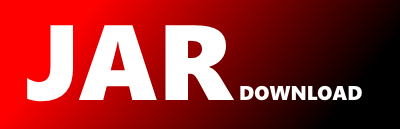
com.github.fmjsjx.libcommon.collection.ListSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of libcommon-collection Show documentation
Show all versions of libcommon-collection Show documentation
A set of some common useful libraries.
package com.github.fmjsjx.libcommon.collection;
import java.util.Collection;
import java.util.List;
import java.util.Objects;
import java.util.Set;
/**
* A {@link Set} backed by a {@link List}.
*
* @param the type of elements maintained by this set
*
* @author MJ Fang
* @see Set
* @see List
* @see ArrayListSet
* @since 2.6
*/
public interface ListSet extends Set {
/**
* Returns the internal {@link List}.
*
* @return the internal {@code List}
*/
List internalList();
/**
* Returns an unmodifiable {@link ListSet} containing zero elements.
*
* @param the {@code ListSet}'s element type
* @return an empty {@code ListSet}
*/
static ListSet of() {
return ImmutableCollections.emptyListSet();
}
/**
* Returns an unmodifiable {@link ListSet} containing one element.
*
* @param the {@code ListSet}'s element type
* @param e1 the single element
* @return a {@code ListSet} containing the specified element
*/
static ListSet of(E e1) {
return new ImmutableCollections.ListSet12<>(e1);
}
/**
* Returns an unmodifiable {@link ListSet} containing two elements.
*
* @param the {@code ListSet}'s element type
* @param e1 the first element
* @param e2 the second element
* @return a {@code ListSet} containing the specified elements
*/
static ListSet of(E e1, E e2) {
return new ImmutableCollections.ListSet12<>(e1, e2);
}
/**
* Returns an unmodifiable {@link ListSet} containing an arbitrary number of
* elements.
*
* @param the {@code ListSet}'s element type
* @param elements elements the elements to be contained in the {@code ListSet}
* @return a {@code ListSet} containing the specified elements
*/
@SafeVarargs
static ListSet of(E... elements) {
switch (elements.length) {
case 0:
return ImmutableCollections.emptyListSet();
case 1:
return new ImmutableCollections.ListSet12<>(elements[0]);
case 2:
var e0 = elements[0];
var e1 = elements[1];
if (e0.equals(Objects.requireNonNull(e1))) { // implicit null check of e0
return new ImmutableCollections.ListSet12<>(e0);
}
return new ImmutableCollections.ListSet12<>(e0, e1);
default:
return new ImmutableCollections.ListSetN<>(elements);
}
}
/**
* Returns an unmodifiable {@link ListSet} containing the elements of the given
* Collection.
*
* @param the {@code ListSet}'s element type
* @param coll a {@code Collection} from which elements are drawn, must be
* non-null
* @return a {@code ListSet} containing the elements of the given
* {@code Collection}
*/
@SuppressWarnings("unchecked")
static ListSet copyOf(Collection extends E> coll) {
if (coll instanceof ImmutableCollections.AbstractImmutableListSet) {
return (ListSet) coll;
} else if (coll instanceof Set) {
return (ListSet) new ImmutableCollections.ListSetN<>(coll.toArray(), false);
} else {
return (ListSet) of(coll.toArray());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy