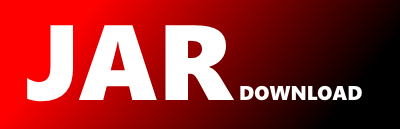
com.github.fluorumlabs.disconnect.polymer.elements.IronIconElement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of disconnect-polymer Show documentation
Show all versions of disconnect-polymer Show documentation
Polymer 3 bindings for Disconnect Zero
package com.github.fluorumlabs.disconnect.polymer.elements;
import com.github.fluorumlabs.disconnect.core.annotations.Import;
import com.github.fluorumlabs.disconnect.core.annotations.NpmPackage;
import com.github.fluorumlabs.disconnect.polymer.Polymer;
import js.web.dom.HTMLElement;
import org.teavm.jso.JSProperty;
import javax.annotation.Nullable;
/**
* The iron-icon
element displays an icon. By default an icon renders as a 24px square.
*
* Example using src:
*
*
<iron-icon src="star.png"></iron-icon>
*
* Example setting size to 32px x 32px:
*
* <iron-icon class="big" src="big_star.png"></iron-icon>
*
* <style is="custom-style">
* .big {
* --iron-icon-height: 32px;
* --iron-icon-width: 32px;
* }
* </style>
*
* The iron elements include several sets of icons. To use the default set of icons, import iron-icons.js
* and use the icon
attribute to specify an icon:
*
* <script type="module">
* import "@polymer/iron-icons/iron-icons.js";
* </script>
*
* <iron-icon icon="menu"></iron-icon>
*
* To use a different built-in set of icons, import the specific
* iron-icons/<iconset>-icons.js
, and specify the icon as <iconset>:<icon>
.
* For example, to use a communication icon, you would use:
*
* <script type="module">
* import "@polymer/iron-icons/communication-icons.js";
* </script>
*
* <iron-icon icon="communication:email"></iron-icon>
*
* You can also create custom icon sets of bitmap or SVG icons.
*
* Example of using an icon named cherry
from a custom iconset with the ID
* fruit
:
*
*
<iron-icon icon="fruit:cherry"></iron-icon>
*
* See <iron-iconset>
and <iron-iconset-svg>
for more information about how to
* create a custom iconset.
*
* See the iron-icons
demo to see the icons available in the various iconsets.
*
*
Styling
* The following custom properties are available for styling:
*
*
* custom properties are available for styling
*
* Custom property Description Default
*
*
* --iron-icon
Mixin applied to the icon {}
* --iron-icon-width
Width of the icon 24px
* --iron-icon-height
Height of the icon 24px
* --iron-icon-fill-color
Fill color of the svg
* icon currentcolor
* --iron-icon-stroke-color
Stroke color of the svg icon none
*
*
*/
@NpmPackage(
name = "@polymer/polymer",
version = Polymer.VERSION
)
@Import(
symbols = "IronIcon",
module = "@polymer/iron-icon/iron-icon.js"
)
public interface IronIconElement extends HTMLElement {
/**
* Tagname string.
*
* @return the string
*/
static String TAGNAME() {
return "iron-icon";
}
/**
* The name of the icon to use. The name should be of the form:
* iconset_name:icon_name
.
*
* @return the icon
*/
@Nullable
@JSProperty
String getIcon();
/**
* The name of the icon to use. The name should be of the form:
* iconset_name:icon_name
.
*
* @param icon the icon
*/
@JSProperty
void setIcon(String icon);
/**
* The name of the theme to used, if one is specified by the iconset.
*
* @return the theme
*/
@Nullable
@JSProperty
String getTheme();
/**
* The name of the theme to used, if one is specified by the iconset.
*
* @param theme the theme
*/
@JSProperty
void setTheme(String theme);
/**
* If using iron-icon without an iconset, you can set the src to be the URL of an individual icon image file. Note
* that this will take precedence over a given icon attribute.
*
* @return the src
*/
@Nullable
@JSProperty
String getSrc();
/**
* If using iron-icon without an iconset, you can set the src to be the URL of an individual icon image file. Note
* that this will take precedence over a given icon attribute.
*
* @param src the src
*/
@JSProperty
void setSrc(String src);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy