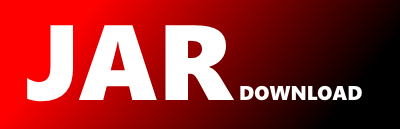
com.github.fluorumlabs.disconnect.polymer.IronMeta Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of disconnect-polymer Show documentation
Show all versions of disconnect-polymer Show documentation
Polymer 3 bindings for Disconnect Zero
package com.github.fluorumlabs.disconnect.polymer;
import com.github.fluorumlabs.disconnect.core.annotations.WebComponent;
import com.github.fluorumlabs.disconnect.polymer.elements.IronMetaElement;
import com.github.fluorumlabs.disconnect.polymer.types.PropertyChangeEvent;
import com.github.fluorumlabs.disconnect.zero.component.AbstractComponent;
import com.github.fluorumlabs.disconnect.zero.observable.ObservableEvent;
import js.lang.Any;
import js.util.collections.Array;
/**
* iron-meta
is a generic element you can use for sharing information across the
* DOM tree. It uses monostate pattern such that any instance of
* iron-meta has access to the shared information. You can use iron-meta
to share whatever you want (or
* create an extension [like x-meta] for enhancements).
*
* The iron-meta
instances containing your actual data can be loaded in an import, or constructed in any
* way you see fit. The only requirement is that you create them before you try to access them.
*
* Examples:
*
* If I create an instance like this:
*
*
<iron-meta key="info" value="foo/bar"></iron-meta>
*
* Note that value="foo/bar" is the metadata I've defined. I could define more attributes or use child nodes
* to define additional metadata.
*
* Now I can access that element (and it's metadata) from any iron-meta instance via the byKey method, e.g.
*
*
meta.byKey('info');
*
* Pure imperative form would be like:
*
* document.createElement('iron-meta').byKey('info');
*
* Or, in a Polymer element, you can include a meta in your template:
*
* <iron-meta id="meta"></iron-meta>
* ...
* this.$.meta.byKey('info');
*
*
* @param - the type parameter
*/
@WebComponent
public class IronMeta
-
extends AbstractComponent
> {
/**
* Instantiates a new Iron meta.
*/
public IronMeta() {
super(IronMetaElement.TAGNAME());
}
/**
* The type of meta-data. All meta-data of the same type is stored together.
*
* @return the string
*/
public String type() {
return getNode().getType();
}
/**
* The type of meta-data. All meta-data of the same type is stored together.
*
* @param type the type
*
* @return the iron meta
*/
public IronMeta- type(String type) {
getNode().setType(type);
return this;
}
/**
* The key used to store
value
under the type
namespace.
*
* @return the string
*/
public String key() {
return getNode().getKey();
}
/**
* The key used to store value
under the type
namespace.
*
* @param key the key
*
* @return the iron meta
*/
public IronMeta- key(String key) {
getNode().setKey(key);
return this;
}
/**
* The meta-data to store or retrieve.
*
* @return the item
*/
public ITEM value() {
return getNode().getValue();
}
/**
* The meta-data to store or retrieve.
*
* @param value the value
*
* @return the iron meta
*/
public IronMeta
- value(ITEM value) {
getNode().setValue(value);
return this;
}
/**
* If true,
value
is set to the iron-meta instance itself.
*
* @return the boolean
*/
public boolean self() {
return getNode().isSelf();
}
/**
* If true, value
is set to the iron-meta instance itself.
*
* @param self the self
*
* @return the iron meta
*/
public IronMeta- self(boolean self) {
getNode().setSelf(self);
return this;
}
/**
* List array.
*
* @return the array
*/
public Array
- list() {
return getNode().getList();
}
/**
* Retrieves meta data value by key.
*
* @param key The key of the meta-data to be returned.
*
* @return the item
*/
public ITEM byKey(String key) {
return getNode().byKey(key);
}
/**
* Fired when the
value
property changes.
*
* @return the observable event
*/
public ObservableEvent> valueChangedEvent() {
return createEvent("value-changed");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy