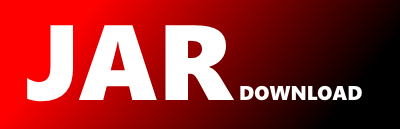
com.github.fommil.netlib.LAPACK Maven / Gradle / Ivy
/* Copyright 2013 Samuel Halliday (generated Java and C).
* Copyright 2003-2007 Keith Seymour (Fortran to Java translation).
* Copyright 1992-2007 The University of Tennessee. All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are
* met:
*
* - Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
*
* - Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer listed
* in this license in the documentation and/or other materials
* provided with the distribution.
*
* - Neither the name of the copyright holders nor the names of its
* contributors may be used to endorse or promote products derived from
* this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
* "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
* LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR
* A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT
* OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
* SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT
* LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
* DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
* THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
* OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package com.github.fommil.netlib;
/**
* Generated by {@code JavaInterfaceGenerator} from {@code org.netlib.lapack} in {@code net.sourceforge.f2j:arpack_combined_all:jar:0.1}.
*
* Property {@value #PROPERTY_KEY} defines the implementation to load,
* defaulting to {@value #FALLBACK}.
*
* This requires 1D column-major linearized arrays, as
* expected by the lower level routines; contrary to
* typical Java 2D row-major arrays.
*/
@lombok.extern.java.Log
public abstract class LAPACK {
private static final String FALLBACK = "com.github.fommil.netlib.F2jLAPACK";
private static final String IMPLS = "com.github.fommil.netlib.NativeSystemLAPACK,com.github.fommil.netlib.NativeRefLAPACK,com.github.fommil.netlib.F2jLAPACK";
private static final String PROPERTY_KEY = "com.github.fommil.netlib.LAPACK";
private static final LAPACK INSTANCE;
static {
try {
String[] classNames = System.getProperty(PROPERTY_KEY, IMPLS).split(",");
LAPACK impl = null;
for (String className: classNames) {
try {
impl = load(className);
break;
} catch (Throwable e) {
log.warning("Failed to load implementation from: " + className);
}
}
if (impl == null) {
log.warning("Using the fallback implementation.");
impl = load(FALLBACK);
}
INSTANCE = impl;
log.config("Implementation provided by " + INSTANCE.getClass());
INSTANCE.slamch("E"); INSTANCE.dlamch("E");
} catch (Exception e) {
throw new ExceptionInInitializerError(e);
}
}
private static LAPACK load(String className) throws Exception {
Class klass = Class.forName(className);
return (LAPACK) klass.newInstance();
}
/**
* @return the environment-defined implementation.
*/
public static LAPACK getInstance() {
return INSTANCE;
}
/**
*
* ..
*
* Purpose
* =======
*
* DBDSDC computes the singular value decomposition (SVD) of a real
* N-by-N (upper or lower) bidiagonal matrix B: B = U * S * VT,
* using a divide and conquer method, where S is a diagonal matrix
* with non-negative diagonal elements (the singular values of B), and
* U and VT are orthogonal matrices of left and right singular vectors,
* respectively. DBDSDC can be used to compute all singular values,
* and optionally, singular vectors or singular vectors in compact form.
*
* This code makes very mild assumptions about floating point
* arithmetic. It will work on machines with a guard digit in
* add/subtract, or on those binary machines without guard digits
* which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or Cray-2.
* It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none. See DLASD3 for details.
*
* The code currently calls DLASDQ if singular values only are desired.
* However, it can be slightly modified to compute singular values
* using the divide and conquer method.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': B is upper bidiagonal.
* = 'L': B is lower bidiagonal.
*
* COMPQ (input) CHARACTER*1
* Specifies whether singular vectors are to be computed
* as follows:
* = 'N': Compute singular values only;
* = 'P': Compute singular values and compute singular
* vectors in compact form;
* = 'I': Compute singular values and singular vectors.
*
* N (input) INTEGER
* The order of the matrix B. N >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the n diagonal elements of the bidiagonal matrix B.
* On exit, if INFO=0, the singular values of B.
*
* E (input/output) DOUBLE PRECISION array, dimension (N-1)
* On entry, the elements of E contain the offdiagonal
* elements of the bidiagonal matrix whose SVD is desired.
* On exit, E has been destroyed.
*
* U (output) DOUBLE PRECISION array, dimension (LDU,N)
* If COMPQ = 'I', then:
* On exit, if INFO = 0, U contains the left singular vectors
* of the bidiagonal matrix.
* For other values of COMPQ, U is not referenced.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= 1.
* If singular vectors are desired, then LDU >= max( 1, N ).
*
* VT (output) DOUBLE PRECISION array, dimension (LDVT,N)
* If COMPQ = 'I', then:
* On exit, if INFO = 0, VT' contains the right singular
* vectors of the bidiagonal matrix.
* For other values of COMPQ, VT is not referenced.
*
* LDVT (input) INTEGER
* The leading dimension of the array VT. LDVT >= 1.
* If singular vectors are desired, then LDVT >= max( 1, N ).
*
* Q (output) DOUBLE PRECISION array, dimension (LDQ)
* If COMPQ = 'P', then:
* On exit, if INFO = 0, Q and IQ contain the left
* and right singular vectors in a compact form,
* requiring O(N log N) space instead of 2*N**2.
* In particular, Q contains all the DOUBLE PRECISION data in
* LDQ >= N*(11 + 2*SMLSIZ + 8*INT(LOG_2(N/(SMLSIZ+1))))
* words of memory, where SMLSIZ is returned by ILAENV and
* is equal to the maximum size of the subproblems at the
* bottom of the computation tree (usually about 25).
* For other values of COMPQ, Q is not referenced.
*
* IQ (output) INTEGER array, dimension (LDIQ)
* If COMPQ = 'P', then:
* On exit, if INFO = 0, Q and IQ contain the left
* and right singular vectors in a compact form,
* requiring O(N log N) space instead of 2*N**2.
* In particular, IQ contains all INTEGER data in
* LDIQ >= N*(3 + 3*INT(LOG_2(N/(SMLSIZ+1))))
* words of memory, where SMLSIZ is returned by ILAENV and
* is equal to the maximum size of the subproblems at the
* bottom of the computation tree (usually about 25).
* For other values of COMPQ, IQ is not referenced.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (MAX(1,LWORK))
* If COMPQ = 'N' then LWORK >= (4 * N).
* If COMPQ = 'P' then LWORK >= (6 * N).
* If COMPQ = 'I' then LWORK >= (3 * N**2 + 4 * N).
*
* IWORK (workspace) INTEGER array, dimension (8*N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: The algorithm failed to compute an singular value.
* The update process of divide and conquer failed.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
* Changed dimension statement in comment describing E from (N) to
* (N-1). Sven, 17 Feb 05.
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param compq
* @param n
* @param d
* @param e
* @param u
* @param ldu
* @param vt
* @param ldvt
* @param q
* @param iq
* @param work
* @param iwork
* @param info
*
*/
abstract public void dbdsdc(java.lang.String uplo, java.lang.String compq, int n, double[] d, double[] e, double[] u, int ldu, double[] vt, int ldvt, double[] q, int[] iq, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DBDSDC computes the singular value decomposition (SVD) of a real
* N-by-N (upper or lower) bidiagonal matrix B: B = U * S * VT,
* using a divide and conquer method, where S is a diagonal matrix
* with non-negative diagonal elements (the singular values of B), and
* U and VT are orthogonal matrices of left and right singular vectors,
* respectively. DBDSDC can be used to compute all singular values,
* and optionally, singular vectors or singular vectors in compact form.
*
* This code makes very mild assumptions about floating point
* arithmetic. It will work on machines with a guard digit in
* add/subtract, or on those binary machines without guard digits
* which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or Cray-2.
* It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none. See DLASD3 for details.
*
* The code currently calls DLASDQ if singular values only are desired.
* However, it can be slightly modified to compute singular values
* using the divide and conquer method.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': B is upper bidiagonal.
* = 'L': B is lower bidiagonal.
*
* COMPQ (input) CHARACTER*1
* Specifies whether singular vectors are to be computed
* as follows:
* = 'N': Compute singular values only;
* = 'P': Compute singular values and compute singular
* vectors in compact form;
* = 'I': Compute singular values and singular vectors.
*
* N (input) INTEGER
* The order of the matrix B. N >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the n diagonal elements of the bidiagonal matrix B.
* On exit, if INFO=0, the singular values of B.
*
* E (input/output) DOUBLE PRECISION array, dimension (N-1)
* On entry, the elements of E contain the offdiagonal
* elements of the bidiagonal matrix whose SVD is desired.
* On exit, E has been destroyed.
*
* U (output) DOUBLE PRECISION array, dimension (LDU,N)
* If COMPQ = 'I', then:
* On exit, if INFO = 0, U contains the left singular vectors
* of the bidiagonal matrix.
* For other values of COMPQ, U is not referenced.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= 1.
* If singular vectors are desired, then LDU >= max( 1, N ).
*
* VT (output) DOUBLE PRECISION array, dimension (LDVT,N)
* If COMPQ = 'I', then:
* On exit, if INFO = 0, VT' contains the right singular
* vectors of the bidiagonal matrix.
* For other values of COMPQ, VT is not referenced.
*
* LDVT (input) INTEGER
* The leading dimension of the array VT. LDVT >= 1.
* If singular vectors are desired, then LDVT >= max( 1, N ).
*
* Q (output) DOUBLE PRECISION array, dimension (LDQ)
* If COMPQ = 'P', then:
* On exit, if INFO = 0, Q and IQ contain the left
* and right singular vectors in a compact form,
* requiring O(N log N) space instead of 2*N**2.
* In particular, Q contains all the DOUBLE PRECISION data in
* LDQ >= N*(11 + 2*SMLSIZ + 8*INT(LOG_2(N/(SMLSIZ+1))))
* words of memory, where SMLSIZ is returned by ILAENV and
* is equal to the maximum size of the subproblems at the
* bottom of the computation tree (usually about 25).
* For other values of COMPQ, Q is not referenced.
*
* IQ (output) INTEGER array, dimension (LDIQ)
* If COMPQ = 'P', then:
* On exit, if INFO = 0, Q and IQ contain the left
* and right singular vectors in a compact form,
* requiring O(N log N) space instead of 2*N**2.
* In particular, IQ contains all INTEGER data in
* LDIQ >= N*(3 + 3*INT(LOG_2(N/(SMLSIZ+1))))
* words of memory, where SMLSIZ is returned by ILAENV and
* is equal to the maximum size of the subproblems at the
* bottom of the computation tree (usually about 25).
* For other values of COMPQ, IQ is not referenced.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (MAX(1,LWORK))
* If COMPQ = 'N' then LWORK >= (4 * N).
* If COMPQ = 'P' then LWORK >= (6 * N).
* If COMPQ = 'I' then LWORK >= (3 * N**2 + 4 * N).
*
* IWORK (workspace) INTEGER array, dimension (8*N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: The algorithm failed to compute an singular value.
* The update process of divide and conquer failed.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
* Changed dimension statement in comment describing E from (N) to
* (N-1). Sven, 17 Feb 05.
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param compq
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param u
* @param _u_offset
* @param ldu
* @param vt
* @param _vt_offset
* @param ldvt
* @param q
* @param _q_offset
* @param iq
* @param _iq_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dbdsdc(java.lang.String uplo, java.lang.String compq, int n, double[] d, int _d_offset, double[] e, int _e_offset, double[] u, int _u_offset, int ldu, double[] vt, int _vt_offset, int ldvt, double[] q, int _q_offset, int[] iq, int _iq_offset, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DBDSQR computes the singular values and, optionally, the right and/or
* left singular vectors from the singular value decomposition (SVD) of
* a real N-by-N (upper or lower) bidiagonal matrix B using the implicit
* zero-shift QR algorithm. The SVD of B has the form
*
* B = Q * S * P**T
*
* where S is the diagonal matrix of singular values, Q is an orthogonal
* matrix of left singular vectors, and P is an orthogonal matrix of
* right singular vectors. If left singular vectors are requested, this
* subroutine actually returns U*Q instead of Q, and, if right singular
* vectors are requested, this subroutine returns P**T*VT instead of
* P**T, for given real input matrices U and VT. When U and VT are the
* orthogonal matrices that reduce a general matrix A to bidiagonal
* form: A = U*B*VT, as computed by DGEBRD, then
*
* A = (U*Q) * S * (P**T*VT)
*
* is the SVD of A. Optionally, the subroutine may also compute Q**T*C
* for a given real input matrix C.
*
* See "Computing Small Singular Values of Bidiagonal Matrices With
* Guaranteed High Relative Accuracy," by J. Demmel and W. Kahan,
* LAPACK Working Note #3 (or SIAM J. Sci. Statist. Comput. vol. 11,
* no. 5, pp. 873-912, Sept 1990) and
* "Accurate singular values and differential qd algorithms," by
* B. Parlett and V. Fernando, Technical Report CPAM-554, Mathematics
* Department, University of California at Berkeley, July 1992
* for a detailed description of the algorithm.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': B is upper bidiagonal;
* = 'L': B is lower bidiagonal.
*
* N (input) INTEGER
* The order of the matrix B. N >= 0.
*
* NCVT (input) INTEGER
* The number of columns of the matrix VT. NCVT >= 0.
*
* NRU (input) INTEGER
* The number of rows of the matrix U. NRU >= 0.
*
* NCC (input) INTEGER
* The number of columns of the matrix C. NCC >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the n diagonal elements of the bidiagonal matrix B.
* On exit, if INFO=0, the singular values of B in decreasing
* order.
*
* E (input/output) DOUBLE PRECISION array, dimension (N-1)
* On entry, the N-1 offdiagonal elements of the bidiagonal
* matrix B.
* On exit, if INFO = 0, E is destroyed; if INFO > 0, D and E
* will contain the diagonal and superdiagonal elements of a
* bidiagonal matrix orthogonally equivalent to the one given
* as input.
*
* VT (input/output) DOUBLE PRECISION array, dimension (LDVT, NCVT)
* On entry, an N-by-NCVT matrix VT.
* On exit, VT is overwritten by P**T * VT.
* Not referenced if NCVT = 0.
*
* LDVT (input) INTEGER
* The leading dimension of the array VT.
* LDVT >= max(1,N) if NCVT > 0; LDVT >= 1 if NCVT = 0.
*
* U (input/output) DOUBLE PRECISION array, dimension (LDU, N)
* On entry, an NRU-by-N matrix U.
* On exit, U is overwritten by U * Q.
* Not referenced if NRU = 0.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= max(1,NRU).
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC, NCC)
* On entry, an N-by-NCC matrix C.
* On exit, C is overwritten by Q**T * C.
* Not referenced if NCC = 0.
*
* LDC (input) INTEGER
* The leading dimension of the array C.
* LDC >= max(1,N) if NCC > 0; LDC >=1 if NCC = 0.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (2*N)
* if NCVT = NRU = NCC = 0, (max(1, 4*N)) otherwise
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: If INFO = -i, the i-th argument had an illegal value
* > 0: the algorithm did not converge; D and E contain the
* elements of a bidiagonal matrix which is orthogonally
* similar to the input matrix B; if INFO = i, i
* elements of E have not converged to zero.
*
* Internal Parameters
* ===================
*
* TOLMUL DOUBLE PRECISION, default = max(10,min(100,EPS**(-1/8)))
* TOLMUL controls the convergence criterion of the QR loop.
* If it is positive, TOLMUL*EPS is the desired relative
* precision in the computed singular values.
* If it is negative, abs(TOLMUL*EPS*sigma_max) is the
* desired absolute accuracy in the computed singular
* values (corresponds to relative accuracy
* abs(TOLMUL*EPS) in the largest singular value.
* abs(TOLMUL) should be between 1 and 1/EPS, and preferably
* between 10 (for fast convergence) and .1/EPS
* (for there to be some accuracy in the results).
* Default is to lose at either one eighth or 2 of the
* available decimal digits in each computed singular value
* (whichever is smaller).
*
* MAXITR INTEGER, default = 6
* MAXITR controls the maximum number of passes of the
* algorithm through its inner loop. The algorithms stops
* (and so fails to converge) if the number of passes
* through the inner loop exceeds MAXITR*N**2.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ncvt
* @param nru
* @param ncc
* @param d
* @param e
* @param vt
* @param ldvt
* @param u
* @param ldu
* @param c
* @param Ldc
* @param work
* @param info
*
*/
abstract public void dbdsqr(java.lang.String uplo, int n, int ncvt, int nru, int ncc, double[] d, double[] e, double[] vt, int ldvt, double[] u, int ldu, double[] c, int Ldc, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DBDSQR computes the singular values and, optionally, the right and/or
* left singular vectors from the singular value decomposition (SVD) of
* a real N-by-N (upper or lower) bidiagonal matrix B using the implicit
* zero-shift QR algorithm. The SVD of B has the form
*
* B = Q * S * P**T
*
* where S is the diagonal matrix of singular values, Q is an orthogonal
* matrix of left singular vectors, and P is an orthogonal matrix of
* right singular vectors. If left singular vectors are requested, this
* subroutine actually returns U*Q instead of Q, and, if right singular
* vectors are requested, this subroutine returns P**T*VT instead of
* P**T, for given real input matrices U and VT. When U and VT are the
* orthogonal matrices that reduce a general matrix A to bidiagonal
* form: A = U*B*VT, as computed by DGEBRD, then
*
* A = (U*Q) * S * (P**T*VT)
*
* is the SVD of A. Optionally, the subroutine may also compute Q**T*C
* for a given real input matrix C.
*
* See "Computing Small Singular Values of Bidiagonal Matrices With
* Guaranteed High Relative Accuracy," by J. Demmel and W. Kahan,
* LAPACK Working Note #3 (or SIAM J. Sci. Statist. Comput. vol. 11,
* no. 5, pp. 873-912, Sept 1990) and
* "Accurate singular values and differential qd algorithms," by
* B. Parlett and V. Fernando, Technical Report CPAM-554, Mathematics
* Department, University of California at Berkeley, July 1992
* for a detailed description of the algorithm.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': B is upper bidiagonal;
* = 'L': B is lower bidiagonal.
*
* N (input) INTEGER
* The order of the matrix B. N >= 0.
*
* NCVT (input) INTEGER
* The number of columns of the matrix VT. NCVT >= 0.
*
* NRU (input) INTEGER
* The number of rows of the matrix U. NRU >= 0.
*
* NCC (input) INTEGER
* The number of columns of the matrix C. NCC >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the n diagonal elements of the bidiagonal matrix B.
* On exit, if INFO=0, the singular values of B in decreasing
* order.
*
* E (input/output) DOUBLE PRECISION array, dimension (N-1)
* On entry, the N-1 offdiagonal elements of the bidiagonal
* matrix B.
* On exit, if INFO = 0, E is destroyed; if INFO > 0, D and E
* will contain the diagonal and superdiagonal elements of a
* bidiagonal matrix orthogonally equivalent to the one given
* as input.
*
* VT (input/output) DOUBLE PRECISION array, dimension (LDVT, NCVT)
* On entry, an N-by-NCVT matrix VT.
* On exit, VT is overwritten by P**T * VT.
* Not referenced if NCVT = 0.
*
* LDVT (input) INTEGER
* The leading dimension of the array VT.
* LDVT >= max(1,N) if NCVT > 0; LDVT >= 1 if NCVT = 0.
*
* U (input/output) DOUBLE PRECISION array, dimension (LDU, N)
* On entry, an NRU-by-N matrix U.
* On exit, U is overwritten by U * Q.
* Not referenced if NRU = 0.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= max(1,NRU).
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC, NCC)
* On entry, an N-by-NCC matrix C.
* On exit, C is overwritten by Q**T * C.
* Not referenced if NCC = 0.
*
* LDC (input) INTEGER
* The leading dimension of the array C.
* LDC >= max(1,N) if NCC > 0; LDC >=1 if NCC = 0.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (2*N)
* if NCVT = NRU = NCC = 0, (max(1, 4*N)) otherwise
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: If INFO = -i, the i-th argument had an illegal value
* > 0: the algorithm did not converge; D and E contain the
* elements of a bidiagonal matrix which is orthogonally
* similar to the input matrix B; if INFO = i, i
* elements of E have not converged to zero.
*
* Internal Parameters
* ===================
*
* TOLMUL DOUBLE PRECISION, default = max(10,min(100,EPS**(-1/8)))
* TOLMUL controls the convergence criterion of the QR loop.
* If it is positive, TOLMUL*EPS is the desired relative
* precision in the computed singular values.
* If it is negative, abs(TOLMUL*EPS*sigma_max) is the
* desired absolute accuracy in the computed singular
* values (corresponds to relative accuracy
* abs(TOLMUL*EPS) in the largest singular value.
* abs(TOLMUL) should be between 1 and 1/EPS, and preferably
* between 10 (for fast convergence) and .1/EPS
* (for there to be some accuracy in the results).
* Default is to lose at either one eighth or 2 of the
* available decimal digits in each computed singular value
* (whichever is smaller).
*
* MAXITR INTEGER, default = 6
* MAXITR controls the maximum number of passes of the
* algorithm through its inner loop. The algorithms stops
* (and so fails to converge) if the number of passes
* through the inner loop exceeds MAXITR*N**2.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ncvt
* @param nru
* @param ncc
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param vt
* @param _vt_offset
* @param ldvt
* @param u
* @param _u_offset
* @param ldu
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dbdsqr(java.lang.String uplo, int n, int ncvt, int nru, int ncc, double[] d, int _d_offset, double[] e, int _e_offset, double[] vt, int _vt_offset, int ldvt, double[] u, int _u_offset, int ldu, double[] c, int _c_offset, int Ldc, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DDISNA computes the reciprocal condition numbers for the eigenvectors
* of a real symmetric or complex Hermitian matrix or for the left or
* right singular vectors of a general m-by-n matrix. The reciprocal
* condition number is the 'gap' between the corresponding eigenvalue or
* singular value and the nearest other one.
*
* The bound on the error, measured by angle in radians, in the I-th
* computed vector is given by
*
* DLAMCH( 'E' ) * ( ANORM / SEP( I ) )
*
* where ANORM = 2-norm(A) = max( abs( D(j) ) ). SEP(I) is not allowed
* to be smaller than DLAMCH( 'E' )*ANORM in order to limit the size of
* the error bound.
*
* DDISNA may also be used to compute error bounds for eigenvectors of
* the generalized symmetric definite eigenproblem.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* Specifies for which problem the reciprocal condition numbers
* should be computed:
* = 'E': the eigenvectors of a symmetric/Hermitian matrix;
* = 'L': the left singular vectors of a general matrix;
* = 'R': the right singular vectors of a general matrix.
*
* M (input) INTEGER
* The number of rows of the matrix. M >= 0.
*
* N (input) INTEGER
* If JOB = 'L' or 'R', the number of columns of the matrix,
* in which case N >= 0. Ignored if JOB = 'E'.
*
* D (input) DOUBLE PRECISION array, dimension (M) if JOB = 'E'
* dimension (min(M,N)) if JOB = 'L' or 'R'
* The eigenvalues (if JOB = 'E') or singular values (if JOB =
* 'L' or 'R') of the matrix, in either increasing or decreasing
* order. If singular values, they must be non-negative.
*
* SEP (output) DOUBLE PRECISION array, dimension (M) if JOB = 'E'
* dimension (min(M,N)) if JOB = 'L' or 'R'
* The reciprocal condition numbers of the vectors.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param m
* @param n
* @param d
* @param sep
* @param info
*
*/
abstract public void ddisna(java.lang.String job, int m, int n, double[] d, double[] sep, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DDISNA computes the reciprocal condition numbers for the eigenvectors
* of a real symmetric or complex Hermitian matrix or for the left or
* right singular vectors of a general m-by-n matrix. The reciprocal
* condition number is the 'gap' between the corresponding eigenvalue or
* singular value and the nearest other one.
*
* The bound on the error, measured by angle in radians, in the I-th
* computed vector is given by
*
* DLAMCH( 'E' ) * ( ANORM / SEP( I ) )
*
* where ANORM = 2-norm(A) = max( abs( D(j) ) ). SEP(I) is not allowed
* to be smaller than DLAMCH( 'E' )*ANORM in order to limit the size of
* the error bound.
*
* DDISNA may also be used to compute error bounds for eigenvectors of
* the generalized symmetric definite eigenproblem.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* Specifies for which problem the reciprocal condition numbers
* should be computed:
* = 'E': the eigenvectors of a symmetric/Hermitian matrix;
* = 'L': the left singular vectors of a general matrix;
* = 'R': the right singular vectors of a general matrix.
*
* M (input) INTEGER
* The number of rows of the matrix. M >= 0.
*
* N (input) INTEGER
* If JOB = 'L' or 'R', the number of columns of the matrix,
* in which case N >= 0. Ignored if JOB = 'E'.
*
* D (input) DOUBLE PRECISION array, dimension (M) if JOB = 'E'
* dimension (min(M,N)) if JOB = 'L' or 'R'
* The eigenvalues (if JOB = 'E') or singular values (if JOB =
* 'L' or 'R') of the matrix, in either increasing or decreasing
* order. If singular values, they must be non-negative.
*
* SEP (output) DOUBLE PRECISION array, dimension (M) if JOB = 'E'
* dimension (min(M,N)) if JOB = 'L' or 'R'
* The reciprocal condition numbers of the vectors.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param m
* @param n
* @param d
* @param _d_offset
* @param sep
* @param _sep_offset
* @param info
*
*/
abstract public void ddisna(java.lang.String job, int m, int n, double[] d, int _d_offset, double[] sep, int _sep_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGBBRD reduces a real general m-by-n band matrix A to upper
* bidiagonal form B by an orthogonal transformation: Q' * A * P = B.
*
* The routine computes B, and optionally forms Q or P', or computes
* Q'*C for a given matrix C.
*
* Arguments
* =========
*
* VECT (input) CHARACTER*1
* Specifies whether or not the matrices Q and P' are to be
* formed.
* = 'N': do not form Q or P';
* = 'Q': form Q only;
* = 'P': form P' only;
* = 'B': form both.
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* NCC (input) INTEGER
* The number of columns of the matrix C. NCC >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals of the matrix A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals of the matrix A. KU >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB,N)
* On entry, the m-by-n band matrix A, stored in rows 1 to
* KL+KU+1. The j-th column of A is stored in the j-th column of
* the array AB as follows:
* AB(ku+1+i-j,j) = A(i,j) for max(1,j-ku)<=i<=min(m,j+kl).
* On exit, A is overwritten by values generated during the
* reduction.
*
* LDAB (input) INTEGER
* The leading dimension of the array A. LDAB >= KL+KU+1.
*
* D (output) DOUBLE PRECISION array, dimension (min(M,N))
* The diagonal elements of the bidiagonal matrix B.
*
* E (output) DOUBLE PRECISION array, dimension (min(M,N)-1)
* The superdiagonal elements of the bidiagonal matrix B.
*
* Q (output) DOUBLE PRECISION array, dimension (LDQ,M)
* If VECT = 'Q' or 'B', the m-by-m orthogonal matrix Q.
* If VECT = 'N' or 'P', the array Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q.
* LDQ >= max(1,M) if VECT = 'Q' or 'B'; LDQ >= 1 otherwise.
*
* PT (output) DOUBLE PRECISION array, dimension (LDPT,N)
* If VECT = 'P' or 'B', the n-by-n orthogonal matrix P'.
* If VECT = 'N' or 'Q', the array PT is not referenced.
*
* LDPT (input) INTEGER
* The leading dimension of the array PT.
* LDPT >= max(1,N) if VECT = 'P' or 'B'; LDPT >= 1 otherwise.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,NCC)
* On entry, an m-by-ncc matrix C.
* On exit, C is overwritten by Q'*C.
* C is not referenced if NCC = 0.
*
* LDC (input) INTEGER
* The leading dimension of the array C.
* LDC >= max(1,M) if NCC > 0; LDC >= 1 if NCC = 0.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (2*max(M,N))
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param vect
* @param m
* @param n
* @param ncc
* @param kl
* @param ku
* @param ab
* @param ldab
* @param d
* @param e
* @param q
* @param ldq
* @param pt
* @param ldpt
* @param c
* @param Ldc
* @param work
* @param info
*
*/
abstract public void dgbbrd(java.lang.String vect, int m, int n, int ncc, int kl, int ku, double[] ab, int ldab, double[] d, double[] e, double[] q, int ldq, double[] pt, int ldpt, double[] c, int Ldc, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGBBRD reduces a real general m-by-n band matrix A to upper
* bidiagonal form B by an orthogonal transformation: Q' * A * P = B.
*
* The routine computes B, and optionally forms Q or P', or computes
* Q'*C for a given matrix C.
*
* Arguments
* =========
*
* VECT (input) CHARACTER*1
* Specifies whether or not the matrices Q and P' are to be
* formed.
* = 'N': do not form Q or P';
* = 'Q': form Q only;
* = 'P': form P' only;
* = 'B': form both.
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* NCC (input) INTEGER
* The number of columns of the matrix C. NCC >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals of the matrix A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals of the matrix A. KU >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB,N)
* On entry, the m-by-n band matrix A, stored in rows 1 to
* KL+KU+1. The j-th column of A is stored in the j-th column of
* the array AB as follows:
* AB(ku+1+i-j,j) = A(i,j) for max(1,j-ku)<=i<=min(m,j+kl).
* On exit, A is overwritten by values generated during the
* reduction.
*
* LDAB (input) INTEGER
* The leading dimension of the array A. LDAB >= KL+KU+1.
*
* D (output) DOUBLE PRECISION array, dimension (min(M,N))
* The diagonal elements of the bidiagonal matrix B.
*
* E (output) DOUBLE PRECISION array, dimension (min(M,N)-1)
* The superdiagonal elements of the bidiagonal matrix B.
*
* Q (output) DOUBLE PRECISION array, dimension (LDQ,M)
* If VECT = 'Q' or 'B', the m-by-m orthogonal matrix Q.
* If VECT = 'N' or 'P', the array Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q.
* LDQ >= max(1,M) if VECT = 'Q' or 'B'; LDQ >= 1 otherwise.
*
* PT (output) DOUBLE PRECISION array, dimension (LDPT,N)
* If VECT = 'P' or 'B', the n-by-n orthogonal matrix P'.
* If VECT = 'N' or 'Q', the array PT is not referenced.
*
* LDPT (input) INTEGER
* The leading dimension of the array PT.
* LDPT >= max(1,N) if VECT = 'P' or 'B'; LDPT >= 1 otherwise.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,NCC)
* On entry, an m-by-ncc matrix C.
* On exit, C is overwritten by Q'*C.
* C is not referenced if NCC = 0.
*
* LDC (input) INTEGER
* The leading dimension of the array C.
* LDC >= max(1,M) if NCC > 0; LDC >= 1 if NCC = 0.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (2*max(M,N))
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param vect
* @param m
* @param n
* @param ncc
* @param kl
* @param ku
* @param ab
* @param _ab_offset
* @param ldab
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param q
* @param _q_offset
* @param ldq
* @param pt
* @param _pt_offset
* @param ldpt
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dgbbrd(java.lang.String vect, int m, int n, int ncc, int kl, int ku, double[] ab, int _ab_offset, int ldab, double[] d, int _d_offset, double[] e, int _e_offset, double[] q, int _q_offset, int ldq, double[] pt, int _pt_offset, int ldpt, double[] c, int _c_offset, int Ldc, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGBCON estimates the reciprocal of the condition number of a real
* general band matrix A, in either the 1-norm or the infinity-norm,
* using the LU factorization computed by DGBTRF.
*
* An estimate is obtained for norm(inv(A)), and the reciprocal of the
* condition number is computed as
* RCOND = 1 / ( norm(A) * norm(inv(A)) ).
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies whether the 1-norm condition number or the
* infinity-norm condition number is required:
* = '1' or 'O': 1-norm;
* = 'I': Infinity-norm.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* AB (input) DOUBLE PRECISION array, dimension (LDAB,N)
* Details of the LU factorization of the band matrix A, as
* computed by DGBTRF. U is stored as an upper triangular band
* matrix with KL+KU superdiagonals in rows 1 to KL+KU+1, and
* the multipliers used during the factorization are stored in
* rows KL+KU+2 to 2*KL+KU+1.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= 2*KL+KU+1.
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices; for 1 <= i <= N, row i of the matrix was
* interchanged with row IPIV(i).
*
* ANORM (input) DOUBLE PRECISION
* If NORM = '1' or 'O', the 1-norm of the original matrix A.
* If NORM = 'I', the infinity-norm of the original matrix A.
*
* RCOND (output) DOUBLE PRECISION
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(norm(A) * norm(inv(A))).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param n
* @param kl
* @param ku
* @param ab
* @param ldab
* @param ipiv
* @param anorm
* @param rcond
* @param work
* @param iwork
* @param info
*
*/
abstract public void dgbcon(java.lang.String norm, int n, int kl, int ku, double[] ab, int ldab, int[] ipiv, double anorm, org.netlib.util.doubleW rcond, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGBCON estimates the reciprocal of the condition number of a real
* general band matrix A, in either the 1-norm or the infinity-norm,
* using the LU factorization computed by DGBTRF.
*
* An estimate is obtained for norm(inv(A)), and the reciprocal of the
* condition number is computed as
* RCOND = 1 / ( norm(A) * norm(inv(A)) ).
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies whether the 1-norm condition number or the
* infinity-norm condition number is required:
* = '1' or 'O': 1-norm;
* = 'I': Infinity-norm.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* AB (input) DOUBLE PRECISION array, dimension (LDAB,N)
* Details of the LU factorization of the band matrix A, as
* computed by DGBTRF. U is stored as an upper triangular band
* matrix with KL+KU superdiagonals in rows 1 to KL+KU+1, and
* the multipliers used during the factorization are stored in
* rows KL+KU+2 to 2*KL+KU+1.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= 2*KL+KU+1.
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices; for 1 <= i <= N, row i of the matrix was
* interchanged with row IPIV(i).
*
* ANORM (input) DOUBLE PRECISION
* If NORM = '1' or 'O', the 1-norm of the original matrix A.
* If NORM = 'I', the infinity-norm of the original matrix A.
*
* RCOND (output) DOUBLE PRECISION
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(norm(A) * norm(inv(A))).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param n
* @param kl
* @param ku
* @param ab
* @param _ab_offset
* @param ldab
* @param ipiv
* @param _ipiv_offset
* @param anorm
* @param rcond
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dgbcon(java.lang.String norm, int n, int kl, int ku, double[] ab, int _ab_offset, int ldab, int[] ipiv, int _ipiv_offset, double anorm, org.netlib.util.doubleW rcond, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGBEQU computes row and column scalings intended to equilibrate an
* M-by-N band matrix A and reduce its condition number. R returns the
* row scale factors and C the column scale factors, chosen to try to
* make the largest element in each row and column of the matrix B with
* elements B(i,j)=R(i)*A(i,j)*C(j) have absolute value 1.
*
* R(i) and C(j) are restricted to be between SMLNUM = smallest safe
* number and BIGNUM = largest safe number. Use of these scaling
* factors is not guaranteed to reduce the condition number of A but
* works well in practice.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* AB (input) DOUBLE PRECISION array, dimension (LDAB,N)
* The band matrix A, stored in rows 1 to KL+KU+1. The j-th
* column of A is stored in the j-th column of the array AB as
* follows:
* AB(ku+1+i-j,j) = A(i,j) for max(1,j-ku)<=i<=min(m,j+kl).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KL+KU+1.
*
* R (output) DOUBLE PRECISION array, dimension (M)
* If INFO = 0, or INFO > M, R contains the row scale factors
* for A.
*
* C (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, C contains the column scale factors for A.
*
* ROWCND (output) DOUBLE PRECISION
* If INFO = 0 or INFO > M, ROWCND contains the ratio of the
* smallest R(i) to the largest R(i). If ROWCND >= 0.1 and
* AMAX is neither too large nor too small, it is not worth
* scaling by R.
*
* COLCND (output) DOUBLE PRECISION
* If INFO = 0, COLCND contains the ratio of the smallest
* C(i) to the largest C(i). If COLCND >= 0.1, it is not
* worth scaling by C.
*
* AMAX (output) DOUBLE PRECISION
* Absolute value of largest matrix element. If AMAX is very
* close to overflow or very close to underflow, the matrix
* should be scaled.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= M: the i-th row of A is exactly zero
* > M: the (i-M)-th column of A is exactly zero
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param kl
* @param ku
* @param ab
* @param ldab
* @param r
* @param c
* @param rowcnd
* @param colcnd
* @param amax
* @param info
*
*/
abstract public void dgbequ(int m, int n, int kl, int ku, double[] ab, int ldab, double[] r, double[] c, org.netlib.util.doubleW rowcnd, org.netlib.util.doubleW colcnd, org.netlib.util.doubleW amax, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGBEQU computes row and column scalings intended to equilibrate an
* M-by-N band matrix A and reduce its condition number. R returns the
* row scale factors and C the column scale factors, chosen to try to
* make the largest element in each row and column of the matrix B with
* elements B(i,j)=R(i)*A(i,j)*C(j) have absolute value 1.
*
* R(i) and C(j) are restricted to be between SMLNUM = smallest safe
* number and BIGNUM = largest safe number. Use of these scaling
* factors is not guaranteed to reduce the condition number of A but
* works well in practice.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* AB (input) DOUBLE PRECISION array, dimension (LDAB,N)
* The band matrix A, stored in rows 1 to KL+KU+1. The j-th
* column of A is stored in the j-th column of the array AB as
* follows:
* AB(ku+1+i-j,j) = A(i,j) for max(1,j-ku)<=i<=min(m,j+kl).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KL+KU+1.
*
* R (output) DOUBLE PRECISION array, dimension (M)
* If INFO = 0, or INFO > M, R contains the row scale factors
* for A.
*
* C (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, C contains the column scale factors for A.
*
* ROWCND (output) DOUBLE PRECISION
* If INFO = 0 or INFO > M, ROWCND contains the ratio of the
* smallest R(i) to the largest R(i). If ROWCND >= 0.1 and
* AMAX is neither too large nor too small, it is not worth
* scaling by R.
*
* COLCND (output) DOUBLE PRECISION
* If INFO = 0, COLCND contains the ratio of the smallest
* C(i) to the largest C(i). If COLCND >= 0.1, it is not
* worth scaling by C.
*
* AMAX (output) DOUBLE PRECISION
* Absolute value of largest matrix element. If AMAX is very
* close to overflow or very close to underflow, the matrix
* should be scaled.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= M: the i-th row of A is exactly zero
* > M: the (i-M)-th column of A is exactly zero
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param kl
* @param ku
* @param ab
* @param _ab_offset
* @param ldab
* @param r
* @param _r_offset
* @param c
* @param _c_offset
* @param rowcnd
* @param colcnd
* @param amax
* @param info
*
*/
abstract public void dgbequ(int m, int n, int kl, int ku, double[] ab, int _ab_offset, int ldab, double[] r, int _r_offset, double[] c, int _c_offset, org.netlib.util.doubleW rowcnd, org.netlib.util.doubleW colcnd, org.netlib.util.doubleW amax, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGBRFS improves the computed solution to a system of linear
* equations when the coefficient matrix is banded, and provides
* error bounds and backward error estimates for the solution.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AB (input) DOUBLE PRECISION array, dimension (LDAB,N)
* The original band matrix A, stored in rows 1 to KL+KU+1.
* The j-th column of A is stored in the j-th column of the
* array AB as follows:
* AB(ku+1+i-j,j) = A(i,j) for max(1,j-ku)<=i<=min(n,j+kl).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KL+KU+1.
*
* AFB (input) DOUBLE PRECISION array, dimension (LDAFB,N)
* Details of the LU factorization of the band matrix A, as
* computed by DGBTRF. U is stored as an upper triangular band
* matrix with KL+KU superdiagonals in rows 1 to KL+KU+1, and
* the multipliers used during the factorization are stored in
* rows KL+KU+2 to 2*KL+KU+1.
*
* LDAFB (input) INTEGER
* The leading dimension of the array AFB. LDAFB >= 2*KL*KU+1.
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices from DGBTRF; for 1<=i<=N, row i of the
* matrix was interchanged with row IPIV(i).
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by DGBTRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param trans
* @param n
* @param kl
* @param ku
* @param nrhs
* @param ab
* @param ldab
* @param afb
* @param ldafb
* @param ipiv
* @param b
* @param ldb
* @param x
* @param ldx
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void dgbrfs(java.lang.String trans, int n, int kl, int ku, int nrhs, double[] ab, int ldab, double[] afb, int ldafb, int[] ipiv, double[] b, int ldb, double[] x, int ldx, double[] ferr, double[] berr, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGBRFS improves the computed solution to a system of linear
* equations when the coefficient matrix is banded, and provides
* error bounds and backward error estimates for the solution.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AB (input) DOUBLE PRECISION array, dimension (LDAB,N)
* The original band matrix A, stored in rows 1 to KL+KU+1.
* The j-th column of A is stored in the j-th column of the
* array AB as follows:
* AB(ku+1+i-j,j) = A(i,j) for max(1,j-ku)<=i<=min(n,j+kl).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KL+KU+1.
*
* AFB (input) DOUBLE PRECISION array, dimension (LDAFB,N)
* Details of the LU factorization of the band matrix A, as
* computed by DGBTRF. U is stored as an upper triangular band
* matrix with KL+KU superdiagonals in rows 1 to KL+KU+1, and
* the multipliers used during the factorization are stored in
* rows KL+KU+2 to 2*KL+KU+1.
*
* LDAFB (input) INTEGER
* The leading dimension of the array AFB. LDAFB >= 2*KL*KU+1.
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices from DGBTRF; for 1<=i<=N, row i of the
* matrix was interchanged with row IPIV(i).
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by DGBTRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param trans
* @param n
* @param kl
* @param ku
* @param nrhs
* @param ab
* @param _ab_offset
* @param ldab
* @param afb
* @param _afb_offset
* @param ldafb
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dgbrfs(java.lang.String trans, int n, int kl, int ku, int nrhs, double[] ab, int _ab_offset, int ldab, double[] afb, int _afb_offset, int ldafb, int[] ipiv, int _ipiv_offset, double[] b, int _b_offset, int ldb, double[] x, int _x_offset, int ldx, double[] ferr, int _ferr_offset, double[] berr, int _berr_offset, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGBSV computes the solution to a real system of linear equations
* A * X = B, where A is a band matrix of order N with KL subdiagonals
* and KU superdiagonals, and X and B are N-by-NRHS matrices.
*
* The LU decomposition with partial pivoting and row interchanges is
* used to factor A as A = L * U, where L is a product of permutation
* and unit lower triangular matrices with KL subdiagonals, and U is
* upper triangular with KL+KU superdiagonals. The factored form of A
* is then used to solve the system of equations A * X = B.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB,N)
* On entry, the matrix A in band storage, in rows KL+1 to
* 2*KL+KU+1; rows 1 to KL of the array need not be set.
* The j-th column of A is stored in the j-th column of the
* array AB as follows:
* AB(KL+KU+1+i-j,j) = A(i,j) for max(1,j-KU)<=i<=min(N,j+KL)
* On exit, details of the factorization: U is stored as an
* upper triangular band matrix with KL+KU superdiagonals in
* rows 1 to KL+KU+1, and the multipliers used during the
* factorization are stored in rows KL+KU+2 to 2*KL+KU+1.
* See below for further details.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= 2*KL+KU+1.
*
* IPIV (output) INTEGER array, dimension (N)
* The pivot indices that define the permutation matrix P;
* row i of the matrix was interchanged with row IPIV(i).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if INFO = 0, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, U(i,i) is exactly zero. The factorization
* has been completed, but the factor U is exactly
* singular, and the solution has not been computed.
*
* Further Details
* ===============
*
* The band storage scheme is illustrated by the following example, when
* M = N = 6, KL = 2, KU = 1:
*
* On entry: On exit:
*
* * * * + + + * * * u14 u25 u36
* * * + + + + * * u13 u24 u35 u46
* * a12 a23 a34 a45 a56 * u12 u23 u34 u45 u56
* a11 a22 a33 a44 a55 a66 u11 u22 u33 u44 u55 u66
* a21 a32 a43 a54 a65 * m21 m32 m43 m54 m65 *
* a31 a42 a53 a64 * * m31 m42 m53 m64 * *
*
* Array elements marked * are not used by the routine; elements marked
* + need not be set on entry, but are required by the routine to store
* elements of U because of fill-in resulting from the row interchanges.
*
* =====================================================================
*
* .. External Subroutines ..
*
*
* @param n
* @param kl
* @param ku
* @param nrhs
* @param ab
* @param ldab
* @param ipiv
* @param b
* @param ldb
* @param info
*
*/
abstract public void dgbsv(int n, int kl, int ku, int nrhs, double[] ab, int ldab, int[] ipiv, double[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGBSV computes the solution to a real system of linear equations
* A * X = B, where A is a band matrix of order N with KL subdiagonals
* and KU superdiagonals, and X and B are N-by-NRHS matrices.
*
* The LU decomposition with partial pivoting and row interchanges is
* used to factor A as A = L * U, where L is a product of permutation
* and unit lower triangular matrices with KL subdiagonals, and U is
* upper triangular with KL+KU superdiagonals. The factored form of A
* is then used to solve the system of equations A * X = B.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB,N)
* On entry, the matrix A in band storage, in rows KL+1 to
* 2*KL+KU+1; rows 1 to KL of the array need not be set.
* The j-th column of A is stored in the j-th column of the
* array AB as follows:
* AB(KL+KU+1+i-j,j) = A(i,j) for max(1,j-KU)<=i<=min(N,j+KL)
* On exit, details of the factorization: U is stored as an
* upper triangular band matrix with KL+KU superdiagonals in
* rows 1 to KL+KU+1, and the multipliers used during the
* factorization are stored in rows KL+KU+2 to 2*KL+KU+1.
* See below for further details.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= 2*KL+KU+1.
*
* IPIV (output) INTEGER array, dimension (N)
* The pivot indices that define the permutation matrix P;
* row i of the matrix was interchanged with row IPIV(i).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if INFO = 0, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, U(i,i) is exactly zero. The factorization
* has been completed, but the factor U is exactly
* singular, and the solution has not been computed.
*
* Further Details
* ===============
*
* The band storage scheme is illustrated by the following example, when
* M = N = 6, KL = 2, KU = 1:
*
* On entry: On exit:
*
* * * * + + + * * * u14 u25 u36
* * * + + + + * * u13 u24 u35 u46
* * a12 a23 a34 a45 a56 * u12 u23 u34 u45 u56
* a11 a22 a33 a44 a55 a66 u11 u22 u33 u44 u55 u66
* a21 a32 a43 a54 a65 * m21 m32 m43 m54 m65 *
* a31 a42 a53 a64 * * m31 m42 m53 m64 * *
*
* Array elements marked * are not used by the routine; elements marked
* + need not be set on entry, but are required by the routine to store
* elements of U because of fill-in resulting from the row interchanges.
*
* =====================================================================
*
* .. External Subroutines ..
*
*
* @param n
* @param kl
* @param ku
* @param nrhs
* @param ab
* @param _ab_offset
* @param ldab
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void dgbsv(int n, int kl, int ku, int nrhs, double[] ab, int _ab_offset, int ldab, int[] ipiv, int _ipiv_offset, double[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGBSVX uses the LU factorization to compute the solution to a real
* system of linear equations A * X = B, A**T * X = B, or A**H * X = B,
* where A is a band matrix of order N with KL subdiagonals and KU
* superdiagonals, and X and B are N-by-NRHS matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed by this subroutine:
*
* 1. If FACT = 'E', real scaling factors are computed to equilibrate
* the system:
* TRANS = 'N': diag(R)*A*diag(C) *inv(diag(C))*X = diag(R)*B
* TRANS = 'T': (diag(R)*A*diag(C))**T *inv(diag(R))*X = diag(C)*B
* TRANS = 'C': (diag(R)*A*diag(C))**H *inv(diag(R))*X = diag(C)*B
* Whether or not the system will be equilibrated depends on the
* scaling of the matrix A, but if equilibration is used, A is
* overwritten by diag(R)*A*diag(C) and B by diag(R)*B (if TRANS='N')
* or diag(C)*B (if TRANS = 'T' or 'C').
*
* 2. If FACT = 'N' or 'E', the LU decomposition is used to factor the
* matrix A (after equilibration if FACT = 'E') as
* A = L * U,
* where L is a product of permutation and unit lower triangular
* matrices with KL subdiagonals, and U is upper triangular with
* KL+KU superdiagonals.
*
* 3. If some U(i,i)=0, so that U is exactly singular, then the routine
* returns with INFO = i. Otherwise, the factored form of A is used
* to estimate the condition number of the matrix A. If the
* reciprocal of the condition number is less than machine precision,
* INFO = N+1 is returned as a warning, but the routine still goes on
* to solve for X and compute error bounds as described below.
*
* 4. The system of equations is solved for X using the factored form
* of A.
*
* 5. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* 6. If equilibration was used, the matrix X is premultiplied by
* diag(C) (if TRANS = 'N') or diag(R) (if TRANS = 'T' or 'C') so
* that it solves the original system before equilibration.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of the matrix A is
* supplied on entry, and if not, whether the matrix A should be
* equilibrated before it is factored.
* = 'F': On entry, AFB and IPIV contain the factored form of
* A. If EQUED is not 'N', the matrix A has been
* equilibrated with scaling factors given by R and C.
* AB, AFB, and IPIV are not modified.
* = 'N': The matrix A will be copied to AFB and factored.
* = 'E': The matrix A will be equilibrated if necessary, then
* copied to AFB and factored.
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations.
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Transpose)
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB,N)
* On entry, the matrix A in band storage, in rows 1 to KL+KU+1.
* The j-th column of A is stored in the j-th column of the
* array AB as follows:
* AB(KU+1+i-j,j) = A(i,j) for max(1,j-KU)<=i<=min(N,j+kl)
*
* If FACT = 'F' and EQUED is not 'N', then A must have been
* equilibrated by the scaling factors in R and/or C. AB is not
* modified if FACT = 'F' or 'N', or if FACT = 'E' and
* EQUED = 'N' on exit.
*
* On exit, if EQUED .ne. 'N', A is scaled as follows:
* EQUED = 'R': A := diag(R) * A
* EQUED = 'C': A := A * diag(C)
* EQUED = 'B': A := diag(R) * A * diag(C).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KL+KU+1.
*
* AFB (input or output) DOUBLE PRECISION array, dimension (LDAFB,N)
* If FACT = 'F', then AFB is an input argument and on entry
* contains details of the LU factorization of the band matrix
* A, as computed by DGBTRF. U is stored as an upper triangular
* band matrix with KL+KU superdiagonals in rows 1 to KL+KU+1,
* and the multipliers used during the factorization are stored
* in rows KL+KU+2 to 2*KL+KU+1. If EQUED .ne. 'N', then AFB is
* the factored form of the equilibrated matrix A.
*
* If FACT = 'N', then AFB is an output argument and on exit
* returns details of the LU factorization of A.
*
* If FACT = 'E', then AFB is an output argument and on exit
* returns details of the LU factorization of the equilibrated
* matrix A (see the description of AB for the form of the
* equilibrated matrix).
*
* LDAFB (input) INTEGER
* The leading dimension of the array AFB. LDAFB >= 2*KL+KU+1.
*
* IPIV (input or output) INTEGER array, dimension (N)
* If FACT = 'F', then IPIV is an input argument and on entry
* contains the pivot indices from the factorization A = L*U
* as computed by DGBTRF; row i of the matrix was interchanged
* with row IPIV(i).
*
* If FACT = 'N', then IPIV is an output argument and on exit
* contains the pivot indices from the factorization A = L*U
* of the original matrix A.
*
* If FACT = 'E', then IPIV is an output argument and on exit
* contains the pivot indices from the factorization A = L*U
* of the equilibrated matrix A.
*
* EQUED (input or output) CHARACTER*1
* Specifies the form of equilibration that was done.
* = 'N': No equilibration (always true if FACT = 'N').
* = 'R': Row equilibration, i.e., A has been premultiplied by
* diag(R).
* = 'C': Column equilibration, i.e., A has been postmultiplied
* by diag(C).
* = 'B': Both row and column equilibration, i.e., A has been
* replaced by diag(R) * A * diag(C).
* EQUED is an input argument if FACT = 'F'; otherwise, it is an
* output argument.
*
* R (input or output) DOUBLE PRECISION array, dimension (N)
* The row scale factors for A. If EQUED = 'R' or 'B', A is
* multiplied on the left by diag(R); if EQUED = 'N' or 'C', R
* is not accessed. R is an input argument if FACT = 'F';
* otherwise, R is an output argument. If FACT = 'F' and
* EQUED = 'R' or 'B', each element of R must be positive.
*
* C (input or output) DOUBLE PRECISION array, dimension (N)
* The column scale factors for A. If EQUED = 'C' or 'B', A is
* multiplied on the right by diag(C); if EQUED = 'N' or 'R', C
* is not accessed. C is an input argument if FACT = 'F';
* otherwise, C is an output argument. If FACT = 'F' and
* EQUED = 'C' or 'B', each element of C must be positive.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit,
* if EQUED = 'N', B is not modified;
* if TRANS = 'N' and EQUED = 'R' or 'B', B is overwritten by
* diag(R)*B;
* if TRANS = 'T' or 'C' and EQUED = 'C' or 'B', B is
* overwritten by diag(C)*B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* If INFO = 0 or INFO = N+1, the N-by-NRHS solution matrix X
* to the original system of equations. Note that A and B are
* modified on exit if EQUED .ne. 'N', and the solution to the
* equilibrated system is inv(diag(C))*X if TRANS = 'N' and
* EQUED = 'C' or 'B', or inv(diag(R))*X if TRANS = 'T' or 'C'
* and EQUED = 'R' or 'B'.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) DOUBLE PRECISION
* The estimate of the reciprocal condition number of the matrix
* A after equilibration (if done). If RCOND is less than the
* machine precision (in particular, if RCOND = 0), the matrix
* is singular to working precision. This condition is
* indicated by a return code of INFO > 0.
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (3*N)
* On exit, WORK(1) contains the reciprocal pivot growth
* factor norm(A)/norm(U). The "max absolute element" norm is
* used. If WORK(1) is much less than 1, then the stability
* of the LU factorization of the (equilibrated) matrix A
* could be poor. This also means that the solution X, condition
* estimator RCOND, and forward error bound FERR could be
* unreliable. If factorization fails with 0 0: if INFO = i, and i is
* <= N: U(i,i) is exactly zero. The factorization
* has been completed, but the factor U is exactly
* singular, so the solution and error bounds
* could not be computed. RCOND = 0 is returned.
* = N+1: U is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param trans
* @param n
* @param kl
* @param ku
* @param nrhs
* @param ab
* @param ldab
* @param afb
* @param ldafb
* @param ipiv
* @param equed
* @param r
* @param c
* @param b
* @param ldb
* @param x
* @param ldx
* @param rcond
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void dgbsvx(java.lang.String fact, java.lang.String trans, int n, int kl, int ku, int nrhs, double[] ab, int ldab, double[] afb, int ldafb, int[] ipiv, org.netlib.util.StringW equed, double[] r, double[] c, double[] b, int ldb, double[] x, int ldx, org.netlib.util.doubleW rcond, double[] ferr, double[] berr, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGBSVX uses the LU factorization to compute the solution to a real
* system of linear equations A * X = B, A**T * X = B, or A**H * X = B,
* where A is a band matrix of order N with KL subdiagonals and KU
* superdiagonals, and X and B are N-by-NRHS matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed by this subroutine:
*
* 1. If FACT = 'E', real scaling factors are computed to equilibrate
* the system:
* TRANS = 'N': diag(R)*A*diag(C) *inv(diag(C))*X = diag(R)*B
* TRANS = 'T': (diag(R)*A*diag(C))**T *inv(diag(R))*X = diag(C)*B
* TRANS = 'C': (diag(R)*A*diag(C))**H *inv(diag(R))*X = diag(C)*B
* Whether or not the system will be equilibrated depends on the
* scaling of the matrix A, but if equilibration is used, A is
* overwritten by diag(R)*A*diag(C) and B by diag(R)*B (if TRANS='N')
* or diag(C)*B (if TRANS = 'T' or 'C').
*
* 2. If FACT = 'N' or 'E', the LU decomposition is used to factor the
* matrix A (after equilibration if FACT = 'E') as
* A = L * U,
* where L is a product of permutation and unit lower triangular
* matrices with KL subdiagonals, and U is upper triangular with
* KL+KU superdiagonals.
*
* 3. If some U(i,i)=0, so that U is exactly singular, then the routine
* returns with INFO = i. Otherwise, the factored form of A is used
* to estimate the condition number of the matrix A. If the
* reciprocal of the condition number is less than machine precision,
* INFO = N+1 is returned as a warning, but the routine still goes on
* to solve for X and compute error bounds as described below.
*
* 4. The system of equations is solved for X using the factored form
* of A.
*
* 5. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* 6. If equilibration was used, the matrix X is premultiplied by
* diag(C) (if TRANS = 'N') or diag(R) (if TRANS = 'T' or 'C') so
* that it solves the original system before equilibration.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of the matrix A is
* supplied on entry, and if not, whether the matrix A should be
* equilibrated before it is factored.
* = 'F': On entry, AFB and IPIV contain the factored form of
* A. If EQUED is not 'N', the matrix A has been
* equilibrated with scaling factors given by R and C.
* AB, AFB, and IPIV are not modified.
* = 'N': The matrix A will be copied to AFB and factored.
* = 'E': The matrix A will be equilibrated if necessary, then
* copied to AFB and factored.
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations.
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Transpose)
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB,N)
* On entry, the matrix A in band storage, in rows 1 to KL+KU+1.
* The j-th column of A is stored in the j-th column of the
* array AB as follows:
* AB(KU+1+i-j,j) = A(i,j) for max(1,j-KU)<=i<=min(N,j+kl)
*
* If FACT = 'F' and EQUED is not 'N', then A must have been
* equilibrated by the scaling factors in R and/or C. AB is not
* modified if FACT = 'F' or 'N', or if FACT = 'E' and
* EQUED = 'N' on exit.
*
* On exit, if EQUED .ne. 'N', A is scaled as follows:
* EQUED = 'R': A := diag(R) * A
* EQUED = 'C': A := A * diag(C)
* EQUED = 'B': A := diag(R) * A * diag(C).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KL+KU+1.
*
* AFB (input or output) DOUBLE PRECISION array, dimension (LDAFB,N)
* If FACT = 'F', then AFB is an input argument and on entry
* contains details of the LU factorization of the band matrix
* A, as computed by DGBTRF. U is stored as an upper triangular
* band matrix with KL+KU superdiagonals in rows 1 to KL+KU+1,
* and the multipliers used during the factorization are stored
* in rows KL+KU+2 to 2*KL+KU+1. If EQUED .ne. 'N', then AFB is
* the factored form of the equilibrated matrix A.
*
* If FACT = 'N', then AFB is an output argument and on exit
* returns details of the LU factorization of A.
*
* If FACT = 'E', then AFB is an output argument and on exit
* returns details of the LU factorization of the equilibrated
* matrix A (see the description of AB for the form of the
* equilibrated matrix).
*
* LDAFB (input) INTEGER
* The leading dimension of the array AFB. LDAFB >= 2*KL+KU+1.
*
* IPIV (input or output) INTEGER array, dimension (N)
* If FACT = 'F', then IPIV is an input argument and on entry
* contains the pivot indices from the factorization A = L*U
* as computed by DGBTRF; row i of the matrix was interchanged
* with row IPIV(i).
*
* If FACT = 'N', then IPIV is an output argument and on exit
* contains the pivot indices from the factorization A = L*U
* of the original matrix A.
*
* If FACT = 'E', then IPIV is an output argument and on exit
* contains the pivot indices from the factorization A = L*U
* of the equilibrated matrix A.
*
* EQUED (input or output) CHARACTER*1
* Specifies the form of equilibration that was done.
* = 'N': No equilibration (always true if FACT = 'N').
* = 'R': Row equilibration, i.e., A has been premultiplied by
* diag(R).
* = 'C': Column equilibration, i.e., A has been postmultiplied
* by diag(C).
* = 'B': Both row and column equilibration, i.e., A has been
* replaced by diag(R) * A * diag(C).
* EQUED is an input argument if FACT = 'F'; otherwise, it is an
* output argument.
*
* R (input or output) DOUBLE PRECISION array, dimension (N)
* The row scale factors for A. If EQUED = 'R' or 'B', A is
* multiplied on the left by diag(R); if EQUED = 'N' or 'C', R
* is not accessed. R is an input argument if FACT = 'F';
* otherwise, R is an output argument. If FACT = 'F' and
* EQUED = 'R' or 'B', each element of R must be positive.
*
* C (input or output) DOUBLE PRECISION array, dimension (N)
* The column scale factors for A. If EQUED = 'C' or 'B', A is
* multiplied on the right by diag(C); if EQUED = 'N' or 'R', C
* is not accessed. C is an input argument if FACT = 'F';
* otherwise, C is an output argument. If FACT = 'F' and
* EQUED = 'C' or 'B', each element of C must be positive.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit,
* if EQUED = 'N', B is not modified;
* if TRANS = 'N' and EQUED = 'R' or 'B', B is overwritten by
* diag(R)*B;
* if TRANS = 'T' or 'C' and EQUED = 'C' or 'B', B is
* overwritten by diag(C)*B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* If INFO = 0 or INFO = N+1, the N-by-NRHS solution matrix X
* to the original system of equations. Note that A and B are
* modified on exit if EQUED .ne. 'N', and the solution to the
* equilibrated system is inv(diag(C))*X if TRANS = 'N' and
* EQUED = 'C' or 'B', or inv(diag(R))*X if TRANS = 'T' or 'C'
* and EQUED = 'R' or 'B'.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) DOUBLE PRECISION
* The estimate of the reciprocal condition number of the matrix
* A after equilibration (if done). If RCOND is less than the
* machine precision (in particular, if RCOND = 0), the matrix
* is singular to working precision. This condition is
* indicated by a return code of INFO > 0.
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (3*N)
* On exit, WORK(1) contains the reciprocal pivot growth
* factor norm(A)/norm(U). The "max absolute element" norm is
* used. If WORK(1) is much less than 1, then the stability
* of the LU factorization of the (equilibrated) matrix A
* could be poor. This also means that the solution X, condition
* estimator RCOND, and forward error bound FERR could be
* unreliable. If factorization fails with 0 0: if INFO = i, and i is
* <= N: U(i,i) is exactly zero. The factorization
* has been completed, but the factor U is exactly
* singular, so the solution and error bounds
* could not be computed. RCOND = 0 is returned.
* = N+1: U is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param trans
* @param n
* @param kl
* @param ku
* @param nrhs
* @param ab
* @param _ab_offset
* @param ldab
* @param afb
* @param _afb_offset
* @param ldafb
* @param ipiv
* @param _ipiv_offset
* @param equed
* @param r
* @param _r_offset
* @param c
* @param _c_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param rcond
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dgbsvx(java.lang.String fact, java.lang.String trans, int n, int kl, int ku, int nrhs, double[] ab, int _ab_offset, int ldab, double[] afb, int _afb_offset, int ldafb, int[] ipiv, int _ipiv_offset, org.netlib.util.StringW equed, double[] r, int _r_offset, double[] c, int _c_offset, double[] b, int _b_offset, int ldb, double[] x, int _x_offset, int ldx, org.netlib.util.doubleW rcond, double[] ferr, int _ferr_offset, double[] berr, int _berr_offset, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGBTF2 computes an LU factorization of a real m-by-n band matrix A
* using partial pivoting with row interchanges.
*
* This is the unblocked version of the algorithm, calling Level 2 BLAS.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB,N)
* On entry, the matrix A in band storage, in rows KL+1 to
* 2*KL+KU+1; rows 1 to KL of the array need not be set.
* The j-th column of A is stored in the j-th column of the
* array AB as follows:
* AB(kl+ku+1+i-j,j) = A(i,j) for max(1,j-ku)<=i<=min(m,j+kl)
*
* On exit, details of the factorization: U is stored as an
* upper triangular band matrix with KL+KU superdiagonals in
* rows 1 to KL+KU+1, and the multipliers used during the
* factorization are stored in rows KL+KU+2 to 2*KL+KU+1.
* See below for further details.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= 2*KL+KU+1.
*
* IPIV (output) INTEGER array, dimension (min(M,N))
* The pivot indices; for 1 <= i <= min(M,N), row i of the
* matrix was interchanged with row IPIV(i).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = +i, U(i,i) is exactly zero. The factorization
* has been completed, but the factor U is exactly
* singular, and division by zero will occur if it is used
* to solve a system of equations.
*
* Further Details
* ===============
*
* The band storage scheme is illustrated by the following example, when
* M = N = 6, KL = 2, KU = 1:
*
* On entry: On exit:
*
* * * * + + + * * * u14 u25 u36
* * * + + + + * * u13 u24 u35 u46
* * a12 a23 a34 a45 a56 * u12 u23 u34 u45 u56
* a11 a22 a33 a44 a55 a66 u11 u22 u33 u44 u55 u66
* a21 a32 a43 a54 a65 * m21 m32 m43 m54 m65 *
* a31 a42 a53 a64 * * m31 m42 m53 m64 * *
*
* Array elements marked * are not used by the routine; elements marked
* + need not be set on entry, but are required by the routine to store
* elements of U, because of fill-in resulting from the row
* interchanges.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param kl
* @param ku
* @param ab
* @param ldab
* @param ipiv
* @param info
*
*/
abstract public void dgbtf2(int m, int n, int kl, int ku, double[] ab, int ldab, int[] ipiv, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGBTF2 computes an LU factorization of a real m-by-n band matrix A
* using partial pivoting with row interchanges.
*
* This is the unblocked version of the algorithm, calling Level 2 BLAS.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB,N)
* On entry, the matrix A in band storage, in rows KL+1 to
* 2*KL+KU+1; rows 1 to KL of the array need not be set.
* The j-th column of A is stored in the j-th column of the
* array AB as follows:
* AB(kl+ku+1+i-j,j) = A(i,j) for max(1,j-ku)<=i<=min(m,j+kl)
*
* On exit, details of the factorization: U is stored as an
* upper triangular band matrix with KL+KU superdiagonals in
* rows 1 to KL+KU+1, and the multipliers used during the
* factorization are stored in rows KL+KU+2 to 2*KL+KU+1.
* See below for further details.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= 2*KL+KU+1.
*
* IPIV (output) INTEGER array, dimension (min(M,N))
* The pivot indices; for 1 <= i <= min(M,N), row i of the
* matrix was interchanged with row IPIV(i).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = +i, U(i,i) is exactly zero. The factorization
* has been completed, but the factor U is exactly
* singular, and division by zero will occur if it is used
* to solve a system of equations.
*
* Further Details
* ===============
*
* The band storage scheme is illustrated by the following example, when
* M = N = 6, KL = 2, KU = 1:
*
* On entry: On exit:
*
* * * * + + + * * * u14 u25 u36
* * * + + + + * * u13 u24 u35 u46
* * a12 a23 a34 a45 a56 * u12 u23 u34 u45 u56
* a11 a22 a33 a44 a55 a66 u11 u22 u33 u44 u55 u66
* a21 a32 a43 a54 a65 * m21 m32 m43 m54 m65 *
* a31 a42 a53 a64 * * m31 m42 m53 m64 * *
*
* Array elements marked * are not used by the routine; elements marked
* + need not be set on entry, but are required by the routine to store
* elements of U, because of fill-in resulting from the row
* interchanges.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param kl
* @param ku
* @param ab
* @param _ab_offset
* @param ldab
* @param ipiv
* @param _ipiv_offset
* @param info
*
*/
abstract public void dgbtf2(int m, int n, int kl, int ku, double[] ab, int _ab_offset, int ldab, int[] ipiv, int _ipiv_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGBTRF computes an LU factorization of a real m-by-n band matrix A
* using partial pivoting with row interchanges.
*
* This is the blocked version of the algorithm, calling Level 3 BLAS.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB,N)
* On entry, the matrix A in band storage, in rows KL+1 to
* 2*KL+KU+1; rows 1 to KL of the array need not be set.
* The j-th column of A is stored in the j-th column of the
* array AB as follows:
* AB(kl+ku+1+i-j,j) = A(i,j) for max(1,j-ku)<=i<=min(m,j+kl)
*
* On exit, details of the factorization: U is stored as an
* upper triangular band matrix with KL+KU superdiagonals in
* rows 1 to KL+KU+1, and the multipliers used during the
* factorization are stored in rows KL+KU+2 to 2*KL+KU+1.
* See below for further details.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= 2*KL+KU+1.
*
* IPIV (output) INTEGER array, dimension (min(M,N))
* The pivot indices; for 1 <= i <= min(M,N), row i of the
* matrix was interchanged with row IPIV(i).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = +i, U(i,i) is exactly zero. The factorization
* has been completed, but the factor U is exactly
* singular, and division by zero will occur if it is used
* to solve a system of equations.
*
* Further Details
* ===============
*
* The band storage scheme is illustrated by the following example, when
* M = N = 6, KL = 2, KU = 1:
*
* On entry: On exit:
*
* * * * + + + * * * u14 u25 u36
* * * + + + + * * u13 u24 u35 u46
* * a12 a23 a34 a45 a56 * u12 u23 u34 u45 u56
* a11 a22 a33 a44 a55 a66 u11 u22 u33 u44 u55 u66
* a21 a32 a43 a54 a65 * m21 m32 m43 m54 m65 *
* a31 a42 a53 a64 * * m31 m42 m53 m64 * *
*
* Array elements marked * are not used by the routine; elements marked
* + need not be set on entry, but are required by the routine to store
* elements of U because of fill-in resulting from the row interchanges.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param kl
* @param ku
* @param ab
* @param ldab
* @param ipiv
* @param info
*
*/
abstract public void dgbtrf(int m, int n, int kl, int ku, double[] ab, int ldab, int[] ipiv, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGBTRF computes an LU factorization of a real m-by-n band matrix A
* using partial pivoting with row interchanges.
*
* This is the blocked version of the algorithm, calling Level 3 BLAS.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB,N)
* On entry, the matrix A in band storage, in rows KL+1 to
* 2*KL+KU+1; rows 1 to KL of the array need not be set.
* The j-th column of A is stored in the j-th column of the
* array AB as follows:
* AB(kl+ku+1+i-j,j) = A(i,j) for max(1,j-ku)<=i<=min(m,j+kl)
*
* On exit, details of the factorization: U is stored as an
* upper triangular band matrix with KL+KU superdiagonals in
* rows 1 to KL+KU+1, and the multipliers used during the
* factorization are stored in rows KL+KU+2 to 2*KL+KU+1.
* See below for further details.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= 2*KL+KU+1.
*
* IPIV (output) INTEGER array, dimension (min(M,N))
* The pivot indices; for 1 <= i <= min(M,N), row i of the
* matrix was interchanged with row IPIV(i).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = +i, U(i,i) is exactly zero. The factorization
* has been completed, but the factor U is exactly
* singular, and division by zero will occur if it is used
* to solve a system of equations.
*
* Further Details
* ===============
*
* The band storage scheme is illustrated by the following example, when
* M = N = 6, KL = 2, KU = 1:
*
* On entry: On exit:
*
* * * * + + + * * * u14 u25 u36
* * * + + + + * * u13 u24 u35 u46
* * a12 a23 a34 a45 a56 * u12 u23 u34 u45 u56
* a11 a22 a33 a44 a55 a66 u11 u22 u33 u44 u55 u66
* a21 a32 a43 a54 a65 * m21 m32 m43 m54 m65 *
* a31 a42 a53 a64 * * m31 m42 m53 m64 * *
*
* Array elements marked * are not used by the routine; elements marked
* + need not be set on entry, but are required by the routine to store
* elements of U because of fill-in resulting from the row interchanges.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param kl
* @param ku
* @param ab
* @param _ab_offset
* @param ldab
* @param ipiv
* @param _ipiv_offset
* @param info
*
*/
abstract public void dgbtrf(int m, int n, int kl, int ku, double[] ab, int _ab_offset, int ldab, int[] ipiv, int _ipiv_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGBTRS solves a system of linear equations
* A * X = B or A' * X = B
* with a general band matrix A using the LU factorization computed
* by DGBTRF.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations.
* = 'N': A * X = B (No transpose)
* = 'T': A'* X = B (Transpose)
* = 'C': A'* X = B (Conjugate transpose = Transpose)
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AB (input) DOUBLE PRECISION array, dimension (LDAB,N)
* Details of the LU factorization of the band matrix A, as
* computed by DGBTRF. U is stored as an upper triangular band
* matrix with KL+KU superdiagonals in rows 1 to KL+KU+1, and
* the multipliers used during the factorization are stored in
* rows KL+KU+2 to 2*KL+KU+1.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= 2*KL+KU+1.
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices; for 1 <= i <= N, row i of the matrix was
* interchanged with row IPIV(i).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param trans
* @param n
* @param kl
* @param ku
* @param nrhs
* @param ab
* @param ldab
* @param ipiv
* @param b
* @param ldb
* @param info
*
*/
abstract public void dgbtrs(java.lang.String trans, int n, int kl, int ku, int nrhs, double[] ab, int ldab, int[] ipiv, double[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGBTRS solves a system of linear equations
* A * X = B or A' * X = B
* with a general band matrix A using the LU factorization computed
* by DGBTRF.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations.
* = 'N': A * X = B (No transpose)
* = 'T': A'* X = B (Transpose)
* = 'C': A'* X = B (Conjugate transpose = Transpose)
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AB (input) DOUBLE PRECISION array, dimension (LDAB,N)
* Details of the LU factorization of the band matrix A, as
* computed by DGBTRF. U is stored as an upper triangular band
* matrix with KL+KU superdiagonals in rows 1 to KL+KU+1, and
* the multipliers used during the factorization are stored in
* rows KL+KU+2 to 2*KL+KU+1.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= 2*KL+KU+1.
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices; for 1 <= i <= N, row i of the matrix was
* interchanged with row IPIV(i).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param trans
* @param n
* @param kl
* @param ku
* @param nrhs
* @param ab
* @param _ab_offset
* @param ldab
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void dgbtrs(java.lang.String trans, int n, int kl, int ku, int nrhs, double[] ab, int _ab_offset, int ldab, int[] ipiv, int _ipiv_offset, double[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGEBAK forms the right or left eigenvectors of a real general matrix
* by backward transformation on the computed eigenvectors of the
* balanced matrix output by DGEBAL.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* Specifies the type of backward transformation required:
* = 'N', do nothing, return immediately;
* = 'P', do backward transformation for permutation only;
* = 'S', do backward transformation for scaling only;
* = 'B', do backward transformations for both permutation and
* scaling.
* JOB must be the same as the argument JOB supplied to DGEBAL.
*
* SIDE (input) CHARACTER*1
* = 'R': V contains right eigenvectors;
* = 'L': V contains left eigenvectors.
*
* N (input) INTEGER
* The number of rows of the matrix V. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* The integers ILO and IHI determined by DGEBAL.
* 1 <= ILO <= IHI <= N, if N > 0; ILO=1 and IHI=0, if N=0.
*
* SCALE (input) DOUBLE PRECISION array, dimension (N)
* Details of the permutation and scaling factors, as returned
* by DGEBAL.
*
* M (input) INTEGER
* The number of columns of the matrix V. M >= 0.
*
* V (input/output) DOUBLE PRECISION array, dimension (LDV,M)
* On entry, the matrix of right or left eigenvectors to be
* transformed, as returned by DHSEIN or DTREVC.
* On exit, V is overwritten by the transformed eigenvectors.
*
* LDV (input) INTEGER
* The leading dimension of the array V. LDV >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param side
* @param n
* @param ilo
* @param ihi
* @param scale
* @param m
* @param v
* @param ldv
* @param info
*
*/
abstract public void dgebak(java.lang.String job, java.lang.String side, int n, int ilo, int ihi, double[] scale, int m, double[] v, int ldv, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGEBAK forms the right or left eigenvectors of a real general matrix
* by backward transformation on the computed eigenvectors of the
* balanced matrix output by DGEBAL.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* Specifies the type of backward transformation required:
* = 'N', do nothing, return immediately;
* = 'P', do backward transformation for permutation only;
* = 'S', do backward transformation for scaling only;
* = 'B', do backward transformations for both permutation and
* scaling.
* JOB must be the same as the argument JOB supplied to DGEBAL.
*
* SIDE (input) CHARACTER*1
* = 'R': V contains right eigenvectors;
* = 'L': V contains left eigenvectors.
*
* N (input) INTEGER
* The number of rows of the matrix V. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* The integers ILO and IHI determined by DGEBAL.
* 1 <= ILO <= IHI <= N, if N > 0; ILO=1 and IHI=0, if N=0.
*
* SCALE (input) DOUBLE PRECISION array, dimension (N)
* Details of the permutation and scaling factors, as returned
* by DGEBAL.
*
* M (input) INTEGER
* The number of columns of the matrix V. M >= 0.
*
* V (input/output) DOUBLE PRECISION array, dimension (LDV,M)
* On entry, the matrix of right or left eigenvectors to be
* transformed, as returned by DHSEIN or DTREVC.
* On exit, V is overwritten by the transformed eigenvectors.
*
* LDV (input) INTEGER
* The leading dimension of the array V. LDV >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param side
* @param n
* @param ilo
* @param ihi
* @param scale
* @param _scale_offset
* @param m
* @param v
* @param _v_offset
* @param ldv
* @param info
*
*/
abstract public void dgebak(java.lang.String job, java.lang.String side, int n, int ilo, int ihi, double[] scale, int _scale_offset, int m, double[] v, int _v_offset, int ldv, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGEBAL balances a general real matrix A. This involves, first,
* permuting A by a similarity transformation to isolate eigenvalues
* in the first 1 to ILO-1 and last IHI+1 to N elements on the
* diagonal; and second, applying a diagonal similarity transformation
* to rows and columns ILO to IHI to make the rows and columns as
* close in norm as possible. Both steps are optional.
*
* Balancing may reduce the 1-norm of the matrix, and improve the
* accuracy of the computed eigenvalues and/or eigenvectors.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* Specifies the operations to be performed on A:
* = 'N': none: simply set ILO = 1, IHI = N, SCALE(I) = 1.0
* for i = 1,...,N;
* = 'P': permute only;
* = 'S': scale only;
* = 'B': both permute and scale.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the input matrix A.
* On exit, A is overwritten by the balanced matrix.
* If JOB = 'N', A is not referenced.
* See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* ILO (output) INTEGER
* IHI (output) INTEGER
* ILO and IHI are set to integers such that on exit
* A(i,j) = 0 if i > j and j = 1,...,ILO-1 or I = IHI+1,...,N.
* If JOB = 'N' or 'S', ILO = 1 and IHI = N.
*
* SCALE (output) DOUBLE PRECISION array, dimension (N)
* Details of the permutations and scaling factors applied to
* A. If P(j) is the index of the row and column interchanged
* with row and column j and D(j) is the scaling factor
* applied to row and column j, then
* SCALE(j) = P(j) for j = 1,...,ILO-1
* = D(j) for j = ILO,...,IHI
* = P(j) for j = IHI+1,...,N.
* The order in which the interchanges are made is N to IHI+1,
* then 1 to ILO-1.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* The permutations consist of row and column interchanges which put
* the matrix in the form
*
* ( T1 X Y )
* P A P = ( 0 B Z )
* ( 0 0 T2 )
*
* where T1 and T2 are upper triangular matrices whose eigenvalues lie
* along the diagonal. The column indices ILO and IHI mark the starting
* and ending columns of the submatrix B. Balancing consists of applying
* a diagonal similarity transformation inv(D) * B * D to make the
* 1-norms of each row of B and its corresponding column nearly equal.
* The output matrix is
*
* ( T1 X*D Y )
* ( 0 inv(D)*B*D inv(D)*Z ).
* ( 0 0 T2 )
*
* Information about the permutations P and the diagonal matrix D is
* returned in the vector SCALE.
*
* This subroutine is based on the EISPACK routine BALANC.
*
* Modified by Tzu-Yi Chen, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param n
* @param a
* @param lda
* @param ilo
* @param ihi
* @param scale
* @param info
*
*/
abstract public void dgebal(java.lang.String job, int n, double[] a, int lda, org.netlib.util.intW ilo, org.netlib.util.intW ihi, double[] scale, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGEBAL balances a general real matrix A. This involves, first,
* permuting A by a similarity transformation to isolate eigenvalues
* in the first 1 to ILO-1 and last IHI+1 to N elements on the
* diagonal; and second, applying a diagonal similarity transformation
* to rows and columns ILO to IHI to make the rows and columns as
* close in norm as possible. Both steps are optional.
*
* Balancing may reduce the 1-norm of the matrix, and improve the
* accuracy of the computed eigenvalues and/or eigenvectors.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* Specifies the operations to be performed on A:
* = 'N': none: simply set ILO = 1, IHI = N, SCALE(I) = 1.0
* for i = 1,...,N;
* = 'P': permute only;
* = 'S': scale only;
* = 'B': both permute and scale.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the input matrix A.
* On exit, A is overwritten by the balanced matrix.
* If JOB = 'N', A is not referenced.
* See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* ILO (output) INTEGER
* IHI (output) INTEGER
* ILO and IHI are set to integers such that on exit
* A(i,j) = 0 if i > j and j = 1,...,ILO-1 or I = IHI+1,...,N.
* If JOB = 'N' or 'S', ILO = 1 and IHI = N.
*
* SCALE (output) DOUBLE PRECISION array, dimension (N)
* Details of the permutations and scaling factors applied to
* A. If P(j) is the index of the row and column interchanged
* with row and column j and D(j) is the scaling factor
* applied to row and column j, then
* SCALE(j) = P(j) for j = 1,...,ILO-1
* = D(j) for j = ILO,...,IHI
* = P(j) for j = IHI+1,...,N.
* The order in which the interchanges are made is N to IHI+1,
* then 1 to ILO-1.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* The permutations consist of row and column interchanges which put
* the matrix in the form
*
* ( T1 X Y )
* P A P = ( 0 B Z )
* ( 0 0 T2 )
*
* where T1 and T2 are upper triangular matrices whose eigenvalues lie
* along the diagonal. The column indices ILO and IHI mark the starting
* and ending columns of the submatrix B. Balancing consists of applying
* a diagonal similarity transformation inv(D) * B * D to make the
* 1-norms of each row of B and its corresponding column nearly equal.
* The output matrix is
*
* ( T1 X*D Y )
* ( 0 inv(D)*B*D inv(D)*Z ).
* ( 0 0 T2 )
*
* Information about the permutations P and the diagonal matrix D is
* returned in the vector SCALE.
*
* This subroutine is based on the EISPACK routine BALANC.
*
* Modified by Tzu-Yi Chen, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param n
* @param a
* @param _a_offset
* @param lda
* @param ilo
* @param ihi
* @param scale
* @param _scale_offset
* @param info
*
*/
abstract public void dgebal(java.lang.String job, int n, double[] a, int _a_offset, int lda, org.netlib.util.intW ilo, org.netlib.util.intW ihi, double[] scale, int _scale_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGEBD2 reduces a real general m by n matrix A to upper or lower
* bidiagonal form B by an orthogonal transformation: Q' * A * P = B.
*
* If m >= n, B is upper bidiagonal; if m < n, B is lower bidiagonal.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows in the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns in the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the m by n general matrix to be reduced.
* On exit,
* if m >= n, the diagonal and the first superdiagonal are
* overwritten with the upper bidiagonal matrix B; the
* elements below the diagonal, with the array TAUQ, represent
* the orthogonal matrix Q as a product of elementary
* reflectors, and the elements above the first superdiagonal,
* with the array TAUP, represent the orthogonal matrix P as
* a product of elementary reflectors;
* if m < n, the diagonal and the first subdiagonal are
* overwritten with the lower bidiagonal matrix B; the
* elements below the first subdiagonal, with the array TAUQ,
* represent the orthogonal matrix Q as a product of
* elementary reflectors, and the elements above the diagonal,
* with the array TAUP, represent the orthogonal matrix P as
* a product of elementary reflectors.
* See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* D (output) DOUBLE PRECISION array, dimension (min(M,N))
* The diagonal elements of the bidiagonal matrix B:
* D(i) = A(i,i).
*
* E (output) DOUBLE PRECISION array, dimension (min(M,N)-1)
* The off-diagonal elements of the bidiagonal matrix B:
* if m >= n, E(i) = A(i,i+1) for i = 1,2,...,n-1;
* if m < n, E(i) = A(i+1,i) for i = 1,2,...,m-1.
*
* TAUQ (output) DOUBLE PRECISION array dimension (min(M,N))
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix Q. See Further Details.
*
* TAUP (output) DOUBLE PRECISION array, dimension (min(M,N))
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix P. See Further Details.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (max(M,N))
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* The matrices Q and P are represented as products of elementary
* reflectors:
*
* If m >= n,
*
* Q = H(1) H(2) . . . H(n) and P = G(1) G(2) . . . G(n-1)
*
* Each H(i) and G(i) has the form:
*
* H(i) = I - tauq * v * v' and G(i) = I - taup * u * u'
*
* where tauq and taup are real scalars, and v and u are real vectors;
* v(1:i-1) = 0, v(i) = 1, and v(i+1:m) is stored on exit in A(i+1:m,i);
* u(1:i) = 0, u(i+1) = 1, and u(i+2:n) is stored on exit in A(i,i+2:n);
* tauq is stored in TAUQ(i) and taup in TAUP(i).
*
* If m < n,
*
* Q = H(1) H(2) . . . H(m-1) and P = G(1) G(2) . . . G(m)
*
* Each H(i) and G(i) has the form:
*
* H(i) = I - tauq * v * v' and G(i) = I - taup * u * u'
*
* where tauq and taup are real scalars, and v and u are real vectors;
* v(1:i) = 0, v(i+1) = 1, and v(i+2:m) is stored on exit in A(i+2:m,i);
* u(1:i-1) = 0, u(i) = 1, and u(i+1:n) is stored on exit in A(i,i+1:n);
* tauq is stored in TAUQ(i) and taup in TAUP(i).
*
* The contents of A on exit are illustrated by the following examples:
*
* m = 6 and n = 5 (m > n): m = 5 and n = 6 (m < n):
*
* ( d e u1 u1 u1 ) ( d u1 u1 u1 u1 u1 )
* ( v1 d e u2 u2 ) ( e d u2 u2 u2 u2 )
* ( v1 v2 d e u3 ) ( v1 e d u3 u3 u3 )
* ( v1 v2 v3 d e ) ( v1 v2 e d u4 u4 )
* ( v1 v2 v3 v4 d ) ( v1 v2 v3 e d u5 )
* ( v1 v2 v3 v4 v5 )
*
* where d and e denote diagonal and off-diagonal elements of B, vi
* denotes an element of the vector defining H(i), and ui an element of
* the vector defining G(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param d
* @param e
* @param tauq
* @param taup
* @param work
* @param info
*
*/
abstract public void dgebd2(int m, int n, double[] a, int lda, double[] d, double[] e, double[] tauq, double[] taup, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGEBD2 reduces a real general m by n matrix A to upper or lower
* bidiagonal form B by an orthogonal transformation: Q' * A * P = B.
*
* If m >= n, B is upper bidiagonal; if m < n, B is lower bidiagonal.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows in the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns in the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the m by n general matrix to be reduced.
* On exit,
* if m >= n, the diagonal and the first superdiagonal are
* overwritten with the upper bidiagonal matrix B; the
* elements below the diagonal, with the array TAUQ, represent
* the orthogonal matrix Q as a product of elementary
* reflectors, and the elements above the first superdiagonal,
* with the array TAUP, represent the orthogonal matrix P as
* a product of elementary reflectors;
* if m < n, the diagonal and the first subdiagonal are
* overwritten with the lower bidiagonal matrix B; the
* elements below the first subdiagonal, with the array TAUQ,
* represent the orthogonal matrix Q as a product of
* elementary reflectors, and the elements above the diagonal,
* with the array TAUP, represent the orthogonal matrix P as
* a product of elementary reflectors.
* See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* D (output) DOUBLE PRECISION array, dimension (min(M,N))
* The diagonal elements of the bidiagonal matrix B:
* D(i) = A(i,i).
*
* E (output) DOUBLE PRECISION array, dimension (min(M,N)-1)
* The off-diagonal elements of the bidiagonal matrix B:
* if m >= n, E(i) = A(i,i+1) for i = 1,2,...,n-1;
* if m < n, E(i) = A(i+1,i) for i = 1,2,...,m-1.
*
* TAUQ (output) DOUBLE PRECISION array dimension (min(M,N))
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix Q. See Further Details.
*
* TAUP (output) DOUBLE PRECISION array, dimension (min(M,N))
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix P. See Further Details.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (max(M,N))
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* The matrices Q and P are represented as products of elementary
* reflectors:
*
* If m >= n,
*
* Q = H(1) H(2) . . . H(n) and P = G(1) G(2) . . . G(n-1)
*
* Each H(i) and G(i) has the form:
*
* H(i) = I - tauq * v * v' and G(i) = I - taup * u * u'
*
* where tauq and taup are real scalars, and v and u are real vectors;
* v(1:i-1) = 0, v(i) = 1, and v(i+1:m) is stored on exit in A(i+1:m,i);
* u(1:i) = 0, u(i+1) = 1, and u(i+2:n) is stored on exit in A(i,i+2:n);
* tauq is stored in TAUQ(i) and taup in TAUP(i).
*
* If m < n,
*
* Q = H(1) H(2) . . . H(m-1) and P = G(1) G(2) . . . G(m)
*
* Each H(i) and G(i) has the form:
*
* H(i) = I - tauq * v * v' and G(i) = I - taup * u * u'
*
* where tauq and taup are real scalars, and v and u are real vectors;
* v(1:i) = 0, v(i+1) = 1, and v(i+2:m) is stored on exit in A(i+2:m,i);
* u(1:i-1) = 0, u(i) = 1, and u(i+1:n) is stored on exit in A(i,i+1:n);
* tauq is stored in TAUQ(i) and taup in TAUP(i).
*
* The contents of A on exit are illustrated by the following examples:
*
* m = 6 and n = 5 (m > n): m = 5 and n = 6 (m < n):
*
* ( d e u1 u1 u1 ) ( d u1 u1 u1 u1 u1 )
* ( v1 d e u2 u2 ) ( e d u2 u2 u2 u2 )
* ( v1 v2 d e u3 ) ( v1 e d u3 u3 u3 )
* ( v1 v2 v3 d e ) ( v1 v2 e d u4 u4 )
* ( v1 v2 v3 v4 d ) ( v1 v2 v3 e d u5 )
* ( v1 v2 v3 v4 v5 )
*
* where d and e denote diagonal and off-diagonal elements of B, vi
* denotes an element of the vector defining H(i), and ui an element of
* the vector defining G(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param tauq
* @param _tauq_offset
* @param taup
* @param _taup_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dgebd2(int m, int n, double[] a, int _a_offset, int lda, double[] d, int _d_offset, double[] e, int _e_offset, double[] tauq, int _tauq_offset, double[] taup, int _taup_offset, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGEBRD reduces a general real M-by-N matrix A to upper or lower
* bidiagonal form B by an orthogonal transformation: Q**T * A * P = B.
*
* If m >= n, B is upper bidiagonal; if m < n, B is lower bidiagonal.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows in the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns in the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N general matrix to be reduced.
* On exit,
* if m >= n, the diagonal and the first superdiagonal are
* overwritten with the upper bidiagonal matrix B; the
* elements below the diagonal, with the array TAUQ, represent
* the orthogonal matrix Q as a product of elementary
* reflectors, and the elements above the first superdiagonal,
* with the array TAUP, represent the orthogonal matrix P as
* a product of elementary reflectors;
* if m < n, the diagonal and the first subdiagonal are
* overwritten with the lower bidiagonal matrix B; the
* elements below the first subdiagonal, with the array TAUQ,
* represent the orthogonal matrix Q as a product of
* elementary reflectors, and the elements above the diagonal,
* with the array TAUP, represent the orthogonal matrix P as
* a product of elementary reflectors.
* See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* D (output) DOUBLE PRECISION array, dimension (min(M,N))
* The diagonal elements of the bidiagonal matrix B:
* D(i) = A(i,i).
*
* E (output) DOUBLE PRECISION array, dimension (min(M,N)-1)
* The off-diagonal elements of the bidiagonal matrix B:
* if m >= n, E(i) = A(i,i+1) for i = 1,2,...,n-1;
* if m < n, E(i) = A(i+1,i) for i = 1,2,...,m-1.
*
* TAUQ (output) DOUBLE PRECISION array dimension (min(M,N))
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix Q. See Further Details.
*
* TAUP (output) DOUBLE PRECISION array, dimension (min(M,N))
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix P. See Further Details.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of the array WORK. LWORK >= max(1,M,N).
* For optimum performance LWORK >= (M+N)*NB, where NB
* is the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* The matrices Q and P are represented as products of elementary
* reflectors:
*
* If m >= n,
*
* Q = H(1) H(2) . . . H(n) and P = G(1) G(2) . . . G(n-1)
*
* Each H(i) and G(i) has the form:
*
* H(i) = I - tauq * v * v' and G(i) = I - taup * u * u'
*
* where tauq and taup are real scalars, and v and u are real vectors;
* v(1:i-1) = 0, v(i) = 1, and v(i+1:m) is stored on exit in A(i+1:m,i);
* u(1:i) = 0, u(i+1) = 1, and u(i+2:n) is stored on exit in A(i,i+2:n);
* tauq is stored in TAUQ(i) and taup in TAUP(i).
*
* If m < n,
*
* Q = H(1) H(2) . . . H(m-1) and P = G(1) G(2) . . . G(m)
*
* Each H(i) and G(i) has the form:
*
* H(i) = I - tauq * v * v' and G(i) = I - taup * u * u'
*
* where tauq and taup are real scalars, and v and u are real vectors;
* v(1:i) = 0, v(i+1) = 1, and v(i+2:m) is stored on exit in A(i+2:m,i);
* u(1:i-1) = 0, u(i) = 1, and u(i+1:n) is stored on exit in A(i,i+1:n);
* tauq is stored in TAUQ(i) and taup in TAUP(i).
*
* The contents of A on exit are illustrated by the following examples:
*
* m = 6 and n = 5 (m > n): m = 5 and n = 6 (m < n):
*
* ( d e u1 u1 u1 ) ( d u1 u1 u1 u1 u1 )
* ( v1 d e u2 u2 ) ( e d u2 u2 u2 u2 )
* ( v1 v2 d e u3 ) ( v1 e d u3 u3 u3 )
* ( v1 v2 v3 d e ) ( v1 v2 e d u4 u4 )
* ( v1 v2 v3 v4 d ) ( v1 v2 v3 e d u5 )
* ( v1 v2 v3 v4 v5 )
*
* where d and e denote diagonal and off-diagonal elements of B, vi
* denotes an element of the vector defining H(i), and ui an element of
* the vector defining G(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param d
* @param e
* @param tauq
* @param taup
* @param work
* @param lwork
* @param info
*
*/
abstract public void dgebrd(int m, int n, double[] a, int lda, double[] d, double[] e, double[] tauq, double[] taup, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGEBRD reduces a general real M-by-N matrix A to upper or lower
* bidiagonal form B by an orthogonal transformation: Q**T * A * P = B.
*
* If m >= n, B is upper bidiagonal; if m < n, B is lower bidiagonal.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows in the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns in the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N general matrix to be reduced.
* On exit,
* if m >= n, the diagonal and the first superdiagonal are
* overwritten with the upper bidiagonal matrix B; the
* elements below the diagonal, with the array TAUQ, represent
* the orthogonal matrix Q as a product of elementary
* reflectors, and the elements above the first superdiagonal,
* with the array TAUP, represent the orthogonal matrix P as
* a product of elementary reflectors;
* if m < n, the diagonal and the first subdiagonal are
* overwritten with the lower bidiagonal matrix B; the
* elements below the first subdiagonal, with the array TAUQ,
* represent the orthogonal matrix Q as a product of
* elementary reflectors, and the elements above the diagonal,
* with the array TAUP, represent the orthogonal matrix P as
* a product of elementary reflectors.
* See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* D (output) DOUBLE PRECISION array, dimension (min(M,N))
* The diagonal elements of the bidiagonal matrix B:
* D(i) = A(i,i).
*
* E (output) DOUBLE PRECISION array, dimension (min(M,N)-1)
* The off-diagonal elements of the bidiagonal matrix B:
* if m >= n, E(i) = A(i,i+1) for i = 1,2,...,n-1;
* if m < n, E(i) = A(i+1,i) for i = 1,2,...,m-1.
*
* TAUQ (output) DOUBLE PRECISION array dimension (min(M,N))
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix Q. See Further Details.
*
* TAUP (output) DOUBLE PRECISION array, dimension (min(M,N))
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix P. See Further Details.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of the array WORK. LWORK >= max(1,M,N).
* For optimum performance LWORK >= (M+N)*NB, where NB
* is the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* The matrices Q and P are represented as products of elementary
* reflectors:
*
* If m >= n,
*
* Q = H(1) H(2) . . . H(n) and P = G(1) G(2) . . . G(n-1)
*
* Each H(i) and G(i) has the form:
*
* H(i) = I - tauq * v * v' and G(i) = I - taup * u * u'
*
* where tauq and taup are real scalars, and v and u are real vectors;
* v(1:i-1) = 0, v(i) = 1, and v(i+1:m) is stored on exit in A(i+1:m,i);
* u(1:i) = 0, u(i+1) = 1, and u(i+2:n) is stored on exit in A(i,i+2:n);
* tauq is stored in TAUQ(i) and taup in TAUP(i).
*
* If m < n,
*
* Q = H(1) H(2) . . . H(m-1) and P = G(1) G(2) . . . G(m)
*
* Each H(i) and G(i) has the form:
*
* H(i) = I - tauq * v * v' and G(i) = I - taup * u * u'
*
* where tauq and taup are real scalars, and v and u are real vectors;
* v(1:i) = 0, v(i+1) = 1, and v(i+2:m) is stored on exit in A(i+2:m,i);
* u(1:i-1) = 0, u(i) = 1, and u(i+1:n) is stored on exit in A(i,i+1:n);
* tauq is stored in TAUQ(i) and taup in TAUP(i).
*
* The contents of A on exit are illustrated by the following examples:
*
* m = 6 and n = 5 (m > n): m = 5 and n = 6 (m < n):
*
* ( d e u1 u1 u1 ) ( d u1 u1 u1 u1 u1 )
* ( v1 d e u2 u2 ) ( e d u2 u2 u2 u2 )
* ( v1 v2 d e u3 ) ( v1 e d u3 u3 u3 )
* ( v1 v2 v3 d e ) ( v1 v2 e d u4 u4 )
* ( v1 v2 v3 v4 d ) ( v1 v2 v3 e d u5 )
* ( v1 v2 v3 v4 v5 )
*
* where d and e denote diagonal and off-diagonal elements of B, vi
* denotes an element of the vector defining H(i), and ui an element of
* the vector defining G(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param tauq
* @param _tauq_offset
* @param taup
* @param _taup_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dgebrd(int m, int n, double[] a, int _a_offset, int lda, double[] d, int _d_offset, double[] e, int _e_offset, double[] tauq, int _tauq_offset, double[] taup, int _taup_offset, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGECON estimates the reciprocal of the condition number of a general
* real matrix A, in either the 1-norm or the infinity-norm, using
* the LU factorization computed by DGETRF.
*
* An estimate is obtained for norm(inv(A)), and the reciprocal of the
* condition number is computed as
* RCOND = 1 / ( norm(A) * norm(inv(A)) ).
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies whether the 1-norm condition number or the
* infinity-norm condition number is required:
* = '1' or 'O': 1-norm;
* = 'I': Infinity-norm.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The factors L and U from the factorization A = P*L*U
* as computed by DGETRF.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* ANORM (input) DOUBLE PRECISION
* If NORM = '1' or 'O', the 1-norm of the original matrix A.
* If NORM = 'I', the infinity-norm of the original matrix A.
*
* RCOND (output) DOUBLE PRECISION
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(norm(A) * norm(inv(A))).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (4*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param n
* @param a
* @param lda
* @param anorm
* @param rcond
* @param work
* @param iwork
* @param info
*
*/
abstract public void dgecon(java.lang.String norm, int n, double[] a, int lda, double anorm, org.netlib.util.doubleW rcond, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGECON estimates the reciprocal of the condition number of a general
* real matrix A, in either the 1-norm or the infinity-norm, using
* the LU factorization computed by DGETRF.
*
* An estimate is obtained for norm(inv(A)), and the reciprocal of the
* condition number is computed as
* RCOND = 1 / ( norm(A) * norm(inv(A)) ).
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies whether the 1-norm condition number or the
* infinity-norm condition number is required:
* = '1' or 'O': 1-norm;
* = 'I': Infinity-norm.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The factors L and U from the factorization A = P*L*U
* as computed by DGETRF.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* ANORM (input) DOUBLE PRECISION
* If NORM = '1' or 'O', the 1-norm of the original matrix A.
* If NORM = 'I', the infinity-norm of the original matrix A.
*
* RCOND (output) DOUBLE PRECISION
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(norm(A) * norm(inv(A))).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (4*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param n
* @param a
* @param _a_offset
* @param lda
* @param anorm
* @param rcond
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dgecon(java.lang.String norm, int n, double[] a, int _a_offset, int lda, double anorm, org.netlib.util.doubleW rcond, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGEEQU computes row and column scalings intended to equilibrate an
* M-by-N matrix A and reduce its condition number. R returns the row
* scale factors and C the column scale factors, chosen to try to make
* the largest element in each row and column of the matrix B with
* elements B(i,j)=R(i)*A(i,j)*C(j) have absolute value 1.
*
* R(i) and C(j) are restricted to be between SMLNUM = smallest safe
* number and BIGNUM = largest safe number. Use of these scaling
* factors is not guaranteed to reduce the condition number of A but
* works well in practice.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The M-by-N matrix whose equilibration factors are
* to be computed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* R (output) DOUBLE PRECISION array, dimension (M)
* If INFO = 0 or INFO > M, R contains the row scale factors
* for A.
*
* C (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, C contains the column scale factors for A.
*
* ROWCND (output) DOUBLE PRECISION
* If INFO = 0 or INFO > M, ROWCND contains the ratio of the
* smallest R(i) to the largest R(i). If ROWCND >= 0.1 and
* AMAX is neither too large nor too small, it is not worth
* scaling by R.
*
* COLCND (output) DOUBLE PRECISION
* If INFO = 0, COLCND contains the ratio of the smallest
* C(i) to the largest C(i). If COLCND >= 0.1, it is not
* worth scaling by C.
*
* AMAX (output) DOUBLE PRECISION
* Absolute value of largest matrix element. If AMAX is very
* close to overflow or very close to underflow, the matrix
* should be scaled.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= M: the i-th row of A is exactly zero
* > M: the (i-M)-th column of A is exactly zero
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param r
* @param c
* @param rowcnd
* @param colcnd
* @param amax
* @param info
*
*/
abstract public void dgeequ(int m, int n, double[] a, int lda, double[] r, double[] c, org.netlib.util.doubleW rowcnd, org.netlib.util.doubleW colcnd, org.netlib.util.doubleW amax, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGEEQU computes row and column scalings intended to equilibrate an
* M-by-N matrix A and reduce its condition number. R returns the row
* scale factors and C the column scale factors, chosen to try to make
* the largest element in each row and column of the matrix B with
* elements B(i,j)=R(i)*A(i,j)*C(j) have absolute value 1.
*
* R(i) and C(j) are restricted to be between SMLNUM = smallest safe
* number and BIGNUM = largest safe number. Use of these scaling
* factors is not guaranteed to reduce the condition number of A but
* works well in practice.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The M-by-N matrix whose equilibration factors are
* to be computed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* R (output) DOUBLE PRECISION array, dimension (M)
* If INFO = 0 or INFO > M, R contains the row scale factors
* for A.
*
* C (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, C contains the column scale factors for A.
*
* ROWCND (output) DOUBLE PRECISION
* If INFO = 0 or INFO > M, ROWCND contains the ratio of the
* smallest R(i) to the largest R(i). If ROWCND >= 0.1 and
* AMAX is neither too large nor too small, it is not worth
* scaling by R.
*
* COLCND (output) DOUBLE PRECISION
* If INFO = 0, COLCND contains the ratio of the smallest
* C(i) to the largest C(i). If COLCND >= 0.1, it is not
* worth scaling by C.
*
* AMAX (output) DOUBLE PRECISION
* Absolute value of largest matrix element. If AMAX is very
* close to overflow or very close to underflow, the matrix
* should be scaled.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= M: the i-th row of A is exactly zero
* > M: the (i-M)-th column of A is exactly zero
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param r
* @param _r_offset
* @param c
* @param _c_offset
* @param rowcnd
* @param colcnd
* @param amax
* @param info
*
*/
abstract public void dgeequ(int m, int n, double[] a, int _a_offset, int lda, double[] r, int _r_offset, double[] c, int _c_offset, org.netlib.util.doubleW rowcnd, org.netlib.util.doubleW colcnd, org.netlib.util.doubleW amax, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGEES computes for an N-by-N real nonsymmetric matrix A, the
* eigenvalues, the real Schur form T, and, optionally, the matrix of
* Schur vectors Z. This gives the Schur factorization A = Z*T*(Z**T).
*
* Optionally, it also orders the eigenvalues on the diagonal of the
* real Schur form so that selected eigenvalues are at the top left.
* The leading columns of Z then form an orthonormal basis for the
* invariant subspace corresponding to the selected eigenvalues.
*
* A matrix is in real Schur form if it is upper quasi-triangular with
* 1-by-1 and 2-by-2 blocks. 2-by-2 blocks will be standardized in the
* form
* [ a b ]
* [ c a ]
*
* where b*c < 0. The eigenvalues of such a block are a +- sqrt(bc).
*
* Arguments
* =========
*
* JOBVS (input) CHARACTER*1
* = 'N': Schur vectors are not computed;
* = 'V': Schur vectors are computed.
*
* SORT (input) CHARACTER*1
* Specifies whether or not to order the eigenvalues on the
* diagonal of the Schur form.
* = 'N': Eigenvalues are not ordered;
* = 'S': Eigenvalues are ordered (see SELECT).
*
* SELECT (external procedure) LOGICAL FUNCTION of two DOUBLE PRECISION
* SELECT must be declared EXTERNAL in the calling subroutine.
* If SORT = 'S', SELECT is used to select eigenvalues to sort
* to the top left of the Schur form.
* If SORT = 'N', SELECT is not referenced.
* An eigenvalue WR(j)+sqrt(-1)*WI(j) is selected if
* SELECT(WR(j),WI(j)) is true; i.e., if either one of a complex
* conjugate pair of eigenvalues is selected, then both complex
* eigenvalues are selected.
* Note that a selected complex eigenvalue may no longer
* satisfy SELECT(WR(j),WI(j)) = .TRUE. after ordering, since
* ordering may change the value of complex eigenvalues
* (especially if the eigenvalue is ill-conditioned); in this
* case INFO is set to N+2 (see INFO below).
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the N-by-N matrix A.
* On exit, A has been overwritten by its real Schur form T.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* SDIM (output) INTEGER
* If SORT = 'N', SDIM = 0.
* If SORT = 'S', SDIM = number of eigenvalues (after sorting)
* for which SELECT is true. (Complex conjugate
* pairs for which SELECT is true for either
* eigenvalue count as 2.)
*
* WR (output) DOUBLE PRECISION array, dimension (N)
* WI (output) DOUBLE PRECISION array, dimension (N)
* WR and WI contain the real and imaginary parts,
* respectively, of the computed eigenvalues in the same order
* that they appear on the diagonal of the output Schur form T.
* Complex conjugate pairs of eigenvalues will appear
* consecutively with the eigenvalue having the positive
* imaginary part first.
*
* VS (output) DOUBLE PRECISION array, dimension (LDVS,N)
* If JOBVS = 'V', VS contains the orthogonal matrix Z of Schur
* vectors.
* If JOBVS = 'N', VS is not referenced.
*
* LDVS (input) INTEGER
* The leading dimension of the array VS. LDVS >= 1; if
* JOBVS = 'V', LDVS >= N.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) contains the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,3*N).
* For good performance, LWORK must generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* BWORK (workspace) LOGICAL array, dimension (N)
* Not referenced if SORT = 'N'.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, and i is
* <= N: the QR algorithm failed to compute all the
* eigenvalues; elements 1:ILO-1 and i+1:N of WR and WI
* contain those eigenvalues which have converged; if
* JOBVS = 'V', VS contains the matrix which reduces A
* to its partially converged Schur form.
* = N+1: the eigenvalues could not be reordered because some
* eigenvalues were too close to separate (the problem
* is very ill-conditioned);
* = N+2: after reordering, roundoff changed values of some
* complex eigenvalues so that leading eigenvalues in
* the Schur form no longer satisfy SELECT=.TRUE. This
* could also be caused by underflow due to scaling.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobvs
* @param sort
* @param select
* @param n
* @param a
* @param lda
* @param sdim
* @param wr
* @param wi
* @param vs
* @param ldvs
* @param work
* @param lwork
* @param bwork
* @param info
*
*/
abstract public void dgees(java.lang.String jobvs, java.lang.String sort, java.lang.Object select, int n, double[] a, int lda, org.netlib.util.intW sdim, double[] wr, double[] wi, double[] vs, int ldvs, double[] work, int lwork, boolean[] bwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGEES computes for an N-by-N real nonsymmetric matrix A, the
* eigenvalues, the real Schur form T, and, optionally, the matrix of
* Schur vectors Z. This gives the Schur factorization A = Z*T*(Z**T).
*
* Optionally, it also orders the eigenvalues on the diagonal of the
* real Schur form so that selected eigenvalues are at the top left.
* The leading columns of Z then form an orthonormal basis for the
* invariant subspace corresponding to the selected eigenvalues.
*
* A matrix is in real Schur form if it is upper quasi-triangular with
* 1-by-1 and 2-by-2 blocks. 2-by-2 blocks will be standardized in the
* form
* [ a b ]
* [ c a ]
*
* where b*c < 0. The eigenvalues of such a block are a +- sqrt(bc).
*
* Arguments
* =========
*
* JOBVS (input) CHARACTER*1
* = 'N': Schur vectors are not computed;
* = 'V': Schur vectors are computed.
*
* SORT (input) CHARACTER*1
* Specifies whether or not to order the eigenvalues on the
* diagonal of the Schur form.
* = 'N': Eigenvalues are not ordered;
* = 'S': Eigenvalues are ordered (see SELECT).
*
* SELECT (external procedure) LOGICAL FUNCTION of two DOUBLE PRECISION
* SELECT must be declared EXTERNAL in the calling subroutine.
* If SORT = 'S', SELECT is used to select eigenvalues to sort
* to the top left of the Schur form.
* If SORT = 'N', SELECT is not referenced.
* An eigenvalue WR(j)+sqrt(-1)*WI(j) is selected if
* SELECT(WR(j),WI(j)) is true; i.e., if either one of a complex
* conjugate pair of eigenvalues is selected, then both complex
* eigenvalues are selected.
* Note that a selected complex eigenvalue may no longer
* satisfy SELECT(WR(j),WI(j)) = .TRUE. after ordering, since
* ordering may change the value of complex eigenvalues
* (especially if the eigenvalue is ill-conditioned); in this
* case INFO is set to N+2 (see INFO below).
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the N-by-N matrix A.
* On exit, A has been overwritten by its real Schur form T.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* SDIM (output) INTEGER
* If SORT = 'N', SDIM = 0.
* If SORT = 'S', SDIM = number of eigenvalues (after sorting)
* for which SELECT is true. (Complex conjugate
* pairs for which SELECT is true for either
* eigenvalue count as 2.)
*
* WR (output) DOUBLE PRECISION array, dimension (N)
* WI (output) DOUBLE PRECISION array, dimension (N)
* WR and WI contain the real and imaginary parts,
* respectively, of the computed eigenvalues in the same order
* that they appear on the diagonal of the output Schur form T.
* Complex conjugate pairs of eigenvalues will appear
* consecutively with the eigenvalue having the positive
* imaginary part first.
*
* VS (output) DOUBLE PRECISION array, dimension (LDVS,N)
* If JOBVS = 'V', VS contains the orthogonal matrix Z of Schur
* vectors.
* If JOBVS = 'N', VS is not referenced.
*
* LDVS (input) INTEGER
* The leading dimension of the array VS. LDVS >= 1; if
* JOBVS = 'V', LDVS >= N.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) contains the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,3*N).
* For good performance, LWORK must generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* BWORK (workspace) LOGICAL array, dimension (N)
* Not referenced if SORT = 'N'.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, and i is
* <= N: the QR algorithm failed to compute all the
* eigenvalues; elements 1:ILO-1 and i+1:N of WR and WI
* contain those eigenvalues which have converged; if
* JOBVS = 'V', VS contains the matrix which reduces A
* to its partially converged Schur form.
* = N+1: the eigenvalues could not be reordered because some
* eigenvalues were too close to separate (the problem
* is very ill-conditioned);
* = N+2: after reordering, roundoff changed values of some
* complex eigenvalues so that leading eigenvalues in
* the Schur form no longer satisfy SELECT=.TRUE. This
* could also be caused by underflow due to scaling.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobvs
* @param sort
* @param select
* @param n
* @param a
* @param _a_offset
* @param lda
* @param sdim
* @param wr
* @param _wr_offset
* @param wi
* @param _wi_offset
* @param vs
* @param _vs_offset
* @param ldvs
* @param work
* @param _work_offset
* @param lwork
* @param bwork
* @param _bwork_offset
* @param info
*
*/
abstract public void dgees(java.lang.String jobvs, java.lang.String sort, java.lang.Object select, int n, double[] a, int _a_offset, int lda, org.netlib.util.intW sdim, double[] wr, int _wr_offset, double[] wi, int _wi_offset, double[] vs, int _vs_offset, int ldvs, double[] work, int _work_offset, int lwork, boolean[] bwork, int _bwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGEESX computes for an N-by-N real nonsymmetric matrix A, the
* eigenvalues, the real Schur form T, and, optionally, the matrix of
* Schur vectors Z. This gives the Schur factorization A = Z*T*(Z**T).
*
* Optionally, it also orders the eigenvalues on the diagonal of the
* real Schur form so that selected eigenvalues are at the top left;
* computes a reciprocal condition number for the average of the
* selected eigenvalues (RCONDE); and computes a reciprocal condition
* number for the right invariant subspace corresponding to the
* selected eigenvalues (RCONDV). The leading columns of Z form an
* orthonormal basis for this invariant subspace.
*
* For further explanation of the reciprocal condition numbers RCONDE
* and RCONDV, see Section 4.10 of the LAPACK Users' Guide (where
* these quantities are called s and sep respectively).
*
* A real matrix is in real Schur form if it is upper quasi-triangular
* with 1-by-1 and 2-by-2 blocks. 2-by-2 blocks will be standardized in
* the form
* [ a b ]
* [ c a ]
*
* where b*c < 0. The eigenvalues of such a block are a +- sqrt(bc).
*
* Arguments
* =========
*
* JOBVS (input) CHARACTER*1
* = 'N': Schur vectors are not computed;
* = 'V': Schur vectors are computed.
*
* SORT (input) CHARACTER*1
* Specifies whether or not to order the eigenvalues on the
* diagonal of the Schur form.
* = 'N': Eigenvalues are not ordered;
* = 'S': Eigenvalues are ordered (see SELECT).
*
* SELECT (external procedure) LOGICAL FUNCTION of two DOUBLE PRECISION
* SELECT must be declared EXTERNAL in the calling subroutine.
* If SORT = 'S', SELECT is used to select eigenvalues to sort
* to the top left of the Schur form.
* If SORT = 'N', SELECT is not referenced.
* An eigenvalue WR(j)+sqrt(-1)*WI(j) is selected if
* SELECT(WR(j),WI(j)) is true; i.e., if either one of a
* complex conjugate pair of eigenvalues is selected, then both
* are. Note that a selected complex eigenvalue may no longer
* satisfy SELECT(WR(j),WI(j)) = .TRUE. after ordering, since
* ordering may change the value of complex eigenvalues
* (especially if the eigenvalue is ill-conditioned); in this
* case INFO may be set to N+3 (see INFO below).
*
* SENSE (input) CHARACTER*1
* Determines which reciprocal condition numbers are computed.
* = 'N': None are computed;
* = 'E': Computed for average of selected eigenvalues only;
* = 'V': Computed for selected right invariant subspace only;
* = 'B': Computed for both.
* If SENSE = 'E', 'V' or 'B', SORT must equal 'S'.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the N-by-N matrix A.
* On exit, A is overwritten by its real Schur form T.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* SDIM (output) INTEGER
* If SORT = 'N', SDIM = 0.
* If SORT = 'S', SDIM = number of eigenvalues (after sorting)
* for which SELECT is true. (Complex conjugate
* pairs for which SELECT is true for either
* eigenvalue count as 2.)
*
* WR (output) DOUBLE PRECISION array, dimension (N)
* WI (output) DOUBLE PRECISION array, dimension (N)
* WR and WI contain the real and imaginary parts, respectively,
* of the computed eigenvalues, in the same order that they
* appear on the diagonal of the output Schur form T. Complex
* conjugate pairs of eigenvalues appear consecutively with the
* eigenvalue having the positive imaginary part first.
*
* VS (output) DOUBLE PRECISION array, dimension (LDVS,N)
* If JOBVS = 'V', VS contains the orthogonal matrix Z of Schur
* vectors.
* If JOBVS = 'N', VS is not referenced.
*
* LDVS (input) INTEGER
* The leading dimension of the array VS. LDVS >= 1, and if
* JOBVS = 'V', LDVS >= N.
*
* RCONDE (output) DOUBLE PRECISION
* If SENSE = 'E' or 'B', RCONDE contains the reciprocal
* condition number for the average of the selected eigenvalues.
* Not referenced if SENSE = 'N' or 'V'.
*
* RCONDV (output) DOUBLE PRECISION
* If SENSE = 'V' or 'B', RCONDV contains the reciprocal
* condition number for the selected right invariant subspace.
* Not referenced if SENSE = 'N' or 'E'.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,3*N).
* Also, if SENSE = 'E' or 'V' or 'B',
* LWORK >= N+2*SDIM*(N-SDIM), where SDIM is the number of
* selected eigenvalues computed by this routine. Note that
* N+2*SDIM*(N-SDIM) <= N+N*N/2. Note also that an error is only
* returned if LWORK < max(1,3*N), but if SENSE = 'E' or 'V' or
* 'B' this may not be large enough.
* For good performance, LWORK must generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates upper bounds on the optimal sizes of the
* arrays WORK and IWORK, returns these values as the first
* entries of the WORK and IWORK arrays, and no error messages
* related to LWORK or LIWORK are issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* LIWORK >= 1; if SENSE = 'V' or 'B', LIWORK >= SDIM*(N-SDIM).
* Note that SDIM*(N-SDIM) <= N*N/4. Note also that an error is
* only returned if LIWORK < 1, but if SENSE = 'V' or 'B' this
* may not be large enough.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates upper bounds on the optimal sizes of
* the arrays WORK and IWORK, returns these values as the first
* entries of the WORK and IWORK arrays, and no error messages
* related to LWORK or LIWORK are issued by XERBLA.
*
* BWORK (workspace) LOGICAL array, dimension (N)
* Not referenced if SORT = 'N'.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, and i is
* <= N: the QR algorithm failed to compute all the
* eigenvalues; elements 1:ILO-1 and i+1:N of WR and WI
* contain those eigenvalues which have converged; if
* JOBVS = 'V', VS contains the transformation which
* reduces A to its partially converged Schur form.
* = N+1: the eigenvalues could not be reordered because some
* eigenvalues were too close to separate (the problem
* is very ill-conditioned);
* = N+2: after reordering, roundoff changed values of some
* complex eigenvalues so that leading eigenvalues in
* the Schur form no longer satisfy SELECT=.TRUE. This
* could also be caused by underflow due to scaling.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobvs
* @param sort
* @param select
* @param sense
* @param n
* @param a
* @param lda
* @param sdim
* @param wr
* @param wi
* @param vs
* @param ldvs
* @param rconde
* @param rcondv
* @param work
* @param lwork
* @param iwork
* @param liwork
* @param bwork
* @param info
*
*/
abstract public void dgeesx(java.lang.String jobvs, java.lang.String sort, java.lang.Object select, java.lang.String sense, int n, double[] a, int lda, org.netlib.util.intW sdim, double[] wr, double[] wi, double[] vs, int ldvs, org.netlib.util.doubleW rconde, org.netlib.util.doubleW rcondv, double[] work, int lwork, int[] iwork, int liwork, boolean[] bwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGEESX computes for an N-by-N real nonsymmetric matrix A, the
* eigenvalues, the real Schur form T, and, optionally, the matrix of
* Schur vectors Z. This gives the Schur factorization A = Z*T*(Z**T).
*
* Optionally, it also orders the eigenvalues on the diagonal of the
* real Schur form so that selected eigenvalues are at the top left;
* computes a reciprocal condition number for the average of the
* selected eigenvalues (RCONDE); and computes a reciprocal condition
* number for the right invariant subspace corresponding to the
* selected eigenvalues (RCONDV). The leading columns of Z form an
* orthonormal basis for this invariant subspace.
*
* For further explanation of the reciprocal condition numbers RCONDE
* and RCONDV, see Section 4.10 of the LAPACK Users' Guide (where
* these quantities are called s and sep respectively).
*
* A real matrix is in real Schur form if it is upper quasi-triangular
* with 1-by-1 and 2-by-2 blocks. 2-by-2 blocks will be standardized in
* the form
* [ a b ]
* [ c a ]
*
* where b*c < 0. The eigenvalues of such a block are a +- sqrt(bc).
*
* Arguments
* =========
*
* JOBVS (input) CHARACTER*1
* = 'N': Schur vectors are not computed;
* = 'V': Schur vectors are computed.
*
* SORT (input) CHARACTER*1
* Specifies whether or not to order the eigenvalues on the
* diagonal of the Schur form.
* = 'N': Eigenvalues are not ordered;
* = 'S': Eigenvalues are ordered (see SELECT).
*
* SELECT (external procedure) LOGICAL FUNCTION of two DOUBLE PRECISION
* SELECT must be declared EXTERNAL in the calling subroutine.
* If SORT = 'S', SELECT is used to select eigenvalues to sort
* to the top left of the Schur form.
* If SORT = 'N', SELECT is not referenced.
* An eigenvalue WR(j)+sqrt(-1)*WI(j) is selected if
* SELECT(WR(j),WI(j)) is true; i.e., if either one of a
* complex conjugate pair of eigenvalues is selected, then both
* are. Note that a selected complex eigenvalue may no longer
* satisfy SELECT(WR(j),WI(j)) = .TRUE. after ordering, since
* ordering may change the value of complex eigenvalues
* (especially if the eigenvalue is ill-conditioned); in this
* case INFO may be set to N+3 (see INFO below).
*
* SENSE (input) CHARACTER*1
* Determines which reciprocal condition numbers are computed.
* = 'N': None are computed;
* = 'E': Computed for average of selected eigenvalues only;
* = 'V': Computed for selected right invariant subspace only;
* = 'B': Computed for both.
* If SENSE = 'E', 'V' or 'B', SORT must equal 'S'.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the N-by-N matrix A.
* On exit, A is overwritten by its real Schur form T.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* SDIM (output) INTEGER
* If SORT = 'N', SDIM = 0.
* If SORT = 'S', SDIM = number of eigenvalues (after sorting)
* for which SELECT is true. (Complex conjugate
* pairs for which SELECT is true for either
* eigenvalue count as 2.)
*
* WR (output) DOUBLE PRECISION array, dimension (N)
* WI (output) DOUBLE PRECISION array, dimension (N)
* WR and WI contain the real and imaginary parts, respectively,
* of the computed eigenvalues, in the same order that they
* appear on the diagonal of the output Schur form T. Complex
* conjugate pairs of eigenvalues appear consecutively with the
* eigenvalue having the positive imaginary part first.
*
* VS (output) DOUBLE PRECISION array, dimension (LDVS,N)
* If JOBVS = 'V', VS contains the orthogonal matrix Z of Schur
* vectors.
* If JOBVS = 'N', VS is not referenced.
*
* LDVS (input) INTEGER
* The leading dimension of the array VS. LDVS >= 1, and if
* JOBVS = 'V', LDVS >= N.
*
* RCONDE (output) DOUBLE PRECISION
* If SENSE = 'E' or 'B', RCONDE contains the reciprocal
* condition number for the average of the selected eigenvalues.
* Not referenced if SENSE = 'N' or 'V'.
*
* RCONDV (output) DOUBLE PRECISION
* If SENSE = 'V' or 'B', RCONDV contains the reciprocal
* condition number for the selected right invariant subspace.
* Not referenced if SENSE = 'N' or 'E'.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,3*N).
* Also, if SENSE = 'E' or 'V' or 'B',
* LWORK >= N+2*SDIM*(N-SDIM), where SDIM is the number of
* selected eigenvalues computed by this routine. Note that
* N+2*SDIM*(N-SDIM) <= N+N*N/2. Note also that an error is only
* returned if LWORK < max(1,3*N), but if SENSE = 'E' or 'V' or
* 'B' this may not be large enough.
* For good performance, LWORK must generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates upper bounds on the optimal sizes of the
* arrays WORK and IWORK, returns these values as the first
* entries of the WORK and IWORK arrays, and no error messages
* related to LWORK or LIWORK are issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* LIWORK >= 1; if SENSE = 'V' or 'B', LIWORK >= SDIM*(N-SDIM).
* Note that SDIM*(N-SDIM) <= N*N/4. Note also that an error is
* only returned if LIWORK < 1, but if SENSE = 'V' or 'B' this
* may not be large enough.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates upper bounds on the optimal sizes of
* the arrays WORK and IWORK, returns these values as the first
* entries of the WORK and IWORK arrays, and no error messages
* related to LWORK or LIWORK are issued by XERBLA.
*
* BWORK (workspace) LOGICAL array, dimension (N)
* Not referenced if SORT = 'N'.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, and i is
* <= N: the QR algorithm failed to compute all the
* eigenvalues; elements 1:ILO-1 and i+1:N of WR and WI
* contain those eigenvalues which have converged; if
* JOBVS = 'V', VS contains the transformation which
* reduces A to its partially converged Schur form.
* = N+1: the eigenvalues could not be reordered because some
* eigenvalues were too close to separate (the problem
* is very ill-conditioned);
* = N+2: after reordering, roundoff changed values of some
* complex eigenvalues so that leading eigenvalues in
* the Schur form no longer satisfy SELECT=.TRUE. This
* could also be caused by underflow due to scaling.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobvs
* @param sort
* @param select
* @param sense
* @param n
* @param a
* @param _a_offset
* @param lda
* @param sdim
* @param wr
* @param _wr_offset
* @param wi
* @param _wi_offset
* @param vs
* @param _vs_offset
* @param ldvs
* @param rconde
* @param rcondv
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param liwork
* @param bwork
* @param _bwork_offset
* @param info
*
*/
abstract public void dgeesx(java.lang.String jobvs, java.lang.String sort, java.lang.Object select, java.lang.String sense, int n, double[] a, int _a_offset, int lda, org.netlib.util.intW sdim, double[] wr, int _wr_offset, double[] wi, int _wi_offset, double[] vs, int _vs_offset, int ldvs, org.netlib.util.doubleW rconde, org.netlib.util.doubleW rcondv, double[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int liwork, boolean[] bwork, int _bwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGEEV computes for an N-by-N real nonsymmetric matrix A, the
* eigenvalues and, optionally, the left and/or right eigenvectors.
*
* The right eigenvector v(j) of A satisfies
* A * v(j) = lambda(j) * v(j)
* where lambda(j) is its eigenvalue.
* The left eigenvector u(j) of A satisfies
* u(j)**H * A = lambda(j) * u(j)**H
* where u(j)**H denotes the conjugate transpose of u(j).
*
* The computed eigenvectors are normalized to have Euclidean norm
* equal to 1 and largest component real.
*
* Arguments
* =========
*
* JOBVL (input) CHARACTER*1
* = 'N': left eigenvectors of A are not computed;
* = 'V': left eigenvectors of A are computed.
*
* JOBVR (input) CHARACTER*1
* = 'N': right eigenvectors of A are not computed;
* = 'V': right eigenvectors of A are computed.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the N-by-N matrix A.
* On exit, A has been overwritten.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* WR (output) DOUBLE PRECISION array, dimension (N)
* WI (output) DOUBLE PRECISION array, dimension (N)
* WR and WI contain the real and imaginary parts,
* respectively, of the computed eigenvalues. Complex
* conjugate pairs of eigenvalues appear consecutively
* with the eigenvalue having the positive imaginary part
* first.
*
* VL (output) DOUBLE PRECISION array, dimension (LDVL,N)
* If JOBVL = 'V', the left eigenvectors u(j) are stored one
* after another in the columns of VL, in the same order
* as their eigenvalues.
* If JOBVL = 'N', VL is not referenced.
* If the j-th eigenvalue is real, then u(j) = VL(:,j),
* the j-th column of VL.
* If the j-th and (j+1)-st eigenvalues form a complex
* conjugate pair, then u(j) = VL(:,j) + i*VL(:,j+1) and
* u(j+1) = VL(:,j) - i*VL(:,j+1).
*
* LDVL (input) INTEGER
* The leading dimension of the array VL. LDVL >= 1; if
* JOBVL = 'V', LDVL >= N.
*
* VR (output) DOUBLE PRECISION array, dimension (LDVR,N)
* If JOBVR = 'V', the right eigenvectors v(j) are stored one
* after another in the columns of VR, in the same order
* as their eigenvalues.
* If JOBVR = 'N', VR is not referenced.
* If the j-th eigenvalue is real, then v(j) = VR(:,j),
* the j-th column of VR.
* If the j-th and (j+1)-st eigenvalues form a complex
* conjugate pair, then v(j) = VR(:,j) + i*VR(:,j+1) and
* v(j+1) = VR(:,j) - i*VR(:,j+1).
*
* LDVR (input) INTEGER
* The leading dimension of the array VR. LDVR >= 1; if
* JOBVR = 'V', LDVR >= N.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,3*N), and
* if JOBVL = 'V' or JOBVR = 'V', LWORK >= 4*N. For good
* performance, LWORK must generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, the QR algorithm failed to compute all the
* eigenvalues, and no eigenvectors have been computed;
* elements i+1:N of WR and WI contain eigenvalues which
* have converged.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobvl
* @param jobvr
* @param n
* @param a
* @param lda
* @param wr
* @param wi
* @param vl
* @param ldvl
* @param vr
* @param ldvr
* @param work
* @param lwork
* @param info
*
*/
abstract public void dgeev(java.lang.String jobvl, java.lang.String jobvr, int n, double[] a, int lda, double[] wr, double[] wi, double[] vl, int ldvl, double[] vr, int ldvr, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGEEV computes for an N-by-N real nonsymmetric matrix A, the
* eigenvalues and, optionally, the left and/or right eigenvectors.
*
* The right eigenvector v(j) of A satisfies
* A * v(j) = lambda(j) * v(j)
* where lambda(j) is its eigenvalue.
* The left eigenvector u(j) of A satisfies
* u(j)**H * A = lambda(j) * u(j)**H
* where u(j)**H denotes the conjugate transpose of u(j).
*
* The computed eigenvectors are normalized to have Euclidean norm
* equal to 1 and largest component real.
*
* Arguments
* =========
*
* JOBVL (input) CHARACTER*1
* = 'N': left eigenvectors of A are not computed;
* = 'V': left eigenvectors of A are computed.
*
* JOBVR (input) CHARACTER*1
* = 'N': right eigenvectors of A are not computed;
* = 'V': right eigenvectors of A are computed.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the N-by-N matrix A.
* On exit, A has been overwritten.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* WR (output) DOUBLE PRECISION array, dimension (N)
* WI (output) DOUBLE PRECISION array, dimension (N)
* WR and WI contain the real and imaginary parts,
* respectively, of the computed eigenvalues. Complex
* conjugate pairs of eigenvalues appear consecutively
* with the eigenvalue having the positive imaginary part
* first.
*
* VL (output) DOUBLE PRECISION array, dimension (LDVL,N)
* If JOBVL = 'V', the left eigenvectors u(j) are stored one
* after another in the columns of VL, in the same order
* as their eigenvalues.
* If JOBVL = 'N', VL is not referenced.
* If the j-th eigenvalue is real, then u(j) = VL(:,j),
* the j-th column of VL.
* If the j-th and (j+1)-st eigenvalues form a complex
* conjugate pair, then u(j) = VL(:,j) + i*VL(:,j+1) and
* u(j+1) = VL(:,j) - i*VL(:,j+1).
*
* LDVL (input) INTEGER
* The leading dimension of the array VL. LDVL >= 1; if
* JOBVL = 'V', LDVL >= N.
*
* VR (output) DOUBLE PRECISION array, dimension (LDVR,N)
* If JOBVR = 'V', the right eigenvectors v(j) are stored one
* after another in the columns of VR, in the same order
* as their eigenvalues.
* If JOBVR = 'N', VR is not referenced.
* If the j-th eigenvalue is real, then v(j) = VR(:,j),
* the j-th column of VR.
* If the j-th and (j+1)-st eigenvalues form a complex
* conjugate pair, then v(j) = VR(:,j) + i*VR(:,j+1) and
* v(j+1) = VR(:,j) - i*VR(:,j+1).
*
* LDVR (input) INTEGER
* The leading dimension of the array VR. LDVR >= 1; if
* JOBVR = 'V', LDVR >= N.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,3*N), and
* if JOBVL = 'V' or JOBVR = 'V', LWORK >= 4*N. For good
* performance, LWORK must generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, the QR algorithm failed to compute all the
* eigenvalues, and no eigenvectors have been computed;
* elements i+1:N of WR and WI contain eigenvalues which
* have converged.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobvl
* @param jobvr
* @param n
* @param a
* @param _a_offset
* @param lda
* @param wr
* @param _wr_offset
* @param wi
* @param _wi_offset
* @param vl
* @param _vl_offset
* @param ldvl
* @param vr
* @param _vr_offset
* @param ldvr
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dgeev(java.lang.String jobvl, java.lang.String jobvr, int n, double[] a, int _a_offset, int lda, double[] wr, int _wr_offset, double[] wi, int _wi_offset, double[] vl, int _vl_offset, int ldvl, double[] vr, int _vr_offset, int ldvr, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGEEVX computes for an N-by-N real nonsymmetric matrix A, the
* eigenvalues and, optionally, the left and/or right eigenvectors.
*
* Optionally also, it computes a balancing transformation to improve
* the conditioning of the eigenvalues and eigenvectors (ILO, IHI,
* SCALE, and ABNRM), reciprocal condition numbers for the eigenvalues
* (RCONDE), and reciprocal condition numbers for the right
* eigenvectors (RCONDV).
*
* The right eigenvector v(j) of A satisfies
* A * v(j) = lambda(j) * v(j)
* where lambda(j) is its eigenvalue.
* The left eigenvector u(j) of A satisfies
* u(j)**H * A = lambda(j) * u(j)**H
* where u(j)**H denotes the conjugate transpose of u(j).
*
* The computed eigenvectors are normalized to have Euclidean norm
* equal to 1 and largest component real.
*
* Balancing a matrix means permuting the rows and columns to make it
* more nearly upper triangular, and applying a diagonal similarity
* transformation D * A * D**(-1), where D is a diagonal matrix, to
* make its rows and columns closer in norm and the condition numbers
* of its eigenvalues and eigenvectors smaller. The computed
* reciprocal condition numbers correspond to the balanced matrix.
* Permuting rows and columns will not change the condition numbers
* (in exact arithmetic) but diagonal scaling will. For further
* explanation of balancing, see section 4.10.2 of the LAPACK
* Users' Guide.
*
* Arguments
* =========
*
* BALANC (input) CHARACTER*1
* Indicates how the input matrix should be diagonally scaled
* and/or permuted to improve the conditioning of its
* eigenvalues.
* = 'N': Do not diagonally scale or permute;
* = 'P': Perform permutations to make the matrix more nearly
* upper triangular. Do not diagonally scale;
* = 'S': Diagonally scale the matrix, i.e. replace A by
* D*A*D**(-1), where D is a diagonal matrix chosen
* to make the rows and columns of A more equal in
* norm. Do not permute;
* = 'B': Both diagonally scale and permute A.
*
* Computed reciprocal condition numbers will be for the matrix
* after balancing and/or permuting. Permuting does not change
* condition numbers (in exact arithmetic), but balancing does.
*
* JOBVL (input) CHARACTER*1
* = 'N': left eigenvectors of A are not computed;
* = 'V': left eigenvectors of A are computed.
* If SENSE = 'E' or 'B', JOBVL must = 'V'.
*
* JOBVR (input) CHARACTER*1
* = 'N': right eigenvectors of A are not computed;
* = 'V': right eigenvectors of A are computed.
* If SENSE = 'E' or 'B', JOBVR must = 'V'.
*
* SENSE (input) CHARACTER*1
* Determines which reciprocal condition numbers are computed.
* = 'N': None are computed;
* = 'E': Computed for eigenvalues only;
* = 'V': Computed for right eigenvectors only;
* = 'B': Computed for eigenvalues and right eigenvectors.
*
* If SENSE = 'E' or 'B', both left and right eigenvectors
* must also be computed (JOBVL = 'V' and JOBVR = 'V').
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the N-by-N matrix A.
* On exit, A has been overwritten. If JOBVL = 'V' or
* JOBVR = 'V', A contains the real Schur form of the balanced
* version of the input matrix A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* WR (output) DOUBLE PRECISION array, dimension (N)
* WI (output) DOUBLE PRECISION array, dimension (N)
* WR and WI contain the real and imaginary parts,
* respectively, of the computed eigenvalues. Complex
* conjugate pairs of eigenvalues will appear consecutively
* with the eigenvalue having the positive imaginary part
* first.
*
* VL (output) DOUBLE PRECISION array, dimension (LDVL,N)
* If JOBVL = 'V', the left eigenvectors u(j) are stored one
* after another in the columns of VL, in the same order
* as their eigenvalues.
* If JOBVL = 'N', VL is not referenced.
* If the j-th eigenvalue is real, then u(j) = VL(:,j),
* the j-th column of VL.
* If the j-th and (j+1)-st eigenvalues form a complex
* conjugate pair, then u(j) = VL(:,j) + i*VL(:,j+1) and
* u(j+1) = VL(:,j) - i*VL(:,j+1).
*
* LDVL (input) INTEGER
* The leading dimension of the array VL. LDVL >= 1; if
* JOBVL = 'V', LDVL >= N.
*
* VR (output) DOUBLE PRECISION array, dimension (LDVR,N)
* If JOBVR = 'V', the right eigenvectors v(j) are stored one
* after another in the columns of VR, in the same order
* as their eigenvalues.
* If JOBVR = 'N', VR is not referenced.
* If the j-th eigenvalue is real, then v(j) = VR(:,j),
* the j-th column of VR.
* If the j-th and (j+1)-st eigenvalues form a complex
* conjugate pair, then v(j) = VR(:,j) + i*VR(:,j+1) and
* v(j+1) = VR(:,j) - i*VR(:,j+1).
*
* LDVR (input) INTEGER
* The leading dimension of the array VR. LDVR >= 1, and if
* JOBVR = 'V', LDVR >= N.
*
* ILO (output) INTEGER
* IHI (output) INTEGER
* ILO and IHI are integer values determined when A was
* balanced. The balanced A(i,j) = 0 if I > J and
* J = 1,...,ILO-1 or I = IHI+1,...,N.
*
* SCALE (output) DOUBLE PRECISION array, dimension (N)
* Details of the permutations and scaling factors applied
* when balancing A. If P(j) is the index of the row and column
* interchanged with row and column j, and D(j) is the scaling
* factor applied to row and column j, then
* SCALE(J) = P(J), for J = 1,...,ILO-1
* = D(J), for J = ILO,...,IHI
* = P(J) for J = IHI+1,...,N.
* The order in which the interchanges are made is N to IHI+1,
* then 1 to ILO-1.
*
* ABNRM (output) DOUBLE PRECISION
* The one-norm of the balanced matrix (the maximum
* of the sum of absolute values of elements of any column).
*
* RCONDE (output) DOUBLE PRECISION array, dimension (N)
* RCONDE(j) is the reciprocal condition number of the j-th
* eigenvalue.
*
* RCONDV (output) DOUBLE PRECISION array, dimension (N)
* RCONDV(j) is the reciprocal condition number of the j-th
* right eigenvector.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. If SENSE = 'N' or 'E',
* LWORK >= max(1,2*N), and if JOBVL = 'V' or JOBVR = 'V',
* LWORK >= 3*N. If SENSE = 'V' or 'B', LWORK >= N*(N+6).
* For good performance, LWORK must generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (2*N-2)
* If SENSE = 'N' or 'E', not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, the QR algorithm failed to compute all the
* eigenvalues, and no eigenvectors or condition numbers
* have been computed; elements 1:ILO-1 and i+1:N of WR
* and WI contain eigenvalues which have converged.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param balanc
* @param jobvl
* @param jobvr
* @param sense
* @param n
* @param a
* @param lda
* @param wr
* @param wi
* @param vl
* @param ldvl
* @param vr
* @param ldvr
* @param ilo
* @param ihi
* @param scale
* @param abnrm
* @param rconde
* @param rcondv
* @param work
* @param lwork
* @param iwork
* @param info
*
*/
abstract public void dgeevx(java.lang.String balanc, java.lang.String jobvl, java.lang.String jobvr, java.lang.String sense, int n, double[] a, int lda, double[] wr, double[] wi, double[] vl, int ldvl, double[] vr, int ldvr, org.netlib.util.intW ilo, org.netlib.util.intW ihi, double[] scale, org.netlib.util.doubleW abnrm, double[] rconde, double[] rcondv, double[] work, int lwork, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGEEVX computes for an N-by-N real nonsymmetric matrix A, the
* eigenvalues and, optionally, the left and/or right eigenvectors.
*
* Optionally also, it computes a balancing transformation to improve
* the conditioning of the eigenvalues and eigenvectors (ILO, IHI,
* SCALE, and ABNRM), reciprocal condition numbers for the eigenvalues
* (RCONDE), and reciprocal condition numbers for the right
* eigenvectors (RCONDV).
*
* The right eigenvector v(j) of A satisfies
* A * v(j) = lambda(j) * v(j)
* where lambda(j) is its eigenvalue.
* The left eigenvector u(j) of A satisfies
* u(j)**H * A = lambda(j) * u(j)**H
* where u(j)**H denotes the conjugate transpose of u(j).
*
* The computed eigenvectors are normalized to have Euclidean norm
* equal to 1 and largest component real.
*
* Balancing a matrix means permuting the rows and columns to make it
* more nearly upper triangular, and applying a diagonal similarity
* transformation D * A * D**(-1), where D is a diagonal matrix, to
* make its rows and columns closer in norm and the condition numbers
* of its eigenvalues and eigenvectors smaller. The computed
* reciprocal condition numbers correspond to the balanced matrix.
* Permuting rows and columns will not change the condition numbers
* (in exact arithmetic) but diagonal scaling will. For further
* explanation of balancing, see section 4.10.2 of the LAPACK
* Users' Guide.
*
* Arguments
* =========
*
* BALANC (input) CHARACTER*1
* Indicates how the input matrix should be diagonally scaled
* and/or permuted to improve the conditioning of its
* eigenvalues.
* = 'N': Do not diagonally scale or permute;
* = 'P': Perform permutations to make the matrix more nearly
* upper triangular. Do not diagonally scale;
* = 'S': Diagonally scale the matrix, i.e. replace A by
* D*A*D**(-1), where D is a diagonal matrix chosen
* to make the rows and columns of A more equal in
* norm. Do not permute;
* = 'B': Both diagonally scale and permute A.
*
* Computed reciprocal condition numbers will be for the matrix
* after balancing and/or permuting. Permuting does not change
* condition numbers (in exact arithmetic), but balancing does.
*
* JOBVL (input) CHARACTER*1
* = 'N': left eigenvectors of A are not computed;
* = 'V': left eigenvectors of A are computed.
* If SENSE = 'E' or 'B', JOBVL must = 'V'.
*
* JOBVR (input) CHARACTER*1
* = 'N': right eigenvectors of A are not computed;
* = 'V': right eigenvectors of A are computed.
* If SENSE = 'E' or 'B', JOBVR must = 'V'.
*
* SENSE (input) CHARACTER*1
* Determines which reciprocal condition numbers are computed.
* = 'N': None are computed;
* = 'E': Computed for eigenvalues only;
* = 'V': Computed for right eigenvectors only;
* = 'B': Computed for eigenvalues and right eigenvectors.
*
* If SENSE = 'E' or 'B', both left and right eigenvectors
* must also be computed (JOBVL = 'V' and JOBVR = 'V').
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the N-by-N matrix A.
* On exit, A has been overwritten. If JOBVL = 'V' or
* JOBVR = 'V', A contains the real Schur form of the balanced
* version of the input matrix A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* WR (output) DOUBLE PRECISION array, dimension (N)
* WI (output) DOUBLE PRECISION array, dimension (N)
* WR and WI contain the real and imaginary parts,
* respectively, of the computed eigenvalues. Complex
* conjugate pairs of eigenvalues will appear consecutively
* with the eigenvalue having the positive imaginary part
* first.
*
* VL (output) DOUBLE PRECISION array, dimension (LDVL,N)
* If JOBVL = 'V', the left eigenvectors u(j) are stored one
* after another in the columns of VL, in the same order
* as their eigenvalues.
* If JOBVL = 'N', VL is not referenced.
* If the j-th eigenvalue is real, then u(j) = VL(:,j),
* the j-th column of VL.
* If the j-th and (j+1)-st eigenvalues form a complex
* conjugate pair, then u(j) = VL(:,j) + i*VL(:,j+1) and
* u(j+1) = VL(:,j) - i*VL(:,j+1).
*
* LDVL (input) INTEGER
* The leading dimension of the array VL. LDVL >= 1; if
* JOBVL = 'V', LDVL >= N.
*
* VR (output) DOUBLE PRECISION array, dimension (LDVR,N)
* If JOBVR = 'V', the right eigenvectors v(j) are stored one
* after another in the columns of VR, in the same order
* as their eigenvalues.
* If JOBVR = 'N', VR is not referenced.
* If the j-th eigenvalue is real, then v(j) = VR(:,j),
* the j-th column of VR.
* If the j-th and (j+1)-st eigenvalues form a complex
* conjugate pair, then v(j) = VR(:,j) + i*VR(:,j+1) and
* v(j+1) = VR(:,j) - i*VR(:,j+1).
*
* LDVR (input) INTEGER
* The leading dimension of the array VR. LDVR >= 1, and if
* JOBVR = 'V', LDVR >= N.
*
* ILO (output) INTEGER
* IHI (output) INTEGER
* ILO and IHI are integer values determined when A was
* balanced. The balanced A(i,j) = 0 if I > J and
* J = 1,...,ILO-1 or I = IHI+1,...,N.
*
* SCALE (output) DOUBLE PRECISION array, dimension (N)
* Details of the permutations and scaling factors applied
* when balancing A. If P(j) is the index of the row and column
* interchanged with row and column j, and D(j) is the scaling
* factor applied to row and column j, then
* SCALE(J) = P(J), for J = 1,...,ILO-1
* = D(J), for J = ILO,...,IHI
* = P(J) for J = IHI+1,...,N.
* The order in which the interchanges are made is N to IHI+1,
* then 1 to ILO-1.
*
* ABNRM (output) DOUBLE PRECISION
* The one-norm of the balanced matrix (the maximum
* of the sum of absolute values of elements of any column).
*
* RCONDE (output) DOUBLE PRECISION array, dimension (N)
* RCONDE(j) is the reciprocal condition number of the j-th
* eigenvalue.
*
* RCONDV (output) DOUBLE PRECISION array, dimension (N)
* RCONDV(j) is the reciprocal condition number of the j-th
* right eigenvector.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. If SENSE = 'N' or 'E',
* LWORK >= max(1,2*N), and if JOBVL = 'V' or JOBVR = 'V',
* LWORK >= 3*N. If SENSE = 'V' or 'B', LWORK >= N*(N+6).
* For good performance, LWORK must generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (2*N-2)
* If SENSE = 'N' or 'E', not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, the QR algorithm failed to compute all the
* eigenvalues, and no eigenvectors or condition numbers
* have been computed; elements 1:ILO-1 and i+1:N of WR
* and WI contain eigenvalues which have converged.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param balanc
* @param jobvl
* @param jobvr
* @param sense
* @param n
* @param a
* @param _a_offset
* @param lda
* @param wr
* @param _wr_offset
* @param wi
* @param _wi_offset
* @param vl
* @param _vl_offset
* @param ldvl
* @param vr
* @param _vr_offset
* @param ldvr
* @param ilo
* @param ihi
* @param scale
* @param _scale_offset
* @param abnrm
* @param rconde
* @param _rconde_offset
* @param rcondv
* @param _rcondv_offset
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dgeevx(java.lang.String balanc, java.lang.String jobvl, java.lang.String jobvr, java.lang.String sense, int n, double[] a, int _a_offset, int lda, double[] wr, int _wr_offset, double[] wi, int _wi_offset, double[] vl, int _vl_offset, int ldvl, double[] vr, int _vr_offset, int ldvr, org.netlib.util.intW ilo, org.netlib.util.intW ihi, double[] scale, int _scale_offset, org.netlib.util.doubleW abnrm, double[] rconde, int _rconde_offset, double[] rcondv, int _rcondv_offset, double[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* This routine is deprecated and has been replaced by routine DGGES.
*
* DGEGS computes the eigenvalues, real Schur form, and, optionally,
* left and or/right Schur vectors of a real matrix pair (A,B).
* Given two square matrices A and B, the generalized real Schur
* factorization has the form
*
* A = Q*S*Z**T, B = Q*T*Z**T
*
* where Q and Z are orthogonal matrices, T is upper triangular, and S
* is an upper quasi-triangular matrix with 1-by-1 and 2-by-2 diagonal
* blocks, the 2-by-2 blocks corresponding to complex conjugate pairs
* of eigenvalues of (A,B). The columns of Q are the left Schur vectors
* and the columns of Z are the right Schur vectors.
*
* If only the eigenvalues of (A,B) are needed, the driver routine
* DGEGV should be used instead. See DGEGV for a description of the
* eigenvalues of the generalized nonsymmetric eigenvalue problem
* (GNEP).
*
* Arguments
* =========
*
* JOBVSL (input) CHARACTER*1
* = 'N': do not compute the left Schur vectors;
* = 'V': compute the left Schur vectors (returned in VSL).
*
* JOBVSR (input) CHARACTER*1
* = 'N': do not compute the right Schur vectors;
* = 'V': compute the right Schur vectors (returned in VSR).
*
* N (input) INTEGER
* The order of the matrices A, B, VSL, and VSR. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the matrix A.
* On exit, the upper quasi-triangular matrix S from the
* generalized real Schur factorization.
*
* LDA (input) INTEGER
* The leading dimension of A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB, N)
* On entry, the matrix B.
* On exit, the upper triangular matrix T from the generalized
* real Schur factorization.
*
* LDB (input) INTEGER
* The leading dimension of B. LDB >= max(1,N).
*
* ALPHAR (output) DOUBLE PRECISION array, dimension (N)
* The real parts of each scalar alpha defining an eigenvalue
* of GNEP.
*
* ALPHAI (output) DOUBLE PRECISION array, dimension (N)
* The imaginary parts of each scalar alpha defining an
* eigenvalue of GNEP. If ALPHAI(j) is zero, then the j-th
* eigenvalue is real; if positive, then the j-th and (j+1)-st
* eigenvalues are a complex conjugate pair, with
* ALPHAI(j+1) = -ALPHAI(j).
*
* BETA (output) DOUBLE PRECISION array, dimension (N)
* The scalars beta that define the eigenvalues of GNEP.
* Together, the quantities alpha = (ALPHAR(j),ALPHAI(j)) and
* beta = BETA(j) represent the j-th eigenvalue of the matrix
* pair (A,B), in one of the forms lambda = alpha/beta or
* mu = beta/alpha. Since either lambda or mu may overflow,
* they should not, in general, be computed.
*
* VSL (output) DOUBLE PRECISION array, dimension (LDVSL,N)
* If JOBVSL = 'V', the matrix of left Schur vectors Q.
* Not referenced if JOBVSL = 'N'.
*
* LDVSL (input) INTEGER
* The leading dimension of the matrix VSL. LDVSL >=1, and
* if JOBVSL = 'V', LDVSL >= N.
*
* VSR (output) DOUBLE PRECISION array, dimension (LDVSR,N)
* If JOBVSR = 'V', the matrix of right Schur vectors Z.
* Not referenced if JOBVSR = 'N'.
*
* LDVSR (input) INTEGER
* The leading dimension of the matrix VSR. LDVSR >= 1, and
* if JOBVSR = 'V', LDVSR >= N.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,4*N).
* For good performance, LWORK must generally be larger.
* To compute the optimal value of LWORK, call ILAENV to get
* blocksizes (for DGEQRF, DORMQR, and DORGQR.) Then compute:
* NB -- MAX of the blocksizes for DGEQRF, DORMQR, and DORGQR
* The optimal LWORK is 2*N + N*(NB+1).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1,...,N:
* The QZ iteration failed. (A,B) are not in Schur
* form, but ALPHAR(j), ALPHAI(j), and BETA(j) should
* be correct for j=INFO+1,...,N.
* > N: errors that usually indicate LAPACK problems:
* =N+1: error return from DGGBAL
* =N+2: error return from DGEQRF
* =N+3: error return from DORMQR
* =N+4: error return from DORGQR
* =N+5: error return from DGGHRD
* =N+6: error return from DHGEQZ (other than failed
* iteration)
* =N+7: error return from DGGBAK (computing VSL)
* =N+8: error return from DGGBAK (computing VSR)
* =N+9: error return from DLASCL (various places)
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobvsl
* @param jobvsr
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param alphar
* @param alphai
* @param beta
* @param vsl
* @param ldvsl
* @param vsr
* @param ldvsr
* @param work
* @param lwork
* @param info
*
*/
abstract public void dgegs(java.lang.String jobvsl, java.lang.String jobvsr, int n, double[] a, int lda, double[] b, int ldb, double[] alphar, double[] alphai, double[] beta, double[] vsl, int ldvsl, double[] vsr, int ldvsr, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* This routine is deprecated and has been replaced by routine DGGES.
*
* DGEGS computes the eigenvalues, real Schur form, and, optionally,
* left and or/right Schur vectors of a real matrix pair (A,B).
* Given two square matrices A and B, the generalized real Schur
* factorization has the form
*
* A = Q*S*Z**T, B = Q*T*Z**T
*
* where Q and Z are orthogonal matrices, T is upper triangular, and S
* is an upper quasi-triangular matrix with 1-by-1 and 2-by-2 diagonal
* blocks, the 2-by-2 blocks corresponding to complex conjugate pairs
* of eigenvalues of (A,B). The columns of Q are the left Schur vectors
* and the columns of Z are the right Schur vectors.
*
* If only the eigenvalues of (A,B) are needed, the driver routine
* DGEGV should be used instead. See DGEGV for a description of the
* eigenvalues of the generalized nonsymmetric eigenvalue problem
* (GNEP).
*
* Arguments
* =========
*
* JOBVSL (input) CHARACTER*1
* = 'N': do not compute the left Schur vectors;
* = 'V': compute the left Schur vectors (returned in VSL).
*
* JOBVSR (input) CHARACTER*1
* = 'N': do not compute the right Schur vectors;
* = 'V': compute the right Schur vectors (returned in VSR).
*
* N (input) INTEGER
* The order of the matrices A, B, VSL, and VSR. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the matrix A.
* On exit, the upper quasi-triangular matrix S from the
* generalized real Schur factorization.
*
* LDA (input) INTEGER
* The leading dimension of A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB, N)
* On entry, the matrix B.
* On exit, the upper triangular matrix T from the generalized
* real Schur factorization.
*
* LDB (input) INTEGER
* The leading dimension of B. LDB >= max(1,N).
*
* ALPHAR (output) DOUBLE PRECISION array, dimension (N)
* The real parts of each scalar alpha defining an eigenvalue
* of GNEP.
*
* ALPHAI (output) DOUBLE PRECISION array, dimension (N)
* The imaginary parts of each scalar alpha defining an
* eigenvalue of GNEP. If ALPHAI(j) is zero, then the j-th
* eigenvalue is real; if positive, then the j-th and (j+1)-st
* eigenvalues are a complex conjugate pair, with
* ALPHAI(j+1) = -ALPHAI(j).
*
* BETA (output) DOUBLE PRECISION array, dimension (N)
* The scalars beta that define the eigenvalues of GNEP.
* Together, the quantities alpha = (ALPHAR(j),ALPHAI(j)) and
* beta = BETA(j) represent the j-th eigenvalue of the matrix
* pair (A,B), in one of the forms lambda = alpha/beta or
* mu = beta/alpha. Since either lambda or mu may overflow,
* they should not, in general, be computed.
*
* VSL (output) DOUBLE PRECISION array, dimension (LDVSL,N)
* If JOBVSL = 'V', the matrix of left Schur vectors Q.
* Not referenced if JOBVSL = 'N'.
*
* LDVSL (input) INTEGER
* The leading dimension of the matrix VSL. LDVSL >=1, and
* if JOBVSL = 'V', LDVSL >= N.
*
* VSR (output) DOUBLE PRECISION array, dimension (LDVSR,N)
* If JOBVSR = 'V', the matrix of right Schur vectors Z.
* Not referenced if JOBVSR = 'N'.
*
* LDVSR (input) INTEGER
* The leading dimension of the matrix VSR. LDVSR >= 1, and
* if JOBVSR = 'V', LDVSR >= N.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,4*N).
* For good performance, LWORK must generally be larger.
* To compute the optimal value of LWORK, call ILAENV to get
* blocksizes (for DGEQRF, DORMQR, and DORGQR.) Then compute:
* NB -- MAX of the blocksizes for DGEQRF, DORMQR, and DORGQR
* The optimal LWORK is 2*N + N*(NB+1).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1,...,N:
* The QZ iteration failed. (A,B) are not in Schur
* form, but ALPHAR(j), ALPHAI(j), and BETA(j) should
* be correct for j=INFO+1,...,N.
* > N: errors that usually indicate LAPACK problems:
* =N+1: error return from DGGBAL
* =N+2: error return from DGEQRF
* =N+3: error return from DORMQR
* =N+4: error return from DORGQR
* =N+5: error return from DGGHRD
* =N+6: error return from DHGEQZ (other than failed
* iteration)
* =N+7: error return from DGGBAK (computing VSL)
* =N+8: error return from DGGBAK (computing VSR)
* =N+9: error return from DLASCL (various places)
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobvsl
* @param jobvsr
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param alphar
* @param _alphar_offset
* @param alphai
* @param _alphai_offset
* @param beta
* @param _beta_offset
* @param vsl
* @param _vsl_offset
* @param ldvsl
* @param vsr
* @param _vsr_offset
* @param ldvsr
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dgegs(java.lang.String jobvsl, java.lang.String jobvsr, int n, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, double[] alphar, int _alphar_offset, double[] alphai, int _alphai_offset, double[] beta, int _beta_offset, double[] vsl, int _vsl_offset, int ldvsl, double[] vsr, int _vsr_offset, int ldvsr, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* This routine is deprecated and has been replaced by routine DGGEV.
*
* DGEGV computes the eigenvalues and, optionally, the left and/or right
* eigenvectors of a real matrix pair (A,B).
* Given two square matrices A and B,
* the generalized nonsymmetric eigenvalue problem (GNEP) is to find the
* eigenvalues lambda and corresponding (non-zero) eigenvectors x such
* that
*
* A*x = lambda*B*x.
*
* An alternate form is to find the eigenvalues mu and corresponding
* eigenvectors y such that
*
* mu*A*y = B*y.
*
* These two forms are equivalent with mu = 1/lambda and x = y if
* neither lambda nor mu is zero. In order to deal with the case that
* lambda or mu is zero or small, two values alpha and beta are returned
* for each eigenvalue, such that lambda = alpha/beta and
* mu = beta/alpha.
*
* The vectors x and y in the above equations are right eigenvectors of
* the matrix pair (A,B). Vectors u and v satisfying
*
* u**H*A = lambda*u**H*B or mu*v**H*A = v**H*B
*
* are left eigenvectors of (A,B).
*
* Note: this routine performs "full balancing" on A and B -- see
* "Further Details", below.
*
* Arguments
* =========
*
* JOBVL (input) CHARACTER*1
* = 'N': do not compute the left generalized eigenvectors;
* = 'V': compute the left generalized eigenvectors (returned
* in VL).
*
* JOBVR (input) CHARACTER*1
* = 'N': do not compute the right generalized eigenvectors;
* = 'V': compute the right generalized eigenvectors (returned
* in VR).
*
* N (input) INTEGER
* The order of the matrices A, B, VL, and VR. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the matrix A.
* If JOBVL = 'V' or JOBVR = 'V', then on exit A
* contains the real Schur form of A from the generalized Schur
* factorization of the pair (A,B) after balancing.
* If no eigenvectors were computed, then only the diagonal
* blocks from the Schur form will be correct. See DGGHRD and
* DHGEQZ for details.
*
* LDA (input) INTEGER
* The leading dimension of A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB, N)
* On entry, the matrix B.
* If JOBVL = 'V' or JOBVR = 'V', then on exit B contains the
* upper triangular matrix obtained from B in the generalized
* Schur factorization of the pair (A,B) after balancing.
* If no eigenvectors were computed, then only those elements of
* B corresponding to the diagonal blocks from the Schur form of
* A will be correct. See DGGHRD and DHGEQZ for details.
*
* LDB (input) INTEGER
* The leading dimension of B. LDB >= max(1,N).
*
* ALPHAR (output) DOUBLE PRECISION array, dimension (N)
* The real parts of each scalar alpha defining an eigenvalue of
* GNEP.
*
* ALPHAI (output) DOUBLE PRECISION array, dimension (N)
* The imaginary parts of each scalar alpha defining an
* eigenvalue of GNEP. If ALPHAI(j) is zero, then the j-th
* eigenvalue is real; if positive, then the j-th and
* (j+1)-st eigenvalues are a complex conjugate pair, with
* ALPHAI(j+1) = -ALPHAI(j).
*
* BETA (output) DOUBLE PRECISION array, dimension (N)
* The scalars beta that define the eigenvalues of GNEP.
*
* Together, the quantities alpha = (ALPHAR(j),ALPHAI(j)) and
* beta = BETA(j) represent the j-th eigenvalue of the matrix
* pair (A,B), in one of the forms lambda = alpha/beta or
* mu = beta/alpha. Since either lambda or mu may overflow,
* they should not, in general, be computed.
*
* VL (output) DOUBLE PRECISION array, dimension (LDVL,N)
* If JOBVL = 'V', the left eigenvectors u(j) are stored
* in the columns of VL, in the same order as their eigenvalues.
* If the j-th eigenvalue is real, then u(j) = VL(:,j).
* If the j-th and (j+1)-st eigenvalues form a complex conjugate
* pair, then
* u(j) = VL(:,j) + i*VL(:,j+1)
* and
* u(j+1) = VL(:,j) - i*VL(:,j+1).
*
* Each eigenvector is scaled so that its largest component has
* abs(real part) + abs(imag. part) = 1, except for eigenvectors
* corresponding to an eigenvalue with alpha = beta = 0, which
* are set to zero.
* Not referenced if JOBVL = 'N'.
*
* LDVL (input) INTEGER
* The leading dimension of the matrix VL. LDVL >= 1, and
* if JOBVL = 'V', LDVL >= N.
*
* VR (output) DOUBLE PRECISION array, dimension (LDVR,N)
* If JOBVR = 'V', the right eigenvectors x(j) are stored
* in the columns of VR, in the same order as their eigenvalues.
* If the j-th eigenvalue is real, then x(j) = VR(:,j).
* If the j-th and (j+1)-st eigenvalues form a complex conjugate
* pair, then
* x(j) = VR(:,j) + i*VR(:,j+1)
* and
* x(j+1) = VR(:,j) - i*VR(:,j+1).
*
* Each eigenvector is scaled so that its largest component has
* abs(real part) + abs(imag. part) = 1, except for eigenvalues
* corresponding to an eigenvalue with alpha = beta = 0, which
* are set to zero.
* Not referenced if JOBVR = 'N'.
*
* LDVR (input) INTEGER
* The leading dimension of the matrix VR. LDVR >= 1, and
* if JOBVR = 'V', LDVR >= N.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,8*N).
* For good performance, LWORK must generally be larger.
* To compute the optimal value of LWORK, call ILAENV to get
* blocksizes (for DGEQRF, DORMQR, and DORGQR.) Then compute:
* NB -- MAX of the blocksizes for DGEQRF, DORMQR, and DORGQR;
* The optimal LWORK is:
* 2*N + MAX( 6*N, N*(NB+1) ).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1,...,N:
* The QZ iteration failed. No eigenvectors have been
* calculated, but ALPHAR(j), ALPHAI(j), and BETA(j)
* should be correct for j=INFO+1,...,N.
* > N: errors that usually indicate LAPACK problems:
* =N+1: error return from DGGBAL
* =N+2: error return from DGEQRF
* =N+3: error return from DORMQR
* =N+4: error return from DORGQR
* =N+5: error return from DGGHRD
* =N+6: error return from DHGEQZ (other than failed
* iteration)
* =N+7: error return from DTGEVC
* =N+8: error return from DGGBAK (computing VL)
* =N+9: error return from DGGBAK (computing VR)
* =N+10: error return from DLASCL (various calls)
*
* Further Details
* ===============
*
* Balancing
* ---------
*
* This driver calls DGGBAL to both permute and scale rows and columns
* of A and B. The permutations PL and PR are chosen so that PL*A*PR
* and PL*B*R will be upper triangular except for the diagonal blocks
* A(i:j,i:j) and B(i:j,i:j), with i and j as close together as
* possible. The diagonal scaling matrices DL and DR are chosen so
* that the pair DL*PL*A*PR*DR, DL*PL*B*PR*DR have elements close to
* one (except for the elements that start out zero.)
*
* After the eigenvalues and eigenvectors of the balanced matrices
* have been computed, DGGBAK transforms the eigenvectors back to what
* they would have been (in perfect arithmetic) if they had not been
* balanced.
*
* Contents of A and B on Exit
* -------- -- - --- - -- ----
*
* If any eigenvectors are computed (either JOBVL='V' or JOBVR='V' or
* both), then on exit the arrays A and B will contain the real Schur
* form[*] of the "balanced" versions of A and B. If no eigenvectors
* are computed, then only the diagonal blocks will be correct.
*
* [*] See DHGEQZ, DGEGS, or read the book "Matrix Computations",
* by Golub & van Loan, pub. by Johns Hopkins U. Press.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobvl
* @param jobvr
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param alphar
* @param alphai
* @param beta
* @param vl
* @param ldvl
* @param vr
* @param ldvr
* @param work
* @param lwork
* @param info
*
*/
abstract public void dgegv(java.lang.String jobvl, java.lang.String jobvr, int n, double[] a, int lda, double[] b, int ldb, double[] alphar, double[] alphai, double[] beta, double[] vl, int ldvl, double[] vr, int ldvr, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* This routine is deprecated and has been replaced by routine DGGEV.
*
* DGEGV computes the eigenvalues and, optionally, the left and/or right
* eigenvectors of a real matrix pair (A,B).
* Given two square matrices A and B,
* the generalized nonsymmetric eigenvalue problem (GNEP) is to find the
* eigenvalues lambda and corresponding (non-zero) eigenvectors x such
* that
*
* A*x = lambda*B*x.
*
* An alternate form is to find the eigenvalues mu and corresponding
* eigenvectors y such that
*
* mu*A*y = B*y.
*
* These two forms are equivalent with mu = 1/lambda and x = y if
* neither lambda nor mu is zero. In order to deal with the case that
* lambda or mu is zero or small, two values alpha and beta are returned
* for each eigenvalue, such that lambda = alpha/beta and
* mu = beta/alpha.
*
* The vectors x and y in the above equations are right eigenvectors of
* the matrix pair (A,B). Vectors u and v satisfying
*
* u**H*A = lambda*u**H*B or mu*v**H*A = v**H*B
*
* are left eigenvectors of (A,B).
*
* Note: this routine performs "full balancing" on A and B -- see
* "Further Details", below.
*
* Arguments
* =========
*
* JOBVL (input) CHARACTER*1
* = 'N': do not compute the left generalized eigenvectors;
* = 'V': compute the left generalized eigenvectors (returned
* in VL).
*
* JOBVR (input) CHARACTER*1
* = 'N': do not compute the right generalized eigenvectors;
* = 'V': compute the right generalized eigenvectors (returned
* in VR).
*
* N (input) INTEGER
* The order of the matrices A, B, VL, and VR. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the matrix A.
* If JOBVL = 'V' or JOBVR = 'V', then on exit A
* contains the real Schur form of A from the generalized Schur
* factorization of the pair (A,B) after balancing.
* If no eigenvectors were computed, then only the diagonal
* blocks from the Schur form will be correct. See DGGHRD and
* DHGEQZ for details.
*
* LDA (input) INTEGER
* The leading dimension of A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB, N)
* On entry, the matrix B.
* If JOBVL = 'V' or JOBVR = 'V', then on exit B contains the
* upper triangular matrix obtained from B in the generalized
* Schur factorization of the pair (A,B) after balancing.
* If no eigenvectors were computed, then only those elements of
* B corresponding to the diagonal blocks from the Schur form of
* A will be correct. See DGGHRD and DHGEQZ for details.
*
* LDB (input) INTEGER
* The leading dimension of B. LDB >= max(1,N).
*
* ALPHAR (output) DOUBLE PRECISION array, dimension (N)
* The real parts of each scalar alpha defining an eigenvalue of
* GNEP.
*
* ALPHAI (output) DOUBLE PRECISION array, dimension (N)
* The imaginary parts of each scalar alpha defining an
* eigenvalue of GNEP. If ALPHAI(j) is zero, then the j-th
* eigenvalue is real; if positive, then the j-th and
* (j+1)-st eigenvalues are a complex conjugate pair, with
* ALPHAI(j+1) = -ALPHAI(j).
*
* BETA (output) DOUBLE PRECISION array, dimension (N)
* The scalars beta that define the eigenvalues of GNEP.
*
* Together, the quantities alpha = (ALPHAR(j),ALPHAI(j)) and
* beta = BETA(j) represent the j-th eigenvalue of the matrix
* pair (A,B), in one of the forms lambda = alpha/beta or
* mu = beta/alpha. Since either lambda or mu may overflow,
* they should not, in general, be computed.
*
* VL (output) DOUBLE PRECISION array, dimension (LDVL,N)
* If JOBVL = 'V', the left eigenvectors u(j) are stored
* in the columns of VL, in the same order as their eigenvalues.
* If the j-th eigenvalue is real, then u(j) = VL(:,j).
* If the j-th and (j+1)-st eigenvalues form a complex conjugate
* pair, then
* u(j) = VL(:,j) + i*VL(:,j+1)
* and
* u(j+1) = VL(:,j) - i*VL(:,j+1).
*
* Each eigenvector is scaled so that its largest component has
* abs(real part) + abs(imag. part) = 1, except for eigenvectors
* corresponding to an eigenvalue with alpha = beta = 0, which
* are set to zero.
* Not referenced if JOBVL = 'N'.
*
* LDVL (input) INTEGER
* The leading dimension of the matrix VL. LDVL >= 1, and
* if JOBVL = 'V', LDVL >= N.
*
* VR (output) DOUBLE PRECISION array, dimension (LDVR,N)
* If JOBVR = 'V', the right eigenvectors x(j) are stored
* in the columns of VR, in the same order as their eigenvalues.
* If the j-th eigenvalue is real, then x(j) = VR(:,j).
* If the j-th and (j+1)-st eigenvalues form a complex conjugate
* pair, then
* x(j) = VR(:,j) + i*VR(:,j+1)
* and
* x(j+1) = VR(:,j) - i*VR(:,j+1).
*
* Each eigenvector is scaled so that its largest component has
* abs(real part) + abs(imag. part) = 1, except for eigenvalues
* corresponding to an eigenvalue with alpha = beta = 0, which
* are set to zero.
* Not referenced if JOBVR = 'N'.
*
* LDVR (input) INTEGER
* The leading dimension of the matrix VR. LDVR >= 1, and
* if JOBVR = 'V', LDVR >= N.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,8*N).
* For good performance, LWORK must generally be larger.
* To compute the optimal value of LWORK, call ILAENV to get
* blocksizes (for DGEQRF, DORMQR, and DORGQR.) Then compute:
* NB -- MAX of the blocksizes for DGEQRF, DORMQR, and DORGQR;
* The optimal LWORK is:
* 2*N + MAX( 6*N, N*(NB+1) ).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1,...,N:
* The QZ iteration failed. No eigenvectors have been
* calculated, but ALPHAR(j), ALPHAI(j), and BETA(j)
* should be correct for j=INFO+1,...,N.
* > N: errors that usually indicate LAPACK problems:
* =N+1: error return from DGGBAL
* =N+2: error return from DGEQRF
* =N+3: error return from DORMQR
* =N+4: error return from DORGQR
* =N+5: error return from DGGHRD
* =N+6: error return from DHGEQZ (other than failed
* iteration)
* =N+7: error return from DTGEVC
* =N+8: error return from DGGBAK (computing VL)
* =N+9: error return from DGGBAK (computing VR)
* =N+10: error return from DLASCL (various calls)
*
* Further Details
* ===============
*
* Balancing
* ---------
*
* This driver calls DGGBAL to both permute and scale rows and columns
* of A and B. The permutations PL and PR are chosen so that PL*A*PR
* and PL*B*R will be upper triangular except for the diagonal blocks
* A(i:j,i:j) and B(i:j,i:j), with i and j as close together as
* possible. The diagonal scaling matrices DL and DR are chosen so
* that the pair DL*PL*A*PR*DR, DL*PL*B*PR*DR have elements close to
* one (except for the elements that start out zero.)
*
* After the eigenvalues and eigenvectors of the balanced matrices
* have been computed, DGGBAK transforms the eigenvectors back to what
* they would have been (in perfect arithmetic) if they had not been
* balanced.
*
* Contents of A and B on Exit
* -------- -- - --- - -- ----
*
* If any eigenvectors are computed (either JOBVL='V' or JOBVR='V' or
* both), then on exit the arrays A and B will contain the real Schur
* form[*] of the "balanced" versions of A and B. If no eigenvectors
* are computed, then only the diagonal blocks will be correct.
*
* [*] See DHGEQZ, DGEGS, or read the book "Matrix Computations",
* by Golub & van Loan, pub. by Johns Hopkins U. Press.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobvl
* @param jobvr
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param alphar
* @param _alphar_offset
* @param alphai
* @param _alphai_offset
* @param beta
* @param _beta_offset
* @param vl
* @param _vl_offset
* @param ldvl
* @param vr
* @param _vr_offset
* @param ldvr
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dgegv(java.lang.String jobvl, java.lang.String jobvr, int n, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, double[] alphar, int _alphar_offset, double[] alphai, int _alphai_offset, double[] beta, int _beta_offset, double[] vl, int _vl_offset, int ldvl, double[] vr, int _vr_offset, int ldvr, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGEHD2 reduces a real general matrix A to upper Hessenberg form H by
* an orthogonal similarity transformation: Q' * A * Q = H .
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* It is assumed that A is already upper triangular in rows
* and columns 1:ILO-1 and IHI+1:N. ILO and IHI are normally
* set by a previous call to DGEBAL; otherwise they should be
* set to 1 and N respectively. See Further Details.
* 1 <= ILO <= IHI <= max(1,N).
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the n by n general matrix to be reduced.
* On exit, the upper triangle and the first subdiagonal of A
* are overwritten with the upper Hessenberg matrix H, and the
* elements below the first subdiagonal, with the array TAU,
* represent the orthogonal matrix Q as a product of elementary
* reflectors. See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* TAU (output) DOUBLE PRECISION array, dimension (N-1)
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of (ihi-ilo) elementary
* reflectors
*
* Q = H(ilo) H(ilo+1) . . . H(ihi-1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i) = 0, v(i+1) = 1 and v(ihi+1:n) = 0; v(i+2:ihi) is stored on
* exit in A(i+2:ihi,i), and tau in TAU(i).
*
* The contents of A are illustrated by the following example, with
* n = 7, ilo = 2 and ihi = 6:
*
* on entry, on exit,
*
* ( a a a a a a a ) ( a a h h h h a )
* ( a a a a a a ) ( a h h h h a )
* ( a a a a a a ) ( h h h h h h )
* ( a a a a a a ) ( v2 h h h h h )
* ( a a a a a a ) ( v2 v3 h h h h )
* ( a a a a a a ) ( v2 v3 v4 h h h )
* ( a ) ( a )
*
* where a denotes an element of the original matrix A, h denotes a
* modified element of the upper Hessenberg matrix H, and vi denotes an
* element of the vector defining H(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param ilo
* @param ihi
* @param a
* @param lda
* @param tau
* @param work
* @param info
*
*/
abstract public void dgehd2(int n, int ilo, int ihi, double[] a, int lda, double[] tau, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGEHD2 reduces a real general matrix A to upper Hessenberg form H by
* an orthogonal similarity transformation: Q' * A * Q = H .
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* It is assumed that A is already upper triangular in rows
* and columns 1:ILO-1 and IHI+1:N. ILO and IHI are normally
* set by a previous call to DGEBAL; otherwise they should be
* set to 1 and N respectively. See Further Details.
* 1 <= ILO <= IHI <= max(1,N).
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the n by n general matrix to be reduced.
* On exit, the upper triangle and the first subdiagonal of A
* are overwritten with the upper Hessenberg matrix H, and the
* elements below the first subdiagonal, with the array TAU,
* represent the orthogonal matrix Q as a product of elementary
* reflectors. See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* TAU (output) DOUBLE PRECISION array, dimension (N-1)
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of (ihi-ilo) elementary
* reflectors
*
* Q = H(ilo) H(ilo+1) . . . H(ihi-1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i) = 0, v(i+1) = 1 and v(ihi+1:n) = 0; v(i+2:ihi) is stored on
* exit in A(i+2:ihi,i), and tau in TAU(i).
*
* The contents of A are illustrated by the following example, with
* n = 7, ilo = 2 and ihi = 6:
*
* on entry, on exit,
*
* ( a a a a a a a ) ( a a h h h h a )
* ( a a a a a a ) ( a h h h h a )
* ( a a a a a a ) ( h h h h h h )
* ( a a a a a a ) ( v2 h h h h h )
* ( a a a a a a ) ( v2 v3 h h h h )
* ( a a a a a a ) ( v2 v3 v4 h h h )
* ( a ) ( a )
*
* where a denotes an element of the original matrix A, h denotes a
* modified element of the upper Hessenberg matrix H, and vi denotes an
* element of the vector defining H(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param ilo
* @param ihi
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dgehd2(int n, int ilo, int ihi, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGEHRD reduces a real general matrix A to upper Hessenberg form H by
* an orthogonal similarity transformation: Q' * A * Q = H .
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* It is assumed that A is already upper triangular in rows
* and columns 1:ILO-1 and IHI+1:N. ILO and IHI are normally
* set by a previous call to DGEBAL; otherwise they should be
* set to 1 and N respectively. See Further Details.
* 1 <= ILO <= IHI <= N, if N > 0; ILO=1 and IHI=0, if N=0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the N-by-N general matrix to be reduced.
* On exit, the upper triangle and the first subdiagonal of A
* are overwritten with the upper Hessenberg matrix H, and the
* elements below the first subdiagonal, with the array TAU,
* represent the orthogonal matrix Q as a product of elementary
* reflectors. See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* TAU (output) DOUBLE PRECISION array, dimension (N-1)
* The scalar factors of the elementary reflectors (see Further
* Details). Elements 1:ILO-1 and IHI:N-1 of TAU are set to
* zero.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (LWORK)
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of the array WORK. LWORK >= max(1,N).
* For optimum performance LWORK >= N*NB, where NB is the
* optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of (ihi-ilo) elementary
* reflectors
*
* Q = H(ilo) H(ilo+1) . . . H(ihi-1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i) = 0, v(i+1) = 1 and v(ihi+1:n) = 0; v(i+2:ihi) is stored on
* exit in A(i+2:ihi,i), and tau in TAU(i).
*
* The contents of A are illustrated by the following example, with
* n = 7, ilo = 2 and ihi = 6:
*
* on entry, on exit,
*
* ( a a a a a a a ) ( a a h h h h a )
* ( a a a a a a ) ( a h h h h a )
* ( a a a a a a ) ( h h h h h h )
* ( a a a a a a ) ( v2 h h h h h )
* ( a a a a a a ) ( v2 v3 h h h h )
* ( a a a a a a ) ( v2 v3 v4 h h h )
* ( a ) ( a )
*
* where a denotes an element of the original matrix A, h denotes a
* modified element of the upper Hessenberg matrix H, and vi denotes an
* element of the vector defining H(i).
*
* This file is a slight modification of LAPACK-3.0's DGEHRD
* subroutine incorporating improvements proposed by Quintana-Orti and
* Van de Geijn (2005).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param ilo
* @param ihi
* @param a
* @param lda
* @param tau
* @param work
* @param lwork
* @param info
*
*/
abstract public void dgehrd(int n, int ilo, int ihi, double[] a, int lda, double[] tau, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGEHRD reduces a real general matrix A to upper Hessenberg form H by
* an orthogonal similarity transformation: Q' * A * Q = H .
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* It is assumed that A is already upper triangular in rows
* and columns 1:ILO-1 and IHI+1:N. ILO and IHI are normally
* set by a previous call to DGEBAL; otherwise they should be
* set to 1 and N respectively. See Further Details.
* 1 <= ILO <= IHI <= N, if N > 0; ILO=1 and IHI=0, if N=0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the N-by-N general matrix to be reduced.
* On exit, the upper triangle and the first subdiagonal of A
* are overwritten with the upper Hessenberg matrix H, and the
* elements below the first subdiagonal, with the array TAU,
* represent the orthogonal matrix Q as a product of elementary
* reflectors. See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* TAU (output) DOUBLE PRECISION array, dimension (N-1)
* The scalar factors of the elementary reflectors (see Further
* Details). Elements 1:ILO-1 and IHI:N-1 of TAU are set to
* zero.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (LWORK)
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of the array WORK. LWORK >= max(1,N).
* For optimum performance LWORK >= N*NB, where NB is the
* optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of (ihi-ilo) elementary
* reflectors
*
* Q = H(ilo) H(ilo+1) . . . H(ihi-1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i) = 0, v(i+1) = 1 and v(ihi+1:n) = 0; v(i+2:ihi) is stored on
* exit in A(i+2:ihi,i), and tau in TAU(i).
*
* The contents of A are illustrated by the following example, with
* n = 7, ilo = 2 and ihi = 6:
*
* on entry, on exit,
*
* ( a a a a a a a ) ( a a h h h h a )
* ( a a a a a a ) ( a h h h h a )
* ( a a a a a a ) ( h h h h h h )
* ( a a a a a a ) ( v2 h h h h h )
* ( a a a a a a ) ( v2 v3 h h h h )
* ( a a a a a a ) ( v2 v3 v4 h h h )
* ( a ) ( a )
*
* where a denotes an element of the original matrix A, h denotes a
* modified element of the upper Hessenberg matrix H, and vi denotes an
* element of the vector defining H(i).
*
* This file is a slight modification of LAPACK-3.0's DGEHRD
* subroutine incorporating improvements proposed by Quintana-Orti and
* Van de Geijn (2005).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param ilo
* @param ihi
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dgehrd(int n, int ilo, int ihi, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGELQ2 computes an LQ factorization of a real m by n matrix A:
* A = L * Q.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the m by n matrix A.
* On exit, the elements on and below the diagonal of the array
* contain the m by min(m,n) lower trapezoidal matrix L (L is
* lower triangular if m <= n); the elements above the diagonal,
* with the array TAU, represent the orthogonal matrix Q as a
* product of elementary reflectors (see Further Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) DOUBLE PRECISION array, dimension (min(M,N))
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (M)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(k) . . . H(2) H(1), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i-1) = 0 and v(i) = 1; v(i+1:n) is stored on exit in A(i,i+1:n),
* and tau in TAU(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param tau
* @param work
* @param info
*
*/
abstract public void dgelq2(int m, int n, double[] a, int lda, double[] tau, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGELQ2 computes an LQ factorization of a real m by n matrix A:
* A = L * Q.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the m by n matrix A.
* On exit, the elements on and below the diagonal of the array
* contain the m by min(m,n) lower trapezoidal matrix L (L is
* lower triangular if m <= n); the elements above the diagonal,
* with the array TAU, represent the orthogonal matrix Q as a
* product of elementary reflectors (see Further Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) DOUBLE PRECISION array, dimension (min(M,N))
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (M)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(k) . . . H(2) H(1), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i-1) = 0 and v(i) = 1; v(i+1:n) is stored on exit in A(i,i+1:n),
* and tau in TAU(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dgelq2(int m, int n, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGELQF computes an LQ factorization of a real M-by-N matrix A:
* A = L * Q.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, the elements on and below the diagonal of the array
* contain the m-by-min(m,n) lower trapezoidal matrix L (L is
* lower triangular if m <= n); the elements above the diagonal,
* with the array TAU, represent the orthogonal matrix Q as a
* product of elementary reflectors (see Further Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) DOUBLE PRECISION array, dimension (min(M,N))
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,M).
* For optimum performance LWORK >= M*NB, where NB is the
* optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(k) . . . H(2) H(1), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i-1) = 0 and v(i) = 1; v(i+1:n) is stored on exit in A(i,i+1:n),
* and tau in TAU(i).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param tau
* @param work
* @param lwork
* @param info
*
*/
abstract public void dgelqf(int m, int n, double[] a, int lda, double[] tau, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGELQF computes an LQ factorization of a real M-by-N matrix A:
* A = L * Q.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, the elements on and below the diagonal of the array
* contain the m-by-min(m,n) lower trapezoidal matrix L (L is
* lower triangular if m <= n); the elements above the diagonal,
* with the array TAU, represent the orthogonal matrix Q as a
* product of elementary reflectors (see Further Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) DOUBLE PRECISION array, dimension (min(M,N))
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,M).
* For optimum performance LWORK >= M*NB, where NB is the
* optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(k) . . . H(2) H(1), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i-1) = 0 and v(i) = 1; v(i+1:n) is stored on exit in A(i,i+1:n),
* and tau in TAU(i).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dgelqf(int m, int n, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGELS solves overdetermined or underdetermined real linear systems
* involving an M-by-N matrix A, or its transpose, using a QR or LQ
* factorization of A. It is assumed that A has full rank.
*
* The following options are provided:
*
* 1. If TRANS = 'N' and m >= n: find the least squares solution of
* an overdetermined system, i.e., solve the least squares problem
* minimize || B - A*X ||.
*
* 2. If TRANS = 'N' and m < n: find the minimum norm solution of
* an underdetermined system A * X = B.
*
* 3. If TRANS = 'T' and m >= n: find the minimum norm solution of
* an undetermined system A**T * X = B.
*
* 4. If TRANS = 'T' and m < n: find the least squares solution of
* an overdetermined system, i.e., solve the least squares problem
* minimize || B - A**T * X ||.
*
* Several right hand side vectors b and solution vectors x can be
* handled in a single call; they are stored as the columns of the
* M-by-NRHS right hand side matrix B and the N-by-NRHS solution
* matrix X.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* = 'N': the linear system involves A;
* = 'T': the linear system involves A**T.
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of
* columns of the matrices B and X. NRHS >=0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit,
* if M >= N, A is overwritten by details of its QR
* factorization as returned by DGEQRF;
* if M < N, A is overwritten by details of its LQ
* factorization as returned by DGELQF.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the matrix B of right hand side vectors, stored
* columnwise; B is M-by-NRHS if TRANS = 'N', or N-by-NRHS
* if TRANS = 'T'.
* On exit, if INFO = 0, B is overwritten by the solution
* vectors, stored columnwise:
* if TRANS = 'N' and m >= n, rows 1 to n of B contain the least
* squares solution vectors; the residual sum of squares for the
* solution in each column is given by the sum of squares of
* elements N+1 to M in that column;
* if TRANS = 'N' and m < n, rows 1 to N of B contain the
* minimum norm solution vectors;
* if TRANS = 'T' and m >= n, rows 1 to M of B contain the
* minimum norm solution vectors;
* if TRANS = 'T' and m < n, rows 1 to M of B contain the
* least squares solution vectors; the residual sum of squares
* for the solution in each column is given by the sum of
* squares of elements M+1 to N in that column.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= MAX(1,M,N).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* LWORK >= max( 1, MN + max( MN, NRHS ) ).
* For optimal performance,
* LWORK >= max( 1, MN + max( MN, NRHS )*NB ).
* where MN = min(M,N) and NB is the optimum block size.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the i-th diagonal element of the
* triangular factor of A is zero, so that A does not have
* full rank; the least squares solution could not be
* computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param trans
* @param m
* @param n
* @param nrhs
* @param a
* @param lda
* @param b
* @param ldb
* @param work
* @param lwork
* @param info
*
*/
abstract public void dgels(java.lang.String trans, int m, int n, int nrhs, double[] a, int lda, double[] b, int ldb, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGELS solves overdetermined or underdetermined real linear systems
* involving an M-by-N matrix A, or its transpose, using a QR or LQ
* factorization of A. It is assumed that A has full rank.
*
* The following options are provided:
*
* 1. If TRANS = 'N' and m >= n: find the least squares solution of
* an overdetermined system, i.e., solve the least squares problem
* minimize || B - A*X ||.
*
* 2. If TRANS = 'N' and m < n: find the minimum norm solution of
* an underdetermined system A * X = B.
*
* 3. If TRANS = 'T' and m >= n: find the minimum norm solution of
* an undetermined system A**T * X = B.
*
* 4. If TRANS = 'T' and m < n: find the least squares solution of
* an overdetermined system, i.e., solve the least squares problem
* minimize || B - A**T * X ||.
*
* Several right hand side vectors b and solution vectors x can be
* handled in a single call; they are stored as the columns of the
* M-by-NRHS right hand side matrix B and the N-by-NRHS solution
* matrix X.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* = 'N': the linear system involves A;
* = 'T': the linear system involves A**T.
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of
* columns of the matrices B and X. NRHS >=0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit,
* if M >= N, A is overwritten by details of its QR
* factorization as returned by DGEQRF;
* if M < N, A is overwritten by details of its LQ
* factorization as returned by DGELQF.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the matrix B of right hand side vectors, stored
* columnwise; B is M-by-NRHS if TRANS = 'N', or N-by-NRHS
* if TRANS = 'T'.
* On exit, if INFO = 0, B is overwritten by the solution
* vectors, stored columnwise:
* if TRANS = 'N' and m >= n, rows 1 to n of B contain the least
* squares solution vectors; the residual sum of squares for the
* solution in each column is given by the sum of squares of
* elements N+1 to M in that column;
* if TRANS = 'N' and m < n, rows 1 to N of B contain the
* minimum norm solution vectors;
* if TRANS = 'T' and m >= n, rows 1 to M of B contain the
* minimum norm solution vectors;
* if TRANS = 'T' and m < n, rows 1 to M of B contain the
* least squares solution vectors; the residual sum of squares
* for the solution in each column is given by the sum of
* squares of elements M+1 to N in that column.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= MAX(1,M,N).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* LWORK >= max( 1, MN + max( MN, NRHS ) ).
* For optimal performance,
* LWORK >= max( 1, MN + max( MN, NRHS )*NB ).
* where MN = min(M,N) and NB is the optimum block size.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the i-th diagonal element of the
* triangular factor of A is zero, so that A does not have
* full rank; the least squares solution could not be
* computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param trans
* @param m
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dgels(java.lang.String trans, int m, int n, int nrhs, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGELSD computes the minimum-norm solution to a real linear least
* squares problem:
* minimize 2-norm(| b - A*x |)
* using the singular value decomposition (SVD) of A. A is an M-by-N
* matrix which may be rank-deficient.
*
* Several right hand side vectors b and solution vectors x can be
* handled in a single call; they are stored as the columns of the
* M-by-NRHS right hand side matrix B and the N-by-NRHS solution
* matrix X.
*
* The problem is solved in three steps:
* (1) Reduce the coefficient matrix A to bidiagonal form with
* Householder transformations, reducing the original problem
* into a "bidiagonal least squares problem" (BLS)
* (2) Solve the BLS using a divide and conquer approach.
* (3) Apply back all the Householder tranformations to solve
* the original least squares problem.
*
* The effective rank of A is determined by treating as zero those
* singular values which are less than RCOND times the largest singular
* value.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of A. M >= 0.
*
* N (input) INTEGER
* The number of columns of A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, A has been destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the M-by-NRHS right hand side matrix B.
* On exit, B is overwritten by the N-by-NRHS solution
* matrix X. If m >= n and RANK = n, the residual
* sum-of-squares for the solution in the i-th column is given
* by the sum of squares of elements n+1:m in that column.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,max(M,N)).
*
* S (output) DOUBLE PRECISION array, dimension (min(M,N))
* The singular values of A in decreasing order.
* The condition number of A in the 2-norm = S(1)/S(min(m,n)).
*
* RCOND (input) DOUBLE PRECISION
* RCOND is used to determine the effective rank of A.
* Singular values S(i) <= RCOND*S(1) are treated as zero.
* If RCOND < 0, machine precision is used instead.
*
* RANK (output) INTEGER
* The effective rank of A, i.e., the number of singular values
* which are greater than RCOND*S(1).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK must be at least 1.
* The exact minimum amount of workspace needed depends on M,
* N and NRHS. As long as LWORK is at least
* 12*N + 2*N*SMLSIZ + 8*N*NLVL + N*NRHS + (SMLSIZ+1)**2,
* if M is greater than or equal to N or
* 12*M + 2*M*SMLSIZ + 8*M*NLVL + M*NRHS + (SMLSIZ+1)**2,
* if M is less than N, the code will execute correctly.
* SMLSIZ is returned by ILAENV and is equal to the maximum
* size of the subproblems at the bottom of the computation
* tree (usually about 25), and
* NLVL = MAX( 0, INT( LOG_2( MIN( M,N )/(SMLSIZ+1) ) ) + 1 )
* For good performance, LWORK should generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (MAX(1,LIWORK))
* LIWORK >= 3 * MINMN * NLVL + 11 * MINMN,
* where MINMN = MIN( M,N ).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: the algorithm for computing the SVD failed to converge;
* if INFO = i, i off-diagonal elements of an intermediate
* bidiagonal form did not converge to zero.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Ren-Cang Li, Computer Science Division, University of
* California at Berkeley, USA
* Osni Marques, LBNL/NERSC, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param nrhs
* @param a
* @param lda
* @param b
* @param ldb
* @param s
* @param rcond
* @param rank
* @param work
* @param lwork
* @param iwork
* @param info
*
*/
abstract public void dgelsd(int m, int n, int nrhs, double[] a, int lda, double[] b, int ldb, double[] s, double rcond, org.netlib.util.intW rank, double[] work, int lwork, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGELSD computes the minimum-norm solution to a real linear least
* squares problem:
* minimize 2-norm(| b - A*x |)
* using the singular value decomposition (SVD) of A. A is an M-by-N
* matrix which may be rank-deficient.
*
* Several right hand side vectors b and solution vectors x can be
* handled in a single call; they are stored as the columns of the
* M-by-NRHS right hand side matrix B and the N-by-NRHS solution
* matrix X.
*
* The problem is solved in three steps:
* (1) Reduce the coefficient matrix A to bidiagonal form with
* Householder transformations, reducing the original problem
* into a "bidiagonal least squares problem" (BLS)
* (2) Solve the BLS using a divide and conquer approach.
* (3) Apply back all the Householder tranformations to solve
* the original least squares problem.
*
* The effective rank of A is determined by treating as zero those
* singular values which are less than RCOND times the largest singular
* value.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of A. M >= 0.
*
* N (input) INTEGER
* The number of columns of A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, A has been destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the M-by-NRHS right hand side matrix B.
* On exit, B is overwritten by the N-by-NRHS solution
* matrix X. If m >= n and RANK = n, the residual
* sum-of-squares for the solution in the i-th column is given
* by the sum of squares of elements n+1:m in that column.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,max(M,N)).
*
* S (output) DOUBLE PRECISION array, dimension (min(M,N))
* The singular values of A in decreasing order.
* The condition number of A in the 2-norm = S(1)/S(min(m,n)).
*
* RCOND (input) DOUBLE PRECISION
* RCOND is used to determine the effective rank of A.
* Singular values S(i) <= RCOND*S(1) are treated as zero.
* If RCOND < 0, machine precision is used instead.
*
* RANK (output) INTEGER
* The effective rank of A, i.e., the number of singular values
* which are greater than RCOND*S(1).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK must be at least 1.
* The exact minimum amount of workspace needed depends on M,
* N and NRHS. As long as LWORK is at least
* 12*N + 2*N*SMLSIZ + 8*N*NLVL + N*NRHS + (SMLSIZ+1)**2,
* if M is greater than or equal to N or
* 12*M + 2*M*SMLSIZ + 8*M*NLVL + M*NRHS + (SMLSIZ+1)**2,
* if M is less than N, the code will execute correctly.
* SMLSIZ is returned by ILAENV and is equal to the maximum
* size of the subproblems at the bottom of the computation
* tree (usually about 25), and
* NLVL = MAX( 0, INT( LOG_2( MIN( M,N )/(SMLSIZ+1) ) ) + 1 )
* For good performance, LWORK should generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (MAX(1,LIWORK))
* LIWORK >= 3 * MINMN * NLVL + 11 * MINMN,
* where MINMN = MIN( M,N ).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: the algorithm for computing the SVD failed to converge;
* if INFO = i, i off-diagonal elements of an intermediate
* bidiagonal form did not converge to zero.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Ren-Cang Li, Computer Science Division, University of
* California at Berkeley, USA
* Osni Marques, LBNL/NERSC, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param s
* @param _s_offset
* @param rcond
* @param rank
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dgelsd(int m, int n, int nrhs, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, double[] s, int _s_offset, double rcond, org.netlib.util.intW rank, double[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGELSS computes the minimum norm solution to a real linear least
* squares problem:
*
* Minimize 2-norm(| b - A*x |).
*
* using the singular value decomposition (SVD) of A. A is an M-by-N
* matrix which may be rank-deficient.
*
* Several right hand side vectors b and solution vectors x can be
* handled in a single call; they are stored as the columns of the
* M-by-NRHS right hand side matrix B and the N-by-NRHS solution matrix
* X.
*
* The effective rank of A is determined by treating as zero those
* singular values which are less than RCOND times the largest singular
* value.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, the first min(m,n) rows of A are overwritten with
* its right singular vectors, stored rowwise.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the M-by-NRHS right hand side matrix B.
* On exit, B is overwritten by the N-by-NRHS solution
* matrix X. If m >= n and RANK = n, the residual
* sum-of-squares for the solution in the i-th column is given
* by the sum of squares of elements n+1:m in that column.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,max(M,N)).
*
* S (output) DOUBLE PRECISION array, dimension (min(M,N))
* The singular values of A in decreasing order.
* The condition number of A in the 2-norm = S(1)/S(min(m,n)).
*
* RCOND (input) DOUBLE PRECISION
* RCOND is used to determine the effective rank of A.
* Singular values S(i) <= RCOND*S(1) are treated as zero.
* If RCOND < 0, machine precision is used instead.
*
* RANK (output) INTEGER
* The effective rank of A, i.e., the number of singular values
* which are greater than RCOND*S(1).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= 1, and also:
* LWORK >= 3*min(M,N) + max( 2*min(M,N), max(M,N), NRHS )
* For good performance, LWORK should generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: the algorithm for computing the SVD failed to converge;
* if INFO = i, i off-diagonal elements of an intermediate
* bidiagonal form did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param nrhs
* @param a
* @param lda
* @param b
* @param ldb
* @param s
* @param rcond
* @param rank
* @param work
* @param lwork
* @param info
*
*/
abstract public void dgelss(int m, int n, int nrhs, double[] a, int lda, double[] b, int ldb, double[] s, double rcond, org.netlib.util.intW rank, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGELSS computes the minimum norm solution to a real linear least
* squares problem:
*
* Minimize 2-norm(| b - A*x |).
*
* using the singular value decomposition (SVD) of A. A is an M-by-N
* matrix which may be rank-deficient.
*
* Several right hand side vectors b and solution vectors x can be
* handled in a single call; they are stored as the columns of the
* M-by-NRHS right hand side matrix B and the N-by-NRHS solution matrix
* X.
*
* The effective rank of A is determined by treating as zero those
* singular values which are less than RCOND times the largest singular
* value.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, the first min(m,n) rows of A are overwritten with
* its right singular vectors, stored rowwise.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the M-by-NRHS right hand side matrix B.
* On exit, B is overwritten by the N-by-NRHS solution
* matrix X. If m >= n and RANK = n, the residual
* sum-of-squares for the solution in the i-th column is given
* by the sum of squares of elements n+1:m in that column.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,max(M,N)).
*
* S (output) DOUBLE PRECISION array, dimension (min(M,N))
* The singular values of A in decreasing order.
* The condition number of A in the 2-norm = S(1)/S(min(m,n)).
*
* RCOND (input) DOUBLE PRECISION
* RCOND is used to determine the effective rank of A.
* Singular values S(i) <= RCOND*S(1) are treated as zero.
* If RCOND < 0, machine precision is used instead.
*
* RANK (output) INTEGER
* The effective rank of A, i.e., the number of singular values
* which are greater than RCOND*S(1).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= 1, and also:
* LWORK >= 3*min(M,N) + max( 2*min(M,N), max(M,N), NRHS )
* For good performance, LWORK should generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: the algorithm for computing the SVD failed to converge;
* if INFO = i, i off-diagonal elements of an intermediate
* bidiagonal form did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param s
* @param _s_offset
* @param rcond
* @param rank
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dgelss(int m, int n, int nrhs, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, double[] s, int _s_offset, double rcond, org.netlib.util.intW rank, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* This routine is deprecated and has been replaced by routine DGELSY.
*
* DGELSX computes the minimum-norm solution to a real linear least
* squares problem:
* minimize || A * X - B ||
* using a complete orthogonal factorization of A. A is an M-by-N
* matrix which may be rank-deficient.
*
* Several right hand side vectors b and solution vectors x can be
* handled in a single call; they are stored as the columns of the
* M-by-NRHS right hand side matrix B and the N-by-NRHS solution
* matrix X.
*
* The routine first computes a QR factorization with column pivoting:
* A * P = Q * [ R11 R12 ]
* [ 0 R22 ]
* with R11 defined as the largest leading submatrix whose estimated
* condition number is less than 1/RCOND. The order of R11, RANK,
* is the effective rank of A.
*
* Then, R22 is considered to be negligible, and R12 is annihilated
* by orthogonal transformations from the right, arriving at the
* complete orthogonal factorization:
* A * P = Q * [ T11 0 ] * Z
* [ 0 0 ]
* The minimum-norm solution is then
* X = P * Z' [ inv(T11)*Q1'*B ]
* [ 0 ]
* where Q1 consists of the first RANK columns of Q.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of
* columns of matrices B and X. NRHS >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, A has been overwritten by details of its
* complete orthogonal factorization.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the M-by-NRHS right hand side matrix B.
* On exit, the N-by-NRHS solution matrix X.
* If m >= n and RANK = n, the residual sum-of-squares for
* the solution in the i-th column is given by the sum of
* squares of elements N+1:M in that column.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,M,N).
*
* JPVT (input/output) INTEGER array, dimension (N)
* On entry, if JPVT(i) .ne. 0, the i-th column of A is an
* initial column, otherwise it is a free column. Before
* the QR factorization of A, all initial columns are
* permuted to the leading positions; only the remaining
* free columns are moved as a result of column pivoting
* during the factorization.
* On exit, if JPVT(i) = k, then the i-th column of A*P
* was the k-th column of A.
*
* RCOND (input) DOUBLE PRECISION
* RCOND is used to determine the effective rank of A, which
* is defined as the order of the largest leading triangular
* submatrix R11 in the QR factorization with pivoting of A,
* whose estimated condition number < 1/RCOND.
*
* RANK (output) INTEGER
* The effective rank of A, i.e., the order of the submatrix
* R11. This is the same as the order of the submatrix T11
* in the complete orthogonal factorization of A.
*
* WORK (workspace) DOUBLE PRECISION array, dimension
* (max( min(M,N)+3*N, 2*min(M,N)+NRHS )),
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param nrhs
* @param a
* @param lda
* @param b
* @param ldb
* @param jpvt
* @param rcond
* @param rank
* @param work
* @param info
*
*/
abstract public void dgelsx(int m, int n, int nrhs, double[] a, int lda, double[] b, int ldb, int[] jpvt, double rcond, org.netlib.util.intW rank, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* This routine is deprecated and has been replaced by routine DGELSY.
*
* DGELSX computes the minimum-norm solution to a real linear least
* squares problem:
* minimize || A * X - B ||
* using a complete orthogonal factorization of A. A is an M-by-N
* matrix which may be rank-deficient.
*
* Several right hand side vectors b and solution vectors x can be
* handled in a single call; they are stored as the columns of the
* M-by-NRHS right hand side matrix B and the N-by-NRHS solution
* matrix X.
*
* The routine first computes a QR factorization with column pivoting:
* A * P = Q * [ R11 R12 ]
* [ 0 R22 ]
* with R11 defined as the largest leading submatrix whose estimated
* condition number is less than 1/RCOND. The order of R11, RANK,
* is the effective rank of A.
*
* Then, R22 is considered to be negligible, and R12 is annihilated
* by orthogonal transformations from the right, arriving at the
* complete orthogonal factorization:
* A * P = Q * [ T11 0 ] * Z
* [ 0 0 ]
* The minimum-norm solution is then
* X = P * Z' [ inv(T11)*Q1'*B ]
* [ 0 ]
* where Q1 consists of the first RANK columns of Q.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of
* columns of matrices B and X. NRHS >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, A has been overwritten by details of its
* complete orthogonal factorization.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the M-by-NRHS right hand side matrix B.
* On exit, the N-by-NRHS solution matrix X.
* If m >= n and RANK = n, the residual sum-of-squares for
* the solution in the i-th column is given by the sum of
* squares of elements N+1:M in that column.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,M,N).
*
* JPVT (input/output) INTEGER array, dimension (N)
* On entry, if JPVT(i) .ne. 0, the i-th column of A is an
* initial column, otherwise it is a free column. Before
* the QR factorization of A, all initial columns are
* permuted to the leading positions; only the remaining
* free columns are moved as a result of column pivoting
* during the factorization.
* On exit, if JPVT(i) = k, then the i-th column of A*P
* was the k-th column of A.
*
* RCOND (input) DOUBLE PRECISION
* RCOND is used to determine the effective rank of A, which
* is defined as the order of the largest leading triangular
* submatrix R11 in the QR factorization with pivoting of A,
* whose estimated condition number < 1/RCOND.
*
* RANK (output) INTEGER
* The effective rank of A, i.e., the order of the submatrix
* R11. This is the same as the order of the submatrix T11
* in the complete orthogonal factorization of A.
*
* WORK (workspace) DOUBLE PRECISION array, dimension
* (max( min(M,N)+3*N, 2*min(M,N)+NRHS )),
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param jpvt
* @param _jpvt_offset
* @param rcond
* @param rank
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dgelsx(int m, int n, int nrhs, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, int[] jpvt, int _jpvt_offset, double rcond, org.netlib.util.intW rank, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGELSY computes the minimum-norm solution to a real linear least
* squares problem:
* minimize || A * X - B ||
* using a complete orthogonal factorization of A. A is an M-by-N
* matrix which may be rank-deficient.
*
* Several right hand side vectors b and solution vectors x can be
* handled in a single call; they are stored as the columns of the
* M-by-NRHS right hand side matrix B and the N-by-NRHS solution
* matrix X.
*
* The routine first computes a QR factorization with column pivoting:
* A * P = Q * [ R11 R12 ]
* [ 0 R22 ]
* with R11 defined as the largest leading submatrix whose estimated
* condition number is less than 1/RCOND. The order of R11, RANK,
* is the effective rank of A.
*
* Then, R22 is considered to be negligible, and R12 is annihilated
* by orthogonal transformations from the right, arriving at the
* complete orthogonal factorization:
* A * P = Q * [ T11 0 ] * Z
* [ 0 0 ]
* The minimum-norm solution is then
* X = P * Z' [ inv(T11)*Q1'*B ]
* [ 0 ]
* where Q1 consists of the first RANK columns of Q.
*
* This routine is basically identical to the original xGELSX except
* three differences:
* o The call to the subroutine xGEQPF has been substituted by the
* the call to the subroutine xGEQP3. This subroutine is a Blas-3
* version of the QR factorization with column pivoting.
* o Matrix B (the right hand side) is updated with Blas-3.
* o The permutation of matrix B (the right hand side) is faster and
* more simple.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of
* columns of matrices B and X. NRHS >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, A has been overwritten by details of its
* complete orthogonal factorization.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the M-by-NRHS right hand side matrix B.
* On exit, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,M,N).
*
* JPVT (input/output) INTEGER array, dimension (N)
* On entry, if JPVT(i) .ne. 0, the i-th column of A is permuted
* to the front of AP, otherwise column i is a free column.
* On exit, if JPVT(i) = k, then the i-th column of AP
* was the k-th column of A.
*
* RCOND (input) DOUBLE PRECISION
* RCOND is used to determine the effective rank of A, which
* is defined as the order of the largest leading triangular
* submatrix R11 in the QR factorization with pivoting of A,
* whose estimated condition number < 1/RCOND.
*
* RANK (output) INTEGER
* The effective rank of A, i.e., the order of the submatrix
* R11. This is the same as the order of the submatrix T11
* in the complete orthogonal factorization of A.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* The unblocked strategy requires that:
* LWORK >= MAX( MN+3*N+1, 2*MN+NRHS ),
* where MN = min( M, N ).
* The block algorithm requires that:
* LWORK >= MAX( MN+2*N+NB*(N+1), 2*MN+NB*NRHS ),
* where NB is an upper bound on the blocksize returned
* by ILAENV for the routines DGEQP3, DTZRZF, STZRQF, DORMQR,
* and DORMRZ.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: If INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* Based on contributions by
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
* E. Quintana-Orti, Depto. de Informatica, Universidad Jaime I, Spain
* G. Quintana-Orti, Depto. de Informatica, Universidad Jaime I, Spain
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param nrhs
* @param a
* @param lda
* @param b
* @param ldb
* @param jpvt
* @param rcond
* @param rank
* @param work
* @param lwork
* @param info
*
*/
abstract public void dgelsy(int m, int n, int nrhs, double[] a, int lda, double[] b, int ldb, int[] jpvt, double rcond, org.netlib.util.intW rank, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGELSY computes the minimum-norm solution to a real linear least
* squares problem:
* minimize || A * X - B ||
* using a complete orthogonal factorization of A. A is an M-by-N
* matrix which may be rank-deficient.
*
* Several right hand side vectors b and solution vectors x can be
* handled in a single call; they are stored as the columns of the
* M-by-NRHS right hand side matrix B and the N-by-NRHS solution
* matrix X.
*
* The routine first computes a QR factorization with column pivoting:
* A * P = Q * [ R11 R12 ]
* [ 0 R22 ]
* with R11 defined as the largest leading submatrix whose estimated
* condition number is less than 1/RCOND. The order of R11, RANK,
* is the effective rank of A.
*
* Then, R22 is considered to be negligible, and R12 is annihilated
* by orthogonal transformations from the right, arriving at the
* complete orthogonal factorization:
* A * P = Q * [ T11 0 ] * Z
* [ 0 0 ]
* The minimum-norm solution is then
* X = P * Z' [ inv(T11)*Q1'*B ]
* [ 0 ]
* where Q1 consists of the first RANK columns of Q.
*
* This routine is basically identical to the original xGELSX except
* three differences:
* o The call to the subroutine xGEQPF has been substituted by the
* the call to the subroutine xGEQP3. This subroutine is a Blas-3
* version of the QR factorization with column pivoting.
* o Matrix B (the right hand side) is updated with Blas-3.
* o The permutation of matrix B (the right hand side) is faster and
* more simple.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of
* columns of matrices B and X. NRHS >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, A has been overwritten by details of its
* complete orthogonal factorization.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the M-by-NRHS right hand side matrix B.
* On exit, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,M,N).
*
* JPVT (input/output) INTEGER array, dimension (N)
* On entry, if JPVT(i) .ne. 0, the i-th column of A is permuted
* to the front of AP, otherwise column i is a free column.
* On exit, if JPVT(i) = k, then the i-th column of AP
* was the k-th column of A.
*
* RCOND (input) DOUBLE PRECISION
* RCOND is used to determine the effective rank of A, which
* is defined as the order of the largest leading triangular
* submatrix R11 in the QR factorization with pivoting of A,
* whose estimated condition number < 1/RCOND.
*
* RANK (output) INTEGER
* The effective rank of A, i.e., the order of the submatrix
* R11. This is the same as the order of the submatrix T11
* in the complete orthogonal factorization of A.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* The unblocked strategy requires that:
* LWORK >= MAX( MN+3*N+1, 2*MN+NRHS ),
* where MN = min( M, N ).
* The block algorithm requires that:
* LWORK >= MAX( MN+2*N+NB*(N+1), 2*MN+NB*NRHS ),
* where NB is an upper bound on the blocksize returned
* by ILAENV for the routines DGEQP3, DTZRZF, STZRQF, DORMQR,
* and DORMRZ.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: If INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* Based on contributions by
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
* E. Quintana-Orti, Depto. de Informatica, Universidad Jaime I, Spain
* G. Quintana-Orti, Depto. de Informatica, Universidad Jaime I, Spain
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param jpvt
* @param _jpvt_offset
* @param rcond
* @param rank
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dgelsy(int m, int n, int nrhs, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, int[] jpvt, int _jpvt_offset, double rcond, org.netlib.util.intW rank, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGEQL2 computes a QL factorization of a real m by n matrix A:
* A = Q * L.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the m by n matrix A.
* On exit, if m >= n, the lower triangle of the subarray
* A(m-n+1:m,1:n) contains the n by n lower triangular matrix L;
* if m <= n, the elements on and below the (n-m)-th
* superdiagonal contain the m by n lower trapezoidal matrix L;
* the remaining elements, with the array TAU, represent the
* orthogonal matrix Q as a product of elementary reflectors
* (see Further Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) DOUBLE PRECISION array, dimension (min(M,N))
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(k) . . . H(2) H(1), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(m-k+i+1:m) = 0 and v(m-k+i) = 1; v(1:m-k+i-1) is stored on exit in
* A(1:m-k+i-1,n-k+i), and tau in TAU(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param tau
* @param work
* @param info
*
*/
abstract public void dgeql2(int m, int n, double[] a, int lda, double[] tau, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGEQL2 computes a QL factorization of a real m by n matrix A:
* A = Q * L.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the m by n matrix A.
* On exit, if m >= n, the lower triangle of the subarray
* A(m-n+1:m,1:n) contains the n by n lower triangular matrix L;
* if m <= n, the elements on and below the (n-m)-th
* superdiagonal contain the m by n lower trapezoidal matrix L;
* the remaining elements, with the array TAU, represent the
* orthogonal matrix Q as a product of elementary reflectors
* (see Further Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) DOUBLE PRECISION array, dimension (min(M,N))
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(k) . . . H(2) H(1), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(m-k+i+1:m) = 0 and v(m-k+i) = 1; v(1:m-k+i-1) is stored on exit in
* A(1:m-k+i-1,n-k+i), and tau in TAU(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dgeql2(int m, int n, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGEQLF computes a QL factorization of a real M-by-N matrix A:
* A = Q * L.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit,
* if m >= n, the lower triangle of the subarray
* A(m-n+1:m,1:n) contains the N-by-N lower triangular matrix L;
* if m <= n, the elements on and below the (n-m)-th
* superdiagonal contain the M-by-N lower trapezoidal matrix L;
* the remaining elements, with the array TAU, represent the
* orthogonal matrix Q as a product of elementary reflectors
* (see Further Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) DOUBLE PRECISION array, dimension (min(M,N))
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N).
* For optimum performance LWORK >= N*NB, where NB is the
* optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(k) . . . H(2) H(1), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(m-k+i+1:m) = 0 and v(m-k+i) = 1; v(1:m-k+i-1) is stored on exit in
* A(1:m-k+i-1,n-k+i), and tau in TAU(i).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param tau
* @param work
* @param lwork
* @param info
*
*/
abstract public void dgeqlf(int m, int n, double[] a, int lda, double[] tau, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGEQLF computes a QL factorization of a real M-by-N matrix A:
* A = Q * L.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit,
* if m >= n, the lower triangle of the subarray
* A(m-n+1:m,1:n) contains the N-by-N lower triangular matrix L;
* if m <= n, the elements on and below the (n-m)-th
* superdiagonal contain the M-by-N lower trapezoidal matrix L;
* the remaining elements, with the array TAU, represent the
* orthogonal matrix Q as a product of elementary reflectors
* (see Further Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) DOUBLE PRECISION array, dimension (min(M,N))
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N).
* For optimum performance LWORK >= N*NB, where NB is the
* optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(k) . . . H(2) H(1), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(m-k+i+1:m) = 0 and v(m-k+i) = 1; v(1:m-k+i-1) is stored on exit in
* A(1:m-k+i-1,n-k+i), and tau in TAU(i).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dgeqlf(int m, int n, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGEQP3 computes a QR factorization with column pivoting of a
* matrix A: A*P = Q*R using Level 3 BLAS.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, the upper triangle of the array contains the
* min(M,N)-by-N upper trapezoidal matrix R; the elements below
* the diagonal, together with the array TAU, represent the
* orthogonal matrix Q as a product of min(M,N) elementary
* reflectors.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* JPVT (input/output) INTEGER array, dimension (N)
* On entry, if JPVT(J).ne.0, the J-th column of A is permuted
* to the front of A*P (a leading column); if JPVT(J)=0,
* the J-th column of A is a free column.
* On exit, if JPVT(J)=K, then the J-th column of A*P was the
* the K-th column of A.
*
* TAU (output) DOUBLE PRECISION array, dimension (min(M,N))
* The scalar factors of the elementary reflectors.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO=0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= 3*N+1.
* For optimal performance LWORK >= 2*N+( N+1 )*NB, where NB
* is the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(1) H(2) . . . H(k), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real/complex scalar, and v is a real/complex vector
* with v(1:i-1) = 0 and v(i) = 1; v(i+1:m) is stored on exit in
* A(i+1:m,i), and tau in TAU(i).
*
* Based on contributions by
* G. Quintana-Orti, Depto. de Informatica, Universidad Jaime I, Spain
* X. Sun, Computer Science Dept., Duke University, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param jpvt
* @param tau
* @param work
* @param lwork
* @param info
*
*/
abstract public void dgeqp3(int m, int n, double[] a, int lda, int[] jpvt, double[] tau, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGEQP3 computes a QR factorization with column pivoting of a
* matrix A: A*P = Q*R using Level 3 BLAS.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, the upper triangle of the array contains the
* min(M,N)-by-N upper trapezoidal matrix R; the elements below
* the diagonal, together with the array TAU, represent the
* orthogonal matrix Q as a product of min(M,N) elementary
* reflectors.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* JPVT (input/output) INTEGER array, dimension (N)
* On entry, if JPVT(J).ne.0, the J-th column of A is permuted
* to the front of A*P (a leading column); if JPVT(J)=0,
* the J-th column of A is a free column.
* On exit, if JPVT(J)=K, then the J-th column of A*P was the
* the K-th column of A.
*
* TAU (output) DOUBLE PRECISION array, dimension (min(M,N))
* The scalar factors of the elementary reflectors.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO=0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= 3*N+1.
* For optimal performance LWORK >= 2*N+( N+1 )*NB, where NB
* is the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(1) H(2) . . . H(k), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real/complex scalar, and v is a real/complex vector
* with v(1:i-1) = 0 and v(i) = 1; v(i+1:m) is stored on exit in
* A(i+1:m,i), and tau in TAU(i).
*
* Based on contributions by
* G. Quintana-Orti, Depto. de Informatica, Universidad Jaime I, Spain
* X. Sun, Computer Science Dept., Duke University, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param jpvt
* @param _jpvt_offset
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dgeqp3(int m, int n, double[] a, int _a_offset, int lda, int[] jpvt, int _jpvt_offset, double[] tau, int _tau_offset, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* This routine is deprecated and has been replaced by routine DGEQP3.
*
* DGEQPF computes a QR factorization with column pivoting of a
* real M-by-N matrix A: A*P = Q*R.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, the upper triangle of the array contains the
* min(M,N)-by-N upper triangular matrix R; the elements
* below the diagonal, together with the array TAU,
* represent the orthogonal matrix Q as a product of
* min(m,n) elementary reflectors.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* JPVT (input/output) INTEGER array, dimension (N)
* On entry, if JPVT(i) .ne. 0, the i-th column of A is permuted
* to the front of A*P (a leading column); if JPVT(i) = 0,
* the i-th column of A is a free column.
* On exit, if JPVT(i) = k, then the i-th column of A*P
* was the k-th column of A.
*
* TAU (output) DOUBLE PRECISION array, dimension (min(M,N))
* The scalar factors of the elementary reflectors.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(1) H(2) . . . H(n)
*
* Each H(i) has the form
*
* H = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i-1) = 0 and v(i) = 1; v(i+1:m) is stored on exit in A(i+1:m,i).
*
* The matrix P is represented in jpvt as follows: If
* jpvt(j) = i
* then the jth column of P is the ith canonical unit vector.
*
* Partial column norm updating strategy modified by
* Z. Drmac and Z. Bujanovic, Dept. of Mathematics,
* University of Zagreb, Croatia.
* June 2006.
* For more details see LAPACK Working Note 176.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param jpvt
* @param tau
* @param work
* @param info
*
*/
abstract public void dgeqpf(int m, int n, double[] a, int lda, int[] jpvt, double[] tau, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* This routine is deprecated and has been replaced by routine DGEQP3.
*
* DGEQPF computes a QR factorization with column pivoting of a
* real M-by-N matrix A: A*P = Q*R.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, the upper triangle of the array contains the
* min(M,N)-by-N upper triangular matrix R; the elements
* below the diagonal, together with the array TAU,
* represent the orthogonal matrix Q as a product of
* min(m,n) elementary reflectors.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* JPVT (input/output) INTEGER array, dimension (N)
* On entry, if JPVT(i) .ne. 0, the i-th column of A is permuted
* to the front of A*P (a leading column); if JPVT(i) = 0,
* the i-th column of A is a free column.
* On exit, if JPVT(i) = k, then the i-th column of A*P
* was the k-th column of A.
*
* TAU (output) DOUBLE PRECISION array, dimension (min(M,N))
* The scalar factors of the elementary reflectors.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(1) H(2) . . . H(n)
*
* Each H(i) has the form
*
* H = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i-1) = 0 and v(i) = 1; v(i+1:m) is stored on exit in A(i+1:m,i).
*
* The matrix P is represented in jpvt as follows: If
* jpvt(j) = i
* then the jth column of P is the ith canonical unit vector.
*
* Partial column norm updating strategy modified by
* Z. Drmac and Z. Bujanovic, Dept. of Mathematics,
* University of Zagreb, Croatia.
* June 2006.
* For more details see LAPACK Working Note 176.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param jpvt
* @param _jpvt_offset
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dgeqpf(int m, int n, double[] a, int _a_offset, int lda, int[] jpvt, int _jpvt_offset, double[] tau, int _tau_offset, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGEQR2 computes a QR factorization of a real m by n matrix A:
* A = Q * R.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the m by n matrix A.
* On exit, the elements on and above the diagonal of the array
* contain the min(m,n) by n upper trapezoidal matrix R (R is
* upper triangular if m >= n); the elements below the diagonal,
* with the array TAU, represent the orthogonal matrix Q as a
* product of elementary reflectors (see Further Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) DOUBLE PRECISION array, dimension (min(M,N))
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(1) H(2) . . . H(k), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i-1) = 0 and v(i) = 1; v(i+1:m) is stored on exit in A(i+1:m,i),
* and tau in TAU(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param tau
* @param work
* @param info
*
*/
abstract public void dgeqr2(int m, int n, double[] a, int lda, double[] tau, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGEQR2 computes a QR factorization of a real m by n matrix A:
* A = Q * R.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the m by n matrix A.
* On exit, the elements on and above the diagonal of the array
* contain the min(m,n) by n upper trapezoidal matrix R (R is
* upper triangular if m >= n); the elements below the diagonal,
* with the array TAU, represent the orthogonal matrix Q as a
* product of elementary reflectors (see Further Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) DOUBLE PRECISION array, dimension (min(M,N))
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(1) H(2) . . . H(k), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i-1) = 0 and v(i) = 1; v(i+1:m) is stored on exit in A(i+1:m,i),
* and tau in TAU(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dgeqr2(int m, int n, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGEQRF computes a QR factorization of a real M-by-N matrix A:
* A = Q * R.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, the elements on and above the diagonal of the array
* contain the min(M,N)-by-N upper trapezoidal matrix R (R is
* upper triangular if m >= n); the elements below the diagonal,
* with the array TAU, represent the orthogonal matrix Q as a
* product of min(m,n) elementary reflectors (see Further
* Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) DOUBLE PRECISION array, dimension (min(M,N))
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N).
* For optimum performance LWORK >= N*NB, where NB is
* the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(1) H(2) . . . H(k), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i-1) = 0 and v(i) = 1; v(i+1:m) is stored on exit in A(i+1:m,i),
* and tau in TAU(i).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param tau
* @param work
* @param lwork
* @param info
*
*/
abstract public void dgeqrf(int m, int n, double[] a, int lda, double[] tau, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGEQRF computes a QR factorization of a real M-by-N matrix A:
* A = Q * R.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, the elements on and above the diagonal of the array
* contain the min(M,N)-by-N upper trapezoidal matrix R (R is
* upper triangular if m >= n); the elements below the diagonal,
* with the array TAU, represent the orthogonal matrix Q as a
* product of min(m,n) elementary reflectors (see Further
* Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) DOUBLE PRECISION array, dimension (min(M,N))
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N).
* For optimum performance LWORK >= N*NB, where NB is
* the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(1) H(2) . . . H(k), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i-1) = 0 and v(i) = 1; v(i+1:m) is stored on exit in A(i+1:m,i),
* and tau in TAU(i).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dgeqrf(int m, int n, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGERFS improves the computed solution to a system of linear
* equations and provides error bounds and backward error estimates for
* the solution.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The original N-by-N matrix A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* AF (input) DOUBLE PRECISION array, dimension (LDAF,N)
* The factors L and U from the factorization A = P*L*U
* as computed by DGETRF.
*
* LDAF (input) INTEGER
* The leading dimension of the array AF. LDAF >= max(1,N).
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices from DGETRF; for 1<=i<=N, row i of the
* matrix was interchanged with row IPIV(i).
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by DGETRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param trans
* @param n
* @param nrhs
* @param a
* @param lda
* @param af
* @param ldaf
* @param ipiv
* @param b
* @param ldb
* @param x
* @param ldx
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void dgerfs(java.lang.String trans, int n, int nrhs, double[] a, int lda, double[] af, int ldaf, int[] ipiv, double[] b, int ldb, double[] x, int ldx, double[] ferr, double[] berr, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGERFS improves the computed solution to a system of linear
* equations and provides error bounds and backward error estimates for
* the solution.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The original N-by-N matrix A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* AF (input) DOUBLE PRECISION array, dimension (LDAF,N)
* The factors L and U from the factorization A = P*L*U
* as computed by DGETRF.
*
* LDAF (input) INTEGER
* The leading dimension of the array AF. LDAF >= max(1,N).
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices from DGETRF; for 1<=i<=N, row i of the
* matrix was interchanged with row IPIV(i).
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by DGETRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param trans
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param af
* @param _af_offset
* @param ldaf
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dgerfs(java.lang.String trans, int n, int nrhs, double[] a, int _a_offset, int lda, double[] af, int _af_offset, int ldaf, int[] ipiv, int _ipiv_offset, double[] b, int _b_offset, int ldb, double[] x, int _x_offset, int ldx, double[] ferr, int _ferr_offset, double[] berr, int _berr_offset, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGERQ2 computes an RQ factorization of a real m by n matrix A:
* A = R * Q.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the m by n matrix A.
* On exit, if m <= n, the upper triangle of the subarray
* A(1:m,n-m+1:n) contains the m by m upper triangular matrix R;
* if m >= n, the elements on and above the (m-n)-th subdiagonal
* contain the m by n upper trapezoidal matrix R; the remaining
* elements, with the array TAU, represent the orthogonal matrix
* Q as a product of elementary reflectors (see Further
* Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) DOUBLE PRECISION array, dimension (min(M,N))
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (M)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(1) H(2) . . . H(k), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(n-k+i+1:n) = 0 and v(n-k+i) = 1; v(1:n-k+i-1) is stored on exit in
* A(m-k+i,1:n-k+i-1), and tau in TAU(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param tau
* @param work
* @param info
*
*/
abstract public void dgerq2(int m, int n, double[] a, int lda, double[] tau, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGERQ2 computes an RQ factorization of a real m by n matrix A:
* A = R * Q.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the m by n matrix A.
* On exit, if m <= n, the upper triangle of the subarray
* A(1:m,n-m+1:n) contains the m by m upper triangular matrix R;
* if m >= n, the elements on and above the (m-n)-th subdiagonal
* contain the m by n upper trapezoidal matrix R; the remaining
* elements, with the array TAU, represent the orthogonal matrix
* Q as a product of elementary reflectors (see Further
* Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) DOUBLE PRECISION array, dimension (min(M,N))
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (M)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(1) H(2) . . . H(k), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(n-k+i+1:n) = 0 and v(n-k+i) = 1; v(1:n-k+i-1) is stored on exit in
* A(m-k+i,1:n-k+i-1), and tau in TAU(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dgerq2(int m, int n, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGERQF computes an RQ factorization of a real M-by-N matrix A:
* A = R * Q.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit,
* if m <= n, the upper triangle of the subarray
* A(1:m,n-m+1:n) contains the M-by-M upper triangular matrix R;
* if m >= n, the elements on and above the (m-n)-th subdiagonal
* contain the M-by-N upper trapezoidal matrix R;
* the remaining elements, with the array TAU, represent the
* orthogonal matrix Q as a product of min(m,n) elementary
* reflectors (see Further Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) DOUBLE PRECISION array, dimension (min(M,N))
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,M).
* For optimum performance LWORK >= M*NB, where NB is
* the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(1) H(2) . . . H(k), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(n-k+i+1:n) = 0 and v(n-k+i) = 1; v(1:n-k+i-1) is stored on exit in
* A(m-k+i,1:n-k+i-1), and tau in TAU(i).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param tau
* @param work
* @param lwork
* @param info
*
*/
abstract public void dgerqf(int m, int n, double[] a, int lda, double[] tau, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGERQF computes an RQ factorization of a real M-by-N matrix A:
* A = R * Q.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit,
* if m <= n, the upper triangle of the subarray
* A(1:m,n-m+1:n) contains the M-by-M upper triangular matrix R;
* if m >= n, the elements on and above the (m-n)-th subdiagonal
* contain the M-by-N upper trapezoidal matrix R;
* the remaining elements, with the array TAU, represent the
* orthogonal matrix Q as a product of min(m,n) elementary
* reflectors (see Further Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) DOUBLE PRECISION array, dimension (min(M,N))
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,M).
* For optimum performance LWORK >= M*NB, where NB is
* the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(1) H(2) . . . H(k), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(n-k+i+1:n) = 0 and v(n-k+i) = 1; v(1:n-k+i-1) is stored on exit in
* A(m-k+i,1:n-k+i-1), and tau in TAU(i).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dgerqf(int m, int n, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGESC2 solves a system of linear equations
*
* A * X = scale* RHS
*
* with a general N-by-N matrix A using the LU factorization with
* complete pivoting computed by DGETC2.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the LU part of the factorization of the n-by-n
* matrix A computed by DGETC2: A = P * L * U * Q
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1, N).
*
* RHS (input/output) DOUBLE PRECISION array, dimension (N).
* On entry, the right hand side vector b.
* On exit, the solution vector X.
*
* IPIV (input) INTEGER array, dimension (N).
* The pivot indices; for 1 <= i <= N, row i of the
* matrix has been interchanged with row IPIV(i).
*
* JPIV (input) INTEGER array, dimension (N).
* The pivot indices; for 1 <= j <= N, column j of the
* matrix has been interchanged with column JPIV(j).
*
* SCALE (output) DOUBLE PRECISION
* On exit, SCALE contains the scale factor. SCALE is chosen
* 0 <= SCALE <= 1 to prevent owerflow in the solution.
*
* Further Details
* ===============
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param a
* @param lda
* @param rhs
* @param ipiv
* @param jpiv
* @param scale
*
*/
abstract public void dgesc2(int n, double[] a, int lda, double[] rhs, int[] ipiv, int[] jpiv, org.netlib.util.doubleW scale);
/**
*
* ..
*
* Purpose
* =======
*
* DGESC2 solves a system of linear equations
*
* A * X = scale* RHS
*
* with a general N-by-N matrix A using the LU factorization with
* complete pivoting computed by DGETC2.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the LU part of the factorization of the n-by-n
* matrix A computed by DGETC2: A = P * L * U * Q
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1, N).
*
* RHS (input/output) DOUBLE PRECISION array, dimension (N).
* On entry, the right hand side vector b.
* On exit, the solution vector X.
*
* IPIV (input) INTEGER array, dimension (N).
* The pivot indices; for 1 <= i <= N, row i of the
* matrix has been interchanged with row IPIV(i).
*
* JPIV (input) INTEGER array, dimension (N).
* The pivot indices; for 1 <= j <= N, column j of the
* matrix has been interchanged with column JPIV(j).
*
* SCALE (output) DOUBLE PRECISION
* On exit, SCALE contains the scale factor. SCALE is chosen
* 0 <= SCALE <= 1 to prevent owerflow in the solution.
*
* Further Details
* ===============
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param a
* @param _a_offset
* @param lda
* @param rhs
* @param _rhs_offset
* @param ipiv
* @param _ipiv_offset
* @param jpiv
* @param _jpiv_offset
* @param scale
*
*/
abstract public void dgesc2(int n, double[] a, int _a_offset, int lda, double[] rhs, int _rhs_offset, int[] ipiv, int _ipiv_offset, int[] jpiv, int _jpiv_offset, org.netlib.util.doubleW scale);
/**
*
* ..
*
* Purpose
* =======
*
* DGESDD computes the singular value decomposition (SVD) of a real
* M-by-N matrix A, optionally computing the left and right singular
* vectors. If singular vectors are desired, it uses a
* divide-and-conquer algorithm.
*
* The SVD is written
*
* A = U * SIGMA * transpose(V)
*
* where SIGMA is an M-by-N matrix which is zero except for its
* min(m,n) diagonal elements, U is an M-by-M orthogonal matrix, and
* V is an N-by-N orthogonal matrix. The diagonal elements of SIGMA
* are the singular values of A; they are real and non-negative, and
* are returned in descending order. The first min(m,n) columns of
* U and V are the left and right singular vectors of A.
*
* Note that the routine returns VT = V**T, not V.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* Specifies options for computing all or part of the matrix U:
* = 'A': all M columns of U and all N rows of V**T are
* returned in the arrays U and VT;
* = 'S': the first min(M,N) columns of U and the first
* min(M,N) rows of V**T are returned in the arrays U
* and VT;
* = 'O': If M >= N, the first N columns of U are overwritten
* on the array A and all rows of V**T are returned in
* the array VT;
* otherwise, all columns of U are returned in the
* array U and the first M rows of V**T are overwritten
* in the array A;
* = 'N': no columns of U or rows of V**T are computed.
*
* M (input) INTEGER
* The number of rows of the input matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the input matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit,
* if JOBZ = 'O', A is overwritten with the first N columns
* of U (the left singular vectors, stored
* columnwise) if M >= N;
* A is overwritten with the first M rows
* of V**T (the right singular vectors, stored
* rowwise) otherwise.
* if JOBZ .ne. 'O', the contents of A are destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* S (output) DOUBLE PRECISION array, dimension (min(M,N))
* The singular values of A, sorted so that S(i) >= S(i+1).
*
* U (output) DOUBLE PRECISION array, dimension (LDU,UCOL)
* UCOL = M if JOBZ = 'A' or JOBZ = 'O' and M < N;
* UCOL = min(M,N) if JOBZ = 'S'.
* If JOBZ = 'A' or JOBZ = 'O' and M < N, U contains the M-by-M
* orthogonal matrix U;
* if JOBZ = 'S', U contains the first min(M,N) columns of U
* (the left singular vectors, stored columnwise);
* if JOBZ = 'O' and M >= N, or JOBZ = 'N', U is not referenced.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= 1; if
* JOBZ = 'S' or 'A' or JOBZ = 'O' and M < N, LDU >= M.
*
* VT (output) DOUBLE PRECISION array, dimension (LDVT,N)
* If JOBZ = 'A' or JOBZ = 'O' and M >= N, VT contains the
* N-by-N orthogonal matrix V**T;
* if JOBZ = 'S', VT contains the first min(M,N) rows of
* V**T (the right singular vectors, stored rowwise);
* if JOBZ = 'O' and M < N, or JOBZ = 'N', VT is not referenced.
*
* LDVT (input) INTEGER
* The leading dimension of the array VT. LDVT >= 1; if
* JOBZ = 'A' or JOBZ = 'O' and M >= N, LDVT >= N;
* if JOBZ = 'S', LDVT >= min(M,N).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK;
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= 1.
* If JOBZ = 'N',
* LWORK >= 3*min(M,N) + max(max(M,N),7*min(M,N)).
* If JOBZ = 'O',
* LWORK >= 3*min(M,N)*min(M,N) +
* max(max(M,N),5*min(M,N)*min(M,N)+4*min(M,N)).
* If JOBZ = 'S' or 'A'
* LWORK >= 3*min(M,N)*min(M,N) +
* max(max(M,N),4*min(M,N)*min(M,N)+4*min(M,N)).
* For good performance, LWORK should generally be larger.
* If LWORK = -1 but other input arguments are legal, WORK(1)
* returns the optimal LWORK.
*
* IWORK (workspace) INTEGER array, dimension (8*min(M,N))
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: DBDSDC did not converge, updating process failed.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param m
* @param n
* @param a
* @param lda
* @param s
* @param u
* @param ldu
* @param vt
* @param ldvt
* @param work
* @param lwork
* @param iwork
* @param info
*
*/
abstract public void dgesdd(java.lang.String jobz, int m, int n, double[] a, int lda, double[] s, double[] u, int ldu, double[] vt, int ldvt, double[] work, int lwork, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGESDD computes the singular value decomposition (SVD) of a real
* M-by-N matrix A, optionally computing the left and right singular
* vectors. If singular vectors are desired, it uses a
* divide-and-conquer algorithm.
*
* The SVD is written
*
* A = U * SIGMA * transpose(V)
*
* where SIGMA is an M-by-N matrix which is zero except for its
* min(m,n) diagonal elements, U is an M-by-M orthogonal matrix, and
* V is an N-by-N orthogonal matrix. The diagonal elements of SIGMA
* are the singular values of A; they are real and non-negative, and
* are returned in descending order. The first min(m,n) columns of
* U and V are the left and right singular vectors of A.
*
* Note that the routine returns VT = V**T, not V.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* Specifies options for computing all or part of the matrix U:
* = 'A': all M columns of U and all N rows of V**T are
* returned in the arrays U and VT;
* = 'S': the first min(M,N) columns of U and the first
* min(M,N) rows of V**T are returned in the arrays U
* and VT;
* = 'O': If M >= N, the first N columns of U are overwritten
* on the array A and all rows of V**T are returned in
* the array VT;
* otherwise, all columns of U are returned in the
* array U and the first M rows of V**T are overwritten
* in the array A;
* = 'N': no columns of U or rows of V**T are computed.
*
* M (input) INTEGER
* The number of rows of the input matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the input matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit,
* if JOBZ = 'O', A is overwritten with the first N columns
* of U (the left singular vectors, stored
* columnwise) if M >= N;
* A is overwritten with the first M rows
* of V**T (the right singular vectors, stored
* rowwise) otherwise.
* if JOBZ .ne. 'O', the contents of A are destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* S (output) DOUBLE PRECISION array, dimension (min(M,N))
* The singular values of A, sorted so that S(i) >= S(i+1).
*
* U (output) DOUBLE PRECISION array, dimension (LDU,UCOL)
* UCOL = M if JOBZ = 'A' or JOBZ = 'O' and M < N;
* UCOL = min(M,N) if JOBZ = 'S'.
* If JOBZ = 'A' or JOBZ = 'O' and M < N, U contains the M-by-M
* orthogonal matrix U;
* if JOBZ = 'S', U contains the first min(M,N) columns of U
* (the left singular vectors, stored columnwise);
* if JOBZ = 'O' and M >= N, or JOBZ = 'N', U is not referenced.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= 1; if
* JOBZ = 'S' or 'A' or JOBZ = 'O' and M < N, LDU >= M.
*
* VT (output) DOUBLE PRECISION array, dimension (LDVT,N)
* If JOBZ = 'A' or JOBZ = 'O' and M >= N, VT contains the
* N-by-N orthogonal matrix V**T;
* if JOBZ = 'S', VT contains the first min(M,N) rows of
* V**T (the right singular vectors, stored rowwise);
* if JOBZ = 'O' and M < N, or JOBZ = 'N', VT is not referenced.
*
* LDVT (input) INTEGER
* The leading dimension of the array VT. LDVT >= 1; if
* JOBZ = 'A' or JOBZ = 'O' and M >= N, LDVT >= N;
* if JOBZ = 'S', LDVT >= min(M,N).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK;
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= 1.
* If JOBZ = 'N',
* LWORK >= 3*min(M,N) + max(max(M,N),7*min(M,N)).
* If JOBZ = 'O',
* LWORK >= 3*min(M,N)*min(M,N) +
* max(max(M,N),5*min(M,N)*min(M,N)+4*min(M,N)).
* If JOBZ = 'S' or 'A'
* LWORK >= 3*min(M,N)*min(M,N) +
* max(max(M,N),4*min(M,N)*min(M,N)+4*min(M,N)).
* For good performance, LWORK should generally be larger.
* If LWORK = -1 but other input arguments are legal, WORK(1)
* returns the optimal LWORK.
*
* IWORK (workspace) INTEGER array, dimension (8*min(M,N))
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: DBDSDC did not converge, updating process failed.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param s
* @param _s_offset
* @param u
* @param _u_offset
* @param ldu
* @param vt
* @param _vt_offset
* @param ldvt
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dgesdd(java.lang.String jobz, int m, int n, double[] a, int _a_offset, int lda, double[] s, int _s_offset, double[] u, int _u_offset, int ldu, double[] vt, int _vt_offset, int ldvt, double[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGESV computes the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N matrix and X and B are N-by-NRHS matrices.
*
* The LU decomposition with partial pivoting and row interchanges is
* used to factor A as
* A = P * L * U,
* where P is a permutation matrix, L is unit lower triangular, and U is
* upper triangular. The factored form of A is then used to solve the
* system of equations A * X = B.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the N-by-N coefficient matrix A.
* On exit, the factors L and U from the factorization
* A = P*L*U; the unit diagonal elements of L are not stored.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (output) INTEGER array, dimension (N)
* The pivot indices that define the permutation matrix P;
* row i of the matrix was interchanged with row IPIV(i).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS matrix of right hand side matrix B.
* On exit, if INFO = 0, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, U(i,i) is exactly zero. The factorization
* has been completed, but the factor U is exactly
* singular, so the solution could not be computed.
*
* =====================================================================
*
* .. External Subroutines ..
*
*
* @param n
* @param nrhs
* @param a
* @param lda
* @param ipiv
* @param b
* @param ldb
* @param info
*
*/
abstract public void dgesv(int n, int nrhs, double[] a, int lda, int[] ipiv, double[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGESV computes the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N matrix and X and B are N-by-NRHS matrices.
*
* The LU decomposition with partial pivoting and row interchanges is
* used to factor A as
* A = P * L * U,
* where P is a permutation matrix, L is unit lower triangular, and U is
* upper triangular. The factored form of A is then used to solve the
* system of equations A * X = B.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the N-by-N coefficient matrix A.
* On exit, the factors L and U from the factorization
* A = P*L*U; the unit diagonal elements of L are not stored.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (output) INTEGER array, dimension (N)
* The pivot indices that define the permutation matrix P;
* row i of the matrix was interchanged with row IPIV(i).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS matrix of right hand side matrix B.
* On exit, if INFO = 0, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, U(i,i) is exactly zero. The factorization
* has been completed, but the factor U is exactly
* singular, so the solution could not be computed.
*
* =====================================================================
*
* .. External Subroutines ..
*
*
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void dgesv(int n, int nrhs, double[] a, int _a_offset, int lda, int[] ipiv, int _ipiv_offset, double[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGESVD computes the singular value decomposition (SVD) of a real
* M-by-N matrix A, optionally computing the left and/or right singular
* vectors. The SVD is written
*
* A = U * SIGMA * transpose(V)
*
* where SIGMA is an M-by-N matrix which is zero except for its
* min(m,n) diagonal elements, U is an M-by-M orthogonal matrix, and
* V is an N-by-N orthogonal matrix. The diagonal elements of SIGMA
* are the singular values of A; they are real and non-negative, and
* are returned in descending order. The first min(m,n) columns of
* U and V are the left and right singular vectors of A.
*
* Note that the routine returns V**T, not V.
*
* Arguments
* =========
*
* JOBU (input) CHARACTER*1
* Specifies options for computing all or part of the matrix U:
* = 'A': all M columns of U are returned in array U:
* = 'S': the first min(m,n) columns of U (the left singular
* vectors) are returned in the array U;
* = 'O': the first min(m,n) columns of U (the left singular
* vectors) are overwritten on the array A;
* = 'N': no columns of U (no left singular vectors) are
* computed.
*
* JOBVT (input) CHARACTER*1
* Specifies options for computing all or part of the matrix
* V**T:
* = 'A': all N rows of V**T are returned in the array VT;
* = 'S': the first min(m,n) rows of V**T (the right singular
* vectors) are returned in the array VT;
* = 'O': the first min(m,n) rows of V**T (the right singular
* vectors) are overwritten on the array A;
* = 'N': no rows of V**T (no right singular vectors) are
* computed.
*
* JOBVT and JOBU cannot both be 'O'.
*
* M (input) INTEGER
* The number of rows of the input matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the input matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit,
* if JOBU = 'O', A is overwritten with the first min(m,n)
* columns of U (the left singular vectors,
* stored columnwise);
* if JOBVT = 'O', A is overwritten with the first min(m,n)
* rows of V**T (the right singular vectors,
* stored rowwise);
* if JOBU .ne. 'O' and JOBVT .ne. 'O', the contents of A
* are destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* S (output) DOUBLE PRECISION array, dimension (min(M,N))
* The singular values of A, sorted so that S(i) >= S(i+1).
*
* U (output) DOUBLE PRECISION array, dimension (LDU,UCOL)
* (LDU,M) if JOBU = 'A' or (LDU,min(M,N)) if JOBU = 'S'.
* If JOBU = 'A', U contains the M-by-M orthogonal matrix U;
* if JOBU = 'S', U contains the first min(m,n) columns of U
* (the left singular vectors, stored columnwise);
* if JOBU = 'N' or 'O', U is not referenced.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= 1; if
* JOBU = 'S' or 'A', LDU >= M.
*
* VT (output) DOUBLE PRECISION array, dimension (LDVT,N)
* If JOBVT = 'A', VT contains the N-by-N orthogonal matrix
* V**T;
* if JOBVT = 'S', VT contains the first min(m,n) rows of
* V**T (the right singular vectors, stored rowwise);
* if JOBVT = 'N' or 'O', VT is not referenced.
*
* LDVT (input) INTEGER
* The leading dimension of the array VT. LDVT >= 1; if
* JOBVT = 'A', LDVT >= N; if JOBVT = 'S', LDVT >= min(M,N).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK;
* if INFO > 0, WORK(2:MIN(M,N)) contains the unconverged
* superdiagonal elements of an upper bidiagonal matrix B
* whose diagonal is in S (not necessarily sorted). B
* satisfies A = U * B * VT, so it has the same singular values
* as A, and singular vectors related by U and VT.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* LWORK >= MAX(1,3*MIN(M,N)+MAX(M,N),5*MIN(M,N)).
* For good performance, LWORK should generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if DBDSQR did not converge, INFO specifies how many
* superdiagonals of an intermediate bidiagonal form B
* did not converge to zero. See the description of WORK
* above for details.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobu
* @param jobvt
* @param m
* @param n
* @param a
* @param lda
* @param s
* @param u
* @param ldu
* @param vt
* @param ldvt
* @param work
* @param lwork
* @param info
*
*/
abstract public void dgesvd(java.lang.String jobu, java.lang.String jobvt, int m, int n, double[] a, int lda, double[] s, double[] u, int ldu, double[] vt, int ldvt, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGESVD computes the singular value decomposition (SVD) of a real
* M-by-N matrix A, optionally computing the left and/or right singular
* vectors. The SVD is written
*
* A = U * SIGMA * transpose(V)
*
* where SIGMA is an M-by-N matrix which is zero except for its
* min(m,n) diagonal elements, U is an M-by-M orthogonal matrix, and
* V is an N-by-N orthogonal matrix. The diagonal elements of SIGMA
* are the singular values of A; they are real and non-negative, and
* are returned in descending order. The first min(m,n) columns of
* U and V are the left and right singular vectors of A.
*
* Note that the routine returns V**T, not V.
*
* Arguments
* =========
*
* JOBU (input) CHARACTER*1
* Specifies options for computing all or part of the matrix U:
* = 'A': all M columns of U are returned in array U:
* = 'S': the first min(m,n) columns of U (the left singular
* vectors) are returned in the array U;
* = 'O': the first min(m,n) columns of U (the left singular
* vectors) are overwritten on the array A;
* = 'N': no columns of U (no left singular vectors) are
* computed.
*
* JOBVT (input) CHARACTER*1
* Specifies options for computing all or part of the matrix
* V**T:
* = 'A': all N rows of V**T are returned in the array VT;
* = 'S': the first min(m,n) rows of V**T (the right singular
* vectors) are returned in the array VT;
* = 'O': the first min(m,n) rows of V**T (the right singular
* vectors) are overwritten on the array A;
* = 'N': no rows of V**T (no right singular vectors) are
* computed.
*
* JOBVT and JOBU cannot both be 'O'.
*
* M (input) INTEGER
* The number of rows of the input matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the input matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit,
* if JOBU = 'O', A is overwritten with the first min(m,n)
* columns of U (the left singular vectors,
* stored columnwise);
* if JOBVT = 'O', A is overwritten with the first min(m,n)
* rows of V**T (the right singular vectors,
* stored rowwise);
* if JOBU .ne. 'O' and JOBVT .ne. 'O', the contents of A
* are destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* S (output) DOUBLE PRECISION array, dimension (min(M,N))
* The singular values of A, sorted so that S(i) >= S(i+1).
*
* U (output) DOUBLE PRECISION array, dimension (LDU,UCOL)
* (LDU,M) if JOBU = 'A' or (LDU,min(M,N)) if JOBU = 'S'.
* If JOBU = 'A', U contains the M-by-M orthogonal matrix U;
* if JOBU = 'S', U contains the first min(m,n) columns of U
* (the left singular vectors, stored columnwise);
* if JOBU = 'N' or 'O', U is not referenced.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= 1; if
* JOBU = 'S' or 'A', LDU >= M.
*
* VT (output) DOUBLE PRECISION array, dimension (LDVT,N)
* If JOBVT = 'A', VT contains the N-by-N orthogonal matrix
* V**T;
* if JOBVT = 'S', VT contains the first min(m,n) rows of
* V**T (the right singular vectors, stored rowwise);
* if JOBVT = 'N' or 'O', VT is not referenced.
*
* LDVT (input) INTEGER
* The leading dimension of the array VT. LDVT >= 1; if
* JOBVT = 'A', LDVT >= N; if JOBVT = 'S', LDVT >= min(M,N).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK;
* if INFO > 0, WORK(2:MIN(M,N)) contains the unconverged
* superdiagonal elements of an upper bidiagonal matrix B
* whose diagonal is in S (not necessarily sorted). B
* satisfies A = U * B * VT, so it has the same singular values
* as A, and singular vectors related by U and VT.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* LWORK >= MAX(1,3*MIN(M,N)+MAX(M,N),5*MIN(M,N)).
* For good performance, LWORK should generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if DBDSQR did not converge, INFO specifies how many
* superdiagonals of an intermediate bidiagonal form B
* did not converge to zero. See the description of WORK
* above for details.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobu
* @param jobvt
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param s
* @param _s_offset
* @param u
* @param _u_offset
* @param ldu
* @param vt
* @param _vt_offset
* @param ldvt
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dgesvd(java.lang.String jobu, java.lang.String jobvt, int m, int n, double[] a, int _a_offset, int lda, double[] s, int _s_offset, double[] u, int _u_offset, int ldu, double[] vt, int _vt_offset, int ldvt, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGESVX uses the LU factorization to compute the solution to a real
* system of linear equations
* A * X = B,
* where A is an N-by-N matrix and X and B are N-by-NRHS matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed:
*
* 1. If FACT = 'E', real scaling factors are computed to equilibrate
* the system:
* TRANS = 'N': diag(R)*A*diag(C) *inv(diag(C))*X = diag(R)*B
* TRANS = 'T': (diag(R)*A*diag(C))**T *inv(diag(R))*X = diag(C)*B
* TRANS = 'C': (diag(R)*A*diag(C))**H *inv(diag(R))*X = diag(C)*B
* Whether or not the system will be equilibrated depends on the
* scaling of the matrix A, but if equilibration is used, A is
* overwritten by diag(R)*A*diag(C) and B by diag(R)*B (if TRANS='N')
* or diag(C)*B (if TRANS = 'T' or 'C').
*
* 2. If FACT = 'N' or 'E', the LU decomposition is used to factor the
* matrix A (after equilibration if FACT = 'E') as
* A = P * L * U,
* where P is a permutation matrix, L is a unit lower triangular
* matrix, and U is upper triangular.
*
* 3. If some U(i,i)=0, so that U is exactly singular, then the routine
* returns with INFO = i. Otherwise, the factored form of A is used
* to estimate the condition number of the matrix A. If the
* reciprocal of the condition number is less than machine precision,
* INFO = N+1 is returned as a warning, but the routine still goes on
* to solve for X and compute error bounds as described below.
*
* 4. The system of equations is solved for X using the factored form
* of A.
*
* 5. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* 6. If equilibration was used, the matrix X is premultiplied by
* diag(C) (if TRANS = 'N') or diag(R) (if TRANS = 'T' or 'C') so
* that it solves the original system before equilibration.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of the matrix A is
* supplied on entry, and if not, whether the matrix A should be
* equilibrated before it is factored.
* = 'F': On entry, AF and IPIV contain the factored form of A.
* If EQUED is not 'N', the matrix A has been
* equilibrated with scaling factors given by R and C.
* A, AF, and IPIV are not modified.
* = 'N': The matrix A will be copied to AF and factored.
* = 'E': The matrix A will be equilibrated if necessary, then
* copied to AF and factored.
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Transpose)
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the N-by-N matrix A. If FACT = 'F' and EQUED is
* not 'N', then A must have been equilibrated by the scaling
* factors in R and/or C. A is not modified if FACT = 'F' or
* 'N', or if FACT = 'E' and EQUED = 'N' on exit.
*
* On exit, if EQUED .ne. 'N', A is scaled as follows:
* EQUED = 'R': A := diag(R) * A
* EQUED = 'C': A := A * diag(C)
* EQUED = 'B': A := diag(R) * A * diag(C).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* AF (input or output) DOUBLE PRECISION array, dimension (LDAF,N)
* If FACT = 'F', then AF is an input argument and on entry
* contains the factors L and U from the factorization
* A = P*L*U as computed by DGETRF. If EQUED .ne. 'N', then
* AF is the factored form of the equilibrated matrix A.
*
* If FACT = 'N', then AF is an output argument and on exit
* returns the factors L and U from the factorization A = P*L*U
* of the original matrix A.
*
* If FACT = 'E', then AF is an output argument and on exit
* returns the factors L and U from the factorization A = P*L*U
* of the equilibrated matrix A (see the description of A for
* the form of the equilibrated matrix).
*
* LDAF (input) INTEGER
* The leading dimension of the array AF. LDAF >= max(1,N).
*
* IPIV (input or output) INTEGER array, dimension (N)
* If FACT = 'F', then IPIV is an input argument and on entry
* contains the pivot indices from the factorization A = P*L*U
* as computed by DGETRF; row i of the matrix was interchanged
* with row IPIV(i).
*
* If FACT = 'N', then IPIV is an output argument and on exit
* contains the pivot indices from the factorization A = P*L*U
* of the original matrix A.
*
* If FACT = 'E', then IPIV is an output argument and on exit
* contains the pivot indices from the factorization A = P*L*U
* of the equilibrated matrix A.
*
* EQUED (input or output) CHARACTER*1
* Specifies the form of equilibration that was done.
* = 'N': No equilibration (always true if FACT = 'N').
* = 'R': Row equilibration, i.e., A has been premultiplied by
* diag(R).
* = 'C': Column equilibration, i.e., A has been postmultiplied
* by diag(C).
* = 'B': Both row and column equilibration, i.e., A has been
* replaced by diag(R) * A * diag(C).
* EQUED is an input argument if FACT = 'F'; otherwise, it is an
* output argument.
*
* R (input or output) DOUBLE PRECISION array, dimension (N)
* The row scale factors for A. If EQUED = 'R' or 'B', A is
* multiplied on the left by diag(R); if EQUED = 'N' or 'C', R
* is not accessed. R is an input argument if FACT = 'F';
* otherwise, R is an output argument. If FACT = 'F' and
* EQUED = 'R' or 'B', each element of R must be positive.
*
* C (input or output) DOUBLE PRECISION array, dimension (N)
* The column scale factors for A. If EQUED = 'C' or 'B', A is
* multiplied on the right by diag(C); if EQUED = 'N' or 'R', C
* is not accessed. C is an input argument if FACT = 'F';
* otherwise, C is an output argument. If FACT = 'F' and
* EQUED = 'C' or 'B', each element of C must be positive.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit,
* if EQUED = 'N', B is not modified;
* if TRANS = 'N' and EQUED = 'R' or 'B', B is overwritten by
* diag(R)*B;
* if TRANS = 'T' or 'C' and EQUED = 'C' or 'B', B is
* overwritten by diag(C)*B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* If INFO = 0 or INFO = N+1, the N-by-NRHS solution matrix X
* to the original system of equations. Note that A and B are
* modified on exit if EQUED .ne. 'N', and the solution to the
* equilibrated system is inv(diag(C))*X if TRANS = 'N' and
* EQUED = 'C' or 'B', or inv(diag(R))*X if TRANS = 'T' or 'C'
* and EQUED = 'R' or 'B'.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) DOUBLE PRECISION
* The estimate of the reciprocal condition number of the matrix
* A after equilibration (if done). If RCOND is less than the
* machine precision (in particular, if RCOND = 0), the matrix
* is singular to working precision. This condition is
* indicated by a return code of INFO > 0.
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (4*N)
* On exit, WORK(1) contains the reciprocal pivot growth
* factor norm(A)/norm(U). The "max absolute element" norm is
* used. If WORK(1) is much less than 1, then the stability
* of the LU factorization of the (equilibrated) matrix A
* could be poor. This also means that the solution X, condition
* estimator RCOND, and forward error bound FERR could be
* unreliable. If factorization fails with 0 0: if INFO = i, and i is
* <= N: U(i,i) is exactly zero. The factorization has
* been completed, but the factor U is exactly
* singular, so the solution and error bounds
* could not be computed. RCOND = 0 is returned.
* = N+1: U is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param trans
* @param n
* @param nrhs
* @param a
* @param lda
* @param af
* @param ldaf
* @param ipiv
* @param equed
* @param r
* @param c
* @param b
* @param ldb
* @param x
* @param ldx
* @param rcond
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void dgesvx(java.lang.String fact, java.lang.String trans, int n, int nrhs, double[] a, int lda, double[] af, int ldaf, int[] ipiv, org.netlib.util.StringW equed, double[] r, double[] c, double[] b, int ldb, double[] x, int ldx, org.netlib.util.doubleW rcond, double[] ferr, double[] berr, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGESVX uses the LU factorization to compute the solution to a real
* system of linear equations
* A * X = B,
* where A is an N-by-N matrix and X and B are N-by-NRHS matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed:
*
* 1. If FACT = 'E', real scaling factors are computed to equilibrate
* the system:
* TRANS = 'N': diag(R)*A*diag(C) *inv(diag(C))*X = diag(R)*B
* TRANS = 'T': (diag(R)*A*diag(C))**T *inv(diag(R))*X = diag(C)*B
* TRANS = 'C': (diag(R)*A*diag(C))**H *inv(diag(R))*X = diag(C)*B
* Whether or not the system will be equilibrated depends on the
* scaling of the matrix A, but if equilibration is used, A is
* overwritten by diag(R)*A*diag(C) and B by diag(R)*B (if TRANS='N')
* or diag(C)*B (if TRANS = 'T' or 'C').
*
* 2. If FACT = 'N' or 'E', the LU decomposition is used to factor the
* matrix A (after equilibration if FACT = 'E') as
* A = P * L * U,
* where P is a permutation matrix, L is a unit lower triangular
* matrix, and U is upper triangular.
*
* 3. If some U(i,i)=0, so that U is exactly singular, then the routine
* returns with INFO = i. Otherwise, the factored form of A is used
* to estimate the condition number of the matrix A. If the
* reciprocal of the condition number is less than machine precision,
* INFO = N+1 is returned as a warning, but the routine still goes on
* to solve for X and compute error bounds as described below.
*
* 4. The system of equations is solved for X using the factored form
* of A.
*
* 5. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* 6. If equilibration was used, the matrix X is premultiplied by
* diag(C) (if TRANS = 'N') or diag(R) (if TRANS = 'T' or 'C') so
* that it solves the original system before equilibration.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of the matrix A is
* supplied on entry, and if not, whether the matrix A should be
* equilibrated before it is factored.
* = 'F': On entry, AF and IPIV contain the factored form of A.
* If EQUED is not 'N', the matrix A has been
* equilibrated with scaling factors given by R and C.
* A, AF, and IPIV are not modified.
* = 'N': The matrix A will be copied to AF and factored.
* = 'E': The matrix A will be equilibrated if necessary, then
* copied to AF and factored.
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Transpose)
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the N-by-N matrix A. If FACT = 'F' and EQUED is
* not 'N', then A must have been equilibrated by the scaling
* factors in R and/or C. A is not modified if FACT = 'F' or
* 'N', or if FACT = 'E' and EQUED = 'N' on exit.
*
* On exit, if EQUED .ne. 'N', A is scaled as follows:
* EQUED = 'R': A := diag(R) * A
* EQUED = 'C': A := A * diag(C)
* EQUED = 'B': A := diag(R) * A * diag(C).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* AF (input or output) DOUBLE PRECISION array, dimension (LDAF,N)
* If FACT = 'F', then AF is an input argument and on entry
* contains the factors L and U from the factorization
* A = P*L*U as computed by DGETRF. If EQUED .ne. 'N', then
* AF is the factored form of the equilibrated matrix A.
*
* If FACT = 'N', then AF is an output argument and on exit
* returns the factors L and U from the factorization A = P*L*U
* of the original matrix A.
*
* If FACT = 'E', then AF is an output argument and on exit
* returns the factors L and U from the factorization A = P*L*U
* of the equilibrated matrix A (see the description of A for
* the form of the equilibrated matrix).
*
* LDAF (input) INTEGER
* The leading dimension of the array AF. LDAF >= max(1,N).
*
* IPIV (input or output) INTEGER array, dimension (N)
* If FACT = 'F', then IPIV is an input argument and on entry
* contains the pivot indices from the factorization A = P*L*U
* as computed by DGETRF; row i of the matrix was interchanged
* with row IPIV(i).
*
* If FACT = 'N', then IPIV is an output argument and on exit
* contains the pivot indices from the factorization A = P*L*U
* of the original matrix A.
*
* If FACT = 'E', then IPIV is an output argument and on exit
* contains the pivot indices from the factorization A = P*L*U
* of the equilibrated matrix A.
*
* EQUED (input or output) CHARACTER*1
* Specifies the form of equilibration that was done.
* = 'N': No equilibration (always true if FACT = 'N').
* = 'R': Row equilibration, i.e., A has been premultiplied by
* diag(R).
* = 'C': Column equilibration, i.e., A has been postmultiplied
* by diag(C).
* = 'B': Both row and column equilibration, i.e., A has been
* replaced by diag(R) * A * diag(C).
* EQUED is an input argument if FACT = 'F'; otherwise, it is an
* output argument.
*
* R (input or output) DOUBLE PRECISION array, dimension (N)
* The row scale factors for A. If EQUED = 'R' or 'B', A is
* multiplied on the left by diag(R); if EQUED = 'N' or 'C', R
* is not accessed. R is an input argument if FACT = 'F';
* otherwise, R is an output argument. If FACT = 'F' and
* EQUED = 'R' or 'B', each element of R must be positive.
*
* C (input or output) DOUBLE PRECISION array, dimension (N)
* The column scale factors for A. If EQUED = 'C' or 'B', A is
* multiplied on the right by diag(C); if EQUED = 'N' or 'R', C
* is not accessed. C is an input argument if FACT = 'F';
* otherwise, C is an output argument. If FACT = 'F' and
* EQUED = 'C' or 'B', each element of C must be positive.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit,
* if EQUED = 'N', B is not modified;
* if TRANS = 'N' and EQUED = 'R' or 'B', B is overwritten by
* diag(R)*B;
* if TRANS = 'T' or 'C' and EQUED = 'C' or 'B', B is
* overwritten by diag(C)*B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* If INFO = 0 or INFO = N+1, the N-by-NRHS solution matrix X
* to the original system of equations. Note that A and B are
* modified on exit if EQUED .ne. 'N', and the solution to the
* equilibrated system is inv(diag(C))*X if TRANS = 'N' and
* EQUED = 'C' or 'B', or inv(diag(R))*X if TRANS = 'T' or 'C'
* and EQUED = 'R' or 'B'.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) DOUBLE PRECISION
* The estimate of the reciprocal condition number of the matrix
* A after equilibration (if done). If RCOND is less than the
* machine precision (in particular, if RCOND = 0), the matrix
* is singular to working precision. This condition is
* indicated by a return code of INFO > 0.
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (4*N)
* On exit, WORK(1) contains the reciprocal pivot growth
* factor norm(A)/norm(U). The "max absolute element" norm is
* used. If WORK(1) is much less than 1, then the stability
* of the LU factorization of the (equilibrated) matrix A
* could be poor. This also means that the solution X, condition
* estimator RCOND, and forward error bound FERR could be
* unreliable. If factorization fails with 0 0: if INFO = i, and i is
* <= N: U(i,i) is exactly zero. The factorization has
* been completed, but the factor U is exactly
* singular, so the solution and error bounds
* could not be computed. RCOND = 0 is returned.
* = N+1: U is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param trans
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param af
* @param _af_offset
* @param ldaf
* @param ipiv
* @param _ipiv_offset
* @param equed
* @param r
* @param _r_offset
* @param c
* @param _c_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param rcond
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dgesvx(java.lang.String fact, java.lang.String trans, int n, int nrhs, double[] a, int _a_offset, int lda, double[] af, int _af_offset, int ldaf, int[] ipiv, int _ipiv_offset, org.netlib.util.StringW equed, double[] r, int _r_offset, double[] c, int _c_offset, double[] b, int _b_offset, int ldb, double[] x, int _x_offset, int ldx, org.netlib.util.doubleW rcond, double[] ferr, int _ferr_offset, double[] berr, int _berr_offset, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGETC2 computes an LU factorization with complete pivoting of the
* n-by-n matrix A. The factorization has the form A = P * L * U * Q,
* where P and Q are permutation matrices, L is lower triangular with
* unit diagonal elements and U is upper triangular.
*
* This is the Level 2 BLAS algorithm.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the n-by-n matrix A to be factored.
* On exit, the factors L and U from the factorization
* A = P*L*U*Q; the unit diagonal elements of L are not stored.
* If U(k, k) appears to be less than SMIN, U(k, k) is given the
* value of SMIN, i.e., giving a nonsingular perturbed system.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (output) INTEGER array, dimension(N).
* The pivot indices; for 1 <= i <= N, row i of the
* matrix has been interchanged with row IPIV(i).
*
* JPIV (output) INTEGER array, dimension(N).
* The pivot indices; for 1 <= j <= N, column j of the
* matrix has been interchanged with column JPIV(j).
*
* INFO (output) INTEGER
* = 0: successful exit
* > 0: if INFO = k, U(k, k) is likely to produce owerflow if
* we try to solve for x in Ax = b. So U is perturbed to
* avoid the overflow.
*
* Further Details
* ===============
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param a
* @param lda
* @param ipiv
* @param jpiv
* @param info
*
*/
abstract public void dgetc2(int n, double[] a, int lda, int[] ipiv, int[] jpiv, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGETC2 computes an LU factorization with complete pivoting of the
* n-by-n matrix A. The factorization has the form A = P * L * U * Q,
* where P and Q are permutation matrices, L is lower triangular with
* unit diagonal elements and U is upper triangular.
*
* This is the Level 2 BLAS algorithm.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the n-by-n matrix A to be factored.
* On exit, the factors L and U from the factorization
* A = P*L*U*Q; the unit diagonal elements of L are not stored.
* If U(k, k) appears to be less than SMIN, U(k, k) is given the
* value of SMIN, i.e., giving a nonsingular perturbed system.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (output) INTEGER array, dimension(N).
* The pivot indices; for 1 <= i <= N, row i of the
* matrix has been interchanged with row IPIV(i).
*
* JPIV (output) INTEGER array, dimension(N).
* The pivot indices; for 1 <= j <= N, column j of the
* matrix has been interchanged with column JPIV(j).
*
* INFO (output) INTEGER
* = 0: successful exit
* > 0: if INFO = k, U(k, k) is likely to produce owerflow if
* we try to solve for x in Ax = b. So U is perturbed to
* avoid the overflow.
*
* Further Details
* ===============
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param a
* @param _a_offset
* @param lda
* @param ipiv
* @param _ipiv_offset
* @param jpiv
* @param _jpiv_offset
* @param info
*
*/
abstract public void dgetc2(int n, double[] a, int _a_offset, int lda, int[] ipiv, int _ipiv_offset, int[] jpiv, int _jpiv_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGETF2 computes an LU factorization of a general m-by-n matrix A
* using partial pivoting with row interchanges.
*
* The factorization has the form
* A = P * L * U
* where P is a permutation matrix, L is lower triangular with unit
* diagonal elements (lower trapezoidal if m > n), and U is upper
* triangular (upper trapezoidal if m < n).
*
* This is the right-looking Level 2 BLAS version of the algorithm.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the m by n matrix to be factored.
* On exit, the factors L and U from the factorization
* A = P*L*U; the unit diagonal elements of L are not stored.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* IPIV (output) INTEGER array, dimension (min(M,N))
* The pivot indices; for 1 <= i <= min(M,N), row i of the
* matrix was interchanged with row IPIV(i).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
* > 0: if INFO = k, U(k,k) is exactly zero. The factorization
* has been completed, but the factor U is exactly
* singular, and division by zero will occur if it is used
* to solve a system of equations.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param ipiv
* @param info
*
*/
abstract public void dgetf2(int m, int n, double[] a, int lda, int[] ipiv, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGETF2 computes an LU factorization of a general m-by-n matrix A
* using partial pivoting with row interchanges.
*
* The factorization has the form
* A = P * L * U
* where P is a permutation matrix, L is lower triangular with unit
* diagonal elements (lower trapezoidal if m > n), and U is upper
* triangular (upper trapezoidal if m < n).
*
* This is the right-looking Level 2 BLAS version of the algorithm.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the m by n matrix to be factored.
* On exit, the factors L and U from the factorization
* A = P*L*U; the unit diagonal elements of L are not stored.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* IPIV (output) INTEGER array, dimension (min(M,N))
* The pivot indices; for 1 <= i <= min(M,N), row i of the
* matrix was interchanged with row IPIV(i).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
* > 0: if INFO = k, U(k,k) is exactly zero. The factorization
* has been completed, but the factor U is exactly
* singular, and division by zero will occur if it is used
* to solve a system of equations.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param ipiv
* @param _ipiv_offset
* @param info
*
*/
abstract public void dgetf2(int m, int n, double[] a, int _a_offset, int lda, int[] ipiv, int _ipiv_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGETRF computes an LU factorization of a general M-by-N matrix A
* using partial pivoting with row interchanges.
*
* The factorization has the form
* A = P * L * U
* where P is a permutation matrix, L is lower triangular with unit
* diagonal elements (lower trapezoidal if m > n), and U is upper
* triangular (upper trapezoidal if m < n).
*
* This is the right-looking Level 3 BLAS version of the algorithm.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix to be factored.
* On exit, the factors L and U from the factorization
* A = P*L*U; the unit diagonal elements of L are not stored.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* IPIV (output) INTEGER array, dimension (min(M,N))
* The pivot indices; for 1 <= i <= min(M,N), row i of the
* matrix was interchanged with row IPIV(i).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, U(i,i) is exactly zero. The factorization
* has been completed, but the factor U is exactly
* singular, and division by zero will occur if it is used
* to solve a system of equations.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param ipiv
* @param info
*
*/
abstract public void dgetrf(int m, int n, double[] a, int lda, int[] ipiv, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGETRF computes an LU factorization of a general M-by-N matrix A
* using partial pivoting with row interchanges.
*
* The factorization has the form
* A = P * L * U
* where P is a permutation matrix, L is lower triangular with unit
* diagonal elements (lower trapezoidal if m > n), and U is upper
* triangular (upper trapezoidal if m < n).
*
* This is the right-looking Level 3 BLAS version of the algorithm.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix to be factored.
* On exit, the factors L and U from the factorization
* A = P*L*U; the unit diagonal elements of L are not stored.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* IPIV (output) INTEGER array, dimension (min(M,N))
* The pivot indices; for 1 <= i <= min(M,N), row i of the
* matrix was interchanged with row IPIV(i).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, U(i,i) is exactly zero. The factorization
* has been completed, but the factor U is exactly
* singular, and division by zero will occur if it is used
* to solve a system of equations.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param ipiv
* @param _ipiv_offset
* @param info
*
*/
abstract public void dgetrf(int m, int n, double[] a, int _a_offset, int lda, int[] ipiv, int _ipiv_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGETRI computes the inverse of a matrix using the LU factorization
* computed by DGETRF.
*
* This method inverts U and then computes inv(A) by solving the system
* inv(A)*L = inv(U) for inv(A).
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the factors L and U from the factorization
* A = P*L*U as computed by DGETRF.
* On exit, if INFO = 0, the inverse of the original matrix A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices from DGETRF; for 1<=i<=N, row i of the
* matrix was interchanged with row IPIV(i).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO=0, then WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N).
* For optimal performance LWORK >= N*NB, where NB is
* the optimal blocksize returned by ILAENV.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, U(i,i) is exactly zero; the matrix is
* singular and its inverse could not be computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param a
* @param lda
* @param ipiv
* @param work
* @param lwork
* @param info
*
*/
abstract public void dgetri(int n, double[] a, int lda, int[] ipiv, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGETRI computes the inverse of a matrix using the LU factorization
* computed by DGETRF.
*
* This method inverts U and then computes inv(A) by solving the system
* inv(A)*L = inv(U) for inv(A).
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the factors L and U from the factorization
* A = P*L*U as computed by DGETRF.
* On exit, if INFO = 0, the inverse of the original matrix A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices from DGETRF; for 1<=i<=N, row i of the
* matrix was interchanged with row IPIV(i).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO=0, then WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N).
* For optimal performance LWORK >= N*NB, where NB is
* the optimal blocksize returned by ILAENV.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, U(i,i) is exactly zero; the matrix is
* singular and its inverse could not be computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param a
* @param _a_offset
* @param lda
* @param ipiv
* @param _ipiv_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dgetri(int n, double[] a, int _a_offset, int lda, int[] ipiv, int _ipiv_offset, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGETRS solves a system of linear equations
* A * X = B or A' * X = B
* with a general N-by-N matrix A using the LU factorization computed
* by DGETRF.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A'* X = B (Transpose)
* = 'C': A'* X = B (Conjugate transpose = Transpose)
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The factors L and U from the factorization A = P*L*U
* as computed by DGETRF.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices from DGETRF; for 1<=i<=N, row i of the
* matrix was interchanged with row IPIV(i).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param trans
* @param n
* @param nrhs
* @param a
* @param lda
* @param ipiv
* @param b
* @param ldb
* @param info
*
*/
abstract public void dgetrs(java.lang.String trans, int n, int nrhs, double[] a, int lda, int[] ipiv, double[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGETRS solves a system of linear equations
* A * X = B or A' * X = B
* with a general N-by-N matrix A using the LU factorization computed
* by DGETRF.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A'* X = B (Transpose)
* = 'C': A'* X = B (Conjugate transpose = Transpose)
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The factors L and U from the factorization A = P*L*U
* as computed by DGETRF.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices from DGETRF; for 1<=i<=N, row i of the
* matrix was interchanged with row IPIV(i).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param trans
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void dgetrs(java.lang.String trans, int n, int nrhs, double[] a, int _a_offset, int lda, int[] ipiv, int _ipiv_offset, double[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGGBAK forms the right or left eigenvectors of a real generalized
* eigenvalue problem A*x = lambda*B*x, by backward transformation on
* the computed eigenvectors of the balanced pair of matrices output by
* DGGBAL.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* Specifies the type of backward transformation required:
* = 'N': do nothing, return immediately;
* = 'P': do backward transformation for permutation only;
* = 'S': do backward transformation for scaling only;
* = 'B': do backward transformations for both permutation and
* scaling.
* JOB must be the same as the argument JOB supplied to DGGBAL.
*
* SIDE (input) CHARACTER*1
* = 'R': V contains right eigenvectors;
* = 'L': V contains left eigenvectors.
*
* N (input) INTEGER
* The number of rows of the matrix V. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* The integers ILO and IHI determined by DGGBAL.
* 1 <= ILO <= IHI <= N, if N > 0; ILO=1 and IHI=0, if N=0.
*
* LSCALE (input) DOUBLE PRECISION array, dimension (N)
* Details of the permutations and/or scaling factors applied
* to the left side of A and B, as returned by DGGBAL.
*
* RSCALE (input) DOUBLE PRECISION array, dimension (N)
* Details of the permutations and/or scaling factors applied
* to the right side of A and B, as returned by DGGBAL.
*
* M (input) INTEGER
* The number of columns of the matrix V. M >= 0.
*
* V (input/output) DOUBLE PRECISION array, dimension (LDV,M)
* On entry, the matrix of right or left eigenvectors to be
* transformed, as returned by DTGEVC.
* On exit, V is overwritten by the transformed eigenvectors.
*
* LDV (input) INTEGER
* The leading dimension of the matrix V. LDV >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* See R.C. Ward, Balancing the generalized eigenvalue problem,
* SIAM J. Sci. Stat. Comp. 2 (1981), 141-152.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param job
* @param side
* @param n
* @param ilo
* @param ihi
* @param lscale
* @param rscale
* @param m
* @param v
* @param ldv
* @param info
*
*/
abstract public void dggbak(java.lang.String job, java.lang.String side, int n, int ilo, int ihi, double[] lscale, double[] rscale, int m, double[] v, int ldv, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGGBAK forms the right or left eigenvectors of a real generalized
* eigenvalue problem A*x = lambda*B*x, by backward transformation on
* the computed eigenvectors of the balanced pair of matrices output by
* DGGBAL.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* Specifies the type of backward transformation required:
* = 'N': do nothing, return immediately;
* = 'P': do backward transformation for permutation only;
* = 'S': do backward transformation for scaling only;
* = 'B': do backward transformations for both permutation and
* scaling.
* JOB must be the same as the argument JOB supplied to DGGBAL.
*
* SIDE (input) CHARACTER*1
* = 'R': V contains right eigenvectors;
* = 'L': V contains left eigenvectors.
*
* N (input) INTEGER
* The number of rows of the matrix V. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* The integers ILO and IHI determined by DGGBAL.
* 1 <= ILO <= IHI <= N, if N > 0; ILO=1 and IHI=0, if N=0.
*
* LSCALE (input) DOUBLE PRECISION array, dimension (N)
* Details of the permutations and/or scaling factors applied
* to the left side of A and B, as returned by DGGBAL.
*
* RSCALE (input) DOUBLE PRECISION array, dimension (N)
* Details of the permutations and/or scaling factors applied
* to the right side of A and B, as returned by DGGBAL.
*
* M (input) INTEGER
* The number of columns of the matrix V. M >= 0.
*
* V (input/output) DOUBLE PRECISION array, dimension (LDV,M)
* On entry, the matrix of right or left eigenvectors to be
* transformed, as returned by DTGEVC.
* On exit, V is overwritten by the transformed eigenvectors.
*
* LDV (input) INTEGER
* The leading dimension of the matrix V. LDV >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* See R.C. Ward, Balancing the generalized eigenvalue problem,
* SIAM J. Sci. Stat. Comp. 2 (1981), 141-152.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param job
* @param side
* @param n
* @param ilo
* @param ihi
* @param lscale
* @param _lscale_offset
* @param rscale
* @param _rscale_offset
* @param m
* @param v
* @param _v_offset
* @param ldv
* @param info
*
*/
abstract public void dggbak(java.lang.String job, java.lang.String side, int n, int ilo, int ihi, double[] lscale, int _lscale_offset, double[] rscale, int _rscale_offset, int m, double[] v, int _v_offset, int ldv, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGGBAL balances a pair of general real matrices (A,B). This
* involves, first, permuting A and B by similarity transformations to
* isolate eigenvalues in the first 1 to ILO$-$1 and last IHI+1 to N
* elements on the diagonal; and second, applying a diagonal similarity
* transformation to rows and columns ILO to IHI to make the rows
* and columns as close in norm as possible. Both steps are optional.
*
* Balancing may reduce the 1-norm of the matrices, and improve the
* accuracy of the computed eigenvalues and/or eigenvectors in the
* generalized eigenvalue problem A*x = lambda*B*x.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* Specifies the operations to be performed on A and B:
* = 'N': none: simply set ILO = 1, IHI = N, LSCALE(I) = 1.0
* and RSCALE(I) = 1.0 for i = 1,...,N.
* = 'P': permute only;
* = 'S': scale only;
* = 'B': both permute and scale.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the input matrix A.
* On exit, A is overwritten by the balanced matrix.
* If JOB = 'N', A is not referenced.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,N)
* On entry, the input matrix B.
* On exit, B is overwritten by the balanced matrix.
* If JOB = 'N', B is not referenced.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* ILO (output) INTEGER
* IHI (output) INTEGER
* ILO and IHI are set to integers such that on exit
* A(i,j) = 0 and B(i,j) = 0 if i > j and
* j = 1,...,ILO-1 or i = IHI+1,...,N.
* If JOB = 'N' or 'S', ILO = 1 and IHI = N.
*
* LSCALE (output) DOUBLE PRECISION array, dimension (N)
* Details of the permutations and scaling factors applied
* to the left side of A and B. If P(j) is the index of the
* row interchanged with row j, and D(j)
* is the scaling factor applied to row j, then
* LSCALE(j) = P(j) for J = 1,...,ILO-1
* = D(j) for J = ILO,...,IHI
* = P(j) for J = IHI+1,...,N.
* The order in which the interchanges are made is N to IHI+1,
* then 1 to ILO-1.
*
* RSCALE (output) DOUBLE PRECISION array, dimension (N)
* Details of the permutations and scaling factors applied
* to the right side of A and B. If P(j) is the index of the
* column interchanged with column j, and D(j)
* is the scaling factor applied to column j, then
* LSCALE(j) = P(j) for J = 1,...,ILO-1
* = D(j) for J = ILO,...,IHI
* = P(j) for J = IHI+1,...,N.
* The order in which the interchanges are made is N to IHI+1,
* then 1 to ILO-1.
*
* WORK (workspace) REAL array, dimension (lwork)
* lwork must be at least max(1,6*N) when JOB = 'S' or 'B', and
* at least 1 when JOB = 'N' or 'P'.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* See R.C. WARD, Balancing the generalized eigenvalue problem,
* SIAM J. Sci. Stat. Comp. 2 (1981), 141-152.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param ilo
* @param ihi
* @param lscale
* @param rscale
* @param work
* @param info
*
*/
abstract public void dggbal(java.lang.String job, int n, double[] a, int lda, double[] b, int ldb, org.netlib.util.intW ilo, org.netlib.util.intW ihi, double[] lscale, double[] rscale, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGGBAL balances a pair of general real matrices (A,B). This
* involves, first, permuting A and B by similarity transformations to
* isolate eigenvalues in the first 1 to ILO$-$1 and last IHI+1 to N
* elements on the diagonal; and second, applying a diagonal similarity
* transformation to rows and columns ILO to IHI to make the rows
* and columns as close in norm as possible. Both steps are optional.
*
* Balancing may reduce the 1-norm of the matrices, and improve the
* accuracy of the computed eigenvalues and/or eigenvectors in the
* generalized eigenvalue problem A*x = lambda*B*x.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* Specifies the operations to be performed on A and B:
* = 'N': none: simply set ILO = 1, IHI = N, LSCALE(I) = 1.0
* and RSCALE(I) = 1.0 for i = 1,...,N.
* = 'P': permute only;
* = 'S': scale only;
* = 'B': both permute and scale.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the input matrix A.
* On exit, A is overwritten by the balanced matrix.
* If JOB = 'N', A is not referenced.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,N)
* On entry, the input matrix B.
* On exit, B is overwritten by the balanced matrix.
* If JOB = 'N', B is not referenced.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* ILO (output) INTEGER
* IHI (output) INTEGER
* ILO and IHI are set to integers such that on exit
* A(i,j) = 0 and B(i,j) = 0 if i > j and
* j = 1,...,ILO-1 or i = IHI+1,...,N.
* If JOB = 'N' or 'S', ILO = 1 and IHI = N.
*
* LSCALE (output) DOUBLE PRECISION array, dimension (N)
* Details of the permutations and scaling factors applied
* to the left side of A and B. If P(j) is the index of the
* row interchanged with row j, and D(j)
* is the scaling factor applied to row j, then
* LSCALE(j) = P(j) for J = 1,...,ILO-1
* = D(j) for J = ILO,...,IHI
* = P(j) for J = IHI+1,...,N.
* The order in which the interchanges are made is N to IHI+1,
* then 1 to ILO-1.
*
* RSCALE (output) DOUBLE PRECISION array, dimension (N)
* Details of the permutations and scaling factors applied
* to the right side of A and B. If P(j) is the index of the
* column interchanged with column j, and D(j)
* is the scaling factor applied to column j, then
* LSCALE(j) = P(j) for J = 1,...,ILO-1
* = D(j) for J = ILO,...,IHI
* = P(j) for J = IHI+1,...,N.
* The order in which the interchanges are made is N to IHI+1,
* then 1 to ILO-1.
*
* WORK (workspace) REAL array, dimension (lwork)
* lwork must be at least max(1,6*N) when JOB = 'S' or 'B', and
* at least 1 when JOB = 'N' or 'P'.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* See R.C. WARD, Balancing the generalized eigenvalue problem,
* SIAM J. Sci. Stat. Comp. 2 (1981), 141-152.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param ilo
* @param ihi
* @param lscale
* @param _lscale_offset
* @param rscale
* @param _rscale_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dggbal(java.lang.String job, int n, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, org.netlib.util.intW ilo, org.netlib.util.intW ihi, double[] lscale, int _lscale_offset, double[] rscale, int _rscale_offset, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGGES computes for a pair of N-by-N real nonsymmetric matrices (A,B),
* the generalized eigenvalues, the generalized real Schur form (S,T),
* optionally, the left and/or right matrices of Schur vectors (VSL and
* VSR). This gives the generalized Schur factorization
*
* (A,B) = ( (VSL)*S*(VSR)**T, (VSL)*T*(VSR)**T )
*
* Optionally, it also orders the eigenvalues so that a selected cluster
* of eigenvalues appears in the leading diagonal blocks of the upper
* quasi-triangular matrix S and the upper triangular matrix T.The
* leading columns of VSL and VSR then form an orthonormal basis for the
* corresponding left and right eigenspaces (deflating subspaces).
*
* (If only the generalized eigenvalues are needed, use the driver
* DGGEV instead, which is faster.)
*
* A generalized eigenvalue for a pair of matrices (A,B) is a scalar w
* or a ratio alpha/beta = w, such that A - w*B is singular. It is
* usually represented as the pair (alpha,beta), as there is a
* reasonable interpretation for beta=0 or both being zero.
*
* A pair of matrices (S,T) is in generalized real Schur form if T is
* upper triangular with non-negative diagonal and S is block upper
* triangular with 1-by-1 and 2-by-2 blocks. 1-by-1 blocks correspond
* to real generalized eigenvalues, while 2-by-2 blocks of S will be
* "standardized" by making the corresponding elements of T have the
* form:
* [ a 0 ]
* [ 0 b ]
*
* and the pair of corresponding 2-by-2 blocks in S and T will have a
* complex conjugate pair of generalized eigenvalues.
*
*
* Arguments
* =========
*
* JOBVSL (input) CHARACTER*1
* = 'N': do not compute the left Schur vectors;
* = 'V': compute the left Schur vectors.
*
* JOBVSR (input) CHARACTER*1
* = 'N': do not compute the right Schur vectors;
* = 'V': compute the right Schur vectors.
*
* SORT (input) CHARACTER*1
* Specifies whether or not to order the eigenvalues on the
* diagonal of the generalized Schur form.
* = 'N': Eigenvalues are not ordered;
* = 'S': Eigenvalues are ordered (see SELCTG);
*
* SELCTG (external procedure) LOGICAL FUNCTION of three DOUBLE PRECISI
* SELCTG must be declared EXTERNAL in the calling subroutine.
* If SORT = 'N', SELCTG is not referenced.
* If SORT = 'S', SELCTG is used to select eigenvalues to sort
* to the top left of the Schur form.
* An eigenvalue (ALPHAR(j)+ALPHAI(j))/BETA(j) is selected if
* SELCTG(ALPHAR(j),ALPHAI(j),BETA(j)) is true; i.e. if either
* one of a complex conjugate pair of eigenvalues is selected,
* then both complex eigenvalues are selected.
*
* Note that in the ill-conditioned case, a selected complex
* eigenvalue may no longer satisfy SELCTG(ALPHAR(j),ALPHAI(j),
* BETA(j)) = .TRUE. after ordering. INFO is to be set to N+2
* in this case.
*
* N (input) INTEGER
* The order of the matrices A, B, VSL, and VSR. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the first of the pair of matrices.
* On exit, A has been overwritten by its generalized Schur
* form S.
*
* LDA (input) INTEGER
* The leading dimension of A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB, N)
* On entry, the second of the pair of matrices.
* On exit, B has been overwritten by its generalized Schur
* form T.
*
* LDB (input) INTEGER
* The leading dimension of B. LDB >= max(1,N).
*
* SDIM (output) INTEGER
* If SORT = 'N', SDIM = 0.
* If SORT = 'S', SDIM = number of eigenvalues (after sorting)
* for which SELCTG is true. (Complex conjugate pairs for which
* SELCTG is true for either eigenvalue count as 2.)
*
* ALPHAR (output) DOUBLE PRECISION array, dimension (N)
* ALPHAI (output) DOUBLE PRECISION array, dimension (N)
* BETA (output) DOUBLE PRECISION array, dimension (N)
* On exit, (ALPHAR(j) + ALPHAI(j)*i)/BETA(j), j=1,...,N, will
* be the generalized eigenvalues. ALPHAR(j) + ALPHAI(j)*i,
* and BETA(j),j=1,...,N are the diagonals of the complex Schur
* form (S,T) that would result if the 2-by-2 diagonal blocks of
* the real Schur form of (A,B) were further reduced to
* triangular form using 2-by-2 complex unitary transformations.
* If ALPHAI(j) is zero, then the j-th eigenvalue is real; if
* positive, then the j-th and (j+1)-st eigenvalues are a
* complex conjugate pair, with ALPHAI(j+1) negative.
*
* Note: the quotients ALPHAR(j)/BETA(j) and ALPHAI(j)/BETA(j)
* may easily over- or underflow, and BETA(j) may even be zero.
* Thus, the user should avoid naively computing the ratio.
* However, ALPHAR and ALPHAI will be always less than and
* usually comparable with norm(A) in magnitude, and BETA always
* less than and usually comparable with norm(B).
*
* VSL (output) DOUBLE PRECISION array, dimension (LDVSL,N)
* If JOBVSL = 'V', VSL will contain the left Schur vectors.
* Not referenced if JOBVSL = 'N'.
*
* LDVSL (input) INTEGER
* The leading dimension of the matrix VSL. LDVSL >=1, and
* if JOBVSL = 'V', LDVSL >= N.
*
* VSR (output) DOUBLE PRECISION array, dimension (LDVSR,N)
* If JOBVSR = 'V', VSR will contain the right Schur vectors.
* Not referenced if JOBVSR = 'N'.
*
* LDVSR (input) INTEGER
* The leading dimension of the matrix VSR. LDVSR >= 1, and
* if JOBVSR = 'V', LDVSR >= N.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If N = 0, LWORK >= 1, else LWORK >= 8*N+16.
* For good performance , LWORK must generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* BWORK (workspace) LOGICAL array, dimension (N)
* Not referenced if SORT = 'N'.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1,...,N:
* The QZ iteration failed. (A,B) are not in Schur
* form, but ALPHAR(j), ALPHAI(j), and BETA(j) should
* be correct for j=INFO+1,...,N.
* > N: =N+1: other than QZ iteration failed in DHGEQZ.
* =N+2: after reordering, roundoff changed values of
* some complex eigenvalues so that leading
* eigenvalues in the Generalized Schur form no
* longer satisfy SELCTG=.TRUE. This could also
* be caused due to scaling.
* =N+3: reordering failed in DTGSEN.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobvsl
* @param jobvsr
* @param sort
* @param selctg
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param sdim
* @param alphar
* @param alphai
* @param beta
* @param vsl
* @param ldvsl
* @param vsr
* @param ldvsr
* @param work
* @param lwork
* @param bwork
* @param info
*
*/
abstract public void dgges(java.lang.String jobvsl, java.lang.String jobvsr, java.lang.String sort, java.lang.Object selctg, int n, double[] a, int lda, double[] b, int ldb, org.netlib.util.intW sdim, double[] alphar, double[] alphai, double[] beta, double[] vsl, int ldvsl, double[] vsr, int ldvsr, double[] work, int lwork, boolean[] bwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGGES computes for a pair of N-by-N real nonsymmetric matrices (A,B),
* the generalized eigenvalues, the generalized real Schur form (S,T),
* optionally, the left and/or right matrices of Schur vectors (VSL and
* VSR). This gives the generalized Schur factorization
*
* (A,B) = ( (VSL)*S*(VSR)**T, (VSL)*T*(VSR)**T )
*
* Optionally, it also orders the eigenvalues so that a selected cluster
* of eigenvalues appears in the leading diagonal blocks of the upper
* quasi-triangular matrix S and the upper triangular matrix T.The
* leading columns of VSL and VSR then form an orthonormal basis for the
* corresponding left and right eigenspaces (deflating subspaces).
*
* (If only the generalized eigenvalues are needed, use the driver
* DGGEV instead, which is faster.)
*
* A generalized eigenvalue for a pair of matrices (A,B) is a scalar w
* or a ratio alpha/beta = w, such that A - w*B is singular. It is
* usually represented as the pair (alpha,beta), as there is a
* reasonable interpretation for beta=0 or both being zero.
*
* A pair of matrices (S,T) is in generalized real Schur form if T is
* upper triangular with non-negative diagonal and S is block upper
* triangular with 1-by-1 and 2-by-2 blocks. 1-by-1 blocks correspond
* to real generalized eigenvalues, while 2-by-2 blocks of S will be
* "standardized" by making the corresponding elements of T have the
* form:
* [ a 0 ]
* [ 0 b ]
*
* and the pair of corresponding 2-by-2 blocks in S and T will have a
* complex conjugate pair of generalized eigenvalues.
*
*
* Arguments
* =========
*
* JOBVSL (input) CHARACTER*1
* = 'N': do not compute the left Schur vectors;
* = 'V': compute the left Schur vectors.
*
* JOBVSR (input) CHARACTER*1
* = 'N': do not compute the right Schur vectors;
* = 'V': compute the right Schur vectors.
*
* SORT (input) CHARACTER*1
* Specifies whether or not to order the eigenvalues on the
* diagonal of the generalized Schur form.
* = 'N': Eigenvalues are not ordered;
* = 'S': Eigenvalues are ordered (see SELCTG);
*
* SELCTG (external procedure) LOGICAL FUNCTION of three DOUBLE PRECISI
* SELCTG must be declared EXTERNAL in the calling subroutine.
* If SORT = 'N', SELCTG is not referenced.
* If SORT = 'S', SELCTG is used to select eigenvalues to sort
* to the top left of the Schur form.
* An eigenvalue (ALPHAR(j)+ALPHAI(j))/BETA(j) is selected if
* SELCTG(ALPHAR(j),ALPHAI(j),BETA(j)) is true; i.e. if either
* one of a complex conjugate pair of eigenvalues is selected,
* then both complex eigenvalues are selected.
*
* Note that in the ill-conditioned case, a selected complex
* eigenvalue may no longer satisfy SELCTG(ALPHAR(j),ALPHAI(j),
* BETA(j)) = .TRUE. after ordering. INFO is to be set to N+2
* in this case.
*
* N (input) INTEGER
* The order of the matrices A, B, VSL, and VSR. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the first of the pair of matrices.
* On exit, A has been overwritten by its generalized Schur
* form S.
*
* LDA (input) INTEGER
* The leading dimension of A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB, N)
* On entry, the second of the pair of matrices.
* On exit, B has been overwritten by its generalized Schur
* form T.
*
* LDB (input) INTEGER
* The leading dimension of B. LDB >= max(1,N).
*
* SDIM (output) INTEGER
* If SORT = 'N', SDIM = 0.
* If SORT = 'S', SDIM = number of eigenvalues (after sorting)
* for which SELCTG is true. (Complex conjugate pairs for which
* SELCTG is true for either eigenvalue count as 2.)
*
* ALPHAR (output) DOUBLE PRECISION array, dimension (N)
* ALPHAI (output) DOUBLE PRECISION array, dimension (N)
* BETA (output) DOUBLE PRECISION array, dimension (N)
* On exit, (ALPHAR(j) + ALPHAI(j)*i)/BETA(j), j=1,...,N, will
* be the generalized eigenvalues. ALPHAR(j) + ALPHAI(j)*i,
* and BETA(j),j=1,...,N are the diagonals of the complex Schur
* form (S,T) that would result if the 2-by-2 diagonal blocks of
* the real Schur form of (A,B) were further reduced to
* triangular form using 2-by-2 complex unitary transformations.
* If ALPHAI(j) is zero, then the j-th eigenvalue is real; if
* positive, then the j-th and (j+1)-st eigenvalues are a
* complex conjugate pair, with ALPHAI(j+1) negative.
*
* Note: the quotients ALPHAR(j)/BETA(j) and ALPHAI(j)/BETA(j)
* may easily over- or underflow, and BETA(j) may even be zero.
* Thus, the user should avoid naively computing the ratio.
* However, ALPHAR and ALPHAI will be always less than and
* usually comparable with norm(A) in magnitude, and BETA always
* less than and usually comparable with norm(B).
*
* VSL (output) DOUBLE PRECISION array, dimension (LDVSL,N)
* If JOBVSL = 'V', VSL will contain the left Schur vectors.
* Not referenced if JOBVSL = 'N'.
*
* LDVSL (input) INTEGER
* The leading dimension of the matrix VSL. LDVSL >=1, and
* if JOBVSL = 'V', LDVSL >= N.
*
* VSR (output) DOUBLE PRECISION array, dimension (LDVSR,N)
* If JOBVSR = 'V', VSR will contain the right Schur vectors.
* Not referenced if JOBVSR = 'N'.
*
* LDVSR (input) INTEGER
* The leading dimension of the matrix VSR. LDVSR >= 1, and
* if JOBVSR = 'V', LDVSR >= N.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If N = 0, LWORK >= 1, else LWORK >= 8*N+16.
* For good performance , LWORK must generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* BWORK (workspace) LOGICAL array, dimension (N)
* Not referenced if SORT = 'N'.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1,...,N:
* The QZ iteration failed. (A,B) are not in Schur
* form, but ALPHAR(j), ALPHAI(j), and BETA(j) should
* be correct for j=INFO+1,...,N.
* > N: =N+1: other than QZ iteration failed in DHGEQZ.
* =N+2: after reordering, roundoff changed values of
* some complex eigenvalues so that leading
* eigenvalues in the Generalized Schur form no
* longer satisfy SELCTG=.TRUE. This could also
* be caused due to scaling.
* =N+3: reordering failed in DTGSEN.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobvsl
* @param jobvsr
* @param sort
* @param selctg
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param sdim
* @param alphar
* @param _alphar_offset
* @param alphai
* @param _alphai_offset
* @param beta
* @param _beta_offset
* @param vsl
* @param _vsl_offset
* @param ldvsl
* @param vsr
* @param _vsr_offset
* @param ldvsr
* @param work
* @param _work_offset
* @param lwork
* @param bwork
* @param _bwork_offset
* @param info
*
*/
abstract public void dgges(java.lang.String jobvsl, java.lang.String jobvsr, java.lang.String sort, java.lang.Object selctg, int n, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, org.netlib.util.intW sdim, double[] alphar, int _alphar_offset, double[] alphai, int _alphai_offset, double[] beta, int _beta_offset, double[] vsl, int _vsl_offset, int ldvsl, double[] vsr, int _vsr_offset, int ldvsr, double[] work, int _work_offset, int lwork, boolean[] bwork, int _bwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGGESX computes for a pair of N-by-N real nonsymmetric matrices
* (A,B), the generalized eigenvalues, the real Schur form (S,T), and,
* optionally, the left and/or right matrices of Schur vectors (VSL and
* VSR). This gives the generalized Schur factorization
*
* (A,B) = ( (VSL) S (VSR)**T, (VSL) T (VSR)**T )
*
* Optionally, it also orders the eigenvalues so that a selected cluster
* of eigenvalues appears in the leading diagonal blocks of the upper
* quasi-triangular matrix S and the upper triangular matrix T; computes
* a reciprocal condition number for the average of the selected
* eigenvalues (RCONDE); and computes a reciprocal condition number for
* the right and left deflating subspaces corresponding to the selected
* eigenvalues (RCONDV). The leading columns of VSL and VSR then form
* an orthonormal basis for the corresponding left and right eigenspaces
* (deflating subspaces).
*
* A generalized eigenvalue for a pair of matrices (A,B) is a scalar w
* or a ratio alpha/beta = w, such that A - w*B is singular. It is
* usually represented as the pair (alpha,beta), as there is a
* reasonable interpretation for beta=0 or for both being zero.
*
* A pair of matrices (S,T) is in generalized real Schur form if T is
* upper triangular with non-negative diagonal and S is block upper
* triangular with 1-by-1 and 2-by-2 blocks. 1-by-1 blocks correspond
* to real generalized eigenvalues, while 2-by-2 blocks of S will be
* "standardized" by making the corresponding elements of T have the
* form:
* [ a 0 ]
* [ 0 b ]
*
* and the pair of corresponding 2-by-2 blocks in S and T will have a
* complex conjugate pair of generalized eigenvalues.
*
*
* Arguments
* =========
*
* JOBVSL (input) CHARACTER*1
* = 'N': do not compute the left Schur vectors;
* = 'V': compute the left Schur vectors.
*
* JOBVSR (input) CHARACTER*1
* = 'N': do not compute the right Schur vectors;
* = 'V': compute the right Schur vectors.
*
* SORT (input) CHARACTER*1
* Specifies whether or not to order the eigenvalues on the
* diagonal of the generalized Schur form.
* = 'N': Eigenvalues are not ordered;
* = 'S': Eigenvalues are ordered (see SELCTG).
*
* SELCTG (external procedure) LOGICAL FUNCTION of three DOUBLE PRECISI
* SELCTG must be declared EXTERNAL in the calling subroutine.
* If SORT = 'N', SELCTG is not referenced.
* If SORT = 'S', SELCTG is used to select eigenvalues to sort
* to the top left of the Schur form.
* An eigenvalue (ALPHAR(j)+ALPHAI(j))/BETA(j) is selected if
* SELCTG(ALPHAR(j),ALPHAI(j),BETA(j)) is true; i.e. if either
* one of a complex conjugate pair of eigenvalues is selected,
* then both complex eigenvalues are selected.
* Note that a selected complex eigenvalue may no longer satisfy
* SELCTG(ALPHAR(j),ALPHAI(j),BETA(j)) = .TRUE. after ordering,
* since ordering may change the value of complex eigenvalues
* (especially if the eigenvalue is ill-conditioned), in this
* case INFO is set to N+3.
*
* SENSE (input) CHARACTER*1
* Determines which reciprocal condition numbers are computed.
* = 'N' : None are computed;
* = 'E' : Computed for average of selected eigenvalues only;
* = 'V' : Computed for selected deflating subspaces only;
* = 'B' : Computed for both.
* If SENSE = 'E', 'V', or 'B', SORT must equal 'S'.
*
* N (input) INTEGER
* The order of the matrices A, B, VSL, and VSR. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the first of the pair of matrices.
* On exit, A has been overwritten by its generalized Schur
* form S.
*
* LDA (input) INTEGER
* The leading dimension of A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB, N)
* On entry, the second of the pair of matrices.
* On exit, B has been overwritten by its generalized Schur
* form T.
*
* LDB (input) INTEGER
* The leading dimension of B. LDB >= max(1,N).
*
* SDIM (output) INTEGER
* If SORT = 'N', SDIM = 0.
* If SORT = 'S', SDIM = number of eigenvalues (after sorting)
* for which SELCTG is true. (Complex conjugate pairs for which
* SELCTG is true for either eigenvalue count as 2.)
*
* ALPHAR (output) DOUBLE PRECISION array, dimension (N)
* ALPHAI (output) DOUBLE PRECISION array, dimension (N)
* BETA (output) DOUBLE PRECISION array, dimension (N)
* On exit, (ALPHAR(j) + ALPHAI(j)*i)/BETA(j), j=1,...,N, will
* be the generalized eigenvalues. ALPHAR(j) + ALPHAI(j)*i
* and BETA(j),j=1,...,N are the diagonals of the complex Schur
* form (S,T) that would result if the 2-by-2 diagonal blocks of
* the real Schur form of (A,B) were further reduced to
* triangular form using 2-by-2 complex unitary transformations.
* If ALPHAI(j) is zero, then the j-th eigenvalue is real; if
* positive, then the j-th and (j+1)-st eigenvalues are a
* complex conjugate pair, with ALPHAI(j+1) negative.
*
* Note: the quotients ALPHAR(j)/BETA(j) and ALPHAI(j)/BETA(j)
* may easily over- or underflow, and BETA(j) may even be zero.
* Thus, the user should avoid naively computing the ratio.
* However, ALPHAR and ALPHAI will be always less than and
* usually comparable with norm(A) in magnitude, and BETA always
* less than and usually comparable with norm(B).
*
* VSL (output) DOUBLE PRECISION array, dimension (LDVSL,N)
* If JOBVSL = 'V', VSL will contain the left Schur vectors.
* Not referenced if JOBVSL = 'N'.
*
* LDVSL (input) INTEGER
* The leading dimension of the matrix VSL. LDVSL >=1, and
* if JOBVSL = 'V', LDVSL >= N.
*
* VSR (output) DOUBLE PRECISION array, dimension (LDVSR,N)
* If JOBVSR = 'V', VSR will contain the right Schur vectors.
* Not referenced if JOBVSR = 'N'.
*
* LDVSR (input) INTEGER
* The leading dimension of the matrix VSR. LDVSR >= 1, and
* if JOBVSR = 'V', LDVSR >= N.
*
* RCONDE (output) DOUBLE PRECISION array, dimension ( 2 )
* If SENSE = 'E' or 'B', RCONDE(1) and RCONDE(2) contain the
* reciprocal condition numbers for the average of the selected
* eigenvalues.
* Not referenced if SENSE = 'N' or 'V'.
*
* RCONDV (output) DOUBLE PRECISION array, dimension ( 2 )
* If SENSE = 'V' or 'B', RCONDV(1) and RCONDV(2) contain the
* reciprocal condition numbers for the selected deflating
* subspaces.
* Not referenced if SENSE = 'N' or 'E'.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If N = 0, LWORK >= 1, else if SENSE = 'E', 'V', or 'B',
* LWORK >= max( 8*N, 6*N+16, 2*SDIM*(N-SDIM) ), else
* LWORK >= max( 8*N, 6*N+16 ).
* Note that 2*SDIM*(N-SDIM) <= N*N/2.
* Note also that an error is only returned if
* LWORK < max( 8*N, 6*N+16), but if SENSE = 'E' or 'V' or 'B'
* this may not be large enough.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the bound on the optimal size of the WORK
* array and the minimum size of the IWORK array, returns these
* values as the first entries of the WORK and IWORK arrays, and
* no error message related to LWORK or LIWORK is issued by
* XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the minimum LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If SENSE = 'N' or N = 0, LIWORK >= 1, otherwise
* LIWORK >= N+6.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the bound on the optimal size of the
* WORK array and the minimum size of the IWORK array, returns
* these values as the first entries of the WORK and IWORK
* arrays, and no error message related to LWORK or LIWORK is
* issued by XERBLA.
*
* BWORK (workspace) LOGICAL array, dimension (N)
* Not referenced if SORT = 'N'.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1,...,N:
* The QZ iteration failed. (A,B) are not in Schur
* form, but ALPHAR(j), ALPHAI(j), and BETA(j) should
* be correct for j=INFO+1,...,N.
* > N: =N+1: other than QZ iteration failed in DHGEQZ
* =N+2: after reordering, roundoff changed values of
* some complex eigenvalues so that leading
* eigenvalues in the Generalized Schur form no
* longer satisfy SELCTG=.TRUE. This could also
* be caused due to scaling.
* =N+3: reordering failed in DTGSEN.
*
* Further details
* ===============
*
* An approximate (asymptotic) bound on the average absolute error of
* the selected eigenvalues is
*
* EPS * norm((A, B)) / RCONDE( 1 ).
*
* An approximate (asymptotic) bound on the maximum angular error in
* the computed deflating subspaces is
*
* EPS * norm((A, B)) / RCONDV( 2 ).
*
* See LAPACK User's Guide, section 4.11 for more information.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobvsl
* @param jobvsr
* @param sort
* @param selctg
* @param sense
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param sdim
* @param alphar
* @param alphai
* @param beta
* @param vsl
* @param ldvsl
* @param vsr
* @param ldvsr
* @param rconde
* @param rcondv
* @param work
* @param lwork
* @param iwork
* @param liwork
* @param bwork
* @param info
*
*/
abstract public void dggesx(java.lang.String jobvsl, java.lang.String jobvsr, java.lang.String sort, java.lang.Object selctg, java.lang.String sense, int n, double[] a, int lda, double[] b, int ldb, org.netlib.util.intW sdim, double[] alphar, double[] alphai, double[] beta, double[] vsl, int ldvsl, double[] vsr, int ldvsr, double[] rconde, double[] rcondv, double[] work, int lwork, int[] iwork, int liwork, boolean[] bwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGGESX computes for a pair of N-by-N real nonsymmetric matrices
* (A,B), the generalized eigenvalues, the real Schur form (S,T), and,
* optionally, the left and/or right matrices of Schur vectors (VSL and
* VSR). This gives the generalized Schur factorization
*
* (A,B) = ( (VSL) S (VSR)**T, (VSL) T (VSR)**T )
*
* Optionally, it also orders the eigenvalues so that a selected cluster
* of eigenvalues appears in the leading diagonal blocks of the upper
* quasi-triangular matrix S and the upper triangular matrix T; computes
* a reciprocal condition number for the average of the selected
* eigenvalues (RCONDE); and computes a reciprocal condition number for
* the right and left deflating subspaces corresponding to the selected
* eigenvalues (RCONDV). The leading columns of VSL and VSR then form
* an orthonormal basis for the corresponding left and right eigenspaces
* (deflating subspaces).
*
* A generalized eigenvalue for a pair of matrices (A,B) is a scalar w
* or a ratio alpha/beta = w, such that A - w*B is singular. It is
* usually represented as the pair (alpha,beta), as there is a
* reasonable interpretation for beta=0 or for both being zero.
*
* A pair of matrices (S,T) is in generalized real Schur form if T is
* upper triangular with non-negative diagonal and S is block upper
* triangular with 1-by-1 and 2-by-2 blocks. 1-by-1 blocks correspond
* to real generalized eigenvalues, while 2-by-2 blocks of S will be
* "standardized" by making the corresponding elements of T have the
* form:
* [ a 0 ]
* [ 0 b ]
*
* and the pair of corresponding 2-by-2 blocks in S and T will have a
* complex conjugate pair of generalized eigenvalues.
*
*
* Arguments
* =========
*
* JOBVSL (input) CHARACTER*1
* = 'N': do not compute the left Schur vectors;
* = 'V': compute the left Schur vectors.
*
* JOBVSR (input) CHARACTER*1
* = 'N': do not compute the right Schur vectors;
* = 'V': compute the right Schur vectors.
*
* SORT (input) CHARACTER*1
* Specifies whether or not to order the eigenvalues on the
* diagonal of the generalized Schur form.
* = 'N': Eigenvalues are not ordered;
* = 'S': Eigenvalues are ordered (see SELCTG).
*
* SELCTG (external procedure) LOGICAL FUNCTION of three DOUBLE PRECISI
* SELCTG must be declared EXTERNAL in the calling subroutine.
* If SORT = 'N', SELCTG is not referenced.
* If SORT = 'S', SELCTG is used to select eigenvalues to sort
* to the top left of the Schur form.
* An eigenvalue (ALPHAR(j)+ALPHAI(j))/BETA(j) is selected if
* SELCTG(ALPHAR(j),ALPHAI(j),BETA(j)) is true; i.e. if either
* one of a complex conjugate pair of eigenvalues is selected,
* then both complex eigenvalues are selected.
* Note that a selected complex eigenvalue may no longer satisfy
* SELCTG(ALPHAR(j),ALPHAI(j),BETA(j)) = .TRUE. after ordering,
* since ordering may change the value of complex eigenvalues
* (especially if the eigenvalue is ill-conditioned), in this
* case INFO is set to N+3.
*
* SENSE (input) CHARACTER*1
* Determines which reciprocal condition numbers are computed.
* = 'N' : None are computed;
* = 'E' : Computed for average of selected eigenvalues only;
* = 'V' : Computed for selected deflating subspaces only;
* = 'B' : Computed for both.
* If SENSE = 'E', 'V', or 'B', SORT must equal 'S'.
*
* N (input) INTEGER
* The order of the matrices A, B, VSL, and VSR. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the first of the pair of matrices.
* On exit, A has been overwritten by its generalized Schur
* form S.
*
* LDA (input) INTEGER
* The leading dimension of A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB, N)
* On entry, the second of the pair of matrices.
* On exit, B has been overwritten by its generalized Schur
* form T.
*
* LDB (input) INTEGER
* The leading dimension of B. LDB >= max(1,N).
*
* SDIM (output) INTEGER
* If SORT = 'N', SDIM = 0.
* If SORT = 'S', SDIM = number of eigenvalues (after sorting)
* for which SELCTG is true. (Complex conjugate pairs for which
* SELCTG is true for either eigenvalue count as 2.)
*
* ALPHAR (output) DOUBLE PRECISION array, dimension (N)
* ALPHAI (output) DOUBLE PRECISION array, dimension (N)
* BETA (output) DOUBLE PRECISION array, dimension (N)
* On exit, (ALPHAR(j) + ALPHAI(j)*i)/BETA(j), j=1,...,N, will
* be the generalized eigenvalues. ALPHAR(j) + ALPHAI(j)*i
* and BETA(j),j=1,...,N are the diagonals of the complex Schur
* form (S,T) that would result if the 2-by-2 diagonal blocks of
* the real Schur form of (A,B) were further reduced to
* triangular form using 2-by-2 complex unitary transformations.
* If ALPHAI(j) is zero, then the j-th eigenvalue is real; if
* positive, then the j-th and (j+1)-st eigenvalues are a
* complex conjugate pair, with ALPHAI(j+1) negative.
*
* Note: the quotients ALPHAR(j)/BETA(j) and ALPHAI(j)/BETA(j)
* may easily over- or underflow, and BETA(j) may even be zero.
* Thus, the user should avoid naively computing the ratio.
* However, ALPHAR and ALPHAI will be always less than and
* usually comparable with norm(A) in magnitude, and BETA always
* less than and usually comparable with norm(B).
*
* VSL (output) DOUBLE PRECISION array, dimension (LDVSL,N)
* If JOBVSL = 'V', VSL will contain the left Schur vectors.
* Not referenced if JOBVSL = 'N'.
*
* LDVSL (input) INTEGER
* The leading dimension of the matrix VSL. LDVSL >=1, and
* if JOBVSL = 'V', LDVSL >= N.
*
* VSR (output) DOUBLE PRECISION array, dimension (LDVSR,N)
* If JOBVSR = 'V', VSR will contain the right Schur vectors.
* Not referenced if JOBVSR = 'N'.
*
* LDVSR (input) INTEGER
* The leading dimension of the matrix VSR. LDVSR >= 1, and
* if JOBVSR = 'V', LDVSR >= N.
*
* RCONDE (output) DOUBLE PRECISION array, dimension ( 2 )
* If SENSE = 'E' or 'B', RCONDE(1) and RCONDE(2) contain the
* reciprocal condition numbers for the average of the selected
* eigenvalues.
* Not referenced if SENSE = 'N' or 'V'.
*
* RCONDV (output) DOUBLE PRECISION array, dimension ( 2 )
* If SENSE = 'V' or 'B', RCONDV(1) and RCONDV(2) contain the
* reciprocal condition numbers for the selected deflating
* subspaces.
* Not referenced if SENSE = 'N' or 'E'.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If N = 0, LWORK >= 1, else if SENSE = 'E', 'V', or 'B',
* LWORK >= max( 8*N, 6*N+16, 2*SDIM*(N-SDIM) ), else
* LWORK >= max( 8*N, 6*N+16 ).
* Note that 2*SDIM*(N-SDIM) <= N*N/2.
* Note also that an error is only returned if
* LWORK < max( 8*N, 6*N+16), but if SENSE = 'E' or 'V' or 'B'
* this may not be large enough.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the bound on the optimal size of the WORK
* array and the minimum size of the IWORK array, returns these
* values as the first entries of the WORK and IWORK arrays, and
* no error message related to LWORK or LIWORK is issued by
* XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the minimum LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If SENSE = 'N' or N = 0, LIWORK >= 1, otherwise
* LIWORK >= N+6.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the bound on the optimal size of the
* WORK array and the minimum size of the IWORK array, returns
* these values as the first entries of the WORK and IWORK
* arrays, and no error message related to LWORK or LIWORK is
* issued by XERBLA.
*
* BWORK (workspace) LOGICAL array, dimension (N)
* Not referenced if SORT = 'N'.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1,...,N:
* The QZ iteration failed. (A,B) are not in Schur
* form, but ALPHAR(j), ALPHAI(j), and BETA(j) should
* be correct for j=INFO+1,...,N.
* > N: =N+1: other than QZ iteration failed in DHGEQZ
* =N+2: after reordering, roundoff changed values of
* some complex eigenvalues so that leading
* eigenvalues in the Generalized Schur form no
* longer satisfy SELCTG=.TRUE. This could also
* be caused due to scaling.
* =N+3: reordering failed in DTGSEN.
*
* Further details
* ===============
*
* An approximate (asymptotic) bound on the average absolute error of
* the selected eigenvalues is
*
* EPS * norm((A, B)) / RCONDE( 1 ).
*
* An approximate (asymptotic) bound on the maximum angular error in
* the computed deflating subspaces is
*
* EPS * norm((A, B)) / RCONDV( 2 ).
*
* See LAPACK User's Guide, section 4.11 for more information.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobvsl
* @param jobvsr
* @param sort
* @param selctg
* @param sense
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param sdim
* @param alphar
* @param _alphar_offset
* @param alphai
* @param _alphai_offset
* @param beta
* @param _beta_offset
* @param vsl
* @param _vsl_offset
* @param ldvsl
* @param vsr
* @param _vsr_offset
* @param ldvsr
* @param rconde
* @param _rconde_offset
* @param rcondv
* @param _rcondv_offset
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param liwork
* @param bwork
* @param _bwork_offset
* @param info
*
*/
abstract public void dggesx(java.lang.String jobvsl, java.lang.String jobvsr, java.lang.String sort, java.lang.Object selctg, java.lang.String sense, int n, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, org.netlib.util.intW sdim, double[] alphar, int _alphar_offset, double[] alphai, int _alphai_offset, double[] beta, int _beta_offset, double[] vsl, int _vsl_offset, int ldvsl, double[] vsr, int _vsr_offset, int ldvsr, double[] rconde, int _rconde_offset, double[] rcondv, int _rcondv_offset, double[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int liwork, boolean[] bwork, int _bwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGGEV computes for a pair of N-by-N real nonsymmetric matrices (A,B)
* the generalized eigenvalues, and optionally, the left and/or right
* generalized eigenvectors.
*
* A generalized eigenvalue for a pair of matrices (A,B) is a scalar
* lambda or a ratio alpha/beta = lambda, such that A - lambda*B is
* singular. It is usually represented as the pair (alpha,beta), as
* there is a reasonable interpretation for beta=0, and even for both
* being zero.
*
* The right eigenvector v(j) corresponding to the eigenvalue lambda(j)
* of (A,B) satisfies
*
* A * v(j) = lambda(j) * B * v(j).
*
* The left eigenvector u(j) corresponding to the eigenvalue lambda(j)
* of (A,B) satisfies
*
* u(j)**H * A = lambda(j) * u(j)**H * B .
*
* where u(j)**H is the conjugate-transpose of u(j).
*
*
* Arguments
* =========
*
* JOBVL (input) CHARACTER*1
* = 'N': do not compute the left generalized eigenvectors;
* = 'V': compute the left generalized eigenvectors.
*
* JOBVR (input) CHARACTER*1
* = 'N': do not compute the right generalized eigenvectors;
* = 'V': compute the right generalized eigenvectors.
*
* N (input) INTEGER
* The order of the matrices A, B, VL, and VR. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the matrix A in the pair (A,B).
* On exit, A has been overwritten.
*
* LDA (input) INTEGER
* The leading dimension of A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB, N)
* On entry, the matrix B in the pair (A,B).
* On exit, B has been overwritten.
*
* LDB (input) INTEGER
* The leading dimension of B. LDB >= max(1,N).
*
* ALPHAR (output) DOUBLE PRECISION array, dimension (N)
* ALPHAI (output) DOUBLE PRECISION array, dimension (N)
* BETA (output) DOUBLE PRECISION array, dimension (N)
* On exit, (ALPHAR(j) + ALPHAI(j)*i)/BETA(j), j=1,...,N, will
* be the generalized eigenvalues. If ALPHAI(j) is zero, then
* the j-th eigenvalue is real; if positive, then the j-th and
* (j+1)-st eigenvalues are a complex conjugate pair, with
* ALPHAI(j+1) negative.
*
* Note: the quotients ALPHAR(j)/BETA(j) and ALPHAI(j)/BETA(j)
* may easily over- or underflow, and BETA(j) may even be zero.
* Thus, the user should avoid naively computing the ratio
* alpha/beta. However, ALPHAR and ALPHAI will be always less
* than and usually comparable with norm(A) in magnitude, and
* BETA always less than and usually comparable with norm(B).
*
* VL (output) DOUBLE PRECISION array, dimension (LDVL,N)
* If JOBVL = 'V', the left eigenvectors u(j) are stored one
* after another in the columns of VL, in the same order as
* their eigenvalues. If the j-th eigenvalue is real, then
* u(j) = VL(:,j), the j-th column of VL. If the j-th and
* (j+1)-th eigenvalues form a complex conjugate pair, then
* u(j) = VL(:,j)+i*VL(:,j+1) and u(j+1) = VL(:,j)-i*VL(:,j+1).
* Each eigenvector is scaled so the largest component has
* abs(real part)+abs(imag. part)=1.
* Not referenced if JOBVL = 'N'.
*
* LDVL (input) INTEGER
* The leading dimension of the matrix VL. LDVL >= 1, and
* if JOBVL = 'V', LDVL >= N.
*
* VR (output) DOUBLE PRECISION array, dimension (LDVR,N)
* If JOBVR = 'V', the right eigenvectors v(j) are stored one
* after another in the columns of VR, in the same order as
* their eigenvalues. If the j-th eigenvalue is real, then
* v(j) = VR(:,j), the j-th column of VR. If the j-th and
* (j+1)-th eigenvalues form a complex conjugate pair, then
* v(j) = VR(:,j)+i*VR(:,j+1) and v(j+1) = VR(:,j)-i*VR(:,j+1).
* Each eigenvector is scaled so the largest component has
* abs(real part)+abs(imag. part)=1.
* Not referenced if JOBVR = 'N'.
*
* LDVR (input) INTEGER
* The leading dimension of the matrix VR. LDVR >= 1, and
* if JOBVR = 'V', LDVR >= N.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,8*N).
* For good performance, LWORK must generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1,...,N:
* The QZ iteration failed. No eigenvectors have been
* calculated, but ALPHAR(j), ALPHAI(j), and BETA(j)
* should be correct for j=INFO+1,...,N.
* > N: =N+1: other than QZ iteration failed in DHGEQZ.
* =N+2: error return from DTGEVC.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobvl
* @param jobvr
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param alphar
* @param alphai
* @param beta
* @param vl
* @param ldvl
* @param vr
* @param ldvr
* @param work
* @param lwork
* @param info
*
*/
abstract public void dggev(java.lang.String jobvl, java.lang.String jobvr, int n, double[] a, int lda, double[] b, int ldb, double[] alphar, double[] alphai, double[] beta, double[] vl, int ldvl, double[] vr, int ldvr, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGGEV computes for a pair of N-by-N real nonsymmetric matrices (A,B)
* the generalized eigenvalues, and optionally, the left and/or right
* generalized eigenvectors.
*
* A generalized eigenvalue for a pair of matrices (A,B) is a scalar
* lambda or a ratio alpha/beta = lambda, such that A - lambda*B is
* singular. It is usually represented as the pair (alpha,beta), as
* there is a reasonable interpretation for beta=0, and even for both
* being zero.
*
* The right eigenvector v(j) corresponding to the eigenvalue lambda(j)
* of (A,B) satisfies
*
* A * v(j) = lambda(j) * B * v(j).
*
* The left eigenvector u(j) corresponding to the eigenvalue lambda(j)
* of (A,B) satisfies
*
* u(j)**H * A = lambda(j) * u(j)**H * B .
*
* where u(j)**H is the conjugate-transpose of u(j).
*
*
* Arguments
* =========
*
* JOBVL (input) CHARACTER*1
* = 'N': do not compute the left generalized eigenvectors;
* = 'V': compute the left generalized eigenvectors.
*
* JOBVR (input) CHARACTER*1
* = 'N': do not compute the right generalized eigenvectors;
* = 'V': compute the right generalized eigenvectors.
*
* N (input) INTEGER
* The order of the matrices A, B, VL, and VR. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the matrix A in the pair (A,B).
* On exit, A has been overwritten.
*
* LDA (input) INTEGER
* The leading dimension of A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB, N)
* On entry, the matrix B in the pair (A,B).
* On exit, B has been overwritten.
*
* LDB (input) INTEGER
* The leading dimension of B. LDB >= max(1,N).
*
* ALPHAR (output) DOUBLE PRECISION array, dimension (N)
* ALPHAI (output) DOUBLE PRECISION array, dimension (N)
* BETA (output) DOUBLE PRECISION array, dimension (N)
* On exit, (ALPHAR(j) + ALPHAI(j)*i)/BETA(j), j=1,...,N, will
* be the generalized eigenvalues. If ALPHAI(j) is zero, then
* the j-th eigenvalue is real; if positive, then the j-th and
* (j+1)-st eigenvalues are a complex conjugate pair, with
* ALPHAI(j+1) negative.
*
* Note: the quotients ALPHAR(j)/BETA(j) and ALPHAI(j)/BETA(j)
* may easily over- or underflow, and BETA(j) may even be zero.
* Thus, the user should avoid naively computing the ratio
* alpha/beta. However, ALPHAR and ALPHAI will be always less
* than and usually comparable with norm(A) in magnitude, and
* BETA always less than and usually comparable with norm(B).
*
* VL (output) DOUBLE PRECISION array, dimension (LDVL,N)
* If JOBVL = 'V', the left eigenvectors u(j) are stored one
* after another in the columns of VL, in the same order as
* their eigenvalues. If the j-th eigenvalue is real, then
* u(j) = VL(:,j), the j-th column of VL. If the j-th and
* (j+1)-th eigenvalues form a complex conjugate pair, then
* u(j) = VL(:,j)+i*VL(:,j+1) and u(j+1) = VL(:,j)-i*VL(:,j+1).
* Each eigenvector is scaled so the largest component has
* abs(real part)+abs(imag. part)=1.
* Not referenced if JOBVL = 'N'.
*
* LDVL (input) INTEGER
* The leading dimension of the matrix VL. LDVL >= 1, and
* if JOBVL = 'V', LDVL >= N.
*
* VR (output) DOUBLE PRECISION array, dimension (LDVR,N)
* If JOBVR = 'V', the right eigenvectors v(j) are stored one
* after another in the columns of VR, in the same order as
* their eigenvalues. If the j-th eigenvalue is real, then
* v(j) = VR(:,j), the j-th column of VR. If the j-th and
* (j+1)-th eigenvalues form a complex conjugate pair, then
* v(j) = VR(:,j)+i*VR(:,j+1) and v(j+1) = VR(:,j)-i*VR(:,j+1).
* Each eigenvector is scaled so the largest component has
* abs(real part)+abs(imag. part)=1.
* Not referenced if JOBVR = 'N'.
*
* LDVR (input) INTEGER
* The leading dimension of the matrix VR. LDVR >= 1, and
* if JOBVR = 'V', LDVR >= N.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,8*N).
* For good performance, LWORK must generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1,...,N:
* The QZ iteration failed. No eigenvectors have been
* calculated, but ALPHAR(j), ALPHAI(j), and BETA(j)
* should be correct for j=INFO+1,...,N.
* > N: =N+1: other than QZ iteration failed in DHGEQZ.
* =N+2: error return from DTGEVC.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobvl
* @param jobvr
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param alphar
* @param _alphar_offset
* @param alphai
* @param _alphai_offset
* @param beta
* @param _beta_offset
* @param vl
* @param _vl_offset
* @param ldvl
* @param vr
* @param _vr_offset
* @param ldvr
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dggev(java.lang.String jobvl, java.lang.String jobvr, int n, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, double[] alphar, int _alphar_offset, double[] alphai, int _alphai_offset, double[] beta, int _beta_offset, double[] vl, int _vl_offset, int ldvl, double[] vr, int _vr_offset, int ldvr, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGGEVX computes for a pair of N-by-N real nonsymmetric matrices (A,B)
* the generalized eigenvalues, and optionally, the left and/or right
* generalized eigenvectors.
*
* Optionally also, it computes a balancing transformation to improve
* the conditioning of the eigenvalues and eigenvectors (ILO, IHI,
* LSCALE, RSCALE, ABNRM, and BBNRM), reciprocal condition numbers for
* the eigenvalues (RCONDE), and reciprocal condition numbers for the
* right eigenvectors (RCONDV).
*
* A generalized eigenvalue for a pair of matrices (A,B) is a scalar
* lambda or a ratio alpha/beta = lambda, such that A - lambda*B is
* singular. It is usually represented as the pair (alpha,beta), as
* there is a reasonable interpretation for beta=0, and even for both
* being zero.
*
* The right eigenvector v(j) corresponding to the eigenvalue lambda(j)
* of (A,B) satisfies
*
* A * v(j) = lambda(j) * B * v(j) .
*
* The left eigenvector u(j) corresponding to the eigenvalue lambda(j)
* of (A,B) satisfies
*
* u(j)**H * A = lambda(j) * u(j)**H * B.
*
* where u(j)**H is the conjugate-transpose of u(j).
*
*
* Arguments
* =========
*
* BALANC (input) CHARACTER*1
* Specifies the balance option to be performed.
* = 'N': do not diagonally scale or permute;
* = 'P': permute only;
* = 'S': scale only;
* = 'B': both permute and scale.
* Computed reciprocal condition numbers will be for the
* matrices after permuting and/or balancing. Permuting does
* not change condition numbers (in exact arithmetic), but
* balancing does.
*
* JOBVL (input) CHARACTER*1
* = 'N': do not compute the left generalized eigenvectors;
* = 'V': compute the left generalized eigenvectors.
*
* JOBVR (input) CHARACTER*1
* = 'N': do not compute the right generalized eigenvectors;
* = 'V': compute the right generalized eigenvectors.
*
* SENSE (input) CHARACTER*1
* Determines which reciprocal condition numbers are computed.
* = 'N': none are computed;
* = 'E': computed for eigenvalues only;
* = 'V': computed for eigenvectors only;
* = 'B': computed for eigenvalues and eigenvectors.
*
* N (input) INTEGER
* The order of the matrices A, B, VL, and VR. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the matrix A in the pair (A,B).
* On exit, A has been overwritten. If JOBVL='V' or JOBVR='V'
* or both, then A contains the first part of the real Schur
* form of the "balanced" versions of the input A and B.
*
* LDA (input) INTEGER
* The leading dimension of A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB, N)
* On entry, the matrix B in the pair (A,B).
* On exit, B has been overwritten. If JOBVL='V' or JOBVR='V'
* or both, then B contains the second part of the real Schur
* form of the "balanced" versions of the input A and B.
*
* LDB (input) INTEGER
* The leading dimension of B. LDB >= max(1,N).
*
* ALPHAR (output) DOUBLE PRECISION array, dimension (N)
* ALPHAI (output) DOUBLE PRECISION array, dimension (N)
* BETA (output) DOUBLE PRECISION array, dimension (N)
* On exit, (ALPHAR(j) + ALPHAI(j)*i)/BETA(j), j=1,...,N, will
* be the generalized eigenvalues. If ALPHAI(j) is zero, then
* the j-th eigenvalue is real; if positive, then the j-th and
* (j+1)-st eigenvalues are a complex conjugate pair, with
* ALPHAI(j+1) negative.
*
* Note: the quotients ALPHAR(j)/BETA(j) and ALPHAI(j)/BETA(j)
* may easily over- or underflow, and BETA(j) may even be zero.
* Thus, the user should avoid naively computing the ratio
* ALPHA/BETA. However, ALPHAR and ALPHAI will be always less
* than and usually comparable with norm(A) in magnitude, and
* BETA always less than and usually comparable with norm(B).
*
* VL (output) DOUBLE PRECISION array, dimension (LDVL,N)
* If JOBVL = 'V', the left eigenvectors u(j) are stored one
* after another in the columns of VL, in the same order as
* their eigenvalues. If the j-th eigenvalue is real, then
* u(j) = VL(:,j), the j-th column of VL. If the j-th and
* (j+1)-th eigenvalues form a complex conjugate pair, then
* u(j) = VL(:,j)+i*VL(:,j+1) and u(j+1) = VL(:,j)-i*VL(:,j+1).
* Each eigenvector will be scaled so the largest component have
* abs(real part) + abs(imag. part) = 1.
* Not referenced if JOBVL = 'N'.
*
* LDVL (input) INTEGER
* The leading dimension of the matrix VL. LDVL >= 1, and
* if JOBVL = 'V', LDVL >= N.
*
* VR (output) DOUBLE PRECISION array, dimension (LDVR,N)
* If JOBVR = 'V', the right eigenvectors v(j) are stored one
* after another in the columns of VR, in the same order as
* their eigenvalues. If the j-th eigenvalue is real, then
* v(j) = VR(:,j), the j-th column of VR. If the j-th and
* (j+1)-th eigenvalues form a complex conjugate pair, then
* v(j) = VR(:,j)+i*VR(:,j+1) and v(j+1) = VR(:,j)-i*VR(:,j+1).
* Each eigenvector will be scaled so the largest component have
* abs(real part) + abs(imag. part) = 1.
* Not referenced if JOBVR = 'N'.
*
* LDVR (input) INTEGER
* The leading dimension of the matrix VR. LDVR >= 1, and
* if JOBVR = 'V', LDVR >= N.
*
* ILO (output) INTEGER
* IHI (output) INTEGER
* ILO and IHI are integer values such that on exit
* A(i,j) = 0 and B(i,j) = 0 if i > j and
* j = 1,...,ILO-1 or i = IHI+1,...,N.
* If BALANC = 'N' or 'S', ILO = 1 and IHI = N.
*
* LSCALE (output) DOUBLE PRECISION array, dimension (N)
* Details of the permutations and scaling factors applied
* to the left side of A and B. If PL(j) is the index of the
* row interchanged with row j, and DL(j) is the scaling
* factor applied to row j, then
* LSCALE(j) = PL(j) for j = 1,...,ILO-1
* = DL(j) for j = ILO,...,IHI
* = PL(j) for j = IHI+1,...,N.
* The order in which the interchanges are made is N to IHI+1,
* then 1 to ILO-1.
*
* RSCALE (output) DOUBLE PRECISION array, dimension (N)
* Details of the permutations and scaling factors applied
* to the right side of A and B. If PR(j) is the index of the
* column interchanged with column j, and DR(j) is the scaling
* factor applied to column j, then
* RSCALE(j) = PR(j) for j = 1,...,ILO-1
* = DR(j) for j = ILO,...,IHI
* = PR(j) for j = IHI+1,...,N
* The order in which the interchanges are made is N to IHI+1,
* then 1 to ILO-1.
*
* ABNRM (output) DOUBLE PRECISION
* The one-norm of the balanced matrix A.
*
* BBNRM (output) DOUBLE PRECISION
* The one-norm of the balanced matrix B.
*
* RCONDE (output) DOUBLE PRECISION array, dimension (N)
* If SENSE = 'E' or 'B', the reciprocal condition numbers of
* the eigenvalues, stored in consecutive elements of the array.
* For a complex conjugate pair of eigenvalues two consecutive
* elements of RCONDE are set to the same value. Thus RCONDE(j),
* RCONDV(j), and the j-th columns of VL and VR all correspond
* to the j-th eigenpair.
* If SENSE = 'N or 'V', RCONDE is not referenced.
*
* RCONDV (output) DOUBLE PRECISION array, dimension (N)
* If SENSE = 'V' or 'B', the estimated reciprocal condition
* numbers of the eigenvectors, stored in consecutive elements
* of the array. For a complex eigenvector two consecutive
* elements of RCONDV are set to the same value. If the
* eigenvalues cannot be reordered to compute RCONDV(j),
* RCONDV(j) is set to 0; this can only occur when the true
* value would be very small anyway.
* If SENSE = 'N' or 'E', RCONDV is not referenced.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,2*N).
* If BALANC = 'S' or 'B', or JOBVL = 'V', or JOBVR = 'V',
* LWORK >= max(1,6*N).
* If SENSE = 'E' or 'B', LWORK >= max(1,10*N).
* If SENSE = 'V' or 'B', LWORK >= 2*N*N+8*N+16.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (N+6)
* If SENSE = 'E', IWORK is not referenced.
*
* BWORK (workspace) LOGICAL array, dimension (N)
* If SENSE = 'N', BWORK is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1,...,N:
* The QZ iteration failed. No eigenvectors have been
* calculated, but ALPHAR(j), ALPHAI(j), and BETA(j)
* should be correct for j=INFO+1,...,N.
* > N: =N+1: other than QZ iteration failed in DHGEQZ.
* =N+2: error return from DTGEVC.
*
* Further Details
* ===============
*
* Balancing a matrix pair (A,B) includes, first, permuting rows and
* columns to isolate eigenvalues, second, applying diagonal similarity
* transformation to the rows and columns to make the rows and columns
* as close in norm as possible. The computed reciprocal condition
* numbers correspond to the balanced matrix. Permuting rows and columns
* will not change the condition numbers (in exact arithmetic) but
* diagonal scaling will. For further explanation of balancing, see
* section 4.11.1.2 of LAPACK Users' Guide.
*
* An approximate error bound on the chordal distance between the i-th
* computed generalized eigenvalue w and the corresponding exact
* eigenvalue lambda is
*
* chord(w, lambda) <= EPS * norm(ABNRM, BBNRM) / RCONDE(I)
*
* An approximate error bound for the angle between the i-th computed
* eigenvector VL(i) or VR(i) is given by
*
* EPS * norm(ABNRM, BBNRM) / DIF(i).
*
* For further explanation of the reciprocal condition numbers RCONDE
* and RCONDV, see section 4.11 of LAPACK User's Guide.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param balanc
* @param jobvl
* @param jobvr
* @param sense
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param alphar
* @param alphai
* @param beta
* @param vl
* @param ldvl
* @param vr
* @param ldvr
* @param ilo
* @param ihi
* @param lscale
* @param rscale
* @param abnrm
* @param bbnrm
* @param rconde
* @param rcondv
* @param work
* @param lwork
* @param iwork
* @param bwork
* @param info
*
*/
abstract public void dggevx(java.lang.String balanc, java.lang.String jobvl, java.lang.String jobvr, java.lang.String sense, int n, double[] a, int lda, double[] b, int ldb, double[] alphar, double[] alphai, double[] beta, double[] vl, int ldvl, double[] vr, int ldvr, org.netlib.util.intW ilo, org.netlib.util.intW ihi, double[] lscale, double[] rscale, org.netlib.util.doubleW abnrm, org.netlib.util.doubleW bbnrm, double[] rconde, double[] rcondv, double[] work, int lwork, int[] iwork, boolean[] bwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGGEVX computes for a pair of N-by-N real nonsymmetric matrices (A,B)
* the generalized eigenvalues, and optionally, the left and/or right
* generalized eigenvectors.
*
* Optionally also, it computes a balancing transformation to improve
* the conditioning of the eigenvalues and eigenvectors (ILO, IHI,
* LSCALE, RSCALE, ABNRM, and BBNRM), reciprocal condition numbers for
* the eigenvalues (RCONDE), and reciprocal condition numbers for the
* right eigenvectors (RCONDV).
*
* A generalized eigenvalue for a pair of matrices (A,B) is a scalar
* lambda or a ratio alpha/beta = lambda, such that A - lambda*B is
* singular. It is usually represented as the pair (alpha,beta), as
* there is a reasonable interpretation for beta=0, and even for both
* being zero.
*
* The right eigenvector v(j) corresponding to the eigenvalue lambda(j)
* of (A,B) satisfies
*
* A * v(j) = lambda(j) * B * v(j) .
*
* The left eigenvector u(j) corresponding to the eigenvalue lambda(j)
* of (A,B) satisfies
*
* u(j)**H * A = lambda(j) * u(j)**H * B.
*
* where u(j)**H is the conjugate-transpose of u(j).
*
*
* Arguments
* =========
*
* BALANC (input) CHARACTER*1
* Specifies the balance option to be performed.
* = 'N': do not diagonally scale or permute;
* = 'P': permute only;
* = 'S': scale only;
* = 'B': both permute and scale.
* Computed reciprocal condition numbers will be for the
* matrices after permuting and/or balancing. Permuting does
* not change condition numbers (in exact arithmetic), but
* balancing does.
*
* JOBVL (input) CHARACTER*1
* = 'N': do not compute the left generalized eigenvectors;
* = 'V': compute the left generalized eigenvectors.
*
* JOBVR (input) CHARACTER*1
* = 'N': do not compute the right generalized eigenvectors;
* = 'V': compute the right generalized eigenvectors.
*
* SENSE (input) CHARACTER*1
* Determines which reciprocal condition numbers are computed.
* = 'N': none are computed;
* = 'E': computed for eigenvalues only;
* = 'V': computed for eigenvectors only;
* = 'B': computed for eigenvalues and eigenvectors.
*
* N (input) INTEGER
* The order of the matrices A, B, VL, and VR. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the matrix A in the pair (A,B).
* On exit, A has been overwritten. If JOBVL='V' or JOBVR='V'
* or both, then A contains the first part of the real Schur
* form of the "balanced" versions of the input A and B.
*
* LDA (input) INTEGER
* The leading dimension of A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB, N)
* On entry, the matrix B in the pair (A,B).
* On exit, B has been overwritten. If JOBVL='V' or JOBVR='V'
* or both, then B contains the second part of the real Schur
* form of the "balanced" versions of the input A and B.
*
* LDB (input) INTEGER
* The leading dimension of B. LDB >= max(1,N).
*
* ALPHAR (output) DOUBLE PRECISION array, dimension (N)
* ALPHAI (output) DOUBLE PRECISION array, dimension (N)
* BETA (output) DOUBLE PRECISION array, dimension (N)
* On exit, (ALPHAR(j) + ALPHAI(j)*i)/BETA(j), j=1,...,N, will
* be the generalized eigenvalues. If ALPHAI(j) is zero, then
* the j-th eigenvalue is real; if positive, then the j-th and
* (j+1)-st eigenvalues are a complex conjugate pair, with
* ALPHAI(j+1) negative.
*
* Note: the quotients ALPHAR(j)/BETA(j) and ALPHAI(j)/BETA(j)
* may easily over- or underflow, and BETA(j) may even be zero.
* Thus, the user should avoid naively computing the ratio
* ALPHA/BETA. However, ALPHAR and ALPHAI will be always less
* than and usually comparable with norm(A) in magnitude, and
* BETA always less than and usually comparable with norm(B).
*
* VL (output) DOUBLE PRECISION array, dimension (LDVL,N)
* If JOBVL = 'V', the left eigenvectors u(j) are stored one
* after another in the columns of VL, in the same order as
* their eigenvalues. If the j-th eigenvalue is real, then
* u(j) = VL(:,j), the j-th column of VL. If the j-th and
* (j+1)-th eigenvalues form a complex conjugate pair, then
* u(j) = VL(:,j)+i*VL(:,j+1) and u(j+1) = VL(:,j)-i*VL(:,j+1).
* Each eigenvector will be scaled so the largest component have
* abs(real part) + abs(imag. part) = 1.
* Not referenced if JOBVL = 'N'.
*
* LDVL (input) INTEGER
* The leading dimension of the matrix VL. LDVL >= 1, and
* if JOBVL = 'V', LDVL >= N.
*
* VR (output) DOUBLE PRECISION array, dimension (LDVR,N)
* If JOBVR = 'V', the right eigenvectors v(j) are stored one
* after another in the columns of VR, in the same order as
* their eigenvalues. If the j-th eigenvalue is real, then
* v(j) = VR(:,j), the j-th column of VR. If the j-th and
* (j+1)-th eigenvalues form a complex conjugate pair, then
* v(j) = VR(:,j)+i*VR(:,j+1) and v(j+1) = VR(:,j)-i*VR(:,j+1).
* Each eigenvector will be scaled so the largest component have
* abs(real part) + abs(imag. part) = 1.
* Not referenced if JOBVR = 'N'.
*
* LDVR (input) INTEGER
* The leading dimension of the matrix VR. LDVR >= 1, and
* if JOBVR = 'V', LDVR >= N.
*
* ILO (output) INTEGER
* IHI (output) INTEGER
* ILO and IHI are integer values such that on exit
* A(i,j) = 0 and B(i,j) = 0 if i > j and
* j = 1,...,ILO-1 or i = IHI+1,...,N.
* If BALANC = 'N' or 'S', ILO = 1 and IHI = N.
*
* LSCALE (output) DOUBLE PRECISION array, dimension (N)
* Details of the permutations and scaling factors applied
* to the left side of A and B. If PL(j) is the index of the
* row interchanged with row j, and DL(j) is the scaling
* factor applied to row j, then
* LSCALE(j) = PL(j) for j = 1,...,ILO-1
* = DL(j) for j = ILO,...,IHI
* = PL(j) for j = IHI+1,...,N.
* The order in which the interchanges are made is N to IHI+1,
* then 1 to ILO-1.
*
* RSCALE (output) DOUBLE PRECISION array, dimension (N)
* Details of the permutations and scaling factors applied
* to the right side of A and B. If PR(j) is the index of the
* column interchanged with column j, and DR(j) is the scaling
* factor applied to column j, then
* RSCALE(j) = PR(j) for j = 1,...,ILO-1
* = DR(j) for j = ILO,...,IHI
* = PR(j) for j = IHI+1,...,N
* The order in which the interchanges are made is N to IHI+1,
* then 1 to ILO-1.
*
* ABNRM (output) DOUBLE PRECISION
* The one-norm of the balanced matrix A.
*
* BBNRM (output) DOUBLE PRECISION
* The one-norm of the balanced matrix B.
*
* RCONDE (output) DOUBLE PRECISION array, dimension (N)
* If SENSE = 'E' or 'B', the reciprocal condition numbers of
* the eigenvalues, stored in consecutive elements of the array.
* For a complex conjugate pair of eigenvalues two consecutive
* elements of RCONDE are set to the same value. Thus RCONDE(j),
* RCONDV(j), and the j-th columns of VL and VR all correspond
* to the j-th eigenpair.
* If SENSE = 'N or 'V', RCONDE is not referenced.
*
* RCONDV (output) DOUBLE PRECISION array, dimension (N)
* If SENSE = 'V' or 'B', the estimated reciprocal condition
* numbers of the eigenvectors, stored in consecutive elements
* of the array. For a complex eigenvector two consecutive
* elements of RCONDV are set to the same value. If the
* eigenvalues cannot be reordered to compute RCONDV(j),
* RCONDV(j) is set to 0; this can only occur when the true
* value would be very small anyway.
* If SENSE = 'N' or 'E', RCONDV is not referenced.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,2*N).
* If BALANC = 'S' or 'B', or JOBVL = 'V', or JOBVR = 'V',
* LWORK >= max(1,6*N).
* If SENSE = 'E' or 'B', LWORK >= max(1,10*N).
* If SENSE = 'V' or 'B', LWORK >= 2*N*N+8*N+16.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (N+6)
* If SENSE = 'E', IWORK is not referenced.
*
* BWORK (workspace) LOGICAL array, dimension (N)
* If SENSE = 'N', BWORK is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1,...,N:
* The QZ iteration failed. No eigenvectors have been
* calculated, but ALPHAR(j), ALPHAI(j), and BETA(j)
* should be correct for j=INFO+1,...,N.
* > N: =N+1: other than QZ iteration failed in DHGEQZ.
* =N+2: error return from DTGEVC.
*
* Further Details
* ===============
*
* Balancing a matrix pair (A,B) includes, first, permuting rows and
* columns to isolate eigenvalues, second, applying diagonal similarity
* transformation to the rows and columns to make the rows and columns
* as close in norm as possible. The computed reciprocal condition
* numbers correspond to the balanced matrix. Permuting rows and columns
* will not change the condition numbers (in exact arithmetic) but
* diagonal scaling will. For further explanation of balancing, see
* section 4.11.1.2 of LAPACK Users' Guide.
*
* An approximate error bound on the chordal distance between the i-th
* computed generalized eigenvalue w and the corresponding exact
* eigenvalue lambda is
*
* chord(w, lambda) <= EPS * norm(ABNRM, BBNRM) / RCONDE(I)
*
* An approximate error bound for the angle between the i-th computed
* eigenvector VL(i) or VR(i) is given by
*
* EPS * norm(ABNRM, BBNRM) / DIF(i).
*
* For further explanation of the reciprocal condition numbers RCONDE
* and RCONDV, see section 4.11 of LAPACK User's Guide.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param balanc
* @param jobvl
* @param jobvr
* @param sense
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param alphar
* @param _alphar_offset
* @param alphai
* @param _alphai_offset
* @param beta
* @param _beta_offset
* @param vl
* @param _vl_offset
* @param ldvl
* @param vr
* @param _vr_offset
* @param ldvr
* @param ilo
* @param ihi
* @param lscale
* @param _lscale_offset
* @param rscale
* @param _rscale_offset
* @param abnrm
* @param bbnrm
* @param rconde
* @param _rconde_offset
* @param rcondv
* @param _rcondv_offset
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param bwork
* @param _bwork_offset
* @param info
*
*/
abstract public void dggevx(java.lang.String balanc, java.lang.String jobvl, java.lang.String jobvr, java.lang.String sense, int n, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, double[] alphar, int _alphar_offset, double[] alphai, int _alphai_offset, double[] beta, int _beta_offset, double[] vl, int _vl_offset, int ldvl, double[] vr, int _vr_offset, int ldvr, org.netlib.util.intW ilo, org.netlib.util.intW ihi, double[] lscale, int _lscale_offset, double[] rscale, int _rscale_offset, org.netlib.util.doubleW abnrm, org.netlib.util.doubleW bbnrm, double[] rconde, int _rconde_offset, double[] rcondv, int _rcondv_offset, double[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, boolean[] bwork, int _bwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGGGLM solves a general Gauss-Markov linear model (GLM) problem:
*
* minimize || y ||_2 subject to d = A*x + B*y
* x
*
* where A is an N-by-M matrix, B is an N-by-P matrix, and d is a
* given N-vector. It is assumed that M <= N <= M+P, and
*
* rank(A) = M and rank( A B ) = N.
*
* Under these assumptions, the constrained equation is always
* consistent, and there is a unique solution x and a minimal 2-norm
* solution y, which is obtained using a generalized QR factorization
* of the matrices (A, B) given by
*
* A = Q*(R), B = Q*T*Z.
* (0)
*
* In particular, if matrix B is square nonsingular, then the problem
* GLM is equivalent to the following weighted linear least squares
* problem
*
* minimize || inv(B)*(d-A*x) ||_2
* x
*
* where inv(B) denotes the inverse of B.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of rows of the matrices A and B. N >= 0.
*
* M (input) INTEGER
* The number of columns of the matrix A. 0 <= M <= N.
*
* P (input) INTEGER
* The number of columns of the matrix B. P >= N-M.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,M)
* On entry, the N-by-M matrix A.
* On exit, the upper triangular part of the array A contains
* the M-by-M upper triangular matrix R.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,P)
* On entry, the N-by-P matrix B.
* On exit, if N <= P, the upper triangle of the subarray
* B(1:N,P-N+1:P) contains the N-by-N upper triangular matrix T;
* if N > P, the elements on and above the (N-P)th subdiagonal
* contain the N-by-P upper trapezoidal matrix T.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, D is the left hand side of the GLM equation.
* On exit, D is destroyed.
*
* X (output) DOUBLE PRECISION array, dimension (M)
* Y (output) DOUBLE PRECISION array, dimension (P)
* On exit, X and Y are the solutions of the GLM problem.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N+M+P).
* For optimum performance, LWORK >= M+min(N,P)+max(N,P)*NB,
* where NB is an upper bound for the optimal blocksizes for
* DGEQRF, SGERQF, DORMQR and SORMRQ.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1: the upper triangular factor R associated with A in the
* generalized QR factorization of the pair (A, B) is
* singular, so that rank(A) < M; the least squares
* solution could not be computed.
* = 2: the bottom (N-M) by (N-M) part of the upper trapezoidal
* factor T associated with B in the generalized QR
* factorization of the pair (A, B) is singular, so that
* rank( A B ) < N; the least squares solution could not
* be computed.
*
* ===================================================================
*
* .. Parameters ..
*
*
* @param n
* @param m
* @param p
* @param a
* @param lda
* @param b
* @param ldb
* @param d
* @param x
* @param y
* @param work
* @param lwork
* @param info
*
*/
abstract public void dggglm(int n, int m, int p, double[] a, int lda, double[] b, int ldb, double[] d, double[] x, double[] y, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGGGLM solves a general Gauss-Markov linear model (GLM) problem:
*
* minimize || y ||_2 subject to d = A*x + B*y
* x
*
* where A is an N-by-M matrix, B is an N-by-P matrix, and d is a
* given N-vector. It is assumed that M <= N <= M+P, and
*
* rank(A) = M and rank( A B ) = N.
*
* Under these assumptions, the constrained equation is always
* consistent, and there is a unique solution x and a minimal 2-norm
* solution y, which is obtained using a generalized QR factorization
* of the matrices (A, B) given by
*
* A = Q*(R), B = Q*T*Z.
* (0)
*
* In particular, if matrix B is square nonsingular, then the problem
* GLM is equivalent to the following weighted linear least squares
* problem
*
* minimize || inv(B)*(d-A*x) ||_2
* x
*
* where inv(B) denotes the inverse of B.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of rows of the matrices A and B. N >= 0.
*
* M (input) INTEGER
* The number of columns of the matrix A. 0 <= M <= N.
*
* P (input) INTEGER
* The number of columns of the matrix B. P >= N-M.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,M)
* On entry, the N-by-M matrix A.
* On exit, the upper triangular part of the array A contains
* the M-by-M upper triangular matrix R.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,P)
* On entry, the N-by-P matrix B.
* On exit, if N <= P, the upper triangle of the subarray
* B(1:N,P-N+1:P) contains the N-by-N upper triangular matrix T;
* if N > P, the elements on and above the (N-P)th subdiagonal
* contain the N-by-P upper trapezoidal matrix T.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, D is the left hand side of the GLM equation.
* On exit, D is destroyed.
*
* X (output) DOUBLE PRECISION array, dimension (M)
* Y (output) DOUBLE PRECISION array, dimension (P)
* On exit, X and Y are the solutions of the GLM problem.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N+M+P).
* For optimum performance, LWORK >= M+min(N,P)+max(N,P)*NB,
* where NB is an upper bound for the optimal blocksizes for
* DGEQRF, SGERQF, DORMQR and SORMRQ.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1: the upper triangular factor R associated with A in the
* generalized QR factorization of the pair (A, B) is
* singular, so that rank(A) < M; the least squares
* solution could not be computed.
* = 2: the bottom (N-M) by (N-M) part of the upper trapezoidal
* factor T associated with B in the generalized QR
* factorization of the pair (A, B) is singular, so that
* rank( A B ) < N; the least squares solution could not
* be computed.
*
* ===================================================================
*
* .. Parameters ..
*
*
* @param n
* @param m
* @param p
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param d
* @param _d_offset
* @param x
* @param _x_offset
* @param y
* @param _y_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dggglm(int n, int m, int p, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, double[] d, int _d_offset, double[] x, int _x_offset, double[] y, int _y_offset, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGGHRD reduces a pair of real matrices (A,B) to generalized upper
* Hessenberg form using orthogonal transformations, where A is a
* general matrix and B is upper triangular. The form of the
* generalized eigenvalue problem is
* A*x = lambda*B*x,
* and B is typically made upper triangular by computing its QR
* factorization and moving the orthogonal matrix Q to the left side
* of the equation.
*
* This subroutine simultaneously reduces A to a Hessenberg matrix H:
* Q**T*A*Z = H
* and transforms B to another upper triangular matrix T:
* Q**T*B*Z = T
* in order to reduce the problem to its standard form
* H*y = lambda*T*y
* where y = Z**T*x.
*
* The orthogonal matrices Q and Z are determined as products of Givens
* rotations. They may either be formed explicitly, or they may be
* postmultiplied into input matrices Q1 and Z1, so that
*
* Q1 * A * Z1**T = (Q1*Q) * H * (Z1*Z)**T
*
* Q1 * B * Z1**T = (Q1*Q) * T * (Z1*Z)**T
*
* If Q1 is the orthogonal matrix from the QR factorization of B in the
* original equation A*x = lambda*B*x, then DGGHRD reduces the original
* problem to generalized Hessenberg form.
*
* Arguments
* =========
*
* COMPQ (input) CHARACTER*1
* = 'N': do not compute Q;
* = 'I': Q is initialized to the unit matrix, and the
* orthogonal matrix Q is returned;
* = 'V': Q must contain an orthogonal matrix Q1 on entry,
* and the product Q1*Q is returned.
*
* COMPZ (input) CHARACTER*1
* = 'N': do not compute Z;
* = 'I': Z is initialized to the unit matrix, and the
* orthogonal matrix Z is returned;
* = 'V': Z must contain an orthogonal matrix Z1 on entry,
* and the product Z1*Z is returned.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* ILO and IHI mark the rows and columns of A which are to be
* reduced. It is assumed that A is already upper triangular
* in rows and columns 1:ILO-1 and IHI+1:N. ILO and IHI are
* normally set by a previous call to SGGBAL; otherwise they
* should be set to 1 and N respectively.
* 1 <= ILO <= IHI <= N, if N > 0; ILO=1 and IHI=0, if N=0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the N-by-N general matrix to be reduced.
* On exit, the upper triangle and the first subdiagonal of A
* are overwritten with the upper Hessenberg matrix H, and the
* rest is set to zero.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB, N)
* On entry, the N-by-N upper triangular matrix B.
* On exit, the upper triangular matrix T = Q**T B Z. The
* elements below the diagonal are set to zero.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* Q (input/output) DOUBLE PRECISION array, dimension (LDQ, N)
* On entry, if COMPQ = 'V', the orthogonal matrix Q1,
* typically from the QR factorization of B.
* On exit, if COMPQ='I', the orthogonal matrix Q, and if
* COMPQ = 'V', the product Q1*Q.
* Not referenced if COMPQ='N'.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q.
* LDQ >= N if COMPQ='V' or 'I'; LDQ >= 1 otherwise.
*
* Z (input/output) DOUBLE PRECISION array, dimension (LDZ, N)
* On entry, if COMPZ = 'V', the orthogonal matrix Z1.
* On exit, if COMPZ='I', the orthogonal matrix Z, and if
* COMPZ = 'V', the product Z1*Z.
* Not referenced if COMPZ='N'.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z.
* LDZ >= N if COMPZ='V' or 'I'; LDZ >= 1 otherwise.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* This routine reduces A to Hessenberg and B to triangular form by
* an unblocked reduction, as described in _Matrix_Computations_,
* by Golub and Van Loan (Johns Hopkins Press.)
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param compq
* @param compz
* @param n
* @param ilo
* @param ihi
* @param a
* @param lda
* @param b
* @param ldb
* @param q
* @param ldq
* @param z
* @param ldz
* @param info
*
*/
abstract public void dgghrd(java.lang.String compq, java.lang.String compz, int n, int ilo, int ihi, double[] a, int lda, double[] b, int ldb, double[] q, int ldq, double[] z, int ldz, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGGHRD reduces a pair of real matrices (A,B) to generalized upper
* Hessenberg form using orthogonal transformations, where A is a
* general matrix and B is upper triangular. The form of the
* generalized eigenvalue problem is
* A*x = lambda*B*x,
* and B is typically made upper triangular by computing its QR
* factorization and moving the orthogonal matrix Q to the left side
* of the equation.
*
* This subroutine simultaneously reduces A to a Hessenberg matrix H:
* Q**T*A*Z = H
* and transforms B to another upper triangular matrix T:
* Q**T*B*Z = T
* in order to reduce the problem to its standard form
* H*y = lambda*T*y
* where y = Z**T*x.
*
* The orthogonal matrices Q and Z are determined as products of Givens
* rotations. They may either be formed explicitly, or they may be
* postmultiplied into input matrices Q1 and Z1, so that
*
* Q1 * A * Z1**T = (Q1*Q) * H * (Z1*Z)**T
*
* Q1 * B * Z1**T = (Q1*Q) * T * (Z1*Z)**T
*
* If Q1 is the orthogonal matrix from the QR factorization of B in the
* original equation A*x = lambda*B*x, then DGGHRD reduces the original
* problem to generalized Hessenberg form.
*
* Arguments
* =========
*
* COMPQ (input) CHARACTER*1
* = 'N': do not compute Q;
* = 'I': Q is initialized to the unit matrix, and the
* orthogonal matrix Q is returned;
* = 'V': Q must contain an orthogonal matrix Q1 on entry,
* and the product Q1*Q is returned.
*
* COMPZ (input) CHARACTER*1
* = 'N': do not compute Z;
* = 'I': Z is initialized to the unit matrix, and the
* orthogonal matrix Z is returned;
* = 'V': Z must contain an orthogonal matrix Z1 on entry,
* and the product Z1*Z is returned.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* ILO and IHI mark the rows and columns of A which are to be
* reduced. It is assumed that A is already upper triangular
* in rows and columns 1:ILO-1 and IHI+1:N. ILO and IHI are
* normally set by a previous call to SGGBAL; otherwise they
* should be set to 1 and N respectively.
* 1 <= ILO <= IHI <= N, if N > 0; ILO=1 and IHI=0, if N=0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the N-by-N general matrix to be reduced.
* On exit, the upper triangle and the first subdiagonal of A
* are overwritten with the upper Hessenberg matrix H, and the
* rest is set to zero.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB, N)
* On entry, the N-by-N upper triangular matrix B.
* On exit, the upper triangular matrix T = Q**T B Z. The
* elements below the diagonal are set to zero.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* Q (input/output) DOUBLE PRECISION array, dimension (LDQ, N)
* On entry, if COMPQ = 'V', the orthogonal matrix Q1,
* typically from the QR factorization of B.
* On exit, if COMPQ='I', the orthogonal matrix Q, and if
* COMPQ = 'V', the product Q1*Q.
* Not referenced if COMPQ='N'.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q.
* LDQ >= N if COMPQ='V' or 'I'; LDQ >= 1 otherwise.
*
* Z (input/output) DOUBLE PRECISION array, dimension (LDZ, N)
* On entry, if COMPZ = 'V', the orthogonal matrix Z1.
* On exit, if COMPZ='I', the orthogonal matrix Z, and if
* COMPZ = 'V', the product Z1*Z.
* Not referenced if COMPZ='N'.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z.
* LDZ >= N if COMPZ='V' or 'I'; LDZ >= 1 otherwise.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* This routine reduces A to Hessenberg and B to triangular form by
* an unblocked reduction, as described in _Matrix_Computations_,
* by Golub and Van Loan (Johns Hopkins Press.)
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param compq
* @param compz
* @param n
* @param ilo
* @param ihi
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param q
* @param _q_offset
* @param ldq
* @param z
* @param _z_offset
* @param ldz
* @param info
*
*/
abstract public void dgghrd(java.lang.String compq, java.lang.String compz, int n, int ilo, int ihi, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, double[] q, int _q_offset, int ldq, double[] z, int _z_offset, int ldz, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGGLSE solves the linear equality-constrained least squares (LSE)
* problem:
*
* minimize || c - A*x ||_2 subject to B*x = d
*
* where A is an M-by-N matrix, B is a P-by-N matrix, c is a given
* M-vector, and d is a given P-vector. It is assumed that
* P <= N <= M+P, and
*
* rank(B) = P and rank( (A) ) = N.
* ( (B) )
*
* These conditions ensure that the LSE problem has a unique solution,
* which is obtained using a generalized RQ factorization of the
* matrices (B, A) given by
*
* B = (0 R)*Q, A = Z*T*Q.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrices A and B. N >= 0.
*
* P (input) INTEGER
* The number of rows of the matrix B. 0 <= P <= N <= M+P.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, the elements on and above the diagonal of the array
* contain the min(M,N)-by-N upper trapezoidal matrix T.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,N)
* On entry, the P-by-N matrix B.
* On exit, the upper triangle of the subarray B(1:P,N-P+1:N)
* contains the P-by-P upper triangular matrix R.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,P).
*
* C (input/output) DOUBLE PRECISION array, dimension (M)
* On entry, C contains the right hand side vector for the
* least squares part of the LSE problem.
* On exit, the residual sum of squares for the solution
* is given by the sum of squares of elements N-P+1 to M of
* vector C.
*
* D (input/output) DOUBLE PRECISION array, dimension (P)
* On entry, D contains the right hand side vector for the
* constrained equation.
* On exit, D is destroyed.
*
* X (output) DOUBLE PRECISION array, dimension (N)
* On exit, X is the solution of the LSE problem.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,M+N+P).
* For optimum performance LWORK >= P+min(M,N)+max(M,N)*NB,
* where NB is an upper bound for the optimal blocksizes for
* DGEQRF, SGERQF, DORMQR and SORMRQ.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1: the upper triangular factor R associated with B in the
* generalized RQ factorization of the pair (B, A) is
* singular, so that rank(B) < P; the least squares
* solution could not be computed.
* = 2: the (N-P) by (N-P) part of the upper trapezoidal factor
* T associated with A in the generalized RQ factorization
* of the pair (B, A) is singular, so that
* rank( (A) ) < N; the least squares solution could not
* ( (B) )
* be computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param p
* @param a
* @param lda
* @param b
* @param ldb
* @param c
* @param d
* @param x
* @param work
* @param lwork
* @param info
*
*/
abstract public void dgglse(int m, int n, int p, double[] a, int lda, double[] b, int ldb, double[] c, double[] d, double[] x, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGGLSE solves the linear equality-constrained least squares (LSE)
* problem:
*
* minimize || c - A*x ||_2 subject to B*x = d
*
* where A is an M-by-N matrix, B is a P-by-N matrix, c is a given
* M-vector, and d is a given P-vector. It is assumed that
* P <= N <= M+P, and
*
* rank(B) = P and rank( (A) ) = N.
* ( (B) )
*
* These conditions ensure that the LSE problem has a unique solution,
* which is obtained using a generalized RQ factorization of the
* matrices (B, A) given by
*
* B = (0 R)*Q, A = Z*T*Q.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrices A and B. N >= 0.
*
* P (input) INTEGER
* The number of rows of the matrix B. 0 <= P <= N <= M+P.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, the elements on and above the diagonal of the array
* contain the min(M,N)-by-N upper trapezoidal matrix T.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,N)
* On entry, the P-by-N matrix B.
* On exit, the upper triangle of the subarray B(1:P,N-P+1:N)
* contains the P-by-P upper triangular matrix R.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,P).
*
* C (input/output) DOUBLE PRECISION array, dimension (M)
* On entry, C contains the right hand side vector for the
* least squares part of the LSE problem.
* On exit, the residual sum of squares for the solution
* is given by the sum of squares of elements N-P+1 to M of
* vector C.
*
* D (input/output) DOUBLE PRECISION array, dimension (P)
* On entry, D contains the right hand side vector for the
* constrained equation.
* On exit, D is destroyed.
*
* X (output) DOUBLE PRECISION array, dimension (N)
* On exit, X is the solution of the LSE problem.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,M+N+P).
* For optimum performance LWORK >= P+min(M,N)+max(M,N)*NB,
* where NB is an upper bound for the optimal blocksizes for
* DGEQRF, SGERQF, DORMQR and SORMRQ.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1: the upper triangular factor R associated with B in the
* generalized RQ factorization of the pair (B, A) is
* singular, so that rank(B) < P; the least squares
* solution could not be computed.
* = 2: the (N-P) by (N-P) part of the upper trapezoidal factor
* T associated with A in the generalized RQ factorization
* of the pair (B, A) is singular, so that
* rank( (A) ) < N; the least squares solution could not
* ( (B) )
* be computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param p
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param c
* @param _c_offset
* @param d
* @param _d_offset
* @param x
* @param _x_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dgglse(int m, int n, int p, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, double[] c, int _c_offset, double[] d, int _d_offset, double[] x, int _x_offset, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGGQRF computes a generalized QR factorization of an N-by-M matrix A
* and an N-by-P matrix B:
*
* A = Q*R, B = Q*T*Z,
*
* where Q is an N-by-N orthogonal matrix, Z is a P-by-P orthogonal
* matrix, and R and T assume one of the forms:
*
* if N >= M, R = ( R11 ) M , or if N < M, R = ( R11 R12 ) N,
* ( 0 ) N-M N M-N
* M
*
* where R11 is upper triangular, and
*
* if N <= P, T = ( 0 T12 ) N, or if N > P, T = ( T11 ) N-P,
* P-N N ( T21 ) P
* P
*
* where T12 or T21 is upper triangular.
*
* In particular, if B is square and nonsingular, the GQR factorization
* of A and B implicitly gives the QR factorization of inv(B)*A:
*
* inv(B)*A = Z'*(inv(T)*R)
*
* where inv(B) denotes the inverse of the matrix B, and Z' denotes the
* transpose of the matrix Z.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of rows of the matrices A and B. N >= 0.
*
* M (input) INTEGER
* The number of columns of the matrix A. M >= 0.
*
* P (input) INTEGER
* The number of columns of the matrix B. P >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,M)
* On entry, the N-by-M matrix A.
* On exit, the elements on and above the diagonal of the array
* contain the min(N,M)-by-M upper trapezoidal matrix R (R is
* upper triangular if N >= M); the elements below the diagonal,
* with the array TAUA, represent the orthogonal matrix Q as a
* product of min(N,M) elementary reflectors (see Further
* Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* TAUA (output) DOUBLE PRECISION array, dimension (min(N,M))
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix Q (see Further Details).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,P)
* On entry, the N-by-P matrix B.
* On exit, if N <= P, the upper triangle of the subarray
* B(1:N,P-N+1:P) contains the N-by-N upper triangular matrix T;
* if N > P, the elements on and above the (N-P)-th subdiagonal
* contain the N-by-P upper trapezoidal matrix T; the remaining
* elements, with the array TAUB, represent the orthogonal
* matrix Z as a product of elementary reflectors (see Further
* Details).
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* TAUB (output) DOUBLE PRECISION array, dimension (min(N,P))
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix Z (see Further Details).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N,M,P).
* For optimum performance LWORK >= max(N,M,P)*max(NB1,NB2,NB3),
* where NB1 is the optimal blocksize for the QR factorization
* of an N-by-M matrix, NB2 is the optimal blocksize for the
* RQ factorization of an N-by-P matrix, and NB3 is the optimal
* blocksize for a call of DORMQR.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(1) H(2) . . . H(k), where k = min(n,m).
*
* Each H(i) has the form
*
* H(i) = I - taua * v * v'
*
* where taua is a real scalar, and v is a real vector with
* v(1:i-1) = 0 and v(i) = 1; v(i+1:n) is stored on exit in A(i+1:n,i),
* and taua in TAUA(i).
* To form Q explicitly, use LAPACK subroutine DORGQR.
* To use Q to update another matrix, use LAPACK subroutine DORMQR.
*
* The matrix Z is represented as a product of elementary reflectors
*
* Z = H(1) H(2) . . . H(k), where k = min(n,p).
*
* Each H(i) has the form
*
* H(i) = I - taub * v * v'
*
* where taub is a real scalar, and v is a real vector with
* v(p-k+i+1:p) = 0 and v(p-k+i) = 1; v(1:p-k+i-1) is stored on exit in
* B(n-k+i,1:p-k+i-1), and taub in TAUB(i).
* To form Z explicitly, use LAPACK subroutine DORGRQ.
* To use Z to update another matrix, use LAPACK subroutine DORMRQ.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n
* @param m
* @param p
* @param a
* @param lda
* @param taua
* @param b
* @param ldb
* @param taub
* @param work
* @param lwork
* @param info
*
*/
abstract public void dggqrf(int n, int m, int p, double[] a, int lda, double[] taua, double[] b, int ldb, double[] taub, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGGQRF computes a generalized QR factorization of an N-by-M matrix A
* and an N-by-P matrix B:
*
* A = Q*R, B = Q*T*Z,
*
* where Q is an N-by-N orthogonal matrix, Z is a P-by-P orthogonal
* matrix, and R and T assume one of the forms:
*
* if N >= M, R = ( R11 ) M , or if N < M, R = ( R11 R12 ) N,
* ( 0 ) N-M N M-N
* M
*
* where R11 is upper triangular, and
*
* if N <= P, T = ( 0 T12 ) N, or if N > P, T = ( T11 ) N-P,
* P-N N ( T21 ) P
* P
*
* where T12 or T21 is upper triangular.
*
* In particular, if B is square and nonsingular, the GQR factorization
* of A and B implicitly gives the QR factorization of inv(B)*A:
*
* inv(B)*A = Z'*(inv(T)*R)
*
* where inv(B) denotes the inverse of the matrix B, and Z' denotes the
* transpose of the matrix Z.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of rows of the matrices A and B. N >= 0.
*
* M (input) INTEGER
* The number of columns of the matrix A. M >= 0.
*
* P (input) INTEGER
* The number of columns of the matrix B. P >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,M)
* On entry, the N-by-M matrix A.
* On exit, the elements on and above the diagonal of the array
* contain the min(N,M)-by-M upper trapezoidal matrix R (R is
* upper triangular if N >= M); the elements below the diagonal,
* with the array TAUA, represent the orthogonal matrix Q as a
* product of min(N,M) elementary reflectors (see Further
* Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* TAUA (output) DOUBLE PRECISION array, dimension (min(N,M))
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix Q (see Further Details).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,P)
* On entry, the N-by-P matrix B.
* On exit, if N <= P, the upper triangle of the subarray
* B(1:N,P-N+1:P) contains the N-by-N upper triangular matrix T;
* if N > P, the elements on and above the (N-P)-th subdiagonal
* contain the N-by-P upper trapezoidal matrix T; the remaining
* elements, with the array TAUB, represent the orthogonal
* matrix Z as a product of elementary reflectors (see Further
* Details).
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* TAUB (output) DOUBLE PRECISION array, dimension (min(N,P))
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix Z (see Further Details).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N,M,P).
* For optimum performance LWORK >= max(N,M,P)*max(NB1,NB2,NB3),
* where NB1 is the optimal blocksize for the QR factorization
* of an N-by-M matrix, NB2 is the optimal blocksize for the
* RQ factorization of an N-by-P matrix, and NB3 is the optimal
* blocksize for a call of DORMQR.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(1) H(2) . . . H(k), where k = min(n,m).
*
* Each H(i) has the form
*
* H(i) = I - taua * v * v'
*
* where taua is a real scalar, and v is a real vector with
* v(1:i-1) = 0 and v(i) = 1; v(i+1:n) is stored on exit in A(i+1:n,i),
* and taua in TAUA(i).
* To form Q explicitly, use LAPACK subroutine DORGQR.
* To use Q to update another matrix, use LAPACK subroutine DORMQR.
*
* The matrix Z is represented as a product of elementary reflectors
*
* Z = H(1) H(2) . . . H(k), where k = min(n,p).
*
* Each H(i) has the form
*
* H(i) = I - taub * v * v'
*
* where taub is a real scalar, and v is a real vector with
* v(p-k+i+1:p) = 0 and v(p-k+i) = 1; v(1:p-k+i-1) is stored on exit in
* B(n-k+i,1:p-k+i-1), and taub in TAUB(i).
* To form Z explicitly, use LAPACK subroutine DORGRQ.
* To use Z to update another matrix, use LAPACK subroutine DORMRQ.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n
* @param m
* @param p
* @param a
* @param _a_offset
* @param lda
* @param taua
* @param _taua_offset
* @param b
* @param _b_offset
* @param ldb
* @param taub
* @param _taub_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dggqrf(int n, int m, int p, double[] a, int _a_offset, int lda, double[] taua, int _taua_offset, double[] b, int _b_offset, int ldb, double[] taub, int _taub_offset, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGGRQF computes a generalized RQ factorization of an M-by-N matrix A
* and a P-by-N matrix B:
*
* A = R*Q, B = Z*T*Q,
*
* where Q is an N-by-N orthogonal matrix, Z is a P-by-P orthogonal
* matrix, and R and T assume one of the forms:
*
* if M <= N, R = ( 0 R12 ) M, or if M > N, R = ( R11 ) M-N,
* N-M M ( R21 ) N
* N
*
* where R12 or R21 is upper triangular, and
*
* if P >= N, T = ( T11 ) N , or if P < N, T = ( T11 T12 ) P,
* ( 0 ) P-N P N-P
* N
*
* where T11 is upper triangular.
*
* In particular, if B is square and nonsingular, the GRQ factorization
* of A and B implicitly gives the RQ factorization of A*inv(B):
*
* A*inv(B) = (R*inv(T))*Z'
*
* where inv(B) denotes the inverse of the matrix B, and Z' denotes the
* transpose of the matrix Z.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* P (input) INTEGER
* The number of rows of the matrix B. P >= 0.
*
* N (input) INTEGER
* The number of columns of the matrices A and B. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, if M <= N, the upper triangle of the subarray
* A(1:M,N-M+1:N) contains the M-by-M upper triangular matrix R;
* if M > N, the elements on and above the (M-N)-th subdiagonal
* contain the M-by-N upper trapezoidal matrix R; the remaining
* elements, with the array TAUA, represent the orthogonal
* matrix Q as a product of elementary reflectors (see Further
* Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAUA (output) DOUBLE PRECISION array, dimension (min(M,N))
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix Q (see Further Details).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,N)
* On entry, the P-by-N matrix B.
* On exit, the elements on and above the diagonal of the array
* contain the min(P,N)-by-N upper trapezoidal matrix T (T is
* upper triangular if P >= N); the elements below the diagonal,
* with the array TAUB, represent the orthogonal matrix Z as a
* product of elementary reflectors (see Further Details).
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,P).
*
* TAUB (output) DOUBLE PRECISION array, dimension (min(P,N))
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix Z (see Further Details).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N,M,P).
* For optimum performance LWORK >= max(N,M,P)*max(NB1,NB2,NB3),
* where NB1 is the optimal blocksize for the RQ factorization
* of an M-by-N matrix, NB2 is the optimal blocksize for the
* QR factorization of a P-by-N matrix, and NB3 is the optimal
* blocksize for a call of DORMRQ.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INF0= -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(1) H(2) . . . H(k), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - taua * v * v'
*
* where taua is a real scalar, and v is a real vector with
* v(n-k+i+1:n) = 0 and v(n-k+i) = 1; v(1:n-k+i-1) is stored on exit in
* A(m-k+i,1:n-k+i-1), and taua in TAUA(i).
* To form Q explicitly, use LAPACK subroutine DORGRQ.
* To use Q to update another matrix, use LAPACK subroutine DORMRQ.
*
* The matrix Z is represented as a product of elementary reflectors
*
* Z = H(1) H(2) . . . H(k), where k = min(p,n).
*
* Each H(i) has the form
*
* H(i) = I - taub * v * v'
*
* where taub is a real scalar, and v is a real vector with
* v(1:i-1) = 0 and v(i) = 1; v(i+1:p) is stored on exit in B(i+1:p,i),
* and taub in TAUB(i).
* To form Z explicitly, use LAPACK subroutine DORGQR.
* To use Z to update another matrix, use LAPACK subroutine DORMQR.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param m
* @param p
* @param n
* @param a
* @param lda
* @param taua
* @param b
* @param ldb
* @param taub
* @param work
* @param lwork
* @param info
*
*/
abstract public void dggrqf(int m, int p, int n, double[] a, int lda, double[] taua, double[] b, int ldb, double[] taub, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGGRQF computes a generalized RQ factorization of an M-by-N matrix A
* and a P-by-N matrix B:
*
* A = R*Q, B = Z*T*Q,
*
* where Q is an N-by-N orthogonal matrix, Z is a P-by-P orthogonal
* matrix, and R and T assume one of the forms:
*
* if M <= N, R = ( 0 R12 ) M, or if M > N, R = ( R11 ) M-N,
* N-M M ( R21 ) N
* N
*
* where R12 or R21 is upper triangular, and
*
* if P >= N, T = ( T11 ) N , or if P < N, T = ( T11 T12 ) P,
* ( 0 ) P-N P N-P
* N
*
* where T11 is upper triangular.
*
* In particular, if B is square and nonsingular, the GRQ factorization
* of A and B implicitly gives the RQ factorization of A*inv(B):
*
* A*inv(B) = (R*inv(T))*Z'
*
* where inv(B) denotes the inverse of the matrix B, and Z' denotes the
* transpose of the matrix Z.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* P (input) INTEGER
* The number of rows of the matrix B. P >= 0.
*
* N (input) INTEGER
* The number of columns of the matrices A and B. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, if M <= N, the upper triangle of the subarray
* A(1:M,N-M+1:N) contains the M-by-M upper triangular matrix R;
* if M > N, the elements on and above the (M-N)-th subdiagonal
* contain the M-by-N upper trapezoidal matrix R; the remaining
* elements, with the array TAUA, represent the orthogonal
* matrix Q as a product of elementary reflectors (see Further
* Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAUA (output) DOUBLE PRECISION array, dimension (min(M,N))
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix Q (see Further Details).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,N)
* On entry, the P-by-N matrix B.
* On exit, the elements on and above the diagonal of the array
* contain the min(P,N)-by-N upper trapezoidal matrix T (T is
* upper triangular if P >= N); the elements below the diagonal,
* with the array TAUB, represent the orthogonal matrix Z as a
* product of elementary reflectors (see Further Details).
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,P).
*
* TAUB (output) DOUBLE PRECISION array, dimension (min(P,N))
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix Z (see Further Details).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N,M,P).
* For optimum performance LWORK >= max(N,M,P)*max(NB1,NB2,NB3),
* where NB1 is the optimal blocksize for the RQ factorization
* of an M-by-N matrix, NB2 is the optimal blocksize for the
* QR factorization of a P-by-N matrix, and NB3 is the optimal
* blocksize for a call of DORMRQ.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INF0= -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(1) H(2) . . . H(k), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - taua * v * v'
*
* where taua is a real scalar, and v is a real vector with
* v(n-k+i+1:n) = 0 and v(n-k+i) = 1; v(1:n-k+i-1) is stored on exit in
* A(m-k+i,1:n-k+i-1), and taua in TAUA(i).
* To form Q explicitly, use LAPACK subroutine DORGRQ.
* To use Q to update another matrix, use LAPACK subroutine DORMRQ.
*
* The matrix Z is represented as a product of elementary reflectors
*
* Z = H(1) H(2) . . . H(k), where k = min(p,n).
*
* Each H(i) has the form
*
* H(i) = I - taub * v * v'
*
* where taub is a real scalar, and v is a real vector with
* v(1:i-1) = 0 and v(i) = 1; v(i+1:p) is stored on exit in B(i+1:p,i),
* and taub in TAUB(i).
* To form Z explicitly, use LAPACK subroutine DORGQR.
* To use Z to update another matrix, use LAPACK subroutine DORMQR.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param m
* @param p
* @param n
* @param a
* @param _a_offset
* @param lda
* @param taua
* @param _taua_offset
* @param b
* @param _b_offset
* @param ldb
* @param taub
* @param _taub_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dggrqf(int m, int p, int n, double[] a, int _a_offset, int lda, double[] taua, int _taua_offset, double[] b, int _b_offset, int ldb, double[] taub, int _taub_offset, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGGSVD computes the generalized singular value decomposition (GSVD)
* of an M-by-N real matrix A and P-by-N real matrix B:
*
* U'*A*Q = D1*( 0 R ), V'*B*Q = D2*( 0 R )
*
* where U, V and Q are orthogonal matrices, and Z' is the transpose
* of Z. Let K+L = the effective numerical rank of the matrix (A',B')',
* then R is a K+L-by-K+L nonsingular upper triangular matrix, D1 and
* D2 are M-by-(K+L) and P-by-(K+L) "diagonal" matrices and of the
* following structures, respectively:
*
* If M-K-L >= 0,
*
* K L
* D1 = K ( I 0 )
* L ( 0 C )
* M-K-L ( 0 0 )
*
* K L
* D2 = L ( 0 S )
* P-L ( 0 0 )
*
* N-K-L K L
* ( 0 R ) = K ( 0 R11 R12 )
* L ( 0 0 R22 )
*
* where
*
* C = diag( ALPHA(K+1), ... , ALPHA(K+L) ),
* S = diag( BETA(K+1), ... , BETA(K+L) ),
* C**2 + S**2 = I.
*
* R is stored in A(1:K+L,N-K-L+1:N) on exit.
*
* If M-K-L < 0,
*
* K M-K K+L-M
* D1 = K ( I 0 0 )
* M-K ( 0 C 0 )
*
* K M-K K+L-M
* D2 = M-K ( 0 S 0 )
* K+L-M ( 0 0 I )
* P-L ( 0 0 0 )
*
* N-K-L K M-K K+L-M
* ( 0 R ) = K ( 0 R11 R12 R13 )
* M-K ( 0 0 R22 R23 )
* K+L-M ( 0 0 0 R33 )
*
* where
*
* C = diag( ALPHA(K+1), ... , ALPHA(M) ),
* S = diag( BETA(K+1), ... , BETA(M) ),
* C**2 + S**2 = I.
*
* (R11 R12 R13 ) is stored in A(1:M, N-K-L+1:N), and R33 is stored
* ( 0 R22 R23 )
* in B(M-K+1:L,N+M-K-L+1:N) on exit.
*
* The routine computes C, S, R, and optionally the orthogonal
* transformation matrices U, V and Q.
*
* In particular, if B is an N-by-N nonsingular matrix, then the GSVD of
* A and B implicitly gives the SVD of A*inv(B):
* A*inv(B) = U*(D1*inv(D2))*V'.
* If ( A',B')' has orthonormal columns, then the GSVD of A and B is
* also equal to the CS decomposition of A and B. Furthermore, the GSVD
* can be used to derive the solution of the eigenvalue problem:
* A'*A x = lambda* B'*B x.
* In some literature, the GSVD of A and B is presented in the form
* U'*A*X = ( 0 D1 ), V'*B*X = ( 0 D2 )
* where U and V are orthogonal and X is nonsingular, D1 and D2 are
* ``diagonal''. The former GSVD form can be converted to the latter
* form by taking the nonsingular matrix X as
*
* X = Q*( I 0 )
* ( 0 inv(R) ).
*
* Arguments
* =========
*
* JOBU (input) CHARACTER*1
* = 'U': Orthogonal matrix U is computed;
* = 'N': U is not computed.
*
* JOBV (input) CHARACTER*1
* = 'V': Orthogonal matrix V is computed;
* = 'N': V is not computed.
*
* JOBQ (input) CHARACTER*1
* = 'Q': Orthogonal matrix Q is computed;
* = 'N': Q is not computed.
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrices A and B. N >= 0.
*
* P (input) INTEGER
* The number of rows of the matrix B. P >= 0.
*
* K (output) INTEGER
* L (output) INTEGER
* On exit, K and L specify the dimension of the subblocks
* described in the Purpose section.
* K + L = effective numerical rank of (A',B')'.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, A contains the triangular matrix R, or part of R.
* See Purpose for details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,N)
* On entry, the P-by-N matrix B.
* On exit, B contains the triangular matrix R if M-K-L < 0.
* See Purpose for details.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,P).
*
* ALPHA (output) DOUBLE PRECISION array, dimension (N)
* BETA (output) DOUBLE PRECISION array, dimension (N)
* On exit, ALPHA and BETA contain the generalized singular
* value pairs of A and B;
* ALPHA(1:K) = 1,
* BETA(1:K) = 0,
* and if M-K-L >= 0,
* ALPHA(K+1:K+L) = C,
* BETA(K+1:K+L) = S,
* or if M-K-L < 0,
* ALPHA(K+1:M)=C, ALPHA(M+1:K+L)=0
* BETA(K+1:M) =S, BETA(M+1:K+L) =1
* and
* ALPHA(K+L+1:N) = 0
* BETA(K+L+1:N) = 0
*
* U (output) DOUBLE PRECISION array, dimension (LDU,M)
* If JOBU = 'U', U contains the M-by-M orthogonal matrix U.
* If JOBU = 'N', U is not referenced.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= max(1,M) if
* JOBU = 'U'; LDU >= 1 otherwise.
*
* V (output) DOUBLE PRECISION array, dimension (LDV,P)
* If JOBV = 'V', V contains the P-by-P orthogonal matrix V.
* If JOBV = 'N', V is not referenced.
*
* LDV (input) INTEGER
* The leading dimension of the array V. LDV >= max(1,P) if
* JOBV = 'V'; LDV >= 1 otherwise.
*
* Q (output) DOUBLE PRECISION array, dimension (LDQ,N)
* If JOBQ = 'Q', Q contains the N-by-N orthogonal matrix Q.
* If JOBQ = 'N', Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N) if
* JOBQ = 'Q'; LDQ >= 1 otherwise.
*
* WORK (workspace) DOUBLE PRECISION array,
* dimension (max(3*N,M,P)+N)
*
* IWORK (workspace/output) INTEGER array, dimension (N)
* On exit, IWORK stores the sorting information. More
* precisely, the following loop will sort ALPHA
* for I = K+1, min(M,K+L)
* swap ALPHA(I) and ALPHA(IWORK(I))
* endfor
* such that ALPHA(1) >= ALPHA(2) >= ... >= ALPHA(N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, the Jacobi-type procedure failed to
* converge. For further details, see subroutine DTGSJA.
*
* Internal Parameters
* ===================
*
* TOLA DOUBLE PRECISION
* TOLB DOUBLE PRECISION
* TOLA and TOLB are the thresholds to determine the effective
* rank of (A',B')'. Generally, they are set to
* TOLA = MAX(M,N)*norm(A)*MAZHEPS,
* TOLB = MAX(P,N)*norm(B)*MAZHEPS.
* The size of TOLA and TOLB may affect the size of backward
* errors of the decomposition.
*
* Further Details
* ===============
*
* 2-96 Based on modifications by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param jobu
* @param jobv
* @param jobq
* @param m
* @param n
* @param p
* @param k
* @param l
* @param a
* @param lda
* @param b
* @param ldb
* @param alpha
* @param beta
* @param u
* @param ldu
* @param v
* @param ldv
* @param q
* @param ldq
* @param work
* @param iwork
* @param info
*
*/
abstract public void dggsvd(java.lang.String jobu, java.lang.String jobv, java.lang.String jobq, int m, int n, int p, org.netlib.util.intW k, org.netlib.util.intW l, double[] a, int lda, double[] b, int ldb, double[] alpha, double[] beta, double[] u, int ldu, double[] v, int ldv, double[] q, int ldq, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGGSVD computes the generalized singular value decomposition (GSVD)
* of an M-by-N real matrix A and P-by-N real matrix B:
*
* U'*A*Q = D1*( 0 R ), V'*B*Q = D2*( 0 R )
*
* where U, V and Q are orthogonal matrices, and Z' is the transpose
* of Z. Let K+L = the effective numerical rank of the matrix (A',B')',
* then R is a K+L-by-K+L nonsingular upper triangular matrix, D1 and
* D2 are M-by-(K+L) and P-by-(K+L) "diagonal" matrices and of the
* following structures, respectively:
*
* If M-K-L >= 0,
*
* K L
* D1 = K ( I 0 )
* L ( 0 C )
* M-K-L ( 0 0 )
*
* K L
* D2 = L ( 0 S )
* P-L ( 0 0 )
*
* N-K-L K L
* ( 0 R ) = K ( 0 R11 R12 )
* L ( 0 0 R22 )
*
* where
*
* C = diag( ALPHA(K+1), ... , ALPHA(K+L) ),
* S = diag( BETA(K+1), ... , BETA(K+L) ),
* C**2 + S**2 = I.
*
* R is stored in A(1:K+L,N-K-L+1:N) on exit.
*
* If M-K-L < 0,
*
* K M-K K+L-M
* D1 = K ( I 0 0 )
* M-K ( 0 C 0 )
*
* K M-K K+L-M
* D2 = M-K ( 0 S 0 )
* K+L-M ( 0 0 I )
* P-L ( 0 0 0 )
*
* N-K-L K M-K K+L-M
* ( 0 R ) = K ( 0 R11 R12 R13 )
* M-K ( 0 0 R22 R23 )
* K+L-M ( 0 0 0 R33 )
*
* where
*
* C = diag( ALPHA(K+1), ... , ALPHA(M) ),
* S = diag( BETA(K+1), ... , BETA(M) ),
* C**2 + S**2 = I.
*
* (R11 R12 R13 ) is stored in A(1:M, N-K-L+1:N), and R33 is stored
* ( 0 R22 R23 )
* in B(M-K+1:L,N+M-K-L+1:N) on exit.
*
* The routine computes C, S, R, and optionally the orthogonal
* transformation matrices U, V and Q.
*
* In particular, if B is an N-by-N nonsingular matrix, then the GSVD of
* A and B implicitly gives the SVD of A*inv(B):
* A*inv(B) = U*(D1*inv(D2))*V'.
* If ( A',B')' has orthonormal columns, then the GSVD of A and B is
* also equal to the CS decomposition of A and B. Furthermore, the GSVD
* can be used to derive the solution of the eigenvalue problem:
* A'*A x = lambda* B'*B x.
* In some literature, the GSVD of A and B is presented in the form
* U'*A*X = ( 0 D1 ), V'*B*X = ( 0 D2 )
* where U and V are orthogonal and X is nonsingular, D1 and D2 are
* ``diagonal''. The former GSVD form can be converted to the latter
* form by taking the nonsingular matrix X as
*
* X = Q*( I 0 )
* ( 0 inv(R) ).
*
* Arguments
* =========
*
* JOBU (input) CHARACTER*1
* = 'U': Orthogonal matrix U is computed;
* = 'N': U is not computed.
*
* JOBV (input) CHARACTER*1
* = 'V': Orthogonal matrix V is computed;
* = 'N': V is not computed.
*
* JOBQ (input) CHARACTER*1
* = 'Q': Orthogonal matrix Q is computed;
* = 'N': Q is not computed.
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrices A and B. N >= 0.
*
* P (input) INTEGER
* The number of rows of the matrix B. P >= 0.
*
* K (output) INTEGER
* L (output) INTEGER
* On exit, K and L specify the dimension of the subblocks
* described in the Purpose section.
* K + L = effective numerical rank of (A',B')'.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, A contains the triangular matrix R, or part of R.
* See Purpose for details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,N)
* On entry, the P-by-N matrix B.
* On exit, B contains the triangular matrix R if M-K-L < 0.
* See Purpose for details.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,P).
*
* ALPHA (output) DOUBLE PRECISION array, dimension (N)
* BETA (output) DOUBLE PRECISION array, dimension (N)
* On exit, ALPHA and BETA contain the generalized singular
* value pairs of A and B;
* ALPHA(1:K) = 1,
* BETA(1:K) = 0,
* and if M-K-L >= 0,
* ALPHA(K+1:K+L) = C,
* BETA(K+1:K+L) = S,
* or if M-K-L < 0,
* ALPHA(K+1:M)=C, ALPHA(M+1:K+L)=0
* BETA(K+1:M) =S, BETA(M+1:K+L) =1
* and
* ALPHA(K+L+1:N) = 0
* BETA(K+L+1:N) = 0
*
* U (output) DOUBLE PRECISION array, dimension (LDU,M)
* If JOBU = 'U', U contains the M-by-M orthogonal matrix U.
* If JOBU = 'N', U is not referenced.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= max(1,M) if
* JOBU = 'U'; LDU >= 1 otherwise.
*
* V (output) DOUBLE PRECISION array, dimension (LDV,P)
* If JOBV = 'V', V contains the P-by-P orthogonal matrix V.
* If JOBV = 'N', V is not referenced.
*
* LDV (input) INTEGER
* The leading dimension of the array V. LDV >= max(1,P) if
* JOBV = 'V'; LDV >= 1 otherwise.
*
* Q (output) DOUBLE PRECISION array, dimension (LDQ,N)
* If JOBQ = 'Q', Q contains the N-by-N orthogonal matrix Q.
* If JOBQ = 'N', Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N) if
* JOBQ = 'Q'; LDQ >= 1 otherwise.
*
* WORK (workspace) DOUBLE PRECISION array,
* dimension (max(3*N,M,P)+N)
*
* IWORK (workspace/output) INTEGER array, dimension (N)
* On exit, IWORK stores the sorting information. More
* precisely, the following loop will sort ALPHA
* for I = K+1, min(M,K+L)
* swap ALPHA(I) and ALPHA(IWORK(I))
* endfor
* such that ALPHA(1) >= ALPHA(2) >= ... >= ALPHA(N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, the Jacobi-type procedure failed to
* converge. For further details, see subroutine DTGSJA.
*
* Internal Parameters
* ===================
*
* TOLA DOUBLE PRECISION
* TOLB DOUBLE PRECISION
* TOLA and TOLB are the thresholds to determine the effective
* rank of (A',B')'. Generally, they are set to
* TOLA = MAX(M,N)*norm(A)*MAZHEPS,
* TOLB = MAX(P,N)*norm(B)*MAZHEPS.
* The size of TOLA and TOLB may affect the size of backward
* errors of the decomposition.
*
* Further Details
* ===============
*
* 2-96 Based on modifications by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param jobu
* @param jobv
* @param jobq
* @param m
* @param n
* @param p
* @param k
* @param l
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param alpha
* @param _alpha_offset
* @param beta
* @param _beta_offset
* @param u
* @param _u_offset
* @param ldu
* @param v
* @param _v_offset
* @param ldv
* @param q
* @param _q_offset
* @param ldq
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dggsvd(java.lang.String jobu, java.lang.String jobv, java.lang.String jobq, int m, int n, int p, org.netlib.util.intW k, org.netlib.util.intW l, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, double[] alpha, int _alpha_offset, double[] beta, int _beta_offset, double[] u, int _u_offset, int ldu, double[] v, int _v_offset, int ldv, double[] q, int _q_offset, int ldq, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGGSVP computes orthogonal matrices U, V and Q such that
*
* N-K-L K L
* U'*A*Q = K ( 0 A12 A13 ) if M-K-L >= 0;
* L ( 0 0 A23 )
* M-K-L ( 0 0 0 )
*
* N-K-L K L
* = K ( 0 A12 A13 ) if M-K-L < 0;
* M-K ( 0 0 A23 )
*
* N-K-L K L
* V'*B*Q = L ( 0 0 B13 )
* P-L ( 0 0 0 )
*
* where the K-by-K matrix A12 and L-by-L matrix B13 are nonsingular
* upper triangular; A23 is L-by-L upper triangular if M-K-L >= 0,
* otherwise A23 is (M-K)-by-L upper trapezoidal. K+L = the effective
* numerical rank of the (M+P)-by-N matrix (A',B')'. Z' denotes the
* transpose of Z.
*
* This decomposition is the preprocessing step for computing the
* Generalized Singular Value Decomposition (GSVD), see subroutine
* DGGSVD.
*
* Arguments
* =========
*
* JOBU (input) CHARACTER*1
* = 'U': Orthogonal matrix U is computed;
* = 'N': U is not computed.
*
* JOBV (input) CHARACTER*1
* = 'V': Orthogonal matrix V is computed;
* = 'N': V is not computed.
*
* JOBQ (input) CHARACTER*1
* = 'Q': Orthogonal matrix Q is computed;
* = 'N': Q is not computed.
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* P (input) INTEGER
* The number of rows of the matrix B. P >= 0.
*
* N (input) INTEGER
* The number of columns of the matrices A and B. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, A contains the triangular (or trapezoidal) matrix
* described in the Purpose section.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,N)
* On entry, the P-by-N matrix B.
* On exit, B contains the triangular matrix described in
* the Purpose section.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,P).
*
* TOLA (input) DOUBLE PRECISION
* TOLB (input) DOUBLE PRECISION
* TOLA and TOLB are the thresholds to determine the effective
* numerical rank of matrix B and a subblock of A. Generally,
* they are set to
* TOLA = MAX(M,N)*norm(A)*MAZHEPS,
* TOLB = MAX(P,N)*norm(B)*MAZHEPS.
* The size of TOLA and TOLB may affect the size of backward
* errors of the decomposition.
*
* K (output) INTEGER
* L (output) INTEGER
* On exit, K and L specify the dimension of the subblocks
* described in Purpose.
* K + L = effective numerical rank of (A',B')'.
*
* U (output) DOUBLE PRECISION array, dimension (LDU,M)
* If JOBU = 'U', U contains the orthogonal matrix U.
* If JOBU = 'N', U is not referenced.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= max(1,M) if
* JOBU = 'U'; LDU >= 1 otherwise.
*
* V (output) DOUBLE PRECISION array, dimension (LDV,M)
* If JOBV = 'V', V contains the orthogonal matrix V.
* If JOBV = 'N', V is not referenced.
*
* LDV (input) INTEGER
* The leading dimension of the array V. LDV >= max(1,P) if
* JOBV = 'V'; LDV >= 1 otherwise.
*
* Q (output) DOUBLE PRECISION array, dimension (LDQ,N)
* If JOBQ = 'Q', Q contains the orthogonal matrix Q.
* If JOBQ = 'N', Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N) if
* JOBQ = 'Q'; LDQ >= 1 otherwise.
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* TAU (workspace) DOUBLE PRECISION array, dimension (N)
*
* WORK (workspace) DOUBLE PRECISION array, dimension (max(3*N,M,P))
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
*
* Further Details
* ===============
*
* The subroutine uses LAPACK subroutine DGEQPF for the QR factorization
* with column pivoting to detect the effective numerical rank of the
* a matrix. It may be replaced by a better rank determination strategy.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobu
* @param jobv
* @param jobq
* @param m
* @param p
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param tola
* @param tolb
* @param k
* @param l
* @param u
* @param ldu
* @param v
* @param ldv
* @param q
* @param ldq
* @param iwork
* @param tau
* @param work
* @param info
*
*/
abstract public void dggsvp(java.lang.String jobu, java.lang.String jobv, java.lang.String jobq, int m, int p, int n, double[] a, int lda, double[] b, int ldb, double tola, double tolb, org.netlib.util.intW k, org.netlib.util.intW l, double[] u, int ldu, double[] v, int ldv, double[] q, int ldq, int[] iwork, double[] tau, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGGSVP computes orthogonal matrices U, V and Q such that
*
* N-K-L K L
* U'*A*Q = K ( 0 A12 A13 ) if M-K-L >= 0;
* L ( 0 0 A23 )
* M-K-L ( 0 0 0 )
*
* N-K-L K L
* = K ( 0 A12 A13 ) if M-K-L < 0;
* M-K ( 0 0 A23 )
*
* N-K-L K L
* V'*B*Q = L ( 0 0 B13 )
* P-L ( 0 0 0 )
*
* where the K-by-K matrix A12 and L-by-L matrix B13 are nonsingular
* upper triangular; A23 is L-by-L upper triangular if M-K-L >= 0,
* otherwise A23 is (M-K)-by-L upper trapezoidal. K+L = the effective
* numerical rank of the (M+P)-by-N matrix (A',B')'. Z' denotes the
* transpose of Z.
*
* This decomposition is the preprocessing step for computing the
* Generalized Singular Value Decomposition (GSVD), see subroutine
* DGGSVD.
*
* Arguments
* =========
*
* JOBU (input) CHARACTER*1
* = 'U': Orthogonal matrix U is computed;
* = 'N': U is not computed.
*
* JOBV (input) CHARACTER*1
* = 'V': Orthogonal matrix V is computed;
* = 'N': V is not computed.
*
* JOBQ (input) CHARACTER*1
* = 'Q': Orthogonal matrix Q is computed;
* = 'N': Q is not computed.
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* P (input) INTEGER
* The number of rows of the matrix B. P >= 0.
*
* N (input) INTEGER
* The number of columns of the matrices A and B. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, A contains the triangular (or trapezoidal) matrix
* described in the Purpose section.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,N)
* On entry, the P-by-N matrix B.
* On exit, B contains the triangular matrix described in
* the Purpose section.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,P).
*
* TOLA (input) DOUBLE PRECISION
* TOLB (input) DOUBLE PRECISION
* TOLA and TOLB are the thresholds to determine the effective
* numerical rank of matrix B and a subblock of A. Generally,
* they are set to
* TOLA = MAX(M,N)*norm(A)*MAZHEPS,
* TOLB = MAX(P,N)*norm(B)*MAZHEPS.
* The size of TOLA and TOLB may affect the size of backward
* errors of the decomposition.
*
* K (output) INTEGER
* L (output) INTEGER
* On exit, K and L specify the dimension of the subblocks
* described in Purpose.
* K + L = effective numerical rank of (A',B')'.
*
* U (output) DOUBLE PRECISION array, dimension (LDU,M)
* If JOBU = 'U', U contains the orthogonal matrix U.
* If JOBU = 'N', U is not referenced.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= max(1,M) if
* JOBU = 'U'; LDU >= 1 otherwise.
*
* V (output) DOUBLE PRECISION array, dimension (LDV,M)
* If JOBV = 'V', V contains the orthogonal matrix V.
* If JOBV = 'N', V is not referenced.
*
* LDV (input) INTEGER
* The leading dimension of the array V. LDV >= max(1,P) if
* JOBV = 'V'; LDV >= 1 otherwise.
*
* Q (output) DOUBLE PRECISION array, dimension (LDQ,N)
* If JOBQ = 'Q', Q contains the orthogonal matrix Q.
* If JOBQ = 'N', Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N) if
* JOBQ = 'Q'; LDQ >= 1 otherwise.
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* TAU (workspace) DOUBLE PRECISION array, dimension (N)
*
* WORK (workspace) DOUBLE PRECISION array, dimension (max(3*N,M,P))
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
*
* Further Details
* ===============
*
* The subroutine uses LAPACK subroutine DGEQPF for the QR factorization
* with column pivoting to detect the effective numerical rank of the
* a matrix. It may be replaced by a better rank determination strategy.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobu
* @param jobv
* @param jobq
* @param m
* @param p
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param tola
* @param tolb
* @param k
* @param l
* @param u
* @param _u_offset
* @param ldu
* @param v
* @param _v_offset
* @param ldv
* @param q
* @param _q_offset
* @param ldq
* @param iwork
* @param _iwork_offset
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dggsvp(java.lang.String jobu, java.lang.String jobv, java.lang.String jobq, int m, int p, int n, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, double tola, double tolb, org.netlib.util.intW k, org.netlib.util.intW l, double[] u, int _u_offset, int ldu, double[] v, int _v_offset, int ldv, double[] q, int _q_offset, int ldq, int[] iwork, int _iwork_offset, double[] tau, int _tau_offset, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGTCON estimates the reciprocal of the condition number of a real
* tridiagonal matrix A using the LU factorization as computed by
* DGTTRF.
*
* An estimate is obtained for norm(inv(A)), and the reciprocal of the
* condition number is computed as RCOND = 1 / (ANORM * norm(inv(A))).
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies whether the 1-norm condition number or the
* infinity-norm condition number is required:
* = '1' or 'O': 1-norm;
* = 'I': Infinity-norm.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* DL (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) multipliers that define the matrix L from the
* LU factorization of A as computed by DGTTRF.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The n diagonal elements of the upper triangular matrix U from
* the LU factorization of A.
*
* DU (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) elements of the first superdiagonal of U.
*
* DU2 (input) DOUBLE PRECISION array, dimension (N-2)
* The (n-2) elements of the second superdiagonal of U.
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices; for 1 <= i <= n, row i of the matrix was
* interchanged with row IPIV(i). IPIV(i) will always be either
* i or i+1; IPIV(i) = i indicates a row interchange was not
* required.
*
* ANORM (input) DOUBLE PRECISION
* If NORM = '1' or 'O', the 1-norm of the original matrix A.
* If NORM = 'I', the infinity-norm of the original matrix A.
*
* RCOND (output) DOUBLE PRECISION
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(ANORM * AINVNM), where AINVNM is an
* estimate of the 1-norm of inv(A) computed in this routine.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (2*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param n
* @param dl
* @param d
* @param du
* @param du2
* @param ipiv
* @param anorm
* @param rcond
* @param work
* @param iwork
* @param info
*
*/
abstract public void dgtcon(java.lang.String norm, int n, double[] dl, double[] d, double[] du, double[] du2, int[] ipiv, double anorm, org.netlib.util.doubleW rcond, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGTCON estimates the reciprocal of the condition number of a real
* tridiagonal matrix A using the LU factorization as computed by
* DGTTRF.
*
* An estimate is obtained for norm(inv(A)), and the reciprocal of the
* condition number is computed as RCOND = 1 / (ANORM * norm(inv(A))).
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies whether the 1-norm condition number or the
* infinity-norm condition number is required:
* = '1' or 'O': 1-norm;
* = 'I': Infinity-norm.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* DL (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) multipliers that define the matrix L from the
* LU factorization of A as computed by DGTTRF.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The n diagonal elements of the upper triangular matrix U from
* the LU factorization of A.
*
* DU (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) elements of the first superdiagonal of U.
*
* DU2 (input) DOUBLE PRECISION array, dimension (N-2)
* The (n-2) elements of the second superdiagonal of U.
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices; for 1 <= i <= n, row i of the matrix was
* interchanged with row IPIV(i). IPIV(i) will always be either
* i or i+1; IPIV(i) = i indicates a row interchange was not
* required.
*
* ANORM (input) DOUBLE PRECISION
* If NORM = '1' or 'O', the 1-norm of the original matrix A.
* If NORM = 'I', the infinity-norm of the original matrix A.
*
* RCOND (output) DOUBLE PRECISION
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(ANORM * AINVNM), where AINVNM is an
* estimate of the 1-norm of inv(A) computed in this routine.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (2*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param n
* @param dl
* @param _dl_offset
* @param d
* @param _d_offset
* @param du
* @param _du_offset
* @param du2
* @param _du2_offset
* @param ipiv
* @param _ipiv_offset
* @param anorm
* @param rcond
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dgtcon(java.lang.String norm, int n, double[] dl, int _dl_offset, double[] d, int _d_offset, double[] du, int _du_offset, double[] du2, int _du2_offset, int[] ipiv, int _ipiv_offset, double anorm, org.netlib.util.doubleW rcond, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGTRFS improves the computed solution to a system of linear
* equations when the coefficient matrix is tridiagonal, and provides
* error bounds and backward error estimates for the solution.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* DL (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) subdiagonal elements of A.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The diagonal elements of A.
*
* DU (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) superdiagonal elements of A.
*
* DLF (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) multipliers that define the matrix L from the
* LU factorization of A as computed by DGTTRF.
*
* DF (input) DOUBLE PRECISION array, dimension (N)
* The n diagonal elements of the upper triangular matrix U from
* the LU factorization of A.
*
* DUF (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) elements of the first superdiagonal of U.
*
* DU2 (input) DOUBLE PRECISION array, dimension (N-2)
* The (n-2) elements of the second superdiagonal of U.
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices; for 1 <= i <= n, row i of the matrix was
* interchanged with row IPIV(i). IPIV(i) will always be either
* i or i+1; IPIV(i) = i indicates a row interchange was not
* required.
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by DGTTRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param trans
* @param n
* @param nrhs
* @param dl
* @param d
* @param du
* @param dlf
* @param df
* @param duf
* @param du2
* @param ipiv
* @param b
* @param ldb
* @param x
* @param ldx
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void dgtrfs(java.lang.String trans, int n, int nrhs, double[] dl, double[] d, double[] du, double[] dlf, double[] df, double[] duf, double[] du2, int[] ipiv, double[] b, int ldb, double[] x, int ldx, double[] ferr, double[] berr, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGTRFS improves the computed solution to a system of linear
* equations when the coefficient matrix is tridiagonal, and provides
* error bounds and backward error estimates for the solution.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* DL (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) subdiagonal elements of A.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The diagonal elements of A.
*
* DU (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) superdiagonal elements of A.
*
* DLF (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) multipliers that define the matrix L from the
* LU factorization of A as computed by DGTTRF.
*
* DF (input) DOUBLE PRECISION array, dimension (N)
* The n diagonal elements of the upper triangular matrix U from
* the LU factorization of A.
*
* DUF (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) elements of the first superdiagonal of U.
*
* DU2 (input) DOUBLE PRECISION array, dimension (N-2)
* The (n-2) elements of the second superdiagonal of U.
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices; for 1 <= i <= n, row i of the matrix was
* interchanged with row IPIV(i). IPIV(i) will always be either
* i or i+1; IPIV(i) = i indicates a row interchange was not
* required.
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by DGTTRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param trans
* @param n
* @param nrhs
* @param dl
* @param _dl_offset
* @param d
* @param _d_offset
* @param du
* @param _du_offset
* @param dlf
* @param _dlf_offset
* @param df
* @param _df_offset
* @param duf
* @param _duf_offset
* @param du2
* @param _du2_offset
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dgtrfs(java.lang.String trans, int n, int nrhs, double[] dl, int _dl_offset, double[] d, int _d_offset, double[] du, int _du_offset, double[] dlf, int _dlf_offset, double[] df, int _df_offset, double[] duf, int _duf_offset, double[] du2, int _du2_offset, int[] ipiv, int _ipiv_offset, double[] b, int _b_offset, int ldb, double[] x, int _x_offset, int ldx, double[] ferr, int _ferr_offset, double[] berr, int _berr_offset, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGTSV solves the equation
*
* A*X = B,
*
* where A is an n by n tridiagonal matrix, by Gaussian elimination with
* partial pivoting.
*
* Note that the equation A'*X = B may be solved by interchanging the
* order of the arguments DU and DL.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* DL (input/output) DOUBLE PRECISION array, dimension (N-1)
* On entry, DL must contain the (n-1) sub-diagonal elements of
* A.
*
* On exit, DL is overwritten by the (n-2) elements of the
* second super-diagonal of the upper triangular matrix U from
* the LU factorization of A, in DL(1), ..., DL(n-2).
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, D must contain the diagonal elements of A.
*
* On exit, D is overwritten by the n diagonal elements of U.
*
* DU (input/output) DOUBLE PRECISION array, dimension (N-1)
* On entry, DU must contain the (n-1) super-diagonal elements
* of A.
*
* On exit, DU is overwritten by the (n-1) elements of the first
* super-diagonal of U.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the N by NRHS matrix of right hand side matrix B.
* On exit, if INFO = 0, the N by NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, U(i,i) is exactly zero, and the solution
* has not been computed. The factorization has not been
* completed unless i = N.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param nrhs
* @param dl
* @param d
* @param du
* @param b
* @param ldb
* @param info
*
*/
abstract public void dgtsv(int n, int nrhs, double[] dl, double[] d, double[] du, double[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGTSV solves the equation
*
* A*X = B,
*
* where A is an n by n tridiagonal matrix, by Gaussian elimination with
* partial pivoting.
*
* Note that the equation A'*X = B may be solved by interchanging the
* order of the arguments DU and DL.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* DL (input/output) DOUBLE PRECISION array, dimension (N-1)
* On entry, DL must contain the (n-1) sub-diagonal elements of
* A.
*
* On exit, DL is overwritten by the (n-2) elements of the
* second super-diagonal of the upper triangular matrix U from
* the LU factorization of A, in DL(1), ..., DL(n-2).
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, D must contain the diagonal elements of A.
*
* On exit, D is overwritten by the n diagonal elements of U.
*
* DU (input/output) DOUBLE PRECISION array, dimension (N-1)
* On entry, DU must contain the (n-1) super-diagonal elements
* of A.
*
* On exit, DU is overwritten by the (n-1) elements of the first
* super-diagonal of U.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the N by NRHS matrix of right hand side matrix B.
* On exit, if INFO = 0, the N by NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, U(i,i) is exactly zero, and the solution
* has not been computed. The factorization has not been
* completed unless i = N.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param nrhs
* @param dl
* @param _dl_offset
* @param d
* @param _d_offset
* @param du
* @param _du_offset
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void dgtsv(int n, int nrhs, double[] dl, int _dl_offset, double[] d, int _d_offset, double[] du, int _du_offset, double[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGTSVX uses the LU factorization to compute the solution to a real
* system of linear equations A * X = B or A**T * X = B,
* where A is a tridiagonal matrix of order N and X and B are N-by-NRHS
* matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed:
*
* 1. If FACT = 'N', the LU decomposition is used to factor the matrix A
* as A = L * U, where L is a product of permutation and unit lower
* bidiagonal matrices and U is upper triangular with nonzeros in
* only the main diagonal and first two superdiagonals.
*
* 2. If some U(i,i)=0, so that U is exactly singular, then the routine
* returns with INFO = i. Otherwise, the factored form of A is used
* to estimate the condition number of the matrix A. If the
* reciprocal of the condition number is less than machine precision,
* INFO = N+1 is returned as a warning, but the routine still goes on
* to solve for X and compute error bounds as described below.
*
* 3. The system of equations is solved for X using the factored form
* of A.
*
* 4. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of A has been
* supplied on entry.
* = 'F': DLF, DF, DUF, DU2, and IPIV contain the factored
* form of A; DL, D, DU, DLF, DF, DUF, DU2 and IPIV
* will not be modified.
* = 'N': The matrix will be copied to DLF, DF, and DUF
* and factored.
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* DL (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) subdiagonal elements of A.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The n diagonal elements of A.
*
* DU (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) superdiagonal elements of A.
*
* DLF (input or output) DOUBLE PRECISION array, dimension (N-1)
* If FACT = 'F', then DLF is an input argument and on entry
* contains the (n-1) multipliers that define the matrix L from
* the LU factorization of A as computed by DGTTRF.
*
* If FACT = 'N', then DLF is an output argument and on exit
* contains the (n-1) multipliers that define the matrix L from
* the LU factorization of A.
*
* DF (input or output) DOUBLE PRECISION array, dimension (N)
* If FACT = 'F', then DF is an input argument and on entry
* contains the n diagonal elements of the upper triangular
* matrix U from the LU factorization of A.
*
* If FACT = 'N', then DF is an output argument and on exit
* contains the n diagonal elements of the upper triangular
* matrix U from the LU factorization of A.
*
* DUF (input or output) DOUBLE PRECISION array, dimension (N-1)
* If FACT = 'F', then DUF is an input argument and on entry
* contains the (n-1) elements of the first superdiagonal of U.
*
* If FACT = 'N', then DUF is an output argument and on exit
* contains the (n-1) elements of the first superdiagonal of U.
*
* DU2 (input or output) DOUBLE PRECISION array, dimension (N-2)
* If FACT = 'F', then DU2 is an input argument and on entry
* contains the (n-2) elements of the second superdiagonal of
* U.
*
* If FACT = 'N', then DU2 is an output argument and on exit
* contains the (n-2) elements of the second superdiagonal of
* U.
*
* IPIV (input or output) INTEGER array, dimension (N)
* If FACT = 'F', then IPIV is an input argument and on entry
* contains the pivot indices from the LU factorization of A as
* computed by DGTTRF.
*
* If FACT = 'N', then IPIV is an output argument and on exit
* contains the pivot indices from the LU factorization of A;
* row i of the matrix was interchanged with row IPIV(i).
* IPIV(i) will always be either i or i+1; IPIV(i) = i indicates
* a row interchange was not required.
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The N-by-NRHS right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* If INFO = 0 or INFO = N+1, the N-by-NRHS solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) DOUBLE PRECISION
* The estimate of the reciprocal condition number of the matrix
* A. If RCOND is less than the machine precision (in
* particular, if RCOND = 0), the matrix is singular to working
* precision. This condition is indicated by a return code of
* INFO > 0.
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= N: U(i,i) is exactly zero. The factorization
* has not been completed unless i = N, but the
* factor U is exactly singular, so the solution
* and error bounds could not be computed.
* RCOND = 0 is returned.
* = N+1: U is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param trans
* @param n
* @param nrhs
* @param dl
* @param d
* @param du
* @param dlf
* @param df
* @param duf
* @param du2
* @param ipiv
* @param b
* @param ldb
* @param x
* @param ldx
* @param rcond
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void dgtsvx(java.lang.String fact, java.lang.String trans, int n, int nrhs, double[] dl, double[] d, double[] du, double[] dlf, double[] df, double[] duf, double[] du2, int[] ipiv, double[] b, int ldb, double[] x, int ldx, org.netlib.util.doubleW rcond, double[] ferr, double[] berr, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGTSVX uses the LU factorization to compute the solution to a real
* system of linear equations A * X = B or A**T * X = B,
* where A is a tridiagonal matrix of order N and X and B are N-by-NRHS
* matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed:
*
* 1. If FACT = 'N', the LU decomposition is used to factor the matrix A
* as A = L * U, where L is a product of permutation and unit lower
* bidiagonal matrices and U is upper triangular with nonzeros in
* only the main diagonal and first two superdiagonals.
*
* 2. If some U(i,i)=0, so that U is exactly singular, then the routine
* returns with INFO = i. Otherwise, the factored form of A is used
* to estimate the condition number of the matrix A. If the
* reciprocal of the condition number is less than machine precision,
* INFO = N+1 is returned as a warning, but the routine still goes on
* to solve for X and compute error bounds as described below.
*
* 3. The system of equations is solved for X using the factored form
* of A.
*
* 4. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of A has been
* supplied on entry.
* = 'F': DLF, DF, DUF, DU2, and IPIV contain the factored
* form of A; DL, D, DU, DLF, DF, DUF, DU2 and IPIV
* will not be modified.
* = 'N': The matrix will be copied to DLF, DF, and DUF
* and factored.
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* DL (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) subdiagonal elements of A.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The n diagonal elements of A.
*
* DU (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) superdiagonal elements of A.
*
* DLF (input or output) DOUBLE PRECISION array, dimension (N-1)
* If FACT = 'F', then DLF is an input argument and on entry
* contains the (n-1) multipliers that define the matrix L from
* the LU factorization of A as computed by DGTTRF.
*
* If FACT = 'N', then DLF is an output argument and on exit
* contains the (n-1) multipliers that define the matrix L from
* the LU factorization of A.
*
* DF (input or output) DOUBLE PRECISION array, dimension (N)
* If FACT = 'F', then DF is an input argument and on entry
* contains the n diagonal elements of the upper triangular
* matrix U from the LU factorization of A.
*
* If FACT = 'N', then DF is an output argument and on exit
* contains the n diagonal elements of the upper triangular
* matrix U from the LU factorization of A.
*
* DUF (input or output) DOUBLE PRECISION array, dimension (N-1)
* If FACT = 'F', then DUF is an input argument and on entry
* contains the (n-1) elements of the first superdiagonal of U.
*
* If FACT = 'N', then DUF is an output argument and on exit
* contains the (n-1) elements of the first superdiagonal of U.
*
* DU2 (input or output) DOUBLE PRECISION array, dimension (N-2)
* If FACT = 'F', then DU2 is an input argument and on entry
* contains the (n-2) elements of the second superdiagonal of
* U.
*
* If FACT = 'N', then DU2 is an output argument and on exit
* contains the (n-2) elements of the second superdiagonal of
* U.
*
* IPIV (input or output) INTEGER array, dimension (N)
* If FACT = 'F', then IPIV is an input argument and on entry
* contains the pivot indices from the LU factorization of A as
* computed by DGTTRF.
*
* If FACT = 'N', then IPIV is an output argument and on exit
* contains the pivot indices from the LU factorization of A;
* row i of the matrix was interchanged with row IPIV(i).
* IPIV(i) will always be either i or i+1; IPIV(i) = i indicates
* a row interchange was not required.
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The N-by-NRHS right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* If INFO = 0 or INFO = N+1, the N-by-NRHS solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) DOUBLE PRECISION
* The estimate of the reciprocal condition number of the matrix
* A. If RCOND is less than the machine precision (in
* particular, if RCOND = 0), the matrix is singular to working
* precision. This condition is indicated by a return code of
* INFO > 0.
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= N: U(i,i) is exactly zero. The factorization
* has not been completed unless i = N, but the
* factor U is exactly singular, so the solution
* and error bounds could not be computed.
* RCOND = 0 is returned.
* = N+1: U is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param trans
* @param n
* @param nrhs
* @param dl
* @param _dl_offset
* @param d
* @param _d_offset
* @param du
* @param _du_offset
* @param dlf
* @param _dlf_offset
* @param df
* @param _df_offset
* @param duf
* @param _duf_offset
* @param du2
* @param _du2_offset
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param rcond
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dgtsvx(java.lang.String fact, java.lang.String trans, int n, int nrhs, double[] dl, int _dl_offset, double[] d, int _d_offset, double[] du, int _du_offset, double[] dlf, int _dlf_offset, double[] df, int _df_offset, double[] duf, int _duf_offset, double[] du2, int _du2_offset, int[] ipiv, int _ipiv_offset, double[] b, int _b_offset, int ldb, double[] x, int _x_offset, int ldx, org.netlib.util.doubleW rcond, double[] ferr, int _ferr_offset, double[] berr, int _berr_offset, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGTTRF computes an LU factorization of a real tridiagonal matrix A
* using elimination with partial pivoting and row interchanges.
*
* The factorization has the form
* A = L * U
* where L is a product of permutation and unit lower bidiagonal
* matrices and U is upper triangular with nonzeros in only the main
* diagonal and first two superdiagonals.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A.
*
* DL (input/output) DOUBLE PRECISION array, dimension (N-1)
* On entry, DL must contain the (n-1) sub-diagonal elements of
* A.
*
* On exit, DL is overwritten by the (n-1) multipliers that
* define the matrix L from the LU factorization of A.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, D must contain the diagonal elements of A.
*
* On exit, D is overwritten by the n diagonal elements of the
* upper triangular matrix U from the LU factorization of A.
*
* DU (input/output) DOUBLE PRECISION array, dimension (N-1)
* On entry, DU must contain the (n-1) super-diagonal elements
* of A.
*
* On exit, DU is overwritten by the (n-1) elements of the first
* super-diagonal of U.
*
* DU2 (output) DOUBLE PRECISION array, dimension (N-2)
* On exit, DU2 is overwritten by the (n-2) elements of the
* second super-diagonal of U.
*
* IPIV (output) INTEGER array, dimension (N)
* The pivot indices; for 1 <= i <= n, row i of the matrix was
* interchanged with row IPIV(i). IPIV(i) will always be either
* i or i+1; IPIV(i) = i indicates a row interchange was not
* required.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
* > 0: if INFO = k, U(k,k) is exactly zero. The factorization
* has been completed, but the factor U is exactly
* singular, and division by zero will occur if it is used
* to solve a system of equations.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param dl
* @param d
* @param du
* @param du2
* @param ipiv
* @param info
*
*/
abstract public void dgttrf(int n, double[] dl, double[] d, double[] du, double[] du2, int[] ipiv, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGTTRF computes an LU factorization of a real tridiagonal matrix A
* using elimination with partial pivoting and row interchanges.
*
* The factorization has the form
* A = L * U
* where L is a product of permutation and unit lower bidiagonal
* matrices and U is upper triangular with nonzeros in only the main
* diagonal and first two superdiagonals.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A.
*
* DL (input/output) DOUBLE PRECISION array, dimension (N-1)
* On entry, DL must contain the (n-1) sub-diagonal elements of
* A.
*
* On exit, DL is overwritten by the (n-1) multipliers that
* define the matrix L from the LU factorization of A.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, D must contain the diagonal elements of A.
*
* On exit, D is overwritten by the n diagonal elements of the
* upper triangular matrix U from the LU factorization of A.
*
* DU (input/output) DOUBLE PRECISION array, dimension (N-1)
* On entry, DU must contain the (n-1) super-diagonal elements
* of A.
*
* On exit, DU is overwritten by the (n-1) elements of the first
* super-diagonal of U.
*
* DU2 (output) DOUBLE PRECISION array, dimension (N-2)
* On exit, DU2 is overwritten by the (n-2) elements of the
* second super-diagonal of U.
*
* IPIV (output) INTEGER array, dimension (N)
* The pivot indices; for 1 <= i <= n, row i of the matrix was
* interchanged with row IPIV(i). IPIV(i) will always be either
* i or i+1; IPIV(i) = i indicates a row interchange was not
* required.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
* > 0: if INFO = k, U(k,k) is exactly zero. The factorization
* has been completed, but the factor U is exactly
* singular, and division by zero will occur if it is used
* to solve a system of equations.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param dl
* @param _dl_offset
* @param d
* @param _d_offset
* @param du
* @param _du_offset
* @param du2
* @param _du2_offset
* @param ipiv
* @param _ipiv_offset
* @param info
*
*/
abstract public void dgttrf(int n, double[] dl, int _dl_offset, double[] d, int _d_offset, double[] du, int _du_offset, double[] du2, int _du2_offset, int[] ipiv, int _ipiv_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGTTRS solves one of the systems of equations
* A*X = B or A'*X = B,
* with a tridiagonal matrix A using the LU factorization computed
* by DGTTRF.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations.
* = 'N': A * X = B (No transpose)
* = 'T': A'* X = B (Transpose)
* = 'C': A'* X = B (Conjugate transpose = Transpose)
*
* N (input) INTEGER
* The order of the matrix A.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* DL (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) multipliers that define the matrix L from the
* LU factorization of A.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The n diagonal elements of the upper triangular matrix U from
* the LU factorization of A.
*
* DU (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) elements of the first super-diagonal of U.
*
* DU2 (input) DOUBLE PRECISION array, dimension (N-2)
* The (n-2) elements of the second super-diagonal of U.
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices; for 1 <= i <= n, row i of the matrix was
* interchanged with row IPIV(i). IPIV(i) will always be either
* i or i+1; IPIV(i) = i indicates a row interchange was not
* required.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the matrix of right hand side vectors B.
* On exit, B is overwritten by the solution vectors X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param trans
* @param n
* @param nrhs
* @param dl
* @param d
* @param du
* @param du2
* @param ipiv
* @param b
* @param ldb
* @param info
*
*/
abstract public void dgttrs(java.lang.String trans, int n, int nrhs, double[] dl, double[] d, double[] du, double[] du2, int[] ipiv, double[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGTTRS solves one of the systems of equations
* A*X = B or A'*X = B,
* with a tridiagonal matrix A using the LU factorization computed
* by DGTTRF.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations.
* = 'N': A * X = B (No transpose)
* = 'T': A'* X = B (Transpose)
* = 'C': A'* X = B (Conjugate transpose = Transpose)
*
* N (input) INTEGER
* The order of the matrix A.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* DL (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) multipliers that define the matrix L from the
* LU factorization of A.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The n diagonal elements of the upper triangular matrix U from
* the LU factorization of A.
*
* DU (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) elements of the first super-diagonal of U.
*
* DU2 (input) DOUBLE PRECISION array, dimension (N-2)
* The (n-2) elements of the second super-diagonal of U.
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices; for 1 <= i <= n, row i of the matrix was
* interchanged with row IPIV(i). IPIV(i) will always be either
* i or i+1; IPIV(i) = i indicates a row interchange was not
* required.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the matrix of right hand side vectors B.
* On exit, B is overwritten by the solution vectors X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param trans
* @param n
* @param nrhs
* @param dl
* @param _dl_offset
* @param d
* @param _d_offset
* @param du
* @param _du_offset
* @param du2
* @param _du2_offset
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void dgttrs(java.lang.String trans, int n, int nrhs, double[] dl, int _dl_offset, double[] d, int _d_offset, double[] du, int _du_offset, double[] du2, int _du2_offset, int[] ipiv, int _ipiv_offset, double[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DGTTS2 solves one of the systems of equations
* A*X = B or A'*X = B,
* with a tridiagonal matrix A using the LU factorization computed
* by DGTTRF.
*
* Arguments
* =========
*
* ITRANS (input) INTEGER
* Specifies the form of the system of equations.
* = 0: A * X = B (No transpose)
* = 1: A'* X = B (Transpose)
* = 2: A'* X = B (Conjugate transpose = Transpose)
*
* N (input) INTEGER
* The order of the matrix A.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* DL (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) multipliers that define the matrix L from the
* LU factorization of A.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The n diagonal elements of the upper triangular matrix U from
* the LU factorization of A.
*
* DU (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) elements of the first super-diagonal of U.
*
* DU2 (input) DOUBLE PRECISION array, dimension (N-2)
* The (n-2) elements of the second super-diagonal of U.
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices; for 1 <= i <= n, row i of the matrix was
* interchanged with row IPIV(i). IPIV(i) will always be either
* i or i+1; IPIV(i) = i indicates a row interchange was not
* required.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the matrix of right hand side vectors B.
* On exit, B is overwritten by the solution vectors X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param itrans
* @param n
* @param nrhs
* @param dl
* @param d
* @param du
* @param du2
* @param ipiv
* @param b
* @param ldb
*
*/
abstract public void dgtts2(int itrans, int n, int nrhs, double[] dl, double[] d, double[] du, double[] du2, int[] ipiv, double[] b, int ldb);
/**
*
* ..
*
* Purpose
* =======
*
* DGTTS2 solves one of the systems of equations
* A*X = B or A'*X = B,
* with a tridiagonal matrix A using the LU factorization computed
* by DGTTRF.
*
* Arguments
* =========
*
* ITRANS (input) INTEGER
* Specifies the form of the system of equations.
* = 0: A * X = B (No transpose)
* = 1: A'* X = B (Transpose)
* = 2: A'* X = B (Conjugate transpose = Transpose)
*
* N (input) INTEGER
* The order of the matrix A.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* DL (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) multipliers that define the matrix L from the
* LU factorization of A.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The n diagonal elements of the upper triangular matrix U from
* the LU factorization of A.
*
* DU (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) elements of the first super-diagonal of U.
*
* DU2 (input) DOUBLE PRECISION array, dimension (N-2)
* The (n-2) elements of the second super-diagonal of U.
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices; for 1 <= i <= n, row i of the matrix was
* interchanged with row IPIV(i). IPIV(i) will always be either
* i or i+1; IPIV(i) = i indicates a row interchange was not
* required.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the matrix of right hand side vectors B.
* On exit, B is overwritten by the solution vectors X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param itrans
* @param n
* @param nrhs
* @param dl
* @param _dl_offset
* @param d
* @param _d_offset
* @param du
* @param _du_offset
* @param du2
* @param _du2_offset
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
*
*/
abstract public void dgtts2(int itrans, int n, int nrhs, double[] dl, int _dl_offset, double[] d, int _d_offset, double[] du, int _du_offset, double[] du2, int _du2_offset, int[] ipiv, int _ipiv_offset, double[] b, int _b_offset, int ldb);
/**
*
* ..
*
* Purpose
* =======
*
* DHGEQZ computes the eigenvalues of a real matrix pair (H,T),
* where H is an upper Hessenberg matrix and T is upper triangular,
* using the double-shift QZ method.
* Matrix pairs of this type are produced by the reduction to
* generalized upper Hessenberg form of a real matrix pair (A,B):
*
* A = Q1*H*Z1**T, B = Q1*T*Z1**T,
*
* as computed by DGGHRD.
*
* If JOB='S', then the Hessenberg-triangular pair (H,T) is
* also reduced to generalized Schur form,
*
* H = Q*S*Z**T, T = Q*P*Z**T,
*
* where Q and Z are orthogonal matrices, P is an upper triangular
* matrix, and S is a quasi-triangular matrix with 1-by-1 and 2-by-2
* diagonal blocks.
*
* The 1-by-1 blocks correspond to real eigenvalues of the matrix pair
* (H,T) and the 2-by-2 blocks correspond to complex conjugate pairs of
* eigenvalues.
*
* Additionally, the 2-by-2 upper triangular diagonal blocks of P
* corresponding to 2-by-2 blocks of S are reduced to positive diagonal
* form, i.e., if S(j+1,j) is non-zero, then P(j+1,j) = P(j,j+1) = 0,
* P(j,j) > 0, and P(j+1,j+1) > 0.
*
* Optionally, the orthogonal matrix Q from the generalized Schur
* factorization may be postmultiplied into an input matrix Q1, and the
* orthogonal matrix Z may be postmultiplied into an input matrix Z1.
* If Q1 and Z1 are the orthogonal matrices from DGGHRD that reduced
* the matrix pair (A,B) to generalized upper Hessenberg form, then the
* output matrices Q1*Q and Z1*Z are the orthogonal factors from the
* generalized Schur factorization of (A,B):
*
* A = (Q1*Q)*S*(Z1*Z)**T, B = (Q1*Q)*P*(Z1*Z)**T.
*
* To avoid overflow, eigenvalues of the matrix pair (H,T) (equivalently
* of (A,B)) are computed as a pair of values (alpha,beta), where alpha
* complex and beta real.
* If beta is nonzero, lambda = alpha / beta is an eigenvalue of the
* generalized nonsymmetric eigenvalue problem (GNEP)
* A*x = lambda*B*x
* and if alpha is nonzero, mu = beta / alpha is an eigenvalue of the
* alternate form of the GNEP
* mu*A*y = B*y.
* Real eigenvalues can be read directly from the generalized Schur
* form:
* alpha = S(i,i), beta = P(i,i).
*
* Ref: C.B. Moler & G.W. Stewart, "An Algorithm for Generalized Matrix
* Eigenvalue Problems", SIAM J. Numer. Anal., 10(1973),
* pp. 241--256.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* = 'E': Compute eigenvalues only;
* = 'S': Compute eigenvalues and the Schur form.
*
* COMPQ (input) CHARACTER*1
* = 'N': Left Schur vectors (Q) are not computed;
* = 'I': Q is initialized to the unit matrix and the matrix Q
* of left Schur vectors of (H,T) is returned;
* = 'V': Q must contain an orthogonal matrix Q1 on entry and
* the product Q1*Q is returned.
*
* COMPZ (input) CHARACTER*1
* = 'N': Right Schur vectors (Z) are not computed;
* = 'I': Z is initialized to the unit matrix and the matrix Z
* of right Schur vectors of (H,T) is returned;
* = 'V': Z must contain an orthogonal matrix Z1 on entry and
* the product Z1*Z is returned.
*
* N (input) INTEGER
* The order of the matrices H, T, Q, and Z. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* ILO and IHI mark the rows and columns of H which are in
* Hessenberg form. It is assumed that A is already upper
* triangular in rows and columns 1:ILO-1 and IHI+1:N.
* If N > 0, 1 <= ILO <= IHI <= N; if N = 0, ILO=1 and IHI=0.
*
* H (input/output) DOUBLE PRECISION array, dimension (LDH, N)
* On entry, the N-by-N upper Hessenberg matrix H.
* On exit, if JOB = 'S', H contains the upper quasi-triangular
* matrix S from the generalized Schur factorization;
* 2-by-2 diagonal blocks (corresponding to complex conjugate
* pairs of eigenvalues) are returned in standard form, with
* H(i,i) = H(i+1,i+1) and H(i+1,i)*H(i,i+1) < 0.
* If JOB = 'E', the diagonal blocks of H match those of S, but
* the rest of H is unspecified.
*
* LDH (input) INTEGER
* The leading dimension of the array H. LDH >= max( 1, N ).
*
* T (input/output) DOUBLE PRECISION array, dimension (LDT, N)
* On entry, the N-by-N upper triangular matrix T.
* On exit, if JOB = 'S', T contains the upper triangular
* matrix P from the generalized Schur factorization;
* 2-by-2 diagonal blocks of P corresponding to 2-by-2 blocks of
* are reduced to positive diagonal form, i.e., if H(j+1,j) is
* non-zero, then T(j+1,j) = T(j,j+1) = 0, T(j,j) > 0, and
* T(j+1,j+1) > 0.
* If JOB = 'E', the diagonal blocks of T match those of P, but
* the rest of T is unspecified.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= max( 1, N ).
*
* ALPHAR (output) DOUBLE PRECISION array, dimension (N)
* The real parts of each scalar alpha defining an eigenvalue
* of GNEP.
*
* ALPHAI (output) DOUBLE PRECISION array, dimension (N)
* The imaginary parts of each scalar alpha defining an
* eigenvalue of GNEP.
* If ALPHAI(j) is zero, then the j-th eigenvalue is real; if
* positive, then the j-th and (j+1)-st eigenvalues are a
* complex conjugate pair, with ALPHAI(j+1) = -ALPHAI(j).
*
* BETA (output) DOUBLE PRECISION array, dimension (N)
* The scalars beta that define the eigenvalues of GNEP.
* Together, the quantities alpha = (ALPHAR(j),ALPHAI(j)) and
* beta = BETA(j) represent the j-th eigenvalue of the matrix
* pair (A,B), in one of the forms lambda = alpha/beta or
* mu = beta/alpha. Since either lambda or mu may overflow,
* they should not, in general, be computed.
*
* Q (input/output) DOUBLE PRECISION array, dimension (LDQ, N)
* On entry, if COMPZ = 'V', the orthogonal matrix Q1 used in
* the reduction of (A,B) to generalized Hessenberg form.
* On exit, if COMPZ = 'I', the orthogonal matrix of left Schur
* vectors of (H,T), and if COMPZ = 'V', the orthogonal matrix
* of left Schur vectors of (A,B).
* Not referenced if COMPZ = 'N'.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= 1.
* If COMPQ='V' or 'I', then LDQ >= N.
*
* Z (input/output) DOUBLE PRECISION array, dimension (LDZ, N)
* On entry, if COMPZ = 'V', the orthogonal matrix Z1 used in
* the reduction of (A,B) to generalized Hessenberg form.
* On exit, if COMPZ = 'I', the orthogonal matrix of
* right Schur vectors of (H,T), and if COMPZ = 'V', the
* orthogonal matrix of right Schur vectors of (A,B).
* Not referenced if COMPZ = 'N'.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1.
* If COMPZ='V' or 'I', then LDZ >= N.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO >= 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* = 1,...,N: the QZ iteration did not converge. (H,T) is not
* in Schur form, but ALPHAR(i), ALPHAI(i), and
* BETA(i), i=INFO+1,...,N should be correct.
* = N+1,...,2*N: the shift calculation failed. (H,T) is not
* in Schur form, but ALPHAR(i), ALPHAI(i), and
* BETA(i), i=INFO-N+1,...,N should be correct.
*
* Further Details
* ===============
*
* Iteration counters:
*
* JITER -- counts iterations.
* IITER -- counts iterations run since ILAST was last
* changed. This is therefore reset only when a 1-by-1 or
* 2-by-2 block deflates off the bottom.
*
* =====================================================================
*
* .. Parameters ..
* $ SAFETY = 1.0E+0 )
*
*
* @param job
* @param compq
* @param compz
* @param n
* @param ilo
* @param ihi
* @param h
* @param ldh
* @param t
* @param ldt
* @param alphar
* @param alphai
* @param beta
* @param q
* @param ldq
* @param z
* @param ldz
* @param work
* @param lwork
* @param info
*
*/
abstract public void dhgeqz(java.lang.String job, java.lang.String compq, java.lang.String compz, int n, int ilo, int ihi, double[] h, int ldh, double[] t, int ldt, double[] alphar, double[] alphai, double[] beta, double[] q, int ldq, double[] z, int ldz, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DHGEQZ computes the eigenvalues of a real matrix pair (H,T),
* where H is an upper Hessenberg matrix and T is upper triangular,
* using the double-shift QZ method.
* Matrix pairs of this type are produced by the reduction to
* generalized upper Hessenberg form of a real matrix pair (A,B):
*
* A = Q1*H*Z1**T, B = Q1*T*Z1**T,
*
* as computed by DGGHRD.
*
* If JOB='S', then the Hessenberg-triangular pair (H,T) is
* also reduced to generalized Schur form,
*
* H = Q*S*Z**T, T = Q*P*Z**T,
*
* where Q and Z are orthogonal matrices, P is an upper triangular
* matrix, and S is a quasi-triangular matrix with 1-by-1 and 2-by-2
* diagonal blocks.
*
* The 1-by-1 blocks correspond to real eigenvalues of the matrix pair
* (H,T) and the 2-by-2 blocks correspond to complex conjugate pairs of
* eigenvalues.
*
* Additionally, the 2-by-2 upper triangular diagonal blocks of P
* corresponding to 2-by-2 blocks of S are reduced to positive diagonal
* form, i.e., if S(j+1,j) is non-zero, then P(j+1,j) = P(j,j+1) = 0,
* P(j,j) > 0, and P(j+1,j+1) > 0.
*
* Optionally, the orthogonal matrix Q from the generalized Schur
* factorization may be postmultiplied into an input matrix Q1, and the
* orthogonal matrix Z may be postmultiplied into an input matrix Z1.
* If Q1 and Z1 are the orthogonal matrices from DGGHRD that reduced
* the matrix pair (A,B) to generalized upper Hessenberg form, then the
* output matrices Q1*Q and Z1*Z are the orthogonal factors from the
* generalized Schur factorization of (A,B):
*
* A = (Q1*Q)*S*(Z1*Z)**T, B = (Q1*Q)*P*(Z1*Z)**T.
*
* To avoid overflow, eigenvalues of the matrix pair (H,T) (equivalently
* of (A,B)) are computed as a pair of values (alpha,beta), where alpha
* complex and beta real.
* If beta is nonzero, lambda = alpha / beta is an eigenvalue of the
* generalized nonsymmetric eigenvalue problem (GNEP)
* A*x = lambda*B*x
* and if alpha is nonzero, mu = beta / alpha is an eigenvalue of the
* alternate form of the GNEP
* mu*A*y = B*y.
* Real eigenvalues can be read directly from the generalized Schur
* form:
* alpha = S(i,i), beta = P(i,i).
*
* Ref: C.B. Moler & G.W. Stewart, "An Algorithm for Generalized Matrix
* Eigenvalue Problems", SIAM J. Numer. Anal., 10(1973),
* pp. 241--256.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* = 'E': Compute eigenvalues only;
* = 'S': Compute eigenvalues and the Schur form.
*
* COMPQ (input) CHARACTER*1
* = 'N': Left Schur vectors (Q) are not computed;
* = 'I': Q is initialized to the unit matrix and the matrix Q
* of left Schur vectors of (H,T) is returned;
* = 'V': Q must contain an orthogonal matrix Q1 on entry and
* the product Q1*Q is returned.
*
* COMPZ (input) CHARACTER*1
* = 'N': Right Schur vectors (Z) are not computed;
* = 'I': Z is initialized to the unit matrix and the matrix Z
* of right Schur vectors of (H,T) is returned;
* = 'V': Z must contain an orthogonal matrix Z1 on entry and
* the product Z1*Z is returned.
*
* N (input) INTEGER
* The order of the matrices H, T, Q, and Z. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* ILO and IHI mark the rows and columns of H which are in
* Hessenberg form. It is assumed that A is already upper
* triangular in rows and columns 1:ILO-1 and IHI+1:N.
* If N > 0, 1 <= ILO <= IHI <= N; if N = 0, ILO=1 and IHI=0.
*
* H (input/output) DOUBLE PRECISION array, dimension (LDH, N)
* On entry, the N-by-N upper Hessenberg matrix H.
* On exit, if JOB = 'S', H contains the upper quasi-triangular
* matrix S from the generalized Schur factorization;
* 2-by-2 diagonal blocks (corresponding to complex conjugate
* pairs of eigenvalues) are returned in standard form, with
* H(i,i) = H(i+1,i+1) and H(i+1,i)*H(i,i+1) < 0.
* If JOB = 'E', the diagonal blocks of H match those of S, but
* the rest of H is unspecified.
*
* LDH (input) INTEGER
* The leading dimension of the array H. LDH >= max( 1, N ).
*
* T (input/output) DOUBLE PRECISION array, dimension (LDT, N)
* On entry, the N-by-N upper triangular matrix T.
* On exit, if JOB = 'S', T contains the upper triangular
* matrix P from the generalized Schur factorization;
* 2-by-2 diagonal blocks of P corresponding to 2-by-2 blocks of
* are reduced to positive diagonal form, i.e., if H(j+1,j) is
* non-zero, then T(j+1,j) = T(j,j+1) = 0, T(j,j) > 0, and
* T(j+1,j+1) > 0.
* If JOB = 'E', the diagonal blocks of T match those of P, but
* the rest of T is unspecified.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= max( 1, N ).
*
* ALPHAR (output) DOUBLE PRECISION array, dimension (N)
* The real parts of each scalar alpha defining an eigenvalue
* of GNEP.
*
* ALPHAI (output) DOUBLE PRECISION array, dimension (N)
* The imaginary parts of each scalar alpha defining an
* eigenvalue of GNEP.
* If ALPHAI(j) is zero, then the j-th eigenvalue is real; if
* positive, then the j-th and (j+1)-st eigenvalues are a
* complex conjugate pair, with ALPHAI(j+1) = -ALPHAI(j).
*
* BETA (output) DOUBLE PRECISION array, dimension (N)
* The scalars beta that define the eigenvalues of GNEP.
* Together, the quantities alpha = (ALPHAR(j),ALPHAI(j)) and
* beta = BETA(j) represent the j-th eigenvalue of the matrix
* pair (A,B), in one of the forms lambda = alpha/beta or
* mu = beta/alpha. Since either lambda or mu may overflow,
* they should not, in general, be computed.
*
* Q (input/output) DOUBLE PRECISION array, dimension (LDQ, N)
* On entry, if COMPZ = 'V', the orthogonal matrix Q1 used in
* the reduction of (A,B) to generalized Hessenberg form.
* On exit, if COMPZ = 'I', the orthogonal matrix of left Schur
* vectors of (H,T), and if COMPZ = 'V', the orthogonal matrix
* of left Schur vectors of (A,B).
* Not referenced if COMPZ = 'N'.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= 1.
* If COMPQ='V' or 'I', then LDQ >= N.
*
* Z (input/output) DOUBLE PRECISION array, dimension (LDZ, N)
* On entry, if COMPZ = 'V', the orthogonal matrix Z1 used in
* the reduction of (A,B) to generalized Hessenberg form.
* On exit, if COMPZ = 'I', the orthogonal matrix of
* right Schur vectors of (H,T), and if COMPZ = 'V', the
* orthogonal matrix of right Schur vectors of (A,B).
* Not referenced if COMPZ = 'N'.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1.
* If COMPZ='V' or 'I', then LDZ >= N.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO >= 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* = 1,...,N: the QZ iteration did not converge. (H,T) is not
* in Schur form, but ALPHAR(i), ALPHAI(i), and
* BETA(i), i=INFO+1,...,N should be correct.
* = N+1,...,2*N: the shift calculation failed. (H,T) is not
* in Schur form, but ALPHAR(i), ALPHAI(i), and
* BETA(i), i=INFO-N+1,...,N should be correct.
*
* Further Details
* ===============
*
* Iteration counters:
*
* JITER -- counts iterations.
* IITER -- counts iterations run since ILAST was last
* changed. This is therefore reset only when a 1-by-1 or
* 2-by-2 block deflates off the bottom.
*
* =====================================================================
*
* .. Parameters ..
* $ SAFETY = 1.0E+0 )
*
*
* @param job
* @param compq
* @param compz
* @param n
* @param ilo
* @param ihi
* @param h
* @param _h_offset
* @param ldh
* @param t
* @param _t_offset
* @param ldt
* @param alphar
* @param _alphar_offset
* @param alphai
* @param _alphai_offset
* @param beta
* @param _beta_offset
* @param q
* @param _q_offset
* @param ldq
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dhgeqz(java.lang.String job, java.lang.String compq, java.lang.String compz, int n, int ilo, int ihi, double[] h, int _h_offset, int ldh, double[] t, int _t_offset, int ldt, double[] alphar, int _alphar_offset, double[] alphai, int _alphai_offset, double[] beta, int _beta_offset, double[] q, int _q_offset, int ldq, double[] z, int _z_offset, int ldz, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DHSEIN uses inverse iteration to find specified right and/or left
* eigenvectors of a real upper Hessenberg matrix H.
*
* The right eigenvector x and the left eigenvector y of the matrix H
* corresponding to an eigenvalue w are defined by:
*
* H * x = w * x, y**h * H = w * y**h
*
* where y**h denotes the conjugate transpose of the vector y.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'R': compute right eigenvectors only;
* = 'L': compute left eigenvectors only;
* = 'B': compute both right and left eigenvectors.
*
* EIGSRC (input) CHARACTER*1
* Specifies the source of eigenvalues supplied in (WR,WI):
* = 'Q': the eigenvalues were found using DHSEQR; thus, if
* H has zero subdiagonal elements, and so is
* block-triangular, then the j-th eigenvalue can be
* assumed to be an eigenvalue of the block containing
* the j-th row/column. This property allows DHSEIN to
* perform inverse iteration on just one diagonal block.
* = 'N': no assumptions are made on the correspondence
* between eigenvalues and diagonal blocks. In this
* case, DHSEIN must always perform inverse iteration
* using the whole matrix H.
*
* INITV (input) CHARACTER*1
* = 'N': no initial vectors are supplied;
* = 'U': user-supplied initial vectors are stored in the arrays
* VL and/or VR.
*
* SELECT (input/output) LOGICAL array, dimension (N)
* Specifies the eigenvectors to be computed. To select the
* real eigenvector corresponding to a real eigenvalue WR(j),
* SELECT(j) must be set to .TRUE.. To select the complex
* eigenvector corresponding to a complex eigenvalue
* (WR(j),WI(j)), with complex conjugate (WR(j+1),WI(j+1)),
* either SELECT(j) or SELECT(j+1) or both must be set to
* .TRUE.; then on exit SELECT(j) is .TRUE. and SELECT(j+1) is
* .FALSE..
*
* N (input) INTEGER
* The order of the matrix H. N >= 0.
*
* H (input) DOUBLE PRECISION array, dimension (LDH,N)
* The upper Hessenberg matrix H.
*
* LDH (input) INTEGER
* The leading dimension of the array H. LDH >= max(1,N).
*
* WR (input/output) DOUBLE PRECISION array, dimension (N)
* WI (input) DOUBLE PRECISION array, dimension (N)
* On entry, the real and imaginary parts of the eigenvalues of
* H; a complex conjugate pair of eigenvalues must be stored in
* consecutive elements of WR and WI.
* On exit, WR may have been altered since close eigenvalues
* are perturbed slightly in searching for independent
* eigenvectors.
*
* VL (input/output) DOUBLE PRECISION array, dimension (LDVL,MM)
* On entry, if INITV = 'U' and SIDE = 'L' or 'B', VL must
* contain starting vectors for the inverse iteration for the
* left eigenvectors; the starting vector for each eigenvector
* must be in the same column(s) in which the eigenvector will
* be stored.
* On exit, if SIDE = 'L' or 'B', the left eigenvectors
* specified by SELECT will be stored consecutively in the
* columns of VL, in the same order as their eigenvalues. A
* complex eigenvector corresponding to a complex eigenvalue is
* stored in two consecutive columns, the first holding the real
* part and the second the imaginary part.
* If SIDE = 'R', VL is not referenced.
*
* LDVL (input) INTEGER
* The leading dimension of the array VL.
* LDVL >= max(1,N) if SIDE = 'L' or 'B'; LDVL >= 1 otherwise.
*
* VR (input/output) DOUBLE PRECISION array, dimension (LDVR,MM)
* On entry, if INITV = 'U' and SIDE = 'R' or 'B', VR must
* contain starting vectors for the inverse iteration for the
* right eigenvectors; the starting vector for each eigenvector
* must be in the same column(s) in which the eigenvector will
* be stored.
* On exit, if SIDE = 'R' or 'B', the right eigenvectors
* specified by SELECT will be stored consecutively in the
* columns of VR, in the same order as their eigenvalues. A
* complex eigenvector corresponding to a complex eigenvalue is
* stored in two consecutive columns, the first holding the real
* part and the second the imaginary part.
* If SIDE = 'L', VR is not referenced.
*
* LDVR (input) INTEGER
* The leading dimension of the array VR.
* LDVR >= max(1,N) if SIDE = 'R' or 'B'; LDVR >= 1 otherwise.
*
* MM (input) INTEGER
* The number of columns in the arrays VL and/or VR. MM >= M.
*
* M (output) INTEGER
* The number of columns in the arrays VL and/or VR required to
* store the eigenvectors; each selected real eigenvector
* occupies one column and each selected complex eigenvector
* occupies two columns.
*
* WORK (workspace) DOUBLE PRECISION array, dimension ((N+2)*N)
*
* IFAILL (output) INTEGER array, dimension (MM)
* If SIDE = 'L' or 'B', IFAILL(i) = j > 0 if the left
* eigenvector in the i-th column of VL (corresponding to the
* eigenvalue w(j)) failed to converge; IFAILL(i) = 0 if the
* eigenvector converged satisfactorily. If the i-th and (i+1)th
* columns of VL hold a complex eigenvector, then IFAILL(i) and
* IFAILL(i+1) are set to the same value.
* If SIDE = 'R', IFAILL is not referenced.
*
* IFAILR (output) INTEGER array, dimension (MM)
* If SIDE = 'R' or 'B', IFAILR(i) = j > 0 if the right
* eigenvector in the i-th column of VR (corresponding to the
* eigenvalue w(j)) failed to converge; IFAILR(i) = 0 if the
* eigenvector converged satisfactorily. If the i-th and (i+1)th
* columns of VR hold a complex eigenvector, then IFAILR(i) and
* IFAILR(i+1) are set to the same value.
* If SIDE = 'L', IFAILR is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, i is the number of eigenvectors which
* failed to converge; see IFAILL and IFAILR for further
* details.
*
* Further Details
* ===============
*
* Each eigenvector is normalized so that the element of largest
* magnitude has magnitude 1; here the magnitude of a complex number
* (x,y) is taken to be |x|+|y|.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param eigsrc
* @param initv
* @param select
* @param n
* @param h
* @param ldh
* @param wr
* @param wi
* @param vl
* @param ldvl
* @param vr
* @param ldvr
* @param mm
* @param m
* @param work
* @param ifaill
* @param ifailr
* @param info
*
*/
abstract public void dhsein(java.lang.String side, java.lang.String eigsrc, java.lang.String initv, boolean[] select, int n, double[] h, int ldh, double[] wr, double[] wi, double[] vl, int ldvl, double[] vr, int ldvr, int mm, org.netlib.util.intW m, double[] work, int[] ifaill, int[] ifailr, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DHSEIN uses inverse iteration to find specified right and/or left
* eigenvectors of a real upper Hessenberg matrix H.
*
* The right eigenvector x and the left eigenvector y of the matrix H
* corresponding to an eigenvalue w are defined by:
*
* H * x = w * x, y**h * H = w * y**h
*
* where y**h denotes the conjugate transpose of the vector y.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'R': compute right eigenvectors only;
* = 'L': compute left eigenvectors only;
* = 'B': compute both right and left eigenvectors.
*
* EIGSRC (input) CHARACTER*1
* Specifies the source of eigenvalues supplied in (WR,WI):
* = 'Q': the eigenvalues were found using DHSEQR; thus, if
* H has zero subdiagonal elements, and so is
* block-triangular, then the j-th eigenvalue can be
* assumed to be an eigenvalue of the block containing
* the j-th row/column. This property allows DHSEIN to
* perform inverse iteration on just one diagonal block.
* = 'N': no assumptions are made on the correspondence
* between eigenvalues and diagonal blocks. In this
* case, DHSEIN must always perform inverse iteration
* using the whole matrix H.
*
* INITV (input) CHARACTER*1
* = 'N': no initial vectors are supplied;
* = 'U': user-supplied initial vectors are stored in the arrays
* VL and/or VR.
*
* SELECT (input/output) LOGICAL array, dimension (N)
* Specifies the eigenvectors to be computed. To select the
* real eigenvector corresponding to a real eigenvalue WR(j),
* SELECT(j) must be set to .TRUE.. To select the complex
* eigenvector corresponding to a complex eigenvalue
* (WR(j),WI(j)), with complex conjugate (WR(j+1),WI(j+1)),
* either SELECT(j) or SELECT(j+1) or both must be set to
* .TRUE.; then on exit SELECT(j) is .TRUE. and SELECT(j+1) is
* .FALSE..
*
* N (input) INTEGER
* The order of the matrix H. N >= 0.
*
* H (input) DOUBLE PRECISION array, dimension (LDH,N)
* The upper Hessenberg matrix H.
*
* LDH (input) INTEGER
* The leading dimension of the array H. LDH >= max(1,N).
*
* WR (input/output) DOUBLE PRECISION array, dimension (N)
* WI (input) DOUBLE PRECISION array, dimension (N)
* On entry, the real and imaginary parts of the eigenvalues of
* H; a complex conjugate pair of eigenvalues must be stored in
* consecutive elements of WR and WI.
* On exit, WR may have been altered since close eigenvalues
* are perturbed slightly in searching for independent
* eigenvectors.
*
* VL (input/output) DOUBLE PRECISION array, dimension (LDVL,MM)
* On entry, if INITV = 'U' and SIDE = 'L' or 'B', VL must
* contain starting vectors for the inverse iteration for the
* left eigenvectors; the starting vector for each eigenvector
* must be in the same column(s) in which the eigenvector will
* be stored.
* On exit, if SIDE = 'L' or 'B', the left eigenvectors
* specified by SELECT will be stored consecutively in the
* columns of VL, in the same order as their eigenvalues. A
* complex eigenvector corresponding to a complex eigenvalue is
* stored in two consecutive columns, the first holding the real
* part and the second the imaginary part.
* If SIDE = 'R', VL is not referenced.
*
* LDVL (input) INTEGER
* The leading dimension of the array VL.
* LDVL >= max(1,N) if SIDE = 'L' or 'B'; LDVL >= 1 otherwise.
*
* VR (input/output) DOUBLE PRECISION array, dimension (LDVR,MM)
* On entry, if INITV = 'U' and SIDE = 'R' or 'B', VR must
* contain starting vectors for the inverse iteration for the
* right eigenvectors; the starting vector for each eigenvector
* must be in the same column(s) in which the eigenvector will
* be stored.
* On exit, if SIDE = 'R' or 'B', the right eigenvectors
* specified by SELECT will be stored consecutively in the
* columns of VR, in the same order as their eigenvalues. A
* complex eigenvector corresponding to a complex eigenvalue is
* stored in two consecutive columns, the first holding the real
* part and the second the imaginary part.
* If SIDE = 'L', VR is not referenced.
*
* LDVR (input) INTEGER
* The leading dimension of the array VR.
* LDVR >= max(1,N) if SIDE = 'R' or 'B'; LDVR >= 1 otherwise.
*
* MM (input) INTEGER
* The number of columns in the arrays VL and/or VR. MM >= M.
*
* M (output) INTEGER
* The number of columns in the arrays VL and/or VR required to
* store the eigenvectors; each selected real eigenvector
* occupies one column and each selected complex eigenvector
* occupies two columns.
*
* WORK (workspace) DOUBLE PRECISION array, dimension ((N+2)*N)
*
* IFAILL (output) INTEGER array, dimension (MM)
* If SIDE = 'L' or 'B', IFAILL(i) = j > 0 if the left
* eigenvector in the i-th column of VL (corresponding to the
* eigenvalue w(j)) failed to converge; IFAILL(i) = 0 if the
* eigenvector converged satisfactorily. If the i-th and (i+1)th
* columns of VL hold a complex eigenvector, then IFAILL(i) and
* IFAILL(i+1) are set to the same value.
* If SIDE = 'R', IFAILL is not referenced.
*
* IFAILR (output) INTEGER array, dimension (MM)
* If SIDE = 'R' or 'B', IFAILR(i) = j > 0 if the right
* eigenvector in the i-th column of VR (corresponding to the
* eigenvalue w(j)) failed to converge; IFAILR(i) = 0 if the
* eigenvector converged satisfactorily. If the i-th and (i+1)th
* columns of VR hold a complex eigenvector, then IFAILR(i) and
* IFAILR(i+1) are set to the same value.
* If SIDE = 'L', IFAILR is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, i is the number of eigenvectors which
* failed to converge; see IFAILL and IFAILR for further
* details.
*
* Further Details
* ===============
*
* Each eigenvector is normalized so that the element of largest
* magnitude has magnitude 1; here the magnitude of a complex number
* (x,y) is taken to be |x|+|y|.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param eigsrc
* @param initv
* @param select
* @param _select_offset
* @param n
* @param h
* @param _h_offset
* @param ldh
* @param wr
* @param _wr_offset
* @param wi
* @param _wi_offset
* @param vl
* @param _vl_offset
* @param ldvl
* @param vr
* @param _vr_offset
* @param ldvr
* @param mm
* @param m
* @param work
* @param _work_offset
* @param ifaill
* @param _ifaill_offset
* @param ifailr
* @param _ifailr_offset
* @param info
*
*/
abstract public void dhsein(java.lang.String side, java.lang.String eigsrc, java.lang.String initv, boolean[] select, int _select_offset, int n, double[] h, int _h_offset, int ldh, double[] wr, int _wr_offset, double[] wi, int _wi_offset, double[] vl, int _vl_offset, int ldvl, double[] vr, int _vr_offset, int ldvr, int mm, org.netlib.util.intW m, double[] work, int _work_offset, int[] ifaill, int _ifaill_offset, int[] ifailr, int _ifailr_offset, org.netlib.util.intW info);
/**
*
* ..
* Purpose
* =======
*
* DHSEQR computes the eigenvalues of a Hessenberg matrix H
* and, optionally, the matrices T and Z from the Schur decomposition
* H = Z T Z**T, where T is an upper quasi-triangular matrix (the
* Schur form), and Z is the orthogonal matrix of Schur vectors.
*
* Optionally Z may be postmultiplied into an input orthogonal
* matrix Q so that this routine can give the Schur factorization
* of a matrix A which has been reduced to the Hessenberg form H
* by the orthogonal matrix Q: A = Q*H*Q**T = (QZ)*T*(QZ)**T.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* = 'E': compute eigenvalues only;
* = 'S': compute eigenvalues and the Schur form T.
*
* COMPZ (input) CHARACTER*1
* = 'N': no Schur vectors are computed;
* = 'I': Z is initialized to the unit matrix and the matrix Z
* of Schur vectors of H is returned;
* = 'V': Z must contain an orthogonal matrix Q on entry, and
* the product Q*Z is returned.
*
* N (input) INTEGER
* The order of the matrix H. N .GE. 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* It is assumed that H is already upper triangular in rows
* and columns 1:ILO-1 and IHI+1:N. ILO and IHI are normally
* set by a previous call to DGEBAL, and then passed to DGEHRD
* when the matrix output by DGEBAL is reduced to Hessenberg
* form. Otherwise ILO and IHI should be set to 1 and N
* respectively. If N.GT.0, then 1.LE.ILO.LE.IHI.LE.N.
* If N = 0, then ILO = 1 and IHI = 0.
*
* H (input/output) DOUBLE PRECISION array, dimension (LDH,N)
* On entry, the upper Hessenberg matrix H.
* On exit, if INFO = 0 and JOB = 'S', then H contains the
* upper quasi-triangular matrix T from the Schur decomposition
* (the Schur form); 2-by-2 diagonal blocks (corresponding to
* complex conjugate pairs of eigenvalues) are returned in
* standard form, with H(i,i) = H(i+1,i+1) and
* H(i+1,i)*H(i,i+1).LT.0. If INFO = 0 and JOB = 'E', the
* contents of H are unspecified on exit. (The output value of
* H when INFO.GT.0 is given under the description of INFO
* below.)
*
* Unlike earlier versions of DHSEQR, this subroutine may
* explicitly H(i,j) = 0 for i.GT.j and j = 1, 2, ... ILO-1
* or j = IHI+1, IHI+2, ... N.
*
* LDH (input) INTEGER
* The leading dimension of the array H. LDH .GE. max(1,N).
*
* WR (output) DOUBLE PRECISION array, dimension (N)
* WI (output) DOUBLE PRECISION array, dimension (N)
* The real and imaginary parts, respectively, of the computed
* eigenvalues. If two eigenvalues are computed as a complex
* conjugate pair, they are stored in consecutive elements of
* WR and WI, say the i-th and (i+1)th, with WI(i) .GT. 0 and
* WI(i+1) .LT. 0. If JOB = 'S', the eigenvalues are stored in
* the same order as on the diagonal of the Schur form returned
* in H, with WR(i) = H(i,i) and, if H(i:i+1,i:i+1) is a 2-by-2
* diagonal block, WI(i) = sqrt(-H(i+1,i)*H(i,i+1)) and
* WI(i+1) = -WI(i).
*
* Z (input/output) DOUBLE PRECISION array, dimension (LDZ,N)
* If COMPZ = 'N', Z is not referenced.
* If COMPZ = 'I', on entry Z need not be set and on exit,
* if INFO = 0, Z contains the orthogonal matrix Z of the Schur
* vectors of H. If COMPZ = 'V', on entry Z must contain an
* N-by-N matrix Q, which is assumed to be equal to the unit
* matrix except for the submatrix Z(ILO:IHI,ILO:IHI). On exit,
* if INFO = 0, Z contains Q*Z.
* Normally Q is the orthogonal matrix generated by DORGHR
* after the call to DGEHRD which formed the Hessenberg matrix
* H. (The output value of Z when INFO.GT.0 is given under
* the description of INFO below.)
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. if COMPZ = 'I' or
* COMPZ = 'V', then LDZ.GE.MAX(1,N). Otherwize, LDZ.GE.1.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (LWORK)
* On exit, if INFO = 0, WORK(1) returns an estimate of
* the optimal value for LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK .GE. max(1,N)
* is sufficient, but LWORK typically as large as 6*N may
* be required for optimal performance. A workspace query
* to determine the optimal workspace size is recommended.
*
* If LWORK = -1, then DHSEQR does a workspace query.
* In this case, DHSEQR checks the input parameters and
* estimates the optimal workspace size for the given
* values of N, ILO and IHI. The estimate is returned
* in WORK(1). No error message related to LWORK is
* issued by XERBLA. Neither H nor Z are accessed.
*
*
* INFO (output) INTEGER
* = 0: successful exit
* .LT. 0: if INFO = -i, the i-th argument had an illegal
* value
* .GT. 0: if INFO = i, DHSEQR failed to compute all of
* the eigenvalues. Elements 1:ilo-1 and i+1:n of WR
* and WI contain those eigenvalues which have been
* successfully computed. (Failures are rare.)
*
* If INFO .GT. 0 and JOB = 'E', then on exit, the
* remaining unconverged eigenvalues are the eigen-
* values of the upper Hessenberg matrix rows and
* columns ILO through INFO of the final, output
* value of H.
*
* If INFO .GT. 0 and JOB = 'S', then on exit
*
* (*) (initial value of H)*U = U*(final value of H)
*
* where U is an orthogonal matrix. The final
* value of H is upper Hessenberg and quasi-triangular
* in rows and columns INFO+1 through IHI.
*
* If INFO .GT. 0 and COMPZ = 'V', then on exit
*
* (final value of Z) = (initial value of Z)*U
*
* where U is the orthogonal matrix in (*) (regard-
* less of the value of JOB.)
*
* If INFO .GT. 0 and COMPZ = 'I', then on exit
* (final value of Z) = U
* where U is the orthogonal matrix in (*) (regard-
* less of the value of JOB.)
*
* If INFO .GT. 0 and COMPZ = 'N', then Z is not
* accessed.
*
* ================================================================
* Default values supplied by
* ILAENV(ISPEC,'DHSEQR',JOB(:1)//COMPZ(:1),N,ILO,IHI,LWORK).
* It is suggested that these defaults be adjusted in order
* to attain best performance in each particular
* computational environment.
*
* ISPEC=1: The DLAHQR vs DLAQR0 crossover point.
* Default: 75. (Must be at least 11.)
*
* ISPEC=2: Recommended deflation window size.
* This depends on ILO, IHI and NS. NS is the
* number of simultaneous shifts returned
* by ILAENV(ISPEC=4). (See ISPEC=4 below.)
* The default for (IHI-ILO+1).LE.500 is NS.
* The default for (IHI-ILO+1).GT.500 is 3*NS/2.
*
* ISPEC=3: Nibble crossover point. (See ILAENV for
* details.) Default: 14% of deflation window
* size.
*
* ISPEC=4: Number of simultaneous shifts, NS, in
* a multi-shift QR iteration.
*
* If IHI-ILO+1 is ...
*
* greater than ...but less ... the
* or equal to ... than default is
*
* 1 30 NS - 2(+)
* 30 60 NS - 4(+)
* 60 150 NS = 10(+)
* 150 590 NS = **
* 590 3000 NS = 64
* 3000 6000 NS = 128
* 6000 infinity NS = 256
*
* (+) By default some or all matrices of this order
* are passed to the implicit double shift routine
* DLAHQR and NS is ignored. See ISPEC=1 above
* and comments in IPARM for details.
*
* The asterisks (**) indicate an ad-hoc
* function of N increasing from 10 to 64.
*
* ISPEC=5: Select structured matrix multiply.
* (See ILAENV for details.) Default: 3.
*
* ================================================================
* Based on contributions by
* Karen Braman and Ralph Byers, Department of Mathematics,
* University of Kansas, USA
*
* ================================================================
* References:
* K. Braman, R. Byers and R. Mathias, The Multi-Shift QR
* Algorithm Part I: Maintaining Well Focused Shifts, and Level 3
* Performance, SIAM Journal of Matrix Analysis, volume 23, pages
* 929--947, 2002.
*
* K. Braman, R. Byers and R. Mathias, The Multi-Shift QR
* Algorithm Part II: Aggressive Early Deflation, SIAM Journal
* of Matrix Analysis, volume 23, pages 948--973, 2002.
*
* ================================================================
* .. Parameters ..
*
* ==== Matrices of order NTINY or smaller must be processed by
* . DLAHQR because of insufficient subdiagonal scratch space.
* . (This is a hard limit.) ====
*
* ==== NL allocates some local workspace to help small matrices
* . through a rare DLAHQR failure. NL .GT. NTINY = 11 is
* . required and NL .LE. NMIN = ILAENV(ISPEC=1,...) is recom-
* . mended. (The default value of NMIN is 75.) Using NL = 49
* . allows up to six simultaneous shifts and a 16-by-16
* . deflation window. ====
*
*
*
* @param job
* @param compz
* @param n
* @param ilo
* @param ihi
* @param h
* @param ldh
* @param wr
* @param wi
* @param z
* @param ldz
* @param work
* @param lwork
* @param info
*
*/
abstract public void dhseqr(java.lang.String job, java.lang.String compz, int n, int ilo, int ihi, double[] h, int ldh, double[] wr, double[] wi, double[] z, int ldz, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
* Purpose
* =======
*
* DHSEQR computes the eigenvalues of a Hessenberg matrix H
* and, optionally, the matrices T and Z from the Schur decomposition
* H = Z T Z**T, where T is an upper quasi-triangular matrix (the
* Schur form), and Z is the orthogonal matrix of Schur vectors.
*
* Optionally Z may be postmultiplied into an input orthogonal
* matrix Q so that this routine can give the Schur factorization
* of a matrix A which has been reduced to the Hessenberg form H
* by the orthogonal matrix Q: A = Q*H*Q**T = (QZ)*T*(QZ)**T.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* = 'E': compute eigenvalues only;
* = 'S': compute eigenvalues and the Schur form T.
*
* COMPZ (input) CHARACTER*1
* = 'N': no Schur vectors are computed;
* = 'I': Z is initialized to the unit matrix and the matrix Z
* of Schur vectors of H is returned;
* = 'V': Z must contain an orthogonal matrix Q on entry, and
* the product Q*Z is returned.
*
* N (input) INTEGER
* The order of the matrix H. N .GE. 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* It is assumed that H is already upper triangular in rows
* and columns 1:ILO-1 and IHI+1:N. ILO and IHI are normally
* set by a previous call to DGEBAL, and then passed to DGEHRD
* when the matrix output by DGEBAL is reduced to Hessenberg
* form. Otherwise ILO and IHI should be set to 1 and N
* respectively. If N.GT.0, then 1.LE.ILO.LE.IHI.LE.N.
* If N = 0, then ILO = 1 and IHI = 0.
*
* H (input/output) DOUBLE PRECISION array, dimension (LDH,N)
* On entry, the upper Hessenberg matrix H.
* On exit, if INFO = 0 and JOB = 'S', then H contains the
* upper quasi-triangular matrix T from the Schur decomposition
* (the Schur form); 2-by-2 diagonal blocks (corresponding to
* complex conjugate pairs of eigenvalues) are returned in
* standard form, with H(i,i) = H(i+1,i+1) and
* H(i+1,i)*H(i,i+1).LT.0. If INFO = 0 and JOB = 'E', the
* contents of H are unspecified on exit. (The output value of
* H when INFO.GT.0 is given under the description of INFO
* below.)
*
* Unlike earlier versions of DHSEQR, this subroutine may
* explicitly H(i,j) = 0 for i.GT.j and j = 1, 2, ... ILO-1
* or j = IHI+1, IHI+2, ... N.
*
* LDH (input) INTEGER
* The leading dimension of the array H. LDH .GE. max(1,N).
*
* WR (output) DOUBLE PRECISION array, dimension (N)
* WI (output) DOUBLE PRECISION array, dimension (N)
* The real and imaginary parts, respectively, of the computed
* eigenvalues. If two eigenvalues are computed as a complex
* conjugate pair, they are stored in consecutive elements of
* WR and WI, say the i-th and (i+1)th, with WI(i) .GT. 0 and
* WI(i+1) .LT. 0. If JOB = 'S', the eigenvalues are stored in
* the same order as on the diagonal of the Schur form returned
* in H, with WR(i) = H(i,i) and, if H(i:i+1,i:i+1) is a 2-by-2
* diagonal block, WI(i) = sqrt(-H(i+1,i)*H(i,i+1)) and
* WI(i+1) = -WI(i).
*
* Z (input/output) DOUBLE PRECISION array, dimension (LDZ,N)
* If COMPZ = 'N', Z is not referenced.
* If COMPZ = 'I', on entry Z need not be set and on exit,
* if INFO = 0, Z contains the orthogonal matrix Z of the Schur
* vectors of H. If COMPZ = 'V', on entry Z must contain an
* N-by-N matrix Q, which is assumed to be equal to the unit
* matrix except for the submatrix Z(ILO:IHI,ILO:IHI). On exit,
* if INFO = 0, Z contains Q*Z.
* Normally Q is the orthogonal matrix generated by DORGHR
* after the call to DGEHRD which formed the Hessenberg matrix
* H. (The output value of Z when INFO.GT.0 is given under
* the description of INFO below.)
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. if COMPZ = 'I' or
* COMPZ = 'V', then LDZ.GE.MAX(1,N). Otherwize, LDZ.GE.1.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (LWORK)
* On exit, if INFO = 0, WORK(1) returns an estimate of
* the optimal value for LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK .GE. max(1,N)
* is sufficient, but LWORK typically as large as 6*N may
* be required for optimal performance. A workspace query
* to determine the optimal workspace size is recommended.
*
* If LWORK = -1, then DHSEQR does a workspace query.
* In this case, DHSEQR checks the input parameters and
* estimates the optimal workspace size for the given
* values of N, ILO and IHI. The estimate is returned
* in WORK(1). No error message related to LWORK is
* issued by XERBLA. Neither H nor Z are accessed.
*
*
* INFO (output) INTEGER
* = 0: successful exit
* .LT. 0: if INFO = -i, the i-th argument had an illegal
* value
* .GT. 0: if INFO = i, DHSEQR failed to compute all of
* the eigenvalues. Elements 1:ilo-1 and i+1:n of WR
* and WI contain those eigenvalues which have been
* successfully computed. (Failures are rare.)
*
* If INFO .GT. 0 and JOB = 'E', then on exit, the
* remaining unconverged eigenvalues are the eigen-
* values of the upper Hessenberg matrix rows and
* columns ILO through INFO of the final, output
* value of H.
*
* If INFO .GT. 0 and JOB = 'S', then on exit
*
* (*) (initial value of H)*U = U*(final value of H)
*
* where U is an orthogonal matrix. The final
* value of H is upper Hessenberg and quasi-triangular
* in rows and columns INFO+1 through IHI.
*
* If INFO .GT. 0 and COMPZ = 'V', then on exit
*
* (final value of Z) = (initial value of Z)*U
*
* where U is the orthogonal matrix in (*) (regard-
* less of the value of JOB.)
*
* If INFO .GT. 0 and COMPZ = 'I', then on exit
* (final value of Z) = U
* where U is the orthogonal matrix in (*) (regard-
* less of the value of JOB.)
*
* If INFO .GT. 0 and COMPZ = 'N', then Z is not
* accessed.
*
* ================================================================
* Default values supplied by
* ILAENV(ISPEC,'DHSEQR',JOB(:1)//COMPZ(:1),N,ILO,IHI,LWORK).
* It is suggested that these defaults be adjusted in order
* to attain best performance in each particular
* computational environment.
*
* ISPEC=1: The DLAHQR vs DLAQR0 crossover point.
* Default: 75. (Must be at least 11.)
*
* ISPEC=2: Recommended deflation window size.
* This depends on ILO, IHI and NS. NS is the
* number of simultaneous shifts returned
* by ILAENV(ISPEC=4). (See ISPEC=4 below.)
* The default for (IHI-ILO+1).LE.500 is NS.
* The default for (IHI-ILO+1).GT.500 is 3*NS/2.
*
* ISPEC=3: Nibble crossover point. (See ILAENV for
* details.) Default: 14% of deflation window
* size.
*
* ISPEC=4: Number of simultaneous shifts, NS, in
* a multi-shift QR iteration.
*
* If IHI-ILO+1 is ...
*
* greater than ...but less ... the
* or equal to ... than default is
*
* 1 30 NS - 2(+)
* 30 60 NS - 4(+)
* 60 150 NS = 10(+)
* 150 590 NS = **
* 590 3000 NS = 64
* 3000 6000 NS = 128
* 6000 infinity NS = 256
*
* (+) By default some or all matrices of this order
* are passed to the implicit double shift routine
* DLAHQR and NS is ignored. See ISPEC=1 above
* and comments in IPARM for details.
*
* The asterisks (**) indicate an ad-hoc
* function of N increasing from 10 to 64.
*
* ISPEC=5: Select structured matrix multiply.
* (See ILAENV for details.) Default: 3.
*
* ================================================================
* Based on contributions by
* Karen Braman and Ralph Byers, Department of Mathematics,
* University of Kansas, USA
*
* ================================================================
* References:
* K. Braman, R. Byers and R. Mathias, The Multi-Shift QR
* Algorithm Part I: Maintaining Well Focused Shifts, and Level 3
* Performance, SIAM Journal of Matrix Analysis, volume 23, pages
* 929--947, 2002.
*
* K. Braman, R. Byers and R. Mathias, The Multi-Shift QR
* Algorithm Part II: Aggressive Early Deflation, SIAM Journal
* of Matrix Analysis, volume 23, pages 948--973, 2002.
*
* ================================================================
* .. Parameters ..
*
* ==== Matrices of order NTINY or smaller must be processed by
* . DLAHQR because of insufficient subdiagonal scratch space.
* . (This is a hard limit.) ====
*
* ==== NL allocates some local workspace to help small matrices
* . through a rare DLAHQR failure. NL .GT. NTINY = 11 is
* . required and NL .LE. NMIN = ILAENV(ISPEC=1,...) is recom-
* . mended. (The default value of NMIN is 75.) Using NL = 49
* . allows up to six simultaneous shifts and a 16-by-16
* . deflation window. ====
*
*
*
* @param job
* @param compz
* @param n
* @param ilo
* @param ihi
* @param h
* @param _h_offset
* @param ldh
* @param wr
* @param _wr_offset
* @param wi
* @param _wi_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dhseqr(java.lang.String job, java.lang.String compz, int n, int ilo, int ihi, double[] h, int _h_offset, int ldh, double[] wr, int _wr_offset, double[] wi, int _wi_offset, double[] z, int _z_offset, int ldz, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DISNAN returns .TRUE. if its argument is NaN, and .FALSE.
* otherwise. To be replaced by the Fortran 2003 intrinsic in the
* future.
*
* Arguments
* =========
*
* DIN (input) DOUBLE PRECISION
* Input to test for NaN.
*
* =====================================================================
*
* .. External Functions ..
*
*
* @param din
* @return
*/
abstract public boolean disnan(double din);
/**
*
* ..
*
* Purpose
* =======
*
* DLABAD takes as input the values computed by DLAMCH for underflow and
* overflow, and returns the square root of each of these values if the
* log of LARGE is sufficiently large. This subroutine is intended to
* identify machines with a large exponent range, such as the Crays, and
* redefine the underflow and overflow limits to be the square roots of
* the values computed by DLAMCH. This subroutine is needed because
* DLAMCH does not compensate for poor arithmetic in the upper half of
* the exponent range, as is found on a Cray.
*
* Arguments
* =========
*
* SMALL (input/output) DOUBLE PRECISION
* On entry, the underflow threshold as computed by DLAMCH.
* On exit, if LOG10(LARGE) is sufficiently large, the square
* root of SMALL, otherwise unchanged.
*
* LARGE (input/output) DOUBLE PRECISION
* On entry, the overflow threshold as computed by DLAMCH.
* On exit, if LOG10(LARGE) is sufficiently large, the square
* root of LARGE, otherwise unchanged.
*
* =====================================================================
*
* .. Intrinsic Functions ..
*
*
* @param small
* @param large
*
*/
abstract public void dlabad(org.netlib.util.doubleW small, org.netlib.util.doubleW large);
/**
*
* ..
*
* Purpose
* =======
*
* DLABRD reduces the first NB rows and columns of a real general
* m by n matrix A to upper or lower bidiagonal form by an orthogonal
* transformation Q' * A * P, and returns the matrices X and Y which
* are needed to apply the transformation to the unreduced part of A.
*
* If m >= n, A is reduced to upper bidiagonal form; if m < n, to lower
* bidiagonal form.
*
* This is an auxiliary routine called by DGEBRD
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows in the matrix A.
*
* N (input) INTEGER
* The number of columns in the matrix A.
*
* NB (input) INTEGER
* The number of leading rows and columns of A to be reduced.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the m by n general matrix to be reduced.
* On exit, the first NB rows and columns of the matrix are
* overwritten; the rest of the array is unchanged.
* If m >= n, elements on and below the diagonal in the first NB
* columns, with the array TAUQ, represent the orthogonal
* matrix Q as a product of elementary reflectors; and
* elements above the diagonal in the first NB rows, with the
* array TAUP, represent the orthogonal matrix P as a product
* of elementary reflectors.
* If m < n, elements below the diagonal in the first NB
* columns, with the array TAUQ, represent the orthogonal
* matrix Q as a product of elementary reflectors, and
* elements on and above the diagonal in the first NB rows,
* with the array TAUP, represent the orthogonal matrix P as
* a product of elementary reflectors.
* See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* D (output) DOUBLE PRECISION array, dimension (NB)
* The diagonal elements of the first NB rows and columns of
* the reduced matrix. D(i) = A(i,i).
*
* E (output) DOUBLE PRECISION array, dimension (NB)
* The off-diagonal elements of the first NB rows and columns of
* the reduced matrix.
*
* TAUQ (output) DOUBLE PRECISION array dimension (NB)
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix Q. See Further Details.
*
* TAUP (output) DOUBLE PRECISION array, dimension (NB)
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix P. See Further Details.
*
* X (output) DOUBLE PRECISION array, dimension (LDX,NB)
* The m-by-nb matrix X required to update the unreduced part
* of A.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= M.
*
* Y (output) DOUBLE PRECISION array, dimension (LDY,NB)
* The n-by-nb matrix Y required to update the unreduced part
* of A.
*
* LDY (input) INTEGER
* The leading dimension of the array Y. LDY >= N.
*
* Further Details
* ===============
*
* The matrices Q and P are represented as products of elementary
* reflectors:
*
* Q = H(1) H(2) . . . H(nb) and P = G(1) G(2) . . . G(nb)
*
* Each H(i) and G(i) has the form:
*
* H(i) = I - tauq * v * v' and G(i) = I - taup * u * u'
*
* where tauq and taup are real scalars, and v and u are real vectors.
*
* If m >= n, v(1:i-1) = 0, v(i) = 1, and v(i:m) is stored on exit in
* A(i:m,i); u(1:i) = 0, u(i+1) = 1, and u(i+1:n) is stored on exit in
* A(i,i+1:n); tauq is stored in TAUQ(i) and taup in TAUP(i).
*
* If m < n, v(1:i) = 0, v(i+1) = 1, and v(i+1:m) is stored on exit in
* A(i+2:m,i); u(1:i-1) = 0, u(i) = 1, and u(i:n) is stored on exit in
* A(i,i+1:n); tauq is stored in TAUQ(i) and taup in TAUP(i).
*
* The elements of the vectors v and u together form the m-by-nb matrix
* V and the nb-by-n matrix U' which are needed, with X and Y, to apply
* the transformation to the unreduced part of the matrix, using a block
* update of the form: A := A - V*Y' - X*U'.
*
* The contents of A on exit are illustrated by the following examples
* with nb = 2:
*
* m = 6 and n = 5 (m > n): m = 5 and n = 6 (m < n):
*
* ( 1 1 u1 u1 u1 ) ( 1 u1 u1 u1 u1 u1 )
* ( v1 1 1 u2 u2 ) ( 1 1 u2 u2 u2 u2 )
* ( v1 v2 a a a ) ( v1 1 a a a a )
* ( v1 v2 a a a ) ( v1 v2 a a a a )
* ( v1 v2 a a a ) ( v1 v2 a a a a )
* ( v1 v2 a a a )
*
* where a denotes an element of the original matrix which is unchanged,
* vi denotes an element of the vector defining H(i), and ui an element
* of the vector defining G(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param nb
* @param a
* @param lda
* @param d
* @param e
* @param tauq
* @param taup
* @param x
* @param ldx
* @param y
* @param ldy
*
*/
abstract public void dlabrd(int m, int n, int nb, double[] a, int lda, double[] d, double[] e, double[] tauq, double[] taup, double[] x, int ldx, double[] y, int ldy);
/**
*
* ..
*
* Purpose
* =======
*
* DLABRD reduces the first NB rows and columns of a real general
* m by n matrix A to upper or lower bidiagonal form by an orthogonal
* transformation Q' * A * P, and returns the matrices X and Y which
* are needed to apply the transformation to the unreduced part of A.
*
* If m >= n, A is reduced to upper bidiagonal form; if m < n, to lower
* bidiagonal form.
*
* This is an auxiliary routine called by DGEBRD
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows in the matrix A.
*
* N (input) INTEGER
* The number of columns in the matrix A.
*
* NB (input) INTEGER
* The number of leading rows and columns of A to be reduced.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the m by n general matrix to be reduced.
* On exit, the first NB rows and columns of the matrix are
* overwritten; the rest of the array is unchanged.
* If m >= n, elements on and below the diagonal in the first NB
* columns, with the array TAUQ, represent the orthogonal
* matrix Q as a product of elementary reflectors; and
* elements above the diagonal in the first NB rows, with the
* array TAUP, represent the orthogonal matrix P as a product
* of elementary reflectors.
* If m < n, elements below the diagonal in the first NB
* columns, with the array TAUQ, represent the orthogonal
* matrix Q as a product of elementary reflectors, and
* elements on and above the diagonal in the first NB rows,
* with the array TAUP, represent the orthogonal matrix P as
* a product of elementary reflectors.
* See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* D (output) DOUBLE PRECISION array, dimension (NB)
* The diagonal elements of the first NB rows and columns of
* the reduced matrix. D(i) = A(i,i).
*
* E (output) DOUBLE PRECISION array, dimension (NB)
* The off-diagonal elements of the first NB rows and columns of
* the reduced matrix.
*
* TAUQ (output) DOUBLE PRECISION array dimension (NB)
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix Q. See Further Details.
*
* TAUP (output) DOUBLE PRECISION array, dimension (NB)
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix P. See Further Details.
*
* X (output) DOUBLE PRECISION array, dimension (LDX,NB)
* The m-by-nb matrix X required to update the unreduced part
* of A.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= M.
*
* Y (output) DOUBLE PRECISION array, dimension (LDY,NB)
* The n-by-nb matrix Y required to update the unreduced part
* of A.
*
* LDY (input) INTEGER
* The leading dimension of the array Y. LDY >= N.
*
* Further Details
* ===============
*
* The matrices Q and P are represented as products of elementary
* reflectors:
*
* Q = H(1) H(2) . . . H(nb) and P = G(1) G(2) . . . G(nb)
*
* Each H(i) and G(i) has the form:
*
* H(i) = I - tauq * v * v' and G(i) = I - taup * u * u'
*
* where tauq and taup are real scalars, and v and u are real vectors.
*
* If m >= n, v(1:i-1) = 0, v(i) = 1, and v(i:m) is stored on exit in
* A(i:m,i); u(1:i) = 0, u(i+1) = 1, and u(i+1:n) is stored on exit in
* A(i,i+1:n); tauq is stored in TAUQ(i) and taup in TAUP(i).
*
* If m < n, v(1:i) = 0, v(i+1) = 1, and v(i+1:m) is stored on exit in
* A(i+2:m,i); u(1:i-1) = 0, u(i) = 1, and u(i:n) is stored on exit in
* A(i,i+1:n); tauq is stored in TAUQ(i) and taup in TAUP(i).
*
* The elements of the vectors v and u together form the m-by-nb matrix
* V and the nb-by-n matrix U' which are needed, with X and Y, to apply
* the transformation to the unreduced part of the matrix, using a block
* update of the form: A := A - V*Y' - X*U'.
*
* The contents of A on exit are illustrated by the following examples
* with nb = 2:
*
* m = 6 and n = 5 (m > n): m = 5 and n = 6 (m < n):
*
* ( 1 1 u1 u1 u1 ) ( 1 u1 u1 u1 u1 u1 )
* ( v1 1 1 u2 u2 ) ( 1 1 u2 u2 u2 u2 )
* ( v1 v2 a a a ) ( v1 1 a a a a )
* ( v1 v2 a a a ) ( v1 v2 a a a a )
* ( v1 v2 a a a ) ( v1 v2 a a a a )
* ( v1 v2 a a a )
*
* where a denotes an element of the original matrix which is unchanged,
* vi denotes an element of the vector defining H(i), and ui an element
* of the vector defining G(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param nb
* @param a
* @param _a_offset
* @param lda
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param tauq
* @param _tauq_offset
* @param taup
* @param _taup_offset
* @param x
* @param _x_offset
* @param ldx
* @param y
* @param _y_offset
* @param ldy
*
*/
abstract public void dlabrd(int m, int n, int nb, double[] a, int _a_offset, int lda, double[] d, int _d_offset, double[] e, int _e_offset, double[] tauq, int _tauq_offset, double[] taup, int _taup_offset, double[] x, int _x_offset, int ldx, double[] y, int _y_offset, int ldy);
/**
*
* ..
*
* Purpose
* =======
*
* DLACN2 estimates the 1-norm of a square, real matrix A.
* Reverse communication is used for evaluating matrix-vector products.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix. N >= 1.
*
* V (workspace) DOUBLE PRECISION array, dimension (N)
* On the final return, V = A*W, where EST = norm(V)/norm(W)
* (W is not returned).
*
* X (input/output) DOUBLE PRECISION array, dimension (N)
* On an intermediate return, X should be overwritten by
* A * X, if KASE=1,
* A' * X, if KASE=2,
* and DLACN2 must be re-called with all the other parameters
* unchanged.
*
* ISGN (workspace) INTEGER array, dimension (N)
*
* EST (input/output) DOUBLE PRECISION
* On entry with KASE = 1 or 2 and ISAVE(1) = 3, EST should be
* unchanged from the previous call to DLACN2.
* On exit, EST is an estimate (a lower bound) for norm(A).
*
* KASE (input/output) INTEGER
* On the initial call to DLACN2, KASE should be 0.
* On an intermediate return, KASE will be 1 or 2, indicating
* whether X should be overwritten by A * X or A' * X.
* On the final return from DLACN2, KASE will again be 0.
*
* ISAVE (input/output) INTEGER array, dimension (3)
* ISAVE is used to save variables between calls to DLACN2
*
* Further Details
* ======= =======
*
* Contributed by Nick Higham, University of Manchester.
* Originally named SONEST, dated March 16, 1988.
*
* Reference: N.J. Higham, "FORTRAN codes for estimating the one-norm of
* a real or complex matrix, with applications to condition estimation",
* ACM Trans. Math. Soft., vol. 14, no. 4, pp. 381-396, December 1988.
*
* This is a thread safe version of DLACON, which uses the array ISAVE
* in place of a SAVE statement, as follows:
*
* DLACON DLACN2
* JUMP ISAVE(1)
* J ISAVE(2)
* ITER ISAVE(3)
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param v
* @param x
* @param isgn
* @param est
* @param kase
* @param isave
*
*/
abstract public void dlacn2(int n, double[] v, double[] x, int[] isgn, org.netlib.util.doubleW est, org.netlib.util.intW kase, int[] isave);
/**
*
* ..
*
* Purpose
* =======
*
* DLACN2 estimates the 1-norm of a square, real matrix A.
* Reverse communication is used for evaluating matrix-vector products.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix. N >= 1.
*
* V (workspace) DOUBLE PRECISION array, dimension (N)
* On the final return, V = A*W, where EST = norm(V)/norm(W)
* (W is not returned).
*
* X (input/output) DOUBLE PRECISION array, dimension (N)
* On an intermediate return, X should be overwritten by
* A * X, if KASE=1,
* A' * X, if KASE=2,
* and DLACN2 must be re-called with all the other parameters
* unchanged.
*
* ISGN (workspace) INTEGER array, dimension (N)
*
* EST (input/output) DOUBLE PRECISION
* On entry with KASE = 1 or 2 and ISAVE(1) = 3, EST should be
* unchanged from the previous call to DLACN2.
* On exit, EST is an estimate (a lower bound) for norm(A).
*
* KASE (input/output) INTEGER
* On the initial call to DLACN2, KASE should be 0.
* On an intermediate return, KASE will be 1 or 2, indicating
* whether X should be overwritten by A * X or A' * X.
* On the final return from DLACN2, KASE will again be 0.
*
* ISAVE (input/output) INTEGER array, dimension (3)
* ISAVE is used to save variables between calls to DLACN2
*
* Further Details
* ======= =======
*
* Contributed by Nick Higham, University of Manchester.
* Originally named SONEST, dated March 16, 1988.
*
* Reference: N.J. Higham, "FORTRAN codes for estimating the one-norm of
* a real or complex matrix, with applications to condition estimation",
* ACM Trans. Math. Soft., vol. 14, no. 4, pp. 381-396, December 1988.
*
* This is a thread safe version of DLACON, which uses the array ISAVE
* in place of a SAVE statement, as follows:
*
* DLACON DLACN2
* JUMP ISAVE(1)
* J ISAVE(2)
* ITER ISAVE(3)
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param v
* @param _v_offset
* @param x
* @param _x_offset
* @param isgn
* @param _isgn_offset
* @param est
* @param kase
* @param isave
* @param _isave_offset
*
*/
abstract public void dlacn2(int n, double[] v, int _v_offset, double[] x, int _x_offset, int[] isgn, int _isgn_offset, org.netlib.util.doubleW est, org.netlib.util.intW kase, int[] isave, int _isave_offset);
/**
*
* ..
*
* Purpose
* =======
*
* DLACON estimates the 1-norm of a square, real matrix A.
* Reverse communication is used for evaluating matrix-vector products.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix. N >= 1.
*
* V (workspace) DOUBLE PRECISION array, dimension (N)
* On the final return, V = A*W, where EST = norm(V)/norm(W)
* (W is not returned).
*
* X (input/output) DOUBLE PRECISION array, dimension (N)
* On an intermediate return, X should be overwritten by
* A * X, if KASE=1,
* A' * X, if KASE=2,
* and DLACON must be re-called with all the other parameters
* unchanged.
*
* ISGN (workspace) INTEGER array, dimension (N)
*
* EST (input/output) DOUBLE PRECISION
* On entry with KASE = 1 or 2 and JUMP = 3, EST should be
* unchanged from the previous call to DLACON.
* On exit, EST is an estimate (a lower bound) for norm(A).
*
* KASE (input/output) INTEGER
* On the initial call to DLACON, KASE should be 0.
* On an intermediate return, KASE will be 1 or 2, indicating
* whether X should be overwritten by A * X or A' * X.
* On the final return from DLACON, KASE will again be 0.
*
* Further Details
* ======= =======
*
* Contributed by Nick Higham, University of Manchester.
* Originally named SONEST, dated March 16, 1988.
*
* Reference: N.J. Higham, "FORTRAN codes for estimating the one-norm of
* a real or complex matrix, with applications to condition estimation",
* ACM Trans. Math. Soft., vol. 14, no. 4, pp. 381-396, December 1988.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param v
* @param x
* @param isgn
* @param est
* @param kase
*
*/
abstract public void dlacon(int n, double[] v, double[] x, int[] isgn, org.netlib.util.doubleW est, org.netlib.util.intW kase);
/**
*
* ..
*
* Purpose
* =======
*
* DLACON estimates the 1-norm of a square, real matrix A.
* Reverse communication is used for evaluating matrix-vector products.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix. N >= 1.
*
* V (workspace) DOUBLE PRECISION array, dimension (N)
* On the final return, V = A*W, where EST = norm(V)/norm(W)
* (W is not returned).
*
* X (input/output) DOUBLE PRECISION array, dimension (N)
* On an intermediate return, X should be overwritten by
* A * X, if KASE=1,
* A' * X, if KASE=2,
* and DLACON must be re-called with all the other parameters
* unchanged.
*
* ISGN (workspace) INTEGER array, dimension (N)
*
* EST (input/output) DOUBLE PRECISION
* On entry with KASE = 1 or 2 and JUMP = 3, EST should be
* unchanged from the previous call to DLACON.
* On exit, EST is an estimate (a lower bound) for norm(A).
*
* KASE (input/output) INTEGER
* On the initial call to DLACON, KASE should be 0.
* On an intermediate return, KASE will be 1 or 2, indicating
* whether X should be overwritten by A * X or A' * X.
* On the final return from DLACON, KASE will again be 0.
*
* Further Details
* ======= =======
*
* Contributed by Nick Higham, University of Manchester.
* Originally named SONEST, dated March 16, 1988.
*
* Reference: N.J. Higham, "FORTRAN codes for estimating the one-norm of
* a real or complex matrix, with applications to condition estimation",
* ACM Trans. Math. Soft., vol. 14, no. 4, pp. 381-396, December 1988.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param v
* @param _v_offset
* @param x
* @param _x_offset
* @param isgn
* @param _isgn_offset
* @param est
* @param kase
*
*/
abstract public void dlacon(int n, double[] v, int _v_offset, double[] x, int _x_offset, int[] isgn, int _isgn_offset, org.netlib.util.doubleW est, org.netlib.util.intW kase);
/**
*
* ..
*
* Purpose
* =======
*
* DLACPY copies all or part of a two-dimensional matrix A to another
* matrix B.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies the part of the matrix A to be copied to B.
* = 'U': Upper triangular part
* = 'L': Lower triangular part
* Otherwise: All of the matrix A
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The m by n matrix A. If UPLO = 'U', only the upper triangle
* or trapezoid is accessed; if UPLO = 'L', only the lower
* triangle or trapezoid is accessed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (output) DOUBLE PRECISION array, dimension (LDB,N)
* On exit, B = A in the locations specified by UPLO.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,M).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param uplo
* @param m
* @param n
* @param a
* @param lda
* @param b
* @param ldb
*
*/
abstract public void dlacpy(java.lang.String uplo, int m, int n, double[] a, int lda, double[] b, int ldb);
/**
*
* ..
*
* Purpose
* =======
*
* DLACPY copies all or part of a two-dimensional matrix A to another
* matrix B.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies the part of the matrix A to be copied to B.
* = 'U': Upper triangular part
* = 'L': Lower triangular part
* Otherwise: All of the matrix A
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The m by n matrix A. If UPLO = 'U', only the upper triangle
* or trapezoid is accessed; if UPLO = 'L', only the lower
* triangle or trapezoid is accessed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (output) DOUBLE PRECISION array, dimension (LDB,N)
* On exit, B = A in the locations specified by UPLO.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,M).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param uplo
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
*
*/
abstract public void dlacpy(java.lang.String uplo, int m, int n, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb);
/**
*
* ..
*
* Purpose
* =======
*
* DLADIV performs complex division in real arithmetic
*
* a + i*b
* p + i*q = ---------
* c + i*d
*
* The algorithm is due to Robert L. Smith and can be found
* in D. Knuth, The art of Computer Programming, Vol.2, p.195
*
* Arguments
* =========
*
* A (input) DOUBLE PRECISION
* B (input) DOUBLE PRECISION
* C (input) DOUBLE PRECISION
* D (input) DOUBLE PRECISION
* The scalars a, b, c, and d in the above expression.
*
* P (output) DOUBLE PRECISION
* Q (output) DOUBLE PRECISION
* The scalars p and q in the above expression.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param a
* @param b
* @param c
* @param d
* @param p
* @param q
*
*/
abstract public void dladiv(double a, double b, double c, double d, org.netlib.util.doubleW p, org.netlib.util.doubleW q);
/**
*
* ..
*
* Purpose
* =======
*
* DLAE2 computes the eigenvalues of a 2-by-2 symmetric matrix
* [ A B ]
* [ B C ].
* On return, RT1 is the eigenvalue of larger absolute value, and RT2
* is the eigenvalue of smaller absolute value.
*
* Arguments
* =========
*
* A (input) DOUBLE PRECISION
* The (1,1) element of the 2-by-2 matrix.
*
* B (input) DOUBLE PRECISION
* The (1,2) and (2,1) elements of the 2-by-2 matrix.
*
* C (input) DOUBLE PRECISION
* The (2,2) element of the 2-by-2 matrix.
*
* RT1 (output) DOUBLE PRECISION
* The eigenvalue of larger absolute value.
*
* RT2 (output) DOUBLE PRECISION
* The eigenvalue of smaller absolute value.
*
* Further Details
* ===============
*
* RT1 is accurate to a few ulps barring over/underflow.
*
* RT2 may be inaccurate if there is massive cancellation in the
* determinant A*C-B*B; higher precision or correctly rounded or
* correctly truncated arithmetic would be needed to compute RT2
* accurately in all cases.
*
* Overflow is possible only if RT1 is within a factor of 5 of overflow.
* Underflow is harmless if the input data is 0 or exceeds
* underflow_threshold / macheps.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param a
* @param b
* @param c
* @param rt1
* @param rt2
*
*/
abstract public void dlae2(double a, double b, double c, org.netlib.util.doubleW rt1, org.netlib.util.doubleW rt2);
/**
*
* ..
*
* Purpose
* =======
*
* DLAEBZ contains the iteration loops which compute and use the
* function N(w), which is the count of eigenvalues of a symmetric
* tridiagonal matrix T less than or equal to its argument w. It
* performs a choice of two types of loops:
*
* IJOB=1, followed by
* IJOB=2: It takes as input a list of intervals and returns a list of
* sufficiently small intervals whose union contains the same
* eigenvalues as the union of the original intervals.
* The input intervals are (AB(j,1),AB(j,2)], j=1,...,MINP.
* The output interval (AB(j,1),AB(j,2)] will contain
* eigenvalues NAB(j,1)+1,...,NAB(j,2), where 1 <= j <= MOUT.
*
* IJOB=3: It performs a binary search in each input interval
* (AB(j,1),AB(j,2)] for a point w(j) such that
* N(w(j))=NVAL(j), and uses C(j) as the starting point of
* the search. If such a w(j) is found, then on output
* AB(j,1)=AB(j,2)=w. If no such w(j) is found, then on output
* (AB(j,1),AB(j,2)] will be a small interval containing the
* point where N(w) jumps through NVAL(j), unless that point
* lies outside the initial interval.
*
* Note that the intervals are in all cases half-open intervals,
* i.e., of the form (a,b] , which includes b but not a .
*
* To avoid underflow, the matrix should be scaled so that its largest
* element is no greater than overflow**(1/2) * underflow**(1/4)
* in absolute value. To assure the most accurate computation
* of small eigenvalues, the matrix should be scaled to be
* not much smaller than that, either.
*
* See W. Kahan "Accurate Eigenvalues of a Symmetric Tridiagonal
* Matrix", Report CS41, Computer Science Dept., Stanford
* University, July 21, 1966
*
* Note: the arguments are, in general, *not* checked for unreasonable
* values.
*
* Arguments
* =========
*
* IJOB (input) INTEGER
* Specifies what is to be done:
* = 1: Compute NAB for the initial intervals.
* = 2: Perform bisection iteration to find eigenvalues of T.
* = 3: Perform bisection iteration to invert N(w), i.e.,
* to find a point which has a specified number of
* eigenvalues of T to its left.
* Other values will cause DLAEBZ to return with INFO=-1.
*
* NITMAX (input) INTEGER
* The maximum number of "levels" of bisection to be
* performed, i.e., an interval of width W will not be made
* smaller than 2^(-NITMAX) * W. If not all intervals
* have converged after NITMAX iterations, then INFO is set
* to the number of non-converged intervals.
*
* N (input) INTEGER
* The dimension n of the tridiagonal matrix T. It must be at
* least 1.
*
* MMAX (input) INTEGER
* The maximum number of intervals. If more than MMAX intervals
* are generated, then DLAEBZ will quit with INFO=MMAX+1.
*
* MINP (input) INTEGER
* The initial number of intervals. It may not be greater than
* MMAX.
*
* NBMIN (input) INTEGER
* The smallest number of intervals that should be processed
* using a vector loop. If zero, then only the scalar loop
* will be used.
*
* ABSTOL (input) DOUBLE PRECISION
* The minimum (absolute) width of an interval. When an
* interval is narrower than ABSTOL, or than RELTOL times the
* larger (in magnitude) endpoint, then it is considered to be
* sufficiently small, i.e., converged. This must be at least
* zero.
*
* RELTOL (input) DOUBLE PRECISION
* The minimum relative width of an interval. When an interval
* is narrower than ABSTOL, or than RELTOL times the larger (in
* magnitude) endpoint, then it is considered to be
* sufficiently small, i.e., converged. Note: this should
* always be at least radix*machine epsilon.
*
* PIVMIN (input) DOUBLE PRECISION
* The minimum absolute value of a "pivot" in the Sturm
* sequence loop. This *must* be at least max |e(j)**2| *
* safe_min and at least safe_min, where safe_min is at least
* the smallest number that can divide one without overflow.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The diagonal elements of the tridiagonal matrix T.
*
* E (input) DOUBLE PRECISION array, dimension (N)
* The offdiagonal elements of the tridiagonal matrix T in
* positions 1 through N-1. E(N) is arbitrary.
*
* E2 (input) DOUBLE PRECISION array, dimension (N)
* The squares of the offdiagonal elements of the tridiagonal
* matrix T. E2(N) is ignored.
*
* NVAL (input/output) INTEGER array, dimension (MINP)
* If IJOB=1 or 2, not referenced.
* If IJOB=3, the desired values of N(w). The elements of NVAL
* will be reordered to correspond with the intervals in AB.
* Thus, NVAL(j) on output will not, in general be the same as
* NVAL(j) on input, but it will correspond with the interval
* (AB(j,1),AB(j,2)] on output.
*
* AB (input/output) DOUBLE PRECISION array, dimension (MMAX,2)
* The endpoints of the intervals. AB(j,1) is a(j), the left
* endpoint of the j-th interval, and AB(j,2) is b(j), the
* right endpoint of the j-th interval. The input intervals
* will, in general, be modified, split, and reordered by the
* calculation.
*
* C (input/output) DOUBLE PRECISION array, dimension (MMAX)
* If IJOB=1, ignored.
* If IJOB=2, workspace.
* If IJOB=3, then on input C(j) should be initialized to the
* first search point in the binary search.
*
* MOUT (output) INTEGER
* If IJOB=1, the number of eigenvalues in the intervals.
* If IJOB=2 or 3, the number of intervals output.
* If IJOB=3, MOUT will equal MINP.
*
* NAB (input/output) INTEGER array, dimension (MMAX,2)
* If IJOB=1, then on output NAB(i,j) will be set to N(AB(i,j)).
* If IJOB=2, then on input, NAB(i,j) should be set. It must
* satisfy the condition:
* N(AB(i,1)) <= NAB(i,1) <= NAB(i,2) <= N(AB(i,2)),
* which means that in interval i only eigenvalues
* NAB(i,1)+1,...,NAB(i,2) will be considered. Usually,
* NAB(i,j)=N(AB(i,j)), from a previous call to DLAEBZ with
* IJOB=1.
* On output, NAB(i,j) will contain
* max(na(k),min(nb(k),N(AB(i,j)))), where k is the index of
* the input interval that the output interval
* (AB(j,1),AB(j,2)] came from, and na(k) and nb(k) are the
* the input values of NAB(k,1) and NAB(k,2).
* If IJOB=3, then on output, NAB(i,j) contains N(AB(i,j)),
* unless N(w) > NVAL(i) for all search points w , in which
* case NAB(i,1) will not be modified, i.e., the output
* value will be the same as the input value (modulo
* reorderings -- see NVAL and AB), or unless N(w) < NVAL(i)
* for all search points w , in which case NAB(i,2) will
* not be modified. Normally, NAB should be set to some
* distinctive value(s) before DLAEBZ is called.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (MMAX)
* Workspace.
*
* IWORK (workspace) INTEGER array, dimension (MMAX)
* Workspace.
*
* INFO (output) INTEGER
* = 0: All intervals converged.
* = 1--MMAX: The last INFO intervals did not converge.
* = MMAX+1: More than MMAX intervals were generated.
*
* Further Details
* ===============
*
* This routine is intended to be called only by other LAPACK
* routines, thus the interface is less user-friendly. It is intended
* for two purposes:
*
* (a) finding eigenvalues. In this case, DLAEBZ should have one or
* more initial intervals set up in AB, and DLAEBZ should be called
* with IJOB=1. This sets up NAB, and also counts the eigenvalues.
* Intervals with no eigenvalues would usually be thrown out at
* this point. Also, if not all the eigenvalues in an interval i
* are desired, NAB(i,1) can be increased or NAB(i,2) decreased.
* For example, set NAB(i,1)=NAB(i,2)-1 to get the largest
* eigenvalue. DLAEBZ is then called with IJOB=2 and MMAX
* no smaller than the value of MOUT returned by the call with
* IJOB=1. After this (IJOB=2) call, eigenvalues NAB(i,1)+1
* through NAB(i,2) are approximately AB(i,1) (or AB(i,2)) to the
* tolerance specified by ABSTOL and RELTOL.
*
* (b) finding an interval (a',b'] containing eigenvalues w(f),...,w(l).
* In this case, start with a Gershgorin interval (a,b). Set up
* AB to contain 2 search intervals, both initially (a,b). One
* NVAL element should contain f-1 and the other should contain l
* , while C should contain a and b, resp. NAB(i,1) should be -1
* and NAB(i,2) should be N+1, to flag an error if the desired
* interval does not lie in (a,b). DLAEBZ is then called with
* IJOB=3. On exit, if w(f-1) < w(f), then one of the intervals --
* j -- will have AB(j,1)=AB(j,2) and NAB(j,1)=NAB(j,2)=f-1, while
* if, to the specified tolerance, w(f-k)=...=w(f+r), k > 0 and r
* >= 0, then the interval will have N(AB(j,1))=NAB(j,1)=f-k and
* N(AB(j,2))=NAB(j,2)=f+r. The cases w(l) < w(l+1) and
* w(l-r)=...=w(l+k) are handled similarly.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param ijob
* @param nitmax
* @param n
* @param mmax
* @param minp
* @param nbmin
* @param abstol
* @param reltol
* @param pivmin
* @param d
* @param e
* @param e2
* @param nval
* @param ab
* @param c
* @param mout
* @param nab
* @param work
* @param iwork
* @param info
*
*/
abstract public void dlaebz(int ijob, int nitmax, int n, int mmax, int minp, int nbmin, double abstol, double reltol, double pivmin, double[] d, double[] e, double[] e2, int[] nval, double[] ab, double[] c, org.netlib.util.intW mout, int[] nab, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAEBZ contains the iteration loops which compute and use the
* function N(w), which is the count of eigenvalues of a symmetric
* tridiagonal matrix T less than or equal to its argument w. It
* performs a choice of two types of loops:
*
* IJOB=1, followed by
* IJOB=2: It takes as input a list of intervals and returns a list of
* sufficiently small intervals whose union contains the same
* eigenvalues as the union of the original intervals.
* The input intervals are (AB(j,1),AB(j,2)], j=1,...,MINP.
* The output interval (AB(j,1),AB(j,2)] will contain
* eigenvalues NAB(j,1)+1,...,NAB(j,2), where 1 <= j <= MOUT.
*
* IJOB=3: It performs a binary search in each input interval
* (AB(j,1),AB(j,2)] for a point w(j) such that
* N(w(j))=NVAL(j), and uses C(j) as the starting point of
* the search. If such a w(j) is found, then on output
* AB(j,1)=AB(j,2)=w. If no such w(j) is found, then on output
* (AB(j,1),AB(j,2)] will be a small interval containing the
* point where N(w) jumps through NVAL(j), unless that point
* lies outside the initial interval.
*
* Note that the intervals are in all cases half-open intervals,
* i.e., of the form (a,b] , which includes b but not a .
*
* To avoid underflow, the matrix should be scaled so that its largest
* element is no greater than overflow**(1/2) * underflow**(1/4)
* in absolute value. To assure the most accurate computation
* of small eigenvalues, the matrix should be scaled to be
* not much smaller than that, either.
*
* See W. Kahan "Accurate Eigenvalues of a Symmetric Tridiagonal
* Matrix", Report CS41, Computer Science Dept., Stanford
* University, July 21, 1966
*
* Note: the arguments are, in general, *not* checked for unreasonable
* values.
*
* Arguments
* =========
*
* IJOB (input) INTEGER
* Specifies what is to be done:
* = 1: Compute NAB for the initial intervals.
* = 2: Perform bisection iteration to find eigenvalues of T.
* = 3: Perform bisection iteration to invert N(w), i.e.,
* to find a point which has a specified number of
* eigenvalues of T to its left.
* Other values will cause DLAEBZ to return with INFO=-1.
*
* NITMAX (input) INTEGER
* The maximum number of "levels" of bisection to be
* performed, i.e., an interval of width W will not be made
* smaller than 2^(-NITMAX) * W. If not all intervals
* have converged after NITMAX iterations, then INFO is set
* to the number of non-converged intervals.
*
* N (input) INTEGER
* The dimension n of the tridiagonal matrix T. It must be at
* least 1.
*
* MMAX (input) INTEGER
* The maximum number of intervals. If more than MMAX intervals
* are generated, then DLAEBZ will quit with INFO=MMAX+1.
*
* MINP (input) INTEGER
* The initial number of intervals. It may not be greater than
* MMAX.
*
* NBMIN (input) INTEGER
* The smallest number of intervals that should be processed
* using a vector loop. If zero, then only the scalar loop
* will be used.
*
* ABSTOL (input) DOUBLE PRECISION
* The minimum (absolute) width of an interval. When an
* interval is narrower than ABSTOL, or than RELTOL times the
* larger (in magnitude) endpoint, then it is considered to be
* sufficiently small, i.e., converged. This must be at least
* zero.
*
* RELTOL (input) DOUBLE PRECISION
* The minimum relative width of an interval. When an interval
* is narrower than ABSTOL, or than RELTOL times the larger (in
* magnitude) endpoint, then it is considered to be
* sufficiently small, i.e., converged. Note: this should
* always be at least radix*machine epsilon.
*
* PIVMIN (input) DOUBLE PRECISION
* The minimum absolute value of a "pivot" in the Sturm
* sequence loop. This *must* be at least max |e(j)**2| *
* safe_min and at least safe_min, where safe_min is at least
* the smallest number that can divide one without overflow.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The diagonal elements of the tridiagonal matrix T.
*
* E (input) DOUBLE PRECISION array, dimension (N)
* The offdiagonal elements of the tridiagonal matrix T in
* positions 1 through N-1. E(N) is arbitrary.
*
* E2 (input) DOUBLE PRECISION array, dimension (N)
* The squares of the offdiagonal elements of the tridiagonal
* matrix T. E2(N) is ignored.
*
* NVAL (input/output) INTEGER array, dimension (MINP)
* If IJOB=1 or 2, not referenced.
* If IJOB=3, the desired values of N(w). The elements of NVAL
* will be reordered to correspond with the intervals in AB.
* Thus, NVAL(j) on output will not, in general be the same as
* NVAL(j) on input, but it will correspond with the interval
* (AB(j,1),AB(j,2)] on output.
*
* AB (input/output) DOUBLE PRECISION array, dimension (MMAX,2)
* The endpoints of the intervals. AB(j,1) is a(j), the left
* endpoint of the j-th interval, and AB(j,2) is b(j), the
* right endpoint of the j-th interval. The input intervals
* will, in general, be modified, split, and reordered by the
* calculation.
*
* C (input/output) DOUBLE PRECISION array, dimension (MMAX)
* If IJOB=1, ignored.
* If IJOB=2, workspace.
* If IJOB=3, then on input C(j) should be initialized to the
* first search point in the binary search.
*
* MOUT (output) INTEGER
* If IJOB=1, the number of eigenvalues in the intervals.
* If IJOB=2 or 3, the number of intervals output.
* If IJOB=3, MOUT will equal MINP.
*
* NAB (input/output) INTEGER array, dimension (MMAX,2)
* If IJOB=1, then on output NAB(i,j) will be set to N(AB(i,j)).
* If IJOB=2, then on input, NAB(i,j) should be set. It must
* satisfy the condition:
* N(AB(i,1)) <= NAB(i,1) <= NAB(i,2) <= N(AB(i,2)),
* which means that in interval i only eigenvalues
* NAB(i,1)+1,...,NAB(i,2) will be considered. Usually,
* NAB(i,j)=N(AB(i,j)), from a previous call to DLAEBZ with
* IJOB=1.
* On output, NAB(i,j) will contain
* max(na(k),min(nb(k),N(AB(i,j)))), where k is the index of
* the input interval that the output interval
* (AB(j,1),AB(j,2)] came from, and na(k) and nb(k) are the
* the input values of NAB(k,1) and NAB(k,2).
* If IJOB=3, then on output, NAB(i,j) contains N(AB(i,j)),
* unless N(w) > NVAL(i) for all search points w , in which
* case NAB(i,1) will not be modified, i.e., the output
* value will be the same as the input value (modulo
* reorderings -- see NVAL and AB), or unless N(w) < NVAL(i)
* for all search points w , in which case NAB(i,2) will
* not be modified. Normally, NAB should be set to some
* distinctive value(s) before DLAEBZ is called.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (MMAX)
* Workspace.
*
* IWORK (workspace) INTEGER array, dimension (MMAX)
* Workspace.
*
* INFO (output) INTEGER
* = 0: All intervals converged.
* = 1--MMAX: The last INFO intervals did not converge.
* = MMAX+1: More than MMAX intervals were generated.
*
* Further Details
* ===============
*
* This routine is intended to be called only by other LAPACK
* routines, thus the interface is less user-friendly. It is intended
* for two purposes:
*
* (a) finding eigenvalues. In this case, DLAEBZ should have one or
* more initial intervals set up in AB, and DLAEBZ should be called
* with IJOB=1. This sets up NAB, and also counts the eigenvalues.
* Intervals with no eigenvalues would usually be thrown out at
* this point. Also, if not all the eigenvalues in an interval i
* are desired, NAB(i,1) can be increased or NAB(i,2) decreased.
* For example, set NAB(i,1)=NAB(i,2)-1 to get the largest
* eigenvalue. DLAEBZ is then called with IJOB=2 and MMAX
* no smaller than the value of MOUT returned by the call with
* IJOB=1. After this (IJOB=2) call, eigenvalues NAB(i,1)+1
* through NAB(i,2) are approximately AB(i,1) (or AB(i,2)) to the
* tolerance specified by ABSTOL and RELTOL.
*
* (b) finding an interval (a',b'] containing eigenvalues w(f),...,w(l).
* In this case, start with a Gershgorin interval (a,b). Set up
* AB to contain 2 search intervals, both initially (a,b). One
* NVAL element should contain f-1 and the other should contain l
* , while C should contain a and b, resp. NAB(i,1) should be -1
* and NAB(i,2) should be N+1, to flag an error if the desired
* interval does not lie in (a,b). DLAEBZ is then called with
* IJOB=3. On exit, if w(f-1) < w(f), then one of the intervals --
* j -- will have AB(j,1)=AB(j,2) and NAB(j,1)=NAB(j,2)=f-1, while
* if, to the specified tolerance, w(f-k)=...=w(f+r), k > 0 and r
* >= 0, then the interval will have N(AB(j,1))=NAB(j,1)=f-k and
* N(AB(j,2))=NAB(j,2)=f+r. The cases w(l) < w(l+1) and
* w(l-r)=...=w(l+k) are handled similarly.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param ijob
* @param nitmax
* @param n
* @param mmax
* @param minp
* @param nbmin
* @param abstol
* @param reltol
* @param pivmin
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param e2
* @param _e2_offset
* @param nval
* @param _nval_offset
* @param ab
* @param _ab_offset
* @param c
* @param _c_offset
* @param mout
* @param nab
* @param _nab_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dlaebz(int ijob, int nitmax, int n, int mmax, int minp, int nbmin, double abstol, double reltol, double pivmin, double[] d, int _d_offset, double[] e, int _e_offset, double[] e2, int _e2_offset, int[] nval, int _nval_offset, double[] ab, int _ab_offset, double[] c, int _c_offset, org.netlib.util.intW mout, int[] nab, int _nab_offset, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAED0 computes all eigenvalues and corresponding eigenvectors of a
* symmetric tridiagonal matrix using the divide and conquer method.
*
* Arguments
* =========
*
* ICOMPQ (input) INTEGER
* = 0: Compute eigenvalues only.
* = 1: Compute eigenvectors of original dense symmetric matrix
* also. On entry, Q contains the orthogonal matrix used
* to reduce the original matrix to tridiagonal form.
* = 2: Compute eigenvalues and eigenvectors of tridiagonal
* matrix.
*
* QSIZ (input) INTEGER
* The dimension of the orthogonal matrix used to reduce
* the full matrix to tridiagonal form. QSIZ >= N if ICOMPQ = 1.
*
* N (input) INTEGER
* The dimension of the symmetric tridiagonal matrix. N >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the main diagonal of the tridiagonal matrix.
* On exit, its eigenvalues.
*
* E (input) DOUBLE PRECISION array, dimension (N-1)
* The off-diagonal elements of the tridiagonal matrix.
* On exit, E has been destroyed.
*
* Q (input/output) DOUBLE PRECISION array, dimension (LDQ, N)
* On entry, Q must contain an N-by-N orthogonal matrix.
* If ICOMPQ = 0 Q is not referenced.
* If ICOMPQ = 1 On entry, Q is a subset of the columns of the
* orthogonal matrix used to reduce the full
* matrix to tridiagonal form corresponding to
* the subset of the full matrix which is being
* decomposed at this time.
* If ICOMPQ = 2 On entry, Q will be the identity matrix.
* On exit, Q contains the eigenvectors of the
* tridiagonal matrix.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. If eigenvectors are
* desired, then LDQ >= max(1,N). In any case, LDQ >= 1.
*
* QSTORE (workspace) DOUBLE PRECISION array, dimension (LDQS, N)
* Referenced only when ICOMPQ = 1. Used to store parts of
* the eigenvector matrix when the updating matrix multiplies
* take place.
*
* LDQS (input) INTEGER
* The leading dimension of the array QSTORE. If ICOMPQ = 1,
* then LDQS >= max(1,N). In any case, LDQS >= 1.
*
* WORK (workspace) DOUBLE PRECISION array,
* If ICOMPQ = 0 or 1, the dimension of WORK must be at least
* 1 + 3*N + 2*N*lg N + 2*N**2
* ( lg( N ) = smallest integer k
* such that 2^k >= N )
* If ICOMPQ = 2, the dimension of WORK must be at least
* 4*N + N**2.
*
* IWORK (workspace) INTEGER array,
* If ICOMPQ = 0 or 1, the dimension of IWORK must be at least
* 6 + 6*N + 5*N*lg N.
* ( lg( N ) = smallest integer k
* such that 2^k >= N )
* If ICOMPQ = 2, the dimension of IWORK must be at least
* 3 + 5*N.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: The algorithm failed to compute an eigenvalue while
* working on the submatrix lying in rows and columns
* INFO/(N+1) through mod(INFO,N+1).
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param qsiz
* @param n
* @param d
* @param e
* @param q
* @param ldq
* @param qstore
* @param ldqs
* @param work
* @param iwork
* @param info
*
*/
abstract public void dlaed0(int icompq, int qsiz, int n, double[] d, double[] e, double[] q, int ldq, double[] qstore, int ldqs, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAED0 computes all eigenvalues and corresponding eigenvectors of a
* symmetric tridiagonal matrix using the divide and conquer method.
*
* Arguments
* =========
*
* ICOMPQ (input) INTEGER
* = 0: Compute eigenvalues only.
* = 1: Compute eigenvectors of original dense symmetric matrix
* also. On entry, Q contains the orthogonal matrix used
* to reduce the original matrix to tridiagonal form.
* = 2: Compute eigenvalues and eigenvectors of tridiagonal
* matrix.
*
* QSIZ (input) INTEGER
* The dimension of the orthogonal matrix used to reduce
* the full matrix to tridiagonal form. QSIZ >= N if ICOMPQ = 1.
*
* N (input) INTEGER
* The dimension of the symmetric tridiagonal matrix. N >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the main diagonal of the tridiagonal matrix.
* On exit, its eigenvalues.
*
* E (input) DOUBLE PRECISION array, dimension (N-1)
* The off-diagonal elements of the tridiagonal matrix.
* On exit, E has been destroyed.
*
* Q (input/output) DOUBLE PRECISION array, dimension (LDQ, N)
* On entry, Q must contain an N-by-N orthogonal matrix.
* If ICOMPQ = 0 Q is not referenced.
* If ICOMPQ = 1 On entry, Q is a subset of the columns of the
* orthogonal matrix used to reduce the full
* matrix to tridiagonal form corresponding to
* the subset of the full matrix which is being
* decomposed at this time.
* If ICOMPQ = 2 On entry, Q will be the identity matrix.
* On exit, Q contains the eigenvectors of the
* tridiagonal matrix.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. If eigenvectors are
* desired, then LDQ >= max(1,N). In any case, LDQ >= 1.
*
* QSTORE (workspace) DOUBLE PRECISION array, dimension (LDQS, N)
* Referenced only when ICOMPQ = 1. Used to store parts of
* the eigenvector matrix when the updating matrix multiplies
* take place.
*
* LDQS (input) INTEGER
* The leading dimension of the array QSTORE. If ICOMPQ = 1,
* then LDQS >= max(1,N). In any case, LDQS >= 1.
*
* WORK (workspace) DOUBLE PRECISION array,
* If ICOMPQ = 0 or 1, the dimension of WORK must be at least
* 1 + 3*N + 2*N*lg N + 2*N**2
* ( lg( N ) = smallest integer k
* such that 2^k >= N )
* If ICOMPQ = 2, the dimension of WORK must be at least
* 4*N + N**2.
*
* IWORK (workspace) INTEGER array,
* If ICOMPQ = 0 or 1, the dimension of IWORK must be at least
* 6 + 6*N + 5*N*lg N.
* ( lg( N ) = smallest integer k
* such that 2^k >= N )
* If ICOMPQ = 2, the dimension of IWORK must be at least
* 3 + 5*N.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: The algorithm failed to compute an eigenvalue while
* working on the submatrix lying in rows and columns
* INFO/(N+1) through mod(INFO,N+1).
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param qsiz
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param q
* @param _q_offset
* @param ldq
* @param qstore
* @param _qstore_offset
* @param ldqs
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dlaed0(int icompq, int qsiz, int n, double[] d, int _d_offset, double[] e, int _e_offset, double[] q, int _q_offset, int ldq, double[] qstore, int _qstore_offset, int ldqs, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAED1 computes the updated eigensystem of a diagonal
* matrix after modification by a rank-one symmetric matrix. This
* routine is used only for the eigenproblem which requires all
* eigenvalues and eigenvectors of a tridiagonal matrix. DLAED7 handles
* the case in which eigenvalues only or eigenvalues and eigenvectors
* of a full symmetric matrix (which was reduced to tridiagonal form)
* are desired.
*
* T = Q(in) ( D(in) + RHO * Z*Z' ) Q'(in) = Q(out) * D(out) * Q'(out)
*
* where Z = Q'u, u is a vector of length N with ones in the
* CUTPNT and CUTPNT + 1 th elements and zeros elsewhere.
*
* The eigenvectors of the original matrix are stored in Q, and the
* eigenvalues are in D. The algorithm consists of three stages:
*
* The first stage consists of deflating the size of the problem
* when there are multiple eigenvalues or if there is a zero in
* the Z vector. For each such occurence the dimension of the
* secular equation problem is reduced by one. This stage is
* performed by the routine DLAED2.
*
* The second stage consists of calculating the updated
* eigenvalues. This is done by finding the roots of the secular
* equation via the routine DLAED4 (as called by DLAED3).
* This routine also calculates the eigenvectors of the current
* problem.
*
* The final stage consists of computing the updated eigenvectors
* directly using the updated eigenvalues. The eigenvectors for
* the current problem are multiplied with the eigenvectors from
* the overall problem.
*
* Arguments
* =========
*
* N (input) INTEGER
* The dimension of the symmetric tridiagonal matrix. N >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the eigenvalues of the rank-1-perturbed matrix.
* On exit, the eigenvalues of the repaired matrix.
*
* Q (input/output) DOUBLE PRECISION array, dimension (LDQ,N)
* On entry, the eigenvectors of the rank-1-perturbed matrix.
* On exit, the eigenvectors of the repaired tridiagonal matrix.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N).
*
* INDXQ (input/output) INTEGER array, dimension (N)
* On entry, the permutation which separately sorts the two
* subproblems in D into ascending order.
* On exit, the permutation which will reintegrate the
* subproblems back into sorted order,
* i.e. D( INDXQ( I = 1, N ) ) will be in ascending order.
*
* RHO (input) DOUBLE PRECISION
* The subdiagonal entry used to create the rank-1 modification.
*
* CUTPNT (input) INTEGER
* The location of the last eigenvalue in the leading sub-matrix.
* min(1,N) <= CUTPNT <= N/2.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (4*N + N**2)
*
* IWORK (workspace) INTEGER array, dimension (4*N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an eigenvalue did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
* Modified by Francoise Tisseur, University of Tennessee.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n
* @param d
* @param q
* @param ldq
* @param indxq
* @param rho
* @param cutpnt
* @param work
* @param iwork
* @param info
*
*/
abstract public void dlaed1(int n, double[] d, double[] q, int ldq, int[] indxq, org.netlib.util.doubleW rho, int cutpnt, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAED1 computes the updated eigensystem of a diagonal
* matrix after modification by a rank-one symmetric matrix. This
* routine is used only for the eigenproblem which requires all
* eigenvalues and eigenvectors of a tridiagonal matrix. DLAED7 handles
* the case in which eigenvalues only or eigenvalues and eigenvectors
* of a full symmetric matrix (which was reduced to tridiagonal form)
* are desired.
*
* T = Q(in) ( D(in) + RHO * Z*Z' ) Q'(in) = Q(out) * D(out) * Q'(out)
*
* where Z = Q'u, u is a vector of length N with ones in the
* CUTPNT and CUTPNT + 1 th elements and zeros elsewhere.
*
* The eigenvectors of the original matrix are stored in Q, and the
* eigenvalues are in D. The algorithm consists of three stages:
*
* The first stage consists of deflating the size of the problem
* when there are multiple eigenvalues or if there is a zero in
* the Z vector. For each such occurence the dimension of the
* secular equation problem is reduced by one. This stage is
* performed by the routine DLAED2.
*
* The second stage consists of calculating the updated
* eigenvalues. This is done by finding the roots of the secular
* equation via the routine DLAED4 (as called by DLAED3).
* This routine also calculates the eigenvectors of the current
* problem.
*
* The final stage consists of computing the updated eigenvectors
* directly using the updated eigenvalues. The eigenvectors for
* the current problem are multiplied with the eigenvectors from
* the overall problem.
*
* Arguments
* =========
*
* N (input) INTEGER
* The dimension of the symmetric tridiagonal matrix. N >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the eigenvalues of the rank-1-perturbed matrix.
* On exit, the eigenvalues of the repaired matrix.
*
* Q (input/output) DOUBLE PRECISION array, dimension (LDQ,N)
* On entry, the eigenvectors of the rank-1-perturbed matrix.
* On exit, the eigenvectors of the repaired tridiagonal matrix.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N).
*
* INDXQ (input/output) INTEGER array, dimension (N)
* On entry, the permutation which separately sorts the two
* subproblems in D into ascending order.
* On exit, the permutation which will reintegrate the
* subproblems back into sorted order,
* i.e. D( INDXQ( I = 1, N ) ) will be in ascending order.
*
* RHO (input) DOUBLE PRECISION
* The subdiagonal entry used to create the rank-1 modification.
*
* CUTPNT (input) INTEGER
* The location of the last eigenvalue in the leading sub-matrix.
* min(1,N) <= CUTPNT <= N/2.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (4*N + N**2)
*
* IWORK (workspace) INTEGER array, dimension (4*N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an eigenvalue did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
* Modified by Francoise Tisseur, University of Tennessee.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n
* @param d
* @param _d_offset
* @param q
* @param _q_offset
* @param ldq
* @param indxq
* @param _indxq_offset
* @param rho
* @param cutpnt
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dlaed1(int n, double[] d, int _d_offset, double[] q, int _q_offset, int ldq, int[] indxq, int _indxq_offset, org.netlib.util.doubleW rho, int cutpnt, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAED2 merges the two sets of eigenvalues together into a single
* sorted set. Then it tries to deflate the size of the problem.
* There are two ways in which deflation can occur: when two or more
* eigenvalues are close together or if there is a tiny entry in the
* Z vector. For each such occurrence the order of the related secular
* equation problem is reduced by one.
*
* Arguments
* =========
*
* K (output) INTEGER
* The number of non-deflated eigenvalues, and the order of the
* related secular equation. 0 <= K <=N.
*
* N (input) INTEGER
* The dimension of the symmetric tridiagonal matrix. N >= 0.
*
* N1 (input) INTEGER
* The location of the last eigenvalue in the leading sub-matrix.
* min(1,N) <= N1 <= N/2.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, D contains the eigenvalues of the two submatrices to
* be combined.
* On exit, D contains the trailing (N-K) updated eigenvalues
* (those which were deflated) sorted into increasing order.
*
* Q (input/output) DOUBLE PRECISION array, dimension (LDQ, N)
* On entry, Q contains the eigenvectors of two submatrices in
* the two square blocks with corners at (1,1), (N1,N1)
* and (N1+1, N1+1), (N,N).
* On exit, Q contains the trailing (N-K) updated eigenvectors
* (those which were deflated) in its last N-K columns.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N).
*
* INDXQ (input/output) INTEGER array, dimension (N)
* The permutation which separately sorts the two sub-problems
* in D into ascending order. Note that elements in the second
* half of this permutation must first have N1 added to their
* values. Destroyed on exit.
*
* RHO (input/output) DOUBLE PRECISION
* On entry, the off-diagonal element associated with the rank-1
* cut which originally split the two submatrices which are now
* being recombined.
* On exit, RHO has been modified to the value required by
* DLAED3.
*
* Z (input) DOUBLE PRECISION array, dimension (N)
* On entry, Z contains the updating vector (the last
* row of the first sub-eigenvector matrix and the first row of
* the second sub-eigenvector matrix).
* On exit, the contents of Z have been destroyed by the updating
* process.
*
* DLAMDA (output) DOUBLE PRECISION array, dimension (N)
* A copy of the first K eigenvalues which will be used by
* DLAED3 to form the secular equation.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* The first k values of the final deflation-altered z-vector
* which will be passed to DLAED3.
*
* Q2 (output) DOUBLE PRECISION array, dimension (N1**2+(N-N1)**2)
* A copy of the first K eigenvectors which will be used by
* DLAED3 in a matrix multiply (DGEMM) to solve for the new
* eigenvectors.
*
* INDX (workspace) INTEGER array, dimension (N)
* The permutation used to sort the contents of DLAMDA into
* ascending order.
*
* INDXC (output) INTEGER array, dimension (N)
* The permutation used to arrange the columns of the deflated
* Q matrix into three groups: the first group contains non-zero
* elements only at and above N1, the second contains
* non-zero elements only below N1, and the third is dense.
*
* INDXP (workspace) INTEGER array, dimension (N)
* The permutation used to place deflated values of D at the end
* of the array. INDXP(1:K) points to the nondeflated D-values
* and INDXP(K+1:N) points to the deflated eigenvalues.
*
* COLTYP (workspace/output) INTEGER array, dimension (N)
* During execution, a label which will indicate which of the
* following types a column in the Q2 matrix is:
* 1 : non-zero in the upper half only;
* 2 : dense;
* 3 : non-zero in the lower half only;
* 4 : deflated.
* On exit, COLTYP(i) is the number of columns of type i,
* for i=1 to 4 only.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
* Modified by Francoise Tisseur, University of Tennessee.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param k
* @param n
* @param n1
* @param d
* @param q
* @param ldq
* @param indxq
* @param rho
* @param z
* @param dlamda
* @param w
* @param q2
* @param indx
* @param indxc
* @param indxp
* @param coltyp
* @param info
*
*/
abstract public void dlaed2(org.netlib.util.intW k, int n, int n1, double[] d, double[] q, int ldq, int[] indxq, org.netlib.util.doubleW rho, double[] z, double[] dlamda, double[] w, double[] q2, int[] indx, int[] indxc, int[] indxp, int[] coltyp, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAED2 merges the two sets of eigenvalues together into a single
* sorted set. Then it tries to deflate the size of the problem.
* There are two ways in which deflation can occur: when two or more
* eigenvalues are close together or if there is a tiny entry in the
* Z vector. For each such occurrence the order of the related secular
* equation problem is reduced by one.
*
* Arguments
* =========
*
* K (output) INTEGER
* The number of non-deflated eigenvalues, and the order of the
* related secular equation. 0 <= K <=N.
*
* N (input) INTEGER
* The dimension of the symmetric tridiagonal matrix. N >= 0.
*
* N1 (input) INTEGER
* The location of the last eigenvalue in the leading sub-matrix.
* min(1,N) <= N1 <= N/2.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, D contains the eigenvalues of the two submatrices to
* be combined.
* On exit, D contains the trailing (N-K) updated eigenvalues
* (those which were deflated) sorted into increasing order.
*
* Q (input/output) DOUBLE PRECISION array, dimension (LDQ, N)
* On entry, Q contains the eigenvectors of two submatrices in
* the two square blocks with corners at (1,1), (N1,N1)
* and (N1+1, N1+1), (N,N).
* On exit, Q contains the trailing (N-K) updated eigenvectors
* (those which were deflated) in its last N-K columns.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N).
*
* INDXQ (input/output) INTEGER array, dimension (N)
* The permutation which separately sorts the two sub-problems
* in D into ascending order. Note that elements in the second
* half of this permutation must first have N1 added to their
* values. Destroyed on exit.
*
* RHO (input/output) DOUBLE PRECISION
* On entry, the off-diagonal element associated with the rank-1
* cut which originally split the two submatrices which are now
* being recombined.
* On exit, RHO has been modified to the value required by
* DLAED3.
*
* Z (input) DOUBLE PRECISION array, dimension (N)
* On entry, Z contains the updating vector (the last
* row of the first sub-eigenvector matrix and the first row of
* the second sub-eigenvector matrix).
* On exit, the contents of Z have been destroyed by the updating
* process.
*
* DLAMDA (output) DOUBLE PRECISION array, dimension (N)
* A copy of the first K eigenvalues which will be used by
* DLAED3 to form the secular equation.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* The first k values of the final deflation-altered z-vector
* which will be passed to DLAED3.
*
* Q2 (output) DOUBLE PRECISION array, dimension (N1**2+(N-N1)**2)
* A copy of the first K eigenvectors which will be used by
* DLAED3 in a matrix multiply (DGEMM) to solve for the new
* eigenvectors.
*
* INDX (workspace) INTEGER array, dimension (N)
* The permutation used to sort the contents of DLAMDA into
* ascending order.
*
* INDXC (output) INTEGER array, dimension (N)
* The permutation used to arrange the columns of the deflated
* Q matrix into three groups: the first group contains non-zero
* elements only at and above N1, the second contains
* non-zero elements only below N1, and the third is dense.
*
* INDXP (workspace) INTEGER array, dimension (N)
* The permutation used to place deflated values of D at the end
* of the array. INDXP(1:K) points to the nondeflated D-values
* and INDXP(K+1:N) points to the deflated eigenvalues.
*
* COLTYP (workspace/output) INTEGER array, dimension (N)
* During execution, a label which will indicate which of the
* following types a column in the Q2 matrix is:
* 1 : non-zero in the upper half only;
* 2 : dense;
* 3 : non-zero in the lower half only;
* 4 : deflated.
* On exit, COLTYP(i) is the number of columns of type i,
* for i=1 to 4 only.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
* Modified by Francoise Tisseur, University of Tennessee.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param k
* @param n
* @param n1
* @param d
* @param _d_offset
* @param q
* @param _q_offset
* @param ldq
* @param indxq
* @param _indxq_offset
* @param rho
* @param z
* @param _z_offset
* @param dlamda
* @param _dlamda_offset
* @param w
* @param _w_offset
* @param q2
* @param _q2_offset
* @param indx
* @param _indx_offset
* @param indxc
* @param _indxc_offset
* @param indxp
* @param _indxp_offset
* @param coltyp
* @param _coltyp_offset
* @param info
*
*/
abstract public void dlaed2(org.netlib.util.intW k, int n, int n1, double[] d, int _d_offset, double[] q, int _q_offset, int ldq, int[] indxq, int _indxq_offset, org.netlib.util.doubleW rho, double[] z, int _z_offset, double[] dlamda, int _dlamda_offset, double[] w, int _w_offset, double[] q2, int _q2_offset, int[] indx, int _indx_offset, int[] indxc, int _indxc_offset, int[] indxp, int _indxp_offset, int[] coltyp, int _coltyp_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAED3 finds the roots of the secular equation, as defined by the
* values in D, W, and RHO, between 1 and K. It makes the
* appropriate calls to DLAED4 and then updates the eigenvectors by
* multiplying the matrix of eigenvectors of the pair of eigensystems
* being combined by the matrix of eigenvectors of the K-by-K system
* which is solved here.
*
* This code makes very mild assumptions about floating point
* arithmetic. It will work on machines with a guard digit in
* add/subtract, or on those binary machines without guard digits
* which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or Cray-2.
* It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* K (input) INTEGER
* The number of terms in the rational function to be solved by
* DLAED4. K >= 0.
*
* N (input) INTEGER
* The number of rows and columns in the Q matrix.
* N >= K (deflation may result in N>K).
*
* N1 (input) INTEGER
* The location of the last eigenvalue in the leading submatrix.
* min(1,N) <= N1 <= N/2.
*
* D (output) DOUBLE PRECISION array, dimension (N)
* D(I) contains the updated eigenvalues for
* 1 <= I <= K.
*
* Q (output) DOUBLE PRECISION array, dimension (LDQ,N)
* Initially the first K columns are used as workspace.
* On output the columns 1 to K contain
* the updated eigenvectors.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N).
*
* RHO (input) DOUBLE PRECISION
* The value of the parameter in the rank one update equation.
* RHO >= 0 required.
*
* DLAMDA (input/output) DOUBLE PRECISION array, dimension (K)
* The first K elements of this array contain the old roots
* of the deflated updating problem. These are the poles
* of the secular equation. May be changed on output by
* having lowest order bit set to zero on Cray X-MP, Cray Y-MP,
* Cray-2, or Cray C-90, as described above.
*
* Q2 (input) DOUBLE PRECISION array, dimension (LDQ2, N)
* The first K columns of this matrix contain the non-deflated
* eigenvectors for the split problem.
*
* INDX (input) INTEGER array, dimension (N)
* The permutation used to arrange the columns of the deflated
* Q matrix into three groups (see DLAED2).
* The rows of the eigenvectors found by DLAED4 must be likewise
* permuted before the matrix multiply can take place.
*
* CTOT (input) INTEGER array, dimension (4)
* A count of the total number of the various types of columns
* in Q, as described in INDX. The fourth column type is any
* column which has been deflated.
*
* W (input/output) DOUBLE PRECISION array, dimension (K)
* The first K elements of this array contain the components
* of the deflation-adjusted updating vector. Destroyed on
* output.
*
* S (workspace) DOUBLE PRECISION array, dimension (N1 + 1)*K
* Will contain the eigenvectors of the repaired matrix which
* will be multiplied by the previously accumulated eigenvectors
* to update the system.
*
* LDS (input) INTEGER
* The leading dimension of S. LDS >= max(1,K).
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an eigenvalue did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
* Modified by Francoise Tisseur, University of Tennessee.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param k
* @param n
* @param n1
* @param d
* @param q
* @param ldq
* @param rho
* @param dlamda
* @param q2
* @param indx
* @param ctot
* @param w
* @param s
* @param info
*
*/
abstract public void dlaed3(int k, int n, int n1, double[] d, double[] q, int ldq, double rho, double[] dlamda, double[] q2, int[] indx, int[] ctot, double[] w, double[] s, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAED3 finds the roots of the secular equation, as defined by the
* values in D, W, and RHO, between 1 and K. It makes the
* appropriate calls to DLAED4 and then updates the eigenvectors by
* multiplying the matrix of eigenvectors of the pair of eigensystems
* being combined by the matrix of eigenvectors of the K-by-K system
* which is solved here.
*
* This code makes very mild assumptions about floating point
* arithmetic. It will work on machines with a guard digit in
* add/subtract, or on those binary machines without guard digits
* which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or Cray-2.
* It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* K (input) INTEGER
* The number of terms in the rational function to be solved by
* DLAED4. K >= 0.
*
* N (input) INTEGER
* The number of rows and columns in the Q matrix.
* N >= K (deflation may result in N>K).
*
* N1 (input) INTEGER
* The location of the last eigenvalue in the leading submatrix.
* min(1,N) <= N1 <= N/2.
*
* D (output) DOUBLE PRECISION array, dimension (N)
* D(I) contains the updated eigenvalues for
* 1 <= I <= K.
*
* Q (output) DOUBLE PRECISION array, dimension (LDQ,N)
* Initially the first K columns are used as workspace.
* On output the columns 1 to K contain
* the updated eigenvectors.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N).
*
* RHO (input) DOUBLE PRECISION
* The value of the parameter in the rank one update equation.
* RHO >= 0 required.
*
* DLAMDA (input/output) DOUBLE PRECISION array, dimension (K)
* The first K elements of this array contain the old roots
* of the deflated updating problem. These are the poles
* of the secular equation. May be changed on output by
* having lowest order bit set to zero on Cray X-MP, Cray Y-MP,
* Cray-2, or Cray C-90, as described above.
*
* Q2 (input) DOUBLE PRECISION array, dimension (LDQ2, N)
* The first K columns of this matrix contain the non-deflated
* eigenvectors for the split problem.
*
* INDX (input) INTEGER array, dimension (N)
* The permutation used to arrange the columns of the deflated
* Q matrix into three groups (see DLAED2).
* The rows of the eigenvectors found by DLAED4 must be likewise
* permuted before the matrix multiply can take place.
*
* CTOT (input) INTEGER array, dimension (4)
* A count of the total number of the various types of columns
* in Q, as described in INDX. The fourth column type is any
* column which has been deflated.
*
* W (input/output) DOUBLE PRECISION array, dimension (K)
* The first K elements of this array contain the components
* of the deflation-adjusted updating vector. Destroyed on
* output.
*
* S (workspace) DOUBLE PRECISION array, dimension (N1 + 1)*K
* Will contain the eigenvectors of the repaired matrix which
* will be multiplied by the previously accumulated eigenvectors
* to update the system.
*
* LDS (input) INTEGER
* The leading dimension of S. LDS >= max(1,K).
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an eigenvalue did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
* Modified by Francoise Tisseur, University of Tennessee.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param k
* @param n
* @param n1
* @param d
* @param _d_offset
* @param q
* @param _q_offset
* @param ldq
* @param rho
* @param dlamda
* @param _dlamda_offset
* @param q2
* @param _q2_offset
* @param indx
* @param _indx_offset
* @param ctot
* @param _ctot_offset
* @param w
* @param _w_offset
* @param s
* @param _s_offset
* @param info
*
*/
abstract public void dlaed3(int k, int n, int n1, double[] d, int _d_offset, double[] q, int _q_offset, int ldq, double rho, double[] dlamda, int _dlamda_offset, double[] q2, int _q2_offset, int[] indx, int _indx_offset, int[] ctot, int _ctot_offset, double[] w, int _w_offset, double[] s, int _s_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* This subroutine computes the I-th updated eigenvalue of a symmetric
* rank-one modification to a diagonal matrix whose elements are
* given in the array d, and that
*
* D(i) < D(j) for i < j
*
* and that RHO > 0. This is arranged by the calling routine, and is
* no loss in generality. The rank-one modified system is thus
*
* diag( D ) + RHO * Z * Z_transpose.
*
* where we assume the Euclidean norm of Z is 1.
*
* The method consists of approximating the rational functions in the
* secular equation by simpler interpolating rational functions.
*
* Arguments
* =========
*
* N (input) INTEGER
* The length of all arrays.
*
* I (input) INTEGER
* The index of the eigenvalue to be computed. 1 <= I <= N.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The original eigenvalues. It is assumed that they are in
* order, D(I) < D(J) for I < J.
*
* Z (input) DOUBLE PRECISION array, dimension (N)
* The components of the updating vector.
*
* DELTA (output) DOUBLE PRECISION array, dimension (N)
* If N .GT. 2, DELTA contains (D(j) - lambda_I) in its j-th
* component. If N = 1, then DELTA(1) = 1. If N = 2, see DLAED5
* for detail. The vector DELTA contains the information necessar
* to construct the eigenvectors by DLAED3 and DLAED9.
*
* RHO (input) DOUBLE PRECISION
* The scalar in the symmetric updating formula.
*
* DLAM (output) DOUBLE PRECISION
* The computed lambda_I, the I-th updated eigenvalue.
*
* INFO (output) INTEGER
* = 0: successful exit
* > 0: if INFO = 1, the updating process failed.
*
* Internal Parameters
* ===================
*
* Logical variable ORGATI (origin-at-i?) is used for distinguishing
* whether D(i) or D(i+1) is treated as the origin.
*
* ORGATI = .true. origin at i
* ORGATI = .false. origin at i+1
*
* Logical variable SWTCH3 (switch-for-3-poles?) is for noting
* if we are working with THREE poles!
*
* MAXIT is the maximum number of iterations allowed for each
* eigenvalue.
*
* Further Details
* ===============
*
* Based on contributions by
* Ren-Cang Li, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param i
* @param d
* @param z
* @param delta
* @param rho
* @param dlam
* @param info
*
*/
abstract public void dlaed4(int n, int i, double[] d, double[] z, double[] delta, double rho, org.netlib.util.doubleW dlam, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* This subroutine computes the I-th updated eigenvalue of a symmetric
* rank-one modification to a diagonal matrix whose elements are
* given in the array d, and that
*
* D(i) < D(j) for i < j
*
* and that RHO > 0. This is arranged by the calling routine, and is
* no loss in generality. The rank-one modified system is thus
*
* diag( D ) + RHO * Z * Z_transpose.
*
* where we assume the Euclidean norm of Z is 1.
*
* The method consists of approximating the rational functions in the
* secular equation by simpler interpolating rational functions.
*
* Arguments
* =========
*
* N (input) INTEGER
* The length of all arrays.
*
* I (input) INTEGER
* The index of the eigenvalue to be computed. 1 <= I <= N.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The original eigenvalues. It is assumed that they are in
* order, D(I) < D(J) for I < J.
*
* Z (input) DOUBLE PRECISION array, dimension (N)
* The components of the updating vector.
*
* DELTA (output) DOUBLE PRECISION array, dimension (N)
* If N .GT. 2, DELTA contains (D(j) - lambda_I) in its j-th
* component. If N = 1, then DELTA(1) = 1. If N = 2, see DLAED5
* for detail. The vector DELTA contains the information necessar
* to construct the eigenvectors by DLAED3 and DLAED9.
*
* RHO (input) DOUBLE PRECISION
* The scalar in the symmetric updating formula.
*
* DLAM (output) DOUBLE PRECISION
* The computed lambda_I, the I-th updated eigenvalue.
*
* INFO (output) INTEGER
* = 0: successful exit
* > 0: if INFO = 1, the updating process failed.
*
* Internal Parameters
* ===================
*
* Logical variable ORGATI (origin-at-i?) is used for distinguishing
* whether D(i) or D(i+1) is treated as the origin.
*
* ORGATI = .true. origin at i
* ORGATI = .false. origin at i+1
*
* Logical variable SWTCH3 (switch-for-3-poles?) is for noting
* if we are working with THREE poles!
*
* MAXIT is the maximum number of iterations allowed for each
* eigenvalue.
*
* Further Details
* ===============
*
* Based on contributions by
* Ren-Cang Li, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param i
* @param d
* @param _d_offset
* @param z
* @param _z_offset
* @param delta
* @param _delta_offset
* @param rho
* @param dlam
* @param info
*
*/
abstract public void dlaed4(int n, int i, double[] d, int _d_offset, double[] z, int _z_offset, double[] delta, int _delta_offset, double rho, org.netlib.util.doubleW dlam, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* This subroutine computes the I-th eigenvalue of a symmetric rank-one
* modification of a 2-by-2 diagonal matrix
*
* diag( D ) + RHO * Z * transpose(Z) .
*
* The diagonal elements in the array D are assumed to satisfy
*
* D(i) < D(j) for i < j .
*
* We also assume RHO > 0 and that the Euclidean norm of the vector
* Z is one.
*
* Arguments
* =========
*
* I (input) INTEGER
* The index of the eigenvalue to be computed. I = 1 or I = 2.
*
* D (input) DOUBLE PRECISION array, dimension (2)
* The original eigenvalues. We assume D(1) < D(2).
*
* Z (input) DOUBLE PRECISION array, dimension (2)
* The components of the updating vector.
*
* DELTA (output) DOUBLE PRECISION array, dimension (2)
* The vector DELTA contains the information necessary
* to construct the eigenvectors.
*
* RHO (input) DOUBLE PRECISION
* The scalar in the symmetric updating formula.
*
* DLAM (output) DOUBLE PRECISION
* The computed lambda_I, the I-th updated eigenvalue.
*
* Further Details
* ===============
*
* Based on contributions by
* Ren-Cang Li, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param i
* @param d
* @param z
* @param delta
* @param rho
* @param dlam
*
*/
abstract public void dlaed5(int i, double[] d, double[] z, double[] delta, double rho, org.netlib.util.doubleW dlam);
/**
*
* ..
*
* Purpose
* =======
*
* This subroutine computes the I-th eigenvalue of a symmetric rank-one
* modification of a 2-by-2 diagonal matrix
*
* diag( D ) + RHO * Z * transpose(Z) .
*
* The diagonal elements in the array D are assumed to satisfy
*
* D(i) < D(j) for i < j .
*
* We also assume RHO > 0 and that the Euclidean norm of the vector
* Z is one.
*
* Arguments
* =========
*
* I (input) INTEGER
* The index of the eigenvalue to be computed. I = 1 or I = 2.
*
* D (input) DOUBLE PRECISION array, dimension (2)
* The original eigenvalues. We assume D(1) < D(2).
*
* Z (input) DOUBLE PRECISION array, dimension (2)
* The components of the updating vector.
*
* DELTA (output) DOUBLE PRECISION array, dimension (2)
* The vector DELTA contains the information necessary
* to construct the eigenvectors.
*
* RHO (input) DOUBLE PRECISION
* The scalar in the symmetric updating formula.
*
* DLAM (output) DOUBLE PRECISION
* The computed lambda_I, the I-th updated eigenvalue.
*
* Further Details
* ===============
*
* Based on contributions by
* Ren-Cang Li, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param i
* @param d
* @param _d_offset
* @param z
* @param _z_offset
* @param delta
* @param _delta_offset
* @param rho
* @param dlam
*
*/
abstract public void dlaed5(int i, double[] d, int _d_offset, double[] z, int _z_offset, double[] delta, int _delta_offset, double rho, org.netlib.util.doubleW dlam);
/**
*
* ..
*
* Purpose
* =======
*
* DLAED6 computes the positive or negative root (closest to the origin)
* of
* z(1) z(2) z(3)
* f(x) = rho + --------- + ---------- + ---------
* d(1)-x d(2)-x d(3)-x
*
* It is assumed that
*
* if ORGATI = .true. the root is between d(2) and d(3);
* otherwise it is between d(1) and d(2)
*
* This routine will be called by DLAED4 when necessary. In most cases,
* the root sought is the smallest in magnitude, though it might not be
* in some extremely rare situations.
*
* Arguments
* =========
*
* KNITER (input) INTEGER
* Refer to DLAED4 for its significance.
*
* ORGATI (input) LOGICAL
* If ORGATI is true, the needed root is between d(2) and
* d(3); otherwise it is between d(1) and d(2). See
* DLAED4 for further details.
*
* RHO (input) DOUBLE PRECISION
* Refer to the equation f(x) above.
*
* D (input) DOUBLE PRECISION array, dimension (3)
* D satisfies d(1) < d(2) < d(3).
*
* Z (input) DOUBLE PRECISION array, dimension (3)
* Each of the elements in z must be positive.
*
* FINIT (input) DOUBLE PRECISION
* The value of f at 0. It is more accurate than the one
* evaluated inside this routine (if someone wants to do
* so).
*
* TAU (output) DOUBLE PRECISION
* The root of the equation f(x).
*
* INFO (output) INTEGER
* = 0: successful exit
* > 0: if INFO = 1, failure to converge
*
* Further Details
* ===============
*
* 30/06/99: Based on contributions by
* Ren-Cang Li, Computer Science Division, University of California
* at Berkeley, USA
*
* 10/02/03: This version has a few statements commented out for thread
* safety (machine parameters are computed on each entry). SJH.
*
* 05/10/06: Modified from a new version of Ren-Cang Li, use
* Gragg-Thornton-Warner cubic convergent scheme for better stability
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param kniter
* @param orgati
* @param rho
* @param d
* @param z
* @param finit
* @param tau
* @param info
*
*/
abstract public void dlaed6(int kniter, boolean orgati, double rho, double[] d, double[] z, double finit, org.netlib.util.doubleW tau, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAED6 computes the positive or negative root (closest to the origin)
* of
* z(1) z(2) z(3)
* f(x) = rho + --------- + ---------- + ---------
* d(1)-x d(2)-x d(3)-x
*
* It is assumed that
*
* if ORGATI = .true. the root is between d(2) and d(3);
* otherwise it is between d(1) and d(2)
*
* This routine will be called by DLAED4 when necessary. In most cases,
* the root sought is the smallest in magnitude, though it might not be
* in some extremely rare situations.
*
* Arguments
* =========
*
* KNITER (input) INTEGER
* Refer to DLAED4 for its significance.
*
* ORGATI (input) LOGICAL
* If ORGATI is true, the needed root is between d(2) and
* d(3); otherwise it is between d(1) and d(2). See
* DLAED4 for further details.
*
* RHO (input) DOUBLE PRECISION
* Refer to the equation f(x) above.
*
* D (input) DOUBLE PRECISION array, dimension (3)
* D satisfies d(1) < d(2) < d(3).
*
* Z (input) DOUBLE PRECISION array, dimension (3)
* Each of the elements in z must be positive.
*
* FINIT (input) DOUBLE PRECISION
* The value of f at 0. It is more accurate than the one
* evaluated inside this routine (if someone wants to do
* so).
*
* TAU (output) DOUBLE PRECISION
* The root of the equation f(x).
*
* INFO (output) INTEGER
* = 0: successful exit
* > 0: if INFO = 1, failure to converge
*
* Further Details
* ===============
*
* 30/06/99: Based on contributions by
* Ren-Cang Li, Computer Science Division, University of California
* at Berkeley, USA
*
* 10/02/03: This version has a few statements commented out for thread
* safety (machine parameters are computed on each entry). SJH.
*
* 05/10/06: Modified from a new version of Ren-Cang Li, use
* Gragg-Thornton-Warner cubic convergent scheme for better stability
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param kniter
* @param orgati
* @param rho
* @param d
* @param _d_offset
* @param z
* @param _z_offset
* @param finit
* @param tau
* @param info
*
*/
abstract public void dlaed6(int kniter, boolean orgati, double rho, double[] d, int _d_offset, double[] z, int _z_offset, double finit, org.netlib.util.doubleW tau, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAED7 computes the updated eigensystem of a diagonal
* matrix after modification by a rank-one symmetric matrix. This
* routine is used only for the eigenproblem which requires all
* eigenvalues and optionally eigenvectors of a dense symmetric matrix
* that has been reduced to tridiagonal form. DLAED1 handles
* the case in which all eigenvalues and eigenvectors of a symmetric
* tridiagonal matrix are desired.
*
* T = Q(in) ( D(in) + RHO * Z*Z' ) Q'(in) = Q(out) * D(out) * Q'(out)
*
* where Z = Q'u, u is a vector of length N with ones in the
* CUTPNT and CUTPNT + 1 th elements and zeros elsewhere.
*
* The eigenvectors of the original matrix are stored in Q, and the
* eigenvalues are in D. The algorithm consists of three stages:
*
* The first stage consists of deflating the size of the problem
* when there are multiple eigenvalues or if there is a zero in
* the Z vector. For each such occurence the dimension of the
* secular equation problem is reduced by one. This stage is
* performed by the routine DLAED8.
*
* The second stage consists of calculating the updated
* eigenvalues. This is done by finding the roots of the secular
* equation via the routine DLAED4 (as called by DLAED9).
* This routine also calculates the eigenvectors of the current
* problem.
*
* The final stage consists of computing the updated eigenvectors
* directly using the updated eigenvalues. The eigenvectors for
* the current problem are multiplied with the eigenvectors from
* the overall problem.
*
* Arguments
* =========
*
* ICOMPQ (input) INTEGER
* = 0: Compute eigenvalues only.
* = 1: Compute eigenvectors of original dense symmetric matrix
* also. On entry, Q contains the orthogonal matrix used
* to reduce the original matrix to tridiagonal form.
*
* N (input) INTEGER
* The dimension of the symmetric tridiagonal matrix. N >= 0.
*
* QSIZ (input) INTEGER
* The dimension of the orthogonal matrix used to reduce
* the full matrix to tridiagonal form. QSIZ >= N if ICOMPQ = 1.
*
* TLVLS (input) INTEGER
* The total number of merging levels in the overall divide and
* conquer tree.
*
* CURLVL (input) INTEGER
* The current level in the overall merge routine,
* 0 <= CURLVL <= TLVLS.
*
* CURPBM (input) INTEGER
* The current problem in the current level in the overall
* merge routine (counting from upper left to lower right).
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the eigenvalues of the rank-1-perturbed matrix.
* On exit, the eigenvalues of the repaired matrix.
*
* Q (input/output) DOUBLE PRECISION array, dimension (LDQ, N)
* On entry, the eigenvectors of the rank-1-perturbed matrix.
* On exit, the eigenvectors of the repaired tridiagonal matrix.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N).
*
* INDXQ (output) INTEGER array, dimension (N)
* The permutation which will reintegrate the subproblem just
* solved back into sorted order, i.e., D( INDXQ( I = 1, N ) )
* will be in ascending order.
*
* RHO (input) DOUBLE PRECISION
* The subdiagonal element used to create the rank-1
* modification.
*
* CUTPNT (input) INTEGER
* Contains the location of the last eigenvalue in the leading
* sub-matrix. min(1,N) <= CUTPNT <= N.
*
* QSTORE (input/output) DOUBLE PRECISION array, dimension (N**2+1)
* Stores eigenvectors of submatrices encountered during
* divide and conquer, packed together. QPTR points to
* beginning of the submatrices.
*
* QPTR (input/output) INTEGER array, dimension (N+2)
* List of indices pointing to beginning of submatrices stored
* in QSTORE. The submatrices are numbered starting at the
* bottom left of the divide and conquer tree, from left to
* right and bottom to top.
*
* PRMPTR (input) INTEGER array, dimension (N lg N)
* Contains a list of pointers which indicate where in PERM a
* level's permutation is stored. PRMPTR(i+1) - PRMPTR(i)
* indicates the size of the permutation and also the size of
* the full, non-deflated problem.
*
* PERM (input) INTEGER array, dimension (N lg N)
* Contains the permutations (from deflation and sorting) to be
* applied to each eigenblock.
*
* GIVPTR (input) INTEGER array, dimension (N lg N)
* Contains a list of pointers which indicate where in GIVCOL a
* level's Givens rotations are stored. GIVPTR(i+1) - GIVPTR(i)
* indicates the number of Givens rotations.
*
* GIVCOL (input) INTEGER array, dimension (2, N lg N)
* Each pair of numbers indicates a pair of columns to take place
* in a Givens rotation.
*
* GIVNUM (input) DOUBLE PRECISION array, dimension (2, N lg N)
* Each number indicates the S value to be used in the
* corresponding Givens rotation.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N+QSIZ*N)
*
* IWORK (workspace) INTEGER array, dimension (4*N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an eigenvalue did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param n
* @param qsiz
* @param tlvls
* @param curlvl
* @param curpbm
* @param d
* @param q
* @param ldq
* @param indxq
* @param rho
* @param cutpnt
* @param qstore
* @param qptr
* @param prmptr
* @param perm
* @param givptr
* @param givcol
* @param givnum
* @param work
* @param iwork
* @param info
*
*/
abstract public void dlaed7(int icompq, int n, int qsiz, int tlvls, int curlvl, int curpbm, double[] d, double[] q, int ldq, int[] indxq, org.netlib.util.doubleW rho, int cutpnt, double[] qstore, int[] qptr, int[] prmptr, int[] perm, int[] givptr, int[] givcol, double[] givnum, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAED7 computes the updated eigensystem of a diagonal
* matrix after modification by a rank-one symmetric matrix. This
* routine is used only for the eigenproblem which requires all
* eigenvalues and optionally eigenvectors of a dense symmetric matrix
* that has been reduced to tridiagonal form. DLAED1 handles
* the case in which all eigenvalues and eigenvectors of a symmetric
* tridiagonal matrix are desired.
*
* T = Q(in) ( D(in) + RHO * Z*Z' ) Q'(in) = Q(out) * D(out) * Q'(out)
*
* where Z = Q'u, u is a vector of length N with ones in the
* CUTPNT and CUTPNT + 1 th elements and zeros elsewhere.
*
* The eigenvectors of the original matrix are stored in Q, and the
* eigenvalues are in D. The algorithm consists of three stages:
*
* The first stage consists of deflating the size of the problem
* when there are multiple eigenvalues or if there is a zero in
* the Z vector. For each such occurence the dimension of the
* secular equation problem is reduced by one. This stage is
* performed by the routine DLAED8.
*
* The second stage consists of calculating the updated
* eigenvalues. This is done by finding the roots of the secular
* equation via the routine DLAED4 (as called by DLAED9).
* This routine also calculates the eigenvectors of the current
* problem.
*
* The final stage consists of computing the updated eigenvectors
* directly using the updated eigenvalues. The eigenvectors for
* the current problem are multiplied with the eigenvectors from
* the overall problem.
*
* Arguments
* =========
*
* ICOMPQ (input) INTEGER
* = 0: Compute eigenvalues only.
* = 1: Compute eigenvectors of original dense symmetric matrix
* also. On entry, Q contains the orthogonal matrix used
* to reduce the original matrix to tridiagonal form.
*
* N (input) INTEGER
* The dimension of the symmetric tridiagonal matrix. N >= 0.
*
* QSIZ (input) INTEGER
* The dimension of the orthogonal matrix used to reduce
* the full matrix to tridiagonal form. QSIZ >= N if ICOMPQ = 1.
*
* TLVLS (input) INTEGER
* The total number of merging levels in the overall divide and
* conquer tree.
*
* CURLVL (input) INTEGER
* The current level in the overall merge routine,
* 0 <= CURLVL <= TLVLS.
*
* CURPBM (input) INTEGER
* The current problem in the current level in the overall
* merge routine (counting from upper left to lower right).
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the eigenvalues of the rank-1-perturbed matrix.
* On exit, the eigenvalues of the repaired matrix.
*
* Q (input/output) DOUBLE PRECISION array, dimension (LDQ, N)
* On entry, the eigenvectors of the rank-1-perturbed matrix.
* On exit, the eigenvectors of the repaired tridiagonal matrix.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N).
*
* INDXQ (output) INTEGER array, dimension (N)
* The permutation which will reintegrate the subproblem just
* solved back into sorted order, i.e., D( INDXQ( I = 1, N ) )
* will be in ascending order.
*
* RHO (input) DOUBLE PRECISION
* The subdiagonal element used to create the rank-1
* modification.
*
* CUTPNT (input) INTEGER
* Contains the location of the last eigenvalue in the leading
* sub-matrix. min(1,N) <= CUTPNT <= N.
*
* QSTORE (input/output) DOUBLE PRECISION array, dimension (N**2+1)
* Stores eigenvectors of submatrices encountered during
* divide and conquer, packed together. QPTR points to
* beginning of the submatrices.
*
* QPTR (input/output) INTEGER array, dimension (N+2)
* List of indices pointing to beginning of submatrices stored
* in QSTORE. The submatrices are numbered starting at the
* bottom left of the divide and conquer tree, from left to
* right and bottom to top.
*
* PRMPTR (input) INTEGER array, dimension (N lg N)
* Contains a list of pointers which indicate where in PERM a
* level's permutation is stored. PRMPTR(i+1) - PRMPTR(i)
* indicates the size of the permutation and also the size of
* the full, non-deflated problem.
*
* PERM (input) INTEGER array, dimension (N lg N)
* Contains the permutations (from deflation and sorting) to be
* applied to each eigenblock.
*
* GIVPTR (input) INTEGER array, dimension (N lg N)
* Contains a list of pointers which indicate where in GIVCOL a
* level's Givens rotations are stored. GIVPTR(i+1) - GIVPTR(i)
* indicates the number of Givens rotations.
*
* GIVCOL (input) INTEGER array, dimension (2, N lg N)
* Each pair of numbers indicates a pair of columns to take place
* in a Givens rotation.
*
* GIVNUM (input) DOUBLE PRECISION array, dimension (2, N lg N)
* Each number indicates the S value to be used in the
* corresponding Givens rotation.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N+QSIZ*N)
*
* IWORK (workspace) INTEGER array, dimension (4*N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an eigenvalue did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param n
* @param qsiz
* @param tlvls
* @param curlvl
* @param curpbm
* @param d
* @param _d_offset
* @param q
* @param _q_offset
* @param ldq
* @param indxq
* @param _indxq_offset
* @param rho
* @param cutpnt
* @param qstore
* @param _qstore_offset
* @param qptr
* @param _qptr_offset
* @param prmptr
* @param _prmptr_offset
* @param perm
* @param _perm_offset
* @param givptr
* @param _givptr_offset
* @param givcol
* @param _givcol_offset
* @param givnum
* @param _givnum_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dlaed7(int icompq, int n, int qsiz, int tlvls, int curlvl, int curpbm, double[] d, int _d_offset, double[] q, int _q_offset, int ldq, int[] indxq, int _indxq_offset, org.netlib.util.doubleW rho, int cutpnt, double[] qstore, int _qstore_offset, int[] qptr, int _qptr_offset, int[] prmptr, int _prmptr_offset, int[] perm, int _perm_offset, int[] givptr, int _givptr_offset, int[] givcol, int _givcol_offset, double[] givnum, int _givnum_offset, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAED8 merges the two sets of eigenvalues together into a single
* sorted set. Then it tries to deflate the size of the problem.
* There are two ways in which deflation can occur: when two or more
* eigenvalues are close together or if there is a tiny element in the
* Z vector. For each such occurrence the order of the related secular
* equation problem is reduced by one.
*
* Arguments
* =========
*
* ICOMPQ (input) INTEGER
* = 0: Compute eigenvalues only.
* = 1: Compute eigenvectors of original dense symmetric matrix
* also. On entry, Q contains the orthogonal matrix used
* to reduce the original matrix to tridiagonal form.
*
* K (output) INTEGER
* The number of non-deflated eigenvalues, and the order of the
* related secular equation.
*
* N (input) INTEGER
* The dimension of the symmetric tridiagonal matrix. N >= 0.
*
* QSIZ (input) INTEGER
* The dimension of the orthogonal matrix used to reduce
* the full matrix to tridiagonal form. QSIZ >= N if ICOMPQ = 1.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the eigenvalues of the two submatrices to be
* combined. On exit, the trailing (N-K) updated eigenvalues
* (those which were deflated) sorted into increasing order.
*
* Q (input/output) DOUBLE PRECISION array, dimension (LDQ,N)
* If ICOMPQ = 0, Q is not referenced. Otherwise,
* on entry, Q contains the eigenvectors of the partially solved
* system which has been previously updated in matrix
* multiplies with other partially solved eigensystems.
* On exit, Q contains the trailing (N-K) updated eigenvectors
* (those which were deflated) in its last N-K columns.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N).
*
* INDXQ (input) INTEGER array, dimension (N)
* The permutation which separately sorts the two sub-problems
* in D into ascending order. Note that elements in the second
* half of this permutation must first have CUTPNT added to
* their values in order to be accurate.
*
* RHO (input/output) DOUBLE PRECISION
* On entry, the off-diagonal element associated with the rank-1
* cut which originally split the two submatrices which are now
* being recombined.
* On exit, RHO has been modified to the value required by
* DLAED3.
*
* CUTPNT (input) INTEGER
* The location of the last eigenvalue in the leading
* sub-matrix. min(1,N) <= CUTPNT <= N.
*
* Z (input) DOUBLE PRECISION array, dimension (N)
* On entry, Z contains the updating vector (the last row of
* the first sub-eigenvector matrix and the first row of the
* second sub-eigenvector matrix).
* On exit, the contents of Z are destroyed by the updating
* process.
*
* DLAMDA (output) DOUBLE PRECISION array, dimension (N)
* A copy of the first K eigenvalues which will be used by
* DLAED3 to form the secular equation.
*
* Q2 (output) DOUBLE PRECISION array, dimension (LDQ2,N)
* If ICOMPQ = 0, Q2 is not referenced. Otherwise,
* a copy of the first K eigenvectors which will be used by
* DLAED7 in a matrix multiply (DGEMM) to update the new
* eigenvectors.
*
* LDQ2 (input) INTEGER
* The leading dimension of the array Q2. LDQ2 >= max(1,N).
*
* W (output) DOUBLE PRECISION array, dimension (N)
* The first k values of the final deflation-altered z-vector and
* will be passed to DLAED3.
*
* PERM (output) INTEGER array, dimension (N)
* The permutations (from deflation and sorting) to be applied
* to each eigenblock.
*
* GIVPTR (output) INTEGER
* The number of Givens rotations which took place in this
* subproblem.
*
* GIVCOL (output) INTEGER array, dimension (2, N)
* Each pair of numbers indicates a pair of columns to take place
* in a Givens rotation.
*
* GIVNUM (output) DOUBLE PRECISION array, dimension (2, N)
* Each number indicates the S value to be used in the
* corresponding Givens rotation.
*
* INDXP (workspace) INTEGER array, dimension (N)
* The permutation used to place deflated values of D at the end
* of the array. INDXP(1:K) points to the nondeflated D-values
* and INDXP(K+1:N) points to the deflated eigenvalues.
*
* INDX (workspace) INTEGER array, dimension (N)
* The permutation used to sort the contents of D into ascending
* order.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param k
* @param n
* @param qsiz
* @param d
* @param q
* @param ldq
* @param indxq
* @param rho
* @param cutpnt
* @param z
* @param dlamda
* @param q2
* @param ldq2
* @param w
* @param perm
* @param givptr
* @param givcol
* @param givnum
* @param indxp
* @param indx
* @param info
*
*/
abstract public void dlaed8(int icompq, org.netlib.util.intW k, int n, int qsiz, double[] d, double[] q, int ldq, int[] indxq, org.netlib.util.doubleW rho, int cutpnt, double[] z, double[] dlamda, double[] q2, int ldq2, double[] w, int[] perm, org.netlib.util.intW givptr, int[] givcol, double[] givnum, int[] indxp, int[] indx, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAED8 merges the two sets of eigenvalues together into a single
* sorted set. Then it tries to deflate the size of the problem.
* There are two ways in which deflation can occur: when two or more
* eigenvalues are close together or if there is a tiny element in the
* Z vector. For each such occurrence the order of the related secular
* equation problem is reduced by one.
*
* Arguments
* =========
*
* ICOMPQ (input) INTEGER
* = 0: Compute eigenvalues only.
* = 1: Compute eigenvectors of original dense symmetric matrix
* also. On entry, Q contains the orthogonal matrix used
* to reduce the original matrix to tridiagonal form.
*
* K (output) INTEGER
* The number of non-deflated eigenvalues, and the order of the
* related secular equation.
*
* N (input) INTEGER
* The dimension of the symmetric tridiagonal matrix. N >= 0.
*
* QSIZ (input) INTEGER
* The dimension of the orthogonal matrix used to reduce
* the full matrix to tridiagonal form. QSIZ >= N if ICOMPQ = 1.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the eigenvalues of the two submatrices to be
* combined. On exit, the trailing (N-K) updated eigenvalues
* (those which were deflated) sorted into increasing order.
*
* Q (input/output) DOUBLE PRECISION array, dimension (LDQ,N)
* If ICOMPQ = 0, Q is not referenced. Otherwise,
* on entry, Q contains the eigenvectors of the partially solved
* system which has been previously updated in matrix
* multiplies with other partially solved eigensystems.
* On exit, Q contains the trailing (N-K) updated eigenvectors
* (those which were deflated) in its last N-K columns.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N).
*
* INDXQ (input) INTEGER array, dimension (N)
* The permutation which separately sorts the two sub-problems
* in D into ascending order. Note that elements in the second
* half of this permutation must first have CUTPNT added to
* their values in order to be accurate.
*
* RHO (input/output) DOUBLE PRECISION
* On entry, the off-diagonal element associated with the rank-1
* cut which originally split the two submatrices which are now
* being recombined.
* On exit, RHO has been modified to the value required by
* DLAED3.
*
* CUTPNT (input) INTEGER
* The location of the last eigenvalue in the leading
* sub-matrix. min(1,N) <= CUTPNT <= N.
*
* Z (input) DOUBLE PRECISION array, dimension (N)
* On entry, Z contains the updating vector (the last row of
* the first sub-eigenvector matrix and the first row of the
* second sub-eigenvector matrix).
* On exit, the contents of Z are destroyed by the updating
* process.
*
* DLAMDA (output) DOUBLE PRECISION array, dimension (N)
* A copy of the first K eigenvalues which will be used by
* DLAED3 to form the secular equation.
*
* Q2 (output) DOUBLE PRECISION array, dimension (LDQ2,N)
* If ICOMPQ = 0, Q2 is not referenced. Otherwise,
* a copy of the first K eigenvectors which will be used by
* DLAED7 in a matrix multiply (DGEMM) to update the new
* eigenvectors.
*
* LDQ2 (input) INTEGER
* The leading dimension of the array Q2. LDQ2 >= max(1,N).
*
* W (output) DOUBLE PRECISION array, dimension (N)
* The first k values of the final deflation-altered z-vector and
* will be passed to DLAED3.
*
* PERM (output) INTEGER array, dimension (N)
* The permutations (from deflation and sorting) to be applied
* to each eigenblock.
*
* GIVPTR (output) INTEGER
* The number of Givens rotations which took place in this
* subproblem.
*
* GIVCOL (output) INTEGER array, dimension (2, N)
* Each pair of numbers indicates a pair of columns to take place
* in a Givens rotation.
*
* GIVNUM (output) DOUBLE PRECISION array, dimension (2, N)
* Each number indicates the S value to be used in the
* corresponding Givens rotation.
*
* INDXP (workspace) INTEGER array, dimension (N)
* The permutation used to place deflated values of D at the end
* of the array. INDXP(1:K) points to the nondeflated D-values
* and INDXP(K+1:N) points to the deflated eigenvalues.
*
* INDX (workspace) INTEGER array, dimension (N)
* The permutation used to sort the contents of D into ascending
* order.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param k
* @param n
* @param qsiz
* @param d
* @param _d_offset
* @param q
* @param _q_offset
* @param ldq
* @param indxq
* @param _indxq_offset
* @param rho
* @param cutpnt
* @param z
* @param _z_offset
* @param dlamda
* @param _dlamda_offset
* @param q2
* @param _q2_offset
* @param ldq2
* @param w
* @param _w_offset
* @param perm
* @param _perm_offset
* @param givptr
* @param givcol
* @param _givcol_offset
* @param givnum
* @param _givnum_offset
* @param indxp
* @param _indxp_offset
* @param indx
* @param _indx_offset
* @param info
*
*/
abstract public void dlaed8(int icompq, org.netlib.util.intW k, int n, int qsiz, double[] d, int _d_offset, double[] q, int _q_offset, int ldq, int[] indxq, int _indxq_offset, org.netlib.util.doubleW rho, int cutpnt, double[] z, int _z_offset, double[] dlamda, int _dlamda_offset, double[] q2, int _q2_offset, int ldq2, double[] w, int _w_offset, int[] perm, int _perm_offset, org.netlib.util.intW givptr, int[] givcol, int _givcol_offset, double[] givnum, int _givnum_offset, int[] indxp, int _indxp_offset, int[] indx, int _indx_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAED9 finds the roots of the secular equation, as defined by the
* values in D, Z, and RHO, between KSTART and KSTOP. It makes the
* appropriate calls to DLAED4 and then stores the new matrix of
* eigenvectors for use in calculating the next level of Z vectors.
*
* Arguments
* =========
*
* K (input) INTEGER
* The number of terms in the rational function to be solved by
* DLAED4. K >= 0.
*
* KSTART (input) INTEGER
* KSTOP (input) INTEGER
* The updated eigenvalues Lambda(I), KSTART <= I <= KSTOP
* are to be computed. 1 <= KSTART <= KSTOP <= K.
*
* N (input) INTEGER
* The number of rows and columns in the Q matrix.
* N >= K (delation may result in N > K).
*
* D (output) DOUBLE PRECISION array, dimension (N)
* D(I) contains the updated eigenvalues
* for KSTART <= I <= KSTOP.
*
* Q (workspace) DOUBLE PRECISION array, dimension (LDQ,N)
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max( 1, N ).
*
* RHO (input) DOUBLE PRECISION
* The value of the parameter in the rank one update equation.
* RHO >= 0 required.
*
* DLAMDA (input) DOUBLE PRECISION array, dimension (K)
* The first K elements of this array contain the old roots
* of the deflated updating problem. These are the poles
* of the secular equation.
*
* W (input) DOUBLE PRECISION array, dimension (K)
* The first K elements of this array contain the components
* of the deflation-adjusted updating vector.
*
* S (output) DOUBLE PRECISION array, dimension (LDS, K)
* Will contain the eigenvectors of the repaired matrix which
* will be stored for subsequent Z vector calculation and
* multiplied by the previously accumulated eigenvectors
* to update the system.
*
* LDS (input) INTEGER
* The leading dimension of S. LDS >= max( 1, K ).
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an eigenvalue did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param k
* @param kstart
* @param kstop
* @param n
* @param d
* @param q
* @param ldq
* @param rho
* @param dlamda
* @param w
* @param s
* @param lds
* @param info
*
*/
abstract public void dlaed9(int k, int kstart, int kstop, int n, double[] d, double[] q, int ldq, double rho, double[] dlamda, double[] w, double[] s, int lds, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAED9 finds the roots of the secular equation, as defined by the
* values in D, Z, and RHO, between KSTART and KSTOP. It makes the
* appropriate calls to DLAED4 and then stores the new matrix of
* eigenvectors for use in calculating the next level of Z vectors.
*
* Arguments
* =========
*
* K (input) INTEGER
* The number of terms in the rational function to be solved by
* DLAED4. K >= 0.
*
* KSTART (input) INTEGER
* KSTOP (input) INTEGER
* The updated eigenvalues Lambda(I), KSTART <= I <= KSTOP
* are to be computed. 1 <= KSTART <= KSTOP <= K.
*
* N (input) INTEGER
* The number of rows and columns in the Q matrix.
* N >= K (delation may result in N > K).
*
* D (output) DOUBLE PRECISION array, dimension (N)
* D(I) contains the updated eigenvalues
* for KSTART <= I <= KSTOP.
*
* Q (workspace) DOUBLE PRECISION array, dimension (LDQ,N)
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max( 1, N ).
*
* RHO (input) DOUBLE PRECISION
* The value of the parameter in the rank one update equation.
* RHO >= 0 required.
*
* DLAMDA (input) DOUBLE PRECISION array, dimension (K)
* The first K elements of this array contain the old roots
* of the deflated updating problem. These are the poles
* of the secular equation.
*
* W (input) DOUBLE PRECISION array, dimension (K)
* The first K elements of this array contain the components
* of the deflation-adjusted updating vector.
*
* S (output) DOUBLE PRECISION array, dimension (LDS, K)
* Will contain the eigenvectors of the repaired matrix which
* will be stored for subsequent Z vector calculation and
* multiplied by the previously accumulated eigenvectors
* to update the system.
*
* LDS (input) INTEGER
* The leading dimension of S. LDS >= max( 1, K ).
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an eigenvalue did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param k
* @param kstart
* @param kstop
* @param n
* @param d
* @param _d_offset
* @param q
* @param _q_offset
* @param ldq
* @param rho
* @param dlamda
* @param _dlamda_offset
* @param w
* @param _w_offset
* @param s
* @param _s_offset
* @param lds
* @param info
*
*/
abstract public void dlaed9(int k, int kstart, int kstop, int n, double[] d, int _d_offset, double[] q, int _q_offset, int ldq, double rho, double[] dlamda, int _dlamda_offset, double[] w, int _w_offset, double[] s, int _s_offset, int lds, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAEDA computes the Z vector corresponding to the merge step in the
* CURLVLth step of the merge process with TLVLS steps for the CURPBMth
* problem.
*
* Arguments
* =========
*
* N (input) INTEGER
* The dimension of the symmetric tridiagonal matrix. N >= 0.
*
* TLVLS (input) INTEGER
* The total number of merging levels in the overall divide and
* conquer tree.
*
* CURLVL (input) INTEGER
* The current level in the overall merge routine,
* 0 <= curlvl <= tlvls.
*
* CURPBM (input) INTEGER
* The current problem in the current level in the overall
* merge routine (counting from upper left to lower right).
*
* PRMPTR (input) INTEGER array, dimension (N lg N)
* Contains a list of pointers which indicate where in PERM a
* level's permutation is stored. PRMPTR(i+1) - PRMPTR(i)
* indicates the size of the permutation and incidentally the
* size of the full, non-deflated problem.
*
* PERM (input) INTEGER array, dimension (N lg N)
* Contains the permutations (from deflation and sorting) to be
* applied to each eigenblock.
*
* GIVPTR (input) INTEGER array, dimension (N lg N)
* Contains a list of pointers which indicate where in GIVCOL a
* level's Givens rotations are stored. GIVPTR(i+1) - GIVPTR(i)
* indicates the number of Givens rotations.
*
* GIVCOL (input) INTEGER array, dimension (2, N lg N)
* Each pair of numbers indicates a pair of columns to take place
* in a Givens rotation.
*
* GIVNUM (input) DOUBLE PRECISION array, dimension (2, N lg N)
* Each number indicates the S value to be used in the
* corresponding Givens rotation.
*
* Q (input) DOUBLE PRECISION array, dimension (N**2)
* Contains the square eigenblocks from previous levels, the
* starting positions for blocks are given by QPTR.
*
* QPTR (input) INTEGER array, dimension (N+2)
* Contains a list of pointers which indicate where in Q an
* eigenblock is stored. SQRT( QPTR(i+1) - QPTR(i) ) indicates
* the size of the block.
*
* Z (output) DOUBLE PRECISION array, dimension (N)
* On output this vector contains the updating vector (the last
* row of the first sub-eigenvector matrix and the first row of
* the second sub-eigenvector matrix).
*
* ZTEMP (workspace) DOUBLE PRECISION array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param tlvls
* @param curlvl
* @param curpbm
* @param prmptr
* @param perm
* @param givptr
* @param givcol
* @param givnum
* @param q
* @param qptr
* @param z
* @param ztemp
* @param info
*
*/
abstract public void dlaeda(int n, int tlvls, int curlvl, int curpbm, int[] prmptr, int[] perm, int[] givptr, int[] givcol, double[] givnum, double[] q, int[] qptr, double[] z, double[] ztemp, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAEDA computes the Z vector corresponding to the merge step in the
* CURLVLth step of the merge process with TLVLS steps for the CURPBMth
* problem.
*
* Arguments
* =========
*
* N (input) INTEGER
* The dimension of the symmetric tridiagonal matrix. N >= 0.
*
* TLVLS (input) INTEGER
* The total number of merging levels in the overall divide and
* conquer tree.
*
* CURLVL (input) INTEGER
* The current level in the overall merge routine,
* 0 <= curlvl <= tlvls.
*
* CURPBM (input) INTEGER
* The current problem in the current level in the overall
* merge routine (counting from upper left to lower right).
*
* PRMPTR (input) INTEGER array, dimension (N lg N)
* Contains a list of pointers which indicate where in PERM a
* level's permutation is stored. PRMPTR(i+1) - PRMPTR(i)
* indicates the size of the permutation and incidentally the
* size of the full, non-deflated problem.
*
* PERM (input) INTEGER array, dimension (N lg N)
* Contains the permutations (from deflation and sorting) to be
* applied to each eigenblock.
*
* GIVPTR (input) INTEGER array, dimension (N lg N)
* Contains a list of pointers which indicate where in GIVCOL a
* level's Givens rotations are stored. GIVPTR(i+1) - GIVPTR(i)
* indicates the number of Givens rotations.
*
* GIVCOL (input) INTEGER array, dimension (2, N lg N)
* Each pair of numbers indicates a pair of columns to take place
* in a Givens rotation.
*
* GIVNUM (input) DOUBLE PRECISION array, dimension (2, N lg N)
* Each number indicates the S value to be used in the
* corresponding Givens rotation.
*
* Q (input) DOUBLE PRECISION array, dimension (N**2)
* Contains the square eigenblocks from previous levels, the
* starting positions for blocks are given by QPTR.
*
* QPTR (input) INTEGER array, dimension (N+2)
* Contains a list of pointers which indicate where in Q an
* eigenblock is stored. SQRT( QPTR(i+1) - QPTR(i) ) indicates
* the size of the block.
*
* Z (output) DOUBLE PRECISION array, dimension (N)
* On output this vector contains the updating vector (the last
* row of the first sub-eigenvector matrix and the first row of
* the second sub-eigenvector matrix).
*
* ZTEMP (workspace) DOUBLE PRECISION array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param tlvls
* @param curlvl
* @param curpbm
* @param prmptr
* @param _prmptr_offset
* @param perm
* @param _perm_offset
* @param givptr
* @param _givptr_offset
* @param givcol
* @param _givcol_offset
* @param givnum
* @param _givnum_offset
* @param q
* @param _q_offset
* @param qptr
* @param _qptr_offset
* @param z
* @param _z_offset
* @param ztemp
* @param _ztemp_offset
* @param info
*
*/
abstract public void dlaeda(int n, int tlvls, int curlvl, int curpbm, int[] prmptr, int _prmptr_offset, int[] perm, int _perm_offset, int[] givptr, int _givptr_offset, int[] givcol, int _givcol_offset, double[] givnum, int _givnum_offset, double[] q, int _q_offset, int[] qptr, int _qptr_offset, double[] z, int _z_offset, double[] ztemp, int _ztemp_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAEIN uses inverse iteration to find a right or left eigenvector
* corresponding to the eigenvalue (WR,WI) of a real upper Hessenberg
* matrix H.
*
* Arguments
* =========
*
* RIGHTV (input) LOGICAL
* = .TRUE. : compute right eigenvector;
* = .FALSE.: compute left eigenvector.
*
* NOINIT (input) LOGICAL
* = .TRUE. : no initial vector supplied in (VR,VI).
* = .FALSE.: initial vector supplied in (VR,VI).
*
* N (input) INTEGER
* The order of the matrix H. N >= 0.
*
* H (input) DOUBLE PRECISION array, dimension (LDH,N)
* The upper Hessenberg matrix H.
*
* LDH (input) INTEGER
* The leading dimension of the array H. LDH >= max(1,N).
*
* WR (input) DOUBLE PRECISION
* WI (input) DOUBLE PRECISION
* The real and imaginary parts of the eigenvalue of H whose
* corresponding right or left eigenvector is to be computed.
*
* VR (input/output) DOUBLE PRECISION array, dimension (N)
* VI (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, if NOINIT = .FALSE. and WI = 0.0, VR must contain
* a real starting vector for inverse iteration using the real
* eigenvalue WR; if NOINIT = .FALSE. and WI.ne.0.0, VR and VI
* must contain the real and imaginary parts of a complex
* starting vector for inverse iteration using the complex
* eigenvalue (WR,WI); otherwise VR and VI need not be set.
* On exit, if WI = 0.0 (real eigenvalue), VR contains the
* computed real eigenvector; if WI.ne.0.0 (complex eigenvalue),
* VR and VI contain the real and imaginary parts of the
* computed complex eigenvector. The eigenvector is normalized
* so that the component of largest magnitude has magnitude 1;
* here the magnitude of a complex number (x,y) is taken to be
* |x| + |y|.
* VI is not referenced if WI = 0.0.
*
* B (workspace) DOUBLE PRECISION array, dimension (LDB,N)
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= N+1.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (N)
*
* EPS3 (input) DOUBLE PRECISION
* A small machine-dependent value which is used to perturb
* close eigenvalues, and to replace zero pivots.
*
* SMLNUM (input) DOUBLE PRECISION
* A machine-dependent value close to the underflow threshold.
*
* BIGNUM (input) DOUBLE PRECISION
* A machine-dependent value close to the overflow threshold.
*
* INFO (output) INTEGER
* = 0: successful exit
* = 1: inverse iteration did not converge; VR is set to the
* last iterate, and so is VI if WI.ne.0.0.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param rightv
* @param noinit
* @param n
* @param h
* @param ldh
* @param wr
* @param wi
* @param vr
* @param vi
* @param b
* @param ldb
* @param work
* @param eps3
* @param smlnum
* @param bignum
* @param info
*
*/
abstract public void dlaein(boolean rightv, boolean noinit, int n, double[] h, int ldh, double wr, double wi, double[] vr, double[] vi, double[] b, int ldb, double[] work, double eps3, double smlnum, double bignum, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAEIN uses inverse iteration to find a right or left eigenvector
* corresponding to the eigenvalue (WR,WI) of a real upper Hessenberg
* matrix H.
*
* Arguments
* =========
*
* RIGHTV (input) LOGICAL
* = .TRUE. : compute right eigenvector;
* = .FALSE.: compute left eigenvector.
*
* NOINIT (input) LOGICAL
* = .TRUE. : no initial vector supplied in (VR,VI).
* = .FALSE.: initial vector supplied in (VR,VI).
*
* N (input) INTEGER
* The order of the matrix H. N >= 0.
*
* H (input) DOUBLE PRECISION array, dimension (LDH,N)
* The upper Hessenberg matrix H.
*
* LDH (input) INTEGER
* The leading dimension of the array H. LDH >= max(1,N).
*
* WR (input) DOUBLE PRECISION
* WI (input) DOUBLE PRECISION
* The real and imaginary parts of the eigenvalue of H whose
* corresponding right or left eigenvector is to be computed.
*
* VR (input/output) DOUBLE PRECISION array, dimension (N)
* VI (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, if NOINIT = .FALSE. and WI = 0.0, VR must contain
* a real starting vector for inverse iteration using the real
* eigenvalue WR; if NOINIT = .FALSE. and WI.ne.0.0, VR and VI
* must contain the real and imaginary parts of a complex
* starting vector for inverse iteration using the complex
* eigenvalue (WR,WI); otherwise VR and VI need not be set.
* On exit, if WI = 0.0 (real eigenvalue), VR contains the
* computed real eigenvector; if WI.ne.0.0 (complex eigenvalue),
* VR and VI contain the real and imaginary parts of the
* computed complex eigenvector. The eigenvector is normalized
* so that the component of largest magnitude has magnitude 1;
* here the magnitude of a complex number (x,y) is taken to be
* |x| + |y|.
* VI is not referenced if WI = 0.0.
*
* B (workspace) DOUBLE PRECISION array, dimension (LDB,N)
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= N+1.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (N)
*
* EPS3 (input) DOUBLE PRECISION
* A small machine-dependent value which is used to perturb
* close eigenvalues, and to replace zero pivots.
*
* SMLNUM (input) DOUBLE PRECISION
* A machine-dependent value close to the underflow threshold.
*
* BIGNUM (input) DOUBLE PRECISION
* A machine-dependent value close to the overflow threshold.
*
* INFO (output) INTEGER
* = 0: successful exit
* = 1: inverse iteration did not converge; VR is set to the
* last iterate, and so is VI if WI.ne.0.0.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param rightv
* @param noinit
* @param n
* @param h
* @param _h_offset
* @param ldh
* @param wr
* @param wi
* @param vr
* @param _vr_offset
* @param vi
* @param _vi_offset
* @param b
* @param _b_offset
* @param ldb
* @param work
* @param _work_offset
* @param eps3
* @param smlnum
* @param bignum
* @param info
*
*/
abstract public void dlaein(boolean rightv, boolean noinit, int n, double[] h, int _h_offset, int ldh, double wr, double wi, double[] vr, int _vr_offset, double[] vi, int _vi_offset, double[] b, int _b_offset, int ldb, double[] work, int _work_offset, double eps3, double smlnum, double bignum, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAEV2 computes the eigendecomposition of a 2-by-2 symmetric matrix
* [ A B ]
* [ B C ].
* On return, RT1 is the eigenvalue of larger absolute value, RT2 is the
* eigenvalue of smaller absolute value, and (CS1,SN1) is the unit right
* eigenvector for RT1, giving the decomposition
*
* [ CS1 SN1 ] [ A B ] [ CS1 -SN1 ] = [ RT1 0 ]
* [-SN1 CS1 ] [ B C ] [ SN1 CS1 ] [ 0 RT2 ].
*
* Arguments
* =========
*
* A (input) DOUBLE PRECISION
* The (1,1) element of the 2-by-2 matrix.
*
* B (input) DOUBLE PRECISION
* The (1,2) element and the conjugate of the (2,1) element of
* the 2-by-2 matrix.
*
* C (input) DOUBLE PRECISION
* The (2,2) element of the 2-by-2 matrix.
*
* RT1 (output) DOUBLE PRECISION
* The eigenvalue of larger absolute value.
*
* RT2 (output) DOUBLE PRECISION
* The eigenvalue of smaller absolute value.
*
* CS1 (output) DOUBLE PRECISION
* SN1 (output) DOUBLE PRECISION
* The vector (CS1, SN1) is a unit right eigenvector for RT1.
*
* Further Details
* ===============
*
* RT1 is accurate to a few ulps barring over/underflow.
*
* RT2 may be inaccurate if there is massive cancellation in the
* determinant A*C-B*B; higher precision or correctly rounded or
* correctly truncated arithmetic would be needed to compute RT2
* accurately in all cases.
*
* CS1 and SN1 are accurate to a few ulps barring over/underflow.
*
* Overflow is possible only if RT1 is within a factor of 5 of overflow.
* Underflow is harmless if the input data is 0 or exceeds
* underflow_threshold / macheps.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param a
* @param b
* @param c
* @param rt1
* @param rt2
* @param cs1
* @param sn1
*
*/
abstract public void dlaev2(double a, double b, double c, org.netlib.util.doubleW rt1, org.netlib.util.doubleW rt2, org.netlib.util.doubleW cs1, org.netlib.util.doubleW sn1);
/**
*
* ..
*
* Purpose
* =======
*
* DLAEXC swaps adjacent diagonal blocks T11 and T22 of order 1 or 2 in
* an upper quasi-triangular matrix T by an orthogonal similarity
* transformation.
*
* T must be in Schur canonical form, that is, block upper triangular
* with 1-by-1 and 2-by-2 diagonal blocks; each 2-by-2 diagonal block
* has its diagonal elemnts equal and its off-diagonal elements of
* opposite sign.
*
* Arguments
* =========
*
* WANTQ (input) LOGICAL
* = .TRUE. : accumulate the transformation in the matrix Q;
* = .FALSE.: do not accumulate the transformation.
*
* N (input) INTEGER
* The order of the matrix T. N >= 0.
*
* T (input/output) DOUBLE PRECISION array, dimension (LDT,N)
* On entry, the upper quasi-triangular matrix T, in Schur
* canonical form.
* On exit, the updated matrix T, again in Schur canonical form.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= max(1,N).
*
* Q (input/output) DOUBLE PRECISION array, dimension (LDQ,N)
* On entry, if WANTQ is .TRUE., the orthogonal matrix Q.
* On exit, if WANTQ is .TRUE., the updated matrix Q.
* If WANTQ is .FALSE., Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q.
* LDQ >= 1; and if WANTQ is .TRUE., LDQ >= N.
*
* J1 (input) INTEGER
* The index of the first row of the first block T11.
*
* N1 (input) INTEGER
* The order of the first block T11. N1 = 0, 1 or 2.
*
* N2 (input) INTEGER
* The order of the second block T22. N2 = 0, 1 or 2.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* = 1: the transformed matrix T would be too far from Schur
* form; the blocks are not swapped and T and Q are
* unchanged.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param wantq
* @param n
* @param t
* @param ldt
* @param q
* @param ldq
* @param j1
* @param n1
* @param n2
* @param work
* @param info
*
*/
abstract public void dlaexc(boolean wantq, int n, double[] t, int ldt, double[] q, int ldq, int j1, int n1, int n2, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAEXC swaps adjacent diagonal blocks T11 and T22 of order 1 or 2 in
* an upper quasi-triangular matrix T by an orthogonal similarity
* transformation.
*
* T must be in Schur canonical form, that is, block upper triangular
* with 1-by-1 and 2-by-2 diagonal blocks; each 2-by-2 diagonal block
* has its diagonal elemnts equal and its off-diagonal elements of
* opposite sign.
*
* Arguments
* =========
*
* WANTQ (input) LOGICAL
* = .TRUE. : accumulate the transformation in the matrix Q;
* = .FALSE.: do not accumulate the transformation.
*
* N (input) INTEGER
* The order of the matrix T. N >= 0.
*
* T (input/output) DOUBLE PRECISION array, dimension (LDT,N)
* On entry, the upper quasi-triangular matrix T, in Schur
* canonical form.
* On exit, the updated matrix T, again in Schur canonical form.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= max(1,N).
*
* Q (input/output) DOUBLE PRECISION array, dimension (LDQ,N)
* On entry, if WANTQ is .TRUE., the orthogonal matrix Q.
* On exit, if WANTQ is .TRUE., the updated matrix Q.
* If WANTQ is .FALSE., Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q.
* LDQ >= 1; and if WANTQ is .TRUE., LDQ >= N.
*
* J1 (input) INTEGER
* The index of the first row of the first block T11.
*
* N1 (input) INTEGER
* The order of the first block T11. N1 = 0, 1 or 2.
*
* N2 (input) INTEGER
* The order of the second block T22. N2 = 0, 1 or 2.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* = 1: the transformed matrix T would be too far from Schur
* form; the blocks are not swapped and T and Q are
* unchanged.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param wantq
* @param n
* @param t
* @param _t_offset
* @param ldt
* @param q
* @param _q_offset
* @param ldq
* @param j1
* @param n1
* @param n2
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dlaexc(boolean wantq, int n, double[] t, int _t_offset, int ldt, double[] q, int _q_offset, int ldq, int j1, int n1, int n2, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAG2 computes the eigenvalues of a 2 x 2 generalized eigenvalue
* problem A - w B, with scaling as necessary to avoid over-/underflow.
*
* The scaling factor "s" results in a modified eigenvalue equation
*
* s A - w B
*
* where s is a non-negative scaling factor chosen so that w, w B,
* and s A do not overflow and, if possible, do not underflow, either.
*
* Arguments
* =========
*
* A (input) DOUBLE PRECISION array, dimension (LDA, 2)
* On entry, the 2 x 2 matrix A. It is assumed that its 1-norm
* is less than 1/SAFMIN. Entries less than
* sqrt(SAFMIN)*norm(A) are subject to being treated as zero.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= 2.
*
* B (input) DOUBLE PRECISION array, dimension (LDB, 2)
* On entry, the 2 x 2 upper triangular matrix B. It is
* assumed that the one-norm of B is less than 1/SAFMIN. The
* diagonals should be at least sqrt(SAFMIN) times the largest
* element of B (in absolute value); if a diagonal is smaller
* than that, then +/- sqrt(SAFMIN) will be used instead of
* that diagonal.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= 2.
*
* SAFMIN (input) DOUBLE PRECISION
* The smallest positive number s.t. 1/SAFMIN does not
* overflow. (This should always be DLAMCH('S') -- it is an
* argument in order to avoid having to call DLAMCH frequently.)
*
* SCALE1 (output) DOUBLE PRECISION
* A scaling factor used to avoid over-/underflow in the
* eigenvalue equation which defines the first eigenvalue. If
* the eigenvalues are complex, then the eigenvalues are
* ( WR1 +/- WI i ) / SCALE1 (which may lie outside the
* exponent range of the machine), SCALE1=SCALE2, and SCALE1
* will always be positive. If the eigenvalues are real, then
* the first (real) eigenvalue is WR1 / SCALE1 , but this may
* overflow or underflow, and in fact, SCALE1 may be zero or
* less than the underflow threshhold if the exact eigenvalue
* is sufficiently large.
*
* SCALE2 (output) DOUBLE PRECISION
* A scaling factor used to avoid over-/underflow in the
* eigenvalue equation which defines the second eigenvalue. If
* the eigenvalues are complex, then SCALE2=SCALE1. If the
* eigenvalues are real, then the second (real) eigenvalue is
* WR2 / SCALE2 , but this may overflow or underflow, and in
* fact, SCALE2 may be zero or less than the underflow
* threshhold if the exact eigenvalue is sufficiently large.
*
* WR1 (output) DOUBLE PRECISION
* If the eigenvalue is real, then WR1 is SCALE1 times the
* eigenvalue closest to the (2,2) element of A B**(-1). If the
* eigenvalue is complex, then WR1=WR2 is SCALE1 times the real
* part of the eigenvalues.
*
* WR2 (output) DOUBLE PRECISION
* If the eigenvalue is real, then WR2 is SCALE2 times the
* other eigenvalue. If the eigenvalue is complex, then
* WR1=WR2 is SCALE1 times the real part of the eigenvalues.
*
* WI (output) DOUBLE PRECISION
* If the eigenvalue is real, then WI is zero. If the
* eigenvalue is complex, then WI is SCALE1 times the imaginary
* part of the eigenvalues. WI will always be non-negative.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param a
* @param lda
* @param b
* @param ldb
* @param safmin
* @param scale1
* @param scale2
* @param wr1
* @param wr2
* @param wi
*
*/
abstract public void dlag2(double[] a, int lda, double[] b, int ldb, double safmin, org.netlib.util.doubleW scale1, org.netlib.util.doubleW scale2, org.netlib.util.doubleW wr1, org.netlib.util.doubleW wr2, org.netlib.util.doubleW wi);
/**
*
* ..
*
* Purpose
* =======
*
* DLAG2 computes the eigenvalues of a 2 x 2 generalized eigenvalue
* problem A - w B, with scaling as necessary to avoid over-/underflow.
*
* The scaling factor "s" results in a modified eigenvalue equation
*
* s A - w B
*
* where s is a non-negative scaling factor chosen so that w, w B,
* and s A do not overflow and, if possible, do not underflow, either.
*
* Arguments
* =========
*
* A (input) DOUBLE PRECISION array, dimension (LDA, 2)
* On entry, the 2 x 2 matrix A. It is assumed that its 1-norm
* is less than 1/SAFMIN. Entries less than
* sqrt(SAFMIN)*norm(A) are subject to being treated as zero.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= 2.
*
* B (input) DOUBLE PRECISION array, dimension (LDB, 2)
* On entry, the 2 x 2 upper triangular matrix B. It is
* assumed that the one-norm of B is less than 1/SAFMIN. The
* diagonals should be at least sqrt(SAFMIN) times the largest
* element of B (in absolute value); if a diagonal is smaller
* than that, then +/- sqrt(SAFMIN) will be used instead of
* that diagonal.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= 2.
*
* SAFMIN (input) DOUBLE PRECISION
* The smallest positive number s.t. 1/SAFMIN does not
* overflow. (This should always be DLAMCH('S') -- it is an
* argument in order to avoid having to call DLAMCH frequently.)
*
* SCALE1 (output) DOUBLE PRECISION
* A scaling factor used to avoid over-/underflow in the
* eigenvalue equation which defines the first eigenvalue. If
* the eigenvalues are complex, then the eigenvalues are
* ( WR1 +/- WI i ) / SCALE1 (which may lie outside the
* exponent range of the machine), SCALE1=SCALE2, and SCALE1
* will always be positive. If the eigenvalues are real, then
* the first (real) eigenvalue is WR1 / SCALE1 , but this may
* overflow or underflow, and in fact, SCALE1 may be zero or
* less than the underflow threshhold if the exact eigenvalue
* is sufficiently large.
*
* SCALE2 (output) DOUBLE PRECISION
* A scaling factor used to avoid over-/underflow in the
* eigenvalue equation which defines the second eigenvalue. If
* the eigenvalues are complex, then SCALE2=SCALE1. If the
* eigenvalues are real, then the second (real) eigenvalue is
* WR2 / SCALE2 , but this may overflow or underflow, and in
* fact, SCALE2 may be zero or less than the underflow
* threshhold if the exact eigenvalue is sufficiently large.
*
* WR1 (output) DOUBLE PRECISION
* If the eigenvalue is real, then WR1 is SCALE1 times the
* eigenvalue closest to the (2,2) element of A B**(-1). If the
* eigenvalue is complex, then WR1=WR2 is SCALE1 times the real
* part of the eigenvalues.
*
* WR2 (output) DOUBLE PRECISION
* If the eigenvalue is real, then WR2 is SCALE2 times the
* other eigenvalue. If the eigenvalue is complex, then
* WR1=WR2 is SCALE1 times the real part of the eigenvalues.
*
* WI (output) DOUBLE PRECISION
* If the eigenvalue is real, then WI is zero. If the
* eigenvalue is complex, then WI is SCALE1 times the imaginary
* part of the eigenvalues. WI will always be non-negative.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param safmin
* @param scale1
* @param scale2
* @param wr1
* @param wr2
* @param wi
*
*/
abstract public void dlag2(double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, double safmin, org.netlib.util.doubleW scale1, org.netlib.util.doubleW scale2, org.netlib.util.doubleW wr1, org.netlib.util.doubleW wr2, org.netlib.util.doubleW wi);
/**
*
* ..
*
* Purpose
* =======
*
* DLAG2S converts a DOUBLE PRECISION matrix, SA, to a SINGLE
* PRECISION matrix, A.
*
* RMAX is the overflow for the SINGLE PRECISION arithmetic
* DLAG2S checks that all the entries of A are between -RMAX and
* RMAX. If not the convertion is aborted and a flag is raised.
*
* This is a helper routine so there is no argument checking.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of lines of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N coefficient matrix A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* SA (output) REAL array, dimension (LDSA,N)
* On exit, if INFO=0, the M-by-N coefficient matrix SA.
*
* LDSA (input) INTEGER
* The leading dimension of the array SA. LDSA >= max(1,M).
*
* INFO (output) INTEGER
* = 0: successful exit
* > 0: if INFO = k, the (i,j) entry of the matrix A has
* overflowed when moving from DOUBLE PRECISION to SINGLE
* k is given by k = (i-1)*LDA+j
*
* =========
*
* .. Local Scalars ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param sa
* @param ldsa
* @param info
*
*/
abstract public void dlag2s(int m, int n, double[] a, int lda, float[] sa, int ldsa, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAG2S converts a DOUBLE PRECISION matrix, SA, to a SINGLE
* PRECISION matrix, A.
*
* RMAX is the overflow for the SINGLE PRECISION arithmetic
* DLAG2S checks that all the entries of A are between -RMAX and
* RMAX. If not the convertion is aborted and a flag is raised.
*
* This is a helper routine so there is no argument checking.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of lines of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N coefficient matrix A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* SA (output) REAL array, dimension (LDSA,N)
* On exit, if INFO=0, the M-by-N coefficient matrix SA.
*
* LDSA (input) INTEGER
* The leading dimension of the array SA. LDSA >= max(1,M).
*
* INFO (output) INTEGER
* = 0: successful exit
* > 0: if INFO = k, the (i,j) entry of the matrix A has
* overflowed when moving from DOUBLE PRECISION to SINGLE
* k is given by k = (i-1)*LDA+j
*
* =========
*
* .. Local Scalars ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param sa
* @param _sa_offset
* @param ldsa
* @param info
*
*/
abstract public void dlag2s(int m, int n, double[] a, int _a_offset, int lda, float[] sa, int _sa_offset, int ldsa, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAGS2 computes 2-by-2 orthogonal matrices U, V and Q, such
* that if ( UPPER ) then
*
* U'*A*Q = U'*( A1 A2 )*Q = ( x 0 )
* ( 0 A3 ) ( x x )
* and
* V'*B*Q = V'*( B1 B2 )*Q = ( x 0 )
* ( 0 B3 ) ( x x )
*
* or if ( .NOT.UPPER ) then
*
* U'*A*Q = U'*( A1 0 )*Q = ( x x )
* ( A2 A3 ) ( 0 x )
* and
* V'*B*Q = V'*( B1 0 )*Q = ( x x )
* ( B2 B3 ) ( 0 x )
*
* The rows of the transformed A and B are parallel, where
*
* U = ( CSU SNU ), V = ( CSV SNV ), Q = ( CSQ SNQ )
* ( -SNU CSU ) ( -SNV CSV ) ( -SNQ CSQ )
*
* Z' denotes the transpose of Z.
*
*
* Arguments
* =========
*
* UPPER (input) LOGICAL
* = .TRUE.: the input matrices A and B are upper triangular.
* = .FALSE.: the input matrices A and B are lower triangular.
*
* A1 (input) DOUBLE PRECISION
* A2 (input) DOUBLE PRECISION
* A3 (input) DOUBLE PRECISION
* On entry, A1, A2 and A3 are elements of the input 2-by-2
* upper (lower) triangular matrix A.
*
* B1 (input) DOUBLE PRECISION
* B2 (input) DOUBLE PRECISION
* B3 (input) DOUBLE PRECISION
* On entry, B1, B2 and B3 are elements of the input 2-by-2
* upper (lower) triangular matrix B.
*
* CSU (output) DOUBLE PRECISION
* SNU (output) DOUBLE PRECISION
* The desired orthogonal matrix U.
*
* CSV (output) DOUBLE PRECISION
* SNV (output) DOUBLE PRECISION
* The desired orthogonal matrix V.
*
* CSQ (output) DOUBLE PRECISION
* SNQ (output) DOUBLE PRECISION
* The desired orthogonal matrix Q.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param upper
* @param a1
* @param a2
* @param a3
* @param b1
* @param b2
* @param b3
* @param csu
* @param snu
* @param csv
* @param snv
* @param csq
* @param snq
*
*/
abstract public void dlags2(boolean upper, double a1, double a2, double a3, double b1, double b2, double b3, org.netlib.util.doubleW csu, org.netlib.util.doubleW snu, org.netlib.util.doubleW csv, org.netlib.util.doubleW snv, org.netlib.util.doubleW csq, org.netlib.util.doubleW snq);
/**
*
* ..
*
* Purpose
* =======
*
* DLAGTF factorizes the matrix (T - lambda*I), where T is an n by n
* tridiagonal matrix and lambda is a scalar, as
*
* T - lambda*I = PLU,
*
* where P is a permutation matrix, L is a unit lower tridiagonal matrix
* with at most one non-zero sub-diagonal elements per column and U is
* an upper triangular matrix with at most two non-zero super-diagonal
* elements per column.
*
* The factorization is obtained by Gaussian elimination with partial
* pivoting and implicit row scaling.
*
* The parameter LAMBDA is included in the routine so that DLAGTF may
* be used, in conjunction with DLAGTS, to obtain eigenvectors of T by
* inverse iteration.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix T.
*
* A (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, A must contain the diagonal elements of T.
*
* On exit, A is overwritten by the n diagonal elements of the
* upper triangular matrix U of the factorization of T.
*
* LAMBDA (input) DOUBLE PRECISION
* On entry, the scalar lambda.
*
* B (input/output) DOUBLE PRECISION array, dimension (N-1)
* On entry, B must contain the (n-1) super-diagonal elements of
* T.
*
* On exit, B is overwritten by the (n-1) super-diagonal
* elements of the matrix U of the factorization of T.
*
* C (input/output) DOUBLE PRECISION array, dimension (N-1)
* On entry, C must contain the (n-1) sub-diagonal elements of
* T.
*
* On exit, C is overwritten by the (n-1) sub-diagonal elements
* of the matrix L of the factorization of T.
*
* TOL (input) DOUBLE PRECISION
* On entry, a relative tolerance used to indicate whether or
* not the matrix (T - lambda*I) is nearly singular. TOL should
* normally be chose as approximately the largest relative error
* in the elements of T. For example, if the elements of T are
* correct to about 4 significant figures, then TOL should be
* set to about 5*10**(-4). If TOL is supplied as less than eps,
* where eps is the relative machine precision, then the value
* eps is used in place of TOL.
*
* D (output) DOUBLE PRECISION array, dimension (N-2)
* On exit, D is overwritten by the (n-2) second super-diagonal
* elements of the matrix U of the factorization of T.
*
* IN (output) INTEGER array, dimension (N)
* On exit, IN contains details of the permutation matrix P. If
* an interchange occurred at the kth step of the elimination,
* then IN(k) = 1, otherwise IN(k) = 0. The element IN(n)
* returns the smallest positive integer j such that
*
* abs( u(j,j) ).le. norm( (T - lambda*I)(j) )*TOL,
*
* where norm( A(j) ) denotes the sum of the absolute values of
* the jth row of the matrix A. If no such j exists then IN(n)
* is returned as zero. If IN(n) is returned as positive, then a
* diagonal element of U is small, indicating that
* (T - lambda*I) is singular or nearly singular,
*
* INFO (output) INTEGER
* = 0 : successful exit
* .lt. 0: if INFO = -k, the kth argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param a
* @param lambda
* @param b
* @param c
* @param tol
* @param d
* @param in
* @param info
*
*/
abstract public void dlagtf(int n, double[] a, double lambda, double[] b, double[] c, double tol, double[] d, int[] in, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAGTF factorizes the matrix (T - lambda*I), where T is an n by n
* tridiagonal matrix and lambda is a scalar, as
*
* T - lambda*I = PLU,
*
* where P is a permutation matrix, L is a unit lower tridiagonal matrix
* with at most one non-zero sub-diagonal elements per column and U is
* an upper triangular matrix with at most two non-zero super-diagonal
* elements per column.
*
* The factorization is obtained by Gaussian elimination with partial
* pivoting and implicit row scaling.
*
* The parameter LAMBDA is included in the routine so that DLAGTF may
* be used, in conjunction with DLAGTS, to obtain eigenvectors of T by
* inverse iteration.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix T.
*
* A (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, A must contain the diagonal elements of T.
*
* On exit, A is overwritten by the n diagonal elements of the
* upper triangular matrix U of the factorization of T.
*
* LAMBDA (input) DOUBLE PRECISION
* On entry, the scalar lambda.
*
* B (input/output) DOUBLE PRECISION array, dimension (N-1)
* On entry, B must contain the (n-1) super-diagonal elements of
* T.
*
* On exit, B is overwritten by the (n-1) super-diagonal
* elements of the matrix U of the factorization of T.
*
* C (input/output) DOUBLE PRECISION array, dimension (N-1)
* On entry, C must contain the (n-1) sub-diagonal elements of
* T.
*
* On exit, C is overwritten by the (n-1) sub-diagonal elements
* of the matrix L of the factorization of T.
*
* TOL (input) DOUBLE PRECISION
* On entry, a relative tolerance used to indicate whether or
* not the matrix (T - lambda*I) is nearly singular. TOL should
* normally be chose as approximately the largest relative error
* in the elements of T. For example, if the elements of T are
* correct to about 4 significant figures, then TOL should be
* set to about 5*10**(-4). If TOL is supplied as less than eps,
* where eps is the relative machine precision, then the value
* eps is used in place of TOL.
*
* D (output) DOUBLE PRECISION array, dimension (N-2)
* On exit, D is overwritten by the (n-2) second super-diagonal
* elements of the matrix U of the factorization of T.
*
* IN (output) INTEGER array, dimension (N)
* On exit, IN contains details of the permutation matrix P. If
* an interchange occurred at the kth step of the elimination,
* then IN(k) = 1, otherwise IN(k) = 0. The element IN(n)
* returns the smallest positive integer j such that
*
* abs( u(j,j) ).le. norm( (T - lambda*I)(j) )*TOL,
*
* where norm( A(j) ) denotes the sum of the absolute values of
* the jth row of the matrix A. If no such j exists then IN(n)
* is returned as zero. If IN(n) is returned as positive, then a
* diagonal element of U is small, indicating that
* (T - lambda*I) is singular or nearly singular,
*
* INFO (output) INTEGER
* = 0 : successful exit
* .lt. 0: if INFO = -k, the kth argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param a
* @param _a_offset
* @param lambda
* @param b
* @param _b_offset
* @param c
* @param _c_offset
* @param tol
* @param d
* @param _d_offset
* @param in
* @param _in_offset
* @param info
*
*/
abstract public void dlagtf(int n, double[] a, int _a_offset, double lambda, double[] b, int _b_offset, double[] c, int _c_offset, double tol, double[] d, int _d_offset, int[] in, int _in_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAGTM performs a matrix-vector product of the form
*
* B := alpha * A * X + beta * B
*
* where A is a tridiagonal matrix of order N, B and X are N by NRHS
* matrices, and alpha and beta are real scalars, each of which may be
* 0., 1., or -1.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* Specifies the operation applied to A.
* = 'N': No transpose, B := alpha * A * X + beta * B
* = 'T': Transpose, B := alpha * A'* X + beta * B
* = 'C': Conjugate transpose = Transpose
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices X and B.
*
* ALPHA (input) DOUBLE PRECISION
* The scalar alpha. ALPHA must be 0., 1., or -1.; otherwise,
* it is assumed to be 0.
*
* DL (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) sub-diagonal elements of T.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The diagonal elements of T.
*
* DU (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) super-diagonal elements of T.
*
* X (input) DOUBLE PRECISION array, dimension (LDX,NRHS)
* The N by NRHS matrix X.
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(N,1).
*
* BETA (input) DOUBLE PRECISION
* The scalar beta. BETA must be 0., 1., or -1.; otherwise,
* it is assumed to be 1.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the N by NRHS matrix B.
* On exit, B is overwritten by the matrix expression
* B := alpha * A * X + beta * B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(N,1).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param trans
* @param n
* @param nrhs
* @param alpha
* @param dl
* @param d
* @param du
* @param x
* @param ldx
* @param beta
* @param b
* @param ldb
*
*/
abstract public void dlagtm(java.lang.String trans, int n, int nrhs, double alpha, double[] dl, double[] d, double[] du, double[] x, int ldx, double beta, double[] b, int ldb);
/**
*
* ..
*
* Purpose
* =======
*
* DLAGTM performs a matrix-vector product of the form
*
* B := alpha * A * X + beta * B
*
* where A is a tridiagonal matrix of order N, B and X are N by NRHS
* matrices, and alpha and beta are real scalars, each of which may be
* 0., 1., or -1.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* Specifies the operation applied to A.
* = 'N': No transpose, B := alpha * A * X + beta * B
* = 'T': Transpose, B := alpha * A'* X + beta * B
* = 'C': Conjugate transpose = Transpose
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices X and B.
*
* ALPHA (input) DOUBLE PRECISION
* The scalar alpha. ALPHA must be 0., 1., or -1.; otherwise,
* it is assumed to be 0.
*
* DL (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) sub-diagonal elements of T.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The diagonal elements of T.
*
* DU (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) super-diagonal elements of T.
*
* X (input) DOUBLE PRECISION array, dimension (LDX,NRHS)
* The N by NRHS matrix X.
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(N,1).
*
* BETA (input) DOUBLE PRECISION
* The scalar beta. BETA must be 0., 1., or -1.; otherwise,
* it is assumed to be 1.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the N by NRHS matrix B.
* On exit, B is overwritten by the matrix expression
* B := alpha * A * X + beta * B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(N,1).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param trans
* @param n
* @param nrhs
* @param alpha
* @param dl
* @param _dl_offset
* @param d
* @param _d_offset
* @param du
* @param _du_offset
* @param x
* @param _x_offset
* @param ldx
* @param beta
* @param b
* @param _b_offset
* @param ldb
*
*/
abstract public void dlagtm(java.lang.String trans, int n, int nrhs, double alpha, double[] dl, int _dl_offset, double[] d, int _d_offset, double[] du, int _du_offset, double[] x, int _x_offset, int ldx, double beta, double[] b, int _b_offset, int ldb);
/**
*
* ..
*
* Purpose
* =======
*
* DLAGTS may be used to solve one of the systems of equations
*
* (T - lambda*I)*x = y or (T - lambda*I)'*x = y,
*
* where T is an n by n tridiagonal matrix, for x, following the
* factorization of (T - lambda*I) as
*
* (T - lambda*I) = P*L*U ,
*
* by routine DLAGTF. The choice of equation to be solved is
* controlled by the argument JOB, and in each case there is an option
* to perturb zero or very small diagonal elements of U, this option
* being intended for use in applications such as inverse iteration.
*
* Arguments
* =========
*
* JOB (input) INTEGER
* Specifies the job to be performed by DLAGTS as follows:
* = 1: The equations (T - lambda*I)x = y are to be solved,
* but diagonal elements of U are not to be perturbed.
* = -1: The equations (T - lambda*I)x = y are to be solved
* and, if overflow would otherwise occur, the diagonal
* elements of U are to be perturbed. See argument TOL
* below.
* = 2: The equations (T - lambda*I)'x = y are to be solved,
* but diagonal elements of U are not to be perturbed.
* = -2: The equations (T - lambda*I)'x = y are to be solved
* and, if overflow would otherwise occur, the diagonal
* elements of U are to be perturbed. See argument TOL
* below.
*
* N (input) INTEGER
* The order of the matrix T.
*
* A (input) DOUBLE PRECISION array, dimension (N)
* On entry, A must contain the diagonal elements of U as
* returned from DLAGTF.
*
* B (input) DOUBLE PRECISION array, dimension (N-1)
* On entry, B must contain the first super-diagonal elements of
* U as returned from DLAGTF.
*
* C (input) DOUBLE PRECISION array, dimension (N-1)
* On entry, C must contain the sub-diagonal elements of L as
* returned from DLAGTF.
*
* D (input) DOUBLE PRECISION array, dimension (N-2)
* On entry, D must contain the second super-diagonal elements
* of U as returned from DLAGTF.
*
* IN (input) INTEGER array, dimension (N)
* On entry, IN must contain details of the matrix P as returned
* from DLAGTF.
*
* Y (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the right hand side vector y.
* On exit, Y is overwritten by the solution vector x.
*
* TOL (input/output) DOUBLE PRECISION
* On entry, with JOB .lt. 0, TOL should be the minimum
* perturbation to be made to very small diagonal elements of U.
* TOL should normally be chosen as about eps*norm(U), where eps
* is the relative machine precision, but if TOL is supplied as
* non-positive, then it is reset to eps*max( abs( u(i,j) ) ).
* If JOB .gt. 0 then TOL is not referenced.
*
* On exit, TOL is changed as described above, only if TOL is
* non-positive on entry. Otherwise TOL is unchanged.
*
* INFO (output) INTEGER
* = 0 : successful exit
* .lt. 0: if INFO = -i, the i-th argument had an illegal value
* .gt. 0: overflow would occur when computing the INFO(th)
* element of the solution vector x. This can only occur
* when JOB is supplied as positive and either means
* that a diagonal element of U is very small, or that
* the elements of the right-hand side vector y are very
* large.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param n
* @param a
* @param b
* @param c
* @param d
* @param in
* @param y
* @param tol
* @param info
*
*/
abstract public void dlagts(int job, int n, double[] a, double[] b, double[] c, double[] d, int[] in, double[] y, org.netlib.util.doubleW tol, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAGTS may be used to solve one of the systems of equations
*
* (T - lambda*I)*x = y or (T - lambda*I)'*x = y,
*
* where T is an n by n tridiagonal matrix, for x, following the
* factorization of (T - lambda*I) as
*
* (T - lambda*I) = P*L*U ,
*
* by routine DLAGTF. The choice of equation to be solved is
* controlled by the argument JOB, and in each case there is an option
* to perturb zero or very small diagonal elements of U, this option
* being intended for use in applications such as inverse iteration.
*
* Arguments
* =========
*
* JOB (input) INTEGER
* Specifies the job to be performed by DLAGTS as follows:
* = 1: The equations (T - lambda*I)x = y are to be solved,
* but diagonal elements of U are not to be perturbed.
* = -1: The equations (T - lambda*I)x = y are to be solved
* and, if overflow would otherwise occur, the diagonal
* elements of U are to be perturbed. See argument TOL
* below.
* = 2: The equations (T - lambda*I)'x = y are to be solved,
* but diagonal elements of U are not to be perturbed.
* = -2: The equations (T - lambda*I)'x = y are to be solved
* and, if overflow would otherwise occur, the diagonal
* elements of U are to be perturbed. See argument TOL
* below.
*
* N (input) INTEGER
* The order of the matrix T.
*
* A (input) DOUBLE PRECISION array, dimension (N)
* On entry, A must contain the diagonal elements of U as
* returned from DLAGTF.
*
* B (input) DOUBLE PRECISION array, dimension (N-1)
* On entry, B must contain the first super-diagonal elements of
* U as returned from DLAGTF.
*
* C (input) DOUBLE PRECISION array, dimension (N-1)
* On entry, C must contain the sub-diagonal elements of L as
* returned from DLAGTF.
*
* D (input) DOUBLE PRECISION array, dimension (N-2)
* On entry, D must contain the second super-diagonal elements
* of U as returned from DLAGTF.
*
* IN (input) INTEGER array, dimension (N)
* On entry, IN must contain details of the matrix P as returned
* from DLAGTF.
*
* Y (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the right hand side vector y.
* On exit, Y is overwritten by the solution vector x.
*
* TOL (input/output) DOUBLE PRECISION
* On entry, with JOB .lt. 0, TOL should be the minimum
* perturbation to be made to very small diagonal elements of U.
* TOL should normally be chosen as about eps*norm(U), where eps
* is the relative machine precision, but if TOL is supplied as
* non-positive, then it is reset to eps*max( abs( u(i,j) ) ).
* If JOB .gt. 0 then TOL is not referenced.
*
* On exit, TOL is changed as described above, only if TOL is
* non-positive on entry. Otherwise TOL is unchanged.
*
* INFO (output) INTEGER
* = 0 : successful exit
* .lt. 0: if INFO = -i, the i-th argument had an illegal value
* .gt. 0: overflow would occur when computing the INFO(th)
* element of the solution vector x. This can only occur
* when JOB is supplied as positive and either means
* that a diagonal element of U is very small, or that
* the elements of the right-hand side vector y are very
* large.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param n
* @param a
* @param _a_offset
* @param b
* @param _b_offset
* @param c
* @param _c_offset
* @param d
* @param _d_offset
* @param in
* @param _in_offset
* @param y
* @param _y_offset
* @param tol
* @param info
*
*/
abstract public void dlagts(int job, int n, double[] a, int _a_offset, double[] b, int _b_offset, double[] c, int _c_offset, double[] d, int _d_offset, int[] in, int _in_offset, double[] y, int _y_offset, org.netlib.util.doubleW tol, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAGV2 computes the Generalized Schur factorization of a real 2-by-2
* matrix pencil (A,B) where B is upper triangular. This routine
* computes orthogonal (rotation) matrices given by CSL, SNL and CSR,
* SNR such that
*
* 1) if the pencil (A,B) has two real eigenvalues (include 0/0 or 1/0
* types), then
*
* [ a11 a12 ] := [ CSL SNL ] [ a11 a12 ] [ CSR -SNR ]
* [ 0 a22 ] [ -SNL CSL ] [ a21 a22 ] [ SNR CSR ]
*
* [ b11 b12 ] := [ CSL SNL ] [ b11 b12 ] [ CSR -SNR ]
* [ 0 b22 ] [ -SNL CSL ] [ 0 b22 ] [ SNR CSR ],
*
* 2) if the pencil (A,B) has a pair of complex conjugate eigenvalues,
* then
*
* [ a11 a12 ] := [ CSL SNL ] [ a11 a12 ] [ CSR -SNR ]
* [ a21 a22 ] [ -SNL CSL ] [ a21 a22 ] [ SNR CSR ]
*
* [ b11 0 ] := [ CSL SNL ] [ b11 b12 ] [ CSR -SNR ]
* [ 0 b22 ] [ -SNL CSL ] [ 0 b22 ] [ SNR CSR ]
*
* where b11 >= b22 > 0.
*
*
* Arguments
* =========
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, 2)
* On entry, the 2 x 2 matrix A.
* On exit, A is overwritten by the ``A-part'' of the
* generalized Schur form.
*
* LDA (input) INTEGER
* THe leading dimension of the array A. LDA >= 2.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB, 2)
* On entry, the upper triangular 2 x 2 matrix B.
* On exit, B is overwritten by the ``B-part'' of the
* generalized Schur form.
*
* LDB (input) INTEGER
* THe leading dimension of the array B. LDB >= 2.
*
* ALPHAR (output) DOUBLE PRECISION array, dimension (2)
* ALPHAI (output) DOUBLE PRECISION array, dimension (2)
* BETA (output) DOUBLE PRECISION array, dimension (2)
* (ALPHAR(k)+i*ALPHAI(k))/BETA(k) are the eigenvalues of the
* pencil (A,B), k=1,2, i = sqrt(-1). Note that BETA(k) may
* be zero.
*
* CSL (output) DOUBLE PRECISION
* The cosine of the left rotation matrix.
*
* SNL (output) DOUBLE PRECISION
* The sine of the left rotation matrix.
*
* CSR (output) DOUBLE PRECISION
* The cosine of the right rotation matrix.
*
* SNR (output) DOUBLE PRECISION
* The sine of the right rotation matrix.
*
* Further Details
* ===============
*
* Based on contributions by
* Mark Fahey, Department of Mathematics, Univ. of Kentucky, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param a
* @param lda
* @param b
* @param ldb
* @param alphar
* @param alphai
* @param beta
* @param csl
* @param snl
* @param csr
* @param snr
*
*/
abstract public void dlagv2(double[] a, int lda, double[] b, int ldb, double[] alphar, double[] alphai, double[] beta, org.netlib.util.doubleW csl, org.netlib.util.doubleW snl, org.netlib.util.doubleW csr, org.netlib.util.doubleW snr);
/**
*
* ..
*
* Purpose
* =======
*
* DLAGV2 computes the Generalized Schur factorization of a real 2-by-2
* matrix pencil (A,B) where B is upper triangular. This routine
* computes orthogonal (rotation) matrices given by CSL, SNL and CSR,
* SNR such that
*
* 1) if the pencil (A,B) has two real eigenvalues (include 0/0 or 1/0
* types), then
*
* [ a11 a12 ] := [ CSL SNL ] [ a11 a12 ] [ CSR -SNR ]
* [ 0 a22 ] [ -SNL CSL ] [ a21 a22 ] [ SNR CSR ]
*
* [ b11 b12 ] := [ CSL SNL ] [ b11 b12 ] [ CSR -SNR ]
* [ 0 b22 ] [ -SNL CSL ] [ 0 b22 ] [ SNR CSR ],
*
* 2) if the pencil (A,B) has a pair of complex conjugate eigenvalues,
* then
*
* [ a11 a12 ] := [ CSL SNL ] [ a11 a12 ] [ CSR -SNR ]
* [ a21 a22 ] [ -SNL CSL ] [ a21 a22 ] [ SNR CSR ]
*
* [ b11 0 ] := [ CSL SNL ] [ b11 b12 ] [ CSR -SNR ]
* [ 0 b22 ] [ -SNL CSL ] [ 0 b22 ] [ SNR CSR ]
*
* where b11 >= b22 > 0.
*
*
* Arguments
* =========
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, 2)
* On entry, the 2 x 2 matrix A.
* On exit, A is overwritten by the ``A-part'' of the
* generalized Schur form.
*
* LDA (input) INTEGER
* THe leading dimension of the array A. LDA >= 2.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB, 2)
* On entry, the upper triangular 2 x 2 matrix B.
* On exit, B is overwritten by the ``B-part'' of the
* generalized Schur form.
*
* LDB (input) INTEGER
* THe leading dimension of the array B. LDB >= 2.
*
* ALPHAR (output) DOUBLE PRECISION array, dimension (2)
* ALPHAI (output) DOUBLE PRECISION array, dimension (2)
* BETA (output) DOUBLE PRECISION array, dimension (2)
* (ALPHAR(k)+i*ALPHAI(k))/BETA(k) are the eigenvalues of the
* pencil (A,B), k=1,2, i = sqrt(-1). Note that BETA(k) may
* be zero.
*
* CSL (output) DOUBLE PRECISION
* The cosine of the left rotation matrix.
*
* SNL (output) DOUBLE PRECISION
* The sine of the left rotation matrix.
*
* CSR (output) DOUBLE PRECISION
* The cosine of the right rotation matrix.
*
* SNR (output) DOUBLE PRECISION
* The sine of the right rotation matrix.
*
* Further Details
* ===============
*
* Based on contributions by
* Mark Fahey, Department of Mathematics, Univ. of Kentucky, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param alphar
* @param _alphar_offset
* @param alphai
* @param _alphai_offset
* @param beta
* @param _beta_offset
* @param csl
* @param snl
* @param csr
* @param snr
*
*/
abstract public void dlagv2(double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, double[] alphar, int _alphar_offset, double[] alphai, int _alphai_offset, double[] beta, int _beta_offset, org.netlib.util.doubleW csl, org.netlib.util.doubleW snl, org.netlib.util.doubleW csr, org.netlib.util.doubleW snr);
/**
*
* ..
*
* Purpose
* =======
*
* DLAHQR is an auxiliary routine called by DHSEQR to update the
* eigenvalues and Schur decomposition already computed by DHSEQR, by
* dealing with the Hessenberg submatrix in rows and columns ILO to
* IHI.
*
* Arguments
* =========
*
* WANTT (input) LOGICAL
* = .TRUE. : the full Schur form T is required;
* = .FALSE.: only eigenvalues are required.
*
* WANTZ (input) LOGICAL
* = .TRUE. : the matrix of Schur vectors Z is required;
* = .FALSE.: Schur vectors are not required.
*
* N (input) INTEGER
* The order of the matrix H. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* It is assumed that H is already upper quasi-triangular in
* rows and columns IHI+1:N, and that H(ILO,ILO-1) = 0 (unless
* ILO = 1). DLAHQR works primarily with the Hessenberg
* submatrix in rows and columns ILO to IHI, but applies
* transformations to all of H if WANTT is .TRUE..
* 1 <= ILO <= max(1,IHI); IHI <= N.
*
* H (input/output) DOUBLE PRECISION array, dimension (LDH,N)
* On entry, the upper Hessenberg matrix H.
* On exit, if INFO is zero and if WANTT is .TRUE., H is upper
* quasi-triangular in rows and columns ILO:IHI, with any
* 2-by-2 diagonal blocks in standard form. If INFO is zero
* and WANTT is .FALSE., the contents of H are unspecified on
* exit. The output state of H if INFO is nonzero is given
* below under the description of INFO.
*
* LDH (input) INTEGER
* The leading dimension of the array H. LDH >= max(1,N).
*
* WR (output) DOUBLE PRECISION array, dimension (N)
* WI (output) DOUBLE PRECISION array, dimension (N)
* The real and imaginary parts, respectively, of the computed
* eigenvalues ILO to IHI are stored in the corresponding
* elements of WR and WI. If two eigenvalues are computed as a
* complex conjugate pair, they are stored in consecutive
* elements of WR and WI, say the i-th and (i+1)th, with
* WI(i) > 0 and WI(i+1) < 0. If WANTT is .TRUE., the
* eigenvalues are stored in the same order as on the diagonal
* of the Schur form returned in H, with WR(i) = H(i,i), and, if
* H(i:i+1,i:i+1) is a 2-by-2 diagonal block,
* WI(i) = sqrt(H(i+1,i)*H(i,i+1)) and WI(i+1) = -WI(i).
*
* ILOZ (input) INTEGER
* IHIZ (input) INTEGER
* Specify the rows of Z to which transformations must be
* applied if WANTZ is .TRUE..
* 1 <= ILOZ <= ILO; IHI <= IHIZ <= N.
*
* Z (input/output) DOUBLE PRECISION array, dimension (LDZ,N)
* If WANTZ is .TRUE., on entry Z must contain the current
* matrix Z of transformations accumulated by DHSEQR, and on
* exit Z has been updated; transformations are applied only to
* the submatrix Z(ILOZ:IHIZ,ILO:IHI).
* If WANTZ is .FALSE., Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* .GT. 0: If INFO = i, DLAHQR failed to compute all the
* eigenvalues ILO to IHI in a total of 30 iterations
* per eigenvalue; elements i+1:ihi of WR and WI
* contain those eigenvalues which have been
* successfully computed.
*
* If INFO .GT. 0 and WANTT is .FALSE., then on exit,
* the remaining unconverged eigenvalues are the
* eigenvalues of the upper Hessenberg matrix rows
* and columns ILO thorugh INFO of the final, output
* value of H.
*
* If INFO .GT. 0 and WANTT is .TRUE., then on exit
* (*) (initial value of H)*U = U*(final value of H)
* where U is an orthognal matrix. The final
* value of H is upper Hessenberg and triangular in
* rows and columns INFO+1 through IHI.
*
* If INFO .GT. 0 and WANTZ is .TRUE., then on exit
* (final value of Z) = (initial value of Z)*U
* where U is the orthogonal matrix in (*)
* (regardless of the value of WANTT.)
*
* Further Details
* ===============
*
* 02-96 Based on modifications by
* David Day, Sandia National Laboratory, USA
*
* 12-04 Further modifications by
* Ralph Byers, University of Kansas, USA
*
* This is a modified version of DLAHQR from LAPACK version 3.0.
* It is (1) more robust against overflow and underflow and
* (2) adopts the more conservative Ahues & Tisseur stopping
* criterion (LAWN 122, 1997).
*
* =========================================================
*
* .. Parameters ..
*
*
* @param wantt
* @param wantz
* @param n
* @param ilo
* @param ihi
* @param h
* @param ldh
* @param wr
* @param wi
* @param iloz
* @param ihiz
* @param z
* @param ldz
* @param info
*
*/
abstract public void dlahqr(boolean wantt, boolean wantz, int n, int ilo, int ihi, double[] h, int ldh, double[] wr, double[] wi, int iloz, int ihiz, double[] z, int ldz, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAHQR is an auxiliary routine called by DHSEQR to update the
* eigenvalues and Schur decomposition already computed by DHSEQR, by
* dealing with the Hessenberg submatrix in rows and columns ILO to
* IHI.
*
* Arguments
* =========
*
* WANTT (input) LOGICAL
* = .TRUE. : the full Schur form T is required;
* = .FALSE.: only eigenvalues are required.
*
* WANTZ (input) LOGICAL
* = .TRUE. : the matrix of Schur vectors Z is required;
* = .FALSE.: Schur vectors are not required.
*
* N (input) INTEGER
* The order of the matrix H. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* It is assumed that H is already upper quasi-triangular in
* rows and columns IHI+1:N, and that H(ILO,ILO-1) = 0 (unless
* ILO = 1). DLAHQR works primarily with the Hessenberg
* submatrix in rows and columns ILO to IHI, but applies
* transformations to all of H if WANTT is .TRUE..
* 1 <= ILO <= max(1,IHI); IHI <= N.
*
* H (input/output) DOUBLE PRECISION array, dimension (LDH,N)
* On entry, the upper Hessenberg matrix H.
* On exit, if INFO is zero and if WANTT is .TRUE., H is upper
* quasi-triangular in rows and columns ILO:IHI, with any
* 2-by-2 diagonal blocks in standard form. If INFO is zero
* and WANTT is .FALSE., the contents of H are unspecified on
* exit. The output state of H if INFO is nonzero is given
* below under the description of INFO.
*
* LDH (input) INTEGER
* The leading dimension of the array H. LDH >= max(1,N).
*
* WR (output) DOUBLE PRECISION array, dimension (N)
* WI (output) DOUBLE PRECISION array, dimension (N)
* The real and imaginary parts, respectively, of the computed
* eigenvalues ILO to IHI are stored in the corresponding
* elements of WR and WI. If two eigenvalues are computed as a
* complex conjugate pair, they are stored in consecutive
* elements of WR and WI, say the i-th and (i+1)th, with
* WI(i) > 0 and WI(i+1) < 0. If WANTT is .TRUE., the
* eigenvalues are stored in the same order as on the diagonal
* of the Schur form returned in H, with WR(i) = H(i,i), and, if
* H(i:i+1,i:i+1) is a 2-by-2 diagonal block,
* WI(i) = sqrt(H(i+1,i)*H(i,i+1)) and WI(i+1) = -WI(i).
*
* ILOZ (input) INTEGER
* IHIZ (input) INTEGER
* Specify the rows of Z to which transformations must be
* applied if WANTZ is .TRUE..
* 1 <= ILOZ <= ILO; IHI <= IHIZ <= N.
*
* Z (input/output) DOUBLE PRECISION array, dimension (LDZ,N)
* If WANTZ is .TRUE., on entry Z must contain the current
* matrix Z of transformations accumulated by DHSEQR, and on
* exit Z has been updated; transformations are applied only to
* the submatrix Z(ILOZ:IHIZ,ILO:IHI).
* If WANTZ is .FALSE., Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* .GT. 0: If INFO = i, DLAHQR failed to compute all the
* eigenvalues ILO to IHI in a total of 30 iterations
* per eigenvalue; elements i+1:ihi of WR and WI
* contain those eigenvalues which have been
* successfully computed.
*
* If INFO .GT. 0 and WANTT is .FALSE., then on exit,
* the remaining unconverged eigenvalues are the
* eigenvalues of the upper Hessenberg matrix rows
* and columns ILO thorugh INFO of the final, output
* value of H.
*
* If INFO .GT. 0 and WANTT is .TRUE., then on exit
* (*) (initial value of H)*U = U*(final value of H)
* where U is an orthognal matrix. The final
* value of H is upper Hessenberg and triangular in
* rows and columns INFO+1 through IHI.
*
* If INFO .GT. 0 and WANTZ is .TRUE., then on exit
* (final value of Z) = (initial value of Z)*U
* where U is the orthogonal matrix in (*)
* (regardless of the value of WANTT.)
*
* Further Details
* ===============
*
* 02-96 Based on modifications by
* David Day, Sandia National Laboratory, USA
*
* 12-04 Further modifications by
* Ralph Byers, University of Kansas, USA
*
* This is a modified version of DLAHQR from LAPACK version 3.0.
* It is (1) more robust against overflow and underflow and
* (2) adopts the more conservative Ahues & Tisseur stopping
* criterion (LAWN 122, 1997).
*
* =========================================================
*
* .. Parameters ..
*
*
* @param wantt
* @param wantz
* @param n
* @param ilo
* @param ihi
* @param h
* @param _h_offset
* @param ldh
* @param wr
* @param _wr_offset
* @param wi
* @param _wi_offset
* @param iloz
* @param ihiz
* @param z
* @param _z_offset
* @param ldz
* @param info
*
*/
abstract public void dlahqr(boolean wantt, boolean wantz, int n, int ilo, int ihi, double[] h, int _h_offset, int ldh, double[] wr, int _wr_offset, double[] wi, int _wi_offset, int iloz, int ihiz, double[] z, int _z_offset, int ldz, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAHR2 reduces the first NB columns of A real general n-BY-(n-k+1)
* matrix A so that elements below the k-th subdiagonal are zero. The
* reduction is performed by an orthogonal similarity transformation
* Q' * A * Q. The routine returns the matrices V and T which determine
* Q as a block reflector I - V*T*V', and also the matrix Y = A * V * T.
*
* This is an auxiliary routine called by DGEHRD.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A.
*
* K (input) INTEGER
* The offset for the reduction. Elements below the k-th
* subdiagonal in the first NB columns are reduced to zero.
* K < N.
*
* NB (input) INTEGER
* The number of columns to be reduced.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N-K+1)
* On entry, the n-by-(n-k+1) general matrix A.
* On exit, the elements on and above the k-th subdiagonal in
* the first NB columns are overwritten with the corresponding
* elements of the reduced matrix; the elements below the k-th
* subdiagonal, with the array TAU, represent the matrix Q as a
* product of elementary reflectors. The other columns of A are
* unchanged. See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* TAU (output) DOUBLE PRECISION array, dimension (NB)
* The scalar factors of the elementary reflectors. See Further
* Details.
*
* T (output) DOUBLE PRECISION array, dimension (LDT,NB)
* The upper triangular matrix T.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= NB.
*
* Y (output) DOUBLE PRECISION array, dimension (LDY,NB)
* The n-by-nb matrix Y.
*
* LDY (input) INTEGER
* The leading dimension of the array Y. LDY >= N.
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of nb elementary reflectors
*
* Q = H(1) H(2) . . . H(nb).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i+k-1) = 0, v(i+k) = 1; v(i+k+1:n) is stored on exit in
* A(i+k+1:n,i), and tau in TAU(i).
*
* The elements of the vectors v together form the (n-k+1)-by-nb matrix
* V which is needed, with T and Y, to apply the transformation to the
* unreduced part of the matrix, using an update of the form:
* A := (I - V*T*V') * (A - Y*V').
*
* The contents of A on exit are illustrated by the following example
* with n = 7, k = 3 and nb = 2:
*
* ( a a a a a )
* ( a a a a a )
* ( a a a a a )
* ( h h a a a )
* ( v1 h a a a )
* ( v1 v2 a a a )
* ( v1 v2 a a a )
*
* where a denotes an element of the original matrix A, h denotes a
* modified element of the upper Hessenberg matrix H, and vi denotes an
* element of the vector defining H(i).
*
* This file is a slight modification of LAPACK-3.0's DLAHRD
* incorporating improvements proposed by Quintana-Orti and Van de
* Gejin. Note that the entries of A(1:K,2:NB) differ from those
* returned by the original LAPACK routine. This function is
* not backward compatible with LAPACK3.0.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param k
* @param nb
* @param a
* @param lda
* @param tau
* @param t
* @param ldt
* @param y
* @param ldy
*
*/
abstract public void dlahr2(int n, int k, int nb, double[] a, int lda, double[] tau, double[] t, int ldt, double[] y, int ldy);
/**
*
* ..
*
* Purpose
* =======
*
* DLAHR2 reduces the first NB columns of A real general n-BY-(n-k+1)
* matrix A so that elements below the k-th subdiagonal are zero. The
* reduction is performed by an orthogonal similarity transformation
* Q' * A * Q. The routine returns the matrices V and T which determine
* Q as a block reflector I - V*T*V', and also the matrix Y = A * V * T.
*
* This is an auxiliary routine called by DGEHRD.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A.
*
* K (input) INTEGER
* The offset for the reduction. Elements below the k-th
* subdiagonal in the first NB columns are reduced to zero.
* K < N.
*
* NB (input) INTEGER
* The number of columns to be reduced.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N-K+1)
* On entry, the n-by-(n-k+1) general matrix A.
* On exit, the elements on and above the k-th subdiagonal in
* the first NB columns are overwritten with the corresponding
* elements of the reduced matrix; the elements below the k-th
* subdiagonal, with the array TAU, represent the matrix Q as a
* product of elementary reflectors. The other columns of A are
* unchanged. See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* TAU (output) DOUBLE PRECISION array, dimension (NB)
* The scalar factors of the elementary reflectors. See Further
* Details.
*
* T (output) DOUBLE PRECISION array, dimension (LDT,NB)
* The upper triangular matrix T.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= NB.
*
* Y (output) DOUBLE PRECISION array, dimension (LDY,NB)
* The n-by-nb matrix Y.
*
* LDY (input) INTEGER
* The leading dimension of the array Y. LDY >= N.
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of nb elementary reflectors
*
* Q = H(1) H(2) . . . H(nb).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i+k-1) = 0, v(i+k) = 1; v(i+k+1:n) is stored on exit in
* A(i+k+1:n,i), and tau in TAU(i).
*
* The elements of the vectors v together form the (n-k+1)-by-nb matrix
* V which is needed, with T and Y, to apply the transformation to the
* unreduced part of the matrix, using an update of the form:
* A := (I - V*T*V') * (A - Y*V').
*
* The contents of A on exit are illustrated by the following example
* with n = 7, k = 3 and nb = 2:
*
* ( a a a a a )
* ( a a a a a )
* ( a a a a a )
* ( h h a a a )
* ( v1 h a a a )
* ( v1 v2 a a a )
* ( v1 v2 a a a )
*
* where a denotes an element of the original matrix A, h denotes a
* modified element of the upper Hessenberg matrix H, and vi denotes an
* element of the vector defining H(i).
*
* This file is a slight modification of LAPACK-3.0's DLAHRD
* incorporating improvements proposed by Quintana-Orti and Van de
* Gejin. Note that the entries of A(1:K,2:NB) differ from those
* returned by the original LAPACK routine. This function is
* not backward compatible with LAPACK3.0.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param k
* @param nb
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param t
* @param _t_offset
* @param ldt
* @param y
* @param _y_offset
* @param ldy
*
*/
abstract public void dlahr2(int n, int k, int nb, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] t, int _t_offset, int ldt, double[] y, int _y_offset, int ldy);
/**
*
* ..
*
* Purpose
* =======
*
* DLAHRD reduces the first NB columns of a real general n-by-(n-k+1)
* matrix A so that elements below the k-th subdiagonal are zero. The
* reduction is performed by an orthogonal similarity transformation
* Q' * A * Q. The routine returns the matrices V and T which determine
* Q as a block reflector I - V*T*V', and also the matrix Y = A * V * T.
*
* This is an OBSOLETE auxiliary routine.
* This routine will be 'deprecated' in a future release.
* Please use the new routine DLAHR2 instead.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A.
*
* K (input) INTEGER
* The offset for the reduction. Elements below the k-th
* subdiagonal in the first NB columns are reduced to zero.
*
* NB (input) INTEGER
* The number of columns to be reduced.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N-K+1)
* On entry, the n-by-(n-k+1) general matrix A.
* On exit, the elements on and above the k-th subdiagonal in
* the first NB columns are overwritten with the corresponding
* elements of the reduced matrix; the elements below the k-th
* subdiagonal, with the array TAU, represent the matrix Q as a
* product of elementary reflectors. The other columns of A are
* unchanged. See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* TAU (output) DOUBLE PRECISION array, dimension (NB)
* The scalar factors of the elementary reflectors. See Further
* Details.
*
* T (output) DOUBLE PRECISION array, dimension (LDT,NB)
* The upper triangular matrix T.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= NB.
*
* Y (output) DOUBLE PRECISION array, dimension (LDY,NB)
* The n-by-nb matrix Y.
*
* LDY (input) INTEGER
* The leading dimension of the array Y. LDY >= N.
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of nb elementary reflectors
*
* Q = H(1) H(2) . . . H(nb).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i+k-1) = 0, v(i+k) = 1; v(i+k+1:n) is stored on exit in
* A(i+k+1:n,i), and tau in TAU(i).
*
* The elements of the vectors v together form the (n-k+1)-by-nb matrix
* V which is needed, with T and Y, to apply the transformation to the
* unreduced part of the matrix, using an update of the form:
* A := (I - V*T*V') * (A - Y*V').
*
* The contents of A on exit are illustrated by the following example
* with n = 7, k = 3 and nb = 2:
*
* ( a h a a a )
* ( a h a a a )
* ( a h a a a )
* ( h h a a a )
* ( v1 h a a a )
* ( v1 v2 a a a )
* ( v1 v2 a a a )
*
* where a denotes an element of the original matrix A, h denotes a
* modified element of the upper Hessenberg matrix H, and vi denotes an
* element of the vector defining H(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param k
* @param nb
* @param a
* @param lda
* @param tau
* @param t
* @param ldt
* @param y
* @param ldy
*
*/
abstract public void dlahrd(int n, int k, int nb, double[] a, int lda, double[] tau, double[] t, int ldt, double[] y, int ldy);
/**
*
* ..
*
* Purpose
* =======
*
* DLAHRD reduces the first NB columns of a real general n-by-(n-k+1)
* matrix A so that elements below the k-th subdiagonal are zero. The
* reduction is performed by an orthogonal similarity transformation
* Q' * A * Q. The routine returns the matrices V and T which determine
* Q as a block reflector I - V*T*V', and also the matrix Y = A * V * T.
*
* This is an OBSOLETE auxiliary routine.
* This routine will be 'deprecated' in a future release.
* Please use the new routine DLAHR2 instead.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A.
*
* K (input) INTEGER
* The offset for the reduction. Elements below the k-th
* subdiagonal in the first NB columns are reduced to zero.
*
* NB (input) INTEGER
* The number of columns to be reduced.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N-K+1)
* On entry, the n-by-(n-k+1) general matrix A.
* On exit, the elements on and above the k-th subdiagonal in
* the first NB columns are overwritten with the corresponding
* elements of the reduced matrix; the elements below the k-th
* subdiagonal, with the array TAU, represent the matrix Q as a
* product of elementary reflectors. The other columns of A are
* unchanged. See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* TAU (output) DOUBLE PRECISION array, dimension (NB)
* The scalar factors of the elementary reflectors. See Further
* Details.
*
* T (output) DOUBLE PRECISION array, dimension (LDT,NB)
* The upper triangular matrix T.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= NB.
*
* Y (output) DOUBLE PRECISION array, dimension (LDY,NB)
* The n-by-nb matrix Y.
*
* LDY (input) INTEGER
* The leading dimension of the array Y. LDY >= N.
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of nb elementary reflectors
*
* Q = H(1) H(2) . . . H(nb).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i+k-1) = 0, v(i+k) = 1; v(i+k+1:n) is stored on exit in
* A(i+k+1:n,i), and tau in TAU(i).
*
* The elements of the vectors v together form the (n-k+1)-by-nb matrix
* V which is needed, with T and Y, to apply the transformation to the
* unreduced part of the matrix, using an update of the form:
* A := (I - V*T*V') * (A - Y*V').
*
* The contents of A on exit are illustrated by the following example
* with n = 7, k = 3 and nb = 2:
*
* ( a h a a a )
* ( a h a a a )
* ( a h a a a )
* ( h h a a a )
* ( v1 h a a a )
* ( v1 v2 a a a )
* ( v1 v2 a a a )
*
* where a denotes an element of the original matrix A, h denotes a
* modified element of the upper Hessenberg matrix H, and vi denotes an
* element of the vector defining H(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param k
* @param nb
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param t
* @param _t_offset
* @param ldt
* @param y
* @param _y_offset
* @param ldy
*
*/
abstract public void dlahrd(int n, int k, int nb, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] t, int _t_offset, int ldt, double[] y, int _y_offset, int ldy);
/**
*
* ..
*
* Purpose
* =======
*
* DLAIC1 applies one step of incremental condition estimation in
* its simplest version:
*
* Let x, twonorm(x) = 1, be an approximate singular vector of an j-by-j
* lower triangular matrix L, such that
* twonorm(L*x) = sest
* Then DLAIC1 computes sestpr, s, c such that
* the vector
* [ s*x ]
* xhat = [ c ]
* is an approximate singular vector of
* [ L 0 ]
* Lhat = [ w' gamma ]
* in the sense that
* twonorm(Lhat*xhat) = sestpr.
*
* Depending on JOB, an estimate for the largest or smallest singular
* value is computed.
*
* Note that [s c]' and sestpr**2 is an eigenpair of the system
*
* diag(sest*sest, 0) + [alpha gamma] * [ alpha ]
* [ gamma ]
*
* where alpha = x'*w.
*
* Arguments
* =========
*
* JOB (input) INTEGER
* = 1: an estimate for the largest singular value is computed.
* = 2: an estimate for the smallest singular value is computed.
*
* J (input) INTEGER
* Length of X and W
*
* X (input) DOUBLE PRECISION array, dimension (J)
* The j-vector x.
*
* SEST (input) DOUBLE PRECISION
* Estimated singular value of j by j matrix L
*
* W (input) DOUBLE PRECISION array, dimension (J)
* The j-vector w.
*
* GAMMA (input) DOUBLE PRECISION
* The diagonal element gamma.
*
* SESTPR (output) DOUBLE PRECISION
* Estimated singular value of (j+1) by (j+1) matrix Lhat.
*
* S (output) DOUBLE PRECISION
* Sine needed in forming xhat.
*
* C (output) DOUBLE PRECISION
* Cosine needed in forming xhat.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param j
* @param x
* @param sest
* @param w
* @param gamma
* @param sestpr
* @param s
* @param c
*
*/
abstract public void dlaic1(int job, int j, double[] x, double sest, double[] w, double gamma, org.netlib.util.doubleW sestpr, org.netlib.util.doubleW s, org.netlib.util.doubleW c);
/**
*
* ..
*
* Purpose
* =======
*
* DLAIC1 applies one step of incremental condition estimation in
* its simplest version:
*
* Let x, twonorm(x) = 1, be an approximate singular vector of an j-by-j
* lower triangular matrix L, such that
* twonorm(L*x) = sest
* Then DLAIC1 computes sestpr, s, c such that
* the vector
* [ s*x ]
* xhat = [ c ]
* is an approximate singular vector of
* [ L 0 ]
* Lhat = [ w' gamma ]
* in the sense that
* twonorm(Lhat*xhat) = sestpr.
*
* Depending on JOB, an estimate for the largest or smallest singular
* value is computed.
*
* Note that [s c]' and sestpr**2 is an eigenpair of the system
*
* diag(sest*sest, 0) + [alpha gamma] * [ alpha ]
* [ gamma ]
*
* where alpha = x'*w.
*
* Arguments
* =========
*
* JOB (input) INTEGER
* = 1: an estimate for the largest singular value is computed.
* = 2: an estimate for the smallest singular value is computed.
*
* J (input) INTEGER
* Length of X and W
*
* X (input) DOUBLE PRECISION array, dimension (J)
* The j-vector x.
*
* SEST (input) DOUBLE PRECISION
* Estimated singular value of j by j matrix L
*
* W (input) DOUBLE PRECISION array, dimension (J)
* The j-vector w.
*
* GAMMA (input) DOUBLE PRECISION
* The diagonal element gamma.
*
* SESTPR (output) DOUBLE PRECISION
* Estimated singular value of (j+1) by (j+1) matrix Lhat.
*
* S (output) DOUBLE PRECISION
* Sine needed in forming xhat.
*
* C (output) DOUBLE PRECISION
* Cosine needed in forming xhat.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param j
* @param x
* @param _x_offset
* @param sest
* @param w
* @param _w_offset
* @param gamma
* @param sestpr
* @param s
* @param c
*
*/
abstract public void dlaic1(int job, int j, double[] x, int _x_offset, double sest, double[] w, int _w_offset, double gamma, org.netlib.util.doubleW sestpr, org.netlib.util.doubleW s, org.netlib.util.doubleW c);
/**
*
* ..
*
* Purpose
* =======
*
* This routine is not for general use. It exists solely to avoid
* over-optimization in DISNAN.
*
* DLAISNAN checks for NaNs by comparing its two arguments for
* inequality. NaN is the only floating-point value where NaN != NaN
* returns .TRUE. To check for NaNs, pass the same variable as both
* arguments.
*
* Strictly speaking, Fortran does not allow aliasing of function
* arguments. So a compiler must assume that the two arguments are
* not the same variable, and the test will not be optimized away.
* Interprocedural or whole-program optimization may delete this
* test. The ISNAN functions will be replaced by the correct
* Fortran 03 intrinsic once the intrinsic is widely available.
*
* Arguments
* =========
*
* DIN1 (input) DOUBLE PRECISION
* DIN2 (input) DOUBLE PRECISION
* Two numbers to compare for inequality.
*
* =====================================================================
*
* .. Executable Statements ..
*
*
* @param din1
* @param din2
* @return
*/
abstract public boolean dlaisnan(double din1, double din2);
/**
*
* ..
*
* Purpose
* =======
*
* DLALN2 solves a system of the form (ca A - w D ) X = s B
* or (ca A' - w D) X = s B with possible scaling ("s") and
* perturbation of A. (A' means A-transpose.)
*
* A is an NA x NA real matrix, ca is a real scalar, D is an NA x NA
* real diagonal matrix, w is a real or complex value, and X and B are
* NA x 1 matrices -- real if w is real, complex if w is complex. NA
* may be 1 or 2.
*
* If w is complex, X and B are represented as NA x 2 matrices,
* the first column of each being the real part and the second
* being the imaginary part.
*
* "s" is a scaling factor (.LE. 1), computed by DLALN2, which is
* so chosen that X can be computed without overflow. X is further
* scaled if necessary to assure that norm(ca A - w D)*norm(X) is less
* than overflow.
*
* If both singular values of (ca A - w D) are less than SMIN,
* SMIN*identity will be used instead of (ca A - w D). If only one
* singular value is less than SMIN, one element of (ca A - w D) will be
* perturbed enough to make the smallest singular value roughly SMIN.
* If both singular values are at least SMIN, (ca A - w D) will not be
* perturbed. In any case, the perturbation will be at most some small
* multiple of max( SMIN, ulp*norm(ca A - w D) ). The singular values
* are computed by infinity-norm approximations, and thus will only be
* correct to a factor of 2 or so.
*
* Note: all input quantities are assumed to be smaller than overflow
* by a reasonable factor. (See BIGNUM.)
*
* Arguments
* ==========
*
* LTRANS (input) LOGICAL
* =.TRUE.: A-transpose will be used.
* =.FALSE.: A will be used (not transposed.)
*
* NA (input) INTEGER
* The size of the matrix A. It may (only) be 1 or 2.
*
* NW (input) INTEGER
* 1 if "w" is real, 2 if "w" is complex. It may only be 1
* or 2.
*
* SMIN (input) DOUBLE PRECISION
* The desired lower bound on the singular values of A. This
* should be a safe distance away from underflow or overflow,
* say, between (underflow/machine precision) and (machine
* precision * overflow ). (See BIGNUM and ULP.)
*
* CA (input) DOUBLE PRECISION
* The coefficient c, which A is multiplied by.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,NA)
* The NA x NA matrix A.
*
* LDA (input) INTEGER
* The leading dimension of A. It must be at least NA.
*
* D1 (input) DOUBLE PRECISION
* The 1,1 element in the diagonal matrix D.
*
* D2 (input) DOUBLE PRECISION
* The 2,2 element in the diagonal matrix D. Not used if NW=1.
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NW)
* The NA x NW matrix B (right-hand side). If NW=2 ("w" is
* complex), column 1 contains the real part of B and column 2
* contains the imaginary part.
*
* LDB (input) INTEGER
* The leading dimension of B. It must be at least NA.
*
* WR (input) DOUBLE PRECISION
* The real part of the scalar "w".
*
* WI (input) DOUBLE PRECISION
* The imaginary part of the scalar "w". Not used if NW=1.
*
* X (output) DOUBLE PRECISION array, dimension (LDX,NW)
* The NA x NW matrix X (unknowns), as computed by DLALN2.
* If NW=2 ("w" is complex), on exit, column 1 will contain
* the real part of X and column 2 will contain the imaginary
* part.
*
* LDX (input) INTEGER
* The leading dimension of X. It must be at least NA.
*
* SCALE (output) DOUBLE PRECISION
* The scale factor that B must be multiplied by to insure
* that overflow does not occur when computing X. Thus,
* (ca A - w D) X will be SCALE*B, not B (ignoring
* perturbations of A.) It will be at most 1.
*
* XNORM (output) DOUBLE PRECISION
* The infinity-norm of X, when X is regarded as an NA x NW
* real matrix.
*
* INFO (output) INTEGER
* An error flag. It will be set to zero if no error occurs,
* a negative number if an argument is in error, or a positive
* number if ca A - w D had to be perturbed.
* The possible values are:
* = 0: No error occurred, and (ca A - w D) did not have to be
* perturbed.
* = 1: (ca A - w D) had to be perturbed to make its smallest
* (or only) singular value greater than SMIN.
* NOTE: In the interests of speed, this routine does not
* check the inputs for errors.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param ltrans
* @param na
* @param nw
* @param smin
* @param ca
* @param a
* @param lda
* @param d1
* @param d2
* @param b
* @param ldb
* @param wr
* @param wi
* @param x
* @param ldx
* @param scale
* @param xnorm
* @param info
*
*/
abstract public void dlaln2(boolean ltrans, int na, int nw, double smin, double ca, double[] a, int lda, double d1, double d2, double[] b, int ldb, double wr, double wi, double[] x, int ldx, org.netlib.util.doubleW scale, org.netlib.util.doubleW xnorm, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLALN2 solves a system of the form (ca A - w D ) X = s B
* or (ca A' - w D) X = s B with possible scaling ("s") and
* perturbation of A. (A' means A-transpose.)
*
* A is an NA x NA real matrix, ca is a real scalar, D is an NA x NA
* real diagonal matrix, w is a real or complex value, and X and B are
* NA x 1 matrices -- real if w is real, complex if w is complex. NA
* may be 1 or 2.
*
* If w is complex, X and B are represented as NA x 2 matrices,
* the first column of each being the real part and the second
* being the imaginary part.
*
* "s" is a scaling factor (.LE. 1), computed by DLALN2, which is
* so chosen that X can be computed without overflow. X is further
* scaled if necessary to assure that norm(ca A - w D)*norm(X) is less
* than overflow.
*
* If both singular values of (ca A - w D) are less than SMIN,
* SMIN*identity will be used instead of (ca A - w D). If only one
* singular value is less than SMIN, one element of (ca A - w D) will be
* perturbed enough to make the smallest singular value roughly SMIN.
* If both singular values are at least SMIN, (ca A - w D) will not be
* perturbed. In any case, the perturbation will be at most some small
* multiple of max( SMIN, ulp*norm(ca A - w D) ). The singular values
* are computed by infinity-norm approximations, and thus will only be
* correct to a factor of 2 or so.
*
* Note: all input quantities are assumed to be smaller than overflow
* by a reasonable factor. (See BIGNUM.)
*
* Arguments
* ==========
*
* LTRANS (input) LOGICAL
* =.TRUE.: A-transpose will be used.
* =.FALSE.: A will be used (not transposed.)
*
* NA (input) INTEGER
* The size of the matrix A. It may (only) be 1 or 2.
*
* NW (input) INTEGER
* 1 if "w" is real, 2 if "w" is complex. It may only be 1
* or 2.
*
* SMIN (input) DOUBLE PRECISION
* The desired lower bound on the singular values of A. This
* should be a safe distance away from underflow or overflow,
* say, between (underflow/machine precision) and (machine
* precision * overflow ). (See BIGNUM and ULP.)
*
* CA (input) DOUBLE PRECISION
* The coefficient c, which A is multiplied by.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,NA)
* The NA x NA matrix A.
*
* LDA (input) INTEGER
* The leading dimension of A. It must be at least NA.
*
* D1 (input) DOUBLE PRECISION
* The 1,1 element in the diagonal matrix D.
*
* D2 (input) DOUBLE PRECISION
* The 2,2 element in the diagonal matrix D. Not used if NW=1.
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NW)
* The NA x NW matrix B (right-hand side). If NW=2 ("w" is
* complex), column 1 contains the real part of B and column 2
* contains the imaginary part.
*
* LDB (input) INTEGER
* The leading dimension of B. It must be at least NA.
*
* WR (input) DOUBLE PRECISION
* The real part of the scalar "w".
*
* WI (input) DOUBLE PRECISION
* The imaginary part of the scalar "w". Not used if NW=1.
*
* X (output) DOUBLE PRECISION array, dimension (LDX,NW)
* The NA x NW matrix X (unknowns), as computed by DLALN2.
* If NW=2 ("w" is complex), on exit, column 1 will contain
* the real part of X and column 2 will contain the imaginary
* part.
*
* LDX (input) INTEGER
* The leading dimension of X. It must be at least NA.
*
* SCALE (output) DOUBLE PRECISION
* The scale factor that B must be multiplied by to insure
* that overflow does not occur when computing X. Thus,
* (ca A - w D) X will be SCALE*B, not B (ignoring
* perturbations of A.) It will be at most 1.
*
* XNORM (output) DOUBLE PRECISION
* The infinity-norm of X, when X is regarded as an NA x NW
* real matrix.
*
* INFO (output) INTEGER
* An error flag. It will be set to zero if no error occurs,
* a negative number if an argument is in error, or a positive
* number if ca A - w D had to be perturbed.
* The possible values are:
* = 0: No error occurred, and (ca A - w D) did not have to be
* perturbed.
* = 1: (ca A - w D) had to be perturbed to make its smallest
* (or only) singular value greater than SMIN.
* NOTE: In the interests of speed, this routine does not
* check the inputs for errors.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param ltrans
* @param na
* @param nw
* @param smin
* @param ca
* @param a
* @param _a_offset
* @param lda
* @param d1
* @param d2
* @param b
* @param _b_offset
* @param ldb
* @param wr
* @param wi
* @param x
* @param _x_offset
* @param ldx
* @param scale
* @param xnorm
* @param info
*
*/
abstract public void dlaln2(boolean ltrans, int na, int nw, double smin, double ca, double[] a, int _a_offset, int lda, double d1, double d2, double[] b, int _b_offset, int ldb, double wr, double wi, double[] x, int _x_offset, int ldx, org.netlib.util.doubleW scale, org.netlib.util.doubleW xnorm, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLALS0 applies back the multiplying factors of either the left or the
* right singular vector matrix of a diagonal matrix appended by a row
* to the right hand side matrix B in solving the least squares problem
* using the divide-and-conquer SVD approach.
*
* For the left singular vector matrix, three types of orthogonal
* matrices are involved:
*
* (1L) Givens rotations: the number of such rotations is GIVPTR; the
* pairs of columns/rows they were applied to are stored in GIVCOL;
* and the C- and S-values of these rotations are stored in GIVNUM.
*
* (2L) Permutation. The (NL+1)-st row of B is to be moved to the first
* row, and for J=2:N, PERM(J)-th row of B is to be moved to the
* J-th row.
*
* (3L) The left singular vector matrix of the remaining matrix.
*
* For the right singular vector matrix, four types of orthogonal
* matrices are involved:
*
* (1R) The right singular vector matrix of the remaining matrix.
*
* (2R) If SQRE = 1, one extra Givens rotation to generate the right
* null space.
*
* (3R) The inverse transformation of (2L).
*
* (4R) The inverse transformation of (1L).
*
* Arguments
* =========
*
* ICOMPQ (input) INTEGER
* Specifies whether singular vectors are to be computed in
* factored form:
* = 0: Left singular vector matrix.
* = 1: Right singular vector matrix.
*
* NL (input) INTEGER
* The row dimension of the upper block. NL >= 1.
*
* NR (input) INTEGER
* The row dimension of the lower block. NR >= 1.
*
* SQRE (input) INTEGER
* = 0: the lower block is an NR-by-NR square matrix.
* = 1: the lower block is an NR-by-(NR+1) rectangular matrix.
*
* The bidiagonal matrix has row dimension N = NL + NR + 1,
* and column dimension M = N + SQRE.
*
* NRHS (input) INTEGER
* The number of columns of B and BX. NRHS must be at least 1.
*
* B (input/output) DOUBLE PRECISION array, dimension ( LDB, NRHS )
* On input, B contains the right hand sides of the least
* squares problem in rows 1 through M. On output, B contains
* the solution X in rows 1 through N.
*
* LDB (input) INTEGER
* The leading dimension of B. LDB must be at least
* max(1,MAX( M, N ) ).
*
* BX (workspace) DOUBLE PRECISION array, dimension ( LDBX, NRHS )
*
* LDBX (input) INTEGER
* The leading dimension of BX.
*
* PERM (input) INTEGER array, dimension ( N )
* The permutations (from deflation and sorting) applied
* to the two blocks.
*
* GIVPTR (input) INTEGER
* The number of Givens rotations which took place in this
* subproblem.
*
* GIVCOL (input) INTEGER array, dimension ( LDGCOL, 2 )
* Each pair of numbers indicates a pair of rows/columns
* involved in a Givens rotation.
*
* LDGCOL (input) INTEGER
* The leading dimension of GIVCOL, must be at least N.
*
* GIVNUM (input) DOUBLE PRECISION array, dimension ( LDGNUM, 2 )
* Each number indicates the C or S value used in the
* corresponding Givens rotation.
*
* LDGNUM (input) INTEGER
* The leading dimension of arrays DIFR, POLES and
* GIVNUM, must be at least K.
*
* POLES (input) DOUBLE PRECISION array, dimension ( LDGNUM, 2 )
* On entry, POLES(1:K, 1) contains the new singular
* values obtained from solving the secular equation, and
* POLES(1:K, 2) is an array containing the poles in the secular
* equation.
*
* DIFL (input) DOUBLE PRECISION array, dimension ( K ).
* On entry, DIFL(I) is the distance between I-th updated
* (undeflated) singular value and the I-th (undeflated) old
* singular value.
*
* DIFR (input) DOUBLE PRECISION array, dimension ( LDGNUM, 2 ).
* On entry, DIFR(I, 1) contains the distances between I-th
* updated (undeflated) singular value and the I+1-th
* (undeflated) old singular value. And DIFR(I, 2) is the
* normalizing factor for the I-th right singular vector.
*
* Z (input) DOUBLE PRECISION array, dimension ( K )
* Contain the components of the deflation-adjusted updating row
* vector.
*
* K (input) INTEGER
* Contains the dimension of the non-deflated matrix,
* This is the order of the related secular equation. 1 <= K <=N.
*
* C (input) DOUBLE PRECISION
* C contains garbage if SQRE =0 and the C-value of a Givens
* rotation related to the right null space if SQRE = 1.
*
* S (input) DOUBLE PRECISION
* S contains garbage if SQRE =0 and the S-value of a Givens
* rotation related to the right null space if SQRE = 1.
*
* WORK (workspace) DOUBLE PRECISION array, dimension ( K )
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Ren-Cang Li, Computer Science Division, University of
* California at Berkeley, USA
* Osni Marques, LBNL/NERSC, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param nl
* @param nr
* @param sqre
* @param nrhs
* @param b
* @param ldb
* @param bx
* @param ldbx
* @param perm
* @param givptr
* @param givcol
* @param ldgcol
* @param givnum
* @param ldgnum
* @param poles
* @param difl
* @param difr
* @param z
* @param k
* @param c
* @param s
* @param work
* @param info
*
*/
abstract public void dlals0(int icompq, int nl, int nr, int sqre, int nrhs, double[] b, int ldb, double[] bx, int ldbx, int[] perm, int givptr, int[] givcol, int ldgcol, double[] givnum, int ldgnum, double[] poles, double[] difl, double[] difr, double[] z, int k, double c, double s, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLALS0 applies back the multiplying factors of either the left or the
* right singular vector matrix of a diagonal matrix appended by a row
* to the right hand side matrix B in solving the least squares problem
* using the divide-and-conquer SVD approach.
*
* For the left singular vector matrix, three types of orthogonal
* matrices are involved:
*
* (1L) Givens rotations: the number of such rotations is GIVPTR; the
* pairs of columns/rows they were applied to are stored in GIVCOL;
* and the C- and S-values of these rotations are stored in GIVNUM.
*
* (2L) Permutation. The (NL+1)-st row of B is to be moved to the first
* row, and for J=2:N, PERM(J)-th row of B is to be moved to the
* J-th row.
*
* (3L) The left singular vector matrix of the remaining matrix.
*
* For the right singular vector matrix, four types of orthogonal
* matrices are involved:
*
* (1R) The right singular vector matrix of the remaining matrix.
*
* (2R) If SQRE = 1, one extra Givens rotation to generate the right
* null space.
*
* (3R) The inverse transformation of (2L).
*
* (4R) The inverse transformation of (1L).
*
* Arguments
* =========
*
* ICOMPQ (input) INTEGER
* Specifies whether singular vectors are to be computed in
* factored form:
* = 0: Left singular vector matrix.
* = 1: Right singular vector matrix.
*
* NL (input) INTEGER
* The row dimension of the upper block. NL >= 1.
*
* NR (input) INTEGER
* The row dimension of the lower block. NR >= 1.
*
* SQRE (input) INTEGER
* = 0: the lower block is an NR-by-NR square matrix.
* = 1: the lower block is an NR-by-(NR+1) rectangular matrix.
*
* The bidiagonal matrix has row dimension N = NL + NR + 1,
* and column dimension M = N + SQRE.
*
* NRHS (input) INTEGER
* The number of columns of B and BX. NRHS must be at least 1.
*
* B (input/output) DOUBLE PRECISION array, dimension ( LDB, NRHS )
* On input, B contains the right hand sides of the least
* squares problem in rows 1 through M. On output, B contains
* the solution X in rows 1 through N.
*
* LDB (input) INTEGER
* The leading dimension of B. LDB must be at least
* max(1,MAX( M, N ) ).
*
* BX (workspace) DOUBLE PRECISION array, dimension ( LDBX, NRHS )
*
* LDBX (input) INTEGER
* The leading dimension of BX.
*
* PERM (input) INTEGER array, dimension ( N )
* The permutations (from deflation and sorting) applied
* to the two blocks.
*
* GIVPTR (input) INTEGER
* The number of Givens rotations which took place in this
* subproblem.
*
* GIVCOL (input) INTEGER array, dimension ( LDGCOL, 2 )
* Each pair of numbers indicates a pair of rows/columns
* involved in a Givens rotation.
*
* LDGCOL (input) INTEGER
* The leading dimension of GIVCOL, must be at least N.
*
* GIVNUM (input) DOUBLE PRECISION array, dimension ( LDGNUM, 2 )
* Each number indicates the C or S value used in the
* corresponding Givens rotation.
*
* LDGNUM (input) INTEGER
* The leading dimension of arrays DIFR, POLES and
* GIVNUM, must be at least K.
*
* POLES (input) DOUBLE PRECISION array, dimension ( LDGNUM, 2 )
* On entry, POLES(1:K, 1) contains the new singular
* values obtained from solving the secular equation, and
* POLES(1:K, 2) is an array containing the poles in the secular
* equation.
*
* DIFL (input) DOUBLE PRECISION array, dimension ( K ).
* On entry, DIFL(I) is the distance between I-th updated
* (undeflated) singular value and the I-th (undeflated) old
* singular value.
*
* DIFR (input) DOUBLE PRECISION array, dimension ( LDGNUM, 2 ).
* On entry, DIFR(I, 1) contains the distances between I-th
* updated (undeflated) singular value and the I+1-th
* (undeflated) old singular value. And DIFR(I, 2) is the
* normalizing factor for the I-th right singular vector.
*
* Z (input) DOUBLE PRECISION array, dimension ( K )
* Contain the components of the deflation-adjusted updating row
* vector.
*
* K (input) INTEGER
* Contains the dimension of the non-deflated matrix,
* This is the order of the related secular equation. 1 <= K <=N.
*
* C (input) DOUBLE PRECISION
* C contains garbage if SQRE =0 and the C-value of a Givens
* rotation related to the right null space if SQRE = 1.
*
* S (input) DOUBLE PRECISION
* S contains garbage if SQRE =0 and the S-value of a Givens
* rotation related to the right null space if SQRE = 1.
*
* WORK (workspace) DOUBLE PRECISION array, dimension ( K )
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Ren-Cang Li, Computer Science Division, University of
* California at Berkeley, USA
* Osni Marques, LBNL/NERSC, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param nl
* @param nr
* @param sqre
* @param nrhs
* @param b
* @param _b_offset
* @param ldb
* @param bx
* @param _bx_offset
* @param ldbx
* @param perm
* @param _perm_offset
* @param givptr
* @param givcol
* @param _givcol_offset
* @param ldgcol
* @param givnum
* @param _givnum_offset
* @param ldgnum
* @param poles
* @param _poles_offset
* @param difl
* @param _difl_offset
* @param difr
* @param _difr_offset
* @param z
* @param _z_offset
* @param k
* @param c
* @param s
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dlals0(int icompq, int nl, int nr, int sqre, int nrhs, double[] b, int _b_offset, int ldb, double[] bx, int _bx_offset, int ldbx, int[] perm, int _perm_offset, int givptr, int[] givcol, int _givcol_offset, int ldgcol, double[] givnum, int _givnum_offset, int ldgnum, double[] poles, int _poles_offset, double[] difl, int _difl_offset, double[] difr, int _difr_offset, double[] z, int _z_offset, int k, double c, double s, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLALSA is an itermediate step in solving the least squares problem
* by computing the SVD of the coefficient matrix in compact form (The
* singular vectors are computed as products of simple orthorgonal
* matrices.).
*
* If ICOMPQ = 0, DLALSA applies the inverse of the left singular vector
* matrix of an upper bidiagonal matrix to the right hand side; and if
* ICOMPQ = 1, DLALSA applies the right singular vector matrix to the
* right hand side. The singular vector matrices were generated in
* compact form by DLALSA.
*
* Arguments
* =========
*
*
* ICOMPQ (input) INTEGER
* Specifies whether the left or the right singular vector
* matrix is involved.
* = 0: Left singular vector matrix
* = 1: Right singular vector matrix
*
* SMLSIZ (input) INTEGER
* The maximum size of the subproblems at the bottom of the
* computation tree.
*
* N (input) INTEGER
* The row and column dimensions of the upper bidiagonal matrix.
*
* NRHS (input) INTEGER
* The number of columns of B and BX. NRHS must be at least 1.
*
* B (input/output) DOUBLE PRECISION array, dimension ( LDB, NRHS )
* On input, B contains the right hand sides of the least
* squares problem in rows 1 through M.
* On output, B contains the solution X in rows 1 through N.
*
* LDB (input) INTEGER
* The leading dimension of B in the calling subprogram.
* LDB must be at least max(1,MAX( M, N ) ).
*
* BX (output) DOUBLE PRECISION array, dimension ( LDBX, NRHS )
* On exit, the result of applying the left or right singular
* vector matrix to B.
*
* LDBX (input) INTEGER
* The leading dimension of BX.
*
* U (input) DOUBLE PRECISION array, dimension ( LDU, SMLSIZ ).
* On entry, U contains the left singular vector matrices of all
* subproblems at the bottom level.
*
* LDU (input) INTEGER, LDU = > N.
* The leading dimension of arrays U, VT, DIFL, DIFR,
* POLES, GIVNUM, and Z.
*
* VT (input) DOUBLE PRECISION array, dimension ( LDU, SMLSIZ+1 ).
* On entry, VT' contains the right singular vector matrices of
* all subproblems at the bottom level.
*
* K (input) INTEGER array, dimension ( N ).
*
* DIFL (input) DOUBLE PRECISION array, dimension ( LDU, NLVL ).
* where NLVL = INT(log_2 (N/(SMLSIZ+1))) + 1.
*
* DIFR (input) DOUBLE PRECISION array, dimension ( LDU, 2 * NLVL ).
* On entry, DIFL(*, I) and DIFR(*, 2 * I -1) record
* distances between singular values on the I-th level and
* singular values on the (I -1)-th level, and DIFR(*, 2 * I)
* record the normalizing factors of the right singular vectors
* matrices of subproblems on I-th level.
*
* Z (input) DOUBLE PRECISION array, dimension ( LDU, NLVL ).
* On entry, Z(1, I) contains the components of the deflation-
* adjusted updating row vector for subproblems on the I-th
* level.
*
* POLES (input) DOUBLE PRECISION array, dimension ( LDU, 2 * NLVL ).
* On entry, POLES(*, 2 * I -1: 2 * I) contains the new and old
* singular values involved in the secular equations on the I-th
* level.
*
* GIVPTR (input) INTEGER array, dimension ( N ).
* On entry, GIVPTR( I ) records the number of Givens
* rotations performed on the I-th problem on the computation
* tree.
*
* GIVCOL (input) INTEGER array, dimension ( LDGCOL, 2 * NLVL ).
* On entry, for each I, GIVCOL(*, 2 * I - 1: 2 * I) records the
* locations of Givens rotations performed on the I-th level on
* the computation tree.
*
* LDGCOL (input) INTEGER, LDGCOL = > N.
* The leading dimension of arrays GIVCOL and PERM.
*
* PERM (input) INTEGER array, dimension ( LDGCOL, NLVL ).
* On entry, PERM(*, I) records permutations done on the I-th
* level of the computation tree.
*
* GIVNUM (input) DOUBLE PRECISION array, dimension ( LDU, 2 * NLVL ).
* On entry, GIVNUM(*, 2 *I -1 : 2 * I) records the C- and S-
* values of Givens rotations performed on the I-th level on the
* computation tree.
*
* C (input) DOUBLE PRECISION array, dimension ( N ).
* On entry, if the I-th subproblem is not square,
* C( I ) contains the C-value of a Givens rotation related to
* the right null space of the I-th subproblem.
*
* S (input) DOUBLE PRECISION array, dimension ( N ).
* On entry, if the I-th subproblem is not square,
* S( I ) contains the S-value of a Givens rotation related to
* the right null space of the I-th subproblem.
*
* WORK (workspace) DOUBLE PRECISION array.
* The dimension must be at least N.
*
* IWORK (workspace) INTEGER array.
* The dimension must be at least 3 * N
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Ren-Cang Li, Computer Science Division, University of
* California at Berkeley, USA
* Osni Marques, LBNL/NERSC, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param smlsiz
* @param n
* @param nrhs
* @param b
* @param ldb
* @param bx
* @param ldbx
* @param u
* @param ldu
* @param vt
* @param k
* @param difl
* @param difr
* @param z
* @param poles
* @param givptr
* @param givcol
* @param ldgcol
* @param perm
* @param givnum
* @param c
* @param s
* @param work
* @param iwork
* @param info
*
*/
abstract public void dlalsa(int icompq, int smlsiz, int n, int nrhs, double[] b, int ldb, double[] bx, int ldbx, double[] u, int ldu, double[] vt, int[] k, double[] difl, double[] difr, double[] z, double[] poles, int[] givptr, int[] givcol, int ldgcol, int[] perm, double[] givnum, double[] c, double[] s, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLALSA is an itermediate step in solving the least squares problem
* by computing the SVD of the coefficient matrix in compact form (The
* singular vectors are computed as products of simple orthorgonal
* matrices.).
*
* If ICOMPQ = 0, DLALSA applies the inverse of the left singular vector
* matrix of an upper bidiagonal matrix to the right hand side; and if
* ICOMPQ = 1, DLALSA applies the right singular vector matrix to the
* right hand side. The singular vector matrices were generated in
* compact form by DLALSA.
*
* Arguments
* =========
*
*
* ICOMPQ (input) INTEGER
* Specifies whether the left or the right singular vector
* matrix is involved.
* = 0: Left singular vector matrix
* = 1: Right singular vector matrix
*
* SMLSIZ (input) INTEGER
* The maximum size of the subproblems at the bottom of the
* computation tree.
*
* N (input) INTEGER
* The row and column dimensions of the upper bidiagonal matrix.
*
* NRHS (input) INTEGER
* The number of columns of B and BX. NRHS must be at least 1.
*
* B (input/output) DOUBLE PRECISION array, dimension ( LDB, NRHS )
* On input, B contains the right hand sides of the least
* squares problem in rows 1 through M.
* On output, B contains the solution X in rows 1 through N.
*
* LDB (input) INTEGER
* The leading dimension of B in the calling subprogram.
* LDB must be at least max(1,MAX( M, N ) ).
*
* BX (output) DOUBLE PRECISION array, dimension ( LDBX, NRHS )
* On exit, the result of applying the left or right singular
* vector matrix to B.
*
* LDBX (input) INTEGER
* The leading dimension of BX.
*
* U (input) DOUBLE PRECISION array, dimension ( LDU, SMLSIZ ).
* On entry, U contains the left singular vector matrices of all
* subproblems at the bottom level.
*
* LDU (input) INTEGER, LDU = > N.
* The leading dimension of arrays U, VT, DIFL, DIFR,
* POLES, GIVNUM, and Z.
*
* VT (input) DOUBLE PRECISION array, dimension ( LDU, SMLSIZ+1 ).
* On entry, VT' contains the right singular vector matrices of
* all subproblems at the bottom level.
*
* K (input) INTEGER array, dimension ( N ).
*
* DIFL (input) DOUBLE PRECISION array, dimension ( LDU, NLVL ).
* where NLVL = INT(log_2 (N/(SMLSIZ+1))) + 1.
*
* DIFR (input) DOUBLE PRECISION array, dimension ( LDU, 2 * NLVL ).
* On entry, DIFL(*, I) and DIFR(*, 2 * I -1) record
* distances between singular values on the I-th level and
* singular values on the (I -1)-th level, and DIFR(*, 2 * I)
* record the normalizing factors of the right singular vectors
* matrices of subproblems on I-th level.
*
* Z (input) DOUBLE PRECISION array, dimension ( LDU, NLVL ).
* On entry, Z(1, I) contains the components of the deflation-
* adjusted updating row vector for subproblems on the I-th
* level.
*
* POLES (input) DOUBLE PRECISION array, dimension ( LDU, 2 * NLVL ).
* On entry, POLES(*, 2 * I -1: 2 * I) contains the new and old
* singular values involved in the secular equations on the I-th
* level.
*
* GIVPTR (input) INTEGER array, dimension ( N ).
* On entry, GIVPTR( I ) records the number of Givens
* rotations performed on the I-th problem on the computation
* tree.
*
* GIVCOL (input) INTEGER array, dimension ( LDGCOL, 2 * NLVL ).
* On entry, for each I, GIVCOL(*, 2 * I - 1: 2 * I) records the
* locations of Givens rotations performed on the I-th level on
* the computation tree.
*
* LDGCOL (input) INTEGER, LDGCOL = > N.
* The leading dimension of arrays GIVCOL and PERM.
*
* PERM (input) INTEGER array, dimension ( LDGCOL, NLVL ).
* On entry, PERM(*, I) records permutations done on the I-th
* level of the computation tree.
*
* GIVNUM (input) DOUBLE PRECISION array, dimension ( LDU, 2 * NLVL ).
* On entry, GIVNUM(*, 2 *I -1 : 2 * I) records the C- and S-
* values of Givens rotations performed on the I-th level on the
* computation tree.
*
* C (input) DOUBLE PRECISION array, dimension ( N ).
* On entry, if the I-th subproblem is not square,
* C( I ) contains the C-value of a Givens rotation related to
* the right null space of the I-th subproblem.
*
* S (input) DOUBLE PRECISION array, dimension ( N ).
* On entry, if the I-th subproblem is not square,
* S( I ) contains the S-value of a Givens rotation related to
* the right null space of the I-th subproblem.
*
* WORK (workspace) DOUBLE PRECISION array.
* The dimension must be at least N.
*
* IWORK (workspace) INTEGER array.
* The dimension must be at least 3 * N
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Ren-Cang Li, Computer Science Division, University of
* California at Berkeley, USA
* Osni Marques, LBNL/NERSC, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param smlsiz
* @param n
* @param nrhs
* @param b
* @param _b_offset
* @param ldb
* @param bx
* @param _bx_offset
* @param ldbx
* @param u
* @param _u_offset
* @param ldu
* @param vt
* @param _vt_offset
* @param k
* @param _k_offset
* @param difl
* @param _difl_offset
* @param difr
* @param _difr_offset
* @param z
* @param _z_offset
* @param poles
* @param _poles_offset
* @param givptr
* @param _givptr_offset
* @param givcol
* @param _givcol_offset
* @param ldgcol
* @param perm
* @param _perm_offset
* @param givnum
* @param _givnum_offset
* @param c
* @param _c_offset
* @param s
* @param _s_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dlalsa(int icompq, int smlsiz, int n, int nrhs, double[] b, int _b_offset, int ldb, double[] bx, int _bx_offset, int ldbx, double[] u, int _u_offset, int ldu, double[] vt, int _vt_offset, int[] k, int _k_offset, double[] difl, int _difl_offset, double[] difr, int _difr_offset, double[] z, int _z_offset, double[] poles, int _poles_offset, int[] givptr, int _givptr_offset, int[] givcol, int _givcol_offset, int ldgcol, int[] perm, int _perm_offset, double[] givnum, int _givnum_offset, double[] c, int _c_offset, double[] s, int _s_offset, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLALSD uses the singular value decomposition of A to solve the least
* squares problem of finding X to minimize the Euclidean norm of each
* column of A*X-B, where A is N-by-N upper bidiagonal, and X and B
* are N-by-NRHS. The solution X overwrites B.
*
* The singular values of A smaller than RCOND times the largest
* singular value are treated as zero in solving the least squares
* problem; in this case a minimum norm solution is returned.
* The actual singular values are returned in D in ascending order.
*
* This code makes very mild assumptions about floating point
* arithmetic. It will work on machines with a guard digit in
* add/subtract, or on those binary machines without guard digits
* which subtract like the Cray XMP, Cray YMP, Cray C 90, or Cray 2.
* It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': D and E define an upper bidiagonal matrix.
* = 'L': D and E define a lower bidiagonal matrix.
*
* SMLSIZ (input) INTEGER
* The maximum size of the subproblems at the bottom of the
* computation tree.
*
* N (input) INTEGER
* The dimension of the bidiagonal matrix. N >= 0.
*
* NRHS (input) INTEGER
* The number of columns of B. NRHS must be at least 1.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry D contains the main diagonal of the bidiagonal
* matrix. On exit, if INFO = 0, D contains its singular values.
*
* E (input/output) DOUBLE PRECISION array, dimension (N-1)
* Contains the super-diagonal entries of the bidiagonal matrix.
* On exit, E has been destroyed.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On input, B contains the right hand sides of the least
* squares problem. On output, B contains the solution X.
*
* LDB (input) INTEGER
* The leading dimension of B in the calling subprogram.
* LDB must be at least max(1,N).
*
* RCOND (input) DOUBLE PRECISION
* The singular values of A less than or equal to RCOND times
* the largest singular value are treated as zero in solving
* the least squares problem. If RCOND is negative,
* machine precision is used instead.
* For example, if diag(S)*X=B were the least squares problem,
* where diag(S) is a diagonal matrix of singular values, the
* solution would be X(i) = B(i) / S(i) if S(i) is greater than
* RCOND*max(S), and X(i) = 0 if S(i) is less than or equal to
* RCOND*max(S).
*
* RANK (output) INTEGER
* The number of singular values of A greater than RCOND times
* the largest singular value.
*
* WORK (workspace) DOUBLE PRECISION array, dimension at least
* (9*N + 2*N*SMLSIZ + 8*N*NLVL + N*NRHS + (SMLSIZ+1)**2),
* where NLVL = max(0, INT(log_2 (N/(SMLSIZ+1))) + 1).
*
* IWORK (workspace) INTEGER array, dimension at least
* (3*N*NLVL + 11*N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: The algorithm failed to compute an singular value while
* working on the submatrix lying in rows and columns
* INFO/(N+1) through MOD(INFO,N+1).
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Ren-Cang Li, Computer Science Division, University of
* California at Berkeley, USA
* Osni Marques, LBNL/NERSC, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param smlsiz
* @param n
* @param nrhs
* @param d
* @param e
* @param b
* @param ldb
* @param rcond
* @param rank
* @param work
* @param iwork
* @param info
*
*/
abstract public void dlalsd(java.lang.String uplo, int smlsiz, int n, int nrhs, double[] d, double[] e, double[] b, int ldb, double rcond, org.netlib.util.intW rank, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLALSD uses the singular value decomposition of A to solve the least
* squares problem of finding X to minimize the Euclidean norm of each
* column of A*X-B, where A is N-by-N upper bidiagonal, and X and B
* are N-by-NRHS. The solution X overwrites B.
*
* The singular values of A smaller than RCOND times the largest
* singular value are treated as zero in solving the least squares
* problem; in this case a minimum norm solution is returned.
* The actual singular values are returned in D in ascending order.
*
* This code makes very mild assumptions about floating point
* arithmetic. It will work on machines with a guard digit in
* add/subtract, or on those binary machines without guard digits
* which subtract like the Cray XMP, Cray YMP, Cray C 90, or Cray 2.
* It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': D and E define an upper bidiagonal matrix.
* = 'L': D and E define a lower bidiagonal matrix.
*
* SMLSIZ (input) INTEGER
* The maximum size of the subproblems at the bottom of the
* computation tree.
*
* N (input) INTEGER
* The dimension of the bidiagonal matrix. N >= 0.
*
* NRHS (input) INTEGER
* The number of columns of B. NRHS must be at least 1.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry D contains the main diagonal of the bidiagonal
* matrix. On exit, if INFO = 0, D contains its singular values.
*
* E (input/output) DOUBLE PRECISION array, dimension (N-1)
* Contains the super-diagonal entries of the bidiagonal matrix.
* On exit, E has been destroyed.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On input, B contains the right hand sides of the least
* squares problem. On output, B contains the solution X.
*
* LDB (input) INTEGER
* The leading dimension of B in the calling subprogram.
* LDB must be at least max(1,N).
*
* RCOND (input) DOUBLE PRECISION
* The singular values of A less than or equal to RCOND times
* the largest singular value are treated as zero in solving
* the least squares problem. If RCOND is negative,
* machine precision is used instead.
* For example, if diag(S)*X=B were the least squares problem,
* where diag(S) is a diagonal matrix of singular values, the
* solution would be X(i) = B(i) / S(i) if S(i) is greater than
* RCOND*max(S), and X(i) = 0 if S(i) is less than or equal to
* RCOND*max(S).
*
* RANK (output) INTEGER
* The number of singular values of A greater than RCOND times
* the largest singular value.
*
* WORK (workspace) DOUBLE PRECISION array, dimension at least
* (9*N + 2*N*SMLSIZ + 8*N*NLVL + N*NRHS + (SMLSIZ+1)**2),
* where NLVL = max(0, INT(log_2 (N/(SMLSIZ+1))) + 1).
*
* IWORK (workspace) INTEGER array, dimension at least
* (3*N*NLVL + 11*N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: The algorithm failed to compute an singular value while
* working on the submatrix lying in rows and columns
* INFO/(N+1) through MOD(INFO,N+1).
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Ren-Cang Li, Computer Science Division, University of
* California at Berkeley, USA
* Osni Marques, LBNL/NERSC, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param smlsiz
* @param n
* @param nrhs
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param b
* @param _b_offset
* @param ldb
* @param rcond
* @param rank
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dlalsd(java.lang.String uplo, int smlsiz, int n, int nrhs, double[] d, int _d_offset, double[] e, int _e_offset, double[] b, int _b_offset, int ldb, double rcond, org.netlib.util.intW rank, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAMRG will create a permutation list which will merge the elements
* of A (which is composed of two independently sorted sets) into a
* single set which is sorted in ascending order.
*
* Arguments
* =========
*
* N1 (input) INTEGER
* N2 (input) INTEGER
* These arguements contain the respective lengths of the two
* sorted lists to be merged.
*
* A (input) DOUBLE PRECISION array, dimension (N1+N2)
* The first N1 elements of A contain a list of numbers which
* are sorted in either ascending or descending order. Likewise
* for the final N2 elements.
*
* DTRD1 (input) INTEGER
* DTRD2 (input) INTEGER
* These are the strides to be taken through the array A.
* Allowable strides are 1 and -1. They indicate whether a
* subset of A is sorted in ascending (DTRDx = 1) or descending
* (DTRDx = -1) order.
*
* INDEX (output) INTEGER array, dimension (N1+N2)
* On exit this array will contain a permutation such that
* if B( I ) = A( INDEX( I ) ) for I=1,N1+N2, then B will be
* sorted in ascending order.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n1
* @param n2
* @param a
* @param dtrd1
* @param dtrd2
* @param index
*
*/
abstract public void dlamrg(int n1, int n2, double[] a, int dtrd1, int dtrd2, int[] index);
/**
*
* ..
*
* Purpose
* =======
*
* DLAMRG will create a permutation list which will merge the elements
* of A (which is composed of two independently sorted sets) into a
* single set which is sorted in ascending order.
*
* Arguments
* =========
*
* N1 (input) INTEGER
* N2 (input) INTEGER
* These arguements contain the respective lengths of the two
* sorted lists to be merged.
*
* A (input) DOUBLE PRECISION array, dimension (N1+N2)
* The first N1 elements of A contain a list of numbers which
* are sorted in either ascending or descending order. Likewise
* for the final N2 elements.
*
* DTRD1 (input) INTEGER
* DTRD2 (input) INTEGER
* These are the strides to be taken through the array A.
* Allowable strides are 1 and -1. They indicate whether a
* subset of A is sorted in ascending (DTRDx = 1) or descending
* (DTRDx = -1) order.
*
* INDEX (output) INTEGER array, dimension (N1+N2)
* On exit this array will contain a permutation such that
* if B( I ) = A( INDEX( I ) ) for I=1,N1+N2, then B will be
* sorted in ascending order.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n1
* @param n2
* @param a
* @param _a_offset
* @param dtrd1
* @param dtrd2
* @param index
* @param _index_offset
*
*/
abstract public void dlamrg(int n1, int n2, double[] a, int _a_offset, int dtrd1, int dtrd2, int[] index, int _index_offset);
/**
*
* ..
*
* Purpose
* =======
*
* DLANEG computes the Sturm count, the number of negative pivots
* encountered while factoring tridiagonal T - sigma I = L D L^T.
* This implementation works directly on the factors without forming
* the tridiagonal matrix T. The Sturm count is also the number of
* eigenvalues of T less than sigma.
*
* This routine is called from DLARRB.
*
* The current routine does not use the PIVMIN parameter but rather
* requires IEEE-754 propagation of Infinities and NaNs. This
* routine also has no input range restrictions but does require
* default exception handling such that x/0 produces Inf when x is
* non-zero, and Inf/Inf produces NaN. For more information, see:
*
* Marques, Riedy, and Voemel, "Benefits of IEEE-754 Features in
* Modern Symmetric Tridiagonal Eigensolvers," SIAM Journal on
* Scientific Computing, v28, n5, 2006. DOI 10.1137/050641624
* (Tech report version in LAWN 172 with the same title.)
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The N diagonal elements of the diagonal matrix D.
*
* LLD (input) DOUBLE PRECISION array, dimension (N-1)
* The (N-1) elements L(i)*L(i)*D(i).
*
* SIGMA (input) DOUBLE PRECISION
* Shift amount in T - sigma I = L D L^T.
*
* PIVMIN (input) DOUBLE PRECISION
* The minimum pivot in the Sturm sequence. May be used
* when zero pivots are encountered on non-IEEE-754
* architectures.
*
* R (input) INTEGER
* The twist index for the twisted factorization that is used
* for the negcount.
*
* Further Details
* ===============
*
* Based on contributions by
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
* Jason Riedy, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param lld
* @param sigma
* @param pivmin
* @param r
* @return
*/
abstract public int dlaneg(int n, double[] d, double[] lld, double sigma, double pivmin, int r);
/**
*
* ..
*
* Purpose
* =======
*
* DLANEG computes the Sturm count, the number of negative pivots
* encountered while factoring tridiagonal T - sigma I = L D L^T.
* This implementation works directly on the factors without forming
* the tridiagonal matrix T. The Sturm count is also the number of
* eigenvalues of T less than sigma.
*
* This routine is called from DLARRB.
*
* The current routine does not use the PIVMIN parameter but rather
* requires IEEE-754 propagation of Infinities and NaNs. This
* routine also has no input range restrictions but does require
* default exception handling such that x/0 produces Inf when x is
* non-zero, and Inf/Inf produces NaN. For more information, see:
*
* Marques, Riedy, and Voemel, "Benefits of IEEE-754 Features in
* Modern Symmetric Tridiagonal Eigensolvers," SIAM Journal on
* Scientific Computing, v28, n5, 2006. DOI 10.1137/050641624
* (Tech report version in LAWN 172 with the same title.)
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The N diagonal elements of the diagonal matrix D.
*
* LLD (input) DOUBLE PRECISION array, dimension (N-1)
* The (N-1) elements L(i)*L(i)*D(i).
*
* SIGMA (input) DOUBLE PRECISION
* Shift amount in T - sigma I = L D L^T.
*
* PIVMIN (input) DOUBLE PRECISION
* The minimum pivot in the Sturm sequence. May be used
* when zero pivots are encountered on non-IEEE-754
* architectures.
*
* R (input) INTEGER
* The twist index for the twisted factorization that is used
* for the negcount.
*
* Further Details
* ===============
*
* Based on contributions by
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
* Jason Riedy, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param _d_offset
* @param lld
* @param _lld_offset
* @param sigma
* @param pivmin
* @param r
* @return
*/
abstract public int dlaneg(int n, double[] d, int _d_offset, double[] lld, int _lld_offset, double sigma, double pivmin, int r);
/**
*
* ..
*
* Purpose
* =======
*
* DLANGB returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of an
* n by n band matrix A, with kl sub-diagonals and ku super-diagonals.
*
* Description
* ===========
*
* DLANGB returns the value
*
* DLANGB = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in DLANGB as described
* above.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, DLANGB is
* set to zero.
*
* KL (input) INTEGER
* The number of sub-diagonals of the matrix A. KL >= 0.
*
* KU (input) INTEGER
* The number of super-diagonals of the matrix A. KU >= 0.
*
* AB (input) DOUBLE PRECISION array, dimension (LDAB,N)
* The band matrix A, stored in rows 1 to KL+KU+1. The j-th
* column of A is stored in the j-th column of the array AB as
* follows:
* AB(ku+1+i-j,j) = A(i,j) for max(1,j-ku)<=i<=min(n,j+kl).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KL+KU+1.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (MAX(1,LWORK)),
* where LWORK >= N when NORM = 'I'; otherwise, WORK is not
* referenced.
*
* =====================================================================
*
*
* .. Parameters ..
*
*
* @param norm
* @param n
* @param kl
* @param ku
* @param ab
* @param ldab
* @param work
* @return
*/
abstract public double dlangb(java.lang.String norm, int n, int kl, int ku, double[] ab, int ldab, double[] work);
/**
*
* ..
*
* Purpose
* =======
*
* DLANGB returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of an
* n by n band matrix A, with kl sub-diagonals and ku super-diagonals.
*
* Description
* ===========
*
* DLANGB returns the value
*
* DLANGB = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in DLANGB as described
* above.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, DLANGB is
* set to zero.
*
* KL (input) INTEGER
* The number of sub-diagonals of the matrix A. KL >= 0.
*
* KU (input) INTEGER
* The number of super-diagonals of the matrix A. KU >= 0.
*
* AB (input) DOUBLE PRECISION array, dimension (LDAB,N)
* The band matrix A, stored in rows 1 to KL+KU+1. The j-th
* column of A is stored in the j-th column of the array AB as
* follows:
* AB(ku+1+i-j,j) = A(i,j) for max(1,j-ku)<=i<=min(n,j+kl).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KL+KU+1.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (MAX(1,LWORK)),
* where LWORK >= N when NORM = 'I'; otherwise, WORK is not
* referenced.
*
* =====================================================================
*
*
* .. Parameters ..
*
*
* @param norm
* @param n
* @param kl
* @param ku
* @param ab
* @param _ab_offset
* @param ldab
* @param work
* @param _work_offset
* @return
*/
abstract public double dlangb(java.lang.String norm, int n, int kl, int ku, double[] ab, int _ab_offset, int ldab, double[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* DLANGE returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of a
* real matrix A.
*
* Description
* ===========
*
* DLANGE returns the value
*
* DLANGE = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in DLANGE as described
* above.
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0. When M = 0,
* DLANGE is set to zero.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0. When N = 0,
* DLANGE is set to zero.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The m by n matrix A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(M,1).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (MAX(1,LWORK)),
* where LWORK >= M when NORM = 'I'; otherwise, WORK is not
* referenced.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param m
* @param n
* @param a
* @param lda
* @param work
* @return
*/
abstract public double dlange(java.lang.String norm, int m, int n, double[] a, int lda, double[] work);
/**
*
* ..
*
* Purpose
* =======
*
* DLANGE returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of a
* real matrix A.
*
* Description
* ===========
*
* DLANGE returns the value
*
* DLANGE = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in DLANGE as described
* above.
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0. When M = 0,
* DLANGE is set to zero.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0. When N = 0,
* DLANGE is set to zero.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The m by n matrix A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(M,1).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (MAX(1,LWORK)),
* where LWORK >= M when NORM = 'I'; otherwise, WORK is not
* referenced.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param work
* @param _work_offset
* @return
*/
abstract public double dlange(java.lang.String norm, int m, int n, double[] a, int _a_offset, int lda, double[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* DLANGT returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of a
* real tridiagonal matrix A.
*
* Description
* ===========
*
* DLANGT returns the value
*
* DLANGT = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in DLANGT as described
* above.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, DLANGT is
* set to zero.
*
* DL (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) sub-diagonal elements of A.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The diagonal elements of A.
*
* DU (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) super-diagonal elements of A.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param n
* @param dl
* @param d
* @param du
* @return
*/
abstract public double dlangt(java.lang.String norm, int n, double[] dl, double[] d, double[] du);
/**
*
* ..
*
* Purpose
* =======
*
* DLANGT returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of a
* real tridiagonal matrix A.
*
* Description
* ===========
*
* DLANGT returns the value
*
* DLANGT = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in DLANGT as described
* above.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, DLANGT is
* set to zero.
*
* DL (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) sub-diagonal elements of A.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The diagonal elements of A.
*
* DU (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) super-diagonal elements of A.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param n
* @param dl
* @param _dl_offset
* @param d
* @param _d_offset
* @param du
* @param _du_offset
* @return
*/
abstract public double dlangt(java.lang.String norm, int n, double[] dl, int _dl_offset, double[] d, int _d_offset, double[] du, int _du_offset);
/**
*
* ..
*
* Purpose
* =======
*
* DLANHS returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of a
* Hessenberg matrix A.
*
* Description
* ===========
*
* DLANHS returns the value
*
* DLANHS = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in DLANHS as described
* above.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, DLANHS is
* set to zero.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The n by n upper Hessenberg matrix A; the part of A below the
* first sub-diagonal is not referenced.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(N,1).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (MAX(1,LWORK)),
* where LWORK >= N when NORM = 'I'; otherwise, WORK is not
* referenced.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param n
* @param a
* @param lda
* @param work
* @return
*/
abstract public double dlanhs(java.lang.String norm, int n, double[] a, int lda, double[] work);
/**
*
* ..
*
* Purpose
* =======
*
* DLANHS returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of a
* Hessenberg matrix A.
*
* Description
* ===========
*
* DLANHS returns the value
*
* DLANHS = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in DLANHS as described
* above.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, DLANHS is
* set to zero.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The n by n upper Hessenberg matrix A; the part of A below the
* first sub-diagonal is not referenced.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(N,1).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (MAX(1,LWORK)),
* where LWORK >= N when NORM = 'I'; otherwise, WORK is not
* referenced.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param n
* @param a
* @param _a_offset
* @param lda
* @param work
* @param _work_offset
* @return
*/
abstract public double dlanhs(java.lang.String norm, int n, double[] a, int _a_offset, int lda, double[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* DLANSB returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of an
* n by n symmetric band matrix A, with k super-diagonals.
*
* Description
* ===========
*
* DLANSB returns the value
*
* DLANSB = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in DLANSB as described
* above.
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* band matrix A is supplied.
* = 'U': Upper triangular part is supplied
* = 'L': Lower triangular part is supplied
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, DLANSB is
* set to zero.
*
* K (input) INTEGER
* The number of super-diagonals or sub-diagonals of the
* band matrix A. K >= 0.
*
* AB (input) DOUBLE PRECISION array, dimension (LDAB,N)
* The upper or lower triangle of the symmetric band matrix A,
* stored in the first K+1 rows of AB. The j-th column of A is
* stored in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(k+1+i-j,j) = A(i,j) for max(1,j-k)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+k).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= K+1.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (MAX(1,LWORK)),
* where LWORK >= N when NORM = 'I' or '1' or 'O'; otherwise,
* WORK is not referenced.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param n
* @param k
* @param ab
* @param ldab
* @param work
* @return
*/
abstract public double dlansb(java.lang.String norm, java.lang.String uplo, int n, int k, double[] ab, int ldab, double[] work);
/**
*
* ..
*
* Purpose
* =======
*
* DLANSB returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of an
* n by n symmetric band matrix A, with k super-diagonals.
*
* Description
* ===========
*
* DLANSB returns the value
*
* DLANSB = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in DLANSB as described
* above.
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* band matrix A is supplied.
* = 'U': Upper triangular part is supplied
* = 'L': Lower triangular part is supplied
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, DLANSB is
* set to zero.
*
* K (input) INTEGER
* The number of super-diagonals or sub-diagonals of the
* band matrix A. K >= 0.
*
* AB (input) DOUBLE PRECISION array, dimension (LDAB,N)
* The upper or lower triangle of the symmetric band matrix A,
* stored in the first K+1 rows of AB. The j-th column of A is
* stored in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(k+1+i-j,j) = A(i,j) for max(1,j-k)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+k).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= K+1.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (MAX(1,LWORK)),
* where LWORK >= N when NORM = 'I' or '1' or 'O'; otherwise,
* WORK is not referenced.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param n
* @param k
* @param ab
* @param _ab_offset
* @param ldab
* @param work
* @param _work_offset
* @return
*/
abstract public double dlansb(java.lang.String norm, java.lang.String uplo, int n, int k, double[] ab, int _ab_offset, int ldab, double[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* DLANSP returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of a
* real symmetric matrix A, supplied in packed form.
*
* Description
* ===========
*
* DLANSP returns the value
*
* DLANSP = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in DLANSP as described
* above.
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is supplied.
* = 'U': Upper triangular part of A is supplied
* = 'L': Lower triangular part of A is supplied
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, DLANSP is
* set to zero.
*
* AP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The upper or lower triangle of the symmetric matrix A, packed
* columnwise in a linear array. The j-th column of A is stored
* in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (MAX(1,LWORK)),
* where LWORK >= N when NORM = 'I' or '1' or 'O'; otherwise,
* WORK is not referenced.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param n
* @param ap
* @param work
* @return
*/
abstract public double dlansp(java.lang.String norm, java.lang.String uplo, int n, double[] ap, double[] work);
/**
*
* ..
*
* Purpose
* =======
*
* DLANSP returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of a
* real symmetric matrix A, supplied in packed form.
*
* Description
* ===========
*
* DLANSP returns the value
*
* DLANSP = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in DLANSP as described
* above.
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is supplied.
* = 'U': Upper triangular part of A is supplied
* = 'L': Lower triangular part of A is supplied
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, DLANSP is
* set to zero.
*
* AP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The upper or lower triangle of the symmetric matrix A, packed
* columnwise in a linear array. The j-th column of A is stored
* in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (MAX(1,LWORK)),
* where LWORK >= N when NORM = 'I' or '1' or 'O'; otherwise,
* WORK is not referenced.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param work
* @param _work_offset
* @return
*/
abstract public double dlansp(java.lang.String norm, java.lang.String uplo, int n, double[] ap, int _ap_offset, double[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* DLANST returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of a
* real symmetric tridiagonal matrix A.
*
* Description
* ===========
*
* DLANST returns the value
*
* DLANST = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in DLANST as described
* above.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, DLANST is
* set to zero.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The diagonal elements of A.
*
* E (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) sub-diagonal or super-diagonal elements of A.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param n
* @param d
* @param e
* @return
*/
abstract public double dlanst(java.lang.String norm, int n, double[] d, double[] e);
/**
*
* ..
*
* Purpose
* =======
*
* DLANST returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of a
* real symmetric tridiagonal matrix A.
*
* Description
* ===========
*
* DLANST returns the value
*
* DLANST = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in DLANST as described
* above.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, DLANST is
* set to zero.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The diagonal elements of A.
*
* E (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) sub-diagonal or super-diagonal elements of A.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @return
*/
abstract public double dlanst(java.lang.String norm, int n, double[] d, int _d_offset, double[] e, int _e_offset);
/**
*
* ..
*
* Purpose
* =======
*
* DLANSY returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of a
* real symmetric matrix A.
*
* Description
* ===========
*
* DLANSY returns the value
*
* DLANSY = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in DLANSY as described
* above.
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is to be referenced.
* = 'U': Upper triangular part of A is referenced
* = 'L': Lower triangular part of A is referenced
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, DLANSY is
* set to zero.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The symmetric matrix A. If UPLO = 'U', the leading n by n
* upper triangular part of A contains the upper triangular part
* of the matrix A, and the strictly lower triangular part of A
* is not referenced. If UPLO = 'L', the leading n by n lower
* triangular part of A contains the lower triangular part of
* the matrix A, and the strictly upper triangular part of A is
* not referenced.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(N,1).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (MAX(1,LWORK)),
* where LWORK >= N when NORM = 'I' or '1' or 'O'; otherwise,
* WORK is not referenced.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param n
* @param a
* @param lda
* @param work
* @return
*/
abstract public double dlansy(java.lang.String norm, java.lang.String uplo, int n, double[] a, int lda, double[] work);
/**
*
* ..
*
* Purpose
* =======
*
* DLANSY returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of a
* real symmetric matrix A.
*
* Description
* ===========
*
* DLANSY returns the value
*
* DLANSY = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in DLANSY as described
* above.
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is to be referenced.
* = 'U': Upper triangular part of A is referenced
* = 'L': Lower triangular part of A is referenced
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, DLANSY is
* set to zero.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The symmetric matrix A. If UPLO = 'U', the leading n by n
* upper triangular part of A contains the upper triangular part
* of the matrix A, and the strictly lower triangular part of A
* is not referenced. If UPLO = 'L', the leading n by n lower
* triangular part of A contains the lower triangular part of
* the matrix A, and the strictly upper triangular part of A is
* not referenced.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(N,1).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (MAX(1,LWORK)),
* where LWORK >= N when NORM = 'I' or '1' or 'O'; otherwise,
* WORK is not referenced.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param work
* @param _work_offset
* @return
*/
abstract public double dlansy(java.lang.String norm, java.lang.String uplo, int n, double[] a, int _a_offset, int lda, double[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* DLANTB returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of an
* n by n triangular band matrix A, with ( k + 1 ) diagonals.
*
* Description
* ===========
*
* DLANTB returns the value
*
* DLANTB = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in DLANTB as described
* above.
*
* UPLO (input) CHARACTER*1
* Specifies whether the matrix A is upper or lower triangular.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* DIAG (input) CHARACTER*1
* Specifies whether or not the matrix A is unit triangular.
* = 'N': Non-unit triangular
* = 'U': Unit triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, DLANTB is
* set to zero.
*
* K (input) INTEGER
* The number of super-diagonals of the matrix A if UPLO = 'U',
* or the number of sub-diagonals of the matrix A if UPLO = 'L'.
* K >= 0.
*
* AB (input) DOUBLE PRECISION array, dimension (LDAB,N)
* The upper or lower triangular band matrix A, stored in the
* first k+1 rows of AB. The j-th column of A is stored
* in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(k+1+i-j,j) = A(i,j) for max(1,j-k)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+k).
* Note that when DIAG = 'U', the elements of the array AB
* corresponding to the diagonal elements of the matrix A are
* not referenced, but are assumed to be one.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= K+1.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (MAX(1,LWORK)),
* where LWORK >= N when NORM = 'I'; otherwise, WORK is not
* referenced.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param diag
* @param n
* @param k
* @param ab
* @param ldab
* @param work
* @return
*/
abstract public double dlantb(java.lang.String norm, java.lang.String uplo, java.lang.String diag, int n, int k, double[] ab, int ldab, double[] work);
/**
*
* ..
*
* Purpose
* =======
*
* DLANTB returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of an
* n by n triangular band matrix A, with ( k + 1 ) diagonals.
*
* Description
* ===========
*
* DLANTB returns the value
*
* DLANTB = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in DLANTB as described
* above.
*
* UPLO (input) CHARACTER*1
* Specifies whether the matrix A is upper or lower triangular.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* DIAG (input) CHARACTER*1
* Specifies whether or not the matrix A is unit triangular.
* = 'N': Non-unit triangular
* = 'U': Unit triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, DLANTB is
* set to zero.
*
* K (input) INTEGER
* The number of super-diagonals of the matrix A if UPLO = 'U',
* or the number of sub-diagonals of the matrix A if UPLO = 'L'.
* K >= 0.
*
* AB (input) DOUBLE PRECISION array, dimension (LDAB,N)
* The upper or lower triangular band matrix A, stored in the
* first k+1 rows of AB. The j-th column of A is stored
* in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(k+1+i-j,j) = A(i,j) for max(1,j-k)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+k).
* Note that when DIAG = 'U', the elements of the array AB
* corresponding to the diagonal elements of the matrix A are
* not referenced, but are assumed to be one.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= K+1.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (MAX(1,LWORK)),
* where LWORK >= N when NORM = 'I'; otherwise, WORK is not
* referenced.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param diag
* @param n
* @param k
* @param ab
* @param _ab_offset
* @param ldab
* @param work
* @param _work_offset
* @return
*/
abstract public double dlantb(java.lang.String norm, java.lang.String uplo, java.lang.String diag, int n, int k, double[] ab, int _ab_offset, int ldab, double[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* DLANTP returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of a
* triangular matrix A, supplied in packed form.
*
* Description
* ===========
*
* DLANTP returns the value
*
* DLANTP = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in DLANTP as described
* above.
*
* UPLO (input) CHARACTER*1
* Specifies whether the matrix A is upper or lower triangular.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* DIAG (input) CHARACTER*1
* Specifies whether or not the matrix A is unit triangular.
* = 'N': Non-unit triangular
* = 'U': Unit triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, DLANTP is
* set to zero.
*
* AP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The upper or lower triangular matrix A, packed columnwise in
* a linear array. The j-th column of A is stored in the array
* AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
* Note that when DIAG = 'U', the elements of the array AP
* corresponding to the diagonal elements of the matrix A are
* not referenced, but are assumed to be one.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (MAX(1,LWORK)),
* where LWORK >= N when NORM = 'I'; otherwise, WORK is not
* referenced.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param diag
* @param n
* @param ap
* @param work
* @return
*/
abstract public double dlantp(java.lang.String norm, java.lang.String uplo, java.lang.String diag, int n, double[] ap, double[] work);
/**
*
* ..
*
* Purpose
* =======
*
* DLANTP returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of a
* triangular matrix A, supplied in packed form.
*
* Description
* ===========
*
* DLANTP returns the value
*
* DLANTP = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in DLANTP as described
* above.
*
* UPLO (input) CHARACTER*1
* Specifies whether the matrix A is upper or lower triangular.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* DIAG (input) CHARACTER*1
* Specifies whether or not the matrix A is unit triangular.
* = 'N': Non-unit triangular
* = 'U': Unit triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, DLANTP is
* set to zero.
*
* AP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The upper or lower triangular matrix A, packed columnwise in
* a linear array. The j-th column of A is stored in the array
* AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
* Note that when DIAG = 'U', the elements of the array AP
* corresponding to the diagonal elements of the matrix A are
* not referenced, but are assumed to be one.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (MAX(1,LWORK)),
* where LWORK >= N when NORM = 'I'; otherwise, WORK is not
* referenced.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param diag
* @param n
* @param ap
* @param _ap_offset
* @param work
* @param _work_offset
* @return
*/
abstract public double dlantp(java.lang.String norm, java.lang.String uplo, java.lang.String diag, int n, double[] ap, int _ap_offset, double[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* DLANTR returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of a
* trapezoidal or triangular matrix A.
*
* Description
* ===========
*
* DLANTR returns the value
*
* DLANTR = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in DLANTR as described
* above.
*
* UPLO (input) CHARACTER*1
* Specifies whether the matrix A is upper or lower trapezoidal.
* = 'U': Upper trapezoidal
* = 'L': Lower trapezoidal
* Note that A is triangular instead of trapezoidal if M = N.
*
* DIAG (input) CHARACTER*1
* Specifies whether or not the matrix A has unit diagonal.
* = 'N': Non-unit diagonal
* = 'U': Unit diagonal
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0, and if
* UPLO = 'U', M <= N. When M = 0, DLANTR is set to zero.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0, and if
* UPLO = 'L', N <= M. When N = 0, DLANTR is set to zero.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The trapezoidal matrix A (A is triangular if M = N).
* If UPLO = 'U', the leading m by n upper trapezoidal part of
* the array A contains the upper trapezoidal matrix, and the
* strictly lower triangular part of A is not referenced.
* If UPLO = 'L', the leading m by n lower trapezoidal part of
* the array A contains the lower trapezoidal matrix, and the
* strictly upper triangular part of A is not referenced. Note
* that when DIAG = 'U', the diagonal elements of A are not
* referenced and are assumed to be one.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(M,1).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (MAX(1,LWORK)),
* where LWORK >= M when NORM = 'I'; otherwise, WORK is not
* referenced.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param diag
* @param m
* @param n
* @param a
* @param lda
* @param work
* @return
*/
abstract public double dlantr(java.lang.String norm, java.lang.String uplo, java.lang.String diag, int m, int n, double[] a, int lda, double[] work);
/**
*
* ..
*
* Purpose
* =======
*
* DLANTR returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of a
* trapezoidal or triangular matrix A.
*
* Description
* ===========
*
* DLANTR returns the value
*
* DLANTR = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in DLANTR as described
* above.
*
* UPLO (input) CHARACTER*1
* Specifies whether the matrix A is upper or lower trapezoidal.
* = 'U': Upper trapezoidal
* = 'L': Lower trapezoidal
* Note that A is triangular instead of trapezoidal if M = N.
*
* DIAG (input) CHARACTER*1
* Specifies whether or not the matrix A has unit diagonal.
* = 'N': Non-unit diagonal
* = 'U': Unit diagonal
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0, and if
* UPLO = 'U', M <= N. When M = 0, DLANTR is set to zero.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0, and if
* UPLO = 'L', N <= M. When N = 0, DLANTR is set to zero.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The trapezoidal matrix A (A is triangular if M = N).
* If UPLO = 'U', the leading m by n upper trapezoidal part of
* the array A contains the upper trapezoidal matrix, and the
* strictly lower triangular part of A is not referenced.
* If UPLO = 'L', the leading m by n lower trapezoidal part of
* the array A contains the lower trapezoidal matrix, and the
* strictly upper triangular part of A is not referenced. Note
* that when DIAG = 'U', the diagonal elements of A are not
* referenced and are assumed to be one.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(M,1).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (MAX(1,LWORK)),
* where LWORK >= M when NORM = 'I'; otherwise, WORK is not
* referenced.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param diag
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param work
* @param _work_offset
* @return
*/
abstract public double dlantr(java.lang.String norm, java.lang.String uplo, java.lang.String diag, int m, int n, double[] a, int _a_offset, int lda, double[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* DLANV2 computes the Schur factorization of a real 2-by-2 nonsymmetric
* matrix in standard form:
*
* [ A B ] = [ CS -SN ] [ AA BB ] [ CS SN ]
* [ C D ] [ SN CS ] [ CC DD ] [-SN CS ]
*
* where either
* 1) CC = 0 so that AA and DD are real eigenvalues of the matrix, or
* 2) AA = DD and BB*CC < 0, so that AA + or - sqrt(BB*CC) are complex
* conjugate eigenvalues.
*
* Arguments
* =========
*
* A (input/output) DOUBLE PRECISION
* B (input/output) DOUBLE PRECISION
* C (input/output) DOUBLE PRECISION
* D (input/output) DOUBLE PRECISION
* On entry, the elements of the input matrix.
* On exit, they are overwritten by the elements of the
* standardised Schur form.
*
* RT1R (output) DOUBLE PRECISION
* RT1I (output) DOUBLE PRECISION
* RT2R (output) DOUBLE PRECISION
* RT2I (output) DOUBLE PRECISION
* The real and imaginary parts of the eigenvalues. If the
* eigenvalues are a complex conjugate pair, RT1I > 0.
*
* CS (output) DOUBLE PRECISION
* SN (output) DOUBLE PRECISION
* Parameters of the rotation matrix.
*
* Further Details
* ===============
*
* Modified by V. Sima, Research Institute for Informatics, Bucharest,
* Romania, to reduce the risk of cancellation errors,
* when computing real eigenvalues, and to ensure, if possible, that
* abs(RT1R) >= abs(RT2R).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param a
* @param b
* @param c
* @param d
* @param rt1r
* @param rt1i
* @param rt2r
* @param rt2i
* @param cs
* @param sn
*
*/
abstract public void dlanv2(org.netlib.util.doubleW a, org.netlib.util.doubleW b, org.netlib.util.doubleW c, org.netlib.util.doubleW d, org.netlib.util.doubleW rt1r, org.netlib.util.doubleW rt1i, org.netlib.util.doubleW rt2r, org.netlib.util.doubleW rt2i, org.netlib.util.doubleW cs, org.netlib.util.doubleW sn);
/**
*
* ..
*
* Purpose
* =======
*
* Given two column vectors X and Y, let
*
* A = ( X Y ).
*
* The subroutine first computes the QR factorization of A = Q*R,
* and then computes the SVD of the 2-by-2 upper triangular matrix R.
* The smaller singular value of R is returned in SSMIN, which is used
* as the measurement of the linear dependency of the vectors X and Y.
*
* Arguments
* =========
*
* N (input) INTEGER
* The length of the vectors X and Y.
*
* X (input/output) DOUBLE PRECISION array,
* dimension (1+(N-1)*INCX)
* On entry, X contains the N-vector X.
* On exit, X is overwritten.
*
* INCX (input) INTEGER
* The increment between successive elements of X. INCX > 0.
*
* Y (input/output) DOUBLE PRECISION array,
* dimension (1+(N-1)*INCY)
* On entry, Y contains the N-vector Y.
* On exit, Y is overwritten.
*
* INCY (input) INTEGER
* The increment between successive elements of Y. INCY > 0.
*
* SSMIN (output) DOUBLE PRECISION
* The smallest singular value of the N-by-2 matrix A = ( X Y ).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param x
* @param incx
* @param y
* @param incy
* @param ssmin
*
*/
abstract public void dlapll(int n, double[] x, int incx, double[] y, int incy, org.netlib.util.doubleW ssmin);
/**
*
* ..
*
* Purpose
* =======
*
* Given two column vectors X and Y, let
*
* A = ( X Y ).
*
* The subroutine first computes the QR factorization of A = Q*R,
* and then computes the SVD of the 2-by-2 upper triangular matrix R.
* The smaller singular value of R is returned in SSMIN, which is used
* as the measurement of the linear dependency of the vectors X and Y.
*
* Arguments
* =========
*
* N (input) INTEGER
* The length of the vectors X and Y.
*
* X (input/output) DOUBLE PRECISION array,
* dimension (1+(N-1)*INCX)
* On entry, X contains the N-vector X.
* On exit, X is overwritten.
*
* INCX (input) INTEGER
* The increment between successive elements of X. INCX > 0.
*
* Y (input/output) DOUBLE PRECISION array,
* dimension (1+(N-1)*INCY)
* On entry, Y contains the N-vector Y.
* On exit, Y is overwritten.
*
* INCY (input) INTEGER
* The increment between successive elements of Y. INCY > 0.
*
* SSMIN (output) DOUBLE PRECISION
* The smallest singular value of the N-by-2 matrix A = ( X Y ).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param x
* @param _x_offset
* @param incx
* @param y
* @param _y_offset
* @param incy
* @param ssmin
*
*/
abstract public void dlapll(int n, double[] x, int _x_offset, int incx, double[] y, int _y_offset, int incy, org.netlib.util.doubleW ssmin);
/**
*
* ..
*
* Purpose
* =======
*
* DLAPMT rearranges the columns of the M by N matrix X as specified
* by the permutation K(1),K(2),...,K(N) of the integers 1,...,N.
* If FORWRD = .TRUE., forward permutation:
*
* X(*,K(J)) is moved X(*,J) for J = 1,2,...,N.
*
* If FORWRD = .FALSE., backward permutation:
*
* X(*,J) is moved to X(*,K(J)) for J = 1,2,...,N.
*
* Arguments
* =========
*
* FORWRD (input) LOGICAL
* = .TRUE., forward permutation
* = .FALSE., backward permutation
*
* M (input) INTEGER
* The number of rows of the matrix X. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix X. N >= 0.
*
* X (input/output) DOUBLE PRECISION array, dimension (LDX,N)
* On entry, the M by N matrix X.
* On exit, X contains the permuted matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X, LDX >= MAX(1,M).
*
* K (input/output) INTEGER array, dimension (N)
* On entry, K contains the permutation vector. K is used as
* internal workspace, but reset to its original value on
* output.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param forwrd
* @param m
* @param n
* @param x
* @param ldx
* @param k
*
*/
abstract public void dlapmt(boolean forwrd, int m, int n, double[] x, int ldx, int[] k);
/**
*
* ..
*
* Purpose
* =======
*
* DLAPMT rearranges the columns of the M by N matrix X as specified
* by the permutation K(1),K(2),...,K(N) of the integers 1,...,N.
* If FORWRD = .TRUE., forward permutation:
*
* X(*,K(J)) is moved X(*,J) for J = 1,2,...,N.
*
* If FORWRD = .FALSE., backward permutation:
*
* X(*,J) is moved to X(*,K(J)) for J = 1,2,...,N.
*
* Arguments
* =========
*
* FORWRD (input) LOGICAL
* = .TRUE., forward permutation
* = .FALSE., backward permutation
*
* M (input) INTEGER
* The number of rows of the matrix X. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix X. N >= 0.
*
* X (input/output) DOUBLE PRECISION array, dimension (LDX,N)
* On entry, the M by N matrix X.
* On exit, X contains the permuted matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X, LDX >= MAX(1,M).
*
* K (input/output) INTEGER array, dimension (N)
* On entry, K contains the permutation vector. K is used as
* internal workspace, but reset to its original value on
* output.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param forwrd
* @param m
* @param n
* @param x
* @param _x_offset
* @param ldx
* @param k
* @param _k_offset
*
*/
abstract public void dlapmt(boolean forwrd, int m, int n, double[] x, int _x_offset, int ldx, int[] k, int _k_offset);
/**
*
* ..
*
* Purpose
* =======
*
* DLAPY2 returns sqrt(x**2+y**2), taking care not to cause unnecessary
* overflow.
*
* Arguments
* =========
*
* X (input) DOUBLE PRECISION
* Y (input) DOUBLE PRECISION
* X and Y specify the values x and y.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param x
* @param y
* @return
*/
abstract public double dlapy2(double x, double y);
/**
*
* ..
*
* Purpose
* =======
*
* DLAPY3 returns sqrt(x**2+y**2+z**2), taking care not to cause
* unnecessary overflow.
*
* Arguments
* =========
*
* X (input) DOUBLE PRECISION
* Y (input) DOUBLE PRECISION
* Z (input) DOUBLE PRECISION
* X, Y and Z specify the values x, y and z.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param x
* @param y
* @param z
* @return
*/
abstract public double dlapy3(double x, double y, double z);
/**
*
* ..
*
* Purpose
* =======
*
* DLAQGB equilibrates a general M by N band matrix A with KL
* subdiagonals and KU superdiagonals using the row and scaling factors
* in the vectors R and C.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB,N)
* On entry, the matrix A in band storage, in rows 1 to KL+KU+1.
* The j-th column of A is stored in the j-th column of the
* array AB as follows:
* AB(ku+1+i-j,j) = A(i,j) for max(1,j-ku)<=i<=min(m,j+kl)
*
* On exit, the equilibrated matrix, in the same storage format
* as A. See EQUED for the form of the equilibrated matrix.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDA >= KL+KU+1.
*
* R (input) DOUBLE PRECISION array, dimension (M)
* The row scale factors for A.
*
* C (input) DOUBLE PRECISION array, dimension (N)
* The column scale factors for A.
*
* ROWCND (input) DOUBLE PRECISION
* Ratio of the smallest R(i) to the largest R(i).
*
* COLCND (input) DOUBLE PRECISION
* Ratio of the smallest C(i) to the largest C(i).
*
* AMAX (input) DOUBLE PRECISION
* Absolute value of largest matrix entry.
*
* EQUED (output) CHARACTER*1
* Specifies the form of equilibration that was done.
* = 'N': No equilibration
* = 'R': Row equilibration, i.e., A has been premultiplied by
* diag(R).
* = 'C': Column equilibration, i.e., A has been postmultiplied
* by diag(C).
* = 'B': Both row and column equilibration, i.e., A has been
* replaced by diag(R) * A * diag(C).
*
* Internal Parameters
* ===================
*
* THRESH is a threshold value used to decide if row or column scaling
* should be done based on the ratio of the row or column scaling
* factors. If ROWCND < THRESH, row scaling is done, and if
* COLCND < THRESH, column scaling is done.
*
* LARGE and SMALL are threshold values used to decide if row scaling
* should be done based on the absolute size of the largest matrix
* element. If AMAX > LARGE or AMAX < SMALL, row scaling is done.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param kl
* @param ku
* @param ab
* @param ldab
* @param r
* @param c
* @param rowcnd
* @param colcnd
* @param amax
* @param equed
*
*/
abstract public void dlaqgb(int m, int n, int kl, int ku, double[] ab, int ldab, double[] r, double[] c, double rowcnd, double colcnd, double amax, org.netlib.util.StringW equed);
/**
*
* ..
*
* Purpose
* =======
*
* DLAQGB equilibrates a general M by N band matrix A with KL
* subdiagonals and KU superdiagonals using the row and scaling factors
* in the vectors R and C.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB,N)
* On entry, the matrix A in band storage, in rows 1 to KL+KU+1.
* The j-th column of A is stored in the j-th column of the
* array AB as follows:
* AB(ku+1+i-j,j) = A(i,j) for max(1,j-ku)<=i<=min(m,j+kl)
*
* On exit, the equilibrated matrix, in the same storage format
* as A. See EQUED for the form of the equilibrated matrix.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDA >= KL+KU+1.
*
* R (input) DOUBLE PRECISION array, dimension (M)
* The row scale factors for A.
*
* C (input) DOUBLE PRECISION array, dimension (N)
* The column scale factors for A.
*
* ROWCND (input) DOUBLE PRECISION
* Ratio of the smallest R(i) to the largest R(i).
*
* COLCND (input) DOUBLE PRECISION
* Ratio of the smallest C(i) to the largest C(i).
*
* AMAX (input) DOUBLE PRECISION
* Absolute value of largest matrix entry.
*
* EQUED (output) CHARACTER*1
* Specifies the form of equilibration that was done.
* = 'N': No equilibration
* = 'R': Row equilibration, i.e., A has been premultiplied by
* diag(R).
* = 'C': Column equilibration, i.e., A has been postmultiplied
* by diag(C).
* = 'B': Both row and column equilibration, i.e., A has been
* replaced by diag(R) * A * diag(C).
*
* Internal Parameters
* ===================
*
* THRESH is a threshold value used to decide if row or column scaling
* should be done based on the ratio of the row or column scaling
* factors. If ROWCND < THRESH, row scaling is done, and if
* COLCND < THRESH, column scaling is done.
*
* LARGE and SMALL are threshold values used to decide if row scaling
* should be done based on the absolute size of the largest matrix
* element. If AMAX > LARGE or AMAX < SMALL, row scaling is done.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param kl
* @param ku
* @param ab
* @param _ab_offset
* @param ldab
* @param r
* @param _r_offset
* @param c
* @param _c_offset
* @param rowcnd
* @param colcnd
* @param amax
* @param equed
*
*/
abstract public void dlaqgb(int m, int n, int kl, int ku, double[] ab, int _ab_offset, int ldab, double[] r, int _r_offset, double[] c, int _c_offset, double rowcnd, double colcnd, double amax, org.netlib.util.StringW equed);
/**
*
* ..
*
* Purpose
* =======
*
* DLAQGE equilibrates a general M by N matrix A using the row and
* column scaling factors in the vectors R and C.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M by N matrix A.
* On exit, the equilibrated matrix. See EQUED for the form of
* the equilibrated matrix.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(M,1).
*
* R (input) DOUBLE PRECISION array, dimension (M)
* The row scale factors for A.
*
* C (input) DOUBLE PRECISION array, dimension (N)
* The column scale factors for A.
*
* ROWCND (input) DOUBLE PRECISION
* Ratio of the smallest R(i) to the largest R(i).
*
* COLCND (input) DOUBLE PRECISION
* Ratio of the smallest C(i) to the largest C(i).
*
* AMAX (input) DOUBLE PRECISION
* Absolute value of largest matrix entry.
*
* EQUED (output) CHARACTER*1
* Specifies the form of equilibration that was done.
* = 'N': No equilibration
* = 'R': Row equilibration, i.e., A has been premultiplied by
* diag(R).
* = 'C': Column equilibration, i.e., A has been postmultiplied
* by diag(C).
* = 'B': Both row and column equilibration, i.e., A has been
* replaced by diag(R) * A * diag(C).
*
* Internal Parameters
* ===================
*
* THRESH is a threshold value used to decide if row or column scaling
* should be done based on the ratio of the row or column scaling
* factors. If ROWCND < THRESH, row scaling is done, and if
* COLCND < THRESH, column scaling is done.
*
* LARGE and SMALL are threshold values used to decide if row scaling
* should be done based on the absolute size of the largest matrix
* element. If AMAX > LARGE or AMAX < SMALL, row scaling is done.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param r
* @param c
* @param rowcnd
* @param colcnd
* @param amax
* @param equed
*
*/
abstract public void dlaqge(int m, int n, double[] a, int lda, double[] r, double[] c, double rowcnd, double colcnd, double amax, org.netlib.util.StringW equed);
/**
*
* ..
*
* Purpose
* =======
*
* DLAQGE equilibrates a general M by N matrix A using the row and
* column scaling factors in the vectors R and C.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M by N matrix A.
* On exit, the equilibrated matrix. See EQUED for the form of
* the equilibrated matrix.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(M,1).
*
* R (input) DOUBLE PRECISION array, dimension (M)
* The row scale factors for A.
*
* C (input) DOUBLE PRECISION array, dimension (N)
* The column scale factors for A.
*
* ROWCND (input) DOUBLE PRECISION
* Ratio of the smallest R(i) to the largest R(i).
*
* COLCND (input) DOUBLE PRECISION
* Ratio of the smallest C(i) to the largest C(i).
*
* AMAX (input) DOUBLE PRECISION
* Absolute value of largest matrix entry.
*
* EQUED (output) CHARACTER*1
* Specifies the form of equilibration that was done.
* = 'N': No equilibration
* = 'R': Row equilibration, i.e., A has been premultiplied by
* diag(R).
* = 'C': Column equilibration, i.e., A has been postmultiplied
* by diag(C).
* = 'B': Both row and column equilibration, i.e., A has been
* replaced by diag(R) * A * diag(C).
*
* Internal Parameters
* ===================
*
* THRESH is a threshold value used to decide if row or column scaling
* should be done based on the ratio of the row or column scaling
* factors. If ROWCND < THRESH, row scaling is done, and if
* COLCND < THRESH, column scaling is done.
*
* LARGE and SMALL are threshold values used to decide if row scaling
* should be done based on the absolute size of the largest matrix
* element. If AMAX > LARGE or AMAX < SMALL, row scaling is done.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param r
* @param _r_offset
* @param c
* @param _c_offset
* @param rowcnd
* @param colcnd
* @param amax
* @param equed
*
*/
abstract public void dlaqge(int m, int n, double[] a, int _a_offset, int lda, double[] r, int _r_offset, double[] c, int _c_offset, double rowcnd, double colcnd, double amax, org.netlib.util.StringW equed);
/**
*
* ..
*
* Purpose
* =======
*
* DLAQP2 computes a QR factorization with column pivoting of
* the block A(OFFSET+1:M,1:N).
* The block A(1:OFFSET,1:N) is accordingly pivoted, but not factorized.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* OFFSET (input) INTEGER
* The number of rows of the matrix A that must be pivoted
* but no factorized. OFFSET >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, the upper triangle of block A(OFFSET+1:M,1:N) is
* the triangular factor obtained; the elements in block
* A(OFFSET+1:M,1:N) below the diagonal, together with the
* array TAU, represent the orthogonal matrix Q as a product of
* elementary reflectors. Block A(1:OFFSET,1:N) has been
* accordingly pivoted, but no factorized.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* JPVT (input/output) INTEGER array, dimension (N)
* On entry, if JPVT(i) .ne. 0, the i-th column of A is permuted
* to the front of A*P (a leading column); if JPVT(i) = 0,
* the i-th column of A is a free column.
* On exit, if JPVT(i) = k, then the i-th column of A*P
* was the k-th column of A.
*
* TAU (output) DOUBLE PRECISION array, dimension (min(M,N))
* The scalar factors of the elementary reflectors.
*
* VN1 (input/output) DOUBLE PRECISION array, dimension (N)
* The vector with the partial column norms.
*
* VN2 (input/output) DOUBLE PRECISION array, dimension (N)
* The vector with the exact column norms.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (N)
*
* Further Details
* ===============
*
* Based on contributions by
* G. Quintana-Orti, Depto. de Informatica, Universidad Jaime I, Spain
* X. Sun, Computer Science Dept., Duke University, USA
*
* Partial column norm updating strategy modified by
* Z. Drmac and Z. Bujanovic, Dept. of Mathematics,
* University of Zagreb, Croatia.
* June 2006.
* For more details see LAPACK Working Note 176.
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param offset
* @param a
* @param lda
* @param jpvt
* @param tau
* @param vn1
* @param vn2
* @param work
*
*/
abstract public void dlaqp2(int m, int n, int offset, double[] a, int lda, int[] jpvt, double[] tau, double[] vn1, double[] vn2, double[] work);
/**
*
* ..
*
* Purpose
* =======
*
* DLAQP2 computes a QR factorization with column pivoting of
* the block A(OFFSET+1:M,1:N).
* The block A(1:OFFSET,1:N) is accordingly pivoted, but not factorized.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* OFFSET (input) INTEGER
* The number of rows of the matrix A that must be pivoted
* but no factorized. OFFSET >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, the upper triangle of block A(OFFSET+1:M,1:N) is
* the triangular factor obtained; the elements in block
* A(OFFSET+1:M,1:N) below the diagonal, together with the
* array TAU, represent the orthogonal matrix Q as a product of
* elementary reflectors. Block A(1:OFFSET,1:N) has been
* accordingly pivoted, but no factorized.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* JPVT (input/output) INTEGER array, dimension (N)
* On entry, if JPVT(i) .ne. 0, the i-th column of A is permuted
* to the front of A*P (a leading column); if JPVT(i) = 0,
* the i-th column of A is a free column.
* On exit, if JPVT(i) = k, then the i-th column of A*P
* was the k-th column of A.
*
* TAU (output) DOUBLE PRECISION array, dimension (min(M,N))
* The scalar factors of the elementary reflectors.
*
* VN1 (input/output) DOUBLE PRECISION array, dimension (N)
* The vector with the partial column norms.
*
* VN2 (input/output) DOUBLE PRECISION array, dimension (N)
* The vector with the exact column norms.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (N)
*
* Further Details
* ===============
*
* Based on contributions by
* G. Quintana-Orti, Depto. de Informatica, Universidad Jaime I, Spain
* X. Sun, Computer Science Dept., Duke University, USA
*
* Partial column norm updating strategy modified by
* Z. Drmac and Z. Bujanovic, Dept. of Mathematics,
* University of Zagreb, Croatia.
* June 2006.
* For more details see LAPACK Working Note 176.
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param offset
* @param a
* @param _a_offset
* @param lda
* @param jpvt
* @param _jpvt_offset
* @param tau
* @param _tau_offset
* @param vn1
* @param _vn1_offset
* @param vn2
* @param _vn2_offset
* @param work
* @param _work_offset
*
*/
abstract public void dlaqp2(int m, int n, int offset, double[] a, int _a_offset, int lda, int[] jpvt, int _jpvt_offset, double[] tau, int _tau_offset, double[] vn1, int _vn1_offset, double[] vn2, int _vn2_offset, double[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* DLAQPS computes a step of QR factorization with column pivoting
* of a real M-by-N matrix A by using Blas-3. It tries to factorize
* NB columns from A starting from the row OFFSET+1, and updates all
* of the matrix with Blas-3 xGEMM.
*
* In some cases, due to catastrophic cancellations, it cannot
* factorize NB columns. Hence, the actual number of factorized
* columns is returned in KB.
*
* Block A(1:OFFSET,1:N) is accordingly pivoted, but not factorized.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0
*
* OFFSET (input) INTEGER
* The number of rows of A that have been factorized in
* previous steps.
*
* NB (input) INTEGER
* The number of columns to factorize.
*
* KB (output) INTEGER
* The number of columns actually factorized.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, block A(OFFSET+1:M,1:KB) is the triangular
* factor obtained and block A(1:OFFSET,1:N) has been
* accordingly pivoted, but no factorized.
* The rest of the matrix, block A(OFFSET+1:M,KB+1:N) has
* been updated.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* JPVT (input/output) INTEGER array, dimension (N)
* JPVT(I) = K <==> Column K of the full matrix A has been
* permuted into position I in AP.
*
* TAU (output) DOUBLE PRECISION array, dimension (KB)
* The scalar factors of the elementary reflectors.
*
* VN1 (input/output) DOUBLE PRECISION array, dimension (N)
* The vector with the partial column norms.
*
* VN2 (input/output) DOUBLE PRECISION array, dimension (N)
* The vector with the exact column norms.
*
* AUXV (input/output) DOUBLE PRECISION array, dimension (NB)
* Auxiliar vector.
*
* F (input/output) DOUBLE PRECISION array, dimension (LDF,NB)
* Matrix F' = L*Y'*A.
*
* LDF (input) INTEGER
* The leading dimension of the array F. LDF >= max(1,N).
*
* Further Details
* ===============
*
* Based on contributions by
* G. Quintana-Orti, Depto. de Informatica, Universidad Jaime I, Spain
* X. Sun, Computer Science Dept., Duke University, USA
*
* Partial column norm updating strategy modified by
* Z. Drmac and Z. Bujanovic, Dept. of Mathematics,
* University of Zagreb, Croatia.
* June 2006.
* For more details see LAPACK Working Note 176.
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param offset
* @param nb
* @param kb
* @param a
* @param lda
* @param jpvt
* @param tau
* @param vn1
* @param vn2
* @param auxv
* @param f
* @param ldf
*
*/
abstract public void dlaqps(int m, int n, int offset, int nb, org.netlib.util.intW kb, double[] a, int lda, int[] jpvt, double[] tau, double[] vn1, double[] vn2, double[] auxv, double[] f, int ldf);
/**
*
* ..
*
* Purpose
* =======
*
* DLAQPS computes a step of QR factorization with column pivoting
* of a real M-by-N matrix A by using Blas-3. It tries to factorize
* NB columns from A starting from the row OFFSET+1, and updates all
* of the matrix with Blas-3 xGEMM.
*
* In some cases, due to catastrophic cancellations, it cannot
* factorize NB columns. Hence, the actual number of factorized
* columns is returned in KB.
*
* Block A(1:OFFSET,1:N) is accordingly pivoted, but not factorized.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0
*
* OFFSET (input) INTEGER
* The number of rows of A that have been factorized in
* previous steps.
*
* NB (input) INTEGER
* The number of columns to factorize.
*
* KB (output) INTEGER
* The number of columns actually factorized.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, block A(OFFSET+1:M,1:KB) is the triangular
* factor obtained and block A(1:OFFSET,1:N) has been
* accordingly pivoted, but no factorized.
* The rest of the matrix, block A(OFFSET+1:M,KB+1:N) has
* been updated.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* JPVT (input/output) INTEGER array, dimension (N)
* JPVT(I) = K <==> Column K of the full matrix A has been
* permuted into position I in AP.
*
* TAU (output) DOUBLE PRECISION array, dimension (KB)
* The scalar factors of the elementary reflectors.
*
* VN1 (input/output) DOUBLE PRECISION array, dimension (N)
* The vector with the partial column norms.
*
* VN2 (input/output) DOUBLE PRECISION array, dimension (N)
* The vector with the exact column norms.
*
* AUXV (input/output) DOUBLE PRECISION array, dimension (NB)
* Auxiliar vector.
*
* F (input/output) DOUBLE PRECISION array, dimension (LDF,NB)
* Matrix F' = L*Y'*A.
*
* LDF (input) INTEGER
* The leading dimension of the array F. LDF >= max(1,N).
*
* Further Details
* ===============
*
* Based on contributions by
* G. Quintana-Orti, Depto. de Informatica, Universidad Jaime I, Spain
* X. Sun, Computer Science Dept., Duke University, USA
*
* Partial column norm updating strategy modified by
* Z. Drmac and Z. Bujanovic, Dept. of Mathematics,
* University of Zagreb, Croatia.
* June 2006.
* For more details see LAPACK Working Note 176.
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param offset
* @param nb
* @param kb
* @param a
* @param _a_offset
* @param lda
* @param jpvt
* @param _jpvt_offset
* @param tau
* @param _tau_offset
* @param vn1
* @param _vn1_offset
* @param vn2
* @param _vn2_offset
* @param auxv
* @param _auxv_offset
* @param f
* @param _f_offset
* @param ldf
*
*/
abstract public void dlaqps(int m, int n, int offset, int nb, org.netlib.util.intW kb, double[] a, int _a_offset, int lda, int[] jpvt, int _jpvt_offset, double[] tau, int _tau_offset, double[] vn1, int _vn1_offset, double[] vn2, int _vn2_offset, double[] auxv, int _auxv_offset, double[] f, int _f_offset, int ldf);
/**
*
* ..
*
* Purpose
* =======
*
* DLAQR0 computes the eigenvalues of a Hessenberg matrix H
* and, optionally, the matrices T and Z from the Schur decomposition
* H = Z T Z**T, where T is an upper quasi-triangular matrix (the
* Schur form), and Z is the orthogonal matrix of Schur vectors.
*
* Optionally Z may be postmultiplied into an input orthogonal
* matrix Q so that this routine can give the Schur factorization
* of a matrix A which has been reduced to the Hessenberg form H
* by the orthogonal matrix Q: A = Q*H*Q**T = (QZ)*T*(QZ)**T.
*
* Arguments
* =========
*
* WANTT (input) LOGICAL
* = .TRUE. : the full Schur form T is required;
* = .FALSE.: only eigenvalues are required.
*
* WANTZ (input) LOGICAL
* = .TRUE. : the matrix of Schur vectors Z is required;
* = .FALSE.: Schur vectors are not required.
*
* N (input) INTEGER
* The order of the matrix H. N .GE. 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* It is assumed that H is already upper triangular in rows
* and columns 1:ILO-1 and IHI+1:N and, if ILO.GT.1,
* H(ILO,ILO-1) is zero. ILO and IHI are normally set by a
* previous call to DGEBAL, and then passed to DGEHRD when the
* matrix output by DGEBAL is reduced to Hessenberg form.
* Otherwise, ILO and IHI should be set to 1 and N,
* respectively. If N.GT.0, then 1.LE.ILO.LE.IHI.LE.N.
* If N = 0, then ILO = 1 and IHI = 0.
*
* H (input/output) DOUBLE PRECISION array, dimension (LDH,N)
* On entry, the upper Hessenberg matrix H.
* On exit, if INFO = 0 and WANTT is .TRUE., then H contains
* the upper quasi-triangular matrix T from the Schur
* decomposition (the Schur form); 2-by-2 diagonal blocks
* (corresponding to complex conjugate pairs of eigenvalues)
* are returned in standard form, with H(i,i) = H(i+1,i+1)
* and H(i+1,i)*H(i,i+1).LT.0. If INFO = 0 and WANTT is
* .FALSE., then the contents of H are unspecified on exit.
* (The output value of H when INFO.GT.0 is given under the
* description of INFO below.)
*
* This subroutine may explicitly set H(i,j) = 0 for i.GT.j and
* j = 1, 2, ... ILO-1 or j = IHI+1, IHI+2, ... N.
*
* LDH (input) INTEGER
* The leading dimension of the array H. LDH .GE. max(1,N).
*
* WR (output) DOUBLE PRECISION array, dimension (IHI)
* WI (output) DOUBLE PRECISION array, dimension (IHI)
* The real and imaginary parts, respectively, of the computed
* eigenvalues of H(ILO:IHI,ILO:IHI) are stored WR(ILO:IHI)
* and WI(ILO:IHI). If two eigenvalues are computed as a
* complex conjugate pair, they are stored in consecutive
* elements of WR and WI, say the i-th and (i+1)th, with
* WI(i) .GT. 0 and WI(i+1) .LT. 0. If WANTT is .TRUE., then
* the eigenvalues are stored in the same order as on the
* diagonal of the Schur form returned in H, with
* WR(i) = H(i,i) and, if H(i:i+1,i:i+1) is a 2-by-2 diagonal
* block, WI(i) = sqrt(-H(i+1,i)*H(i,i+1)) and
* WI(i+1) = -WI(i).
*
* ILOZ (input) INTEGER
* IHIZ (input) INTEGER
* Specify the rows of Z to which transformations must be
* applied if WANTZ is .TRUE..
* 1 .LE. ILOZ .LE. ILO; IHI .LE. IHIZ .LE. N.
*
* Z (input/output) DOUBLE PRECISION array, dimension (LDZ,IHI)
* If WANTZ is .FALSE., then Z is not referenced.
* If WANTZ is .TRUE., then Z(ILO:IHI,ILOZ:IHIZ) is
* replaced by Z(ILO:IHI,ILOZ:IHIZ)*U where U is the
* orthogonal Schur factor of H(ILO:IHI,ILO:IHI).
* (The output value of Z when INFO.GT.0 is given under
* the description of INFO below.)
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. if WANTZ is .TRUE.
* then LDZ.GE.MAX(1,IHIZ). Otherwize, LDZ.GE.1.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension LWORK
* On exit, if LWORK = -1, WORK(1) returns an estimate of
* the optimal value for LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK .GE. max(1,N)
* is sufficient, but LWORK typically as large as 6*N may
* be required for optimal performance. A workspace query
* to determine the optimal workspace size is recommended.
*
* If LWORK = -1, then DLAQR0 does a workspace query.
* In this case, DLAQR0 checks the input parameters and
* estimates the optimal workspace size for the given
* values of N, ILO and IHI. The estimate is returned
* in WORK(1). No error message related to LWORK is
* issued by XERBLA. Neither H nor Z are accessed.
*
*
* INFO (output) INTEGER
* = 0: successful exit
* .GT. 0: if INFO = i, DLAQR0 failed to compute all of
* the eigenvalues. Elements 1:ilo-1 and i+1:n of WR
* and WI contain those eigenvalues which have been
* successfully computed. (Failures are rare.)
*
* If INFO .GT. 0 and WANT is .FALSE., then on exit,
* the remaining unconverged eigenvalues are the eigen-
* values of the upper Hessenberg matrix rows and
* columns ILO through INFO of the final, output
* value of H.
*
* If INFO .GT. 0 and WANTT is .TRUE., then on exit
*
* (*) (initial value of H)*U = U*(final value of H)
*
* where U is an orthogonal matrix. The final
* value of H is upper Hessenberg and quasi-triangular
* in rows and columns INFO+1 through IHI.
*
* If INFO .GT. 0 and WANTZ is .TRUE., then on exit
*
* (final value of Z(ILO:IHI,ILOZ:IHIZ)
* = (initial value of Z(ILO:IHI,ILOZ:IHIZ)*U
*
* where U is the orthogonal matrix in (*) (regard-
* less of the value of WANTT.)
*
* If INFO .GT. 0 and WANTZ is .FALSE., then Z is not
* accessed.
*
*
* ================================================================
* Based on contributions by
* Karen Braman and Ralph Byers, Department of Mathematics,
* University of Kansas, USA
*
* ================================================================
*
* References:
* K. Braman, R. Byers and R. Mathias, The Multi-Shift QR
* Algorithm Part I: Maintaining Well Focused Shifts, and Level 3
* Performance, SIAM Journal of Matrix Analysis, volume 23, pages
* 929--947, 2002.
*
* K. Braman, R. Byers and R. Mathias, The Multi-Shift QR
* Algorithm Part II: Aggressive Early Deflation, SIAM Journal
* of Matrix Analysis, volume 23, pages 948--973, 2002.
*
* ================================================================
* .. Parameters ..
*
* ==== Matrices of order NTINY or smaller must be processed by
* . DLAHQR because of insufficient subdiagonal scratch space.
* . (This is a hard limit.) ====
*
* ==== Exceptional deflation windows: try to cure rare
* . slow convergence by increasing the size of the
* . deflation window after KEXNW iterations. =====
*
* ==== Exceptional shifts: try to cure rare slow convergence
* . with ad-hoc exceptional shifts every KEXSH iterations.
* . The constants WILK1 and WILK2 are used to form the
* . exceptional shifts. ====
*
*
*
* @param wantt
* @param wantz
* @param n
* @param ilo
* @param ihi
* @param h
* @param ldh
* @param wr
* @param wi
* @param iloz
* @param ihiz
* @param z
* @param ldz
* @param work
* @param lwork
* @param info
*
*/
abstract public void dlaqr0(boolean wantt, boolean wantz, int n, int ilo, int ihi, double[] h, int ldh, double[] wr, double[] wi, int iloz, int ihiz, double[] z, int ldz, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAQR0 computes the eigenvalues of a Hessenberg matrix H
* and, optionally, the matrices T and Z from the Schur decomposition
* H = Z T Z**T, where T is an upper quasi-triangular matrix (the
* Schur form), and Z is the orthogonal matrix of Schur vectors.
*
* Optionally Z may be postmultiplied into an input orthogonal
* matrix Q so that this routine can give the Schur factorization
* of a matrix A which has been reduced to the Hessenberg form H
* by the orthogonal matrix Q: A = Q*H*Q**T = (QZ)*T*(QZ)**T.
*
* Arguments
* =========
*
* WANTT (input) LOGICAL
* = .TRUE. : the full Schur form T is required;
* = .FALSE.: only eigenvalues are required.
*
* WANTZ (input) LOGICAL
* = .TRUE. : the matrix of Schur vectors Z is required;
* = .FALSE.: Schur vectors are not required.
*
* N (input) INTEGER
* The order of the matrix H. N .GE. 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* It is assumed that H is already upper triangular in rows
* and columns 1:ILO-1 and IHI+1:N and, if ILO.GT.1,
* H(ILO,ILO-1) is zero. ILO and IHI are normally set by a
* previous call to DGEBAL, and then passed to DGEHRD when the
* matrix output by DGEBAL is reduced to Hessenberg form.
* Otherwise, ILO and IHI should be set to 1 and N,
* respectively. If N.GT.0, then 1.LE.ILO.LE.IHI.LE.N.
* If N = 0, then ILO = 1 and IHI = 0.
*
* H (input/output) DOUBLE PRECISION array, dimension (LDH,N)
* On entry, the upper Hessenberg matrix H.
* On exit, if INFO = 0 and WANTT is .TRUE., then H contains
* the upper quasi-triangular matrix T from the Schur
* decomposition (the Schur form); 2-by-2 diagonal blocks
* (corresponding to complex conjugate pairs of eigenvalues)
* are returned in standard form, with H(i,i) = H(i+1,i+1)
* and H(i+1,i)*H(i,i+1).LT.0. If INFO = 0 and WANTT is
* .FALSE., then the contents of H are unspecified on exit.
* (The output value of H when INFO.GT.0 is given under the
* description of INFO below.)
*
* This subroutine may explicitly set H(i,j) = 0 for i.GT.j and
* j = 1, 2, ... ILO-1 or j = IHI+1, IHI+2, ... N.
*
* LDH (input) INTEGER
* The leading dimension of the array H. LDH .GE. max(1,N).
*
* WR (output) DOUBLE PRECISION array, dimension (IHI)
* WI (output) DOUBLE PRECISION array, dimension (IHI)
* The real and imaginary parts, respectively, of the computed
* eigenvalues of H(ILO:IHI,ILO:IHI) are stored WR(ILO:IHI)
* and WI(ILO:IHI). If two eigenvalues are computed as a
* complex conjugate pair, they are stored in consecutive
* elements of WR and WI, say the i-th and (i+1)th, with
* WI(i) .GT. 0 and WI(i+1) .LT. 0. If WANTT is .TRUE., then
* the eigenvalues are stored in the same order as on the
* diagonal of the Schur form returned in H, with
* WR(i) = H(i,i) and, if H(i:i+1,i:i+1) is a 2-by-2 diagonal
* block, WI(i) = sqrt(-H(i+1,i)*H(i,i+1)) and
* WI(i+1) = -WI(i).
*
* ILOZ (input) INTEGER
* IHIZ (input) INTEGER
* Specify the rows of Z to which transformations must be
* applied if WANTZ is .TRUE..
* 1 .LE. ILOZ .LE. ILO; IHI .LE. IHIZ .LE. N.
*
* Z (input/output) DOUBLE PRECISION array, dimension (LDZ,IHI)
* If WANTZ is .FALSE., then Z is not referenced.
* If WANTZ is .TRUE., then Z(ILO:IHI,ILOZ:IHIZ) is
* replaced by Z(ILO:IHI,ILOZ:IHIZ)*U where U is the
* orthogonal Schur factor of H(ILO:IHI,ILO:IHI).
* (The output value of Z when INFO.GT.0 is given under
* the description of INFO below.)
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. if WANTZ is .TRUE.
* then LDZ.GE.MAX(1,IHIZ). Otherwize, LDZ.GE.1.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension LWORK
* On exit, if LWORK = -1, WORK(1) returns an estimate of
* the optimal value for LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK .GE. max(1,N)
* is sufficient, but LWORK typically as large as 6*N may
* be required for optimal performance. A workspace query
* to determine the optimal workspace size is recommended.
*
* If LWORK = -1, then DLAQR0 does a workspace query.
* In this case, DLAQR0 checks the input parameters and
* estimates the optimal workspace size for the given
* values of N, ILO and IHI. The estimate is returned
* in WORK(1). No error message related to LWORK is
* issued by XERBLA. Neither H nor Z are accessed.
*
*
* INFO (output) INTEGER
* = 0: successful exit
* .GT. 0: if INFO = i, DLAQR0 failed to compute all of
* the eigenvalues. Elements 1:ilo-1 and i+1:n of WR
* and WI contain those eigenvalues which have been
* successfully computed. (Failures are rare.)
*
* If INFO .GT. 0 and WANT is .FALSE., then on exit,
* the remaining unconverged eigenvalues are the eigen-
* values of the upper Hessenberg matrix rows and
* columns ILO through INFO of the final, output
* value of H.
*
* If INFO .GT. 0 and WANTT is .TRUE., then on exit
*
* (*) (initial value of H)*U = U*(final value of H)
*
* where U is an orthogonal matrix. The final
* value of H is upper Hessenberg and quasi-triangular
* in rows and columns INFO+1 through IHI.
*
* If INFO .GT. 0 and WANTZ is .TRUE., then on exit
*
* (final value of Z(ILO:IHI,ILOZ:IHIZ)
* = (initial value of Z(ILO:IHI,ILOZ:IHIZ)*U
*
* where U is the orthogonal matrix in (*) (regard-
* less of the value of WANTT.)
*
* If INFO .GT. 0 and WANTZ is .FALSE., then Z is not
* accessed.
*
*
* ================================================================
* Based on contributions by
* Karen Braman and Ralph Byers, Department of Mathematics,
* University of Kansas, USA
*
* ================================================================
*
* References:
* K. Braman, R. Byers and R. Mathias, The Multi-Shift QR
* Algorithm Part I: Maintaining Well Focused Shifts, and Level 3
* Performance, SIAM Journal of Matrix Analysis, volume 23, pages
* 929--947, 2002.
*
* K. Braman, R. Byers and R. Mathias, The Multi-Shift QR
* Algorithm Part II: Aggressive Early Deflation, SIAM Journal
* of Matrix Analysis, volume 23, pages 948--973, 2002.
*
* ================================================================
* .. Parameters ..
*
* ==== Matrices of order NTINY or smaller must be processed by
* . DLAHQR because of insufficient subdiagonal scratch space.
* . (This is a hard limit.) ====
*
* ==== Exceptional deflation windows: try to cure rare
* . slow convergence by increasing the size of the
* . deflation window after KEXNW iterations. =====
*
* ==== Exceptional shifts: try to cure rare slow convergence
* . with ad-hoc exceptional shifts every KEXSH iterations.
* . The constants WILK1 and WILK2 are used to form the
* . exceptional shifts. ====
*
*
*
* @param wantt
* @param wantz
* @param n
* @param ilo
* @param ihi
* @param h
* @param _h_offset
* @param ldh
* @param wr
* @param _wr_offset
* @param wi
* @param _wi_offset
* @param iloz
* @param ihiz
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dlaqr0(boolean wantt, boolean wantz, int n, int ilo, int ihi, double[] h, int _h_offset, int ldh, double[] wr, int _wr_offset, double[] wi, int _wi_offset, int iloz, int ihiz, double[] z, int _z_offset, int ldz, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Given a 2-by-2 or 3-by-3 matrix H, DLAQR1 sets v to a
* scalar multiple of the first column of the product
*
* (*) K = (H - (sr1 + i*si1)*I)*(H - (sr2 + i*si2)*I)
*
* scaling to avoid overflows and most underflows. It
* is assumed that either
*
* 1) sr1 = sr2 and si1 = -si2
* or
* 2) si1 = si2 = 0.
*
* This is useful for starting double implicit shift bulges
* in the QR algorithm.
*
*
* N (input) integer
* Order of the matrix H. N must be either 2 or 3.
*
* H (input) DOUBLE PRECISION array of dimension (LDH,N)
* The 2-by-2 or 3-by-3 matrix H in (*).
*
* LDH (input) integer
* The leading dimension of H as declared in
* the calling procedure. LDH.GE.N
*
* SR1 (input) DOUBLE PRECISION
* SI1 The shifts in (*).
* SR2
* SI2
*
* V (output) DOUBLE PRECISION array of dimension N
* A scalar multiple of the first column of the
* matrix K in (*).
*
* ================================================================
* Based on contributions by
* Karen Braman and Ralph Byers, Department of Mathematics,
* University of Kansas, USA
*
* ================================================================
*
* .. Parameters ..
*
*
* @param n
* @param h
* @param ldh
* @param sr1
* @param si1
* @param sr2
* @param si2
* @param v
*
*/
abstract public void dlaqr1(int n, double[] h, int ldh, double sr1, double si1, double sr2, double si2, double[] v);
/**
*
* ..
*
* Given a 2-by-2 or 3-by-3 matrix H, DLAQR1 sets v to a
* scalar multiple of the first column of the product
*
* (*) K = (H - (sr1 + i*si1)*I)*(H - (sr2 + i*si2)*I)
*
* scaling to avoid overflows and most underflows. It
* is assumed that either
*
* 1) sr1 = sr2 and si1 = -si2
* or
* 2) si1 = si2 = 0.
*
* This is useful for starting double implicit shift bulges
* in the QR algorithm.
*
*
* N (input) integer
* Order of the matrix H. N must be either 2 or 3.
*
* H (input) DOUBLE PRECISION array of dimension (LDH,N)
* The 2-by-2 or 3-by-3 matrix H in (*).
*
* LDH (input) integer
* The leading dimension of H as declared in
* the calling procedure. LDH.GE.N
*
* SR1 (input) DOUBLE PRECISION
* SI1 The shifts in (*).
* SR2
* SI2
*
* V (output) DOUBLE PRECISION array of dimension N
* A scalar multiple of the first column of the
* matrix K in (*).
*
* ================================================================
* Based on contributions by
* Karen Braman and Ralph Byers, Department of Mathematics,
* University of Kansas, USA
*
* ================================================================
*
* .. Parameters ..
*
*
* @param n
* @param h
* @param _h_offset
* @param ldh
* @param sr1
* @param si1
* @param sr2
* @param si2
* @param v
* @param _v_offset
*
*/
abstract public void dlaqr1(int n, double[] h, int _h_offset, int ldh, double sr1, double si1, double sr2, double si2, double[] v, int _v_offset);
/**
*
* ..
*
* This subroutine is identical to DLAQR3 except that it avoids
* recursion by calling DLAHQR instead of DLAQR4.
*
*
* ******************************************************************
* Aggressive early deflation:
*
* This subroutine accepts as input an upper Hessenberg matrix
* H and performs an orthogonal similarity transformation
* designed to detect and deflate fully converged eigenvalues from
* a trailing principal submatrix. On output H has been over-
* written by a new Hessenberg matrix that is a perturbation of
* an orthogonal similarity transformation of H. It is to be
* hoped that the final version of H has many zero subdiagonal
* entries.
*
* ******************************************************************
* WANTT (input) LOGICAL
* If .TRUE., then the Hessenberg matrix H is fully updated
* so that the quasi-triangular Schur factor may be
* computed (in cooperation with the calling subroutine).
* If .FALSE., then only enough of H is updated to preserve
* the eigenvalues.
*
* WANTZ (input) LOGICAL
* If .TRUE., then the orthogonal matrix Z is updated so
* so that the orthogonal Schur factor may be computed
* (in cooperation with the calling subroutine).
* If .FALSE., then Z is not referenced.
*
* N (input) INTEGER
* The order of the matrix H and (if WANTZ is .TRUE.) the
* order of the orthogonal matrix Z.
*
* KTOP (input) INTEGER
* It is assumed that either KTOP = 1 or H(KTOP,KTOP-1)=0.
* KBOT and KTOP together determine an isolated block
* along the diagonal of the Hessenberg matrix.
*
* KBOT (input) INTEGER
* It is assumed without a check that either
* KBOT = N or H(KBOT+1,KBOT)=0. KBOT and KTOP together
* determine an isolated block along the diagonal of the
* Hessenberg matrix.
*
* NW (input) INTEGER
* Deflation window size. 1 .LE. NW .LE. (KBOT-KTOP+1).
*
* H (input/output) DOUBLE PRECISION array, dimension (LDH,N)
* On input the initial N-by-N section of H stores the
* Hessenberg matrix undergoing aggressive early deflation.
* On output H has been transformed by an orthogonal
* similarity transformation, perturbed, and the returned
* to Hessenberg form that (it is to be hoped) has some
* zero subdiagonal entries.
*
* LDH (input) integer
* Leading dimension of H just as declared in the calling
* subroutine. N .LE. LDH
*
* ILOZ (input) INTEGER
* IHIZ (input) INTEGER
* Specify the rows of Z to which transformations must be
* applied if WANTZ is .TRUE.. 1 .LE. ILOZ .LE. IHIZ .LE. N.
*
* Z (input/output) DOUBLE PRECISION array, dimension (LDZ,IHI)
* IF WANTZ is .TRUE., then on output, the orthogonal
* similarity transformation mentioned above has been
* accumulated into Z(ILOZ:IHIZ,ILO:IHI) from the right.
* If WANTZ is .FALSE., then Z is unreferenced.
*
* LDZ (input) integer
* The leading dimension of Z just as declared in the
* calling subroutine. 1 .LE. LDZ.
*
* NS (output) integer
* The number of unconverged (ie approximate) eigenvalues
* returned in SR and SI that may be used as shifts by the
* calling subroutine.
*
* ND (output) integer
* The number of converged eigenvalues uncovered by this
* subroutine.
*
* SR (output) DOUBLE PRECISION array, dimension KBOT
* SI (output) DOUBLE PRECISION array, dimension KBOT
* On output, the real and imaginary parts of approximate
* eigenvalues that may be used for shifts are stored in
* SR(KBOT-ND-NS+1) through SR(KBOT-ND) and
* SI(KBOT-ND-NS+1) through SI(KBOT-ND), respectively.
* The real and imaginary parts of converged eigenvalues
* are stored in SR(KBOT-ND+1) through SR(KBOT) and
* SI(KBOT-ND+1) through SI(KBOT), respectively.
*
* V (workspace) DOUBLE PRECISION array, dimension (LDV,NW)
* An NW-by-NW work array.
*
* LDV (input) integer scalar
* The leading dimension of V just as declared in the
* calling subroutine. NW .LE. LDV
*
* NH (input) integer scalar
* The number of columns of T. NH.GE.NW.
*
* T (workspace) DOUBLE PRECISION array, dimension (LDT,NW)
*
* LDT (input) integer
* The leading dimension of T just as declared in the
* calling subroutine. NW .LE. LDT
*
* NV (input) integer
* The number of rows of work array WV available for
* workspace. NV.GE.NW.
*
* WV (workspace) DOUBLE PRECISION array, dimension (LDWV,NW)
*
* LDWV (input) integer
* The leading dimension of W just as declared in the
* calling subroutine. NW .LE. LDV
*
* WORK (workspace) DOUBLE PRECISION array, dimension LWORK.
* On exit, WORK(1) is set to an estimate of the optimal value
* of LWORK for the given values of N, NW, KTOP and KBOT.
*
* LWORK (input) integer
* The dimension of the work array WORK. LWORK = 2*NW
* suffices, but greater efficiency may result from larger
* values of LWORK.
*
* If LWORK = -1, then a workspace query is assumed; DLAQR2
* only estimates the optimal workspace size for the given
* values of N, NW, KTOP and KBOT. The estimate is returned
* in WORK(1). No error message related to LWORK is issued
* by XERBLA. Neither H nor Z are accessed.
*
* ================================================================
* Based on contributions by
* Karen Braman and Ralph Byers, Department of Mathematics,
* University of Kansas, USA
*
* ================================================================
* .. Parameters ..
*
*
* @param wantt
* @param wantz
* @param n
* @param ktop
* @param kbot
* @param nw
* @param h
* @param ldh
* @param iloz
* @param ihiz
* @param z
* @param ldz
* @param ns
* @param nd
* @param sr
* @param si
* @param v
* @param ldv
* @param nh
* @param t
* @param ldt
* @param nv
* @param wv
* @param ldwv
* @param work
* @param lwork
*
*/
abstract public void dlaqr2(boolean wantt, boolean wantz, int n, int ktop, int kbot, int nw, double[] h, int ldh, int iloz, int ihiz, double[] z, int ldz, org.netlib.util.intW ns, org.netlib.util.intW nd, double[] sr, double[] si, double[] v, int ldv, int nh, double[] t, int ldt, int nv, double[] wv, int ldwv, double[] work, int lwork);
/**
*
* ..
*
* This subroutine is identical to DLAQR3 except that it avoids
* recursion by calling DLAHQR instead of DLAQR4.
*
*
* ******************************************************************
* Aggressive early deflation:
*
* This subroutine accepts as input an upper Hessenberg matrix
* H and performs an orthogonal similarity transformation
* designed to detect and deflate fully converged eigenvalues from
* a trailing principal submatrix. On output H has been over-
* written by a new Hessenberg matrix that is a perturbation of
* an orthogonal similarity transformation of H. It is to be
* hoped that the final version of H has many zero subdiagonal
* entries.
*
* ******************************************************************
* WANTT (input) LOGICAL
* If .TRUE., then the Hessenberg matrix H is fully updated
* so that the quasi-triangular Schur factor may be
* computed (in cooperation with the calling subroutine).
* If .FALSE., then only enough of H is updated to preserve
* the eigenvalues.
*
* WANTZ (input) LOGICAL
* If .TRUE., then the orthogonal matrix Z is updated so
* so that the orthogonal Schur factor may be computed
* (in cooperation with the calling subroutine).
* If .FALSE., then Z is not referenced.
*
* N (input) INTEGER
* The order of the matrix H and (if WANTZ is .TRUE.) the
* order of the orthogonal matrix Z.
*
* KTOP (input) INTEGER
* It is assumed that either KTOP = 1 or H(KTOP,KTOP-1)=0.
* KBOT and KTOP together determine an isolated block
* along the diagonal of the Hessenberg matrix.
*
* KBOT (input) INTEGER
* It is assumed without a check that either
* KBOT = N or H(KBOT+1,KBOT)=0. KBOT and KTOP together
* determine an isolated block along the diagonal of the
* Hessenberg matrix.
*
* NW (input) INTEGER
* Deflation window size. 1 .LE. NW .LE. (KBOT-KTOP+1).
*
* H (input/output) DOUBLE PRECISION array, dimension (LDH,N)
* On input the initial N-by-N section of H stores the
* Hessenberg matrix undergoing aggressive early deflation.
* On output H has been transformed by an orthogonal
* similarity transformation, perturbed, and the returned
* to Hessenberg form that (it is to be hoped) has some
* zero subdiagonal entries.
*
* LDH (input) integer
* Leading dimension of H just as declared in the calling
* subroutine. N .LE. LDH
*
* ILOZ (input) INTEGER
* IHIZ (input) INTEGER
* Specify the rows of Z to which transformations must be
* applied if WANTZ is .TRUE.. 1 .LE. ILOZ .LE. IHIZ .LE. N.
*
* Z (input/output) DOUBLE PRECISION array, dimension (LDZ,IHI)
* IF WANTZ is .TRUE., then on output, the orthogonal
* similarity transformation mentioned above has been
* accumulated into Z(ILOZ:IHIZ,ILO:IHI) from the right.
* If WANTZ is .FALSE., then Z is unreferenced.
*
* LDZ (input) integer
* The leading dimension of Z just as declared in the
* calling subroutine. 1 .LE. LDZ.
*
* NS (output) integer
* The number of unconverged (ie approximate) eigenvalues
* returned in SR and SI that may be used as shifts by the
* calling subroutine.
*
* ND (output) integer
* The number of converged eigenvalues uncovered by this
* subroutine.
*
* SR (output) DOUBLE PRECISION array, dimension KBOT
* SI (output) DOUBLE PRECISION array, dimension KBOT
* On output, the real and imaginary parts of approximate
* eigenvalues that may be used for shifts are stored in
* SR(KBOT-ND-NS+1) through SR(KBOT-ND) and
* SI(KBOT-ND-NS+1) through SI(KBOT-ND), respectively.
* The real and imaginary parts of converged eigenvalues
* are stored in SR(KBOT-ND+1) through SR(KBOT) and
* SI(KBOT-ND+1) through SI(KBOT), respectively.
*
* V (workspace) DOUBLE PRECISION array, dimension (LDV,NW)
* An NW-by-NW work array.
*
* LDV (input) integer scalar
* The leading dimension of V just as declared in the
* calling subroutine. NW .LE. LDV
*
* NH (input) integer scalar
* The number of columns of T. NH.GE.NW.
*
* T (workspace) DOUBLE PRECISION array, dimension (LDT,NW)
*
* LDT (input) integer
* The leading dimension of T just as declared in the
* calling subroutine. NW .LE. LDT
*
* NV (input) integer
* The number of rows of work array WV available for
* workspace. NV.GE.NW.
*
* WV (workspace) DOUBLE PRECISION array, dimension (LDWV,NW)
*
* LDWV (input) integer
* The leading dimension of W just as declared in the
* calling subroutine. NW .LE. LDV
*
* WORK (workspace) DOUBLE PRECISION array, dimension LWORK.
* On exit, WORK(1) is set to an estimate of the optimal value
* of LWORK for the given values of N, NW, KTOP and KBOT.
*
* LWORK (input) integer
* The dimension of the work array WORK. LWORK = 2*NW
* suffices, but greater efficiency may result from larger
* values of LWORK.
*
* If LWORK = -1, then a workspace query is assumed; DLAQR2
* only estimates the optimal workspace size for the given
* values of N, NW, KTOP and KBOT. The estimate is returned
* in WORK(1). No error message related to LWORK is issued
* by XERBLA. Neither H nor Z are accessed.
*
* ================================================================
* Based on contributions by
* Karen Braman and Ralph Byers, Department of Mathematics,
* University of Kansas, USA
*
* ================================================================
* .. Parameters ..
*
*
* @param wantt
* @param wantz
* @param n
* @param ktop
* @param kbot
* @param nw
* @param h
* @param _h_offset
* @param ldh
* @param iloz
* @param ihiz
* @param z
* @param _z_offset
* @param ldz
* @param ns
* @param nd
* @param sr
* @param _sr_offset
* @param si
* @param _si_offset
* @param v
* @param _v_offset
* @param ldv
* @param nh
* @param t
* @param _t_offset
* @param ldt
* @param nv
* @param wv
* @param _wv_offset
* @param ldwv
* @param work
* @param _work_offset
* @param lwork
*
*/
abstract public void dlaqr2(boolean wantt, boolean wantz, int n, int ktop, int kbot, int nw, double[] h, int _h_offset, int ldh, int iloz, int ihiz, double[] z, int _z_offset, int ldz, org.netlib.util.intW ns, org.netlib.util.intW nd, double[] sr, int _sr_offset, double[] si, int _si_offset, double[] v, int _v_offset, int ldv, int nh, double[] t, int _t_offset, int ldt, int nv, double[] wv, int _wv_offset, int ldwv, double[] work, int _work_offset, int lwork);
/**
*
* ..
*
* ******************************************************************
* Aggressive early deflation:
*
* This subroutine accepts as input an upper Hessenberg matrix
* H and performs an orthogonal similarity transformation
* designed to detect and deflate fully converged eigenvalues from
* a trailing principal submatrix. On output H has been over-
* written by a new Hessenberg matrix that is a perturbation of
* an orthogonal similarity transformation of H. It is to be
* hoped that the final version of H has many zero subdiagonal
* entries.
*
* ******************************************************************
* WANTT (input) LOGICAL
* If .TRUE., then the Hessenberg matrix H is fully updated
* so that the quasi-triangular Schur factor may be
* computed (in cooperation with the calling subroutine).
* If .FALSE., then only enough of H is updated to preserve
* the eigenvalues.
*
* WANTZ (input) LOGICAL
* If .TRUE., then the orthogonal matrix Z is updated so
* so that the orthogonal Schur factor may be computed
* (in cooperation with the calling subroutine).
* If .FALSE., then Z is not referenced.
*
* N (input) INTEGER
* The order of the matrix H and (if WANTZ is .TRUE.) the
* order of the orthogonal matrix Z.
*
* KTOP (input) INTEGER
* It is assumed that either KTOP = 1 or H(KTOP,KTOP-1)=0.
* KBOT and KTOP together determine an isolated block
* along the diagonal of the Hessenberg matrix.
*
* KBOT (input) INTEGER
* It is assumed without a check that either
* KBOT = N or H(KBOT+1,KBOT)=0. KBOT and KTOP together
* determine an isolated block along the diagonal of the
* Hessenberg matrix.
*
* NW (input) INTEGER
* Deflation window size. 1 .LE. NW .LE. (KBOT-KTOP+1).
*
* H (input/output) DOUBLE PRECISION array, dimension (LDH,N)
* On input the initial N-by-N section of H stores the
* Hessenberg matrix undergoing aggressive early deflation.
* On output H has been transformed by an orthogonal
* similarity transformation, perturbed, and the returned
* to Hessenberg form that (it is to be hoped) has some
* zero subdiagonal entries.
*
* LDH (input) integer
* Leading dimension of H just as declared in the calling
* subroutine. N .LE. LDH
*
* ILOZ (input) INTEGER
* IHIZ (input) INTEGER
* Specify the rows of Z to which transformations must be
* applied if WANTZ is .TRUE.. 1 .LE. ILOZ .LE. IHIZ .LE. N.
*
* Z (input/output) DOUBLE PRECISION array, dimension (LDZ,IHI)
* IF WANTZ is .TRUE., then on output, the orthogonal
* similarity transformation mentioned above has been
* accumulated into Z(ILOZ:IHIZ,ILO:IHI) from the right.
* If WANTZ is .FALSE., then Z is unreferenced.
*
* LDZ (input) integer
* The leading dimension of Z just as declared in the
* calling subroutine. 1 .LE. LDZ.
*
* NS (output) integer
* The number of unconverged (ie approximate) eigenvalues
* returned in SR and SI that may be used as shifts by the
* calling subroutine.
*
* ND (output) integer
* The number of converged eigenvalues uncovered by this
* subroutine.
*
* SR (output) DOUBLE PRECISION array, dimension KBOT
* SI (output) DOUBLE PRECISION array, dimension KBOT
* On output, the real and imaginary parts of approximate
* eigenvalues that may be used for shifts are stored in
* SR(KBOT-ND-NS+1) through SR(KBOT-ND) and
* SI(KBOT-ND-NS+1) through SI(KBOT-ND), respectively.
* The real and imaginary parts of converged eigenvalues
* are stored in SR(KBOT-ND+1) through SR(KBOT) and
* SI(KBOT-ND+1) through SI(KBOT), respectively.
*
* V (workspace) DOUBLE PRECISION array, dimension (LDV,NW)
* An NW-by-NW work array.
*
* LDV (input) integer scalar
* The leading dimension of V just as declared in the
* calling subroutine. NW .LE. LDV
*
* NH (input) integer scalar
* The number of columns of T. NH.GE.NW.
*
* T (workspace) DOUBLE PRECISION array, dimension (LDT,NW)
*
* LDT (input) integer
* The leading dimension of T just as declared in the
* calling subroutine. NW .LE. LDT
*
* NV (input) integer
* The number of rows of work array WV available for
* workspace. NV.GE.NW.
*
* WV (workspace) DOUBLE PRECISION array, dimension (LDWV,NW)
*
* LDWV (input) integer
* The leading dimension of W just as declared in the
* calling subroutine. NW .LE. LDV
*
* WORK (workspace) DOUBLE PRECISION array, dimension LWORK.
* On exit, WORK(1) is set to an estimate of the optimal value
* of LWORK for the given values of N, NW, KTOP and KBOT.
*
* LWORK (input) integer
* The dimension of the work array WORK. LWORK = 2*NW
* suffices, but greater efficiency may result from larger
* values of LWORK.
*
* If LWORK = -1, then a workspace query is assumed; DLAQR3
* only estimates the optimal workspace size for the given
* values of N, NW, KTOP and KBOT. The estimate is returned
* in WORK(1). No error message related to LWORK is issued
* by XERBLA. Neither H nor Z are accessed.
*
* ================================================================
* Based on contributions by
* Karen Braman and Ralph Byers, Department of Mathematics,
* University of Kansas, USA
*
* ==================================================================
* .. Parameters ..
*
*
* @param wantt
* @param wantz
* @param n
* @param ktop
* @param kbot
* @param nw
* @param h
* @param ldh
* @param iloz
* @param ihiz
* @param z
* @param ldz
* @param ns
* @param nd
* @param sr
* @param si
* @param v
* @param ldv
* @param nh
* @param t
* @param ldt
* @param nv
* @param wv
* @param ldwv
* @param work
* @param lwork
*
*/
abstract public void dlaqr3(boolean wantt, boolean wantz, int n, int ktop, int kbot, int nw, double[] h, int ldh, int iloz, int ihiz, double[] z, int ldz, org.netlib.util.intW ns, org.netlib.util.intW nd, double[] sr, double[] si, double[] v, int ldv, int nh, double[] t, int ldt, int nv, double[] wv, int ldwv, double[] work, int lwork);
/**
*
* ..
*
* ******************************************************************
* Aggressive early deflation:
*
* This subroutine accepts as input an upper Hessenberg matrix
* H and performs an orthogonal similarity transformation
* designed to detect and deflate fully converged eigenvalues from
* a trailing principal submatrix. On output H has been over-
* written by a new Hessenberg matrix that is a perturbation of
* an orthogonal similarity transformation of H. It is to be
* hoped that the final version of H has many zero subdiagonal
* entries.
*
* ******************************************************************
* WANTT (input) LOGICAL
* If .TRUE., then the Hessenberg matrix H is fully updated
* so that the quasi-triangular Schur factor may be
* computed (in cooperation with the calling subroutine).
* If .FALSE., then only enough of H is updated to preserve
* the eigenvalues.
*
* WANTZ (input) LOGICAL
* If .TRUE., then the orthogonal matrix Z is updated so
* so that the orthogonal Schur factor may be computed
* (in cooperation with the calling subroutine).
* If .FALSE., then Z is not referenced.
*
* N (input) INTEGER
* The order of the matrix H and (if WANTZ is .TRUE.) the
* order of the orthogonal matrix Z.
*
* KTOP (input) INTEGER
* It is assumed that either KTOP = 1 or H(KTOP,KTOP-1)=0.
* KBOT and KTOP together determine an isolated block
* along the diagonal of the Hessenberg matrix.
*
* KBOT (input) INTEGER
* It is assumed without a check that either
* KBOT = N or H(KBOT+1,KBOT)=0. KBOT and KTOP together
* determine an isolated block along the diagonal of the
* Hessenberg matrix.
*
* NW (input) INTEGER
* Deflation window size. 1 .LE. NW .LE. (KBOT-KTOP+1).
*
* H (input/output) DOUBLE PRECISION array, dimension (LDH,N)
* On input the initial N-by-N section of H stores the
* Hessenberg matrix undergoing aggressive early deflation.
* On output H has been transformed by an orthogonal
* similarity transformation, perturbed, and the returned
* to Hessenberg form that (it is to be hoped) has some
* zero subdiagonal entries.
*
* LDH (input) integer
* Leading dimension of H just as declared in the calling
* subroutine. N .LE. LDH
*
* ILOZ (input) INTEGER
* IHIZ (input) INTEGER
* Specify the rows of Z to which transformations must be
* applied if WANTZ is .TRUE.. 1 .LE. ILOZ .LE. IHIZ .LE. N.
*
* Z (input/output) DOUBLE PRECISION array, dimension (LDZ,IHI)
* IF WANTZ is .TRUE., then on output, the orthogonal
* similarity transformation mentioned above has been
* accumulated into Z(ILOZ:IHIZ,ILO:IHI) from the right.
* If WANTZ is .FALSE., then Z is unreferenced.
*
* LDZ (input) integer
* The leading dimension of Z just as declared in the
* calling subroutine. 1 .LE. LDZ.
*
* NS (output) integer
* The number of unconverged (ie approximate) eigenvalues
* returned in SR and SI that may be used as shifts by the
* calling subroutine.
*
* ND (output) integer
* The number of converged eigenvalues uncovered by this
* subroutine.
*
* SR (output) DOUBLE PRECISION array, dimension KBOT
* SI (output) DOUBLE PRECISION array, dimension KBOT
* On output, the real and imaginary parts of approximate
* eigenvalues that may be used for shifts are stored in
* SR(KBOT-ND-NS+1) through SR(KBOT-ND) and
* SI(KBOT-ND-NS+1) through SI(KBOT-ND), respectively.
* The real and imaginary parts of converged eigenvalues
* are stored in SR(KBOT-ND+1) through SR(KBOT) and
* SI(KBOT-ND+1) through SI(KBOT), respectively.
*
* V (workspace) DOUBLE PRECISION array, dimension (LDV,NW)
* An NW-by-NW work array.
*
* LDV (input) integer scalar
* The leading dimension of V just as declared in the
* calling subroutine. NW .LE. LDV
*
* NH (input) integer scalar
* The number of columns of T. NH.GE.NW.
*
* T (workspace) DOUBLE PRECISION array, dimension (LDT,NW)
*
* LDT (input) integer
* The leading dimension of T just as declared in the
* calling subroutine. NW .LE. LDT
*
* NV (input) integer
* The number of rows of work array WV available for
* workspace. NV.GE.NW.
*
* WV (workspace) DOUBLE PRECISION array, dimension (LDWV,NW)
*
* LDWV (input) integer
* The leading dimension of W just as declared in the
* calling subroutine. NW .LE. LDV
*
* WORK (workspace) DOUBLE PRECISION array, dimension LWORK.
* On exit, WORK(1) is set to an estimate of the optimal value
* of LWORK for the given values of N, NW, KTOP and KBOT.
*
* LWORK (input) integer
* The dimension of the work array WORK. LWORK = 2*NW
* suffices, but greater efficiency may result from larger
* values of LWORK.
*
* If LWORK = -1, then a workspace query is assumed; DLAQR3
* only estimates the optimal workspace size for the given
* values of N, NW, KTOP and KBOT. The estimate is returned
* in WORK(1). No error message related to LWORK is issued
* by XERBLA. Neither H nor Z are accessed.
*
* ================================================================
* Based on contributions by
* Karen Braman and Ralph Byers, Department of Mathematics,
* University of Kansas, USA
*
* ==================================================================
* .. Parameters ..
*
*
* @param wantt
* @param wantz
* @param n
* @param ktop
* @param kbot
* @param nw
* @param h
* @param _h_offset
* @param ldh
* @param iloz
* @param ihiz
* @param z
* @param _z_offset
* @param ldz
* @param ns
* @param nd
* @param sr
* @param _sr_offset
* @param si
* @param _si_offset
* @param v
* @param _v_offset
* @param ldv
* @param nh
* @param t
* @param _t_offset
* @param ldt
* @param nv
* @param wv
* @param _wv_offset
* @param ldwv
* @param work
* @param _work_offset
* @param lwork
*
*/
abstract public void dlaqr3(boolean wantt, boolean wantz, int n, int ktop, int kbot, int nw, double[] h, int _h_offset, int ldh, int iloz, int ihiz, double[] z, int _z_offset, int ldz, org.netlib.util.intW ns, org.netlib.util.intW nd, double[] sr, int _sr_offset, double[] si, int _si_offset, double[] v, int _v_offset, int ldv, int nh, double[] t, int _t_offset, int ldt, int nv, double[] wv, int _wv_offset, int ldwv, double[] work, int _work_offset, int lwork);
/**
*
* ..
*
* This subroutine implements one level of recursion for DLAQR0.
* It is a complete implementation of the small bulge multi-shift
* QR algorithm. It may be called by DLAQR0 and, for large enough
* deflation window size, it may be called by DLAQR3. This
* subroutine is identical to DLAQR0 except that it calls DLAQR2
* instead of DLAQR3.
*
* Purpose
* =======
*
* DLAQR4 computes the eigenvalues of a Hessenberg matrix H
* and, optionally, the matrices T and Z from the Schur decomposition
* H = Z T Z**T, where T is an upper quasi-triangular matrix (the
* Schur form), and Z is the orthogonal matrix of Schur vectors.
*
* Optionally Z may be postmultiplied into an input orthogonal
* matrix Q so that this routine can give the Schur factorization
* of a matrix A which has been reduced to the Hessenberg form H
* by the orthogonal matrix Q: A = Q*H*Q**T = (QZ)*T*(QZ)**T.
*
* Arguments
* =========
*
* WANTT (input) LOGICAL
* = .TRUE. : the full Schur form T is required;
* = .FALSE.: only eigenvalues are required.
*
* WANTZ (input) LOGICAL
* = .TRUE. : the matrix of Schur vectors Z is required;
* = .FALSE.: Schur vectors are not required.
*
* N (input) INTEGER
* The order of the matrix H. N .GE. 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* It is assumed that H is already upper triangular in rows
* and columns 1:ILO-1 and IHI+1:N and, if ILO.GT.1,
* H(ILO,ILO-1) is zero. ILO and IHI are normally set by a
* previous call to DGEBAL, and then passed to DGEHRD when the
* matrix output by DGEBAL is reduced to Hessenberg form.
* Otherwise, ILO and IHI should be set to 1 and N,
* respectively. If N.GT.0, then 1.LE.ILO.LE.IHI.LE.N.
* If N = 0, then ILO = 1 and IHI = 0.
*
* H (input/output) DOUBLE PRECISION array, dimension (LDH,N)
* On entry, the upper Hessenberg matrix H.
* On exit, if INFO = 0 and WANTT is .TRUE., then H contains
* the upper quasi-triangular matrix T from the Schur
* decomposition (the Schur form); 2-by-2 diagonal blocks
* (corresponding to complex conjugate pairs of eigenvalues)
* are returned in standard form, with H(i,i) = H(i+1,i+1)
* and H(i+1,i)*H(i,i+1).LT.0. If INFO = 0 and WANTT is
* .FALSE., then the contents of H are unspecified on exit.
* (The output value of H when INFO.GT.0 is given under the
* description of INFO below.)
*
* This subroutine may explicitly set H(i,j) = 0 for i.GT.j and
* j = 1, 2, ... ILO-1 or j = IHI+1, IHI+2, ... N.
*
* LDH (input) INTEGER
* The leading dimension of the array H. LDH .GE. max(1,N).
*
* WR (output) DOUBLE PRECISION array, dimension (IHI)
* WI (output) DOUBLE PRECISION array, dimension (IHI)
* The real and imaginary parts, respectively, of the computed
* eigenvalues of H(ILO:IHI,ILO:IHI) are stored WR(ILO:IHI)
* and WI(ILO:IHI). If two eigenvalues are computed as a
* complex conjugate pair, they are stored in consecutive
* elements of WR and WI, say the i-th and (i+1)th, with
* WI(i) .GT. 0 and WI(i+1) .LT. 0. If WANTT is .TRUE., then
* the eigenvalues are stored in the same order as on the
* diagonal of the Schur form returned in H, with
* WR(i) = H(i,i) and, if H(i:i+1,i:i+1) is a 2-by-2 diagonal
* block, WI(i) = sqrt(-H(i+1,i)*H(i,i+1)) and
* WI(i+1) = -WI(i).
*
* ILOZ (input) INTEGER
* IHIZ (input) INTEGER
* Specify the rows of Z to which transformations must be
* applied if WANTZ is .TRUE..
* 1 .LE. ILOZ .LE. ILO; IHI .LE. IHIZ .LE. N.
*
* Z (input/output) DOUBLE PRECISION array, dimension (LDZ,IHI)
* If WANTZ is .FALSE., then Z is not referenced.
* If WANTZ is .TRUE., then Z(ILO:IHI,ILOZ:IHIZ) is
* replaced by Z(ILO:IHI,ILOZ:IHIZ)*U where U is the
* orthogonal Schur factor of H(ILO:IHI,ILO:IHI).
* (The output value of Z when INFO.GT.0 is given under
* the description of INFO below.)
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. if WANTZ is .TRUE.
* then LDZ.GE.MAX(1,IHIZ). Otherwize, LDZ.GE.1.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension LWORK
* On exit, if LWORK = -1, WORK(1) returns an estimate of
* the optimal value for LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK .GE. max(1,N)
* is sufficient, but LWORK typically as large as 6*N may
* be required for optimal performance. A workspace query
* to determine the optimal workspace size is recommended.
*
* If LWORK = -1, then DLAQR4 does a workspace query.
* In this case, DLAQR4 checks the input parameters and
* estimates the optimal workspace size for the given
* values of N, ILO and IHI. The estimate is returned
* in WORK(1). No error message related to LWORK is
* issued by XERBLA. Neither H nor Z are accessed.
*
*
* INFO (output) INTEGER
* = 0: successful exit
* .GT. 0: if INFO = i, DLAQR4 failed to compute all of
* the eigenvalues. Elements 1:ilo-1 and i+1:n of WR
* and WI contain those eigenvalues which have been
* successfully computed. (Failures are rare.)
*
* If INFO .GT. 0 and WANT is .FALSE., then on exit,
* the remaining unconverged eigenvalues are the eigen-
* values of the upper Hessenberg matrix rows and
* columns ILO through INFO of the final, output
* value of H.
*
* If INFO .GT. 0 and WANTT is .TRUE., then on exit
*
* (*) (initial value of H)*U = U*(final value of H)
*
* where U is an orthogonal matrix. The final
* value of H is upper Hessenberg and quasi-triangular
* in rows and columns INFO+1 through IHI.
*
* If INFO .GT. 0 and WANTZ is .TRUE., then on exit
*
* (final value of Z(ILO:IHI,ILOZ:IHIZ)
* = (initial value of Z(ILO:IHI,ILOZ:IHIZ)*U
*
* where U is the orthogonal matrix in (*) (regard-
* less of the value of WANTT.)
*
* If INFO .GT. 0 and WANTZ is .FALSE., then Z is not
* accessed.
*
* ================================================================
* Based on contributions by
* Karen Braman and Ralph Byers, Department of Mathematics,
* University of Kansas, USA
*
* ================================================================
* References:
* K. Braman, R. Byers and R. Mathias, The Multi-Shift QR
* Algorithm Part I: Maintaining Well Focused Shifts, and Level 3
* Performance, SIAM Journal of Matrix Analysis, volume 23, pages
* 929--947, 2002.
*
* K. Braman, R. Byers and R. Mathias, The Multi-Shift QR
* Algorithm Part II: Aggressive Early Deflation, SIAM Journal
* of Matrix Analysis, volume 23, pages 948--973, 2002.
*
* ================================================================
* .. Parameters ..
*
* ==== Matrices of order NTINY or smaller must be processed by
* . DLAHQR because of insufficient subdiagonal scratch space.
* . (This is a hard limit.) ====
*
* ==== Exceptional deflation windows: try to cure rare
* . slow convergence by increasing the size of the
* . deflation window after KEXNW iterations. =====
*
* ==== Exceptional shifts: try to cure rare slow convergence
* . with ad-hoc exceptional shifts every KEXSH iterations.
* . The constants WILK1 and WILK2 are used to form the
* . exceptional shifts. ====
*
*
*
* @param wantt
* @param wantz
* @param n
* @param ilo
* @param ihi
* @param h
* @param ldh
* @param wr
* @param wi
* @param iloz
* @param ihiz
* @param z
* @param ldz
* @param work
* @param lwork
* @param info
*
*/
abstract public void dlaqr4(boolean wantt, boolean wantz, int n, int ilo, int ihi, double[] h, int ldh, double[] wr, double[] wi, int iloz, int ihiz, double[] z, int ldz, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* This subroutine implements one level of recursion for DLAQR0.
* It is a complete implementation of the small bulge multi-shift
* QR algorithm. It may be called by DLAQR0 and, for large enough
* deflation window size, it may be called by DLAQR3. This
* subroutine is identical to DLAQR0 except that it calls DLAQR2
* instead of DLAQR3.
*
* Purpose
* =======
*
* DLAQR4 computes the eigenvalues of a Hessenberg matrix H
* and, optionally, the matrices T and Z from the Schur decomposition
* H = Z T Z**T, where T is an upper quasi-triangular matrix (the
* Schur form), and Z is the orthogonal matrix of Schur vectors.
*
* Optionally Z may be postmultiplied into an input orthogonal
* matrix Q so that this routine can give the Schur factorization
* of a matrix A which has been reduced to the Hessenberg form H
* by the orthogonal matrix Q: A = Q*H*Q**T = (QZ)*T*(QZ)**T.
*
* Arguments
* =========
*
* WANTT (input) LOGICAL
* = .TRUE. : the full Schur form T is required;
* = .FALSE.: only eigenvalues are required.
*
* WANTZ (input) LOGICAL
* = .TRUE. : the matrix of Schur vectors Z is required;
* = .FALSE.: Schur vectors are not required.
*
* N (input) INTEGER
* The order of the matrix H. N .GE. 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* It is assumed that H is already upper triangular in rows
* and columns 1:ILO-1 and IHI+1:N and, if ILO.GT.1,
* H(ILO,ILO-1) is zero. ILO and IHI are normally set by a
* previous call to DGEBAL, and then passed to DGEHRD when the
* matrix output by DGEBAL is reduced to Hessenberg form.
* Otherwise, ILO and IHI should be set to 1 and N,
* respectively. If N.GT.0, then 1.LE.ILO.LE.IHI.LE.N.
* If N = 0, then ILO = 1 and IHI = 0.
*
* H (input/output) DOUBLE PRECISION array, dimension (LDH,N)
* On entry, the upper Hessenberg matrix H.
* On exit, if INFO = 0 and WANTT is .TRUE., then H contains
* the upper quasi-triangular matrix T from the Schur
* decomposition (the Schur form); 2-by-2 diagonal blocks
* (corresponding to complex conjugate pairs of eigenvalues)
* are returned in standard form, with H(i,i) = H(i+1,i+1)
* and H(i+1,i)*H(i,i+1).LT.0. If INFO = 0 and WANTT is
* .FALSE., then the contents of H are unspecified on exit.
* (The output value of H when INFO.GT.0 is given under the
* description of INFO below.)
*
* This subroutine may explicitly set H(i,j) = 0 for i.GT.j and
* j = 1, 2, ... ILO-1 or j = IHI+1, IHI+2, ... N.
*
* LDH (input) INTEGER
* The leading dimension of the array H. LDH .GE. max(1,N).
*
* WR (output) DOUBLE PRECISION array, dimension (IHI)
* WI (output) DOUBLE PRECISION array, dimension (IHI)
* The real and imaginary parts, respectively, of the computed
* eigenvalues of H(ILO:IHI,ILO:IHI) are stored WR(ILO:IHI)
* and WI(ILO:IHI). If two eigenvalues are computed as a
* complex conjugate pair, they are stored in consecutive
* elements of WR and WI, say the i-th and (i+1)th, with
* WI(i) .GT. 0 and WI(i+1) .LT. 0. If WANTT is .TRUE., then
* the eigenvalues are stored in the same order as on the
* diagonal of the Schur form returned in H, with
* WR(i) = H(i,i) and, if H(i:i+1,i:i+1) is a 2-by-2 diagonal
* block, WI(i) = sqrt(-H(i+1,i)*H(i,i+1)) and
* WI(i+1) = -WI(i).
*
* ILOZ (input) INTEGER
* IHIZ (input) INTEGER
* Specify the rows of Z to which transformations must be
* applied if WANTZ is .TRUE..
* 1 .LE. ILOZ .LE. ILO; IHI .LE. IHIZ .LE. N.
*
* Z (input/output) DOUBLE PRECISION array, dimension (LDZ,IHI)
* If WANTZ is .FALSE., then Z is not referenced.
* If WANTZ is .TRUE., then Z(ILO:IHI,ILOZ:IHIZ) is
* replaced by Z(ILO:IHI,ILOZ:IHIZ)*U where U is the
* orthogonal Schur factor of H(ILO:IHI,ILO:IHI).
* (The output value of Z when INFO.GT.0 is given under
* the description of INFO below.)
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. if WANTZ is .TRUE.
* then LDZ.GE.MAX(1,IHIZ). Otherwize, LDZ.GE.1.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension LWORK
* On exit, if LWORK = -1, WORK(1) returns an estimate of
* the optimal value for LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK .GE. max(1,N)
* is sufficient, but LWORK typically as large as 6*N may
* be required for optimal performance. A workspace query
* to determine the optimal workspace size is recommended.
*
* If LWORK = -1, then DLAQR4 does a workspace query.
* In this case, DLAQR4 checks the input parameters and
* estimates the optimal workspace size for the given
* values of N, ILO and IHI. The estimate is returned
* in WORK(1). No error message related to LWORK is
* issued by XERBLA. Neither H nor Z are accessed.
*
*
* INFO (output) INTEGER
* = 0: successful exit
* .GT. 0: if INFO = i, DLAQR4 failed to compute all of
* the eigenvalues. Elements 1:ilo-1 and i+1:n of WR
* and WI contain those eigenvalues which have been
* successfully computed. (Failures are rare.)
*
* If INFO .GT. 0 and WANT is .FALSE., then on exit,
* the remaining unconverged eigenvalues are the eigen-
* values of the upper Hessenberg matrix rows and
* columns ILO through INFO of the final, output
* value of H.
*
* If INFO .GT. 0 and WANTT is .TRUE., then on exit
*
* (*) (initial value of H)*U = U*(final value of H)
*
* where U is an orthogonal matrix. The final
* value of H is upper Hessenberg and quasi-triangular
* in rows and columns INFO+1 through IHI.
*
* If INFO .GT. 0 and WANTZ is .TRUE., then on exit
*
* (final value of Z(ILO:IHI,ILOZ:IHIZ)
* = (initial value of Z(ILO:IHI,ILOZ:IHIZ)*U
*
* where U is the orthogonal matrix in (*) (regard-
* less of the value of WANTT.)
*
* If INFO .GT. 0 and WANTZ is .FALSE., then Z is not
* accessed.
*
* ================================================================
* Based on contributions by
* Karen Braman and Ralph Byers, Department of Mathematics,
* University of Kansas, USA
*
* ================================================================
* References:
* K. Braman, R. Byers and R. Mathias, The Multi-Shift QR
* Algorithm Part I: Maintaining Well Focused Shifts, and Level 3
* Performance, SIAM Journal of Matrix Analysis, volume 23, pages
* 929--947, 2002.
*
* K. Braman, R. Byers and R. Mathias, The Multi-Shift QR
* Algorithm Part II: Aggressive Early Deflation, SIAM Journal
* of Matrix Analysis, volume 23, pages 948--973, 2002.
*
* ================================================================
* .. Parameters ..
*
* ==== Matrices of order NTINY or smaller must be processed by
* . DLAHQR because of insufficient subdiagonal scratch space.
* . (This is a hard limit.) ====
*
* ==== Exceptional deflation windows: try to cure rare
* . slow convergence by increasing the size of the
* . deflation window after KEXNW iterations. =====
*
* ==== Exceptional shifts: try to cure rare slow convergence
* . with ad-hoc exceptional shifts every KEXSH iterations.
* . The constants WILK1 and WILK2 are used to form the
* . exceptional shifts. ====
*
*
*
* @param wantt
* @param wantz
* @param n
* @param ilo
* @param ihi
* @param h
* @param _h_offset
* @param ldh
* @param wr
* @param _wr_offset
* @param wi
* @param _wi_offset
* @param iloz
* @param ihiz
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dlaqr4(boolean wantt, boolean wantz, int n, int ilo, int ihi, double[] h, int _h_offset, int ldh, double[] wr, int _wr_offset, double[] wi, int _wi_offset, int iloz, int ihiz, double[] z, int _z_offset, int ldz, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* This auxiliary subroutine called by DLAQR0 performs a
* single small-bulge multi-shift QR sweep.
*
* WANTT (input) logical scalar
* WANTT = .true. if the quasi-triangular Schur factor
* is being computed. WANTT is set to .false. otherwise.
*
* WANTZ (input) logical scalar
* WANTZ = .true. if the orthogonal Schur factor is being
* computed. WANTZ is set to .false. otherwise.
*
* KACC22 (input) integer with value 0, 1, or 2.
* Specifies the computation mode of far-from-diagonal
* orthogonal updates.
* = 0: DLAQR5 does not accumulate reflections and does not
* use matrix-matrix multiply to update far-from-diagonal
* matrix entries.
* = 1: DLAQR5 accumulates reflections and uses matrix-matrix
* multiply to update the far-from-diagonal matrix entries.
* = 2: DLAQR5 accumulates reflections, uses matrix-matrix
* multiply to update the far-from-diagonal matrix entries,
* and takes advantage of 2-by-2 block structure during
* matrix multiplies.
*
* N (input) integer scalar
* N is the order of the Hessenberg matrix H upon which this
* subroutine operates.
*
* KTOP (input) integer scalar
* KBOT (input) integer scalar
* These are the first and last rows and columns of an
* isolated diagonal block upon which the QR sweep is to be
* applied. It is assumed without a check that
* either KTOP = 1 or H(KTOP,KTOP-1) = 0
* and
* either KBOT = N or H(KBOT+1,KBOT) = 0.
*
* NSHFTS (input) integer scalar
* NSHFTS gives the number of simultaneous shifts. NSHFTS
* must be positive and even.
*
* SR (input) DOUBLE PRECISION array of size (NSHFTS)
* SI (input) DOUBLE PRECISION array of size (NSHFTS)
* SR contains the real parts and SI contains the imaginary
* parts of the NSHFTS shifts of origin that define the
* multi-shift QR sweep.
*
* H (input/output) DOUBLE PRECISION array of size (LDH,N)
* On input H contains a Hessenberg matrix. On output a
* multi-shift QR sweep with shifts SR(J)+i*SI(J) is applied
* to the isolated diagonal block in rows and columns KTOP
* through KBOT.
*
* LDH (input) integer scalar
* LDH is the leading dimension of H just as declared in the
* calling procedure. LDH.GE.MAX(1,N).
*
* ILOZ (input) INTEGER
* IHIZ (input) INTEGER
* Specify the rows of Z to which transformations must be
* applied if WANTZ is .TRUE.. 1 .LE. ILOZ .LE. IHIZ .LE. N
*
* Z (input/output) DOUBLE PRECISION array of size (LDZ,IHI)
* If WANTZ = .TRUE., then the QR Sweep orthogonal
* similarity transformation is accumulated into
* Z(ILOZ:IHIZ,ILO:IHI) from the right.
* If WANTZ = .FALSE., then Z is unreferenced.
*
* LDZ (input) integer scalar
* LDA is the leading dimension of Z just as declared in
* the calling procedure. LDZ.GE.N.
*
* V (workspace) DOUBLE PRECISION array of size (LDV,NSHFTS/2)
*
* LDV (input) integer scalar
* LDV is the leading dimension of V as declared in the
* calling procedure. LDV.GE.3.
*
* U (workspace) DOUBLE PRECISION array of size
* (LDU,3*NSHFTS-3)
*
* LDU (input) integer scalar
* LDU is the leading dimension of U just as declared in the
* in the calling subroutine. LDU.GE.3*NSHFTS-3.
*
* NH (input) integer scalar
* NH is the number of columns in array WH available for
* workspace. NH.GE.1.
*
* WH (workspace) DOUBLE PRECISION array of size (LDWH,NH)
*
* LDWH (input) integer scalar
* Leading dimension of WH just as declared in the
* calling procedure. LDWH.GE.3*NSHFTS-3.
*
* NV (input) integer scalar
* NV is the number of rows in WV agailable for workspace.
* NV.GE.1.
*
* WV (workspace) DOUBLE PRECISION array of size
* (LDWV,3*NSHFTS-3)
*
* LDWV (input) integer scalar
* LDWV is the leading dimension of WV as declared in the
* in the calling subroutine. LDWV.GE.NV.
*
*
* ================================================================
* Based on contributions by
* Karen Braman and Ralph Byers, Department of Mathematics,
* University of Kansas, USA
*
* ============================================================
* Reference:
*
* K. Braman, R. Byers and R. Mathias, The Multi-Shift QR
* Algorithm Part I: Maintaining Well Focused Shifts, and
* Level 3 Performance, SIAM Journal of Matrix Analysis,
* volume 23, pages 929--947, 2002.
*
* ============================================================
* .. Parameters ..
*
*
* @param wantt
* @param wantz
* @param kacc22
* @param n
* @param ktop
* @param kbot
* @param nshfts
* @param sr
* @param si
* @param h
* @param ldh
* @param iloz
* @param ihiz
* @param z
* @param ldz
* @param v
* @param ldv
* @param u
* @param ldu
* @param nv
* @param wv
* @param ldwv
* @param nh
* @param wh
* @param ldwh
*
*/
abstract public void dlaqr5(boolean wantt, boolean wantz, int kacc22, int n, int ktop, int kbot, int nshfts, double[] sr, double[] si, double[] h, int ldh, int iloz, int ihiz, double[] z, int ldz, double[] v, int ldv, double[] u, int ldu, int nv, double[] wv, int ldwv, int nh, double[] wh, int ldwh);
/**
*
* ..
*
* This auxiliary subroutine called by DLAQR0 performs a
* single small-bulge multi-shift QR sweep.
*
* WANTT (input) logical scalar
* WANTT = .true. if the quasi-triangular Schur factor
* is being computed. WANTT is set to .false. otherwise.
*
* WANTZ (input) logical scalar
* WANTZ = .true. if the orthogonal Schur factor is being
* computed. WANTZ is set to .false. otherwise.
*
* KACC22 (input) integer with value 0, 1, or 2.
* Specifies the computation mode of far-from-diagonal
* orthogonal updates.
* = 0: DLAQR5 does not accumulate reflections and does not
* use matrix-matrix multiply to update far-from-diagonal
* matrix entries.
* = 1: DLAQR5 accumulates reflections and uses matrix-matrix
* multiply to update the far-from-diagonal matrix entries.
* = 2: DLAQR5 accumulates reflections, uses matrix-matrix
* multiply to update the far-from-diagonal matrix entries,
* and takes advantage of 2-by-2 block structure during
* matrix multiplies.
*
* N (input) integer scalar
* N is the order of the Hessenberg matrix H upon which this
* subroutine operates.
*
* KTOP (input) integer scalar
* KBOT (input) integer scalar
* These are the first and last rows and columns of an
* isolated diagonal block upon which the QR sweep is to be
* applied. It is assumed without a check that
* either KTOP = 1 or H(KTOP,KTOP-1) = 0
* and
* either KBOT = N or H(KBOT+1,KBOT) = 0.
*
* NSHFTS (input) integer scalar
* NSHFTS gives the number of simultaneous shifts. NSHFTS
* must be positive and even.
*
* SR (input) DOUBLE PRECISION array of size (NSHFTS)
* SI (input) DOUBLE PRECISION array of size (NSHFTS)
* SR contains the real parts and SI contains the imaginary
* parts of the NSHFTS shifts of origin that define the
* multi-shift QR sweep.
*
* H (input/output) DOUBLE PRECISION array of size (LDH,N)
* On input H contains a Hessenberg matrix. On output a
* multi-shift QR sweep with shifts SR(J)+i*SI(J) is applied
* to the isolated diagonal block in rows and columns KTOP
* through KBOT.
*
* LDH (input) integer scalar
* LDH is the leading dimension of H just as declared in the
* calling procedure. LDH.GE.MAX(1,N).
*
* ILOZ (input) INTEGER
* IHIZ (input) INTEGER
* Specify the rows of Z to which transformations must be
* applied if WANTZ is .TRUE.. 1 .LE. ILOZ .LE. IHIZ .LE. N
*
* Z (input/output) DOUBLE PRECISION array of size (LDZ,IHI)
* If WANTZ = .TRUE., then the QR Sweep orthogonal
* similarity transformation is accumulated into
* Z(ILOZ:IHIZ,ILO:IHI) from the right.
* If WANTZ = .FALSE., then Z is unreferenced.
*
* LDZ (input) integer scalar
* LDA is the leading dimension of Z just as declared in
* the calling procedure. LDZ.GE.N.
*
* V (workspace) DOUBLE PRECISION array of size (LDV,NSHFTS/2)
*
* LDV (input) integer scalar
* LDV is the leading dimension of V as declared in the
* calling procedure. LDV.GE.3.
*
* U (workspace) DOUBLE PRECISION array of size
* (LDU,3*NSHFTS-3)
*
* LDU (input) integer scalar
* LDU is the leading dimension of U just as declared in the
* in the calling subroutine. LDU.GE.3*NSHFTS-3.
*
* NH (input) integer scalar
* NH is the number of columns in array WH available for
* workspace. NH.GE.1.
*
* WH (workspace) DOUBLE PRECISION array of size (LDWH,NH)
*
* LDWH (input) integer scalar
* Leading dimension of WH just as declared in the
* calling procedure. LDWH.GE.3*NSHFTS-3.
*
* NV (input) integer scalar
* NV is the number of rows in WV agailable for workspace.
* NV.GE.1.
*
* WV (workspace) DOUBLE PRECISION array of size
* (LDWV,3*NSHFTS-3)
*
* LDWV (input) integer scalar
* LDWV is the leading dimension of WV as declared in the
* in the calling subroutine. LDWV.GE.NV.
*
*
* ================================================================
* Based on contributions by
* Karen Braman and Ralph Byers, Department of Mathematics,
* University of Kansas, USA
*
* ============================================================
* Reference:
*
* K. Braman, R. Byers and R. Mathias, The Multi-Shift QR
* Algorithm Part I: Maintaining Well Focused Shifts, and
* Level 3 Performance, SIAM Journal of Matrix Analysis,
* volume 23, pages 929--947, 2002.
*
* ============================================================
* .. Parameters ..
*
*
* @param wantt
* @param wantz
* @param kacc22
* @param n
* @param ktop
* @param kbot
* @param nshfts
* @param sr
* @param _sr_offset
* @param si
* @param _si_offset
* @param h
* @param _h_offset
* @param ldh
* @param iloz
* @param ihiz
* @param z
* @param _z_offset
* @param ldz
* @param v
* @param _v_offset
* @param ldv
* @param u
* @param _u_offset
* @param ldu
* @param nv
* @param wv
* @param _wv_offset
* @param ldwv
* @param nh
* @param wh
* @param _wh_offset
* @param ldwh
*
*/
abstract public void dlaqr5(boolean wantt, boolean wantz, int kacc22, int n, int ktop, int kbot, int nshfts, double[] sr, int _sr_offset, double[] si, int _si_offset, double[] h, int _h_offset, int ldh, int iloz, int ihiz, double[] z, int _z_offset, int ldz, double[] v, int _v_offset, int ldv, double[] u, int _u_offset, int ldu, int nv, double[] wv, int _wv_offset, int ldwv, int nh, double[] wh, int _wh_offset, int ldwh);
/**
*
* ..
*
* Purpose
* =======
*
* DLAQSB equilibrates a symmetric band matrix A using the scaling
* factors in the vector S.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of super-diagonals of the matrix A if UPLO = 'U',
* or the number of sub-diagonals if UPLO = 'L'. KD >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB,N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* On exit, if INFO = 0, the triangular factor U or L from the
* Cholesky factorization A = U'*U or A = L*L' of the band
* matrix A, in the same storage format as A.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* S (input) DOUBLE PRECISION array, dimension (N)
* The scale factors for A.
*
* SCOND (input) DOUBLE PRECISION
* Ratio of the smallest S(i) to the largest S(i).
*
* AMAX (input) DOUBLE PRECISION
* Absolute value of largest matrix entry.
*
* EQUED (output) CHARACTER*1
* Specifies whether or not equilibration was done.
* = 'N': No equilibration.
* = 'Y': Equilibration was done, i.e., A has been replaced by
* diag(S) * A * diag(S).
*
* Internal Parameters
* ===================
*
* THRESH is a threshold value used to decide if scaling should be done
* based on the ratio of the scaling factors. If SCOND < THRESH,
* scaling is done.
*
* LARGE and SMALL are threshold values used to decide if scaling should
* be done based on the absolute size of the largest matrix element.
* If AMAX > LARGE or AMAX < SMALL, scaling is done.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param kd
* @param ab
* @param ldab
* @param s
* @param scond
* @param amax
* @param equed
*
*/
abstract public void dlaqsb(java.lang.String uplo, int n, int kd, double[] ab, int ldab, double[] s, double scond, double amax, org.netlib.util.StringW equed);
/**
*
* ..
*
* Purpose
* =======
*
* DLAQSB equilibrates a symmetric band matrix A using the scaling
* factors in the vector S.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of super-diagonals of the matrix A if UPLO = 'U',
* or the number of sub-diagonals if UPLO = 'L'. KD >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB,N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* On exit, if INFO = 0, the triangular factor U or L from the
* Cholesky factorization A = U'*U or A = L*L' of the band
* matrix A, in the same storage format as A.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* S (input) DOUBLE PRECISION array, dimension (N)
* The scale factors for A.
*
* SCOND (input) DOUBLE PRECISION
* Ratio of the smallest S(i) to the largest S(i).
*
* AMAX (input) DOUBLE PRECISION
* Absolute value of largest matrix entry.
*
* EQUED (output) CHARACTER*1
* Specifies whether or not equilibration was done.
* = 'N': No equilibration.
* = 'Y': Equilibration was done, i.e., A has been replaced by
* diag(S) * A * diag(S).
*
* Internal Parameters
* ===================
*
* THRESH is a threshold value used to decide if scaling should be done
* based on the ratio of the scaling factors. If SCOND < THRESH,
* scaling is done.
*
* LARGE and SMALL are threshold values used to decide if scaling should
* be done based on the absolute size of the largest matrix element.
* If AMAX > LARGE or AMAX < SMALL, scaling is done.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param kd
* @param ab
* @param _ab_offset
* @param ldab
* @param s
* @param _s_offset
* @param scond
* @param amax
* @param equed
*
*/
abstract public void dlaqsb(java.lang.String uplo, int n, int kd, double[] ab, int _ab_offset, int ldab, double[] s, int _s_offset, double scond, double amax, org.netlib.util.StringW equed);
/**
*
* ..
*
* Purpose
* =======
*
* DLAQSP equilibrates a symmetric matrix A using the scaling factors
* in the vector S.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, the equilibrated matrix: diag(S) * A * diag(S), in
* the same storage format as A.
*
* S (input) DOUBLE PRECISION array, dimension (N)
* The scale factors for A.
*
* SCOND (input) DOUBLE PRECISION
* Ratio of the smallest S(i) to the largest S(i).
*
* AMAX (input) DOUBLE PRECISION
* Absolute value of largest matrix entry.
*
* EQUED (output) CHARACTER*1
* Specifies whether or not equilibration was done.
* = 'N': No equilibration.
* = 'Y': Equilibration was done, i.e., A has been replaced by
* diag(S) * A * diag(S).
*
* Internal Parameters
* ===================
*
* THRESH is a threshold value used to decide if scaling should be done
* based on the ratio of the scaling factors. If SCOND < THRESH,
* scaling is done.
*
* LARGE and SMALL are threshold values used to decide if scaling should
* be done based on the absolute size of the largest matrix element.
* If AMAX > LARGE or AMAX < SMALL, scaling is done.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param s
* @param scond
* @param amax
* @param equed
*
*/
abstract public void dlaqsp(java.lang.String uplo, int n, double[] ap, double[] s, double scond, double amax, org.netlib.util.StringW equed);
/**
*
* ..
*
* Purpose
* =======
*
* DLAQSP equilibrates a symmetric matrix A using the scaling factors
* in the vector S.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, the equilibrated matrix: diag(S) * A * diag(S), in
* the same storage format as A.
*
* S (input) DOUBLE PRECISION array, dimension (N)
* The scale factors for A.
*
* SCOND (input) DOUBLE PRECISION
* Ratio of the smallest S(i) to the largest S(i).
*
* AMAX (input) DOUBLE PRECISION
* Absolute value of largest matrix entry.
*
* EQUED (output) CHARACTER*1
* Specifies whether or not equilibration was done.
* = 'N': No equilibration.
* = 'Y': Equilibration was done, i.e., A has been replaced by
* diag(S) * A * diag(S).
*
* Internal Parameters
* ===================
*
* THRESH is a threshold value used to decide if scaling should be done
* based on the ratio of the scaling factors. If SCOND < THRESH,
* scaling is done.
*
* LARGE and SMALL are threshold values used to decide if scaling should
* be done based on the absolute size of the largest matrix element.
* If AMAX > LARGE or AMAX < SMALL, scaling is done.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param s
* @param _s_offset
* @param scond
* @param amax
* @param equed
*
*/
abstract public void dlaqsp(java.lang.String uplo, int n, double[] ap, int _ap_offset, double[] s, int _s_offset, double scond, double amax, org.netlib.util.StringW equed);
/**
*
* ..
*
* Purpose
* =======
*
* DLAQSY equilibrates a symmetric matrix A using the scaling factors
* in the vector S.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* n by n upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading n by n lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, if EQUED = 'Y', the equilibrated matrix:
* diag(S) * A * diag(S).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(N,1).
*
* S (input) DOUBLE PRECISION array, dimension (N)
* The scale factors for A.
*
* SCOND (input) DOUBLE PRECISION
* Ratio of the smallest S(i) to the largest S(i).
*
* AMAX (input) DOUBLE PRECISION
* Absolute value of largest matrix entry.
*
* EQUED (output) CHARACTER*1
* Specifies whether or not equilibration was done.
* = 'N': No equilibration.
* = 'Y': Equilibration was done, i.e., A has been replaced by
* diag(S) * A * diag(S).
*
* Internal Parameters
* ===================
*
* THRESH is a threshold value used to decide if scaling should be done
* based on the ratio of the scaling factors. If SCOND < THRESH,
* scaling is done.
*
* LARGE and SMALL are threshold values used to decide if scaling should
* be done based on the absolute size of the largest matrix element.
* If AMAX > LARGE or AMAX < SMALL, scaling is done.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param lda
* @param s
* @param scond
* @param amax
* @param equed
*
*/
abstract public void dlaqsy(java.lang.String uplo, int n, double[] a, int lda, double[] s, double scond, double amax, org.netlib.util.StringW equed);
/**
*
* ..
*
* Purpose
* =======
*
* DLAQSY equilibrates a symmetric matrix A using the scaling factors
* in the vector S.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* n by n upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading n by n lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, if EQUED = 'Y', the equilibrated matrix:
* diag(S) * A * diag(S).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(N,1).
*
* S (input) DOUBLE PRECISION array, dimension (N)
* The scale factors for A.
*
* SCOND (input) DOUBLE PRECISION
* Ratio of the smallest S(i) to the largest S(i).
*
* AMAX (input) DOUBLE PRECISION
* Absolute value of largest matrix entry.
*
* EQUED (output) CHARACTER*1
* Specifies whether or not equilibration was done.
* = 'N': No equilibration.
* = 'Y': Equilibration was done, i.e., A has been replaced by
* diag(S) * A * diag(S).
*
* Internal Parameters
* ===================
*
* THRESH is a threshold value used to decide if scaling should be done
* based on the ratio of the scaling factors. If SCOND < THRESH,
* scaling is done.
*
* LARGE and SMALL are threshold values used to decide if scaling should
* be done based on the absolute size of the largest matrix element.
* If AMAX > LARGE or AMAX < SMALL, scaling is done.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param s
* @param _s_offset
* @param scond
* @param amax
* @param equed
*
*/
abstract public void dlaqsy(java.lang.String uplo, int n, double[] a, int _a_offset, int lda, double[] s, int _s_offset, double scond, double amax, org.netlib.util.StringW equed);
/**
*
* ..
*
* Purpose
* =======
*
* DLAQTR solves the real quasi-triangular system
*
* op(T)*p = scale*c, if LREAL = .TRUE.
*
* or the complex quasi-triangular systems
*
* op(T + iB)*(p+iq) = scale*(c+id), if LREAL = .FALSE.
*
* in real arithmetic, where T is upper quasi-triangular.
* If LREAL = .FALSE., then the first diagonal block of T must be
* 1 by 1, B is the specially structured matrix
*
* B = [ b(1) b(2) ... b(n) ]
* [ w ]
* [ w ]
* [ . ]
* [ w ]
*
* op(A) = A or A', A' denotes the conjugate transpose of
* matrix A.
*
* On input, X = [ c ]. On output, X = [ p ].
* [ d ] [ q ]
*
* This subroutine is designed for the condition number estimation
* in routine DTRSNA.
*
* Arguments
* =========
*
* LTRAN (input) LOGICAL
* On entry, LTRAN specifies the option of conjugate transpose:
* = .FALSE., op(T+i*B) = T+i*B,
* = .TRUE., op(T+i*B) = (T+i*B)'.
*
* LREAL (input) LOGICAL
* On entry, LREAL specifies the input matrix structure:
* = .FALSE., the input is complex
* = .TRUE., the input is real
*
* N (input) INTEGER
* On entry, N specifies the order of T+i*B. N >= 0.
*
* T (input) DOUBLE PRECISION array, dimension (LDT,N)
* On entry, T contains a matrix in Schur canonical form.
* If LREAL = .FALSE., then the first diagonal block of T mu
* be 1 by 1.
*
* LDT (input) INTEGER
* The leading dimension of the matrix T. LDT >= max(1,N).
*
* B (input) DOUBLE PRECISION array, dimension (N)
* On entry, B contains the elements to form the matrix
* B as described above.
* If LREAL = .TRUE., B is not referenced.
*
* W (input) DOUBLE PRECISION
* On entry, W is the diagonal element of the matrix B.
* If LREAL = .TRUE., W is not referenced.
*
* SCALE (output) DOUBLE PRECISION
* On exit, SCALE is the scale factor.
*
* X (input/output) DOUBLE PRECISION array, dimension (2*N)
* On entry, X contains the right hand side of the system.
* On exit, X is overwritten by the solution.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (N)
*
* INFO (output) INTEGER
* On exit, INFO is set to
* 0: successful exit.
* 1: the some diagonal 1 by 1 block has been perturbed by
* a small number SMIN to keep nonsingularity.
* 2: the some diagonal 2 by 2 block has been perturbed by
* a small number in DLALN2 to keep nonsingularity.
* NOTE: In the interests of speed, this routine does not
* check the inputs for errors.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param ltran
* @param lreal
* @param n
* @param t
* @param ldt
* @param b
* @param w
* @param scale
* @param x
* @param work
* @param info
*
*/
abstract public void dlaqtr(boolean ltran, boolean lreal, int n, double[] t, int ldt, double[] b, double w, org.netlib.util.doubleW scale, double[] x, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAQTR solves the real quasi-triangular system
*
* op(T)*p = scale*c, if LREAL = .TRUE.
*
* or the complex quasi-triangular systems
*
* op(T + iB)*(p+iq) = scale*(c+id), if LREAL = .FALSE.
*
* in real arithmetic, where T is upper quasi-triangular.
* If LREAL = .FALSE., then the first diagonal block of T must be
* 1 by 1, B is the specially structured matrix
*
* B = [ b(1) b(2) ... b(n) ]
* [ w ]
* [ w ]
* [ . ]
* [ w ]
*
* op(A) = A or A', A' denotes the conjugate transpose of
* matrix A.
*
* On input, X = [ c ]. On output, X = [ p ].
* [ d ] [ q ]
*
* This subroutine is designed for the condition number estimation
* in routine DTRSNA.
*
* Arguments
* =========
*
* LTRAN (input) LOGICAL
* On entry, LTRAN specifies the option of conjugate transpose:
* = .FALSE., op(T+i*B) = T+i*B,
* = .TRUE., op(T+i*B) = (T+i*B)'.
*
* LREAL (input) LOGICAL
* On entry, LREAL specifies the input matrix structure:
* = .FALSE., the input is complex
* = .TRUE., the input is real
*
* N (input) INTEGER
* On entry, N specifies the order of T+i*B. N >= 0.
*
* T (input) DOUBLE PRECISION array, dimension (LDT,N)
* On entry, T contains a matrix in Schur canonical form.
* If LREAL = .FALSE., then the first diagonal block of T mu
* be 1 by 1.
*
* LDT (input) INTEGER
* The leading dimension of the matrix T. LDT >= max(1,N).
*
* B (input) DOUBLE PRECISION array, dimension (N)
* On entry, B contains the elements to form the matrix
* B as described above.
* If LREAL = .TRUE., B is not referenced.
*
* W (input) DOUBLE PRECISION
* On entry, W is the diagonal element of the matrix B.
* If LREAL = .TRUE., W is not referenced.
*
* SCALE (output) DOUBLE PRECISION
* On exit, SCALE is the scale factor.
*
* X (input/output) DOUBLE PRECISION array, dimension (2*N)
* On entry, X contains the right hand side of the system.
* On exit, X is overwritten by the solution.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (N)
*
* INFO (output) INTEGER
* On exit, INFO is set to
* 0: successful exit.
* 1: the some diagonal 1 by 1 block has been perturbed by
* a small number SMIN to keep nonsingularity.
* 2: the some diagonal 2 by 2 block has been perturbed by
* a small number in DLALN2 to keep nonsingularity.
* NOTE: In the interests of speed, this routine does not
* check the inputs for errors.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param ltran
* @param lreal
* @param n
* @param t
* @param _t_offset
* @param ldt
* @param b
* @param _b_offset
* @param w
* @param scale
* @param x
* @param _x_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dlaqtr(boolean ltran, boolean lreal, int n, double[] t, int _t_offset, int ldt, double[] b, int _b_offset, double w, org.netlib.util.doubleW scale, double[] x, int _x_offset, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAR1V computes the (scaled) r-th column of the inverse of
* the sumbmatrix in rows B1 through BN of the tridiagonal matrix
* L D L^T - sigma I. When sigma is close to an eigenvalue, the
* computed vector is an accurate eigenvector. Usually, r corresponds
* to the index where the eigenvector is largest in magnitude.
* The following steps accomplish this computation :
* (a) Stationary qd transform, L D L^T - sigma I = L(+) D(+) L(+)^T,
* (b) Progressive qd transform, L D L^T - sigma I = U(-) D(-) U(-)^T,
* (c) Computation of the diagonal elements of the inverse of
* L D L^T - sigma I by combining the above transforms, and choosing
* r as the index where the diagonal of the inverse is (one of the)
* largest in magnitude.
* (d) Computation of the (scaled) r-th column of the inverse using the
* twisted factorization obtained by combining the top part of the
* the stationary and the bottom part of the progressive transform.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix L D L^T.
*
* B1 (input) INTEGER
* First index of the submatrix of L D L^T.
*
* BN (input) INTEGER
* Last index of the submatrix of L D L^T.
*
* LAMBDA (input) DOUBLE PRECISION
* The shift. In order to compute an accurate eigenvector,
* LAMBDA should be a good approximation to an eigenvalue
* of L D L^T.
*
* L (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) subdiagonal elements of the unit bidiagonal matrix
* L, in elements 1 to N-1.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The n diagonal elements of the diagonal matrix D.
*
* LD (input) DOUBLE PRECISION array, dimension (N-1)
* The n-1 elements L(i)*D(i).
*
* LLD (input) DOUBLE PRECISION array, dimension (N-1)
* The n-1 elements L(i)*L(i)*D(i).
*
* PIVMIN (input) DOUBLE PRECISION
* The minimum pivot in the Sturm sequence.
*
* GAPTOL (input) DOUBLE PRECISION
* Tolerance that indicates when eigenvector entries are neglig
* w.r.t. their contribution to the residual.
*
* Z (input/output) DOUBLE PRECISION array, dimension (N)
* On input, all entries of Z must be set to 0.
* On output, Z contains the (scaled) r-th column of the
* inverse. The scaling is such that Z(R) equals 1.
*
* WANTNC (input) LOGICAL
* Specifies whether NEGCNT has to be computed.
*
* NEGCNT (output) INTEGER
* If WANTNC is .TRUE. then NEGCNT = the number of pivots < piv
* in the matrix factorization L D L^T, and NEGCNT = -1 otherw
*
* ZTZ (output) DOUBLE PRECISION
* The square of the 2-norm of Z.
*
* MINGMA (output) DOUBLE PRECISION
* The reciprocal of the largest (in magnitude) diagonal
* element of the inverse of L D L^T - sigma I.
*
* R (input/output) INTEGER
* The twist index for the twisted factorization used to
* compute Z.
* On input, 0 <= R <= N. If R is input as 0, R is set to
* the index where (L D L^T - sigma I)^{-1} is largest
* in magnitude. If 1 <= R <= N, R is unchanged.
* On output, R contains the twist index used to compute Z.
* Ideally, R designates the position of the maximum entry in t
* eigenvector.
*
* ISUPPZ (output) INTEGER array, dimension (2)
* The support of the vector in Z, i.e., the vector Z is
* nonzero only in elements ISUPPZ(1) through ISUPPZ( 2 ).
*
* NRMINV (output) DOUBLE PRECISION
* NRMINV = 1/SQRT( ZTZ )
*
* RESID (output) DOUBLE PRECISION
* The residual of the FP vector.
* RESID = ABS( MINGMA )/SQRT( ZTZ )
*
* RQCORR (output) DOUBLE PRECISION
* The Rayleigh Quotient correction to LAMBDA.
* RQCORR = MINGMA*TMP
*
* WORK (workspace) DOUBLE PRECISION array, dimension (4*N)
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param b1
* @param bn
* @param lambda
* @param d
* @param l
* @param ld
* @param lld
* @param pivmin
* @param gaptol
* @param z
* @param wantnc
* @param negcnt
* @param ztz
* @param mingma
* @param r
* @param isuppz
* @param nrminv
* @param resid
* @param rqcorr
* @param work
*
*/
abstract public void dlar1v(int n, int b1, int bn, double lambda, double[] d, double[] l, double[] ld, double[] lld, double pivmin, double gaptol, double[] z, boolean wantnc, org.netlib.util.intW negcnt, org.netlib.util.doubleW ztz, org.netlib.util.doubleW mingma, org.netlib.util.intW r, int[] isuppz, org.netlib.util.doubleW nrminv, org.netlib.util.doubleW resid, org.netlib.util.doubleW rqcorr, double[] work);
/**
*
* ..
*
* Purpose
* =======
*
* DLAR1V computes the (scaled) r-th column of the inverse of
* the sumbmatrix in rows B1 through BN of the tridiagonal matrix
* L D L^T - sigma I. When sigma is close to an eigenvalue, the
* computed vector is an accurate eigenvector. Usually, r corresponds
* to the index where the eigenvector is largest in magnitude.
* The following steps accomplish this computation :
* (a) Stationary qd transform, L D L^T - sigma I = L(+) D(+) L(+)^T,
* (b) Progressive qd transform, L D L^T - sigma I = U(-) D(-) U(-)^T,
* (c) Computation of the diagonal elements of the inverse of
* L D L^T - sigma I by combining the above transforms, and choosing
* r as the index where the diagonal of the inverse is (one of the)
* largest in magnitude.
* (d) Computation of the (scaled) r-th column of the inverse using the
* twisted factorization obtained by combining the top part of the
* the stationary and the bottom part of the progressive transform.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix L D L^T.
*
* B1 (input) INTEGER
* First index of the submatrix of L D L^T.
*
* BN (input) INTEGER
* Last index of the submatrix of L D L^T.
*
* LAMBDA (input) DOUBLE PRECISION
* The shift. In order to compute an accurate eigenvector,
* LAMBDA should be a good approximation to an eigenvalue
* of L D L^T.
*
* L (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) subdiagonal elements of the unit bidiagonal matrix
* L, in elements 1 to N-1.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The n diagonal elements of the diagonal matrix D.
*
* LD (input) DOUBLE PRECISION array, dimension (N-1)
* The n-1 elements L(i)*D(i).
*
* LLD (input) DOUBLE PRECISION array, dimension (N-1)
* The n-1 elements L(i)*L(i)*D(i).
*
* PIVMIN (input) DOUBLE PRECISION
* The minimum pivot in the Sturm sequence.
*
* GAPTOL (input) DOUBLE PRECISION
* Tolerance that indicates when eigenvector entries are neglig
* w.r.t. their contribution to the residual.
*
* Z (input/output) DOUBLE PRECISION array, dimension (N)
* On input, all entries of Z must be set to 0.
* On output, Z contains the (scaled) r-th column of the
* inverse. The scaling is such that Z(R) equals 1.
*
* WANTNC (input) LOGICAL
* Specifies whether NEGCNT has to be computed.
*
* NEGCNT (output) INTEGER
* If WANTNC is .TRUE. then NEGCNT = the number of pivots < piv
* in the matrix factorization L D L^T, and NEGCNT = -1 otherw
*
* ZTZ (output) DOUBLE PRECISION
* The square of the 2-norm of Z.
*
* MINGMA (output) DOUBLE PRECISION
* The reciprocal of the largest (in magnitude) diagonal
* element of the inverse of L D L^T - sigma I.
*
* R (input/output) INTEGER
* The twist index for the twisted factorization used to
* compute Z.
* On input, 0 <= R <= N. If R is input as 0, R is set to
* the index where (L D L^T - sigma I)^{-1} is largest
* in magnitude. If 1 <= R <= N, R is unchanged.
* On output, R contains the twist index used to compute Z.
* Ideally, R designates the position of the maximum entry in t
* eigenvector.
*
* ISUPPZ (output) INTEGER array, dimension (2)
* The support of the vector in Z, i.e., the vector Z is
* nonzero only in elements ISUPPZ(1) through ISUPPZ( 2 ).
*
* NRMINV (output) DOUBLE PRECISION
* NRMINV = 1/SQRT( ZTZ )
*
* RESID (output) DOUBLE PRECISION
* The residual of the FP vector.
* RESID = ABS( MINGMA )/SQRT( ZTZ )
*
* RQCORR (output) DOUBLE PRECISION
* The Rayleigh Quotient correction to LAMBDA.
* RQCORR = MINGMA*TMP
*
* WORK (workspace) DOUBLE PRECISION array, dimension (4*N)
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param b1
* @param bn
* @param lambda
* @param d
* @param _d_offset
* @param l
* @param _l_offset
* @param ld
* @param _ld_offset
* @param lld
* @param _lld_offset
* @param pivmin
* @param gaptol
* @param z
* @param _z_offset
* @param wantnc
* @param negcnt
* @param ztz
* @param mingma
* @param r
* @param isuppz
* @param _isuppz_offset
* @param nrminv
* @param resid
* @param rqcorr
* @param work
* @param _work_offset
*
*/
abstract public void dlar1v(int n, int b1, int bn, double lambda, double[] d, int _d_offset, double[] l, int _l_offset, double[] ld, int _ld_offset, double[] lld, int _lld_offset, double pivmin, double gaptol, double[] z, int _z_offset, boolean wantnc, org.netlib.util.intW negcnt, org.netlib.util.doubleW ztz, org.netlib.util.doubleW mingma, org.netlib.util.intW r, int[] isuppz, int _isuppz_offset, org.netlib.util.doubleW nrminv, org.netlib.util.doubleW resid, org.netlib.util.doubleW rqcorr, double[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* DLAR2V applies a vector of real plane rotations from both sides to
* a sequence of 2-by-2 real symmetric matrices, defined by the elements
* of the vectors x, y and z. For i = 1,2,...,n
*
* ( x(i) z(i) ) := ( c(i) s(i) ) ( x(i) z(i) ) ( c(i) -s(i) )
* ( z(i) y(i) ) ( -s(i) c(i) ) ( z(i) y(i) ) ( s(i) c(i) )
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of plane rotations to be applied.
*
* X (input/output) DOUBLE PRECISION array,
* dimension (1+(N-1)*INCX)
* The vector x.
*
* Y (input/output) DOUBLE PRECISION array,
* dimension (1+(N-1)*INCX)
* The vector y.
*
* Z (input/output) DOUBLE PRECISION array,
* dimension (1+(N-1)*INCX)
* The vector z.
*
* INCX (input) INTEGER
* The increment between elements of X, Y and Z. INCX > 0.
*
* C (input) DOUBLE PRECISION array, dimension (1+(N-1)*INCC)
* The cosines of the plane rotations.
*
* S (input) DOUBLE PRECISION array, dimension (1+(N-1)*INCC)
* The sines of the plane rotations.
*
* INCC (input) INTEGER
* The increment between elements of C and S. INCC > 0.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n
* @param x
* @param y
* @param z
* @param incx
* @param c
* @param s
* @param incc
*
*/
abstract public void dlar2v(int n, double[] x, double[] y, double[] z, int incx, double[] c, double[] s, int incc);
/**
*
* ..
*
* Purpose
* =======
*
* DLAR2V applies a vector of real plane rotations from both sides to
* a sequence of 2-by-2 real symmetric matrices, defined by the elements
* of the vectors x, y and z. For i = 1,2,...,n
*
* ( x(i) z(i) ) := ( c(i) s(i) ) ( x(i) z(i) ) ( c(i) -s(i) )
* ( z(i) y(i) ) ( -s(i) c(i) ) ( z(i) y(i) ) ( s(i) c(i) )
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of plane rotations to be applied.
*
* X (input/output) DOUBLE PRECISION array,
* dimension (1+(N-1)*INCX)
* The vector x.
*
* Y (input/output) DOUBLE PRECISION array,
* dimension (1+(N-1)*INCX)
* The vector y.
*
* Z (input/output) DOUBLE PRECISION array,
* dimension (1+(N-1)*INCX)
* The vector z.
*
* INCX (input) INTEGER
* The increment between elements of X, Y and Z. INCX > 0.
*
* C (input) DOUBLE PRECISION array, dimension (1+(N-1)*INCC)
* The cosines of the plane rotations.
*
* S (input) DOUBLE PRECISION array, dimension (1+(N-1)*INCC)
* The sines of the plane rotations.
*
* INCC (input) INTEGER
* The increment between elements of C and S. INCC > 0.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n
* @param x
* @param _x_offset
* @param y
* @param _y_offset
* @param z
* @param _z_offset
* @param incx
* @param c
* @param _c_offset
* @param s
* @param _s_offset
* @param incc
*
*/
abstract public void dlar2v(int n, double[] x, int _x_offset, double[] y, int _y_offset, double[] z, int _z_offset, int incx, double[] c, int _c_offset, double[] s, int _s_offset, int incc);
/**
*
* ..
*
* Purpose
* =======
*
* DLARF applies a real elementary reflector H to a real m by n matrix
* C, from either the left or the right. H is represented in the form
*
* H = I - tau * v * v'
*
* where tau is a real scalar and v is a real vector.
*
* If tau = 0, then H is taken to be the unit matrix.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': form H * C
* = 'R': form C * H
*
* M (input) INTEGER
* The number of rows of the matrix C.
*
* N (input) INTEGER
* The number of columns of the matrix C.
*
* V (input) DOUBLE PRECISION array, dimension
* (1 + (M-1)*abs(INCV)) if SIDE = 'L'
* or (1 + (N-1)*abs(INCV)) if SIDE = 'R'
* The vector v in the representation of H. V is not used if
* TAU = 0.
*
* INCV (input) INTEGER
* The increment between elements of v. INCV <> 0.
*
* TAU (input) DOUBLE PRECISION
* The value tau in the representation of H.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the m by n matrix C.
* On exit, C is overwritten by the matrix H * C if SIDE = 'L',
* or C * H if SIDE = 'R'.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) DOUBLE PRECISION array, dimension
* (N) if SIDE = 'L'
* or (M) if SIDE = 'R'
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param m
* @param n
* @param v
* @param incv
* @param tau
* @param c
* @param Ldc
* @param work
*
*/
abstract public void dlarf(java.lang.String side, int m, int n, double[] v, int incv, double tau, double[] c, int Ldc, double[] work);
/**
*
* ..
*
* Purpose
* =======
*
* DLARF applies a real elementary reflector H to a real m by n matrix
* C, from either the left or the right. H is represented in the form
*
* H = I - tau * v * v'
*
* where tau is a real scalar and v is a real vector.
*
* If tau = 0, then H is taken to be the unit matrix.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': form H * C
* = 'R': form C * H
*
* M (input) INTEGER
* The number of rows of the matrix C.
*
* N (input) INTEGER
* The number of columns of the matrix C.
*
* V (input) DOUBLE PRECISION array, dimension
* (1 + (M-1)*abs(INCV)) if SIDE = 'L'
* or (1 + (N-1)*abs(INCV)) if SIDE = 'R'
* The vector v in the representation of H. V is not used if
* TAU = 0.
*
* INCV (input) INTEGER
* The increment between elements of v. INCV <> 0.
*
* TAU (input) DOUBLE PRECISION
* The value tau in the representation of H.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the m by n matrix C.
* On exit, C is overwritten by the matrix H * C if SIDE = 'L',
* or C * H if SIDE = 'R'.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) DOUBLE PRECISION array, dimension
* (N) if SIDE = 'L'
* or (M) if SIDE = 'R'
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param m
* @param n
* @param v
* @param _v_offset
* @param incv
* @param tau
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
*
*/
abstract public void dlarf(java.lang.String side, int m, int n, double[] v, int _v_offset, int incv, double tau, double[] c, int _c_offset, int Ldc, double[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* DLARFB applies a real block reflector H or its transpose H' to a
* real m by n matrix C, from either the left or the right.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply H or H' from the Left
* = 'R': apply H or H' from the Right
*
* TRANS (input) CHARACTER*1
* = 'N': apply H (No transpose)
* = 'T': apply H' (Transpose)
*
* DIRECT (input) CHARACTER*1
* Indicates how H is formed from a product of elementary
* reflectors
* = 'F': H = H(1) H(2) . . . H(k) (Forward)
* = 'B': H = H(k) . . . H(2) H(1) (Backward)
*
* STOREV (input) CHARACTER*1
* Indicates how the vectors which define the elementary
* reflectors are stored:
* = 'C': Columnwise
* = 'R': Rowwise
*
* M (input) INTEGER
* The number of rows of the matrix C.
*
* N (input) INTEGER
* The number of columns of the matrix C.
*
* K (input) INTEGER
* The order of the matrix T (= the number of elementary
* reflectors whose product defines the block reflector).
*
* V (input) DOUBLE PRECISION array, dimension
* (LDV,K) if STOREV = 'C'
* (LDV,M) if STOREV = 'R' and SIDE = 'L'
* (LDV,N) if STOREV = 'R' and SIDE = 'R'
* The matrix V. See further details.
*
* LDV (input) INTEGER
* The leading dimension of the array V.
* If STOREV = 'C' and SIDE = 'L', LDV >= max(1,M);
* if STOREV = 'C' and SIDE = 'R', LDV >= max(1,N);
* if STOREV = 'R', LDV >= K.
*
* T (input) DOUBLE PRECISION array, dimension (LDT,K)
* The triangular k by k matrix T in the representation of the
* block reflector.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= K.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the m by n matrix C.
* On exit, C is overwritten by H*C or H'*C or C*H or C*H'.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDA >= max(1,M).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (LDWORK,K)
*
* LDWORK (input) INTEGER
* The leading dimension of the array WORK.
* If SIDE = 'L', LDWORK >= max(1,N);
* if SIDE = 'R', LDWORK >= max(1,M).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param direct
* @param storev
* @param m
* @param n
* @param k
* @param v
* @param ldv
* @param t
* @param ldt
* @param c
* @param Ldc
* @param work
* @param ldwork
*
*/
abstract public void dlarfb(java.lang.String side, java.lang.String trans, java.lang.String direct, java.lang.String storev, int m, int n, int k, double[] v, int ldv, double[] t, int ldt, double[] c, int Ldc, double[] work, int ldwork);
/**
*
* ..
*
* Purpose
* =======
*
* DLARFB applies a real block reflector H or its transpose H' to a
* real m by n matrix C, from either the left or the right.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply H or H' from the Left
* = 'R': apply H or H' from the Right
*
* TRANS (input) CHARACTER*1
* = 'N': apply H (No transpose)
* = 'T': apply H' (Transpose)
*
* DIRECT (input) CHARACTER*1
* Indicates how H is formed from a product of elementary
* reflectors
* = 'F': H = H(1) H(2) . . . H(k) (Forward)
* = 'B': H = H(k) . . . H(2) H(1) (Backward)
*
* STOREV (input) CHARACTER*1
* Indicates how the vectors which define the elementary
* reflectors are stored:
* = 'C': Columnwise
* = 'R': Rowwise
*
* M (input) INTEGER
* The number of rows of the matrix C.
*
* N (input) INTEGER
* The number of columns of the matrix C.
*
* K (input) INTEGER
* The order of the matrix T (= the number of elementary
* reflectors whose product defines the block reflector).
*
* V (input) DOUBLE PRECISION array, dimension
* (LDV,K) if STOREV = 'C'
* (LDV,M) if STOREV = 'R' and SIDE = 'L'
* (LDV,N) if STOREV = 'R' and SIDE = 'R'
* The matrix V. See further details.
*
* LDV (input) INTEGER
* The leading dimension of the array V.
* If STOREV = 'C' and SIDE = 'L', LDV >= max(1,M);
* if STOREV = 'C' and SIDE = 'R', LDV >= max(1,N);
* if STOREV = 'R', LDV >= K.
*
* T (input) DOUBLE PRECISION array, dimension (LDT,K)
* The triangular k by k matrix T in the representation of the
* block reflector.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= K.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the m by n matrix C.
* On exit, C is overwritten by H*C or H'*C or C*H or C*H'.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDA >= max(1,M).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (LDWORK,K)
*
* LDWORK (input) INTEGER
* The leading dimension of the array WORK.
* If SIDE = 'L', LDWORK >= max(1,N);
* if SIDE = 'R', LDWORK >= max(1,M).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param direct
* @param storev
* @param m
* @param n
* @param k
* @param v
* @param _v_offset
* @param ldv
* @param t
* @param _t_offset
* @param ldt
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param ldwork
*
*/
abstract public void dlarfb(java.lang.String side, java.lang.String trans, java.lang.String direct, java.lang.String storev, int m, int n, int k, double[] v, int _v_offset, int ldv, double[] t, int _t_offset, int ldt, double[] c, int _c_offset, int Ldc, double[] work, int _work_offset, int ldwork);
/**
*
* ..
*
* Purpose
* =======
*
* DLARFG generates a real elementary reflector H of order n, such
* that
*
* H * ( alpha ) = ( beta ), H' * H = I.
* ( x ) ( 0 )
*
* where alpha and beta are scalars, and x is an (n-1)-element real
* vector. H is represented in the form
*
* H = I - tau * ( 1 ) * ( 1 v' ) ,
* ( v )
*
* where tau is a real scalar and v is a real (n-1)-element
* vector.
*
* If the elements of x are all zero, then tau = 0 and H is taken to be
* the unit matrix.
*
* Otherwise 1 <= tau <= 2.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the elementary reflector.
*
* ALPHA (input/output) DOUBLE PRECISION
* On entry, the value alpha.
* On exit, it is overwritten with the value beta.
*
* X (input/output) DOUBLE PRECISION array, dimension
* (1+(N-2)*abs(INCX))
* On entry, the vector x.
* On exit, it is overwritten with the vector v.
*
* INCX (input) INTEGER
* The increment between elements of X. INCX > 0.
*
* TAU (output) DOUBLE PRECISION
* The value tau.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param alpha
* @param x
* @param incx
* @param tau
*
*/
abstract public void dlarfg(int n, org.netlib.util.doubleW alpha, double[] x, int incx, org.netlib.util.doubleW tau);
/**
*
* ..
*
* Purpose
* =======
*
* DLARFG generates a real elementary reflector H of order n, such
* that
*
* H * ( alpha ) = ( beta ), H' * H = I.
* ( x ) ( 0 )
*
* where alpha and beta are scalars, and x is an (n-1)-element real
* vector. H is represented in the form
*
* H = I - tau * ( 1 ) * ( 1 v' ) ,
* ( v )
*
* where tau is a real scalar and v is a real (n-1)-element
* vector.
*
* If the elements of x are all zero, then tau = 0 and H is taken to be
* the unit matrix.
*
* Otherwise 1 <= tau <= 2.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the elementary reflector.
*
* ALPHA (input/output) DOUBLE PRECISION
* On entry, the value alpha.
* On exit, it is overwritten with the value beta.
*
* X (input/output) DOUBLE PRECISION array, dimension
* (1+(N-2)*abs(INCX))
* On entry, the vector x.
* On exit, it is overwritten with the vector v.
*
* INCX (input) INTEGER
* The increment between elements of X. INCX > 0.
*
* TAU (output) DOUBLE PRECISION
* The value tau.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param alpha
* @param x
* @param _x_offset
* @param incx
* @param tau
*
*/
abstract public void dlarfg(int n, org.netlib.util.doubleW alpha, double[] x, int _x_offset, int incx, org.netlib.util.doubleW tau);
/**
*
* ..
*
* Purpose
* =======
*
* DLARFT forms the triangular factor T of a real block reflector H
* of order n, which is defined as a product of k elementary reflectors.
*
* If DIRECT = 'F', H = H(1) H(2) . . . H(k) and T is upper triangular;
*
* If DIRECT = 'B', H = H(k) . . . H(2) H(1) and T is lower triangular.
*
* If STOREV = 'C', the vector which defines the elementary reflector
* H(i) is stored in the i-th column of the array V, and
*
* H = I - V * T * V'
*
* If STOREV = 'R', the vector which defines the elementary reflector
* H(i) is stored in the i-th row of the array V, and
*
* H = I - V' * T * V
*
* Arguments
* =========
*
* DIRECT (input) CHARACTER*1
* Specifies the order in which the elementary reflectors are
* multiplied to form the block reflector:
* = 'F': H = H(1) H(2) . . . H(k) (Forward)
* = 'B': H = H(k) . . . H(2) H(1) (Backward)
*
* STOREV (input) CHARACTER*1
* Specifies how the vectors which define the elementary
* reflectors are stored (see also Further Details):
* = 'C': columnwise
* = 'R': rowwise
*
* N (input) INTEGER
* The order of the block reflector H. N >= 0.
*
* K (input) INTEGER
* The order of the triangular factor T (= the number of
* elementary reflectors). K >= 1.
*
* V (input/output) DOUBLE PRECISION array, dimension
* (LDV,K) if STOREV = 'C'
* (LDV,N) if STOREV = 'R'
* The matrix V. See further details.
*
* LDV (input) INTEGER
* The leading dimension of the array V.
* If STOREV = 'C', LDV >= max(1,N); if STOREV = 'R', LDV >= K.
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i).
*
* T (output) DOUBLE PRECISION array, dimension (LDT,K)
* The k by k triangular factor T of the block reflector.
* If DIRECT = 'F', T is upper triangular; if DIRECT = 'B', T is
* lower triangular. The rest of the array is not used.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= K.
*
* Further Details
* ===============
*
* The shape of the matrix V and the storage of the vectors which define
* the H(i) is best illustrated by the following example with n = 5 and
* k = 3. The elements equal to 1 are not stored; the corresponding
* array elements are modified but restored on exit. The rest of the
* array is not used.
*
* DIRECT = 'F' and STOREV = 'C': DIRECT = 'F' and STOREV = 'R':
*
* V = ( 1 ) V = ( 1 v1 v1 v1 v1 )
* ( v1 1 ) ( 1 v2 v2 v2 )
* ( v1 v2 1 ) ( 1 v3 v3 )
* ( v1 v2 v3 )
* ( v1 v2 v3 )
*
* DIRECT = 'B' and STOREV = 'C': DIRECT = 'B' and STOREV = 'R':
*
* V = ( v1 v2 v3 ) V = ( v1 v1 1 )
* ( v1 v2 v3 ) ( v2 v2 v2 1 )
* ( 1 v2 v3 ) ( v3 v3 v3 v3 1 )
* ( 1 v3 )
* ( 1 )
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param direct
* @param storev
* @param n
* @param k
* @param v
* @param ldv
* @param tau
* @param t
* @param ldt
*
*/
abstract public void dlarft(java.lang.String direct, java.lang.String storev, int n, int k, double[] v, int ldv, double[] tau, double[] t, int ldt);
/**
*
* ..
*
* Purpose
* =======
*
* DLARFT forms the triangular factor T of a real block reflector H
* of order n, which is defined as a product of k elementary reflectors.
*
* If DIRECT = 'F', H = H(1) H(2) . . . H(k) and T is upper triangular;
*
* If DIRECT = 'B', H = H(k) . . . H(2) H(1) and T is lower triangular.
*
* If STOREV = 'C', the vector which defines the elementary reflector
* H(i) is stored in the i-th column of the array V, and
*
* H = I - V * T * V'
*
* If STOREV = 'R', the vector which defines the elementary reflector
* H(i) is stored in the i-th row of the array V, and
*
* H = I - V' * T * V
*
* Arguments
* =========
*
* DIRECT (input) CHARACTER*1
* Specifies the order in which the elementary reflectors are
* multiplied to form the block reflector:
* = 'F': H = H(1) H(2) . . . H(k) (Forward)
* = 'B': H = H(k) . . . H(2) H(1) (Backward)
*
* STOREV (input) CHARACTER*1
* Specifies how the vectors which define the elementary
* reflectors are stored (see also Further Details):
* = 'C': columnwise
* = 'R': rowwise
*
* N (input) INTEGER
* The order of the block reflector H. N >= 0.
*
* K (input) INTEGER
* The order of the triangular factor T (= the number of
* elementary reflectors). K >= 1.
*
* V (input/output) DOUBLE PRECISION array, dimension
* (LDV,K) if STOREV = 'C'
* (LDV,N) if STOREV = 'R'
* The matrix V. See further details.
*
* LDV (input) INTEGER
* The leading dimension of the array V.
* If STOREV = 'C', LDV >= max(1,N); if STOREV = 'R', LDV >= K.
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i).
*
* T (output) DOUBLE PRECISION array, dimension (LDT,K)
* The k by k triangular factor T of the block reflector.
* If DIRECT = 'F', T is upper triangular; if DIRECT = 'B', T is
* lower triangular. The rest of the array is not used.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= K.
*
* Further Details
* ===============
*
* The shape of the matrix V and the storage of the vectors which define
* the H(i) is best illustrated by the following example with n = 5 and
* k = 3. The elements equal to 1 are not stored; the corresponding
* array elements are modified but restored on exit. The rest of the
* array is not used.
*
* DIRECT = 'F' and STOREV = 'C': DIRECT = 'F' and STOREV = 'R':
*
* V = ( 1 ) V = ( 1 v1 v1 v1 v1 )
* ( v1 1 ) ( 1 v2 v2 v2 )
* ( v1 v2 1 ) ( 1 v3 v3 )
* ( v1 v2 v3 )
* ( v1 v2 v3 )
*
* DIRECT = 'B' and STOREV = 'C': DIRECT = 'B' and STOREV = 'R':
*
* V = ( v1 v2 v3 ) V = ( v1 v1 1 )
* ( v1 v2 v3 ) ( v2 v2 v2 1 )
* ( 1 v2 v3 ) ( v3 v3 v3 v3 1 )
* ( 1 v3 )
* ( 1 )
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param direct
* @param storev
* @param n
* @param k
* @param v
* @param _v_offset
* @param ldv
* @param tau
* @param _tau_offset
* @param t
* @param _t_offset
* @param ldt
*
*/
abstract public void dlarft(java.lang.String direct, java.lang.String storev, int n, int k, double[] v, int _v_offset, int ldv, double[] tau, int _tau_offset, double[] t, int _t_offset, int ldt);
/**
*
* ..
*
* Purpose
* =======
*
* DLARFX applies a real elementary reflector H to a real m by n
* matrix C, from either the left or the right. H is represented in the
* form
*
* H = I - tau * v * v'
*
* where tau is a real scalar and v is a real vector.
*
* If tau = 0, then H is taken to be the unit matrix
*
* This version uses inline code if H has order < 11.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': form H * C
* = 'R': form C * H
*
* M (input) INTEGER
* The number of rows of the matrix C.
*
* N (input) INTEGER
* The number of columns of the matrix C.
*
* V (input) DOUBLE PRECISION array, dimension (M) if SIDE = 'L'
* or (N) if SIDE = 'R'
* The vector v in the representation of H.
*
* TAU (input) DOUBLE PRECISION
* The value tau in the representation of H.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the m by n matrix C.
* On exit, C is overwritten by the matrix H * C if SIDE = 'L',
* or C * H if SIDE = 'R'.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDA >= (1,M).
*
* WORK (workspace) DOUBLE PRECISION array, dimension
* (N) if SIDE = 'L'
* or (M) if SIDE = 'R'
* WORK is not referenced if H has order < 11.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param m
* @param n
* @param v
* @param tau
* @param c
* @param Ldc
* @param work
*
*/
abstract public void dlarfx(java.lang.String side, int m, int n, double[] v, double tau, double[] c, int Ldc, double[] work);
/**
*
* ..
*
* Purpose
* =======
*
* DLARFX applies a real elementary reflector H to a real m by n
* matrix C, from either the left or the right. H is represented in the
* form
*
* H = I - tau * v * v'
*
* where tau is a real scalar and v is a real vector.
*
* If tau = 0, then H is taken to be the unit matrix
*
* This version uses inline code if H has order < 11.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': form H * C
* = 'R': form C * H
*
* M (input) INTEGER
* The number of rows of the matrix C.
*
* N (input) INTEGER
* The number of columns of the matrix C.
*
* V (input) DOUBLE PRECISION array, dimension (M) if SIDE = 'L'
* or (N) if SIDE = 'R'
* The vector v in the representation of H.
*
* TAU (input) DOUBLE PRECISION
* The value tau in the representation of H.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the m by n matrix C.
* On exit, C is overwritten by the matrix H * C if SIDE = 'L',
* or C * H if SIDE = 'R'.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDA >= (1,M).
*
* WORK (workspace) DOUBLE PRECISION array, dimension
* (N) if SIDE = 'L'
* or (M) if SIDE = 'R'
* WORK is not referenced if H has order < 11.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param m
* @param n
* @param v
* @param _v_offset
* @param tau
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
*
*/
abstract public void dlarfx(java.lang.String side, int m, int n, double[] v, int _v_offset, double tau, double[] c, int _c_offset, int Ldc, double[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* DLARGV generates a vector of real plane rotations, determined by
* elements of the real vectors x and y. For i = 1,2,...,n
*
* ( c(i) s(i) ) ( x(i) ) = ( a(i) )
* ( -s(i) c(i) ) ( y(i) ) = ( 0 )
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of plane rotations to be generated.
*
* X (input/output) DOUBLE PRECISION array,
* dimension (1+(N-1)*INCX)
* On entry, the vector x.
* On exit, x(i) is overwritten by a(i), for i = 1,...,n.
*
* INCX (input) INTEGER
* The increment between elements of X. INCX > 0.
*
* Y (input/output) DOUBLE PRECISION array,
* dimension (1+(N-1)*INCY)
* On entry, the vector y.
* On exit, the sines of the plane rotations.
*
* INCY (input) INTEGER
* The increment between elements of Y. INCY > 0.
*
* C (output) DOUBLE PRECISION array, dimension (1+(N-1)*INCC)
* The cosines of the plane rotations.
*
* INCC (input) INTEGER
* The increment between elements of C. INCC > 0.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param x
* @param incx
* @param y
* @param incy
* @param c
* @param incc
*
*/
abstract public void dlargv(int n, double[] x, int incx, double[] y, int incy, double[] c, int incc);
/**
*
* ..
*
* Purpose
* =======
*
* DLARGV generates a vector of real plane rotations, determined by
* elements of the real vectors x and y. For i = 1,2,...,n
*
* ( c(i) s(i) ) ( x(i) ) = ( a(i) )
* ( -s(i) c(i) ) ( y(i) ) = ( 0 )
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of plane rotations to be generated.
*
* X (input/output) DOUBLE PRECISION array,
* dimension (1+(N-1)*INCX)
* On entry, the vector x.
* On exit, x(i) is overwritten by a(i), for i = 1,...,n.
*
* INCX (input) INTEGER
* The increment between elements of X. INCX > 0.
*
* Y (input/output) DOUBLE PRECISION array,
* dimension (1+(N-1)*INCY)
* On entry, the vector y.
* On exit, the sines of the plane rotations.
*
* INCY (input) INTEGER
* The increment between elements of Y. INCY > 0.
*
* C (output) DOUBLE PRECISION array, dimension (1+(N-1)*INCC)
* The cosines of the plane rotations.
*
* INCC (input) INTEGER
* The increment between elements of C. INCC > 0.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param x
* @param _x_offset
* @param incx
* @param y
* @param _y_offset
* @param incy
* @param c
* @param _c_offset
* @param incc
*
*/
abstract public void dlargv(int n, double[] x, int _x_offset, int incx, double[] y, int _y_offset, int incy, double[] c, int _c_offset, int incc);
/**
*
* ..
*
* Purpose
* =======
*
* DLARNV returns a vector of n random real numbers from a uniform or
* normal distribution.
*
* Arguments
* =========
*
* IDIST (input) INTEGER
* Specifies the distribution of the random numbers:
* = 1: uniform (0,1)
* = 2: uniform (-1,1)
* = 3: normal (0,1)
*
* ISEED (input/output) INTEGER array, dimension (4)
* On entry, the seed of the random number generator; the array
* elements must be between 0 and 4095, and ISEED(4) must be
* odd.
* On exit, the seed is updated.
*
* N (input) INTEGER
* The number of random numbers to be generated.
*
* X (output) DOUBLE PRECISION array, dimension (N)
* The generated random numbers.
*
* Further Details
* ===============
*
* This routine calls the auxiliary routine DLARUV to generate random
* real numbers from a uniform (0,1) distribution, in batches of up to
* 128 using vectorisable code. The Box-Muller method is used to
* transform numbers from a uniform to a normal distribution.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param idist
* @param iseed
* @param n
* @param x
*
*/
abstract public void dlarnv(int idist, int[] iseed, int n, double[] x);
/**
*
* ..
*
* Purpose
* =======
*
* DLARNV returns a vector of n random real numbers from a uniform or
* normal distribution.
*
* Arguments
* =========
*
* IDIST (input) INTEGER
* Specifies the distribution of the random numbers:
* = 1: uniform (0,1)
* = 2: uniform (-1,1)
* = 3: normal (0,1)
*
* ISEED (input/output) INTEGER array, dimension (4)
* On entry, the seed of the random number generator; the array
* elements must be between 0 and 4095, and ISEED(4) must be
* odd.
* On exit, the seed is updated.
*
* N (input) INTEGER
* The number of random numbers to be generated.
*
* X (output) DOUBLE PRECISION array, dimension (N)
* The generated random numbers.
*
* Further Details
* ===============
*
* This routine calls the auxiliary routine DLARUV to generate random
* real numbers from a uniform (0,1) distribution, in batches of up to
* 128 using vectorisable code. The Box-Muller method is used to
* transform numbers from a uniform to a normal distribution.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param idist
* @param iseed
* @param _iseed_offset
* @param n
* @param x
* @param _x_offset
*
*/
abstract public void dlarnv(int idist, int[] iseed, int _iseed_offset, int n, double[] x, int _x_offset);
/**
*
* ..
*
* Purpose
* =======
*
* Compute the splitting points with threshold SPLTOL.
* DLARRA sets any "small" off-diagonal elements to zero.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix. N > 0.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* On entry, the N diagonal elements of the tridiagonal
* matrix T.
*
* E (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the first (N-1) entries contain the subdiagonal
* elements of the tridiagonal matrix T; E(N) need not be set.
* On exit, the entries E( ISPLIT( I ) ), 1 <= I <= NSPLIT,
* are set to zero, the other entries of E are untouched.
*
* E2 (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the first (N-1) entries contain the SQUARES of the
* subdiagonal elements of the tridiagonal matrix T;
* E2(N) need not be set.
* On exit, the entries E2( ISPLIT( I ) ),
* 1 <= I <= NSPLIT, have been set to zero
*
* SPLTOL (input) DOUBLE PRECISION
* The threshold for splitting. Two criteria can be used:
* SPLTOL<0 : criterion based on absolute off-diagonal value
* SPLTOL>0 : criterion that preserves relative accuracy
*
* TNRM (input) DOUBLE PRECISION
* The norm of the matrix.
*
* NSPLIT (output) INTEGER
* The number of blocks T splits into. 1 <= NSPLIT <= N.
*
* ISPLIT (output) INTEGER array, dimension (N)
* The splitting points, at which T breaks up into blocks.
* The first block consists of rows/columns 1 to ISPLIT(1),
* the second of rows/columns ISPLIT(1)+1 through ISPLIT(2),
* etc., and the NSPLIT-th consists of rows/columns
* ISPLIT(NSPLIT-1)+1 through ISPLIT(NSPLIT)=N.
*
*
* INFO (output) INTEGER
* = 0: successful exit
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param e
* @param e2
* @param spltol
* @param tnrm
* @param nsplit
* @param isplit
* @param info
*
*/
abstract public void dlarra(int n, double[] d, double[] e, double[] e2, double spltol, double tnrm, org.netlib.util.intW nsplit, int[] isplit, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* Compute the splitting points with threshold SPLTOL.
* DLARRA sets any "small" off-diagonal elements to zero.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix. N > 0.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* On entry, the N diagonal elements of the tridiagonal
* matrix T.
*
* E (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the first (N-1) entries contain the subdiagonal
* elements of the tridiagonal matrix T; E(N) need not be set.
* On exit, the entries E( ISPLIT( I ) ), 1 <= I <= NSPLIT,
* are set to zero, the other entries of E are untouched.
*
* E2 (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the first (N-1) entries contain the SQUARES of the
* subdiagonal elements of the tridiagonal matrix T;
* E2(N) need not be set.
* On exit, the entries E2( ISPLIT( I ) ),
* 1 <= I <= NSPLIT, have been set to zero
*
* SPLTOL (input) DOUBLE PRECISION
* The threshold for splitting. Two criteria can be used:
* SPLTOL<0 : criterion based on absolute off-diagonal value
* SPLTOL>0 : criterion that preserves relative accuracy
*
* TNRM (input) DOUBLE PRECISION
* The norm of the matrix.
*
* NSPLIT (output) INTEGER
* The number of blocks T splits into. 1 <= NSPLIT <= N.
*
* ISPLIT (output) INTEGER array, dimension (N)
* The splitting points, at which T breaks up into blocks.
* The first block consists of rows/columns 1 to ISPLIT(1),
* the second of rows/columns ISPLIT(1)+1 through ISPLIT(2),
* etc., and the NSPLIT-th consists of rows/columns
* ISPLIT(NSPLIT-1)+1 through ISPLIT(NSPLIT)=N.
*
*
* INFO (output) INTEGER
* = 0: successful exit
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param e2
* @param _e2_offset
* @param spltol
* @param tnrm
* @param nsplit
* @param isplit
* @param _isplit_offset
* @param info
*
*/
abstract public void dlarra(int n, double[] d, int _d_offset, double[] e, int _e_offset, double[] e2, int _e2_offset, double spltol, double tnrm, org.netlib.util.intW nsplit, int[] isplit, int _isplit_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* Given the relatively robust representation(RRR) L D L^T, DLARRB
* does "limited" bisection to refine the eigenvalues of L D L^T,
* W( IFIRST-OFFSET ) through W( ILAST-OFFSET ), to more accuracy. Initi
* guesses for these eigenvalues are input in W, the corresponding estim
* of the error in these guesses and their gaps are input in WERR
* and WGAP, respectively. During bisection, intervals
* [left, right] are maintained by storing their mid-points and
* semi-widths in the arrays W and WERR respectively.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The N diagonal elements of the diagonal matrix D.
*
* LLD (input) DOUBLE PRECISION array, dimension (N-1)
* The (N-1) elements L(i)*L(i)*D(i).
*
* IFIRST (input) INTEGER
* The index of the first eigenvalue to be computed.
*
* ILAST (input) INTEGER
* The index of the last eigenvalue to be computed.
*
* RTOL1 (input) DOUBLE PRECISION
* RTOL2 (input) DOUBLE PRECISION
* Tolerance for the convergence of the bisection intervals.
* An interval [LEFT,RIGHT] has converged if
* RIGHT-LEFT.LT.MAX( RTOL1*GAP, RTOL2*MAX(|LEFT|,|RIGHT|) )
* where GAP is the (estimated) distance to the nearest
* eigenvalue.
*
* OFFSET (input) INTEGER
* Offset for the arrays W, WGAP and WERR, i.e., the IFIRST-OFFS
* through ILAST-OFFSET elements of these arrays are to be used.
*
* W (input/output) DOUBLE PRECISION array, dimension (N)
* On input, W( IFIRST-OFFSET ) through W( ILAST-OFFSET ) are
* estimates of the eigenvalues of L D L^T indexed IFIRST throug
* ILAST.
* On output, these estimates are refined.
*
* WGAP (input/output) DOUBLE PRECISION array, dimension (N-1)
* On input, the (estimated) gaps between consecutive
* eigenvalues of L D L^T, i.e., WGAP(I-OFFSET) is the gap betwe
* eigenvalues I and I+1. Note that if IFIRST.EQ.ILAST
* then WGAP(IFIRST-OFFSET) must be set to ZERO.
* On output, these gaps are refined.
*
* WERR (input/output) DOUBLE PRECISION array, dimension (N)
* On input, WERR( IFIRST-OFFSET ) through WERR( ILAST-OFFSET )
* the errors in the estimates of the corresponding elements in
* On output, these errors are refined.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (2*N)
* Workspace.
*
* IWORK (workspace) INTEGER array, dimension (2*N)
* Workspace.
*
* PIVMIN (input) DOUBLE PRECISION
* The minimum pivot in the Sturm sequence.
*
* SPDIAM (input) DOUBLE PRECISION
* The spectral diameter of the matrix.
*
* TWIST (input) INTEGER
* The twist index for the twisted factorization that is used
* for the negcount.
* TWIST = N: Compute negcount from L D L^T - LAMBDA I = L+ D+ L
* TWIST = 1: Compute negcount from L D L^T - LAMBDA I = U- D- U
* TWIST = R: Compute negcount from L D L^T - LAMBDA I = N(r) D(
*
* INFO (output) INTEGER
* Error flag.
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param lld
* @param ifirst
* @param ilast
* @param rtol1
* @param rtol2
* @param offset
* @param w
* @param wgap
* @param werr
* @param work
* @param iwork
* @param pivmin
* @param spdiam
* @param twist
* @param info
*
*/
abstract public void dlarrb(int n, double[] d, double[] lld, int ifirst, int ilast, double rtol1, double rtol2, int offset, double[] w, double[] wgap, double[] werr, double[] work, int[] iwork, double pivmin, double spdiam, int twist, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* Given the relatively robust representation(RRR) L D L^T, DLARRB
* does "limited" bisection to refine the eigenvalues of L D L^T,
* W( IFIRST-OFFSET ) through W( ILAST-OFFSET ), to more accuracy. Initi
* guesses for these eigenvalues are input in W, the corresponding estim
* of the error in these guesses and their gaps are input in WERR
* and WGAP, respectively. During bisection, intervals
* [left, right] are maintained by storing their mid-points and
* semi-widths in the arrays W and WERR respectively.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The N diagonal elements of the diagonal matrix D.
*
* LLD (input) DOUBLE PRECISION array, dimension (N-1)
* The (N-1) elements L(i)*L(i)*D(i).
*
* IFIRST (input) INTEGER
* The index of the first eigenvalue to be computed.
*
* ILAST (input) INTEGER
* The index of the last eigenvalue to be computed.
*
* RTOL1 (input) DOUBLE PRECISION
* RTOL2 (input) DOUBLE PRECISION
* Tolerance for the convergence of the bisection intervals.
* An interval [LEFT,RIGHT] has converged if
* RIGHT-LEFT.LT.MAX( RTOL1*GAP, RTOL2*MAX(|LEFT|,|RIGHT|) )
* where GAP is the (estimated) distance to the nearest
* eigenvalue.
*
* OFFSET (input) INTEGER
* Offset for the arrays W, WGAP and WERR, i.e., the IFIRST-OFFS
* through ILAST-OFFSET elements of these arrays are to be used.
*
* W (input/output) DOUBLE PRECISION array, dimension (N)
* On input, W( IFIRST-OFFSET ) through W( ILAST-OFFSET ) are
* estimates of the eigenvalues of L D L^T indexed IFIRST throug
* ILAST.
* On output, these estimates are refined.
*
* WGAP (input/output) DOUBLE PRECISION array, dimension (N-1)
* On input, the (estimated) gaps between consecutive
* eigenvalues of L D L^T, i.e., WGAP(I-OFFSET) is the gap betwe
* eigenvalues I and I+1. Note that if IFIRST.EQ.ILAST
* then WGAP(IFIRST-OFFSET) must be set to ZERO.
* On output, these gaps are refined.
*
* WERR (input/output) DOUBLE PRECISION array, dimension (N)
* On input, WERR( IFIRST-OFFSET ) through WERR( ILAST-OFFSET )
* the errors in the estimates of the corresponding elements in
* On output, these errors are refined.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (2*N)
* Workspace.
*
* IWORK (workspace) INTEGER array, dimension (2*N)
* Workspace.
*
* PIVMIN (input) DOUBLE PRECISION
* The minimum pivot in the Sturm sequence.
*
* SPDIAM (input) DOUBLE PRECISION
* The spectral diameter of the matrix.
*
* TWIST (input) INTEGER
* The twist index for the twisted factorization that is used
* for the negcount.
* TWIST = N: Compute negcount from L D L^T - LAMBDA I = L+ D+ L
* TWIST = 1: Compute negcount from L D L^T - LAMBDA I = U- D- U
* TWIST = R: Compute negcount from L D L^T - LAMBDA I = N(r) D(
*
* INFO (output) INTEGER
* Error flag.
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param _d_offset
* @param lld
* @param _lld_offset
* @param ifirst
* @param ilast
* @param rtol1
* @param rtol2
* @param offset
* @param w
* @param _w_offset
* @param wgap
* @param _wgap_offset
* @param werr
* @param _werr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param pivmin
* @param spdiam
* @param twist
* @param info
*
*/
abstract public void dlarrb(int n, double[] d, int _d_offset, double[] lld, int _lld_offset, int ifirst, int ilast, double rtol1, double rtol2, int offset, double[] w, int _w_offset, double[] wgap, int _wgap_offset, double[] werr, int _werr_offset, double[] work, int _work_offset, int[] iwork, int _iwork_offset, double pivmin, double spdiam, int twist, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* Find the number of eigenvalues of the symmetric tridiagonal matrix T
* that are in the interval (VL,VU] if JOBT = 'T', and of L D L^T
* if JOBT = 'L'.
*
* Arguments
* =========
*
* JOBT (input) CHARACTER*1
* = 'T': Compute Sturm count for matrix T.
* = 'L': Compute Sturm count for matrix L D L^T.
*
* N (input) INTEGER
* The order of the matrix. N > 0.
*
* VL (input) DOUBLE PRECISION
* VU (input) DOUBLE PRECISION
* The lower and upper bounds for the eigenvalues.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* JOBT = 'T': The N diagonal elements of the tridiagonal matrix
* JOBT = 'L': The N diagonal elements of the diagonal matrix D.
*
* E (input) DOUBLE PRECISION array, dimension (N)
* JOBT = 'T': The N-1 offdiagonal elements of the matrix T.
* JOBT = 'L': The N-1 offdiagonal elements of the matrix L.
*
* PIVMIN (input) DOUBLE PRECISION
* The minimum pivot in the Sturm sequence for T.
*
* EIGCNT (output) INTEGER
* The number of eigenvalues of the symmetric tridiagonal matrix
* that are in the interval (VL,VU]
*
* LCNT (output) INTEGER
* RCNT (output) INTEGER
* The left and right negcounts of the interval.
*
* INFO (output) INTEGER
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobt
* @param n
* @param vl
* @param vu
* @param d
* @param e
* @param pivmin
* @param eigcnt
* @param lcnt
* @param rcnt
* @param info
*
*/
abstract public void dlarrc(java.lang.String jobt, int n, double vl, double vu, double[] d, double[] e, double pivmin, org.netlib.util.intW eigcnt, org.netlib.util.intW lcnt, org.netlib.util.intW rcnt, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* Find the number of eigenvalues of the symmetric tridiagonal matrix T
* that are in the interval (VL,VU] if JOBT = 'T', and of L D L^T
* if JOBT = 'L'.
*
* Arguments
* =========
*
* JOBT (input) CHARACTER*1
* = 'T': Compute Sturm count for matrix T.
* = 'L': Compute Sturm count for matrix L D L^T.
*
* N (input) INTEGER
* The order of the matrix. N > 0.
*
* VL (input) DOUBLE PRECISION
* VU (input) DOUBLE PRECISION
* The lower and upper bounds for the eigenvalues.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* JOBT = 'T': The N diagonal elements of the tridiagonal matrix
* JOBT = 'L': The N diagonal elements of the diagonal matrix D.
*
* E (input) DOUBLE PRECISION array, dimension (N)
* JOBT = 'T': The N-1 offdiagonal elements of the matrix T.
* JOBT = 'L': The N-1 offdiagonal elements of the matrix L.
*
* PIVMIN (input) DOUBLE PRECISION
* The minimum pivot in the Sturm sequence for T.
*
* EIGCNT (output) INTEGER
* The number of eigenvalues of the symmetric tridiagonal matrix
* that are in the interval (VL,VU]
*
* LCNT (output) INTEGER
* RCNT (output) INTEGER
* The left and right negcounts of the interval.
*
* INFO (output) INTEGER
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobt
* @param n
* @param vl
* @param vu
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param pivmin
* @param eigcnt
* @param lcnt
* @param rcnt
* @param info
*
*/
abstract public void dlarrc(java.lang.String jobt, int n, double vl, double vu, double[] d, int _d_offset, double[] e, int _e_offset, double pivmin, org.netlib.util.intW eigcnt, org.netlib.util.intW lcnt, org.netlib.util.intW rcnt, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLARRD computes the eigenvalues of a symmetric tridiagonal
* matrix T to suitable accuracy. This is an auxiliary code to be
* called from DSTEMR.
* The user may ask for all eigenvalues, all eigenvalues
* in the half-open interval (VL, VU], or the IL-th through IU-th
* eigenvalues.
*
* To avoid overflow, the matrix must be scaled so that its
* largest element is no greater than overflow**(1/2) *
* underflow**(1/4) in absolute value, and for greatest
* accuracy, it should not be much smaller than that.
*
* See W. Kahan "Accurate Eigenvalues of a Symmetric Tridiagonal
* Matrix", Report CS41, Computer Science Dept., Stanford
* University, July 21, 1966.
*
* Arguments
* =========
*
* RANGE (input) CHARACTER
* = 'A': ("All") all eigenvalues will be found.
* = 'V': ("Value") all eigenvalues in the half-open interval
* (VL, VU] will be found.
* = 'I': ("Index") the IL-th through IU-th eigenvalues (of the
* entire matrix) will be found.
*
* ORDER (input) CHARACTER
* = 'B': ("By Block") the eigenvalues will be grouped by
* split-off block (see IBLOCK, ISPLIT) and
* ordered from smallest to largest within
* the block.
* = 'E': ("Entire matrix")
* the eigenvalues for the entire matrix
* will be ordered from smallest to
* largest.
*
* N (input) INTEGER
* The order of the tridiagonal matrix T. N >= 0.
*
* VL (input) DOUBLE PRECISION
* VU (input) DOUBLE PRECISION
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. Eigenvalues less than or equal
* to VL, or greater than VU, will not be returned. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* GERS (input) DOUBLE PRECISION array, dimension (2*N)
* The N Gerschgorin intervals (the i-th Gerschgorin interval
* is (GERS(2*i-1), GERS(2*i)).
*
* RELTOL (input) DOUBLE PRECISION
* The minimum relative width of an interval. When an interval
* is narrower than RELTOL times the larger (in
* magnitude) endpoint, then it is considered to be
* sufficiently small, i.e., converged. Note: this should
* always be at least radix*machine epsilon.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The n diagonal elements of the tridiagonal matrix T.
*
* E (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) off-diagonal elements of the tridiagonal matrix T.
*
* E2 (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) squared off-diagonal elements of the tridiagonal ma
*
* PIVMIN (input) DOUBLE PRECISION
* The minimum pivot allowed in the Sturm sequence for T.
*
* NSPLIT (input) INTEGER
* The number of diagonal blocks in the matrix T.
* 1 <= NSPLIT <= N.
*
* ISPLIT (input) INTEGER array, dimension (N)
* The splitting points, at which T breaks up into submatrices.
* The first submatrix consists of rows/columns 1 to ISPLIT(1),
* the second of rows/columns ISPLIT(1)+1 through ISPLIT(2),
* etc., and the NSPLIT-th consists of rows/columns
* ISPLIT(NSPLIT-1)+1 through ISPLIT(NSPLIT)=N.
* (Only the first NSPLIT elements will actually be used, but
* since the user cannot know a priori what value NSPLIT will
* have, N words must be reserved for ISPLIT.)
*
* M (output) INTEGER
* The actual number of eigenvalues found. 0 <= M <= N.
* (See also the description of INFO=2,3.)
*
* W (output) DOUBLE PRECISION array, dimension (N)
* On exit, the first M elements of W will contain the
* eigenvalue approximations. DLARRD computes an interval
* I_j = (a_j, b_j] that includes eigenvalue j. The eigenvalue
* approximation is given as the interval midpoint
* W(j)= ( a_j + b_j)/2. The corresponding error is bounded by
* WERR(j) = abs( a_j - b_j)/2
*
* WERR (output) DOUBLE PRECISION array, dimension (N)
* The error bound on the corresponding eigenvalue approximation
* in W.
*
* WL (output) DOUBLE PRECISION
* WU (output) DOUBLE PRECISION
* The interval (WL, WU] contains all the wanted eigenvalues.
* If RANGE='V', then WL=VL and WU=VU.
* If RANGE='A', then WL and WU are the global Gerschgorin bound
* on the spectrum.
* If RANGE='I', then WL and WU are computed by DLAEBZ from the
* index range specified.
*
* IBLOCK (output) INTEGER array, dimension (N)
* At each row/column j where E(j) is zero or small, the
* matrix T is considered to split into a block diagonal
* matrix. On exit, if INFO = 0, IBLOCK(i) specifies to which
* block (from 1 to the number of blocks) the eigenvalue W(i)
* belongs. (DLARRD may use the remaining N-M elements as
* workspace.)
*
* INDEXW (output) INTEGER array, dimension (N)
* The indices of the eigenvalues within each block (submatrix);
* for example, INDEXW(i)= j and IBLOCK(i)=k imply that the
* i-th eigenvalue W(i) is the j-th eigenvalue in block k.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (4*N)
*
* IWORK (workspace) INTEGER array, dimension (3*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: some or all of the eigenvalues failed to converge or
* were not computed:
* =1 or 3: Bisection failed to converge for some
* eigenvalues; these eigenvalues are flagged by a
* negative block number. The effect is that the
* eigenvalues may not be as accurate as the
* absolute and relative tolerances. This is
* generally caused by unexpectedly inaccurate
* arithmetic.
* =2 or 3: RANGE='I' only: Not all of the eigenvalues
* IL:IU were found.
* Effect: M < IU+1-IL
* Cause: non-monotonic arithmetic, causing the
* Sturm sequence to be non-monotonic.
* Cure: recalculate, using RANGE='A', and pick
* out eigenvalues IL:IU. In some cases,
* increasing the PARAMETER "FUDGE" may
* make things work.
* = 4: RANGE='I', and the Gershgorin interval
* initially used was too small. No eigenvalues
* were computed.
* Probable cause: your machine has sloppy
* floating-point arithmetic.
* Cure: Increase the PARAMETER "FUDGE",
* recompile, and try again.
*
* Internal Parameters
* ===================
*
* FUDGE DOUBLE PRECISION, default = 2
* A "fudge factor" to widen the Gershgorin intervals. Ideally,
* a value of 1 should work, but on machines with sloppy
* arithmetic, this needs to be larger. The default for
* publicly released versions should be large enough to handle
* the worst machine around. Note that this has no effect
* on accuracy of the solution.
*
* Based on contributions by
* W. Kahan, University of California, Berkeley, USA
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param range
* @param order
* @param n
* @param vl
* @param vu
* @param il
* @param iu
* @param gers
* @param reltol
* @param d
* @param e
* @param e2
* @param pivmin
* @param nsplit
* @param isplit
* @param m
* @param w
* @param werr
* @param wl
* @param wu
* @param iblock
* @param indexw
* @param work
* @param iwork
* @param info
*
*/
abstract public void dlarrd(java.lang.String range, java.lang.String order, int n, double vl, double vu, int il, int iu, double[] gers, double reltol, double[] d, double[] e, double[] e2, double pivmin, int nsplit, int[] isplit, org.netlib.util.intW m, double[] w, double[] werr, org.netlib.util.doubleW wl, org.netlib.util.doubleW wu, int[] iblock, int[] indexw, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLARRD computes the eigenvalues of a symmetric tridiagonal
* matrix T to suitable accuracy. This is an auxiliary code to be
* called from DSTEMR.
* The user may ask for all eigenvalues, all eigenvalues
* in the half-open interval (VL, VU], or the IL-th through IU-th
* eigenvalues.
*
* To avoid overflow, the matrix must be scaled so that its
* largest element is no greater than overflow**(1/2) *
* underflow**(1/4) in absolute value, and for greatest
* accuracy, it should not be much smaller than that.
*
* See W. Kahan "Accurate Eigenvalues of a Symmetric Tridiagonal
* Matrix", Report CS41, Computer Science Dept., Stanford
* University, July 21, 1966.
*
* Arguments
* =========
*
* RANGE (input) CHARACTER
* = 'A': ("All") all eigenvalues will be found.
* = 'V': ("Value") all eigenvalues in the half-open interval
* (VL, VU] will be found.
* = 'I': ("Index") the IL-th through IU-th eigenvalues (of the
* entire matrix) will be found.
*
* ORDER (input) CHARACTER
* = 'B': ("By Block") the eigenvalues will be grouped by
* split-off block (see IBLOCK, ISPLIT) and
* ordered from smallest to largest within
* the block.
* = 'E': ("Entire matrix")
* the eigenvalues for the entire matrix
* will be ordered from smallest to
* largest.
*
* N (input) INTEGER
* The order of the tridiagonal matrix T. N >= 0.
*
* VL (input) DOUBLE PRECISION
* VU (input) DOUBLE PRECISION
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. Eigenvalues less than or equal
* to VL, or greater than VU, will not be returned. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* GERS (input) DOUBLE PRECISION array, dimension (2*N)
* The N Gerschgorin intervals (the i-th Gerschgorin interval
* is (GERS(2*i-1), GERS(2*i)).
*
* RELTOL (input) DOUBLE PRECISION
* The minimum relative width of an interval. When an interval
* is narrower than RELTOL times the larger (in
* magnitude) endpoint, then it is considered to be
* sufficiently small, i.e., converged. Note: this should
* always be at least radix*machine epsilon.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The n diagonal elements of the tridiagonal matrix T.
*
* E (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) off-diagonal elements of the tridiagonal matrix T.
*
* E2 (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) squared off-diagonal elements of the tridiagonal ma
*
* PIVMIN (input) DOUBLE PRECISION
* The minimum pivot allowed in the Sturm sequence for T.
*
* NSPLIT (input) INTEGER
* The number of diagonal blocks in the matrix T.
* 1 <= NSPLIT <= N.
*
* ISPLIT (input) INTEGER array, dimension (N)
* The splitting points, at which T breaks up into submatrices.
* The first submatrix consists of rows/columns 1 to ISPLIT(1),
* the second of rows/columns ISPLIT(1)+1 through ISPLIT(2),
* etc., and the NSPLIT-th consists of rows/columns
* ISPLIT(NSPLIT-1)+1 through ISPLIT(NSPLIT)=N.
* (Only the first NSPLIT elements will actually be used, but
* since the user cannot know a priori what value NSPLIT will
* have, N words must be reserved for ISPLIT.)
*
* M (output) INTEGER
* The actual number of eigenvalues found. 0 <= M <= N.
* (See also the description of INFO=2,3.)
*
* W (output) DOUBLE PRECISION array, dimension (N)
* On exit, the first M elements of W will contain the
* eigenvalue approximations. DLARRD computes an interval
* I_j = (a_j, b_j] that includes eigenvalue j. The eigenvalue
* approximation is given as the interval midpoint
* W(j)= ( a_j + b_j)/2. The corresponding error is bounded by
* WERR(j) = abs( a_j - b_j)/2
*
* WERR (output) DOUBLE PRECISION array, dimension (N)
* The error bound on the corresponding eigenvalue approximation
* in W.
*
* WL (output) DOUBLE PRECISION
* WU (output) DOUBLE PRECISION
* The interval (WL, WU] contains all the wanted eigenvalues.
* If RANGE='V', then WL=VL and WU=VU.
* If RANGE='A', then WL and WU are the global Gerschgorin bound
* on the spectrum.
* If RANGE='I', then WL and WU are computed by DLAEBZ from the
* index range specified.
*
* IBLOCK (output) INTEGER array, dimension (N)
* At each row/column j where E(j) is zero or small, the
* matrix T is considered to split into a block diagonal
* matrix. On exit, if INFO = 0, IBLOCK(i) specifies to which
* block (from 1 to the number of blocks) the eigenvalue W(i)
* belongs. (DLARRD may use the remaining N-M elements as
* workspace.)
*
* INDEXW (output) INTEGER array, dimension (N)
* The indices of the eigenvalues within each block (submatrix);
* for example, INDEXW(i)= j and IBLOCK(i)=k imply that the
* i-th eigenvalue W(i) is the j-th eigenvalue in block k.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (4*N)
*
* IWORK (workspace) INTEGER array, dimension (3*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: some or all of the eigenvalues failed to converge or
* were not computed:
* =1 or 3: Bisection failed to converge for some
* eigenvalues; these eigenvalues are flagged by a
* negative block number. The effect is that the
* eigenvalues may not be as accurate as the
* absolute and relative tolerances. This is
* generally caused by unexpectedly inaccurate
* arithmetic.
* =2 or 3: RANGE='I' only: Not all of the eigenvalues
* IL:IU were found.
* Effect: M < IU+1-IL
* Cause: non-monotonic arithmetic, causing the
* Sturm sequence to be non-monotonic.
* Cure: recalculate, using RANGE='A', and pick
* out eigenvalues IL:IU. In some cases,
* increasing the PARAMETER "FUDGE" may
* make things work.
* = 4: RANGE='I', and the Gershgorin interval
* initially used was too small. No eigenvalues
* were computed.
* Probable cause: your machine has sloppy
* floating-point arithmetic.
* Cure: Increase the PARAMETER "FUDGE",
* recompile, and try again.
*
* Internal Parameters
* ===================
*
* FUDGE DOUBLE PRECISION, default = 2
* A "fudge factor" to widen the Gershgorin intervals. Ideally,
* a value of 1 should work, but on machines with sloppy
* arithmetic, this needs to be larger. The default for
* publicly released versions should be large enough to handle
* the worst machine around. Note that this has no effect
* on accuracy of the solution.
*
* Based on contributions by
* W. Kahan, University of California, Berkeley, USA
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param range
* @param order
* @param n
* @param vl
* @param vu
* @param il
* @param iu
* @param gers
* @param _gers_offset
* @param reltol
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param e2
* @param _e2_offset
* @param pivmin
* @param nsplit
* @param isplit
* @param _isplit_offset
* @param m
* @param w
* @param _w_offset
* @param werr
* @param _werr_offset
* @param wl
* @param wu
* @param iblock
* @param _iblock_offset
* @param indexw
* @param _indexw_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dlarrd(java.lang.String range, java.lang.String order, int n, double vl, double vu, int il, int iu, double[] gers, int _gers_offset, double reltol, double[] d, int _d_offset, double[] e, int _e_offset, double[] e2, int _e2_offset, double pivmin, int nsplit, int[] isplit, int _isplit_offset, org.netlib.util.intW m, double[] w, int _w_offset, double[] werr, int _werr_offset, org.netlib.util.doubleW wl, org.netlib.util.doubleW wu, int[] iblock, int _iblock_offset, int[] indexw, int _indexw_offset, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* To find the desired eigenvalues of a given real symmetric
* tridiagonal matrix T, DLARRE sets any "small" off-diagonal
* elements to zero, and for each unreduced block T_i, it finds
* (a) a suitable shift at one end of the block's spectrum,
* (b) the base representation, T_i - sigma_i I = L_i D_i L_i^T, and
* (c) eigenvalues of each L_i D_i L_i^T.
* The representations and eigenvalues found are then used by
* DSTEMR to compute the eigenvectors of T.
* The accuracy varies depending on whether bisection is used to
* find a few eigenvalues or the dqds algorithm (subroutine DLASQ2) to
* conpute all and then discard any unwanted one.
* As an added benefit, DLARRE also outputs the n
* Gerschgorin intervals for the matrices L_i D_i L_i^T.
*
* Arguments
* =========
*
* RANGE (input) CHARACTER
* = 'A': ("All") all eigenvalues will be found.
* = 'V': ("Value") all eigenvalues in the half-open interval
* (VL, VU] will be found.
* = 'I': ("Index") the IL-th through IU-th eigenvalues (of the
* entire matrix) will be found.
*
* N (input) INTEGER
* The order of the matrix. N > 0.
*
* VL (input/output) DOUBLE PRECISION
* VU (input/output) DOUBLE PRECISION
* If RANGE='V', the lower and upper bounds for the eigenvalues.
* Eigenvalues less than or equal to VL, or greater than VU,
* will not be returned. VL < VU.
* If RANGE='I' or ='A', DLARRE computes bounds on the desired
* part of the spectrum.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the N diagonal elements of the tridiagonal
* matrix T.
* On exit, the N diagonal elements of the diagonal
* matrices D_i.
*
* E (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the first (N-1) entries contain the subdiagonal
* elements of the tridiagonal matrix T; E(N) need not be set.
* On exit, E contains the subdiagonal elements of the unit
* bidiagonal matrices L_i. The entries E( ISPLIT( I ) ),
* 1 <= I <= NSPLIT, contain the base points sigma_i on output.
*
* E2 (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the first (N-1) entries contain the SQUARES of the
* subdiagonal elements of the tridiagonal matrix T;
* E2(N) need not be set.
* On exit, the entries E2( ISPLIT( I ) ),
* 1 <= I <= NSPLIT, have been set to zero
*
* RTOL1 (input) DOUBLE PRECISION
* RTOL2 (input) DOUBLE PRECISION
* Parameters for bisection.
* An interval [LEFT,RIGHT] has converged if
* RIGHT-LEFT.LT.MAX( RTOL1*GAP, RTOL2*MAX(|LEFT|,|RIGHT|) )
*
* SPLTOL (input) DOUBLE PRECISION
* The threshold for splitting.
*
* NSPLIT (output) INTEGER
* The number of blocks T splits into. 1 <= NSPLIT <= N.
*
* ISPLIT (output) INTEGER array, dimension (N)
* The splitting points, at which T breaks up into blocks.
* The first block consists of rows/columns 1 to ISPLIT(1),
* the second of rows/columns ISPLIT(1)+1 through ISPLIT(2),
* etc., and the NSPLIT-th consists of rows/columns
* ISPLIT(NSPLIT-1)+1 through ISPLIT(NSPLIT)=N.
*
* M (output) INTEGER
* The total number of eigenvalues (of all L_i D_i L_i^T)
* found.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* The first M elements contain the eigenvalues. The
* eigenvalues of each of the blocks, L_i D_i L_i^T, are
* sorted in ascending order ( DLARRE may use the
* remaining N-M elements as workspace).
*
* WERR (output) DOUBLE PRECISION array, dimension (N)
* The error bound on the corresponding eigenvalue in W.
*
* WGAP (output) DOUBLE PRECISION array, dimension (N)
* The separation from the right neighbor eigenvalue in W.
* The gap is only with respect to the eigenvalues of the same b
* as each block has its own representation tree.
* Exception: at the right end of a block we store the left gap
*
* IBLOCK (output) INTEGER array, dimension (N)
* The indices of the blocks (submatrices) associated with the
* corresponding eigenvalues in W; IBLOCK(i)=1 if eigenvalue
* W(i) belongs to the first block from the top, =2 if W(i)
* belongs to the second block, etc.
*
* INDEXW (output) INTEGER array, dimension (N)
* The indices of the eigenvalues within each block (submatrix);
* for example, INDEXW(i)= 10 and IBLOCK(i)=2 imply that the
* i-th eigenvalue W(i) is the 10-th eigenvalue in block 2
*
* GERS (output) DOUBLE PRECISION array, dimension (2*N)
* The N Gerschgorin intervals (the i-th Gerschgorin interval
* is (GERS(2*i-1), GERS(2*i)).
*
* PIVMIN (output) DOUBLE PRECISION
* The minimum pivot in the Sturm sequence for T.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (6*N)
* Workspace.
*
* IWORK (workspace) INTEGER array, dimension (5*N)
* Workspace.
*
* INFO (output) INTEGER
* = 0: successful exit
* > 0: A problem occured in DLARRE.
* < 0: One of the called subroutines signaled an internal prob
* Needs inspection of the corresponding parameter IINFO
* for further information.
*
* =-1: Problem in DLARRD.
* = 2: No base representation could be found in MAXTRY iterati
* Increasing MAXTRY and recompilation might be a remedy.
* =-3: Problem in DLARRB when computing the refined root
* representation for DLASQ2.
* =-4: Problem in DLARRB when preforming bisection on the
* desired part of the spectrum.
* =-5: Problem in DLASQ2.
* =-6: Problem in DLASQ2.
*
* Further Details
* The base representations are required to suffer very little
* element growth and consequently define all their eigenvalues to
* high relative accuracy.
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param range
* @param n
* @param vl
* @param vu
* @param il
* @param iu
* @param d
* @param e
* @param e2
* @param rtol1
* @param rtol2
* @param spltol
* @param nsplit
* @param isplit
* @param m
* @param w
* @param werr
* @param wgap
* @param iblock
* @param indexw
* @param gers
* @param pivmin
* @param work
* @param iwork
* @param info
*
*/
abstract public void dlarre(java.lang.String range, int n, org.netlib.util.doubleW vl, org.netlib.util.doubleW vu, int il, int iu, double[] d, double[] e, double[] e2, double rtol1, double rtol2, double spltol, org.netlib.util.intW nsplit, int[] isplit, org.netlib.util.intW m, double[] w, double[] werr, double[] wgap, int[] iblock, int[] indexw, double[] gers, org.netlib.util.doubleW pivmin, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* To find the desired eigenvalues of a given real symmetric
* tridiagonal matrix T, DLARRE sets any "small" off-diagonal
* elements to zero, and for each unreduced block T_i, it finds
* (a) a suitable shift at one end of the block's spectrum,
* (b) the base representation, T_i - sigma_i I = L_i D_i L_i^T, and
* (c) eigenvalues of each L_i D_i L_i^T.
* The representations and eigenvalues found are then used by
* DSTEMR to compute the eigenvectors of T.
* The accuracy varies depending on whether bisection is used to
* find a few eigenvalues or the dqds algorithm (subroutine DLASQ2) to
* conpute all and then discard any unwanted one.
* As an added benefit, DLARRE also outputs the n
* Gerschgorin intervals for the matrices L_i D_i L_i^T.
*
* Arguments
* =========
*
* RANGE (input) CHARACTER
* = 'A': ("All") all eigenvalues will be found.
* = 'V': ("Value") all eigenvalues in the half-open interval
* (VL, VU] will be found.
* = 'I': ("Index") the IL-th through IU-th eigenvalues (of the
* entire matrix) will be found.
*
* N (input) INTEGER
* The order of the matrix. N > 0.
*
* VL (input/output) DOUBLE PRECISION
* VU (input/output) DOUBLE PRECISION
* If RANGE='V', the lower and upper bounds for the eigenvalues.
* Eigenvalues less than or equal to VL, or greater than VU,
* will not be returned. VL < VU.
* If RANGE='I' or ='A', DLARRE computes bounds on the desired
* part of the spectrum.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the N diagonal elements of the tridiagonal
* matrix T.
* On exit, the N diagonal elements of the diagonal
* matrices D_i.
*
* E (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the first (N-1) entries contain the subdiagonal
* elements of the tridiagonal matrix T; E(N) need not be set.
* On exit, E contains the subdiagonal elements of the unit
* bidiagonal matrices L_i. The entries E( ISPLIT( I ) ),
* 1 <= I <= NSPLIT, contain the base points sigma_i on output.
*
* E2 (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the first (N-1) entries contain the SQUARES of the
* subdiagonal elements of the tridiagonal matrix T;
* E2(N) need not be set.
* On exit, the entries E2( ISPLIT( I ) ),
* 1 <= I <= NSPLIT, have been set to zero
*
* RTOL1 (input) DOUBLE PRECISION
* RTOL2 (input) DOUBLE PRECISION
* Parameters for bisection.
* An interval [LEFT,RIGHT] has converged if
* RIGHT-LEFT.LT.MAX( RTOL1*GAP, RTOL2*MAX(|LEFT|,|RIGHT|) )
*
* SPLTOL (input) DOUBLE PRECISION
* The threshold for splitting.
*
* NSPLIT (output) INTEGER
* The number of blocks T splits into. 1 <= NSPLIT <= N.
*
* ISPLIT (output) INTEGER array, dimension (N)
* The splitting points, at which T breaks up into blocks.
* The first block consists of rows/columns 1 to ISPLIT(1),
* the second of rows/columns ISPLIT(1)+1 through ISPLIT(2),
* etc., and the NSPLIT-th consists of rows/columns
* ISPLIT(NSPLIT-1)+1 through ISPLIT(NSPLIT)=N.
*
* M (output) INTEGER
* The total number of eigenvalues (of all L_i D_i L_i^T)
* found.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* The first M elements contain the eigenvalues. The
* eigenvalues of each of the blocks, L_i D_i L_i^T, are
* sorted in ascending order ( DLARRE may use the
* remaining N-M elements as workspace).
*
* WERR (output) DOUBLE PRECISION array, dimension (N)
* The error bound on the corresponding eigenvalue in W.
*
* WGAP (output) DOUBLE PRECISION array, dimension (N)
* The separation from the right neighbor eigenvalue in W.
* The gap is only with respect to the eigenvalues of the same b
* as each block has its own representation tree.
* Exception: at the right end of a block we store the left gap
*
* IBLOCK (output) INTEGER array, dimension (N)
* The indices of the blocks (submatrices) associated with the
* corresponding eigenvalues in W; IBLOCK(i)=1 if eigenvalue
* W(i) belongs to the first block from the top, =2 if W(i)
* belongs to the second block, etc.
*
* INDEXW (output) INTEGER array, dimension (N)
* The indices of the eigenvalues within each block (submatrix);
* for example, INDEXW(i)= 10 and IBLOCK(i)=2 imply that the
* i-th eigenvalue W(i) is the 10-th eigenvalue in block 2
*
* GERS (output) DOUBLE PRECISION array, dimension (2*N)
* The N Gerschgorin intervals (the i-th Gerschgorin interval
* is (GERS(2*i-1), GERS(2*i)).
*
* PIVMIN (output) DOUBLE PRECISION
* The minimum pivot in the Sturm sequence for T.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (6*N)
* Workspace.
*
* IWORK (workspace) INTEGER array, dimension (5*N)
* Workspace.
*
* INFO (output) INTEGER
* = 0: successful exit
* > 0: A problem occured in DLARRE.
* < 0: One of the called subroutines signaled an internal prob
* Needs inspection of the corresponding parameter IINFO
* for further information.
*
* =-1: Problem in DLARRD.
* = 2: No base representation could be found in MAXTRY iterati
* Increasing MAXTRY and recompilation might be a remedy.
* =-3: Problem in DLARRB when computing the refined root
* representation for DLASQ2.
* =-4: Problem in DLARRB when preforming bisection on the
* desired part of the spectrum.
* =-5: Problem in DLASQ2.
* =-6: Problem in DLASQ2.
*
* Further Details
* The base representations are required to suffer very little
* element growth and consequently define all their eigenvalues to
* high relative accuracy.
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param range
* @param n
* @param vl
* @param vu
* @param il
* @param iu
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param e2
* @param _e2_offset
* @param rtol1
* @param rtol2
* @param spltol
* @param nsplit
* @param isplit
* @param _isplit_offset
* @param m
* @param w
* @param _w_offset
* @param werr
* @param _werr_offset
* @param wgap
* @param _wgap_offset
* @param iblock
* @param _iblock_offset
* @param indexw
* @param _indexw_offset
* @param gers
* @param _gers_offset
* @param pivmin
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dlarre(java.lang.String range, int n, org.netlib.util.doubleW vl, org.netlib.util.doubleW vu, int il, int iu, double[] d, int _d_offset, double[] e, int _e_offset, double[] e2, int _e2_offset, double rtol1, double rtol2, double spltol, org.netlib.util.intW nsplit, int[] isplit, int _isplit_offset, org.netlib.util.intW m, double[] w, int _w_offset, double[] werr, int _werr_offset, double[] wgap, int _wgap_offset, int[] iblock, int _iblock_offset, int[] indexw, int _indexw_offset, double[] gers, int _gers_offset, org.netlib.util.doubleW pivmin, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* Given the initial representation L D L^T and its cluster of close
* eigenvalues (in a relative measure), W( CLSTRT ), W( CLSTRT+1 ), ...
* W( CLEND ), DLARRF finds a new relatively robust representation
* L D L^T - SIGMA I = L(+) D(+) L(+)^T such that at least one of the
* eigenvalues of L(+) D(+) L(+)^T is relatively isolated.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix (subblock, if the matrix splitted).
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The N diagonal elements of the diagonal matrix D.
*
* L (input) DOUBLE PRECISION array, dimension (N-1)
* The (N-1) subdiagonal elements of the unit bidiagonal
* matrix L.
*
* LD (input) DOUBLE PRECISION array, dimension (N-1)
* The (N-1) elements L(i)*D(i).
*
* CLSTRT (input) INTEGER
* The index of the first eigenvalue in the cluster.
*
* CLEND (input) INTEGER
* The index of the last eigenvalue in the cluster.
*
* W (input) DOUBLE PRECISION array, dimension >= (CLEND-CLSTRT+1
* The eigenvalue APPROXIMATIONS of L D L^T in ascending order.
* W( CLSTRT ) through W( CLEND ) form the cluster of relatively
* close eigenalues.
*
* WGAP (input/output) DOUBLE PRECISION array, dimension >= (CLEND-C
* The separation from the right neighbor eigenvalue in W.
*
* WERR (input) DOUBLE PRECISION array, dimension >= (CLEND-CLSTRT+1
* WERR contain the semiwidth of the uncertainty
* interval of the corresponding eigenvalue APPROXIMATION in W
*
* SPDIAM (input) estimate of the spectral diameter obtained from the
* Gerschgorin intervals
*
* CLGAPL, CLGAPR (input) absolute gap on each end of the cluster.
* Set by the calling routine to protect against shifts too clos
* to eigenvalues outside the cluster.
*
* PIVMIN (input) DOUBLE PRECISION
* The minimum pivot allowed in the Sturm sequence.
*
* SIGMA (output) DOUBLE PRECISION
* The shift used to form L(+) D(+) L(+)^T.
*
* DPLUS (output) DOUBLE PRECISION array, dimension (N)
* The N diagonal elements of the diagonal matrix D(+).
*
* LPLUS (output) DOUBLE PRECISION array, dimension (N-1)
* The first (N-1) elements of LPLUS contain the subdiagonal
* elements of the unit bidiagonal matrix L(+).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (2*N)
* Workspace.
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param l
* @param ld
* @param clstrt
* @param clend
* @param w
* @param wgap
* @param werr
* @param spdiam
* @param clgapl
* @param clgapr
* @param pivmin
* @param sigma
* @param dplus
* @param lplus
* @param work
* @param info
*
*/
abstract public void dlarrf(int n, double[] d, double[] l, double[] ld, int clstrt, int clend, double[] w, double[] wgap, double[] werr, double spdiam, double clgapl, double clgapr, double pivmin, org.netlib.util.doubleW sigma, double[] dplus, double[] lplus, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* Given the initial representation L D L^T and its cluster of close
* eigenvalues (in a relative measure), W( CLSTRT ), W( CLSTRT+1 ), ...
* W( CLEND ), DLARRF finds a new relatively robust representation
* L D L^T - SIGMA I = L(+) D(+) L(+)^T such that at least one of the
* eigenvalues of L(+) D(+) L(+)^T is relatively isolated.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix (subblock, if the matrix splitted).
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The N diagonal elements of the diagonal matrix D.
*
* L (input) DOUBLE PRECISION array, dimension (N-1)
* The (N-1) subdiagonal elements of the unit bidiagonal
* matrix L.
*
* LD (input) DOUBLE PRECISION array, dimension (N-1)
* The (N-1) elements L(i)*D(i).
*
* CLSTRT (input) INTEGER
* The index of the first eigenvalue in the cluster.
*
* CLEND (input) INTEGER
* The index of the last eigenvalue in the cluster.
*
* W (input) DOUBLE PRECISION array, dimension >= (CLEND-CLSTRT+1
* The eigenvalue APPROXIMATIONS of L D L^T in ascending order.
* W( CLSTRT ) through W( CLEND ) form the cluster of relatively
* close eigenalues.
*
* WGAP (input/output) DOUBLE PRECISION array, dimension >= (CLEND-C
* The separation from the right neighbor eigenvalue in W.
*
* WERR (input) DOUBLE PRECISION array, dimension >= (CLEND-CLSTRT+1
* WERR contain the semiwidth of the uncertainty
* interval of the corresponding eigenvalue APPROXIMATION in W
*
* SPDIAM (input) estimate of the spectral diameter obtained from the
* Gerschgorin intervals
*
* CLGAPL, CLGAPR (input) absolute gap on each end of the cluster.
* Set by the calling routine to protect against shifts too clos
* to eigenvalues outside the cluster.
*
* PIVMIN (input) DOUBLE PRECISION
* The minimum pivot allowed in the Sturm sequence.
*
* SIGMA (output) DOUBLE PRECISION
* The shift used to form L(+) D(+) L(+)^T.
*
* DPLUS (output) DOUBLE PRECISION array, dimension (N)
* The N diagonal elements of the diagonal matrix D(+).
*
* LPLUS (output) DOUBLE PRECISION array, dimension (N-1)
* The first (N-1) elements of LPLUS contain the subdiagonal
* elements of the unit bidiagonal matrix L(+).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (2*N)
* Workspace.
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param _d_offset
* @param l
* @param _l_offset
* @param ld
* @param _ld_offset
* @param clstrt
* @param clend
* @param w
* @param _w_offset
* @param wgap
* @param _wgap_offset
* @param werr
* @param _werr_offset
* @param spdiam
* @param clgapl
* @param clgapr
* @param pivmin
* @param sigma
* @param dplus
* @param _dplus_offset
* @param lplus
* @param _lplus_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dlarrf(int n, double[] d, int _d_offset, double[] l, int _l_offset, double[] ld, int _ld_offset, int clstrt, int clend, double[] w, int _w_offset, double[] wgap, int _wgap_offset, double[] werr, int _werr_offset, double spdiam, double clgapl, double clgapr, double pivmin, org.netlib.util.doubleW sigma, double[] dplus, int _dplus_offset, double[] lplus, int _lplus_offset, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* Given the initial eigenvalue approximations of T, DLARRJ
* does bisection to refine the eigenvalues of T,
* W( IFIRST-OFFSET ) through W( ILAST-OFFSET ), to more accuracy. Initi
* guesses for these eigenvalues are input in W, the corresponding estim
* of the error in these guesses in WERR. During bisection, intervals
* [left, right] are maintained by storing their mid-points and
* semi-widths in the arrays W and WERR respectively.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The N diagonal elements of T.
*
* E2 (input) DOUBLE PRECISION array, dimension (N-1)
* The Squares of the (N-1) subdiagonal elements of T.
*
* IFIRST (input) INTEGER
* The index of the first eigenvalue to be computed.
*
* ILAST (input) INTEGER
* The index of the last eigenvalue to be computed.
*
* RTOL (input) DOUBLE PRECISION
* Tolerance for the convergence of the bisection intervals.
* An interval [LEFT,RIGHT] has converged if
* RIGHT-LEFT.LT.RTOL*MAX(|LEFT|,|RIGHT|).
*
* OFFSET (input) INTEGER
* Offset for the arrays W and WERR, i.e., the IFIRST-OFFSET
* through ILAST-OFFSET elements of these arrays are to be used.
*
* W (input/output) DOUBLE PRECISION array, dimension (N)
* On input, W( IFIRST-OFFSET ) through W( ILAST-OFFSET ) are
* estimates of the eigenvalues of L D L^T indexed IFIRST throug
* ILAST.
* On output, these estimates are refined.
*
* WERR (input/output) DOUBLE PRECISION array, dimension (N)
* On input, WERR( IFIRST-OFFSET ) through WERR( ILAST-OFFSET )
* the errors in the estimates of the corresponding elements in
* On output, these errors are refined.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (2*N)
* Workspace.
*
* IWORK (workspace) INTEGER array, dimension (2*N)
* Workspace.
*
* PIVMIN (input) DOUBLE PRECISION
* The minimum pivot in the Sturm sequence for T.
*
* SPDIAM (input) DOUBLE PRECISION
* The spectral diameter of T.
*
* INFO (output) INTEGER
* Error flag.
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param e2
* @param ifirst
* @param ilast
* @param rtol
* @param offset
* @param w
* @param werr
* @param work
* @param iwork
* @param pivmin
* @param spdiam
* @param info
*
*/
abstract public void dlarrj(int n, double[] d, double[] e2, int ifirst, int ilast, double rtol, int offset, double[] w, double[] werr, double[] work, int[] iwork, double pivmin, double spdiam, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* Given the initial eigenvalue approximations of T, DLARRJ
* does bisection to refine the eigenvalues of T,
* W( IFIRST-OFFSET ) through W( ILAST-OFFSET ), to more accuracy. Initi
* guesses for these eigenvalues are input in W, the corresponding estim
* of the error in these guesses in WERR. During bisection, intervals
* [left, right] are maintained by storing their mid-points and
* semi-widths in the arrays W and WERR respectively.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The N diagonal elements of T.
*
* E2 (input) DOUBLE PRECISION array, dimension (N-1)
* The Squares of the (N-1) subdiagonal elements of T.
*
* IFIRST (input) INTEGER
* The index of the first eigenvalue to be computed.
*
* ILAST (input) INTEGER
* The index of the last eigenvalue to be computed.
*
* RTOL (input) DOUBLE PRECISION
* Tolerance for the convergence of the bisection intervals.
* An interval [LEFT,RIGHT] has converged if
* RIGHT-LEFT.LT.RTOL*MAX(|LEFT|,|RIGHT|).
*
* OFFSET (input) INTEGER
* Offset for the arrays W and WERR, i.e., the IFIRST-OFFSET
* through ILAST-OFFSET elements of these arrays are to be used.
*
* W (input/output) DOUBLE PRECISION array, dimension (N)
* On input, W( IFIRST-OFFSET ) through W( ILAST-OFFSET ) are
* estimates of the eigenvalues of L D L^T indexed IFIRST throug
* ILAST.
* On output, these estimates are refined.
*
* WERR (input/output) DOUBLE PRECISION array, dimension (N)
* On input, WERR( IFIRST-OFFSET ) through WERR( ILAST-OFFSET )
* the errors in the estimates of the corresponding elements in
* On output, these errors are refined.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (2*N)
* Workspace.
*
* IWORK (workspace) INTEGER array, dimension (2*N)
* Workspace.
*
* PIVMIN (input) DOUBLE PRECISION
* The minimum pivot in the Sturm sequence for T.
*
* SPDIAM (input) DOUBLE PRECISION
* The spectral diameter of T.
*
* INFO (output) INTEGER
* Error flag.
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param _d_offset
* @param e2
* @param _e2_offset
* @param ifirst
* @param ilast
* @param rtol
* @param offset
* @param w
* @param _w_offset
* @param werr
* @param _werr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param pivmin
* @param spdiam
* @param info
*
*/
abstract public void dlarrj(int n, double[] d, int _d_offset, double[] e2, int _e2_offset, int ifirst, int ilast, double rtol, int offset, double[] w, int _w_offset, double[] werr, int _werr_offset, double[] work, int _work_offset, int[] iwork, int _iwork_offset, double pivmin, double spdiam, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLARRK computes one eigenvalue of a symmetric tridiagonal
* matrix T to suitable accuracy. This is an auxiliary code to be
* called from DSTEMR.
*
* To avoid overflow, the matrix must be scaled so that its
* largest element is no greater than overflow**(1/2) *
* underflow**(1/4) in absolute value, and for greatest
* accuracy, it should not be much smaller than that.
*
* See W. Kahan "Accurate Eigenvalues of a Symmetric Tridiagonal
* Matrix", Report CS41, Computer Science Dept., Stanford
* University, July 21, 1966.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the tridiagonal matrix T. N >= 0.
*
* IW (input) INTEGER
* The index of the eigenvalues to be returned.
*
* GL (input) DOUBLE PRECISION
* GU (input) DOUBLE PRECISION
* An upper and a lower bound on the eigenvalue.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The n diagonal elements of the tridiagonal matrix T.
*
* E2 (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) squared off-diagonal elements of the tridiagonal ma
*
* PIVMIN (input) DOUBLE PRECISION
* The minimum pivot allowed in the Sturm sequence for T.
*
* RELTOL (input) DOUBLE PRECISION
* The minimum relative width of an interval. When an interval
* is narrower than RELTOL times the larger (in
* magnitude) endpoint, then it is considered to be
* sufficiently small, i.e., converged. Note: this should
* always be at least radix*machine epsilon.
*
* W (output) DOUBLE PRECISION
*
* WERR (output) DOUBLE PRECISION
* The error bound on the corresponding eigenvalue approximation
* in W.
*
* INFO (output) INTEGER
* = 0: Eigenvalue converged
* = -1: Eigenvalue did NOT converge
*
* Internal Parameters
* ===================
*
* FUDGE DOUBLE PRECISION, default = 2
* A "fudge factor" to widen the Gershgorin intervals.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param iw
* @param gl
* @param gu
* @param d
* @param e2
* @param pivmin
* @param reltol
* @param w
* @param werr
* @param info
*
*/
abstract public void dlarrk(int n, int iw, double gl, double gu, double[] d, double[] e2, double pivmin, double reltol, org.netlib.util.doubleW w, org.netlib.util.doubleW werr, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLARRK computes one eigenvalue of a symmetric tridiagonal
* matrix T to suitable accuracy. This is an auxiliary code to be
* called from DSTEMR.
*
* To avoid overflow, the matrix must be scaled so that its
* largest element is no greater than overflow**(1/2) *
* underflow**(1/4) in absolute value, and for greatest
* accuracy, it should not be much smaller than that.
*
* See W. Kahan "Accurate Eigenvalues of a Symmetric Tridiagonal
* Matrix", Report CS41, Computer Science Dept., Stanford
* University, July 21, 1966.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the tridiagonal matrix T. N >= 0.
*
* IW (input) INTEGER
* The index of the eigenvalues to be returned.
*
* GL (input) DOUBLE PRECISION
* GU (input) DOUBLE PRECISION
* An upper and a lower bound on the eigenvalue.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The n diagonal elements of the tridiagonal matrix T.
*
* E2 (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) squared off-diagonal elements of the tridiagonal ma
*
* PIVMIN (input) DOUBLE PRECISION
* The minimum pivot allowed in the Sturm sequence for T.
*
* RELTOL (input) DOUBLE PRECISION
* The minimum relative width of an interval. When an interval
* is narrower than RELTOL times the larger (in
* magnitude) endpoint, then it is considered to be
* sufficiently small, i.e., converged. Note: this should
* always be at least radix*machine epsilon.
*
* W (output) DOUBLE PRECISION
*
* WERR (output) DOUBLE PRECISION
* The error bound on the corresponding eigenvalue approximation
* in W.
*
* INFO (output) INTEGER
* = 0: Eigenvalue converged
* = -1: Eigenvalue did NOT converge
*
* Internal Parameters
* ===================
*
* FUDGE DOUBLE PRECISION, default = 2
* A "fudge factor" to widen the Gershgorin intervals.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param iw
* @param gl
* @param gu
* @param d
* @param _d_offset
* @param e2
* @param _e2_offset
* @param pivmin
* @param reltol
* @param w
* @param werr
* @param info
*
*/
abstract public void dlarrk(int n, int iw, double gl, double gu, double[] d, int _d_offset, double[] e2, int _e2_offset, double pivmin, double reltol, org.netlib.util.doubleW w, org.netlib.util.doubleW werr, org.netlib.util.intW info);
/**
*
* ..
*
*
* Purpose
* =======
*
* Perform tests to decide whether the symmetric tridiagonal matrix T
* warrants expensive computations which guarantee high relative accurac
* in the eigenvalues.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix. N > 0.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The N diagonal elements of the tridiagonal matrix T.
*
* E (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the first (N-1) entries contain the subdiagonal
* elements of the tridiagonal matrix T; E(N) is set to ZERO.
*
* INFO (output) INTEGER
* INFO = 0(default) : the matrix warrants computations preservi
* relative accuracy.
* INFO = 1 : the matrix warrants computations guarante
* only absolute accuracy.
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param e
* @param info
*
*/
abstract public void dlarrr(int n, double[] d, double[] e, org.netlib.util.intW info);
/**
*
* ..
*
*
* Purpose
* =======
*
* Perform tests to decide whether the symmetric tridiagonal matrix T
* warrants expensive computations which guarantee high relative accurac
* in the eigenvalues.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix. N > 0.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The N diagonal elements of the tridiagonal matrix T.
*
* E (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the first (N-1) entries contain the subdiagonal
* elements of the tridiagonal matrix T; E(N) is set to ZERO.
*
* INFO (output) INTEGER
* INFO = 0(default) : the matrix warrants computations preservi
* relative accuracy.
* INFO = 1 : the matrix warrants computations guarante
* only absolute accuracy.
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param info
*
*/
abstract public void dlarrr(int n, double[] d, int _d_offset, double[] e, int _e_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLARRV computes the eigenvectors of the tridiagonal matrix
* T = L D L^T given L, D and APPROXIMATIONS to the eigenvalues of L D L
* The input eigenvalues should have been computed by DLARRE.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* VL (input) DOUBLE PRECISION
* VU (input) DOUBLE PRECISION
* Lower and upper bounds of the interval that contains the desi
* eigenvalues. VL < VU. Needed to compute gaps on the left or r
* end of the extremal eigenvalues in the desired RANGE.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the N diagonal elements of the diagonal matrix D.
* On exit, D may be overwritten.
*
* L (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the (N-1) subdiagonal elements of the unit
* bidiagonal matrix L are in elements 1 to N-1 of L
* (if the matrix is not splitted.) At the end of each block
* is stored the corresponding shift as given by DLARRE.
* On exit, L is overwritten.
*
* PIVMIN (in) DOUBLE PRECISION
* The minimum pivot allowed in the Sturm sequence.
*
* ISPLIT (input) INTEGER array, dimension (N)
* The splitting points, at which T breaks up into blocks.
* The first block consists of rows/columns 1 to
* ISPLIT( 1 ), the second of rows/columns ISPLIT( 1 )+1
* through ISPLIT( 2 ), etc.
*
* M (input) INTEGER
* The total number of input eigenvalues. 0 <= M <= N.
*
* DOL (input) INTEGER
* DOU (input) INTEGER
* If the user wants to compute only selected eigenvectors from
* the eigenvalues supplied, he can specify an index range DOL:D
* Or else the setting DOL=1, DOU=M should be applied.
* Note that DOL and DOU refer to the order in which the eigenva
* are stored in W.
* If the user wants to compute only selected eigenpairs, then
* the columns DOL-1 to DOU+1 of the eigenvector space Z contain
* computed eigenvectors. All other columns of Z are set to zero
*
* MINRGP (input) DOUBLE PRECISION
*
* RTOL1 (input) DOUBLE PRECISION
* RTOL2 (input) DOUBLE PRECISION
* Parameters for bisection.
* An interval [LEFT,RIGHT] has converged if
* RIGHT-LEFT.LT.MAX( RTOL1*GAP, RTOL2*MAX(|LEFT|,|RIGHT|) )
*
* W (input/output) DOUBLE PRECISION array, dimension (N)
* The first M elements of W contain the APPROXIMATE eigenvalues
* which eigenvectors are to be computed. The eigenvalues
* should be grouped by split-off block and ordered from
* smallest to largest within the block ( The output array
* W from DLARRE is expected here ). Furthermore, they are with
* respect to the shift of the corresponding root representation
* for their block. On exit, W holds the eigenvalues of the
* UNshifted matrix.
*
* WERR (input/output) DOUBLE PRECISION array, dimension (N)
* The first M elements contain the semiwidth of the uncertainty
* interval of the corresponding eigenvalue in W
*
* WGAP (input/output) DOUBLE PRECISION array, dimension (N)
* The separation from the right neighbor eigenvalue in W.
*
* IBLOCK (input) INTEGER array, dimension (N)
* The indices of the blocks (submatrices) associated with the
* corresponding eigenvalues in W; IBLOCK(i)=1 if eigenvalue
* W(i) belongs to the first block from the top, =2 if W(i)
* belongs to the second block, etc.
*
* INDEXW (input) INTEGER array, dimension (N)
* The indices of the eigenvalues within each block (submatrix);
* for example, INDEXW(i)= 10 and IBLOCK(i)=2 imply that the
* i-th eigenvalue W(i) is the 10-th eigenvalue in the second bl
*
* GERS (input) DOUBLE PRECISION array, dimension (2*N)
* The N Gerschgorin intervals (the i-th Gerschgorin interval
* is (GERS(2*i-1), GERS(2*i)). The Gerschgorin intervals should
* be computed from the original UNshifted matrix.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, max(1,M) )
* If INFO = 0, the first M columns of Z contain the
* orthonormal eigenvectors of the matrix T
* corresponding to the input eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* ISUPPZ (output) INTEGER array, dimension ( 2*max(1,M) )
* The support of the eigenvectors in Z, i.e., the indices
* indicating the nonzero elements in Z. The I-th eigenvector
* is nonzero only in elements ISUPPZ( 2*I-1 ) through
* ISUPPZ( 2*I ).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (12*N)
*
* IWORK (workspace) INTEGER array, dimension (7*N)
*
* INFO (output) INTEGER
* = 0: successful exit
*
* > 0: A problem occured in DLARRV.
* < 0: One of the called subroutines signaled an internal prob
* Needs inspection of the corresponding parameter IINFO
* for further information.
*
* =-1: Problem in DLARRB when refining a child's eigenvalues.
* =-2: Problem in DLARRF when computing the RRR of a child.
* When a child is inside a tight cluster, it can be diffi
* to find an RRR. A partial remedy from the user's point
* view is to make the parameter MINRGP smaller and recomp
* However, as the orthogonality of the computed vectors i
* proportional to 1/MINRGP, the user should be aware that
* he might be trading in precision when he decreases MINR
* =-3: Problem in DLARRB when refining a single eigenvalue
* after the Rayleigh correction was rejected.
* = 5: The Rayleigh Quotient Iteration failed to converge to
* full accuracy in MAXITR steps.
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param vl
* @param vu
* @param d
* @param l
* @param pivmin
* @param isplit
* @param m
* @param dol
* @param dou
* @param minrgp
* @param rtol1
* @param rtol2
* @param w
* @param werr
* @param wgap
* @param iblock
* @param indexw
* @param gers
* @param z
* @param ldz
* @param isuppz
* @param work
* @param iwork
* @param info
*
*/
abstract public void dlarrv(int n, double vl, double vu, double[] d, double[] l, double pivmin, int[] isplit, int m, int dol, int dou, double minrgp, org.netlib.util.doubleW rtol1, org.netlib.util.doubleW rtol2, double[] w, double[] werr, double[] wgap, int[] iblock, int[] indexw, double[] gers, double[] z, int ldz, int[] isuppz, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLARRV computes the eigenvectors of the tridiagonal matrix
* T = L D L^T given L, D and APPROXIMATIONS to the eigenvalues of L D L
* The input eigenvalues should have been computed by DLARRE.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* VL (input) DOUBLE PRECISION
* VU (input) DOUBLE PRECISION
* Lower and upper bounds of the interval that contains the desi
* eigenvalues. VL < VU. Needed to compute gaps on the left or r
* end of the extremal eigenvalues in the desired RANGE.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the N diagonal elements of the diagonal matrix D.
* On exit, D may be overwritten.
*
* L (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the (N-1) subdiagonal elements of the unit
* bidiagonal matrix L are in elements 1 to N-1 of L
* (if the matrix is not splitted.) At the end of each block
* is stored the corresponding shift as given by DLARRE.
* On exit, L is overwritten.
*
* PIVMIN (in) DOUBLE PRECISION
* The minimum pivot allowed in the Sturm sequence.
*
* ISPLIT (input) INTEGER array, dimension (N)
* The splitting points, at which T breaks up into blocks.
* The first block consists of rows/columns 1 to
* ISPLIT( 1 ), the second of rows/columns ISPLIT( 1 )+1
* through ISPLIT( 2 ), etc.
*
* M (input) INTEGER
* The total number of input eigenvalues. 0 <= M <= N.
*
* DOL (input) INTEGER
* DOU (input) INTEGER
* If the user wants to compute only selected eigenvectors from
* the eigenvalues supplied, he can specify an index range DOL:D
* Or else the setting DOL=1, DOU=M should be applied.
* Note that DOL and DOU refer to the order in which the eigenva
* are stored in W.
* If the user wants to compute only selected eigenpairs, then
* the columns DOL-1 to DOU+1 of the eigenvector space Z contain
* computed eigenvectors. All other columns of Z are set to zero
*
* MINRGP (input) DOUBLE PRECISION
*
* RTOL1 (input) DOUBLE PRECISION
* RTOL2 (input) DOUBLE PRECISION
* Parameters for bisection.
* An interval [LEFT,RIGHT] has converged if
* RIGHT-LEFT.LT.MAX( RTOL1*GAP, RTOL2*MAX(|LEFT|,|RIGHT|) )
*
* W (input/output) DOUBLE PRECISION array, dimension (N)
* The first M elements of W contain the APPROXIMATE eigenvalues
* which eigenvectors are to be computed. The eigenvalues
* should be grouped by split-off block and ordered from
* smallest to largest within the block ( The output array
* W from DLARRE is expected here ). Furthermore, they are with
* respect to the shift of the corresponding root representation
* for their block. On exit, W holds the eigenvalues of the
* UNshifted matrix.
*
* WERR (input/output) DOUBLE PRECISION array, dimension (N)
* The first M elements contain the semiwidth of the uncertainty
* interval of the corresponding eigenvalue in W
*
* WGAP (input/output) DOUBLE PRECISION array, dimension (N)
* The separation from the right neighbor eigenvalue in W.
*
* IBLOCK (input) INTEGER array, dimension (N)
* The indices of the blocks (submatrices) associated with the
* corresponding eigenvalues in W; IBLOCK(i)=1 if eigenvalue
* W(i) belongs to the first block from the top, =2 if W(i)
* belongs to the second block, etc.
*
* INDEXW (input) INTEGER array, dimension (N)
* The indices of the eigenvalues within each block (submatrix);
* for example, INDEXW(i)= 10 and IBLOCK(i)=2 imply that the
* i-th eigenvalue W(i) is the 10-th eigenvalue in the second bl
*
* GERS (input) DOUBLE PRECISION array, dimension (2*N)
* The N Gerschgorin intervals (the i-th Gerschgorin interval
* is (GERS(2*i-1), GERS(2*i)). The Gerschgorin intervals should
* be computed from the original UNshifted matrix.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, max(1,M) )
* If INFO = 0, the first M columns of Z contain the
* orthonormal eigenvectors of the matrix T
* corresponding to the input eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* ISUPPZ (output) INTEGER array, dimension ( 2*max(1,M) )
* The support of the eigenvectors in Z, i.e., the indices
* indicating the nonzero elements in Z. The I-th eigenvector
* is nonzero only in elements ISUPPZ( 2*I-1 ) through
* ISUPPZ( 2*I ).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (12*N)
*
* IWORK (workspace) INTEGER array, dimension (7*N)
*
* INFO (output) INTEGER
* = 0: successful exit
*
* > 0: A problem occured in DLARRV.
* < 0: One of the called subroutines signaled an internal prob
* Needs inspection of the corresponding parameter IINFO
* for further information.
*
* =-1: Problem in DLARRB when refining a child's eigenvalues.
* =-2: Problem in DLARRF when computing the RRR of a child.
* When a child is inside a tight cluster, it can be diffi
* to find an RRR. A partial remedy from the user's point
* view is to make the parameter MINRGP smaller and recomp
* However, as the orthogonality of the computed vectors i
* proportional to 1/MINRGP, the user should be aware that
* he might be trading in precision when he decreases MINR
* =-3: Problem in DLARRB when refining a single eigenvalue
* after the Rayleigh correction was rejected.
* = 5: The Rayleigh Quotient Iteration failed to converge to
* full accuracy in MAXITR steps.
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param vl
* @param vu
* @param d
* @param _d_offset
* @param l
* @param _l_offset
* @param pivmin
* @param isplit
* @param _isplit_offset
* @param m
* @param dol
* @param dou
* @param minrgp
* @param rtol1
* @param rtol2
* @param w
* @param _w_offset
* @param werr
* @param _werr_offset
* @param wgap
* @param _wgap_offset
* @param iblock
* @param _iblock_offset
* @param indexw
* @param _indexw_offset
* @param gers
* @param _gers_offset
* @param z
* @param _z_offset
* @param ldz
* @param isuppz
* @param _isuppz_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dlarrv(int n, double vl, double vu, double[] d, int _d_offset, double[] l, int _l_offset, double pivmin, int[] isplit, int _isplit_offset, int m, int dol, int dou, double minrgp, org.netlib.util.doubleW rtol1, org.netlib.util.doubleW rtol2, double[] w, int _w_offset, double[] werr, int _werr_offset, double[] wgap, int _wgap_offset, int[] iblock, int _iblock_offset, int[] indexw, int _indexw_offset, double[] gers, int _gers_offset, double[] z, int _z_offset, int ldz, int[] isuppz, int _isuppz_offset, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLARTG generate a plane rotation so that
*
* [ CS SN ] . [ F ] = [ R ] where CS**2 + SN**2 = 1.
* [ -SN CS ] [ G ] [ 0 ]
*
* This is a slower, more accurate version of the BLAS1 routine DROTG,
* with the following other differences:
* F and G are unchanged on return.
* If G=0, then CS=1 and SN=0.
* If F=0 and (G .ne. 0), then CS=0 and SN=1 without doing any
* floating point operations (saves work in DBDSQR when
* there are zeros on the diagonal).
*
* If F exceeds G in magnitude, CS will be positive.
*
* Arguments
* =========
*
* F (input) DOUBLE PRECISION
* The first component of vector to be rotated.
*
* G (input) DOUBLE PRECISION
* The second component of vector to be rotated.
*
* CS (output) DOUBLE PRECISION
* The cosine of the rotation.
*
* SN (output) DOUBLE PRECISION
* The sine of the rotation.
*
* R (output) DOUBLE PRECISION
* The nonzero component of the rotated vector.
*
* This version has a few statements commented out for thread safety
* (machine parameters are computed on each entry). 10 feb 03, SJH.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param f
* @param g
* @param cs
* @param sn
* @param r
*
*/
abstract public void dlartg(double f, double g, org.netlib.util.doubleW cs, org.netlib.util.doubleW sn, org.netlib.util.doubleW r);
/**
*
* ..
*
* Purpose
* =======
*
* DLARTV applies a vector of real plane rotations to elements of the
* real vectors x and y. For i = 1,2,...,n
*
* ( x(i) ) := ( c(i) s(i) ) ( x(i) )
* ( y(i) ) ( -s(i) c(i) ) ( y(i) )
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of plane rotations to be applied.
*
* X (input/output) DOUBLE PRECISION array,
* dimension (1+(N-1)*INCX)
* The vector x.
*
* INCX (input) INTEGER
* The increment between elements of X. INCX > 0.
*
* Y (input/output) DOUBLE PRECISION array,
* dimension (1+(N-1)*INCY)
* The vector y.
*
* INCY (input) INTEGER
* The increment between elements of Y. INCY > 0.
*
* C (input) DOUBLE PRECISION array, dimension (1+(N-1)*INCC)
* The cosines of the plane rotations.
*
* S (input) DOUBLE PRECISION array, dimension (1+(N-1)*INCC)
* The sines of the plane rotations.
*
* INCC (input) INTEGER
* The increment between elements of C and S. INCC > 0.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n
* @param x
* @param incx
* @param y
* @param incy
* @param c
* @param s
* @param incc
*
*/
abstract public void dlartv(int n, double[] x, int incx, double[] y, int incy, double[] c, double[] s, int incc);
/**
*
* ..
*
* Purpose
* =======
*
* DLARTV applies a vector of real plane rotations to elements of the
* real vectors x and y. For i = 1,2,...,n
*
* ( x(i) ) := ( c(i) s(i) ) ( x(i) )
* ( y(i) ) ( -s(i) c(i) ) ( y(i) )
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of plane rotations to be applied.
*
* X (input/output) DOUBLE PRECISION array,
* dimension (1+(N-1)*INCX)
* The vector x.
*
* INCX (input) INTEGER
* The increment between elements of X. INCX > 0.
*
* Y (input/output) DOUBLE PRECISION array,
* dimension (1+(N-1)*INCY)
* The vector y.
*
* INCY (input) INTEGER
* The increment between elements of Y. INCY > 0.
*
* C (input) DOUBLE PRECISION array, dimension (1+(N-1)*INCC)
* The cosines of the plane rotations.
*
* S (input) DOUBLE PRECISION array, dimension (1+(N-1)*INCC)
* The sines of the plane rotations.
*
* INCC (input) INTEGER
* The increment between elements of C and S. INCC > 0.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n
* @param x
* @param _x_offset
* @param incx
* @param y
* @param _y_offset
* @param incy
* @param c
* @param _c_offset
* @param s
* @param _s_offset
* @param incc
*
*/
abstract public void dlartv(int n, double[] x, int _x_offset, int incx, double[] y, int _y_offset, int incy, double[] c, int _c_offset, double[] s, int _s_offset, int incc);
/**
*
* ..
*
* Purpose
* =======
*
* DLARUV returns a vector of n random real numbers from a uniform (0,1)
* distribution (n <= 128).
*
* This is an auxiliary routine called by DLARNV and ZLARNV.
*
* Arguments
* =========
*
* ISEED (input/output) INTEGER array, dimension (4)
* On entry, the seed of the random number generator; the array
* elements must be between 0 and 4095, and ISEED(4) must be
* odd.
* On exit, the seed is updated.
*
* N (input) INTEGER
* The number of random numbers to be generated. N <= 128.
*
* X (output) DOUBLE PRECISION array, dimension (N)
* The generated random numbers.
*
* Further Details
* ===============
*
* This routine uses a multiplicative congruential method with modulus
* 2**48 and multiplier 33952834046453 (see G.S.Fishman,
* 'Multiplicative congruential random number generators with modulus
* 2**b: an exhaustive analysis for b = 32 and a partial analysis for
* b = 48', Math. Comp. 189, pp 331-344, 1990).
*
* 48-bit integers are stored in 4 integer array elements with 12 bits
* per element. Hence the routine is portable across machines with
* integers of 32 bits or more.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param iseed
* @param n
* @param x
*
*/
abstract public void dlaruv(int[] iseed, int n, double[] x);
/**
*
* ..
*
* Purpose
* =======
*
* DLARUV returns a vector of n random real numbers from a uniform (0,1)
* distribution (n <= 128).
*
* This is an auxiliary routine called by DLARNV and ZLARNV.
*
* Arguments
* =========
*
* ISEED (input/output) INTEGER array, dimension (4)
* On entry, the seed of the random number generator; the array
* elements must be between 0 and 4095, and ISEED(4) must be
* odd.
* On exit, the seed is updated.
*
* N (input) INTEGER
* The number of random numbers to be generated. N <= 128.
*
* X (output) DOUBLE PRECISION array, dimension (N)
* The generated random numbers.
*
* Further Details
* ===============
*
* This routine uses a multiplicative congruential method with modulus
* 2**48 and multiplier 33952834046453 (see G.S.Fishman,
* 'Multiplicative congruential random number generators with modulus
* 2**b: an exhaustive analysis for b = 32 and a partial analysis for
* b = 48', Math. Comp. 189, pp 331-344, 1990).
*
* 48-bit integers are stored in 4 integer array elements with 12 bits
* per element. Hence the routine is portable across machines with
* integers of 32 bits or more.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param iseed
* @param _iseed_offset
* @param n
* @param x
* @param _x_offset
*
*/
abstract public void dlaruv(int[] iseed, int _iseed_offset, int n, double[] x, int _x_offset);
/**
*
* ..
*
* Purpose
* =======
*
* DLARZ applies a real elementary reflector H to a real M-by-N
* matrix C, from either the left or the right. H is represented in the
* form
*
* H = I - tau * v * v'
*
* where tau is a real scalar and v is a real vector.
*
* If tau = 0, then H is taken to be the unit matrix.
*
*
* H is a product of k elementary reflectors as returned by DTZRZF.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': form H * C
* = 'R': form C * H
*
* M (input) INTEGER
* The number of rows of the matrix C.
*
* N (input) INTEGER
* The number of columns of the matrix C.
*
* L (input) INTEGER
* The number of entries of the vector V containing
* the meaningful part of the Householder vectors.
* If SIDE = 'L', M >= L >= 0, if SIDE = 'R', N >= L >= 0.
*
* V (input) DOUBLE PRECISION array, dimension (1+(L-1)*abs(INCV))
* The vector v in the representation of H as returned by
* DTZRZF. V is not used if TAU = 0.
*
* INCV (input) INTEGER
* The increment between elements of v. INCV <> 0.
*
* TAU (input) DOUBLE PRECISION
* The value tau in the representation of H.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by the matrix H * C if SIDE = 'L',
* or C * H if SIDE = 'R'.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) DOUBLE PRECISION array, dimension
* (N) if SIDE = 'L'
* or (M) if SIDE = 'R'
*
* Further Details
* ===============
*
* Based on contributions by
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param m
* @param n
* @param l
* @param v
* @param incv
* @param tau
* @param c
* @param Ldc
* @param work
*
*/
abstract public void dlarz(java.lang.String side, int m, int n, int l, double[] v, int incv, double tau, double[] c, int Ldc, double[] work);
/**
*
* ..
*
* Purpose
* =======
*
* DLARZ applies a real elementary reflector H to a real M-by-N
* matrix C, from either the left or the right. H is represented in the
* form
*
* H = I - tau * v * v'
*
* where tau is a real scalar and v is a real vector.
*
* If tau = 0, then H is taken to be the unit matrix.
*
*
* H is a product of k elementary reflectors as returned by DTZRZF.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': form H * C
* = 'R': form C * H
*
* M (input) INTEGER
* The number of rows of the matrix C.
*
* N (input) INTEGER
* The number of columns of the matrix C.
*
* L (input) INTEGER
* The number of entries of the vector V containing
* the meaningful part of the Householder vectors.
* If SIDE = 'L', M >= L >= 0, if SIDE = 'R', N >= L >= 0.
*
* V (input) DOUBLE PRECISION array, dimension (1+(L-1)*abs(INCV))
* The vector v in the representation of H as returned by
* DTZRZF. V is not used if TAU = 0.
*
* INCV (input) INTEGER
* The increment between elements of v. INCV <> 0.
*
* TAU (input) DOUBLE PRECISION
* The value tau in the representation of H.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by the matrix H * C if SIDE = 'L',
* or C * H if SIDE = 'R'.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) DOUBLE PRECISION array, dimension
* (N) if SIDE = 'L'
* or (M) if SIDE = 'R'
*
* Further Details
* ===============
*
* Based on contributions by
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param m
* @param n
* @param l
* @param v
* @param _v_offset
* @param incv
* @param tau
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
*
*/
abstract public void dlarz(java.lang.String side, int m, int n, int l, double[] v, int _v_offset, int incv, double tau, double[] c, int _c_offset, int Ldc, double[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* DLARZB applies a real block reflector H or its transpose H**T to
* a real distributed M-by-N C from the left or the right.
*
* Currently, only STOREV = 'R' and DIRECT = 'B' are supported.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply H or H' from the Left
* = 'R': apply H or H' from the Right
*
* TRANS (input) CHARACTER*1
* = 'N': apply H (No transpose)
* = 'C': apply H' (Transpose)
*
* DIRECT (input) CHARACTER*1
* Indicates how H is formed from a product of elementary
* reflectors
* = 'F': H = H(1) H(2) . . . H(k) (Forward, not supported yet)
* = 'B': H = H(k) . . . H(2) H(1) (Backward)
*
* STOREV (input) CHARACTER*1
* Indicates how the vectors which define the elementary
* reflectors are stored:
* = 'C': Columnwise (not supported yet)
* = 'R': Rowwise
*
* M (input) INTEGER
* The number of rows of the matrix C.
*
* N (input) INTEGER
* The number of columns of the matrix C.
*
* K (input) INTEGER
* The order of the matrix T (= the number of elementary
* reflectors whose product defines the block reflector).
*
* L (input) INTEGER
* The number of columns of the matrix V containing the
* meaningful part of the Householder reflectors.
* If SIDE = 'L', M >= L >= 0, if SIDE = 'R', N >= L >= 0.
*
* V (input) DOUBLE PRECISION array, dimension (LDV,NV).
* If STOREV = 'C', NV = K; if STOREV = 'R', NV = L.
*
* LDV (input) INTEGER
* The leading dimension of the array V.
* If STOREV = 'C', LDV >= L; if STOREV = 'R', LDV >= K.
*
* T (input) DOUBLE PRECISION array, dimension (LDT,K)
* The triangular K-by-K matrix T in the representation of the
* block reflector.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= K.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by H*C or H'*C or C*H or C*H'.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (LDWORK,K)
*
* LDWORK (input) INTEGER
* The leading dimension of the array WORK.
* If SIDE = 'L', LDWORK >= max(1,N);
* if SIDE = 'R', LDWORK >= max(1,M).
*
* Further Details
* ===============
*
* Based on contributions by
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param direct
* @param storev
* @param m
* @param n
* @param k
* @param l
* @param v
* @param ldv
* @param t
* @param ldt
* @param c
* @param Ldc
* @param work
* @param ldwork
*
*/
abstract public void dlarzb(java.lang.String side, java.lang.String trans, java.lang.String direct, java.lang.String storev, int m, int n, int k, int l, double[] v, int ldv, double[] t, int ldt, double[] c, int Ldc, double[] work, int ldwork);
/**
*
* ..
*
* Purpose
* =======
*
* DLARZB applies a real block reflector H or its transpose H**T to
* a real distributed M-by-N C from the left or the right.
*
* Currently, only STOREV = 'R' and DIRECT = 'B' are supported.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply H or H' from the Left
* = 'R': apply H or H' from the Right
*
* TRANS (input) CHARACTER*1
* = 'N': apply H (No transpose)
* = 'C': apply H' (Transpose)
*
* DIRECT (input) CHARACTER*1
* Indicates how H is formed from a product of elementary
* reflectors
* = 'F': H = H(1) H(2) . . . H(k) (Forward, not supported yet)
* = 'B': H = H(k) . . . H(2) H(1) (Backward)
*
* STOREV (input) CHARACTER*1
* Indicates how the vectors which define the elementary
* reflectors are stored:
* = 'C': Columnwise (not supported yet)
* = 'R': Rowwise
*
* M (input) INTEGER
* The number of rows of the matrix C.
*
* N (input) INTEGER
* The number of columns of the matrix C.
*
* K (input) INTEGER
* The order of the matrix T (= the number of elementary
* reflectors whose product defines the block reflector).
*
* L (input) INTEGER
* The number of columns of the matrix V containing the
* meaningful part of the Householder reflectors.
* If SIDE = 'L', M >= L >= 0, if SIDE = 'R', N >= L >= 0.
*
* V (input) DOUBLE PRECISION array, dimension (LDV,NV).
* If STOREV = 'C', NV = K; if STOREV = 'R', NV = L.
*
* LDV (input) INTEGER
* The leading dimension of the array V.
* If STOREV = 'C', LDV >= L; if STOREV = 'R', LDV >= K.
*
* T (input) DOUBLE PRECISION array, dimension (LDT,K)
* The triangular K-by-K matrix T in the representation of the
* block reflector.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= K.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by H*C or H'*C or C*H or C*H'.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (LDWORK,K)
*
* LDWORK (input) INTEGER
* The leading dimension of the array WORK.
* If SIDE = 'L', LDWORK >= max(1,N);
* if SIDE = 'R', LDWORK >= max(1,M).
*
* Further Details
* ===============
*
* Based on contributions by
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param direct
* @param storev
* @param m
* @param n
* @param k
* @param l
* @param v
* @param _v_offset
* @param ldv
* @param t
* @param _t_offset
* @param ldt
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param ldwork
*
*/
abstract public void dlarzb(java.lang.String side, java.lang.String trans, java.lang.String direct, java.lang.String storev, int m, int n, int k, int l, double[] v, int _v_offset, int ldv, double[] t, int _t_offset, int ldt, double[] c, int _c_offset, int Ldc, double[] work, int _work_offset, int ldwork);
/**
*
* ..
*
* Purpose
* =======
*
* DLARZT forms the triangular factor T of a real block reflector
* H of order > n, which is defined as a product of k elementary
* reflectors.
*
* If DIRECT = 'F', H = H(1) H(2) . . . H(k) and T is upper triangular;
*
* If DIRECT = 'B', H = H(k) . . . H(2) H(1) and T is lower triangular.
*
* If STOREV = 'C', the vector which defines the elementary reflector
* H(i) is stored in the i-th column of the array V, and
*
* H = I - V * T * V'
*
* If STOREV = 'R', the vector which defines the elementary reflector
* H(i) is stored in the i-th row of the array V, and
*
* H = I - V' * T * V
*
* Currently, only STOREV = 'R' and DIRECT = 'B' are supported.
*
* Arguments
* =========
*
* DIRECT (input) CHARACTER*1
* Specifies the order in which the elementary reflectors are
* multiplied to form the block reflector:
* = 'F': H = H(1) H(2) . . . H(k) (Forward, not supported yet)
* = 'B': H = H(k) . . . H(2) H(1) (Backward)
*
* STOREV (input) CHARACTER*1
* Specifies how the vectors which define the elementary
* reflectors are stored (see also Further Details):
* = 'C': columnwise (not supported yet)
* = 'R': rowwise
*
* N (input) INTEGER
* The order of the block reflector H. N >= 0.
*
* K (input) INTEGER
* The order of the triangular factor T (= the number of
* elementary reflectors). K >= 1.
*
* V (input/output) DOUBLE PRECISION array, dimension
* (LDV,K) if STOREV = 'C'
* (LDV,N) if STOREV = 'R'
* The matrix V. See further details.
*
* LDV (input) INTEGER
* The leading dimension of the array V.
* If STOREV = 'C', LDV >= max(1,N); if STOREV = 'R', LDV >= K.
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i).
*
* T (output) DOUBLE PRECISION array, dimension (LDT,K)
* The k by k triangular factor T of the block reflector.
* If DIRECT = 'F', T is upper triangular; if DIRECT = 'B', T is
* lower triangular. The rest of the array is not used.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= K.
*
* Further Details
* ===============
*
* Based on contributions by
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
*
* The shape of the matrix V and the storage of the vectors which define
* the H(i) is best illustrated by the following example with n = 5 and
* k = 3. The elements equal to 1 are not stored; the corresponding
* array elements are modified but restored on exit. The rest of the
* array is not used.
*
* DIRECT = 'F' and STOREV = 'C': DIRECT = 'F' and STOREV = 'R':
*
* ______V_____
* ( v1 v2 v3 ) / \
* ( v1 v2 v3 ) ( v1 v1 v1 v1 v1 . . . . 1 )
* V = ( v1 v2 v3 ) ( v2 v2 v2 v2 v2 . . . 1 )
* ( v1 v2 v3 ) ( v3 v3 v3 v3 v3 . . 1 )
* ( v1 v2 v3 )
* . . .
* . . .
* 1 . .
* 1 .
* 1
*
* DIRECT = 'B' and STOREV = 'C': DIRECT = 'B' and STOREV = 'R':
*
* ______V_____
* 1 / \
* . 1 ( 1 . . . . v1 v1 v1 v1 v1 )
* . . 1 ( . 1 . . . v2 v2 v2 v2 v2 )
* . . . ( . . 1 . . v3 v3 v3 v3 v3 )
* . . .
* ( v1 v2 v3 )
* ( v1 v2 v3 )
* V = ( v1 v2 v3 )
* ( v1 v2 v3 )
* ( v1 v2 v3 )
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param direct
* @param storev
* @param n
* @param k
* @param v
* @param ldv
* @param tau
* @param t
* @param ldt
*
*/
abstract public void dlarzt(java.lang.String direct, java.lang.String storev, int n, int k, double[] v, int ldv, double[] tau, double[] t, int ldt);
/**
*
* ..
*
* Purpose
* =======
*
* DLARZT forms the triangular factor T of a real block reflector
* H of order > n, which is defined as a product of k elementary
* reflectors.
*
* If DIRECT = 'F', H = H(1) H(2) . . . H(k) and T is upper triangular;
*
* If DIRECT = 'B', H = H(k) . . . H(2) H(1) and T is lower triangular.
*
* If STOREV = 'C', the vector which defines the elementary reflector
* H(i) is stored in the i-th column of the array V, and
*
* H = I - V * T * V'
*
* If STOREV = 'R', the vector which defines the elementary reflector
* H(i) is stored in the i-th row of the array V, and
*
* H = I - V' * T * V
*
* Currently, only STOREV = 'R' and DIRECT = 'B' are supported.
*
* Arguments
* =========
*
* DIRECT (input) CHARACTER*1
* Specifies the order in which the elementary reflectors are
* multiplied to form the block reflector:
* = 'F': H = H(1) H(2) . . . H(k) (Forward, not supported yet)
* = 'B': H = H(k) . . . H(2) H(1) (Backward)
*
* STOREV (input) CHARACTER*1
* Specifies how the vectors which define the elementary
* reflectors are stored (see also Further Details):
* = 'C': columnwise (not supported yet)
* = 'R': rowwise
*
* N (input) INTEGER
* The order of the block reflector H. N >= 0.
*
* K (input) INTEGER
* The order of the triangular factor T (= the number of
* elementary reflectors). K >= 1.
*
* V (input/output) DOUBLE PRECISION array, dimension
* (LDV,K) if STOREV = 'C'
* (LDV,N) if STOREV = 'R'
* The matrix V. See further details.
*
* LDV (input) INTEGER
* The leading dimension of the array V.
* If STOREV = 'C', LDV >= max(1,N); if STOREV = 'R', LDV >= K.
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i).
*
* T (output) DOUBLE PRECISION array, dimension (LDT,K)
* The k by k triangular factor T of the block reflector.
* If DIRECT = 'F', T is upper triangular; if DIRECT = 'B', T is
* lower triangular. The rest of the array is not used.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= K.
*
* Further Details
* ===============
*
* Based on contributions by
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
*
* The shape of the matrix V and the storage of the vectors which define
* the H(i) is best illustrated by the following example with n = 5 and
* k = 3. The elements equal to 1 are not stored; the corresponding
* array elements are modified but restored on exit. The rest of the
* array is not used.
*
* DIRECT = 'F' and STOREV = 'C': DIRECT = 'F' and STOREV = 'R':
*
* ______V_____
* ( v1 v2 v3 ) / \
* ( v1 v2 v3 ) ( v1 v1 v1 v1 v1 . . . . 1 )
* V = ( v1 v2 v3 ) ( v2 v2 v2 v2 v2 . . . 1 )
* ( v1 v2 v3 ) ( v3 v3 v3 v3 v3 . . 1 )
* ( v1 v2 v3 )
* . . .
* . . .
* 1 . .
* 1 .
* 1
*
* DIRECT = 'B' and STOREV = 'C': DIRECT = 'B' and STOREV = 'R':
*
* ______V_____
* 1 / \
* . 1 ( 1 . . . . v1 v1 v1 v1 v1 )
* . . 1 ( . 1 . . . v2 v2 v2 v2 v2 )
* . . . ( . . 1 . . v3 v3 v3 v3 v3 )
* . . .
* ( v1 v2 v3 )
* ( v1 v2 v3 )
* V = ( v1 v2 v3 )
* ( v1 v2 v3 )
* ( v1 v2 v3 )
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param direct
* @param storev
* @param n
* @param k
* @param v
* @param _v_offset
* @param ldv
* @param tau
* @param _tau_offset
* @param t
* @param _t_offset
* @param ldt
*
*/
abstract public void dlarzt(java.lang.String direct, java.lang.String storev, int n, int k, double[] v, int _v_offset, int ldv, double[] tau, int _tau_offset, double[] t, int _t_offset, int ldt);
/**
*
* ..
*
* Purpose
* =======
*
* DLAS2 computes the singular values of the 2-by-2 matrix
* [ F G ]
* [ 0 H ].
* On return, SSMIN is the smaller singular value and SSMAX is the
* larger singular value.
*
* Arguments
* =========
*
* F (input) DOUBLE PRECISION
* The (1,1) element of the 2-by-2 matrix.
*
* G (input) DOUBLE PRECISION
* The (1,2) element of the 2-by-2 matrix.
*
* H (input) DOUBLE PRECISION
* The (2,2) element of the 2-by-2 matrix.
*
* SSMIN (output) DOUBLE PRECISION
* The smaller singular value.
*
* SSMAX (output) DOUBLE PRECISION
* The larger singular value.
*
* Further Details
* ===============
*
* Barring over/underflow, all output quantities are correct to within
* a few units in the last place (ulps), even in the absence of a guard
* digit in addition/subtraction.
*
* In IEEE arithmetic, the code works correctly if one matrix element is
* infinite.
*
* Overflow will not occur unless the largest singular value itself
* overflows, or is within a few ulps of overflow. (On machines with
* partial overflow, like the Cray, overflow may occur if the largest
* singular value is within a factor of 2 of overflow.)
*
* Underflow is harmless if underflow is gradual. Otherwise, results
* may correspond to a matrix modified by perturbations of size near
* the underflow threshold.
*
* ====================================================================
*
* .. Parameters ..
*
*
* @param f
* @param g
* @param h
* @param ssmin
* @param ssmax
*
*/
abstract public void dlas2(double f, double g, double h, org.netlib.util.doubleW ssmin, org.netlib.util.doubleW ssmax);
/**
*
* ..
*
* Purpose
* =======
*
* DLASCL multiplies the M by N real matrix A by the real scalar
* CTO/CFROM. This is done without over/underflow as long as the final
* result CTO*A(I,J)/CFROM does not over/underflow. TYPE specifies that
* A may be full, upper triangular, lower triangular, upper Hessenberg,
* or banded.
*
* Arguments
* =========
*
* TYPE (input) CHARACTER*1
* TYPE indices the storage type of the input matrix.
* = 'G': A is a full matrix.
* = 'L': A is a lower triangular matrix.
* = 'U': A is an upper triangular matrix.
* = 'H': A is an upper Hessenberg matrix.
* = 'B': A is a symmetric band matrix with lower bandwidth KL
* and upper bandwidth KU and with the only the lower
* half stored.
* = 'Q': A is a symmetric band matrix with lower bandwidth KL
* and upper bandwidth KU and with the only the upper
* half stored.
* = 'Z': A is a band matrix with lower bandwidth KL and upper
* bandwidth KU.
*
* KL (input) INTEGER
* The lower bandwidth of A. Referenced only if TYPE = 'B',
* 'Q' or 'Z'.
*
* KU (input) INTEGER
* The upper bandwidth of A. Referenced only if TYPE = 'B',
* 'Q' or 'Z'.
*
* CFROM (input) DOUBLE PRECISION
* CTO (input) DOUBLE PRECISION
* The matrix A is multiplied by CTO/CFROM. A(I,J) is computed
* without over/underflow if the final result CTO*A(I,J)/CFROM
* can be represented without over/underflow. CFROM must be
* nonzero.
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* The matrix to be multiplied by CTO/CFROM. See TYPE for the
* storage type.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* INFO (output) INTEGER
* 0 - successful exit
* <0 - if INFO = -i, the i-th argument had an illegal value.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param type
* @param kl
* @param ku
* @param cfrom
* @param cto
* @param m
* @param n
* @param a
* @param lda
* @param info
*
*/
abstract public void dlascl(java.lang.String type, int kl, int ku, double cfrom, double cto, int m, int n, double[] a, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLASCL multiplies the M by N real matrix A by the real scalar
* CTO/CFROM. This is done without over/underflow as long as the final
* result CTO*A(I,J)/CFROM does not over/underflow. TYPE specifies that
* A may be full, upper triangular, lower triangular, upper Hessenberg,
* or banded.
*
* Arguments
* =========
*
* TYPE (input) CHARACTER*1
* TYPE indices the storage type of the input matrix.
* = 'G': A is a full matrix.
* = 'L': A is a lower triangular matrix.
* = 'U': A is an upper triangular matrix.
* = 'H': A is an upper Hessenberg matrix.
* = 'B': A is a symmetric band matrix with lower bandwidth KL
* and upper bandwidth KU and with the only the lower
* half stored.
* = 'Q': A is a symmetric band matrix with lower bandwidth KL
* and upper bandwidth KU and with the only the upper
* half stored.
* = 'Z': A is a band matrix with lower bandwidth KL and upper
* bandwidth KU.
*
* KL (input) INTEGER
* The lower bandwidth of A. Referenced only if TYPE = 'B',
* 'Q' or 'Z'.
*
* KU (input) INTEGER
* The upper bandwidth of A. Referenced only if TYPE = 'B',
* 'Q' or 'Z'.
*
* CFROM (input) DOUBLE PRECISION
* CTO (input) DOUBLE PRECISION
* The matrix A is multiplied by CTO/CFROM. A(I,J) is computed
* without over/underflow if the final result CTO*A(I,J)/CFROM
* can be represented without over/underflow. CFROM must be
* nonzero.
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* The matrix to be multiplied by CTO/CFROM. See TYPE for the
* storage type.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* INFO (output) INTEGER
* 0 - successful exit
* <0 - if INFO = -i, the i-th argument had an illegal value.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param type
* @param kl
* @param ku
* @param cfrom
* @param cto
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param info
*
*/
abstract public void dlascl(java.lang.String type, int kl, int ku, double cfrom, double cto, int m, int n, double[] a, int _a_offset, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* Using a divide and conquer approach, DLASD0 computes the singular
* value decomposition (SVD) of a real upper bidiagonal N-by-M
* matrix B with diagonal D and offdiagonal E, where M = N + SQRE.
* The algorithm computes orthogonal matrices U and VT such that
* B = U * S * VT. The singular values S are overwritten on D.
*
* A related subroutine, DLASDA, computes only the singular values,
* and optionally, the singular vectors in compact form.
*
* Arguments
* =========
*
* N (input) INTEGER
* On entry, the row dimension of the upper bidiagonal matrix.
* This is also the dimension of the main diagonal array D.
*
* SQRE (input) INTEGER
* Specifies the column dimension of the bidiagonal matrix.
* = 0: The bidiagonal matrix has column dimension M = N;
* = 1: The bidiagonal matrix has column dimension M = N+1;
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry D contains the main diagonal of the bidiagonal
* matrix.
* On exit D, if INFO = 0, contains its singular values.
*
* E (input) DOUBLE PRECISION array, dimension (M-1)
* Contains the subdiagonal entries of the bidiagonal matrix.
* On exit, E has been destroyed.
*
* U (output) DOUBLE PRECISION array, dimension at least (LDQ, N)
* On exit, U contains the left singular vectors.
*
* LDU (input) INTEGER
* On entry, leading dimension of U.
*
* VT (output) DOUBLE PRECISION array, dimension at least (LDVT, M)
* On exit, VT' contains the right singular vectors.
*
* LDVT (input) INTEGER
* On entry, leading dimension of VT.
*
* SMLSIZ (input) INTEGER
* On entry, maximum size of the subproblems at the
* bottom of the computation tree.
*
* IWORK (workspace) INTEGER work array.
* Dimension must be at least (8 * N)
*
* WORK (workspace) DOUBLE PRECISION work array.
* Dimension must be at least (3 * M**2 + 2 * M)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an singular value did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n
* @param sqre
* @param d
* @param e
* @param u
* @param ldu
* @param vt
* @param ldvt
* @param smlsiz
* @param iwork
* @param work
* @param info
*
*/
abstract public void dlasd0(int n, int sqre, double[] d, double[] e, double[] u, int ldu, double[] vt, int ldvt, int smlsiz, int[] iwork, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* Using a divide and conquer approach, DLASD0 computes the singular
* value decomposition (SVD) of a real upper bidiagonal N-by-M
* matrix B with diagonal D and offdiagonal E, where M = N + SQRE.
* The algorithm computes orthogonal matrices U and VT such that
* B = U * S * VT. The singular values S are overwritten on D.
*
* A related subroutine, DLASDA, computes only the singular values,
* and optionally, the singular vectors in compact form.
*
* Arguments
* =========
*
* N (input) INTEGER
* On entry, the row dimension of the upper bidiagonal matrix.
* This is also the dimension of the main diagonal array D.
*
* SQRE (input) INTEGER
* Specifies the column dimension of the bidiagonal matrix.
* = 0: The bidiagonal matrix has column dimension M = N;
* = 1: The bidiagonal matrix has column dimension M = N+1;
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry D contains the main diagonal of the bidiagonal
* matrix.
* On exit D, if INFO = 0, contains its singular values.
*
* E (input) DOUBLE PRECISION array, dimension (M-1)
* Contains the subdiagonal entries of the bidiagonal matrix.
* On exit, E has been destroyed.
*
* U (output) DOUBLE PRECISION array, dimension at least (LDQ, N)
* On exit, U contains the left singular vectors.
*
* LDU (input) INTEGER
* On entry, leading dimension of U.
*
* VT (output) DOUBLE PRECISION array, dimension at least (LDVT, M)
* On exit, VT' contains the right singular vectors.
*
* LDVT (input) INTEGER
* On entry, leading dimension of VT.
*
* SMLSIZ (input) INTEGER
* On entry, maximum size of the subproblems at the
* bottom of the computation tree.
*
* IWORK (workspace) INTEGER work array.
* Dimension must be at least (8 * N)
*
* WORK (workspace) DOUBLE PRECISION work array.
* Dimension must be at least (3 * M**2 + 2 * M)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an singular value did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n
* @param sqre
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param u
* @param _u_offset
* @param ldu
* @param vt
* @param _vt_offset
* @param ldvt
* @param smlsiz
* @param iwork
* @param _iwork_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dlasd0(int n, int sqre, double[] d, int _d_offset, double[] e, int _e_offset, double[] u, int _u_offset, int ldu, double[] vt, int _vt_offset, int ldvt, int smlsiz, int[] iwork, int _iwork_offset, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLASD1 computes the SVD of an upper bidiagonal N-by-M matrix B,
* where N = NL + NR + 1 and M = N + SQRE. DLASD1 is called from DLASD0.
*
* A related subroutine DLASD7 handles the case in which the singular
* values (and the singular vectors in factored form) are desired.
*
* DLASD1 computes the SVD as follows:
*
* ( D1(in) 0 0 0 )
* B = U(in) * ( Z1' a Z2' b ) * VT(in)
* ( 0 0 D2(in) 0 )
*
* = U(out) * ( D(out) 0) * VT(out)
*
* where Z' = (Z1' a Z2' b) = u' VT', and u is a vector of dimension M
* with ALPHA and BETA in the NL+1 and NL+2 th entries and zeros
* elsewhere; and the entry b is empty if SQRE = 0.
*
* The left singular vectors of the original matrix are stored in U, and
* the transpose of the right singular vectors are stored in VT, and the
* singular values are in D. The algorithm consists of three stages:
*
* The first stage consists of deflating the size of the problem
* when there are multiple singular values or when there are zeros in
* the Z vector. For each such occurence the dimension of the
* secular equation problem is reduced by one. This stage is
* performed by the routine DLASD2.
*
* The second stage consists of calculating the updated
* singular values. This is done by finding the square roots of the
* roots of the secular equation via the routine DLASD4 (as called
* by DLASD3). This routine also calculates the singular vectors of
* the current problem.
*
* The final stage consists of computing the updated singular vectors
* directly using the updated singular values. The singular vectors
* for the current problem are multiplied with the singular vectors
* from the overall problem.
*
* Arguments
* =========
*
* NL (input) INTEGER
* The row dimension of the upper block. NL >= 1.
*
* NR (input) INTEGER
* The row dimension of the lower block. NR >= 1.
*
* SQRE (input) INTEGER
* = 0: the lower block is an NR-by-NR square matrix.
* = 1: the lower block is an NR-by-(NR+1) rectangular matrix.
*
* The bidiagonal matrix has row dimension N = NL + NR + 1,
* and column dimension M = N + SQRE.
*
* D (input/output) DOUBLE PRECISION array,
* dimension (N = NL+NR+1).
* On entry D(1:NL,1:NL) contains the singular values of the
* upper block; and D(NL+2:N) contains the singular values of
* the lower block. On exit D(1:N) contains the singular values
* of the modified matrix.
*
* ALPHA (input/output) DOUBLE PRECISION
* Contains the diagonal element associated with the added row.
*
* BETA (input/output) DOUBLE PRECISION
* Contains the off-diagonal element associated with the added
* row.
*
* U (input/output) DOUBLE PRECISION array, dimension(LDU,N)
* On entry U(1:NL, 1:NL) contains the left singular vectors of
* the upper block; U(NL+2:N, NL+2:N) contains the left singular
* vectors of the lower block. On exit U contains the left
* singular vectors of the bidiagonal matrix.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= max( 1, N ).
*
* VT (input/output) DOUBLE PRECISION array, dimension(LDVT,M)
* where M = N + SQRE.
* On entry VT(1:NL+1, 1:NL+1)' contains the right singular
* vectors of the upper block; VT(NL+2:M, NL+2:M)' contains
* the right singular vectors of the lower block. On exit
* VT' contains the right singular vectors of the
* bidiagonal matrix.
*
* LDVT (input) INTEGER
* The leading dimension of the array VT. LDVT >= max( 1, M ).
*
* IDXQ (output) INTEGER array, dimension(N)
* This contains the permutation which will reintegrate the
* subproblem just solved back into sorted order, i.e.
* D( IDXQ( I = 1, N ) ) will be in ascending order.
*
* IWORK (workspace) INTEGER array, dimension( 4 * N )
*
* WORK (workspace) DOUBLE PRECISION array, dimension( 3*M**2 + 2*M )
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an singular value did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
*
* @param nl
* @param nr
* @param sqre
* @param d
* @param alpha
* @param beta
* @param u
* @param ldu
* @param vt
* @param ldvt
* @param idxq
* @param iwork
* @param work
* @param info
*
*/
abstract public void dlasd1(int nl, int nr, int sqre, double[] d, org.netlib.util.doubleW alpha, org.netlib.util.doubleW beta, double[] u, int ldu, double[] vt, int ldvt, int[] idxq, int[] iwork, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLASD1 computes the SVD of an upper bidiagonal N-by-M matrix B,
* where N = NL + NR + 1 and M = N + SQRE. DLASD1 is called from DLASD0.
*
* A related subroutine DLASD7 handles the case in which the singular
* values (and the singular vectors in factored form) are desired.
*
* DLASD1 computes the SVD as follows:
*
* ( D1(in) 0 0 0 )
* B = U(in) * ( Z1' a Z2' b ) * VT(in)
* ( 0 0 D2(in) 0 )
*
* = U(out) * ( D(out) 0) * VT(out)
*
* where Z' = (Z1' a Z2' b) = u' VT', and u is a vector of dimension M
* with ALPHA and BETA in the NL+1 and NL+2 th entries and zeros
* elsewhere; and the entry b is empty if SQRE = 0.
*
* The left singular vectors of the original matrix are stored in U, and
* the transpose of the right singular vectors are stored in VT, and the
* singular values are in D. The algorithm consists of three stages:
*
* The first stage consists of deflating the size of the problem
* when there are multiple singular values or when there are zeros in
* the Z vector. For each such occurence the dimension of the
* secular equation problem is reduced by one. This stage is
* performed by the routine DLASD2.
*
* The second stage consists of calculating the updated
* singular values. This is done by finding the square roots of the
* roots of the secular equation via the routine DLASD4 (as called
* by DLASD3). This routine also calculates the singular vectors of
* the current problem.
*
* The final stage consists of computing the updated singular vectors
* directly using the updated singular values. The singular vectors
* for the current problem are multiplied with the singular vectors
* from the overall problem.
*
* Arguments
* =========
*
* NL (input) INTEGER
* The row dimension of the upper block. NL >= 1.
*
* NR (input) INTEGER
* The row dimension of the lower block. NR >= 1.
*
* SQRE (input) INTEGER
* = 0: the lower block is an NR-by-NR square matrix.
* = 1: the lower block is an NR-by-(NR+1) rectangular matrix.
*
* The bidiagonal matrix has row dimension N = NL + NR + 1,
* and column dimension M = N + SQRE.
*
* D (input/output) DOUBLE PRECISION array,
* dimension (N = NL+NR+1).
* On entry D(1:NL,1:NL) contains the singular values of the
* upper block; and D(NL+2:N) contains the singular values of
* the lower block. On exit D(1:N) contains the singular values
* of the modified matrix.
*
* ALPHA (input/output) DOUBLE PRECISION
* Contains the diagonal element associated with the added row.
*
* BETA (input/output) DOUBLE PRECISION
* Contains the off-diagonal element associated with the added
* row.
*
* U (input/output) DOUBLE PRECISION array, dimension(LDU,N)
* On entry U(1:NL, 1:NL) contains the left singular vectors of
* the upper block; U(NL+2:N, NL+2:N) contains the left singular
* vectors of the lower block. On exit U contains the left
* singular vectors of the bidiagonal matrix.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= max( 1, N ).
*
* VT (input/output) DOUBLE PRECISION array, dimension(LDVT,M)
* where M = N + SQRE.
* On entry VT(1:NL+1, 1:NL+1)' contains the right singular
* vectors of the upper block; VT(NL+2:M, NL+2:M)' contains
* the right singular vectors of the lower block. On exit
* VT' contains the right singular vectors of the
* bidiagonal matrix.
*
* LDVT (input) INTEGER
* The leading dimension of the array VT. LDVT >= max( 1, M ).
*
* IDXQ (output) INTEGER array, dimension(N)
* This contains the permutation which will reintegrate the
* subproblem just solved back into sorted order, i.e.
* D( IDXQ( I = 1, N ) ) will be in ascending order.
*
* IWORK (workspace) INTEGER array, dimension( 4 * N )
*
* WORK (workspace) DOUBLE PRECISION array, dimension( 3*M**2 + 2*M )
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an singular value did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
*
* @param nl
* @param nr
* @param sqre
* @param d
* @param _d_offset
* @param alpha
* @param beta
* @param u
* @param _u_offset
* @param ldu
* @param vt
* @param _vt_offset
* @param ldvt
* @param idxq
* @param _idxq_offset
* @param iwork
* @param _iwork_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dlasd1(int nl, int nr, int sqre, double[] d, int _d_offset, org.netlib.util.doubleW alpha, org.netlib.util.doubleW beta, double[] u, int _u_offset, int ldu, double[] vt, int _vt_offset, int ldvt, int[] idxq, int _idxq_offset, int[] iwork, int _iwork_offset, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLASD2 merges the two sets of singular values together into a single
* sorted set. Then it tries to deflate the size of the problem.
* There are two ways in which deflation can occur: when two or more
* singular values are close together or if there is a tiny entry in the
* Z vector. For each such occurrence the order of the related secular
* equation problem is reduced by one.
*
* DLASD2 is called from DLASD1.
*
* Arguments
* =========
*
* NL (input) INTEGER
* The row dimension of the upper block. NL >= 1.
*
* NR (input) INTEGER
* The row dimension of the lower block. NR >= 1.
*
* SQRE (input) INTEGER
* = 0: the lower block is an NR-by-NR square matrix.
* = 1: the lower block is an NR-by-(NR+1) rectangular matrix.
*
* The bidiagonal matrix has N = NL + NR + 1 rows and
* M = N + SQRE >= N columns.
*
* K (output) INTEGER
* Contains the dimension of the non-deflated matrix,
* This is the order of the related secular equation. 1 <= K <=N.
*
* D (input/output) DOUBLE PRECISION array, dimension(N)
* On entry D contains the singular values of the two submatrices
* to be combined. On exit D contains the trailing (N-K) updated
* singular values (those which were deflated) sorted into
* increasing order.
*
* Z (output) DOUBLE PRECISION array, dimension(N)
* On exit Z contains the updating row vector in the secular
* equation.
*
* ALPHA (input) DOUBLE PRECISION
* Contains the diagonal element associated with the added row.
*
* BETA (input) DOUBLE PRECISION
* Contains the off-diagonal element associated with the added
* row.
*
* U (input/output) DOUBLE PRECISION array, dimension(LDU,N)
* On entry U contains the left singular vectors of two
* submatrices in the two square blocks with corners at (1,1),
* (NL, NL), and (NL+2, NL+2), (N,N).
* On exit U contains the trailing (N-K) updated left singular
* vectors (those which were deflated) in its last N-K columns.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= N.
*
* VT (input/output) DOUBLE PRECISION array, dimension(LDVT,M)
* On entry VT' contains the right singular vectors of two
* submatrices in the two square blocks with corners at (1,1),
* (NL+1, NL+1), and (NL+2, NL+2), (M,M).
* On exit VT' contains the trailing (N-K) updated right singular
* vectors (those which were deflated) in its last N-K columns.
* In case SQRE =1, the last row of VT spans the right null
* space.
*
* LDVT (input) INTEGER
* The leading dimension of the array VT. LDVT >= M.
*
* DSIGMA (output) DOUBLE PRECISION array, dimension (N)
* Contains a copy of the diagonal elements (K-1 singular values
* and one zero) in the secular equation.
*
* U2 (output) DOUBLE PRECISION array, dimension(LDU2,N)
* Contains a copy of the first K-1 left singular vectors which
* will be used by DLASD3 in a matrix multiply (DGEMM) to solve
* for the new left singular vectors. U2 is arranged into four
* blocks. The first block contains a column with 1 at NL+1 and
* zero everywhere else; the second block contains non-zero
* entries only at and above NL; the third contains non-zero
* entries only below NL+1; and the fourth is dense.
*
* LDU2 (input) INTEGER
* The leading dimension of the array U2. LDU2 >= N.
*
* VT2 (output) DOUBLE PRECISION array, dimension(LDVT2,N)
* VT2' contains a copy of the first K right singular vectors
* which will be used by DLASD3 in a matrix multiply (DGEMM) to
* solve for the new right singular vectors. VT2 is arranged into
* three blocks. The first block contains a row that corresponds
* to the special 0 diagonal element in SIGMA; the second block
* contains non-zeros only at and before NL +1; the third block
* contains non-zeros only at and after NL +2.
*
* LDVT2 (input) INTEGER
* The leading dimension of the array VT2. LDVT2 >= M.
*
* IDXP (workspace) INTEGER array dimension(N)
* This will contain the permutation used to place deflated
* values of D at the end of the array. On output IDXP(2:K)
* points to the nondeflated D-values and IDXP(K+1:N)
* points to the deflated singular values.
*
* IDX (workspace) INTEGER array dimension(N)
* This will contain the permutation used to sort the contents of
* D into ascending order.
*
* IDXC (output) INTEGER array dimension(N)
* This will contain the permutation used to arrange the columns
* of the deflated U matrix into three groups: the first group
* contains non-zero entries only at and above NL, the second
* contains non-zero entries only below NL+2, and the third is
* dense.
*
* IDXQ (input/output) INTEGER array dimension(N)
* This contains the permutation which separately sorts the two
* sub-problems in D into ascending order. Note that entries in
* the first hlaf of this permutation must first be moved one
* position backward; and entries in the second half
* must first have NL+1 added to their values.
*
* COLTYP (workspace/output) INTEGER array dimension(N)
* As workspace, this will contain a label which will indicate
* which of the following types a column in the U2 matrix or a
* row in the VT2 matrix is:
* 1 : non-zero in the upper half only
* 2 : non-zero in the lower half only
* 3 : dense
* 4 : deflated
*
* On exit, it is an array of dimension 4, with COLTYP(I) being
* the dimension of the I-th type columns.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param nl
* @param nr
* @param sqre
* @param k
* @param d
* @param z
* @param alpha
* @param beta
* @param u
* @param ldu
* @param vt
* @param ldvt
* @param dsigma
* @param u2
* @param ldu2
* @param vt2
* @param ldvt2
* @param idxp
* @param idx
* @param idxc
* @param idxq
* @param coltyp
* @param info
*
*/
abstract public void dlasd2(int nl, int nr, int sqre, org.netlib.util.intW k, double[] d, double[] z, double alpha, double beta, double[] u, int ldu, double[] vt, int ldvt, double[] dsigma, double[] u2, int ldu2, double[] vt2, int ldvt2, int[] idxp, int[] idx, int[] idxc, int[] idxq, int[] coltyp, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLASD2 merges the two sets of singular values together into a single
* sorted set. Then it tries to deflate the size of the problem.
* There are two ways in which deflation can occur: when two or more
* singular values are close together or if there is a tiny entry in the
* Z vector. For each such occurrence the order of the related secular
* equation problem is reduced by one.
*
* DLASD2 is called from DLASD1.
*
* Arguments
* =========
*
* NL (input) INTEGER
* The row dimension of the upper block. NL >= 1.
*
* NR (input) INTEGER
* The row dimension of the lower block. NR >= 1.
*
* SQRE (input) INTEGER
* = 0: the lower block is an NR-by-NR square matrix.
* = 1: the lower block is an NR-by-(NR+1) rectangular matrix.
*
* The bidiagonal matrix has N = NL + NR + 1 rows and
* M = N + SQRE >= N columns.
*
* K (output) INTEGER
* Contains the dimension of the non-deflated matrix,
* This is the order of the related secular equation. 1 <= K <=N.
*
* D (input/output) DOUBLE PRECISION array, dimension(N)
* On entry D contains the singular values of the two submatrices
* to be combined. On exit D contains the trailing (N-K) updated
* singular values (those which were deflated) sorted into
* increasing order.
*
* Z (output) DOUBLE PRECISION array, dimension(N)
* On exit Z contains the updating row vector in the secular
* equation.
*
* ALPHA (input) DOUBLE PRECISION
* Contains the diagonal element associated with the added row.
*
* BETA (input) DOUBLE PRECISION
* Contains the off-diagonal element associated with the added
* row.
*
* U (input/output) DOUBLE PRECISION array, dimension(LDU,N)
* On entry U contains the left singular vectors of two
* submatrices in the two square blocks with corners at (1,1),
* (NL, NL), and (NL+2, NL+2), (N,N).
* On exit U contains the trailing (N-K) updated left singular
* vectors (those which were deflated) in its last N-K columns.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= N.
*
* VT (input/output) DOUBLE PRECISION array, dimension(LDVT,M)
* On entry VT' contains the right singular vectors of two
* submatrices in the two square blocks with corners at (1,1),
* (NL+1, NL+1), and (NL+2, NL+2), (M,M).
* On exit VT' contains the trailing (N-K) updated right singular
* vectors (those which were deflated) in its last N-K columns.
* In case SQRE =1, the last row of VT spans the right null
* space.
*
* LDVT (input) INTEGER
* The leading dimension of the array VT. LDVT >= M.
*
* DSIGMA (output) DOUBLE PRECISION array, dimension (N)
* Contains a copy of the diagonal elements (K-1 singular values
* and one zero) in the secular equation.
*
* U2 (output) DOUBLE PRECISION array, dimension(LDU2,N)
* Contains a copy of the first K-1 left singular vectors which
* will be used by DLASD3 in a matrix multiply (DGEMM) to solve
* for the new left singular vectors. U2 is arranged into four
* blocks. The first block contains a column with 1 at NL+1 and
* zero everywhere else; the second block contains non-zero
* entries only at and above NL; the third contains non-zero
* entries only below NL+1; and the fourth is dense.
*
* LDU2 (input) INTEGER
* The leading dimension of the array U2. LDU2 >= N.
*
* VT2 (output) DOUBLE PRECISION array, dimension(LDVT2,N)
* VT2' contains a copy of the first K right singular vectors
* which will be used by DLASD3 in a matrix multiply (DGEMM) to
* solve for the new right singular vectors. VT2 is arranged into
* three blocks. The first block contains a row that corresponds
* to the special 0 diagonal element in SIGMA; the second block
* contains non-zeros only at and before NL +1; the third block
* contains non-zeros only at and after NL +2.
*
* LDVT2 (input) INTEGER
* The leading dimension of the array VT2. LDVT2 >= M.
*
* IDXP (workspace) INTEGER array dimension(N)
* This will contain the permutation used to place deflated
* values of D at the end of the array. On output IDXP(2:K)
* points to the nondeflated D-values and IDXP(K+1:N)
* points to the deflated singular values.
*
* IDX (workspace) INTEGER array dimension(N)
* This will contain the permutation used to sort the contents of
* D into ascending order.
*
* IDXC (output) INTEGER array dimension(N)
* This will contain the permutation used to arrange the columns
* of the deflated U matrix into three groups: the first group
* contains non-zero entries only at and above NL, the second
* contains non-zero entries only below NL+2, and the third is
* dense.
*
* IDXQ (input/output) INTEGER array dimension(N)
* This contains the permutation which separately sorts the two
* sub-problems in D into ascending order. Note that entries in
* the first hlaf of this permutation must first be moved one
* position backward; and entries in the second half
* must first have NL+1 added to their values.
*
* COLTYP (workspace/output) INTEGER array dimension(N)
* As workspace, this will contain a label which will indicate
* which of the following types a column in the U2 matrix or a
* row in the VT2 matrix is:
* 1 : non-zero in the upper half only
* 2 : non-zero in the lower half only
* 3 : dense
* 4 : deflated
*
* On exit, it is an array of dimension 4, with COLTYP(I) being
* the dimension of the I-th type columns.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param nl
* @param nr
* @param sqre
* @param k
* @param d
* @param _d_offset
* @param z
* @param _z_offset
* @param alpha
* @param beta
* @param u
* @param _u_offset
* @param ldu
* @param vt
* @param _vt_offset
* @param ldvt
* @param dsigma
* @param _dsigma_offset
* @param u2
* @param _u2_offset
* @param ldu2
* @param vt2
* @param _vt2_offset
* @param ldvt2
* @param idxp
* @param _idxp_offset
* @param idx
* @param _idx_offset
* @param idxc
* @param _idxc_offset
* @param idxq
* @param _idxq_offset
* @param coltyp
* @param _coltyp_offset
* @param info
*
*/
abstract public void dlasd2(int nl, int nr, int sqre, org.netlib.util.intW k, double[] d, int _d_offset, double[] z, int _z_offset, double alpha, double beta, double[] u, int _u_offset, int ldu, double[] vt, int _vt_offset, int ldvt, double[] dsigma, int _dsigma_offset, double[] u2, int _u2_offset, int ldu2, double[] vt2, int _vt2_offset, int ldvt2, int[] idxp, int _idxp_offset, int[] idx, int _idx_offset, int[] idxc, int _idxc_offset, int[] idxq, int _idxq_offset, int[] coltyp, int _coltyp_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLASD3 finds all the square roots of the roots of the secular
* equation, as defined by the values in D and Z. It makes the
* appropriate calls to DLASD4 and then updates the singular
* vectors by matrix multiplication.
*
* This code makes very mild assumptions about floating point
* arithmetic. It will work on machines with a guard digit in
* add/subtract, or on those binary machines without guard digits
* which subtract like the Cray XMP, Cray YMP, Cray C 90, or Cray 2.
* It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* DLASD3 is called from DLASD1.
*
* Arguments
* =========
*
* NL (input) INTEGER
* The row dimension of the upper block. NL >= 1.
*
* NR (input) INTEGER
* The row dimension of the lower block. NR >= 1.
*
* SQRE (input) INTEGER
* = 0: the lower block is an NR-by-NR square matrix.
* = 1: the lower block is an NR-by-(NR+1) rectangular matrix.
*
* The bidiagonal matrix has N = NL + NR + 1 rows and
* M = N + SQRE >= N columns.
*
* K (input) INTEGER
* The size of the secular equation, 1 =< K = < N.
*
* D (output) DOUBLE PRECISION array, dimension(K)
* On exit the square roots of the roots of the secular equation,
* in ascending order.
*
* Q (workspace) DOUBLE PRECISION array,
* dimension at least (LDQ,K).
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= K.
*
* DSIGMA (input) DOUBLE PRECISION array, dimension(K)
* The first K elements of this array contain the old roots
* of the deflated updating problem. These are the poles
* of the secular equation.
*
* U (output) DOUBLE PRECISION array, dimension (LDU, N)
* The last N - K columns of this matrix contain the deflated
* left singular vectors.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= N.
*
* U2 (input/output) DOUBLE PRECISION array, dimension (LDU2, N)
* The first K columns of this matrix contain the non-deflated
* left singular vectors for the split problem.
*
* LDU2 (input) INTEGER
* The leading dimension of the array U2. LDU2 >= N.
*
* VT (output) DOUBLE PRECISION array, dimension (LDVT, M)
* The last M - K columns of VT' contain the deflated
* right singular vectors.
*
* LDVT (input) INTEGER
* The leading dimension of the array VT. LDVT >= N.
*
* VT2 (input/output) DOUBLE PRECISION array, dimension (LDVT2, N)
* The first K columns of VT2' contain the non-deflated
* right singular vectors for the split problem.
*
* LDVT2 (input) INTEGER
* The leading dimension of the array VT2. LDVT2 >= N.
*
* IDXC (input) INTEGER array, dimension ( N )
* The permutation used to arrange the columns of U (and rows of
* VT) into three groups: the first group contains non-zero
* entries only at and above (or before) NL +1; the second
* contains non-zero entries only at and below (or after) NL+2;
* and the third is dense. The first column of U and the row of
* VT are treated separately, however.
*
* The rows of the singular vectors found by DLASD4
* must be likewise permuted before the matrix multiplies can
* take place.
*
* CTOT (input) INTEGER array, dimension ( 4 )
* A count of the total number of the various types of columns
* in U (or rows in VT), as described in IDXC. The fourth column
* type is any column which has been deflated.
*
* Z (input) DOUBLE PRECISION array, dimension (K)
* The first K elements of this array contain the components
* of the deflation-adjusted updating row vector.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an singular value did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param nl
* @param nr
* @param sqre
* @param k
* @param d
* @param q
* @param ldq
* @param dsigma
* @param u
* @param ldu
* @param u2
* @param ldu2
* @param vt
* @param ldvt
* @param vt2
* @param ldvt2
* @param idxc
* @param ctot
* @param z
* @param info
*
*/
abstract public void dlasd3(int nl, int nr, int sqre, int k, double[] d, double[] q, int ldq, double[] dsigma, double[] u, int ldu, double[] u2, int ldu2, double[] vt, int ldvt, double[] vt2, int ldvt2, int[] idxc, int[] ctot, double[] z, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLASD3 finds all the square roots of the roots of the secular
* equation, as defined by the values in D and Z. It makes the
* appropriate calls to DLASD4 and then updates the singular
* vectors by matrix multiplication.
*
* This code makes very mild assumptions about floating point
* arithmetic. It will work on machines with a guard digit in
* add/subtract, or on those binary machines without guard digits
* which subtract like the Cray XMP, Cray YMP, Cray C 90, or Cray 2.
* It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* DLASD3 is called from DLASD1.
*
* Arguments
* =========
*
* NL (input) INTEGER
* The row dimension of the upper block. NL >= 1.
*
* NR (input) INTEGER
* The row dimension of the lower block. NR >= 1.
*
* SQRE (input) INTEGER
* = 0: the lower block is an NR-by-NR square matrix.
* = 1: the lower block is an NR-by-(NR+1) rectangular matrix.
*
* The bidiagonal matrix has N = NL + NR + 1 rows and
* M = N + SQRE >= N columns.
*
* K (input) INTEGER
* The size of the secular equation, 1 =< K = < N.
*
* D (output) DOUBLE PRECISION array, dimension(K)
* On exit the square roots of the roots of the secular equation,
* in ascending order.
*
* Q (workspace) DOUBLE PRECISION array,
* dimension at least (LDQ,K).
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= K.
*
* DSIGMA (input) DOUBLE PRECISION array, dimension(K)
* The first K elements of this array contain the old roots
* of the deflated updating problem. These are the poles
* of the secular equation.
*
* U (output) DOUBLE PRECISION array, dimension (LDU, N)
* The last N - K columns of this matrix contain the deflated
* left singular vectors.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= N.
*
* U2 (input/output) DOUBLE PRECISION array, dimension (LDU2, N)
* The first K columns of this matrix contain the non-deflated
* left singular vectors for the split problem.
*
* LDU2 (input) INTEGER
* The leading dimension of the array U2. LDU2 >= N.
*
* VT (output) DOUBLE PRECISION array, dimension (LDVT, M)
* The last M - K columns of VT' contain the deflated
* right singular vectors.
*
* LDVT (input) INTEGER
* The leading dimension of the array VT. LDVT >= N.
*
* VT2 (input/output) DOUBLE PRECISION array, dimension (LDVT2, N)
* The first K columns of VT2' contain the non-deflated
* right singular vectors for the split problem.
*
* LDVT2 (input) INTEGER
* The leading dimension of the array VT2. LDVT2 >= N.
*
* IDXC (input) INTEGER array, dimension ( N )
* The permutation used to arrange the columns of U (and rows of
* VT) into three groups: the first group contains non-zero
* entries only at and above (or before) NL +1; the second
* contains non-zero entries only at and below (or after) NL+2;
* and the third is dense. The first column of U and the row of
* VT are treated separately, however.
*
* The rows of the singular vectors found by DLASD4
* must be likewise permuted before the matrix multiplies can
* take place.
*
* CTOT (input) INTEGER array, dimension ( 4 )
* A count of the total number of the various types of columns
* in U (or rows in VT), as described in IDXC. The fourth column
* type is any column which has been deflated.
*
* Z (input) DOUBLE PRECISION array, dimension (K)
* The first K elements of this array contain the components
* of the deflation-adjusted updating row vector.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an singular value did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param nl
* @param nr
* @param sqre
* @param k
* @param d
* @param _d_offset
* @param q
* @param _q_offset
* @param ldq
* @param dsigma
* @param _dsigma_offset
* @param u
* @param _u_offset
* @param ldu
* @param u2
* @param _u2_offset
* @param ldu2
* @param vt
* @param _vt_offset
* @param ldvt
* @param vt2
* @param _vt2_offset
* @param ldvt2
* @param idxc
* @param _idxc_offset
* @param ctot
* @param _ctot_offset
* @param z
* @param _z_offset
* @param info
*
*/
abstract public void dlasd3(int nl, int nr, int sqre, int k, double[] d, int _d_offset, double[] q, int _q_offset, int ldq, double[] dsigma, int _dsigma_offset, double[] u, int _u_offset, int ldu, double[] u2, int _u2_offset, int ldu2, double[] vt, int _vt_offset, int ldvt, double[] vt2, int _vt2_offset, int ldvt2, int[] idxc, int _idxc_offset, int[] ctot, int _ctot_offset, double[] z, int _z_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* This subroutine computes the square root of the I-th updated
* eigenvalue of a positive symmetric rank-one modification to
* a positive diagonal matrix whose entries are given as the squares
* of the corresponding entries in the array d, and that
*
* 0 <= D(i) < D(j) for i < j
*
* and that RHO > 0. This is arranged by the calling routine, and is
* no loss in generality. The rank-one modified system is thus
*
* diag( D ) * diag( D ) + RHO * Z * Z_transpose.
*
* where we assume the Euclidean norm of Z is 1.
*
* The method consists of approximating the rational functions in the
* secular equation by simpler interpolating rational functions.
*
* Arguments
* =========
*
* N (input) INTEGER
* The length of all arrays.
*
* I (input) INTEGER
* The index of the eigenvalue to be computed. 1 <= I <= N.
*
* D (input) DOUBLE PRECISION array, dimension ( N )
* The original eigenvalues. It is assumed that they are in
* order, 0 <= D(I) < D(J) for I < J.
*
* Z (input) DOUBLE PRECISION array, dimension ( N )
* The components of the updating vector.
*
* DELTA (output) DOUBLE PRECISION array, dimension ( N )
* If N .ne. 1, DELTA contains (D(j) - sigma_I) in its j-th
* component. If N = 1, then DELTA(1) = 1. The vector DELTA
* contains the information necessary to construct the
* (singular) eigenvectors.
*
* RHO (input) DOUBLE PRECISION
* The scalar in the symmetric updating formula.
*
* SIGMA (output) DOUBLE PRECISION
* The computed sigma_I, the I-th updated eigenvalue.
*
* WORK (workspace) DOUBLE PRECISION array, dimension ( N )
* If N .ne. 1, WORK contains (D(j) + sigma_I) in its j-th
* component. If N = 1, then WORK( 1 ) = 1.
*
* INFO (output) INTEGER
* = 0: successful exit
* > 0: if INFO = 1, the updating process failed.
*
* Internal Parameters
* ===================
*
* Logical variable ORGATI (origin-at-i?) is used for distinguishing
* whether D(i) or D(i+1) is treated as the origin.
*
* ORGATI = .true. origin at i
* ORGATI = .false. origin at i+1
*
* Logical variable SWTCH3 (switch-for-3-poles?) is for noting
* if we are working with THREE poles!
*
* MAXIT is the maximum number of iterations allowed for each
* eigenvalue.
*
* Further Details
* ===============
*
* Based on contributions by
* Ren-Cang Li, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param i
* @param d
* @param z
* @param delta
* @param rho
* @param sigma
* @param work
* @param info
*
*/
abstract public void dlasd4(int n, int i, double[] d, double[] z, double[] delta, double rho, org.netlib.util.doubleW sigma, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* This subroutine computes the square root of the I-th updated
* eigenvalue of a positive symmetric rank-one modification to
* a positive diagonal matrix whose entries are given as the squares
* of the corresponding entries in the array d, and that
*
* 0 <= D(i) < D(j) for i < j
*
* and that RHO > 0. This is arranged by the calling routine, and is
* no loss in generality. The rank-one modified system is thus
*
* diag( D ) * diag( D ) + RHO * Z * Z_transpose.
*
* where we assume the Euclidean norm of Z is 1.
*
* The method consists of approximating the rational functions in the
* secular equation by simpler interpolating rational functions.
*
* Arguments
* =========
*
* N (input) INTEGER
* The length of all arrays.
*
* I (input) INTEGER
* The index of the eigenvalue to be computed. 1 <= I <= N.
*
* D (input) DOUBLE PRECISION array, dimension ( N )
* The original eigenvalues. It is assumed that they are in
* order, 0 <= D(I) < D(J) for I < J.
*
* Z (input) DOUBLE PRECISION array, dimension ( N )
* The components of the updating vector.
*
* DELTA (output) DOUBLE PRECISION array, dimension ( N )
* If N .ne. 1, DELTA contains (D(j) - sigma_I) in its j-th
* component. If N = 1, then DELTA(1) = 1. The vector DELTA
* contains the information necessary to construct the
* (singular) eigenvectors.
*
* RHO (input) DOUBLE PRECISION
* The scalar in the symmetric updating formula.
*
* SIGMA (output) DOUBLE PRECISION
* The computed sigma_I, the I-th updated eigenvalue.
*
* WORK (workspace) DOUBLE PRECISION array, dimension ( N )
* If N .ne. 1, WORK contains (D(j) + sigma_I) in its j-th
* component. If N = 1, then WORK( 1 ) = 1.
*
* INFO (output) INTEGER
* = 0: successful exit
* > 0: if INFO = 1, the updating process failed.
*
* Internal Parameters
* ===================
*
* Logical variable ORGATI (origin-at-i?) is used for distinguishing
* whether D(i) or D(i+1) is treated as the origin.
*
* ORGATI = .true. origin at i
* ORGATI = .false. origin at i+1
*
* Logical variable SWTCH3 (switch-for-3-poles?) is for noting
* if we are working with THREE poles!
*
* MAXIT is the maximum number of iterations allowed for each
* eigenvalue.
*
* Further Details
* ===============
*
* Based on contributions by
* Ren-Cang Li, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param i
* @param d
* @param _d_offset
* @param z
* @param _z_offset
* @param delta
* @param _delta_offset
* @param rho
* @param sigma
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dlasd4(int n, int i, double[] d, int _d_offset, double[] z, int _z_offset, double[] delta, int _delta_offset, double rho, org.netlib.util.doubleW sigma, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* This subroutine computes the square root of the I-th eigenvalue
* of a positive symmetric rank-one modification of a 2-by-2 diagonal
* matrix
*
* diag( D ) * diag( D ) + RHO * Z * transpose(Z) .
*
* The diagonal entries in the array D are assumed to satisfy
*
* 0 <= D(i) < D(j) for i < j .
*
* We also assume RHO > 0 and that the Euclidean norm of the vector
* Z is one.
*
* Arguments
* =========
*
* I (input) INTEGER
* The index of the eigenvalue to be computed. I = 1 or I = 2.
*
* D (input) DOUBLE PRECISION array, dimension ( 2 )
* The original eigenvalues. We assume 0 <= D(1) < D(2).
*
* Z (input) DOUBLE PRECISION array, dimension ( 2 )
* The components of the updating vector.
*
* DELTA (output) DOUBLE PRECISION array, dimension ( 2 )
* Contains (D(j) - sigma_I) in its j-th component.
* The vector DELTA contains the information necessary
* to construct the eigenvectors.
*
* RHO (input) DOUBLE PRECISION
* The scalar in the symmetric updating formula.
*
* DSIGMA (output) DOUBLE PRECISION
* The computed sigma_I, the I-th updated eigenvalue.
*
* WORK (workspace) DOUBLE PRECISION array, dimension ( 2 )
* WORK contains (D(j) + sigma_I) in its j-th component.
*
* Further Details
* ===============
*
* Based on contributions by
* Ren-Cang Li, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param i
* @param d
* @param z
* @param delta
* @param rho
* @param dsigma
* @param work
*
*/
abstract public void dlasd5(int i, double[] d, double[] z, double[] delta, double rho, org.netlib.util.doubleW dsigma, double[] work);
/**
*
* ..
*
* Purpose
* =======
*
* This subroutine computes the square root of the I-th eigenvalue
* of a positive symmetric rank-one modification of a 2-by-2 diagonal
* matrix
*
* diag( D ) * diag( D ) + RHO * Z * transpose(Z) .
*
* The diagonal entries in the array D are assumed to satisfy
*
* 0 <= D(i) < D(j) for i < j .
*
* We also assume RHO > 0 and that the Euclidean norm of the vector
* Z is one.
*
* Arguments
* =========
*
* I (input) INTEGER
* The index of the eigenvalue to be computed. I = 1 or I = 2.
*
* D (input) DOUBLE PRECISION array, dimension ( 2 )
* The original eigenvalues. We assume 0 <= D(1) < D(2).
*
* Z (input) DOUBLE PRECISION array, dimension ( 2 )
* The components of the updating vector.
*
* DELTA (output) DOUBLE PRECISION array, dimension ( 2 )
* Contains (D(j) - sigma_I) in its j-th component.
* The vector DELTA contains the information necessary
* to construct the eigenvectors.
*
* RHO (input) DOUBLE PRECISION
* The scalar in the symmetric updating formula.
*
* DSIGMA (output) DOUBLE PRECISION
* The computed sigma_I, the I-th updated eigenvalue.
*
* WORK (workspace) DOUBLE PRECISION array, dimension ( 2 )
* WORK contains (D(j) + sigma_I) in its j-th component.
*
* Further Details
* ===============
*
* Based on contributions by
* Ren-Cang Li, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param i
* @param d
* @param _d_offset
* @param z
* @param _z_offset
* @param delta
* @param _delta_offset
* @param rho
* @param dsigma
* @param work
* @param _work_offset
*
*/
abstract public void dlasd5(int i, double[] d, int _d_offset, double[] z, int _z_offset, double[] delta, int _delta_offset, double rho, org.netlib.util.doubleW dsigma, double[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* DLASD6 computes the SVD of an updated upper bidiagonal matrix B
* obtained by merging two smaller ones by appending a row. This
* routine is used only for the problem which requires all singular
* values and optionally singular vector matrices in factored form.
* B is an N-by-M matrix with N = NL + NR + 1 and M = N + SQRE.
* A related subroutine, DLASD1, handles the case in which all singular
* values and singular vectors of the bidiagonal matrix are desired.
*
* DLASD6 computes the SVD as follows:
*
* ( D1(in) 0 0 0 )
* B = U(in) * ( Z1' a Z2' b ) * VT(in)
* ( 0 0 D2(in) 0 )
*
* = U(out) * ( D(out) 0) * VT(out)
*
* where Z' = (Z1' a Z2' b) = u' VT', and u is a vector of dimension M
* with ALPHA and BETA in the NL+1 and NL+2 th entries and zeros
* elsewhere; and the entry b is empty if SQRE = 0.
*
* The singular values of B can be computed using D1, D2, the first
* components of all the right singular vectors of the lower block, and
* the last components of all the right singular vectors of the upper
* block. These components are stored and updated in VF and VL,
* respectively, in DLASD6. Hence U and VT are not explicitly
* referenced.
*
* The singular values are stored in D. The algorithm consists of two
* stages:
*
* The first stage consists of deflating the size of the problem
* when there are multiple singular values or if there is a zero
* in the Z vector. For each such occurence the dimension of the
* secular equation problem is reduced by one. This stage is
* performed by the routine DLASD7.
*
* The second stage consists of calculating the updated
* singular values. This is done by finding the roots of the
* secular equation via the routine DLASD4 (as called by DLASD8).
* This routine also updates VF and VL and computes the distances
* between the updated singular values and the old singular
* values.
*
* DLASD6 is called from DLASDA.
*
* Arguments
* =========
*
* ICOMPQ (input) INTEGER
* Specifies whether singular vectors are to be computed in
* factored form:
* = 0: Compute singular values only.
* = 1: Compute singular vectors in factored form as well.
*
* NL (input) INTEGER
* The row dimension of the upper block. NL >= 1.
*
* NR (input) INTEGER
* The row dimension of the lower block. NR >= 1.
*
* SQRE (input) INTEGER
* = 0: the lower block is an NR-by-NR square matrix.
* = 1: the lower block is an NR-by-(NR+1) rectangular matrix.
*
* The bidiagonal matrix has row dimension N = NL + NR + 1,
* and column dimension M = N + SQRE.
*
* D (input/output) DOUBLE PRECISION array, dimension ( NL+NR+1 ).
* On entry D(1:NL,1:NL) contains the singular values of the
* upper block, and D(NL+2:N) contains the singular values
* of the lower block. On exit D(1:N) contains the singular
* values of the modified matrix.
*
* VF (input/output) DOUBLE PRECISION array, dimension ( M )
* On entry, VF(1:NL+1) contains the first components of all
* right singular vectors of the upper block; and VF(NL+2:M)
* contains the first components of all right singular vectors
* of the lower block. On exit, VF contains the first components
* of all right singular vectors of the bidiagonal matrix.
*
* VL (input/output) DOUBLE PRECISION array, dimension ( M )
* On entry, VL(1:NL+1) contains the last components of all
* right singular vectors of the upper block; and VL(NL+2:M)
* contains the last components of all right singular vectors of
* the lower block. On exit, VL contains the last components of
* all right singular vectors of the bidiagonal matrix.
*
* ALPHA (input/output) DOUBLE PRECISION
* Contains the diagonal element associated with the added row.
*
* BETA (input/output) DOUBLE PRECISION
* Contains the off-diagonal element associated with the added
* row.
*
* IDXQ (output) INTEGER array, dimension ( N )
* This contains the permutation which will reintegrate the
* subproblem just solved back into sorted order, i.e.
* D( IDXQ( I = 1, N ) ) will be in ascending order.
*
* PERM (output) INTEGER array, dimension ( N )
* The permutations (from deflation and sorting) to be applied
* to each block. Not referenced if ICOMPQ = 0.
*
* GIVPTR (output) INTEGER
* The number of Givens rotations which took place in this
* subproblem. Not referenced if ICOMPQ = 0.
*
* GIVCOL (output) INTEGER array, dimension ( LDGCOL, 2 )
* Each pair of numbers indicates a pair of columns to take place
* in a Givens rotation. Not referenced if ICOMPQ = 0.
*
* LDGCOL (input) INTEGER
* leading dimension of GIVCOL, must be at least N.
*
* GIVNUM (output) DOUBLE PRECISION array, dimension ( LDGNUM, 2 )
* Each number indicates the C or S value to be used in the
* corresponding Givens rotation. Not referenced if ICOMPQ = 0.
*
* LDGNUM (input) INTEGER
* The leading dimension of GIVNUM and POLES, must be at least N.
*
* POLES (output) DOUBLE PRECISION array, dimension ( LDGNUM, 2 )
* On exit, POLES(1,*) is an array containing the new singular
* values obtained from solving the secular equation, and
* POLES(2,*) is an array containing the poles in the secular
* equation. Not referenced if ICOMPQ = 0.
*
* DIFL (output) DOUBLE PRECISION array, dimension ( N )
* On exit, DIFL(I) is the distance between I-th updated
* (undeflated) singular value and the I-th (undeflated) old
* singular value.
*
* DIFR (output) DOUBLE PRECISION array,
* dimension ( LDGNUM, 2 ) if ICOMPQ = 1 and
* dimension ( N ) if ICOMPQ = 0.
* On exit, DIFR(I, 1) is the distance between I-th updated
* (undeflated) singular value and the I+1-th (undeflated) old
* singular value.
*
* If ICOMPQ = 1, DIFR(1:K,2) is an array containing the
* normalizing factors for the right singular vector matrix.
*
* See DLASD8 for details on DIFL and DIFR.
*
* Z (output) DOUBLE PRECISION array, dimension ( M )
* The first elements of this array contain the components
* of the deflation-adjusted updating row vector.
*
* K (output) INTEGER
* Contains the dimension of the non-deflated matrix,
* This is the order of the related secular equation. 1 <= K <=N.
*
* C (output) DOUBLE PRECISION
* C contains garbage if SQRE =0 and the C-value of a Givens
* rotation related to the right null space if SQRE = 1.
*
* S (output) DOUBLE PRECISION
* S contains garbage if SQRE =0 and the S-value of a Givens
* rotation related to the right null space if SQRE = 1.
*
* WORK (workspace) DOUBLE PRECISION array, dimension ( 4 * M )
*
* IWORK (workspace) INTEGER array, dimension ( 3 * N )
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an singular value did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param nl
* @param nr
* @param sqre
* @param d
* @param vf
* @param vl
* @param alpha
* @param beta
* @param idxq
* @param perm
* @param givptr
* @param givcol
* @param ldgcol
* @param givnum
* @param ldgnum
* @param poles
* @param difl
* @param difr
* @param z
* @param k
* @param c
* @param s
* @param work
* @param iwork
* @param info
*
*/
abstract public void dlasd6(int icompq, int nl, int nr, int sqre, double[] d, double[] vf, double[] vl, org.netlib.util.doubleW alpha, org.netlib.util.doubleW beta, int[] idxq, int[] perm, org.netlib.util.intW givptr, int[] givcol, int ldgcol, double[] givnum, int ldgnum, double[] poles, double[] difl, double[] difr, double[] z, org.netlib.util.intW k, org.netlib.util.doubleW c, org.netlib.util.doubleW s, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLASD6 computes the SVD of an updated upper bidiagonal matrix B
* obtained by merging two smaller ones by appending a row. This
* routine is used only for the problem which requires all singular
* values and optionally singular vector matrices in factored form.
* B is an N-by-M matrix with N = NL + NR + 1 and M = N + SQRE.
* A related subroutine, DLASD1, handles the case in which all singular
* values and singular vectors of the bidiagonal matrix are desired.
*
* DLASD6 computes the SVD as follows:
*
* ( D1(in) 0 0 0 )
* B = U(in) * ( Z1' a Z2' b ) * VT(in)
* ( 0 0 D2(in) 0 )
*
* = U(out) * ( D(out) 0) * VT(out)
*
* where Z' = (Z1' a Z2' b) = u' VT', and u is a vector of dimension M
* with ALPHA and BETA in the NL+1 and NL+2 th entries and zeros
* elsewhere; and the entry b is empty if SQRE = 0.
*
* The singular values of B can be computed using D1, D2, the first
* components of all the right singular vectors of the lower block, and
* the last components of all the right singular vectors of the upper
* block. These components are stored and updated in VF and VL,
* respectively, in DLASD6. Hence U and VT are not explicitly
* referenced.
*
* The singular values are stored in D. The algorithm consists of two
* stages:
*
* The first stage consists of deflating the size of the problem
* when there are multiple singular values or if there is a zero
* in the Z vector. For each such occurence the dimension of the
* secular equation problem is reduced by one. This stage is
* performed by the routine DLASD7.
*
* The second stage consists of calculating the updated
* singular values. This is done by finding the roots of the
* secular equation via the routine DLASD4 (as called by DLASD8).
* This routine also updates VF and VL and computes the distances
* between the updated singular values and the old singular
* values.
*
* DLASD6 is called from DLASDA.
*
* Arguments
* =========
*
* ICOMPQ (input) INTEGER
* Specifies whether singular vectors are to be computed in
* factored form:
* = 0: Compute singular values only.
* = 1: Compute singular vectors in factored form as well.
*
* NL (input) INTEGER
* The row dimension of the upper block. NL >= 1.
*
* NR (input) INTEGER
* The row dimension of the lower block. NR >= 1.
*
* SQRE (input) INTEGER
* = 0: the lower block is an NR-by-NR square matrix.
* = 1: the lower block is an NR-by-(NR+1) rectangular matrix.
*
* The bidiagonal matrix has row dimension N = NL + NR + 1,
* and column dimension M = N + SQRE.
*
* D (input/output) DOUBLE PRECISION array, dimension ( NL+NR+1 ).
* On entry D(1:NL,1:NL) contains the singular values of the
* upper block, and D(NL+2:N) contains the singular values
* of the lower block. On exit D(1:N) contains the singular
* values of the modified matrix.
*
* VF (input/output) DOUBLE PRECISION array, dimension ( M )
* On entry, VF(1:NL+1) contains the first components of all
* right singular vectors of the upper block; and VF(NL+2:M)
* contains the first components of all right singular vectors
* of the lower block. On exit, VF contains the first components
* of all right singular vectors of the bidiagonal matrix.
*
* VL (input/output) DOUBLE PRECISION array, dimension ( M )
* On entry, VL(1:NL+1) contains the last components of all
* right singular vectors of the upper block; and VL(NL+2:M)
* contains the last components of all right singular vectors of
* the lower block. On exit, VL contains the last components of
* all right singular vectors of the bidiagonal matrix.
*
* ALPHA (input/output) DOUBLE PRECISION
* Contains the diagonal element associated with the added row.
*
* BETA (input/output) DOUBLE PRECISION
* Contains the off-diagonal element associated with the added
* row.
*
* IDXQ (output) INTEGER array, dimension ( N )
* This contains the permutation which will reintegrate the
* subproblem just solved back into sorted order, i.e.
* D( IDXQ( I = 1, N ) ) will be in ascending order.
*
* PERM (output) INTEGER array, dimension ( N )
* The permutations (from deflation and sorting) to be applied
* to each block. Not referenced if ICOMPQ = 0.
*
* GIVPTR (output) INTEGER
* The number of Givens rotations which took place in this
* subproblem. Not referenced if ICOMPQ = 0.
*
* GIVCOL (output) INTEGER array, dimension ( LDGCOL, 2 )
* Each pair of numbers indicates a pair of columns to take place
* in a Givens rotation. Not referenced if ICOMPQ = 0.
*
* LDGCOL (input) INTEGER
* leading dimension of GIVCOL, must be at least N.
*
* GIVNUM (output) DOUBLE PRECISION array, dimension ( LDGNUM, 2 )
* Each number indicates the C or S value to be used in the
* corresponding Givens rotation. Not referenced if ICOMPQ = 0.
*
* LDGNUM (input) INTEGER
* The leading dimension of GIVNUM and POLES, must be at least N.
*
* POLES (output) DOUBLE PRECISION array, dimension ( LDGNUM, 2 )
* On exit, POLES(1,*) is an array containing the new singular
* values obtained from solving the secular equation, and
* POLES(2,*) is an array containing the poles in the secular
* equation. Not referenced if ICOMPQ = 0.
*
* DIFL (output) DOUBLE PRECISION array, dimension ( N )
* On exit, DIFL(I) is the distance between I-th updated
* (undeflated) singular value and the I-th (undeflated) old
* singular value.
*
* DIFR (output) DOUBLE PRECISION array,
* dimension ( LDGNUM, 2 ) if ICOMPQ = 1 and
* dimension ( N ) if ICOMPQ = 0.
* On exit, DIFR(I, 1) is the distance between I-th updated
* (undeflated) singular value and the I+1-th (undeflated) old
* singular value.
*
* If ICOMPQ = 1, DIFR(1:K,2) is an array containing the
* normalizing factors for the right singular vector matrix.
*
* See DLASD8 for details on DIFL and DIFR.
*
* Z (output) DOUBLE PRECISION array, dimension ( M )
* The first elements of this array contain the components
* of the deflation-adjusted updating row vector.
*
* K (output) INTEGER
* Contains the dimension of the non-deflated matrix,
* This is the order of the related secular equation. 1 <= K <=N.
*
* C (output) DOUBLE PRECISION
* C contains garbage if SQRE =0 and the C-value of a Givens
* rotation related to the right null space if SQRE = 1.
*
* S (output) DOUBLE PRECISION
* S contains garbage if SQRE =0 and the S-value of a Givens
* rotation related to the right null space if SQRE = 1.
*
* WORK (workspace) DOUBLE PRECISION array, dimension ( 4 * M )
*
* IWORK (workspace) INTEGER array, dimension ( 3 * N )
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an singular value did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param nl
* @param nr
* @param sqre
* @param d
* @param _d_offset
* @param vf
* @param _vf_offset
* @param vl
* @param _vl_offset
* @param alpha
* @param beta
* @param idxq
* @param _idxq_offset
* @param perm
* @param _perm_offset
* @param givptr
* @param givcol
* @param _givcol_offset
* @param ldgcol
* @param givnum
* @param _givnum_offset
* @param ldgnum
* @param poles
* @param _poles_offset
* @param difl
* @param _difl_offset
* @param difr
* @param _difr_offset
* @param z
* @param _z_offset
* @param k
* @param c
* @param s
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dlasd6(int icompq, int nl, int nr, int sqre, double[] d, int _d_offset, double[] vf, int _vf_offset, double[] vl, int _vl_offset, org.netlib.util.doubleW alpha, org.netlib.util.doubleW beta, int[] idxq, int _idxq_offset, int[] perm, int _perm_offset, org.netlib.util.intW givptr, int[] givcol, int _givcol_offset, int ldgcol, double[] givnum, int _givnum_offset, int ldgnum, double[] poles, int _poles_offset, double[] difl, int _difl_offset, double[] difr, int _difr_offset, double[] z, int _z_offset, org.netlib.util.intW k, org.netlib.util.doubleW c, org.netlib.util.doubleW s, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLASD7 merges the two sets of singular values together into a single
* sorted set. Then it tries to deflate the size of the problem. There
* are two ways in which deflation can occur: when two or more singular
* values are close together or if there is a tiny entry in the Z
* vector. For each such occurrence the order of the related
* secular equation problem is reduced by one.
*
* DLASD7 is called from DLASD6.
*
* Arguments
* =========
*
* ICOMPQ (input) INTEGER
* Specifies whether singular vectors are to be computed
* in compact form, as follows:
* = 0: Compute singular values only.
* = 1: Compute singular vectors of upper
* bidiagonal matrix in compact form.
*
* NL (input) INTEGER
* The row dimension of the upper block. NL >= 1.
*
* NR (input) INTEGER
* The row dimension of the lower block. NR >= 1.
*
* SQRE (input) INTEGER
* = 0: the lower block is an NR-by-NR square matrix.
* = 1: the lower block is an NR-by-(NR+1) rectangular matrix.
*
* The bidiagonal matrix has
* N = NL + NR + 1 rows and
* M = N + SQRE >= N columns.
*
* K (output) INTEGER
* Contains the dimension of the non-deflated matrix, this is
* the order of the related secular equation. 1 <= K <=N.
*
* D (input/output) DOUBLE PRECISION array, dimension ( N )
* On entry D contains the singular values of the two submatrices
* to be combined. On exit D contains the trailing (N-K) updated
* singular values (those which were deflated) sorted into
* increasing order.
*
* Z (output) DOUBLE PRECISION array, dimension ( M )
* On exit Z contains the updating row vector in the secular
* equation.
*
* ZW (workspace) DOUBLE PRECISION array, dimension ( M )
* Workspace for Z.
*
* VF (input/output) DOUBLE PRECISION array, dimension ( M )
* On entry, VF(1:NL+1) contains the first components of all
* right singular vectors of the upper block; and VF(NL+2:M)
* contains the first components of all right singular vectors
* of the lower block. On exit, VF contains the first components
* of all right singular vectors of the bidiagonal matrix.
*
* VFW (workspace) DOUBLE PRECISION array, dimension ( M )
* Workspace for VF.
*
* VL (input/output) DOUBLE PRECISION array, dimension ( M )
* On entry, VL(1:NL+1) contains the last components of all
* right singular vectors of the upper block; and VL(NL+2:M)
* contains the last components of all right singular vectors
* of the lower block. On exit, VL contains the last components
* of all right singular vectors of the bidiagonal matrix.
*
* VLW (workspace) DOUBLE PRECISION array, dimension ( M )
* Workspace for VL.
*
* ALPHA (input) DOUBLE PRECISION
* Contains the diagonal element associated with the added row.
*
* BETA (input) DOUBLE PRECISION
* Contains the off-diagonal element associated with the added
* row.
*
* DSIGMA (output) DOUBLE PRECISION array, dimension ( N )
* Contains a copy of the diagonal elements (K-1 singular values
* and one zero) in the secular equation.
*
* IDX (workspace) INTEGER array, dimension ( N )
* This will contain the permutation used to sort the contents of
* D into ascending order.
*
* IDXP (workspace) INTEGER array, dimension ( N )
* This will contain the permutation used to place deflated
* values of D at the end of the array. On output IDXP(2:K)
* points to the nondeflated D-values and IDXP(K+1:N)
* points to the deflated singular values.
*
* IDXQ (input) INTEGER array, dimension ( N )
* This contains the permutation which separately sorts the two
* sub-problems in D into ascending order. Note that entries in
* the first half of this permutation must first be moved one
* position backward; and entries in the second half
* must first have NL+1 added to their values.
*
* PERM (output) INTEGER array, dimension ( N )
* The permutations (from deflation and sorting) to be applied
* to each singular block. Not referenced if ICOMPQ = 0.
*
* GIVPTR (output) INTEGER
* The number of Givens rotations which took place in this
* subproblem. Not referenced if ICOMPQ = 0.
*
* GIVCOL (output) INTEGER array, dimension ( LDGCOL, 2 )
* Each pair of numbers indicates a pair of columns to take place
* in a Givens rotation. Not referenced if ICOMPQ = 0.
*
* LDGCOL (input) INTEGER
* The leading dimension of GIVCOL, must be at least N.
*
* GIVNUM (output) DOUBLE PRECISION array, dimension ( LDGNUM, 2 )
* Each number indicates the C or S value to be used in the
* corresponding Givens rotation. Not referenced if ICOMPQ = 0.
*
* LDGNUM (input) INTEGER
* The leading dimension of GIVNUM, must be at least N.
*
* C (output) DOUBLE PRECISION
* C contains garbage if SQRE =0 and the C-value of a Givens
* rotation related to the right null space if SQRE = 1.
*
* S (output) DOUBLE PRECISION
* S contains garbage if SQRE =0 and the S-value of a Givens
* rotation related to the right null space if SQRE = 1.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param nl
* @param nr
* @param sqre
* @param k
* @param d
* @param z
* @param zw
* @param vf
* @param vfw
* @param vl
* @param vlw
* @param alpha
* @param beta
* @param dsigma
* @param idx
* @param idxp
* @param idxq
* @param perm
* @param givptr
* @param givcol
* @param ldgcol
* @param givnum
* @param ldgnum
* @param c
* @param s
* @param info
*
*/
abstract public void dlasd7(int icompq, int nl, int nr, int sqre, org.netlib.util.intW k, double[] d, double[] z, double[] zw, double[] vf, double[] vfw, double[] vl, double[] vlw, double alpha, double beta, double[] dsigma, int[] idx, int[] idxp, int[] idxq, int[] perm, org.netlib.util.intW givptr, int[] givcol, int ldgcol, double[] givnum, int ldgnum, org.netlib.util.doubleW c, org.netlib.util.doubleW s, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLASD7 merges the two sets of singular values together into a single
* sorted set. Then it tries to deflate the size of the problem. There
* are two ways in which deflation can occur: when two or more singular
* values are close together or if there is a tiny entry in the Z
* vector. For each such occurrence the order of the related
* secular equation problem is reduced by one.
*
* DLASD7 is called from DLASD6.
*
* Arguments
* =========
*
* ICOMPQ (input) INTEGER
* Specifies whether singular vectors are to be computed
* in compact form, as follows:
* = 0: Compute singular values only.
* = 1: Compute singular vectors of upper
* bidiagonal matrix in compact form.
*
* NL (input) INTEGER
* The row dimension of the upper block. NL >= 1.
*
* NR (input) INTEGER
* The row dimension of the lower block. NR >= 1.
*
* SQRE (input) INTEGER
* = 0: the lower block is an NR-by-NR square matrix.
* = 1: the lower block is an NR-by-(NR+1) rectangular matrix.
*
* The bidiagonal matrix has
* N = NL + NR + 1 rows and
* M = N + SQRE >= N columns.
*
* K (output) INTEGER
* Contains the dimension of the non-deflated matrix, this is
* the order of the related secular equation. 1 <= K <=N.
*
* D (input/output) DOUBLE PRECISION array, dimension ( N )
* On entry D contains the singular values of the two submatrices
* to be combined. On exit D contains the trailing (N-K) updated
* singular values (those which were deflated) sorted into
* increasing order.
*
* Z (output) DOUBLE PRECISION array, dimension ( M )
* On exit Z contains the updating row vector in the secular
* equation.
*
* ZW (workspace) DOUBLE PRECISION array, dimension ( M )
* Workspace for Z.
*
* VF (input/output) DOUBLE PRECISION array, dimension ( M )
* On entry, VF(1:NL+1) contains the first components of all
* right singular vectors of the upper block; and VF(NL+2:M)
* contains the first components of all right singular vectors
* of the lower block. On exit, VF contains the first components
* of all right singular vectors of the bidiagonal matrix.
*
* VFW (workspace) DOUBLE PRECISION array, dimension ( M )
* Workspace for VF.
*
* VL (input/output) DOUBLE PRECISION array, dimension ( M )
* On entry, VL(1:NL+1) contains the last components of all
* right singular vectors of the upper block; and VL(NL+2:M)
* contains the last components of all right singular vectors
* of the lower block. On exit, VL contains the last components
* of all right singular vectors of the bidiagonal matrix.
*
* VLW (workspace) DOUBLE PRECISION array, dimension ( M )
* Workspace for VL.
*
* ALPHA (input) DOUBLE PRECISION
* Contains the diagonal element associated with the added row.
*
* BETA (input) DOUBLE PRECISION
* Contains the off-diagonal element associated with the added
* row.
*
* DSIGMA (output) DOUBLE PRECISION array, dimension ( N )
* Contains a copy of the diagonal elements (K-1 singular values
* and one zero) in the secular equation.
*
* IDX (workspace) INTEGER array, dimension ( N )
* This will contain the permutation used to sort the contents of
* D into ascending order.
*
* IDXP (workspace) INTEGER array, dimension ( N )
* This will contain the permutation used to place deflated
* values of D at the end of the array. On output IDXP(2:K)
* points to the nondeflated D-values and IDXP(K+1:N)
* points to the deflated singular values.
*
* IDXQ (input) INTEGER array, dimension ( N )
* This contains the permutation which separately sorts the two
* sub-problems in D into ascending order. Note that entries in
* the first half of this permutation must first be moved one
* position backward; and entries in the second half
* must first have NL+1 added to their values.
*
* PERM (output) INTEGER array, dimension ( N )
* The permutations (from deflation and sorting) to be applied
* to each singular block. Not referenced if ICOMPQ = 0.
*
* GIVPTR (output) INTEGER
* The number of Givens rotations which took place in this
* subproblem. Not referenced if ICOMPQ = 0.
*
* GIVCOL (output) INTEGER array, dimension ( LDGCOL, 2 )
* Each pair of numbers indicates a pair of columns to take place
* in a Givens rotation. Not referenced if ICOMPQ = 0.
*
* LDGCOL (input) INTEGER
* The leading dimension of GIVCOL, must be at least N.
*
* GIVNUM (output) DOUBLE PRECISION array, dimension ( LDGNUM, 2 )
* Each number indicates the C or S value to be used in the
* corresponding Givens rotation. Not referenced if ICOMPQ = 0.
*
* LDGNUM (input) INTEGER
* The leading dimension of GIVNUM, must be at least N.
*
* C (output) DOUBLE PRECISION
* C contains garbage if SQRE =0 and the C-value of a Givens
* rotation related to the right null space if SQRE = 1.
*
* S (output) DOUBLE PRECISION
* S contains garbage if SQRE =0 and the S-value of a Givens
* rotation related to the right null space if SQRE = 1.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param nl
* @param nr
* @param sqre
* @param k
* @param d
* @param _d_offset
* @param z
* @param _z_offset
* @param zw
* @param _zw_offset
* @param vf
* @param _vf_offset
* @param vfw
* @param _vfw_offset
* @param vl
* @param _vl_offset
* @param vlw
* @param _vlw_offset
* @param alpha
* @param beta
* @param dsigma
* @param _dsigma_offset
* @param idx
* @param _idx_offset
* @param idxp
* @param _idxp_offset
* @param idxq
* @param _idxq_offset
* @param perm
* @param _perm_offset
* @param givptr
* @param givcol
* @param _givcol_offset
* @param ldgcol
* @param givnum
* @param _givnum_offset
* @param ldgnum
* @param c
* @param s
* @param info
*
*/
abstract public void dlasd7(int icompq, int nl, int nr, int sqre, org.netlib.util.intW k, double[] d, int _d_offset, double[] z, int _z_offset, double[] zw, int _zw_offset, double[] vf, int _vf_offset, double[] vfw, int _vfw_offset, double[] vl, int _vl_offset, double[] vlw, int _vlw_offset, double alpha, double beta, double[] dsigma, int _dsigma_offset, int[] idx, int _idx_offset, int[] idxp, int _idxp_offset, int[] idxq, int _idxq_offset, int[] perm, int _perm_offset, org.netlib.util.intW givptr, int[] givcol, int _givcol_offset, int ldgcol, double[] givnum, int _givnum_offset, int ldgnum, org.netlib.util.doubleW c, org.netlib.util.doubleW s, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLASD8 finds the square roots of the roots of the secular equation,
* as defined by the values in DSIGMA and Z. It makes the appropriate
* calls to DLASD4, and stores, for each element in D, the distance
* to its two nearest poles (elements in DSIGMA). It also updates
* the arrays VF and VL, the first and last components of all the
* right singular vectors of the original bidiagonal matrix.
*
* DLASD8 is called from DLASD6.
*
* Arguments
* =========
*
* ICOMPQ (input) INTEGER
* Specifies whether singular vectors are to be computed in
* factored form in the calling routine:
* = 0: Compute singular values only.
* = 1: Compute singular vectors in factored form as well.
*
* K (input) INTEGER
* The number of terms in the rational function to be solved
* by DLASD4. K >= 1.
*
* D (output) DOUBLE PRECISION array, dimension ( K )
* On output, D contains the updated singular values.
*
* Z (input) DOUBLE PRECISION array, dimension ( K )
* The first K elements of this array contain the components
* of the deflation-adjusted updating row vector.
*
* VF (input/output) DOUBLE PRECISION array, dimension ( K )
* On entry, VF contains information passed through DBEDE8.
* On exit, VF contains the first K components of the first
* components of all right singular vectors of the bidiagonal
* matrix.
*
* VL (input/output) DOUBLE PRECISION array, dimension ( K )
* On entry, VL contains information passed through DBEDE8.
* On exit, VL contains the first K components of the last
* components of all right singular vectors of the bidiagonal
* matrix.
*
* DIFL (output) DOUBLE PRECISION array, dimension ( K )
* On exit, DIFL(I) = D(I) - DSIGMA(I).
*
* DIFR (output) DOUBLE PRECISION array,
* dimension ( LDDIFR, 2 ) if ICOMPQ = 1 and
* dimension ( K ) if ICOMPQ = 0.
* On exit, DIFR(I,1) = D(I) - DSIGMA(I+1), DIFR(K,1) is not
* defined and will not be referenced.
*
* If ICOMPQ = 1, DIFR(1:K,2) is an array containing the
* normalizing factors for the right singular vector matrix.
*
* LDDIFR (input) INTEGER
* The leading dimension of DIFR, must be at least K.
*
* DSIGMA (input) DOUBLE PRECISION array, dimension ( K )
* The first K elements of this array contain the old roots
* of the deflated updating problem. These are the poles
* of the secular equation.
*
* WORK (workspace) DOUBLE PRECISION array, dimension at least 3 * K
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an singular value did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param k
* @param d
* @param z
* @param vf
* @param vl
* @param difl
* @param difr
* @param lddifr
* @param dsigma
* @param work
* @param info
*
*/
abstract public void dlasd8(int icompq, int k, double[] d, double[] z, double[] vf, double[] vl, double[] difl, double[] difr, int lddifr, double[] dsigma, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLASD8 finds the square roots of the roots of the secular equation,
* as defined by the values in DSIGMA and Z. It makes the appropriate
* calls to DLASD4, and stores, for each element in D, the distance
* to its two nearest poles (elements in DSIGMA). It also updates
* the arrays VF and VL, the first and last components of all the
* right singular vectors of the original bidiagonal matrix.
*
* DLASD8 is called from DLASD6.
*
* Arguments
* =========
*
* ICOMPQ (input) INTEGER
* Specifies whether singular vectors are to be computed in
* factored form in the calling routine:
* = 0: Compute singular values only.
* = 1: Compute singular vectors in factored form as well.
*
* K (input) INTEGER
* The number of terms in the rational function to be solved
* by DLASD4. K >= 1.
*
* D (output) DOUBLE PRECISION array, dimension ( K )
* On output, D contains the updated singular values.
*
* Z (input) DOUBLE PRECISION array, dimension ( K )
* The first K elements of this array contain the components
* of the deflation-adjusted updating row vector.
*
* VF (input/output) DOUBLE PRECISION array, dimension ( K )
* On entry, VF contains information passed through DBEDE8.
* On exit, VF contains the first K components of the first
* components of all right singular vectors of the bidiagonal
* matrix.
*
* VL (input/output) DOUBLE PRECISION array, dimension ( K )
* On entry, VL contains information passed through DBEDE8.
* On exit, VL contains the first K components of the last
* components of all right singular vectors of the bidiagonal
* matrix.
*
* DIFL (output) DOUBLE PRECISION array, dimension ( K )
* On exit, DIFL(I) = D(I) - DSIGMA(I).
*
* DIFR (output) DOUBLE PRECISION array,
* dimension ( LDDIFR, 2 ) if ICOMPQ = 1 and
* dimension ( K ) if ICOMPQ = 0.
* On exit, DIFR(I,1) = D(I) - DSIGMA(I+1), DIFR(K,1) is not
* defined and will not be referenced.
*
* If ICOMPQ = 1, DIFR(1:K,2) is an array containing the
* normalizing factors for the right singular vector matrix.
*
* LDDIFR (input) INTEGER
* The leading dimension of DIFR, must be at least K.
*
* DSIGMA (input) DOUBLE PRECISION array, dimension ( K )
* The first K elements of this array contain the old roots
* of the deflated updating problem. These are the poles
* of the secular equation.
*
* WORK (workspace) DOUBLE PRECISION array, dimension at least 3 * K
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an singular value did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param k
* @param d
* @param _d_offset
* @param z
* @param _z_offset
* @param vf
* @param _vf_offset
* @param vl
* @param _vl_offset
* @param difl
* @param _difl_offset
* @param difr
* @param _difr_offset
* @param lddifr
* @param dsigma
* @param _dsigma_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dlasd8(int icompq, int k, double[] d, int _d_offset, double[] z, int _z_offset, double[] vf, int _vf_offset, double[] vl, int _vl_offset, double[] difl, int _difl_offset, double[] difr, int _difr_offset, int lddifr, double[] dsigma, int _dsigma_offset, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* Using a divide and conquer approach, DLASDA computes the singular
* value decomposition (SVD) of a real upper bidiagonal N-by-M matrix
* B with diagonal D and offdiagonal E, where M = N + SQRE. The
* algorithm computes the singular values in the SVD B = U * S * VT.
* The orthogonal matrices U and VT are optionally computed in
* compact form.
*
* A related subroutine, DLASD0, computes the singular values and
* the singular vectors in explicit form.
*
* Arguments
* =========
*
* ICOMPQ (input) INTEGER
* Specifies whether singular vectors are to be computed
* in compact form, as follows
* = 0: Compute singular values only.
* = 1: Compute singular vectors of upper bidiagonal
* matrix in compact form.
*
* SMLSIZ (input) INTEGER
* The maximum size of the subproblems at the bottom of the
* computation tree.
*
* N (input) INTEGER
* The row dimension of the upper bidiagonal matrix. This is
* also the dimension of the main diagonal array D.
*
* SQRE (input) INTEGER
* Specifies the column dimension of the bidiagonal matrix.
* = 0: The bidiagonal matrix has column dimension M = N;
* = 1: The bidiagonal matrix has column dimension M = N + 1.
*
* D (input/output) DOUBLE PRECISION array, dimension ( N )
* On entry D contains the main diagonal of the bidiagonal
* matrix. On exit D, if INFO = 0, contains its singular values.
*
* E (input) DOUBLE PRECISION array, dimension ( M-1 )
* Contains the subdiagonal entries of the bidiagonal matrix.
* On exit, E has been destroyed.
*
* U (output) DOUBLE PRECISION array,
* dimension ( LDU, SMLSIZ ) if ICOMPQ = 1, and not referenced
* if ICOMPQ = 0. If ICOMPQ = 1, on exit, U contains the left
* singular vector matrices of all subproblems at the bottom
* level.
*
* LDU (input) INTEGER, LDU = > N.
* The leading dimension of arrays U, VT, DIFL, DIFR, POLES,
* GIVNUM, and Z.
*
* VT (output) DOUBLE PRECISION array,
* dimension ( LDU, SMLSIZ+1 ) if ICOMPQ = 1, and not referenced
* if ICOMPQ = 0. If ICOMPQ = 1, on exit, VT' contains the right
* singular vector matrices of all subproblems at the bottom
* level.
*
* K (output) INTEGER array,
* dimension ( N ) if ICOMPQ = 1 and dimension 1 if ICOMPQ = 0.
* If ICOMPQ = 1, on exit, K(I) is the dimension of the I-th
* secular equation on the computation tree.
*
* DIFL (output) DOUBLE PRECISION array, dimension ( LDU, NLVL ),
* where NLVL = floor(log_2 (N/SMLSIZ))).
*
* DIFR (output) DOUBLE PRECISION array,
* dimension ( LDU, 2 * NLVL ) if ICOMPQ = 1 and
* dimension ( N ) if ICOMPQ = 0.
* If ICOMPQ = 1, on exit, DIFL(1:N, I) and DIFR(1:N, 2 * I - 1)
* record distances between singular values on the I-th
* level and singular values on the (I -1)-th level, and
* DIFR(1:N, 2 * I ) contains the normalizing factors for
* the right singular vector matrix. See DLASD8 for details.
*
* Z (output) DOUBLE PRECISION array,
* dimension ( LDU, NLVL ) if ICOMPQ = 1 and
* dimension ( N ) if ICOMPQ = 0.
* The first K elements of Z(1, I) contain the components of
* the deflation-adjusted updating row vector for subproblems
* on the I-th level.
*
* POLES (output) DOUBLE PRECISION array,
* dimension ( LDU, 2 * NLVL ) if ICOMPQ = 1, and not referenced
* if ICOMPQ = 0. If ICOMPQ = 1, on exit, POLES(1, 2*I - 1) and
* POLES(1, 2*I) contain the new and old singular values
* involved in the secular equations on the I-th level.
*
* GIVPTR (output) INTEGER array,
* dimension ( N ) if ICOMPQ = 1, and not referenced if
* ICOMPQ = 0. If ICOMPQ = 1, on exit, GIVPTR( I ) records
* the number of Givens rotations performed on the I-th
* problem on the computation tree.
*
* GIVCOL (output) INTEGER array,
* dimension ( LDGCOL, 2 * NLVL ) if ICOMPQ = 1, and not
* referenced if ICOMPQ = 0. If ICOMPQ = 1, on exit, for each I,
* GIVCOL(1, 2 *I - 1) and GIVCOL(1, 2 *I) record the locations
* of Givens rotations performed on the I-th level on the
* computation tree.
*
* LDGCOL (input) INTEGER, LDGCOL = > N.
* The leading dimension of arrays GIVCOL and PERM.
*
* PERM (output) INTEGER array,
* dimension ( LDGCOL, NLVL ) if ICOMPQ = 1, and not referenced
* if ICOMPQ = 0. If ICOMPQ = 1, on exit, PERM(1, I) records
* permutations done on the I-th level of the computation tree.
*
* GIVNUM (output) DOUBLE PRECISION array,
* dimension ( LDU, 2 * NLVL ) if ICOMPQ = 1, and not
* referenced if ICOMPQ = 0. If ICOMPQ = 1, on exit, for each I,
* GIVNUM(1, 2 *I - 1) and GIVNUM(1, 2 *I) record the C- and S-
* values of Givens rotations performed on the I-th level on
* the computation tree.
*
* C (output) DOUBLE PRECISION array,
* dimension ( N ) if ICOMPQ = 1, and dimension 1 if ICOMPQ = 0.
* If ICOMPQ = 1 and the I-th subproblem is not square, on exit,
* C( I ) contains the C-value of a Givens rotation related to
* the right null space of the I-th subproblem.
*
* S (output) DOUBLE PRECISION array, dimension ( N ) if
* ICOMPQ = 1, and dimension 1 if ICOMPQ = 0. If ICOMPQ = 1
* and the I-th subproblem is not square, on exit, S( I )
* contains the S-value of a Givens rotation related to
* the right null space of the I-th subproblem.
*
* WORK (workspace) DOUBLE PRECISION array, dimension
* (6 * N + (SMLSIZ + 1)*(SMLSIZ + 1)).
*
* IWORK (workspace) INTEGER array.
* Dimension must be at least (7 * N).
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an singular value did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param smlsiz
* @param n
* @param sqre
* @param d
* @param e
* @param u
* @param ldu
* @param vt
* @param k
* @param difl
* @param difr
* @param z
* @param poles
* @param givptr
* @param givcol
* @param ldgcol
* @param perm
* @param givnum
* @param c
* @param s
* @param work
* @param iwork
* @param info
*
*/
abstract public void dlasda(int icompq, int smlsiz, int n, int sqre, double[] d, double[] e, double[] u, int ldu, double[] vt, int[] k, double[] difl, double[] difr, double[] z, double[] poles, int[] givptr, int[] givcol, int ldgcol, int[] perm, double[] givnum, double[] c, double[] s, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* Using a divide and conquer approach, DLASDA computes the singular
* value decomposition (SVD) of a real upper bidiagonal N-by-M matrix
* B with diagonal D and offdiagonal E, where M = N + SQRE. The
* algorithm computes the singular values in the SVD B = U * S * VT.
* The orthogonal matrices U and VT are optionally computed in
* compact form.
*
* A related subroutine, DLASD0, computes the singular values and
* the singular vectors in explicit form.
*
* Arguments
* =========
*
* ICOMPQ (input) INTEGER
* Specifies whether singular vectors are to be computed
* in compact form, as follows
* = 0: Compute singular values only.
* = 1: Compute singular vectors of upper bidiagonal
* matrix in compact form.
*
* SMLSIZ (input) INTEGER
* The maximum size of the subproblems at the bottom of the
* computation tree.
*
* N (input) INTEGER
* The row dimension of the upper bidiagonal matrix. This is
* also the dimension of the main diagonal array D.
*
* SQRE (input) INTEGER
* Specifies the column dimension of the bidiagonal matrix.
* = 0: The bidiagonal matrix has column dimension M = N;
* = 1: The bidiagonal matrix has column dimension M = N + 1.
*
* D (input/output) DOUBLE PRECISION array, dimension ( N )
* On entry D contains the main diagonal of the bidiagonal
* matrix. On exit D, if INFO = 0, contains its singular values.
*
* E (input) DOUBLE PRECISION array, dimension ( M-1 )
* Contains the subdiagonal entries of the bidiagonal matrix.
* On exit, E has been destroyed.
*
* U (output) DOUBLE PRECISION array,
* dimension ( LDU, SMLSIZ ) if ICOMPQ = 1, and not referenced
* if ICOMPQ = 0. If ICOMPQ = 1, on exit, U contains the left
* singular vector matrices of all subproblems at the bottom
* level.
*
* LDU (input) INTEGER, LDU = > N.
* The leading dimension of arrays U, VT, DIFL, DIFR, POLES,
* GIVNUM, and Z.
*
* VT (output) DOUBLE PRECISION array,
* dimension ( LDU, SMLSIZ+1 ) if ICOMPQ = 1, and not referenced
* if ICOMPQ = 0. If ICOMPQ = 1, on exit, VT' contains the right
* singular vector matrices of all subproblems at the bottom
* level.
*
* K (output) INTEGER array,
* dimension ( N ) if ICOMPQ = 1 and dimension 1 if ICOMPQ = 0.
* If ICOMPQ = 1, on exit, K(I) is the dimension of the I-th
* secular equation on the computation tree.
*
* DIFL (output) DOUBLE PRECISION array, dimension ( LDU, NLVL ),
* where NLVL = floor(log_2 (N/SMLSIZ))).
*
* DIFR (output) DOUBLE PRECISION array,
* dimension ( LDU, 2 * NLVL ) if ICOMPQ = 1 and
* dimension ( N ) if ICOMPQ = 0.
* If ICOMPQ = 1, on exit, DIFL(1:N, I) and DIFR(1:N, 2 * I - 1)
* record distances between singular values on the I-th
* level and singular values on the (I -1)-th level, and
* DIFR(1:N, 2 * I ) contains the normalizing factors for
* the right singular vector matrix. See DLASD8 for details.
*
* Z (output) DOUBLE PRECISION array,
* dimension ( LDU, NLVL ) if ICOMPQ = 1 and
* dimension ( N ) if ICOMPQ = 0.
* The first K elements of Z(1, I) contain the components of
* the deflation-adjusted updating row vector for subproblems
* on the I-th level.
*
* POLES (output) DOUBLE PRECISION array,
* dimension ( LDU, 2 * NLVL ) if ICOMPQ = 1, and not referenced
* if ICOMPQ = 0. If ICOMPQ = 1, on exit, POLES(1, 2*I - 1) and
* POLES(1, 2*I) contain the new and old singular values
* involved in the secular equations on the I-th level.
*
* GIVPTR (output) INTEGER array,
* dimension ( N ) if ICOMPQ = 1, and not referenced if
* ICOMPQ = 0. If ICOMPQ = 1, on exit, GIVPTR( I ) records
* the number of Givens rotations performed on the I-th
* problem on the computation tree.
*
* GIVCOL (output) INTEGER array,
* dimension ( LDGCOL, 2 * NLVL ) if ICOMPQ = 1, and not
* referenced if ICOMPQ = 0. If ICOMPQ = 1, on exit, for each I,
* GIVCOL(1, 2 *I - 1) and GIVCOL(1, 2 *I) record the locations
* of Givens rotations performed on the I-th level on the
* computation tree.
*
* LDGCOL (input) INTEGER, LDGCOL = > N.
* The leading dimension of arrays GIVCOL and PERM.
*
* PERM (output) INTEGER array,
* dimension ( LDGCOL, NLVL ) if ICOMPQ = 1, and not referenced
* if ICOMPQ = 0. If ICOMPQ = 1, on exit, PERM(1, I) records
* permutations done on the I-th level of the computation tree.
*
* GIVNUM (output) DOUBLE PRECISION array,
* dimension ( LDU, 2 * NLVL ) if ICOMPQ = 1, and not
* referenced if ICOMPQ = 0. If ICOMPQ = 1, on exit, for each I,
* GIVNUM(1, 2 *I - 1) and GIVNUM(1, 2 *I) record the C- and S-
* values of Givens rotations performed on the I-th level on
* the computation tree.
*
* C (output) DOUBLE PRECISION array,
* dimension ( N ) if ICOMPQ = 1, and dimension 1 if ICOMPQ = 0.
* If ICOMPQ = 1 and the I-th subproblem is not square, on exit,
* C( I ) contains the C-value of a Givens rotation related to
* the right null space of the I-th subproblem.
*
* S (output) DOUBLE PRECISION array, dimension ( N ) if
* ICOMPQ = 1, and dimension 1 if ICOMPQ = 0. If ICOMPQ = 1
* and the I-th subproblem is not square, on exit, S( I )
* contains the S-value of a Givens rotation related to
* the right null space of the I-th subproblem.
*
* WORK (workspace) DOUBLE PRECISION array, dimension
* (6 * N + (SMLSIZ + 1)*(SMLSIZ + 1)).
*
* IWORK (workspace) INTEGER array.
* Dimension must be at least (7 * N).
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an singular value did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param smlsiz
* @param n
* @param sqre
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param u
* @param _u_offset
* @param ldu
* @param vt
* @param _vt_offset
* @param k
* @param _k_offset
* @param difl
* @param _difl_offset
* @param difr
* @param _difr_offset
* @param z
* @param _z_offset
* @param poles
* @param _poles_offset
* @param givptr
* @param _givptr_offset
* @param givcol
* @param _givcol_offset
* @param ldgcol
* @param perm
* @param _perm_offset
* @param givnum
* @param _givnum_offset
* @param c
* @param _c_offset
* @param s
* @param _s_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dlasda(int icompq, int smlsiz, int n, int sqre, double[] d, int _d_offset, double[] e, int _e_offset, double[] u, int _u_offset, int ldu, double[] vt, int _vt_offset, int[] k, int _k_offset, double[] difl, int _difl_offset, double[] difr, int _difr_offset, double[] z, int _z_offset, double[] poles, int _poles_offset, int[] givptr, int _givptr_offset, int[] givcol, int _givcol_offset, int ldgcol, int[] perm, int _perm_offset, double[] givnum, int _givnum_offset, double[] c, int _c_offset, double[] s, int _s_offset, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLASDQ computes the singular value decomposition (SVD) of a real
* (upper or lower) bidiagonal matrix with diagonal D and offdiagonal
* E, accumulating the transformations if desired. Letting B denote
* the input bidiagonal matrix, the algorithm computes orthogonal
* matrices Q and P such that B = Q * S * P' (P' denotes the transpose
* of P). The singular values S are overwritten on D.
*
* The input matrix U is changed to U * Q if desired.
* The input matrix VT is changed to P' * VT if desired.
* The input matrix C is changed to Q' * C if desired.
*
* See "Computing Small Singular Values of Bidiagonal Matrices With
* Guaranteed High Relative Accuracy," by J. Demmel and W. Kahan,
* LAPACK Working Note #3, for a detailed description of the algorithm.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* On entry, UPLO specifies whether the input bidiagonal matrix
* is upper or lower bidiagonal, and wether it is square are
* not.
* UPLO = 'U' or 'u' B is upper bidiagonal.
* UPLO = 'L' or 'l' B is lower bidiagonal.
*
* SQRE (input) INTEGER
* = 0: then the input matrix is N-by-N.
* = 1: then the input matrix is N-by-(N+1) if UPLU = 'U' and
* (N+1)-by-N if UPLU = 'L'.
*
* The bidiagonal matrix has
* N = NL + NR + 1 rows and
* M = N + SQRE >= N columns.
*
* N (input) INTEGER
* On entry, N specifies the number of rows and columns
* in the matrix. N must be at least 0.
*
* NCVT (input) INTEGER
* On entry, NCVT specifies the number of columns of
* the matrix VT. NCVT must be at least 0.
*
* NRU (input) INTEGER
* On entry, NRU specifies the number of rows of
* the matrix U. NRU must be at least 0.
*
* NCC (input) INTEGER
* On entry, NCC specifies the number of columns of
* the matrix C. NCC must be at least 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, D contains the diagonal entries of the
* bidiagonal matrix whose SVD is desired. On normal exit,
* D contains the singular values in ascending order.
*
* E (input/output) DOUBLE PRECISION array.
* dimension is (N-1) if SQRE = 0 and N if SQRE = 1.
* On entry, the entries of E contain the offdiagonal entries
* of the bidiagonal matrix whose SVD is desired. On normal
* exit, E will contain 0. If the algorithm does not converge,
* D and E will contain the diagonal and superdiagonal entries
* of a bidiagonal matrix orthogonally equivalent to the one
* given as input.
*
* VT (input/output) DOUBLE PRECISION array, dimension (LDVT, NCVT)
* On entry, contains a matrix which on exit has been
* premultiplied by P', dimension N-by-NCVT if SQRE = 0
* and (N+1)-by-NCVT if SQRE = 1 (not referenced if NCVT=0).
*
* LDVT (input) INTEGER
* On entry, LDVT specifies the leading dimension of VT as
* declared in the calling (sub) program. LDVT must be at
* least 1. If NCVT is nonzero LDVT must also be at least N.
*
* U (input/output) DOUBLE PRECISION array, dimension (LDU, N)
* On entry, contains a matrix which on exit has been
* postmultiplied by Q, dimension NRU-by-N if SQRE = 0
* and NRU-by-(N+1) if SQRE = 1 (not referenced if NRU=0).
*
* LDU (input) INTEGER
* On entry, LDU specifies the leading dimension of U as
* declared in the calling (sub) program. LDU must be at
* least max( 1, NRU ) .
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC, NCC)
* On entry, contains an N-by-NCC matrix which on exit
* has been premultiplied by Q' dimension N-by-NCC if SQRE = 0
* and (N+1)-by-NCC if SQRE = 1 (not referenced if NCC=0).
*
* LDC (input) INTEGER
* On entry, LDC specifies the leading dimension of C as
* declared in the calling (sub) program. LDC must be at
* least 1. If NCC is nonzero, LDC must also be at least N.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (4*N)
* Workspace. Only referenced if one of NCVT, NRU, or NCC is
* nonzero, and if N is at least 2.
*
* INFO (output) INTEGER
* On exit, a value of 0 indicates a successful exit.
* If INFO < 0, argument number -INFO is illegal.
* If INFO > 0, the algorithm did not converge, and INFO
* specifies how many superdiagonals did not converge.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param sqre
* @param n
* @param ncvt
* @param nru
* @param ncc
* @param d
* @param e
* @param vt
* @param ldvt
* @param u
* @param ldu
* @param c
* @param Ldc
* @param work
* @param info
*
*/
abstract public void dlasdq(java.lang.String uplo, int sqre, int n, int ncvt, int nru, int ncc, double[] d, double[] e, double[] vt, int ldvt, double[] u, int ldu, double[] c, int Ldc, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLASDQ computes the singular value decomposition (SVD) of a real
* (upper or lower) bidiagonal matrix with diagonal D and offdiagonal
* E, accumulating the transformations if desired. Letting B denote
* the input bidiagonal matrix, the algorithm computes orthogonal
* matrices Q and P such that B = Q * S * P' (P' denotes the transpose
* of P). The singular values S are overwritten on D.
*
* The input matrix U is changed to U * Q if desired.
* The input matrix VT is changed to P' * VT if desired.
* The input matrix C is changed to Q' * C if desired.
*
* See "Computing Small Singular Values of Bidiagonal Matrices With
* Guaranteed High Relative Accuracy," by J. Demmel and W. Kahan,
* LAPACK Working Note #3, for a detailed description of the algorithm.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* On entry, UPLO specifies whether the input bidiagonal matrix
* is upper or lower bidiagonal, and wether it is square are
* not.
* UPLO = 'U' or 'u' B is upper bidiagonal.
* UPLO = 'L' or 'l' B is lower bidiagonal.
*
* SQRE (input) INTEGER
* = 0: then the input matrix is N-by-N.
* = 1: then the input matrix is N-by-(N+1) if UPLU = 'U' and
* (N+1)-by-N if UPLU = 'L'.
*
* The bidiagonal matrix has
* N = NL + NR + 1 rows and
* M = N + SQRE >= N columns.
*
* N (input) INTEGER
* On entry, N specifies the number of rows and columns
* in the matrix. N must be at least 0.
*
* NCVT (input) INTEGER
* On entry, NCVT specifies the number of columns of
* the matrix VT. NCVT must be at least 0.
*
* NRU (input) INTEGER
* On entry, NRU specifies the number of rows of
* the matrix U. NRU must be at least 0.
*
* NCC (input) INTEGER
* On entry, NCC specifies the number of columns of
* the matrix C. NCC must be at least 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, D contains the diagonal entries of the
* bidiagonal matrix whose SVD is desired. On normal exit,
* D contains the singular values in ascending order.
*
* E (input/output) DOUBLE PRECISION array.
* dimension is (N-1) if SQRE = 0 and N if SQRE = 1.
* On entry, the entries of E contain the offdiagonal entries
* of the bidiagonal matrix whose SVD is desired. On normal
* exit, E will contain 0. If the algorithm does not converge,
* D and E will contain the diagonal and superdiagonal entries
* of a bidiagonal matrix orthogonally equivalent to the one
* given as input.
*
* VT (input/output) DOUBLE PRECISION array, dimension (LDVT, NCVT)
* On entry, contains a matrix which on exit has been
* premultiplied by P', dimension N-by-NCVT if SQRE = 0
* and (N+1)-by-NCVT if SQRE = 1 (not referenced if NCVT=0).
*
* LDVT (input) INTEGER
* On entry, LDVT specifies the leading dimension of VT as
* declared in the calling (sub) program. LDVT must be at
* least 1. If NCVT is nonzero LDVT must also be at least N.
*
* U (input/output) DOUBLE PRECISION array, dimension (LDU, N)
* On entry, contains a matrix which on exit has been
* postmultiplied by Q, dimension NRU-by-N if SQRE = 0
* and NRU-by-(N+1) if SQRE = 1 (not referenced if NRU=0).
*
* LDU (input) INTEGER
* On entry, LDU specifies the leading dimension of U as
* declared in the calling (sub) program. LDU must be at
* least max( 1, NRU ) .
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC, NCC)
* On entry, contains an N-by-NCC matrix which on exit
* has been premultiplied by Q' dimension N-by-NCC if SQRE = 0
* and (N+1)-by-NCC if SQRE = 1 (not referenced if NCC=0).
*
* LDC (input) INTEGER
* On entry, LDC specifies the leading dimension of C as
* declared in the calling (sub) program. LDC must be at
* least 1. If NCC is nonzero, LDC must also be at least N.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (4*N)
* Workspace. Only referenced if one of NCVT, NRU, or NCC is
* nonzero, and if N is at least 2.
*
* INFO (output) INTEGER
* On exit, a value of 0 indicates a successful exit.
* If INFO < 0, argument number -INFO is illegal.
* If INFO > 0, the algorithm did not converge, and INFO
* specifies how many superdiagonals did not converge.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param sqre
* @param n
* @param ncvt
* @param nru
* @param ncc
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param vt
* @param _vt_offset
* @param ldvt
* @param u
* @param _u_offset
* @param ldu
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dlasdq(java.lang.String uplo, int sqre, int n, int ncvt, int nru, int ncc, double[] d, int _d_offset, double[] e, int _e_offset, double[] vt, int _vt_offset, int ldvt, double[] u, int _u_offset, int ldu, double[] c, int _c_offset, int Ldc, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLASDT creates a tree of subproblems for bidiagonal divide and
* conquer.
*
* Arguments
* =========
*
* N (input) INTEGER
* On entry, the number of diagonal elements of the
* bidiagonal matrix.
*
* LVL (output) INTEGER
* On exit, the number of levels on the computation tree.
*
* ND (output) INTEGER
* On exit, the number of nodes on the tree.
*
* INODE (output) INTEGER array, dimension ( N )
* On exit, centers of subproblems.
*
* NDIML (output) INTEGER array, dimension ( N )
* On exit, row dimensions of left children.
*
* NDIMR (output) INTEGER array, dimension ( N )
* On exit, row dimensions of right children.
*
* MSUB (input) INTEGER.
* On entry, the maximum row dimension each subproblem at the
* bottom of the tree can be of.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param lvl
* @param nd
* @param inode
* @param ndiml
* @param ndimr
* @param msub
*
*/
abstract public void dlasdt(int n, org.netlib.util.intW lvl, org.netlib.util.intW nd, int[] inode, int[] ndiml, int[] ndimr, int msub);
/**
*
* ..
*
* Purpose
* =======
*
* DLASDT creates a tree of subproblems for bidiagonal divide and
* conquer.
*
* Arguments
* =========
*
* N (input) INTEGER
* On entry, the number of diagonal elements of the
* bidiagonal matrix.
*
* LVL (output) INTEGER
* On exit, the number of levels on the computation tree.
*
* ND (output) INTEGER
* On exit, the number of nodes on the tree.
*
* INODE (output) INTEGER array, dimension ( N )
* On exit, centers of subproblems.
*
* NDIML (output) INTEGER array, dimension ( N )
* On exit, row dimensions of left children.
*
* NDIMR (output) INTEGER array, dimension ( N )
* On exit, row dimensions of right children.
*
* MSUB (input) INTEGER.
* On entry, the maximum row dimension each subproblem at the
* bottom of the tree can be of.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param lvl
* @param nd
* @param inode
* @param _inode_offset
* @param ndiml
* @param _ndiml_offset
* @param ndimr
* @param _ndimr_offset
* @param msub
*
*/
abstract public void dlasdt(int n, org.netlib.util.intW lvl, org.netlib.util.intW nd, int[] inode, int _inode_offset, int[] ndiml, int _ndiml_offset, int[] ndimr, int _ndimr_offset, int msub);
/**
*
* ..
*
* Purpose
* =======
*
* DLASET initializes an m-by-n matrix A to BETA on the diagonal and
* ALPHA on the offdiagonals.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies the part of the matrix A to be set.
* = 'U': Upper triangular part is set; the strictly lower
* triangular part of A is not changed.
* = 'L': Lower triangular part is set; the strictly upper
* triangular part of A is not changed.
* Otherwise: All of the matrix A is set.
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* ALPHA (input) DOUBLE PRECISION
* The constant to which the offdiagonal elements are to be set.
*
* BETA (input) DOUBLE PRECISION
* The constant to which the diagonal elements are to be set.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On exit, the leading m-by-n submatrix of A is set as follows:
*
* if UPLO = 'U', A(i,j) = ALPHA, 1<=i<=j-1, 1<=j<=n,
* if UPLO = 'L', A(i,j) = ALPHA, j+1<=i<=m, 1<=j<=n,
* otherwise, A(i,j) = ALPHA, 1<=i<=m, 1<=j<=n, i.ne.j,
*
* and, for all UPLO, A(i,i) = BETA, 1<=i<=min(m,n).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param uplo
* @param m
* @param n
* @param alpha
* @param beta
* @param a
* @param lda
*
*/
abstract public void dlaset(java.lang.String uplo, int m, int n, double alpha, double beta, double[] a, int lda);
/**
*
* ..
*
* Purpose
* =======
*
* DLASET initializes an m-by-n matrix A to BETA on the diagonal and
* ALPHA on the offdiagonals.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies the part of the matrix A to be set.
* = 'U': Upper triangular part is set; the strictly lower
* triangular part of A is not changed.
* = 'L': Lower triangular part is set; the strictly upper
* triangular part of A is not changed.
* Otherwise: All of the matrix A is set.
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* ALPHA (input) DOUBLE PRECISION
* The constant to which the offdiagonal elements are to be set.
*
* BETA (input) DOUBLE PRECISION
* The constant to which the diagonal elements are to be set.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On exit, the leading m-by-n submatrix of A is set as follows:
*
* if UPLO = 'U', A(i,j) = ALPHA, 1<=i<=j-1, 1<=j<=n,
* if UPLO = 'L', A(i,j) = ALPHA, j+1<=i<=m, 1<=j<=n,
* otherwise, A(i,j) = ALPHA, 1<=i<=m, 1<=j<=n, i.ne.j,
*
* and, for all UPLO, A(i,i) = BETA, 1<=i<=min(m,n).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param uplo
* @param m
* @param n
* @param alpha
* @param beta
* @param a
* @param _a_offset
* @param lda
*
*/
abstract public void dlaset(java.lang.String uplo, int m, int n, double alpha, double beta, double[] a, int _a_offset, int lda);
/**
*
* ..
*
* Purpose
* =======
*
* DLASQ1 computes the singular values of a real N-by-N bidiagonal
* matrix with diagonal D and off-diagonal E. The singular values
* are computed to high relative accuracy, in the absence of
* denormalization, underflow and overflow. The algorithm was first
* presented in
*
* "Accurate singular values and differential qd algorithms" by K. V.
* Fernando and B. N. Parlett, Numer. Math., Vol-67, No. 2, pp. 191-230,
* 1994,
*
* and the present implementation is described in "An implementation of
* the dqds Algorithm (Positive Case)", LAPACK Working Note.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of rows and columns in the matrix. N >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, D contains the diagonal elements of the
* bidiagonal matrix whose SVD is desired. On normal exit,
* D contains the singular values in decreasing order.
*
* E (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, elements E(1:N-1) contain the off-diagonal elements
* of the bidiagonal matrix whose SVD is desired.
* On exit, E is overwritten.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (4*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: the algorithm failed
* = 1, a split was marked by a positive value in E
* = 2, current block of Z not diagonalized after 30*N
* iterations (in inner while loop)
* = 3, termination criterion of outer while loop not met
* (program created more than N unreduced blocks)
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param e
* @param work
* @param info
*
*/
abstract public void dlasq1(int n, double[] d, double[] e, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLASQ1 computes the singular values of a real N-by-N bidiagonal
* matrix with diagonal D and off-diagonal E. The singular values
* are computed to high relative accuracy, in the absence of
* denormalization, underflow and overflow. The algorithm was first
* presented in
*
* "Accurate singular values and differential qd algorithms" by K. V.
* Fernando and B. N. Parlett, Numer. Math., Vol-67, No. 2, pp. 191-230,
* 1994,
*
* and the present implementation is described in "An implementation of
* the dqds Algorithm (Positive Case)", LAPACK Working Note.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of rows and columns in the matrix. N >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, D contains the diagonal elements of the
* bidiagonal matrix whose SVD is desired. On normal exit,
* D contains the singular values in decreasing order.
*
* E (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, elements E(1:N-1) contain the off-diagonal elements
* of the bidiagonal matrix whose SVD is desired.
* On exit, E is overwritten.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (4*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: the algorithm failed
* = 1, a split was marked by a positive value in E
* = 2, current block of Z not diagonalized after 30*N
* iterations (in inner while loop)
* = 3, termination criterion of outer while loop not met
* (program created more than N unreduced blocks)
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dlasq1(int n, double[] d, int _d_offset, double[] e, int _e_offset, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLASQ2 computes all the eigenvalues of the symmetric positive
* definite tridiagonal matrix associated with the qd array Z to high
* relative accuracy are computed to high relative accuracy, in the
* absence of denormalization, underflow and overflow.
*
* To see the relation of Z to the tridiagonal matrix, let L be a
* unit lower bidiagonal matrix with subdiagonals Z(2,4,6,,..) and
* let U be an upper bidiagonal matrix with 1's above and diagonal
* Z(1,3,5,,..). The tridiagonal is L*U or, if you prefer, the
* symmetric tridiagonal to which it is similar.
*
* Note : DLASQ2 defines a logical variable, IEEE, which is true
* on machines which follow ieee-754 floating-point standard in their
* handling of infinities and NaNs, and false otherwise. This variable
* is passed to DLAZQ3.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of rows and columns in the matrix. N >= 0.
*
* Z (workspace) DOUBLE PRECISION array, dimension ( 4*N )
* On entry Z holds the qd array. On exit, entries 1 to N hold
* the eigenvalues in decreasing order, Z( 2*N+1 ) holds the
* trace, and Z( 2*N+2 ) holds the sum of the eigenvalues. If
* N > 2, then Z( 2*N+3 ) holds the iteration count, Z( 2*N+4 )
* holds NDIVS/NIN^2, and Z( 2*N+5 ) holds the percentage of
* shifts that failed.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if the i-th argument is a scalar and had an illegal
* value, then INFO = -i, if the i-th argument is an
* array and the j-entry had an illegal value, then
* INFO = -(i*100+j)
* > 0: the algorithm failed
* = 1, a split was marked by a positive value in E
* = 2, current block of Z not diagonalized after 30*N
* iterations (in inner while loop)
* = 3, termination criterion of outer while loop not met
* (program created more than N unreduced blocks)
*
* Further Details
* ===============
* Local Variables: I0:N0 defines a current unreduced segment of Z.
* The shifts are accumulated in SIGMA. Iteration count is in ITER.
* Ping-pong is controlled by PP (alternates between 0 and 1).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param z
* @param info
*
*/
abstract public void dlasq2(int n, double[] z, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLASQ2 computes all the eigenvalues of the symmetric positive
* definite tridiagonal matrix associated with the qd array Z to high
* relative accuracy are computed to high relative accuracy, in the
* absence of denormalization, underflow and overflow.
*
* To see the relation of Z to the tridiagonal matrix, let L be a
* unit lower bidiagonal matrix with subdiagonals Z(2,4,6,,..) and
* let U be an upper bidiagonal matrix with 1's above and diagonal
* Z(1,3,5,,..). The tridiagonal is L*U or, if you prefer, the
* symmetric tridiagonal to which it is similar.
*
* Note : DLASQ2 defines a logical variable, IEEE, which is true
* on machines which follow ieee-754 floating-point standard in their
* handling of infinities and NaNs, and false otherwise. This variable
* is passed to DLAZQ3.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of rows and columns in the matrix. N >= 0.
*
* Z (workspace) DOUBLE PRECISION array, dimension ( 4*N )
* On entry Z holds the qd array. On exit, entries 1 to N hold
* the eigenvalues in decreasing order, Z( 2*N+1 ) holds the
* trace, and Z( 2*N+2 ) holds the sum of the eigenvalues. If
* N > 2, then Z( 2*N+3 ) holds the iteration count, Z( 2*N+4 )
* holds NDIVS/NIN^2, and Z( 2*N+5 ) holds the percentage of
* shifts that failed.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if the i-th argument is a scalar and had an illegal
* value, then INFO = -i, if the i-th argument is an
* array and the j-entry had an illegal value, then
* INFO = -(i*100+j)
* > 0: the algorithm failed
* = 1, a split was marked by a positive value in E
* = 2, current block of Z not diagonalized after 30*N
* iterations (in inner while loop)
* = 3, termination criterion of outer while loop not met
* (program created more than N unreduced blocks)
*
* Further Details
* ===============
* Local Variables: I0:N0 defines a current unreduced segment of Z.
* The shifts are accumulated in SIGMA. Iteration count is in ITER.
* Ping-pong is controlled by PP (alternates between 0 and 1).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param z
* @param _z_offset
* @param info
*
*/
abstract public void dlasq2(int n, double[] z, int _z_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLASQ3 checks for deflation, computes a shift (TAU) and calls dqds.
* In case of failure it changes shifts, and tries again until output
* is positive.
*
* Arguments
* =========
*
* I0 (input) INTEGER
* First index.
*
* N0 (input) INTEGER
* Last index.
*
* Z (input) DOUBLE PRECISION array, dimension ( 4*N )
* Z holds the qd array.
*
* PP (input) INTEGER
* PP=0 for ping, PP=1 for pong.
*
* DMIN (output) DOUBLE PRECISION
* Minimum value of d.
*
* SIGMA (output) DOUBLE PRECISION
* Sum of shifts used in current segment.
*
* DESIG (input/output) DOUBLE PRECISION
* Lower order part of SIGMA
*
* QMAX (input) DOUBLE PRECISION
* Maximum value of q.
*
* NFAIL (output) INTEGER
* Number of times shift was too big.
*
* ITER (output) INTEGER
* Number of iterations.
*
* NDIV (output) INTEGER
* Number of divisions.
*
* TTYPE (output) INTEGER
* Shift type.
*
* IEEE (input) LOGICAL
* Flag for IEEE or non IEEE arithmetic (passed to DLASQ5).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param i0
* @param n0
* @param z
* @param pp
* @param dmin
* @param sigma
* @param desig
* @param qmax
* @param nfail
* @param iter
* @param ndiv
* @param ieee
*
*/
abstract public void dlasq3(int i0, org.netlib.util.intW n0, double[] z, int pp, org.netlib.util.doubleW dmin, org.netlib.util.doubleW sigma, org.netlib.util.doubleW desig, org.netlib.util.doubleW qmax, org.netlib.util.intW nfail, org.netlib.util.intW iter, org.netlib.util.intW ndiv, boolean ieee);
/**
*
* ..
*
* Purpose
* =======
*
* DLASQ3 checks for deflation, computes a shift (TAU) and calls dqds.
* In case of failure it changes shifts, and tries again until output
* is positive.
*
* Arguments
* =========
*
* I0 (input) INTEGER
* First index.
*
* N0 (input) INTEGER
* Last index.
*
* Z (input) DOUBLE PRECISION array, dimension ( 4*N )
* Z holds the qd array.
*
* PP (input) INTEGER
* PP=0 for ping, PP=1 for pong.
*
* DMIN (output) DOUBLE PRECISION
* Minimum value of d.
*
* SIGMA (output) DOUBLE PRECISION
* Sum of shifts used in current segment.
*
* DESIG (input/output) DOUBLE PRECISION
* Lower order part of SIGMA
*
* QMAX (input) DOUBLE PRECISION
* Maximum value of q.
*
* NFAIL (output) INTEGER
* Number of times shift was too big.
*
* ITER (output) INTEGER
* Number of iterations.
*
* NDIV (output) INTEGER
* Number of divisions.
*
* TTYPE (output) INTEGER
* Shift type.
*
* IEEE (input) LOGICAL
* Flag for IEEE or non IEEE arithmetic (passed to DLASQ5).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param i0
* @param n0
* @param z
* @param _z_offset
* @param pp
* @param dmin
* @param sigma
* @param desig
* @param qmax
* @param nfail
* @param iter
* @param ndiv
* @param ieee
*
*/
abstract public void dlasq3(int i0, org.netlib.util.intW n0, double[] z, int _z_offset, int pp, org.netlib.util.doubleW dmin, org.netlib.util.doubleW sigma, org.netlib.util.doubleW desig, org.netlib.util.doubleW qmax, org.netlib.util.intW nfail, org.netlib.util.intW iter, org.netlib.util.intW ndiv, boolean ieee);
/**
*
* ..
*
* Purpose
* =======
*
* DLASQ4 computes an approximation TAU to the smallest eigenvalue
* using values of d from the previous transform.
*
* I0 (input) INTEGER
* First index.
*
* N0 (input) INTEGER
* Last index.
*
* Z (input) DOUBLE PRECISION array, dimension ( 4*N )
* Z holds the qd array.
*
* PP (input) INTEGER
* PP=0 for ping, PP=1 for pong.
*
* N0IN (input) INTEGER
* The value of N0 at start of EIGTEST.
*
* DMIN (input) DOUBLE PRECISION
* Minimum value of d.
*
* DMIN1 (input) DOUBLE PRECISION
* Minimum value of d, excluding D( N0 ).
*
* DMIN2 (input) DOUBLE PRECISION
* Minimum value of d, excluding D( N0 ) and D( N0-1 ).
*
* DN (input) DOUBLE PRECISION
* d(N)
*
* DN1 (input) DOUBLE PRECISION
* d(N-1)
*
* DN2 (input) DOUBLE PRECISION
* d(N-2)
*
* TAU (output) DOUBLE PRECISION
* This is the shift.
*
* TTYPE (output) INTEGER
* Shift type.
*
* Further Details
* ===============
* CNST1 = 9/16
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param i0
* @param n0
* @param z
* @param pp
* @param n0in
* @param dmin
* @param dmin1
* @param dmin2
* @param dn
* @param dn1
* @param dn2
* @param tau
* @param ttype
*
*/
abstract public void dlasq4(int i0, int n0, double[] z, int pp, int n0in, double dmin, double dmin1, double dmin2, double dn, double dn1, double dn2, org.netlib.util.doubleW tau, org.netlib.util.intW ttype);
/**
*
* ..
*
* Purpose
* =======
*
* DLASQ4 computes an approximation TAU to the smallest eigenvalue
* using values of d from the previous transform.
*
* I0 (input) INTEGER
* First index.
*
* N0 (input) INTEGER
* Last index.
*
* Z (input) DOUBLE PRECISION array, dimension ( 4*N )
* Z holds the qd array.
*
* PP (input) INTEGER
* PP=0 for ping, PP=1 for pong.
*
* N0IN (input) INTEGER
* The value of N0 at start of EIGTEST.
*
* DMIN (input) DOUBLE PRECISION
* Minimum value of d.
*
* DMIN1 (input) DOUBLE PRECISION
* Minimum value of d, excluding D( N0 ).
*
* DMIN2 (input) DOUBLE PRECISION
* Minimum value of d, excluding D( N0 ) and D( N0-1 ).
*
* DN (input) DOUBLE PRECISION
* d(N)
*
* DN1 (input) DOUBLE PRECISION
* d(N-1)
*
* DN2 (input) DOUBLE PRECISION
* d(N-2)
*
* TAU (output) DOUBLE PRECISION
* This is the shift.
*
* TTYPE (output) INTEGER
* Shift type.
*
* Further Details
* ===============
* CNST1 = 9/16
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param i0
* @param n0
* @param z
* @param _z_offset
* @param pp
* @param n0in
* @param dmin
* @param dmin1
* @param dmin2
* @param dn
* @param dn1
* @param dn2
* @param tau
* @param ttype
*
*/
abstract public void dlasq4(int i0, int n0, double[] z, int _z_offset, int pp, int n0in, double dmin, double dmin1, double dmin2, double dn, double dn1, double dn2, org.netlib.util.doubleW tau, org.netlib.util.intW ttype);
/**
*
* ..
*
* Purpose
* =======
*
* DLASQ5 computes one dqds transform in ping-pong form, one
* version for IEEE machines another for non IEEE machines.
*
* Arguments
* =========
*
* I0 (input) INTEGER
* First index.
*
* N0 (input) INTEGER
* Last index.
*
* Z (input) DOUBLE PRECISION array, dimension ( 4*N )
* Z holds the qd array. EMIN is stored in Z(4*N0) to avoid
* an extra argument.
*
* PP (input) INTEGER
* PP=0 for ping, PP=1 for pong.
*
* TAU (input) DOUBLE PRECISION
* This is the shift.
*
* DMIN (output) DOUBLE PRECISION
* Minimum value of d.
*
* DMIN1 (output) DOUBLE PRECISION
* Minimum value of d, excluding D( N0 ).
*
* DMIN2 (output) DOUBLE PRECISION
* Minimum value of d, excluding D( N0 ) and D( N0-1 ).
*
* DN (output) DOUBLE PRECISION
* d(N0), the last value of d.
*
* DNM1 (output) DOUBLE PRECISION
* d(N0-1).
*
* DNM2 (output) DOUBLE PRECISION
* d(N0-2).
*
* IEEE (input) LOGICAL
* Flag for IEEE or non IEEE arithmetic.
*
* =====================================================================
*
* .. Parameter ..
*
*
* @param i0
* @param n0
* @param z
* @param pp
* @param tau
* @param dmin
* @param dmin1
* @param dmin2
* @param dn
* @param dnm1
* @param dnm2
* @param ieee
*
*/
abstract public void dlasq5(int i0, int n0, double[] z, int pp, double tau, org.netlib.util.doubleW dmin, org.netlib.util.doubleW dmin1, org.netlib.util.doubleW dmin2, org.netlib.util.doubleW dn, org.netlib.util.doubleW dnm1, org.netlib.util.doubleW dnm2, boolean ieee);
/**
*
* ..
*
* Purpose
* =======
*
* DLASQ5 computes one dqds transform in ping-pong form, one
* version for IEEE machines another for non IEEE machines.
*
* Arguments
* =========
*
* I0 (input) INTEGER
* First index.
*
* N0 (input) INTEGER
* Last index.
*
* Z (input) DOUBLE PRECISION array, dimension ( 4*N )
* Z holds the qd array. EMIN is stored in Z(4*N0) to avoid
* an extra argument.
*
* PP (input) INTEGER
* PP=0 for ping, PP=1 for pong.
*
* TAU (input) DOUBLE PRECISION
* This is the shift.
*
* DMIN (output) DOUBLE PRECISION
* Minimum value of d.
*
* DMIN1 (output) DOUBLE PRECISION
* Minimum value of d, excluding D( N0 ).
*
* DMIN2 (output) DOUBLE PRECISION
* Minimum value of d, excluding D( N0 ) and D( N0-1 ).
*
* DN (output) DOUBLE PRECISION
* d(N0), the last value of d.
*
* DNM1 (output) DOUBLE PRECISION
* d(N0-1).
*
* DNM2 (output) DOUBLE PRECISION
* d(N0-2).
*
* IEEE (input) LOGICAL
* Flag for IEEE or non IEEE arithmetic.
*
* =====================================================================
*
* .. Parameter ..
*
*
* @param i0
* @param n0
* @param z
* @param _z_offset
* @param pp
* @param tau
* @param dmin
* @param dmin1
* @param dmin2
* @param dn
* @param dnm1
* @param dnm2
* @param ieee
*
*/
abstract public void dlasq5(int i0, int n0, double[] z, int _z_offset, int pp, double tau, org.netlib.util.doubleW dmin, org.netlib.util.doubleW dmin1, org.netlib.util.doubleW dmin2, org.netlib.util.doubleW dn, org.netlib.util.doubleW dnm1, org.netlib.util.doubleW dnm2, boolean ieee);
/**
*
* ..
*
* Purpose
* =======
*
* DLASQ6 computes one dqd (shift equal to zero) transform in
* ping-pong form, with protection against underflow and overflow.
*
* Arguments
* =========
*
* I0 (input) INTEGER
* First index.
*
* N0 (input) INTEGER
* Last index.
*
* Z (input) DOUBLE PRECISION array, dimension ( 4*N )
* Z holds the qd array. EMIN is stored in Z(4*N0) to avoid
* an extra argument.
*
* PP (input) INTEGER
* PP=0 for ping, PP=1 for pong.
*
* DMIN (output) DOUBLE PRECISION
* Minimum value of d.
*
* DMIN1 (output) DOUBLE PRECISION
* Minimum value of d, excluding D( N0 ).
*
* DMIN2 (output) DOUBLE PRECISION
* Minimum value of d, excluding D( N0 ) and D( N0-1 ).
*
* DN (output) DOUBLE PRECISION
* d(N0), the last value of d.
*
* DNM1 (output) DOUBLE PRECISION
* d(N0-1).
*
* DNM2 (output) DOUBLE PRECISION
* d(N0-2).
*
* =====================================================================
*
* .. Parameter ..
*
*
* @param i0
* @param n0
* @param z
* @param pp
* @param dmin
* @param dmin1
* @param dmin2
* @param dn
* @param dnm1
* @param dnm2
*
*/
abstract public void dlasq6(int i0, int n0, double[] z, int pp, org.netlib.util.doubleW dmin, org.netlib.util.doubleW dmin1, org.netlib.util.doubleW dmin2, org.netlib.util.doubleW dn, org.netlib.util.doubleW dnm1, org.netlib.util.doubleW dnm2);
/**
*
* ..
*
* Purpose
* =======
*
* DLASQ6 computes one dqd (shift equal to zero) transform in
* ping-pong form, with protection against underflow and overflow.
*
* Arguments
* =========
*
* I0 (input) INTEGER
* First index.
*
* N0 (input) INTEGER
* Last index.
*
* Z (input) DOUBLE PRECISION array, dimension ( 4*N )
* Z holds the qd array. EMIN is stored in Z(4*N0) to avoid
* an extra argument.
*
* PP (input) INTEGER
* PP=0 for ping, PP=1 for pong.
*
* DMIN (output) DOUBLE PRECISION
* Minimum value of d.
*
* DMIN1 (output) DOUBLE PRECISION
* Minimum value of d, excluding D( N0 ).
*
* DMIN2 (output) DOUBLE PRECISION
* Minimum value of d, excluding D( N0 ) and D( N0-1 ).
*
* DN (output) DOUBLE PRECISION
* d(N0), the last value of d.
*
* DNM1 (output) DOUBLE PRECISION
* d(N0-1).
*
* DNM2 (output) DOUBLE PRECISION
* d(N0-2).
*
* =====================================================================
*
* .. Parameter ..
*
*
* @param i0
* @param n0
* @param z
* @param _z_offset
* @param pp
* @param dmin
* @param dmin1
* @param dmin2
* @param dn
* @param dnm1
* @param dnm2
*
*/
abstract public void dlasq6(int i0, int n0, double[] z, int _z_offset, int pp, org.netlib.util.doubleW dmin, org.netlib.util.doubleW dmin1, org.netlib.util.doubleW dmin2, org.netlib.util.doubleW dn, org.netlib.util.doubleW dnm1, org.netlib.util.doubleW dnm2);
/**
*
* ..
*
* Purpose
* =======
*
* DLASR applies a sequence of plane rotations to a real matrix A,
* from either the left or the right.
*
* When SIDE = 'L', the transformation takes the form
*
* A := P*A
*
* and when SIDE = 'R', the transformation takes the form
*
* A := A*P**T
*
* where P is an orthogonal matrix consisting of a sequence of z plane
* rotations, with z = M when SIDE = 'L' and z = N when SIDE = 'R',
* and P**T is the transpose of P.
*
* When DIRECT = 'F' (Forward sequence), then
*
* P = P(z-1) * ... * P(2) * P(1)
*
* and when DIRECT = 'B' (Backward sequence), then
*
* P = P(1) * P(2) * ... * P(z-1)
*
* where P(k) is a plane rotation matrix defined by the 2-by-2 rotation
*
* R(k) = ( c(k) s(k) )
* = ( -s(k) c(k) ).
*
* When PIVOT = 'V' (Variable pivot), the rotation is performed
* for the plane (k,k+1), i.e., P(k) has the form
*
* P(k) = ( 1 )
* ( ... )
* ( 1 )
* ( c(k) s(k) )
* ( -s(k) c(k) )
* ( 1 )
* ( ... )
* ( 1 )
*
* where R(k) appears as a rank-2 modification to the identity matrix in
* rows and columns k and k+1.
*
* When PIVOT = 'T' (Top pivot), the rotation is performed for the
* plane (1,k+1), so P(k) has the form
*
* P(k) = ( c(k) s(k) )
* ( 1 )
* ( ... )
* ( 1 )
* ( -s(k) c(k) )
* ( 1 )
* ( ... )
* ( 1 )
*
* where R(k) appears in rows and columns 1 and k+1.
*
* Similarly, when PIVOT = 'B' (Bottom pivot), the rotation is
* performed for the plane (k,z), giving P(k) the form
*
* P(k) = ( 1 )
* ( ... )
* ( 1 )
* ( c(k) s(k) )
* ( 1 )
* ( ... )
* ( 1 )
* ( -s(k) c(k) )
*
* where R(k) appears in rows and columns k and z. The rotations are
* performed without ever forming P(k) explicitly.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* Specifies whether the plane rotation matrix P is applied to
* A on the left or the right.
* = 'L': Left, compute A := P*A
* = 'R': Right, compute A:= A*P**T
*
* PIVOT (input) CHARACTER*1
* Specifies the plane for which P(k) is a plane rotation
* matrix.
* = 'V': Variable pivot, the plane (k,k+1)
* = 'T': Top pivot, the plane (1,k+1)
* = 'B': Bottom pivot, the plane (k,z)
*
* DIRECT (input) CHARACTER*1
* Specifies whether P is a forward or backward sequence of
* plane rotations.
* = 'F': Forward, P = P(z-1)*...*P(2)*P(1)
* = 'B': Backward, P = P(1)*P(2)*...*P(z-1)
*
* M (input) INTEGER
* The number of rows of the matrix A. If m <= 1, an immediate
* return is effected.
*
* N (input) INTEGER
* The number of columns of the matrix A. If n <= 1, an
* immediate return is effected.
*
* C (input) DOUBLE PRECISION array, dimension
* (M-1) if SIDE = 'L'
* (N-1) if SIDE = 'R'
* The cosines c(k) of the plane rotations.
*
* S (input) DOUBLE PRECISION array, dimension
* (M-1) if SIDE = 'L'
* (N-1) if SIDE = 'R'
* The sines s(k) of the plane rotations. The 2-by-2 plane
* rotation part of the matrix P(k), R(k), has the form
* R(k) = ( c(k) s(k) )
* ( -s(k) c(k) ).
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* The M-by-N matrix A. On exit, A is overwritten by P*A if
* SIDE = 'R' or by A*P**T if SIDE = 'L'.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param pivot
* @param direct
* @param m
* @param n
* @param c
* @param s
* @param a
* @param lda
*
*/
abstract public void dlasr(java.lang.String side, java.lang.String pivot, java.lang.String direct, int m, int n, double[] c, double[] s, double[] a, int lda);
/**
*
* ..
*
* Purpose
* =======
*
* DLASR applies a sequence of plane rotations to a real matrix A,
* from either the left or the right.
*
* When SIDE = 'L', the transformation takes the form
*
* A := P*A
*
* and when SIDE = 'R', the transformation takes the form
*
* A := A*P**T
*
* where P is an orthogonal matrix consisting of a sequence of z plane
* rotations, with z = M when SIDE = 'L' and z = N when SIDE = 'R',
* and P**T is the transpose of P.
*
* When DIRECT = 'F' (Forward sequence), then
*
* P = P(z-1) * ... * P(2) * P(1)
*
* and when DIRECT = 'B' (Backward sequence), then
*
* P = P(1) * P(2) * ... * P(z-1)
*
* where P(k) is a plane rotation matrix defined by the 2-by-2 rotation
*
* R(k) = ( c(k) s(k) )
* = ( -s(k) c(k) ).
*
* When PIVOT = 'V' (Variable pivot), the rotation is performed
* for the plane (k,k+1), i.e., P(k) has the form
*
* P(k) = ( 1 )
* ( ... )
* ( 1 )
* ( c(k) s(k) )
* ( -s(k) c(k) )
* ( 1 )
* ( ... )
* ( 1 )
*
* where R(k) appears as a rank-2 modification to the identity matrix in
* rows and columns k and k+1.
*
* When PIVOT = 'T' (Top pivot), the rotation is performed for the
* plane (1,k+1), so P(k) has the form
*
* P(k) = ( c(k) s(k) )
* ( 1 )
* ( ... )
* ( 1 )
* ( -s(k) c(k) )
* ( 1 )
* ( ... )
* ( 1 )
*
* where R(k) appears in rows and columns 1 and k+1.
*
* Similarly, when PIVOT = 'B' (Bottom pivot), the rotation is
* performed for the plane (k,z), giving P(k) the form
*
* P(k) = ( 1 )
* ( ... )
* ( 1 )
* ( c(k) s(k) )
* ( 1 )
* ( ... )
* ( 1 )
* ( -s(k) c(k) )
*
* where R(k) appears in rows and columns k and z. The rotations are
* performed without ever forming P(k) explicitly.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* Specifies whether the plane rotation matrix P is applied to
* A on the left or the right.
* = 'L': Left, compute A := P*A
* = 'R': Right, compute A:= A*P**T
*
* PIVOT (input) CHARACTER*1
* Specifies the plane for which P(k) is a plane rotation
* matrix.
* = 'V': Variable pivot, the plane (k,k+1)
* = 'T': Top pivot, the plane (1,k+1)
* = 'B': Bottom pivot, the plane (k,z)
*
* DIRECT (input) CHARACTER*1
* Specifies whether P is a forward or backward sequence of
* plane rotations.
* = 'F': Forward, P = P(z-1)*...*P(2)*P(1)
* = 'B': Backward, P = P(1)*P(2)*...*P(z-1)
*
* M (input) INTEGER
* The number of rows of the matrix A. If m <= 1, an immediate
* return is effected.
*
* N (input) INTEGER
* The number of columns of the matrix A. If n <= 1, an
* immediate return is effected.
*
* C (input) DOUBLE PRECISION array, dimension
* (M-1) if SIDE = 'L'
* (N-1) if SIDE = 'R'
* The cosines c(k) of the plane rotations.
*
* S (input) DOUBLE PRECISION array, dimension
* (M-1) if SIDE = 'L'
* (N-1) if SIDE = 'R'
* The sines s(k) of the plane rotations. The 2-by-2 plane
* rotation part of the matrix P(k), R(k), has the form
* R(k) = ( c(k) s(k) )
* ( -s(k) c(k) ).
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* The M-by-N matrix A. On exit, A is overwritten by P*A if
* SIDE = 'R' or by A*P**T if SIDE = 'L'.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param pivot
* @param direct
* @param m
* @param n
* @param c
* @param _c_offset
* @param s
* @param _s_offset
* @param a
* @param _a_offset
* @param lda
*
*/
abstract public void dlasr(java.lang.String side, java.lang.String pivot, java.lang.String direct, int m, int n, double[] c, int _c_offset, double[] s, int _s_offset, double[] a, int _a_offset, int lda);
/**
*
* ..
*
* Purpose
* =======
*
* Sort the numbers in D in increasing order (if ID = 'I') or
* in decreasing order (if ID = 'D' ).
*
* Use Quick Sort, reverting to Insertion sort on arrays of
* size <= 20. Dimension of STACK limits N to about 2**32.
*
* Arguments
* =========
*
* ID (input) CHARACTER*1
* = 'I': sort D in increasing order;
* = 'D': sort D in decreasing order.
*
* N (input) INTEGER
* The length of the array D.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the array to be sorted.
* On exit, D has been sorted into increasing order
* (D(1) <= ... <= D(N) ) or into decreasing order
* (D(1) >= ... >= D(N) ), depending on ID.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param id
* @param n
* @param d
* @param info
*
*/
abstract public void dlasrt(java.lang.String id, int n, double[] d, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* Sort the numbers in D in increasing order (if ID = 'I') or
* in decreasing order (if ID = 'D' ).
*
* Use Quick Sort, reverting to Insertion sort on arrays of
* size <= 20. Dimension of STACK limits N to about 2**32.
*
* Arguments
* =========
*
* ID (input) CHARACTER*1
* = 'I': sort D in increasing order;
* = 'D': sort D in decreasing order.
*
* N (input) INTEGER
* The length of the array D.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the array to be sorted.
* On exit, D has been sorted into increasing order
* (D(1) <= ... <= D(N) ) or into decreasing order
* (D(1) >= ... >= D(N) ), depending on ID.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param id
* @param n
* @param d
* @param _d_offset
* @param info
*
*/
abstract public void dlasrt(java.lang.String id, int n, double[] d, int _d_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLASSQ returns the values scl and smsq such that
*
* ( scl**2 )*smsq = x( 1 )**2 +...+ x( n )**2 + ( scale**2 )*sumsq,
*
* where x( i ) = X( 1 + ( i - 1 )*INCX ). The value of sumsq is
* assumed to be non-negative and scl returns the value
*
* scl = max( scale, abs( x( i ) ) ).
*
* scale and sumsq must be supplied in SCALE and SUMSQ and
* scl and smsq are overwritten on SCALE and SUMSQ respectively.
*
* The routine makes only one pass through the vector x.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of elements to be used from the vector X.
*
* X (input) DOUBLE PRECISION array, dimension (N)
* The vector for which a scaled sum of squares is computed.
* x( i ) = X( 1 + ( i - 1 )*INCX ), 1 <= i <= n.
*
* INCX (input) INTEGER
* The increment between successive values of the vector X.
* INCX > 0.
*
* SCALE (input/output) DOUBLE PRECISION
* On entry, the value scale in the equation above.
* On exit, SCALE is overwritten with scl , the scaling factor
* for the sum of squares.
*
* SUMSQ (input/output) DOUBLE PRECISION
* On entry, the value sumsq in the equation above.
* On exit, SUMSQ is overwritten with smsq , the basic sum of
* squares from which scl has been factored out.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param x
* @param incx
* @param scale
* @param sumsq
*
*/
abstract public void dlassq(int n, double[] x, int incx, org.netlib.util.doubleW scale, org.netlib.util.doubleW sumsq);
/**
*
* ..
*
* Purpose
* =======
*
* DLASSQ returns the values scl and smsq such that
*
* ( scl**2 )*smsq = x( 1 )**2 +...+ x( n )**2 + ( scale**2 )*sumsq,
*
* where x( i ) = X( 1 + ( i - 1 )*INCX ). The value of sumsq is
* assumed to be non-negative and scl returns the value
*
* scl = max( scale, abs( x( i ) ) ).
*
* scale and sumsq must be supplied in SCALE and SUMSQ and
* scl and smsq are overwritten on SCALE and SUMSQ respectively.
*
* The routine makes only one pass through the vector x.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of elements to be used from the vector X.
*
* X (input) DOUBLE PRECISION array, dimension (N)
* The vector for which a scaled sum of squares is computed.
* x( i ) = X( 1 + ( i - 1 )*INCX ), 1 <= i <= n.
*
* INCX (input) INTEGER
* The increment between successive values of the vector X.
* INCX > 0.
*
* SCALE (input/output) DOUBLE PRECISION
* On entry, the value scale in the equation above.
* On exit, SCALE is overwritten with scl , the scaling factor
* for the sum of squares.
*
* SUMSQ (input/output) DOUBLE PRECISION
* On entry, the value sumsq in the equation above.
* On exit, SUMSQ is overwritten with smsq , the basic sum of
* squares from which scl has been factored out.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param x
* @param _x_offset
* @param incx
* @param scale
* @param sumsq
*
*/
abstract public void dlassq(int n, double[] x, int _x_offset, int incx, org.netlib.util.doubleW scale, org.netlib.util.doubleW sumsq);
/**
*
* ..
*
* Purpose
* =======
*
* DLASV2 computes the singular value decomposition of a 2-by-2
* triangular matrix
* [ F G ]
* [ 0 H ].
* On return, abs(SSMAX) is the larger singular value, abs(SSMIN) is the
* smaller singular value, and (CSL,SNL) and (CSR,SNR) are the left and
* right singular vectors for abs(SSMAX), giving the decomposition
*
* [ CSL SNL ] [ F G ] [ CSR -SNR ] = [ SSMAX 0 ]
* [-SNL CSL ] [ 0 H ] [ SNR CSR ] [ 0 SSMIN ].
*
* Arguments
* =========
*
* F (input) DOUBLE PRECISION
* The (1,1) element of the 2-by-2 matrix.
*
* G (input) DOUBLE PRECISION
* The (1,2) element of the 2-by-2 matrix.
*
* H (input) DOUBLE PRECISION
* The (2,2) element of the 2-by-2 matrix.
*
* SSMIN (output) DOUBLE PRECISION
* abs(SSMIN) is the smaller singular value.
*
* SSMAX (output) DOUBLE PRECISION
* abs(SSMAX) is the larger singular value.
*
* SNL (output) DOUBLE PRECISION
* CSL (output) DOUBLE PRECISION
* The vector (CSL, SNL) is a unit left singular vector for the
* singular value abs(SSMAX).
*
* SNR (output) DOUBLE PRECISION
* CSR (output) DOUBLE PRECISION
* The vector (CSR, SNR) is a unit right singular vector for the
* singular value abs(SSMAX).
*
* Further Details
* ===============
*
* Any input parameter may be aliased with any output parameter.
*
* Barring over/underflow and assuming a guard digit in subtraction, all
* output quantities are correct to within a few units in the last
* place (ulps).
*
* In IEEE arithmetic, the code works correctly if one matrix element is
* infinite.
*
* Overflow will not occur unless the largest singular value itself
* overflows or is within a few ulps of overflow. (On machines with
* partial overflow, like the Cray, overflow may occur if the largest
* singular value is within a factor of 2 of overflow.)
*
* Underflow is harmless if underflow is gradual. Otherwise, results
* may correspond to a matrix modified by perturbations of size near
* the underflow threshold.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param f
* @param g
* @param h
* @param ssmin
* @param ssmax
* @param snr
* @param csr
* @param snl
* @param csl
*
*/
abstract public void dlasv2(double f, double g, double h, org.netlib.util.doubleW ssmin, org.netlib.util.doubleW ssmax, org.netlib.util.doubleW snr, org.netlib.util.doubleW csr, org.netlib.util.doubleW snl, org.netlib.util.doubleW csl);
/**
*
* ..
*
* Purpose
* =======
*
* DLASWP performs a series of row interchanges on the matrix A.
* One row interchange is initiated for each of rows K1 through K2 of A.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of columns of the matrix A.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the matrix of column dimension N to which the row
* interchanges will be applied.
* On exit, the permuted matrix.
*
* LDA (input) INTEGER
* The leading dimension of the array A.
*
* K1 (input) INTEGER
* The first element of IPIV for which a row interchange will
* be done.
*
* K2 (input) INTEGER
* The last element of IPIV for which a row interchange will
* be done.
*
* IPIV (input) INTEGER array, dimension (K2*abs(INCX))
* The vector of pivot indices. Only the elements in positions
* K1 through K2 of IPIV are accessed.
* IPIV(K) = L implies rows K and L are to be interchanged.
*
* INCX (input) INTEGER
* The increment between successive values of IPIV. If IPIV
* is negative, the pivots are applied in reverse order.
*
* Further Details
* ===============
*
* Modified by
* R. C. Whaley, Computer Science Dept., Univ. of Tenn., Knoxville, USA
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n
* @param a
* @param lda
* @param k1
* @param k2
* @param ipiv
* @param incx
*
*/
abstract public void dlaswp(int n, double[] a, int lda, int k1, int k2, int[] ipiv, int incx);
/**
*
* ..
*
* Purpose
* =======
*
* DLASWP performs a series of row interchanges on the matrix A.
* One row interchange is initiated for each of rows K1 through K2 of A.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of columns of the matrix A.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the matrix of column dimension N to which the row
* interchanges will be applied.
* On exit, the permuted matrix.
*
* LDA (input) INTEGER
* The leading dimension of the array A.
*
* K1 (input) INTEGER
* The first element of IPIV for which a row interchange will
* be done.
*
* K2 (input) INTEGER
* The last element of IPIV for which a row interchange will
* be done.
*
* IPIV (input) INTEGER array, dimension (K2*abs(INCX))
* The vector of pivot indices. Only the elements in positions
* K1 through K2 of IPIV are accessed.
* IPIV(K) = L implies rows K and L are to be interchanged.
*
* INCX (input) INTEGER
* The increment between successive values of IPIV. If IPIV
* is negative, the pivots are applied in reverse order.
*
* Further Details
* ===============
*
* Modified by
* R. C. Whaley, Computer Science Dept., Univ. of Tenn., Knoxville, USA
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n
* @param a
* @param _a_offset
* @param lda
* @param k1
* @param k2
* @param ipiv
* @param _ipiv_offset
* @param incx
*
*/
abstract public void dlaswp(int n, double[] a, int _a_offset, int lda, int k1, int k2, int[] ipiv, int _ipiv_offset, int incx);
/**
*
* ..
*
* Purpose
* =======
*
* DLASY2 solves for the N1 by N2 matrix X, 1 <= N1,N2 <= 2, in
*
* op(TL)*X + ISGN*X*op(TR) = SCALE*B,
*
* where TL is N1 by N1, TR is N2 by N2, B is N1 by N2, and ISGN = 1 or
* -1. op(T) = T or T', where T' denotes the transpose of T.
*
* Arguments
* =========
*
* LTRANL (input) LOGICAL
* On entry, LTRANL specifies the op(TL):
* = .FALSE., op(TL) = TL,
* = .TRUE., op(TL) = TL'.
*
* LTRANR (input) LOGICAL
* On entry, LTRANR specifies the op(TR):
* = .FALSE., op(TR) = TR,
* = .TRUE., op(TR) = TR'.
*
* ISGN (input) INTEGER
* On entry, ISGN specifies the sign of the equation
* as described before. ISGN may only be 1 or -1.
*
* N1 (input) INTEGER
* On entry, N1 specifies the order of matrix TL.
* N1 may only be 0, 1 or 2.
*
* N2 (input) INTEGER
* On entry, N2 specifies the order of matrix TR.
* N2 may only be 0, 1 or 2.
*
* TL (input) DOUBLE PRECISION array, dimension (LDTL,2)
* On entry, TL contains an N1 by N1 matrix.
*
* LDTL (input) INTEGER
* The leading dimension of the matrix TL. LDTL >= max(1,N1).
*
* TR (input) DOUBLE PRECISION array, dimension (LDTR,2)
* On entry, TR contains an N2 by N2 matrix.
*
* LDTR (input) INTEGER
* The leading dimension of the matrix TR. LDTR >= max(1,N2).
*
* B (input) DOUBLE PRECISION array, dimension (LDB,2)
* On entry, the N1 by N2 matrix B contains the right-hand
* side of the equation.
*
* LDB (input) INTEGER
* The leading dimension of the matrix B. LDB >= max(1,N1).
*
* SCALE (output) DOUBLE PRECISION
* On exit, SCALE contains the scale factor. SCALE is chosen
* less than or equal to 1 to prevent the solution overflowing.
*
* X (output) DOUBLE PRECISION array, dimension (LDX,2)
* On exit, X contains the N1 by N2 solution.
*
* LDX (input) INTEGER
* The leading dimension of the matrix X. LDX >= max(1,N1).
*
* XNORM (output) DOUBLE PRECISION
* On exit, XNORM is the infinity-norm of the solution.
*
* INFO (output) INTEGER
* On exit, INFO is set to
* 0: successful exit.
* 1: TL and TR have too close eigenvalues, so TL or
* TR is perturbed to get a nonsingular equation.
* NOTE: In the interests of speed, this routine does not
* check the inputs for errors.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param ltranl
* @param ltranr
* @param isgn
* @param n1
* @param n2
* @param tl
* @param ldtl
* @param tr
* @param ldtr
* @param b
* @param ldb
* @param scale
* @param x
* @param ldx
* @param xnorm
* @param info
*
*/
abstract public void dlasy2(boolean ltranl, boolean ltranr, int isgn, int n1, int n2, double[] tl, int ldtl, double[] tr, int ldtr, double[] b, int ldb, org.netlib.util.doubleW scale, double[] x, int ldx, org.netlib.util.doubleW xnorm, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLASY2 solves for the N1 by N2 matrix X, 1 <= N1,N2 <= 2, in
*
* op(TL)*X + ISGN*X*op(TR) = SCALE*B,
*
* where TL is N1 by N1, TR is N2 by N2, B is N1 by N2, and ISGN = 1 or
* -1. op(T) = T or T', where T' denotes the transpose of T.
*
* Arguments
* =========
*
* LTRANL (input) LOGICAL
* On entry, LTRANL specifies the op(TL):
* = .FALSE., op(TL) = TL,
* = .TRUE., op(TL) = TL'.
*
* LTRANR (input) LOGICAL
* On entry, LTRANR specifies the op(TR):
* = .FALSE., op(TR) = TR,
* = .TRUE., op(TR) = TR'.
*
* ISGN (input) INTEGER
* On entry, ISGN specifies the sign of the equation
* as described before. ISGN may only be 1 or -1.
*
* N1 (input) INTEGER
* On entry, N1 specifies the order of matrix TL.
* N1 may only be 0, 1 or 2.
*
* N2 (input) INTEGER
* On entry, N2 specifies the order of matrix TR.
* N2 may only be 0, 1 or 2.
*
* TL (input) DOUBLE PRECISION array, dimension (LDTL,2)
* On entry, TL contains an N1 by N1 matrix.
*
* LDTL (input) INTEGER
* The leading dimension of the matrix TL. LDTL >= max(1,N1).
*
* TR (input) DOUBLE PRECISION array, dimension (LDTR,2)
* On entry, TR contains an N2 by N2 matrix.
*
* LDTR (input) INTEGER
* The leading dimension of the matrix TR. LDTR >= max(1,N2).
*
* B (input) DOUBLE PRECISION array, dimension (LDB,2)
* On entry, the N1 by N2 matrix B contains the right-hand
* side of the equation.
*
* LDB (input) INTEGER
* The leading dimension of the matrix B. LDB >= max(1,N1).
*
* SCALE (output) DOUBLE PRECISION
* On exit, SCALE contains the scale factor. SCALE is chosen
* less than or equal to 1 to prevent the solution overflowing.
*
* X (output) DOUBLE PRECISION array, dimension (LDX,2)
* On exit, X contains the N1 by N2 solution.
*
* LDX (input) INTEGER
* The leading dimension of the matrix X. LDX >= max(1,N1).
*
* XNORM (output) DOUBLE PRECISION
* On exit, XNORM is the infinity-norm of the solution.
*
* INFO (output) INTEGER
* On exit, INFO is set to
* 0: successful exit.
* 1: TL and TR have too close eigenvalues, so TL or
* TR is perturbed to get a nonsingular equation.
* NOTE: In the interests of speed, this routine does not
* check the inputs for errors.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param ltranl
* @param ltranr
* @param isgn
* @param n1
* @param n2
* @param tl
* @param _tl_offset
* @param ldtl
* @param tr
* @param _tr_offset
* @param ldtr
* @param b
* @param _b_offset
* @param ldb
* @param scale
* @param x
* @param _x_offset
* @param ldx
* @param xnorm
* @param info
*
*/
abstract public void dlasy2(boolean ltranl, boolean ltranr, int isgn, int n1, int n2, double[] tl, int _tl_offset, int ldtl, double[] tr, int _tr_offset, int ldtr, double[] b, int _b_offset, int ldb, org.netlib.util.doubleW scale, double[] x, int _x_offset, int ldx, org.netlib.util.doubleW xnorm, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLASYF computes a partial factorization of a real symmetric matrix A
* using the Bunch-Kaufman diagonal pivoting method. The partial
* factorization has the form:
*
* A = ( I U12 ) ( A11 0 ) ( I 0 ) if UPLO = 'U', or:
* ( 0 U22 ) ( 0 D ) ( U12' U22' )
*
* A = ( L11 0 ) ( D 0 ) ( L11' L21' ) if UPLO = 'L'
* ( L21 I ) ( 0 A22 ) ( 0 I )
*
* where the order of D is at most NB. The actual order is returned in
* the argument KB, and is either NB or NB-1, or N if N <= NB.
*
* DLASYF is an auxiliary routine called by DSYTRF. It uses blocked code
* (calling Level 3 BLAS) to update the submatrix A11 (if UPLO = 'U') or
* A22 (if UPLO = 'L').
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored:
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NB (input) INTEGER
* The maximum number of columns of the matrix A that should be
* factored. NB should be at least 2 to allow for 2-by-2 pivot
* blocks.
*
* KB (output) INTEGER
* The number of columns of A that were actually factored.
* KB is either NB-1 or NB, or N if N <= NB.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* n-by-n upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading n-by-n lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
* On exit, A contains details of the partial factorization.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (output) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D.
* If UPLO = 'U', only the last KB elements of IPIV are set;
* if UPLO = 'L', only the first KB elements are set.
*
* If IPIV(k) > 0, then rows and columns k and IPIV(k) were
* interchanged and D(k,k) is a 1-by-1 diagonal block.
* If UPLO = 'U' and IPIV(k) = IPIV(k-1) < 0, then rows and
* columns k-1 and -IPIV(k) were interchanged and D(k-1:k,k-1:k)
* is a 2-by-2 diagonal block. If UPLO = 'L' and IPIV(k) =
* IPIV(k+1) < 0, then rows and columns k+1 and -IPIV(k) were
* interchanged and D(k:k+1,k:k+1) is a 2-by-2 diagonal block.
*
* W (workspace) DOUBLE PRECISION array, dimension (LDW,NB)
*
* LDW (input) INTEGER
* The leading dimension of the array W. LDW >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* > 0: if INFO = k, D(k,k) is exactly zero. The factorization
* has been completed, but the block diagonal matrix D is
* exactly singular.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nb
* @param kb
* @param a
* @param lda
* @param ipiv
* @param w
* @param ldw
* @param info
*
*/
abstract public void dlasyf(java.lang.String uplo, int n, int nb, org.netlib.util.intW kb, double[] a, int lda, int[] ipiv, double[] w, int ldw, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLASYF computes a partial factorization of a real symmetric matrix A
* using the Bunch-Kaufman diagonal pivoting method. The partial
* factorization has the form:
*
* A = ( I U12 ) ( A11 0 ) ( I 0 ) if UPLO = 'U', or:
* ( 0 U22 ) ( 0 D ) ( U12' U22' )
*
* A = ( L11 0 ) ( D 0 ) ( L11' L21' ) if UPLO = 'L'
* ( L21 I ) ( 0 A22 ) ( 0 I )
*
* where the order of D is at most NB. The actual order is returned in
* the argument KB, and is either NB or NB-1, or N if N <= NB.
*
* DLASYF is an auxiliary routine called by DSYTRF. It uses blocked code
* (calling Level 3 BLAS) to update the submatrix A11 (if UPLO = 'U') or
* A22 (if UPLO = 'L').
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored:
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NB (input) INTEGER
* The maximum number of columns of the matrix A that should be
* factored. NB should be at least 2 to allow for 2-by-2 pivot
* blocks.
*
* KB (output) INTEGER
* The number of columns of A that were actually factored.
* KB is either NB-1 or NB, or N if N <= NB.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* n-by-n upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading n-by-n lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
* On exit, A contains details of the partial factorization.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (output) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D.
* If UPLO = 'U', only the last KB elements of IPIV are set;
* if UPLO = 'L', only the first KB elements are set.
*
* If IPIV(k) > 0, then rows and columns k and IPIV(k) were
* interchanged and D(k,k) is a 1-by-1 diagonal block.
* If UPLO = 'U' and IPIV(k) = IPIV(k-1) < 0, then rows and
* columns k-1 and -IPIV(k) were interchanged and D(k-1:k,k-1:k)
* is a 2-by-2 diagonal block. If UPLO = 'L' and IPIV(k) =
* IPIV(k+1) < 0, then rows and columns k+1 and -IPIV(k) were
* interchanged and D(k:k+1,k:k+1) is a 2-by-2 diagonal block.
*
* W (workspace) DOUBLE PRECISION array, dimension (LDW,NB)
*
* LDW (input) INTEGER
* The leading dimension of the array W. LDW >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* > 0: if INFO = k, D(k,k) is exactly zero. The factorization
* has been completed, but the block diagonal matrix D is
* exactly singular.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nb
* @param kb
* @param a
* @param _a_offset
* @param lda
* @param ipiv
* @param _ipiv_offset
* @param w
* @param _w_offset
* @param ldw
* @param info
*
*/
abstract public void dlasyf(java.lang.String uplo, int n, int nb, org.netlib.util.intW kb, double[] a, int _a_offset, int lda, int[] ipiv, int _ipiv_offset, double[] w, int _w_offset, int ldw, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLATBS solves one of the triangular systems
*
* A *x = s*b or A'*x = s*b
*
* with scaling to prevent overflow, where A is an upper or lower
* triangular band matrix. Here A' denotes the transpose of A, x and b
* are n-element vectors, and s is a scaling factor, usually less than
* or equal to 1, chosen so that the components of x will be less than
* the overflow threshold. If the unscaled problem will not cause
* overflow, the Level 2 BLAS routine DTBSV is called. If the matrix A
* is singular (A(j,j) = 0 for some j), then s is set to 0 and a
* non-trivial solution to A*x = 0 is returned.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the matrix A is upper or lower triangular.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* TRANS (input) CHARACTER*1
* Specifies the operation applied to A.
* = 'N': Solve A * x = s*b (No transpose)
* = 'T': Solve A'* x = s*b (Transpose)
* = 'C': Solve A'* x = s*b (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* Specifies whether or not the matrix A is unit triangular.
* = 'N': Non-unit triangular
* = 'U': Unit triangular
*
* NORMIN (input) CHARACTER*1
* Specifies whether CNORM has been set or not.
* = 'Y': CNORM contains the column norms on entry
* = 'N': CNORM is not set on entry. On exit, the norms will
* be computed and stored in CNORM.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of subdiagonals or superdiagonals in the
* triangular matrix A. KD >= 0.
*
* AB (input) DOUBLE PRECISION array, dimension (LDAB,N)
* The upper or lower triangular band matrix A, stored in the
* first KD+1 rows of the array. The j-th column of A is stored
* in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* X (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the right hand side b of the triangular system.
* On exit, X is overwritten by the solution vector x.
*
* SCALE (output) DOUBLE PRECISION
* The scaling factor s for the triangular system
* A * x = s*b or A'* x = s*b.
* If SCALE = 0, the matrix A is singular or badly scaled, and
* the vector x is an exact or approximate solution to A*x = 0.
*
* CNORM (input or output) DOUBLE PRECISION array, dimension (N)
*
* If NORMIN = 'Y', CNORM is an input argument and CNORM(j)
* contains the norm of the off-diagonal part of the j-th column
* of A. If TRANS = 'N', CNORM(j) must be greater than or equal
* to the infinity-norm, and if TRANS = 'T' or 'C', CNORM(j)
* must be greater than or equal to the 1-norm.
*
* If NORMIN = 'N', CNORM is an output argument and CNORM(j)
* returns the 1-norm of the offdiagonal part of the j-th column
* of A.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
*
* Further Details
* ======= =======
*
* A rough bound on x is computed; if that is less than overflow, DTBSV
* is called, otherwise, specific code is used which checks for possible
* overflow or divide-by-zero at every operation.
*
* A columnwise scheme is used for solving A*x = b. The basic algorithm
* if A is lower triangular is
*
* x[1:n] := b[1:n]
* for j = 1, ..., n
* x(j) := x(j) / A(j,j)
* x[j+1:n] := x[j+1:n] - x(j) * A[j+1:n,j]
* end
*
* Define bounds on the components of x after j iterations of the loop:
* M(j) = bound on x[1:j]
* G(j) = bound on x[j+1:n]
* Initially, let M(0) = 0 and G(0) = max{x(i), i=1,...,n}.
*
* Then for iteration j+1 we have
* M(j+1) <= G(j) / | A(j+1,j+1) |
* G(j+1) <= G(j) + M(j+1) * | A[j+2:n,j+1] |
* <= G(j) ( 1 + CNORM(j+1) / | A(j+1,j+1) | )
*
* where CNORM(j+1) is greater than or equal to the infinity-norm of
* column j+1 of A, not counting the diagonal. Hence
*
* G(j) <= G(0) product ( 1 + CNORM(i) / | A(i,i) | )
* 1<=i<=j
* and
*
* |x(j)| <= ( G(0) / |A(j,j)| ) product ( 1 + CNORM(i) / |A(i,i)| )
* 1<=i< j
*
* Since |x(j)| <= M(j), we use the Level 2 BLAS routine DTBSV if the
* reciprocal of the largest M(j), j=1,..,n, is larger than
* max(underflow, 1/overflow).
*
* The bound on x(j) is also used to determine when a step in the
* columnwise method can be performed without fear of overflow. If
* the computed bound is greater than a large constant, x is scaled to
* prevent overflow, but if the bound overflows, x is set to 0, x(j) to
* 1, and scale to 0, and a non-trivial solution to A*x = 0 is found.
*
* Similarly, a row-wise scheme is used to solve A'*x = b. The basic
* algorithm for A upper triangular is
*
* for j = 1, ..., n
* x(j) := ( b(j) - A[1:j-1,j]' * x[1:j-1] ) / A(j,j)
* end
*
* We simultaneously compute two bounds
* G(j) = bound on ( b(i) - A[1:i-1,i]' * x[1:i-1] ), 1<=i<=j
* M(j) = bound on x(i), 1<=i<=j
*
* The initial values are G(0) = 0, M(0) = max{b(i), i=1,..,n}, and we
* add the constraint G(j) >= G(j-1) and M(j) >= M(j-1) for j >= 1.
* Then the bound on x(j) is
*
* M(j) <= M(j-1) * ( 1 + CNORM(j) ) / | A(j,j) |
*
* <= M(0) * product ( ( 1 + CNORM(i) ) / |A(i,i)| )
* 1<=i<=j
*
* and we can safely call DTBSV if 1/M(n) and 1/G(n) are both greater
* than max(underflow, 1/overflow).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param normin
* @param n
* @param kd
* @param ab
* @param ldab
* @param x
* @param scale
* @param cnorm
* @param info
*
*/
abstract public void dlatbs(java.lang.String uplo, java.lang.String trans, java.lang.String diag, java.lang.String normin, int n, int kd, double[] ab, int ldab, double[] x, org.netlib.util.doubleW scale, double[] cnorm, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLATBS solves one of the triangular systems
*
* A *x = s*b or A'*x = s*b
*
* with scaling to prevent overflow, where A is an upper or lower
* triangular band matrix. Here A' denotes the transpose of A, x and b
* are n-element vectors, and s is a scaling factor, usually less than
* or equal to 1, chosen so that the components of x will be less than
* the overflow threshold. If the unscaled problem will not cause
* overflow, the Level 2 BLAS routine DTBSV is called. If the matrix A
* is singular (A(j,j) = 0 for some j), then s is set to 0 and a
* non-trivial solution to A*x = 0 is returned.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the matrix A is upper or lower triangular.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* TRANS (input) CHARACTER*1
* Specifies the operation applied to A.
* = 'N': Solve A * x = s*b (No transpose)
* = 'T': Solve A'* x = s*b (Transpose)
* = 'C': Solve A'* x = s*b (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* Specifies whether or not the matrix A is unit triangular.
* = 'N': Non-unit triangular
* = 'U': Unit triangular
*
* NORMIN (input) CHARACTER*1
* Specifies whether CNORM has been set or not.
* = 'Y': CNORM contains the column norms on entry
* = 'N': CNORM is not set on entry. On exit, the norms will
* be computed and stored in CNORM.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of subdiagonals or superdiagonals in the
* triangular matrix A. KD >= 0.
*
* AB (input) DOUBLE PRECISION array, dimension (LDAB,N)
* The upper or lower triangular band matrix A, stored in the
* first KD+1 rows of the array. The j-th column of A is stored
* in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* X (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the right hand side b of the triangular system.
* On exit, X is overwritten by the solution vector x.
*
* SCALE (output) DOUBLE PRECISION
* The scaling factor s for the triangular system
* A * x = s*b or A'* x = s*b.
* If SCALE = 0, the matrix A is singular or badly scaled, and
* the vector x is an exact or approximate solution to A*x = 0.
*
* CNORM (input or output) DOUBLE PRECISION array, dimension (N)
*
* If NORMIN = 'Y', CNORM is an input argument and CNORM(j)
* contains the norm of the off-diagonal part of the j-th column
* of A. If TRANS = 'N', CNORM(j) must be greater than or equal
* to the infinity-norm, and if TRANS = 'T' or 'C', CNORM(j)
* must be greater than or equal to the 1-norm.
*
* If NORMIN = 'N', CNORM is an output argument and CNORM(j)
* returns the 1-norm of the offdiagonal part of the j-th column
* of A.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
*
* Further Details
* ======= =======
*
* A rough bound on x is computed; if that is less than overflow, DTBSV
* is called, otherwise, specific code is used which checks for possible
* overflow or divide-by-zero at every operation.
*
* A columnwise scheme is used for solving A*x = b. The basic algorithm
* if A is lower triangular is
*
* x[1:n] := b[1:n]
* for j = 1, ..., n
* x(j) := x(j) / A(j,j)
* x[j+1:n] := x[j+1:n] - x(j) * A[j+1:n,j]
* end
*
* Define bounds on the components of x after j iterations of the loop:
* M(j) = bound on x[1:j]
* G(j) = bound on x[j+1:n]
* Initially, let M(0) = 0 and G(0) = max{x(i), i=1,...,n}.
*
* Then for iteration j+1 we have
* M(j+1) <= G(j) / | A(j+1,j+1) |
* G(j+1) <= G(j) + M(j+1) * | A[j+2:n,j+1] |
* <= G(j) ( 1 + CNORM(j+1) / | A(j+1,j+1) | )
*
* where CNORM(j+1) is greater than or equal to the infinity-norm of
* column j+1 of A, not counting the diagonal. Hence
*
* G(j) <= G(0) product ( 1 + CNORM(i) / | A(i,i) | )
* 1<=i<=j
* and
*
* |x(j)| <= ( G(0) / |A(j,j)| ) product ( 1 + CNORM(i) / |A(i,i)| )
* 1<=i< j
*
* Since |x(j)| <= M(j), we use the Level 2 BLAS routine DTBSV if the
* reciprocal of the largest M(j), j=1,..,n, is larger than
* max(underflow, 1/overflow).
*
* The bound on x(j) is also used to determine when a step in the
* columnwise method can be performed without fear of overflow. If
* the computed bound is greater than a large constant, x is scaled to
* prevent overflow, but if the bound overflows, x is set to 0, x(j) to
* 1, and scale to 0, and a non-trivial solution to A*x = 0 is found.
*
* Similarly, a row-wise scheme is used to solve A'*x = b. The basic
* algorithm for A upper triangular is
*
* for j = 1, ..., n
* x(j) := ( b(j) - A[1:j-1,j]' * x[1:j-1] ) / A(j,j)
* end
*
* We simultaneously compute two bounds
* G(j) = bound on ( b(i) - A[1:i-1,i]' * x[1:i-1] ), 1<=i<=j
* M(j) = bound on x(i), 1<=i<=j
*
* The initial values are G(0) = 0, M(0) = max{b(i), i=1,..,n}, and we
* add the constraint G(j) >= G(j-1) and M(j) >= M(j-1) for j >= 1.
* Then the bound on x(j) is
*
* M(j) <= M(j-1) * ( 1 + CNORM(j) ) / | A(j,j) |
*
* <= M(0) * product ( ( 1 + CNORM(i) ) / |A(i,i)| )
* 1<=i<=j
*
* and we can safely call DTBSV if 1/M(n) and 1/G(n) are both greater
* than max(underflow, 1/overflow).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param normin
* @param n
* @param kd
* @param ab
* @param _ab_offset
* @param ldab
* @param x
* @param _x_offset
* @param scale
* @param cnorm
* @param _cnorm_offset
* @param info
*
*/
abstract public void dlatbs(java.lang.String uplo, java.lang.String trans, java.lang.String diag, java.lang.String normin, int n, int kd, double[] ab, int _ab_offset, int ldab, double[] x, int _x_offset, org.netlib.util.doubleW scale, double[] cnorm, int _cnorm_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLATDF uses the LU factorization of the n-by-n matrix Z computed by
* DGETC2 and computes a contribution to the reciprocal Dif-estimate
* by solving Z * x = b for x, and choosing the r.h.s. b such that
* the norm of x is as large as possible. On entry RHS = b holds the
* contribution from earlier solved sub-systems, and on return RHS = x.
*
* The factorization of Z returned by DGETC2 has the form Z = P*L*U*Q,
* where P and Q are permutation matrices. L is lower triangular with
* unit diagonal elements and U is upper triangular.
*
* Arguments
* =========
*
* IJOB (input) INTEGER
* IJOB = 2: First compute an approximative null-vector e
* of Z using DGECON, e is normalized and solve for
* Zx = +-e - f with the sign giving the greater value
* of 2-norm(x). About 5 times as expensive as Default.
* IJOB .ne. 2: Local look ahead strategy where all entries of
* the r.h.s. b is choosen as either +1 or -1 (Default).
*
* N (input) INTEGER
* The number of columns of the matrix Z.
*
* Z (input) DOUBLE PRECISION array, dimension (LDZ, N)
* On entry, the LU part of the factorization of the n-by-n
* matrix Z computed by DGETC2: Z = P * L * U * Q
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDA >= max(1, N).
*
* RHS (input/output) DOUBLE PRECISION array, dimension N.
* On entry, RHS contains contributions from other subsystems.
* On exit, RHS contains the solution of the subsystem with
* entries acoording to the value of IJOB (see above).
*
* RDSUM (input/output) DOUBLE PRECISION
* On entry, the sum of squares of computed contributions to
* the Dif-estimate under computation by DTGSYL, where the
* scaling factor RDSCAL (see below) has been factored out.
* On exit, the corresponding sum of squares updated with the
* contributions from the current sub-system.
* If TRANS = 'T' RDSUM is not touched.
* NOTE: RDSUM only makes sense when DTGSY2 is called by STGSYL.
*
* RDSCAL (input/output) DOUBLE PRECISION
* On entry, scaling factor used to prevent overflow in RDSUM.
* On exit, RDSCAL is updated w.r.t. the current contributions
* in RDSUM.
* If TRANS = 'T', RDSCAL is not touched.
* NOTE: RDSCAL only makes sense when DTGSY2 is called by
* DTGSYL.
*
* IPIV (input) INTEGER array, dimension (N).
* The pivot indices; for 1 <= i <= N, row i of the
* matrix has been interchanged with row IPIV(i).
*
* JPIV (input) INTEGER array, dimension (N).
* The pivot indices; for 1 <= j <= N, column j of the
* matrix has been interchanged with column JPIV(j).
*
* Further Details
* ===============
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* This routine is a further developed implementation of algorithm
* BSOLVE in [1] using complete pivoting in the LU factorization.
*
* [1] Bo Kagstrom and Lars Westin,
* Generalized Schur Methods with Condition Estimators for
* Solving the Generalized Sylvester Equation, IEEE Transactions
* on Automatic Control, Vol. 34, No. 7, July 1989, pp 745-751.
*
* [2] Peter Poromaa,
* On Efficient and Robust Estimators for the Separation
* between two Regular Matrix Pairs with Applications in
* Condition Estimation. Report IMINF-95.05, Departement of
* Computing Science, Umea University, S-901 87 Umea, Sweden, 1995.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param ijob
* @param n
* @param z
* @param ldz
* @param rhs
* @param rdsum
* @param rdscal
* @param ipiv
* @param jpiv
*
*/
abstract public void dlatdf(int ijob, int n, double[] z, int ldz, double[] rhs, org.netlib.util.doubleW rdsum, org.netlib.util.doubleW rdscal, int[] ipiv, int[] jpiv);
/**
*
* ..
*
* Purpose
* =======
*
* DLATDF uses the LU factorization of the n-by-n matrix Z computed by
* DGETC2 and computes a contribution to the reciprocal Dif-estimate
* by solving Z * x = b for x, and choosing the r.h.s. b such that
* the norm of x is as large as possible. On entry RHS = b holds the
* contribution from earlier solved sub-systems, and on return RHS = x.
*
* The factorization of Z returned by DGETC2 has the form Z = P*L*U*Q,
* where P and Q are permutation matrices. L is lower triangular with
* unit diagonal elements and U is upper triangular.
*
* Arguments
* =========
*
* IJOB (input) INTEGER
* IJOB = 2: First compute an approximative null-vector e
* of Z using DGECON, e is normalized and solve for
* Zx = +-e - f with the sign giving the greater value
* of 2-norm(x). About 5 times as expensive as Default.
* IJOB .ne. 2: Local look ahead strategy where all entries of
* the r.h.s. b is choosen as either +1 or -1 (Default).
*
* N (input) INTEGER
* The number of columns of the matrix Z.
*
* Z (input) DOUBLE PRECISION array, dimension (LDZ, N)
* On entry, the LU part of the factorization of the n-by-n
* matrix Z computed by DGETC2: Z = P * L * U * Q
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDA >= max(1, N).
*
* RHS (input/output) DOUBLE PRECISION array, dimension N.
* On entry, RHS contains contributions from other subsystems.
* On exit, RHS contains the solution of the subsystem with
* entries acoording to the value of IJOB (see above).
*
* RDSUM (input/output) DOUBLE PRECISION
* On entry, the sum of squares of computed contributions to
* the Dif-estimate under computation by DTGSYL, where the
* scaling factor RDSCAL (see below) has been factored out.
* On exit, the corresponding sum of squares updated with the
* contributions from the current sub-system.
* If TRANS = 'T' RDSUM is not touched.
* NOTE: RDSUM only makes sense when DTGSY2 is called by STGSYL.
*
* RDSCAL (input/output) DOUBLE PRECISION
* On entry, scaling factor used to prevent overflow in RDSUM.
* On exit, RDSCAL is updated w.r.t. the current contributions
* in RDSUM.
* If TRANS = 'T', RDSCAL is not touched.
* NOTE: RDSCAL only makes sense when DTGSY2 is called by
* DTGSYL.
*
* IPIV (input) INTEGER array, dimension (N).
* The pivot indices; for 1 <= i <= N, row i of the
* matrix has been interchanged with row IPIV(i).
*
* JPIV (input) INTEGER array, dimension (N).
* The pivot indices; for 1 <= j <= N, column j of the
* matrix has been interchanged with column JPIV(j).
*
* Further Details
* ===============
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* This routine is a further developed implementation of algorithm
* BSOLVE in [1] using complete pivoting in the LU factorization.
*
* [1] Bo Kagstrom and Lars Westin,
* Generalized Schur Methods with Condition Estimators for
* Solving the Generalized Sylvester Equation, IEEE Transactions
* on Automatic Control, Vol. 34, No. 7, July 1989, pp 745-751.
*
* [2] Peter Poromaa,
* On Efficient and Robust Estimators for the Separation
* between two Regular Matrix Pairs with Applications in
* Condition Estimation. Report IMINF-95.05, Departement of
* Computing Science, Umea University, S-901 87 Umea, Sweden, 1995.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param ijob
* @param n
* @param z
* @param _z_offset
* @param ldz
* @param rhs
* @param _rhs_offset
* @param rdsum
* @param rdscal
* @param ipiv
* @param _ipiv_offset
* @param jpiv
* @param _jpiv_offset
*
*/
abstract public void dlatdf(int ijob, int n, double[] z, int _z_offset, int ldz, double[] rhs, int _rhs_offset, org.netlib.util.doubleW rdsum, org.netlib.util.doubleW rdscal, int[] ipiv, int _ipiv_offset, int[] jpiv, int _jpiv_offset);
/**
*
* ..
*
* Purpose
* =======
*
* DLATPS solves one of the triangular systems
*
* A *x = s*b or A'*x = s*b
*
* with scaling to prevent overflow, where A is an upper or lower
* triangular matrix stored in packed form. Here A' denotes the
* transpose of A, x and b are n-element vectors, and s is a scaling
* factor, usually less than or equal to 1, chosen so that the
* components of x will be less than the overflow threshold. If the
* unscaled problem will not cause overflow, the Level 2 BLAS routine
* DTPSV is called. If the matrix A is singular (A(j,j) = 0 for some j),
* then s is set to 0 and a non-trivial solution to A*x = 0 is returned.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the matrix A is upper or lower triangular.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* TRANS (input) CHARACTER*1
* Specifies the operation applied to A.
* = 'N': Solve A * x = s*b (No transpose)
* = 'T': Solve A'* x = s*b (Transpose)
* = 'C': Solve A'* x = s*b (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* Specifies whether or not the matrix A is unit triangular.
* = 'N': Non-unit triangular
* = 'U': Unit triangular
*
* NORMIN (input) CHARACTER*1
* Specifies whether CNORM has been set or not.
* = 'Y': CNORM contains the column norms on entry
* = 'N': CNORM is not set on entry. On exit, the norms will
* be computed and stored in CNORM.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The upper or lower triangular matrix A, packed columnwise in
* a linear array. The j-th column of A is stored in the array
* AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
*
* X (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the right hand side b of the triangular system.
* On exit, X is overwritten by the solution vector x.
*
* SCALE (output) DOUBLE PRECISION
* The scaling factor s for the triangular system
* A * x = s*b or A'* x = s*b.
* If SCALE = 0, the matrix A is singular or badly scaled, and
* the vector x is an exact or approximate solution to A*x = 0.
*
* CNORM (input or output) DOUBLE PRECISION array, dimension (N)
*
* If NORMIN = 'Y', CNORM is an input argument and CNORM(j)
* contains the norm of the off-diagonal part of the j-th column
* of A. If TRANS = 'N', CNORM(j) must be greater than or equal
* to the infinity-norm, and if TRANS = 'T' or 'C', CNORM(j)
* must be greater than or equal to the 1-norm.
*
* If NORMIN = 'N', CNORM is an output argument and CNORM(j)
* returns the 1-norm of the offdiagonal part of the j-th column
* of A.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
*
* Further Details
* ======= =======
*
* A rough bound on x is computed; if that is less than overflow, DTPSV
* is called, otherwise, specific code is used which checks for possible
* overflow or divide-by-zero at every operation.
*
* A columnwise scheme is used for solving A*x = b. The basic algorithm
* if A is lower triangular is
*
* x[1:n] := b[1:n]
* for j = 1, ..., n
* x(j) := x(j) / A(j,j)
* x[j+1:n] := x[j+1:n] - x(j) * A[j+1:n,j]
* end
*
* Define bounds on the components of x after j iterations of the loop:
* M(j) = bound on x[1:j]
* G(j) = bound on x[j+1:n]
* Initially, let M(0) = 0 and G(0) = max{x(i), i=1,...,n}.
*
* Then for iteration j+1 we have
* M(j+1) <= G(j) / | A(j+1,j+1) |
* G(j+1) <= G(j) + M(j+1) * | A[j+2:n,j+1] |
* <= G(j) ( 1 + CNORM(j+1) / | A(j+1,j+1) | )
*
* where CNORM(j+1) is greater than or equal to the infinity-norm of
* column j+1 of A, not counting the diagonal. Hence
*
* G(j) <= G(0) product ( 1 + CNORM(i) / | A(i,i) | )
* 1<=i<=j
* and
*
* |x(j)| <= ( G(0) / |A(j,j)| ) product ( 1 + CNORM(i) / |A(i,i)| )
* 1<=i< j
*
* Since |x(j)| <= M(j), we use the Level 2 BLAS routine DTPSV if the
* reciprocal of the largest M(j), j=1,..,n, is larger than
* max(underflow, 1/overflow).
*
* The bound on x(j) is also used to determine when a step in the
* columnwise method can be performed without fear of overflow. If
* the computed bound is greater than a large constant, x is scaled to
* prevent overflow, but if the bound overflows, x is set to 0, x(j) to
* 1, and scale to 0, and a non-trivial solution to A*x = 0 is found.
*
* Similarly, a row-wise scheme is used to solve A'*x = b. The basic
* algorithm for A upper triangular is
*
* for j = 1, ..., n
* x(j) := ( b(j) - A[1:j-1,j]' * x[1:j-1] ) / A(j,j)
* end
*
* We simultaneously compute two bounds
* G(j) = bound on ( b(i) - A[1:i-1,i]' * x[1:i-1] ), 1<=i<=j
* M(j) = bound on x(i), 1<=i<=j
*
* The initial values are G(0) = 0, M(0) = max{b(i), i=1,..,n}, and we
* add the constraint G(j) >= G(j-1) and M(j) >= M(j-1) for j >= 1.
* Then the bound on x(j) is
*
* M(j) <= M(j-1) * ( 1 + CNORM(j) ) / | A(j,j) |
*
* <= M(0) * product ( ( 1 + CNORM(i) ) / |A(i,i)| )
* 1<=i<=j
*
* and we can safely call DTPSV if 1/M(n) and 1/G(n) are both greater
* than max(underflow, 1/overflow).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param normin
* @param n
* @param ap
* @param x
* @param scale
* @param cnorm
* @param info
*
*/
abstract public void dlatps(java.lang.String uplo, java.lang.String trans, java.lang.String diag, java.lang.String normin, int n, double[] ap, double[] x, org.netlib.util.doubleW scale, double[] cnorm, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLATPS solves one of the triangular systems
*
* A *x = s*b or A'*x = s*b
*
* with scaling to prevent overflow, where A is an upper or lower
* triangular matrix stored in packed form. Here A' denotes the
* transpose of A, x and b are n-element vectors, and s is a scaling
* factor, usually less than or equal to 1, chosen so that the
* components of x will be less than the overflow threshold. If the
* unscaled problem will not cause overflow, the Level 2 BLAS routine
* DTPSV is called. If the matrix A is singular (A(j,j) = 0 for some j),
* then s is set to 0 and a non-trivial solution to A*x = 0 is returned.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the matrix A is upper or lower triangular.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* TRANS (input) CHARACTER*1
* Specifies the operation applied to A.
* = 'N': Solve A * x = s*b (No transpose)
* = 'T': Solve A'* x = s*b (Transpose)
* = 'C': Solve A'* x = s*b (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* Specifies whether or not the matrix A is unit triangular.
* = 'N': Non-unit triangular
* = 'U': Unit triangular
*
* NORMIN (input) CHARACTER*1
* Specifies whether CNORM has been set or not.
* = 'Y': CNORM contains the column norms on entry
* = 'N': CNORM is not set on entry. On exit, the norms will
* be computed and stored in CNORM.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The upper or lower triangular matrix A, packed columnwise in
* a linear array. The j-th column of A is stored in the array
* AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
*
* X (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the right hand side b of the triangular system.
* On exit, X is overwritten by the solution vector x.
*
* SCALE (output) DOUBLE PRECISION
* The scaling factor s for the triangular system
* A * x = s*b or A'* x = s*b.
* If SCALE = 0, the matrix A is singular or badly scaled, and
* the vector x is an exact or approximate solution to A*x = 0.
*
* CNORM (input or output) DOUBLE PRECISION array, dimension (N)
*
* If NORMIN = 'Y', CNORM is an input argument and CNORM(j)
* contains the norm of the off-diagonal part of the j-th column
* of A. If TRANS = 'N', CNORM(j) must be greater than or equal
* to the infinity-norm, and if TRANS = 'T' or 'C', CNORM(j)
* must be greater than or equal to the 1-norm.
*
* If NORMIN = 'N', CNORM is an output argument and CNORM(j)
* returns the 1-norm of the offdiagonal part of the j-th column
* of A.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
*
* Further Details
* ======= =======
*
* A rough bound on x is computed; if that is less than overflow, DTPSV
* is called, otherwise, specific code is used which checks for possible
* overflow or divide-by-zero at every operation.
*
* A columnwise scheme is used for solving A*x = b. The basic algorithm
* if A is lower triangular is
*
* x[1:n] := b[1:n]
* for j = 1, ..., n
* x(j) := x(j) / A(j,j)
* x[j+1:n] := x[j+1:n] - x(j) * A[j+1:n,j]
* end
*
* Define bounds on the components of x after j iterations of the loop:
* M(j) = bound on x[1:j]
* G(j) = bound on x[j+1:n]
* Initially, let M(0) = 0 and G(0) = max{x(i), i=1,...,n}.
*
* Then for iteration j+1 we have
* M(j+1) <= G(j) / | A(j+1,j+1) |
* G(j+1) <= G(j) + M(j+1) * | A[j+2:n,j+1] |
* <= G(j) ( 1 + CNORM(j+1) / | A(j+1,j+1) | )
*
* where CNORM(j+1) is greater than or equal to the infinity-norm of
* column j+1 of A, not counting the diagonal. Hence
*
* G(j) <= G(0) product ( 1 + CNORM(i) / | A(i,i) | )
* 1<=i<=j
* and
*
* |x(j)| <= ( G(0) / |A(j,j)| ) product ( 1 + CNORM(i) / |A(i,i)| )
* 1<=i< j
*
* Since |x(j)| <= M(j), we use the Level 2 BLAS routine DTPSV if the
* reciprocal of the largest M(j), j=1,..,n, is larger than
* max(underflow, 1/overflow).
*
* The bound on x(j) is also used to determine when a step in the
* columnwise method can be performed without fear of overflow. If
* the computed bound is greater than a large constant, x is scaled to
* prevent overflow, but if the bound overflows, x is set to 0, x(j) to
* 1, and scale to 0, and a non-trivial solution to A*x = 0 is found.
*
* Similarly, a row-wise scheme is used to solve A'*x = b. The basic
* algorithm for A upper triangular is
*
* for j = 1, ..., n
* x(j) := ( b(j) - A[1:j-1,j]' * x[1:j-1] ) / A(j,j)
* end
*
* We simultaneously compute two bounds
* G(j) = bound on ( b(i) - A[1:i-1,i]' * x[1:i-1] ), 1<=i<=j
* M(j) = bound on x(i), 1<=i<=j
*
* The initial values are G(0) = 0, M(0) = max{b(i), i=1,..,n}, and we
* add the constraint G(j) >= G(j-1) and M(j) >= M(j-1) for j >= 1.
* Then the bound on x(j) is
*
* M(j) <= M(j-1) * ( 1 + CNORM(j) ) / | A(j,j) |
*
* <= M(0) * product ( ( 1 + CNORM(i) ) / |A(i,i)| )
* 1<=i<=j
*
* and we can safely call DTPSV if 1/M(n) and 1/G(n) are both greater
* than max(underflow, 1/overflow).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param normin
* @param n
* @param ap
* @param _ap_offset
* @param x
* @param _x_offset
* @param scale
* @param cnorm
* @param _cnorm_offset
* @param info
*
*/
abstract public void dlatps(java.lang.String uplo, java.lang.String trans, java.lang.String diag, java.lang.String normin, int n, double[] ap, int _ap_offset, double[] x, int _x_offset, org.netlib.util.doubleW scale, double[] cnorm, int _cnorm_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLATRD reduces NB rows and columns of a real symmetric matrix A to
* symmetric tridiagonal form by an orthogonal similarity
* transformation Q' * A * Q, and returns the matrices V and W which are
* needed to apply the transformation to the unreduced part of A.
*
* If UPLO = 'U', DLATRD reduces the last NB rows and columns of a
* matrix, of which the upper triangle is supplied;
* if UPLO = 'L', DLATRD reduces the first NB rows and columns of a
* matrix, of which the lower triangle is supplied.
*
* This is an auxiliary routine called by DSYTRD.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored:
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A.
*
* NB (input) INTEGER
* The number of rows and columns to be reduced.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* n-by-n upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading n-by-n lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
* On exit:
* if UPLO = 'U', the last NB columns have been reduced to
* tridiagonal form, with the diagonal elements overwriting
* the diagonal elements of A; the elements above the diagonal
* with the array TAU, represent the orthogonal matrix Q as a
* product of elementary reflectors;
* if UPLO = 'L', the first NB columns have been reduced to
* tridiagonal form, with the diagonal elements overwriting
* the diagonal elements of A; the elements below the diagonal
* with the array TAU, represent the orthogonal matrix Q as a
* product of elementary reflectors.
* See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= (1,N).
*
* E (output) DOUBLE PRECISION array, dimension (N-1)
* If UPLO = 'U', E(n-nb:n-1) contains the superdiagonal
* elements of the last NB columns of the reduced matrix;
* if UPLO = 'L', E(1:nb) contains the subdiagonal elements of
* the first NB columns of the reduced matrix.
*
* TAU (output) DOUBLE PRECISION array, dimension (N-1)
* The scalar factors of the elementary reflectors, stored in
* TAU(n-nb:n-1) if UPLO = 'U', and in TAU(1:nb) if UPLO = 'L'.
* See Further Details.
*
* W (output) DOUBLE PRECISION array, dimension (LDW,NB)
* The n-by-nb matrix W required to update the unreduced part
* of A.
*
* LDW (input) INTEGER
* The leading dimension of the array W. LDW >= max(1,N).
*
* Further Details
* ===============
*
* If UPLO = 'U', the matrix Q is represented as a product of elementary
* reflectors
*
* Q = H(n) H(n-1) . . . H(n-nb+1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(i:n) = 0 and v(i-1) = 1; v(1:i-1) is stored on exit in A(1:i-1,i),
* and tau in TAU(i-1).
*
* If UPLO = 'L', the matrix Q is represented as a product of elementary
* reflectors
*
* Q = H(1) H(2) . . . H(nb).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i) = 0 and v(i+1) = 1; v(i+1:n) is stored on exit in A(i+1:n,i),
* and tau in TAU(i).
*
* The elements of the vectors v together form the n-by-nb matrix V
* which is needed, with W, to apply the transformation to the unreduced
* part of the matrix, using a symmetric rank-2k update of the form:
* A := A - V*W' - W*V'.
*
* The contents of A on exit are illustrated by the following examples
* with n = 5 and nb = 2:
*
* if UPLO = 'U': if UPLO = 'L':
*
* ( a a a v4 v5 ) ( d )
* ( a a v4 v5 ) ( 1 d )
* ( a 1 v5 ) ( v1 1 a )
* ( d 1 ) ( v1 v2 a a )
* ( d ) ( v1 v2 a a a )
*
* where d denotes a diagonal element of the reduced matrix, a denotes
* an element of the original matrix that is unchanged, and vi denotes
* an element of the vector defining H(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nb
* @param a
* @param lda
* @param e
* @param tau
* @param w
* @param ldw
*
*/
abstract public void dlatrd(java.lang.String uplo, int n, int nb, double[] a, int lda, double[] e, double[] tau, double[] w, int ldw);
/**
*
* ..
*
* Purpose
* =======
*
* DLATRD reduces NB rows and columns of a real symmetric matrix A to
* symmetric tridiagonal form by an orthogonal similarity
* transformation Q' * A * Q, and returns the matrices V and W which are
* needed to apply the transformation to the unreduced part of A.
*
* If UPLO = 'U', DLATRD reduces the last NB rows and columns of a
* matrix, of which the upper triangle is supplied;
* if UPLO = 'L', DLATRD reduces the first NB rows and columns of a
* matrix, of which the lower triangle is supplied.
*
* This is an auxiliary routine called by DSYTRD.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored:
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A.
*
* NB (input) INTEGER
* The number of rows and columns to be reduced.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* n-by-n upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading n-by-n lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
* On exit:
* if UPLO = 'U', the last NB columns have been reduced to
* tridiagonal form, with the diagonal elements overwriting
* the diagonal elements of A; the elements above the diagonal
* with the array TAU, represent the orthogonal matrix Q as a
* product of elementary reflectors;
* if UPLO = 'L', the first NB columns have been reduced to
* tridiagonal form, with the diagonal elements overwriting
* the diagonal elements of A; the elements below the diagonal
* with the array TAU, represent the orthogonal matrix Q as a
* product of elementary reflectors.
* See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= (1,N).
*
* E (output) DOUBLE PRECISION array, dimension (N-1)
* If UPLO = 'U', E(n-nb:n-1) contains the superdiagonal
* elements of the last NB columns of the reduced matrix;
* if UPLO = 'L', E(1:nb) contains the subdiagonal elements of
* the first NB columns of the reduced matrix.
*
* TAU (output) DOUBLE PRECISION array, dimension (N-1)
* The scalar factors of the elementary reflectors, stored in
* TAU(n-nb:n-1) if UPLO = 'U', and in TAU(1:nb) if UPLO = 'L'.
* See Further Details.
*
* W (output) DOUBLE PRECISION array, dimension (LDW,NB)
* The n-by-nb matrix W required to update the unreduced part
* of A.
*
* LDW (input) INTEGER
* The leading dimension of the array W. LDW >= max(1,N).
*
* Further Details
* ===============
*
* If UPLO = 'U', the matrix Q is represented as a product of elementary
* reflectors
*
* Q = H(n) H(n-1) . . . H(n-nb+1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(i:n) = 0 and v(i-1) = 1; v(1:i-1) is stored on exit in A(1:i-1,i),
* and tau in TAU(i-1).
*
* If UPLO = 'L', the matrix Q is represented as a product of elementary
* reflectors
*
* Q = H(1) H(2) . . . H(nb).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i) = 0 and v(i+1) = 1; v(i+1:n) is stored on exit in A(i+1:n,i),
* and tau in TAU(i).
*
* The elements of the vectors v together form the n-by-nb matrix V
* which is needed, with W, to apply the transformation to the unreduced
* part of the matrix, using a symmetric rank-2k update of the form:
* A := A - V*W' - W*V'.
*
* The contents of A on exit are illustrated by the following examples
* with n = 5 and nb = 2:
*
* if UPLO = 'U': if UPLO = 'L':
*
* ( a a a v4 v5 ) ( d )
* ( a a v4 v5 ) ( 1 d )
* ( a 1 v5 ) ( v1 1 a )
* ( d 1 ) ( v1 v2 a a )
* ( d ) ( v1 v2 a a a )
*
* where d denotes a diagonal element of the reduced matrix, a denotes
* an element of the original matrix that is unchanged, and vi denotes
* an element of the vector defining H(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nb
* @param a
* @param _a_offset
* @param lda
* @param e
* @param _e_offset
* @param tau
* @param _tau_offset
* @param w
* @param _w_offset
* @param ldw
*
*/
abstract public void dlatrd(java.lang.String uplo, int n, int nb, double[] a, int _a_offset, int lda, double[] e, int _e_offset, double[] tau, int _tau_offset, double[] w, int _w_offset, int ldw);
/**
*
* ..
*
* Purpose
* =======
*
* DLATRS solves one of the triangular systems
*
* A *x = s*b or A'*x = s*b
*
* with scaling to prevent overflow. Here A is an upper or lower
* triangular matrix, A' denotes the transpose of A, x and b are
* n-element vectors, and s is a scaling factor, usually less than
* or equal to 1, chosen so that the components of x will be less than
* the overflow threshold. If the unscaled problem will not cause
* overflow, the Level 2 BLAS routine DTRSV is called. If the matrix A
* is singular (A(j,j) = 0 for some j), then s is set to 0 and a
* non-trivial solution to A*x = 0 is returned.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the matrix A is upper or lower triangular.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* TRANS (input) CHARACTER*1
* Specifies the operation applied to A.
* = 'N': Solve A * x = s*b (No transpose)
* = 'T': Solve A'* x = s*b (Transpose)
* = 'C': Solve A'* x = s*b (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* Specifies whether or not the matrix A is unit triangular.
* = 'N': Non-unit triangular
* = 'U': Unit triangular
*
* NORMIN (input) CHARACTER*1
* Specifies whether CNORM has been set or not.
* = 'Y': CNORM contains the column norms on entry
* = 'N': CNORM is not set on entry. On exit, the norms will
* be computed and stored in CNORM.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The triangular matrix A. If UPLO = 'U', the leading n by n
* upper triangular part of the array A contains the upper
* triangular matrix, and the strictly lower triangular part of
* A is not referenced. If UPLO = 'L', the leading n by n lower
* triangular part of the array A contains the lower triangular
* matrix, and the strictly upper triangular part of A is not
* referenced. If DIAG = 'U', the diagonal elements of A are
* also not referenced and are assumed to be 1.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max (1,N).
*
* X (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the right hand side b of the triangular system.
* On exit, X is overwritten by the solution vector x.
*
* SCALE (output) DOUBLE PRECISION
* The scaling factor s for the triangular system
* A * x = s*b or A'* x = s*b.
* If SCALE = 0, the matrix A is singular or badly scaled, and
* the vector x is an exact or approximate solution to A*x = 0.
*
* CNORM (input or output) DOUBLE PRECISION array, dimension (N)
*
* If NORMIN = 'Y', CNORM is an input argument and CNORM(j)
* contains the norm of the off-diagonal part of the j-th column
* of A. If TRANS = 'N', CNORM(j) must be greater than or equal
* to the infinity-norm, and if TRANS = 'T' or 'C', CNORM(j)
* must be greater than or equal to the 1-norm.
*
* If NORMIN = 'N', CNORM is an output argument and CNORM(j)
* returns the 1-norm of the offdiagonal part of the j-th column
* of A.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
*
* Further Details
* ======= =======
*
* A rough bound on x is computed; if that is less than overflow, DTRSV
* is called, otherwise, specific code is used which checks for possible
* overflow or divide-by-zero at every operation.
*
* A columnwise scheme is used for solving A*x = b. The basic algorithm
* if A is lower triangular is
*
* x[1:n] := b[1:n]
* for j = 1, ..., n
* x(j) := x(j) / A(j,j)
* x[j+1:n] := x[j+1:n] - x(j) * A[j+1:n,j]
* end
*
* Define bounds on the components of x after j iterations of the loop:
* M(j) = bound on x[1:j]
* G(j) = bound on x[j+1:n]
* Initially, let M(0) = 0 and G(0) = max{x(i), i=1,...,n}.
*
* Then for iteration j+1 we have
* M(j+1) <= G(j) / | A(j+1,j+1) |
* G(j+1) <= G(j) + M(j+1) * | A[j+2:n,j+1] |
* <= G(j) ( 1 + CNORM(j+1) / | A(j+1,j+1) | )
*
* where CNORM(j+1) is greater than or equal to the infinity-norm of
* column j+1 of A, not counting the diagonal. Hence
*
* G(j) <= G(0) product ( 1 + CNORM(i) / | A(i,i) | )
* 1<=i<=j
* and
*
* |x(j)| <= ( G(0) / |A(j,j)| ) product ( 1 + CNORM(i) / |A(i,i)| )
* 1<=i< j
*
* Since |x(j)| <= M(j), we use the Level 2 BLAS routine DTRSV if the
* reciprocal of the largest M(j), j=1,..,n, is larger than
* max(underflow, 1/overflow).
*
* The bound on x(j) is also used to determine when a step in the
* columnwise method can be performed without fear of overflow. If
* the computed bound is greater than a large constant, x is scaled to
* prevent overflow, but if the bound overflows, x is set to 0, x(j) to
* 1, and scale to 0, and a non-trivial solution to A*x = 0 is found.
*
* Similarly, a row-wise scheme is used to solve A'*x = b. The basic
* algorithm for A upper triangular is
*
* for j = 1, ..., n
* x(j) := ( b(j) - A[1:j-1,j]' * x[1:j-1] ) / A(j,j)
* end
*
* We simultaneously compute two bounds
* G(j) = bound on ( b(i) - A[1:i-1,i]' * x[1:i-1] ), 1<=i<=j
* M(j) = bound on x(i), 1<=i<=j
*
* The initial values are G(0) = 0, M(0) = max{b(i), i=1,..,n}, and we
* add the constraint G(j) >= G(j-1) and M(j) >= M(j-1) for j >= 1.
* Then the bound on x(j) is
*
* M(j) <= M(j-1) * ( 1 + CNORM(j) ) / | A(j,j) |
*
* <= M(0) * product ( ( 1 + CNORM(i) ) / |A(i,i)| )
* 1<=i<=j
*
* and we can safely call DTRSV if 1/M(n) and 1/G(n) are both greater
* than max(underflow, 1/overflow).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param normin
* @param n
* @param a
* @param lda
* @param x
* @param scale
* @param cnorm
* @param info
*
*/
abstract public void dlatrs(java.lang.String uplo, java.lang.String trans, java.lang.String diag, java.lang.String normin, int n, double[] a, int lda, double[] x, org.netlib.util.doubleW scale, double[] cnorm, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLATRS solves one of the triangular systems
*
* A *x = s*b or A'*x = s*b
*
* with scaling to prevent overflow. Here A is an upper or lower
* triangular matrix, A' denotes the transpose of A, x and b are
* n-element vectors, and s is a scaling factor, usually less than
* or equal to 1, chosen so that the components of x will be less than
* the overflow threshold. If the unscaled problem will not cause
* overflow, the Level 2 BLAS routine DTRSV is called. If the matrix A
* is singular (A(j,j) = 0 for some j), then s is set to 0 and a
* non-trivial solution to A*x = 0 is returned.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the matrix A is upper or lower triangular.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* TRANS (input) CHARACTER*1
* Specifies the operation applied to A.
* = 'N': Solve A * x = s*b (No transpose)
* = 'T': Solve A'* x = s*b (Transpose)
* = 'C': Solve A'* x = s*b (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* Specifies whether or not the matrix A is unit triangular.
* = 'N': Non-unit triangular
* = 'U': Unit triangular
*
* NORMIN (input) CHARACTER*1
* Specifies whether CNORM has been set or not.
* = 'Y': CNORM contains the column norms on entry
* = 'N': CNORM is not set on entry. On exit, the norms will
* be computed and stored in CNORM.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The triangular matrix A. If UPLO = 'U', the leading n by n
* upper triangular part of the array A contains the upper
* triangular matrix, and the strictly lower triangular part of
* A is not referenced. If UPLO = 'L', the leading n by n lower
* triangular part of the array A contains the lower triangular
* matrix, and the strictly upper triangular part of A is not
* referenced. If DIAG = 'U', the diagonal elements of A are
* also not referenced and are assumed to be 1.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max (1,N).
*
* X (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the right hand side b of the triangular system.
* On exit, X is overwritten by the solution vector x.
*
* SCALE (output) DOUBLE PRECISION
* The scaling factor s for the triangular system
* A * x = s*b or A'* x = s*b.
* If SCALE = 0, the matrix A is singular or badly scaled, and
* the vector x is an exact or approximate solution to A*x = 0.
*
* CNORM (input or output) DOUBLE PRECISION array, dimension (N)
*
* If NORMIN = 'Y', CNORM is an input argument and CNORM(j)
* contains the norm of the off-diagonal part of the j-th column
* of A. If TRANS = 'N', CNORM(j) must be greater than or equal
* to the infinity-norm, and if TRANS = 'T' or 'C', CNORM(j)
* must be greater than or equal to the 1-norm.
*
* If NORMIN = 'N', CNORM is an output argument and CNORM(j)
* returns the 1-norm of the offdiagonal part of the j-th column
* of A.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
*
* Further Details
* ======= =======
*
* A rough bound on x is computed; if that is less than overflow, DTRSV
* is called, otherwise, specific code is used which checks for possible
* overflow or divide-by-zero at every operation.
*
* A columnwise scheme is used for solving A*x = b. The basic algorithm
* if A is lower triangular is
*
* x[1:n] := b[1:n]
* for j = 1, ..., n
* x(j) := x(j) / A(j,j)
* x[j+1:n] := x[j+1:n] - x(j) * A[j+1:n,j]
* end
*
* Define bounds on the components of x after j iterations of the loop:
* M(j) = bound on x[1:j]
* G(j) = bound on x[j+1:n]
* Initially, let M(0) = 0 and G(0) = max{x(i), i=1,...,n}.
*
* Then for iteration j+1 we have
* M(j+1) <= G(j) / | A(j+1,j+1) |
* G(j+1) <= G(j) + M(j+1) * | A[j+2:n,j+1] |
* <= G(j) ( 1 + CNORM(j+1) / | A(j+1,j+1) | )
*
* where CNORM(j+1) is greater than or equal to the infinity-norm of
* column j+1 of A, not counting the diagonal. Hence
*
* G(j) <= G(0) product ( 1 + CNORM(i) / | A(i,i) | )
* 1<=i<=j
* and
*
* |x(j)| <= ( G(0) / |A(j,j)| ) product ( 1 + CNORM(i) / |A(i,i)| )
* 1<=i< j
*
* Since |x(j)| <= M(j), we use the Level 2 BLAS routine DTRSV if the
* reciprocal of the largest M(j), j=1,..,n, is larger than
* max(underflow, 1/overflow).
*
* The bound on x(j) is also used to determine when a step in the
* columnwise method can be performed without fear of overflow. If
* the computed bound is greater than a large constant, x is scaled to
* prevent overflow, but if the bound overflows, x is set to 0, x(j) to
* 1, and scale to 0, and a non-trivial solution to A*x = 0 is found.
*
* Similarly, a row-wise scheme is used to solve A'*x = b. The basic
* algorithm for A upper triangular is
*
* for j = 1, ..., n
* x(j) := ( b(j) - A[1:j-1,j]' * x[1:j-1] ) / A(j,j)
* end
*
* We simultaneously compute two bounds
* G(j) = bound on ( b(i) - A[1:i-1,i]' * x[1:i-1] ), 1<=i<=j
* M(j) = bound on x(i), 1<=i<=j
*
* The initial values are G(0) = 0, M(0) = max{b(i), i=1,..,n}, and we
* add the constraint G(j) >= G(j-1) and M(j) >= M(j-1) for j >= 1.
* Then the bound on x(j) is
*
* M(j) <= M(j-1) * ( 1 + CNORM(j) ) / | A(j,j) |
*
* <= M(0) * product ( ( 1 + CNORM(i) ) / |A(i,i)| )
* 1<=i<=j
*
* and we can safely call DTRSV if 1/M(n) and 1/G(n) are both greater
* than max(underflow, 1/overflow).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param normin
* @param n
* @param a
* @param _a_offset
* @param lda
* @param x
* @param _x_offset
* @param scale
* @param cnorm
* @param _cnorm_offset
* @param info
*
*/
abstract public void dlatrs(java.lang.String uplo, java.lang.String trans, java.lang.String diag, java.lang.String normin, int n, double[] a, int _a_offset, int lda, double[] x, int _x_offset, org.netlib.util.doubleW scale, double[] cnorm, int _cnorm_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLATRZ factors the M-by-(M+L) real upper trapezoidal matrix
* [ A1 A2 ] = [ A(1:M,1:M) A(1:M,N-L+1:N) ] as ( R 0 ) * Z, by means
* of orthogonal transformations. Z is an (M+L)-by-(M+L) orthogonal
* matrix and, R and A1 are M-by-M upper triangular matrices.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* L (input) INTEGER
* The number of columns of the matrix A containing the
* meaningful part of the Householder vectors. N-M >= L >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the leading M-by-N upper trapezoidal part of the
* array A must contain the matrix to be factorized.
* On exit, the leading M-by-M upper triangular part of A
* contains the upper triangular matrix R, and elements N-L+1 to
* N of the first M rows of A, with the array TAU, represent the
* orthogonal matrix Z as a product of M elementary reflectors.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) DOUBLE PRECISION array, dimension (M)
* The scalar factors of the elementary reflectors.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (M)
*
* Further Details
* ===============
*
* Based on contributions by
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
*
* The factorization is obtained by Householder's method. The kth
* transformation matrix, Z( k ), which is used to introduce zeros into
* the ( m - k + 1 )th row of A, is given in the form
*
* Z( k ) = ( I 0 ),
* ( 0 T( k ) )
*
* where
*
* T( k ) = I - tau*u( k )*u( k )', u( k ) = ( 1 ),
* ( 0 )
* ( z( k ) )
*
* tau is a scalar and z( k ) is an l element vector. tau and z( k )
* are chosen to annihilate the elements of the kth row of A2.
*
* The scalar tau is returned in the kth element of TAU and the vector
* u( k ) in the kth row of A2, such that the elements of z( k ) are
* in a( k, l + 1 ), ..., a( k, n ). The elements of R are returned in
* the upper triangular part of A1.
*
* Z is given by
*
* Z = Z( 1 ) * Z( 2 ) * ... * Z( m ).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param l
* @param a
* @param lda
* @param tau
* @param work
*
*/
abstract public void dlatrz(int m, int n, int l, double[] a, int lda, double[] tau, double[] work);
/**
*
* ..
*
* Purpose
* =======
*
* DLATRZ factors the M-by-(M+L) real upper trapezoidal matrix
* [ A1 A2 ] = [ A(1:M,1:M) A(1:M,N-L+1:N) ] as ( R 0 ) * Z, by means
* of orthogonal transformations. Z is an (M+L)-by-(M+L) orthogonal
* matrix and, R and A1 are M-by-M upper triangular matrices.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* L (input) INTEGER
* The number of columns of the matrix A containing the
* meaningful part of the Householder vectors. N-M >= L >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the leading M-by-N upper trapezoidal part of the
* array A must contain the matrix to be factorized.
* On exit, the leading M-by-M upper triangular part of A
* contains the upper triangular matrix R, and elements N-L+1 to
* N of the first M rows of A, with the array TAU, represent the
* orthogonal matrix Z as a product of M elementary reflectors.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) DOUBLE PRECISION array, dimension (M)
* The scalar factors of the elementary reflectors.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (M)
*
* Further Details
* ===============
*
* Based on contributions by
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
*
* The factorization is obtained by Householder's method. The kth
* transformation matrix, Z( k ), which is used to introduce zeros into
* the ( m - k + 1 )th row of A, is given in the form
*
* Z( k ) = ( I 0 ),
* ( 0 T( k ) )
*
* where
*
* T( k ) = I - tau*u( k )*u( k )', u( k ) = ( 1 ),
* ( 0 )
* ( z( k ) )
*
* tau is a scalar and z( k ) is an l element vector. tau and z( k )
* are chosen to annihilate the elements of the kth row of A2.
*
* The scalar tau is returned in the kth element of TAU and the vector
* u( k ) in the kth row of A2, such that the elements of z( k ) are
* in a( k, l + 1 ), ..., a( k, n ). The elements of R are returned in
* the upper triangular part of A1.
*
* Z is given by
*
* Z = Z( 1 ) * Z( 2 ) * ... * Z( m ).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param l
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
*
*/
abstract public void dlatrz(int m, int n, int l, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* This routine is deprecated and has been replaced by routine DORMRZ.
*
* DLATZM applies a Householder matrix generated by DTZRQF to a matrix.
*
* Let P = I - tau*u*u', u = ( 1 ),
* ( v )
* where v is an (m-1) vector if SIDE = 'L', or a (n-1) vector if
* SIDE = 'R'.
*
* If SIDE equals 'L', let
* C = [ C1 ] 1
* [ C2 ] m-1
* n
* Then C is overwritten by P*C.
*
* If SIDE equals 'R', let
* C = [ C1, C2 ] m
* 1 n-1
* Then C is overwritten by C*P.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': form P * C
* = 'R': form C * P
*
* M (input) INTEGER
* The number of rows of the matrix C.
*
* N (input) INTEGER
* The number of columns of the matrix C.
*
* V (input) DOUBLE PRECISION array, dimension
* (1 + (M-1)*abs(INCV)) if SIDE = 'L'
* (1 + (N-1)*abs(INCV)) if SIDE = 'R'
* The vector v in the representation of P. V is not used
* if TAU = 0.
*
* INCV (input) INTEGER
* The increment between elements of v. INCV <> 0
*
* TAU (input) DOUBLE PRECISION
* The value tau in the representation of P.
*
* C1 (input/output) DOUBLE PRECISION array, dimension
* (LDC,N) if SIDE = 'L'
* (M,1) if SIDE = 'R'
* On entry, the n-vector C1 if SIDE = 'L', or the m-vector C1
* if SIDE = 'R'.
*
* On exit, the first row of P*C if SIDE = 'L', or the first
* column of C*P if SIDE = 'R'.
*
* C2 (input/output) DOUBLE PRECISION array, dimension
* (LDC, N) if SIDE = 'L'
* (LDC, N-1) if SIDE = 'R'
* On entry, the (m - 1) x n matrix C2 if SIDE = 'L', or the
* m x (n - 1) matrix C2 if SIDE = 'R'.
*
* On exit, rows 2:m of P*C if SIDE = 'L', or columns 2:m of C*P
* if SIDE = 'R'.
*
* LDC (input) INTEGER
* The leading dimension of the arrays C1 and C2. LDC >= (1,M).
*
* WORK (workspace) DOUBLE PRECISION array, dimension
* (N) if SIDE = 'L'
* (M) if SIDE = 'R'
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param m
* @param n
* @param v
* @param incv
* @param tau
* @param c1
* @param c2
* @param Ldc
* @param work
*
*/
abstract public void dlatzm(java.lang.String side, int m, int n, double[] v, int incv, double tau, double[] c1, double[] c2, int Ldc, double[] work);
/**
*
* ..
*
* Purpose
* =======
*
* This routine is deprecated and has been replaced by routine DORMRZ.
*
* DLATZM applies a Householder matrix generated by DTZRQF to a matrix.
*
* Let P = I - tau*u*u', u = ( 1 ),
* ( v )
* where v is an (m-1) vector if SIDE = 'L', or a (n-1) vector if
* SIDE = 'R'.
*
* If SIDE equals 'L', let
* C = [ C1 ] 1
* [ C2 ] m-1
* n
* Then C is overwritten by P*C.
*
* If SIDE equals 'R', let
* C = [ C1, C2 ] m
* 1 n-1
* Then C is overwritten by C*P.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': form P * C
* = 'R': form C * P
*
* M (input) INTEGER
* The number of rows of the matrix C.
*
* N (input) INTEGER
* The number of columns of the matrix C.
*
* V (input) DOUBLE PRECISION array, dimension
* (1 + (M-1)*abs(INCV)) if SIDE = 'L'
* (1 + (N-1)*abs(INCV)) if SIDE = 'R'
* The vector v in the representation of P. V is not used
* if TAU = 0.
*
* INCV (input) INTEGER
* The increment between elements of v. INCV <> 0
*
* TAU (input) DOUBLE PRECISION
* The value tau in the representation of P.
*
* C1 (input/output) DOUBLE PRECISION array, dimension
* (LDC,N) if SIDE = 'L'
* (M,1) if SIDE = 'R'
* On entry, the n-vector C1 if SIDE = 'L', or the m-vector C1
* if SIDE = 'R'.
*
* On exit, the first row of P*C if SIDE = 'L', or the first
* column of C*P if SIDE = 'R'.
*
* C2 (input/output) DOUBLE PRECISION array, dimension
* (LDC, N) if SIDE = 'L'
* (LDC, N-1) if SIDE = 'R'
* On entry, the (m - 1) x n matrix C2 if SIDE = 'L', or the
* m x (n - 1) matrix C2 if SIDE = 'R'.
*
* On exit, rows 2:m of P*C if SIDE = 'L', or columns 2:m of C*P
* if SIDE = 'R'.
*
* LDC (input) INTEGER
* The leading dimension of the arrays C1 and C2. LDC >= (1,M).
*
* WORK (workspace) DOUBLE PRECISION array, dimension
* (N) if SIDE = 'L'
* (M) if SIDE = 'R'
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param m
* @param n
* @param v
* @param _v_offset
* @param incv
* @param tau
* @param c1
* @param _c1_offset
* @param c2
* @param _c2_offset
* @param Ldc
* @param work
* @param _work_offset
*
*/
abstract public void dlatzm(java.lang.String side, int m, int n, double[] v, int _v_offset, int incv, double tau, double[] c1, int _c1_offset, double[] c2, int _c2_offset, int Ldc, double[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* DLAUU2 computes the product U * U' or L' * L, where the triangular
* factor U or L is stored in the upper or lower triangular part of
* the array A.
*
* If UPLO = 'U' or 'u' then the upper triangle of the result is stored,
* overwriting the factor U in A.
* If UPLO = 'L' or 'l' then the lower triangle of the result is stored,
* overwriting the factor L in A.
*
* This is the unblocked form of the algorithm, calling Level 2 BLAS.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the triangular factor stored in the array A
* is upper or lower triangular:
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the triangular factor U or L. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the triangular factor U or L.
* On exit, if UPLO = 'U', the upper triangle of A is
* overwritten with the upper triangle of the product U * U';
* if UPLO = 'L', the lower triangle of A is overwritten with
* the lower triangle of the product L' * L.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param lda
* @param info
*
*/
abstract public void dlauu2(java.lang.String uplo, int n, double[] a, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAUU2 computes the product U * U' or L' * L, where the triangular
* factor U or L is stored in the upper or lower triangular part of
* the array A.
*
* If UPLO = 'U' or 'u' then the upper triangle of the result is stored,
* overwriting the factor U in A.
* If UPLO = 'L' or 'l' then the lower triangle of the result is stored,
* overwriting the factor L in A.
*
* This is the unblocked form of the algorithm, calling Level 2 BLAS.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the triangular factor stored in the array A
* is upper or lower triangular:
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the triangular factor U or L. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the triangular factor U or L.
* On exit, if UPLO = 'U', the upper triangle of A is
* overwritten with the upper triangle of the product U * U';
* if UPLO = 'L', the lower triangle of A is overwritten with
* the lower triangle of the product L' * L.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param info
*
*/
abstract public void dlauu2(java.lang.String uplo, int n, double[] a, int _a_offset, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAUUM computes the product U * U' or L' * L, where the triangular
* factor U or L is stored in the upper or lower triangular part of
* the array A.
*
* If UPLO = 'U' or 'u' then the upper triangle of the result is stored,
* overwriting the factor U in A.
* If UPLO = 'L' or 'l' then the lower triangle of the result is stored,
* overwriting the factor L in A.
*
* This is the blocked form of the algorithm, calling Level 3 BLAS.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the triangular factor stored in the array A
* is upper or lower triangular:
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the triangular factor U or L. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the triangular factor U or L.
* On exit, if UPLO = 'U', the upper triangle of A is
* overwritten with the upper triangle of the product U * U';
* if UPLO = 'L', the lower triangle of A is overwritten with
* the lower triangle of the product L' * L.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param lda
* @param info
*
*/
abstract public void dlauum(java.lang.String uplo, int n, double[] a, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAUUM computes the product U * U' or L' * L, where the triangular
* factor U or L is stored in the upper or lower triangular part of
* the array A.
*
* If UPLO = 'U' or 'u' then the upper triangle of the result is stored,
* overwriting the factor U in A.
* If UPLO = 'L' or 'l' then the lower triangle of the result is stored,
* overwriting the factor L in A.
*
* This is the blocked form of the algorithm, calling Level 3 BLAS.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the triangular factor stored in the array A
* is upper or lower triangular:
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the triangular factor U or L. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the triangular factor U or L.
* On exit, if UPLO = 'U', the upper triangle of A is
* overwritten with the upper triangle of the product U * U';
* if UPLO = 'L', the lower triangle of A is overwritten with
* the lower triangle of the product L' * L.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param info
*
*/
abstract public void dlauum(java.lang.String uplo, int n, double[] a, int _a_offset, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAZQ3 checks for deflation, computes a shift (TAU) and calls dqds.
* In case of failure it changes shifts, and tries again until output
* is positive.
*
* Arguments
* =========
*
* I0 (input) INTEGER
* First index.
*
* N0 (input) INTEGER
* Last index.
*
* Z (input) DOUBLE PRECISION array, dimension ( 4*N )
* Z holds the qd array.
*
* PP (input) INTEGER
* PP=0 for ping, PP=1 for pong.
*
* DMIN (output) DOUBLE PRECISION
* Minimum value of d.
*
* SIGMA (output) DOUBLE PRECISION
* Sum of shifts used in current segment.
*
* DESIG (input/output) DOUBLE PRECISION
* Lower order part of SIGMA
*
* QMAX (input) DOUBLE PRECISION
* Maximum value of q.
*
* NFAIL (output) INTEGER
* Number of times shift was too big.
*
* ITER (output) INTEGER
* Number of iterations.
*
* NDIV (output) INTEGER
* Number of divisions.
*
* IEEE (input) LOGICAL
* Flag for IEEE or non IEEE arithmetic (passed to DLASQ5).
*
* TTYPE (input/output) INTEGER
* Shift type. TTYPE is passed as an argument in order to save
* its value between calls to DLAZQ3
*
* DMIN1 (input/output) REAL
* DMIN2 (input/output) REAL
* DN (input/output) REAL
* DN1 (input/output) REAL
* DN2 (input/output) REAL
* TAU (input/output) REAL
* These are passed as arguments in order to save their values
* between calls to DLAZQ3
*
* This is a thread safe version of DLASQ3, which passes TTYPE, DMIN1,
* DMIN2, DN, DN1. DN2 and TAU through the argument list in place of
* declaring them in a SAVE statment.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param i0
* @param n0
* @param z
* @param pp
* @param dmin
* @param sigma
* @param desig
* @param qmax
* @param nfail
* @param iter
* @param ndiv
* @param ieee
* @param ttype
* @param dmin1
* @param dmin2
* @param dn
* @param dn1
* @param dn2
* @param tau
*
*/
abstract public void dlazq3(int i0, org.netlib.util.intW n0, double[] z, int pp, org.netlib.util.doubleW dmin, org.netlib.util.doubleW sigma, org.netlib.util.doubleW desig, org.netlib.util.doubleW qmax, org.netlib.util.intW nfail, org.netlib.util.intW iter, org.netlib.util.intW ndiv, boolean ieee, org.netlib.util.intW ttype, org.netlib.util.doubleW dmin1, org.netlib.util.doubleW dmin2, org.netlib.util.doubleW dn, org.netlib.util.doubleW dn1, org.netlib.util.doubleW dn2, org.netlib.util.doubleW tau);
/**
*
* ..
*
* Purpose
* =======
*
* DLAZQ3 checks for deflation, computes a shift (TAU) and calls dqds.
* In case of failure it changes shifts, and tries again until output
* is positive.
*
* Arguments
* =========
*
* I0 (input) INTEGER
* First index.
*
* N0 (input) INTEGER
* Last index.
*
* Z (input) DOUBLE PRECISION array, dimension ( 4*N )
* Z holds the qd array.
*
* PP (input) INTEGER
* PP=0 for ping, PP=1 for pong.
*
* DMIN (output) DOUBLE PRECISION
* Minimum value of d.
*
* SIGMA (output) DOUBLE PRECISION
* Sum of shifts used in current segment.
*
* DESIG (input/output) DOUBLE PRECISION
* Lower order part of SIGMA
*
* QMAX (input) DOUBLE PRECISION
* Maximum value of q.
*
* NFAIL (output) INTEGER
* Number of times shift was too big.
*
* ITER (output) INTEGER
* Number of iterations.
*
* NDIV (output) INTEGER
* Number of divisions.
*
* IEEE (input) LOGICAL
* Flag for IEEE or non IEEE arithmetic (passed to DLASQ5).
*
* TTYPE (input/output) INTEGER
* Shift type. TTYPE is passed as an argument in order to save
* its value between calls to DLAZQ3
*
* DMIN1 (input/output) REAL
* DMIN2 (input/output) REAL
* DN (input/output) REAL
* DN1 (input/output) REAL
* DN2 (input/output) REAL
* TAU (input/output) REAL
* These are passed as arguments in order to save their values
* between calls to DLAZQ3
*
* This is a thread safe version of DLASQ3, which passes TTYPE, DMIN1,
* DMIN2, DN, DN1. DN2 and TAU through the argument list in place of
* declaring them in a SAVE statment.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param i0
* @param n0
* @param z
* @param _z_offset
* @param pp
* @param dmin
* @param sigma
* @param desig
* @param qmax
* @param nfail
* @param iter
* @param ndiv
* @param ieee
* @param ttype
* @param dmin1
* @param dmin2
* @param dn
* @param dn1
* @param dn2
* @param tau
*
*/
abstract public void dlazq3(int i0, org.netlib.util.intW n0, double[] z, int _z_offset, int pp, org.netlib.util.doubleW dmin, org.netlib.util.doubleW sigma, org.netlib.util.doubleW desig, org.netlib.util.doubleW qmax, org.netlib.util.intW nfail, org.netlib.util.intW iter, org.netlib.util.intW ndiv, boolean ieee, org.netlib.util.intW ttype, org.netlib.util.doubleW dmin1, org.netlib.util.doubleW dmin2, org.netlib.util.doubleW dn, org.netlib.util.doubleW dn1, org.netlib.util.doubleW dn2, org.netlib.util.doubleW tau);
/**
*
* ..
*
* Purpose
* =======
*
* DLAZQ4 computes an approximation TAU to the smallest eigenvalue
* using values of d from the previous transform.
*
* I0 (input) INTEGER
* First index.
*
* N0 (input) INTEGER
* Last index.
*
* Z (input) DOUBLE PRECISION array, dimension ( 4*N )
* Z holds the qd array.
*
* PP (input) INTEGER
* PP=0 for ping, PP=1 for pong.
*
* N0IN (input) INTEGER
* The value of N0 at start of EIGTEST.
*
* DMIN (input) DOUBLE PRECISION
* Minimum value of d.
*
* DMIN1 (input) DOUBLE PRECISION
* Minimum value of d, excluding D( N0 ).
*
* DMIN2 (input) DOUBLE PRECISION
* Minimum value of d, excluding D( N0 ) and D( N0-1 ).
*
* DN (input) DOUBLE PRECISION
* d(N)
*
* DN1 (input) DOUBLE PRECISION
* d(N-1)
*
* DN2 (input) DOUBLE PRECISION
* d(N-2)
*
* TAU (output) DOUBLE PRECISION
* This is the shift.
*
* TTYPE (output) INTEGER
* Shift type.
*
* G (input/output) DOUBLE PRECISION
* G is passed as an argument in order to save its value between
* calls to DLAZQ4
*
* Further Details
* ===============
* CNST1 = 9/16
*
* This is a thread safe version of DLASQ4, which passes G through the
* argument list in place of declaring G in a SAVE statment.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param i0
* @param n0
* @param z
* @param pp
* @param n0in
* @param dmin
* @param dmin1
* @param dmin2
* @param dn
* @param dn1
* @param dn2
* @param tau
* @param ttype
* @param g
*
*/
abstract public void dlazq4(int i0, int n0, double[] z, int pp, int n0in, double dmin, double dmin1, double dmin2, double dn, double dn1, double dn2, org.netlib.util.doubleW tau, org.netlib.util.intW ttype, org.netlib.util.doubleW g);
/**
*
* ..
*
* Purpose
* =======
*
* DLAZQ4 computes an approximation TAU to the smallest eigenvalue
* using values of d from the previous transform.
*
* I0 (input) INTEGER
* First index.
*
* N0 (input) INTEGER
* Last index.
*
* Z (input) DOUBLE PRECISION array, dimension ( 4*N )
* Z holds the qd array.
*
* PP (input) INTEGER
* PP=0 for ping, PP=1 for pong.
*
* N0IN (input) INTEGER
* The value of N0 at start of EIGTEST.
*
* DMIN (input) DOUBLE PRECISION
* Minimum value of d.
*
* DMIN1 (input) DOUBLE PRECISION
* Minimum value of d, excluding D( N0 ).
*
* DMIN2 (input) DOUBLE PRECISION
* Minimum value of d, excluding D( N0 ) and D( N0-1 ).
*
* DN (input) DOUBLE PRECISION
* d(N)
*
* DN1 (input) DOUBLE PRECISION
* d(N-1)
*
* DN2 (input) DOUBLE PRECISION
* d(N-2)
*
* TAU (output) DOUBLE PRECISION
* This is the shift.
*
* TTYPE (output) INTEGER
* Shift type.
*
* G (input/output) DOUBLE PRECISION
* G is passed as an argument in order to save its value between
* calls to DLAZQ4
*
* Further Details
* ===============
* CNST1 = 9/16
*
* This is a thread safe version of DLASQ4, which passes G through the
* argument list in place of declaring G in a SAVE statment.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param i0
* @param n0
* @param z
* @param _z_offset
* @param pp
* @param n0in
* @param dmin
* @param dmin1
* @param dmin2
* @param dn
* @param dn1
* @param dn2
* @param tau
* @param ttype
* @param g
*
*/
abstract public void dlazq4(int i0, int n0, double[] z, int _z_offset, int pp, int n0in, double dmin, double dmin1, double dmin2, double dn, double dn1, double dn2, org.netlib.util.doubleW tau, org.netlib.util.intW ttype, org.netlib.util.doubleW g);
/**
*
* ..
*
* Purpose
* =======
*
* DOPGTR generates a real orthogonal matrix Q which is defined as the
* product of n-1 elementary reflectors H(i) of order n, as returned by
* DSPTRD using packed storage:
*
* if UPLO = 'U', Q = H(n-1) . . . H(2) H(1),
*
* if UPLO = 'L', Q = H(1) H(2) . . . H(n-1).
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangular packed storage used in previous
* call to DSPTRD;
* = 'L': Lower triangular packed storage used in previous
* call to DSPTRD.
*
* N (input) INTEGER
* The order of the matrix Q. N >= 0.
*
* AP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The vectors which define the elementary reflectors, as
* returned by DSPTRD.
*
* TAU (input) DOUBLE PRECISION array, dimension (N-1)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DSPTRD.
*
* Q (output) DOUBLE PRECISION array, dimension (LDQ,N)
* The N-by-N orthogonal matrix Q.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (N-1)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param tau
* @param q
* @param ldq
* @param work
* @param info
*
*/
abstract public void dopgtr(java.lang.String uplo, int n, double[] ap, double[] tau, double[] q, int ldq, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DOPGTR generates a real orthogonal matrix Q which is defined as the
* product of n-1 elementary reflectors H(i) of order n, as returned by
* DSPTRD using packed storage:
*
* if UPLO = 'U', Q = H(n-1) . . . H(2) H(1),
*
* if UPLO = 'L', Q = H(1) H(2) . . . H(n-1).
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangular packed storage used in previous
* call to DSPTRD;
* = 'L': Lower triangular packed storage used in previous
* call to DSPTRD.
*
* N (input) INTEGER
* The order of the matrix Q. N >= 0.
*
* AP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The vectors which define the elementary reflectors, as
* returned by DSPTRD.
*
* TAU (input) DOUBLE PRECISION array, dimension (N-1)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DSPTRD.
*
* Q (output) DOUBLE PRECISION array, dimension (LDQ,N)
* The N-by-N orthogonal matrix Q.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (N-1)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param tau
* @param _tau_offset
* @param q
* @param _q_offset
* @param ldq
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dopgtr(java.lang.String uplo, int n, double[] ap, int _ap_offset, double[] tau, int _tau_offset, double[] q, int _q_offset, int ldq, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DOPMTR overwrites the general real M-by-N matrix C with
*
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* where Q is a real orthogonal matrix of order nq, with nq = m if
* SIDE = 'L' and nq = n if SIDE = 'R'. Q is defined as the product of
* nq-1 elementary reflectors, as returned by DSPTRD using packed
* storage:
*
* if UPLO = 'U', Q = H(nq-1) . . . H(2) H(1);
*
* if UPLO = 'L', Q = H(1) H(2) . . . H(nq-1).
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q**T from the Left;
* = 'R': apply Q or Q**T from the Right.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangular packed storage used in previous
* call to DSPTRD;
* = 'L': Lower triangular packed storage used in previous
* call to DSPTRD.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q;
* = 'T': Transpose, apply Q**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* AP (input) DOUBLE PRECISION array, dimension
* (M*(M+1)/2) if SIDE = 'L'
* (N*(N+1)/2) if SIDE = 'R'
* The vectors which define the elementary reflectors, as
* returned by DSPTRD. AP is modified by the routine but
* restored on exit.
*
* TAU (input) DOUBLE PRECISION array, dimension (M-1) if SIDE = 'L'
* or (N-1) if SIDE = 'R'
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DSPTRD.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**T*C or C*Q**T or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) DOUBLE PRECISION array, dimension
* (N) if SIDE = 'L'
* (M) if SIDE = 'R'
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param uplo
* @param trans
* @param m
* @param n
* @param ap
* @param tau
* @param c
* @param Ldc
* @param work
* @param info
*
*/
abstract public void dopmtr(java.lang.String side, java.lang.String uplo, java.lang.String trans, int m, int n, double[] ap, double[] tau, double[] c, int Ldc, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DOPMTR overwrites the general real M-by-N matrix C with
*
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* where Q is a real orthogonal matrix of order nq, with nq = m if
* SIDE = 'L' and nq = n if SIDE = 'R'. Q is defined as the product of
* nq-1 elementary reflectors, as returned by DSPTRD using packed
* storage:
*
* if UPLO = 'U', Q = H(nq-1) . . . H(2) H(1);
*
* if UPLO = 'L', Q = H(1) H(2) . . . H(nq-1).
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q**T from the Left;
* = 'R': apply Q or Q**T from the Right.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangular packed storage used in previous
* call to DSPTRD;
* = 'L': Lower triangular packed storage used in previous
* call to DSPTRD.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q;
* = 'T': Transpose, apply Q**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* AP (input) DOUBLE PRECISION array, dimension
* (M*(M+1)/2) if SIDE = 'L'
* (N*(N+1)/2) if SIDE = 'R'
* The vectors which define the elementary reflectors, as
* returned by DSPTRD. AP is modified by the routine but
* restored on exit.
*
* TAU (input) DOUBLE PRECISION array, dimension (M-1) if SIDE = 'L'
* or (N-1) if SIDE = 'R'
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DSPTRD.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**T*C or C*Q**T or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) DOUBLE PRECISION array, dimension
* (N) if SIDE = 'L'
* (M) if SIDE = 'R'
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param uplo
* @param trans
* @param m
* @param n
* @param ap
* @param _ap_offset
* @param tau
* @param _tau_offset
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dopmtr(java.lang.String side, java.lang.String uplo, java.lang.String trans, int m, int n, double[] ap, int _ap_offset, double[] tau, int _tau_offset, double[] c, int _c_offset, int Ldc, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORG2L generates an m by n real matrix Q with orthonormal columns,
* which is defined as the last n columns of a product of k elementary
* reflectors of order m
*
* Q = H(k) . . . H(2) H(1)
*
* as returned by DGEQLF.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix Q. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q. M >= N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines the
* matrix Q. N >= K >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the (n-k+i)-th column must contain the vector which
* defines the elementary reflector H(i), for i = 1,2,...,k, as
* returned by DGEQLF in the last k columns of its array
* argument A.
* On exit, the m by n matrix Q.
*
* LDA (input) INTEGER
* The first dimension of the array A. LDA >= max(1,M).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGEQLF.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument has an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param work
* @param info
*
*/
abstract public void dorg2l(int m, int n, int k, double[] a, int lda, double[] tau, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORG2L generates an m by n real matrix Q with orthonormal columns,
* which is defined as the last n columns of a product of k elementary
* reflectors of order m
*
* Q = H(k) . . . H(2) H(1)
*
* as returned by DGEQLF.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix Q. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q. M >= N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines the
* matrix Q. N >= K >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the (n-k+i)-th column must contain the vector which
* defines the elementary reflector H(i), for i = 1,2,...,k, as
* returned by DGEQLF in the last k columns of its array
* argument A.
* On exit, the m by n matrix Q.
*
* LDA (input) INTEGER
* The first dimension of the array A. LDA >= max(1,M).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGEQLF.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument has an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dorg2l(int m, int n, int k, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORG2R generates an m by n real matrix Q with orthonormal columns,
* which is defined as the first n columns of a product of k elementary
* reflectors of order m
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by DGEQRF.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix Q. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q. M >= N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines the
* matrix Q. N >= K >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the i-th column must contain the vector which
* defines the elementary reflector H(i), for i = 1,2,...,k, as
* returned by DGEQRF in the first k columns of its array
* argument A.
* On exit, the m-by-n matrix Q.
*
* LDA (input) INTEGER
* The first dimension of the array A. LDA >= max(1,M).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGEQRF.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument has an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param work
* @param info
*
*/
abstract public void dorg2r(int m, int n, int k, double[] a, int lda, double[] tau, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORG2R generates an m by n real matrix Q with orthonormal columns,
* which is defined as the first n columns of a product of k elementary
* reflectors of order m
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by DGEQRF.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix Q. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q. M >= N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines the
* matrix Q. N >= K >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the i-th column must contain the vector which
* defines the elementary reflector H(i), for i = 1,2,...,k, as
* returned by DGEQRF in the first k columns of its array
* argument A.
* On exit, the m-by-n matrix Q.
*
* LDA (input) INTEGER
* The first dimension of the array A. LDA >= max(1,M).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGEQRF.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument has an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dorg2r(int m, int n, int k, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORGBR generates one of the real orthogonal matrices Q or P**T
* determined by DGEBRD when reducing a real matrix A to bidiagonal
* form: A = Q * B * P**T. Q and P**T are defined as products of
* elementary reflectors H(i) or G(i) respectively.
*
* If VECT = 'Q', A is assumed to have been an M-by-K matrix, and Q
* is of order M:
* if m >= k, Q = H(1) H(2) . . . H(k) and DORGBR returns the first n
* columns of Q, where m >= n >= k;
* if m < k, Q = H(1) H(2) . . . H(m-1) and DORGBR returns Q as an
* M-by-M matrix.
*
* If VECT = 'P', A is assumed to have been a K-by-N matrix, and P**T
* is of order N:
* if k < n, P**T = G(k) . . . G(2) G(1) and DORGBR returns the first m
* rows of P**T, where n >= m >= k;
* if k >= n, P**T = G(n-1) . . . G(2) G(1) and DORGBR returns P**T as
* an N-by-N matrix.
*
* Arguments
* =========
*
* VECT (input) CHARACTER*1
* Specifies whether the matrix Q or the matrix P**T is
* required, as defined in the transformation applied by DGEBRD:
* = 'Q': generate Q;
* = 'P': generate P**T.
*
* M (input) INTEGER
* The number of rows of the matrix Q or P**T to be returned.
* M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q or P**T to be returned.
* N >= 0.
* If VECT = 'Q', M >= N >= min(M,K);
* if VECT = 'P', N >= M >= min(N,K).
*
* K (input) INTEGER
* If VECT = 'Q', the number of columns in the original M-by-K
* matrix reduced by DGEBRD.
* If VECT = 'P', the number of rows in the original K-by-N
* matrix reduced by DGEBRD.
* K >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the vectors which define the elementary reflectors,
* as returned by DGEBRD.
* On exit, the M-by-N matrix Q or P**T.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (input) DOUBLE PRECISION array, dimension
* (min(M,K)) if VECT = 'Q'
* (min(N,K)) if VECT = 'P'
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i) or G(i), which determines Q or P**T, as
* returned by DGEBRD in its array argument TAUQ or TAUP.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,min(M,N)).
* For optimum performance LWORK >= min(M,N)*NB, where NB
* is the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param vect
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param work
* @param lwork
* @param info
*
*/
abstract public void dorgbr(java.lang.String vect, int m, int n, int k, double[] a, int lda, double[] tau, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORGBR generates one of the real orthogonal matrices Q or P**T
* determined by DGEBRD when reducing a real matrix A to bidiagonal
* form: A = Q * B * P**T. Q and P**T are defined as products of
* elementary reflectors H(i) or G(i) respectively.
*
* If VECT = 'Q', A is assumed to have been an M-by-K matrix, and Q
* is of order M:
* if m >= k, Q = H(1) H(2) . . . H(k) and DORGBR returns the first n
* columns of Q, where m >= n >= k;
* if m < k, Q = H(1) H(2) . . . H(m-1) and DORGBR returns Q as an
* M-by-M matrix.
*
* If VECT = 'P', A is assumed to have been a K-by-N matrix, and P**T
* is of order N:
* if k < n, P**T = G(k) . . . G(2) G(1) and DORGBR returns the first m
* rows of P**T, where n >= m >= k;
* if k >= n, P**T = G(n-1) . . . G(2) G(1) and DORGBR returns P**T as
* an N-by-N matrix.
*
* Arguments
* =========
*
* VECT (input) CHARACTER*1
* Specifies whether the matrix Q or the matrix P**T is
* required, as defined in the transformation applied by DGEBRD:
* = 'Q': generate Q;
* = 'P': generate P**T.
*
* M (input) INTEGER
* The number of rows of the matrix Q or P**T to be returned.
* M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q or P**T to be returned.
* N >= 0.
* If VECT = 'Q', M >= N >= min(M,K);
* if VECT = 'P', N >= M >= min(N,K).
*
* K (input) INTEGER
* If VECT = 'Q', the number of columns in the original M-by-K
* matrix reduced by DGEBRD.
* If VECT = 'P', the number of rows in the original K-by-N
* matrix reduced by DGEBRD.
* K >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the vectors which define the elementary reflectors,
* as returned by DGEBRD.
* On exit, the M-by-N matrix Q or P**T.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (input) DOUBLE PRECISION array, dimension
* (min(M,K)) if VECT = 'Q'
* (min(N,K)) if VECT = 'P'
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i) or G(i), which determines Q or P**T, as
* returned by DGEBRD in its array argument TAUQ or TAUP.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,min(M,N)).
* For optimum performance LWORK >= min(M,N)*NB, where NB
* is the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param vect
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dorgbr(java.lang.String vect, int m, int n, int k, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORGHR generates a real orthogonal matrix Q which is defined as the
* product of IHI-ILO elementary reflectors of order N, as returned by
* DGEHRD:
*
* Q = H(ilo) H(ilo+1) . . . H(ihi-1).
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix Q. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* ILO and IHI must have the same values as in the previous call
* of DGEHRD. Q is equal to the unit matrix except in the
* submatrix Q(ilo+1:ihi,ilo+1:ihi).
* 1 <= ILO <= IHI <= N, if N > 0; ILO=1 and IHI=0, if N=0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the vectors which define the elementary reflectors,
* as returned by DGEHRD.
* On exit, the N-by-N orthogonal matrix Q.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* TAU (input) DOUBLE PRECISION array, dimension (N-1)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGEHRD.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= IHI-ILO.
* For optimum performance LWORK >= (IHI-ILO)*NB, where NB is
* the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param ilo
* @param ihi
* @param a
* @param lda
* @param tau
* @param work
* @param lwork
* @param info
*
*/
abstract public void dorghr(int n, int ilo, int ihi, double[] a, int lda, double[] tau, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORGHR generates a real orthogonal matrix Q which is defined as the
* product of IHI-ILO elementary reflectors of order N, as returned by
* DGEHRD:
*
* Q = H(ilo) H(ilo+1) . . . H(ihi-1).
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix Q. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* ILO and IHI must have the same values as in the previous call
* of DGEHRD. Q is equal to the unit matrix except in the
* submatrix Q(ilo+1:ihi,ilo+1:ihi).
* 1 <= ILO <= IHI <= N, if N > 0; ILO=1 and IHI=0, if N=0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the vectors which define the elementary reflectors,
* as returned by DGEHRD.
* On exit, the N-by-N orthogonal matrix Q.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* TAU (input) DOUBLE PRECISION array, dimension (N-1)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGEHRD.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= IHI-ILO.
* For optimum performance LWORK >= (IHI-ILO)*NB, where NB is
* the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param ilo
* @param ihi
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dorghr(int n, int ilo, int ihi, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORGL2 generates an m by n real matrix Q with orthonormal rows,
* which is defined as the first m rows of a product of k elementary
* reflectors of order n
*
* Q = H(k) . . . H(2) H(1)
*
* as returned by DGELQF.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix Q. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q. N >= M.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines the
* matrix Q. M >= K >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the i-th row must contain the vector which defines
* the elementary reflector H(i), for i = 1,2,...,k, as returned
* by DGELQF in the first k rows of its array argument A.
* On exit, the m-by-n matrix Q.
*
* LDA (input) INTEGER
* The first dimension of the array A. LDA >= max(1,M).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGELQF.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (M)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument has an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param work
* @param info
*
*/
abstract public void dorgl2(int m, int n, int k, double[] a, int lda, double[] tau, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORGL2 generates an m by n real matrix Q with orthonormal rows,
* which is defined as the first m rows of a product of k elementary
* reflectors of order n
*
* Q = H(k) . . . H(2) H(1)
*
* as returned by DGELQF.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix Q. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q. N >= M.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines the
* matrix Q. M >= K >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the i-th row must contain the vector which defines
* the elementary reflector H(i), for i = 1,2,...,k, as returned
* by DGELQF in the first k rows of its array argument A.
* On exit, the m-by-n matrix Q.
*
* LDA (input) INTEGER
* The first dimension of the array A. LDA >= max(1,M).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGELQF.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (M)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument has an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dorgl2(int m, int n, int k, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORGLQ generates an M-by-N real matrix Q with orthonormal rows,
* which is defined as the first M rows of a product of K elementary
* reflectors of order N
*
* Q = H(k) . . . H(2) H(1)
*
* as returned by DGELQF.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix Q. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q. N >= M.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines the
* matrix Q. M >= K >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the i-th row must contain the vector which defines
* the elementary reflector H(i), for i = 1,2,...,k, as returned
* by DGELQF in the first k rows of its array argument A.
* On exit, the M-by-N matrix Q.
*
* LDA (input) INTEGER
* The first dimension of the array A. LDA >= max(1,M).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGELQF.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,M).
* For optimum performance LWORK >= M*NB, where NB is
* the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument has an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param work
* @param lwork
* @param info
*
*/
abstract public void dorglq(int m, int n, int k, double[] a, int lda, double[] tau, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORGLQ generates an M-by-N real matrix Q with orthonormal rows,
* which is defined as the first M rows of a product of K elementary
* reflectors of order N
*
* Q = H(k) . . . H(2) H(1)
*
* as returned by DGELQF.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix Q. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q. N >= M.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines the
* matrix Q. M >= K >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the i-th row must contain the vector which defines
* the elementary reflector H(i), for i = 1,2,...,k, as returned
* by DGELQF in the first k rows of its array argument A.
* On exit, the M-by-N matrix Q.
*
* LDA (input) INTEGER
* The first dimension of the array A. LDA >= max(1,M).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGELQF.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,M).
* For optimum performance LWORK >= M*NB, where NB is
* the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument has an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dorglq(int m, int n, int k, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORGQL generates an M-by-N real matrix Q with orthonormal columns,
* which is defined as the last N columns of a product of K elementary
* reflectors of order M
*
* Q = H(k) . . . H(2) H(1)
*
* as returned by DGEQLF.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix Q. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q. M >= N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines the
* matrix Q. N >= K >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the (n-k+i)-th column must contain the vector which
* defines the elementary reflector H(i), for i = 1,2,...,k, as
* returned by DGEQLF in the last k columns of its array
* argument A.
* On exit, the M-by-N matrix Q.
*
* LDA (input) INTEGER
* The first dimension of the array A. LDA >= max(1,M).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGEQLF.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N).
* For optimum performance LWORK >= N*NB, where NB is the
* optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument has an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param work
* @param lwork
* @param info
*
*/
abstract public void dorgql(int m, int n, int k, double[] a, int lda, double[] tau, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORGQL generates an M-by-N real matrix Q with orthonormal columns,
* which is defined as the last N columns of a product of K elementary
* reflectors of order M
*
* Q = H(k) . . . H(2) H(1)
*
* as returned by DGEQLF.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix Q. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q. M >= N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines the
* matrix Q. N >= K >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the (n-k+i)-th column must contain the vector which
* defines the elementary reflector H(i), for i = 1,2,...,k, as
* returned by DGEQLF in the last k columns of its array
* argument A.
* On exit, the M-by-N matrix Q.
*
* LDA (input) INTEGER
* The first dimension of the array A. LDA >= max(1,M).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGEQLF.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N).
* For optimum performance LWORK >= N*NB, where NB is the
* optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument has an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dorgql(int m, int n, int k, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORGQR generates an M-by-N real matrix Q with orthonormal columns,
* which is defined as the first N columns of a product of K elementary
* reflectors of order M
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by DGEQRF.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix Q. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q. M >= N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines the
* matrix Q. N >= K >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the i-th column must contain the vector which
* defines the elementary reflector H(i), for i = 1,2,...,k, as
* returned by DGEQRF in the first k columns of its array
* argument A.
* On exit, the M-by-N matrix Q.
*
* LDA (input) INTEGER
* The first dimension of the array A. LDA >= max(1,M).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGEQRF.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N).
* For optimum performance LWORK >= N*NB, where NB is the
* optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument has an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param work
* @param lwork
* @param info
*
*/
abstract public void dorgqr(int m, int n, int k, double[] a, int lda, double[] tau, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORGQR generates an M-by-N real matrix Q with orthonormal columns,
* which is defined as the first N columns of a product of K elementary
* reflectors of order M
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by DGEQRF.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix Q. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q. M >= N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines the
* matrix Q. N >= K >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the i-th column must contain the vector which
* defines the elementary reflector H(i), for i = 1,2,...,k, as
* returned by DGEQRF in the first k columns of its array
* argument A.
* On exit, the M-by-N matrix Q.
*
* LDA (input) INTEGER
* The first dimension of the array A. LDA >= max(1,M).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGEQRF.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N).
* For optimum performance LWORK >= N*NB, where NB is the
* optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument has an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dorgqr(int m, int n, int k, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORGR2 generates an m by n real matrix Q with orthonormal rows,
* which is defined as the last m rows of a product of k elementary
* reflectors of order n
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by DGERQF.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix Q. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q. N >= M.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines the
* matrix Q. M >= K >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the (m-k+i)-th row must contain the vector which
* defines the elementary reflector H(i), for i = 1,2,...,k, as
* returned by DGERQF in the last k rows of its array argument
* A.
* On exit, the m by n matrix Q.
*
* LDA (input) INTEGER
* The first dimension of the array A. LDA >= max(1,M).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGERQF.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (M)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument has an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param work
* @param info
*
*/
abstract public void dorgr2(int m, int n, int k, double[] a, int lda, double[] tau, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORGR2 generates an m by n real matrix Q with orthonormal rows,
* which is defined as the last m rows of a product of k elementary
* reflectors of order n
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by DGERQF.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix Q. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q. N >= M.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines the
* matrix Q. M >= K >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the (m-k+i)-th row must contain the vector which
* defines the elementary reflector H(i), for i = 1,2,...,k, as
* returned by DGERQF in the last k rows of its array argument
* A.
* On exit, the m by n matrix Q.
*
* LDA (input) INTEGER
* The first dimension of the array A. LDA >= max(1,M).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGERQF.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (M)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument has an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dorgr2(int m, int n, int k, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORGRQ generates an M-by-N real matrix Q with orthonormal rows,
* which is defined as the last M rows of a product of K elementary
* reflectors of order N
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by DGERQF.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix Q. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q. N >= M.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines the
* matrix Q. M >= K >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the (m-k+i)-th row must contain the vector which
* defines the elementary reflector H(i), for i = 1,2,...,k, as
* returned by DGERQF in the last k rows of its array argument
* A.
* On exit, the M-by-N matrix Q.
*
* LDA (input) INTEGER
* The first dimension of the array A. LDA >= max(1,M).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGERQF.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,M).
* For optimum performance LWORK >= M*NB, where NB is the
* optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument has an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param work
* @param lwork
* @param info
*
*/
abstract public void dorgrq(int m, int n, int k, double[] a, int lda, double[] tau, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORGRQ generates an M-by-N real matrix Q with orthonormal rows,
* which is defined as the last M rows of a product of K elementary
* reflectors of order N
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by DGERQF.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix Q. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q. N >= M.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines the
* matrix Q. M >= K >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the (m-k+i)-th row must contain the vector which
* defines the elementary reflector H(i), for i = 1,2,...,k, as
* returned by DGERQF in the last k rows of its array argument
* A.
* On exit, the M-by-N matrix Q.
*
* LDA (input) INTEGER
* The first dimension of the array A. LDA >= max(1,M).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGERQF.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,M).
* For optimum performance LWORK >= M*NB, where NB is the
* optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument has an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dorgrq(int m, int n, int k, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORGTR generates a real orthogonal matrix Q which is defined as the
* product of n-1 elementary reflectors of order N, as returned by
* DSYTRD:
*
* if UPLO = 'U', Q = H(n-1) . . . H(2) H(1),
*
* if UPLO = 'L', Q = H(1) H(2) . . . H(n-1).
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A contains elementary reflectors
* from DSYTRD;
* = 'L': Lower triangle of A contains elementary reflectors
* from DSYTRD.
*
* N (input) INTEGER
* The order of the matrix Q. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the vectors which define the elementary reflectors,
* as returned by DSYTRD.
* On exit, the N-by-N orthogonal matrix Q.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* TAU (input) DOUBLE PRECISION array, dimension (N-1)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DSYTRD.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N-1).
* For optimum performance LWORK >= (N-1)*NB, where NB is
* the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param lda
* @param tau
* @param work
* @param lwork
* @param info
*
*/
abstract public void dorgtr(java.lang.String uplo, int n, double[] a, int lda, double[] tau, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORGTR generates a real orthogonal matrix Q which is defined as the
* product of n-1 elementary reflectors of order N, as returned by
* DSYTRD:
*
* if UPLO = 'U', Q = H(n-1) . . . H(2) H(1),
*
* if UPLO = 'L', Q = H(1) H(2) . . . H(n-1).
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A contains elementary reflectors
* from DSYTRD;
* = 'L': Lower triangle of A contains elementary reflectors
* from DSYTRD.
*
* N (input) INTEGER
* The order of the matrix Q. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the vectors which define the elementary reflectors,
* as returned by DSYTRD.
* On exit, the N-by-N orthogonal matrix Q.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* TAU (input) DOUBLE PRECISION array, dimension (N-1)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DSYTRD.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N-1).
* For optimum performance LWORK >= (N-1)*NB, where NB is
* the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dorgtr(java.lang.String uplo, int n, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORM2L overwrites the general real m by n matrix C with
*
* Q * C if SIDE = 'L' and TRANS = 'N', or
*
* Q'* C if SIDE = 'L' and TRANS = 'T', or
*
* C * Q if SIDE = 'R' and TRANS = 'N', or
*
* C * Q' if SIDE = 'R' and TRANS = 'T',
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(k) . . . H(2) H(1)
*
* as returned by DGEQLF. Q is of order m if SIDE = 'L' and of order n
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q' from the Left
* = 'R': apply Q or Q' from the Right
*
* TRANS (input) CHARACTER*1
* = 'N': apply Q (No transpose)
* = 'T': apply Q' (Transpose)
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,K)
* The i-th column must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* DGEQLF in the last k columns of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A.
* If SIDE = 'L', LDA >= max(1,M);
* if SIDE = 'R', LDA >= max(1,N).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGEQLF.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the m by n matrix C.
* On exit, C is overwritten by Q*C or Q'*C or C*Q' or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) DOUBLE PRECISION array, dimension
* (N) if SIDE = 'L',
* (M) if SIDE = 'R'
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param c
* @param Ldc
* @param work
* @param info
*
*/
abstract public void dorm2l(java.lang.String side, java.lang.String trans, int m, int n, int k, double[] a, int lda, double[] tau, double[] c, int Ldc, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORM2L overwrites the general real m by n matrix C with
*
* Q * C if SIDE = 'L' and TRANS = 'N', or
*
* Q'* C if SIDE = 'L' and TRANS = 'T', or
*
* C * Q if SIDE = 'R' and TRANS = 'N', or
*
* C * Q' if SIDE = 'R' and TRANS = 'T',
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(k) . . . H(2) H(1)
*
* as returned by DGEQLF. Q is of order m if SIDE = 'L' and of order n
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q' from the Left
* = 'R': apply Q or Q' from the Right
*
* TRANS (input) CHARACTER*1
* = 'N': apply Q (No transpose)
* = 'T': apply Q' (Transpose)
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,K)
* The i-th column must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* DGEQLF in the last k columns of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A.
* If SIDE = 'L', LDA >= max(1,M);
* if SIDE = 'R', LDA >= max(1,N).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGEQLF.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the m by n matrix C.
* On exit, C is overwritten by Q*C or Q'*C or C*Q' or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) DOUBLE PRECISION array, dimension
* (N) if SIDE = 'L',
* (M) if SIDE = 'R'
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dorm2l(java.lang.String side, java.lang.String trans, int m, int n, int k, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] c, int _c_offset, int Ldc, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORM2R overwrites the general real m by n matrix C with
*
* Q * C if SIDE = 'L' and TRANS = 'N', or
*
* Q'* C if SIDE = 'L' and TRANS = 'T', or
*
* C * Q if SIDE = 'R' and TRANS = 'N', or
*
* C * Q' if SIDE = 'R' and TRANS = 'T',
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by DGEQRF. Q is of order m if SIDE = 'L' and of order n
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q' from the Left
* = 'R': apply Q or Q' from the Right
*
* TRANS (input) CHARACTER*1
* = 'N': apply Q (No transpose)
* = 'T': apply Q' (Transpose)
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,K)
* The i-th column must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* DGEQRF in the first k columns of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A.
* If SIDE = 'L', LDA >= max(1,M);
* if SIDE = 'R', LDA >= max(1,N).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGEQRF.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the m by n matrix C.
* On exit, C is overwritten by Q*C or Q'*C or C*Q' or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) DOUBLE PRECISION array, dimension
* (N) if SIDE = 'L',
* (M) if SIDE = 'R'
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param c
* @param Ldc
* @param work
* @param info
*
*/
abstract public void dorm2r(java.lang.String side, java.lang.String trans, int m, int n, int k, double[] a, int lda, double[] tau, double[] c, int Ldc, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORM2R overwrites the general real m by n matrix C with
*
* Q * C if SIDE = 'L' and TRANS = 'N', or
*
* Q'* C if SIDE = 'L' and TRANS = 'T', or
*
* C * Q if SIDE = 'R' and TRANS = 'N', or
*
* C * Q' if SIDE = 'R' and TRANS = 'T',
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by DGEQRF. Q is of order m if SIDE = 'L' and of order n
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q' from the Left
* = 'R': apply Q or Q' from the Right
*
* TRANS (input) CHARACTER*1
* = 'N': apply Q (No transpose)
* = 'T': apply Q' (Transpose)
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,K)
* The i-th column must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* DGEQRF in the first k columns of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A.
* If SIDE = 'L', LDA >= max(1,M);
* if SIDE = 'R', LDA >= max(1,N).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGEQRF.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the m by n matrix C.
* On exit, C is overwritten by Q*C or Q'*C or C*Q' or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) DOUBLE PRECISION array, dimension
* (N) if SIDE = 'L',
* (M) if SIDE = 'R'
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dorm2r(java.lang.String side, java.lang.String trans, int m, int n, int k, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] c, int _c_offset, int Ldc, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* If VECT = 'Q', DORMBR overwrites the general real M-by-N matrix C
* with
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* If VECT = 'P', DORMBR overwrites the general real M-by-N matrix C
* with
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': P * C C * P
* TRANS = 'T': P**T * C C * P**T
*
* Here Q and P**T are the orthogonal matrices determined by DGEBRD when
* reducing a real matrix A to bidiagonal form: A = Q * B * P**T. Q and
* P**T are defined as products of elementary reflectors H(i) and G(i)
* respectively.
*
* Let nq = m if SIDE = 'L' and nq = n if SIDE = 'R'. Thus nq is the
* order of the orthogonal matrix Q or P**T that is applied.
*
* If VECT = 'Q', A is assumed to have been an NQ-by-K matrix:
* if nq >= k, Q = H(1) H(2) . . . H(k);
* if nq < k, Q = H(1) H(2) . . . H(nq-1).
*
* If VECT = 'P', A is assumed to have been a K-by-NQ matrix:
* if k < nq, P = G(1) G(2) . . . G(k);
* if k >= nq, P = G(1) G(2) . . . G(nq-1).
*
* Arguments
* =========
*
* VECT (input) CHARACTER*1
* = 'Q': apply Q or Q**T;
* = 'P': apply P or P**T.
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q, Q**T, P or P**T from the Left;
* = 'R': apply Q, Q**T, P or P**T from the Right.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q or P;
* = 'T': Transpose, apply Q**T or P**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* If VECT = 'Q', the number of columns in the original
* matrix reduced by DGEBRD.
* If VECT = 'P', the number of rows in the original
* matrix reduced by DGEBRD.
* K >= 0.
*
* A (input) DOUBLE PRECISION array, dimension
* (LDA,min(nq,K)) if VECT = 'Q'
* (LDA,nq) if VECT = 'P'
* The vectors which define the elementary reflectors H(i) and
* G(i), whose products determine the matrices Q and P, as
* returned by DGEBRD.
*
* LDA (input) INTEGER
* The leading dimension of the array A.
* If VECT = 'Q', LDA >= max(1,nq);
* if VECT = 'P', LDA >= max(1,min(nq,K)).
*
* TAU (input) DOUBLE PRECISION array, dimension (min(nq,K))
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i) or G(i) which determines Q or P, as returned
* by DGEBRD in the array argument TAUQ or TAUP.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**T*C or C*Q**T or C*Q
* or P*C or P**T*C or C*P or C*P**T.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If SIDE = 'L', LWORK >= max(1,N);
* if SIDE = 'R', LWORK >= max(1,M).
* For optimum performance LWORK >= N*NB if SIDE = 'L', and
* LWORK >= M*NB if SIDE = 'R', where NB is the optimal
* blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param vect
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param c
* @param Ldc
* @param work
* @param lwork
* @param info
*
*/
abstract public void dormbr(java.lang.String vect, java.lang.String side, java.lang.String trans, int m, int n, int k, double[] a, int lda, double[] tau, double[] c, int Ldc, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* If VECT = 'Q', DORMBR overwrites the general real M-by-N matrix C
* with
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* If VECT = 'P', DORMBR overwrites the general real M-by-N matrix C
* with
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': P * C C * P
* TRANS = 'T': P**T * C C * P**T
*
* Here Q and P**T are the orthogonal matrices determined by DGEBRD when
* reducing a real matrix A to bidiagonal form: A = Q * B * P**T. Q and
* P**T are defined as products of elementary reflectors H(i) and G(i)
* respectively.
*
* Let nq = m if SIDE = 'L' and nq = n if SIDE = 'R'. Thus nq is the
* order of the orthogonal matrix Q or P**T that is applied.
*
* If VECT = 'Q', A is assumed to have been an NQ-by-K matrix:
* if nq >= k, Q = H(1) H(2) . . . H(k);
* if nq < k, Q = H(1) H(2) . . . H(nq-1).
*
* If VECT = 'P', A is assumed to have been a K-by-NQ matrix:
* if k < nq, P = G(1) G(2) . . . G(k);
* if k >= nq, P = G(1) G(2) . . . G(nq-1).
*
* Arguments
* =========
*
* VECT (input) CHARACTER*1
* = 'Q': apply Q or Q**T;
* = 'P': apply P or P**T.
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q, Q**T, P or P**T from the Left;
* = 'R': apply Q, Q**T, P or P**T from the Right.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q or P;
* = 'T': Transpose, apply Q**T or P**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* If VECT = 'Q', the number of columns in the original
* matrix reduced by DGEBRD.
* If VECT = 'P', the number of rows in the original
* matrix reduced by DGEBRD.
* K >= 0.
*
* A (input) DOUBLE PRECISION array, dimension
* (LDA,min(nq,K)) if VECT = 'Q'
* (LDA,nq) if VECT = 'P'
* The vectors which define the elementary reflectors H(i) and
* G(i), whose products determine the matrices Q and P, as
* returned by DGEBRD.
*
* LDA (input) INTEGER
* The leading dimension of the array A.
* If VECT = 'Q', LDA >= max(1,nq);
* if VECT = 'P', LDA >= max(1,min(nq,K)).
*
* TAU (input) DOUBLE PRECISION array, dimension (min(nq,K))
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i) or G(i) which determines Q or P, as returned
* by DGEBRD in the array argument TAUQ or TAUP.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**T*C or C*Q**T or C*Q
* or P*C or P**T*C or C*P or C*P**T.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If SIDE = 'L', LWORK >= max(1,N);
* if SIDE = 'R', LWORK >= max(1,M).
* For optimum performance LWORK >= N*NB if SIDE = 'L', and
* LWORK >= M*NB if SIDE = 'R', where NB is the optimal
* blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param vect
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dormbr(java.lang.String vect, java.lang.String side, java.lang.String trans, int m, int n, int k, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] c, int _c_offset, int Ldc, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORMHR overwrites the general real M-by-N matrix C with
*
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* where Q is a real orthogonal matrix of order nq, with nq = m if
* SIDE = 'L' and nq = n if SIDE = 'R'. Q is defined as the product of
* IHI-ILO elementary reflectors, as returned by DGEHRD:
*
* Q = H(ilo) H(ilo+1) . . . H(ihi-1).
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q**T from the Left;
* = 'R': apply Q or Q**T from the Right.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q;
* = 'T': Transpose, apply Q**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* ILO and IHI must have the same values as in the previous call
* of DGEHRD. Q is equal to the unit matrix except in the
* submatrix Q(ilo+1:ihi,ilo+1:ihi).
* If SIDE = 'L', then 1 <= ILO <= IHI <= M, if M > 0, and
* ILO = 1 and IHI = 0, if M = 0;
* if SIDE = 'R', then 1 <= ILO <= IHI <= N, if N > 0, and
* ILO = 1 and IHI = 0, if N = 0.
*
* A (input) DOUBLE PRECISION array, dimension
* (LDA,M) if SIDE = 'L'
* (LDA,N) if SIDE = 'R'
* The vectors which define the elementary reflectors, as
* returned by DGEHRD.
*
* LDA (input) INTEGER
* The leading dimension of the array A.
* LDA >= max(1,M) if SIDE = 'L'; LDA >= max(1,N) if SIDE = 'R'.
*
* TAU (input) DOUBLE PRECISION array, dimension
* (M-1) if SIDE = 'L'
* (N-1) if SIDE = 'R'
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGEHRD.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**T*C or C*Q**T or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If SIDE = 'L', LWORK >= max(1,N);
* if SIDE = 'R', LWORK >= max(1,M).
* For optimum performance LWORK >= N*NB if SIDE = 'L', and
* LWORK >= M*NB if SIDE = 'R', where NB is the optimal
* blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param ilo
* @param ihi
* @param a
* @param lda
* @param tau
* @param c
* @param Ldc
* @param work
* @param lwork
* @param info
*
*/
abstract public void dormhr(java.lang.String side, java.lang.String trans, int m, int n, int ilo, int ihi, double[] a, int lda, double[] tau, double[] c, int Ldc, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORMHR overwrites the general real M-by-N matrix C with
*
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* where Q is a real orthogonal matrix of order nq, with nq = m if
* SIDE = 'L' and nq = n if SIDE = 'R'. Q is defined as the product of
* IHI-ILO elementary reflectors, as returned by DGEHRD:
*
* Q = H(ilo) H(ilo+1) . . . H(ihi-1).
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q**T from the Left;
* = 'R': apply Q or Q**T from the Right.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q;
* = 'T': Transpose, apply Q**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* ILO and IHI must have the same values as in the previous call
* of DGEHRD. Q is equal to the unit matrix except in the
* submatrix Q(ilo+1:ihi,ilo+1:ihi).
* If SIDE = 'L', then 1 <= ILO <= IHI <= M, if M > 0, and
* ILO = 1 and IHI = 0, if M = 0;
* if SIDE = 'R', then 1 <= ILO <= IHI <= N, if N > 0, and
* ILO = 1 and IHI = 0, if N = 0.
*
* A (input) DOUBLE PRECISION array, dimension
* (LDA,M) if SIDE = 'L'
* (LDA,N) if SIDE = 'R'
* The vectors which define the elementary reflectors, as
* returned by DGEHRD.
*
* LDA (input) INTEGER
* The leading dimension of the array A.
* LDA >= max(1,M) if SIDE = 'L'; LDA >= max(1,N) if SIDE = 'R'.
*
* TAU (input) DOUBLE PRECISION array, dimension
* (M-1) if SIDE = 'L'
* (N-1) if SIDE = 'R'
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGEHRD.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**T*C or C*Q**T or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If SIDE = 'L', LWORK >= max(1,N);
* if SIDE = 'R', LWORK >= max(1,M).
* For optimum performance LWORK >= N*NB if SIDE = 'L', and
* LWORK >= M*NB if SIDE = 'R', where NB is the optimal
* blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param ilo
* @param ihi
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dormhr(java.lang.String side, java.lang.String trans, int m, int n, int ilo, int ihi, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] c, int _c_offset, int Ldc, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORML2 overwrites the general real m by n matrix C with
*
* Q * C if SIDE = 'L' and TRANS = 'N', or
*
* Q'* C if SIDE = 'L' and TRANS = 'T', or
*
* C * Q if SIDE = 'R' and TRANS = 'N', or
*
* C * Q' if SIDE = 'R' and TRANS = 'T',
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(k) . . . H(2) H(1)
*
* as returned by DGELQF. Q is of order m if SIDE = 'L' and of order n
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q' from the Left
* = 'R': apply Q or Q' from the Right
*
* TRANS (input) CHARACTER*1
* = 'N': apply Q (No transpose)
* = 'T': apply Q' (Transpose)
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* A (input) DOUBLE PRECISION array, dimension
* (LDA,M) if SIDE = 'L',
* (LDA,N) if SIDE = 'R'
* The i-th row must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* DGELQF in the first k rows of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,K).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGELQF.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the m by n matrix C.
* On exit, C is overwritten by Q*C or Q'*C or C*Q' or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) DOUBLE PRECISION array, dimension
* (N) if SIDE = 'L',
* (M) if SIDE = 'R'
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param c
* @param Ldc
* @param work
* @param info
*
*/
abstract public void dorml2(java.lang.String side, java.lang.String trans, int m, int n, int k, double[] a, int lda, double[] tau, double[] c, int Ldc, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORML2 overwrites the general real m by n matrix C with
*
* Q * C if SIDE = 'L' and TRANS = 'N', or
*
* Q'* C if SIDE = 'L' and TRANS = 'T', or
*
* C * Q if SIDE = 'R' and TRANS = 'N', or
*
* C * Q' if SIDE = 'R' and TRANS = 'T',
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(k) . . . H(2) H(1)
*
* as returned by DGELQF. Q is of order m if SIDE = 'L' and of order n
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q' from the Left
* = 'R': apply Q or Q' from the Right
*
* TRANS (input) CHARACTER*1
* = 'N': apply Q (No transpose)
* = 'T': apply Q' (Transpose)
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* A (input) DOUBLE PRECISION array, dimension
* (LDA,M) if SIDE = 'L',
* (LDA,N) if SIDE = 'R'
* The i-th row must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* DGELQF in the first k rows of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,K).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGELQF.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the m by n matrix C.
* On exit, C is overwritten by Q*C or Q'*C or C*Q' or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) DOUBLE PRECISION array, dimension
* (N) if SIDE = 'L',
* (M) if SIDE = 'R'
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dorml2(java.lang.String side, java.lang.String trans, int m, int n, int k, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] c, int _c_offset, int Ldc, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORMLQ overwrites the general real M-by-N matrix C with
*
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(k) . . . H(2) H(1)
*
* as returned by DGELQF. Q is of order M if SIDE = 'L' and of order N
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q**T from the Left;
* = 'R': apply Q or Q**T from the Right.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q;
* = 'T': Transpose, apply Q**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* A (input) DOUBLE PRECISION array, dimension
* (LDA,M) if SIDE = 'L',
* (LDA,N) if SIDE = 'R'
* The i-th row must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* DGELQF in the first k rows of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,K).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGELQF.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**T*C or C*Q**T or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If SIDE = 'L', LWORK >= max(1,N);
* if SIDE = 'R', LWORK >= max(1,M).
* For optimum performance LWORK >= N*NB if SIDE = 'L', and
* LWORK >= M*NB if SIDE = 'R', where NB is the optimal
* blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param c
* @param Ldc
* @param work
* @param lwork
* @param info
*
*/
abstract public void dormlq(java.lang.String side, java.lang.String trans, int m, int n, int k, double[] a, int lda, double[] tau, double[] c, int Ldc, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORMLQ overwrites the general real M-by-N matrix C with
*
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(k) . . . H(2) H(1)
*
* as returned by DGELQF. Q is of order M if SIDE = 'L' and of order N
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q**T from the Left;
* = 'R': apply Q or Q**T from the Right.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q;
* = 'T': Transpose, apply Q**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* A (input) DOUBLE PRECISION array, dimension
* (LDA,M) if SIDE = 'L',
* (LDA,N) if SIDE = 'R'
* The i-th row must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* DGELQF in the first k rows of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,K).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGELQF.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**T*C or C*Q**T or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If SIDE = 'L', LWORK >= max(1,N);
* if SIDE = 'R', LWORK >= max(1,M).
* For optimum performance LWORK >= N*NB if SIDE = 'L', and
* LWORK >= M*NB if SIDE = 'R', where NB is the optimal
* blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dormlq(java.lang.String side, java.lang.String trans, int m, int n, int k, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] c, int _c_offset, int Ldc, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORMQL overwrites the general real M-by-N matrix C with
*
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(k) . . . H(2) H(1)
*
* as returned by DGEQLF. Q is of order M if SIDE = 'L' and of order N
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q**T from the Left;
* = 'R': apply Q or Q**T from the Right.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q;
* = 'T': Transpose, apply Q**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,K)
* The i-th column must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* DGEQLF in the last k columns of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A.
* If SIDE = 'L', LDA >= max(1,M);
* if SIDE = 'R', LDA >= max(1,N).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGEQLF.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**T*C or C*Q**T or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If SIDE = 'L', LWORK >= max(1,N);
* if SIDE = 'R', LWORK >= max(1,M).
* For optimum performance LWORK >= N*NB if SIDE = 'L', and
* LWORK >= M*NB if SIDE = 'R', where NB is the optimal
* blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param c
* @param Ldc
* @param work
* @param lwork
* @param info
*
*/
abstract public void dormql(java.lang.String side, java.lang.String trans, int m, int n, int k, double[] a, int lda, double[] tau, double[] c, int Ldc, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORMQL overwrites the general real M-by-N matrix C with
*
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(k) . . . H(2) H(1)
*
* as returned by DGEQLF. Q is of order M if SIDE = 'L' and of order N
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q**T from the Left;
* = 'R': apply Q or Q**T from the Right.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q;
* = 'T': Transpose, apply Q**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,K)
* The i-th column must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* DGEQLF in the last k columns of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A.
* If SIDE = 'L', LDA >= max(1,M);
* if SIDE = 'R', LDA >= max(1,N).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGEQLF.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**T*C or C*Q**T or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If SIDE = 'L', LWORK >= max(1,N);
* if SIDE = 'R', LWORK >= max(1,M).
* For optimum performance LWORK >= N*NB if SIDE = 'L', and
* LWORK >= M*NB if SIDE = 'R', where NB is the optimal
* blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dormql(java.lang.String side, java.lang.String trans, int m, int n, int k, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] c, int _c_offset, int Ldc, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORMQR overwrites the general real M-by-N matrix C with
*
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by DGEQRF. Q is of order M if SIDE = 'L' and of order N
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q**T from the Left;
* = 'R': apply Q or Q**T from the Right.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q;
* = 'T': Transpose, apply Q**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,K)
* The i-th column must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* DGEQRF in the first k columns of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A.
* If SIDE = 'L', LDA >= max(1,M);
* if SIDE = 'R', LDA >= max(1,N).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGEQRF.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**T*C or C*Q**T or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If SIDE = 'L', LWORK >= max(1,N);
* if SIDE = 'R', LWORK >= max(1,M).
* For optimum performance LWORK >= N*NB if SIDE = 'L', and
* LWORK >= M*NB if SIDE = 'R', where NB is the optimal
* blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param c
* @param Ldc
* @param work
* @param lwork
* @param info
*
*/
abstract public void dormqr(java.lang.String side, java.lang.String trans, int m, int n, int k, double[] a, int lda, double[] tau, double[] c, int Ldc, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORMQR overwrites the general real M-by-N matrix C with
*
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by DGEQRF. Q is of order M if SIDE = 'L' and of order N
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q**T from the Left;
* = 'R': apply Q or Q**T from the Right.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q;
* = 'T': Transpose, apply Q**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,K)
* The i-th column must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* DGEQRF in the first k columns of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A.
* If SIDE = 'L', LDA >= max(1,M);
* if SIDE = 'R', LDA >= max(1,N).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGEQRF.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**T*C or C*Q**T or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If SIDE = 'L', LWORK >= max(1,N);
* if SIDE = 'R', LWORK >= max(1,M).
* For optimum performance LWORK >= N*NB if SIDE = 'L', and
* LWORK >= M*NB if SIDE = 'R', where NB is the optimal
* blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dormqr(java.lang.String side, java.lang.String trans, int m, int n, int k, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] c, int _c_offset, int Ldc, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORMR2 overwrites the general real m by n matrix C with
*
* Q * C if SIDE = 'L' and TRANS = 'N', or
*
* Q'* C if SIDE = 'L' and TRANS = 'T', or
*
* C * Q if SIDE = 'R' and TRANS = 'N', or
*
* C * Q' if SIDE = 'R' and TRANS = 'T',
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by DGERQF. Q is of order m if SIDE = 'L' and of order n
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q' from the Left
* = 'R': apply Q or Q' from the Right
*
* TRANS (input) CHARACTER*1
* = 'N': apply Q (No transpose)
* = 'T': apply Q' (Transpose)
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* A (input) DOUBLE PRECISION array, dimension
* (LDA,M) if SIDE = 'L',
* (LDA,N) if SIDE = 'R'
* The i-th row must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* DGERQF in the last k rows of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,K).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGERQF.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the m by n matrix C.
* On exit, C is overwritten by Q*C or Q'*C or C*Q' or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) DOUBLE PRECISION array, dimension
* (N) if SIDE = 'L',
* (M) if SIDE = 'R'
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param c
* @param Ldc
* @param work
* @param info
*
*/
abstract public void dormr2(java.lang.String side, java.lang.String trans, int m, int n, int k, double[] a, int lda, double[] tau, double[] c, int Ldc, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORMR2 overwrites the general real m by n matrix C with
*
* Q * C if SIDE = 'L' and TRANS = 'N', or
*
* Q'* C if SIDE = 'L' and TRANS = 'T', or
*
* C * Q if SIDE = 'R' and TRANS = 'N', or
*
* C * Q' if SIDE = 'R' and TRANS = 'T',
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by DGERQF. Q is of order m if SIDE = 'L' and of order n
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q' from the Left
* = 'R': apply Q or Q' from the Right
*
* TRANS (input) CHARACTER*1
* = 'N': apply Q (No transpose)
* = 'T': apply Q' (Transpose)
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* A (input) DOUBLE PRECISION array, dimension
* (LDA,M) if SIDE = 'L',
* (LDA,N) if SIDE = 'R'
* The i-th row must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* DGERQF in the last k rows of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,K).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGERQF.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the m by n matrix C.
* On exit, C is overwritten by Q*C or Q'*C or C*Q' or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) DOUBLE PRECISION array, dimension
* (N) if SIDE = 'L',
* (M) if SIDE = 'R'
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dormr2(java.lang.String side, java.lang.String trans, int m, int n, int k, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] c, int _c_offset, int Ldc, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORMR3 overwrites the general real m by n matrix C with
*
* Q * C if SIDE = 'L' and TRANS = 'N', or
*
* Q'* C if SIDE = 'L' and TRANS = 'T', or
*
* C * Q if SIDE = 'R' and TRANS = 'N', or
*
* C * Q' if SIDE = 'R' and TRANS = 'T',
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by DTZRZF. Q is of order m if SIDE = 'L' and of order n
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q' from the Left
* = 'R': apply Q or Q' from the Right
*
* TRANS (input) CHARACTER*1
* = 'N': apply Q (No transpose)
* = 'T': apply Q' (Transpose)
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* L (input) INTEGER
* The number of columns of the matrix A containing
* the meaningful part of the Householder reflectors.
* If SIDE = 'L', M >= L >= 0, if SIDE = 'R', N >= L >= 0.
*
* A (input) DOUBLE PRECISION array, dimension
* (LDA,M) if SIDE = 'L',
* (LDA,N) if SIDE = 'R'
* The i-th row must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* DTZRZF in the last k rows of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,K).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DTZRZF.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the m-by-n matrix C.
* On exit, C is overwritten by Q*C or Q'*C or C*Q' or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) DOUBLE PRECISION array, dimension
* (N) if SIDE = 'L',
* (M) if SIDE = 'R'
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* Based on contributions by
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param l
* @param a
* @param lda
* @param tau
* @param c
* @param Ldc
* @param work
* @param info
*
*/
abstract public void dormr3(java.lang.String side, java.lang.String trans, int m, int n, int k, int l, double[] a, int lda, double[] tau, double[] c, int Ldc, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORMR3 overwrites the general real m by n matrix C with
*
* Q * C if SIDE = 'L' and TRANS = 'N', or
*
* Q'* C if SIDE = 'L' and TRANS = 'T', or
*
* C * Q if SIDE = 'R' and TRANS = 'N', or
*
* C * Q' if SIDE = 'R' and TRANS = 'T',
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by DTZRZF. Q is of order m if SIDE = 'L' and of order n
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q' from the Left
* = 'R': apply Q or Q' from the Right
*
* TRANS (input) CHARACTER*1
* = 'N': apply Q (No transpose)
* = 'T': apply Q' (Transpose)
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* L (input) INTEGER
* The number of columns of the matrix A containing
* the meaningful part of the Householder reflectors.
* If SIDE = 'L', M >= L >= 0, if SIDE = 'R', N >= L >= 0.
*
* A (input) DOUBLE PRECISION array, dimension
* (LDA,M) if SIDE = 'L',
* (LDA,N) if SIDE = 'R'
* The i-th row must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* DTZRZF in the last k rows of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,K).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DTZRZF.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the m-by-n matrix C.
* On exit, C is overwritten by Q*C or Q'*C or C*Q' or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) DOUBLE PRECISION array, dimension
* (N) if SIDE = 'L',
* (M) if SIDE = 'R'
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* Based on contributions by
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param l
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dormr3(java.lang.String side, java.lang.String trans, int m, int n, int k, int l, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] c, int _c_offset, int Ldc, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORMRQ overwrites the general real M-by-N matrix C with
*
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by DGERQF. Q is of order M if SIDE = 'L' and of order N
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q**T from the Left;
* = 'R': apply Q or Q**T from the Right.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q;
* = 'T': Transpose, apply Q**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* A (input) DOUBLE PRECISION array, dimension
* (LDA,M) if SIDE = 'L',
* (LDA,N) if SIDE = 'R'
* The i-th row must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* DGERQF in the last k rows of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,K).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGERQF.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**T*C or C*Q**T or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If SIDE = 'L', LWORK >= max(1,N);
* if SIDE = 'R', LWORK >= max(1,M).
* For optimum performance LWORK >= N*NB if SIDE = 'L', and
* LWORK >= M*NB if SIDE = 'R', where NB is the optimal
* blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param c
* @param Ldc
* @param work
* @param lwork
* @param info
*
*/
abstract public void dormrq(java.lang.String side, java.lang.String trans, int m, int n, int k, double[] a, int lda, double[] tau, double[] c, int Ldc, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORMRQ overwrites the general real M-by-N matrix C with
*
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by DGERQF. Q is of order M if SIDE = 'L' and of order N
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q**T from the Left;
* = 'R': apply Q or Q**T from the Right.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q;
* = 'T': Transpose, apply Q**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* A (input) DOUBLE PRECISION array, dimension
* (LDA,M) if SIDE = 'L',
* (LDA,N) if SIDE = 'R'
* The i-th row must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* DGERQF in the last k rows of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,K).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DGERQF.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**T*C or C*Q**T or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If SIDE = 'L', LWORK >= max(1,N);
* if SIDE = 'R', LWORK >= max(1,M).
* For optimum performance LWORK >= N*NB if SIDE = 'L', and
* LWORK >= M*NB if SIDE = 'R', where NB is the optimal
* blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dormrq(java.lang.String side, java.lang.String trans, int m, int n, int k, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] c, int _c_offset, int Ldc, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORMRZ overwrites the general real M-by-N matrix C with
*
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by DTZRZF. Q is of order M if SIDE = 'L' and of order N
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q**T from the Left;
* = 'R': apply Q or Q**T from the Right.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q;
* = 'T': Transpose, apply Q**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* L (input) INTEGER
* The number of columns of the matrix A containing
* the meaningful part of the Householder reflectors.
* If SIDE = 'L', M >= L >= 0, if SIDE = 'R', N >= L >= 0.
*
* A (input) DOUBLE PRECISION array, dimension
* (LDA,M) if SIDE = 'L',
* (LDA,N) if SIDE = 'R'
* The i-th row must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* DTZRZF in the last k rows of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,K).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DTZRZF.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**H*C or C*Q**H or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If SIDE = 'L', LWORK >= max(1,N);
* if SIDE = 'R', LWORK >= max(1,M).
* For optimum performance LWORK >= N*NB if SIDE = 'L', and
* LWORK >= M*NB if SIDE = 'R', where NB is the optimal
* blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* Based on contributions by
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param l
* @param a
* @param lda
* @param tau
* @param c
* @param Ldc
* @param work
* @param lwork
* @param info
*
*/
abstract public void dormrz(java.lang.String side, java.lang.String trans, int m, int n, int k, int l, double[] a, int lda, double[] tau, double[] c, int Ldc, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORMRZ overwrites the general real M-by-N matrix C with
*
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by DTZRZF. Q is of order M if SIDE = 'L' and of order N
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q**T from the Left;
* = 'R': apply Q or Q**T from the Right.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q;
* = 'T': Transpose, apply Q**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* L (input) INTEGER
* The number of columns of the matrix A containing
* the meaningful part of the Householder reflectors.
* If SIDE = 'L', M >= L >= 0, if SIDE = 'R', N >= L >= 0.
*
* A (input) DOUBLE PRECISION array, dimension
* (LDA,M) if SIDE = 'L',
* (LDA,N) if SIDE = 'R'
* The i-th row must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* DTZRZF in the last k rows of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,K).
*
* TAU (input) DOUBLE PRECISION array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DTZRZF.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**H*C or C*Q**H or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If SIDE = 'L', LWORK >= max(1,N);
* if SIDE = 'R', LWORK >= max(1,M).
* For optimum performance LWORK >= N*NB if SIDE = 'L', and
* LWORK >= M*NB if SIDE = 'R', where NB is the optimal
* blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* Based on contributions by
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param l
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dormrz(java.lang.String side, java.lang.String trans, int m, int n, int k, int l, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] c, int _c_offset, int Ldc, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORMTR overwrites the general real M-by-N matrix C with
*
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* where Q is a real orthogonal matrix of order nq, with nq = m if
* SIDE = 'L' and nq = n if SIDE = 'R'. Q is defined as the product of
* nq-1 elementary reflectors, as returned by DSYTRD:
*
* if UPLO = 'U', Q = H(nq-1) . . . H(2) H(1);
*
* if UPLO = 'L', Q = H(1) H(2) . . . H(nq-1).
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q**T from the Left;
* = 'R': apply Q or Q**T from the Right.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A contains elementary reflectors
* from DSYTRD;
* = 'L': Lower triangle of A contains elementary reflectors
* from DSYTRD.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q;
* = 'T': Transpose, apply Q**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* A (input) DOUBLE PRECISION array, dimension
* (LDA,M) if SIDE = 'L'
* (LDA,N) if SIDE = 'R'
* The vectors which define the elementary reflectors, as
* returned by DSYTRD.
*
* LDA (input) INTEGER
* The leading dimension of the array A.
* LDA >= max(1,M) if SIDE = 'L'; LDA >= max(1,N) if SIDE = 'R'.
*
* TAU (input) DOUBLE PRECISION array, dimension
* (M-1) if SIDE = 'L'
* (N-1) if SIDE = 'R'
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DSYTRD.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**T*C or C*Q**T or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If SIDE = 'L', LWORK >= max(1,N);
* if SIDE = 'R', LWORK >= max(1,M).
* For optimum performance LWORK >= N*NB if SIDE = 'L', and
* LWORK >= M*NB if SIDE = 'R', where NB is the optimal
* blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param side
* @param uplo
* @param trans
* @param m
* @param n
* @param a
* @param lda
* @param tau
* @param c
* @param Ldc
* @param work
* @param lwork
* @param info
*
*/
abstract public void dormtr(java.lang.String side, java.lang.String uplo, java.lang.String trans, int m, int n, double[] a, int lda, double[] tau, double[] c, int Ldc, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DORMTR overwrites the general real M-by-N matrix C with
*
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* where Q is a real orthogonal matrix of order nq, with nq = m if
* SIDE = 'L' and nq = n if SIDE = 'R'. Q is defined as the product of
* nq-1 elementary reflectors, as returned by DSYTRD:
*
* if UPLO = 'U', Q = H(nq-1) . . . H(2) H(1);
*
* if UPLO = 'L', Q = H(1) H(2) . . . H(nq-1).
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q**T from the Left;
* = 'R': apply Q or Q**T from the Right.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A contains elementary reflectors
* from DSYTRD;
* = 'L': Lower triangle of A contains elementary reflectors
* from DSYTRD.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q;
* = 'T': Transpose, apply Q**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* A (input) DOUBLE PRECISION array, dimension
* (LDA,M) if SIDE = 'L'
* (LDA,N) if SIDE = 'R'
* The vectors which define the elementary reflectors, as
* returned by DSYTRD.
*
* LDA (input) INTEGER
* The leading dimension of the array A.
* LDA >= max(1,M) if SIDE = 'L'; LDA >= max(1,N) if SIDE = 'R'.
*
* TAU (input) DOUBLE PRECISION array, dimension
* (M-1) if SIDE = 'L'
* (N-1) if SIDE = 'R'
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by DSYTRD.
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**T*C or C*Q**T or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If SIDE = 'L', LWORK >= max(1,N);
* if SIDE = 'R', LWORK >= max(1,M).
* For optimum performance LWORK >= N*NB if SIDE = 'L', and
* LWORK >= M*NB if SIDE = 'R', where NB is the optimal
* blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param side
* @param uplo
* @param trans
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dormtr(java.lang.String side, java.lang.String uplo, java.lang.String trans, int m, int n, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] c, int _c_offset, int Ldc, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPBCON estimates the reciprocal of the condition number (in the
* 1-norm) of a real symmetric positive definite band matrix using the
* Cholesky factorization A = U**T*U or A = L*L**T computed by DPBTRF.
*
* An estimate is obtained for norm(inv(A)), and the reciprocal of the
* condition number is computed as RCOND = 1 / (ANORM * norm(inv(A))).
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangular factor stored in AB;
* = 'L': Lower triangular factor stored in AB.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* AB (input) DOUBLE PRECISION array, dimension (LDAB,N)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T of the band matrix A, stored in the
* first KD+1 rows of the array. The j-th column of U or L is
* stored in the j-th column of the array AB as follows:
* if UPLO ='U', AB(kd+1+i-j,j) = U(i,j) for max(1,j-kd)<=i<=j;
* if UPLO ='L', AB(1+i-j,j) = L(i,j) for j<=i<=min(n,j+kd).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* ANORM (input) DOUBLE PRECISION
* The 1-norm (or infinity-norm) of the symmetric band matrix A.
*
* RCOND (output) DOUBLE PRECISION
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(ANORM * AINVNM), where AINVNM is an
* estimate of the 1-norm of inv(A) computed in this routine.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param kd
* @param ab
* @param ldab
* @param anorm
* @param rcond
* @param work
* @param iwork
* @param info
*
*/
abstract public void dpbcon(java.lang.String uplo, int n, int kd, double[] ab, int ldab, double anorm, org.netlib.util.doubleW rcond, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPBCON estimates the reciprocal of the condition number (in the
* 1-norm) of a real symmetric positive definite band matrix using the
* Cholesky factorization A = U**T*U or A = L*L**T computed by DPBTRF.
*
* An estimate is obtained for norm(inv(A)), and the reciprocal of the
* condition number is computed as RCOND = 1 / (ANORM * norm(inv(A))).
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangular factor stored in AB;
* = 'L': Lower triangular factor stored in AB.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* AB (input) DOUBLE PRECISION array, dimension (LDAB,N)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T of the band matrix A, stored in the
* first KD+1 rows of the array. The j-th column of U or L is
* stored in the j-th column of the array AB as follows:
* if UPLO ='U', AB(kd+1+i-j,j) = U(i,j) for max(1,j-kd)<=i<=j;
* if UPLO ='L', AB(1+i-j,j) = L(i,j) for j<=i<=min(n,j+kd).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* ANORM (input) DOUBLE PRECISION
* The 1-norm (or infinity-norm) of the symmetric band matrix A.
*
* RCOND (output) DOUBLE PRECISION
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(ANORM * AINVNM), where AINVNM is an
* estimate of the 1-norm of inv(A) computed in this routine.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param kd
* @param ab
* @param _ab_offset
* @param ldab
* @param anorm
* @param rcond
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dpbcon(java.lang.String uplo, int n, int kd, double[] ab, int _ab_offset, int ldab, double anorm, org.netlib.util.doubleW rcond, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPBEQU computes row and column scalings intended to equilibrate a
* symmetric positive definite band matrix A and reduce its condition
* number (with respect to the two-norm). S contains the scale factors,
* S(i) = 1/sqrt(A(i,i)), chosen so that the scaled matrix B with
* elements B(i,j) = S(i)*A(i,j)*S(j) has ones on the diagonal. This
* choice of S puts the condition number of B within a factor N of the
* smallest possible condition number over all possible diagonal
* scalings.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangular of A is stored;
* = 'L': Lower triangular of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* AB (input) DOUBLE PRECISION array, dimension (LDAB,N)
* The upper or lower triangle of the symmetric band matrix A,
* stored in the first KD+1 rows of the array. The j-th column
* of A is stored in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* LDAB (input) INTEGER
* The leading dimension of the array A. LDAB >= KD+1.
*
* S (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, S contains the scale factors for A.
*
* SCOND (output) DOUBLE PRECISION
* If INFO = 0, S contains the ratio of the smallest S(i) to
* the largest S(i). If SCOND >= 0.1 and AMAX is neither too
* large nor too small, it is not worth scaling by S.
*
* AMAX (output) DOUBLE PRECISION
* Absolute value of largest matrix element. If AMAX is very
* close to overflow or very close to underflow, the matrix
* should be scaled.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, the i-th diagonal element is nonpositive.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param kd
* @param ab
* @param ldab
* @param s
* @param scond
* @param amax
* @param info
*
*/
abstract public void dpbequ(java.lang.String uplo, int n, int kd, double[] ab, int ldab, double[] s, org.netlib.util.doubleW scond, org.netlib.util.doubleW amax, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPBEQU computes row and column scalings intended to equilibrate a
* symmetric positive definite band matrix A and reduce its condition
* number (with respect to the two-norm). S contains the scale factors,
* S(i) = 1/sqrt(A(i,i)), chosen so that the scaled matrix B with
* elements B(i,j) = S(i)*A(i,j)*S(j) has ones on the diagonal. This
* choice of S puts the condition number of B within a factor N of the
* smallest possible condition number over all possible diagonal
* scalings.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangular of A is stored;
* = 'L': Lower triangular of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* AB (input) DOUBLE PRECISION array, dimension (LDAB,N)
* The upper or lower triangle of the symmetric band matrix A,
* stored in the first KD+1 rows of the array. The j-th column
* of A is stored in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* LDAB (input) INTEGER
* The leading dimension of the array A. LDAB >= KD+1.
*
* S (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, S contains the scale factors for A.
*
* SCOND (output) DOUBLE PRECISION
* If INFO = 0, S contains the ratio of the smallest S(i) to
* the largest S(i). If SCOND >= 0.1 and AMAX is neither too
* large nor too small, it is not worth scaling by S.
*
* AMAX (output) DOUBLE PRECISION
* Absolute value of largest matrix element. If AMAX is very
* close to overflow or very close to underflow, the matrix
* should be scaled.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, the i-th diagonal element is nonpositive.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param kd
* @param ab
* @param _ab_offset
* @param ldab
* @param s
* @param _s_offset
* @param scond
* @param amax
* @param info
*
*/
abstract public void dpbequ(java.lang.String uplo, int n, int kd, double[] ab, int _ab_offset, int ldab, double[] s, int _s_offset, org.netlib.util.doubleW scond, org.netlib.util.doubleW amax, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPBRFS improves the computed solution to a system of linear
* equations when the coefficient matrix is symmetric positive definite
* and banded, and provides error bounds and backward error estimates
* for the solution.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AB (input) DOUBLE PRECISION array, dimension (LDAB,N)
* The upper or lower triangle of the symmetric band matrix A,
* stored in the first KD+1 rows of the array. The j-th column
* of A is stored in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* AFB (input) DOUBLE PRECISION array, dimension (LDAFB,N)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T of the band matrix A as computed by
* DPBTRF, in the same storage format as A (see AB).
*
* LDAFB (input) INTEGER
* The leading dimension of the array AFB. LDAFB >= KD+1.
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by DPBTRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param kd
* @param nrhs
* @param ab
* @param ldab
* @param afb
* @param ldafb
* @param b
* @param ldb
* @param x
* @param ldx
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void dpbrfs(java.lang.String uplo, int n, int kd, int nrhs, double[] ab, int ldab, double[] afb, int ldafb, double[] b, int ldb, double[] x, int ldx, double[] ferr, double[] berr, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPBRFS improves the computed solution to a system of linear
* equations when the coefficient matrix is symmetric positive definite
* and banded, and provides error bounds and backward error estimates
* for the solution.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AB (input) DOUBLE PRECISION array, dimension (LDAB,N)
* The upper or lower triangle of the symmetric band matrix A,
* stored in the first KD+1 rows of the array. The j-th column
* of A is stored in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* AFB (input) DOUBLE PRECISION array, dimension (LDAFB,N)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T of the band matrix A as computed by
* DPBTRF, in the same storage format as A (see AB).
*
* LDAFB (input) INTEGER
* The leading dimension of the array AFB. LDAFB >= KD+1.
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by DPBTRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param kd
* @param nrhs
* @param ab
* @param _ab_offset
* @param ldab
* @param afb
* @param _afb_offset
* @param ldafb
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dpbrfs(java.lang.String uplo, int n, int kd, int nrhs, double[] ab, int _ab_offset, int ldab, double[] afb, int _afb_offset, int ldafb, double[] b, int _b_offset, int ldb, double[] x, int _x_offset, int ldx, double[] ferr, int _ferr_offset, double[] berr, int _berr_offset, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPBSTF computes a split Cholesky factorization of a real
* symmetric positive definite band matrix A.
*
* This routine is designed to be used in conjunction with DSBGST.
*
* The factorization has the form A = S**T*S where S is a band matrix
* of the same bandwidth as A and the following structure:
*
* S = ( U )
* ( M L )
*
* where U is upper triangular of order m = (n+kd)/2, and L is lower
* triangular of order n-m.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB,N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first kd+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* On exit, if INFO = 0, the factor S from the split Cholesky
* factorization A = S**T*S. See Further Details.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the factorization could not be completed,
* because the updated element a(i,i) was negative; the
* matrix A is not positive definite.
*
* Further Details
* ===============
*
* The band storage scheme is illustrated by the following example, when
* N = 7, KD = 2:
*
* S = ( s11 s12 s13 )
* ( s22 s23 s24 )
* ( s33 s34 )
* ( s44 )
* ( s53 s54 s55 )
* ( s64 s65 s66 )
* ( s75 s76 s77 )
*
* If UPLO = 'U', the array AB holds:
*
* on entry: on exit:
*
* * * a13 a24 a35 a46 a57 * * s13 s24 s53 s64 s75
* * a12 a23 a34 a45 a56 a67 * s12 s23 s34 s54 s65 s76
* a11 a22 a33 a44 a55 a66 a77 s11 s22 s33 s44 s55 s66 s77
*
* If UPLO = 'L', the array AB holds:
*
* on entry: on exit:
*
* a11 a22 a33 a44 a55 a66 a77 s11 s22 s33 s44 s55 s66 s77
* a21 a32 a43 a54 a65 a76 * s12 s23 s34 s54 s65 s76 *
* a31 a42 a53 a64 a64 * * s13 s24 s53 s64 s75 * *
*
* Array elements marked * are not used by the routine.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param kd
* @param ab
* @param ldab
* @param info
*
*/
abstract public void dpbstf(java.lang.String uplo, int n, int kd, double[] ab, int ldab, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPBSTF computes a split Cholesky factorization of a real
* symmetric positive definite band matrix A.
*
* This routine is designed to be used in conjunction with DSBGST.
*
* The factorization has the form A = S**T*S where S is a band matrix
* of the same bandwidth as A and the following structure:
*
* S = ( U )
* ( M L )
*
* where U is upper triangular of order m = (n+kd)/2, and L is lower
* triangular of order n-m.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB,N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first kd+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* On exit, if INFO = 0, the factor S from the split Cholesky
* factorization A = S**T*S. See Further Details.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the factorization could not be completed,
* because the updated element a(i,i) was negative; the
* matrix A is not positive definite.
*
* Further Details
* ===============
*
* The band storage scheme is illustrated by the following example, when
* N = 7, KD = 2:
*
* S = ( s11 s12 s13 )
* ( s22 s23 s24 )
* ( s33 s34 )
* ( s44 )
* ( s53 s54 s55 )
* ( s64 s65 s66 )
* ( s75 s76 s77 )
*
* If UPLO = 'U', the array AB holds:
*
* on entry: on exit:
*
* * * a13 a24 a35 a46 a57 * * s13 s24 s53 s64 s75
* * a12 a23 a34 a45 a56 a67 * s12 s23 s34 s54 s65 s76
* a11 a22 a33 a44 a55 a66 a77 s11 s22 s33 s44 s55 s66 s77
*
* If UPLO = 'L', the array AB holds:
*
* on entry: on exit:
*
* a11 a22 a33 a44 a55 a66 a77 s11 s22 s33 s44 s55 s66 s77
* a21 a32 a43 a54 a65 a76 * s12 s23 s34 s54 s65 s76 *
* a31 a42 a53 a64 a64 * * s13 s24 s53 s64 s75 * *
*
* Array elements marked * are not used by the routine.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param kd
* @param ab
* @param _ab_offset
* @param ldab
* @param info
*
*/
abstract public void dpbstf(java.lang.String uplo, int n, int kd, double[] ab, int _ab_offset, int ldab, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPBSV computes the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N symmetric positive definite band matrix and X
* and B are N-by-NRHS matrices.
*
* The Cholesky decomposition is used to factor A as
* A = U**T * U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular band matrix, and L is a lower
* triangular band matrix, with the same number of superdiagonals or
* subdiagonals as A. The factored form of A is then used to solve the
* system of equations A * X = B.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB,N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(KD+1+i-j,j) = A(i,j) for max(1,j-KD)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(N,j+KD).
* See below for further details.
*
* On exit, if INFO = 0, the triangular factor U or L from the
* Cholesky factorization A = U**T*U or A = L*L**T of the band
* matrix A, in the same storage format as A.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if INFO = 0, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the leading minor of order i of A is not
* positive definite, so the factorization could not be
* completed, and the solution has not been computed.
*
* Further Details
* ===============
*
* The band storage scheme is illustrated by the following example, when
* N = 6, KD = 2, and UPLO = 'U':
*
* On entry: On exit:
*
* * * a13 a24 a35 a46 * * u13 u24 u35 u46
* * a12 a23 a34 a45 a56 * u12 u23 u34 u45 u56
* a11 a22 a33 a44 a55 a66 u11 u22 u33 u44 u55 u66
*
* Similarly, if UPLO = 'L' the format of A is as follows:
*
* On entry: On exit:
*
* a11 a22 a33 a44 a55 a66 l11 l22 l33 l44 l55 l66
* a21 a32 a43 a54 a65 * l21 l32 l43 l54 l65 *
* a31 a42 a53 a64 * * l31 l42 l53 l64 * *
*
* Array elements marked * are not used by the routine.
*
* =====================================================================
*
* .. External Functions ..
*
*
* @param uplo
* @param n
* @param kd
* @param nrhs
* @param ab
* @param ldab
* @param b
* @param ldb
* @param info
*
*/
abstract public void dpbsv(java.lang.String uplo, int n, int kd, int nrhs, double[] ab, int ldab, double[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPBSV computes the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N symmetric positive definite band matrix and X
* and B are N-by-NRHS matrices.
*
* The Cholesky decomposition is used to factor A as
* A = U**T * U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular band matrix, and L is a lower
* triangular band matrix, with the same number of superdiagonals or
* subdiagonals as A. The factored form of A is then used to solve the
* system of equations A * X = B.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB,N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(KD+1+i-j,j) = A(i,j) for max(1,j-KD)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(N,j+KD).
* See below for further details.
*
* On exit, if INFO = 0, the triangular factor U or L from the
* Cholesky factorization A = U**T*U or A = L*L**T of the band
* matrix A, in the same storage format as A.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if INFO = 0, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the leading minor of order i of A is not
* positive definite, so the factorization could not be
* completed, and the solution has not been computed.
*
* Further Details
* ===============
*
* The band storage scheme is illustrated by the following example, when
* N = 6, KD = 2, and UPLO = 'U':
*
* On entry: On exit:
*
* * * a13 a24 a35 a46 * * u13 u24 u35 u46
* * a12 a23 a34 a45 a56 * u12 u23 u34 u45 u56
* a11 a22 a33 a44 a55 a66 u11 u22 u33 u44 u55 u66
*
* Similarly, if UPLO = 'L' the format of A is as follows:
*
* On entry: On exit:
*
* a11 a22 a33 a44 a55 a66 l11 l22 l33 l44 l55 l66
* a21 a32 a43 a54 a65 * l21 l32 l43 l54 l65 *
* a31 a42 a53 a64 * * l31 l42 l53 l64 * *
*
* Array elements marked * are not used by the routine.
*
* =====================================================================
*
* .. External Functions ..
*
*
* @param uplo
* @param n
* @param kd
* @param nrhs
* @param ab
* @param _ab_offset
* @param ldab
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void dpbsv(java.lang.String uplo, int n, int kd, int nrhs, double[] ab, int _ab_offset, int ldab, double[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPBSVX uses the Cholesky factorization A = U**T*U or A = L*L**T to
* compute the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N symmetric positive definite band matrix and X
* and B are N-by-NRHS matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed:
*
* 1. If FACT = 'E', real scaling factors are computed to equilibrate
* the system:
* diag(S) * A * diag(S) * inv(diag(S)) * X = diag(S) * B
* Whether or not the system will be equilibrated depends on the
* scaling of the matrix A, but if equilibration is used, A is
* overwritten by diag(S)*A*diag(S) and B by diag(S)*B.
*
* 2. If FACT = 'N' or 'E', the Cholesky decomposition is used to
* factor the matrix A (after equilibration if FACT = 'E') as
* A = U**T * U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular band matrix, and L is a lower
* triangular band matrix.
*
* 3. If the leading i-by-i principal minor is not positive definite,
* then the routine returns with INFO = i. Otherwise, the factored
* form of A is used to estimate the condition number of the matrix
* A. If the reciprocal of the condition number is less than machine
* precision, INFO = N+1 is returned as a warning, but the routine
* still goes on to solve for X and compute error bounds as
* described below.
*
* 4. The system of equations is solved for X using the factored form
* of A.
*
* 5. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* 6. If equilibration was used, the matrix X is premultiplied by
* diag(S) so that it solves the original system before
* equilibration.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of the matrix A is
* supplied on entry, and if not, whether the matrix A should be
* equilibrated before it is factored.
* = 'F': On entry, AFB contains the factored form of A.
* If EQUED = 'Y', the matrix A has been equilibrated
* with scaling factors given by S. AB and AFB will not
* be modified.
* = 'N': The matrix A will be copied to AFB and factored.
* = 'E': The matrix A will be equilibrated if necessary, then
* copied to AFB and factored.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* NRHS (input) INTEGER
* The number of right-hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB,N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array, except
* if FACT = 'F' and EQUED = 'Y', then A must contain the
* equilibrated matrix diag(S)*A*diag(S). The j-th column of A
* is stored in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(KD+1+i-j,j) = A(i,j) for max(1,j-KD)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(N,j+KD).
* See below for further details.
*
* On exit, if FACT = 'E' and EQUED = 'Y', A is overwritten by
* diag(S)*A*diag(S).
*
* LDAB (input) INTEGER
* The leading dimension of the array A. LDAB >= KD+1.
*
* AFB (input or output) DOUBLE PRECISION array, dimension (LDAFB,N)
* If FACT = 'F', then AFB is an input argument and on entry
* contains the triangular factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T of the band matrix
* A, in the same storage format as A (see AB). If EQUED = 'Y',
* then AFB is the factored form of the equilibrated matrix A.
*
* If FACT = 'N', then AFB is an output argument and on exit
* returns the triangular factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T.
*
* If FACT = 'E', then AFB is an output argument and on exit
* returns the triangular factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T of the equilibrated
* matrix A (see the description of A for the form of the
* equilibrated matrix).
*
* LDAFB (input) INTEGER
* The leading dimension of the array AFB. LDAFB >= KD+1.
*
* EQUED (input or output) CHARACTER*1
* Specifies the form of equilibration that was done.
* = 'N': No equilibration (always true if FACT = 'N').
* = 'Y': Equilibration was done, i.e., A has been replaced by
* diag(S) * A * diag(S).
* EQUED is an input argument if FACT = 'F'; otherwise, it is an
* output argument.
*
* S (input or output) DOUBLE PRECISION array, dimension (N)
* The scale factors for A; not accessed if EQUED = 'N'. S is
* an input argument if FACT = 'F'; otherwise, S is an output
* argument. If FACT = 'F' and EQUED = 'Y', each element of S
* must be positive.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if EQUED = 'N', B is not modified; if EQUED = 'Y',
* B is overwritten by diag(S) * B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* If INFO = 0 or INFO = N+1, the N-by-NRHS solution matrix X to
* the original system of equations. Note that if EQUED = 'Y',
* A and B are modified on exit, and the solution to the
* equilibrated system is inv(diag(S))*X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) DOUBLE PRECISION
* The estimate of the reciprocal condition number of the matrix
* A after equilibration (if done). If RCOND is less than the
* machine precision (in particular, if RCOND = 0), the matrix
* is singular to working precision. This condition is
* indicated by a return code of INFO > 0.
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= N: the leading minor of order i of A is
* not positive definite, so the factorization
* could not be completed, and the solution has not
* been computed. RCOND = 0 is returned.
* = N+1: U is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* Further Details
* ===============
*
* The band storage scheme is illustrated by the following example, when
* N = 6, KD = 2, and UPLO = 'U':
*
* Two-dimensional storage of the symmetric matrix A:
*
* a11 a12 a13
* a22 a23 a24
* a33 a34 a35
* a44 a45 a46
* a55 a56
* (aij=conjg(aji)) a66
*
* Band storage of the upper triangle of A:
*
* * * a13 a24 a35 a46
* * a12 a23 a34 a45 a56
* a11 a22 a33 a44 a55 a66
*
* Similarly, if UPLO = 'L' the format of A is as follows:
*
* a11 a22 a33 a44 a55 a66
* a21 a32 a43 a54 a65 *
* a31 a42 a53 a64 * *
*
* Array elements marked * are not used by the routine.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param uplo
* @param n
* @param kd
* @param nrhs
* @param ab
* @param ldab
* @param afb
* @param ldafb
* @param equed
* @param s
* @param b
* @param ldb
* @param x
* @param ldx
* @param rcond
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void dpbsvx(java.lang.String fact, java.lang.String uplo, int n, int kd, int nrhs, double[] ab, int ldab, double[] afb, int ldafb, org.netlib.util.StringW equed, double[] s, double[] b, int ldb, double[] x, int ldx, org.netlib.util.doubleW rcond, double[] ferr, double[] berr, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPBSVX uses the Cholesky factorization A = U**T*U or A = L*L**T to
* compute the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N symmetric positive definite band matrix and X
* and B are N-by-NRHS matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed:
*
* 1. If FACT = 'E', real scaling factors are computed to equilibrate
* the system:
* diag(S) * A * diag(S) * inv(diag(S)) * X = diag(S) * B
* Whether or not the system will be equilibrated depends on the
* scaling of the matrix A, but if equilibration is used, A is
* overwritten by diag(S)*A*diag(S) and B by diag(S)*B.
*
* 2. If FACT = 'N' or 'E', the Cholesky decomposition is used to
* factor the matrix A (after equilibration if FACT = 'E') as
* A = U**T * U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular band matrix, and L is a lower
* triangular band matrix.
*
* 3. If the leading i-by-i principal minor is not positive definite,
* then the routine returns with INFO = i. Otherwise, the factored
* form of A is used to estimate the condition number of the matrix
* A. If the reciprocal of the condition number is less than machine
* precision, INFO = N+1 is returned as a warning, but the routine
* still goes on to solve for X and compute error bounds as
* described below.
*
* 4. The system of equations is solved for X using the factored form
* of A.
*
* 5. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* 6. If equilibration was used, the matrix X is premultiplied by
* diag(S) so that it solves the original system before
* equilibration.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of the matrix A is
* supplied on entry, and if not, whether the matrix A should be
* equilibrated before it is factored.
* = 'F': On entry, AFB contains the factored form of A.
* If EQUED = 'Y', the matrix A has been equilibrated
* with scaling factors given by S. AB and AFB will not
* be modified.
* = 'N': The matrix A will be copied to AFB and factored.
* = 'E': The matrix A will be equilibrated if necessary, then
* copied to AFB and factored.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* NRHS (input) INTEGER
* The number of right-hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB,N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array, except
* if FACT = 'F' and EQUED = 'Y', then A must contain the
* equilibrated matrix diag(S)*A*diag(S). The j-th column of A
* is stored in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(KD+1+i-j,j) = A(i,j) for max(1,j-KD)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(N,j+KD).
* See below for further details.
*
* On exit, if FACT = 'E' and EQUED = 'Y', A is overwritten by
* diag(S)*A*diag(S).
*
* LDAB (input) INTEGER
* The leading dimension of the array A. LDAB >= KD+1.
*
* AFB (input or output) DOUBLE PRECISION array, dimension (LDAFB,N)
* If FACT = 'F', then AFB is an input argument and on entry
* contains the triangular factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T of the band matrix
* A, in the same storage format as A (see AB). If EQUED = 'Y',
* then AFB is the factored form of the equilibrated matrix A.
*
* If FACT = 'N', then AFB is an output argument and on exit
* returns the triangular factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T.
*
* If FACT = 'E', then AFB is an output argument and on exit
* returns the triangular factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T of the equilibrated
* matrix A (see the description of A for the form of the
* equilibrated matrix).
*
* LDAFB (input) INTEGER
* The leading dimension of the array AFB. LDAFB >= KD+1.
*
* EQUED (input or output) CHARACTER*1
* Specifies the form of equilibration that was done.
* = 'N': No equilibration (always true if FACT = 'N').
* = 'Y': Equilibration was done, i.e., A has been replaced by
* diag(S) * A * diag(S).
* EQUED is an input argument if FACT = 'F'; otherwise, it is an
* output argument.
*
* S (input or output) DOUBLE PRECISION array, dimension (N)
* The scale factors for A; not accessed if EQUED = 'N'. S is
* an input argument if FACT = 'F'; otherwise, S is an output
* argument. If FACT = 'F' and EQUED = 'Y', each element of S
* must be positive.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if EQUED = 'N', B is not modified; if EQUED = 'Y',
* B is overwritten by diag(S) * B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* If INFO = 0 or INFO = N+1, the N-by-NRHS solution matrix X to
* the original system of equations. Note that if EQUED = 'Y',
* A and B are modified on exit, and the solution to the
* equilibrated system is inv(diag(S))*X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) DOUBLE PRECISION
* The estimate of the reciprocal condition number of the matrix
* A after equilibration (if done). If RCOND is less than the
* machine precision (in particular, if RCOND = 0), the matrix
* is singular to working precision. This condition is
* indicated by a return code of INFO > 0.
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= N: the leading minor of order i of A is
* not positive definite, so the factorization
* could not be completed, and the solution has not
* been computed. RCOND = 0 is returned.
* = N+1: U is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* Further Details
* ===============
*
* The band storage scheme is illustrated by the following example, when
* N = 6, KD = 2, and UPLO = 'U':
*
* Two-dimensional storage of the symmetric matrix A:
*
* a11 a12 a13
* a22 a23 a24
* a33 a34 a35
* a44 a45 a46
* a55 a56
* (aij=conjg(aji)) a66
*
* Band storage of the upper triangle of A:
*
* * * a13 a24 a35 a46
* * a12 a23 a34 a45 a56
* a11 a22 a33 a44 a55 a66
*
* Similarly, if UPLO = 'L' the format of A is as follows:
*
* a11 a22 a33 a44 a55 a66
* a21 a32 a43 a54 a65 *
* a31 a42 a53 a64 * *
*
* Array elements marked * are not used by the routine.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param uplo
* @param n
* @param kd
* @param nrhs
* @param ab
* @param _ab_offset
* @param ldab
* @param afb
* @param _afb_offset
* @param ldafb
* @param equed
* @param s
* @param _s_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param rcond
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dpbsvx(java.lang.String fact, java.lang.String uplo, int n, int kd, int nrhs, double[] ab, int _ab_offset, int ldab, double[] afb, int _afb_offset, int ldafb, org.netlib.util.StringW equed, double[] s, int _s_offset, double[] b, int _b_offset, int ldb, double[] x, int _x_offset, int ldx, org.netlib.util.doubleW rcond, double[] ferr, int _ferr_offset, double[] berr, int _berr_offset, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPBTF2 computes the Cholesky factorization of a real symmetric
* positive definite band matrix A.
*
* The factorization has the form
* A = U' * U , if UPLO = 'U', or
* A = L * L', if UPLO = 'L',
* where U is an upper triangular matrix, U' is the transpose of U, and
* L is lower triangular.
*
* This is the unblocked version of the algorithm, calling Level 2 BLAS.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored:
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of super-diagonals of the matrix A if UPLO = 'U',
* or the number of sub-diagonals if UPLO = 'L'. KD >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB,N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* On exit, if INFO = 0, the triangular factor U or L from the
* Cholesky factorization A = U'*U or A = L*L' of the band
* matrix A, in the same storage format as A.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
* > 0: if INFO = k, the leading minor of order k is not
* positive definite, and the factorization could not be
* completed.
*
* Further Details
* ===============
*
* The band storage scheme is illustrated by the following example, when
* N = 6, KD = 2, and UPLO = 'U':
*
* On entry: On exit:
*
* * * a13 a24 a35 a46 * * u13 u24 u35 u46
* * a12 a23 a34 a45 a56 * u12 u23 u34 u45 u56
* a11 a22 a33 a44 a55 a66 u11 u22 u33 u44 u55 u66
*
* Similarly, if UPLO = 'L' the format of A is as follows:
*
* On entry: On exit:
*
* a11 a22 a33 a44 a55 a66 l11 l22 l33 l44 l55 l66
* a21 a32 a43 a54 a65 * l21 l32 l43 l54 l65 *
* a31 a42 a53 a64 * * l31 l42 l53 l64 * *
*
* Array elements marked * are not used by the routine.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param kd
* @param ab
* @param ldab
* @param info
*
*/
abstract public void dpbtf2(java.lang.String uplo, int n, int kd, double[] ab, int ldab, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPBTF2 computes the Cholesky factorization of a real symmetric
* positive definite band matrix A.
*
* The factorization has the form
* A = U' * U , if UPLO = 'U', or
* A = L * L', if UPLO = 'L',
* where U is an upper triangular matrix, U' is the transpose of U, and
* L is lower triangular.
*
* This is the unblocked version of the algorithm, calling Level 2 BLAS.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored:
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of super-diagonals of the matrix A if UPLO = 'U',
* or the number of sub-diagonals if UPLO = 'L'. KD >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB,N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* On exit, if INFO = 0, the triangular factor U or L from the
* Cholesky factorization A = U'*U or A = L*L' of the band
* matrix A, in the same storage format as A.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
* > 0: if INFO = k, the leading minor of order k is not
* positive definite, and the factorization could not be
* completed.
*
* Further Details
* ===============
*
* The band storage scheme is illustrated by the following example, when
* N = 6, KD = 2, and UPLO = 'U':
*
* On entry: On exit:
*
* * * a13 a24 a35 a46 * * u13 u24 u35 u46
* * a12 a23 a34 a45 a56 * u12 u23 u34 u45 u56
* a11 a22 a33 a44 a55 a66 u11 u22 u33 u44 u55 u66
*
* Similarly, if UPLO = 'L' the format of A is as follows:
*
* On entry: On exit:
*
* a11 a22 a33 a44 a55 a66 l11 l22 l33 l44 l55 l66
* a21 a32 a43 a54 a65 * l21 l32 l43 l54 l65 *
* a31 a42 a53 a64 * * l31 l42 l53 l64 * *
*
* Array elements marked * are not used by the routine.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param kd
* @param ab
* @param _ab_offset
* @param ldab
* @param info
*
*/
abstract public void dpbtf2(java.lang.String uplo, int n, int kd, double[] ab, int _ab_offset, int ldab, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPBTRF computes the Cholesky factorization of a real symmetric
* positive definite band matrix A.
*
* The factorization has the form
* A = U**T * U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular matrix and L is lower triangular.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB,N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* On exit, if INFO = 0, the triangular factor U or L from the
* Cholesky factorization A = U**T*U or A = L*L**T of the band
* matrix A, in the same storage format as A.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the leading minor of order i is not
* positive definite, and the factorization could not be
* completed.
*
* Further Details
* ===============
*
* The band storage scheme is illustrated by the following example, when
* N = 6, KD = 2, and UPLO = 'U':
*
* On entry: On exit:
*
* * * a13 a24 a35 a46 * * u13 u24 u35 u46
* * a12 a23 a34 a45 a56 * u12 u23 u34 u45 u56
* a11 a22 a33 a44 a55 a66 u11 u22 u33 u44 u55 u66
*
* Similarly, if UPLO = 'L' the format of A is as follows:
*
* On entry: On exit:
*
* a11 a22 a33 a44 a55 a66 l11 l22 l33 l44 l55 l66
* a21 a32 a43 a54 a65 * l21 l32 l43 l54 l65 *
* a31 a42 a53 a64 * * l31 l42 l53 l64 * *
*
* Array elements marked * are not used by the routine.
*
* Contributed by
* Peter Mayes and Giuseppe Radicati, IBM ECSEC, Rome, March 23, 1989
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param kd
* @param ab
* @param ldab
* @param info
*
*/
abstract public void dpbtrf(java.lang.String uplo, int n, int kd, double[] ab, int ldab, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPBTRF computes the Cholesky factorization of a real symmetric
* positive definite band matrix A.
*
* The factorization has the form
* A = U**T * U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular matrix and L is lower triangular.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB,N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* On exit, if INFO = 0, the triangular factor U or L from the
* Cholesky factorization A = U**T*U or A = L*L**T of the band
* matrix A, in the same storage format as A.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the leading minor of order i is not
* positive definite, and the factorization could not be
* completed.
*
* Further Details
* ===============
*
* The band storage scheme is illustrated by the following example, when
* N = 6, KD = 2, and UPLO = 'U':
*
* On entry: On exit:
*
* * * a13 a24 a35 a46 * * u13 u24 u35 u46
* * a12 a23 a34 a45 a56 * u12 u23 u34 u45 u56
* a11 a22 a33 a44 a55 a66 u11 u22 u33 u44 u55 u66
*
* Similarly, if UPLO = 'L' the format of A is as follows:
*
* On entry: On exit:
*
* a11 a22 a33 a44 a55 a66 l11 l22 l33 l44 l55 l66
* a21 a32 a43 a54 a65 * l21 l32 l43 l54 l65 *
* a31 a42 a53 a64 * * l31 l42 l53 l64 * *
*
* Array elements marked * are not used by the routine.
*
* Contributed by
* Peter Mayes and Giuseppe Radicati, IBM ECSEC, Rome, March 23, 1989
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param kd
* @param ab
* @param _ab_offset
* @param ldab
* @param info
*
*/
abstract public void dpbtrf(java.lang.String uplo, int n, int kd, double[] ab, int _ab_offset, int ldab, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPBTRS solves a system of linear equations A*X = B with a symmetric
* positive definite band matrix A using the Cholesky factorization
* A = U**T*U or A = L*L**T computed by DPBTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangular factor stored in AB;
* = 'L': Lower triangular factor stored in AB.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AB (input) DOUBLE PRECISION array, dimension (LDAB,N)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T of the band matrix A, stored in the
* first KD+1 rows of the array. The j-th column of U or L is
* stored in the j-th column of the array AB as follows:
* if UPLO ='U', AB(kd+1+i-j,j) = U(i,j) for max(1,j-kd)<=i<=j;
* if UPLO ='L', AB(1+i-j,j) = L(i,j) for j<=i<=min(n,j+kd).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param uplo
* @param n
* @param kd
* @param nrhs
* @param ab
* @param ldab
* @param b
* @param ldb
* @param info
*
*/
abstract public void dpbtrs(java.lang.String uplo, int n, int kd, int nrhs, double[] ab, int ldab, double[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPBTRS solves a system of linear equations A*X = B with a symmetric
* positive definite band matrix A using the Cholesky factorization
* A = U**T*U or A = L*L**T computed by DPBTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangular factor stored in AB;
* = 'L': Lower triangular factor stored in AB.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AB (input) DOUBLE PRECISION array, dimension (LDAB,N)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T of the band matrix A, stored in the
* first KD+1 rows of the array. The j-th column of U or L is
* stored in the j-th column of the array AB as follows:
* if UPLO ='U', AB(kd+1+i-j,j) = U(i,j) for max(1,j-kd)<=i<=j;
* if UPLO ='L', AB(1+i-j,j) = L(i,j) for j<=i<=min(n,j+kd).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param uplo
* @param n
* @param kd
* @param nrhs
* @param ab
* @param _ab_offset
* @param ldab
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void dpbtrs(java.lang.String uplo, int n, int kd, int nrhs, double[] ab, int _ab_offset, int ldab, double[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPOCON estimates the reciprocal of the condition number (in the
* 1-norm) of a real symmetric positive definite matrix using the
* Cholesky factorization A = U**T*U or A = L*L**T computed by DPOTRF.
*
* An estimate is obtained for norm(inv(A)), and the reciprocal of the
* condition number is computed as RCOND = 1 / (ANORM * norm(inv(A))).
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T, as computed by DPOTRF.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* ANORM (input) DOUBLE PRECISION
* The 1-norm (or infinity-norm) of the symmetric matrix A.
*
* RCOND (output) DOUBLE PRECISION
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(ANORM * AINVNM), where AINVNM is an
* estimate of the 1-norm of inv(A) computed in this routine.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param lda
* @param anorm
* @param rcond
* @param work
* @param iwork
* @param info
*
*/
abstract public void dpocon(java.lang.String uplo, int n, double[] a, int lda, double anorm, org.netlib.util.doubleW rcond, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPOCON estimates the reciprocal of the condition number (in the
* 1-norm) of a real symmetric positive definite matrix using the
* Cholesky factorization A = U**T*U or A = L*L**T computed by DPOTRF.
*
* An estimate is obtained for norm(inv(A)), and the reciprocal of the
* condition number is computed as RCOND = 1 / (ANORM * norm(inv(A))).
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T, as computed by DPOTRF.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* ANORM (input) DOUBLE PRECISION
* The 1-norm (or infinity-norm) of the symmetric matrix A.
*
* RCOND (output) DOUBLE PRECISION
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(ANORM * AINVNM), where AINVNM is an
* estimate of the 1-norm of inv(A) computed in this routine.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param anorm
* @param rcond
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dpocon(java.lang.String uplo, int n, double[] a, int _a_offset, int lda, double anorm, org.netlib.util.doubleW rcond, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPOEQU computes row and column scalings intended to equilibrate a
* symmetric positive definite matrix A and reduce its condition number
* (with respect to the two-norm). S contains the scale factors,
* S(i) = 1/sqrt(A(i,i)), chosen so that the scaled matrix B with
* elements B(i,j) = S(i)*A(i,j)*S(j) has ones on the diagonal. This
* choice of S puts the condition number of B within a factor N of the
* smallest possible condition number over all possible diagonal
* scalings.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The N-by-N symmetric positive definite matrix whose scaling
* factors are to be computed. Only the diagonal elements of A
* are referenced.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* S (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, S contains the scale factors for A.
*
* SCOND (output) DOUBLE PRECISION
* If INFO = 0, S contains the ratio of the smallest S(i) to
* the largest S(i). If SCOND >= 0.1 and AMAX is neither too
* large nor too small, it is not worth scaling by S.
*
* AMAX (output) DOUBLE PRECISION
* Absolute value of largest matrix element. If AMAX is very
* close to overflow or very close to underflow, the matrix
* should be scaled.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the i-th diagonal element is nonpositive.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param a
* @param lda
* @param s
* @param scond
* @param amax
* @param info
*
*/
abstract public void dpoequ(int n, double[] a, int lda, double[] s, org.netlib.util.doubleW scond, org.netlib.util.doubleW amax, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPOEQU computes row and column scalings intended to equilibrate a
* symmetric positive definite matrix A and reduce its condition number
* (with respect to the two-norm). S contains the scale factors,
* S(i) = 1/sqrt(A(i,i)), chosen so that the scaled matrix B with
* elements B(i,j) = S(i)*A(i,j)*S(j) has ones on the diagonal. This
* choice of S puts the condition number of B within a factor N of the
* smallest possible condition number over all possible diagonal
* scalings.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The N-by-N symmetric positive definite matrix whose scaling
* factors are to be computed. Only the diagonal elements of A
* are referenced.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* S (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, S contains the scale factors for A.
*
* SCOND (output) DOUBLE PRECISION
* If INFO = 0, S contains the ratio of the smallest S(i) to
* the largest S(i). If SCOND >= 0.1 and AMAX is neither too
* large nor too small, it is not worth scaling by S.
*
* AMAX (output) DOUBLE PRECISION
* Absolute value of largest matrix element. If AMAX is very
* close to overflow or very close to underflow, the matrix
* should be scaled.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the i-th diagonal element is nonpositive.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param a
* @param _a_offset
* @param lda
* @param s
* @param _s_offset
* @param scond
* @param amax
* @param info
*
*/
abstract public void dpoequ(int n, double[] a, int _a_offset, int lda, double[] s, int _s_offset, org.netlib.util.doubleW scond, org.netlib.util.doubleW amax, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPORFS improves the computed solution to a system of linear
* equations when the coefficient matrix is symmetric positive definite,
* and provides error bounds and backward error estimates for the
* solution.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The symmetric matrix A. If UPLO = 'U', the leading N-by-N
* upper triangular part of A contains the upper triangular part
* of the matrix A, and the strictly lower triangular part of A
* is not referenced. If UPLO = 'L', the leading N-by-N lower
* triangular part of A contains the lower triangular part of
* the matrix A, and the strictly upper triangular part of A is
* not referenced.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* AF (input) DOUBLE PRECISION array, dimension (LDAF,N)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T, as computed by DPOTRF.
*
* LDAF (input) INTEGER
* The leading dimension of the array AF. LDAF >= max(1,N).
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by DPOTRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param a
* @param lda
* @param af
* @param ldaf
* @param b
* @param ldb
* @param x
* @param ldx
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void dporfs(java.lang.String uplo, int n, int nrhs, double[] a, int lda, double[] af, int ldaf, double[] b, int ldb, double[] x, int ldx, double[] ferr, double[] berr, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPORFS improves the computed solution to a system of linear
* equations when the coefficient matrix is symmetric positive definite,
* and provides error bounds and backward error estimates for the
* solution.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The symmetric matrix A. If UPLO = 'U', the leading N-by-N
* upper triangular part of A contains the upper triangular part
* of the matrix A, and the strictly lower triangular part of A
* is not referenced. If UPLO = 'L', the leading N-by-N lower
* triangular part of A contains the lower triangular part of
* the matrix A, and the strictly upper triangular part of A is
* not referenced.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* AF (input) DOUBLE PRECISION array, dimension (LDAF,N)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T, as computed by DPOTRF.
*
* LDAF (input) INTEGER
* The leading dimension of the array AF. LDAF >= max(1,N).
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by DPOTRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param af
* @param _af_offset
* @param ldaf
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dporfs(java.lang.String uplo, int n, int nrhs, double[] a, int _a_offset, int lda, double[] af, int _af_offset, int ldaf, double[] b, int _b_offset, int ldb, double[] x, int _x_offset, int ldx, double[] ferr, int _ferr_offset, double[] berr, int _berr_offset, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPOSV computes the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N symmetric positive definite matrix and X and B
* are N-by-NRHS matrices.
*
* The Cholesky decomposition is used to factor A as
* A = U**T* U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular matrix and L is a lower triangular
* matrix. The factored form of A is then used to solve the system of
* equations A * X = B.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* N-by-N upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading N-by-N lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, if INFO = 0, the factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if INFO = 0, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the leading minor of order i of A is not
* positive definite, so the factorization could not be
* completed, and the solution has not been computed.
*
* =====================================================================
*
* .. External Functions ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param a
* @param lda
* @param b
* @param ldb
* @param info
*
*/
abstract public void dposv(java.lang.String uplo, int n, int nrhs, double[] a, int lda, double[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPOSV computes the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N symmetric positive definite matrix and X and B
* are N-by-NRHS matrices.
*
* The Cholesky decomposition is used to factor A as
* A = U**T* U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular matrix and L is a lower triangular
* matrix. The factored form of A is then used to solve the system of
* equations A * X = B.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* N-by-N upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading N-by-N lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, if INFO = 0, the factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if INFO = 0, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the leading minor of order i of A is not
* positive definite, so the factorization could not be
* completed, and the solution has not been computed.
*
* =====================================================================
*
* .. External Functions ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void dposv(java.lang.String uplo, int n, int nrhs, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPOSVX uses the Cholesky factorization A = U**T*U or A = L*L**T to
* compute the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N symmetric positive definite matrix and X and B
* are N-by-NRHS matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed:
*
* 1. If FACT = 'E', real scaling factors are computed to equilibrate
* the system:
* diag(S) * A * diag(S) * inv(diag(S)) * X = diag(S) * B
* Whether or not the system will be equilibrated depends on the
* scaling of the matrix A, but if equilibration is used, A is
* overwritten by diag(S)*A*diag(S) and B by diag(S)*B.
*
* 2. If FACT = 'N' or 'E', the Cholesky decomposition is used to
* factor the matrix A (after equilibration if FACT = 'E') as
* A = U**T* U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular matrix and L is a lower triangular
* matrix.
*
* 3. If the leading i-by-i principal minor is not positive definite,
* then the routine returns with INFO = i. Otherwise, the factored
* form of A is used to estimate the condition number of the matrix
* A. If the reciprocal of the condition number is less than machine
* precision, INFO = N+1 is returned as a warning, but the routine
* still goes on to solve for X and compute error bounds as
* described below.
*
* 4. The system of equations is solved for X using the factored form
* of A.
*
* 5. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* 6. If equilibration was used, the matrix X is premultiplied by
* diag(S) so that it solves the original system before
* equilibration.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of the matrix A is
* supplied on entry, and if not, whether the matrix A should be
* equilibrated before it is factored.
* = 'F': On entry, AF contains the factored form of A.
* If EQUED = 'Y', the matrix A has been equilibrated
* with scaling factors given by S. A and AF will not
* be modified.
* = 'N': The matrix A will be copied to AF and factored.
* = 'E': The matrix A will be equilibrated if necessary, then
* copied to AF and factored.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the symmetric matrix A, except if FACT = 'F' and
* EQUED = 'Y', then A must contain the equilibrated matrix
* diag(S)*A*diag(S). If UPLO = 'U', the leading
* N-by-N upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading N-by-N lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced. A is not modified if
* FACT = 'F' or 'N', or if FACT = 'E' and EQUED = 'N' on exit.
*
* On exit, if FACT = 'E' and EQUED = 'Y', A is overwritten by
* diag(S)*A*diag(S).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* AF (input or output) DOUBLE PRECISION array, dimension (LDAF,N)
* If FACT = 'F', then AF is an input argument and on entry
* contains the triangular factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T, in the same storage
* format as A. If EQUED .ne. 'N', then AF is the factored form
* of the equilibrated matrix diag(S)*A*diag(S).
*
* If FACT = 'N', then AF is an output argument and on exit
* returns the triangular factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T of the original
* matrix A.
*
* If FACT = 'E', then AF is an output argument and on exit
* returns the triangular factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T of the equilibrated
* matrix A (see the description of A for the form of the
* equilibrated matrix).
*
* LDAF (input) INTEGER
* The leading dimension of the array AF. LDAF >= max(1,N).
*
* EQUED (input or output) CHARACTER*1
* Specifies the form of equilibration that was done.
* = 'N': No equilibration (always true if FACT = 'N').
* = 'Y': Equilibration was done, i.e., A has been replaced by
* diag(S) * A * diag(S).
* EQUED is an input argument if FACT = 'F'; otherwise, it is an
* output argument.
*
* S (input or output) DOUBLE PRECISION array, dimension (N)
* The scale factors for A; not accessed if EQUED = 'N'. S is
* an input argument if FACT = 'F'; otherwise, S is an output
* argument. If FACT = 'F' and EQUED = 'Y', each element of S
* must be positive.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if EQUED = 'N', B is not modified; if EQUED = 'Y',
* B is overwritten by diag(S) * B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* If INFO = 0 or INFO = N+1, the N-by-NRHS solution matrix X to
* the original system of equations. Note that if EQUED = 'Y',
* A and B are modified on exit, and the solution to the
* equilibrated system is inv(diag(S))*X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) DOUBLE PRECISION
* The estimate of the reciprocal condition number of the matrix
* A after equilibration (if done). If RCOND is less than the
* machine precision (in particular, if RCOND = 0), the matrix
* is singular to working precision. This condition is
* indicated by a return code of INFO > 0.
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= N: the leading minor of order i of A is
* not positive definite, so the factorization
* could not be completed, and the solution has not
* been computed. RCOND = 0 is returned.
* = N+1: U is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param uplo
* @param n
* @param nrhs
* @param a
* @param lda
* @param af
* @param ldaf
* @param equed
* @param s
* @param b
* @param ldb
* @param x
* @param ldx
* @param rcond
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void dposvx(java.lang.String fact, java.lang.String uplo, int n, int nrhs, double[] a, int lda, double[] af, int ldaf, org.netlib.util.StringW equed, double[] s, double[] b, int ldb, double[] x, int ldx, org.netlib.util.doubleW rcond, double[] ferr, double[] berr, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPOSVX uses the Cholesky factorization A = U**T*U or A = L*L**T to
* compute the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N symmetric positive definite matrix and X and B
* are N-by-NRHS matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed:
*
* 1. If FACT = 'E', real scaling factors are computed to equilibrate
* the system:
* diag(S) * A * diag(S) * inv(diag(S)) * X = diag(S) * B
* Whether or not the system will be equilibrated depends on the
* scaling of the matrix A, but if equilibration is used, A is
* overwritten by diag(S)*A*diag(S) and B by diag(S)*B.
*
* 2. If FACT = 'N' or 'E', the Cholesky decomposition is used to
* factor the matrix A (after equilibration if FACT = 'E') as
* A = U**T* U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular matrix and L is a lower triangular
* matrix.
*
* 3. If the leading i-by-i principal minor is not positive definite,
* then the routine returns with INFO = i. Otherwise, the factored
* form of A is used to estimate the condition number of the matrix
* A. If the reciprocal of the condition number is less than machine
* precision, INFO = N+1 is returned as a warning, but the routine
* still goes on to solve for X and compute error bounds as
* described below.
*
* 4. The system of equations is solved for X using the factored form
* of A.
*
* 5. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* 6. If equilibration was used, the matrix X is premultiplied by
* diag(S) so that it solves the original system before
* equilibration.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of the matrix A is
* supplied on entry, and if not, whether the matrix A should be
* equilibrated before it is factored.
* = 'F': On entry, AF contains the factored form of A.
* If EQUED = 'Y', the matrix A has been equilibrated
* with scaling factors given by S. A and AF will not
* be modified.
* = 'N': The matrix A will be copied to AF and factored.
* = 'E': The matrix A will be equilibrated if necessary, then
* copied to AF and factored.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the symmetric matrix A, except if FACT = 'F' and
* EQUED = 'Y', then A must contain the equilibrated matrix
* diag(S)*A*diag(S). If UPLO = 'U', the leading
* N-by-N upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading N-by-N lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced. A is not modified if
* FACT = 'F' or 'N', or if FACT = 'E' and EQUED = 'N' on exit.
*
* On exit, if FACT = 'E' and EQUED = 'Y', A is overwritten by
* diag(S)*A*diag(S).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* AF (input or output) DOUBLE PRECISION array, dimension (LDAF,N)
* If FACT = 'F', then AF is an input argument and on entry
* contains the triangular factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T, in the same storage
* format as A. If EQUED .ne. 'N', then AF is the factored form
* of the equilibrated matrix diag(S)*A*diag(S).
*
* If FACT = 'N', then AF is an output argument and on exit
* returns the triangular factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T of the original
* matrix A.
*
* If FACT = 'E', then AF is an output argument and on exit
* returns the triangular factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T of the equilibrated
* matrix A (see the description of A for the form of the
* equilibrated matrix).
*
* LDAF (input) INTEGER
* The leading dimension of the array AF. LDAF >= max(1,N).
*
* EQUED (input or output) CHARACTER*1
* Specifies the form of equilibration that was done.
* = 'N': No equilibration (always true if FACT = 'N').
* = 'Y': Equilibration was done, i.e., A has been replaced by
* diag(S) * A * diag(S).
* EQUED is an input argument if FACT = 'F'; otherwise, it is an
* output argument.
*
* S (input or output) DOUBLE PRECISION array, dimension (N)
* The scale factors for A; not accessed if EQUED = 'N'. S is
* an input argument if FACT = 'F'; otherwise, S is an output
* argument. If FACT = 'F' and EQUED = 'Y', each element of S
* must be positive.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if EQUED = 'N', B is not modified; if EQUED = 'Y',
* B is overwritten by diag(S) * B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* If INFO = 0 or INFO = N+1, the N-by-NRHS solution matrix X to
* the original system of equations. Note that if EQUED = 'Y',
* A and B are modified on exit, and the solution to the
* equilibrated system is inv(diag(S))*X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) DOUBLE PRECISION
* The estimate of the reciprocal condition number of the matrix
* A after equilibration (if done). If RCOND is less than the
* machine precision (in particular, if RCOND = 0), the matrix
* is singular to working precision. This condition is
* indicated by a return code of INFO > 0.
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= N: the leading minor of order i of A is
* not positive definite, so the factorization
* could not be completed, and the solution has not
* been computed. RCOND = 0 is returned.
* = N+1: U is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param uplo
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param af
* @param _af_offset
* @param ldaf
* @param equed
* @param s
* @param _s_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param rcond
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dposvx(java.lang.String fact, java.lang.String uplo, int n, int nrhs, double[] a, int _a_offset, int lda, double[] af, int _af_offset, int ldaf, org.netlib.util.StringW equed, double[] s, int _s_offset, double[] b, int _b_offset, int ldb, double[] x, int _x_offset, int ldx, org.netlib.util.doubleW rcond, double[] ferr, int _ferr_offset, double[] berr, int _berr_offset, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPOTF2 computes the Cholesky factorization of a real symmetric
* positive definite matrix A.
*
* The factorization has the form
* A = U' * U , if UPLO = 'U', or
* A = L * L', if UPLO = 'L',
* where U is an upper triangular matrix and L is lower triangular.
*
* This is the unblocked version of the algorithm, calling Level 2 BLAS.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* n by n upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading n by n lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, if INFO = 0, the factor U or L from the Cholesky
* factorization A = U'*U or A = L*L'.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
* > 0: if INFO = k, the leading minor of order k is not
* positive definite, and the factorization could not be
* completed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param lda
* @param info
*
*/
abstract public void dpotf2(java.lang.String uplo, int n, double[] a, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPOTF2 computes the Cholesky factorization of a real symmetric
* positive definite matrix A.
*
* The factorization has the form
* A = U' * U , if UPLO = 'U', or
* A = L * L', if UPLO = 'L',
* where U is an upper triangular matrix and L is lower triangular.
*
* This is the unblocked version of the algorithm, calling Level 2 BLAS.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* n by n upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading n by n lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, if INFO = 0, the factor U or L from the Cholesky
* factorization A = U'*U or A = L*L'.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
* > 0: if INFO = k, the leading minor of order k is not
* positive definite, and the factorization could not be
* completed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param info
*
*/
abstract public void dpotf2(java.lang.String uplo, int n, double[] a, int _a_offset, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPOTRF computes the Cholesky factorization of a real symmetric
* positive definite matrix A.
*
* The factorization has the form
* A = U**T * U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular matrix and L is lower triangular.
*
* This is the block version of the algorithm, calling Level 3 BLAS.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* N-by-N upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading N-by-N lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, if INFO = 0, the factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the leading minor of order i is not
* positive definite, and the factorization could not be
* completed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param lda
* @param info
*
*/
abstract public void dpotrf(java.lang.String uplo, int n, double[] a, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPOTRF computes the Cholesky factorization of a real symmetric
* positive definite matrix A.
*
* The factorization has the form
* A = U**T * U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular matrix and L is lower triangular.
*
* This is the block version of the algorithm, calling Level 3 BLAS.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* N-by-N upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading N-by-N lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, if INFO = 0, the factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the leading minor of order i is not
* positive definite, and the factorization could not be
* completed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param info
*
*/
abstract public void dpotrf(java.lang.String uplo, int n, double[] a, int _a_offset, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPOTRI computes the inverse of a real symmetric positive definite
* matrix A using the Cholesky factorization A = U**T*U or A = L*L**T
* computed by DPOTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the triangular factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T, as computed by
* DPOTRF.
* On exit, the upper or lower triangle of the (symmetric)
* inverse of A, overwriting the input factor U or L.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the (i,i) element of the factor U or L is
* zero, and the inverse could not be computed.
*
* =====================================================================
*
* .. External Functions ..
*
*
* @param uplo
* @param n
* @param a
* @param lda
* @param info
*
*/
abstract public void dpotri(java.lang.String uplo, int n, double[] a, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPOTRI computes the inverse of a real symmetric positive definite
* matrix A using the Cholesky factorization A = U**T*U or A = L*L**T
* computed by DPOTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the triangular factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T, as computed by
* DPOTRF.
* On exit, the upper or lower triangle of the (symmetric)
* inverse of A, overwriting the input factor U or L.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the (i,i) element of the factor U or L is
* zero, and the inverse could not be computed.
*
* =====================================================================
*
* .. External Functions ..
*
*
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param info
*
*/
abstract public void dpotri(java.lang.String uplo, int n, double[] a, int _a_offset, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPOTRS solves a system of linear equations A*X = B with a symmetric
* positive definite matrix A using the Cholesky factorization
* A = U**T*U or A = L*L**T computed by DPOTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T, as computed by DPOTRF.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param a
* @param lda
* @param b
* @param ldb
* @param info
*
*/
abstract public void dpotrs(java.lang.String uplo, int n, int nrhs, double[] a, int lda, double[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPOTRS solves a system of linear equations A*X = B with a symmetric
* positive definite matrix A using the Cholesky factorization
* A = U**T*U or A = L*L**T computed by DPOTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T, as computed by DPOTRF.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void dpotrs(java.lang.String uplo, int n, int nrhs, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPPCON estimates the reciprocal of the condition number (in the
* 1-norm) of a real symmetric positive definite packed matrix using
* the Cholesky factorization A = U**T*U or A = L*L**T computed by
* DPPTRF.
*
* An estimate is obtained for norm(inv(A)), and the reciprocal of the
* condition number is computed as RCOND = 1 / (ANORM * norm(inv(A))).
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T, packed columnwise in a linear
* array. The j-th column of U or L is stored in the array AP
* as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = U(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = L(i,j) for j<=i<=n.
*
* ANORM (input) DOUBLE PRECISION
* The 1-norm (or infinity-norm) of the symmetric matrix A.
*
* RCOND (output) DOUBLE PRECISION
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(ANORM * AINVNM), where AINVNM is an
* estimate of the 1-norm of inv(A) computed in this routine.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param anorm
* @param rcond
* @param work
* @param iwork
* @param info
*
*/
abstract public void dppcon(java.lang.String uplo, int n, double[] ap, double anorm, org.netlib.util.doubleW rcond, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPPCON estimates the reciprocal of the condition number (in the
* 1-norm) of a real symmetric positive definite packed matrix using
* the Cholesky factorization A = U**T*U or A = L*L**T computed by
* DPPTRF.
*
* An estimate is obtained for norm(inv(A)), and the reciprocal of the
* condition number is computed as RCOND = 1 / (ANORM * norm(inv(A))).
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T, packed columnwise in a linear
* array. The j-th column of U or L is stored in the array AP
* as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = U(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = L(i,j) for j<=i<=n.
*
* ANORM (input) DOUBLE PRECISION
* The 1-norm (or infinity-norm) of the symmetric matrix A.
*
* RCOND (output) DOUBLE PRECISION
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(ANORM * AINVNM), where AINVNM is an
* estimate of the 1-norm of inv(A) computed in this routine.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param anorm
* @param rcond
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dppcon(java.lang.String uplo, int n, double[] ap, int _ap_offset, double anorm, org.netlib.util.doubleW rcond, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPPEQU computes row and column scalings intended to equilibrate a
* symmetric positive definite matrix A in packed storage and reduce
* its condition number (with respect to the two-norm). S contains the
* scale factors, S(i)=1/sqrt(A(i,i)), chosen so that the scaled matrix
* B with elements B(i,j)=S(i)*A(i,j)*S(j) has ones on the diagonal.
* This choice of S puts the condition number of B within a factor N of
* the smallest possible condition number over all possible diagonal
* scalings.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The upper or lower triangle of the symmetric matrix A, packed
* columnwise in a linear array. The j-th column of A is stored
* in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
*
* S (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, S contains the scale factors for A.
*
* SCOND (output) DOUBLE PRECISION
* If INFO = 0, S contains the ratio of the smallest S(i) to
* the largest S(i). If SCOND >= 0.1 and AMAX is neither too
* large nor too small, it is not worth scaling by S.
*
* AMAX (output) DOUBLE PRECISION
* Absolute value of largest matrix element. If AMAX is very
* close to overflow or very close to underflow, the matrix
* should be scaled.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the i-th diagonal element is nonpositive.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param s
* @param scond
* @param amax
* @param info
*
*/
abstract public void dppequ(java.lang.String uplo, int n, double[] ap, double[] s, org.netlib.util.doubleW scond, org.netlib.util.doubleW amax, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPPEQU computes row and column scalings intended to equilibrate a
* symmetric positive definite matrix A in packed storage and reduce
* its condition number (with respect to the two-norm). S contains the
* scale factors, S(i)=1/sqrt(A(i,i)), chosen so that the scaled matrix
* B with elements B(i,j)=S(i)*A(i,j)*S(j) has ones on the diagonal.
* This choice of S puts the condition number of B within a factor N of
* the smallest possible condition number over all possible diagonal
* scalings.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The upper or lower triangle of the symmetric matrix A, packed
* columnwise in a linear array. The j-th column of A is stored
* in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
*
* S (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, S contains the scale factors for A.
*
* SCOND (output) DOUBLE PRECISION
* If INFO = 0, S contains the ratio of the smallest S(i) to
* the largest S(i). If SCOND >= 0.1 and AMAX is neither too
* large nor too small, it is not worth scaling by S.
*
* AMAX (output) DOUBLE PRECISION
* Absolute value of largest matrix element. If AMAX is very
* close to overflow or very close to underflow, the matrix
* should be scaled.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the i-th diagonal element is nonpositive.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param s
* @param _s_offset
* @param scond
* @param amax
* @param info
*
*/
abstract public void dppequ(java.lang.String uplo, int n, double[] ap, int _ap_offset, double[] s, int _s_offset, org.netlib.util.doubleW scond, org.netlib.util.doubleW amax, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPPRFS improves the computed solution to a system of linear
* equations when the coefficient matrix is symmetric positive definite
* and packed, and provides error bounds and backward error estimates
* for the solution.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The upper or lower triangle of the symmetric matrix A, packed
* columnwise in a linear array. The j-th column of A is stored
* in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
*
* AFP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T, as computed by DPPTRF/ZPPTRF,
* packed columnwise in a linear array in the same format as A
* (see AP).
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by DPPTRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param ap
* @param afp
* @param b
* @param ldb
* @param x
* @param ldx
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void dpprfs(java.lang.String uplo, int n, int nrhs, double[] ap, double[] afp, double[] b, int ldb, double[] x, int ldx, double[] ferr, double[] berr, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPPRFS improves the computed solution to a system of linear
* equations when the coefficient matrix is symmetric positive definite
* and packed, and provides error bounds and backward error estimates
* for the solution.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The upper or lower triangle of the symmetric matrix A, packed
* columnwise in a linear array. The j-th column of A is stored
* in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
*
* AFP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T, as computed by DPPTRF/ZPPTRF,
* packed columnwise in a linear array in the same format as A
* (see AP).
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by DPPTRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param ap
* @param _ap_offset
* @param afp
* @param _afp_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dpprfs(java.lang.String uplo, int n, int nrhs, double[] ap, int _ap_offset, double[] afp, int _afp_offset, double[] b, int _b_offset, int ldb, double[] x, int _x_offset, int ldx, double[] ferr, int _ferr_offset, double[] berr, int _berr_offset, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPPSV computes the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N symmetric positive definite matrix stored in
* packed format and X and B are N-by-NRHS matrices.
*
* The Cholesky decomposition is used to factor A as
* A = U**T* U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular matrix and L is a lower triangular
* matrix. The factored form of A is then used to solve the system of
* equations A * X = B.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
* See below for further details.
*
* On exit, if INFO = 0, the factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T, in the same storage
* format as A.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if INFO = 0, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the leading minor of order i of A is not
* positive definite, so the factorization could not be
* completed, and the solution has not been computed.
*
* Further Details
* ===============
*
* The packed storage scheme is illustrated by the following example
* when N = 4, UPLO = 'U':
*
* Two-dimensional storage of the symmetric matrix A:
*
* a11 a12 a13 a14
* a22 a23 a24
* a33 a34 (aij = conjg(aji))
* a44
*
* Packed storage of the upper triangle of A:
*
* AP = [ a11, a12, a22, a13, a23, a33, a14, a24, a34, a44 ]
*
* =====================================================================
*
* .. External Functions ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param ap
* @param b
* @param ldb
* @param info
*
*/
abstract public void dppsv(java.lang.String uplo, int n, int nrhs, double[] ap, double[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPPSV computes the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N symmetric positive definite matrix stored in
* packed format and X and B are N-by-NRHS matrices.
*
* The Cholesky decomposition is used to factor A as
* A = U**T* U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular matrix and L is a lower triangular
* matrix. The factored form of A is then used to solve the system of
* equations A * X = B.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
* See below for further details.
*
* On exit, if INFO = 0, the factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T, in the same storage
* format as A.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if INFO = 0, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the leading minor of order i of A is not
* positive definite, so the factorization could not be
* completed, and the solution has not been computed.
*
* Further Details
* ===============
*
* The packed storage scheme is illustrated by the following example
* when N = 4, UPLO = 'U':
*
* Two-dimensional storage of the symmetric matrix A:
*
* a11 a12 a13 a14
* a22 a23 a24
* a33 a34 (aij = conjg(aji))
* a44
*
* Packed storage of the upper triangle of A:
*
* AP = [ a11, a12, a22, a13, a23, a33, a14, a24, a34, a44 ]
*
* =====================================================================
*
* .. External Functions ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param ap
* @param _ap_offset
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void dppsv(java.lang.String uplo, int n, int nrhs, double[] ap, int _ap_offset, double[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPPSVX uses the Cholesky factorization A = U**T*U or A = L*L**T to
* compute the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N symmetric positive definite matrix stored in
* packed format and X and B are N-by-NRHS matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed:
*
* 1. If FACT = 'E', real scaling factors are computed to equilibrate
* the system:
* diag(S) * A * diag(S) * inv(diag(S)) * X = diag(S) * B
* Whether or not the system will be equilibrated depends on the
* scaling of the matrix A, but if equilibration is used, A is
* overwritten by diag(S)*A*diag(S) and B by diag(S)*B.
*
* 2. If FACT = 'N' or 'E', the Cholesky decomposition is used to
* factor the matrix A (after equilibration if FACT = 'E') as
* A = U**T* U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular matrix and L is a lower triangular
* matrix.
*
* 3. If the leading i-by-i principal minor is not positive definite,
* then the routine returns with INFO = i. Otherwise, the factored
* form of A is used to estimate the condition number of the matrix
* A. If the reciprocal of the condition number is less than machine
* precision, INFO = N+1 is returned as a warning, but the routine
* still goes on to solve for X and compute error bounds as
* described below.
*
* 4. The system of equations is solved for X using the factored form
* of A.
*
* 5. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* 6. If equilibration was used, the matrix X is premultiplied by
* diag(S) so that it solves the original system before
* equilibration.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of the matrix A is
* supplied on entry, and if not, whether the matrix A should be
* equilibrated before it is factored.
* = 'F': On entry, AFP contains the factored form of A.
* If EQUED = 'Y', the matrix A has been equilibrated
* with scaling factors given by S. AP and AFP will not
* be modified.
* = 'N': The matrix A will be copied to AFP and factored.
* = 'E': The matrix A will be equilibrated if necessary, then
* copied to AFP and factored.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array, except if FACT = 'F'
* and EQUED = 'Y', then A must contain the equilibrated matrix
* diag(S)*A*diag(S). The j-th column of A is stored in the
* array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
* See below for further details. A is not modified if
* FACT = 'F' or 'N', or if FACT = 'E' and EQUED = 'N' on exit.
*
* On exit, if FACT = 'E' and EQUED = 'Y', A is overwritten by
* diag(S)*A*diag(S).
*
* AFP (input or output) DOUBLE PRECISION array, dimension
* (N*(N+1)/2)
* If FACT = 'F', then AFP is an input argument and on entry
* contains the triangular factor U or L from the Cholesky
* factorization A = U'*U or A = L*L', in the same storage
* format as A. If EQUED .ne. 'N', then AFP is the factored
* form of the equilibrated matrix A.
*
* If FACT = 'N', then AFP is an output argument and on exit
* returns the triangular factor U or L from the Cholesky
* factorization A = U'*U or A = L*L' of the original matrix A.
*
* If FACT = 'E', then AFP is an output argument and on exit
* returns the triangular factor U or L from the Cholesky
* factorization A = U'*U or A = L*L' of the equilibrated
* matrix A (see the description of AP for the form of the
* equilibrated matrix).
*
* EQUED (input or output) CHARACTER*1
* Specifies the form of equilibration that was done.
* = 'N': No equilibration (always true if FACT = 'N').
* = 'Y': Equilibration was done, i.e., A has been replaced by
* diag(S) * A * diag(S).
* EQUED is an input argument if FACT = 'F'; otherwise, it is an
* output argument.
*
* S (input or output) DOUBLE PRECISION array, dimension (N)
* The scale factors for A; not accessed if EQUED = 'N'. S is
* an input argument if FACT = 'F'; otherwise, S is an output
* argument. If FACT = 'F' and EQUED = 'Y', each element of S
* must be positive.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if EQUED = 'N', B is not modified; if EQUED = 'Y',
* B is overwritten by diag(S) * B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* If INFO = 0 or INFO = N+1, the N-by-NRHS solution matrix X to
* the original system of equations. Note that if EQUED = 'Y',
* A and B are modified on exit, and the solution to the
* equilibrated system is inv(diag(S))*X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) DOUBLE PRECISION
* The estimate of the reciprocal condition number of the matrix
* A after equilibration (if done). If RCOND is less than the
* machine precision (in particular, if RCOND = 0), the matrix
* is singular to working precision. This condition is
* indicated by a return code of INFO > 0.
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= N: the leading minor of order i of A is
* not positive definite, so the factorization
* could not be completed, and the solution has not
* been computed. RCOND = 0 is returned.
* = N+1: U is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* Further Details
* ===============
*
* The packed storage scheme is illustrated by the following example
* when N = 4, UPLO = 'U':
*
* Two-dimensional storage of the symmetric matrix A:
*
* a11 a12 a13 a14
* a22 a23 a24
* a33 a34 (aij = conjg(aji))
* a44
*
* Packed storage of the upper triangle of A:
*
* AP = [ a11, a12, a22, a13, a23, a33, a14, a24, a34, a44 ]
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param uplo
* @param n
* @param nrhs
* @param ap
* @param afp
* @param equed
* @param s
* @param b
* @param ldb
* @param x
* @param ldx
* @param rcond
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void dppsvx(java.lang.String fact, java.lang.String uplo, int n, int nrhs, double[] ap, double[] afp, org.netlib.util.StringW equed, double[] s, double[] b, int ldb, double[] x, int ldx, org.netlib.util.doubleW rcond, double[] ferr, double[] berr, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPPSVX uses the Cholesky factorization A = U**T*U or A = L*L**T to
* compute the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N symmetric positive definite matrix stored in
* packed format and X and B are N-by-NRHS matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed:
*
* 1. If FACT = 'E', real scaling factors are computed to equilibrate
* the system:
* diag(S) * A * diag(S) * inv(diag(S)) * X = diag(S) * B
* Whether or not the system will be equilibrated depends on the
* scaling of the matrix A, but if equilibration is used, A is
* overwritten by diag(S)*A*diag(S) and B by diag(S)*B.
*
* 2. If FACT = 'N' or 'E', the Cholesky decomposition is used to
* factor the matrix A (after equilibration if FACT = 'E') as
* A = U**T* U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular matrix and L is a lower triangular
* matrix.
*
* 3. If the leading i-by-i principal minor is not positive definite,
* then the routine returns with INFO = i. Otherwise, the factored
* form of A is used to estimate the condition number of the matrix
* A. If the reciprocal of the condition number is less than machine
* precision, INFO = N+1 is returned as a warning, but the routine
* still goes on to solve for X and compute error bounds as
* described below.
*
* 4. The system of equations is solved for X using the factored form
* of A.
*
* 5. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* 6. If equilibration was used, the matrix X is premultiplied by
* diag(S) so that it solves the original system before
* equilibration.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of the matrix A is
* supplied on entry, and if not, whether the matrix A should be
* equilibrated before it is factored.
* = 'F': On entry, AFP contains the factored form of A.
* If EQUED = 'Y', the matrix A has been equilibrated
* with scaling factors given by S. AP and AFP will not
* be modified.
* = 'N': The matrix A will be copied to AFP and factored.
* = 'E': The matrix A will be equilibrated if necessary, then
* copied to AFP and factored.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array, except if FACT = 'F'
* and EQUED = 'Y', then A must contain the equilibrated matrix
* diag(S)*A*diag(S). The j-th column of A is stored in the
* array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
* See below for further details. A is not modified if
* FACT = 'F' or 'N', or if FACT = 'E' and EQUED = 'N' on exit.
*
* On exit, if FACT = 'E' and EQUED = 'Y', A is overwritten by
* diag(S)*A*diag(S).
*
* AFP (input or output) DOUBLE PRECISION array, dimension
* (N*(N+1)/2)
* If FACT = 'F', then AFP is an input argument and on entry
* contains the triangular factor U or L from the Cholesky
* factorization A = U'*U or A = L*L', in the same storage
* format as A. If EQUED .ne. 'N', then AFP is the factored
* form of the equilibrated matrix A.
*
* If FACT = 'N', then AFP is an output argument and on exit
* returns the triangular factor U or L from the Cholesky
* factorization A = U'*U or A = L*L' of the original matrix A.
*
* If FACT = 'E', then AFP is an output argument and on exit
* returns the triangular factor U or L from the Cholesky
* factorization A = U'*U or A = L*L' of the equilibrated
* matrix A (see the description of AP for the form of the
* equilibrated matrix).
*
* EQUED (input or output) CHARACTER*1
* Specifies the form of equilibration that was done.
* = 'N': No equilibration (always true if FACT = 'N').
* = 'Y': Equilibration was done, i.e., A has been replaced by
* diag(S) * A * diag(S).
* EQUED is an input argument if FACT = 'F'; otherwise, it is an
* output argument.
*
* S (input or output) DOUBLE PRECISION array, dimension (N)
* The scale factors for A; not accessed if EQUED = 'N'. S is
* an input argument if FACT = 'F'; otherwise, S is an output
* argument. If FACT = 'F' and EQUED = 'Y', each element of S
* must be positive.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if EQUED = 'N', B is not modified; if EQUED = 'Y',
* B is overwritten by diag(S) * B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* If INFO = 0 or INFO = N+1, the N-by-NRHS solution matrix X to
* the original system of equations. Note that if EQUED = 'Y',
* A and B are modified on exit, and the solution to the
* equilibrated system is inv(diag(S))*X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) DOUBLE PRECISION
* The estimate of the reciprocal condition number of the matrix
* A after equilibration (if done). If RCOND is less than the
* machine precision (in particular, if RCOND = 0), the matrix
* is singular to working precision. This condition is
* indicated by a return code of INFO > 0.
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= N: the leading minor of order i of A is
* not positive definite, so the factorization
* could not be completed, and the solution has not
* been computed. RCOND = 0 is returned.
* = N+1: U is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* Further Details
* ===============
*
* The packed storage scheme is illustrated by the following example
* when N = 4, UPLO = 'U':
*
* Two-dimensional storage of the symmetric matrix A:
*
* a11 a12 a13 a14
* a22 a23 a24
* a33 a34 (aij = conjg(aji))
* a44
*
* Packed storage of the upper triangle of A:
*
* AP = [ a11, a12, a22, a13, a23, a33, a14, a24, a34, a44 ]
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param uplo
* @param n
* @param nrhs
* @param ap
* @param _ap_offset
* @param afp
* @param _afp_offset
* @param equed
* @param s
* @param _s_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param rcond
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dppsvx(java.lang.String fact, java.lang.String uplo, int n, int nrhs, double[] ap, int _ap_offset, double[] afp, int _afp_offset, org.netlib.util.StringW equed, double[] s, int _s_offset, double[] b, int _b_offset, int ldb, double[] x, int _x_offset, int ldx, org.netlib.util.doubleW rcond, double[] ferr, int _ferr_offset, double[] berr, int _berr_offset, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPPTRF computes the Cholesky factorization of a real symmetric
* positive definite matrix A stored in packed format.
*
* The factorization has the form
* A = U**T * U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular matrix and L is lower triangular.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
* See below for further details.
*
* On exit, if INFO = 0, the triangular factor U or L from the
* Cholesky factorization A = U**T*U or A = L*L**T, in the same
* storage format as A.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the leading minor of order i is not
* positive definite, and the factorization could not be
* completed.
*
* Further Details
* ======= =======
*
* The packed storage scheme is illustrated by the following example
* when N = 4, UPLO = 'U':
*
* Two-dimensional storage of the symmetric matrix A:
*
* a11 a12 a13 a14
* a22 a23 a24
* a33 a34 (aij = aji)
* a44
*
* Packed storage of the upper triangle of A:
*
* AP = [ a11, a12, a22, a13, a23, a33, a14, a24, a34, a44 ]
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param info
*
*/
abstract public void dpptrf(java.lang.String uplo, int n, double[] ap, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPPTRF computes the Cholesky factorization of a real symmetric
* positive definite matrix A stored in packed format.
*
* The factorization has the form
* A = U**T * U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular matrix and L is lower triangular.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
* See below for further details.
*
* On exit, if INFO = 0, the triangular factor U or L from the
* Cholesky factorization A = U**T*U or A = L*L**T, in the same
* storage format as A.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the leading minor of order i is not
* positive definite, and the factorization could not be
* completed.
*
* Further Details
* ======= =======
*
* The packed storage scheme is illustrated by the following example
* when N = 4, UPLO = 'U':
*
* Two-dimensional storage of the symmetric matrix A:
*
* a11 a12 a13 a14
* a22 a23 a24
* a33 a34 (aij = aji)
* a44
*
* Packed storage of the upper triangle of A:
*
* AP = [ a11, a12, a22, a13, a23, a33, a14, a24, a34, a44 ]
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param info
*
*/
abstract public void dpptrf(java.lang.String uplo, int n, double[] ap, int _ap_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPPTRI computes the inverse of a real symmetric positive definite
* matrix A using the Cholesky factorization A = U**T*U or A = L*L**T
* computed by DPPTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangular factor is stored in AP;
* = 'L': Lower triangular factor is stored in AP.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the triangular factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T, packed columnwise as
* a linear array. The j-th column of U or L is stored in the
* array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = U(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = L(i,j) for j<=i<=n.
*
* On exit, the upper or lower triangle of the (symmetric)
* inverse of A, overwriting the input factor U or L.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the (i,i) element of the factor U or L is
* zero, and the inverse could not be computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param info
*
*/
abstract public void dpptri(java.lang.String uplo, int n, double[] ap, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPPTRI computes the inverse of a real symmetric positive definite
* matrix A using the Cholesky factorization A = U**T*U or A = L*L**T
* computed by DPPTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangular factor is stored in AP;
* = 'L': Lower triangular factor is stored in AP.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the triangular factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T, packed columnwise as
* a linear array. The j-th column of U or L is stored in the
* array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = U(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = L(i,j) for j<=i<=n.
*
* On exit, the upper or lower triangle of the (symmetric)
* inverse of A, overwriting the input factor U or L.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the (i,i) element of the factor U or L is
* zero, and the inverse could not be computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param info
*
*/
abstract public void dpptri(java.lang.String uplo, int n, double[] ap, int _ap_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPPTRS solves a system of linear equations A*X = B with a symmetric
* positive definite matrix A in packed storage using the Cholesky
* factorization A = U**T*U or A = L*L**T computed by DPPTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T, packed columnwise in a linear
* array. The j-th column of U or L is stored in the array AP
* as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = U(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = L(i,j) for j<=i<=n.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param ap
* @param b
* @param ldb
* @param info
*
*/
abstract public void dpptrs(java.lang.String uplo, int n, int nrhs, double[] ap, double[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPPTRS solves a system of linear equations A*X = B with a symmetric
* positive definite matrix A in packed storage using the Cholesky
* factorization A = U**T*U or A = L*L**T computed by DPPTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T, packed columnwise in a linear
* array. The j-th column of U or L is stored in the array AP
* as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = U(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = L(i,j) for j<=i<=n.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param ap
* @param _ap_offset
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void dpptrs(java.lang.String uplo, int n, int nrhs, double[] ap, int _ap_offset, double[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPTCON computes the reciprocal of the condition number (in the
* 1-norm) of a real symmetric positive definite tridiagonal matrix
* using the factorization A = L*D*L**T or A = U**T*D*U computed by
* DPTTRF.
*
* Norm(inv(A)) is computed by a direct method, and the reciprocal of
* the condition number is computed as
* RCOND = 1 / (ANORM * norm(inv(A))).
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The n diagonal elements of the diagonal matrix D from the
* factorization of A, as computed by DPTTRF.
*
* E (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) off-diagonal elements of the unit bidiagonal factor
* U or L from the factorization of A, as computed by DPTTRF.
*
* ANORM (input) DOUBLE PRECISION
* The 1-norm of the original matrix A.
*
* RCOND (output) DOUBLE PRECISION
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(ANORM * AINVNM), where AINVNM is the
* 1-norm of inv(A) computed in this routine.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The method used is described in Nicholas J. Higham, "Efficient
* Algorithms for Computing the Condition Number of a Tridiagonal
* Matrix", SIAM J. Sci. Stat. Comput., Vol. 7, No. 1, January 1986.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param e
* @param anorm
* @param rcond
* @param work
* @param info
*
*/
abstract public void dptcon(int n, double[] d, double[] e, double anorm, org.netlib.util.doubleW rcond, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPTCON computes the reciprocal of the condition number (in the
* 1-norm) of a real symmetric positive definite tridiagonal matrix
* using the factorization A = L*D*L**T or A = U**T*D*U computed by
* DPTTRF.
*
* Norm(inv(A)) is computed by a direct method, and the reciprocal of
* the condition number is computed as
* RCOND = 1 / (ANORM * norm(inv(A))).
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The n diagonal elements of the diagonal matrix D from the
* factorization of A, as computed by DPTTRF.
*
* E (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) off-diagonal elements of the unit bidiagonal factor
* U or L from the factorization of A, as computed by DPTTRF.
*
* ANORM (input) DOUBLE PRECISION
* The 1-norm of the original matrix A.
*
* RCOND (output) DOUBLE PRECISION
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(ANORM * AINVNM), where AINVNM is the
* 1-norm of inv(A) computed in this routine.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The method used is described in Nicholas J. Higham, "Efficient
* Algorithms for Computing the Condition Number of a Tridiagonal
* Matrix", SIAM J. Sci. Stat. Comput., Vol. 7, No. 1, January 1986.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param anorm
* @param rcond
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dptcon(int n, double[] d, int _d_offset, double[] e, int _e_offset, double anorm, org.netlib.util.doubleW rcond, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPTEQR computes all eigenvalues and, optionally, eigenvectors of a
* symmetric positive definite tridiagonal matrix by first factoring the
* matrix using DPTTRF, and then calling DBDSQR to compute the singular
* values of the bidiagonal factor.
*
* This routine computes the eigenvalues of the positive definite
* tridiagonal matrix to high relative accuracy. This means that if the
* eigenvalues range over many orders of magnitude in size, then the
* small eigenvalues and corresponding eigenvectors will be computed
* more accurately than, for example, with the standard QR method.
*
* The eigenvectors of a full or band symmetric positive definite matrix
* can also be found if DSYTRD, DSPTRD, or DSBTRD has been used to
* reduce this matrix to tridiagonal form. (The reduction to tridiagonal
* form, however, may preclude the possibility of obtaining high
* relative accuracy in the small eigenvalues of the original matrix, if
* these eigenvalues range over many orders of magnitude.)
*
* Arguments
* =========
*
* COMPZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only.
* = 'V': Compute eigenvectors of original symmetric
* matrix also. Array Z contains the orthogonal
* matrix used to reduce the original matrix to
* tridiagonal form.
* = 'I': Compute eigenvectors of tridiagonal matrix also.
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the n diagonal elements of the tridiagonal
* matrix.
* On normal exit, D contains the eigenvalues, in descending
* order.
*
* E (input/output) DOUBLE PRECISION array, dimension (N-1)
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix.
* On exit, E has been destroyed.
*
* Z (input/output) DOUBLE PRECISION array, dimension (LDZ, N)
* On entry, if COMPZ = 'V', the orthogonal matrix used in the
* reduction to tridiagonal form.
* On exit, if COMPZ = 'V', the orthonormal eigenvectors of the
* original symmetric matrix;
* if COMPZ = 'I', the orthonormal eigenvectors of the
* tridiagonal matrix.
* If INFO > 0 on exit, Z contains the eigenvectors associated
* with only the stored eigenvalues.
* If COMPZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* COMPZ = 'V' or 'I', LDZ >= max(1,N).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (4*N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, and i is:
* <= N the Cholesky factorization of the matrix could
* not be performed because the i-th principal minor
* was not positive definite.
* > N the SVD algorithm failed to converge;
* if INFO = N+i, i off-diagonal elements of the
* bidiagonal factor did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param compz
* @param n
* @param d
* @param e
* @param z
* @param ldz
* @param work
* @param info
*
*/
abstract public void dpteqr(java.lang.String compz, int n, double[] d, double[] e, double[] z, int ldz, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPTEQR computes all eigenvalues and, optionally, eigenvectors of a
* symmetric positive definite tridiagonal matrix by first factoring the
* matrix using DPTTRF, and then calling DBDSQR to compute the singular
* values of the bidiagonal factor.
*
* This routine computes the eigenvalues of the positive definite
* tridiagonal matrix to high relative accuracy. This means that if the
* eigenvalues range over many orders of magnitude in size, then the
* small eigenvalues and corresponding eigenvectors will be computed
* more accurately than, for example, with the standard QR method.
*
* The eigenvectors of a full or band symmetric positive definite matrix
* can also be found if DSYTRD, DSPTRD, or DSBTRD has been used to
* reduce this matrix to tridiagonal form. (The reduction to tridiagonal
* form, however, may preclude the possibility of obtaining high
* relative accuracy in the small eigenvalues of the original matrix, if
* these eigenvalues range over many orders of magnitude.)
*
* Arguments
* =========
*
* COMPZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only.
* = 'V': Compute eigenvectors of original symmetric
* matrix also. Array Z contains the orthogonal
* matrix used to reduce the original matrix to
* tridiagonal form.
* = 'I': Compute eigenvectors of tridiagonal matrix also.
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the n diagonal elements of the tridiagonal
* matrix.
* On normal exit, D contains the eigenvalues, in descending
* order.
*
* E (input/output) DOUBLE PRECISION array, dimension (N-1)
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix.
* On exit, E has been destroyed.
*
* Z (input/output) DOUBLE PRECISION array, dimension (LDZ, N)
* On entry, if COMPZ = 'V', the orthogonal matrix used in the
* reduction to tridiagonal form.
* On exit, if COMPZ = 'V', the orthonormal eigenvectors of the
* original symmetric matrix;
* if COMPZ = 'I', the orthonormal eigenvectors of the
* tridiagonal matrix.
* If INFO > 0 on exit, Z contains the eigenvectors associated
* with only the stored eigenvalues.
* If COMPZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* COMPZ = 'V' or 'I', LDZ >= max(1,N).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (4*N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, and i is:
* <= N the Cholesky factorization of the matrix could
* not be performed because the i-th principal minor
* was not positive definite.
* > N the SVD algorithm failed to converge;
* if INFO = N+i, i off-diagonal elements of the
* bidiagonal factor did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param compz
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dpteqr(java.lang.String compz, int n, double[] d, int _d_offset, double[] e, int _e_offset, double[] z, int _z_offset, int ldz, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPTRFS improves the computed solution to a system of linear
* equations when the coefficient matrix is symmetric positive definite
* and tridiagonal, and provides error bounds and backward error
* estimates for the solution.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The n diagonal elements of the tridiagonal matrix A.
*
* E (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) subdiagonal elements of the tridiagonal matrix A.
*
* DF (input) DOUBLE PRECISION array, dimension (N)
* The n diagonal elements of the diagonal matrix D from the
* factorization computed by DPTTRF.
*
* EF (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) subdiagonal elements of the unit bidiagonal factor
* L from the factorization computed by DPTTRF.
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by DPTTRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j).
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (2*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param nrhs
* @param d
* @param e
* @param df
* @param ef
* @param b
* @param ldb
* @param x
* @param ldx
* @param ferr
* @param berr
* @param work
* @param info
*
*/
abstract public void dptrfs(int n, int nrhs, double[] d, double[] e, double[] df, double[] ef, double[] b, int ldb, double[] x, int ldx, double[] ferr, double[] berr, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPTRFS improves the computed solution to a system of linear
* equations when the coefficient matrix is symmetric positive definite
* and tridiagonal, and provides error bounds and backward error
* estimates for the solution.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The n diagonal elements of the tridiagonal matrix A.
*
* E (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) subdiagonal elements of the tridiagonal matrix A.
*
* DF (input) DOUBLE PRECISION array, dimension (N)
* The n diagonal elements of the diagonal matrix D from the
* factorization computed by DPTTRF.
*
* EF (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) subdiagonal elements of the unit bidiagonal factor
* L from the factorization computed by DPTTRF.
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by DPTTRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j).
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (2*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param nrhs
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param df
* @param _df_offset
* @param ef
* @param _ef_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dptrfs(int n, int nrhs, double[] d, int _d_offset, double[] e, int _e_offset, double[] df, int _df_offset, double[] ef, int _ef_offset, double[] b, int _b_offset, int ldb, double[] x, int _x_offset, int ldx, double[] ferr, int _ferr_offset, double[] berr, int _berr_offset, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPTSV computes the solution to a real system of linear equations
* A*X = B, where A is an N-by-N symmetric positive definite tridiagonal
* matrix, and X and B are N-by-NRHS matrices.
*
* A is factored as A = L*D*L**T, and the factored form of A is then
* used to solve the system of equations.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the n diagonal elements of the tridiagonal matrix
* A. On exit, the n diagonal elements of the diagonal matrix
* D from the factorization A = L*D*L**T.
*
* E (input/output) DOUBLE PRECISION array, dimension (N-1)
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix A. On exit, the (n-1) subdiagonal elements of the
* unit bidiagonal factor L from the L*D*L**T factorization of
* A. (E can also be regarded as the superdiagonal of the unit
* bidiagonal factor U from the U**T*D*U factorization of A.)
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if INFO = 0, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the leading minor of order i is not
* positive definite, and the solution has not been
* computed. The factorization has not been completed
* unless i = N.
*
* =====================================================================
*
* .. External Subroutines ..
*
*
* @param n
* @param nrhs
* @param d
* @param e
* @param b
* @param ldb
* @param info
*
*/
abstract public void dptsv(int n, int nrhs, double[] d, double[] e, double[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPTSV computes the solution to a real system of linear equations
* A*X = B, where A is an N-by-N symmetric positive definite tridiagonal
* matrix, and X and B are N-by-NRHS matrices.
*
* A is factored as A = L*D*L**T, and the factored form of A is then
* used to solve the system of equations.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the n diagonal elements of the tridiagonal matrix
* A. On exit, the n diagonal elements of the diagonal matrix
* D from the factorization A = L*D*L**T.
*
* E (input/output) DOUBLE PRECISION array, dimension (N-1)
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix A. On exit, the (n-1) subdiagonal elements of the
* unit bidiagonal factor L from the L*D*L**T factorization of
* A. (E can also be regarded as the superdiagonal of the unit
* bidiagonal factor U from the U**T*D*U factorization of A.)
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if INFO = 0, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the leading minor of order i is not
* positive definite, and the solution has not been
* computed. The factorization has not been completed
* unless i = N.
*
* =====================================================================
*
* .. External Subroutines ..
*
*
* @param n
* @param nrhs
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void dptsv(int n, int nrhs, double[] d, int _d_offset, double[] e, int _e_offset, double[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPTSVX uses the factorization A = L*D*L**T to compute the solution
* to a real system of linear equations A*X = B, where A is an N-by-N
* symmetric positive definite tridiagonal matrix and X and B are
* N-by-NRHS matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed:
*
* 1. If FACT = 'N', the matrix A is factored as A = L*D*L**T, where L
* is a unit lower bidiagonal matrix and D is diagonal. The
* factorization can also be regarded as having the form
* A = U**T*D*U.
*
* 2. If the leading i-by-i principal minor is not positive definite,
* then the routine returns with INFO = i. Otherwise, the factored
* form of A is used to estimate the condition number of the matrix
* A. If the reciprocal of the condition number is less than machine
* precision, INFO = N+1 is returned as a warning, but the routine
* still goes on to solve for X and compute error bounds as
* described below.
*
* 3. The system of equations is solved for X using the factored form
* of A.
*
* 4. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of A has been
* supplied on entry.
* = 'F': On entry, DF and EF contain the factored form of A.
* D, E, DF, and EF will not be modified.
* = 'N': The matrix A will be copied to DF and EF and
* factored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The n diagonal elements of the tridiagonal matrix A.
*
* E (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) subdiagonal elements of the tridiagonal matrix A.
*
* DF (input or output) DOUBLE PRECISION array, dimension (N)
* If FACT = 'F', then DF is an input argument and on entry
* contains the n diagonal elements of the diagonal matrix D
* from the L*D*L**T factorization of A.
* If FACT = 'N', then DF is an output argument and on exit
* contains the n diagonal elements of the diagonal matrix D
* from the L*D*L**T factorization of A.
*
* EF (input or output) DOUBLE PRECISION array, dimension (N-1)
* If FACT = 'F', then EF is an input argument and on entry
* contains the (n-1) subdiagonal elements of the unit
* bidiagonal factor L from the L*D*L**T factorization of A.
* If FACT = 'N', then EF is an output argument and on exit
* contains the (n-1) subdiagonal elements of the unit
* bidiagonal factor L from the L*D*L**T factorization of A.
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The N-by-NRHS right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* If INFO = 0 of INFO = N+1, the N-by-NRHS solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) DOUBLE PRECISION
* The reciprocal condition number of the matrix A. If RCOND
* is less than the machine precision (in particular, if
* RCOND = 0), the matrix is singular to working precision.
* This condition is indicated by a return code of INFO > 0.
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j).
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in any
* element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (2*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= N: the leading minor of order i of A is
* not positive definite, so the factorization
* could not be completed, and the solution has not
* been computed. RCOND = 0 is returned.
* = N+1: U is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param n
* @param nrhs
* @param d
* @param e
* @param df
* @param ef
* @param b
* @param ldb
* @param x
* @param ldx
* @param rcond
* @param ferr
* @param berr
* @param work
* @param info
*
*/
abstract public void dptsvx(java.lang.String fact, int n, int nrhs, double[] d, double[] e, double[] df, double[] ef, double[] b, int ldb, double[] x, int ldx, org.netlib.util.doubleW rcond, double[] ferr, double[] berr, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPTSVX uses the factorization A = L*D*L**T to compute the solution
* to a real system of linear equations A*X = B, where A is an N-by-N
* symmetric positive definite tridiagonal matrix and X and B are
* N-by-NRHS matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed:
*
* 1. If FACT = 'N', the matrix A is factored as A = L*D*L**T, where L
* is a unit lower bidiagonal matrix and D is diagonal. The
* factorization can also be regarded as having the form
* A = U**T*D*U.
*
* 2. If the leading i-by-i principal minor is not positive definite,
* then the routine returns with INFO = i. Otherwise, the factored
* form of A is used to estimate the condition number of the matrix
* A. If the reciprocal of the condition number is less than machine
* precision, INFO = N+1 is returned as a warning, but the routine
* still goes on to solve for X and compute error bounds as
* described below.
*
* 3. The system of equations is solved for X using the factored form
* of A.
*
* 4. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of A has been
* supplied on entry.
* = 'F': On entry, DF and EF contain the factored form of A.
* D, E, DF, and EF will not be modified.
* = 'N': The matrix A will be copied to DF and EF and
* factored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The n diagonal elements of the tridiagonal matrix A.
*
* E (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) subdiagonal elements of the tridiagonal matrix A.
*
* DF (input or output) DOUBLE PRECISION array, dimension (N)
* If FACT = 'F', then DF is an input argument and on entry
* contains the n diagonal elements of the diagonal matrix D
* from the L*D*L**T factorization of A.
* If FACT = 'N', then DF is an output argument and on exit
* contains the n diagonal elements of the diagonal matrix D
* from the L*D*L**T factorization of A.
*
* EF (input or output) DOUBLE PRECISION array, dimension (N-1)
* If FACT = 'F', then EF is an input argument and on entry
* contains the (n-1) subdiagonal elements of the unit
* bidiagonal factor L from the L*D*L**T factorization of A.
* If FACT = 'N', then EF is an output argument and on exit
* contains the (n-1) subdiagonal elements of the unit
* bidiagonal factor L from the L*D*L**T factorization of A.
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The N-by-NRHS right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* If INFO = 0 of INFO = N+1, the N-by-NRHS solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) DOUBLE PRECISION
* The reciprocal condition number of the matrix A. If RCOND
* is less than the machine precision (in particular, if
* RCOND = 0), the matrix is singular to working precision.
* This condition is indicated by a return code of INFO > 0.
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j).
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in any
* element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (2*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= N: the leading minor of order i of A is
* not positive definite, so the factorization
* could not be completed, and the solution has not
* been computed. RCOND = 0 is returned.
* = N+1: U is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param n
* @param nrhs
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param df
* @param _df_offset
* @param ef
* @param _ef_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param rcond
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dptsvx(java.lang.String fact, int n, int nrhs, double[] d, int _d_offset, double[] e, int _e_offset, double[] df, int _df_offset, double[] ef, int _ef_offset, double[] b, int _b_offset, int ldb, double[] x, int _x_offset, int ldx, org.netlib.util.doubleW rcond, double[] ferr, int _ferr_offset, double[] berr, int _berr_offset, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPTTRF computes the L*D*L' factorization of a real symmetric
* positive definite tridiagonal matrix A. The factorization may also
* be regarded as having the form A = U'*D*U.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the n diagonal elements of the tridiagonal matrix
* A. On exit, the n diagonal elements of the diagonal matrix
* D from the L*D*L' factorization of A.
*
* E (input/output) DOUBLE PRECISION array, dimension (N-1)
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix A. On exit, the (n-1) subdiagonal elements of the
* unit bidiagonal factor L from the L*D*L' factorization of A.
* E can also be regarded as the superdiagonal of the unit
* bidiagonal factor U from the U'*D*U factorization of A.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
* > 0: if INFO = k, the leading minor of order k is not
* positive definite; if k < N, the factorization could not
* be completed, while if k = N, the factorization was
* completed, but D(N) <= 0.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param e
* @param info
*
*/
abstract public void dpttrf(int n, double[] d, double[] e, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPTTRF computes the L*D*L' factorization of a real symmetric
* positive definite tridiagonal matrix A. The factorization may also
* be regarded as having the form A = U'*D*U.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the n diagonal elements of the tridiagonal matrix
* A. On exit, the n diagonal elements of the diagonal matrix
* D from the L*D*L' factorization of A.
*
* E (input/output) DOUBLE PRECISION array, dimension (N-1)
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix A. On exit, the (n-1) subdiagonal elements of the
* unit bidiagonal factor L from the L*D*L' factorization of A.
* E can also be regarded as the superdiagonal of the unit
* bidiagonal factor U from the U'*D*U factorization of A.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
* > 0: if INFO = k, the leading minor of order k is not
* positive definite; if k < N, the factorization could not
* be completed, while if k = N, the factorization was
* completed, but D(N) <= 0.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param info
*
*/
abstract public void dpttrf(int n, double[] d, int _d_offset, double[] e, int _e_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPTTRS solves a tridiagonal system of the form
* A * X = B
* using the L*D*L' factorization of A computed by DPTTRF. D is a
* diagonal matrix specified in the vector D, L is a unit bidiagonal
* matrix whose subdiagonal is specified in the vector E, and X and B
* are N by NRHS matrices.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the tridiagonal matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The n diagonal elements of the diagonal matrix D from the
* L*D*L' factorization of A.
*
* E (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) subdiagonal elements of the unit bidiagonal factor
* L from the L*D*L' factorization of A. E can also be regarded
* as the superdiagonal of the unit bidiagonal factor U from the
* factorization A = U'*D*U.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the right hand side vectors B for the system of
* linear equations.
* On exit, the solution vectors, X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n
* @param nrhs
* @param d
* @param e
* @param b
* @param ldb
* @param info
*
*/
abstract public void dpttrs(int n, int nrhs, double[] d, double[] e, double[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPTTRS solves a tridiagonal system of the form
* A * X = B
* using the L*D*L' factorization of A computed by DPTTRF. D is a
* diagonal matrix specified in the vector D, L is a unit bidiagonal
* matrix whose subdiagonal is specified in the vector E, and X and B
* are N by NRHS matrices.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the tridiagonal matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The n diagonal elements of the diagonal matrix D from the
* L*D*L' factorization of A.
*
* E (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) subdiagonal elements of the unit bidiagonal factor
* L from the L*D*L' factorization of A. E can also be regarded
* as the superdiagonal of the unit bidiagonal factor U from the
* factorization A = U'*D*U.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the right hand side vectors B for the system of
* linear equations.
* On exit, the solution vectors, X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n
* @param nrhs
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void dpttrs(int n, int nrhs, double[] d, int _d_offset, double[] e, int _e_offset, double[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DPTTS2 solves a tridiagonal system of the form
* A * X = B
* using the L*D*L' factorization of A computed by DPTTRF. D is a
* diagonal matrix specified in the vector D, L is a unit bidiagonal
* matrix whose subdiagonal is specified in the vector E, and X and B
* are N by NRHS matrices.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the tridiagonal matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The n diagonal elements of the diagonal matrix D from the
* L*D*L' factorization of A.
*
* E (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) subdiagonal elements of the unit bidiagonal factor
* L from the L*D*L' factorization of A. E can also be regarded
* as the superdiagonal of the unit bidiagonal factor U from the
* factorization A = U'*D*U.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the right hand side vectors B for the system of
* linear equations.
* On exit, the solution vectors, X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n
* @param nrhs
* @param d
* @param e
* @param b
* @param ldb
*
*/
abstract public void dptts2(int n, int nrhs, double[] d, double[] e, double[] b, int ldb);
/**
*
* ..
*
* Purpose
* =======
*
* DPTTS2 solves a tridiagonal system of the form
* A * X = B
* using the L*D*L' factorization of A computed by DPTTRF. D is a
* diagonal matrix specified in the vector D, L is a unit bidiagonal
* matrix whose subdiagonal is specified in the vector E, and X and B
* are N by NRHS matrices.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the tridiagonal matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The n diagonal elements of the diagonal matrix D from the
* L*D*L' factorization of A.
*
* E (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) subdiagonal elements of the unit bidiagonal factor
* L from the L*D*L' factorization of A. E can also be regarded
* as the superdiagonal of the unit bidiagonal factor U from the
* factorization A = U'*D*U.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the right hand side vectors B for the system of
* linear equations.
* On exit, the solution vectors, X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n
* @param nrhs
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param b
* @param _b_offset
* @param ldb
*
*/
abstract public void dptts2(int n, int nrhs, double[] d, int _d_offset, double[] e, int _e_offset, double[] b, int _b_offset, int ldb);
/**
*
* ..
*
* Purpose
* =======
*
* DRSCL multiplies an n-element real vector x by the real scalar 1/a.
* This is done without overflow or underflow as long as
* the final result x/a does not overflow or underflow.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of components of the vector x.
*
* SA (input) DOUBLE PRECISION
* The scalar a which is used to divide each component of x.
* SA must be >= 0, or the subroutine will divide by zero.
*
* SX (input/output) DOUBLE PRECISION array, dimension
* (1+(N-1)*abs(INCX))
* The n-element vector x.
*
* INCX (input) INTEGER
* The increment between successive values of the vector SX.
* > 0: SX(1) = X(1) and SX(1+(i-1)*INCX) = x(i), 1< i<= n
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param sa
* @param sx
* @param incx
*
*/
abstract public void drscl(int n, double sa, double[] sx, int incx);
/**
*
* ..
*
* Purpose
* =======
*
* DRSCL multiplies an n-element real vector x by the real scalar 1/a.
* This is done without overflow or underflow as long as
* the final result x/a does not overflow or underflow.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of components of the vector x.
*
* SA (input) DOUBLE PRECISION
* The scalar a which is used to divide each component of x.
* SA must be >= 0, or the subroutine will divide by zero.
*
* SX (input/output) DOUBLE PRECISION array, dimension
* (1+(N-1)*abs(INCX))
* The n-element vector x.
*
* INCX (input) INTEGER
* The increment between successive values of the vector SX.
* > 0: SX(1) = X(1) and SX(1+(i-1)*INCX) = x(i), 1< i<= n
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param sa
* @param sx
* @param _sx_offset
* @param incx
*
*/
abstract public void drscl(int n, double sa, double[] sx, int _sx_offset, int incx);
/**
*
* ..
*
* Purpose
* =======
*
* DSBEV computes all the eigenvalues and, optionally, eigenvectors of
* a real symmetric band matrix A.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* On exit, AB is overwritten by values generated during the
* reduction to tridiagonal form. If UPLO = 'U', the first
* superdiagonal and the diagonal of the tridiagonal matrix T
* are returned in rows KD and KD+1 of AB, and if UPLO = 'L',
* the diagonal and first subdiagonal of T are returned in the
* first two rows of AB.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD + 1.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the orthonormal
* eigenvectors of the matrix A, with the i-th column of Z
* holding the eigenvector associated with W(i).
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (max(1,3*N-2))
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the algorithm failed to converge; i
* off-diagonal elements of an intermediate tridiagonal
* form did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param uplo
* @param n
* @param kd
* @param ab
* @param ldab
* @param w
* @param z
* @param ldz
* @param work
* @param info
*
*/
abstract public void dsbev(java.lang.String jobz, java.lang.String uplo, int n, int kd, double[] ab, int ldab, double[] w, double[] z, int ldz, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSBEV computes all the eigenvalues and, optionally, eigenvectors of
* a real symmetric band matrix A.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* On exit, AB is overwritten by values generated during the
* reduction to tridiagonal form. If UPLO = 'U', the first
* superdiagonal and the diagonal of the tridiagonal matrix T
* are returned in rows KD and KD+1 of AB, and if UPLO = 'L',
* the diagonal and first subdiagonal of T are returned in the
* first two rows of AB.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD + 1.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the orthonormal
* eigenvectors of the matrix A, with the i-th column of Z
* holding the eigenvector associated with W(i).
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (max(1,3*N-2))
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the algorithm failed to converge; i
* off-diagonal elements of an intermediate tridiagonal
* form did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param uplo
* @param n
* @param kd
* @param ab
* @param _ab_offset
* @param ldab
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dsbev(java.lang.String jobz, java.lang.String uplo, int n, int kd, double[] ab, int _ab_offset, int ldab, double[] w, int _w_offset, double[] z, int _z_offset, int ldz, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSBEVD computes all the eigenvalues and, optionally, eigenvectors of
* a real symmetric band matrix A. If eigenvectors are desired, it uses
* a divide and conquer algorithm.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* On exit, AB is overwritten by values generated during the
* reduction to tridiagonal form. If UPLO = 'U', the first
* superdiagonal and the diagonal of the tridiagonal matrix T
* are returned in rows KD and KD+1 of AB, and if UPLO = 'L',
* the diagonal and first subdiagonal of T are returned in the
* first two rows of AB.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD + 1.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the orthonormal
* eigenvectors of the matrix A, with the i-th column of Z
* holding the eigenvector associated with W(i).
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace/output) DOUBLE PRECISION array,
* dimension (LWORK)
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* IF N <= 1, LWORK must be at least 1.
* If JOBZ = 'N' and N > 2, LWORK must be at least 2*N.
* If JOBZ = 'V' and N > 2, LWORK must be at least
* ( 1 + 5*N + 2*N**2 ).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array LIWORK.
* If JOBZ = 'N' or N <= 1, LIWORK must be at least 1.
* If JOBZ = 'V' and N > 2, LIWORK must be at least 3 + 5*N.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the algorithm failed to converge; i
* off-diagonal elements of an intermediate tridiagonal
* form did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param uplo
* @param n
* @param kd
* @param ab
* @param ldab
* @param w
* @param z
* @param ldz
* @param work
* @param lwork
* @param iwork
* @param liwork
* @param info
*
*/
abstract public void dsbevd(java.lang.String jobz, java.lang.String uplo, int n, int kd, double[] ab, int ldab, double[] w, double[] z, int ldz, double[] work, int lwork, int[] iwork, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSBEVD computes all the eigenvalues and, optionally, eigenvectors of
* a real symmetric band matrix A. If eigenvectors are desired, it uses
* a divide and conquer algorithm.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* On exit, AB is overwritten by values generated during the
* reduction to tridiagonal form. If UPLO = 'U', the first
* superdiagonal and the diagonal of the tridiagonal matrix T
* are returned in rows KD and KD+1 of AB, and if UPLO = 'L',
* the diagonal and first subdiagonal of T are returned in the
* first two rows of AB.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD + 1.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the orthonormal
* eigenvectors of the matrix A, with the i-th column of Z
* holding the eigenvector associated with W(i).
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace/output) DOUBLE PRECISION array,
* dimension (LWORK)
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* IF N <= 1, LWORK must be at least 1.
* If JOBZ = 'N' and N > 2, LWORK must be at least 2*N.
* If JOBZ = 'V' and N > 2, LWORK must be at least
* ( 1 + 5*N + 2*N**2 ).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array LIWORK.
* If JOBZ = 'N' or N <= 1, LIWORK must be at least 1.
* If JOBZ = 'V' and N > 2, LIWORK must be at least 3 + 5*N.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the algorithm failed to converge; i
* off-diagonal elements of an intermediate tridiagonal
* form did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param uplo
* @param n
* @param kd
* @param ab
* @param _ab_offset
* @param ldab
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param liwork
* @param info
*
*/
abstract public void dsbevd(java.lang.String jobz, java.lang.String uplo, int n, int kd, double[] ab, int _ab_offset, int ldab, double[] w, int _w_offset, double[] z, int _z_offset, int ldz, double[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSBEVX computes selected eigenvalues and, optionally, eigenvectors
* of a real symmetric band matrix A. Eigenvalues and eigenvectors can
* be selected by specifying either a range of values or a range of
* indices for the desired eigenvalues.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found;
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found;
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* On exit, AB is overwritten by values generated during the
* reduction to tridiagonal form. If UPLO = 'U', the first
* superdiagonal and the diagonal of the tridiagonal matrix T
* are returned in rows KD and KD+1 of AB, and if UPLO = 'L',
* the diagonal and first subdiagonal of T are returned in the
* first two rows of AB.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD + 1.
*
* Q (output) DOUBLE PRECISION array, dimension (LDQ, N)
* If JOBZ = 'V', the N-by-N orthogonal matrix used in the
* reduction to tridiagonal form.
* If JOBZ = 'N', the array Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. If JOBZ = 'V', then
* LDQ >= max(1,N).
*
* VL (input) DOUBLE PRECISION
* VU (input) DOUBLE PRECISION
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) DOUBLE PRECISION
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less than
* or equal to zero, then EPS*|T| will be used in its place,
* where |T| is the 1-norm of the tridiagonal matrix obtained
* by reducing AB to tridiagonal form.
*
* Eigenvalues will be computed most accurately when ABSTOL is
* set to twice the underflow threshold 2*DLAMCH('S'), not zero.
* If this routine returns with INFO>0, indicating that some
* eigenvectors did not converge, try setting ABSTOL to
* 2*DLAMCH('S').
*
* See "Computing Small Singular Values of Bidiagonal Matrices
* with Guaranteed High Relative Accuracy," by Demmel and
* Kahan, LAPACK Working Note #3.
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* The first M elements contain the selected eigenvalues in
* ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, max(1,M))
* If JOBZ = 'V', then if INFO = 0, the first M columns of Z
* contain the orthonormal eigenvectors of the matrix A
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* If an eigenvector fails to converge, then that column of Z
* contains the latest approximation to the eigenvector, and the
* index of the eigenvector is returned in IFAIL.
* If JOBZ = 'N', then Z is not referenced.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (7*N)
*
* IWORK (workspace) INTEGER array, dimension (5*N)
*
* IFAIL (output) INTEGER array, dimension (N)
* If JOBZ = 'V', then if INFO = 0, the first M elements of
* IFAIL are zero. If INFO > 0, then IFAIL contains the
* indices of the eigenvectors that failed to converge.
* If JOBZ = 'N', then IFAIL is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, then i eigenvectors failed to converge.
* Their indices are stored in array IFAIL.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param range
* @param uplo
* @param n
* @param kd
* @param ab
* @param ldab
* @param q
* @param ldq
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param z
* @param ldz
* @param work
* @param iwork
* @param ifail
* @param info
*
*/
abstract public void dsbevx(java.lang.String jobz, java.lang.String range, java.lang.String uplo, int n, int kd, double[] ab, int ldab, double[] q, int ldq, double vl, double vu, int il, int iu, double abstol, org.netlib.util.intW m, double[] w, double[] z, int ldz, double[] work, int[] iwork, int[] ifail, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSBEVX computes selected eigenvalues and, optionally, eigenvectors
* of a real symmetric band matrix A. Eigenvalues and eigenvectors can
* be selected by specifying either a range of values or a range of
* indices for the desired eigenvalues.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found;
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found;
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* On exit, AB is overwritten by values generated during the
* reduction to tridiagonal form. If UPLO = 'U', the first
* superdiagonal and the diagonal of the tridiagonal matrix T
* are returned in rows KD and KD+1 of AB, and if UPLO = 'L',
* the diagonal and first subdiagonal of T are returned in the
* first two rows of AB.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD + 1.
*
* Q (output) DOUBLE PRECISION array, dimension (LDQ, N)
* If JOBZ = 'V', the N-by-N orthogonal matrix used in the
* reduction to tridiagonal form.
* If JOBZ = 'N', the array Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. If JOBZ = 'V', then
* LDQ >= max(1,N).
*
* VL (input) DOUBLE PRECISION
* VU (input) DOUBLE PRECISION
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) DOUBLE PRECISION
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less than
* or equal to zero, then EPS*|T| will be used in its place,
* where |T| is the 1-norm of the tridiagonal matrix obtained
* by reducing AB to tridiagonal form.
*
* Eigenvalues will be computed most accurately when ABSTOL is
* set to twice the underflow threshold 2*DLAMCH('S'), not zero.
* If this routine returns with INFO>0, indicating that some
* eigenvectors did not converge, try setting ABSTOL to
* 2*DLAMCH('S').
*
* See "Computing Small Singular Values of Bidiagonal Matrices
* with Guaranteed High Relative Accuracy," by Demmel and
* Kahan, LAPACK Working Note #3.
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* The first M elements contain the selected eigenvalues in
* ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, max(1,M))
* If JOBZ = 'V', then if INFO = 0, the first M columns of Z
* contain the orthonormal eigenvectors of the matrix A
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* If an eigenvector fails to converge, then that column of Z
* contains the latest approximation to the eigenvector, and the
* index of the eigenvector is returned in IFAIL.
* If JOBZ = 'N', then Z is not referenced.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (7*N)
*
* IWORK (workspace) INTEGER array, dimension (5*N)
*
* IFAIL (output) INTEGER array, dimension (N)
* If JOBZ = 'V', then if INFO = 0, the first M elements of
* IFAIL are zero. If INFO > 0, then IFAIL contains the
* indices of the eigenvectors that failed to converge.
* If JOBZ = 'N', then IFAIL is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, then i eigenvectors failed to converge.
* Their indices are stored in array IFAIL.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param range
* @param uplo
* @param n
* @param kd
* @param ab
* @param _ab_offset
* @param ldab
* @param q
* @param _q_offset
* @param ldq
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param ifail
* @param _ifail_offset
* @param info
*
*/
abstract public void dsbevx(java.lang.String jobz, java.lang.String range, java.lang.String uplo, int n, int kd, double[] ab, int _ab_offset, int ldab, double[] q, int _q_offset, int ldq, double vl, double vu, int il, int iu, double abstol, org.netlib.util.intW m, double[] w, int _w_offset, double[] z, int _z_offset, int ldz, double[] work, int _work_offset, int[] iwork, int _iwork_offset, int[] ifail, int _ifail_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSBGST reduces a real symmetric-definite banded generalized
* eigenproblem A*x = lambda*B*x to standard form C*y = lambda*y,
* such that C has the same bandwidth as A.
*
* B must have been previously factorized as S**T*S by DPBSTF, using a
* split Cholesky factorization. A is overwritten by C = X**T*A*X, where
* X = S**(-1)*Q and Q is an orthogonal matrix chosen to preserve the
* bandwidth of A.
*
* Arguments
* =========
*
* VECT (input) CHARACTER*1
* = 'N': do not form the transformation matrix X;
* = 'V': form X.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* KA (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KA >= 0.
*
* KB (input) INTEGER
* The number of superdiagonals of the matrix B if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KA >= KB >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB,N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first ka+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(ka+1+i-j,j) = A(i,j) for max(1,j-ka)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+ka).
*
* On exit, the transformed matrix X**T*A*X, stored in the same
* format as A.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KA+1.
*
* BB (input) DOUBLE PRECISION array, dimension (LDBB,N)
* The banded factor S from the split Cholesky factorization of
* B, as returned by DPBSTF, stored in the first KB+1 rows of
* the array.
*
* LDBB (input) INTEGER
* The leading dimension of the array BB. LDBB >= KB+1.
*
* X (output) DOUBLE PRECISION array, dimension (LDX,N)
* If VECT = 'V', the n-by-n matrix X.
* If VECT = 'N', the array X is not referenced.
*
* LDX (input) INTEGER
* The leading dimension of the array X.
* LDX >= max(1,N) if VECT = 'V'; LDX >= 1 otherwise.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (2*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param vect
* @param uplo
* @param n
* @param ka
* @param kb
* @param ab
* @param ldab
* @param bb
* @param ldbb
* @param x
* @param ldx
* @param work
* @param info
*
*/
abstract public void dsbgst(java.lang.String vect, java.lang.String uplo, int n, int ka, int kb, double[] ab, int ldab, double[] bb, int ldbb, double[] x, int ldx, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSBGST reduces a real symmetric-definite banded generalized
* eigenproblem A*x = lambda*B*x to standard form C*y = lambda*y,
* such that C has the same bandwidth as A.
*
* B must have been previously factorized as S**T*S by DPBSTF, using a
* split Cholesky factorization. A is overwritten by C = X**T*A*X, where
* X = S**(-1)*Q and Q is an orthogonal matrix chosen to preserve the
* bandwidth of A.
*
* Arguments
* =========
*
* VECT (input) CHARACTER*1
* = 'N': do not form the transformation matrix X;
* = 'V': form X.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* KA (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KA >= 0.
*
* KB (input) INTEGER
* The number of superdiagonals of the matrix B if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KA >= KB >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB,N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first ka+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(ka+1+i-j,j) = A(i,j) for max(1,j-ka)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+ka).
*
* On exit, the transformed matrix X**T*A*X, stored in the same
* format as A.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KA+1.
*
* BB (input) DOUBLE PRECISION array, dimension (LDBB,N)
* The banded factor S from the split Cholesky factorization of
* B, as returned by DPBSTF, stored in the first KB+1 rows of
* the array.
*
* LDBB (input) INTEGER
* The leading dimension of the array BB. LDBB >= KB+1.
*
* X (output) DOUBLE PRECISION array, dimension (LDX,N)
* If VECT = 'V', the n-by-n matrix X.
* If VECT = 'N', the array X is not referenced.
*
* LDX (input) INTEGER
* The leading dimension of the array X.
* LDX >= max(1,N) if VECT = 'V'; LDX >= 1 otherwise.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (2*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param vect
* @param uplo
* @param n
* @param ka
* @param kb
* @param ab
* @param _ab_offset
* @param ldab
* @param bb
* @param _bb_offset
* @param ldbb
* @param x
* @param _x_offset
* @param ldx
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dsbgst(java.lang.String vect, java.lang.String uplo, int n, int ka, int kb, double[] ab, int _ab_offset, int ldab, double[] bb, int _bb_offset, int ldbb, double[] x, int _x_offset, int ldx, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSBGV computes all the eigenvalues, and optionally, the eigenvectors
* of a real generalized symmetric-definite banded eigenproblem, of
* the form A*x=(lambda)*B*x. Here A and B are assumed to be symmetric
* and banded, and B is also positive definite.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangles of A and B are stored;
* = 'L': Lower triangles of A and B are stored.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* KA (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KA >= 0.
*
* KB (input) INTEGER
* The number of superdiagonals of the matrix B if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KB >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first ka+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(ka+1+i-j,j) = A(i,j) for max(1,j-ka)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+ka).
*
* On exit, the contents of AB are destroyed.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KA+1.
*
* BB (input/output) DOUBLE PRECISION array, dimension (LDBB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix B, stored in the first kb+1 rows of the array. The
* j-th column of B is stored in the j-th column of the array BB
* as follows:
* if UPLO = 'U', BB(kb+1+i-j,j) = B(i,j) for max(1,j-kb)<=i<=j;
* if UPLO = 'L', BB(1+i-j,j) = B(i,j) for j<=i<=min(n,j+kb).
*
* On exit, the factor S from the split Cholesky factorization
* B = S**T*S, as returned by DPBSTF.
*
* LDBB (input) INTEGER
* The leading dimension of the array BB. LDBB >= KB+1.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the matrix Z of
* eigenvectors, with the i-th column of Z holding the
* eigenvector associated with W(i). The eigenvectors are
* normalized so that Z**T*B*Z = I.
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= N.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is:
* <= N: the algorithm failed to converge:
* i off-diagonal elements of an intermediate
* tridiagonal form did not converge to zero;
* > N: if INFO = N + i, for 1 <= i <= N, then DPBSTF
* returned INFO = i: B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param jobz
* @param uplo
* @param n
* @param ka
* @param kb
* @param ab
* @param ldab
* @param bb
* @param ldbb
* @param w
* @param z
* @param ldz
* @param work
* @param info
*
*/
abstract public void dsbgv(java.lang.String jobz, java.lang.String uplo, int n, int ka, int kb, double[] ab, int ldab, double[] bb, int ldbb, double[] w, double[] z, int ldz, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSBGV computes all the eigenvalues, and optionally, the eigenvectors
* of a real generalized symmetric-definite banded eigenproblem, of
* the form A*x=(lambda)*B*x. Here A and B are assumed to be symmetric
* and banded, and B is also positive definite.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangles of A and B are stored;
* = 'L': Lower triangles of A and B are stored.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* KA (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KA >= 0.
*
* KB (input) INTEGER
* The number of superdiagonals of the matrix B if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KB >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first ka+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(ka+1+i-j,j) = A(i,j) for max(1,j-ka)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+ka).
*
* On exit, the contents of AB are destroyed.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KA+1.
*
* BB (input/output) DOUBLE PRECISION array, dimension (LDBB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix B, stored in the first kb+1 rows of the array. The
* j-th column of B is stored in the j-th column of the array BB
* as follows:
* if UPLO = 'U', BB(kb+1+i-j,j) = B(i,j) for max(1,j-kb)<=i<=j;
* if UPLO = 'L', BB(1+i-j,j) = B(i,j) for j<=i<=min(n,j+kb).
*
* On exit, the factor S from the split Cholesky factorization
* B = S**T*S, as returned by DPBSTF.
*
* LDBB (input) INTEGER
* The leading dimension of the array BB. LDBB >= KB+1.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the matrix Z of
* eigenvectors, with the i-th column of Z holding the
* eigenvector associated with W(i). The eigenvectors are
* normalized so that Z**T*B*Z = I.
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= N.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is:
* <= N: the algorithm failed to converge:
* i off-diagonal elements of an intermediate
* tridiagonal form did not converge to zero;
* > N: if INFO = N + i, for 1 <= i <= N, then DPBSTF
* returned INFO = i: B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param jobz
* @param uplo
* @param n
* @param ka
* @param kb
* @param ab
* @param _ab_offset
* @param ldab
* @param bb
* @param _bb_offset
* @param ldbb
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dsbgv(java.lang.String jobz, java.lang.String uplo, int n, int ka, int kb, double[] ab, int _ab_offset, int ldab, double[] bb, int _bb_offset, int ldbb, double[] w, int _w_offset, double[] z, int _z_offset, int ldz, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSBGVD computes all the eigenvalues, and optionally, the eigenvectors
* of a real generalized symmetric-definite banded eigenproblem, of the
* form A*x=(lambda)*B*x. Here A and B are assumed to be symmetric and
* banded, and B is also positive definite. If eigenvectors are
* desired, it uses a divide and conquer algorithm.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangles of A and B are stored;
* = 'L': Lower triangles of A and B are stored.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* KA (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KA >= 0.
*
* KB (input) INTEGER
* The number of superdiagonals of the matrix B if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KB >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first ka+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(ka+1+i-j,j) = A(i,j) for max(1,j-ka)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+ka).
*
* On exit, the contents of AB are destroyed.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KA+1.
*
* BB (input/output) DOUBLE PRECISION array, dimension (LDBB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix B, stored in the first kb+1 rows of the array. The
* j-th column of B is stored in the j-th column of the array BB
* as follows:
* if UPLO = 'U', BB(ka+1+i-j,j) = B(i,j) for max(1,j-kb)<=i<=j;
* if UPLO = 'L', BB(1+i-j,j) = B(i,j) for j<=i<=min(n,j+kb).
*
* On exit, the factor S from the split Cholesky factorization
* B = S**T*S, as returned by DPBSTF.
*
* LDBB (input) INTEGER
* The leading dimension of the array BB. LDBB >= KB+1.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the matrix Z of
* eigenvectors, with the i-th column of Z holding the
* eigenvector associated with W(i). The eigenvectors are
* normalized so Z**T*B*Z = I.
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If N <= 1, LWORK >= 1.
* If JOBZ = 'N' and N > 1, LWORK >= 3*N.
* If JOBZ = 'V' and N > 1, LWORK >= 1 + 5*N + 2*N**2.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if LIWORK > 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If JOBZ = 'N' or N <= 1, LIWORK >= 1.
* If JOBZ = 'V' and N > 1, LIWORK >= 3 + 5*N.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is:
* <= N: the algorithm failed to converge:
* i off-diagonal elements of an intermediate
* tridiagonal form did not converge to zero;
* > N: if INFO = N + i, for 1 <= i <= N, then DPBSTF
* returned INFO = i: B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* Further Details
* ===============
*
* Based on contributions by
* Mark Fahey, Department of Mathematics, Univ. of Kentucky, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param uplo
* @param n
* @param ka
* @param kb
* @param ab
* @param ldab
* @param bb
* @param ldbb
* @param w
* @param z
* @param ldz
* @param work
* @param lwork
* @param iwork
* @param liwork
* @param info
*
*/
abstract public void dsbgvd(java.lang.String jobz, java.lang.String uplo, int n, int ka, int kb, double[] ab, int ldab, double[] bb, int ldbb, double[] w, double[] z, int ldz, double[] work, int lwork, int[] iwork, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSBGVD computes all the eigenvalues, and optionally, the eigenvectors
* of a real generalized symmetric-definite banded eigenproblem, of the
* form A*x=(lambda)*B*x. Here A and B are assumed to be symmetric and
* banded, and B is also positive definite. If eigenvectors are
* desired, it uses a divide and conquer algorithm.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangles of A and B are stored;
* = 'L': Lower triangles of A and B are stored.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* KA (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KA >= 0.
*
* KB (input) INTEGER
* The number of superdiagonals of the matrix B if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KB >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first ka+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(ka+1+i-j,j) = A(i,j) for max(1,j-ka)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+ka).
*
* On exit, the contents of AB are destroyed.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KA+1.
*
* BB (input/output) DOUBLE PRECISION array, dimension (LDBB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix B, stored in the first kb+1 rows of the array. The
* j-th column of B is stored in the j-th column of the array BB
* as follows:
* if UPLO = 'U', BB(ka+1+i-j,j) = B(i,j) for max(1,j-kb)<=i<=j;
* if UPLO = 'L', BB(1+i-j,j) = B(i,j) for j<=i<=min(n,j+kb).
*
* On exit, the factor S from the split Cholesky factorization
* B = S**T*S, as returned by DPBSTF.
*
* LDBB (input) INTEGER
* The leading dimension of the array BB. LDBB >= KB+1.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the matrix Z of
* eigenvectors, with the i-th column of Z holding the
* eigenvector associated with W(i). The eigenvectors are
* normalized so Z**T*B*Z = I.
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If N <= 1, LWORK >= 1.
* If JOBZ = 'N' and N > 1, LWORK >= 3*N.
* If JOBZ = 'V' and N > 1, LWORK >= 1 + 5*N + 2*N**2.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if LIWORK > 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If JOBZ = 'N' or N <= 1, LIWORK >= 1.
* If JOBZ = 'V' and N > 1, LIWORK >= 3 + 5*N.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is:
* <= N: the algorithm failed to converge:
* i off-diagonal elements of an intermediate
* tridiagonal form did not converge to zero;
* > N: if INFO = N + i, for 1 <= i <= N, then DPBSTF
* returned INFO = i: B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* Further Details
* ===============
*
* Based on contributions by
* Mark Fahey, Department of Mathematics, Univ. of Kentucky, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param uplo
* @param n
* @param ka
* @param kb
* @param ab
* @param _ab_offset
* @param ldab
* @param bb
* @param _bb_offset
* @param ldbb
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param liwork
* @param info
*
*/
abstract public void dsbgvd(java.lang.String jobz, java.lang.String uplo, int n, int ka, int kb, double[] ab, int _ab_offset, int ldab, double[] bb, int _bb_offset, int ldbb, double[] w, int _w_offset, double[] z, int _z_offset, int ldz, double[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSBGVX computes selected eigenvalues, and optionally, eigenvectors
* of a real generalized symmetric-definite banded eigenproblem, of
* the form A*x=(lambda)*B*x. Here A and B are assumed to be symmetric
* and banded, and B is also positive definite. Eigenvalues and
* eigenvectors can be selected by specifying either all eigenvalues,
* a range of values or a range of indices for the desired eigenvalues.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangles of A and B are stored;
* = 'L': Lower triangles of A and B are stored.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* KA (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KA >= 0.
*
* KB (input) INTEGER
* The number of superdiagonals of the matrix B if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KB >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first ka+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(ka+1+i-j,j) = A(i,j) for max(1,j-ka)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+ka).
*
* On exit, the contents of AB are destroyed.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KA+1.
*
* BB (input/output) DOUBLE PRECISION array, dimension (LDBB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix B, stored in the first kb+1 rows of the array. The
* j-th column of B is stored in the j-th column of the array BB
* as follows:
* if UPLO = 'U', BB(ka+1+i-j,j) = B(i,j) for max(1,j-kb)<=i<=j;
* if UPLO = 'L', BB(1+i-j,j) = B(i,j) for j<=i<=min(n,j+kb).
*
* On exit, the factor S from the split Cholesky factorization
* B = S**T*S, as returned by DPBSTF.
*
* LDBB (input) INTEGER
* The leading dimension of the array BB. LDBB >= KB+1.
*
* Q (output) DOUBLE PRECISION array, dimension (LDQ, N)
* If JOBZ = 'V', the n-by-n matrix used in the reduction of
* A*x = (lambda)*B*x to standard form, i.e. C*x = (lambda)*x,
* and consequently C to tridiagonal form.
* If JOBZ = 'N', the array Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. If JOBZ = 'N',
* LDQ >= 1. If JOBZ = 'V', LDQ >= max(1,N).
*
* VL (input) DOUBLE PRECISION
* VU (input) DOUBLE PRECISION
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) DOUBLE PRECISION
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less than
* or equal to zero, then EPS*|T| will be used in its place,
* where |T| is the 1-norm of the tridiagonal matrix obtained
* by reducing A to tridiagonal form.
*
* Eigenvalues will be computed most accurately when ABSTOL is
* set to twice the underflow threshold 2*DLAMCH('S'), not zero.
* If this routine returns with INFO>0, indicating that some
* eigenvectors did not converge, try setting ABSTOL to
* 2*DLAMCH('S').
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the matrix Z of
* eigenvectors, with the i-th column of Z holding the
* eigenvector associated with W(i). The eigenvectors are
* normalized so Z**T*B*Z = I.
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (7*N)
*
* IWORK (workspace/output) INTEGER array, dimension (5*N)
*
* IFAIL (output) INTEGER array, dimension (M)
* If JOBZ = 'V', then if INFO = 0, the first M elements of
* IFAIL are zero. If INFO > 0, then IFAIL contains the
* indices of the eigenvalues that failed to converge.
* If JOBZ = 'N', then IFAIL is not referenced.
*
* INFO (output) INTEGER
* = 0 : successful exit
* < 0 : if INFO = -i, the i-th argument had an illegal value
* <= N: if INFO = i, then i eigenvectors failed to converge.
* Their indices are stored in IFAIL.
* > N : DPBSTF returned an error code; i.e.,
* if INFO = N + i, for 1 <= i <= N, then the leading
* minor of order i of B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* Further Details
* ===============
*
* Based on contributions by
* Mark Fahey, Department of Mathematics, Univ. of Kentucky, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param range
* @param uplo
* @param n
* @param ka
* @param kb
* @param ab
* @param ldab
* @param bb
* @param ldbb
* @param q
* @param ldq
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param z
* @param ldz
* @param work
* @param iwork
* @param ifail
* @param info
*
*/
abstract public void dsbgvx(java.lang.String jobz, java.lang.String range, java.lang.String uplo, int n, int ka, int kb, double[] ab, int ldab, double[] bb, int ldbb, double[] q, int ldq, double vl, double vu, int il, int iu, double abstol, org.netlib.util.intW m, double[] w, double[] z, int ldz, double[] work, int[] iwork, int[] ifail, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSBGVX computes selected eigenvalues, and optionally, eigenvectors
* of a real generalized symmetric-definite banded eigenproblem, of
* the form A*x=(lambda)*B*x. Here A and B are assumed to be symmetric
* and banded, and B is also positive definite. Eigenvalues and
* eigenvectors can be selected by specifying either all eigenvalues,
* a range of values or a range of indices for the desired eigenvalues.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangles of A and B are stored;
* = 'L': Lower triangles of A and B are stored.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* KA (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KA >= 0.
*
* KB (input) INTEGER
* The number of superdiagonals of the matrix B if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KB >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first ka+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(ka+1+i-j,j) = A(i,j) for max(1,j-ka)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+ka).
*
* On exit, the contents of AB are destroyed.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KA+1.
*
* BB (input/output) DOUBLE PRECISION array, dimension (LDBB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix B, stored in the first kb+1 rows of the array. The
* j-th column of B is stored in the j-th column of the array BB
* as follows:
* if UPLO = 'U', BB(ka+1+i-j,j) = B(i,j) for max(1,j-kb)<=i<=j;
* if UPLO = 'L', BB(1+i-j,j) = B(i,j) for j<=i<=min(n,j+kb).
*
* On exit, the factor S from the split Cholesky factorization
* B = S**T*S, as returned by DPBSTF.
*
* LDBB (input) INTEGER
* The leading dimension of the array BB. LDBB >= KB+1.
*
* Q (output) DOUBLE PRECISION array, dimension (LDQ, N)
* If JOBZ = 'V', the n-by-n matrix used in the reduction of
* A*x = (lambda)*B*x to standard form, i.e. C*x = (lambda)*x,
* and consequently C to tridiagonal form.
* If JOBZ = 'N', the array Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. If JOBZ = 'N',
* LDQ >= 1. If JOBZ = 'V', LDQ >= max(1,N).
*
* VL (input) DOUBLE PRECISION
* VU (input) DOUBLE PRECISION
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) DOUBLE PRECISION
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less than
* or equal to zero, then EPS*|T| will be used in its place,
* where |T| is the 1-norm of the tridiagonal matrix obtained
* by reducing A to tridiagonal form.
*
* Eigenvalues will be computed most accurately when ABSTOL is
* set to twice the underflow threshold 2*DLAMCH('S'), not zero.
* If this routine returns with INFO>0, indicating that some
* eigenvectors did not converge, try setting ABSTOL to
* 2*DLAMCH('S').
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the matrix Z of
* eigenvectors, with the i-th column of Z holding the
* eigenvector associated with W(i). The eigenvectors are
* normalized so Z**T*B*Z = I.
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (7*N)
*
* IWORK (workspace/output) INTEGER array, dimension (5*N)
*
* IFAIL (output) INTEGER array, dimension (M)
* If JOBZ = 'V', then if INFO = 0, the first M elements of
* IFAIL are zero. If INFO > 0, then IFAIL contains the
* indices of the eigenvalues that failed to converge.
* If JOBZ = 'N', then IFAIL is not referenced.
*
* INFO (output) INTEGER
* = 0 : successful exit
* < 0 : if INFO = -i, the i-th argument had an illegal value
* <= N: if INFO = i, then i eigenvectors failed to converge.
* Their indices are stored in IFAIL.
* > N : DPBSTF returned an error code; i.e.,
* if INFO = N + i, for 1 <= i <= N, then the leading
* minor of order i of B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* Further Details
* ===============
*
* Based on contributions by
* Mark Fahey, Department of Mathematics, Univ. of Kentucky, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param range
* @param uplo
* @param n
* @param ka
* @param kb
* @param ab
* @param _ab_offset
* @param ldab
* @param bb
* @param _bb_offset
* @param ldbb
* @param q
* @param _q_offset
* @param ldq
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param ifail
* @param _ifail_offset
* @param info
*
*/
abstract public void dsbgvx(java.lang.String jobz, java.lang.String range, java.lang.String uplo, int n, int ka, int kb, double[] ab, int _ab_offset, int ldab, double[] bb, int _bb_offset, int ldbb, double[] q, int _q_offset, int ldq, double vl, double vu, int il, int iu, double abstol, org.netlib.util.intW m, double[] w, int _w_offset, double[] z, int _z_offset, int ldz, double[] work, int _work_offset, int[] iwork, int _iwork_offset, int[] ifail, int _ifail_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSBTRD reduces a real symmetric band matrix A to symmetric
* tridiagonal form T by an orthogonal similarity transformation:
* Q**T * A * Q = T.
*
* Arguments
* =========
*
* VECT (input) CHARACTER*1
* = 'N': do not form Q;
* = 'V': form Q;
* = 'U': update a matrix X, by forming X*Q.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB,N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
* On exit, the diagonal elements of AB are overwritten by the
* diagonal elements of the tridiagonal matrix T; if KD > 0, the
* elements on the first superdiagonal (if UPLO = 'U') or the
* first subdiagonal (if UPLO = 'L') are overwritten by the
* off-diagonal elements of T; the rest of AB is overwritten by
* values generated during the reduction.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* D (output) DOUBLE PRECISION array, dimension (N)
* The diagonal elements of the tridiagonal matrix T.
*
* E (output) DOUBLE PRECISION array, dimension (N-1)
* The off-diagonal elements of the tridiagonal matrix T:
* E(i) = T(i,i+1) if UPLO = 'U'; E(i) = T(i+1,i) if UPLO = 'L'.
*
* Q (input/output) DOUBLE PRECISION array, dimension (LDQ,N)
* On entry, if VECT = 'U', then Q must contain an N-by-N
* matrix X; if VECT = 'N' or 'V', then Q need not be set.
*
* On exit:
* if VECT = 'V', Q contains the N-by-N orthogonal matrix Q;
* if VECT = 'U', Q contains the product X*Q;
* if VECT = 'N', the array Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q.
* LDQ >= 1, and LDQ >= N if VECT = 'V' or 'U'.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* Modified by Linda Kaufman, Bell Labs.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param vect
* @param uplo
* @param n
* @param kd
* @param ab
* @param ldab
* @param d
* @param e
* @param q
* @param ldq
* @param work
* @param info
*
*/
abstract public void dsbtrd(java.lang.String vect, java.lang.String uplo, int n, int kd, double[] ab, int ldab, double[] d, double[] e, double[] q, int ldq, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSBTRD reduces a real symmetric band matrix A to symmetric
* tridiagonal form T by an orthogonal similarity transformation:
* Q**T * A * Q = T.
*
* Arguments
* =========
*
* VECT (input) CHARACTER*1
* = 'N': do not form Q;
* = 'V': form Q;
* = 'U': update a matrix X, by forming X*Q.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* AB (input/output) DOUBLE PRECISION array, dimension (LDAB,N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
* On exit, the diagonal elements of AB are overwritten by the
* diagonal elements of the tridiagonal matrix T; if KD > 0, the
* elements on the first superdiagonal (if UPLO = 'U') or the
* first subdiagonal (if UPLO = 'L') are overwritten by the
* off-diagonal elements of T; the rest of AB is overwritten by
* values generated during the reduction.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* D (output) DOUBLE PRECISION array, dimension (N)
* The diagonal elements of the tridiagonal matrix T.
*
* E (output) DOUBLE PRECISION array, dimension (N-1)
* The off-diagonal elements of the tridiagonal matrix T:
* E(i) = T(i,i+1) if UPLO = 'U'; E(i) = T(i+1,i) if UPLO = 'L'.
*
* Q (input/output) DOUBLE PRECISION array, dimension (LDQ,N)
* On entry, if VECT = 'U', then Q must contain an N-by-N
* matrix X; if VECT = 'N' or 'V', then Q need not be set.
*
* On exit:
* if VECT = 'V', Q contains the N-by-N orthogonal matrix Q;
* if VECT = 'U', Q contains the product X*Q;
* if VECT = 'N', the array Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q.
* LDQ >= 1, and LDQ >= N if VECT = 'V' or 'U'.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* Modified by Linda Kaufman, Bell Labs.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param vect
* @param uplo
* @param n
* @param kd
* @param ab
* @param _ab_offset
* @param ldab
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param q
* @param _q_offset
* @param ldq
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dsbtrd(java.lang.String vect, java.lang.String uplo, int n, int kd, double[] ab, int _ab_offset, int ldab, double[] d, int _d_offset, double[] e, int _e_offset, double[] q, int _q_offset, int ldq, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSGESV computes the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N matrix and X and B are N-by-NRHS matrices.
*
* DSGESV first attempts to factorize the matrix in SINGLE PRECISION
* and use this factorization within an iterative refinement procedure t
* produce a solution with DOUBLE PRECISION normwise backward error
* quality (see below). If the approach fails the method switches to a
* DOUBLE PRECISION factorization and solve.
*
* The iterative refinement is not going to be a winning strategy if
* the ratio SINGLE PRECISION performance over DOUBLE PRECISION performa
* is too small. A reasonable strategy should take the number of right-h
* sides and the size of the matrix into account. This might be done wit
* call to ILAENV in the future. Up to now, we always try iterative refi
*
* The iterative refinement process is stopped if
* ITER > ITERMAX
* or for all the RHS we have:
* RNRM < SQRT(N)*XNRM*ANRM*EPS*BWDMAX
* where
* o ITER is the number of the current iteration in the iterative
* refinement process
* o RNRM is the infinity-norm of the residual
* o XNRM is the infinity-norm of the solution
* o ANRM is the infinity-operator-norm of the matrix A
* o EPS is the machine epsilon returned by DLAMCH('Epsilon')
* The value ITERMAX and BWDMAX are fixed to 30 and 1.0D+00 respectively
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* A (input or input/ouptut) DOUBLE PRECISION array,
* dimension (LDA,N)
* On entry, the N-by-N coefficient matrix A.
* On exit, if iterative refinement has been successfully used
* (INFO.EQ.0 and ITER.GE.0, see description below), then A is
* unchanged, if double precision factorization has been used
* (INFO.EQ.0 and ITER.LT.0, see description below), then the
* array A contains the factors L and U from the factorization
* A = P*L*U; the unit diagonal elements of L are not stored.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (output) INTEGER array, dimension (N)
* The pivot indices that define the permutation matrix P;
* row i of the matrix was interchanged with row IPIV(i).
* Corresponds either to the single precision factorization
* (if INFO.EQ.0 and ITER.GE.0) or the double precision
* factorization (if INFO.EQ.0 and ITER.LT.0).
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The N-by-NRHS matrix of right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* If INFO = 0, the N-by-NRHS solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (N*NRHS)
* This array is used to hold the residual vectors.
*
* SWORK (workspace) REAL array, dimension (N*(N+NRHS))
* This array is used to use the single precision matrix and the
* right-hand sides or solutions in single precision.
*
* ITER (output) INTEGER
* < 0: iterative refinement has failed, double precision
* factorization has been performed
* -1 : taking into account machine parameters, N, NRHS, it
* is a priori not worth working in SINGLE PRECISION
* -2 : overflow of an entry when moving from double to
* SINGLE PRECISION
* -3 : failure of SGETRF
* -31: stop the iterative refinement after the 30th
* iterations
* > 0: iterative refinement has been sucessfully used.
* Returns the number of iterations
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, U(i,i) computed in DOUBLE PRECISION is
* exactly zero. The factorization has been completed,
* but the factor U is exactly singular, so the solution
* could not be computed.
*
* =========
*
* .. Parameters ..
*
*
* @param n
* @param nrhs
* @param a
* @param lda
* @param ipiv
* @param b
* @param ldb
* @param x
* @param ldx
* @param work
* @param swork
* @param iter
* @param info
*
*/
abstract public void dsgesv(int n, int nrhs, double[] a, int lda, int[] ipiv, double[] b, int ldb, double[] x, int ldx, double[] work, float[] swork, org.netlib.util.intW iter, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSGESV computes the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N matrix and X and B are N-by-NRHS matrices.
*
* DSGESV first attempts to factorize the matrix in SINGLE PRECISION
* and use this factorization within an iterative refinement procedure t
* produce a solution with DOUBLE PRECISION normwise backward error
* quality (see below). If the approach fails the method switches to a
* DOUBLE PRECISION factorization and solve.
*
* The iterative refinement is not going to be a winning strategy if
* the ratio SINGLE PRECISION performance over DOUBLE PRECISION performa
* is too small. A reasonable strategy should take the number of right-h
* sides and the size of the matrix into account. This might be done wit
* call to ILAENV in the future. Up to now, we always try iterative refi
*
* The iterative refinement process is stopped if
* ITER > ITERMAX
* or for all the RHS we have:
* RNRM < SQRT(N)*XNRM*ANRM*EPS*BWDMAX
* where
* o ITER is the number of the current iteration in the iterative
* refinement process
* o RNRM is the infinity-norm of the residual
* o XNRM is the infinity-norm of the solution
* o ANRM is the infinity-operator-norm of the matrix A
* o EPS is the machine epsilon returned by DLAMCH('Epsilon')
* The value ITERMAX and BWDMAX are fixed to 30 and 1.0D+00 respectively
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* A (input or input/ouptut) DOUBLE PRECISION array,
* dimension (LDA,N)
* On entry, the N-by-N coefficient matrix A.
* On exit, if iterative refinement has been successfully used
* (INFO.EQ.0 and ITER.GE.0, see description below), then A is
* unchanged, if double precision factorization has been used
* (INFO.EQ.0 and ITER.LT.0, see description below), then the
* array A contains the factors L and U from the factorization
* A = P*L*U; the unit diagonal elements of L are not stored.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (output) INTEGER array, dimension (N)
* The pivot indices that define the permutation matrix P;
* row i of the matrix was interchanged with row IPIV(i).
* Corresponds either to the single precision factorization
* (if INFO.EQ.0 and ITER.GE.0) or the double precision
* factorization (if INFO.EQ.0 and ITER.LT.0).
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The N-by-NRHS matrix of right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* If INFO = 0, the N-by-NRHS solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (N*NRHS)
* This array is used to hold the residual vectors.
*
* SWORK (workspace) REAL array, dimension (N*(N+NRHS))
* This array is used to use the single precision matrix and the
* right-hand sides or solutions in single precision.
*
* ITER (output) INTEGER
* < 0: iterative refinement has failed, double precision
* factorization has been performed
* -1 : taking into account machine parameters, N, NRHS, it
* is a priori not worth working in SINGLE PRECISION
* -2 : overflow of an entry when moving from double to
* SINGLE PRECISION
* -3 : failure of SGETRF
* -31: stop the iterative refinement after the 30th
* iterations
* > 0: iterative refinement has been sucessfully used.
* Returns the number of iterations
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, U(i,i) computed in DOUBLE PRECISION is
* exactly zero. The factorization has been completed,
* but the factor U is exactly singular, so the solution
* could not be computed.
*
* =========
*
* .. Parameters ..
*
*
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param work
* @param _work_offset
* @param swork
* @param _swork_offset
* @param iter
* @param info
*
*/
abstract public void dsgesv(int n, int nrhs, double[] a, int _a_offset, int lda, int[] ipiv, int _ipiv_offset, double[] b, int _b_offset, int ldb, double[] x, int _x_offset, int ldx, double[] work, int _work_offset, float[] swork, int _swork_offset, org.netlib.util.intW iter, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSPCON estimates the reciprocal of the condition number (in the
* 1-norm) of a real symmetric packed matrix A using the factorization
* A = U*D*U**T or A = L*D*L**T computed by DSPTRF.
*
* An estimate is obtained for norm(inv(A)), and the reciprocal of the
* condition number is computed as RCOND = 1 / (ANORM * norm(inv(A))).
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the details of the factorization are stored
* as an upper or lower triangular matrix.
* = 'U': Upper triangular, form is A = U*D*U**T;
* = 'L': Lower triangular, form is A = L*D*L**T.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The block diagonal matrix D and the multipliers used to
* obtain the factor U or L as computed by DSPTRF, stored as a
* packed triangular matrix.
*
* IPIV (input) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D
* as determined by DSPTRF.
*
* ANORM (input) DOUBLE PRECISION
* The 1-norm of the original matrix A.
*
* RCOND (output) DOUBLE PRECISION
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(ANORM * AINVNM), where AINVNM is an
* estimate of the 1-norm of inv(A) computed in this routine.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (2*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param ipiv
* @param anorm
* @param rcond
* @param work
* @param iwork
* @param info
*
*/
abstract public void dspcon(java.lang.String uplo, int n, double[] ap, int[] ipiv, double anorm, org.netlib.util.doubleW rcond, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSPCON estimates the reciprocal of the condition number (in the
* 1-norm) of a real symmetric packed matrix A using the factorization
* A = U*D*U**T or A = L*D*L**T computed by DSPTRF.
*
* An estimate is obtained for norm(inv(A)), and the reciprocal of the
* condition number is computed as RCOND = 1 / (ANORM * norm(inv(A))).
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the details of the factorization are stored
* as an upper or lower triangular matrix.
* = 'U': Upper triangular, form is A = U*D*U**T;
* = 'L': Lower triangular, form is A = L*D*L**T.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The block diagonal matrix D and the multipliers used to
* obtain the factor U or L as computed by DSPTRF, stored as a
* packed triangular matrix.
*
* IPIV (input) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D
* as determined by DSPTRF.
*
* ANORM (input) DOUBLE PRECISION
* The 1-norm of the original matrix A.
*
* RCOND (output) DOUBLE PRECISION
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(ANORM * AINVNM), where AINVNM is an
* estimate of the 1-norm of inv(A) computed in this routine.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (2*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param ipiv
* @param _ipiv_offset
* @param anorm
* @param rcond
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dspcon(java.lang.String uplo, int n, double[] ap, int _ap_offset, int[] ipiv, int _ipiv_offset, double anorm, org.netlib.util.doubleW rcond, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSPEV computes all the eigenvalues and, optionally, eigenvectors of a
* real symmetric matrix A in packed storage.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, AP is overwritten by values generated during the
* reduction to tridiagonal form. If UPLO = 'U', the diagonal
* and first superdiagonal of the tridiagonal matrix T overwrite
* the corresponding elements of A, and if UPLO = 'L', the
* diagonal and first subdiagonal of T overwrite the
* corresponding elements of A.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the orthonormal
* eigenvectors of the matrix A, with the i-th column of Z
* holding the eigenvector associated with W(i).
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, the algorithm failed to converge; i
* off-diagonal elements of an intermediate tridiagonal
* form did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param uplo
* @param n
* @param ap
* @param w
* @param z
* @param ldz
* @param work
* @param info
*
*/
abstract public void dspev(java.lang.String jobz, java.lang.String uplo, int n, double[] ap, double[] w, double[] z, int ldz, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSPEV computes all the eigenvalues and, optionally, eigenvectors of a
* real symmetric matrix A in packed storage.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, AP is overwritten by values generated during the
* reduction to tridiagonal form. If UPLO = 'U', the diagonal
* and first superdiagonal of the tridiagonal matrix T overwrite
* the corresponding elements of A, and if UPLO = 'L', the
* diagonal and first subdiagonal of T overwrite the
* corresponding elements of A.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the orthonormal
* eigenvectors of the matrix A, with the i-th column of Z
* holding the eigenvector associated with W(i).
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, the algorithm failed to converge; i
* off-diagonal elements of an intermediate tridiagonal
* form did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dspev(java.lang.String jobz, java.lang.String uplo, int n, double[] ap, int _ap_offset, double[] w, int _w_offset, double[] z, int _z_offset, int ldz, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSPEVD computes all the eigenvalues and, optionally, eigenvectors
* of a real symmetric matrix A in packed storage. If eigenvectors are
* desired, it uses a divide and conquer algorithm.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, AP is overwritten by values generated during the
* reduction to tridiagonal form. If UPLO = 'U', the diagonal
* and first superdiagonal of the tridiagonal matrix T overwrite
* the corresponding elements of A, and if UPLO = 'L', the
* diagonal and first subdiagonal of T overwrite the
* corresponding elements of A.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the orthonormal
* eigenvectors of the matrix A, with the i-th column of Z
* holding the eigenvector associated with W(i).
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace/output) DOUBLE PRECISION array,
* dimension (LWORK)
* On exit, if INFO = 0, WORK(1) returns the required LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If N <= 1, LWORK must be at least 1.
* If JOBZ = 'N' and N > 1, LWORK must be at least 2*N.
* If JOBZ = 'V' and N > 1, LWORK must be at least
* 1 + 6*N + N**2.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the required sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the required LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If JOBZ = 'N' or N <= 1, LIWORK must be at least 1.
* If JOBZ = 'V' and N > 1, LIWORK must be at least 3 + 5*N.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the required sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, the algorithm failed to converge; i
* off-diagonal elements of an intermediate tridiagonal
* form did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param uplo
* @param n
* @param ap
* @param w
* @param z
* @param ldz
* @param work
* @param lwork
* @param iwork
* @param liwork
* @param info
*
*/
abstract public void dspevd(java.lang.String jobz, java.lang.String uplo, int n, double[] ap, double[] w, double[] z, int ldz, double[] work, int lwork, int[] iwork, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSPEVD computes all the eigenvalues and, optionally, eigenvectors
* of a real symmetric matrix A in packed storage. If eigenvectors are
* desired, it uses a divide and conquer algorithm.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, AP is overwritten by values generated during the
* reduction to tridiagonal form. If UPLO = 'U', the diagonal
* and first superdiagonal of the tridiagonal matrix T overwrite
* the corresponding elements of A, and if UPLO = 'L', the
* diagonal and first subdiagonal of T overwrite the
* corresponding elements of A.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the orthonormal
* eigenvectors of the matrix A, with the i-th column of Z
* holding the eigenvector associated with W(i).
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace/output) DOUBLE PRECISION array,
* dimension (LWORK)
* On exit, if INFO = 0, WORK(1) returns the required LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If N <= 1, LWORK must be at least 1.
* If JOBZ = 'N' and N > 1, LWORK must be at least 2*N.
* If JOBZ = 'V' and N > 1, LWORK must be at least
* 1 + 6*N + N**2.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the required sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the required LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If JOBZ = 'N' or N <= 1, LIWORK must be at least 1.
* If JOBZ = 'V' and N > 1, LIWORK must be at least 3 + 5*N.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the required sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, the algorithm failed to converge; i
* off-diagonal elements of an intermediate tridiagonal
* form did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param liwork
* @param info
*
*/
abstract public void dspevd(java.lang.String jobz, java.lang.String uplo, int n, double[] ap, int _ap_offset, double[] w, int _w_offset, double[] z, int _z_offset, int ldz, double[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSPEVX computes selected eigenvalues and, optionally, eigenvectors
* of a real symmetric matrix A in packed storage. Eigenvalues/vectors
* can be selected by specifying either a range of values or a range of
* indices for the desired eigenvalues.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found;
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found;
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, AP is overwritten by values generated during the
* reduction to tridiagonal form. If UPLO = 'U', the diagonal
* and first superdiagonal of the tridiagonal matrix T overwrite
* the corresponding elements of A, and if UPLO = 'L', the
* diagonal and first subdiagonal of T overwrite the
* corresponding elements of A.
*
* VL (input) DOUBLE PRECISION
* VU (input) DOUBLE PRECISION
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) DOUBLE PRECISION
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less than
* or equal to zero, then EPS*|T| will be used in its place,
* where |T| is the 1-norm of the tridiagonal matrix obtained
* by reducing AP to tridiagonal form.
*
* Eigenvalues will be computed most accurately when ABSTOL is
* set to twice the underflow threshold 2*DLAMCH('S'), not zero.
* If this routine returns with INFO>0, indicating that some
* eigenvectors did not converge, try setting ABSTOL to
* 2*DLAMCH('S').
*
* See "Computing Small Singular Values of Bidiagonal Matrices
* with Guaranteed High Relative Accuracy," by Demmel and
* Kahan, LAPACK Working Note #3.
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, the selected eigenvalues in ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, max(1,M))
* If JOBZ = 'V', then if INFO = 0, the first M columns of Z
* contain the orthonormal eigenvectors of the matrix A
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* If an eigenvector fails to converge, then that column of Z
* contains the latest approximation to the eigenvector, and the
* index of the eigenvector is returned in IFAIL.
* If JOBZ = 'N', then Z is not referenced.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (8*N)
*
* IWORK (workspace) INTEGER array, dimension (5*N)
*
* IFAIL (output) INTEGER array, dimension (N)
* If JOBZ = 'V', then if INFO = 0, the first M elements of
* IFAIL are zero. If INFO > 0, then IFAIL contains the
* indices of the eigenvectors that failed to converge.
* If JOBZ = 'N', then IFAIL is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, then i eigenvectors failed to converge.
* Their indices are stored in array IFAIL.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param range
* @param uplo
* @param n
* @param ap
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param z
* @param ldz
* @param work
* @param iwork
* @param ifail
* @param info
*
*/
abstract public void dspevx(java.lang.String jobz, java.lang.String range, java.lang.String uplo, int n, double[] ap, double vl, double vu, int il, int iu, double abstol, org.netlib.util.intW m, double[] w, double[] z, int ldz, double[] work, int[] iwork, int[] ifail, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSPEVX computes selected eigenvalues and, optionally, eigenvectors
* of a real symmetric matrix A in packed storage. Eigenvalues/vectors
* can be selected by specifying either a range of values or a range of
* indices for the desired eigenvalues.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found;
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found;
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, AP is overwritten by values generated during the
* reduction to tridiagonal form. If UPLO = 'U', the diagonal
* and first superdiagonal of the tridiagonal matrix T overwrite
* the corresponding elements of A, and if UPLO = 'L', the
* diagonal and first subdiagonal of T overwrite the
* corresponding elements of A.
*
* VL (input) DOUBLE PRECISION
* VU (input) DOUBLE PRECISION
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) DOUBLE PRECISION
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less than
* or equal to zero, then EPS*|T| will be used in its place,
* where |T| is the 1-norm of the tridiagonal matrix obtained
* by reducing AP to tridiagonal form.
*
* Eigenvalues will be computed most accurately when ABSTOL is
* set to twice the underflow threshold 2*DLAMCH('S'), not zero.
* If this routine returns with INFO>0, indicating that some
* eigenvectors did not converge, try setting ABSTOL to
* 2*DLAMCH('S').
*
* See "Computing Small Singular Values of Bidiagonal Matrices
* with Guaranteed High Relative Accuracy," by Demmel and
* Kahan, LAPACK Working Note #3.
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, the selected eigenvalues in ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, max(1,M))
* If JOBZ = 'V', then if INFO = 0, the first M columns of Z
* contain the orthonormal eigenvectors of the matrix A
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* If an eigenvector fails to converge, then that column of Z
* contains the latest approximation to the eigenvector, and the
* index of the eigenvector is returned in IFAIL.
* If JOBZ = 'N', then Z is not referenced.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (8*N)
*
* IWORK (workspace) INTEGER array, dimension (5*N)
*
* IFAIL (output) INTEGER array, dimension (N)
* If JOBZ = 'V', then if INFO = 0, the first M elements of
* IFAIL are zero. If INFO > 0, then IFAIL contains the
* indices of the eigenvectors that failed to converge.
* If JOBZ = 'N', then IFAIL is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, then i eigenvectors failed to converge.
* Their indices are stored in array IFAIL.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param range
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param ifail
* @param _ifail_offset
* @param info
*
*/
abstract public void dspevx(java.lang.String jobz, java.lang.String range, java.lang.String uplo, int n, double[] ap, int _ap_offset, double vl, double vu, int il, int iu, double abstol, org.netlib.util.intW m, double[] w, int _w_offset, double[] z, int _z_offset, int ldz, double[] work, int _work_offset, int[] iwork, int _iwork_offset, int[] ifail, int _ifail_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSPGST reduces a real symmetric-definite generalized eigenproblem
* to standard form, using packed storage.
*
* If ITYPE = 1, the problem is A*x = lambda*B*x,
* and A is overwritten by inv(U**T)*A*inv(U) or inv(L)*A*inv(L**T)
*
* If ITYPE = 2 or 3, the problem is A*B*x = lambda*x or
* B*A*x = lambda*x, and A is overwritten by U*A*U**T or L**T*A*L.
*
* B must have been previously factorized as U**T*U or L*L**T by DPPTRF.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* = 1: compute inv(U**T)*A*inv(U) or inv(L)*A*inv(L**T);
* = 2 or 3: compute U*A*U**T or L**T*A*L.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored and B is factored as
* U**T*U;
* = 'L': Lower triangle of A is stored and B is factored as
* L*L**T.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, if INFO = 0, the transformed matrix, stored in the
* same format as A.
*
* BP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The triangular factor from the Cholesky factorization of B,
* stored in the same format as A, as returned by DPPTRF.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param itype
* @param uplo
* @param n
* @param ap
* @param bp
* @param info
*
*/
abstract public void dspgst(int itype, java.lang.String uplo, int n, double[] ap, double[] bp, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSPGST reduces a real symmetric-definite generalized eigenproblem
* to standard form, using packed storage.
*
* If ITYPE = 1, the problem is A*x = lambda*B*x,
* and A is overwritten by inv(U**T)*A*inv(U) or inv(L)*A*inv(L**T)
*
* If ITYPE = 2 or 3, the problem is A*B*x = lambda*x or
* B*A*x = lambda*x, and A is overwritten by U*A*U**T or L**T*A*L.
*
* B must have been previously factorized as U**T*U or L*L**T by DPPTRF.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* = 1: compute inv(U**T)*A*inv(U) or inv(L)*A*inv(L**T);
* = 2 or 3: compute U*A*U**T or L**T*A*L.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored and B is factored as
* U**T*U;
* = 'L': Lower triangle of A is stored and B is factored as
* L*L**T.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, if INFO = 0, the transformed matrix, stored in the
* same format as A.
*
* BP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The triangular factor from the Cholesky factorization of B,
* stored in the same format as A, as returned by DPPTRF.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param itype
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param bp
* @param _bp_offset
* @param info
*
*/
abstract public void dspgst(int itype, java.lang.String uplo, int n, double[] ap, int _ap_offset, double[] bp, int _bp_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSPGV computes all the eigenvalues and, optionally, the eigenvectors
* of a real generalized symmetric-definite eigenproblem, of the form
* A*x=(lambda)*B*x, A*Bx=(lambda)*x, or B*A*x=(lambda)*x.
* Here A and B are assumed to be symmetric, stored in packed format,
* and B is also positive definite.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* Specifies the problem type to be solved:
* = 1: A*x = (lambda)*B*x
* = 2: A*B*x = (lambda)*x
* = 3: B*A*x = (lambda)*x
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangles of A and B are stored;
* = 'L': Lower triangles of A and B are stored.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension
* (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, the contents of AP are destroyed.
*
* BP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* B, packed columnwise in a linear array. The j-th column of B
* is stored in the array BP as follows:
* if UPLO = 'U', BP(i + (j-1)*j/2) = B(i,j) for 1<=i<=j;
* if UPLO = 'L', BP(i + (j-1)*(2*n-j)/2) = B(i,j) for j<=i<=n.
*
* On exit, the triangular factor U or L from the Cholesky
* factorization B = U**T*U or B = L*L**T, in the same storage
* format as B.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the matrix Z of
* eigenvectors. The eigenvectors are normalized as follows:
* if ITYPE = 1 or 2, Z**T*B*Z = I;
* if ITYPE = 3, Z**T*inv(B)*Z = I.
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: DPPTRF or DSPEV returned an error code:
* <= N: if INFO = i, DSPEV failed to converge;
* i off-diagonal elements of an intermediate
* tridiagonal form did not converge to zero.
* > N: if INFO = n + i, for 1 <= i <= n, then the leading
* minor of order i of B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param itype
* @param jobz
* @param uplo
* @param n
* @param ap
* @param bp
* @param w
* @param z
* @param ldz
* @param work
* @param info
*
*/
abstract public void dspgv(int itype, java.lang.String jobz, java.lang.String uplo, int n, double[] ap, double[] bp, double[] w, double[] z, int ldz, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSPGV computes all the eigenvalues and, optionally, the eigenvectors
* of a real generalized symmetric-definite eigenproblem, of the form
* A*x=(lambda)*B*x, A*Bx=(lambda)*x, or B*A*x=(lambda)*x.
* Here A and B are assumed to be symmetric, stored in packed format,
* and B is also positive definite.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* Specifies the problem type to be solved:
* = 1: A*x = (lambda)*B*x
* = 2: A*B*x = (lambda)*x
* = 3: B*A*x = (lambda)*x
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangles of A and B are stored;
* = 'L': Lower triangles of A and B are stored.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension
* (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, the contents of AP are destroyed.
*
* BP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* B, packed columnwise in a linear array. The j-th column of B
* is stored in the array BP as follows:
* if UPLO = 'U', BP(i + (j-1)*j/2) = B(i,j) for 1<=i<=j;
* if UPLO = 'L', BP(i + (j-1)*(2*n-j)/2) = B(i,j) for j<=i<=n.
*
* On exit, the triangular factor U or L from the Cholesky
* factorization B = U**T*U or B = L*L**T, in the same storage
* format as B.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the matrix Z of
* eigenvectors. The eigenvectors are normalized as follows:
* if ITYPE = 1 or 2, Z**T*B*Z = I;
* if ITYPE = 3, Z**T*inv(B)*Z = I.
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: DPPTRF or DSPEV returned an error code:
* <= N: if INFO = i, DSPEV failed to converge;
* i off-diagonal elements of an intermediate
* tridiagonal form did not converge to zero.
* > N: if INFO = n + i, for 1 <= i <= n, then the leading
* minor of order i of B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param itype
* @param jobz
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param bp
* @param _bp_offset
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dspgv(int itype, java.lang.String jobz, java.lang.String uplo, int n, double[] ap, int _ap_offset, double[] bp, int _bp_offset, double[] w, int _w_offset, double[] z, int _z_offset, int ldz, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSPGVD computes all the eigenvalues, and optionally, the eigenvectors
* of a real generalized symmetric-definite eigenproblem, of the form
* A*x=(lambda)*B*x, A*Bx=(lambda)*x, or B*A*x=(lambda)*x. Here A and
* B are assumed to be symmetric, stored in packed format, and B is also
* positive definite.
* If eigenvectors are desired, it uses a divide and conquer algorithm.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* Specifies the problem type to be solved:
* = 1: A*x = (lambda)*B*x
* = 2: A*B*x = (lambda)*x
* = 3: B*A*x = (lambda)*x
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangles of A and B are stored;
* = 'L': Lower triangles of A and B are stored.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, the contents of AP are destroyed.
*
* BP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* B, packed columnwise in a linear array. The j-th column of B
* is stored in the array BP as follows:
* if UPLO = 'U', BP(i + (j-1)*j/2) = B(i,j) for 1<=i<=j;
* if UPLO = 'L', BP(i + (j-1)*(2*n-j)/2) = B(i,j) for j<=i<=n.
*
* On exit, the triangular factor U or L from the Cholesky
* factorization B = U**T*U or B = L*L**T, in the same storage
* format as B.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the matrix Z of
* eigenvectors. The eigenvectors are normalized as follows:
* if ITYPE = 1 or 2, Z**T*B*Z = I;
* if ITYPE = 3, Z**T*inv(B)*Z = I.
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the required LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If N <= 1, LWORK >= 1.
* If JOBZ = 'N' and N > 1, LWORK >= 2*N.
* If JOBZ = 'V' and N > 1, LWORK >= 1 + 6*N + 2*N**2.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the required sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the required LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If JOBZ = 'N' or N <= 1, LIWORK >= 1.
* If JOBZ = 'V' and N > 1, LIWORK >= 3 + 5*N.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the required sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: DPPTRF or DSPEVD returned an error code:
* <= N: if INFO = i, DSPEVD failed to converge;
* i off-diagonal elements of an intermediate
* tridiagonal form did not converge to zero;
* > N: if INFO = N + i, for 1 <= i <= N, then the leading
* minor of order i of B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* Further Details
* ===============
*
* Based on contributions by
* Mark Fahey, Department of Mathematics, Univ. of Kentucky, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param itype
* @param jobz
* @param uplo
* @param n
* @param ap
* @param bp
* @param w
* @param z
* @param ldz
* @param work
* @param lwork
* @param iwork
* @param liwork
* @param info
*
*/
abstract public void dspgvd(int itype, java.lang.String jobz, java.lang.String uplo, int n, double[] ap, double[] bp, double[] w, double[] z, int ldz, double[] work, int lwork, int[] iwork, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSPGVD computes all the eigenvalues, and optionally, the eigenvectors
* of a real generalized symmetric-definite eigenproblem, of the form
* A*x=(lambda)*B*x, A*Bx=(lambda)*x, or B*A*x=(lambda)*x. Here A and
* B are assumed to be symmetric, stored in packed format, and B is also
* positive definite.
* If eigenvectors are desired, it uses a divide and conquer algorithm.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* Specifies the problem type to be solved:
* = 1: A*x = (lambda)*B*x
* = 2: A*B*x = (lambda)*x
* = 3: B*A*x = (lambda)*x
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangles of A and B are stored;
* = 'L': Lower triangles of A and B are stored.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, the contents of AP are destroyed.
*
* BP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* B, packed columnwise in a linear array. The j-th column of B
* is stored in the array BP as follows:
* if UPLO = 'U', BP(i + (j-1)*j/2) = B(i,j) for 1<=i<=j;
* if UPLO = 'L', BP(i + (j-1)*(2*n-j)/2) = B(i,j) for j<=i<=n.
*
* On exit, the triangular factor U or L from the Cholesky
* factorization B = U**T*U or B = L*L**T, in the same storage
* format as B.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the matrix Z of
* eigenvectors. The eigenvectors are normalized as follows:
* if ITYPE = 1 or 2, Z**T*B*Z = I;
* if ITYPE = 3, Z**T*inv(B)*Z = I.
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the required LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If N <= 1, LWORK >= 1.
* If JOBZ = 'N' and N > 1, LWORK >= 2*N.
* If JOBZ = 'V' and N > 1, LWORK >= 1 + 6*N + 2*N**2.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the required sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the required LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If JOBZ = 'N' or N <= 1, LIWORK >= 1.
* If JOBZ = 'V' and N > 1, LIWORK >= 3 + 5*N.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the required sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: DPPTRF or DSPEVD returned an error code:
* <= N: if INFO = i, DSPEVD failed to converge;
* i off-diagonal elements of an intermediate
* tridiagonal form did not converge to zero;
* > N: if INFO = N + i, for 1 <= i <= N, then the leading
* minor of order i of B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* Further Details
* ===============
*
* Based on contributions by
* Mark Fahey, Department of Mathematics, Univ. of Kentucky, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param itype
* @param jobz
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param bp
* @param _bp_offset
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param liwork
* @param info
*
*/
abstract public void dspgvd(int itype, java.lang.String jobz, java.lang.String uplo, int n, double[] ap, int _ap_offset, double[] bp, int _bp_offset, double[] w, int _w_offset, double[] z, int _z_offset, int ldz, double[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSPGVX computes selected eigenvalues, and optionally, eigenvectors
* of a real generalized symmetric-definite eigenproblem, of the form
* A*x=(lambda)*B*x, A*Bx=(lambda)*x, or B*A*x=(lambda)*x. Here A
* and B are assumed to be symmetric, stored in packed storage, and B
* is also positive definite. Eigenvalues and eigenvectors can be
* selected by specifying either a range of values or a range of indices
* for the desired eigenvalues.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* Specifies the problem type to be solved:
* = 1: A*x = (lambda)*B*x
* = 2: A*B*x = (lambda)*x
* = 3: B*A*x = (lambda)*x
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A and B are stored;
* = 'L': Lower triangle of A and B are stored.
*
* N (input) INTEGER
* The order of the matrix pencil (A,B). N >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, the contents of AP are destroyed.
*
* BP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* B, packed columnwise in a linear array. The j-th column of B
* is stored in the array BP as follows:
* if UPLO = 'U', BP(i + (j-1)*j/2) = B(i,j) for 1<=i<=j;
* if UPLO = 'L', BP(i + (j-1)*(2*n-j)/2) = B(i,j) for j<=i<=n.
*
* On exit, the triangular factor U or L from the Cholesky
* factorization B = U**T*U or B = L*L**T, in the same storage
* format as B.
*
* VL (input) DOUBLE PRECISION
* VU (input) DOUBLE PRECISION
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) DOUBLE PRECISION
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less than
* or equal to zero, then EPS*|T| will be used in its place,
* where |T| is the 1-norm of the tridiagonal matrix obtained
* by reducing A to tridiagonal form.
*
* Eigenvalues will be computed most accurately when ABSTOL is
* set to twice the underflow threshold 2*DLAMCH('S'), not zero.
* If this routine returns with INFO>0, indicating that some
* eigenvectors did not converge, try setting ABSTOL to
* 2*DLAMCH('S').
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* On normal exit, the first M elements contain the selected
* eigenvalues in ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, max(1,M))
* If JOBZ = 'N', then Z is not referenced.
* If JOBZ = 'V', then if INFO = 0, the first M columns of Z
* contain the orthonormal eigenvectors of the matrix A
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* The eigenvectors are normalized as follows:
* if ITYPE = 1 or 2, Z**T*B*Z = I;
* if ITYPE = 3, Z**T*inv(B)*Z = I.
*
* If an eigenvector fails to converge, then that column of Z
* contains the latest approximation to the eigenvector, and the
* index of the eigenvector is returned in IFAIL.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (8*N)
*
* IWORK (workspace) INTEGER array, dimension (5*N)
*
* IFAIL (output) INTEGER array, dimension (N)
* If JOBZ = 'V', then if INFO = 0, the first M elements of
* IFAIL are zero. If INFO > 0, then IFAIL contains the
* indices of the eigenvectors that failed to converge.
* If JOBZ = 'N', then IFAIL is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: DPPTRF or DSPEVX returned an error code:
* <= N: if INFO = i, DSPEVX failed to converge;
* i eigenvectors failed to converge. Their indices
* are stored in array IFAIL.
* > N: if INFO = N + i, for 1 <= i <= N, then the leading
* minor of order i of B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* Further Details
* ===============
*
* Based on contributions by
* Mark Fahey, Department of Mathematics, Univ. of Kentucky, USA
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param itype
* @param jobz
* @param range
* @param uplo
* @param n
* @param ap
* @param bp
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param z
* @param ldz
* @param work
* @param iwork
* @param ifail
* @param info
*
*/
abstract public void dspgvx(int itype, java.lang.String jobz, java.lang.String range, java.lang.String uplo, int n, double[] ap, double[] bp, double vl, double vu, int il, int iu, double abstol, org.netlib.util.intW m, double[] w, double[] z, int ldz, double[] work, int[] iwork, int[] ifail, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSPGVX computes selected eigenvalues, and optionally, eigenvectors
* of a real generalized symmetric-definite eigenproblem, of the form
* A*x=(lambda)*B*x, A*Bx=(lambda)*x, or B*A*x=(lambda)*x. Here A
* and B are assumed to be symmetric, stored in packed storage, and B
* is also positive definite. Eigenvalues and eigenvectors can be
* selected by specifying either a range of values or a range of indices
* for the desired eigenvalues.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* Specifies the problem type to be solved:
* = 1: A*x = (lambda)*B*x
* = 2: A*B*x = (lambda)*x
* = 3: B*A*x = (lambda)*x
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A and B are stored;
* = 'L': Lower triangle of A and B are stored.
*
* N (input) INTEGER
* The order of the matrix pencil (A,B). N >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, the contents of AP are destroyed.
*
* BP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* B, packed columnwise in a linear array. The j-th column of B
* is stored in the array BP as follows:
* if UPLO = 'U', BP(i + (j-1)*j/2) = B(i,j) for 1<=i<=j;
* if UPLO = 'L', BP(i + (j-1)*(2*n-j)/2) = B(i,j) for j<=i<=n.
*
* On exit, the triangular factor U or L from the Cholesky
* factorization B = U**T*U or B = L*L**T, in the same storage
* format as B.
*
* VL (input) DOUBLE PRECISION
* VU (input) DOUBLE PRECISION
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) DOUBLE PRECISION
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less than
* or equal to zero, then EPS*|T| will be used in its place,
* where |T| is the 1-norm of the tridiagonal matrix obtained
* by reducing A to tridiagonal form.
*
* Eigenvalues will be computed most accurately when ABSTOL is
* set to twice the underflow threshold 2*DLAMCH('S'), not zero.
* If this routine returns with INFO>0, indicating that some
* eigenvectors did not converge, try setting ABSTOL to
* 2*DLAMCH('S').
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* On normal exit, the first M elements contain the selected
* eigenvalues in ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, max(1,M))
* If JOBZ = 'N', then Z is not referenced.
* If JOBZ = 'V', then if INFO = 0, the first M columns of Z
* contain the orthonormal eigenvectors of the matrix A
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* The eigenvectors are normalized as follows:
* if ITYPE = 1 or 2, Z**T*B*Z = I;
* if ITYPE = 3, Z**T*inv(B)*Z = I.
*
* If an eigenvector fails to converge, then that column of Z
* contains the latest approximation to the eigenvector, and the
* index of the eigenvector is returned in IFAIL.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (8*N)
*
* IWORK (workspace) INTEGER array, dimension (5*N)
*
* IFAIL (output) INTEGER array, dimension (N)
* If JOBZ = 'V', then if INFO = 0, the first M elements of
* IFAIL are zero. If INFO > 0, then IFAIL contains the
* indices of the eigenvectors that failed to converge.
* If JOBZ = 'N', then IFAIL is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: DPPTRF or DSPEVX returned an error code:
* <= N: if INFO = i, DSPEVX failed to converge;
* i eigenvectors failed to converge. Their indices
* are stored in array IFAIL.
* > N: if INFO = N + i, for 1 <= i <= N, then the leading
* minor of order i of B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* Further Details
* ===============
*
* Based on contributions by
* Mark Fahey, Department of Mathematics, Univ. of Kentucky, USA
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param itype
* @param jobz
* @param range
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param bp
* @param _bp_offset
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param ifail
* @param _ifail_offset
* @param info
*
*/
abstract public void dspgvx(int itype, java.lang.String jobz, java.lang.String range, java.lang.String uplo, int n, double[] ap, int _ap_offset, double[] bp, int _bp_offset, double vl, double vu, int il, int iu, double abstol, org.netlib.util.intW m, double[] w, int _w_offset, double[] z, int _z_offset, int ldz, double[] work, int _work_offset, int[] iwork, int _iwork_offset, int[] ifail, int _ifail_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSPRFS improves the computed solution to a system of linear
* equations when the coefficient matrix is symmetric indefinite
* and packed, and provides error bounds and backward error estimates
* for the solution.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The upper or lower triangle of the symmetric matrix A, packed
* columnwise in a linear array. The j-th column of A is stored
* in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
*
* AFP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The factored form of the matrix A. AFP contains the block
* diagonal matrix D and the multipliers used to obtain the
* factor U or L from the factorization A = U*D*U**T or
* A = L*D*L**T as computed by DSPTRF, stored as a packed
* triangular matrix.
*
* IPIV (input) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D
* as determined by DSPTRF.
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by DSPTRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param ap
* @param afp
* @param ipiv
* @param b
* @param ldb
* @param x
* @param ldx
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void dsprfs(java.lang.String uplo, int n, int nrhs, double[] ap, double[] afp, int[] ipiv, double[] b, int ldb, double[] x, int ldx, double[] ferr, double[] berr, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSPRFS improves the computed solution to a system of linear
* equations when the coefficient matrix is symmetric indefinite
* and packed, and provides error bounds and backward error estimates
* for the solution.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The upper or lower triangle of the symmetric matrix A, packed
* columnwise in a linear array. The j-th column of A is stored
* in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
*
* AFP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The factored form of the matrix A. AFP contains the block
* diagonal matrix D and the multipliers used to obtain the
* factor U or L from the factorization A = U*D*U**T or
* A = L*D*L**T as computed by DSPTRF, stored as a packed
* triangular matrix.
*
* IPIV (input) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D
* as determined by DSPTRF.
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by DSPTRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param ap
* @param _ap_offset
* @param afp
* @param _afp_offset
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dsprfs(java.lang.String uplo, int n, int nrhs, double[] ap, int _ap_offset, double[] afp, int _afp_offset, int[] ipiv, int _ipiv_offset, double[] b, int _b_offset, int ldb, double[] x, int _x_offset, int ldx, double[] ferr, int _ferr_offset, double[] berr, int _berr_offset, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSPSV computes the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N symmetric matrix stored in packed format and X
* and B are N-by-NRHS matrices.
*
* The diagonal pivoting method is used to factor A as
* A = U * D * U**T, if UPLO = 'U', or
* A = L * D * L**T, if UPLO = 'L',
* where U (or L) is a product of permutation and unit upper (lower)
* triangular matrices, D is symmetric and block diagonal with 1-by-1
* and 2-by-2 diagonal blocks. The factored form of A is then used to
* solve the system of equations A * X = B.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
* See below for further details.
*
* On exit, the block diagonal matrix D and the multipliers used
* to obtain the factor U or L from the factorization
* A = U*D*U**T or A = L*D*L**T as computed by DSPTRF, stored as
* a packed triangular matrix in the same storage format as A.
*
* IPIV (output) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D, as
* determined by DSPTRF. If IPIV(k) > 0, then rows and columns
* k and IPIV(k) were interchanged, and D(k,k) is a 1-by-1
* diagonal block. If UPLO = 'U' and IPIV(k) = IPIV(k-1) < 0,
* then rows and columns k-1 and -IPIV(k) were interchanged and
* D(k-1:k,k-1:k) is a 2-by-2 diagonal block. If UPLO = 'L' and
* IPIV(k) = IPIV(k+1) < 0, then rows and columns k+1 and
* -IPIV(k) were interchanged and D(k:k+1,k:k+1) is a 2-by-2
* diagonal block.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if INFO = 0, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, D(i,i) is exactly zero. The factorization
* has been completed, but the block diagonal matrix D is
* exactly singular, so the solution could not be
* computed.
*
* Further Details
* ===============
*
* The packed storage scheme is illustrated by the following example
* when N = 4, UPLO = 'U':
*
* Two-dimensional storage of the symmetric matrix A:
*
* a11 a12 a13 a14
* a22 a23 a24
* a33 a34 (aij = aji)
* a44
*
* Packed storage of the upper triangle of A:
*
* AP = [ a11, a12, a22, a13, a23, a33, a14, a24, a34, a44 ]
*
* =====================================================================
*
* .. External Functions ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param ap
* @param ipiv
* @param b
* @param ldb
* @param info
*
*/
abstract public void dspsv(java.lang.String uplo, int n, int nrhs, double[] ap, int[] ipiv, double[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSPSV computes the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N symmetric matrix stored in packed format and X
* and B are N-by-NRHS matrices.
*
* The diagonal pivoting method is used to factor A as
* A = U * D * U**T, if UPLO = 'U', or
* A = L * D * L**T, if UPLO = 'L',
* where U (or L) is a product of permutation and unit upper (lower)
* triangular matrices, D is symmetric and block diagonal with 1-by-1
* and 2-by-2 diagonal blocks. The factored form of A is then used to
* solve the system of equations A * X = B.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
* See below for further details.
*
* On exit, the block diagonal matrix D and the multipliers used
* to obtain the factor U or L from the factorization
* A = U*D*U**T or A = L*D*L**T as computed by DSPTRF, stored as
* a packed triangular matrix in the same storage format as A.
*
* IPIV (output) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D, as
* determined by DSPTRF. If IPIV(k) > 0, then rows and columns
* k and IPIV(k) were interchanged, and D(k,k) is a 1-by-1
* diagonal block. If UPLO = 'U' and IPIV(k) = IPIV(k-1) < 0,
* then rows and columns k-1 and -IPIV(k) were interchanged and
* D(k-1:k,k-1:k) is a 2-by-2 diagonal block. If UPLO = 'L' and
* IPIV(k) = IPIV(k+1) < 0, then rows and columns k+1 and
* -IPIV(k) were interchanged and D(k:k+1,k:k+1) is a 2-by-2
* diagonal block.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if INFO = 0, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, D(i,i) is exactly zero. The factorization
* has been completed, but the block diagonal matrix D is
* exactly singular, so the solution could not be
* computed.
*
* Further Details
* ===============
*
* The packed storage scheme is illustrated by the following example
* when N = 4, UPLO = 'U':
*
* Two-dimensional storage of the symmetric matrix A:
*
* a11 a12 a13 a14
* a22 a23 a24
* a33 a34 (aij = aji)
* a44
*
* Packed storage of the upper triangle of A:
*
* AP = [ a11, a12, a22, a13, a23, a33, a14, a24, a34, a44 ]
*
* =====================================================================
*
* .. External Functions ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param ap
* @param _ap_offset
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void dspsv(java.lang.String uplo, int n, int nrhs, double[] ap, int _ap_offset, int[] ipiv, int _ipiv_offset, double[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSPSVX uses the diagonal pivoting factorization A = U*D*U**T or
* A = L*D*L**T to compute the solution to a real system of linear
* equations A * X = B, where A is an N-by-N symmetric matrix stored
* in packed format and X and B are N-by-NRHS matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed:
*
* 1. If FACT = 'N', the diagonal pivoting method is used to factor A as
* A = U * D * U**T, if UPLO = 'U', or
* A = L * D * L**T, if UPLO = 'L',
* where U (or L) is a product of permutation and unit upper (lower)
* triangular matrices and D is symmetric and block diagonal with
* 1-by-1 and 2-by-2 diagonal blocks.
*
* 2. If some D(i,i)=0, so that D is exactly singular, then the routine
* returns with INFO = i. Otherwise, the factored form of A is used
* to estimate the condition number of the matrix A. If the
* reciprocal of the condition number is less than machine precision,
* INFO = N+1 is returned as a warning, but the routine still goes on
* to solve for X and compute error bounds as described below.
*
* 3. The system of equations is solved for X using the factored form
* of A.
*
* 4. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of A has been
* supplied on entry.
* = 'F': On entry, AFP and IPIV contain the factored form of
* A. AP, AFP and IPIV will not be modified.
* = 'N': The matrix A will be copied to AFP and factored.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The upper or lower triangle of the symmetric matrix A, packed
* columnwise in a linear array. The j-th column of A is stored
* in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
* See below for further details.
*
* AFP (input or output) DOUBLE PRECISION array, dimension
* (N*(N+1)/2)
* If FACT = 'F', then AFP is an input argument and on entry
* contains the block diagonal matrix D and the multipliers used
* to obtain the factor U or L from the factorization
* A = U*D*U**T or A = L*D*L**T as computed by DSPTRF, stored as
* a packed triangular matrix in the same storage format as A.
*
* If FACT = 'N', then AFP is an output argument and on exit
* contains the block diagonal matrix D and the multipliers used
* to obtain the factor U or L from the factorization
* A = U*D*U**T or A = L*D*L**T as computed by DSPTRF, stored as
* a packed triangular matrix in the same storage format as A.
*
* IPIV (input or output) INTEGER array, dimension (N)
* If FACT = 'F', then IPIV is an input argument and on entry
* contains details of the interchanges and the block structure
* of D, as determined by DSPTRF.
* If IPIV(k) > 0, then rows and columns k and IPIV(k) were
* interchanged and D(k,k) is a 1-by-1 diagonal block.
* If UPLO = 'U' and IPIV(k) = IPIV(k-1) < 0, then rows and
* columns k-1 and -IPIV(k) were interchanged and D(k-1:k,k-1:k)
* is a 2-by-2 diagonal block. If UPLO = 'L' and IPIV(k) =
* IPIV(k+1) < 0, then rows and columns k+1 and -IPIV(k) were
* interchanged and D(k:k+1,k:k+1) is a 2-by-2 diagonal block.
*
* If FACT = 'N', then IPIV is an output argument and on exit
* contains details of the interchanges and the block structure
* of D, as determined by DSPTRF.
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The N-by-NRHS right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* If INFO = 0 or INFO = N+1, the N-by-NRHS solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) DOUBLE PRECISION
* The estimate of the reciprocal condition number of the matrix
* A. If RCOND is less than the machine precision (in
* particular, if RCOND = 0), the matrix is singular to working
* precision. This condition is indicated by a return code of
* INFO > 0.
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= N: D(i,i) is exactly zero. The factorization
* has been completed but the factor D is exactly
* singular, so the solution and error bounds could
* not be computed. RCOND = 0 is returned.
* = N+1: D is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* Further Details
* ===============
*
* The packed storage scheme is illustrated by the following example
* when N = 4, UPLO = 'U':
*
* Two-dimensional storage of the symmetric matrix A:
*
* a11 a12 a13 a14
* a22 a23 a24
* a33 a34 (aij = aji)
* a44
*
* Packed storage of the upper triangle of A:
*
* AP = [ a11, a12, a22, a13, a23, a33, a14, a24, a34, a44 ]
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param uplo
* @param n
* @param nrhs
* @param ap
* @param afp
* @param ipiv
* @param b
* @param ldb
* @param x
* @param ldx
* @param rcond
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void dspsvx(java.lang.String fact, java.lang.String uplo, int n, int nrhs, double[] ap, double[] afp, int[] ipiv, double[] b, int ldb, double[] x, int ldx, org.netlib.util.doubleW rcond, double[] ferr, double[] berr, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSPSVX uses the diagonal pivoting factorization A = U*D*U**T or
* A = L*D*L**T to compute the solution to a real system of linear
* equations A * X = B, where A is an N-by-N symmetric matrix stored
* in packed format and X and B are N-by-NRHS matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed:
*
* 1. If FACT = 'N', the diagonal pivoting method is used to factor A as
* A = U * D * U**T, if UPLO = 'U', or
* A = L * D * L**T, if UPLO = 'L',
* where U (or L) is a product of permutation and unit upper (lower)
* triangular matrices and D is symmetric and block diagonal with
* 1-by-1 and 2-by-2 diagonal blocks.
*
* 2. If some D(i,i)=0, so that D is exactly singular, then the routine
* returns with INFO = i. Otherwise, the factored form of A is used
* to estimate the condition number of the matrix A. If the
* reciprocal of the condition number is less than machine precision,
* INFO = N+1 is returned as a warning, but the routine still goes on
* to solve for X and compute error bounds as described below.
*
* 3. The system of equations is solved for X using the factored form
* of A.
*
* 4. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of A has been
* supplied on entry.
* = 'F': On entry, AFP and IPIV contain the factored form of
* A. AP, AFP and IPIV will not be modified.
* = 'N': The matrix A will be copied to AFP and factored.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The upper or lower triangle of the symmetric matrix A, packed
* columnwise in a linear array. The j-th column of A is stored
* in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
* See below for further details.
*
* AFP (input or output) DOUBLE PRECISION array, dimension
* (N*(N+1)/2)
* If FACT = 'F', then AFP is an input argument and on entry
* contains the block diagonal matrix D and the multipliers used
* to obtain the factor U or L from the factorization
* A = U*D*U**T or A = L*D*L**T as computed by DSPTRF, stored as
* a packed triangular matrix in the same storage format as A.
*
* If FACT = 'N', then AFP is an output argument and on exit
* contains the block diagonal matrix D and the multipliers used
* to obtain the factor U or L from the factorization
* A = U*D*U**T or A = L*D*L**T as computed by DSPTRF, stored as
* a packed triangular matrix in the same storage format as A.
*
* IPIV (input or output) INTEGER array, dimension (N)
* If FACT = 'F', then IPIV is an input argument and on entry
* contains details of the interchanges and the block structure
* of D, as determined by DSPTRF.
* If IPIV(k) > 0, then rows and columns k and IPIV(k) were
* interchanged and D(k,k) is a 1-by-1 diagonal block.
* If UPLO = 'U' and IPIV(k) = IPIV(k-1) < 0, then rows and
* columns k-1 and -IPIV(k) were interchanged and D(k-1:k,k-1:k)
* is a 2-by-2 diagonal block. If UPLO = 'L' and IPIV(k) =
* IPIV(k+1) < 0, then rows and columns k+1 and -IPIV(k) were
* interchanged and D(k:k+1,k:k+1) is a 2-by-2 diagonal block.
*
* If FACT = 'N', then IPIV is an output argument and on exit
* contains details of the interchanges and the block structure
* of D, as determined by DSPTRF.
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The N-by-NRHS right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* If INFO = 0 or INFO = N+1, the N-by-NRHS solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) DOUBLE PRECISION
* The estimate of the reciprocal condition number of the matrix
* A. If RCOND is less than the machine precision (in
* particular, if RCOND = 0), the matrix is singular to working
* precision. This condition is indicated by a return code of
* INFO > 0.
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= N: D(i,i) is exactly zero. The factorization
* has been completed but the factor D is exactly
* singular, so the solution and error bounds could
* not be computed. RCOND = 0 is returned.
* = N+1: D is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* Further Details
* ===============
*
* The packed storage scheme is illustrated by the following example
* when N = 4, UPLO = 'U':
*
* Two-dimensional storage of the symmetric matrix A:
*
* a11 a12 a13 a14
* a22 a23 a24
* a33 a34 (aij = aji)
* a44
*
* Packed storage of the upper triangle of A:
*
* AP = [ a11, a12, a22, a13, a23, a33, a14, a24, a34, a44 ]
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param uplo
* @param n
* @param nrhs
* @param ap
* @param _ap_offset
* @param afp
* @param _afp_offset
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param rcond
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dspsvx(java.lang.String fact, java.lang.String uplo, int n, int nrhs, double[] ap, int _ap_offset, double[] afp, int _afp_offset, int[] ipiv, int _ipiv_offset, double[] b, int _b_offset, int ldb, double[] x, int _x_offset, int ldx, org.netlib.util.doubleW rcond, double[] ferr, int _ferr_offset, double[] berr, int _berr_offset, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSPTRD reduces a real symmetric matrix A stored in packed form to
* symmetric tridiagonal form T by an orthogonal similarity
* transformation: Q**T * A * Q = T.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
* On exit, if UPLO = 'U', the diagonal and first superdiagonal
* of A are overwritten by the corresponding elements of the
* tridiagonal matrix T, and the elements above the first
* superdiagonal, with the array TAU, represent the orthogonal
* matrix Q as a product of elementary reflectors; if UPLO
* = 'L', the diagonal and first subdiagonal of A are over-
* written by the corresponding elements of the tridiagonal
* matrix T, and the elements below the first subdiagonal, with
* the array TAU, represent the orthogonal matrix Q as a product
* of elementary reflectors. See Further Details.
*
* D (output) DOUBLE PRECISION array, dimension (N)
* The diagonal elements of the tridiagonal matrix T:
* D(i) = A(i,i).
*
* E (output) DOUBLE PRECISION array, dimension (N-1)
* The off-diagonal elements of the tridiagonal matrix T:
* E(i) = A(i,i+1) if UPLO = 'U', E(i) = A(i+1,i) if UPLO = 'L'.
*
* TAU (output) DOUBLE PRECISION array, dimension (N-1)
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* If UPLO = 'U', the matrix Q is represented as a product of elementary
* reflectors
*
* Q = H(n-1) . . . H(2) H(1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(i+1:n) = 0 and v(i) = 1; v(1:i-1) is stored on exit in AP,
* overwriting A(1:i-1,i+1), and tau is stored in TAU(i).
*
* If UPLO = 'L', the matrix Q is represented as a product of elementary
* reflectors
*
* Q = H(1) H(2) . . . H(n-1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i) = 0 and v(i+1) = 1; v(i+2:n) is stored on exit in AP,
* overwriting A(i+2:n,i), and tau is stored in TAU(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param d
* @param e
* @param tau
* @param info
*
*/
abstract public void dsptrd(java.lang.String uplo, int n, double[] ap, double[] d, double[] e, double[] tau, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSPTRD reduces a real symmetric matrix A stored in packed form to
* symmetric tridiagonal form T by an orthogonal similarity
* transformation: Q**T * A * Q = T.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
* On exit, if UPLO = 'U', the diagonal and first superdiagonal
* of A are overwritten by the corresponding elements of the
* tridiagonal matrix T, and the elements above the first
* superdiagonal, with the array TAU, represent the orthogonal
* matrix Q as a product of elementary reflectors; if UPLO
* = 'L', the diagonal and first subdiagonal of A are over-
* written by the corresponding elements of the tridiagonal
* matrix T, and the elements below the first subdiagonal, with
* the array TAU, represent the orthogonal matrix Q as a product
* of elementary reflectors. See Further Details.
*
* D (output) DOUBLE PRECISION array, dimension (N)
* The diagonal elements of the tridiagonal matrix T:
* D(i) = A(i,i).
*
* E (output) DOUBLE PRECISION array, dimension (N-1)
* The off-diagonal elements of the tridiagonal matrix T:
* E(i) = A(i,i+1) if UPLO = 'U', E(i) = A(i+1,i) if UPLO = 'L'.
*
* TAU (output) DOUBLE PRECISION array, dimension (N-1)
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* If UPLO = 'U', the matrix Q is represented as a product of elementary
* reflectors
*
* Q = H(n-1) . . . H(2) H(1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(i+1:n) = 0 and v(i) = 1; v(1:i-1) is stored on exit in AP,
* overwriting A(1:i-1,i+1), and tau is stored in TAU(i).
*
* If UPLO = 'L', the matrix Q is represented as a product of elementary
* reflectors
*
* Q = H(1) H(2) . . . H(n-1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i) = 0 and v(i+1) = 1; v(i+2:n) is stored on exit in AP,
* overwriting A(i+2:n,i), and tau is stored in TAU(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param tau
* @param _tau_offset
* @param info
*
*/
abstract public void dsptrd(java.lang.String uplo, int n, double[] ap, int _ap_offset, double[] d, int _d_offset, double[] e, int _e_offset, double[] tau, int _tau_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSPTRF computes the factorization of a real symmetric matrix A stored
* in packed format using the Bunch-Kaufman diagonal pivoting method:
*
* A = U*D*U**T or A = L*D*L**T
*
* where U (or L) is a product of permutation and unit upper (lower)
* triangular matrices, and D is symmetric and block diagonal with
* 1-by-1 and 2-by-2 diagonal blocks.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, the block diagonal matrix D and the multipliers used
* to obtain the factor U or L, stored as a packed triangular
* matrix overwriting A (see below for further details).
*
* IPIV (output) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D.
* If IPIV(k) > 0, then rows and columns k and IPIV(k) were
* interchanged and D(k,k) is a 1-by-1 diagonal block.
* If UPLO = 'U' and IPIV(k) = IPIV(k-1) < 0, then rows and
* columns k-1 and -IPIV(k) were interchanged and D(k-1:k,k-1:k)
* is a 2-by-2 diagonal block. If UPLO = 'L' and IPIV(k) =
* IPIV(k+1) < 0, then rows and columns k+1 and -IPIV(k) were
* interchanged and D(k:k+1,k:k+1) is a 2-by-2 diagonal block.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, D(i,i) is exactly zero. The factorization
* has been completed, but the block diagonal matrix D is
* exactly singular, and division by zero will occur if it
* is used to solve a system of equations.
*
* Further Details
* ===============
*
* 5-96 - Based on modifications by J. Lewis, Boeing Computer Services
* Company
*
* If UPLO = 'U', then A = U*D*U', where
* U = P(n)*U(n)* ... *P(k)U(k)* ...,
* i.e., U is a product of terms P(k)*U(k), where k decreases from n to
* 1 in steps of 1 or 2, and D is a block diagonal matrix with 1-by-1
* and 2-by-2 diagonal blocks D(k). P(k) is a permutation matrix as
* defined by IPIV(k), and U(k) is a unit upper triangular matrix, such
* that if the diagonal block D(k) is of order s (s = 1 or 2), then
*
* ( I v 0 ) k-s
* U(k) = ( 0 I 0 ) s
* ( 0 0 I ) n-k
* k-s s n-k
*
* If s = 1, D(k) overwrites A(k,k), and v overwrites A(1:k-1,k).
* If s = 2, the upper triangle of D(k) overwrites A(k-1,k-1), A(k-1,k),
* and A(k,k), and v overwrites A(1:k-2,k-1:k).
*
* If UPLO = 'L', then A = L*D*L', where
* L = P(1)*L(1)* ... *P(k)*L(k)* ...,
* i.e., L is a product of terms P(k)*L(k), where k increases from 1 to
* n in steps of 1 or 2, and D is a block diagonal matrix with 1-by-1
* and 2-by-2 diagonal blocks D(k). P(k) is a permutation matrix as
* defined by IPIV(k), and L(k) is a unit lower triangular matrix, such
* that if the diagonal block D(k) is of order s (s = 1 or 2), then
*
* ( I 0 0 ) k-1
* L(k) = ( 0 I 0 ) s
* ( 0 v I ) n-k-s+1
* k-1 s n-k-s+1
*
* If s = 1, D(k) overwrites A(k,k), and v overwrites A(k+1:n,k).
* If s = 2, the lower triangle of D(k) overwrites A(k,k), A(k+1,k),
* and A(k+1,k+1), and v overwrites A(k+2:n,k:k+1).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param ipiv
* @param info
*
*/
abstract public void dsptrf(java.lang.String uplo, int n, double[] ap, int[] ipiv, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSPTRF computes the factorization of a real symmetric matrix A stored
* in packed format using the Bunch-Kaufman diagonal pivoting method:
*
* A = U*D*U**T or A = L*D*L**T
*
* where U (or L) is a product of permutation and unit upper (lower)
* triangular matrices, and D is symmetric and block diagonal with
* 1-by-1 and 2-by-2 diagonal blocks.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, the block diagonal matrix D and the multipliers used
* to obtain the factor U or L, stored as a packed triangular
* matrix overwriting A (see below for further details).
*
* IPIV (output) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D.
* If IPIV(k) > 0, then rows and columns k and IPIV(k) were
* interchanged and D(k,k) is a 1-by-1 diagonal block.
* If UPLO = 'U' and IPIV(k) = IPIV(k-1) < 0, then rows and
* columns k-1 and -IPIV(k) were interchanged and D(k-1:k,k-1:k)
* is a 2-by-2 diagonal block. If UPLO = 'L' and IPIV(k) =
* IPIV(k+1) < 0, then rows and columns k+1 and -IPIV(k) were
* interchanged and D(k:k+1,k:k+1) is a 2-by-2 diagonal block.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, D(i,i) is exactly zero. The factorization
* has been completed, but the block diagonal matrix D is
* exactly singular, and division by zero will occur if it
* is used to solve a system of equations.
*
* Further Details
* ===============
*
* 5-96 - Based on modifications by J. Lewis, Boeing Computer Services
* Company
*
* If UPLO = 'U', then A = U*D*U', where
* U = P(n)*U(n)* ... *P(k)U(k)* ...,
* i.e., U is a product of terms P(k)*U(k), where k decreases from n to
* 1 in steps of 1 or 2, and D is a block diagonal matrix with 1-by-1
* and 2-by-2 diagonal blocks D(k). P(k) is a permutation matrix as
* defined by IPIV(k), and U(k) is a unit upper triangular matrix, such
* that if the diagonal block D(k) is of order s (s = 1 or 2), then
*
* ( I v 0 ) k-s
* U(k) = ( 0 I 0 ) s
* ( 0 0 I ) n-k
* k-s s n-k
*
* If s = 1, D(k) overwrites A(k,k), and v overwrites A(1:k-1,k).
* If s = 2, the upper triangle of D(k) overwrites A(k-1,k-1), A(k-1,k),
* and A(k,k), and v overwrites A(1:k-2,k-1:k).
*
* If UPLO = 'L', then A = L*D*L', where
* L = P(1)*L(1)* ... *P(k)*L(k)* ...,
* i.e., L is a product of terms P(k)*L(k), where k increases from 1 to
* n in steps of 1 or 2, and D is a block diagonal matrix with 1-by-1
* and 2-by-2 diagonal blocks D(k). P(k) is a permutation matrix as
* defined by IPIV(k), and L(k) is a unit lower triangular matrix, such
* that if the diagonal block D(k) is of order s (s = 1 or 2), then
*
* ( I 0 0 ) k-1
* L(k) = ( 0 I 0 ) s
* ( 0 v I ) n-k-s+1
* k-1 s n-k-s+1
*
* If s = 1, D(k) overwrites A(k,k), and v overwrites A(k+1:n,k).
* If s = 2, the lower triangle of D(k) overwrites A(k,k), A(k+1,k),
* and A(k+1,k+1), and v overwrites A(k+2:n,k:k+1).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param ipiv
* @param _ipiv_offset
* @param info
*
*/
abstract public void dsptrf(java.lang.String uplo, int n, double[] ap, int _ap_offset, int[] ipiv, int _ipiv_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSPTRI computes the inverse of a real symmetric indefinite matrix
* A in packed storage using the factorization A = U*D*U**T or
* A = L*D*L**T computed by DSPTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the details of the factorization are stored
* as an upper or lower triangular matrix.
* = 'U': Upper triangular, form is A = U*D*U**T;
* = 'L': Lower triangular, form is A = L*D*L**T.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the block diagonal matrix D and the multipliers
* used to obtain the factor U or L as computed by DSPTRF,
* stored as a packed triangular matrix.
*
* On exit, if INFO = 0, the (symmetric) inverse of the original
* matrix, stored as a packed triangular matrix. The j-th column
* of inv(A) is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = inv(A)(i,j) for 1<=i<=j;
* if UPLO = 'L',
* AP(i + (j-1)*(2n-j)/2) = inv(A)(i,j) for j<=i<=n.
*
* IPIV (input) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D
* as determined by DSPTRF.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, D(i,i) = 0; the matrix is singular and its
* inverse could not be computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param ipiv
* @param work
* @param info
*
*/
abstract public void dsptri(java.lang.String uplo, int n, double[] ap, int[] ipiv, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSPTRI computes the inverse of a real symmetric indefinite matrix
* A in packed storage using the factorization A = U*D*U**T or
* A = L*D*L**T computed by DSPTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the details of the factorization are stored
* as an upper or lower triangular matrix.
* = 'U': Upper triangular, form is A = U*D*U**T;
* = 'L': Lower triangular, form is A = L*D*L**T.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the block diagonal matrix D and the multipliers
* used to obtain the factor U or L as computed by DSPTRF,
* stored as a packed triangular matrix.
*
* On exit, if INFO = 0, the (symmetric) inverse of the original
* matrix, stored as a packed triangular matrix. The j-th column
* of inv(A) is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = inv(A)(i,j) for 1<=i<=j;
* if UPLO = 'L',
* AP(i + (j-1)*(2n-j)/2) = inv(A)(i,j) for j<=i<=n.
*
* IPIV (input) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D
* as determined by DSPTRF.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, D(i,i) = 0; the matrix is singular and its
* inverse could not be computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param ipiv
* @param _ipiv_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dsptri(java.lang.String uplo, int n, double[] ap, int _ap_offset, int[] ipiv, int _ipiv_offset, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSPTRS solves a system of linear equations A*X = B with a real
* symmetric matrix A stored in packed format using the factorization
* A = U*D*U**T or A = L*D*L**T computed by DSPTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the details of the factorization are stored
* as an upper or lower triangular matrix.
* = 'U': Upper triangular, form is A = U*D*U**T;
* = 'L': Lower triangular, form is A = L*D*L**T.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The block diagonal matrix D and the multipliers used to
* obtain the factor U or L as computed by DSPTRF, stored as a
* packed triangular matrix.
*
* IPIV (input) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D
* as determined by DSPTRF.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param ap
* @param ipiv
* @param b
* @param ldb
* @param info
*
*/
abstract public void dsptrs(java.lang.String uplo, int n, int nrhs, double[] ap, int[] ipiv, double[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSPTRS solves a system of linear equations A*X = B with a real
* symmetric matrix A stored in packed format using the factorization
* A = U*D*U**T or A = L*D*L**T computed by DSPTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the details of the factorization are stored
* as an upper or lower triangular matrix.
* = 'U': Upper triangular, form is A = U*D*U**T;
* = 'L': Lower triangular, form is A = L*D*L**T.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The block diagonal matrix D and the multipliers used to
* obtain the factor U or L as computed by DSPTRF, stored as a
* packed triangular matrix.
*
* IPIV (input) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D
* as determined by DSPTRF.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param ap
* @param _ap_offset
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void dsptrs(java.lang.String uplo, int n, int nrhs, double[] ap, int _ap_offset, int[] ipiv, int _ipiv_offset, double[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSTEBZ computes the eigenvalues of a symmetric tridiagonal
* matrix T. The user may ask for all eigenvalues, all eigenvalues
* in the half-open interval (VL, VU], or the IL-th through IU-th
* eigenvalues.
*
* To avoid overflow, the matrix must be scaled so that its
* largest element is no greater than overflow**(1/2) *
* underflow**(1/4) in absolute value, and for greatest
* accuracy, it should not be much smaller than that.
*
* See W. Kahan "Accurate Eigenvalues of a Symmetric Tridiagonal
* Matrix", Report CS41, Computer Science Dept., Stanford
* University, July 21, 1966.
*
* Arguments
* =========
*
* RANGE (input) CHARACTER*1
* = 'A': ("All") all eigenvalues will be found.
* = 'V': ("Value") all eigenvalues in the half-open interval
* (VL, VU] will be found.
* = 'I': ("Index") the IL-th through IU-th eigenvalues (of the
* entire matrix) will be found.
*
* ORDER (input) CHARACTER*1
* = 'B': ("By Block") the eigenvalues will be grouped by
* split-off block (see IBLOCK, ISPLIT) and
* ordered from smallest to largest within
* the block.
* = 'E': ("Entire matrix")
* the eigenvalues for the entire matrix
* will be ordered from smallest to
* largest.
*
* N (input) INTEGER
* The order of the tridiagonal matrix T. N >= 0.
*
* VL (input) DOUBLE PRECISION
* VU (input) DOUBLE PRECISION
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. Eigenvalues less than or equal
* to VL, or greater than VU, will not be returned. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) DOUBLE PRECISION
* The absolute tolerance for the eigenvalues. An eigenvalue
* (or cluster) is considered to be located if it has been
* determined to lie in an interval whose width is ABSTOL or
* less. If ABSTOL is less than or equal to zero, then ULP*|T|
* will be used, where |T| means the 1-norm of T.
*
* Eigenvalues will be computed most accurately when ABSTOL is
* set to twice the underflow threshold 2*DLAMCH('S'), not zero.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The n diagonal elements of the tridiagonal matrix T.
*
* E (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) off-diagonal elements of the tridiagonal matrix T.
*
* M (output) INTEGER
* The actual number of eigenvalues found. 0 <= M <= N.
* (See also the description of INFO=2,3.)
*
* NSPLIT (output) INTEGER
* The number of diagonal blocks in the matrix T.
* 1 <= NSPLIT <= N.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* On exit, the first M elements of W will contain the
* eigenvalues. (DSTEBZ may use the remaining N-M elements as
* workspace.)
*
* IBLOCK (output) INTEGER array, dimension (N)
* At each row/column j where E(j) is zero or small, the
* matrix T is considered to split into a block diagonal
* matrix. On exit, if INFO = 0, IBLOCK(i) specifies to which
* block (from 1 to the number of blocks) the eigenvalue W(i)
* belongs. (DSTEBZ may use the remaining N-M elements as
* workspace.)
*
* ISPLIT (output) INTEGER array, dimension (N)
* The splitting points, at which T breaks up into submatrices.
* The first submatrix consists of rows/columns 1 to ISPLIT(1),
* the second of rows/columns ISPLIT(1)+1 through ISPLIT(2),
* etc., and the NSPLIT-th consists of rows/columns
* ISPLIT(NSPLIT-1)+1 through ISPLIT(NSPLIT)=N.
* (Only the first NSPLIT elements will actually be used, but
* since the user cannot know a priori what value NSPLIT will
* have, N words must be reserved for ISPLIT.)
*
* WORK (workspace) DOUBLE PRECISION array, dimension (4*N)
*
* IWORK (workspace) INTEGER array, dimension (3*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: some or all of the eigenvalues failed to converge or
* were not computed:
* =1 or 3: Bisection failed to converge for some
* eigenvalues; these eigenvalues are flagged by a
* negative block number. The effect is that the
* eigenvalues may not be as accurate as the
* absolute and relative tolerances. This is
* generally caused by unexpectedly inaccurate
* arithmetic.
* =2 or 3: RANGE='I' only: Not all of the eigenvalues
* IL:IU were found.
* Effect: M < IU+1-IL
* Cause: non-monotonic arithmetic, causing the
* Sturm sequence to be non-monotonic.
* Cure: recalculate, using RANGE='A', and pick
* out eigenvalues IL:IU. In some cases,
* increasing the PARAMETER "FUDGE" may
* make things work.
* = 4: RANGE='I', and the Gershgorin interval
* initially used was too small. No eigenvalues
* were computed.
* Probable cause: your machine has sloppy
* floating-point arithmetic.
* Cure: Increase the PARAMETER "FUDGE",
* recompile, and try again.
*
* Internal Parameters
* ===================
*
* RELFAC DOUBLE PRECISION, default = 2.0e0
* The relative tolerance. An interval (a,b] lies within
* "relative tolerance" if b-a < RELFAC*ulp*max(|a|,|b|),
* where "ulp" is the machine precision (distance from 1 to
* the next larger floating point number.)
*
* FUDGE DOUBLE PRECISION, default = 2
* A "fudge factor" to widen the Gershgorin intervals. Ideally,
* a value of 1 should work, but on machines with sloppy
* arithmetic, this needs to be larger. The default for
* publicly released versions should be large enough to handle
* the worst machine around. Note that this has no effect
* on accuracy of the solution.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param range
* @param order
* @param n
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param d
* @param e
* @param m
* @param nsplit
* @param w
* @param iblock
* @param isplit
* @param work
* @param iwork
* @param info
*
*/
abstract public void dstebz(java.lang.String range, java.lang.String order, int n, double vl, double vu, int il, int iu, double abstol, double[] d, double[] e, org.netlib.util.intW m, org.netlib.util.intW nsplit, double[] w, int[] iblock, int[] isplit, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSTEBZ computes the eigenvalues of a symmetric tridiagonal
* matrix T. The user may ask for all eigenvalues, all eigenvalues
* in the half-open interval (VL, VU], or the IL-th through IU-th
* eigenvalues.
*
* To avoid overflow, the matrix must be scaled so that its
* largest element is no greater than overflow**(1/2) *
* underflow**(1/4) in absolute value, and for greatest
* accuracy, it should not be much smaller than that.
*
* See W. Kahan "Accurate Eigenvalues of a Symmetric Tridiagonal
* Matrix", Report CS41, Computer Science Dept., Stanford
* University, July 21, 1966.
*
* Arguments
* =========
*
* RANGE (input) CHARACTER*1
* = 'A': ("All") all eigenvalues will be found.
* = 'V': ("Value") all eigenvalues in the half-open interval
* (VL, VU] will be found.
* = 'I': ("Index") the IL-th through IU-th eigenvalues (of the
* entire matrix) will be found.
*
* ORDER (input) CHARACTER*1
* = 'B': ("By Block") the eigenvalues will be grouped by
* split-off block (see IBLOCK, ISPLIT) and
* ordered from smallest to largest within
* the block.
* = 'E': ("Entire matrix")
* the eigenvalues for the entire matrix
* will be ordered from smallest to
* largest.
*
* N (input) INTEGER
* The order of the tridiagonal matrix T. N >= 0.
*
* VL (input) DOUBLE PRECISION
* VU (input) DOUBLE PRECISION
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. Eigenvalues less than or equal
* to VL, or greater than VU, will not be returned. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) DOUBLE PRECISION
* The absolute tolerance for the eigenvalues. An eigenvalue
* (or cluster) is considered to be located if it has been
* determined to lie in an interval whose width is ABSTOL or
* less. If ABSTOL is less than or equal to zero, then ULP*|T|
* will be used, where |T| means the 1-norm of T.
*
* Eigenvalues will be computed most accurately when ABSTOL is
* set to twice the underflow threshold 2*DLAMCH('S'), not zero.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The n diagonal elements of the tridiagonal matrix T.
*
* E (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) off-diagonal elements of the tridiagonal matrix T.
*
* M (output) INTEGER
* The actual number of eigenvalues found. 0 <= M <= N.
* (See also the description of INFO=2,3.)
*
* NSPLIT (output) INTEGER
* The number of diagonal blocks in the matrix T.
* 1 <= NSPLIT <= N.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* On exit, the first M elements of W will contain the
* eigenvalues. (DSTEBZ may use the remaining N-M elements as
* workspace.)
*
* IBLOCK (output) INTEGER array, dimension (N)
* At each row/column j where E(j) is zero or small, the
* matrix T is considered to split into a block diagonal
* matrix. On exit, if INFO = 0, IBLOCK(i) specifies to which
* block (from 1 to the number of blocks) the eigenvalue W(i)
* belongs. (DSTEBZ may use the remaining N-M elements as
* workspace.)
*
* ISPLIT (output) INTEGER array, dimension (N)
* The splitting points, at which T breaks up into submatrices.
* The first submatrix consists of rows/columns 1 to ISPLIT(1),
* the second of rows/columns ISPLIT(1)+1 through ISPLIT(2),
* etc., and the NSPLIT-th consists of rows/columns
* ISPLIT(NSPLIT-1)+1 through ISPLIT(NSPLIT)=N.
* (Only the first NSPLIT elements will actually be used, but
* since the user cannot know a priori what value NSPLIT will
* have, N words must be reserved for ISPLIT.)
*
* WORK (workspace) DOUBLE PRECISION array, dimension (4*N)
*
* IWORK (workspace) INTEGER array, dimension (3*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: some or all of the eigenvalues failed to converge or
* were not computed:
* =1 or 3: Bisection failed to converge for some
* eigenvalues; these eigenvalues are flagged by a
* negative block number. The effect is that the
* eigenvalues may not be as accurate as the
* absolute and relative tolerances. This is
* generally caused by unexpectedly inaccurate
* arithmetic.
* =2 or 3: RANGE='I' only: Not all of the eigenvalues
* IL:IU were found.
* Effect: M < IU+1-IL
* Cause: non-monotonic arithmetic, causing the
* Sturm sequence to be non-monotonic.
* Cure: recalculate, using RANGE='A', and pick
* out eigenvalues IL:IU. In some cases,
* increasing the PARAMETER "FUDGE" may
* make things work.
* = 4: RANGE='I', and the Gershgorin interval
* initially used was too small. No eigenvalues
* were computed.
* Probable cause: your machine has sloppy
* floating-point arithmetic.
* Cure: Increase the PARAMETER "FUDGE",
* recompile, and try again.
*
* Internal Parameters
* ===================
*
* RELFAC DOUBLE PRECISION, default = 2.0e0
* The relative tolerance. An interval (a,b] lies within
* "relative tolerance" if b-a < RELFAC*ulp*max(|a|,|b|),
* where "ulp" is the machine precision (distance from 1 to
* the next larger floating point number.)
*
* FUDGE DOUBLE PRECISION, default = 2
* A "fudge factor" to widen the Gershgorin intervals. Ideally,
* a value of 1 should work, but on machines with sloppy
* arithmetic, this needs to be larger. The default for
* publicly released versions should be large enough to handle
* the worst machine around. Note that this has no effect
* on accuracy of the solution.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param range
* @param order
* @param n
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param m
* @param nsplit
* @param w
* @param _w_offset
* @param iblock
* @param _iblock_offset
* @param isplit
* @param _isplit_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dstebz(java.lang.String range, java.lang.String order, int n, double vl, double vu, int il, int iu, double abstol, double[] d, int _d_offset, double[] e, int _e_offset, org.netlib.util.intW m, org.netlib.util.intW nsplit, double[] w, int _w_offset, int[] iblock, int _iblock_offset, int[] isplit, int _isplit_offset, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSTEDC computes all eigenvalues and, optionally, eigenvectors of a
* symmetric tridiagonal matrix using the divide and conquer method.
* The eigenvectors of a full or band real symmetric matrix can also be
* found if DSYTRD or DSPTRD or DSBTRD has been used to reduce this
* matrix to tridiagonal form.
*
* This code makes very mild assumptions about floating point
* arithmetic. It will work on machines with a guard digit in
* add/subtract, or on those binary machines without guard digits
* which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or Cray-2.
* It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none. See DLAED3 for details.
*
* Arguments
* =========
*
* COMPZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only.
* = 'I': Compute eigenvectors of tridiagonal matrix also.
* = 'V': Compute eigenvectors of original dense symmetric
* matrix also. On entry, Z contains the orthogonal
* matrix used to reduce the original matrix to
* tridiagonal form.
*
* N (input) INTEGER
* The dimension of the symmetric tridiagonal matrix. N >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the diagonal elements of the tridiagonal matrix.
* On exit, if INFO = 0, the eigenvalues in ascending order.
*
* E (input/output) DOUBLE PRECISION array, dimension (N-1)
* On entry, the subdiagonal elements of the tridiagonal matrix.
* On exit, E has been destroyed.
*
* Z (input/output) DOUBLE PRECISION array, dimension (LDZ,N)
* On entry, if COMPZ = 'V', then Z contains the orthogonal
* matrix used in the reduction to tridiagonal form.
* On exit, if INFO = 0, then if COMPZ = 'V', Z contains the
* orthonormal eigenvectors of the original symmetric matrix,
* and if COMPZ = 'I', Z contains the orthonormal eigenvectors
* of the symmetric tridiagonal matrix.
* If COMPZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1.
* If eigenvectors are desired, then LDZ >= max(1,N).
*
* WORK (workspace/output) DOUBLE PRECISION array,
* dimension (LWORK)
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If COMPZ = 'N' or N <= 1 then LWORK must be at least 1.
* If COMPZ = 'V' and N > 1 then LWORK must be at least
* ( 1 + 3*N + 2*N*lg N + 3*N**2 ),
* where lg( N ) = smallest integer k such
* that 2**k >= N.
* If COMPZ = 'I' and N > 1 then LWORK must be at least
* ( 1 + 4*N + N**2 ).
* Note that for COMPZ = 'I' or 'V', then if N is less than or
* equal to the minimum divide size, usually 25, then LWORK need
* only be max(1,2*(N-1)).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If COMPZ = 'N' or N <= 1 then LIWORK must be at least 1.
* If COMPZ = 'V' and N > 1 then LIWORK must be at least
* ( 6 + 6*N + 5*N*lg N ).
* If COMPZ = 'I' and N > 1 then LIWORK must be at least
* ( 3 + 5*N ).
* Note that for COMPZ = 'I' or 'V', then if N is less than or
* equal to the minimum divide size, usually 25, then LIWORK
* need only be 1.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal size of the IWORK array,
* returns this value as the first entry of the IWORK array, and
* no error message related to LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: The algorithm failed to compute an eigenvalue while
* working on the submatrix lying in rows and columns
* INFO/(N+1) through mod(INFO,N+1).
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
* Modified by Francoise Tisseur, University of Tennessee.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param compz
* @param n
* @param d
* @param e
* @param z
* @param ldz
* @param work
* @param lwork
* @param iwork
* @param liwork
* @param info
*
*/
abstract public void dstedc(java.lang.String compz, int n, double[] d, double[] e, double[] z, int ldz, double[] work, int lwork, int[] iwork, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSTEDC computes all eigenvalues and, optionally, eigenvectors of a
* symmetric tridiagonal matrix using the divide and conquer method.
* The eigenvectors of a full or band real symmetric matrix can also be
* found if DSYTRD or DSPTRD or DSBTRD has been used to reduce this
* matrix to tridiagonal form.
*
* This code makes very mild assumptions about floating point
* arithmetic. It will work on machines with a guard digit in
* add/subtract, or on those binary machines without guard digits
* which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or Cray-2.
* It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none. See DLAED3 for details.
*
* Arguments
* =========
*
* COMPZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only.
* = 'I': Compute eigenvectors of tridiagonal matrix also.
* = 'V': Compute eigenvectors of original dense symmetric
* matrix also. On entry, Z contains the orthogonal
* matrix used to reduce the original matrix to
* tridiagonal form.
*
* N (input) INTEGER
* The dimension of the symmetric tridiagonal matrix. N >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the diagonal elements of the tridiagonal matrix.
* On exit, if INFO = 0, the eigenvalues in ascending order.
*
* E (input/output) DOUBLE PRECISION array, dimension (N-1)
* On entry, the subdiagonal elements of the tridiagonal matrix.
* On exit, E has been destroyed.
*
* Z (input/output) DOUBLE PRECISION array, dimension (LDZ,N)
* On entry, if COMPZ = 'V', then Z contains the orthogonal
* matrix used in the reduction to tridiagonal form.
* On exit, if INFO = 0, then if COMPZ = 'V', Z contains the
* orthonormal eigenvectors of the original symmetric matrix,
* and if COMPZ = 'I', Z contains the orthonormal eigenvectors
* of the symmetric tridiagonal matrix.
* If COMPZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1.
* If eigenvectors are desired, then LDZ >= max(1,N).
*
* WORK (workspace/output) DOUBLE PRECISION array,
* dimension (LWORK)
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If COMPZ = 'N' or N <= 1 then LWORK must be at least 1.
* If COMPZ = 'V' and N > 1 then LWORK must be at least
* ( 1 + 3*N + 2*N*lg N + 3*N**2 ),
* where lg( N ) = smallest integer k such
* that 2**k >= N.
* If COMPZ = 'I' and N > 1 then LWORK must be at least
* ( 1 + 4*N + N**2 ).
* Note that for COMPZ = 'I' or 'V', then if N is less than or
* equal to the minimum divide size, usually 25, then LWORK need
* only be max(1,2*(N-1)).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If COMPZ = 'N' or N <= 1 then LIWORK must be at least 1.
* If COMPZ = 'V' and N > 1 then LIWORK must be at least
* ( 6 + 6*N + 5*N*lg N ).
* If COMPZ = 'I' and N > 1 then LIWORK must be at least
* ( 3 + 5*N ).
* Note that for COMPZ = 'I' or 'V', then if N is less than or
* equal to the minimum divide size, usually 25, then LIWORK
* need only be 1.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal size of the IWORK array,
* returns this value as the first entry of the IWORK array, and
* no error message related to LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: The algorithm failed to compute an eigenvalue while
* working on the submatrix lying in rows and columns
* INFO/(N+1) through mod(INFO,N+1).
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
* Modified by Francoise Tisseur, University of Tennessee.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param compz
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param liwork
* @param info
*
*/
abstract public void dstedc(java.lang.String compz, int n, double[] d, int _d_offset, double[] e, int _e_offset, double[] z, int _z_offset, int ldz, double[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSTEGR computes selected eigenvalues and, optionally, eigenvectors
* of a real symmetric tridiagonal matrix T. Any such unreduced matrix h
* a well defined set of pairwise different real eigenvalues, the corres
* real eigenvectors are pairwise orthogonal.
*
* The spectrum may be computed either completely or partially by specif
* either an interval (VL,VU] or a range of indices IL:IU for the desire
* eigenvalues.
*
* DSTEGR is a compatability wrapper around the improved DSTEMR routine.
* See DSTEMR for further details.
*
* One important change is that the ABSTOL parameter no longer provides
* benefit and hence is no longer used.
*
* Note : DSTEGR and DSTEMR work only on machines which follow
* IEEE-754 floating-point standard in their handling of infinities and
* NaNs. Normal execution may create these exceptiona values and hence
* may abort due to a floating point exception in environments which
* do not conform to the IEEE-754 standard.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the N diagonal elements of the tridiagonal matrix
* T. On exit, D is overwritten.
*
* E (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the (N-1) subdiagonal elements of the tridiagonal
* matrix T in elements 1 to N-1 of E. E(N) need not be set on
* input, but is used internally as workspace.
* On exit, E is overwritten.
*
* VL (input) DOUBLE PRECISION
* VU (input) DOUBLE PRECISION
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) DOUBLE PRECISION
* Unused. Was the absolute error tolerance for the
* eigenvalues/eigenvectors in previous versions.
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* The first M elements contain the selected eigenvalues in
* ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, max(1,M) )
* If JOBZ = 'V', and if INFO = 0, then the first M columns of Z
* contain the orthonormal eigenvectors of the matrix T
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* If JOBZ = 'N', then Z is not referenced.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
* Supplying N columns is always safe.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', then LDZ >= max(1,N).
*
* ISUPPZ (output) INTEGER ARRAY, dimension ( 2*max(1,M) )
* The support of the eigenvectors in Z, i.e., the indices
* indicating the nonzero elements in Z. The i-th computed eigen
* is nonzero only in elements ISUPPZ( 2*i-1 ) through
* ISUPPZ( 2*i ). This is relevant in the case when the matrix
* is split. ISUPPZ is only accessed when JOBZ is 'V' and N > 0.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (LWORK)
* On exit, if INFO = 0, WORK(1) returns the optimal
* (and minimal) LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,18*N)
* if JOBZ = 'V', and LWORK >= max(1,12*N) if JOBZ = 'N'.
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (LIWORK)
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK. LIWORK >= max(1,10*N)
* if the eigenvectors are desired, and LIWORK >= max(1,8*N)
* if only the eigenvalues are to be computed.
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal size of the IWORK array,
* returns this value as the first entry of the IWORK array, and
* no error message related to LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* On exit, INFO
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = 1X, internal error in DLARRE,
* if INFO = 2X, internal error in DLARRV.
* Here, the digit X = ABS( IINFO ) < 10, where IINFO is
* the nonzero error code returned by DLARRE or
* DLARRV, respectively.
*
* Further Details
* ===============
*
* Based on contributions by
* Inderjit Dhillon, IBM Almaden, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, LBNL/NERSC, USA
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param jobz
* @param range
* @param n
* @param d
* @param e
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param z
* @param ldz
* @param isuppz
* @param work
* @param lwork
* @param iwork
* @param liwork
* @param info
*
*/
abstract public void dstegr(java.lang.String jobz, java.lang.String range, int n, double[] d, double[] e, double vl, double vu, int il, int iu, double abstol, org.netlib.util.intW m, double[] w, double[] z, int ldz, int[] isuppz, double[] work, int lwork, int[] iwork, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSTEGR computes selected eigenvalues and, optionally, eigenvectors
* of a real symmetric tridiagonal matrix T. Any such unreduced matrix h
* a well defined set of pairwise different real eigenvalues, the corres
* real eigenvectors are pairwise orthogonal.
*
* The spectrum may be computed either completely or partially by specif
* either an interval (VL,VU] or a range of indices IL:IU for the desire
* eigenvalues.
*
* DSTEGR is a compatability wrapper around the improved DSTEMR routine.
* See DSTEMR for further details.
*
* One important change is that the ABSTOL parameter no longer provides
* benefit and hence is no longer used.
*
* Note : DSTEGR and DSTEMR work only on machines which follow
* IEEE-754 floating-point standard in their handling of infinities and
* NaNs. Normal execution may create these exceptiona values and hence
* may abort due to a floating point exception in environments which
* do not conform to the IEEE-754 standard.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the N diagonal elements of the tridiagonal matrix
* T. On exit, D is overwritten.
*
* E (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the (N-1) subdiagonal elements of the tridiagonal
* matrix T in elements 1 to N-1 of E. E(N) need not be set on
* input, but is used internally as workspace.
* On exit, E is overwritten.
*
* VL (input) DOUBLE PRECISION
* VU (input) DOUBLE PRECISION
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) DOUBLE PRECISION
* Unused. Was the absolute error tolerance for the
* eigenvalues/eigenvectors in previous versions.
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* The first M elements contain the selected eigenvalues in
* ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, max(1,M) )
* If JOBZ = 'V', and if INFO = 0, then the first M columns of Z
* contain the orthonormal eigenvectors of the matrix T
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* If JOBZ = 'N', then Z is not referenced.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
* Supplying N columns is always safe.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', then LDZ >= max(1,N).
*
* ISUPPZ (output) INTEGER ARRAY, dimension ( 2*max(1,M) )
* The support of the eigenvectors in Z, i.e., the indices
* indicating the nonzero elements in Z. The i-th computed eigen
* is nonzero only in elements ISUPPZ( 2*i-1 ) through
* ISUPPZ( 2*i ). This is relevant in the case when the matrix
* is split. ISUPPZ is only accessed when JOBZ is 'V' and N > 0.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (LWORK)
* On exit, if INFO = 0, WORK(1) returns the optimal
* (and minimal) LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,18*N)
* if JOBZ = 'V', and LWORK >= max(1,12*N) if JOBZ = 'N'.
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (LIWORK)
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK. LIWORK >= max(1,10*N)
* if the eigenvectors are desired, and LIWORK >= max(1,8*N)
* if only the eigenvalues are to be computed.
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal size of the IWORK array,
* returns this value as the first entry of the IWORK array, and
* no error message related to LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* On exit, INFO
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = 1X, internal error in DLARRE,
* if INFO = 2X, internal error in DLARRV.
* Here, the digit X = ABS( IINFO ) < 10, where IINFO is
* the nonzero error code returned by DLARRE or
* DLARRV, respectively.
*
* Further Details
* ===============
*
* Based on contributions by
* Inderjit Dhillon, IBM Almaden, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, LBNL/NERSC, USA
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param jobz
* @param range
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param isuppz
* @param _isuppz_offset
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param liwork
* @param info
*
*/
abstract public void dstegr(java.lang.String jobz, java.lang.String range, int n, double[] d, int _d_offset, double[] e, int _e_offset, double vl, double vu, int il, int iu, double abstol, org.netlib.util.intW m, double[] w, int _w_offset, double[] z, int _z_offset, int ldz, int[] isuppz, int _isuppz_offset, double[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSTEIN computes the eigenvectors of a real symmetric tridiagonal
* matrix T corresponding to specified eigenvalues, using inverse
* iteration.
*
* The maximum number of iterations allowed for each eigenvector is
* specified by an internal parameter MAXITS (currently set to 5).
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The n diagonal elements of the tridiagonal matrix T.
*
* E (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) subdiagonal elements of the tridiagonal matrix
* T, in elements 1 to N-1.
*
* M (input) INTEGER
* The number of eigenvectors to be found. 0 <= M <= N.
*
* W (input) DOUBLE PRECISION array, dimension (N)
* The first M elements of W contain the eigenvalues for
* which eigenvectors are to be computed. The eigenvalues
* should be grouped by split-off block and ordered from
* smallest to largest within the block. ( The output array
* W from DSTEBZ with ORDER = 'B' is expected here. )
*
* IBLOCK (input) INTEGER array, dimension (N)
* The submatrix indices associated with the corresponding
* eigenvalues in W; IBLOCK(i)=1 if eigenvalue W(i) belongs to
* the first submatrix from the top, =2 if W(i) belongs to
* the second submatrix, etc. ( The output array IBLOCK
* from DSTEBZ is expected here. )
*
* ISPLIT (input) INTEGER array, dimension (N)
* The splitting points, at which T breaks up into submatrices.
* The first submatrix consists of rows/columns 1 to
* ISPLIT( 1 ), the second of rows/columns ISPLIT( 1 )+1
* through ISPLIT( 2 ), etc.
* ( The output array ISPLIT from DSTEBZ is expected here. )
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, M)
* The computed eigenvectors. The eigenvector associated
* with the eigenvalue W(i) is stored in the i-th column of
* Z. Any vector which fails to converge is set to its current
* iterate after MAXITS iterations.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= max(1,N).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (5*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* IFAIL (output) INTEGER array, dimension (M)
* On normal exit, all elements of IFAIL are zero.
* If one or more eigenvectors fail to converge after
* MAXITS iterations, then their indices are stored in
* array IFAIL.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, then i eigenvectors failed to converge
* in MAXITS iterations. Their indices are stored in
* array IFAIL.
*
* Internal Parameters
* ===================
*
* MAXITS INTEGER, default = 5
* The maximum number of iterations performed.
*
* EXTRA INTEGER, default = 2
* The number of iterations performed after norm growth
* criterion is satisfied, should be at least 1.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param e
* @param m
* @param w
* @param iblock
* @param isplit
* @param z
* @param ldz
* @param work
* @param iwork
* @param ifail
* @param info
*
*/
abstract public void dstein(int n, double[] d, double[] e, int m, double[] w, int[] iblock, int[] isplit, double[] z, int ldz, double[] work, int[] iwork, int[] ifail, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSTEIN computes the eigenvectors of a real symmetric tridiagonal
* matrix T corresponding to specified eigenvalues, using inverse
* iteration.
*
* The maximum number of iterations allowed for each eigenvector is
* specified by an internal parameter MAXITS (currently set to 5).
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* The n diagonal elements of the tridiagonal matrix T.
*
* E (input) DOUBLE PRECISION array, dimension (N-1)
* The (n-1) subdiagonal elements of the tridiagonal matrix
* T, in elements 1 to N-1.
*
* M (input) INTEGER
* The number of eigenvectors to be found. 0 <= M <= N.
*
* W (input) DOUBLE PRECISION array, dimension (N)
* The first M elements of W contain the eigenvalues for
* which eigenvectors are to be computed. The eigenvalues
* should be grouped by split-off block and ordered from
* smallest to largest within the block. ( The output array
* W from DSTEBZ with ORDER = 'B' is expected here. )
*
* IBLOCK (input) INTEGER array, dimension (N)
* The submatrix indices associated with the corresponding
* eigenvalues in W; IBLOCK(i)=1 if eigenvalue W(i) belongs to
* the first submatrix from the top, =2 if W(i) belongs to
* the second submatrix, etc. ( The output array IBLOCK
* from DSTEBZ is expected here. )
*
* ISPLIT (input) INTEGER array, dimension (N)
* The splitting points, at which T breaks up into submatrices.
* The first submatrix consists of rows/columns 1 to
* ISPLIT( 1 ), the second of rows/columns ISPLIT( 1 )+1
* through ISPLIT( 2 ), etc.
* ( The output array ISPLIT from DSTEBZ is expected here. )
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, M)
* The computed eigenvectors. The eigenvector associated
* with the eigenvalue W(i) is stored in the i-th column of
* Z. Any vector which fails to converge is set to its current
* iterate after MAXITS iterations.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= max(1,N).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (5*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* IFAIL (output) INTEGER array, dimension (M)
* On normal exit, all elements of IFAIL are zero.
* If one or more eigenvectors fail to converge after
* MAXITS iterations, then their indices are stored in
* array IFAIL.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, then i eigenvectors failed to converge
* in MAXITS iterations. Their indices are stored in
* array IFAIL.
*
* Internal Parameters
* ===================
*
* MAXITS INTEGER, default = 5
* The maximum number of iterations performed.
*
* EXTRA INTEGER, default = 2
* The number of iterations performed after norm growth
* criterion is satisfied, should be at least 1.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param m
* @param w
* @param _w_offset
* @param iblock
* @param _iblock_offset
* @param isplit
* @param _isplit_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param ifail
* @param _ifail_offset
* @param info
*
*/
abstract public void dstein(int n, double[] d, int _d_offset, double[] e, int _e_offset, int m, double[] w, int _w_offset, int[] iblock, int _iblock_offset, int[] isplit, int _isplit_offset, double[] z, int _z_offset, int ldz, double[] work, int _work_offset, int[] iwork, int _iwork_offset, int[] ifail, int _ifail_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSTEMR computes selected eigenvalues and, optionally, eigenvectors
* of a real symmetric tridiagonal matrix T. Any such unreduced matrix h
* a well defined set of pairwise different real eigenvalues, the corres
* real eigenvectors are pairwise orthogonal.
*
* The spectrum may be computed either completely or partially by specif
* either an interval (VL,VU] or a range of indices IL:IU for the desire
* eigenvalues.
*
* Depending on the number of desired eigenvalues, these are computed ei
* by bisection or the dqds algorithm. Numerically orthogonal eigenvecto
* computed by the use of various suitable L D L^T factorizations near c
* of close eigenvalues (referred to as RRRs, Relatively Robust
* Representations). An informal sketch of the algorithm follows.
*
* For each unreduced block (submatrix) of T,
* (a) Compute T - sigma I = L D L^T, so that L and D
* define all the wanted eigenvalues to high relative accuracy.
* This means that small relative changes in the entries of D and
* cause only small relative changes in the eigenvalues and
* eigenvectors. The standard (unfactored) representation of the
* tridiagonal matrix T does not have this property in general.
* (b) Compute the eigenvalues to suitable accuracy.
* If the eigenvectors are desired, the algorithm attains full
* accuracy of the computed eigenvalues only right before
* the corresponding vectors have to be computed, see steps c) an
* (c) For each cluster of close eigenvalues, select a new
* shift close to the cluster, find a new factorization, and refi
* the shifted eigenvalues to suitable accuracy.
* (d) For each eigenvalue with a large enough relative separation co
* the corresponding eigenvector by forming a rank revealing twis
* factorization. Go back to (c) for any clusters that remain.
*
* For more details, see:
* - Inderjit S. Dhillon and Beresford N. Parlett: "Multiple representat
* to compute orthogonal eigenvectors of symmetric tridiagonal matrice
* Linear Algebra and its Applications, 387(1), pp. 1-28, August 2004.
* - Inderjit Dhillon and Beresford Parlett: "Orthogonal Eigenvectors an
* Relative Gaps," SIAM Journal on Matrix Analysis and Applications, V
* 2004. Also LAPACK Working Note 154.
* - Inderjit Dhillon: "A new O(n^2) algorithm for the symmetric
* tridiagonal eigenvalue/eigenvector problem",
* Computer Science Division Technical Report No. UCB/CSD-97-971,
* UC Berkeley, May 1997.
*
* Notes:
* 1.DSTEMR works only on machines which follow IEEE-754
* floating-point standard in their handling of infinities and NaNs.
* This permits the use of efficient inner loops avoiding a check for
* zero divisors.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the N diagonal elements of the tridiagonal matrix
* T. On exit, D is overwritten.
*
* E (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the (N-1) subdiagonal elements of the tridiagonal
* matrix T in elements 1 to N-1 of E. E(N) need not be set on
* input, but is used internally as workspace.
* On exit, E is overwritten.
*
* VL (input) DOUBLE PRECISION
* VU (input) DOUBLE PRECISION
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* The first M elements contain the selected eigenvalues in
* ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, max(1,M) )
* If JOBZ = 'V', and if INFO = 0, then the first M columns of Z
* contain the orthonormal eigenvectors of the matrix T
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* If JOBZ = 'N', then Z is not referenced.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and can be computed with a workspace
* query by setting NZC = -1, see below.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', then LDZ >= max(1,N).
*
* NZC (input) INTEGER
* The number of eigenvectors to be held in the array Z.
* If RANGE = 'A', then NZC >= max(1,N).
* If RANGE = 'V', then NZC >= the number of eigenvalues in (VL,
* If RANGE = 'I', then NZC >= IU-IL+1.
* If NZC = -1, then a workspace query is assumed; the
* routine calculates the number of columns of the array Z that
* are needed to hold the eigenvectors.
* This value is returned as the first entry of the Z array, and
* no error message related to NZC is issued by XERBLA.
*
* ISUPPZ (output) INTEGER ARRAY, dimension ( 2*max(1,M) )
* The support of the eigenvectors in Z, i.e., the indices
* indicating the nonzero elements in Z. The i-th computed eigen
* is nonzero only in elements ISUPPZ( 2*i-1 ) through
* ISUPPZ( 2*i ). This is relevant in the case when the matrix
* is split. ISUPPZ is only accessed when JOBZ is 'V' and N > 0.
*
* TRYRAC (input/output) LOGICAL
* If TRYRAC.EQ..TRUE., indicates that the code should check whe
* the tridiagonal matrix defines its eigenvalues to high relati
* accuracy. If so, the code uses relative-accuracy preserving
* algorithms that might be (a bit) slower depending on the matr
* If the matrix does not define its eigenvalues to high relativ
* accuracy, the code can uses possibly faster algorithms.
* If TRYRAC.EQ..FALSE., the code is not required to guarantee
* relatively accurate eigenvalues and can use the fastest possi
* techniques.
* On exit, a .TRUE. TRYRAC will be set to .FALSE. if the matrix
* does not define its eigenvalues to high relative accuracy.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (LWORK)
* On exit, if INFO = 0, WORK(1) returns the optimal
* (and minimal) LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,18*N)
* if JOBZ = 'V', and LWORK >= max(1,12*N) if JOBZ = 'N'.
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (LIWORK)
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK. LIWORK >= max(1,10*N)
* if the eigenvectors are desired, and LIWORK >= max(1,8*N)
* if only the eigenvalues are to be computed.
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal size of the IWORK array,
* returns this value as the first entry of the IWORK array, and
* no error message related to LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* On exit, INFO
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = 1X, internal error in DLARRE,
* if INFO = 2X, internal error in DLARRV.
* Here, the digit X = ABS( IINFO ) < 10, where IINFO is
* the nonzero error code returned by DLARRE or
* DLARRV, respectively.
*
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param range
* @param n
* @param d
* @param e
* @param vl
* @param vu
* @param il
* @param iu
* @param m
* @param w
* @param z
* @param ldz
* @param nzc
* @param isuppz
* @param tryrac
* @param work
* @param lwork
* @param iwork
* @param liwork
* @param info
*
*/
abstract public void dstemr(java.lang.String jobz, java.lang.String range, int n, double[] d, double[] e, double vl, double vu, int il, int iu, org.netlib.util.intW m, double[] w, double[] z, int ldz, int nzc, int[] isuppz, org.netlib.util.booleanW tryrac, double[] work, int lwork, int[] iwork, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSTEMR computes selected eigenvalues and, optionally, eigenvectors
* of a real symmetric tridiagonal matrix T. Any such unreduced matrix h
* a well defined set of pairwise different real eigenvalues, the corres
* real eigenvectors are pairwise orthogonal.
*
* The spectrum may be computed either completely or partially by specif
* either an interval (VL,VU] or a range of indices IL:IU for the desire
* eigenvalues.
*
* Depending on the number of desired eigenvalues, these are computed ei
* by bisection or the dqds algorithm. Numerically orthogonal eigenvecto
* computed by the use of various suitable L D L^T factorizations near c
* of close eigenvalues (referred to as RRRs, Relatively Robust
* Representations). An informal sketch of the algorithm follows.
*
* For each unreduced block (submatrix) of T,
* (a) Compute T - sigma I = L D L^T, so that L and D
* define all the wanted eigenvalues to high relative accuracy.
* This means that small relative changes in the entries of D and
* cause only small relative changes in the eigenvalues and
* eigenvectors. The standard (unfactored) representation of the
* tridiagonal matrix T does not have this property in general.
* (b) Compute the eigenvalues to suitable accuracy.
* If the eigenvectors are desired, the algorithm attains full
* accuracy of the computed eigenvalues only right before
* the corresponding vectors have to be computed, see steps c) an
* (c) For each cluster of close eigenvalues, select a new
* shift close to the cluster, find a new factorization, and refi
* the shifted eigenvalues to suitable accuracy.
* (d) For each eigenvalue with a large enough relative separation co
* the corresponding eigenvector by forming a rank revealing twis
* factorization. Go back to (c) for any clusters that remain.
*
* For more details, see:
* - Inderjit S. Dhillon and Beresford N. Parlett: "Multiple representat
* to compute orthogonal eigenvectors of symmetric tridiagonal matrice
* Linear Algebra and its Applications, 387(1), pp. 1-28, August 2004.
* - Inderjit Dhillon and Beresford Parlett: "Orthogonal Eigenvectors an
* Relative Gaps," SIAM Journal on Matrix Analysis and Applications, V
* 2004. Also LAPACK Working Note 154.
* - Inderjit Dhillon: "A new O(n^2) algorithm for the symmetric
* tridiagonal eigenvalue/eigenvector problem",
* Computer Science Division Technical Report No. UCB/CSD-97-971,
* UC Berkeley, May 1997.
*
* Notes:
* 1.DSTEMR works only on machines which follow IEEE-754
* floating-point standard in their handling of infinities and NaNs.
* This permits the use of efficient inner loops avoiding a check for
* zero divisors.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the N diagonal elements of the tridiagonal matrix
* T. On exit, D is overwritten.
*
* E (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the (N-1) subdiagonal elements of the tridiagonal
* matrix T in elements 1 to N-1 of E. E(N) need not be set on
* input, but is used internally as workspace.
* On exit, E is overwritten.
*
* VL (input) DOUBLE PRECISION
* VU (input) DOUBLE PRECISION
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* The first M elements contain the selected eigenvalues in
* ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, max(1,M) )
* If JOBZ = 'V', and if INFO = 0, then the first M columns of Z
* contain the orthonormal eigenvectors of the matrix T
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* If JOBZ = 'N', then Z is not referenced.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and can be computed with a workspace
* query by setting NZC = -1, see below.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', then LDZ >= max(1,N).
*
* NZC (input) INTEGER
* The number of eigenvectors to be held in the array Z.
* If RANGE = 'A', then NZC >= max(1,N).
* If RANGE = 'V', then NZC >= the number of eigenvalues in (VL,
* If RANGE = 'I', then NZC >= IU-IL+1.
* If NZC = -1, then a workspace query is assumed; the
* routine calculates the number of columns of the array Z that
* are needed to hold the eigenvectors.
* This value is returned as the first entry of the Z array, and
* no error message related to NZC is issued by XERBLA.
*
* ISUPPZ (output) INTEGER ARRAY, dimension ( 2*max(1,M) )
* The support of the eigenvectors in Z, i.e., the indices
* indicating the nonzero elements in Z. The i-th computed eigen
* is nonzero only in elements ISUPPZ( 2*i-1 ) through
* ISUPPZ( 2*i ). This is relevant in the case when the matrix
* is split. ISUPPZ is only accessed when JOBZ is 'V' and N > 0.
*
* TRYRAC (input/output) LOGICAL
* If TRYRAC.EQ..TRUE., indicates that the code should check whe
* the tridiagonal matrix defines its eigenvalues to high relati
* accuracy. If so, the code uses relative-accuracy preserving
* algorithms that might be (a bit) slower depending on the matr
* If the matrix does not define its eigenvalues to high relativ
* accuracy, the code can uses possibly faster algorithms.
* If TRYRAC.EQ..FALSE., the code is not required to guarantee
* relatively accurate eigenvalues and can use the fastest possi
* techniques.
* On exit, a .TRUE. TRYRAC will be set to .FALSE. if the matrix
* does not define its eigenvalues to high relative accuracy.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (LWORK)
* On exit, if INFO = 0, WORK(1) returns the optimal
* (and minimal) LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,18*N)
* if JOBZ = 'V', and LWORK >= max(1,12*N) if JOBZ = 'N'.
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (LIWORK)
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK. LIWORK >= max(1,10*N)
* if the eigenvectors are desired, and LIWORK >= max(1,8*N)
* if only the eigenvalues are to be computed.
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal size of the IWORK array,
* returns this value as the first entry of the IWORK array, and
* no error message related to LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* On exit, INFO
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = 1X, internal error in DLARRE,
* if INFO = 2X, internal error in DLARRV.
* Here, the digit X = ABS( IINFO ) < 10, where IINFO is
* the nonzero error code returned by DLARRE or
* DLARRV, respectively.
*
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param range
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param vl
* @param vu
* @param il
* @param iu
* @param m
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param nzc
* @param isuppz
* @param _isuppz_offset
* @param tryrac
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param liwork
* @param info
*
*/
abstract public void dstemr(java.lang.String jobz, java.lang.String range, int n, double[] d, int _d_offset, double[] e, int _e_offset, double vl, double vu, int il, int iu, org.netlib.util.intW m, double[] w, int _w_offset, double[] z, int _z_offset, int ldz, int nzc, int[] isuppz, int _isuppz_offset, org.netlib.util.booleanW tryrac, double[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSTEQR computes all eigenvalues and, optionally, eigenvectors of a
* symmetric tridiagonal matrix using the implicit QL or QR method.
* The eigenvectors of a full or band symmetric matrix can also be found
* if DSYTRD or DSPTRD or DSBTRD has been used to reduce this matrix to
* tridiagonal form.
*
* Arguments
* =========
*
* COMPZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only.
* = 'V': Compute eigenvalues and eigenvectors of the original
* symmetric matrix. On entry, Z must contain the
* orthogonal matrix used to reduce the original matrix
* to tridiagonal form.
* = 'I': Compute eigenvalues and eigenvectors of the
* tridiagonal matrix. Z is initialized to the identity
* matrix.
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the diagonal elements of the tridiagonal matrix.
* On exit, if INFO = 0, the eigenvalues in ascending order.
*
* E (input/output) DOUBLE PRECISION array, dimension (N-1)
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix.
* On exit, E has been destroyed.
*
* Z (input/output) DOUBLE PRECISION array, dimension (LDZ, N)
* On entry, if COMPZ = 'V', then Z contains the orthogonal
* matrix used in the reduction to tridiagonal form.
* On exit, if INFO = 0, then if COMPZ = 'V', Z contains the
* orthonormal eigenvectors of the original symmetric matrix,
* and if COMPZ = 'I', Z contains the orthonormal eigenvectors
* of the symmetric tridiagonal matrix.
* If COMPZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* eigenvectors are desired, then LDZ >= max(1,N).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (max(1,2*N-2))
* If COMPZ = 'N', then WORK is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: the algorithm has failed to find all the eigenvalues in
* a total of 30*N iterations; if INFO = i, then i
* elements of E have not converged to zero; on exit, D
* and E contain the elements of a symmetric tridiagonal
* matrix which is orthogonally similar to the original
* matrix.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param compz
* @param n
* @param d
* @param e
* @param z
* @param ldz
* @param work
* @param info
*
*/
abstract public void dsteqr(java.lang.String compz, int n, double[] d, double[] e, double[] z, int ldz, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSTEQR computes all eigenvalues and, optionally, eigenvectors of a
* symmetric tridiagonal matrix using the implicit QL or QR method.
* The eigenvectors of a full or band symmetric matrix can also be found
* if DSYTRD or DSPTRD or DSBTRD has been used to reduce this matrix to
* tridiagonal form.
*
* Arguments
* =========
*
* COMPZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only.
* = 'V': Compute eigenvalues and eigenvectors of the original
* symmetric matrix. On entry, Z must contain the
* orthogonal matrix used to reduce the original matrix
* to tridiagonal form.
* = 'I': Compute eigenvalues and eigenvectors of the
* tridiagonal matrix. Z is initialized to the identity
* matrix.
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the diagonal elements of the tridiagonal matrix.
* On exit, if INFO = 0, the eigenvalues in ascending order.
*
* E (input/output) DOUBLE PRECISION array, dimension (N-1)
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix.
* On exit, E has been destroyed.
*
* Z (input/output) DOUBLE PRECISION array, dimension (LDZ, N)
* On entry, if COMPZ = 'V', then Z contains the orthogonal
* matrix used in the reduction to tridiagonal form.
* On exit, if INFO = 0, then if COMPZ = 'V', Z contains the
* orthonormal eigenvectors of the original symmetric matrix,
* and if COMPZ = 'I', Z contains the orthonormal eigenvectors
* of the symmetric tridiagonal matrix.
* If COMPZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* eigenvectors are desired, then LDZ >= max(1,N).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (max(1,2*N-2))
* If COMPZ = 'N', then WORK is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: the algorithm has failed to find all the eigenvalues in
* a total of 30*N iterations; if INFO = i, then i
* elements of E have not converged to zero; on exit, D
* and E contain the elements of a symmetric tridiagonal
* matrix which is orthogonally similar to the original
* matrix.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param compz
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dsteqr(java.lang.String compz, int n, double[] d, int _d_offset, double[] e, int _e_offset, double[] z, int _z_offset, int ldz, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSTERF computes all eigenvalues of a symmetric tridiagonal matrix
* using the Pal-Walker-Kahan variant of the QL or QR algorithm.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the n diagonal elements of the tridiagonal matrix.
* On exit, if INFO = 0, the eigenvalues in ascending order.
*
* E (input/output) DOUBLE PRECISION array, dimension (N-1)
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix.
* On exit, E has been destroyed.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: the algorithm failed to find all of the eigenvalues in
* a total of 30*N iterations; if INFO = i, then i
* elements of E have not converged to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param e
* @param info
*
*/
abstract public void dsterf(int n, double[] d, double[] e, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSTERF computes all eigenvalues of a symmetric tridiagonal matrix
* using the Pal-Walker-Kahan variant of the QL or QR algorithm.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the n diagonal elements of the tridiagonal matrix.
* On exit, if INFO = 0, the eigenvalues in ascending order.
*
* E (input/output) DOUBLE PRECISION array, dimension (N-1)
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix.
* On exit, E has been destroyed.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: the algorithm failed to find all of the eigenvalues in
* a total of 30*N iterations; if INFO = i, then i
* elements of E have not converged to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param info
*
*/
abstract public void dsterf(int n, double[] d, int _d_offset, double[] e, int _e_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSTEV computes all eigenvalues and, optionally, eigenvectors of a
* real symmetric tridiagonal matrix A.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the n diagonal elements of the tridiagonal matrix
* A.
* On exit, if INFO = 0, the eigenvalues in ascending order.
*
* E (input/output) DOUBLE PRECISION array, dimension (N-1)
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix A, stored in elements 1 to N-1 of E.
* On exit, the contents of E are destroyed.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the orthonormal
* eigenvectors of the matrix A, with the i-th column of Z
* holding the eigenvector associated with D(i).
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (max(1,2*N-2))
* If JOBZ = 'N', WORK is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the algorithm failed to converge; i
* off-diagonal elements of E did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param n
* @param d
* @param e
* @param z
* @param ldz
* @param work
* @param info
*
*/
abstract public void dstev(java.lang.String jobz, int n, double[] d, double[] e, double[] z, int ldz, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSTEV computes all eigenvalues and, optionally, eigenvectors of a
* real symmetric tridiagonal matrix A.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the n diagonal elements of the tridiagonal matrix
* A.
* On exit, if INFO = 0, the eigenvalues in ascending order.
*
* E (input/output) DOUBLE PRECISION array, dimension (N-1)
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix A, stored in elements 1 to N-1 of E.
* On exit, the contents of E are destroyed.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the orthonormal
* eigenvectors of the matrix A, with the i-th column of Z
* holding the eigenvector associated with D(i).
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (max(1,2*N-2))
* If JOBZ = 'N', WORK is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the algorithm failed to converge; i
* off-diagonal elements of E did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dstev(java.lang.String jobz, int n, double[] d, int _d_offset, double[] e, int _e_offset, double[] z, int _z_offset, int ldz, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSTEVD computes all eigenvalues and, optionally, eigenvectors of a
* real symmetric tridiagonal matrix. If eigenvectors are desired, it
* uses a divide and conquer algorithm.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the n diagonal elements of the tridiagonal matrix
* A.
* On exit, if INFO = 0, the eigenvalues in ascending order.
*
* E (input/output) DOUBLE PRECISION array, dimension (N-1)
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix A, stored in elements 1 to N-1 of E.
* On exit, the contents of E are destroyed.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the orthonormal
* eigenvectors of the matrix A, with the i-th column of Z
* holding the eigenvector associated with D(i).
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace/output) DOUBLE PRECISION array,
* dimension (LWORK)
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If JOBZ = 'N' or N <= 1 then LWORK must be at least 1.
* If JOBZ = 'V' and N > 1 then LWORK must be at least
* ( 1 + 4*N + N**2 ).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If JOBZ = 'N' or N <= 1 then LIWORK must be at least 1.
* If JOBZ = 'V' and N > 1 then LIWORK must be at least 3+5*N.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the algorithm failed to converge; i
* off-diagonal elements of E did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param n
* @param d
* @param e
* @param z
* @param ldz
* @param work
* @param lwork
* @param iwork
* @param liwork
* @param info
*
*/
abstract public void dstevd(java.lang.String jobz, int n, double[] d, double[] e, double[] z, int ldz, double[] work, int lwork, int[] iwork, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSTEVD computes all eigenvalues and, optionally, eigenvectors of a
* real symmetric tridiagonal matrix. If eigenvectors are desired, it
* uses a divide and conquer algorithm.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the n diagonal elements of the tridiagonal matrix
* A.
* On exit, if INFO = 0, the eigenvalues in ascending order.
*
* E (input/output) DOUBLE PRECISION array, dimension (N-1)
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix A, stored in elements 1 to N-1 of E.
* On exit, the contents of E are destroyed.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the orthonormal
* eigenvectors of the matrix A, with the i-th column of Z
* holding the eigenvector associated with D(i).
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace/output) DOUBLE PRECISION array,
* dimension (LWORK)
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If JOBZ = 'N' or N <= 1 then LWORK must be at least 1.
* If JOBZ = 'V' and N > 1 then LWORK must be at least
* ( 1 + 4*N + N**2 ).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If JOBZ = 'N' or N <= 1 then LIWORK must be at least 1.
* If JOBZ = 'V' and N > 1 then LIWORK must be at least 3+5*N.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the algorithm failed to converge; i
* off-diagonal elements of E did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param liwork
* @param info
*
*/
abstract public void dstevd(java.lang.String jobz, int n, double[] d, int _d_offset, double[] e, int _e_offset, double[] z, int _z_offset, int ldz, double[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSTEVR computes selected eigenvalues and, optionally, eigenvectors
* of a real symmetric tridiagonal matrix T. Eigenvalues and
* eigenvectors can be selected by specifying either a range of values
* or a range of indices for the desired eigenvalues.
*
* Whenever possible, DSTEVR calls DSTEMR to compute the
* eigenspectrum using Relatively Robust Representations. DSTEMR
* computes eigenvalues by the dqds algorithm, while orthogonal
* eigenvectors are computed from various "good" L D L^T representations
* (also known as Relatively Robust Representations). Gram-Schmidt
* orthogonalization is avoided as far as possible. More specifically,
* the various steps of the algorithm are as follows. For the i-th
* unreduced block of T,
* (a) Compute T - sigma_i = L_i D_i L_i^T, such that L_i D_i L_i^T
* is a relatively robust representation,
* (b) Compute the eigenvalues, lambda_j, of L_i D_i L_i^T to high
* relative accuracy by the dqds algorithm,
* (c) If there is a cluster of close eigenvalues, "choose" sigma_i
* close to the cluster, and go to step (a),
* (d) Given the approximate eigenvalue lambda_j of L_i D_i L_i^T,
* compute the corresponding eigenvector by forming a
* rank-revealing twisted factorization.
* The desired accuracy of the output can be specified by the input
* parameter ABSTOL.
*
* For more details, see "A new O(n^2) algorithm for the symmetric
* tridiagonal eigenvalue/eigenvector problem", by Inderjit Dhillon,
* Computer Science Division Technical Report No. UCB//CSD-97-971,
* UC Berkeley, May 1997.
*
*
* Note 1 : DSTEVR calls DSTEMR when the full spectrum is requested
* on machines which conform to the ieee-754 floating point standard.
* DSTEVR calls DSTEBZ and DSTEIN on non-ieee machines and
* when partial spectrum requests are made.
*
* Normal execution of DSTEMR may create NaNs and infinities and
* hence may abort due to a floating point exception in environments
* which do not handle NaNs and infinities in the ieee standard default
* manner.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
********** For RANGE = 'V' or 'I' and IU - IL < N - 1, DSTEBZ and
********** DSTEIN are called
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the n diagonal elements of the tridiagonal matrix
* A.
* On exit, D may be multiplied by a constant factor chosen
* to avoid over/underflow in computing the eigenvalues.
*
* E (input/output) DOUBLE PRECISION array, dimension (max(1,N-1))
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix A in elements 1 to N-1 of E.
* On exit, E may be multiplied by a constant factor chosen
* to avoid over/underflow in computing the eigenvalues.
*
* VL (input) DOUBLE PRECISION
* VU (input) DOUBLE PRECISION
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) DOUBLE PRECISION
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less than
* or equal to zero, then EPS*|T| will be used in its place,
* where |T| is the 1-norm of the tridiagonal matrix obtained
* by reducing A to tridiagonal form.
*
* See "Computing Small Singular Values of Bidiagonal Matrices
* with Guaranteed High Relative Accuracy," by Demmel and
* Kahan, LAPACK Working Note #3.
*
* If high relative accuracy is important, set ABSTOL to
* DLAMCH( 'Safe minimum' ). Doing so will guarantee that
* eigenvalues are computed to high relative accuracy when
* possible in future releases. The current code does not
* make any guarantees about high relative accuracy, but
* future releases will. See J. Barlow and J. Demmel,
* "Computing Accurate Eigensystems of Scaled Diagonally
* Dominant Matrices", LAPACK Working Note #7, for a discussion
* of which matrices define their eigenvalues to high relative
* accuracy.
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* The first M elements contain the selected eigenvalues in
* ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, max(1,M) )
* If JOBZ = 'V', then if INFO = 0, the first M columns of Z
* contain the orthonormal eigenvectors of the matrix A
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* ISUPPZ (output) INTEGER array, dimension ( 2*max(1,M) )
* The support of the eigenvectors in Z, i.e., the indices
* indicating the nonzero elements in Z. The i-th eigenvector
* is nonzero only in elements ISUPPZ( 2*i-1 ) through
* ISUPPZ( 2*i ).
********** Implemented only for RANGE = 'A' or 'I' and IU - IL = N - 1
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal (and
* minimal) LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,20*N).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal (and
* minimal) LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK. LIWORK >= max(1,10*N).
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: Internal error
*
* Further Details
* ===============
*
* Based on contributions by
* Inderjit Dhillon, IBM Almaden, USA
* Osni Marques, LBNL/NERSC, USA
* Ken Stanley, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param range
* @param n
* @param d
* @param e
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param z
* @param ldz
* @param isuppz
* @param work
* @param lwork
* @param iwork
* @param liwork
* @param info
*
*/
abstract public void dstevr(java.lang.String jobz, java.lang.String range, int n, double[] d, double[] e, double vl, double vu, int il, int iu, double abstol, org.netlib.util.intW m, double[] w, double[] z, int ldz, int[] isuppz, double[] work, int lwork, int[] iwork, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSTEVR computes selected eigenvalues and, optionally, eigenvectors
* of a real symmetric tridiagonal matrix T. Eigenvalues and
* eigenvectors can be selected by specifying either a range of values
* or a range of indices for the desired eigenvalues.
*
* Whenever possible, DSTEVR calls DSTEMR to compute the
* eigenspectrum using Relatively Robust Representations. DSTEMR
* computes eigenvalues by the dqds algorithm, while orthogonal
* eigenvectors are computed from various "good" L D L^T representations
* (also known as Relatively Robust Representations). Gram-Schmidt
* orthogonalization is avoided as far as possible. More specifically,
* the various steps of the algorithm are as follows. For the i-th
* unreduced block of T,
* (a) Compute T - sigma_i = L_i D_i L_i^T, such that L_i D_i L_i^T
* is a relatively robust representation,
* (b) Compute the eigenvalues, lambda_j, of L_i D_i L_i^T to high
* relative accuracy by the dqds algorithm,
* (c) If there is a cluster of close eigenvalues, "choose" sigma_i
* close to the cluster, and go to step (a),
* (d) Given the approximate eigenvalue lambda_j of L_i D_i L_i^T,
* compute the corresponding eigenvector by forming a
* rank-revealing twisted factorization.
* The desired accuracy of the output can be specified by the input
* parameter ABSTOL.
*
* For more details, see "A new O(n^2) algorithm for the symmetric
* tridiagonal eigenvalue/eigenvector problem", by Inderjit Dhillon,
* Computer Science Division Technical Report No. UCB//CSD-97-971,
* UC Berkeley, May 1997.
*
*
* Note 1 : DSTEVR calls DSTEMR when the full spectrum is requested
* on machines which conform to the ieee-754 floating point standard.
* DSTEVR calls DSTEBZ and DSTEIN on non-ieee machines and
* when partial spectrum requests are made.
*
* Normal execution of DSTEMR may create NaNs and infinities and
* hence may abort due to a floating point exception in environments
* which do not handle NaNs and infinities in the ieee standard default
* manner.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
********** For RANGE = 'V' or 'I' and IU - IL < N - 1, DSTEBZ and
********** DSTEIN are called
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the n diagonal elements of the tridiagonal matrix
* A.
* On exit, D may be multiplied by a constant factor chosen
* to avoid over/underflow in computing the eigenvalues.
*
* E (input/output) DOUBLE PRECISION array, dimension (max(1,N-1))
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix A in elements 1 to N-1 of E.
* On exit, E may be multiplied by a constant factor chosen
* to avoid over/underflow in computing the eigenvalues.
*
* VL (input) DOUBLE PRECISION
* VU (input) DOUBLE PRECISION
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) DOUBLE PRECISION
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less than
* or equal to zero, then EPS*|T| will be used in its place,
* where |T| is the 1-norm of the tridiagonal matrix obtained
* by reducing A to tridiagonal form.
*
* See "Computing Small Singular Values of Bidiagonal Matrices
* with Guaranteed High Relative Accuracy," by Demmel and
* Kahan, LAPACK Working Note #3.
*
* If high relative accuracy is important, set ABSTOL to
* DLAMCH( 'Safe minimum' ). Doing so will guarantee that
* eigenvalues are computed to high relative accuracy when
* possible in future releases. The current code does not
* make any guarantees about high relative accuracy, but
* future releases will. See J. Barlow and J. Demmel,
* "Computing Accurate Eigensystems of Scaled Diagonally
* Dominant Matrices", LAPACK Working Note #7, for a discussion
* of which matrices define their eigenvalues to high relative
* accuracy.
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* The first M elements contain the selected eigenvalues in
* ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, max(1,M) )
* If JOBZ = 'V', then if INFO = 0, the first M columns of Z
* contain the orthonormal eigenvectors of the matrix A
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* ISUPPZ (output) INTEGER array, dimension ( 2*max(1,M) )
* The support of the eigenvectors in Z, i.e., the indices
* indicating the nonzero elements in Z. The i-th eigenvector
* is nonzero only in elements ISUPPZ( 2*i-1 ) through
* ISUPPZ( 2*i ).
********** Implemented only for RANGE = 'A' or 'I' and IU - IL = N - 1
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal (and
* minimal) LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,20*N).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal (and
* minimal) LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK. LIWORK >= max(1,10*N).
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: Internal error
*
* Further Details
* ===============
*
* Based on contributions by
* Inderjit Dhillon, IBM Almaden, USA
* Osni Marques, LBNL/NERSC, USA
* Ken Stanley, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param range
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param isuppz
* @param _isuppz_offset
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param liwork
* @param info
*
*/
abstract public void dstevr(java.lang.String jobz, java.lang.String range, int n, double[] d, int _d_offset, double[] e, int _e_offset, double vl, double vu, int il, int iu, double abstol, org.netlib.util.intW m, double[] w, int _w_offset, double[] z, int _z_offset, int ldz, int[] isuppz, int _isuppz_offset, double[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSTEVX computes selected eigenvalues and, optionally, eigenvectors
* of a real symmetric tridiagonal matrix A. Eigenvalues and
* eigenvectors can be selected by specifying either a range of values
* or a range of indices for the desired eigenvalues.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the n diagonal elements of the tridiagonal matrix
* A.
* On exit, D may be multiplied by a constant factor chosen
* to avoid over/underflow in computing the eigenvalues.
*
* E (input/output) DOUBLE PRECISION array, dimension (max(1,N-1))
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix A in elements 1 to N-1 of E.
* On exit, E may be multiplied by a constant factor chosen
* to avoid over/underflow in computing the eigenvalues.
*
* VL (input) DOUBLE PRECISION
* VU (input) DOUBLE PRECISION
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) DOUBLE PRECISION
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less
* than or equal to zero, then EPS*|T| will be used in
* its place, where |T| is the 1-norm of the tridiagonal
* matrix.
*
* Eigenvalues will be computed most accurately when ABSTOL is
* set to twice the underflow threshold 2*DLAMCH('S'), not zero.
* If this routine returns with INFO>0, indicating that some
* eigenvectors did not converge, try setting ABSTOL to
* 2*DLAMCH('S').
*
* See "Computing Small Singular Values of Bidiagonal Matrices
* with Guaranteed High Relative Accuracy," by Demmel and
* Kahan, LAPACK Working Note #3.
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* The first M elements contain the selected eigenvalues in
* ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, max(1,M) )
* If JOBZ = 'V', then if INFO = 0, the first M columns of Z
* contain the orthonormal eigenvectors of the matrix A
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* If an eigenvector fails to converge (INFO > 0), then that
* column of Z contains the latest approximation to the
* eigenvector, and the index of the eigenvector is returned
* in IFAIL. If JOBZ = 'N', then Z is not referenced.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (5*N)
*
* IWORK (workspace) INTEGER array, dimension (5*N)
*
* IFAIL (output) INTEGER array, dimension (N)
* If JOBZ = 'V', then if INFO = 0, the first M elements of
* IFAIL are zero. If INFO > 0, then IFAIL contains the
* indices of the eigenvectors that failed to converge.
* If JOBZ = 'N', then IFAIL is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, then i eigenvectors failed to converge.
* Their indices are stored in array IFAIL.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param range
* @param n
* @param d
* @param e
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param z
* @param ldz
* @param work
* @param iwork
* @param ifail
* @param info
*
*/
abstract public void dstevx(java.lang.String jobz, java.lang.String range, int n, double[] d, double[] e, double vl, double vu, int il, int iu, double abstol, org.netlib.util.intW m, double[] w, double[] z, int ldz, double[] work, int[] iwork, int[] ifail, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSTEVX computes selected eigenvalues and, optionally, eigenvectors
* of a real symmetric tridiagonal matrix A. Eigenvalues and
* eigenvectors can be selected by specifying either a range of values
* or a range of indices for the desired eigenvalues.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) DOUBLE PRECISION array, dimension (N)
* On entry, the n diagonal elements of the tridiagonal matrix
* A.
* On exit, D may be multiplied by a constant factor chosen
* to avoid over/underflow in computing the eigenvalues.
*
* E (input/output) DOUBLE PRECISION array, dimension (max(1,N-1))
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix A in elements 1 to N-1 of E.
* On exit, E may be multiplied by a constant factor chosen
* to avoid over/underflow in computing the eigenvalues.
*
* VL (input) DOUBLE PRECISION
* VU (input) DOUBLE PRECISION
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) DOUBLE PRECISION
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less
* than or equal to zero, then EPS*|T| will be used in
* its place, where |T| is the 1-norm of the tridiagonal
* matrix.
*
* Eigenvalues will be computed most accurately when ABSTOL is
* set to twice the underflow threshold 2*DLAMCH('S'), not zero.
* If this routine returns with INFO>0, indicating that some
* eigenvectors did not converge, try setting ABSTOL to
* 2*DLAMCH('S').
*
* See "Computing Small Singular Values of Bidiagonal Matrices
* with Guaranteed High Relative Accuracy," by Demmel and
* Kahan, LAPACK Working Note #3.
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* The first M elements contain the selected eigenvalues in
* ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, max(1,M) )
* If JOBZ = 'V', then if INFO = 0, the first M columns of Z
* contain the orthonormal eigenvectors of the matrix A
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* If an eigenvector fails to converge (INFO > 0), then that
* column of Z contains the latest approximation to the
* eigenvector, and the index of the eigenvector is returned
* in IFAIL. If JOBZ = 'N', then Z is not referenced.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (5*N)
*
* IWORK (workspace) INTEGER array, dimension (5*N)
*
* IFAIL (output) INTEGER array, dimension (N)
* If JOBZ = 'V', then if INFO = 0, the first M elements of
* IFAIL are zero. If INFO > 0, then IFAIL contains the
* indices of the eigenvectors that failed to converge.
* If JOBZ = 'N', then IFAIL is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, then i eigenvectors failed to converge.
* Their indices are stored in array IFAIL.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param range
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param ifail
* @param _ifail_offset
* @param info
*
*/
abstract public void dstevx(java.lang.String jobz, java.lang.String range, int n, double[] d, int _d_offset, double[] e, int _e_offset, double vl, double vu, int il, int iu, double abstol, org.netlib.util.intW m, double[] w, int _w_offset, double[] z, int _z_offset, int ldz, double[] work, int _work_offset, int[] iwork, int _iwork_offset, int[] ifail, int _ifail_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYCON estimates the reciprocal of the condition number (in the
* 1-norm) of a real symmetric matrix A using the factorization
* A = U*D*U**T or A = L*D*L**T computed by DSYTRF.
*
* An estimate is obtained for norm(inv(A)), and the reciprocal of the
* condition number is computed as RCOND = 1 / (ANORM * norm(inv(A))).
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the details of the factorization are stored
* as an upper or lower triangular matrix.
* = 'U': Upper triangular, form is A = U*D*U**T;
* = 'L': Lower triangular, form is A = L*D*L**T.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The block diagonal matrix D and the multipliers used to
* obtain the factor U or L as computed by DSYTRF.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (input) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D
* as determined by DSYTRF.
*
* ANORM (input) DOUBLE PRECISION
* The 1-norm of the original matrix A.
*
* RCOND (output) DOUBLE PRECISION
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(ANORM * AINVNM), where AINVNM is an
* estimate of the 1-norm of inv(A) computed in this routine.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (2*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param lda
* @param ipiv
* @param anorm
* @param rcond
* @param work
* @param iwork
* @param info
*
*/
abstract public void dsycon(java.lang.String uplo, int n, double[] a, int lda, int[] ipiv, double anorm, org.netlib.util.doubleW rcond, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYCON estimates the reciprocal of the condition number (in the
* 1-norm) of a real symmetric matrix A using the factorization
* A = U*D*U**T or A = L*D*L**T computed by DSYTRF.
*
* An estimate is obtained for norm(inv(A)), and the reciprocal of the
* condition number is computed as RCOND = 1 / (ANORM * norm(inv(A))).
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the details of the factorization are stored
* as an upper or lower triangular matrix.
* = 'U': Upper triangular, form is A = U*D*U**T;
* = 'L': Lower triangular, form is A = L*D*L**T.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The block diagonal matrix D and the multipliers used to
* obtain the factor U or L as computed by DSYTRF.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (input) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D
* as determined by DSYTRF.
*
* ANORM (input) DOUBLE PRECISION
* The 1-norm of the original matrix A.
*
* RCOND (output) DOUBLE PRECISION
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(ANORM * AINVNM), where AINVNM is an
* estimate of the 1-norm of inv(A) computed in this routine.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (2*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param ipiv
* @param _ipiv_offset
* @param anorm
* @param rcond
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dsycon(java.lang.String uplo, int n, double[] a, int _a_offset, int lda, int[] ipiv, int _ipiv_offset, double anorm, org.netlib.util.doubleW rcond, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYEV computes all eigenvalues and, optionally, eigenvectors of a
* real symmetric matrix A.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the symmetric matrix A. If UPLO = 'U', the
* leading N-by-N upper triangular part of A contains the
* upper triangular part of the matrix A. If UPLO = 'L',
* the leading N-by-N lower triangular part of A contains
* the lower triangular part of the matrix A.
* On exit, if JOBZ = 'V', then if INFO = 0, A contains the
* orthonormal eigenvectors of the matrix A.
* If JOBZ = 'N', then on exit the lower triangle (if UPLO='L')
* or the upper triangle (if UPLO='U') of A, including the
* diagonal, is destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* W (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of the array WORK. LWORK >= max(1,3*N-1).
* For optimal efficiency, LWORK >= (NB+2)*N,
* where NB is the blocksize for DSYTRD returned by ILAENV.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the algorithm failed to converge; i
* off-diagonal elements of an intermediate tridiagonal
* form did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param uplo
* @param n
* @param a
* @param lda
* @param w
* @param work
* @param lwork
* @param info
*
*/
abstract public void dsyev(java.lang.String jobz, java.lang.String uplo, int n, double[] a, int lda, double[] w, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYEV computes all eigenvalues and, optionally, eigenvectors of a
* real symmetric matrix A.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the symmetric matrix A. If UPLO = 'U', the
* leading N-by-N upper triangular part of A contains the
* upper triangular part of the matrix A. If UPLO = 'L',
* the leading N-by-N lower triangular part of A contains
* the lower triangular part of the matrix A.
* On exit, if JOBZ = 'V', then if INFO = 0, A contains the
* orthonormal eigenvectors of the matrix A.
* If JOBZ = 'N', then on exit the lower triangle (if UPLO='L')
* or the upper triangle (if UPLO='U') of A, including the
* diagonal, is destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* W (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of the array WORK. LWORK >= max(1,3*N-1).
* For optimal efficiency, LWORK >= (NB+2)*N,
* where NB is the blocksize for DSYTRD returned by ILAENV.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the algorithm failed to converge; i
* off-diagonal elements of an intermediate tridiagonal
* form did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param w
* @param _w_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dsyev(java.lang.String jobz, java.lang.String uplo, int n, double[] a, int _a_offset, int lda, double[] w, int _w_offset, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYEVD computes all eigenvalues and, optionally, eigenvectors of a
* real symmetric matrix A. If eigenvectors are desired, it uses a
* divide and conquer algorithm.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Because of large use of BLAS of level 3, DSYEVD needs N**2 more
* workspace than DSYEVX.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the symmetric matrix A. If UPLO = 'U', the
* leading N-by-N upper triangular part of A contains the
* upper triangular part of the matrix A. If UPLO = 'L',
* the leading N-by-N lower triangular part of A contains
* the lower triangular part of the matrix A.
* On exit, if JOBZ = 'V', then if INFO = 0, A contains the
* orthonormal eigenvectors of the matrix A.
* If JOBZ = 'N', then on exit the lower triangle (if UPLO='L')
* or the upper triangle (if UPLO='U') of A, including the
* diagonal, is destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* W (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* WORK (workspace/output) DOUBLE PRECISION array,
* dimension (LWORK)
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If N <= 1, LWORK must be at least 1.
* If JOBZ = 'N' and N > 1, LWORK must be at least 2*N+1.
* If JOBZ = 'V' and N > 1, LWORK must be at least
* 1 + 6*N + 2*N**2.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If N <= 1, LIWORK must be at least 1.
* If JOBZ = 'N' and N > 1, LIWORK must be at least 1.
* If JOBZ = 'V' and N > 1, LIWORK must be at least 3 + 5*N.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i and JOBZ = 'N', then the algorithm failed
* to converge; i off-diagonal elements of an intermediate
* tridiagonal form did not converge to zero;
* if INFO = i and JOBZ = 'V', then the algorithm failed
* to compute an eigenvalue while working on the submatrix
* lying in rows and columns INFO/(N+1) through
* mod(INFO,N+1).
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
* Modified by Francoise Tisseur, University of Tennessee.
*
* Modified description of INFO. Sven, 16 Feb 05.
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param uplo
* @param n
* @param a
* @param lda
* @param w
* @param work
* @param lwork
* @param iwork
* @param liwork
* @param info
*
*/
abstract public void dsyevd(java.lang.String jobz, java.lang.String uplo, int n, double[] a, int lda, double[] w, double[] work, int lwork, int[] iwork, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYEVD computes all eigenvalues and, optionally, eigenvectors of a
* real symmetric matrix A. If eigenvectors are desired, it uses a
* divide and conquer algorithm.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Because of large use of BLAS of level 3, DSYEVD needs N**2 more
* workspace than DSYEVX.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the symmetric matrix A. If UPLO = 'U', the
* leading N-by-N upper triangular part of A contains the
* upper triangular part of the matrix A. If UPLO = 'L',
* the leading N-by-N lower triangular part of A contains
* the lower triangular part of the matrix A.
* On exit, if JOBZ = 'V', then if INFO = 0, A contains the
* orthonormal eigenvectors of the matrix A.
* If JOBZ = 'N', then on exit the lower triangle (if UPLO='L')
* or the upper triangle (if UPLO='U') of A, including the
* diagonal, is destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* W (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* WORK (workspace/output) DOUBLE PRECISION array,
* dimension (LWORK)
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If N <= 1, LWORK must be at least 1.
* If JOBZ = 'N' and N > 1, LWORK must be at least 2*N+1.
* If JOBZ = 'V' and N > 1, LWORK must be at least
* 1 + 6*N + 2*N**2.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If N <= 1, LIWORK must be at least 1.
* If JOBZ = 'N' and N > 1, LIWORK must be at least 1.
* If JOBZ = 'V' and N > 1, LIWORK must be at least 3 + 5*N.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i and JOBZ = 'N', then the algorithm failed
* to converge; i off-diagonal elements of an intermediate
* tridiagonal form did not converge to zero;
* if INFO = i and JOBZ = 'V', then the algorithm failed
* to compute an eigenvalue while working on the submatrix
* lying in rows and columns INFO/(N+1) through
* mod(INFO,N+1).
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
* Modified by Francoise Tisseur, University of Tennessee.
*
* Modified description of INFO. Sven, 16 Feb 05.
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param w
* @param _w_offset
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param liwork
* @param info
*
*/
abstract public void dsyevd(java.lang.String jobz, java.lang.String uplo, int n, double[] a, int _a_offset, int lda, double[] w, int _w_offset, double[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYEVR computes selected eigenvalues and, optionally, eigenvectors
* of a real symmetric matrix A. Eigenvalues and eigenvectors can be
* selected by specifying either a range of values or a range of
* indices for the desired eigenvalues.
*
* DSYEVR first reduces the matrix A to tridiagonal form T with a call
* to DSYTRD. Then, whenever possible, DSYEVR calls DSTEMR to compute
* the eigenspectrum using Relatively Robust Representations. DSTEMR
* computes eigenvalues by the dqds algorithm, while orthogonal
* eigenvectors are computed from various "good" L D L^T representations
* (also known as Relatively Robust Representations). Gram-Schmidt
* orthogonalization is avoided as far as possible. More specifically,
* the various steps of the algorithm are as follows.
*
* For each unreduced block (submatrix) of T,
* (a) Compute T - sigma I = L D L^T, so that L and D
* define all the wanted eigenvalues to high relative accuracy.
* This means that small relative changes in the entries of D and
* cause only small relative changes in the eigenvalues and
* eigenvectors. The standard (unfactored) representation of the
* tridiagonal matrix T does not have this property in general.
* (b) Compute the eigenvalues to suitable accuracy.
* If the eigenvectors are desired, the algorithm attains full
* accuracy of the computed eigenvalues only right before
* the corresponding vectors have to be computed, see steps c) an
* (c) For each cluster of close eigenvalues, select a new
* shift close to the cluster, find a new factorization, and refi
* the shifted eigenvalues to suitable accuracy.
* (d) For each eigenvalue with a large enough relative separation co
* the corresponding eigenvector by forming a rank revealing twis
* factorization. Go back to (c) for any clusters that remain.
*
* The desired accuracy of the output can be specified by the input
* parameter ABSTOL.
*
* For more details, see DSTEMR's documentation and:
* - Inderjit S. Dhillon and Beresford N. Parlett: "Multiple representat
* to compute orthogonal eigenvectors of symmetric tridiagonal matrice
* Linear Algebra and its Applications, 387(1), pp. 1-28, August 2004.
* - Inderjit Dhillon and Beresford Parlett: "Orthogonal Eigenvectors an
* Relative Gaps," SIAM Journal on Matrix Analysis and Applications, V
* 2004. Also LAPACK Working Note 154.
* - Inderjit Dhillon: "A new O(n^2) algorithm for the symmetric
* tridiagonal eigenvalue/eigenvector problem",
* Computer Science Division Technical Report No. UCB/CSD-97-971,
* UC Berkeley, May 1997.
*
*
* Note 1 : DSYEVR calls DSTEMR when the full spectrum is requested
* on machines which conform to the ieee-754 floating point standard.
* DSYEVR calls DSTEBZ and SSTEIN on non-ieee machines and
* when partial spectrum requests are made.
*
* Normal execution of DSTEMR may create NaNs and infinities and
* hence may abort due to a floating point exception in environments
* which do not handle NaNs and infinities in the ieee standard default
* manner.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
********** For RANGE = 'V' or 'I' and IU - IL < N - 1, DSTEBZ and
********** DSTEIN are called
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the symmetric matrix A. If UPLO = 'U', the
* leading N-by-N upper triangular part of A contains the
* upper triangular part of the matrix A. If UPLO = 'L',
* the leading N-by-N lower triangular part of A contains
* the lower triangular part of the matrix A.
* On exit, the lower triangle (if UPLO='L') or the upper
* triangle (if UPLO='U') of A, including the diagonal, is
* destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* VL (input) DOUBLE PRECISION
* VU (input) DOUBLE PRECISION
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) DOUBLE PRECISION
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less than
* or equal to zero, then EPS*|T| will be used in its place,
* where |T| is the 1-norm of the tridiagonal matrix obtained
* by reducing A to tridiagonal form.
*
* See "Computing Small Singular Values of Bidiagonal Matrices
* with Guaranteed High Relative Accuracy," by Demmel and
* Kahan, LAPACK Working Note #3.
*
* If high relative accuracy is important, set ABSTOL to
* DLAMCH( 'Safe minimum' ). Doing so will guarantee that
* eigenvalues are computed to high relative accuracy when
* possible in future releases. The current code does not
* make any guarantees about high relative accuracy, but
* future releases will. See J. Barlow and J. Demmel,
* "Computing Accurate Eigensystems of Scaled Diagonally
* Dominant Matrices", LAPACK Working Note #7, for a discussion
* of which matrices define their eigenvalues to high relative
* accuracy.
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* The first M elements contain the selected eigenvalues in
* ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, max(1,M))
* If JOBZ = 'V', then if INFO = 0, the first M columns of Z
* contain the orthonormal eigenvectors of the matrix A
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* If JOBZ = 'N', then Z is not referenced.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
* Supplying N columns is always safe.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* ISUPPZ (output) INTEGER array, dimension ( 2*max(1,M) )
* The support of the eigenvectors in Z, i.e., the indices
* indicating the nonzero elements in Z. The i-th eigenvector
* is nonzero only in elements ISUPPZ( 2*i-1 ) through
* ISUPPZ( 2*i ).
********** Implemented only for RANGE = 'A' or 'I' and IU - IL = N - 1
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,26*N).
* For optimal efficiency, LWORK >= (NB+6)*N,
* where NB is the max of the blocksize for DSYTRD and DORMTR
* returned by ILAENV.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal LWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK. LIWORK >= max(1,10*N).
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal size of the IWORK array,
* returns this value as the first entry of the IWORK array, and
* no error message related to LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: Internal error
*
* Further Details
* ===============
*
* Based on contributions by
* Inderjit Dhillon, IBM Almaden, USA
* Osni Marques, LBNL/NERSC, USA
* Ken Stanley, Computer Science Division, University of
* California at Berkeley, USA
* Jason Riedy, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param range
* @param uplo
* @param n
* @param a
* @param lda
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param z
* @param ldz
* @param isuppz
* @param work
* @param lwork
* @param iwork
* @param liwork
* @param info
*
*/
abstract public void dsyevr(java.lang.String jobz, java.lang.String range, java.lang.String uplo, int n, double[] a, int lda, double vl, double vu, int il, int iu, double abstol, org.netlib.util.intW m, double[] w, double[] z, int ldz, int[] isuppz, double[] work, int lwork, int[] iwork, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYEVR computes selected eigenvalues and, optionally, eigenvectors
* of a real symmetric matrix A. Eigenvalues and eigenvectors can be
* selected by specifying either a range of values or a range of
* indices for the desired eigenvalues.
*
* DSYEVR first reduces the matrix A to tridiagonal form T with a call
* to DSYTRD. Then, whenever possible, DSYEVR calls DSTEMR to compute
* the eigenspectrum using Relatively Robust Representations. DSTEMR
* computes eigenvalues by the dqds algorithm, while orthogonal
* eigenvectors are computed from various "good" L D L^T representations
* (also known as Relatively Robust Representations). Gram-Schmidt
* orthogonalization is avoided as far as possible. More specifically,
* the various steps of the algorithm are as follows.
*
* For each unreduced block (submatrix) of T,
* (a) Compute T - sigma I = L D L^T, so that L and D
* define all the wanted eigenvalues to high relative accuracy.
* This means that small relative changes in the entries of D and
* cause only small relative changes in the eigenvalues and
* eigenvectors. The standard (unfactored) representation of the
* tridiagonal matrix T does not have this property in general.
* (b) Compute the eigenvalues to suitable accuracy.
* If the eigenvectors are desired, the algorithm attains full
* accuracy of the computed eigenvalues only right before
* the corresponding vectors have to be computed, see steps c) an
* (c) For each cluster of close eigenvalues, select a new
* shift close to the cluster, find a new factorization, and refi
* the shifted eigenvalues to suitable accuracy.
* (d) For each eigenvalue with a large enough relative separation co
* the corresponding eigenvector by forming a rank revealing twis
* factorization. Go back to (c) for any clusters that remain.
*
* The desired accuracy of the output can be specified by the input
* parameter ABSTOL.
*
* For more details, see DSTEMR's documentation and:
* - Inderjit S. Dhillon and Beresford N. Parlett: "Multiple representat
* to compute orthogonal eigenvectors of symmetric tridiagonal matrice
* Linear Algebra and its Applications, 387(1), pp. 1-28, August 2004.
* - Inderjit Dhillon and Beresford Parlett: "Orthogonal Eigenvectors an
* Relative Gaps," SIAM Journal on Matrix Analysis and Applications, V
* 2004. Also LAPACK Working Note 154.
* - Inderjit Dhillon: "A new O(n^2) algorithm for the symmetric
* tridiagonal eigenvalue/eigenvector problem",
* Computer Science Division Technical Report No. UCB/CSD-97-971,
* UC Berkeley, May 1997.
*
*
* Note 1 : DSYEVR calls DSTEMR when the full spectrum is requested
* on machines which conform to the ieee-754 floating point standard.
* DSYEVR calls DSTEBZ and SSTEIN on non-ieee machines and
* when partial spectrum requests are made.
*
* Normal execution of DSTEMR may create NaNs and infinities and
* hence may abort due to a floating point exception in environments
* which do not handle NaNs and infinities in the ieee standard default
* manner.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
********** For RANGE = 'V' or 'I' and IU - IL < N - 1, DSTEBZ and
********** DSTEIN are called
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the symmetric matrix A. If UPLO = 'U', the
* leading N-by-N upper triangular part of A contains the
* upper triangular part of the matrix A. If UPLO = 'L',
* the leading N-by-N lower triangular part of A contains
* the lower triangular part of the matrix A.
* On exit, the lower triangle (if UPLO='L') or the upper
* triangle (if UPLO='U') of A, including the diagonal, is
* destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* VL (input) DOUBLE PRECISION
* VU (input) DOUBLE PRECISION
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) DOUBLE PRECISION
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less than
* or equal to zero, then EPS*|T| will be used in its place,
* where |T| is the 1-norm of the tridiagonal matrix obtained
* by reducing A to tridiagonal form.
*
* See "Computing Small Singular Values of Bidiagonal Matrices
* with Guaranteed High Relative Accuracy," by Demmel and
* Kahan, LAPACK Working Note #3.
*
* If high relative accuracy is important, set ABSTOL to
* DLAMCH( 'Safe minimum' ). Doing so will guarantee that
* eigenvalues are computed to high relative accuracy when
* possible in future releases. The current code does not
* make any guarantees about high relative accuracy, but
* future releases will. See J. Barlow and J. Demmel,
* "Computing Accurate Eigensystems of Scaled Diagonally
* Dominant Matrices", LAPACK Working Note #7, for a discussion
* of which matrices define their eigenvalues to high relative
* accuracy.
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* The first M elements contain the selected eigenvalues in
* ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, max(1,M))
* If JOBZ = 'V', then if INFO = 0, the first M columns of Z
* contain the orthonormal eigenvectors of the matrix A
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* If JOBZ = 'N', then Z is not referenced.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
* Supplying N columns is always safe.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* ISUPPZ (output) INTEGER array, dimension ( 2*max(1,M) )
* The support of the eigenvectors in Z, i.e., the indices
* indicating the nonzero elements in Z. The i-th eigenvector
* is nonzero only in elements ISUPPZ( 2*i-1 ) through
* ISUPPZ( 2*i ).
********** Implemented only for RANGE = 'A' or 'I' and IU - IL = N - 1
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,26*N).
* For optimal efficiency, LWORK >= (NB+6)*N,
* where NB is the max of the blocksize for DSYTRD and DORMTR
* returned by ILAENV.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal LWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK. LIWORK >= max(1,10*N).
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal size of the IWORK array,
* returns this value as the first entry of the IWORK array, and
* no error message related to LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: Internal error
*
* Further Details
* ===============
*
* Based on contributions by
* Inderjit Dhillon, IBM Almaden, USA
* Osni Marques, LBNL/NERSC, USA
* Ken Stanley, Computer Science Division, University of
* California at Berkeley, USA
* Jason Riedy, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param range
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param isuppz
* @param _isuppz_offset
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param liwork
* @param info
*
*/
abstract public void dsyevr(java.lang.String jobz, java.lang.String range, java.lang.String uplo, int n, double[] a, int _a_offset, int lda, double vl, double vu, int il, int iu, double abstol, org.netlib.util.intW m, double[] w, int _w_offset, double[] z, int _z_offset, int ldz, int[] isuppz, int _isuppz_offset, double[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYEVX computes selected eigenvalues and, optionally, eigenvectors
* of a real symmetric matrix A. Eigenvalues and eigenvectors can be
* selected by specifying either a range of values or a range of indices
* for the desired eigenvalues.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the symmetric matrix A. If UPLO = 'U', the
* leading N-by-N upper triangular part of A contains the
* upper triangular part of the matrix A. If UPLO = 'L',
* the leading N-by-N lower triangular part of A contains
* the lower triangular part of the matrix A.
* On exit, the lower triangle (if UPLO='L') or the upper
* triangle (if UPLO='U') of A, including the diagonal, is
* destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* VL (input) DOUBLE PRECISION
* VU (input) DOUBLE PRECISION
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) DOUBLE PRECISION
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less than
* or equal to zero, then EPS*|T| will be used in its place,
* where |T| is the 1-norm of the tridiagonal matrix obtained
* by reducing A to tridiagonal form.
*
* Eigenvalues will be computed most accurately when ABSTOL is
* set to twice the underflow threshold 2*DLAMCH('S'), not zero.
* If this routine returns with INFO>0, indicating that some
* eigenvectors did not converge, try setting ABSTOL to
* 2*DLAMCH('S').
*
* See "Computing Small Singular Values of Bidiagonal Matrices
* with Guaranteed High Relative Accuracy," by Demmel and
* Kahan, LAPACK Working Note #3.
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* On normal exit, the first M elements contain the selected
* eigenvalues in ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, max(1,M))
* If JOBZ = 'V', then if INFO = 0, the first M columns of Z
* contain the orthonormal eigenvectors of the matrix A
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* If an eigenvector fails to converge, then that column of Z
* contains the latest approximation to the eigenvector, and the
* index of the eigenvector is returned in IFAIL.
* If JOBZ = 'N', then Z is not referenced.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of the array WORK. LWORK >= 1, when N <= 1;
* otherwise 8*N.
* For optimal efficiency, LWORK >= (NB+3)*N,
* where NB is the max of the blocksize for DSYTRD and DORMTR
* returned by ILAENV.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (5*N)
*
* IFAIL (output) INTEGER array, dimension (N)
* If JOBZ = 'V', then if INFO = 0, the first M elements of
* IFAIL are zero. If INFO > 0, then IFAIL contains the
* indices of the eigenvectors that failed to converge.
* If JOBZ = 'N', then IFAIL is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, then i eigenvectors failed to converge.
* Their indices are stored in array IFAIL.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param range
* @param uplo
* @param n
* @param a
* @param lda
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param z
* @param ldz
* @param work
* @param lwork
* @param iwork
* @param ifail
* @param info
*
*/
abstract public void dsyevx(java.lang.String jobz, java.lang.String range, java.lang.String uplo, int n, double[] a, int lda, double vl, double vu, int il, int iu, double abstol, org.netlib.util.intW m, double[] w, double[] z, int ldz, double[] work, int lwork, int[] iwork, int[] ifail, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYEVX computes selected eigenvalues and, optionally, eigenvectors
* of a real symmetric matrix A. Eigenvalues and eigenvectors can be
* selected by specifying either a range of values or a range of indices
* for the desired eigenvalues.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the symmetric matrix A. If UPLO = 'U', the
* leading N-by-N upper triangular part of A contains the
* upper triangular part of the matrix A. If UPLO = 'L',
* the leading N-by-N lower triangular part of A contains
* the lower triangular part of the matrix A.
* On exit, the lower triangle (if UPLO='L') or the upper
* triangle (if UPLO='U') of A, including the diagonal, is
* destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* VL (input) DOUBLE PRECISION
* VU (input) DOUBLE PRECISION
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) DOUBLE PRECISION
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less than
* or equal to zero, then EPS*|T| will be used in its place,
* where |T| is the 1-norm of the tridiagonal matrix obtained
* by reducing A to tridiagonal form.
*
* Eigenvalues will be computed most accurately when ABSTOL is
* set to twice the underflow threshold 2*DLAMCH('S'), not zero.
* If this routine returns with INFO>0, indicating that some
* eigenvectors did not converge, try setting ABSTOL to
* 2*DLAMCH('S').
*
* See "Computing Small Singular Values of Bidiagonal Matrices
* with Guaranteed High Relative Accuracy," by Demmel and
* Kahan, LAPACK Working Note #3.
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* On normal exit, the first M elements contain the selected
* eigenvalues in ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, max(1,M))
* If JOBZ = 'V', then if INFO = 0, the first M columns of Z
* contain the orthonormal eigenvectors of the matrix A
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* If an eigenvector fails to converge, then that column of Z
* contains the latest approximation to the eigenvector, and the
* index of the eigenvector is returned in IFAIL.
* If JOBZ = 'N', then Z is not referenced.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of the array WORK. LWORK >= 1, when N <= 1;
* otherwise 8*N.
* For optimal efficiency, LWORK >= (NB+3)*N,
* where NB is the max of the blocksize for DSYTRD and DORMTR
* returned by ILAENV.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (5*N)
*
* IFAIL (output) INTEGER array, dimension (N)
* If JOBZ = 'V', then if INFO = 0, the first M elements of
* IFAIL are zero. If INFO > 0, then IFAIL contains the
* indices of the eigenvectors that failed to converge.
* If JOBZ = 'N', then IFAIL is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, then i eigenvectors failed to converge.
* Their indices are stored in array IFAIL.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param range
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param ifail
* @param _ifail_offset
* @param info
*
*/
abstract public void dsyevx(java.lang.String jobz, java.lang.String range, java.lang.String uplo, int n, double[] a, int _a_offset, int lda, double vl, double vu, int il, int iu, double abstol, org.netlib.util.intW m, double[] w, int _w_offset, double[] z, int _z_offset, int ldz, double[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int[] ifail, int _ifail_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYGS2 reduces a real symmetric-definite generalized eigenproblem
* to standard form.
*
* If ITYPE = 1, the problem is A*x = lambda*B*x,
* and A is overwritten by inv(U')*A*inv(U) or inv(L)*A*inv(L')
*
* If ITYPE = 2 or 3, the problem is A*B*x = lambda*x or
* B*A*x = lambda*x, and A is overwritten by U*A*U` or L'*A*L.
*
* B must have been previously factorized as U'*U or L*L' by DPOTRF.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* = 1: compute inv(U')*A*inv(U) or inv(L)*A*inv(L');
* = 2 or 3: compute U*A*U' or L'*A*L.
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored, and how B has been factorized.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* n by n upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading n by n lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, if INFO = 0, the transformed matrix, stored in the
* same format as A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input) DOUBLE PRECISION array, dimension (LDB,N)
* The triangular factor from the Cholesky factorization of B,
* as returned by DPOTRF.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param itype
* @param uplo
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param info
*
*/
abstract public void dsygs2(int itype, java.lang.String uplo, int n, double[] a, int lda, double[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYGS2 reduces a real symmetric-definite generalized eigenproblem
* to standard form.
*
* If ITYPE = 1, the problem is A*x = lambda*B*x,
* and A is overwritten by inv(U')*A*inv(U) or inv(L)*A*inv(L')
*
* If ITYPE = 2 or 3, the problem is A*B*x = lambda*x or
* B*A*x = lambda*x, and A is overwritten by U*A*U` or L'*A*L.
*
* B must have been previously factorized as U'*U or L*L' by DPOTRF.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* = 1: compute inv(U')*A*inv(U) or inv(L)*A*inv(L');
* = 2 or 3: compute U*A*U' or L'*A*L.
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored, and how B has been factorized.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* n by n upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading n by n lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, if INFO = 0, the transformed matrix, stored in the
* same format as A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input) DOUBLE PRECISION array, dimension (LDB,N)
* The triangular factor from the Cholesky factorization of B,
* as returned by DPOTRF.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param itype
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void dsygs2(int itype, java.lang.String uplo, int n, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYGST reduces a real symmetric-definite generalized eigenproblem
* to standard form.
*
* If ITYPE = 1, the problem is A*x = lambda*B*x,
* and A is overwritten by inv(U**T)*A*inv(U) or inv(L)*A*inv(L**T)
*
* If ITYPE = 2 or 3, the problem is A*B*x = lambda*x or
* B*A*x = lambda*x, and A is overwritten by U*A*U**T or L**T*A*L.
*
* B must have been previously factorized as U**T*U or L*L**T by DPOTRF.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* = 1: compute inv(U**T)*A*inv(U) or inv(L)*A*inv(L**T);
* = 2 or 3: compute U*A*U**T or L**T*A*L.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored and B is factored as
* U**T*U;
* = 'L': Lower triangle of A is stored and B is factored as
* L*L**T.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* N-by-N upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading N-by-N lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, if INFO = 0, the transformed matrix, stored in the
* same format as A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input) DOUBLE PRECISION array, dimension (LDB,N)
* The triangular factor from the Cholesky factorization of B,
* as returned by DPOTRF.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param itype
* @param uplo
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param info
*
*/
abstract public void dsygst(int itype, java.lang.String uplo, int n, double[] a, int lda, double[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYGST reduces a real symmetric-definite generalized eigenproblem
* to standard form.
*
* If ITYPE = 1, the problem is A*x = lambda*B*x,
* and A is overwritten by inv(U**T)*A*inv(U) or inv(L)*A*inv(L**T)
*
* If ITYPE = 2 or 3, the problem is A*B*x = lambda*x or
* B*A*x = lambda*x, and A is overwritten by U*A*U**T or L**T*A*L.
*
* B must have been previously factorized as U**T*U or L*L**T by DPOTRF.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* = 1: compute inv(U**T)*A*inv(U) or inv(L)*A*inv(L**T);
* = 2 or 3: compute U*A*U**T or L**T*A*L.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored and B is factored as
* U**T*U;
* = 'L': Lower triangle of A is stored and B is factored as
* L*L**T.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* N-by-N upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading N-by-N lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, if INFO = 0, the transformed matrix, stored in the
* same format as A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input) DOUBLE PRECISION array, dimension (LDB,N)
* The triangular factor from the Cholesky factorization of B,
* as returned by DPOTRF.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param itype
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void dsygst(int itype, java.lang.String uplo, int n, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYGV computes all the eigenvalues, and optionally, the eigenvectors
* of a real generalized symmetric-definite eigenproblem, of the form
* A*x=(lambda)*B*x, A*Bx=(lambda)*x, or B*A*x=(lambda)*x.
* Here A and B are assumed to be symmetric and B is also
* positive definite.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* Specifies the problem type to be solved:
* = 1: A*x = (lambda)*B*x
* = 2: A*B*x = (lambda)*x
* = 3: B*A*x = (lambda)*x
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangles of A and B are stored;
* = 'L': Lower triangles of A and B are stored.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the symmetric matrix A. If UPLO = 'U', the
* leading N-by-N upper triangular part of A contains the
* upper triangular part of the matrix A. If UPLO = 'L',
* the leading N-by-N lower triangular part of A contains
* the lower triangular part of the matrix A.
*
* On exit, if JOBZ = 'V', then if INFO = 0, A contains the
* matrix Z of eigenvectors. The eigenvectors are normalized
* as follows:
* if ITYPE = 1 or 2, Z**T*B*Z = I;
* if ITYPE = 3, Z**T*inv(B)*Z = I.
* If JOBZ = 'N', then on exit the upper triangle (if UPLO='U')
* or the lower triangle (if UPLO='L') of A, including the
* diagonal, is destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB, N)
* On entry, the symmetric positive definite matrix B.
* If UPLO = 'U', the leading N-by-N upper triangular part of B
* contains the upper triangular part of the matrix B.
* If UPLO = 'L', the leading N-by-N lower triangular part of B
* contains the lower triangular part of the matrix B.
*
* On exit, if INFO <= N, the part of B containing the matrix is
* overwritten by the triangular factor U or L from the Cholesky
* factorization B = U**T*U or B = L*L**T.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* W (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of the array WORK. LWORK >= max(1,3*N-1).
* For optimal efficiency, LWORK >= (NB+2)*N,
* where NB is the blocksize for DSYTRD returned by ILAENV.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: DPOTRF or DSYEV returned an error code:
* <= N: if INFO = i, DSYEV failed to converge;
* i off-diagonal elements of an intermediate
* tridiagonal form did not converge to zero;
* > N: if INFO = N + i, for 1 <= i <= N, then the leading
* minor of order i of B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param itype
* @param jobz
* @param uplo
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param w
* @param work
* @param lwork
* @param info
*
*/
abstract public void dsygv(int itype, java.lang.String jobz, java.lang.String uplo, int n, double[] a, int lda, double[] b, int ldb, double[] w, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYGV computes all the eigenvalues, and optionally, the eigenvectors
* of a real generalized symmetric-definite eigenproblem, of the form
* A*x=(lambda)*B*x, A*Bx=(lambda)*x, or B*A*x=(lambda)*x.
* Here A and B are assumed to be symmetric and B is also
* positive definite.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* Specifies the problem type to be solved:
* = 1: A*x = (lambda)*B*x
* = 2: A*B*x = (lambda)*x
* = 3: B*A*x = (lambda)*x
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangles of A and B are stored;
* = 'L': Lower triangles of A and B are stored.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the symmetric matrix A. If UPLO = 'U', the
* leading N-by-N upper triangular part of A contains the
* upper triangular part of the matrix A. If UPLO = 'L',
* the leading N-by-N lower triangular part of A contains
* the lower triangular part of the matrix A.
*
* On exit, if JOBZ = 'V', then if INFO = 0, A contains the
* matrix Z of eigenvectors. The eigenvectors are normalized
* as follows:
* if ITYPE = 1 or 2, Z**T*B*Z = I;
* if ITYPE = 3, Z**T*inv(B)*Z = I.
* If JOBZ = 'N', then on exit the upper triangle (if UPLO='U')
* or the lower triangle (if UPLO='L') of A, including the
* diagonal, is destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB, N)
* On entry, the symmetric positive definite matrix B.
* If UPLO = 'U', the leading N-by-N upper triangular part of B
* contains the upper triangular part of the matrix B.
* If UPLO = 'L', the leading N-by-N lower triangular part of B
* contains the lower triangular part of the matrix B.
*
* On exit, if INFO <= N, the part of B containing the matrix is
* overwritten by the triangular factor U or L from the Cholesky
* factorization B = U**T*U or B = L*L**T.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* W (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of the array WORK. LWORK >= max(1,3*N-1).
* For optimal efficiency, LWORK >= (NB+2)*N,
* where NB is the blocksize for DSYTRD returned by ILAENV.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: DPOTRF or DSYEV returned an error code:
* <= N: if INFO = i, DSYEV failed to converge;
* i off-diagonal elements of an intermediate
* tridiagonal form did not converge to zero;
* > N: if INFO = N + i, for 1 <= i <= N, then the leading
* minor of order i of B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param itype
* @param jobz
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param w
* @param _w_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dsygv(int itype, java.lang.String jobz, java.lang.String uplo, int n, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, double[] w, int _w_offset, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYGVD computes all the eigenvalues, and optionally, the eigenvectors
* of a real generalized symmetric-definite eigenproblem, of the form
* A*x=(lambda)*B*x, A*Bx=(lambda)*x, or B*A*x=(lambda)*x. Here A and
* B are assumed to be symmetric and B is also positive definite.
* If eigenvectors are desired, it uses a divide and conquer algorithm.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* Specifies the problem type to be solved:
* = 1: A*x = (lambda)*B*x
* = 2: A*B*x = (lambda)*x
* = 3: B*A*x = (lambda)*x
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangles of A and B are stored;
* = 'L': Lower triangles of A and B are stored.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the symmetric matrix A. If UPLO = 'U', the
* leading N-by-N upper triangular part of A contains the
* upper triangular part of the matrix A. If UPLO = 'L',
* the leading N-by-N lower triangular part of A contains
* the lower triangular part of the matrix A.
*
* On exit, if JOBZ = 'V', then if INFO = 0, A contains the
* matrix Z of eigenvectors. The eigenvectors are normalized
* as follows:
* if ITYPE = 1 or 2, Z**T*B*Z = I;
* if ITYPE = 3, Z**T*inv(B)*Z = I.
* If JOBZ = 'N', then on exit the upper triangle (if UPLO='U')
* or the lower triangle (if UPLO='L') of A, including the
* diagonal, is destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB, N)
* On entry, the symmetric matrix B. If UPLO = 'U', the
* leading N-by-N upper triangular part of B contains the
* upper triangular part of the matrix B. If UPLO = 'L',
* the leading N-by-N lower triangular part of B contains
* the lower triangular part of the matrix B.
*
* On exit, if INFO <= N, the part of B containing the matrix is
* overwritten by the triangular factor U or L from the Cholesky
* factorization B = U**T*U or B = L*L**T.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* W (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If N <= 1, LWORK >= 1.
* If JOBZ = 'N' and N > 1, LWORK >= 2*N+1.
* If JOBZ = 'V' and N > 1, LWORK >= 1 + 6*N + 2*N**2.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If N <= 1, LIWORK >= 1.
* If JOBZ = 'N' and N > 1, LIWORK >= 1.
* If JOBZ = 'V' and N > 1, LIWORK >= 3 + 5*N.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: DPOTRF or DSYEVD returned an error code:
* <= N: if INFO = i and JOBZ = 'N', then the algorithm
* failed to converge; i off-diagonal elements of an
* intermediate tridiagonal form did not converge to
* zero;
* if INFO = i and JOBZ = 'V', then the algorithm
* failed to compute an eigenvalue while working on
* the submatrix lying in rows and columns INFO/(N+1)
* through mod(INFO,N+1);
* > N: if INFO = N + i, for 1 <= i <= N, then the leading
* minor of order i of B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* Further Details
* ===============
*
* Based on contributions by
* Mark Fahey, Department of Mathematics, Univ. of Kentucky, USA
*
* Modified so that no backsubstitution is performed if DSYEVD fails to
* converge (NEIG in old code could be greater than N causing out of
* bounds reference to A - reported by Ralf Meyer). Also corrected the
* description of INFO and the test on ITYPE. Sven, 16 Feb 05.
* =====================================================================
*
* .. Parameters ..
*
*
* @param itype
* @param jobz
* @param uplo
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param w
* @param work
* @param lwork
* @param iwork
* @param liwork
* @param info
*
*/
abstract public void dsygvd(int itype, java.lang.String jobz, java.lang.String uplo, int n, double[] a, int lda, double[] b, int ldb, double[] w, double[] work, int lwork, int[] iwork, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYGVD computes all the eigenvalues, and optionally, the eigenvectors
* of a real generalized symmetric-definite eigenproblem, of the form
* A*x=(lambda)*B*x, A*Bx=(lambda)*x, or B*A*x=(lambda)*x. Here A and
* B are assumed to be symmetric and B is also positive definite.
* If eigenvectors are desired, it uses a divide and conquer algorithm.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* Specifies the problem type to be solved:
* = 1: A*x = (lambda)*B*x
* = 2: A*B*x = (lambda)*x
* = 3: B*A*x = (lambda)*x
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangles of A and B are stored;
* = 'L': Lower triangles of A and B are stored.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the symmetric matrix A. If UPLO = 'U', the
* leading N-by-N upper triangular part of A contains the
* upper triangular part of the matrix A. If UPLO = 'L',
* the leading N-by-N lower triangular part of A contains
* the lower triangular part of the matrix A.
*
* On exit, if JOBZ = 'V', then if INFO = 0, A contains the
* matrix Z of eigenvectors. The eigenvectors are normalized
* as follows:
* if ITYPE = 1 or 2, Z**T*B*Z = I;
* if ITYPE = 3, Z**T*inv(B)*Z = I.
* If JOBZ = 'N', then on exit the upper triangle (if UPLO='U')
* or the lower triangle (if UPLO='L') of A, including the
* diagonal, is destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB, N)
* On entry, the symmetric matrix B. If UPLO = 'U', the
* leading N-by-N upper triangular part of B contains the
* upper triangular part of the matrix B. If UPLO = 'L',
* the leading N-by-N lower triangular part of B contains
* the lower triangular part of the matrix B.
*
* On exit, if INFO <= N, the part of B containing the matrix is
* overwritten by the triangular factor U or L from the Cholesky
* factorization B = U**T*U or B = L*L**T.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* W (output) DOUBLE PRECISION array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If N <= 1, LWORK >= 1.
* If JOBZ = 'N' and N > 1, LWORK >= 2*N+1.
* If JOBZ = 'V' and N > 1, LWORK >= 1 + 6*N + 2*N**2.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If N <= 1, LIWORK >= 1.
* If JOBZ = 'N' and N > 1, LIWORK >= 1.
* If JOBZ = 'V' and N > 1, LIWORK >= 3 + 5*N.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: DPOTRF or DSYEVD returned an error code:
* <= N: if INFO = i and JOBZ = 'N', then the algorithm
* failed to converge; i off-diagonal elements of an
* intermediate tridiagonal form did not converge to
* zero;
* if INFO = i and JOBZ = 'V', then the algorithm
* failed to compute an eigenvalue while working on
* the submatrix lying in rows and columns INFO/(N+1)
* through mod(INFO,N+1);
* > N: if INFO = N + i, for 1 <= i <= N, then the leading
* minor of order i of B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* Further Details
* ===============
*
* Based on contributions by
* Mark Fahey, Department of Mathematics, Univ. of Kentucky, USA
*
* Modified so that no backsubstitution is performed if DSYEVD fails to
* converge (NEIG in old code could be greater than N causing out of
* bounds reference to A - reported by Ralf Meyer). Also corrected the
* description of INFO and the test on ITYPE. Sven, 16 Feb 05.
* =====================================================================
*
* .. Parameters ..
*
*
* @param itype
* @param jobz
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param w
* @param _w_offset
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param liwork
* @param info
*
*/
abstract public void dsygvd(int itype, java.lang.String jobz, java.lang.String uplo, int n, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, double[] w, int _w_offset, double[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYGVX computes selected eigenvalues, and optionally, eigenvectors
* of a real generalized symmetric-definite eigenproblem, of the form
* A*x=(lambda)*B*x, A*Bx=(lambda)*x, or B*A*x=(lambda)*x. Here A
* and B are assumed to be symmetric and B is also positive definite.
* Eigenvalues and eigenvectors can be selected by specifying either a
* range of values or a range of indices for the desired eigenvalues.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* Specifies the problem type to be solved:
* = 1: A*x = (lambda)*B*x
* = 2: A*B*x = (lambda)*x
* = 3: B*A*x = (lambda)*x
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A and B are stored;
* = 'L': Lower triangle of A and B are stored.
*
* N (input) INTEGER
* The order of the matrix pencil (A,B). N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the symmetric matrix A. If UPLO = 'U', the
* leading N-by-N upper triangular part of A contains the
* upper triangular part of the matrix A. If UPLO = 'L',
* the leading N-by-N lower triangular part of A contains
* the lower triangular part of the matrix A.
*
* On exit, the lower triangle (if UPLO='L') or the upper
* triangle (if UPLO='U') of A, including the diagonal, is
* destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the symmetric matrix B. If UPLO = 'U', the
* leading N-by-N upper triangular part of B contains the
* upper triangular part of the matrix B. If UPLO = 'L',
* the leading N-by-N lower triangular part of B contains
* the lower triangular part of the matrix B.
*
* On exit, if INFO <= N, the part of B containing the matrix is
* overwritten by the triangular factor U or L from the Cholesky
* factorization B = U**T*U or B = L*L**T.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* VL (input) DOUBLE PRECISION
* VU (input) DOUBLE PRECISION
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) DOUBLE PRECISION
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less than
* or equal to zero, then EPS*|T| will be used in its place,
* where |T| is the 1-norm of the tridiagonal matrix obtained
* by reducing A to tridiagonal form.
*
* Eigenvalues will be computed most accurately when ABSTOL is
* set to twice the underflow threshold 2*DLAMCH('S'), not zero.
* If this routine returns with INFO>0, indicating that some
* eigenvectors did not converge, try setting ABSTOL to
* 2*DLAMCH('S').
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* On normal exit, the first M elements contain the selected
* eigenvalues in ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, max(1,M))
* If JOBZ = 'N', then Z is not referenced.
* If JOBZ = 'V', then if INFO = 0, the first M columns of Z
* contain the orthonormal eigenvectors of the matrix A
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* The eigenvectors are normalized as follows:
* if ITYPE = 1 or 2, Z**T*B*Z = I;
* if ITYPE = 3, Z**T*inv(B)*Z = I.
*
* If an eigenvector fails to converge, then that column of Z
* contains the latest approximation to the eigenvector, and the
* index of the eigenvector is returned in IFAIL.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of the array WORK. LWORK >= max(1,8*N).
* For optimal efficiency, LWORK >= (NB+3)*N,
* where NB is the blocksize for DSYTRD returned by ILAENV.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (5*N)
*
* IFAIL (output) INTEGER array, dimension (N)
* If JOBZ = 'V', then if INFO = 0, the first M elements of
* IFAIL are zero. If INFO > 0, then IFAIL contains the
* indices of the eigenvectors that failed to converge.
* If JOBZ = 'N', then IFAIL is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: DPOTRF or DSYEVX returned an error code:
* <= N: if INFO = i, DSYEVX failed to converge;
* i eigenvectors failed to converge. Their indices
* are stored in array IFAIL.
* > N: if INFO = N + i, for 1 <= i <= N, then the leading
* minor of order i of B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* Further Details
* ===============
*
* Based on contributions by
* Mark Fahey, Department of Mathematics, Univ. of Kentucky, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param itype
* @param jobz
* @param range
* @param uplo
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param z
* @param ldz
* @param work
* @param lwork
* @param iwork
* @param ifail
* @param info
*
*/
abstract public void dsygvx(int itype, java.lang.String jobz, java.lang.String range, java.lang.String uplo, int n, double[] a, int lda, double[] b, int ldb, double vl, double vu, int il, int iu, double abstol, org.netlib.util.intW m, double[] w, double[] z, int ldz, double[] work, int lwork, int[] iwork, int[] ifail, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYGVX computes selected eigenvalues, and optionally, eigenvectors
* of a real generalized symmetric-definite eigenproblem, of the form
* A*x=(lambda)*B*x, A*Bx=(lambda)*x, or B*A*x=(lambda)*x. Here A
* and B are assumed to be symmetric and B is also positive definite.
* Eigenvalues and eigenvectors can be selected by specifying either a
* range of values or a range of indices for the desired eigenvalues.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* Specifies the problem type to be solved:
* = 1: A*x = (lambda)*B*x
* = 2: A*B*x = (lambda)*x
* = 3: B*A*x = (lambda)*x
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A and B are stored;
* = 'L': Lower triangle of A and B are stored.
*
* N (input) INTEGER
* The order of the matrix pencil (A,B). N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the symmetric matrix A. If UPLO = 'U', the
* leading N-by-N upper triangular part of A contains the
* upper triangular part of the matrix A. If UPLO = 'L',
* the leading N-by-N lower triangular part of A contains
* the lower triangular part of the matrix A.
*
* On exit, the lower triangle (if UPLO='L') or the upper
* triangle (if UPLO='U') of A, including the diagonal, is
* destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDA, N)
* On entry, the symmetric matrix B. If UPLO = 'U', the
* leading N-by-N upper triangular part of B contains the
* upper triangular part of the matrix B. If UPLO = 'L',
* the leading N-by-N lower triangular part of B contains
* the lower triangular part of the matrix B.
*
* On exit, if INFO <= N, the part of B containing the matrix is
* overwritten by the triangular factor U or L from the Cholesky
* factorization B = U**T*U or B = L*L**T.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* VL (input) DOUBLE PRECISION
* VU (input) DOUBLE PRECISION
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) DOUBLE PRECISION
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less than
* or equal to zero, then EPS*|T| will be used in its place,
* where |T| is the 1-norm of the tridiagonal matrix obtained
* by reducing A to tridiagonal form.
*
* Eigenvalues will be computed most accurately when ABSTOL is
* set to twice the underflow threshold 2*DLAMCH('S'), not zero.
* If this routine returns with INFO>0, indicating that some
* eigenvectors did not converge, try setting ABSTOL to
* 2*DLAMCH('S').
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) DOUBLE PRECISION array, dimension (N)
* On normal exit, the first M elements contain the selected
* eigenvalues in ascending order.
*
* Z (output) DOUBLE PRECISION array, dimension (LDZ, max(1,M))
* If JOBZ = 'N', then Z is not referenced.
* If JOBZ = 'V', then if INFO = 0, the first M columns of Z
* contain the orthonormal eigenvectors of the matrix A
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* The eigenvectors are normalized as follows:
* if ITYPE = 1 or 2, Z**T*B*Z = I;
* if ITYPE = 3, Z**T*inv(B)*Z = I.
*
* If an eigenvector fails to converge, then that column of Z
* contains the latest approximation to the eigenvector, and the
* index of the eigenvector is returned in IFAIL.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of the array WORK. LWORK >= max(1,8*N).
* For optimal efficiency, LWORK >= (NB+3)*N,
* where NB is the blocksize for DSYTRD returned by ILAENV.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (5*N)
*
* IFAIL (output) INTEGER array, dimension (N)
* If JOBZ = 'V', then if INFO = 0, the first M elements of
* IFAIL are zero. If INFO > 0, then IFAIL contains the
* indices of the eigenvectors that failed to converge.
* If JOBZ = 'N', then IFAIL is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: DPOTRF or DSYEVX returned an error code:
* <= N: if INFO = i, DSYEVX failed to converge;
* i eigenvectors failed to converge. Their indices
* are stored in array IFAIL.
* > N: if INFO = N + i, for 1 <= i <= N, then the leading
* minor of order i of B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* Further Details
* ===============
*
* Based on contributions by
* Mark Fahey, Department of Mathematics, Univ. of Kentucky, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param itype
* @param jobz
* @param range
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param ifail
* @param _ifail_offset
* @param info
*
*/
abstract public void dsygvx(int itype, java.lang.String jobz, java.lang.String range, java.lang.String uplo, int n, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, double vl, double vu, int il, int iu, double abstol, org.netlib.util.intW m, double[] w, int _w_offset, double[] z, int _z_offset, int ldz, double[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int[] ifail, int _ifail_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYRFS improves the computed solution to a system of linear
* equations when the coefficient matrix is symmetric indefinite, and
* provides error bounds and backward error estimates for the solution.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The symmetric matrix A. If UPLO = 'U', the leading N-by-N
* upper triangular part of A contains the upper triangular part
* of the matrix A, and the strictly lower triangular part of A
* is not referenced. If UPLO = 'L', the leading N-by-N lower
* triangular part of A contains the lower triangular part of
* the matrix A, and the strictly upper triangular part of A is
* not referenced.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* AF (input) DOUBLE PRECISION array, dimension (LDAF,N)
* The factored form of the matrix A. AF contains the block
* diagonal matrix D and the multipliers used to obtain the
* factor U or L from the factorization A = U*D*U**T or
* A = L*D*L**T as computed by DSYTRF.
*
* LDAF (input) INTEGER
* The leading dimension of the array AF. LDAF >= max(1,N).
*
* IPIV (input) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D
* as determined by DSYTRF.
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by DSYTRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param a
* @param lda
* @param af
* @param ldaf
* @param ipiv
* @param b
* @param ldb
* @param x
* @param ldx
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void dsyrfs(java.lang.String uplo, int n, int nrhs, double[] a, int lda, double[] af, int ldaf, int[] ipiv, double[] b, int ldb, double[] x, int ldx, double[] ferr, double[] berr, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYRFS improves the computed solution to a system of linear
* equations when the coefficient matrix is symmetric indefinite, and
* provides error bounds and backward error estimates for the solution.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The symmetric matrix A. If UPLO = 'U', the leading N-by-N
* upper triangular part of A contains the upper triangular part
* of the matrix A, and the strictly lower triangular part of A
* is not referenced. If UPLO = 'L', the leading N-by-N lower
* triangular part of A contains the lower triangular part of
* the matrix A, and the strictly upper triangular part of A is
* not referenced.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* AF (input) DOUBLE PRECISION array, dimension (LDAF,N)
* The factored form of the matrix A. AF contains the block
* diagonal matrix D and the multipliers used to obtain the
* factor U or L from the factorization A = U*D*U**T or
* A = L*D*L**T as computed by DSYTRF.
*
* LDAF (input) INTEGER
* The leading dimension of the array AF. LDAF >= max(1,N).
*
* IPIV (input) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D
* as determined by DSYTRF.
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by DSYTRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param af
* @param _af_offset
* @param ldaf
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dsyrfs(java.lang.String uplo, int n, int nrhs, double[] a, int _a_offset, int lda, double[] af, int _af_offset, int ldaf, int[] ipiv, int _ipiv_offset, double[] b, int _b_offset, int ldb, double[] x, int _x_offset, int ldx, double[] ferr, int _ferr_offset, double[] berr, int _berr_offset, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYSV computes the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N symmetric matrix and X and B are N-by-NRHS
* matrices.
*
* The diagonal pivoting method is used to factor A as
* A = U * D * U**T, if UPLO = 'U', or
* A = L * D * L**T, if UPLO = 'L',
* where U (or L) is a product of permutation and unit upper (lower)
* triangular matrices, and D is symmetric and block diagonal with
* 1-by-1 and 2-by-2 diagonal blocks. The factored form of A is then
* used to solve the system of equations A * X = B.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* N-by-N upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading N-by-N lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, if INFO = 0, the block diagonal matrix D and the
* multipliers used to obtain the factor U or L from the
* factorization A = U*D*U**T or A = L*D*L**T as computed by
* DSYTRF.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (output) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D, as
* determined by DSYTRF. If IPIV(k) > 0, then rows and columns
* k and IPIV(k) were interchanged, and D(k,k) is a 1-by-1
* diagonal block. If UPLO = 'U' and IPIV(k) = IPIV(k-1) < 0,
* then rows and columns k-1 and -IPIV(k) were interchanged and
* D(k-1:k,k-1:k) is a 2-by-2 diagonal block. If UPLO = 'L' and
* IPIV(k) = IPIV(k+1) < 0, then rows and columns k+1 and
* -IPIV(k) were interchanged and D(k:k+1,k:k+1) is a 2-by-2
* diagonal block.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if INFO = 0, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of WORK. LWORK >= 1, and for best performance
* LWORK >= max(1,N*NB), where NB is the optimal blocksize for
* DSYTRF.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, D(i,i) is exactly zero. The factorization
* has been completed, but the block diagonal matrix D is
* exactly singular, so the solution could not be computed.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param a
* @param lda
* @param ipiv
* @param b
* @param ldb
* @param work
* @param lwork
* @param info
*
*/
abstract public void dsysv(java.lang.String uplo, int n, int nrhs, double[] a, int lda, int[] ipiv, double[] b, int ldb, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYSV computes the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N symmetric matrix and X and B are N-by-NRHS
* matrices.
*
* The diagonal pivoting method is used to factor A as
* A = U * D * U**T, if UPLO = 'U', or
* A = L * D * L**T, if UPLO = 'L',
* where U (or L) is a product of permutation and unit upper (lower)
* triangular matrices, and D is symmetric and block diagonal with
* 1-by-1 and 2-by-2 diagonal blocks. The factored form of A is then
* used to solve the system of equations A * X = B.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* N-by-N upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading N-by-N lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, if INFO = 0, the block diagonal matrix D and the
* multipliers used to obtain the factor U or L from the
* factorization A = U*D*U**T or A = L*D*L**T as computed by
* DSYTRF.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (output) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D, as
* determined by DSYTRF. If IPIV(k) > 0, then rows and columns
* k and IPIV(k) were interchanged, and D(k,k) is a 1-by-1
* diagonal block. If UPLO = 'U' and IPIV(k) = IPIV(k-1) < 0,
* then rows and columns k-1 and -IPIV(k) were interchanged and
* D(k-1:k,k-1:k) is a 2-by-2 diagonal block. If UPLO = 'L' and
* IPIV(k) = IPIV(k+1) < 0, then rows and columns k+1 and
* -IPIV(k) were interchanged and D(k:k+1,k:k+1) is a 2-by-2
* diagonal block.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if INFO = 0, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of WORK. LWORK >= 1, and for best performance
* LWORK >= max(1,N*NB), where NB is the optimal blocksize for
* DSYTRF.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, D(i,i) is exactly zero. The factorization
* has been completed, but the block diagonal matrix D is
* exactly singular, so the solution could not be computed.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dsysv(java.lang.String uplo, int n, int nrhs, double[] a, int _a_offset, int lda, int[] ipiv, int _ipiv_offset, double[] b, int _b_offset, int ldb, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYSVX uses the diagonal pivoting factorization to compute the
* solution to a real system of linear equations A * X = B,
* where A is an N-by-N symmetric matrix and X and B are N-by-NRHS
* matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed:
*
* 1. If FACT = 'N', the diagonal pivoting method is used to factor A.
* The form of the factorization is
* A = U * D * U**T, if UPLO = 'U', or
* A = L * D * L**T, if UPLO = 'L',
* where U (or L) is a product of permutation and unit upper (lower)
* triangular matrices, and D is symmetric and block diagonal with
* 1-by-1 and 2-by-2 diagonal blocks.
*
* 2. If some D(i,i)=0, so that D is exactly singular, then the routine
* returns with INFO = i. Otherwise, the factored form of A is used
* to estimate the condition number of the matrix A. If the
* reciprocal of the condition number is less than machine precision,
* INFO = N+1 is returned as a warning, but the routine still goes on
* to solve for X and compute error bounds as described below.
*
* 3. The system of equations is solved for X using the factored form
* of A.
*
* 4. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of A has been
* supplied on entry.
* = 'F': On entry, AF and IPIV contain the factored form of
* A. AF and IPIV will not be modified.
* = 'N': The matrix A will be copied to AF and factored.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The symmetric matrix A. If UPLO = 'U', the leading N-by-N
* upper triangular part of A contains the upper triangular part
* of the matrix A, and the strictly lower triangular part of A
* is not referenced. If UPLO = 'L', the leading N-by-N lower
* triangular part of A contains the lower triangular part of
* the matrix A, and the strictly upper triangular part of A is
* not referenced.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* AF (input or output) DOUBLE PRECISION array, dimension (LDAF,N)
* If FACT = 'F', then AF is an input argument and on entry
* contains the block diagonal matrix D and the multipliers used
* to obtain the factor U or L from the factorization
* A = U*D*U**T or A = L*D*L**T as computed by DSYTRF.
*
* If FACT = 'N', then AF is an output argument and on exit
* returns the block diagonal matrix D and the multipliers used
* to obtain the factor U or L from the factorization
* A = U*D*U**T or A = L*D*L**T.
*
* LDAF (input) INTEGER
* The leading dimension of the array AF. LDAF >= max(1,N).
*
* IPIV (input or output) INTEGER array, dimension (N)
* If FACT = 'F', then IPIV is an input argument and on entry
* contains details of the interchanges and the block structure
* of D, as determined by DSYTRF.
* If IPIV(k) > 0, then rows and columns k and IPIV(k) were
* interchanged and D(k,k) is a 1-by-1 diagonal block.
* If UPLO = 'U' and IPIV(k) = IPIV(k-1) < 0, then rows and
* columns k-1 and -IPIV(k) were interchanged and D(k-1:k,k-1:k)
* is a 2-by-2 diagonal block. If UPLO = 'L' and IPIV(k) =
* IPIV(k+1) < 0, then rows and columns k+1 and -IPIV(k) were
* interchanged and D(k:k+1,k:k+1) is a 2-by-2 diagonal block.
*
* If FACT = 'N', then IPIV is an output argument and on exit
* contains details of the interchanges and the block structure
* of D, as determined by DSYTRF.
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The N-by-NRHS right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* If INFO = 0 or INFO = N+1, the N-by-NRHS solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) DOUBLE PRECISION
* The estimate of the reciprocal condition number of the matrix
* A. If RCOND is less than the machine precision (in
* particular, if RCOND = 0), the matrix is singular to working
* precision. This condition is indicated by a return code of
* INFO > 0.
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of WORK. LWORK >= max(1,3*N), and for best
* performance, when FACT = 'N', LWORK >= max(1,3*N,N*NB), where
* NB is the optimal blocksize for DSYTRF.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= N: D(i,i) is exactly zero. The factorization
* has been completed but the factor D is exactly
* singular, so the solution and error bounds could
* not be computed. RCOND = 0 is returned.
* = N+1: D is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param uplo
* @param n
* @param nrhs
* @param a
* @param lda
* @param af
* @param ldaf
* @param ipiv
* @param b
* @param ldb
* @param x
* @param ldx
* @param rcond
* @param ferr
* @param berr
* @param work
* @param lwork
* @param iwork
* @param info
*
*/
abstract public void dsysvx(java.lang.String fact, java.lang.String uplo, int n, int nrhs, double[] a, int lda, double[] af, int ldaf, int[] ipiv, double[] b, int ldb, double[] x, int ldx, org.netlib.util.doubleW rcond, double[] ferr, double[] berr, double[] work, int lwork, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYSVX uses the diagonal pivoting factorization to compute the
* solution to a real system of linear equations A * X = B,
* where A is an N-by-N symmetric matrix and X and B are N-by-NRHS
* matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed:
*
* 1. If FACT = 'N', the diagonal pivoting method is used to factor A.
* The form of the factorization is
* A = U * D * U**T, if UPLO = 'U', or
* A = L * D * L**T, if UPLO = 'L',
* where U (or L) is a product of permutation and unit upper (lower)
* triangular matrices, and D is symmetric and block diagonal with
* 1-by-1 and 2-by-2 diagonal blocks.
*
* 2. If some D(i,i)=0, so that D is exactly singular, then the routine
* returns with INFO = i. Otherwise, the factored form of A is used
* to estimate the condition number of the matrix A. If the
* reciprocal of the condition number is less than machine precision,
* INFO = N+1 is returned as a warning, but the routine still goes on
* to solve for X and compute error bounds as described below.
*
* 3. The system of equations is solved for X using the factored form
* of A.
*
* 4. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of A has been
* supplied on entry.
* = 'F': On entry, AF and IPIV contain the factored form of
* A. AF and IPIV will not be modified.
* = 'N': The matrix A will be copied to AF and factored.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The symmetric matrix A. If UPLO = 'U', the leading N-by-N
* upper triangular part of A contains the upper triangular part
* of the matrix A, and the strictly lower triangular part of A
* is not referenced. If UPLO = 'L', the leading N-by-N lower
* triangular part of A contains the lower triangular part of
* the matrix A, and the strictly upper triangular part of A is
* not referenced.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* AF (input or output) DOUBLE PRECISION array, dimension (LDAF,N)
* If FACT = 'F', then AF is an input argument and on entry
* contains the block diagonal matrix D and the multipliers used
* to obtain the factor U or L from the factorization
* A = U*D*U**T or A = L*D*L**T as computed by DSYTRF.
*
* If FACT = 'N', then AF is an output argument and on exit
* returns the block diagonal matrix D and the multipliers used
* to obtain the factor U or L from the factorization
* A = U*D*U**T or A = L*D*L**T.
*
* LDAF (input) INTEGER
* The leading dimension of the array AF. LDAF >= max(1,N).
*
* IPIV (input or output) INTEGER array, dimension (N)
* If FACT = 'F', then IPIV is an input argument and on entry
* contains details of the interchanges and the block structure
* of D, as determined by DSYTRF.
* If IPIV(k) > 0, then rows and columns k and IPIV(k) were
* interchanged and D(k,k) is a 1-by-1 diagonal block.
* If UPLO = 'U' and IPIV(k) = IPIV(k-1) < 0, then rows and
* columns k-1 and -IPIV(k) were interchanged and D(k-1:k,k-1:k)
* is a 2-by-2 diagonal block. If UPLO = 'L' and IPIV(k) =
* IPIV(k+1) < 0, then rows and columns k+1 and -IPIV(k) were
* interchanged and D(k:k+1,k:k+1) is a 2-by-2 diagonal block.
*
* If FACT = 'N', then IPIV is an output argument and on exit
* contains details of the interchanges and the block structure
* of D, as determined by DSYTRF.
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The N-by-NRHS right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) DOUBLE PRECISION array, dimension (LDX,NRHS)
* If INFO = 0 or INFO = N+1, the N-by-NRHS solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) DOUBLE PRECISION
* The estimate of the reciprocal condition number of the matrix
* A. If RCOND is less than the machine precision (in
* particular, if RCOND = 0), the matrix is singular to working
* precision. This condition is indicated by a return code of
* INFO > 0.
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of WORK. LWORK >= max(1,3*N), and for best
* performance, when FACT = 'N', LWORK >= max(1,3*N,N*NB), where
* NB is the optimal blocksize for DSYTRF.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= N: D(i,i) is exactly zero. The factorization
* has been completed but the factor D is exactly
* singular, so the solution and error bounds could
* not be computed. RCOND = 0 is returned.
* = N+1: D is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param uplo
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param af
* @param _af_offset
* @param ldaf
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param rcond
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dsysvx(java.lang.String fact, java.lang.String uplo, int n, int nrhs, double[] a, int _a_offset, int lda, double[] af, int _af_offset, int ldaf, int[] ipiv, int _ipiv_offset, double[] b, int _b_offset, int ldb, double[] x, int _x_offset, int ldx, org.netlib.util.doubleW rcond, double[] ferr, int _ferr_offset, double[] berr, int _berr_offset, double[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYTD2 reduces a real symmetric matrix A to symmetric tridiagonal
* form T by an orthogonal similarity transformation: Q' * A * Q = T.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored:
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* n-by-n upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading n-by-n lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
* On exit, if UPLO = 'U', the diagonal and first superdiagonal
* of A are overwritten by the corresponding elements of the
* tridiagonal matrix T, and the elements above the first
* superdiagonal, with the array TAU, represent the orthogonal
* matrix Q as a product of elementary reflectors; if UPLO
* = 'L', the diagonal and first subdiagonal of A are over-
* written by the corresponding elements of the tridiagonal
* matrix T, and the elements below the first subdiagonal, with
* the array TAU, represent the orthogonal matrix Q as a product
* of elementary reflectors. See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* D (output) DOUBLE PRECISION array, dimension (N)
* The diagonal elements of the tridiagonal matrix T:
* D(i) = A(i,i).
*
* E (output) DOUBLE PRECISION array, dimension (N-1)
* The off-diagonal elements of the tridiagonal matrix T:
* E(i) = A(i,i+1) if UPLO = 'U', E(i) = A(i+1,i) if UPLO = 'L'.
*
* TAU (output) DOUBLE PRECISION array, dimension (N-1)
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* If UPLO = 'U', the matrix Q is represented as a product of elementary
* reflectors
*
* Q = H(n-1) . . . H(2) H(1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(i+1:n) = 0 and v(i) = 1; v(1:i-1) is stored on exit in
* A(1:i-1,i+1), and tau in TAU(i).
*
* If UPLO = 'L', the matrix Q is represented as a product of elementary
* reflectors
*
* Q = H(1) H(2) . . . H(n-1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i) = 0 and v(i+1) = 1; v(i+2:n) is stored on exit in A(i+2:n,i),
* and tau in TAU(i).
*
* The contents of A on exit are illustrated by the following examples
* with n = 5:
*
* if UPLO = 'U': if UPLO = 'L':
*
* ( d e v2 v3 v4 ) ( d )
* ( d e v3 v4 ) ( e d )
* ( d e v4 ) ( v1 e d )
* ( d e ) ( v1 v2 e d )
* ( d ) ( v1 v2 v3 e d )
*
* where d and e denote diagonal and off-diagonal elements of T, and vi
* denotes an element of the vector defining H(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param lda
* @param d
* @param e
* @param tau
* @param info
*
*/
abstract public void dsytd2(java.lang.String uplo, int n, double[] a, int lda, double[] d, double[] e, double[] tau, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYTD2 reduces a real symmetric matrix A to symmetric tridiagonal
* form T by an orthogonal similarity transformation: Q' * A * Q = T.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored:
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* n-by-n upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading n-by-n lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
* On exit, if UPLO = 'U', the diagonal and first superdiagonal
* of A are overwritten by the corresponding elements of the
* tridiagonal matrix T, and the elements above the first
* superdiagonal, with the array TAU, represent the orthogonal
* matrix Q as a product of elementary reflectors; if UPLO
* = 'L', the diagonal and first subdiagonal of A are over-
* written by the corresponding elements of the tridiagonal
* matrix T, and the elements below the first subdiagonal, with
* the array TAU, represent the orthogonal matrix Q as a product
* of elementary reflectors. See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* D (output) DOUBLE PRECISION array, dimension (N)
* The diagonal elements of the tridiagonal matrix T:
* D(i) = A(i,i).
*
* E (output) DOUBLE PRECISION array, dimension (N-1)
* The off-diagonal elements of the tridiagonal matrix T:
* E(i) = A(i,i+1) if UPLO = 'U', E(i) = A(i+1,i) if UPLO = 'L'.
*
* TAU (output) DOUBLE PRECISION array, dimension (N-1)
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* If UPLO = 'U', the matrix Q is represented as a product of elementary
* reflectors
*
* Q = H(n-1) . . . H(2) H(1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(i+1:n) = 0 and v(i) = 1; v(1:i-1) is stored on exit in
* A(1:i-1,i+1), and tau in TAU(i).
*
* If UPLO = 'L', the matrix Q is represented as a product of elementary
* reflectors
*
* Q = H(1) H(2) . . . H(n-1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i) = 0 and v(i+1) = 1; v(i+2:n) is stored on exit in A(i+2:n,i),
* and tau in TAU(i).
*
* The contents of A on exit are illustrated by the following examples
* with n = 5:
*
* if UPLO = 'U': if UPLO = 'L':
*
* ( d e v2 v3 v4 ) ( d )
* ( d e v3 v4 ) ( e d )
* ( d e v4 ) ( v1 e d )
* ( d e ) ( v1 v2 e d )
* ( d ) ( v1 v2 v3 e d )
*
* where d and e denote diagonal and off-diagonal elements of T, and vi
* denotes an element of the vector defining H(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param tau
* @param _tau_offset
* @param info
*
*/
abstract public void dsytd2(java.lang.String uplo, int n, double[] a, int _a_offset, int lda, double[] d, int _d_offset, double[] e, int _e_offset, double[] tau, int _tau_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYTF2 computes the factorization of a real symmetric matrix A using
* the Bunch-Kaufman diagonal pivoting method:
*
* A = U*D*U' or A = L*D*L'
*
* where U (or L) is a product of permutation and unit upper (lower)
* triangular matrices, U' is the transpose of U, and D is symmetric and
* block diagonal with 1-by-1 and 2-by-2 diagonal blocks.
*
* This is the unblocked version of the algorithm, calling Level 2 BLAS.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored:
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* n-by-n upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading n-by-n lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, the block diagonal matrix D and the multipliers used
* to obtain the factor U or L (see below for further details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (output) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D.
* If IPIV(k) > 0, then rows and columns k and IPIV(k) were
* interchanged and D(k,k) is a 1-by-1 diagonal block.
* If UPLO = 'U' and IPIV(k) = IPIV(k-1) < 0, then rows and
* columns k-1 and -IPIV(k) were interchanged and D(k-1:k,k-1:k)
* is a 2-by-2 diagonal block. If UPLO = 'L' and IPIV(k) =
* IPIV(k+1) < 0, then rows and columns k+1 and -IPIV(k) were
* interchanged and D(k:k+1,k:k+1) is a 2-by-2 diagonal block.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
* > 0: if INFO = k, D(k,k) is exactly zero. The factorization
* has been completed, but the block diagonal matrix D is
* exactly singular, and division by zero will occur if it
* is used to solve a system of equations.
*
* Further Details
* ===============
*
* 09-29-06 - patch from
* Bobby Cheng, MathWorks
*
* Replace l.204 and l.372
* IF( MAX( ABSAKK, COLMAX ).EQ.ZERO ) THEN
* by
* IF( (MAX( ABSAKK, COLMAX ).EQ.ZERO) .OR. DISNAN(ABSAKK) ) THEN
*
* 01-01-96 - Based on modifications by
* J. Lewis, Boeing Computer Services Company
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
* 1-96 - Based on modifications by J. Lewis, Boeing Computer Services
* Company
*
* If UPLO = 'U', then A = U*D*U', where
* U = P(n)*U(n)* ... *P(k)U(k)* ...,
* i.e., U is a product of terms P(k)*U(k), where k decreases from n to
* 1 in steps of 1 or 2, and D is a block diagonal matrix with 1-by-1
* and 2-by-2 diagonal blocks D(k). P(k) is a permutation matrix as
* defined by IPIV(k), and U(k) is a unit upper triangular matrix, such
* that if the diagonal block D(k) is of order s (s = 1 or 2), then
*
* ( I v 0 ) k-s
* U(k) = ( 0 I 0 ) s
* ( 0 0 I ) n-k
* k-s s n-k
*
* If s = 1, D(k) overwrites A(k,k), and v overwrites A(1:k-1,k).
* If s = 2, the upper triangle of D(k) overwrites A(k-1,k-1), A(k-1,k),
* and A(k,k), and v overwrites A(1:k-2,k-1:k).
*
* If UPLO = 'L', then A = L*D*L', where
* L = P(1)*L(1)* ... *P(k)*L(k)* ...,
* i.e., L is a product of terms P(k)*L(k), where k increases from 1 to
* n in steps of 1 or 2, and D is a block diagonal matrix with 1-by-1
* and 2-by-2 diagonal blocks D(k). P(k) is a permutation matrix as
* defined by IPIV(k), and L(k) is a unit lower triangular matrix, such
* that if the diagonal block D(k) is of order s (s = 1 or 2), then
*
* ( I 0 0 ) k-1
* L(k) = ( 0 I 0 ) s
* ( 0 v I ) n-k-s+1
* k-1 s n-k-s+1
*
* If s = 1, D(k) overwrites A(k,k), and v overwrites A(k+1:n,k).
* If s = 2, the lower triangle of D(k) overwrites A(k,k), A(k+1,k),
* and A(k+1,k+1), and v overwrites A(k+2:n,k:k+1).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param lda
* @param ipiv
* @param info
*
*/
abstract public void dsytf2(java.lang.String uplo, int n, double[] a, int lda, int[] ipiv, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYTF2 computes the factorization of a real symmetric matrix A using
* the Bunch-Kaufman diagonal pivoting method:
*
* A = U*D*U' or A = L*D*L'
*
* where U (or L) is a product of permutation and unit upper (lower)
* triangular matrices, U' is the transpose of U, and D is symmetric and
* block diagonal with 1-by-1 and 2-by-2 diagonal blocks.
*
* This is the unblocked version of the algorithm, calling Level 2 BLAS.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored:
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* n-by-n upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading n-by-n lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, the block diagonal matrix D and the multipliers used
* to obtain the factor U or L (see below for further details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (output) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D.
* If IPIV(k) > 0, then rows and columns k and IPIV(k) were
* interchanged and D(k,k) is a 1-by-1 diagonal block.
* If UPLO = 'U' and IPIV(k) = IPIV(k-1) < 0, then rows and
* columns k-1 and -IPIV(k) were interchanged and D(k-1:k,k-1:k)
* is a 2-by-2 diagonal block. If UPLO = 'L' and IPIV(k) =
* IPIV(k+1) < 0, then rows and columns k+1 and -IPIV(k) were
* interchanged and D(k:k+1,k:k+1) is a 2-by-2 diagonal block.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
* > 0: if INFO = k, D(k,k) is exactly zero. The factorization
* has been completed, but the block diagonal matrix D is
* exactly singular, and division by zero will occur if it
* is used to solve a system of equations.
*
* Further Details
* ===============
*
* 09-29-06 - patch from
* Bobby Cheng, MathWorks
*
* Replace l.204 and l.372
* IF( MAX( ABSAKK, COLMAX ).EQ.ZERO ) THEN
* by
* IF( (MAX( ABSAKK, COLMAX ).EQ.ZERO) .OR. DISNAN(ABSAKK) ) THEN
*
* 01-01-96 - Based on modifications by
* J. Lewis, Boeing Computer Services Company
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
* 1-96 - Based on modifications by J. Lewis, Boeing Computer Services
* Company
*
* If UPLO = 'U', then A = U*D*U', where
* U = P(n)*U(n)* ... *P(k)U(k)* ...,
* i.e., U is a product of terms P(k)*U(k), where k decreases from n to
* 1 in steps of 1 or 2, and D is a block diagonal matrix with 1-by-1
* and 2-by-2 diagonal blocks D(k). P(k) is a permutation matrix as
* defined by IPIV(k), and U(k) is a unit upper triangular matrix, such
* that if the diagonal block D(k) is of order s (s = 1 or 2), then
*
* ( I v 0 ) k-s
* U(k) = ( 0 I 0 ) s
* ( 0 0 I ) n-k
* k-s s n-k
*
* If s = 1, D(k) overwrites A(k,k), and v overwrites A(1:k-1,k).
* If s = 2, the upper triangle of D(k) overwrites A(k-1,k-1), A(k-1,k),
* and A(k,k), and v overwrites A(1:k-2,k-1:k).
*
* If UPLO = 'L', then A = L*D*L', where
* L = P(1)*L(1)* ... *P(k)*L(k)* ...,
* i.e., L is a product of terms P(k)*L(k), where k increases from 1 to
* n in steps of 1 or 2, and D is a block diagonal matrix with 1-by-1
* and 2-by-2 diagonal blocks D(k). P(k) is a permutation matrix as
* defined by IPIV(k), and L(k) is a unit lower triangular matrix, such
* that if the diagonal block D(k) is of order s (s = 1 or 2), then
*
* ( I 0 0 ) k-1
* L(k) = ( 0 I 0 ) s
* ( 0 v I ) n-k-s+1
* k-1 s n-k-s+1
*
* If s = 1, D(k) overwrites A(k,k), and v overwrites A(k+1:n,k).
* If s = 2, the lower triangle of D(k) overwrites A(k,k), A(k+1,k),
* and A(k+1,k+1), and v overwrites A(k+2:n,k:k+1).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param ipiv
* @param _ipiv_offset
* @param info
*
*/
abstract public void dsytf2(java.lang.String uplo, int n, double[] a, int _a_offset, int lda, int[] ipiv, int _ipiv_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYTRD reduces a real symmetric matrix A to real symmetric
* tridiagonal form T by an orthogonal similarity transformation:
* Q**T * A * Q = T.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* N-by-N upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading N-by-N lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
* On exit, if UPLO = 'U', the diagonal and first superdiagonal
* of A are overwritten by the corresponding elements of the
* tridiagonal matrix T, and the elements above the first
* superdiagonal, with the array TAU, represent the orthogonal
* matrix Q as a product of elementary reflectors; if UPLO
* = 'L', the diagonal and first subdiagonal of A are over-
* written by the corresponding elements of the tridiagonal
* matrix T, and the elements below the first subdiagonal, with
* the array TAU, represent the orthogonal matrix Q as a product
* of elementary reflectors. See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* D (output) DOUBLE PRECISION array, dimension (N)
* The diagonal elements of the tridiagonal matrix T:
* D(i) = A(i,i).
*
* E (output) DOUBLE PRECISION array, dimension (N-1)
* The off-diagonal elements of the tridiagonal matrix T:
* E(i) = A(i,i+1) if UPLO = 'U', E(i) = A(i+1,i) if UPLO = 'L'.
*
* TAU (output) DOUBLE PRECISION array, dimension (N-1)
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= 1.
* For optimum performance LWORK >= N*NB, where NB is the
* optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* If UPLO = 'U', the matrix Q is represented as a product of elementary
* reflectors
*
* Q = H(n-1) . . . H(2) H(1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(i+1:n) = 0 and v(i) = 1; v(1:i-1) is stored on exit in
* A(1:i-1,i+1), and tau in TAU(i).
*
* If UPLO = 'L', the matrix Q is represented as a product of elementary
* reflectors
*
* Q = H(1) H(2) . . . H(n-1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i) = 0 and v(i+1) = 1; v(i+2:n) is stored on exit in A(i+2:n,i),
* and tau in TAU(i).
*
* The contents of A on exit are illustrated by the following examples
* with n = 5:
*
* if UPLO = 'U': if UPLO = 'L':
*
* ( d e v2 v3 v4 ) ( d )
* ( d e v3 v4 ) ( e d )
* ( d e v4 ) ( v1 e d )
* ( d e ) ( v1 v2 e d )
* ( d ) ( v1 v2 v3 e d )
*
* where d and e denote diagonal and off-diagonal elements of T, and vi
* denotes an element of the vector defining H(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param lda
* @param d
* @param e
* @param tau
* @param work
* @param lwork
* @param info
*
*/
abstract public void dsytrd(java.lang.String uplo, int n, double[] a, int lda, double[] d, double[] e, double[] tau, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYTRD reduces a real symmetric matrix A to real symmetric
* tridiagonal form T by an orthogonal similarity transformation:
* Q**T * A * Q = T.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* N-by-N upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading N-by-N lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
* On exit, if UPLO = 'U', the diagonal and first superdiagonal
* of A are overwritten by the corresponding elements of the
* tridiagonal matrix T, and the elements above the first
* superdiagonal, with the array TAU, represent the orthogonal
* matrix Q as a product of elementary reflectors; if UPLO
* = 'L', the diagonal and first subdiagonal of A are over-
* written by the corresponding elements of the tridiagonal
* matrix T, and the elements below the first subdiagonal, with
* the array TAU, represent the orthogonal matrix Q as a product
* of elementary reflectors. See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* D (output) DOUBLE PRECISION array, dimension (N)
* The diagonal elements of the tridiagonal matrix T:
* D(i) = A(i,i).
*
* E (output) DOUBLE PRECISION array, dimension (N-1)
* The off-diagonal elements of the tridiagonal matrix T:
* E(i) = A(i,i+1) if UPLO = 'U', E(i) = A(i+1,i) if UPLO = 'L'.
*
* TAU (output) DOUBLE PRECISION array, dimension (N-1)
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= 1.
* For optimum performance LWORK >= N*NB, where NB is the
* optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* If UPLO = 'U', the matrix Q is represented as a product of elementary
* reflectors
*
* Q = H(n-1) . . . H(2) H(1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(i+1:n) = 0 and v(i) = 1; v(1:i-1) is stored on exit in
* A(1:i-1,i+1), and tau in TAU(i).
*
* If UPLO = 'L', the matrix Q is represented as a product of elementary
* reflectors
*
* Q = H(1) H(2) . . . H(n-1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i) = 0 and v(i+1) = 1; v(i+2:n) is stored on exit in A(i+2:n,i),
* and tau in TAU(i).
*
* The contents of A on exit are illustrated by the following examples
* with n = 5:
*
* if UPLO = 'U': if UPLO = 'L':
*
* ( d e v2 v3 v4 ) ( d )
* ( d e v3 v4 ) ( e d )
* ( d e v4 ) ( v1 e d )
* ( d e ) ( v1 v2 e d )
* ( d ) ( v1 v2 v3 e d )
*
* where d and e denote diagonal and off-diagonal elements of T, and vi
* denotes an element of the vector defining H(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dsytrd(java.lang.String uplo, int n, double[] a, int _a_offset, int lda, double[] d, int _d_offset, double[] e, int _e_offset, double[] tau, int _tau_offset, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYTRF computes the factorization of a real symmetric matrix A using
* the Bunch-Kaufman diagonal pivoting method. The form of the
* factorization is
*
* A = U*D*U**T or A = L*D*L**T
*
* where U (or L) is a product of permutation and unit upper (lower)
* triangular matrices, and D is symmetric and block diagonal with
* 1-by-1 and 2-by-2 diagonal blocks.
*
* This is the blocked version of the algorithm, calling Level 3 BLAS.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* N-by-N upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading N-by-N lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, the block diagonal matrix D and the multipliers used
* to obtain the factor U or L (see below for further details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (output) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D.
* If IPIV(k) > 0, then rows and columns k and IPIV(k) were
* interchanged and D(k,k) is a 1-by-1 diagonal block.
* If UPLO = 'U' and IPIV(k) = IPIV(k-1) < 0, then rows and
* columns k-1 and -IPIV(k) were interchanged and D(k-1:k,k-1:k)
* is a 2-by-2 diagonal block. If UPLO = 'L' and IPIV(k) =
* IPIV(k+1) < 0, then rows and columns k+1 and -IPIV(k) were
* interchanged and D(k:k+1,k:k+1) is a 2-by-2 diagonal block.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of WORK. LWORK >=1. For best performance
* LWORK >= N*NB, where NB is the block size returned by ILAENV.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, D(i,i) is exactly zero. The factorization
* has been completed, but the block diagonal matrix D is
* exactly singular, and division by zero will occur if it
* is used to solve a system of equations.
*
* Further Details
* ===============
*
* If UPLO = 'U', then A = U*D*U', where
* U = P(n)*U(n)* ... *P(k)U(k)* ...,
* i.e., U is a product of terms P(k)*U(k), where k decreases from n to
* 1 in steps of 1 or 2, and D is a block diagonal matrix with 1-by-1
* and 2-by-2 diagonal blocks D(k). P(k) is a permutation matrix as
* defined by IPIV(k), and U(k) is a unit upper triangular matrix, such
* that if the diagonal block D(k) is of order s (s = 1 or 2), then
*
* ( I v 0 ) k-s
* U(k) = ( 0 I 0 ) s
* ( 0 0 I ) n-k
* k-s s n-k
*
* If s = 1, D(k) overwrites A(k,k), and v overwrites A(1:k-1,k).
* If s = 2, the upper triangle of D(k) overwrites A(k-1,k-1), A(k-1,k),
* and A(k,k), and v overwrites A(1:k-2,k-1:k).
*
* If UPLO = 'L', then A = L*D*L', where
* L = P(1)*L(1)* ... *P(k)*L(k)* ...,
* i.e., L is a product of terms P(k)*L(k), where k increases from 1 to
* n in steps of 1 or 2, and D is a block diagonal matrix with 1-by-1
* and 2-by-2 diagonal blocks D(k). P(k) is a permutation matrix as
* defined by IPIV(k), and L(k) is a unit lower triangular matrix, such
* that if the diagonal block D(k) is of order s (s = 1 or 2), then
*
* ( I 0 0 ) k-1
* L(k) = ( 0 I 0 ) s
* ( 0 v I ) n-k-s+1
* k-1 s n-k-s+1
*
* If s = 1, D(k) overwrites A(k,k), and v overwrites A(k+1:n,k).
* If s = 2, the lower triangle of D(k) overwrites A(k,k), A(k+1,k),
* and A(k+1,k+1), and v overwrites A(k+2:n,k:k+1).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param uplo
* @param n
* @param a
* @param lda
* @param ipiv
* @param work
* @param lwork
* @param info
*
*/
abstract public void dsytrf(java.lang.String uplo, int n, double[] a, int lda, int[] ipiv, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYTRF computes the factorization of a real symmetric matrix A using
* the Bunch-Kaufman diagonal pivoting method. The form of the
* factorization is
*
* A = U*D*U**T or A = L*D*L**T
*
* where U (or L) is a product of permutation and unit upper (lower)
* triangular matrices, and D is symmetric and block diagonal with
* 1-by-1 and 2-by-2 diagonal blocks.
*
* This is the blocked version of the algorithm, calling Level 3 BLAS.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* N-by-N upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading N-by-N lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, the block diagonal matrix D and the multipliers used
* to obtain the factor U or L (see below for further details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (output) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D.
* If IPIV(k) > 0, then rows and columns k and IPIV(k) were
* interchanged and D(k,k) is a 1-by-1 diagonal block.
* If UPLO = 'U' and IPIV(k) = IPIV(k-1) < 0, then rows and
* columns k-1 and -IPIV(k) were interchanged and D(k-1:k,k-1:k)
* is a 2-by-2 diagonal block. If UPLO = 'L' and IPIV(k) =
* IPIV(k+1) < 0, then rows and columns k+1 and -IPIV(k) were
* interchanged and D(k:k+1,k:k+1) is a 2-by-2 diagonal block.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of WORK. LWORK >=1. For best performance
* LWORK >= N*NB, where NB is the block size returned by ILAENV.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, D(i,i) is exactly zero. The factorization
* has been completed, but the block diagonal matrix D is
* exactly singular, and division by zero will occur if it
* is used to solve a system of equations.
*
* Further Details
* ===============
*
* If UPLO = 'U', then A = U*D*U', where
* U = P(n)*U(n)* ... *P(k)U(k)* ...,
* i.e., U is a product of terms P(k)*U(k), where k decreases from n to
* 1 in steps of 1 or 2, and D is a block diagonal matrix with 1-by-1
* and 2-by-2 diagonal blocks D(k). P(k) is a permutation matrix as
* defined by IPIV(k), and U(k) is a unit upper triangular matrix, such
* that if the diagonal block D(k) is of order s (s = 1 or 2), then
*
* ( I v 0 ) k-s
* U(k) = ( 0 I 0 ) s
* ( 0 0 I ) n-k
* k-s s n-k
*
* If s = 1, D(k) overwrites A(k,k), and v overwrites A(1:k-1,k).
* If s = 2, the upper triangle of D(k) overwrites A(k-1,k-1), A(k-1,k),
* and A(k,k), and v overwrites A(1:k-2,k-1:k).
*
* If UPLO = 'L', then A = L*D*L', where
* L = P(1)*L(1)* ... *P(k)*L(k)* ...,
* i.e., L is a product of terms P(k)*L(k), where k increases from 1 to
* n in steps of 1 or 2, and D is a block diagonal matrix with 1-by-1
* and 2-by-2 diagonal blocks D(k). P(k) is a permutation matrix as
* defined by IPIV(k), and L(k) is a unit lower triangular matrix, such
* that if the diagonal block D(k) is of order s (s = 1 or 2), then
*
* ( I 0 0 ) k-1
* L(k) = ( 0 I 0 ) s
* ( 0 v I ) n-k-s+1
* k-1 s n-k-s+1
*
* If s = 1, D(k) overwrites A(k,k), and v overwrites A(k+1:n,k).
* If s = 2, the lower triangle of D(k) overwrites A(k,k), A(k+1,k),
* and A(k+1,k+1), and v overwrites A(k+2:n,k:k+1).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param ipiv
* @param _ipiv_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dsytrf(java.lang.String uplo, int n, double[] a, int _a_offset, int lda, int[] ipiv, int _ipiv_offset, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYTRI computes the inverse of a real symmetric indefinite matrix
* A using the factorization A = U*D*U**T or A = L*D*L**T computed by
* DSYTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the details of the factorization are stored
* as an upper or lower triangular matrix.
* = 'U': Upper triangular, form is A = U*D*U**T;
* = 'L': Lower triangular, form is A = L*D*L**T.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the block diagonal matrix D and the multipliers
* used to obtain the factor U or L as computed by DSYTRF.
*
* On exit, if INFO = 0, the (symmetric) inverse of the original
* matrix. If UPLO = 'U', the upper triangular part of the
* inverse is formed and the part of A below the diagonal is not
* referenced; if UPLO = 'L' the lower triangular part of the
* inverse is formed and the part of A above the diagonal is
* not referenced.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (input) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D
* as determined by DSYTRF.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, D(i,i) = 0; the matrix is singular and its
* inverse could not be computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param lda
* @param ipiv
* @param work
* @param info
*
*/
abstract public void dsytri(java.lang.String uplo, int n, double[] a, int lda, int[] ipiv, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYTRI computes the inverse of a real symmetric indefinite matrix
* A using the factorization A = U*D*U**T or A = L*D*L**T computed by
* DSYTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the details of the factorization are stored
* as an upper or lower triangular matrix.
* = 'U': Upper triangular, form is A = U*D*U**T;
* = 'L': Lower triangular, form is A = L*D*L**T.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the block diagonal matrix D and the multipliers
* used to obtain the factor U or L as computed by DSYTRF.
*
* On exit, if INFO = 0, the (symmetric) inverse of the original
* matrix. If UPLO = 'U', the upper triangular part of the
* inverse is formed and the part of A below the diagonal is not
* referenced; if UPLO = 'L' the lower triangular part of the
* inverse is formed and the part of A above the diagonal is
* not referenced.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (input) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D
* as determined by DSYTRF.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, D(i,i) = 0; the matrix is singular and its
* inverse could not be computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param ipiv
* @param _ipiv_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dsytri(java.lang.String uplo, int n, double[] a, int _a_offset, int lda, int[] ipiv, int _ipiv_offset, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYTRS solves a system of linear equations A*X = B with a real
* symmetric matrix A using the factorization A = U*D*U**T or
* A = L*D*L**T computed by DSYTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the details of the factorization are stored
* as an upper or lower triangular matrix.
* = 'U': Upper triangular, form is A = U*D*U**T;
* = 'L': Lower triangular, form is A = L*D*L**T.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The block diagonal matrix D and the multipliers used to
* obtain the factor U or L as computed by DSYTRF.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (input) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D
* as determined by DSYTRF.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param a
* @param lda
* @param ipiv
* @param b
* @param ldb
* @param info
*
*/
abstract public void dsytrs(java.lang.String uplo, int n, int nrhs, double[] a, int lda, int[] ipiv, double[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DSYTRS solves a system of linear equations A*X = B with a real
* symmetric matrix A using the factorization A = U*D*U**T or
* A = L*D*L**T computed by DSYTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the details of the factorization are stored
* as an upper or lower triangular matrix.
* = 'U': Upper triangular, form is A = U*D*U**T;
* = 'L': Lower triangular, form is A = L*D*L**T.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The block diagonal matrix D and the multipliers used to
* obtain the factor U or L as computed by DSYTRF.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (input) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D
* as determined by DSYTRF.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void dsytrs(java.lang.String uplo, int n, int nrhs, double[] a, int _a_offset, int lda, int[] ipiv, int _ipiv_offset, double[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTBCON estimates the reciprocal of the condition number of a
* triangular band matrix A, in either the 1-norm or the infinity-norm.
*
* The norm of A is computed and an estimate is obtained for
* norm(inv(A)), then the reciprocal of the condition number is
* computed as
* RCOND = 1 / ( norm(A) * norm(inv(A)) ).
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies whether the 1-norm condition number or the
* infinity-norm condition number is required:
* = '1' or 'O': 1-norm;
* = 'I': Infinity-norm.
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals or subdiagonals of the
* triangular band matrix A. KD >= 0.
*
* AB (input) DOUBLE PRECISION array, dimension (LDAB,N)
* The upper or lower triangular band matrix A, stored in the
* first kd+1 rows of the array. The j-th column of A is stored
* in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
* If DIAG = 'U', the diagonal elements of A are not referenced
* and are assumed to be 1.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* RCOND (output) DOUBLE PRECISION
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(norm(A) * norm(inv(A))).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param diag
* @param n
* @param kd
* @param ab
* @param ldab
* @param rcond
* @param work
* @param iwork
* @param info
*
*/
abstract public void dtbcon(java.lang.String norm, java.lang.String uplo, java.lang.String diag, int n, int kd, double[] ab, int ldab, org.netlib.util.doubleW rcond, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTBCON estimates the reciprocal of the condition number of a
* triangular band matrix A, in either the 1-norm or the infinity-norm.
*
* The norm of A is computed and an estimate is obtained for
* norm(inv(A)), then the reciprocal of the condition number is
* computed as
* RCOND = 1 / ( norm(A) * norm(inv(A)) ).
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies whether the 1-norm condition number or the
* infinity-norm condition number is required:
* = '1' or 'O': 1-norm;
* = 'I': Infinity-norm.
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals or subdiagonals of the
* triangular band matrix A. KD >= 0.
*
* AB (input) DOUBLE PRECISION array, dimension (LDAB,N)
* The upper or lower triangular band matrix A, stored in the
* first kd+1 rows of the array. The j-th column of A is stored
* in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
* If DIAG = 'U', the diagonal elements of A are not referenced
* and are assumed to be 1.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* RCOND (output) DOUBLE PRECISION
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(norm(A) * norm(inv(A))).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param diag
* @param n
* @param kd
* @param ab
* @param _ab_offset
* @param ldab
* @param rcond
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dtbcon(java.lang.String norm, java.lang.String uplo, java.lang.String diag, int n, int kd, double[] ab, int _ab_offset, int ldab, org.netlib.util.doubleW rcond, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTBRFS provides error bounds and backward error estimates for the
* solution to a system of linear equations with a triangular band
* coefficient matrix.
*
* The solution matrix X must be computed by DTBTRS or some other
* means before entering this routine. DTBRFS does not do iterative
* refinement because doing so cannot improve the backward error.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals or subdiagonals of the
* triangular band matrix A. KD >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AB (input) DOUBLE PRECISION array, dimension (LDAB,N)
* The upper or lower triangular band matrix A, stored in the
* first kd+1 rows of the array. The j-th column of A is stored
* in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
* If DIAG = 'U', the diagonal elements of A are not referenced
* and are assumed to be 1.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input) DOUBLE PRECISION array, dimension (LDX,NRHS)
* The solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param n
* @param kd
* @param nrhs
* @param ab
* @param ldab
* @param b
* @param ldb
* @param x
* @param ldx
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void dtbrfs(java.lang.String uplo, java.lang.String trans, java.lang.String diag, int n, int kd, int nrhs, double[] ab, int ldab, double[] b, int ldb, double[] x, int ldx, double[] ferr, double[] berr, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTBRFS provides error bounds and backward error estimates for the
* solution to a system of linear equations with a triangular band
* coefficient matrix.
*
* The solution matrix X must be computed by DTBTRS or some other
* means before entering this routine. DTBRFS does not do iterative
* refinement because doing so cannot improve the backward error.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals or subdiagonals of the
* triangular band matrix A. KD >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AB (input) DOUBLE PRECISION array, dimension (LDAB,N)
* The upper or lower triangular band matrix A, stored in the
* first kd+1 rows of the array. The j-th column of A is stored
* in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
* If DIAG = 'U', the diagonal elements of A are not referenced
* and are assumed to be 1.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input) DOUBLE PRECISION array, dimension (LDX,NRHS)
* The solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param n
* @param kd
* @param nrhs
* @param ab
* @param _ab_offset
* @param ldab
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dtbrfs(java.lang.String uplo, java.lang.String trans, java.lang.String diag, int n, int kd, int nrhs, double[] ab, int _ab_offset, int ldab, double[] b, int _b_offset, int ldb, double[] x, int _x_offset, int ldx, double[] ferr, int _ferr_offset, double[] berr, int _berr_offset, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTBTRS solves a triangular system of the form
*
* A * X = B or A**T * X = B,
*
* where A is a triangular band matrix of order N, and B is an
* N-by NRHS matrix. A check is made to verify that A is nonsingular.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* TRANS (input) CHARACTER*1
* Specifies the form the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals or subdiagonals of the
* triangular band matrix A. KD >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AB (input) DOUBLE PRECISION array, dimension (LDAB,N)
* The upper or lower triangular band matrix A, stored in the
* first kd+1 rows of AB. The j-th column of A is stored
* in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
* If DIAG = 'U', the diagonal elements of A are not referenced
* and are assumed to be 1.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, if INFO = 0, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the i-th diagonal element of A is zero,
* indicating that the matrix is singular and the
* solutions X have not been computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param n
* @param kd
* @param nrhs
* @param ab
* @param ldab
* @param b
* @param ldb
* @param info
*
*/
abstract public void dtbtrs(java.lang.String uplo, java.lang.String trans, java.lang.String diag, int n, int kd, int nrhs, double[] ab, int ldab, double[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTBTRS solves a triangular system of the form
*
* A * X = B or A**T * X = B,
*
* where A is a triangular band matrix of order N, and B is an
* N-by NRHS matrix. A check is made to verify that A is nonsingular.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* TRANS (input) CHARACTER*1
* Specifies the form the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals or subdiagonals of the
* triangular band matrix A. KD >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AB (input) DOUBLE PRECISION array, dimension (LDAB,N)
* The upper or lower triangular band matrix A, stored in the
* first kd+1 rows of AB. The j-th column of A is stored
* in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
* If DIAG = 'U', the diagonal elements of A are not referenced
* and are assumed to be 1.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, if INFO = 0, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the i-th diagonal element of A is zero,
* indicating that the matrix is singular and the
* solutions X have not been computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param n
* @param kd
* @param nrhs
* @param ab
* @param _ab_offset
* @param ldab
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void dtbtrs(java.lang.String uplo, java.lang.String trans, java.lang.String diag, int n, int kd, int nrhs, double[] ab, int _ab_offset, int ldab, double[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
*
* Purpose
* =======
*
* DTGEVC computes some or all of the right and/or left eigenvectors of
* a pair of real matrices (S,P), where S is a quasi-triangular matrix
* and P is upper triangular. Matrix pairs of this type are produced by
* the generalized Schur factorization of a matrix pair (A,B):
*
* A = Q*S*Z**T, B = Q*P*Z**T
*
* as computed by DGGHRD + DHGEQZ.
*
* The right eigenvector x and the left eigenvector y of (S,P)
* corresponding to an eigenvalue w are defined by:
*
* S*x = w*P*x, (y**H)*S = w*(y**H)*P,
*
* where y**H denotes the conjugate tranpose of y.
* The eigenvalues are not input to this routine, but are computed
* directly from the diagonal blocks of S and P.
*
* This routine returns the matrices X and/or Y of right and left
* eigenvectors of (S,P), or the products Z*X and/or Q*Y,
* where Z and Q are input matrices.
* If Q and Z are the orthogonal factors from the generalized Schur
* factorization of a matrix pair (A,B), then Z*X and Q*Y
* are the matrices of right and left eigenvectors of (A,B).
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'R': compute right eigenvectors only;
* = 'L': compute left eigenvectors only;
* = 'B': compute both right and left eigenvectors.
*
* HOWMNY (input) CHARACTER*1
* = 'A': compute all right and/or left eigenvectors;
* = 'B': compute all right and/or left eigenvectors,
* backtransformed by the matrices in VR and/or VL;
* = 'S': compute selected right and/or left eigenvectors,
* specified by the logical array SELECT.
*
* SELECT (input) LOGICAL array, dimension (N)
* If HOWMNY='S', SELECT specifies the eigenvectors to be
* computed. If w(j) is a real eigenvalue, the corresponding
* real eigenvector is computed if SELECT(j) is .TRUE..
* If w(j) and w(j+1) are the real and imaginary parts of a
* complex eigenvalue, the corresponding complex eigenvector
* is computed if either SELECT(j) or SELECT(j+1) is .TRUE.,
* and on exit SELECT(j) is set to .TRUE. and SELECT(j+1) is
* set to .FALSE..
* Not referenced if HOWMNY = 'A' or 'B'.
*
* N (input) INTEGER
* The order of the matrices S and P. N >= 0.
*
* S (input) DOUBLE PRECISION array, dimension (LDS,N)
* The upper quasi-triangular matrix S from a generalized Schur
* factorization, as computed by DHGEQZ.
*
* LDS (input) INTEGER
* The leading dimension of array S. LDS >= max(1,N).
*
* P (input) DOUBLE PRECISION array, dimension (LDP,N)
* The upper triangular matrix P from a generalized Schur
* factorization, as computed by DHGEQZ.
* 2-by-2 diagonal blocks of P corresponding to 2-by-2 blocks
* of S must be in positive diagonal form.
*
* LDP (input) INTEGER
* The leading dimension of array P. LDP >= max(1,N).
*
* VL (input/output) DOUBLE PRECISION array, dimension (LDVL,MM)
* On entry, if SIDE = 'L' or 'B' and HOWMNY = 'B', VL must
* contain an N-by-N matrix Q (usually the orthogonal matrix Q
* of left Schur vectors returned by DHGEQZ).
* On exit, if SIDE = 'L' or 'B', VL contains:
* if HOWMNY = 'A', the matrix Y of left eigenvectors of (S,P);
* if HOWMNY = 'B', the matrix Q*Y;
* if HOWMNY = 'S', the left eigenvectors of (S,P) specified by
* SELECT, stored consecutively in the columns of
* VL, in the same order as their eigenvalues.
*
* A complex eigenvector corresponding to a complex eigenvalue
* is stored in two consecutive columns, the first holding the
* real part, and the second the imaginary part.
*
* Not referenced if SIDE = 'R'.
*
* LDVL (input) INTEGER
* The leading dimension of array VL. LDVL >= 1, and if
* SIDE = 'L' or 'B', LDVL >= N.
*
* VR (input/output) DOUBLE PRECISION array, dimension (LDVR,MM)
* On entry, if SIDE = 'R' or 'B' and HOWMNY = 'B', VR must
* contain an N-by-N matrix Z (usually the orthogonal matrix Z
* of right Schur vectors returned by DHGEQZ).
*
* On exit, if SIDE = 'R' or 'B', VR contains:
* if HOWMNY = 'A', the matrix X of right eigenvectors of (S,P);
* if HOWMNY = 'B' or 'b', the matrix Z*X;
* if HOWMNY = 'S' or 's', the right eigenvectors of (S,P)
* specified by SELECT, stored consecutively in the
* columns of VR, in the same order as their
* eigenvalues.
*
* A complex eigenvector corresponding to a complex eigenvalue
* is stored in two consecutive columns, the first holding the
* real part and the second the imaginary part.
*
* Not referenced if SIDE = 'L'.
*
* LDVR (input) INTEGER
* The leading dimension of the array VR. LDVR >= 1, and if
* SIDE = 'R' or 'B', LDVR >= N.
*
* MM (input) INTEGER
* The number of columns in the arrays VL and/or VR. MM >= M.
*
* M (output) INTEGER
* The number of columns in the arrays VL and/or VR actually
* used to store the eigenvectors. If HOWMNY = 'A' or 'B', M
* is set to N. Each selected real eigenvector occupies one
* column and each selected complex eigenvector occupies two
* columns.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (6*N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: the 2-by-2 block (INFO:INFO+1) does not have a complex
* eigenvalue.
*
* Further Details
* ===============
*
* Allocation of workspace:
* ---------- -- ---------
*
* WORK( j ) = 1-norm of j-th column of A, above the diagonal
* WORK( N+j ) = 1-norm of j-th column of B, above the diagonal
* WORK( 2*N+1:3*N ) = real part of eigenvector
* WORK( 3*N+1:4*N ) = imaginary part of eigenvector
* WORK( 4*N+1:5*N ) = real part of back-transformed eigenvector
* WORK( 5*N+1:6*N ) = imaginary part of back-transformed eigenvector
*
* Rowwise vs. columnwise solution methods:
* ------- -- ---------- -------- -------
*
* Finding a generalized eigenvector consists basically of solving the
* singular triangular system
*
* (A - w B) x = 0 (for right) or: (A - w B)**H y = 0 (for left)
*
* Consider finding the i-th right eigenvector (assume all eigenvalues
* are real). The equation to be solved is:
* n i
* 0 = sum C(j,k) v(k) = sum C(j,k) v(k) for j = i,. . .,1
* k=j k=j
*
* where C = (A - w B) (The components v(i+1:n) are 0.)
*
* The "rowwise" method is:
*
* (1) v(i) := 1
* for j = i-1,. . .,1:
* i
* (2) compute s = - sum C(j,k) v(k) and
* k=j+1
*
* (3) v(j) := s / C(j,j)
*
* Step 2 is sometimes called the "dot product" step, since it is an
* inner product between the j-th row and the portion of the eigenvector
* that has been computed so far.
*
* The "columnwise" method consists basically in doing the sums
* for all the rows in parallel. As each v(j) is computed, the
* contribution of v(j) times the j-th column of C is added to the
* partial sums. Since FORTRAN arrays are stored columnwise, this has
* the advantage that at each step, the elements of C that are accessed
* are adjacent to one another, whereas with the rowwise method, the
* elements accessed at a step are spaced LDS (and LDP) words apart.
*
* When finding left eigenvectors, the matrix in question is the
* transpose of the one in storage, so the rowwise method then
* actually accesses columns of A and B at each step, and so is the
* preferred method.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param howmny
* @param select
* @param n
* @param s
* @param lds
* @param p
* @param ldp
* @param vl
* @param ldvl
* @param vr
* @param ldvr
* @param mm
* @param m
* @param work
* @param info
*
*/
abstract public void dtgevc(java.lang.String side, java.lang.String howmny, boolean[] select, int n, double[] s, int lds, double[] p, int ldp, double[] vl, int ldvl, double[] vr, int ldvr, int mm, org.netlib.util.intW m, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
*
* Purpose
* =======
*
* DTGEVC computes some or all of the right and/or left eigenvectors of
* a pair of real matrices (S,P), where S is a quasi-triangular matrix
* and P is upper triangular. Matrix pairs of this type are produced by
* the generalized Schur factorization of a matrix pair (A,B):
*
* A = Q*S*Z**T, B = Q*P*Z**T
*
* as computed by DGGHRD + DHGEQZ.
*
* The right eigenvector x and the left eigenvector y of (S,P)
* corresponding to an eigenvalue w are defined by:
*
* S*x = w*P*x, (y**H)*S = w*(y**H)*P,
*
* where y**H denotes the conjugate tranpose of y.
* The eigenvalues are not input to this routine, but are computed
* directly from the diagonal blocks of S and P.
*
* This routine returns the matrices X and/or Y of right and left
* eigenvectors of (S,P), or the products Z*X and/or Q*Y,
* where Z and Q are input matrices.
* If Q and Z are the orthogonal factors from the generalized Schur
* factorization of a matrix pair (A,B), then Z*X and Q*Y
* are the matrices of right and left eigenvectors of (A,B).
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'R': compute right eigenvectors only;
* = 'L': compute left eigenvectors only;
* = 'B': compute both right and left eigenvectors.
*
* HOWMNY (input) CHARACTER*1
* = 'A': compute all right and/or left eigenvectors;
* = 'B': compute all right and/or left eigenvectors,
* backtransformed by the matrices in VR and/or VL;
* = 'S': compute selected right and/or left eigenvectors,
* specified by the logical array SELECT.
*
* SELECT (input) LOGICAL array, dimension (N)
* If HOWMNY='S', SELECT specifies the eigenvectors to be
* computed. If w(j) is a real eigenvalue, the corresponding
* real eigenvector is computed if SELECT(j) is .TRUE..
* If w(j) and w(j+1) are the real and imaginary parts of a
* complex eigenvalue, the corresponding complex eigenvector
* is computed if either SELECT(j) or SELECT(j+1) is .TRUE.,
* and on exit SELECT(j) is set to .TRUE. and SELECT(j+1) is
* set to .FALSE..
* Not referenced if HOWMNY = 'A' or 'B'.
*
* N (input) INTEGER
* The order of the matrices S and P. N >= 0.
*
* S (input) DOUBLE PRECISION array, dimension (LDS,N)
* The upper quasi-triangular matrix S from a generalized Schur
* factorization, as computed by DHGEQZ.
*
* LDS (input) INTEGER
* The leading dimension of array S. LDS >= max(1,N).
*
* P (input) DOUBLE PRECISION array, dimension (LDP,N)
* The upper triangular matrix P from a generalized Schur
* factorization, as computed by DHGEQZ.
* 2-by-2 diagonal blocks of P corresponding to 2-by-2 blocks
* of S must be in positive diagonal form.
*
* LDP (input) INTEGER
* The leading dimension of array P. LDP >= max(1,N).
*
* VL (input/output) DOUBLE PRECISION array, dimension (LDVL,MM)
* On entry, if SIDE = 'L' or 'B' and HOWMNY = 'B', VL must
* contain an N-by-N matrix Q (usually the orthogonal matrix Q
* of left Schur vectors returned by DHGEQZ).
* On exit, if SIDE = 'L' or 'B', VL contains:
* if HOWMNY = 'A', the matrix Y of left eigenvectors of (S,P);
* if HOWMNY = 'B', the matrix Q*Y;
* if HOWMNY = 'S', the left eigenvectors of (S,P) specified by
* SELECT, stored consecutively in the columns of
* VL, in the same order as their eigenvalues.
*
* A complex eigenvector corresponding to a complex eigenvalue
* is stored in two consecutive columns, the first holding the
* real part, and the second the imaginary part.
*
* Not referenced if SIDE = 'R'.
*
* LDVL (input) INTEGER
* The leading dimension of array VL. LDVL >= 1, and if
* SIDE = 'L' or 'B', LDVL >= N.
*
* VR (input/output) DOUBLE PRECISION array, dimension (LDVR,MM)
* On entry, if SIDE = 'R' or 'B' and HOWMNY = 'B', VR must
* contain an N-by-N matrix Z (usually the orthogonal matrix Z
* of right Schur vectors returned by DHGEQZ).
*
* On exit, if SIDE = 'R' or 'B', VR contains:
* if HOWMNY = 'A', the matrix X of right eigenvectors of (S,P);
* if HOWMNY = 'B' or 'b', the matrix Z*X;
* if HOWMNY = 'S' or 's', the right eigenvectors of (S,P)
* specified by SELECT, stored consecutively in the
* columns of VR, in the same order as their
* eigenvalues.
*
* A complex eigenvector corresponding to a complex eigenvalue
* is stored in two consecutive columns, the first holding the
* real part and the second the imaginary part.
*
* Not referenced if SIDE = 'L'.
*
* LDVR (input) INTEGER
* The leading dimension of the array VR. LDVR >= 1, and if
* SIDE = 'R' or 'B', LDVR >= N.
*
* MM (input) INTEGER
* The number of columns in the arrays VL and/or VR. MM >= M.
*
* M (output) INTEGER
* The number of columns in the arrays VL and/or VR actually
* used to store the eigenvectors. If HOWMNY = 'A' or 'B', M
* is set to N. Each selected real eigenvector occupies one
* column and each selected complex eigenvector occupies two
* columns.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (6*N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: the 2-by-2 block (INFO:INFO+1) does not have a complex
* eigenvalue.
*
* Further Details
* ===============
*
* Allocation of workspace:
* ---------- -- ---------
*
* WORK( j ) = 1-norm of j-th column of A, above the diagonal
* WORK( N+j ) = 1-norm of j-th column of B, above the diagonal
* WORK( 2*N+1:3*N ) = real part of eigenvector
* WORK( 3*N+1:4*N ) = imaginary part of eigenvector
* WORK( 4*N+1:5*N ) = real part of back-transformed eigenvector
* WORK( 5*N+1:6*N ) = imaginary part of back-transformed eigenvector
*
* Rowwise vs. columnwise solution methods:
* ------- -- ---------- -------- -------
*
* Finding a generalized eigenvector consists basically of solving the
* singular triangular system
*
* (A - w B) x = 0 (for right) or: (A - w B)**H y = 0 (for left)
*
* Consider finding the i-th right eigenvector (assume all eigenvalues
* are real). The equation to be solved is:
* n i
* 0 = sum C(j,k) v(k) = sum C(j,k) v(k) for j = i,. . .,1
* k=j k=j
*
* where C = (A - w B) (The components v(i+1:n) are 0.)
*
* The "rowwise" method is:
*
* (1) v(i) := 1
* for j = i-1,. . .,1:
* i
* (2) compute s = - sum C(j,k) v(k) and
* k=j+1
*
* (3) v(j) := s / C(j,j)
*
* Step 2 is sometimes called the "dot product" step, since it is an
* inner product between the j-th row and the portion of the eigenvector
* that has been computed so far.
*
* The "columnwise" method consists basically in doing the sums
* for all the rows in parallel. As each v(j) is computed, the
* contribution of v(j) times the j-th column of C is added to the
* partial sums. Since FORTRAN arrays are stored columnwise, this has
* the advantage that at each step, the elements of C that are accessed
* are adjacent to one another, whereas with the rowwise method, the
* elements accessed at a step are spaced LDS (and LDP) words apart.
*
* When finding left eigenvectors, the matrix in question is the
* transpose of the one in storage, so the rowwise method then
* actually accesses columns of A and B at each step, and so is the
* preferred method.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param howmny
* @param select
* @param _select_offset
* @param n
* @param s
* @param _s_offset
* @param lds
* @param p
* @param _p_offset
* @param ldp
* @param vl
* @param _vl_offset
* @param ldvl
* @param vr
* @param _vr_offset
* @param ldvr
* @param mm
* @param m
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dtgevc(java.lang.String side, java.lang.String howmny, boolean[] select, int _select_offset, int n, double[] s, int _s_offset, int lds, double[] p, int _p_offset, int ldp, double[] vl, int _vl_offset, int ldvl, double[] vr, int _vr_offset, int ldvr, int mm, org.netlib.util.intW m, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTGEX2 swaps adjacent diagonal blocks (A11, B11) and (A22, B22)
* of size 1-by-1 or 2-by-2 in an upper (quasi) triangular matrix pair
* (A, B) by an orthogonal equivalence transformation.
*
* (A, B) must be in generalized real Schur canonical form (as returned
* by DGGES), i.e. A is block upper triangular with 1-by-1 and 2-by-2
* diagonal blocks. B is upper triangular.
*
* Optionally, the matrices Q and Z of generalized Schur vectors are
* updated.
*
* Q(in) * A(in) * Z(in)' = Q(out) * A(out) * Z(out)'
* Q(in) * B(in) * Z(in)' = Q(out) * B(out) * Z(out)'
*
*
* Arguments
* =========
*
* WANTQ (input) LOGICAL
* .TRUE. : update the left transformation matrix Q;
* .FALSE.: do not update Q.
*
* WANTZ (input) LOGICAL
* .TRUE. : update the right transformation matrix Z;
* .FALSE.: do not update Z.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* A (input/output) DOUBLE PRECISION arrays, dimensions (LDA,N)
* On entry, the matrix A in the pair (A, B).
* On exit, the updated matrix A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION arrays, dimensions (LDB,N)
* On entry, the matrix B in the pair (A, B).
* On exit, the updated matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* Q (input/output) DOUBLE PRECISION array, dimension (LDZ,N)
* On entry, if WANTQ = .TRUE., the orthogonal matrix Q.
* On exit, the updated matrix Q.
* Not referenced if WANTQ = .FALSE..
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= 1.
* If WANTQ = .TRUE., LDQ >= N.
*
* Z (input/output) DOUBLE PRECISION array, dimension (LDZ,N)
* On entry, if WANTZ =.TRUE., the orthogonal matrix Z.
* On exit, the updated matrix Z.
* Not referenced if WANTZ = .FALSE..
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1.
* If WANTZ = .TRUE., LDZ >= N.
*
* J1 (input) INTEGER
* The index to the first block (A11, B11). 1 <= J1 <= N.
*
* N1 (input) INTEGER
* The order of the first block (A11, B11). N1 = 0, 1 or 2.
*
* N2 (input) INTEGER
* The order of the second block (A22, B22). N2 = 0, 1 or 2.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (MAX(1,LWORK)).
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* LWORK >= MAX( 1, N*(N2+N1), (N2+N1)*(N2+N1)*2 )
*
* INFO (output) INTEGER
* =0: Successful exit
* >0: If INFO = 1, the transformed matrix (A, B) would be
* too far from generalized Schur form; the blocks are
* not swapped and (A, B) and (Q, Z) are unchanged.
* The problem of swapping is too ill-conditioned.
* <0: If INFO = -16: LWORK is too small. Appropriate value
* for LWORK is returned in WORK(1).
*
* Further Details
* ===============
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* In the current code both weak and strong stability tests are
* performed. The user can omit the strong stability test by changing
* the internal logical parameter WANDS to .FALSE.. See ref. [2] for
* details.
*
* [1] B. Kagstrom; A Direct Method for Reordering Eigenvalues in the
* Generalized Real Schur Form of a Regular Matrix Pair (A, B), in
* M.S. Moonen et al (eds), Linear Algebra for Large Scale and
* Real-Time Applications, Kluwer Academic Publ. 1993, pp 195-218.
*
* [2] B. Kagstrom and P. Poromaa; Computing Eigenspaces with Specified
* Eigenvalues of a Regular Matrix Pair (A, B) and Condition
* Estimation: Theory, Algorithms and Software,
* Report UMINF - 94.04, Department of Computing Science, Umea
* University, S-901 87 Umea, Sweden, 1994. Also as LAPACK Working
* Note 87. To appear in Numerical Algorithms, 1996.
*
* =====================================================================
* Replaced various illegal calls to DCOPY by calls to DLASET, or by DO
* loops. Sven Hammarling, 1/5/02.
*
* .. Parameters ..
*
*
* @param wantq
* @param wantz
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param q
* @param ldq
* @param z
* @param ldz
* @param j1
* @param n1
* @param n2
* @param work
* @param lwork
* @param info
*
*/
abstract public void dtgex2(boolean wantq, boolean wantz, int n, double[] a, int lda, double[] b, int ldb, double[] q, int ldq, double[] z, int ldz, int j1, int n1, int n2, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTGEX2 swaps adjacent diagonal blocks (A11, B11) and (A22, B22)
* of size 1-by-1 or 2-by-2 in an upper (quasi) triangular matrix pair
* (A, B) by an orthogonal equivalence transformation.
*
* (A, B) must be in generalized real Schur canonical form (as returned
* by DGGES), i.e. A is block upper triangular with 1-by-1 and 2-by-2
* diagonal blocks. B is upper triangular.
*
* Optionally, the matrices Q and Z of generalized Schur vectors are
* updated.
*
* Q(in) * A(in) * Z(in)' = Q(out) * A(out) * Z(out)'
* Q(in) * B(in) * Z(in)' = Q(out) * B(out) * Z(out)'
*
*
* Arguments
* =========
*
* WANTQ (input) LOGICAL
* .TRUE. : update the left transformation matrix Q;
* .FALSE.: do not update Q.
*
* WANTZ (input) LOGICAL
* .TRUE. : update the right transformation matrix Z;
* .FALSE.: do not update Z.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* A (input/output) DOUBLE PRECISION arrays, dimensions (LDA,N)
* On entry, the matrix A in the pair (A, B).
* On exit, the updated matrix A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION arrays, dimensions (LDB,N)
* On entry, the matrix B in the pair (A, B).
* On exit, the updated matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* Q (input/output) DOUBLE PRECISION array, dimension (LDZ,N)
* On entry, if WANTQ = .TRUE., the orthogonal matrix Q.
* On exit, the updated matrix Q.
* Not referenced if WANTQ = .FALSE..
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= 1.
* If WANTQ = .TRUE., LDQ >= N.
*
* Z (input/output) DOUBLE PRECISION array, dimension (LDZ,N)
* On entry, if WANTZ =.TRUE., the orthogonal matrix Z.
* On exit, the updated matrix Z.
* Not referenced if WANTZ = .FALSE..
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1.
* If WANTZ = .TRUE., LDZ >= N.
*
* J1 (input) INTEGER
* The index to the first block (A11, B11). 1 <= J1 <= N.
*
* N1 (input) INTEGER
* The order of the first block (A11, B11). N1 = 0, 1 or 2.
*
* N2 (input) INTEGER
* The order of the second block (A22, B22). N2 = 0, 1 or 2.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (MAX(1,LWORK)).
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* LWORK >= MAX( 1, N*(N2+N1), (N2+N1)*(N2+N1)*2 )
*
* INFO (output) INTEGER
* =0: Successful exit
* >0: If INFO = 1, the transformed matrix (A, B) would be
* too far from generalized Schur form; the blocks are
* not swapped and (A, B) and (Q, Z) are unchanged.
* The problem of swapping is too ill-conditioned.
* <0: If INFO = -16: LWORK is too small. Appropriate value
* for LWORK is returned in WORK(1).
*
* Further Details
* ===============
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* In the current code both weak and strong stability tests are
* performed. The user can omit the strong stability test by changing
* the internal logical parameter WANDS to .FALSE.. See ref. [2] for
* details.
*
* [1] B. Kagstrom; A Direct Method for Reordering Eigenvalues in the
* Generalized Real Schur Form of a Regular Matrix Pair (A, B), in
* M.S. Moonen et al (eds), Linear Algebra for Large Scale and
* Real-Time Applications, Kluwer Academic Publ. 1993, pp 195-218.
*
* [2] B. Kagstrom and P. Poromaa; Computing Eigenspaces with Specified
* Eigenvalues of a Regular Matrix Pair (A, B) and Condition
* Estimation: Theory, Algorithms and Software,
* Report UMINF - 94.04, Department of Computing Science, Umea
* University, S-901 87 Umea, Sweden, 1994. Also as LAPACK Working
* Note 87. To appear in Numerical Algorithms, 1996.
*
* =====================================================================
* Replaced various illegal calls to DCOPY by calls to DLASET, or by DO
* loops. Sven Hammarling, 1/5/02.
*
* .. Parameters ..
*
*
* @param wantq
* @param wantz
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param q
* @param _q_offset
* @param ldq
* @param z
* @param _z_offset
* @param ldz
* @param j1
* @param n1
* @param n2
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dtgex2(boolean wantq, boolean wantz, int n, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, double[] q, int _q_offset, int ldq, double[] z, int _z_offset, int ldz, int j1, int n1, int n2, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTGEXC reorders the generalized real Schur decomposition of a real
* matrix pair (A,B) using an orthogonal equivalence transformation
*
* (A, B) = Q * (A, B) * Z',
*
* so that the diagonal block of (A, B) with row index IFST is moved
* to row ILST.
*
* (A, B) must be in generalized real Schur canonical form (as returned
* by DGGES), i.e. A is block upper triangular with 1-by-1 and 2-by-2
* diagonal blocks. B is upper triangular.
*
* Optionally, the matrices Q and Z of generalized Schur vectors are
* updated.
*
* Q(in) * A(in) * Z(in)' = Q(out) * A(out) * Z(out)'
* Q(in) * B(in) * Z(in)' = Q(out) * B(out) * Z(out)'
*
*
* Arguments
* =========
*
* WANTQ (input) LOGICAL
* .TRUE. : update the left transformation matrix Q;
* .FALSE.: do not update Q.
*
* WANTZ (input) LOGICAL
* .TRUE. : update the right transformation matrix Z;
* .FALSE.: do not update Z.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the matrix A in generalized real Schur canonical
* form.
* On exit, the updated matrix A, again in generalized
* real Schur canonical form.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,N)
* On entry, the matrix B in generalized real Schur canonical
* form (A,B).
* On exit, the updated matrix B, again in generalized
* real Schur canonical form (A,B).
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* Q (input/output) DOUBLE PRECISION array, dimension (LDZ,N)
* On entry, if WANTQ = .TRUE., the orthogonal matrix Q.
* On exit, the updated matrix Q.
* If WANTQ = .FALSE., Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= 1.
* If WANTQ = .TRUE., LDQ >= N.
*
* Z (input/output) DOUBLE PRECISION array, dimension (LDZ,N)
* On entry, if WANTZ = .TRUE., the orthogonal matrix Z.
* On exit, the updated matrix Z.
* If WANTZ = .FALSE., Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1.
* If WANTZ = .TRUE., LDZ >= N.
*
* IFST (input/output) INTEGER
* ILST (input/output) INTEGER
* Specify the reordering of the diagonal blocks of (A, B).
* The block with row index IFST is moved to row ILST, by a
* sequence of swapping between adjacent blocks.
* On exit, if IFST pointed on entry to the second row of
* a 2-by-2 block, it is changed to point to the first row;
* ILST always points to the first row of the block in its
* final position (which may differ from its input value by
* +1 or -1). 1 <= IFST, ILST <= N.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* LWORK >= 1 when N <= 1, otherwise LWORK >= 4*N + 16.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* =0: successful exit.
* <0: if INFO = -i, the i-th argument had an illegal value.
* =1: The transformed matrix pair (A, B) would be too far
* from generalized Schur form; the problem is ill-
* conditioned. (A, B) may have been partially reordered,
* and ILST points to the first row of the current
* position of the block being moved.
*
* Further Details
* ===============
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* [1] B. Kagstrom; A Direct Method for Reordering Eigenvalues in the
* Generalized Real Schur Form of a Regular Matrix Pair (A, B), in
* M.S. Moonen et al (eds), Linear Algebra for Large Scale and
* Real-Time Applications, Kluwer Academic Publ. 1993, pp 195-218.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param wantq
* @param wantz
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param q
* @param ldq
* @param z
* @param ldz
* @param ifst
* @param ilst
* @param work
* @param lwork
* @param info
*
*/
abstract public void dtgexc(boolean wantq, boolean wantz, int n, double[] a, int lda, double[] b, int ldb, double[] q, int ldq, double[] z, int ldz, org.netlib.util.intW ifst, org.netlib.util.intW ilst, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTGEXC reorders the generalized real Schur decomposition of a real
* matrix pair (A,B) using an orthogonal equivalence transformation
*
* (A, B) = Q * (A, B) * Z',
*
* so that the diagonal block of (A, B) with row index IFST is moved
* to row ILST.
*
* (A, B) must be in generalized real Schur canonical form (as returned
* by DGGES), i.e. A is block upper triangular with 1-by-1 and 2-by-2
* diagonal blocks. B is upper triangular.
*
* Optionally, the matrices Q and Z of generalized Schur vectors are
* updated.
*
* Q(in) * A(in) * Z(in)' = Q(out) * A(out) * Z(out)'
* Q(in) * B(in) * Z(in)' = Q(out) * B(out) * Z(out)'
*
*
* Arguments
* =========
*
* WANTQ (input) LOGICAL
* .TRUE. : update the left transformation matrix Q;
* .FALSE.: do not update Q.
*
* WANTZ (input) LOGICAL
* .TRUE. : update the right transformation matrix Z;
* .FALSE.: do not update Z.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the matrix A in generalized real Schur canonical
* form.
* On exit, the updated matrix A, again in generalized
* real Schur canonical form.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,N)
* On entry, the matrix B in generalized real Schur canonical
* form (A,B).
* On exit, the updated matrix B, again in generalized
* real Schur canonical form (A,B).
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* Q (input/output) DOUBLE PRECISION array, dimension (LDZ,N)
* On entry, if WANTQ = .TRUE., the orthogonal matrix Q.
* On exit, the updated matrix Q.
* If WANTQ = .FALSE., Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= 1.
* If WANTQ = .TRUE., LDQ >= N.
*
* Z (input/output) DOUBLE PRECISION array, dimension (LDZ,N)
* On entry, if WANTZ = .TRUE., the orthogonal matrix Z.
* On exit, the updated matrix Z.
* If WANTZ = .FALSE., Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1.
* If WANTZ = .TRUE., LDZ >= N.
*
* IFST (input/output) INTEGER
* ILST (input/output) INTEGER
* Specify the reordering of the diagonal blocks of (A, B).
* The block with row index IFST is moved to row ILST, by a
* sequence of swapping between adjacent blocks.
* On exit, if IFST pointed on entry to the second row of
* a 2-by-2 block, it is changed to point to the first row;
* ILST always points to the first row of the block in its
* final position (which may differ from its input value by
* +1 or -1). 1 <= IFST, ILST <= N.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* LWORK >= 1 when N <= 1, otherwise LWORK >= 4*N + 16.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* =0: successful exit.
* <0: if INFO = -i, the i-th argument had an illegal value.
* =1: The transformed matrix pair (A, B) would be too far
* from generalized Schur form; the problem is ill-
* conditioned. (A, B) may have been partially reordered,
* and ILST points to the first row of the current
* position of the block being moved.
*
* Further Details
* ===============
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* [1] B. Kagstrom; A Direct Method for Reordering Eigenvalues in the
* Generalized Real Schur Form of a Regular Matrix Pair (A, B), in
* M.S. Moonen et al (eds), Linear Algebra for Large Scale and
* Real-Time Applications, Kluwer Academic Publ. 1993, pp 195-218.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param wantq
* @param wantz
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param q
* @param _q_offset
* @param ldq
* @param z
* @param _z_offset
* @param ldz
* @param ifst
* @param ilst
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dtgexc(boolean wantq, boolean wantz, int n, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, double[] q, int _q_offset, int ldq, double[] z, int _z_offset, int ldz, org.netlib.util.intW ifst, org.netlib.util.intW ilst, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTGSEN reorders the generalized real Schur decomposition of a real
* matrix pair (A, B) (in terms of an orthonormal equivalence trans-
* formation Q' * (A, B) * Z), so that a selected cluster of eigenvalues
* appears in the leading diagonal blocks of the upper quasi-triangular
* matrix A and the upper triangular B. The leading columns of Q and
* Z form orthonormal bases of the corresponding left and right eigen-
* spaces (deflating subspaces). (A, B) must be in generalized real
* Schur canonical form (as returned by DGGES), i.e. A is block upper
* triangular with 1-by-1 and 2-by-2 diagonal blocks. B is upper
* triangular.
*
* DTGSEN also computes the generalized eigenvalues
*
* w(j) = (ALPHAR(j) + i*ALPHAI(j))/BETA(j)
*
* of the reordered matrix pair (A, B).
*
* Optionally, DTGSEN computes the estimates of reciprocal condition
* numbers for eigenvalues and eigenspaces. These are Difu[(A11,B11),
* (A22,B22)] and Difl[(A11,B11), (A22,B22)], i.e. the separation(s)
* between the matrix pairs (A11, B11) and (A22,B22) that correspond to
* the selected cluster and the eigenvalues outside the cluster, resp.,
* and norms of "projections" onto left and right eigenspaces w.r.t.
* the selected cluster in the (1,1)-block.
*
* Arguments
* =========
*
* IJOB (input) INTEGER
* Specifies whether condition numbers are required for the
* cluster of eigenvalues (PL and PR) or the deflating subspaces
* (Difu and Difl):
* =0: Only reorder w.r.t. SELECT. No extras.
* =1: Reciprocal of norms of "projections" onto left and right
* eigenspaces w.r.t. the selected cluster (PL and PR).
* =2: Upper bounds on Difu and Difl. F-norm-based estimate
* (DIF(1:2)).
* =3: Estimate of Difu and Difl. 1-norm-based estimate
* (DIF(1:2)).
* About 5 times as expensive as IJOB = 2.
* =4: Compute PL, PR and DIF (i.e. 0, 1 and 2 above): Economic
* version to get it all.
* =5: Compute PL, PR and DIF (i.e. 0, 1 and 3 above)
*
* WANTQ (input) LOGICAL
* .TRUE. : update the left transformation matrix Q;
* .FALSE.: do not update Q.
*
* WANTZ (input) LOGICAL
* .TRUE. : update the right transformation matrix Z;
* .FALSE.: do not update Z.
*
* SELECT (input) LOGICAL array, dimension (N)
* SELECT specifies the eigenvalues in the selected cluster.
* To select a real eigenvalue w(j), SELECT(j) must be set to
* .TRUE.. To select a complex conjugate pair of eigenvalues
* w(j) and w(j+1), corresponding to a 2-by-2 diagonal block,
* either SELECT(j) or SELECT(j+1) or both must be set to
* .TRUE.; a complex conjugate pair of eigenvalues must be
* either both included in the cluster or both excluded.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension(LDA,N)
* On entry, the upper quasi-triangular matrix A, with (A, B) in
* generalized real Schur canonical form.
* On exit, A is overwritten by the reordered matrix A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension(LDB,N)
* On entry, the upper triangular matrix B, with (A, B) in
* generalized real Schur canonical form.
* On exit, B is overwritten by the reordered matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* ALPHAR (output) DOUBLE PRECISION array, dimension (N)
* ALPHAI (output) DOUBLE PRECISION array, dimension (N)
* BETA (output) DOUBLE PRECISION array, dimension (N)
* On exit, (ALPHAR(j) + ALPHAI(j)*i)/BETA(j), j=1,...,N, will
* be the generalized eigenvalues. ALPHAR(j) + ALPHAI(j)*i
* and BETA(j),j=1,...,N are the diagonals of the complex Schur
* form (S,T) that would result if the 2-by-2 diagonal blocks of
* the real generalized Schur form of (A,B) were further reduced
* to triangular form using complex unitary transformations.
* If ALPHAI(j) is zero, then the j-th eigenvalue is real; if
* positive, then the j-th and (j+1)-st eigenvalues are a
* complex conjugate pair, with ALPHAI(j+1) negative.
*
* Q (input/output) DOUBLE PRECISION array, dimension (LDQ,N)
* On entry, if WANTQ = .TRUE., Q is an N-by-N matrix.
* On exit, Q has been postmultiplied by the left orthogonal
* transformation matrix which reorder (A, B); The leading M
* columns of Q form orthonormal bases for the specified pair of
* left eigenspaces (deflating subspaces).
* If WANTQ = .FALSE., Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= 1;
* and if WANTQ = .TRUE., LDQ >= N.
*
* Z (input/output) DOUBLE PRECISION array, dimension (LDZ,N)
* On entry, if WANTZ = .TRUE., Z is an N-by-N matrix.
* On exit, Z has been postmultiplied by the left orthogonal
* transformation matrix which reorder (A, B); The leading M
* columns of Z form orthonormal bases for the specified pair of
* left eigenspaces (deflating subspaces).
* If WANTZ = .FALSE., Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1;
* If WANTZ = .TRUE., LDZ >= N.
*
* M (output) INTEGER
* The dimension of the specified pair of left and right eigen-
* spaces (deflating subspaces). 0 <= M <= N.
*
* PL (output) DOUBLE PRECISION
* PR (output) DOUBLE PRECISION
* If IJOB = 1, 4 or 5, PL, PR are lower bounds on the
* reciprocal of the norm of "projections" onto left and right
* eigenspaces with respect to the selected cluster.
* 0 < PL, PR <= 1.
* If M = 0 or M = N, PL = PR = 1.
* If IJOB = 0, 2 or 3, PL and PR are not referenced.
*
* DIF (output) DOUBLE PRECISION array, dimension (2).
* If IJOB >= 2, DIF(1:2) store the estimates of Difu and Difl.
* If IJOB = 2 or 4, DIF(1:2) are F-norm-based upper bounds on
* Difu and Difl. If IJOB = 3 or 5, DIF(1:2) are 1-norm-based
* estimates of Difu and Difl.
* If M = 0 or N, DIF(1:2) = F-norm([A, B]).
* If IJOB = 0 or 1, DIF is not referenced.
*
* WORK (workspace/output) DOUBLE PRECISION array,
* dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= 4*N+16.
* If IJOB = 1, 2 or 4, LWORK >= MAX(4*N+16, 2*M*(N-M)).
* If IJOB = 3 or 5, LWORK >= MAX(4*N+16, 4*M*(N-M)).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* IF IJOB = 0, IWORK is not referenced. Otherwise,
* on exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK. LIWORK >= 1.
* If IJOB = 1, 2 or 4, LIWORK >= N+6.
* If IJOB = 3 or 5, LIWORK >= MAX(2*M*(N-M), N+6).
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal size of the IWORK array,
* returns this value as the first entry of the IWORK array, and
* no error message related to LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* =0: Successful exit.
* <0: If INFO = -i, the i-th argument had an illegal value.
* =1: Reordering of (A, B) failed because the transformed
* matrix pair (A, B) would be too far from generalized
* Schur form; the problem is very ill-conditioned.
* (A, B) may have been partially reordered.
* If requested, 0 is returned in DIF(*), PL and PR.
*
* Further Details
* ===============
*
* DTGSEN first collects the selected eigenvalues by computing
* orthogonal U and W that move them to the top left corner of (A, B).
* In other words, the selected eigenvalues are the eigenvalues of
* (A11, B11) in:
*
* U'*(A, B)*W = (A11 A12) (B11 B12) n1
* ( 0 A22),( 0 B22) n2
* n1 n2 n1 n2
*
* where N = n1+n2 and U' means the transpose of U. The first n1 columns
* of U and W span the specified pair of left and right eigenspaces
* (deflating subspaces) of (A, B).
*
* If (A, B) has been obtained from the generalized real Schur
* decomposition of a matrix pair (C, D) = Q*(A, B)*Z', then the
* reordered generalized real Schur form of (C, D) is given by
*
* (C, D) = (Q*U)*(U'*(A, B)*W)*(Z*W)',
*
* and the first n1 columns of Q*U and Z*W span the corresponding
* deflating subspaces of (C, D) (Q and Z store Q*U and Z*W, resp.).
*
* Note that if the selected eigenvalue is sufficiently ill-conditioned,
* then its value may differ significantly from its value before
* reordering.
*
* The reciprocal condition numbers of the left and right eigenspaces
* spanned by the first n1 columns of U and W (or Q*U and Z*W) may
* be returned in DIF(1:2), corresponding to Difu and Difl, resp.
*
* The Difu and Difl are defined as:
*
* Difu[(A11, B11), (A22, B22)] = sigma-min( Zu )
* and
* Difl[(A11, B11), (A22, B22)] = Difu[(A22, B22), (A11, B11)],
*
* where sigma-min(Zu) is the smallest singular value of the
* (2*n1*n2)-by-(2*n1*n2) matrix
*
* Zu = [ kron(In2, A11) -kron(A22', In1) ]
* [ kron(In2, B11) -kron(B22', In1) ].
*
* Here, Inx is the identity matrix of size nx and A22' is the
* transpose of A22. kron(X, Y) is the Kronecker product between
* the matrices X and Y.
*
* When DIF(2) is small, small changes in (A, B) can cause large changes
* in the deflating subspace. An approximate (asymptotic) bound on the
* maximum angular error in the computed deflating subspaces is
*
* EPS * norm((A, B)) / DIF(2),
*
* where EPS is the machine precision.
*
* The reciprocal norm of the projectors on the left and right
* eigenspaces associated with (A11, B11) may be returned in PL and PR.
* They are computed as follows. First we compute L and R so that
* P*(A, B)*Q is block diagonal, where
*
* P = ( I -L ) n1 Q = ( I R ) n1
* ( 0 I ) n2 and ( 0 I ) n2
* n1 n2 n1 n2
*
* and (L, R) is the solution to the generalized Sylvester equation
*
* A11*R - L*A22 = -A12
* B11*R - L*B22 = -B12
*
* Then PL = (F-norm(L)**2+1)**(-1/2) and PR = (F-norm(R)**2+1)**(-1/2).
* An approximate (asymptotic) bound on the average absolute error of
* the selected eigenvalues is
*
* EPS * norm((A, B)) / PL.
*
* There are also global error bounds which valid for perturbations up
* to a certain restriction: A lower bound (x) on the smallest
* F-norm(E,F) for which an eigenvalue of (A11, B11) may move and
* coalesce with an eigenvalue of (A22, B22) under perturbation (E,F),
* (i.e. (A + E, B + F), is
*
* x = min(Difu,Difl)/((1/(PL*PL)+1/(PR*PR))**(1/2)+2*max(1/PL,1/PR)).
*
* An approximate bound on x can be computed from DIF(1:2), PL and PR.
*
* If y = ( F-norm(E,F) / x) <= 1, the angles between the perturbed
* (L', R') and unperturbed (L, R) left and right deflating subspaces
* associated with the selected cluster in the (1,1)-blocks can be
* bounded as
*
* max-angle(L, L') <= arctan( y * PL / (1 - y * (1 - PL * PL)**(1/2))
* max-angle(R, R') <= arctan( y * PR / (1 - y * (1 - PR * PR)**(1/2))
*
* See LAPACK User's Guide section 4.11 or the following references
* for more information.
*
* Note that if the default method for computing the Frobenius-norm-
* based estimate DIF is not wanted (see DLATDF), then the parameter
* IDIFJB (see below) should be changed from 3 to 4 (routine DLATDF
* (IJOB = 2 will be used)). See DTGSYL for more details.
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* References
* ==========
*
* [1] B. Kagstrom; A Direct Method for Reordering Eigenvalues in the
* Generalized Real Schur Form of a Regular Matrix Pair (A, B), in
* M.S. Moonen et al (eds), Linear Algebra for Large Scale and
* Real-Time Applications, Kluwer Academic Publ. 1993, pp 195-218.
*
* [2] B. Kagstrom and P. Poromaa; Computing Eigenspaces with Specified
* Eigenvalues of a Regular Matrix Pair (A, B) and Condition
* Estimation: Theory, Algorithms and Software,
* Report UMINF - 94.04, Department of Computing Science, Umea
* University, S-901 87 Umea, Sweden, 1994. Also as LAPACK Working
* Note 87. To appear in Numerical Algorithms, 1996.
*
* [3] B. Kagstrom and P. Poromaa, LAPACK-Style Algorithms and Software
* for Solving the Generalized Sylvester Equation and Estimating the
* Separation between Regular Matrix Pairs, Report UMINF - 93.23,
* Department of Computing Science, Umea University, S-901 87 Umea,
* Sweden, December 1993, Revised April 1994, Also as LAPACK Working
* Note 75. To appear in ACM Trans. on Math. Software, Vol 22, No 1,
* 1996.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param ijob
* @param wantq
* @param wantz
* @param select
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param alphar
* @param alphai
* @param beta
* @param q
* @param ldq
* @param z
* @param ldz
* @param m
* @param pl
* @param pr
* @param dif
* @param work
* @param lwork
* @param iwork
* @param liwork
* @param info
*
*/
abstract public void dtgsen(int ijob, boolean wantq, boolean wantz, boolean[] select, int n, double[] a, int lda, double[] b, int ldb, double[] alphar, double[] alphai, double[] beta, double[] q, int ldq, double[] z, int ldz, org.netlib.util.intW m, org.netlib.util.doubleW pl, org.netlib.util.doubleW pr, double[] dif, double[] work, int lwork, int[] iwork, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTGSEN reorders the generalized real Schur decomposition of a real
* matrix pair (A, B) (in terms of an orthonormal equivalence trans-
* formation Q' * (A, B) * Z), so that a selected cluster of eigenvalues
* appears in the leading diagonal blocks of the upper quasi-triangular
* matrix A and the upper triangular B. The leading columns of Q and
* Z form orthonormal bases of the corresponding left and right eigen-
* spaces (deflating subspaces). (A, B) must be in generalized real
* Schur canonical form (as returned by DGGES), i.e. A is block upper
* triangular with 1-by-1 and 2-by-2 diagonal blocks. B is upper
* triangular.
*
* DTGSEN also computes the generalized eigenvalues
*
* w(j) = (ALPHAR(j) + i*ALPHAI(j))/BETA(j)
*
* of the reordered matrix pair (A, B).
*
* Optionally, DTGSEN computes the estimates of reciprocal condition
* numbers for eigenvalues and eigenspaces. These are Difu[(A11,B11),
* (A22,B22)] and Difl[(A11,B11), (A22,B22)], i.e. the separation(s)
* between the matrix pairs (A11, B11) and (A22,B22) that correspond to
* the selected cluster and the eigenvalues outside the cluster, resp.,
* and norms of "projections" onto left and right eigenspaces w.r.t.
* the selected cluster in the (1,1)-block.
*
* Arguments
* =========
*
* IJOB (input) INTEGER
* Specifies whether condition numbers are required for the
* cluster of eigenvalues (PL and PR) or the deflating subspaces
* (Difu and Difl):
* =0: Only reorder w.r.t. SELECT. No extras.
* =1: Reciprocal of norms of "projections" onto left and right
* eigenspaces w.r.t. the selected cluster (PL and PR).
* =2: Upper bounds on Difu and Difl. F-norm-based estimate
* (DIF(1:2)).
* =3: Estimate of Difu and Difl. 1-norm-based estimate
* (DIF(1:2)).
* About 5 times as expensive as IJOB = 2.
* =4: Compute PL, PR and DIF (i.e. 0, 1 and 2 above): Economic
* version to get it all.
* =5: Compute PL, PR and DIF (i.e. 0, 1 and 3 above)
*
* WANTQ (input) LOGICAL
* .TRUE. : update the left transformation matrix Q;
* .FALSE.: do not update Q.
*
* WANTZ (input) LOGICAL
* .TRUE. : update the right transformation matrix Z;
* .FALSE.: do not update Z.
*
* SELECT (input) LOGICAL array, dimension (N)
* SELECT specifies the eigenvalues in the selected cluster.
* To select a real eigenvalue w(j), SELECT(j) must be set to
* .TRUE.. To select a complex conjugate pair of eigenvalues
* w(j) and w(j+1), corresponding to a 2-by-2 diagonal block,
* either SELECT(j) or SELECT(j+1) or both must be set to
* .TRUE.; a complex conjugate pair of eigenvalues must be
* either both included in the cluster or both excluded.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension(LDA,N)
* On entry, the upper quasi-triangular matrix A, with (A, B) in
* generalized real Schur canonical form.
* On exit, A is overwritten by the reordered matrix A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension(LDB,N)
* On entry, the upper triangular matrix B, with (A, B) in
* generalized real Schur canonical form.
* On exit, B is overwritten by the reordered matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* ALPHAR (output) DOUBLE PRECISION array, dimension (N)
* ALPHAI (output) DOUBLE PRECISION array, dimension (N)
* BETA (output) DOUBLE PRECISION array, dimension (N)
* On exit, (ALPHAR(j) + ALPHAI(j)*i)/BETA(j), j=1,...,N, will
* be the generalized eigenvalues. ALPHAR(j) + ALPHAI(j)*i
* and BETA(j),j=1,...,N are the diagonals of the complex Schur
* form (S,T) that would result if the 2-by-2 diagonal blocks of
* the real generalized Schur form of (A,B) were further reduced
* to triangular form using complex unitary transformations.
* If ALPHAI(j) is zero, then the j-th eigenvalue is real; if
* positive, then the j-th and (j+1)-st eigenvalues are a
* complex conjugate pair, with ALPHAI(j+1) negative.
*
* Q (input/output) DOUBLE PRECISION array, dimension (LDQ,N)
* On entry, if WANTQ = .TRUE., Q is an N-by-N matrix.
* On exit, Q has been postmultiplied by the left orthogonal
* transformation matrix which reorder (A, B); The leading M
* columns of Q form orthonormal bases for the specified pair of
* left eigenspaces (deflating subspaces).
* If WANTQ = .FALSE., Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= 1;
* and if WANTQ = .TRUE., LDQ >= N.
*
* Z (input/output) DOUBLE PRECISION array, dimension (LDZ,N)
* On entry, if WANTZ = .TRUE., Z is an N-by-N matrix.
* On exit, Z has been postmultiplied by the left orthogonal
* transformation matrix which reorder (A, B); The leading M
* columns of Z form orthonormal bases for the specified pair of
* left eigenspaces (deflating subspaces).
* If WANTZ = .FALSE., Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1;
* If WANTZ = .TRUE., LDZ >= N.
*
* M (output) INTEGER
* The dimension of the specified pair of left and right eigen-
* spaces (deflating subspaces). 0 <= M <= N.
*
* PL (output) DOUBLE PRECISION
* PR (output) DOUBLE PRECISION
* If IJOB = 1, 4 or 5, PL, PR are lower bounds on the
* reciprocal of the norm of "projections" onto left and right
* eigenspaces with respect to the selected cluster.
* 0 < PL, PR <= 1.
* If M = 0 or M = N, PL = PR = 1.
* If IJOB = 0, 2 or 3, PL and PR are not referenced.
*
* DIF (output) DOUBLE PRECISION array, dimension (2).
* If IJOB >= 2, DIF(1:2) store the estimates of Difu and Difl.
* If IJOB = 2 or 4, DIF(1:2) are F-norm-based upper bounds on
* Difu and Difl. If IJOB = 3 or 5, DIF(1:2) are 1-norm-based
* estimates of Difu and Difl.
* If M = 0 or N, DIF(1:2) = F-norm([A, B]).
* If IJOB = 0 or 1, DIF is not referenced.
*
* WORK (workspace/output) DOUBLE PRECISION array,
* dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= 4*N+16.
* If IJOB = 1, 2 or 4, LWORK >= MAX(4*N+16, 2*M*(N-M)).
* If IJOB = 3 or 5, LWORK >= MAX(4*N+16, 4*M*(N-M)).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* IF IJOB = 0, IWORK is not referenced. Otherwise,
* on exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK. LIWORK >= 1.
* If IJOB = 1, 2 or 4, LIWORK >= N+6.
* If IJOB = 3 or 5, LIWORK >= MAX(2*M*(N-M), N+6).
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal size of the IWORK array,
* returns this value as the first entry of the IWORK array, and
* no error message related to LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* =0: Successful exit.
* <0: If INFO = -i, the i-th argument had an illegal value.
* =1: Reordering of (A, B) failed because the transformed
* matrix pair (A, B) would be too far from generalized
* Schur form; the problem is very ill-conditioned.
* (A, B) may have been partially reordered.
* If requested, 0 is returned in DIF(*), PL and PR.
*
* Further Details
* ===============
*
* DTGSEN first collects the selected eigenvalues by computing
* orthogonal U and W that move them to the top left corner of (A, B).
* In other words, the selected eigenvalues are the eigenvalues of
* (A11, B11) in:
*
* U'*(A, B)*W = (A11 A12) (B11 B12) n1
* ( 0 A22),( 0 B22) n2
* n1 n2 n1 n2
*
* where N = n1+n2 and U' means the transpose of U. The first n1 columns
* of U and W span the specified pair of left and right eigenspaces
* (deflating subspaces) of (A, B).
*
* If (A, B) has been obtained from the generalized real Schur
* decomposition of a matrix pair (C, D) = Q*(A, B)*Z', then the
* reordered generalized real Schur form of (C, D) is given by
*
* (C, D) = (Q*U)*(U'*(A, B)*W)*(Z*W)',
*
* and the first n1 columns of Q*U and Z*W span the corresponding
* deflating subspaces of (C, D) (Q and Z store Q*U and Z*W, resp.).
*
* Note that if the selected eigenvalue is sufficiently ill-conditioned,
* then its value may differ significantly from its value before
* reordering.
*
* The reciprocal condition numbers of the left and right eigenspaces
* spanned by the first n1 columns of U and W (or Q*U and Z*W) may
* be returned in DIF(1:2), corresponding to Difu and Difl, resp.
*
* The Difu and Difl are defined as:
*
* Difu[(A11, B11), (A22, B22)] = sigma-min( Zu )
* and
* Difl[(A11, B11), (A22, B22)] = Difu[(A22, B22), (A11, B11)],
*
* where sigma-min(Zu) is the smallest singular value of the
* (2*n1*n2)-by-(2*n1*n2) matrix
*
* Zu = [ kron(In2, A11) -kron(A22', In1) ]
* [ kron(In2, B11) -kron(B22', In1) ].
*
* Here, Inx is the identity matrix of size nx and A22' is the
* transpose of A22. kron(X, Y) is the Kronecker product between
* the matrices X and Y.
*
* When DIF(2) is small, small changes in (A, B) can cause large changes
* in the deflating subspace. An approximate (asymptotic) bound on the
* maximum angular error in the computed deflating subspaces is
*
* EPS * norm((A, B)) / DIF(2),
*
* where EPS is the machine precision.
*
* The reciprocal norm of the projectors on the left and right
* eigenspaces associated with (A11, B11) may be returned in PL and PR.
* They are computed as follows. First we compute L and R so that
* P*(A, B)*Q is block diagonal, where
*
* P = ( I -L ) n1 Q = ( I R ) n1
* ( 0 I ) n2 and ( 0 I ) n2
* n1 n2 n1 n2
*
* and (L, R) is the solution to the generalized Sylvester equation
*
* A11*R - L*A22 = -A12
* B11*R - L*B22 = -B12
*
* Then PL = (F-norm(L)**2+1)**(-1/2) and PR = (F-norm(R)**2+1)**(-1/2).
* An approximate (asymptotic) bound on the average absolute error of
* the selected eigenvalues is
*
* EPS * norm((A, B)) / PL.
*
* There are also global error bounds which valid for perturbations up
* to a certain restriction: A lower bound (x) on the smallest
* F-norm(E,F) for which an eigenvalue of (A11, B11) may move and
* coalesce with an eigenvalue of (A22, B22) under perturbation (E,F),
* (i.e. (A + E, B + F), is
*
* x = min(Difu,Difl)/((1/(PL*PL)+1/(PR*PR))**(1/2)+2*max(1/PL,1/PR)).
*
* An approximate bound on x can be computed from DIF(1:2), PL and PR.
*
* If y = ( F-norm(E,F) / x) <= 1, the angles between the perturbed
* (L', R') and unperturbed (L, R) left and right deflating subspaces
* associated with the selected cluster in the (1,1)-blocks can be
* bounded as
*
* max-angle(L, L') <= arctan( y * PL / (1 - y * (1 - PL * PL)**(1/2))
* max-angle(R, R') <= arctan( y * PR / (1 - y * (1 - PR * PR)**(1/2))
*
* See LAPACK User's Guide section 4.11 or the following references
* for more information.
*
* Note that if the default method for computing the Frobenius-norm-
* based estimate DIF is not wanted (see DLATDF), then the parameter
* IDIFJB (see below) should be changed from 3 to 4 (routine DLATDF
* (IJOB = 2 will be used)). See DTGSYL for more details.
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* References
* ==========
*
* [1] B. Kagstrom; A Direct Method for Reordering Eigenvalues in the
* Generalized Real Schur Form of a Regular Matrix Pair (A, B), in
* M.S. Moonen et al (eds), Linear Algebra for Large Scale and
* Real-Time Applications, Kluwer Academic Publ. 1993, pp 195-218.
*
* [2] B. Kagstrom and P. Poromaa; Computing Eigenspaces with Specified
* Eigenvalues of a Regular Matrix Pair (A, B) and Condition
* Estimation: Theory, Algorithms and Software,
* Report UMINF - 94.04, Department of Computing Science, Umea
* University, S-901 87 Umea, Sweden, 1994. Also as LAPACK Working
* Note 87. To appear in Numerical Algorithms, 1996.
*
* [3] B. Kagstrom and P. Poromaa, LAPACK-Style Algorithms and Software
* for Solving the Generalized Sylvester Equation and Estimating the
* Separation between Regular Matrix Pairs, Report UMINF - 93.23,
* Department of Computing Science, Umea University, S-901 87 Umea,
* Sweden, December 1993, Revised April 1994, Also as LAPACK Working
* Note 75. To appear in ACM Trans. on Math. Software, Vol 22, No 1,
* 1996.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param ijob
* @param wantq
* @param wantz
* @param select
* @param _select_offset
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param alphar
* @param _alphar_offset
* @param alphai
* @param _alphai_offset
* @param beta
* @param _beta_offset
* @param q
* @param _q_offset
* @param ldq
* @param z
* @param _z_offset
* @param ldz
* @param m
* @param pl
* @param pr
* @param dif
* @param _dif_offset
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param liwork
* @param info
*
*/
abstract public void dtgsen(int ijob, boolean wantq, boolean wantz, boolean[] select, int _select_offset, int n, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, double[] alphar, int _alphar_offset, double[] alphai, int _alphai_offset, double[] beta, int _beta_offset, double[] q, int _q_offset, int ldq, double[] z, int _z_offset, int ldz, org.netlib.util.intW m, org.netlib.util.doubleW pl, org.netlib.util.doubleW pr, double[] dif, int _dif_offset, double[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTGSJA computes the generalized singular value decomposition (GSVD)
* of two real upper triangular (or trapezoidal) matrices A and B.
*
* On entry, it is assumed that matrices A and B have the following
* forms, which may be obtained by the preprocessing subroutine DGGSVP
* from a general M-by-N matrix A and P-by-N matrix B:
*
* N-K-L K L
* A = K ( 0 A12 A13 ) if M-K-L >= 0;
* L ( 0 0 A23 )
* M-K-L ( 0 0 0 )
*
* N-K-L K L
* A = K ( 0 A12 A13 ) if M-K-L < 0;
* M-K ( 0 0 A23 )
*
* N-K-L K L
* B = L ( 0 0 B13 )
* P-L ( 0 0 0 )
*
* where the K-by-K matrix A12 and L-by-L matrix B13 are nonsingular
* upper triangular; A23 is L-by-L upper triangular if M-K-L >= 0,
* otherwise A23 is (M-K)-by-L upper trapezoidal.
*
* On exit,
*
* U'*A*Q = D1*( 0 R ), V'*B*Q = D2*( 0 R ),
*
* where U, V and Q are orthogonal matrices, Z' denotes the transpose
* of Z, R is a nonsingular upper triangular matrix, and D1 and D2 are
* ``diagonal'' matrices, which are of the following structures:
*
* If M-K-L >= 0,
*
* K L
* D1 = K ( I 0 )
* L ( 0 C )
* M-K-L ( 0 0 )
*
* K L
* D2 = L ( 0 S )
* P-L ( 0 0 )
*
* N-K-L K L
* ( 0 R ) = K ( 0 R11 R12 ) K
* L ( 0 0 R22 ) L
*
* where
*
* C = diag( ALPHA(K+1), ... , ALPHA(K+L) ),
* S = diag( BETA(K+1), ... , BETA(K+L) ),
* C**2 + S**2 = I.
*
* R is stored in A(1:K+L,N-K-L+1:N) on exit.
*
* If M-K-L < 0,
*
* K M-K K+L-M
* D1 = K ( I 0 0 )
* M-K ( 0 C 0 )
*
* K M-K K+L-M
* D2 = M-K ( 0 S 0 )
* K+L-M ( 0 0 I )
* P-L ( 0 0 0 )
*
* N-K-L K M-K K+L-M
* ( 0 R ) = K ( 0 R11 R12 R13 )
* M-K ( 0 0 R22 R23 )
* K+L-M ( 0 0 0 R33 )
*
* where
* C = diag( ALPHA(K+1), ... , ALPHA(M) ),
* S = diag( BETA(K+1), ... , BETA(M) ),
* C**2 + S**2 = I.
*
* R = ( R11 R12 R13 ) is stored in A(1:M, N-K-L+1:N) and R33 is stored
* ( 0 R22 R23 )
* in B(M-K+1:L,N+M-K-L+1:N) on exit.
*
* The computation of the orthogonal transformation matrices U, V or Q
* is optional. These matrices may either be formed explicitly, or they
* may be postmultiplied into input matrices U1, V1, or Q1.
*
* Arguments
* =========
*
* JOBU (input) CHARACTER*1
* = 'U': U must contain an orthogonal matrix U1 on entry, and
* the product U1*U is returned;
* = 'I': U is initialized to the unit matrix, and the
* orthogonal matrix U is returned;
* = 'N': U is not computed.
*
* JOBV (input) CHARACTER*1
* = 'V': V must contain an orthogonal matrix V1 on entry, and
* the product V1*V is returned;
* = 'I': V is initialized to the unit matrix, and the
* orthogonal matrix V is returned;
* = 'N': V is not computed.
*
* JOBQ (input) CHARACTER*1
* = 'Q': Q must contain an orthogonal matrix Q1 on entry, and
* the product Q1*Q is returned;
* = 'I': Q is initialized to the unit matrix, and the
* orthogonal matrix Q is returned;
* = 'N': Q is not computed.
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* P (input) INTEGER
* The number of rows of the matrix B. P >= 0.
*
* N (input) INTEGER
* The number of columns of the matrices A and B. N >= 0.
*
* K (input) INTEGER
* L (input) INTEGER
* K and L specify the subblocks in the input matrices A and B:
* A23 = A(K+1:MIN(K+L,M),N-L+1:N) and B13 = B(1:L,N-L+1:N)
* of A and B, whose GSVD is going to be computed by DTGSJA.
* See Further details.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, A(N-K+1:N,1:MIN(K+L,M) ) contains the triangular
* matrix R or part of R. See Purpose for details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,N)
* On entry, the P-by-N matrix B.
* On exit, if necessary, B(M-K+1:L,N+M-K-L+1:N) contains
* a part of R. See Purpose for details.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,P).
*
* TOLA (input) DOUBLE PRECISION
* TOLB (input) DOUBLE PRECISION
* TOLA and TOLB are the convergence criteria for the Jacobi-
* Kogbetliantz iteration procedure. Generally, they are the
* same as used in the preprocessing step, say
* TOLA = max(M,N)*norm(A)*MAZHEPS,
* TOLB = max(P,N)*norm(B)*MAZHEPS.
*
* ALPHA (output) DOUBLE PRECISION array, dimension (N)
* BETA (output) DOUBLE PRECISION array, dimension (N)
* On exit, ALPHA and BETA contain the generalized singular
* value pairs of A and B;
* ALPHA(1:K) = 1,
* BETA(1:K) = 0,
* and if M-K-L >= 0,
* ALPHA(K+1:K+L) = diag(C),
* BETA(K+1:K+L) = diag(S),
* or if M-K-L < 0,
* ALPHA(K+1:M)= C, ALPHA(M+1:K+L)= 0
* BETA(K+1:M) = S, BETA(M+1:K+L) = 1.
* Furthermore, if K+L < N,
* ALPHA(K+L+1:N) = 0 and
* BETA(K+L+1:N) = 0.
*
* U (input/output) DOUBLE PRECISION array, dimension (LDU,M)
* On entry, if JOBU = 'U', U must contain a matrix U1 (usually
* the orthogonal matrix returned by DGGSVP).
* On exit,
* if JOBU = 'I', U contains the orthogonal matrix U;
* if JOBU = 'U', U contains the product U1*U.
* If JOBU = 'N', U is not referenced.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= max(1,M) if
* JOBU = 'U'; LDU >= 1 otherwise.
*
* V (input/output) DOUBLE PRECISION array, dimension (LDV,P)
* On entry, if JOBV = 'V', V must contain a matrix V1 (usually
* the orthogonal matrix returned by DGGSVP).
* On exit,
* if JOBV = 'I', V contains the orthogonal matrix V;
* if JOBV = 'V', V contains the product V1*V.
* If JOBV = 'N', V is not referenced.
*
* LDV (input) INTEGER
* The leading dimension of the array V. LDV >= max(1,P) if
* JOBV = 'V'; LDV >= 1 otherwise.
*
* Q (input/output) DOUBLE PRECISION array, dimension (LDQ,N)
* On entry, if JOBQ = 'Q', Q must contain a matrix Q1 (usually
* the orthogonal matrix returned by DGGSVP).
* On exit,
* if JOBQ = 'I', Q contains the orthogonal matrix Q;
* if JOBQ = 'Q', Q contains the product Q1*Q.
* If JOBQ = 'N', Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N) if
* JOBQ = 'Q'; LDQ >= 1 otherwise.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (2*N)
*
* NCYCLE (output) INTEGER
* The number of cycles required for convergence.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1: the procedure does not converge after MAXIT cycles.
*
* Internal Parameters
* ===================
*
* MAXIT INTEGER
* MAXIT specifies the total loops that the iterative procedure
* may take. If after MAXIT cycles, the routine fails to
* converge, we return INFO = 1.
*
* Further Details
* ===============
*
* DTGSJA essentially uses a variant of Kogbetliantz algorithm to reduce
* min(L,M-K)-by-L triangular (or trapezoidal) matrix A23 and L-by-L
* matrix B13 to the form:
*
* U1'*A13*Q1 = C1*R1; V1'*B13*Q1 = S1*R1,
*
* where U1, V1 and Q1 are orthogonal matrix, and Z' is the transpose
* of Z. C1 and S1 are diagonal matrices satisfying
*
* C1**2 + S1**2 = I,
*
* and R1 is an L-by-L nonsingular upper triangular matrix.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobu
* @param jobv
* @param jobq
* @param m
* @param p
* @param n
* @param k
* @param l
* @param a
* @param lda
* @param b
* @param ldb
* @param tola
* @param tolb
* @param alpha
* @param beta
* @param u
* @param ldu
* @param v
* @param ldv
* @param q
* @param ldq
* @param work
* @param ncycle
* @param info
*
*/
abstract public void dtgsja(java.lang.String jobu, java.lang.String jobv, java.lang.String jobq, int m, int p, int n, int k, int l, double[] a, int lda, double[] b, int ldb, double tola, double tolb, double[] alpha, double[] beta, double[] u, int ldu, double[] v, int ldv, double[] q, int ldq, double[] work, org.netlib.util.intW ncycle, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTGSJA computes the generalized singular value decomposition (GSVD)
* of two real upper triangular (or trapezoidal) matrices A and B.
*
* On entry, it is assumed that matrices A and B have the following
* forms, which may be obtained by the preprocessing subroutine DGGSVP
* from a general M-by-N matrix A and P-by-N matrix B:
*
* N-K-L K L
* A = K ( 0 A12 A13 ) if M-K-L >= 0;
* L ( 0 0 A23 )
* M-K-L ( 0 0 0 )
*
* N-K-L K L
* A = K ( 0 A12 A13 ) if M-K-L < 0;
* M-K ( 0 0 A23 )
*
* N-K-L K L
* B = L ( 0 0 B13 )
* P-L ( 0 0 0 )
*
* where the K-by-K matrix A12 and L-by-L matrix B13 are nonsingular
* upper triangular; A23 is L-by-L upper triangular if M-K-L >= 0,
* otherwise A23 is (M-K)-by-L upper trapezoidal.
*
* On exit,
*
* U'*A*Q = D1*( 0 R ), V'*B*Q = D2*( 0 R ),
*
* where U, V and Q are orthogonal matrices, Z' denotes the transpose
* of Z, R is a nonsingular upper triangular matrix, and D1 and D2 are
* ``diagonal'' matrices, which are of the following structures:
*
* If M-K-L >= 0,
*
* K L
* D1 = K ( I 0 )
* L ( 0 C )
* M-K-L ( 0 0 )
*
* K L
* D2 = L ( 0 S )
* P-L ( 0 0 )
*
* N-K-L K L
* ( 0 R ) = K ( 0 R11 R12 ) K
* L ( 0 0 R22 ) L
*
* where
*
* C = diag( ALPHA(K+1), ... , ALPHA(K+L) ),
* S = diag( BETA(K+1), ... , BETA(K+L) ),
* C**2 + S**2 = I.
*
* R is stored in A(1:K+L,N-K-L+1:N) on exit.
*
* If M-K-L < 0,
*
* K M-K K+L-M
* D1 = K ( I 0 0 )
* M-K ( 0 C 0 )
*
* K M-K K+L-M
* D2 = M-K ( 0 S 0 )
* K+L-M ( 0 0 I )
* P-L ( 0 0 0 )
*
* N-K-L K M-K K+L-M
* ( 0 R ) = K ( 0 R11 R12 R13 )
* M-K ( 0 0 R22 R23 )
* K+L-M ( 0 0 0 R33 )
*
* where
* C = diag( ALPHA(K+1), ... , ALPHA(M) ),
* S = diag( BETA(K+1), ... , BETA(M) ),
* C**2 + S**2 = I.
*
* R = ( R11 R12 R13 ) is stored in A(1:M, N-K-L+1:N) and R33 is stored
* ( 0 R22 R23 )
* in B(M-K+1:L,N+M-K-L+1:N) on exit.
*
* The computation of the orthogonal transformation matrices U, V or Q
* is optional. These matrices may either be formed explicitly, or they
* may be postmultiplied into input matrices U1, V1, or Q1.
*
* Arguments
* =========
*
* JOBU (input) CHARACTER*1
* = 'U': U must contain an orthogonal matrix U1 on entry, and
* the product U1*U is returned;
* = 'I': U is initialized to the unit matrix, and the
* orthogonal matrix U is returned;
* = 'N': U is not computed.
*
* JOBV (input) CHARACTER*1
* = 'V': V must contain an orthogonal matrix V1 on entry, and
* the product V1*V is returned;
* = 'I': V is initialized to the unit matrix, and the
* orthogonal matrix V is returned;
* = 'N': V is not computed.
*
* JOBQ (input) CHARACTER*1
* = 'Q': Q must contain an orthogonal matrix Q1 on entry, and
* the product Q1*Q is returned;
* = 'I': Q is initialized to the unit matrix, and the
* orthogonal matrix Q is returned;
* = 'N': Q is not computed.
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* P (input) INTEGER
* The number of rows of the matrix B. P >= 0.
*
* N (input) INTEGER
* The number of columns of the matrices A and B. N >= 0.
*
* K (input) INTEGER
* L (input) INTEGER
* K and L specify the subblocks in the input matrices A and B:
* A23 = A(K+1:MIN(K+L,M),N-L+1:N) and B13 = B(1:L,N-L+1:N)
* of A and B, whose GSVD is going to be computed by DTGSJA.
* See Further details.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, A(N-K+1:N,1:MIN(K+L,M) ) contains the triangular
* matrix R or part of R. See Purpose for details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,N)
* On entry, the P-by-N matrix B.
* On exit, if necessary, B(M-K+1:L,N+M-K-L+1:N) contains
* a part of R. See Purpose for details.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,P).
*
* TOLA (input) DOUBLE PRECISION
* TOLB (input) DOUBLE PRECISION
* TOLA and TOLB are the convergence criteria for the Jacobi-
* Kogbetliantz iteration procedure. Generally, they are the
* same as used in the preprocessing step, say
* TOLA = max(M,N)*norm(A)*MAZHEPS,
* TOLB = max(P,N)*norm(B)*MAZHEPS.
*
* ALPHA (output) DOUBLE PRECISION array, dimension (N)
* BETA (output) DOUBLE PRECISION array, dimension (N)
* On exit, ALPHA and BETA contain the generalized singular
* value pairs of A and B;
* ALPHA(1:K) = 1,
* BETA(1:K) = 0,
* and if M-K-L >= 0,
* ALPHA(K+1:K+L) = diag(C),
* BETA(K+1:K+L) = diag(S),
* or if M-K-L < 0,
* ALPHA(K+1:M)= C, ALPHA(M+1:K+L)= 0
* BETA(K+1:M) = S, BETA(M+1:K+L) = 1.
* Furthermore, if K+L < N,
* ALPHA(K+L+1:N) = 0 and
* BETA(K+L+1:N) = 0.
*
* U (input/output) DOUBLE PRECISION array, dimension (LDU,M)
* On entry, if JOBU = 'U', U must contain a matrix U1 (usually
* the orthogonal matrix returned by DGGSVP).
* On exit,
* if JOBU = 'I', U contains the orthogonal matrix U;
* if JOBU = 'U', U contains the product U1*U.
* If JOBU = 'N', U is not referenced.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= max(1,M) if
* JOBU = 'U'; LDU >= 1 otherwise.
*
* V (input/output) DOUBLE PRECISION array, dimension (LDV,P)
* On entry, if JOBV = 'V', V must contain a matrix V1 (usually
* the orthogonal matrix returned by DGGSVP).
* On exit,
* if JOBV = 'I', V contains the orthogonal matrix V;
* if JOBV = 'V', V contains the product V1*V.
* If JOBV = 'N', V is not referenced.
*
* LDV (input) INTEGER
* The leading dimension of the array V. LDV >= max(1,P) if
* JOBV = 'V'; LDV >= 1 otherwise.
*
* Q (input/output) DOUBLE PRECISION array, dimension (LDQ,N)
* On entry, if JOBQ = 'Q', Q must contain a matrix Q1 (usually
* the orthogonal matrix returned by DGGSVP).
* On exit,
* if JOBQ = 'I', Q contains the orthogonal matrix Q;
* if JOBQ = 'Q', Q contains the product Q1*Q.
* If JOBQ = 'N', Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N) if
* JOBQ = 'Q'; LDQ >= 1 otherwise.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (2*N)
*
* NCYCLE (output) INTEGER
* The number of cycles required for convergence.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1: the procedure does not converge after MAXIT cycles.
*
* Internal Parameters
* ===================
*
* MAXIT INTEGER
* MAXIT specifies the total loops that the iterative procedure
* may take. If after MAXIT cycles, the routine fails to
* converge, we return INFO = 1.
*
* Further Details
* ===============
*
* DTGSJA essentially uses a variant of Kogbetliantz algorithm to reduce
* min(L,M-K)-by-L triangular (or trapezoidal) matrix A23 and L-by-L
* matrix B13 to the form:
*
* U1'*A13*Q1 = C1*R1; V1'*B13*Q1 = S1*R1,
*
* where U1, V1 and Q1 are orthogonal matrix, and Z' is the transpose
* of Z. C1 and S1 are diagonal matrices satisfying
*
* C1**2 + S1**2 = I,
*
* and R1 is an L-by-L nonsingular upper triangular matrix.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobu
* @param jobv
* @param jobq
* @param m
* @param p
* @param n
* @param k
* @param l
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param tola
* @param tolb
* @param alpha
* @param _alpha_offset
* @param beta
* @param _beta_offset
* @param u
* @param _u_offset
* @param ldu
* @param v
* @param _v_offset
* @param ldv
* @param q
* @param _q_offset
* @param ldq
* @param work
* @param _work_offset
* @param ncycle
* @param info
*
*/
abstract public void dtgsja(java.lang.String jobu, java.lang.String jobv, java.lang.String jobq, int m, int p, int n, int k, int l, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, double tola, double tolb, double[] alpha, int _alpha_offset, double[] beta, int _beta_offset, double[] u, int _u_offset, int ldu, double[] v, int _v_offset, int ldv, double[] q, int _q_offset, int ldq, double[] work, int _work_offset, org.netlib.util.intW ncycle, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTGSNA estimates reciprocal condition numbers for specified
* eigenvalues and/or eigenvectors of a matrix pair (A, B) in
* generalized real Schur canonical form (or of any matrix pair
* (Q*A*Z', Q*B*Z') with orthogonal matrices Q and Z, where
* Z' denotes the transpose of Z.
*
* (A, B) must be in generalized real Schur form (as returned by DGGES),
* i.e. A is block upper triangular with 1-by-1 and 2-by-2 diagonal
* blocks. B is upper triangular.
*
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* Specifies whether condition numbers are required for
* eigenvalues (S) or eigenvectors (DIF):
* = 'E': for eigenvalues only (S);
* = 'V': for eigenvectors only (DIF);
* = 'B': for both eigenvalues and eigenvectors (S and DIF).
*
* HOWMNY (input) CHARACTER*1
* = 'A': compute condition numbers for all eigenpairs;
* = 'S': compute condition numbers for selected eigenpairs
* specified by the array SELECT.
*
* SELECT (input) LOGICAL array, dimension (N)
* If HOWMNY = 'S', SELECT specifies the eigenpairs for which
* condition numbers are required. To select condition numbers
* for the eigenpair corresponding to a real eigenvalue w(j),
* SELECT(j) must be set to .TRUE.. To select condition numbers
* corresponding to a complex conjugate pair of eigenvalues w(j)
* and w(j+1), either SELECT(j) or SELECT(j+1) or both, must be
* set to .TRUE..
* If HOWMNY = 'A', SELECT is not referenced.
*
* N (input) INTEGER
* The order of the square matrix pair (A, B). N >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The upper quasi-triangular matrix A in the pair (A,B).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input) DOUBLE PRECISION array, dimension (LDB,N)
* The upper triangular matrix B in the pair (A,B).
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* VL (input) DOUBLE PRECISION array, dimension (LDVL,M)
* If JOB = 'E' or 'B', VL must contain left eigenvectors of
* (A, B), corresponding to the eigenpairs specified by HOWMNY
* and SELECT. The eigenvectors must be stored in consecutive
* columns of VL, as returned by DTGEVC.
* If JOB = 'V', VL is not referenced.
*
* LDVL (input) INTEGER
* The leading dimension of the array VL. LDVL >= 1.
* If JOB = 'E' or 'B', LDVL >= N.
*
* VR (input) DOUBLE PRECISION array, dimension (LDVR,M)
* If JOB = 'E' or 'B', VR must contain right eigenvectors of
* (A, B), corresponding to the eigenpairs specified by HOWMNY
* and SELECT. The eigenvectors must be stored in consecutive
* columns ov VR, as returned by DTGEVC.
* If JOB = 'V', VR is not referenced.
*
* LDVR (input) INTEGER
* The leading dimension of the array VR. LDVR >= 1.
* If JOB = 'E' or 'B', LDVR >= N.
*
* S (output) DOUBLE PRECISION array, dimension (MM)
* If JOB = 'E' or 'B', the reciprocal condition numbers of the
* selected eigenvalues, stored in consecutive elements of the
* array. For a complex conjugate pair of eigenvalues two
* consecutive elements of S are set to the same value. Thus
* S(j), DIF(j), and the j-th columns of VL and VR all
* correspond to the same eigenpair (but not in general the
* j-th eigenpair, unless all eigenpairs are selected).
* If JOB = 'V', S is not referenced.
*
* DIF (output) DOUBLE PRECISION array, dimension (MM)
* If JOB = 'V' or 'B', the estimated reciprocal condition
* numbers of the selected eigenvectors, stored in consecutive
* elements of the array. For a complex eigenvector two
* consecutive elements of DIF are set to the same value. If
* the eigenvalues cannot be reordered to compute DIF(j), DIF(j)
* is set to 0; this can only occur when the true value would be
* very small anyway.
* If JOB = 'E', DIF is not referenced.
*
* MM (input) INTEGER
* The number of elements in the arrays S and DIF. MM >= M.
*
* M (output) INTEGER
* The number of elements of the arrays S and DIF used to store
* the specified condition numbers; for each selected real
* eigenvalue one element is used, and for each selected complex
* conjugate pair of eigenvalues, two elements are used.
* If HOWMNY = 'A', M is set to N.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N).
* If JOB = 'V' or 'B' LWORK >= 2*N*(N+2)+16.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (N + 6)
* If JOB = 'E', IWORK is not referenced.
*
* INFO (output) INTEGER
* =0: Successful exit
* <0: If INFO = -i, the i-th argument had an illegal value
*
*
* Further Details
* ===============
*
* The reciprocal of the condition number of a generalized eigenvalue
* w = (a, b) is defined as
*
* S(w) = (|u'Av|**2 + |u'Bv|**2)**(1/2) / (norm(u)*norm(v))
*
* where u and v are the left and right eigenvectors of (A, B)
* corresponding to w; |z| denotes the absolute value of the complex
* number, and norm(u) denotes the 2-norm of the vector u.
* The pair (a, b) corresponds to an eigenvalue w = a/b (= u'Av/u'Bv)
* of the matrix pair (A, B). If both a and b equal zero, then (A B) is
* singular and S(I) = -1 is returned.
*
* An approximate error bound on the chordal distance between the i-th
* computed generalized eigenvalue w and the corresponding exact
* eigenvalue lambda is
*
* chord(w, lambda) <= EPS * norm(A, B) / S(I)
*
* where EPS is the machine precision.
*
* The reciprocal of the condition number DIF(i) of right eigenvector u
* and left eigenvector v corresponding to the generalized eigenvalue w
* is defined as follows:
*
* a) If the i-th eigenvalue w = (a,b) is real
*
* Suppose U and V are orthogonal transformations such that
*
* U'*(A, B)*V = (S, T) = ( a * ) ( b * ) 1
* ( 0 S22 ),( 0 T22 ) n-1
* 1 n-1 1 n-1
*
* Then the reciprocal condition number DIF(i) is
*
* Difl((a, b), (S22, T22)) = sigma-min( Zl ),
*
* where sigma-min(Zl) denotes the smallest singular value of the
* 2(n-1)-by-2(n-1) matrix
*
* Zl = [ kron(a, In-1) -kron(1, S22) ]
* [ kron(b, In-1) -kron(1, T22) ] .
*
* Here In-1 is the identity matrix of size n-1. kron(X, Y) is the
* Kronecker product between the matrices X and Y.
*
* Note that if the default method for computing DIF(i) is wanted
* (see DLATDF), then the parameter DIFDRI (see below) should be
* changed from 3 to 4 (routine DLATDF(IJOB = 2 will be used)).
* See DTGSYL for more details.
*
* b) If the i-th and (i+1)-th eigenvalues are complex conjugate pair,
*
* Suppose U and V are orthogonal transformations such that
*
* U'*(A, B)*V = (S, T) = ( S11 * ) ( T11 * ) 2
* ( 0 S22 ),( 0 T22) n-2
* 2 n-2 2 n-2
*
* and (S11, T11) corresponds to the complex conjugate eigenvalue
* pair (w, conjg(w)). There exist unitary matrices U1 and V1 such
* that
*
* U1'*S11*V1 = ( s11 s12 ) and U1'*T11*V1 = ( t11 t12 )
* ( 0 s22 ) ( 0 t22 )
*
* where the generalized eigenvalues w = s11/t11 and
* conjg(w) = s22/t22.
*
* Then the reciprocal condition number DIF(i) is bounded by
*
* min( d1, max( 1, |real(s11)/real(s22)| )*d2 )
*
* where, d1 = Difl((s11, t11), (s22, t22)) = sigma-min(Z1), where
* Z1 is the complex 2-by-2 matrix
*
* Z1 = [ s11 -s22 ]
* [ t11 -t22 ],
*
* This is done by computing (using real arithmetic) the
* roots of the characteristical polynomial det(Z1' * Z1 - lambda I),
* where Z1' denotes the conjugate transpose of Z1 and det(X) denotes
* the determinant of X.
*
* and d2 is an upper bound on Difl((S11, T11), (S22, T22)), i.e. an
* upper bound on sigma-min(Z2), where Z2 is (2n-2)-by-(2n-2)
*
* Z2 = [ kron(S11', In-2) -kron(I2, S22) ]
* [ kron(T11', In-2) -kron(I2, T22) ]
*
* Note that if the default method for computing DIF is wanted (see
* DLATDF), then the parameter DIFDRI (see below) should be changed
* from 3 to 4 (routine DLATDF(IJOB = 2 will be used)). See DTGSYL
* for more details.
*
* For each eigenvalue/vector specified by SELECT, DIF stores a
* Frobenius norm-based estimate of Difl.
*
* An approximate error bound for the i-th computed eigenvector VL(i) or
* VR(i) is given by
*
* EPS * norm(A, B) / DIF(i).
*
* See ref. [2-3] for more details and further references.
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* References
* ==========
*
* [1] B. Kagstrom; A Direct Method for Reordering Eigenvalues in the
* Generalized Real Schur Form of a Regular Matrix Pair (A, B), in
* M.S. Moonen et al (eds), Linear Algebra for Large Scale and
* Real-Time Applications, Kluwer Academic Publ. 1993, pp 195-218.
*
* [2] B. Kagstrom and P. Poromaa; Computing Eigenspaces with Specified
* Eigenvalues of a Regular Matrix Pair (A, B) and Condition
* Estimation: Theory, Algorithms and Software,
* Report UMINF - 94.04, Department of Computing Science, Umea
* University, S-901 87 Umea, Sweden, 1994. Also as LAPACK Working
* Note 87. To appear in Numerical Algorithms, 1996.
*
* [3] B. Kagstrom and P. Poromaa, LAPACK-Style Algorithms and Software
* for Solving the Generalized Sylvester Equation and Estimating the
* Separation between Regular Matrix Pairs, Report UMINF - 93.23,
* Department of Computing Science, Umea University, S-901 87 Umea,
* Sweden, December 1993, Revised April 1994, Also as LAPACK Working
* Note 75. To appear in ACM Trans. on Math. Software, Vol 22,
* No 1, 1996.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param howmny
* @param select
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param vl
* @param ldvl
* @param vr
* @param ldvr
* @param s
* @param dif
* @param mm
* @param m
* @param work
* @param lwork
* @param iwork
* @param info
*
*/
abstract public void dtgsna(java.lang.String job, java.lang.String howmny, boolean[] select, int n, double[] a, int lda, double[] b, int ldb, double[] vl, int ldvl, double[] vr, int ldvr, double[] s, double[] dif, int mm, org.netlib.util.intW m, double[] work, int lwork, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTGSNA estimates reciprocal condition numbers for specified
* eigenvalues and/or eigenvectors of a matrix pair (A, B) in
* generalized real Schur canonical form (or of any matrix pair
* (Q*A*Z', Q*B*Z') with orthogonal matrices Q and Z, where
* Z' denotes the transpose of Z.
*
* (A, B) must be in generalized real Schur form (as returned by DGGES),
* i.e. A is block upper triangular with 1-by-1 and 2-by-2 diagonal
* blocks. B is upper triangular.
*
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* Specifies whether condition numbers are required for
* eigenvalues (S) or eigenvectors (DIF):
* = 'E': for eigenvalues only (S);
* = 'V': for eigenvectors only (DIF);
* = 'B': for both eigenvalues and eigenvectors (S and DIF).
*
* HOWMNY (input) CHARACTER*1
* = 'A': compute condition numbers for all eigenpairs;
* = 'S': compute condition numbers for selected eigenpairs
* specified by the array SELECT.
*
* SELECT (input) LOGICAL array, dimension (N)
* If HOWMNY = 'S', SELECT specifies the eigenpairs for which
* condition numbers are required. To select condition numbers
* for the eigenpair corresponding to a real eigenvalue w(j),
* SELECT(j) must be set to .TRUE.. To select condition numbers
* corresponding to a complex conjugate pair of eigenvalues w(j)
* and w(j+1), either SELECT(j) or SELECT(j+1) or both, must be
* set to .TRUE..
* If HOWMNY = 'A', SELECT is not referenced.
*
* N (input) INTEGER
* The order of the square matrix pair (A, B). N >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The upper quasi-triangular matrix A in the pair (A,B).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input) DOUBLE PRECISION array, dimension (LDB,N)
* The upper triangular matrix B in the pair (A,B).
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* VL (input) DOUBLE PRECISION array, dimension (LDVL,M)
* If JOB = 'E' or 'B', VL must contain left eigenvectors of
* (A, B), corresponding to the eigenpairs specified by HOWMNY
* and SELECT. The eigenvectors must be stored in consecutive
* columns of VL, as returned by DTGEVC.
* If JOB = 'V', VL is not referenced.
*
* LDVL (input) INTEGER
* The leading dimension of the array VL. LDVL >= 1.
* If JOB = 'E' or 'B', LDVL >= N.
*
* VR (input) DOUBLE PRECISION array, dimension (LDVR,M)
* If JOB = 'E' or 'B', VR must contain right eigenvectors of
* (A, B), corresponding to the eigenpairs specified by HOWMNY
* and SELECT. The eigenvectors must be stored in consecutive
* columns ov VR, as returned by DTGEVC.
* If JOB = 'V', VR is not referenced.
*
* LDVR (input) INTEGER
* The leading dimension of the array VR. LDVR >= 1.
* If JOB = 'E' or 'B', LDVR >= N.
*
* S (output) DOUBLE PRECISION array, dimension (MM)
* If JOB = 'E' or 'B', the reciprocal condition numbers of the
* selected eigenvalues, stored in consecutive elements of the
* array. For a complex conjugate pair of eigenvalues two
* consecutive elements of S are set to the same value. Thus
* S(j), DIF(j), and the j-th columns of VL and VR all
* correspond to the same eigenpair (but not in general the
* j-th eigenpair, unless all eigenpairs are selected).
* If JOB = 'V', S is not referenced.
*
* DIF (output) DOUBLE PRECISION array, dimension (MM)
* If JOB = 'V' or 'B', the estimated reciprocal condition
* numbers of the selected eigenvectors, stored in consecutive
* elements of the array. For a complex eigenvector two
* consecutive elements of DIF are set to the same value. If
* the eigenvalues cannot be reordered to compute DIF(j), DIF(j)
* is set to 0; this can only occur when the true value would be
* very small anyway.
* If JOB = 'E', DIF is not referenced.
*
* MM (input) INTEGER
* The number of elements in the arrays S and DIF. MM >= M.
*
* M (output) INTEGER
* The number of elements of the arrays S and DIF used to store
* the specified condition numbers; for each selected real
* eigenvalue one element is used, and for each selected complex
* conjugate pair of eigenvalues, two elements are used.
* If HOWMNY = 'A', M is set to N.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N).
* If JOB = 'V' or 'B' LWORK >= 2*N*(N+2)+16.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (N + 6)
* If JOB = 'E', IWORK is not referenced.
*
* INFO (output) INTEGER
* =0: Successful exit
* <0: If INFO = -i, the i-th argument had an illegal value
*
*
* Further Details
* ===============
*
* The reciprocal of the condition number of a generalized eigenvalue
* w = (a, b) is defined as
*
* S(w) = (|u'Av|**2 + |u'Bv|**2)**(1/2) / (norm(u)*norm(v))
*
* where u and v are the left and right eigenvectors of (A, B)
* corresponding to w; |z| denotes the absolute value of the complex
* number, and norm(u) denotes the 2-norm of the vector u.
* The pair (a, b) corresponds to an eigenvalue w = a/b (= u'Av/u'Bv)
* of the matrix pair (A, B). If both a and b equal zero, then (A B) is
* singular and S(I) = -1 is returned.
*
* An approximate error bound on the chordal distance between the i-th
* computed generalized eigenvalue w and the corresponding exact
* eigenvalue lambda is
*
* chord(w, lambda) <= EPS * norm(A, B) / S(I)
*
* where EPS is the machine precision.
*
* The reciprocal of the condition number DIF(i) of right eigenvector u
* and left eigenvector v corresponding to the generalized eigenvalue w
* is defined as follows:
*
* a) If the i-th eigenvalue w = (a,b) is real
*
* Suppose U and V are orthogonal transformations such that
*
* U'*(A, B)*V = (S, T) = ( a * ) ( b * ) 1
* ( 0 S22 ),( 0 T22 ) n-1
* 1 n-1 1 n-1
*
* Then the reciprocal condition number DIF(i) is
*
* Difl((a, b), (S22, T22)) = sigma-min( Zl ),
*
* where sigma-min(Zl) denotes the smallest singular value of the
* 2(n-1)-by-2(n-1) matrix
*
* Zl = [ kron(a, In-1) -kron(1, S22) ]
* [ kron(b, In-1) -kron(1, T22) ] .
*
* Here In-1 is the identity matrix of size n-1. kron(X, Y) is the
* Kronecker product between the matrices X and Y.
*
* Note that if the default method for computing DIF(i) is wanted
* (see DLATDF), then the parameter DIFDRI (see below) should be
* changed from 3 to 4 (routine DLATDF(IJOB = 2 will be used)).
* See DTGSYL for more details.
*
* b) If the i-th and (i+1)-th eigenvalues are complex conjugate pair,
*
* Suppose U and V are orthogonal transformations such that
*
* U'*(A, B)*V = (S, T) = ( S11 * ) ( T11 * ) 2
* ( 0 S22 ),( 0 T22) n-2
* 2 n-2 2 n-2
*
* and (S11, T11) corresponds to the complex conjugate eigenvalue
* pair (w, conjg(w)). There exist unitary matrices U1 and V1 such
* that
*
* U1'*S11*V1 = ( s11 s12 ) and U1'*T11*V1 = ( t11 t12 )
* ( 0 s22 ) ( 0 t22 )
*
* where the generalized eigenvalues w = s11/t11 and
* conjg(w) = s22/t22.
*
* Then the reciprocal condition number DIF(i) is bounded by
*
* min( d1, max( 1, |real(s11)/real(s22)| )*d2 )
*
* where, d1 = Difl((s11, t11), (s22, t22)) = sigma-min(Z1), where
* Z1 is the complex 2-by-2 matrix
*
* Z1 = [ s11 -s22 ]
* [ t11 -t22 ],
*
* This is done by computing (using real arithmetic) the
* roots of the characteristical polynomial det(Z1' * Z1 - lambda I),
* where Z1' denotes the conjugate transpose of Z1 and det(X) denotes
* the determinant of X.
*
* and d2 is an upper bound on Difl((S11, T11), (S22, T22)), i.e. an
* upper bound on sigma-min(Z2), where Z2 is (2n-2)-by-(2n-2)
*
* Z2 = [ kron(S11', In-2) -kron(I2, S22) ]
* [ kron(T11', In-2) -kron(I2, T22) ]
*
* Note that if the default method for computing DIF is wanted (see
* DLATDF), then the parameter DIFDRI (see below) should be changed
* from 3 to 4 (routine DLATDF(IJOB = 2 will be used)). See DTGSYL
* for more details.
*
* For each eigenvalue/vector specified by SELECT, DIF stores a
* Frobenius norm-based estimate of Difl.
*
* An approximate error bound for the i-th computed eigenvector VL(i) or
* VR(i) is given by
*
* EPS * norm(A, B) / DIF(i).
*
* See ref. [2-3] for more details and further references.
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* References
* ==========
*
* [1] B. Kagstrom; A Direct Method for Reordering Eigenvalues in the
* Generalized Real Schur Form of a Regular Matrix Pair (A, B), in
* M.S. Moonen et al (eds), Linear Algebra for Large Scale and
* Real-Time Applications, Kluwer Academic Publ. 1993, pp 195-218.
*
* [2] B. Kagstrom and P. Poromaa; Computing Eigenspaces with Specified
* Eigenvalues of a Regular Matrix Pair (A, B) and Condition
* Estimation: Theory, Algorithms and Software,
* Report UMINF - 94.04, Department of Computing Science, Umea
* University, S-901 87 Umea, Sweden, 1994. Also as LAPACK Working
* Note 87. To appear in Numerical Algorithms, 1996.
*
* [3] B. Kagstrom and P. Poromaa, LAPACK-Style Algorithms and Software
* for Solving the Generalized Sylvester Equation and Estimating the
* Separation between Regular Matrix Pairs, Report UMINF - 93.23,
* Department of Computing Science, Umea University, S-901 87 Umea,
* Sweden, December 1993, Revised April 1994, Also as LAPACK Working
* Note 75. To appear in ACM Trans. on Math. Software, Vol 22,
* No 1, 1996.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param howmny
* @param select
* @param _select_offset
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param vl
* @param _vl_offset
* @param ldvl
* @param vr
* @param _vr_offset
* @param ldvr
* @param s
* @param _s_offset
* @param dif
* @param _dif_offset
* @param mm
* @param m
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dtgsna(java.lang.String job, java.lang.String howmny, boolean[] select, int _select_offset, int n, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, double[] vl, int _vl_offset, int ldvl, double[] vr, int _vr_offset, int ldvr, double[] s, int _s_offset, double[] dif, int _dif_offset, int mm, org.netlib.util.intW m, double[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTGSY2 solves the generalized Sylvester equation:
*
* A * R - L * B = scale * C (1)
* D * R - L * E = scale * F,
*
* using Level 1 and 2 BLAS. where R and L are unknown M-by-N matrices,
* (A, D), (B, E) and (C, F) are given matrix pairs of size M-by-M,
* N-by-N and M-by-N, respectively, with real entries. (A, D) and (B, E)
* must be in generalized Schur canonical form, i.e. A, B are upper
* quasi triangular and D, E are upper triangular. The solution (R, L)
* overwrites (C, F). 0 <= SCALE <= 1 is an output scaling factor
* chosen to avoid overflow.
*
* In matrix notation solving equation (1) corresponds to solve
* Z*x = scale*b, where Z is defined as
*
* Z = [ kron(In, A) -kron(B', Im) ] (2)
* [ kron(In, D) -kron(E', Im) ],
*
* Ik is the identity matrix of size k and X' is the transpose of X.
* kron(X, Y) is the Kronecker product between the matrices X and Y.
* In the process of solving (1), we solve a number of such systems
* where Dim(In), Dim(In) = 1 or 2.
*
* If TRANS = 'T', solve the transposed system Z'*y = scale*b for y,
* which is equivalent to solve for R and L in
*
* A' * R + D' * L = scale * C (3)
* R * B' + L * E' = scale * -F
*
* This case is used to compute an estimate of Dif[(A, D), (B, E)] =
* sigma_min(Z) using reverse communicaton with DLACON.
*
* DTGSY2 also (IJOB >= 1) contributes to the computation in DTGSYL
* of an upper bound on the separation between to matrix pairs. Then
* the input (A, D), (B, E) are sub-pencils of the matrix pair in
* DTGSYL. See DTGSYL for details.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* = 'N', solve the generalized Sylvester equation (1).
* = 'T': solve the 'transposed' system (3).
*
* IJOB (input) INTEGER
* Specifies what kind of functionality to be performed.
* = 0: solve (1) only.
* = 1: A contribution from this subsystem to a Frobenius
* norm-based estimate of the separation between two matrix
* pairs is computed. (look ahead strategy is used).
* = 2: A contribution from this subsystem to a Frobenius
* norm-based estimate of the separation between two matrix
* pairs is computed. (DGECON on sub-systems is used.)
* Not referenced if TRANS = 'T'.
*
* M (input) INTEGER
* On entry, M specifies the order of A and D, and the row
* dimension of C, F, R and L.
*
* N (input) INTEGER
* On entry, N specifies the order of B and E, and the column
* dimension of C, F, R and L.
*
* A (input) DOUBLE PRECISION array, dimension (LDA, M)
* On entry, A contains an upper quasi triangular matrix.
*
* LDA (input) INTEGER
* The leading dimension of the matrix A. LDA >= max(1, M).
*
* B (input) DOUBLE PRECISION array, dimension (LDB, N)
* On entry, B contains an upper quasi triangular matrix.
*
* LDB (input) INTEGER
* The leading dimension of the matrix B. LDB >= max(1, N).
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC, N)
* On entry, C contains the right-hand-side of the first matrix
* equation in (1).
* On exit, if IJOB = 0, C has been overwritten by the
* solution R.
*
* LDC (input) INTEGER
* The leading dimension of the matrix C. LDC >= max(1, M).
*
* D (input) DOUBLE PRECISION array, dimension (LDD, M)
* On entry, D contains an upper triangular matrix.
*
* LDD (input) INTEGER
* The leading dimension of the matrix D. LDD >= max(1, M).
*
* E (input) DOUBLE PRECISION array, dimension (LDE, N)
* On entry, E contains an upper triangular matrix.
*
* LDE (input) INTEGER
* The leading dimension of the matrix E. LDE >= max(1, N).
*
* F (input/output) DOUBLE PRECISION array, dimension (LDF, N)
* On entry, F contains the right-hand-side of the second matrix
* equation in (1).
* On exit, if IJOB = 0, F has been overwritten by the
* solution L.
*
* LDF (input) INTEGER
* The leading dimension of the matrix F. LDF >= max(1, M).
*
* SCALE (output) DOUBLE PRECISION
* On exit, 0 <= SCALE <= 1. If 0 < SCALE < 1, the solutions
* R and L (C and F on entry) will hold the solutions to a
* slightly perturbed system but the input matrices A, B, D and
* E have not been changed. If SCALE = 0, R and L will hold the
* solutions to the homogeneous system with C = F = 0. Normally,
* SCALE = 1.
*
* RDSUM (input/output) DOUBLE PRECISION
* On entry, the sum of squares of computed contributions to
* the Dif-estimate under computation by DTGSYL, where the
* scaling factor RDSCAL (see below) has been factored out.
* On exit, the corresponding sum of squares updated with the
* contributions from the current sub-system.
* If TRANS = 'T' RDSUM is not touched.
* NOTE: RDSUM only makes sense when DTGSY2 is called by DTGSYL.
*
* RDSCAL (input/output) DOUBLE PRECISION
* On entry, scaling factor used to prevent overflow in RDSUM.
* On exit, RDSCAL is updated w.r.t. the current contributions
* in RDSUM.
* If TRANS = 'T', RDSCAL is not touched.
* NOTE: RDSCAL only makes sense when DTGSY2 is called by
* DTGSYL.
*
* IWORK (workspace) INTEGER array, dimension (M+N+2)
*
* PQ (output) INTEGER
* On exit, the number of subsystems (of size 2-by-2, 4-by-4 and
* 8-by-8) solved by this routine.
*
* INFO (output) INTEGER
* On exit, if INFO is set to
* =0: Successful exit
* <0: If INFO = -i, the i-th argument had an illegal value.
* >0: The matrix pairs (A, D) and (B, E) have common or very
* close eigenvalues.
*
* Further Details
* ===============
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* =====================================================================
* Replaced various illegal calls to DCOPY by calls to DLASET.
* Sven Hammarling, 27/5/02.
*
* .. Parameters ..
*
*
* @param trans
* @param ijob
* @param m
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param c
* @param Ldc
* @param d
* @param ldd
* @param e
* @param lde
* @param f
* @param ldf
* @param scale
* @param rdsum
* @param rdscal
* @param iwork
* @param pq
* @param info
*
*/
abstract public void dtgsy2(java.lang.String trans, int ijob, int m, int n, double[] a, int lda, double[] b, int ldb, double[] c, int Ldc, double[] d, int ldd, double[] e, int lde, double[] f, int ldf, org.netlib.util.doubleW scale, org.netlib.util.doubleW rdsum, org.netlib.util.doubleW rdscal, int[] iwork, org.netlib.util.intW pq, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTGSY2 solves the generalized Sylvester equation:
*
* A * R - L * B = scale * C (1)
* D * R - L * E = scale * F,
*
* using Level 1 and 2 BLAS. where R and L are unknown M-by-N matrices,
* (A, D), (B, E) and (C, F) are given matrix pairs of size M-by-M,
* N-by-N and M-by-N, respectively, with real entries. (A, D) and (B, E)
* must be in generalized Schur canonical form, i.e. A, B are upper
* quasi triangular and D, E are upper triangular. The solution (R, L)
* overwrites (C, F). 0 <= SCALE <= 1 is an output scaling factor
* chosen to avoid overflow.
*
* In matrix notation solving equation (1) corresponds to solve
* Z*x = scale*b, where Z is defined as
*
* Z = [ kron(In, A) -kron(B', Im) ] (2)
* [ kron(In, D) -kron(E', Im) ],
*
* Ik is the identity matrix of size k and X' is the transpose of X.
* kron(X, Y) is the Kronecker product between the matrices X and Y.
* In the process of solving (1), we solve a number of such systems
* where Dim(In), Dim(In) = 1 or 2.
*
* If TRANS = 'T', solve the transposed system Z'*y = scale*b for y,
* which is equivalent to solve for R and L in
*
* A' * R + D' * L = scale * C (3)
* R * B' + L * E' = scale * -F
*
* This case is used to compute an estimate of Dif[(A, D), (B, E)] =
* sigma_min(Z) using reverse communicaton with DLACON.
*
* DTGSY2 also (IJOB >= 1) contributes to the computation in DTGSYL
* of an upper bound on the separation between to matrix pairs. Then
* the input (A, D), (B, E) are sub-pencils of the matrix pair in
* DTGSYL. See DTGSYL for details.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* = 'N', solve the generalized Sylvester equation (1).
* = 'T': solve the 'transposed' system (3).
*
* IJOB (input) INTEGER
* Specifies what kind of functionality to be performed.
* = 0: solve (1) only.
* = 1: A contribution from this subsystem to a Frobenius
* norm-based estimate of the separation between two matrix
* pairs is computed. (look ahead strategy is used).
* = 2: A contribution from this subsystem to a Frobenius
* norm-based estimate of the separation between two matrix
* pairs is computed. (DGECON on sub-systems is used.)
* Not referenced if TRANS = 'T'.
*
* M (input) INTEGER
* On entry, M specifies the order of A and D, and the row
* dimension of C, F, R and L.
*
* N (input) INTEGER
* On entry, N specifies the order of B and E, and the column
* dimension of C, F, R and L.
*
* A (input) DOUBLE PRECISION array, dimension (LDA, M)
* On entry, A contains an upper quasi triangular matrix.
*
* LDA (input) INTEGER
* The leading dimension of the matrix A. LDA >= max(1, M).
*
* B (input) DOUBLE PRECISION array, dimension (LDB, N)
* On entry, B contains an upper quasi triangular matrix.
*
* LDB (input) INTEGER
* The leading dimension of the matrix B. LDB >= max(1, N).
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC, N)
* On entry, C contains the right-hand-side of the first matrix
* equation in (1).
* On exit, if IJOB = 0, C has been overwritten by the
* solution R.
*
* LDC (input) INTEGER
* The leading dimension of the matrix C. LDC >= max(1, M).
*
* D (input) DOUBLE PRECISION array, dimension (LDD, M)
* On entry, D contains an upper triangular matrix.
*
* LDD (input) INTEGER
* The leading dimension of the matrix D. LDD >= max(1, M).
*
* E (input) DOUBLE PRECISION array, dimension (LDE, N)
* On entry, E contains an upper triangular matrix.
*
* LDE (input) INTEGER
* The leading dimension of the matrix E. LDE >= max(1, N).
*
* F (input/output) DOUBLE PRECISION array, dimension (LDF, N)
* On entry, F contains the right-hand-side of the second matrix
* equation in (1).
* On exit, if IJOB = 0, F has been overwritten by the
* solution L.
*
* LDF (input) INTEGER
* The leading dimension of the matrix F. LDF >= max(1, M).
*
* SCALE (output) DOUBLE PRECISION
* On exit, 0 <= SCALE <= 1. If 0 < SCALE < 1, the solutions
* R and L (C and F on entry) will hold the solutions to a
* slightly perturbed system but the input matrices A, B, D and
* E have not been changed. If SCALE = 0, R and L will hold the
* solutions to the homogeneous system with C = F = 0. Normally,
* SCALE = 1.
*
* RDSUM (input/output) DOUBLE PRECISION
* On entry, the sum of squares of computed contributions to
* the Dif-estimate under computation by DTGSYL, where the
* scaling factor RDSCAL (see below) has been factored out.
* On exit, the corresponding sum of squares updated with the
* contributions from the current sub-system.
* If TRANS = 'T' RDSUM is not touched.
* NOTE: RDSUM only makes sense when DTGSY2 is called by DTGSYL.
*
* RDSCAL (input/output) DOUBLE PRECISION
* On entry, scaling factor used to prevent overflow in RDSUM.
* On exit, RDSCAL is updated w.r.t. the current contributions
* in RDSUM.
* If TRANS = 'T', RDSCAL is not touched.
* NOTE: RDSCAL only makes sense when DTGSY2 is called by
* DTGSYL.
*
* IWORK (workspace) INTEGER array, dimension (M+N+2)
*
* PQ (output) INTEGER
* On exit, the number of subsystems (of size 2-by-2, 4-by-4 and
* 8-by-8) solved by this routine.
*
* INFO (output) INTEGER
* On exit, if INFO is set to
* =0: Successful exit
* <0: If INFO = -i, the i-th argument had an illegal value.
* >0: The matrix pairs (A, D) and (B, E) have common or very
* close eigenvalues.
*
* Further Details
* ===============
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* =====================================================================
* Replaced various illegal calls to DCOPY by calls to DLASET.
* Sven Hammarling, 27/5/02.
*
* .. Parameters ..
*
*
* @param trans
* @param ijob
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param c
* @param _c_offset
* @param Ldc
* @param d
* @param _d_offset
* @param ldd
* @param e
* @param _e_offset
* @param lde
* @param f
* @param _f_offset
* @param ldf
* @param scale
* @param rdsum
* @param rdscal
* @param iwork
* @param _iwork_offset
* @param pq
* @param info
*
*/
abstract public void dtgsy2(java.lang.String trans, int ijob, int m, int n, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, double[] c, int _c_offset, int Ldc, double[] d, int _d_offset, int ldd, double[] e, int _e_offset, int lde, double[] f, int _f_offset, int ldf, org.netlib.util.doubleW scale, org.netlib.util.doubleW rdsum, org.netlib.util.doubleW rdscal, int[] iwork, int _iwork_offset, org.netlib.util.intW pq, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTGSYL solves the generalized Sylvester equation:
*
* A * R - L * B = scale * C (1)
* D * R - L * E = scale * F
*
* where R and L are unknown m-by-n matrices, (A, D), (B, E) and
* (C, F) are given matrix pairs of size m-by-m, n-by-n and m-by-n,
* respectively, with real entries. (A, D) and (B, E) must be in
* generalized (real) Schur canonical form, i.e. A, B are upper quasi
* triangular and D, E are upper triangular.
*
* The solution (R, L) overwrites (C, F). 0 <= SCALE <= 1 is an output
* scaling factor chosen to avoid overflow.
*
* In matrix notation (1) is equivalent to solve Zx = scale b, where
* Z is defined as
*
* Z = [ kron(In, A) -kron(B', Im) ] (2)
* [ kron(In, D) -kron(E', Im) ].
*
* Here Ik is the identity matrix of size k and X' is the transpose of
* X. kron(X, Y) is the Kronecker product between the matrices X and Y.
*
* If TRANS = 'T', DTGSYL solves the transposed system Z'*y = scale*b,
* which is equivalent to solve for R and L in
*
* A' * R + D' * L = scale * C (3)
* R * B' + L * E' = scale * (-F)
*
* This case (TRANS = 'T') is used to compute an one-norm-based estimate
* of Dif[(A,D), (B,E)], the separation between the matrix pairs (A,D)
* and (B,E), using DLACON.
*
* If IJOB >= 1, DTGSYL computes a Frobenius norm-based estimate
* of Dif[(A,D),(B,E)]. That is, the reciprocal of a lower bound on the
* reciprocal of the smallest singular value of Z. See [1-2] for more
* information.
*
* This is a level 3 BLAS algorithm.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* = 'N', solve the generalized Sylvester equation (1).
* = 'T', solve the 'transposed' system (3).
*
* IJOB (input) INTEGER
* Specifies what kind of functionality to be performed.
* =0: solve (1) only.
* =1: The functionality of 0 and 3.
* =2: The functionality of 0 and 4.
* =3: Only an estimate of Dif[(A,D), (B,E)] is computed.
* (look ahead strategy IJOB = 1 is used).
* =4: Only an estimate of Dif[(A,D), (B,E)] is computed.
* ( DGECON on sub-systems is used ).
* Not referenced if TRANS = 'T'.
*
* M (input) INTEGER
* The order of the matrices A and D, and the row dimension of
* the matrices C, F, R and L.
*
* N (input) INTEGER
* The order of the matrices B and E, and the column dimension
* of the matrices C, F, R and L.
*
* A (input) DOUBLE PRECISION array, dimension (LDA, M)
* The upper quasi triangular matrix A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1, M).
*
* B (input) DOUBLE PRECISION array, dimension (LDB, N)
* The upper quasi triangular matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1, N).
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC, N)
* On entry, C contains the right-hand-side of the first matrix
* equation in (1) or (3).
* On exit, if IJOB = 0, 1 or 2, C has been overwritten by
* the solution R. If IJOB = 3 or 4 and TRANS = 'N', C holds R,
* the solution achieved during the computation of the
* Dif-estimate.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1, M).
*
* D (input) DOUBLE PRECISION array, dimension (LDD, M)
* The upper triangular matrix D.
*
* LDD (input) INTEGER
* The leading dimension of the array D. LDD >= max(1, M).
*
* E (input) DOUBLE PRECISION array, dimension (LDE, N)
* The upper triangular matrix E.
*
* LDE (input) INTEGER
* The leading dimension of the array E. LDE >= max(1, N).
*
* F (input/output) DOUBLE PRECISION array, dimension (LDF, N)
* On entry, F contains the right-hand-side of the second matrix
* equation in (1) or (3).
* On exit, if IJOB = 0, 1 or 2, F has been overwritten by
* the solution L. If IJOB = 3 or 4 and TRANS = 'N', F holds L,
* the solution achieved during the computation of the
* Dif-estimate.
*
* LDF (input) INTEGER
* The leading dimension of the array F. LDF >= max(1, M).
*
* DIF (output) DOUBLE PRECISION
* On exit DIF is the reciprocal of a lower bound of the
* reciprocal of the Dif-function, i.e. DIF is an upper bound of
* Dif[(A,D), (B,E)] = sigma_min(Z), where Z as in (2).
* IF IJOB = 0 or TRANS = 'T', DIF is not touched.
*
* SCALE (output) DOUBLE PRECISION
* On exit SCALE is the scaling factor in (1) or (3).
* If 0 < SCALE < 1, C and F hold the solutions R and L, resp.,
* to a slightly perturbed system but the input matrices A, B, D
* and E have not been changed. If SCALE = 0, C and F hold the
* solutions R and L, respectively, to the homogeneous system
* with C = F = 0. Normally, SCALE = 1.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK > = 1.
* If IJOB = 1 or 2 and TRANS = 'N', LWORK >= max(1,2*M*N).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (M+N+6)
*
* INFO (output) INTEGER
* =0: successful exit
* <0: If INFO = -i, the i-th argument had an illegal value.
* >0: (A, D) and (B, E) have common or close eigenvalues.
*
* Further Details
* ===============
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* [1] B. Kagstrom and P. Poromaa, LAPACK-Style Algorithms and Software
* for Solving the Generalized Sylvester Equation and Estimating the
* Separation between Regular Matrix Pairs, Report UMINF - 93.23,
* Department of Computing Science, Umea University, S-901 87 Umea,
* Sweden, December 1993, Revised April 1994, Also as LAPACK Working
* Note 75. To appear in ACM Trans. on Math. Software, Vol 22,
* No 1, 1996.
*
* [2] B. Kagstrom, A Perturbation Analysis of the Generalized Sylvester
* Equation (AR - LB, DR - LE ) = (C, F), SIAM J. Matrix Anal.
* Appl., 15(4):1045-1060, 1994
*
* [3] B. Kagstrom and L. Westin, Generalized Schur Methods with
* Condition Estimators for Solving the Generalized Sylvester
* Equation, IEEE Transactions on Automatic Control, Vol. 34, No. 7,
* July 1989, pp 745-751.
*
* =====================================================================
* Replaced various illegal calls to DCOPY by calls to DLASET.
* Sven Hammarling, 1/5/02.
*
* .. Parameters ..
*
*
* @param trans
* @param ijob
* @param m
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param c
* @param Ldc
* @param d
* @param ldd
* @param e
* @param lde
* @param f
* @param ldf
* @param scale
* @param dif
* @param work
* @param lwork
* @param iwork
* @param info
*
*/
abstract public void dtgsyl(java.lang.String trans, int ijob, int m, int n, double[] a, int lda, double[] b, int ldb, double[] c, int Ldc, double[] d, int ldd, double[] e, int lde, double[] f, int ldf, org.netlib.util.doubleW scale, org.netlib.util.doubleW dif, double[] work, int lwork, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTGSYL solves the generalized Sylvester equation:
*
* A * R - L * B = scale * C (1)
* D * R - L * E = scale * F
*
* where R and L are unknown m-by-n matrices, (A, D), (B, E) and
* (C, F) are given matrix pairs of size m-by-m, n-by-n and m-by-n,
* respectively, with real entries. (A, D) and (B, E) must be in
* generalized (real) Schur canonical form, i.e. A, B are upper quasi
* triangular and D, E are upper triangular.
*
* The solution (R, L) overwrites (C, F). 0 <= SCALE <= 1 is an output
* scaling factor chosen to avoid overflow.
*
* In matrix notation (1) is equivalent to solve Zx = scale b, where
* Z is defined as
*
* Z = [ kron(In, A) -kron(B', Im) ] (2)
* [ kron(In, D) -kron(E', Im) ].
*
* Here Ik is the identity matrix of size k and X' is the transpose of
* X. kron(X, Y) is the Kronecker product between the matrices X and Y.
*
* If TRANS = 'T', DTGSYL solves the transposed system Z'*y = scale*b,
* which is equivalent to solve for R and L in
*
* A' * R + D' * L = scale * C (3)
* R * B' + L * E' = scale * (-F)
*
* This case (TRANS = 'T') is used to compute an one-norm-based estimate
* of Dif[(A,D), (B,E)], the separation between the matrix pairs (A,D)
* and (B,E), using DLACON.
*
* If IJOB >= 1, DTGSYL computes a Frobenius norm-based estimate
* of Dif[(A,D),(B,E)]. That is, the reciprocal of a lower bound on the
* reciprocal of the smallest singular value of Z. See [1-2] for more
* information.
*
* This is a level 3 BLAS algorithm.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* = 'N', solve the generalized Sylvester equation (1).
* = 'T', solve the 'transposed' system (3).
*
* IJOB (input) INTEGER
* Specifies what kind of functionality to be performed.
* =0: solve (1) only.
* =1: The functionality of 0 and 3.
* =2: The functionality of 0 and 4.
* =3: Only an estimate of Dif[(A,D), (B,E)] is computed.
* (look ahead strategy IJOB = 1 is used).
* =4: Only an estimate of Dif[(A,D), (B,E)] is computed.
* ( DGECON on sub-systems is used ).
* Not referenced if TRANS = 'T'.
*
* M (input) INTEGER
* The order of the matrices A and D, and the row dimension of
* the matrices C, F, R and L.
*
* N (input) INTEGER
* The order of the matrices B and E, and the column dimension
* of the matrices C, F, R and L.
*
* A (input) DOUBLE PRECISION array, dimension (LDA, M)
* The upper quasi triangular matrix A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1, M).
*
* B (input) DOUBLE PRECISION array, dimension (LDB, N)
* The upper quasi triangular matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1, N).
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC, N)
* On entry, C contains the right-hand-side of the first matrix
* equation in (1) or (3).
* On exit, if IJOB = 0, 1 or 2, C has been overwritten by
* the solution R. If IJOB = 3 or 4 and TRANS = 'N', C holds R,
* the solution achieved during the computation of the
* Dif-estimate.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1, M).
*
* D (input) DOUBLE PRECISION array, dimension (LDD, M)
* The upper triangular matrix D.
*
* LDD (input) INTEGER
* The leading dimension of the array D. LDD >= max(1, M).
*
* E (input) DOUBLE PRECISION array, dimension (LDE, N)
* The upper triangular matrix E.
*
* LDE (input) INTEGER
* The leading dimension of the array E. LDE >= max(1, N).
*
* F (input/output) DOUBLE PRECISION array, dimension (LDF, N)
* On entry, F contains the right-hand-side of the second matrix
* equation in (1) or (3).
* On exit, if IJOB = 0, 1 or 2, F has been overwritten by
* the solution L. If IJOB = 3 or 4 and TRANS = 'N', F holds L,
* the solution achieved during the computation of the
* Dif-estimate.
*
* LDF (input) INTEGER
* The leading dimension of the array F. LDF >= max(1, M).
*
* DIF (output) DOUBLE PRECISION
* On exit DIF is the reciprocal of a lower bound of the
* reciprocal of the Dif-function, i.e. DIF is an upper bound of
* Dif[(A,D), (B,E)] = sigma_min(Z), where Z as in (2).
* IF IJOB = 0 or TRANS = 'T', DIF is not touched.
*
* SCALE (output) DOUBLE PRECISION
* On exit SCALE is the scaling factor in (1) or (3).
* If 0 < SCALE < 1, C and F hold the solutions R and L, resp.,
* to a slightly perturbed system but the input matrices A, B, D
* and E have not been changed. If SCALE = 0, C and F hold the
* solutions R and L, respectively, to the homogeneous system
* with C = F = 0. Normally, SCALE = 1.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK > = 1.
* If IJOB = 1 or 2 and TRANS = 'N', LWORK >= max(1,2*M*N).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (M+N+6)
*
* INFO (output) INTEGER
* =0: successful exit
* <0: If INFO = -i, the i-th argument had an illegal value.
* >0: (A, D) and (B, E) have common or close eigenvalues.
*
* Further Details
* ===============
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* [1] B. Kagstrom and P. Poromaa, LAPACK-Style Algorithms and Software
* for Solving the Generalized Sylvester Equation and Estimating the
* Separation between Regular Matrix Pairs, Report UMINF - 93.23,
* Department of Computing Science, Umea University, S-901 87 Umea,
* Sweden, December 1993, Revised April 1994, Also as LAPACK Working
* Note 75. To appear in ACM Trans. on Math. Software, Vol 22,
* No 1, 1996.
*
* [2] B. Kagstrom, A Perturbation Analysis of the Generalized Sylvester
* Equation (AR - LB, DR - LE ) = (C, F), SIAM J. Matrix Anal.
* Appl., 15(4):1045-1060, 1994
*
* [3] B. Kagstrom and L. Westin, Generalized Schur Methods with
* Condition Estimators for Solving the Generalized Sylvester
* Equation, IEEE Transactions on Automatic Control, Vol. 34, No. 7,
* July 1989, pp 745-751.
*
* =====================================================================
* Replaced various illegal calls to DCOPY by calls to DLASET.
* Sven Hammarling, 1/5/02.
*
* .. Parameters ..
*
*
* @param trans
* @param ijob
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param c
* @param _c_offset
* @param Ldc
* @param d
* @param _d_offset
* @param ldd
* @param e
* @param _e_offset
* @param lde
* @param f
* @param _f_offset
* @param ldf
* @param scale
* @param dif
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dtgsyl(java.lang.String trans, int ijob, int m, int n, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, double[] c, int _c_offset, int Ldc, double[] d, int _d_offset, int ldd, double[] e, int _e_offset, int lde, double[] f, int _f_offset, int ldf, org.netlib.util.doubleW scale, org.netlib.util.doubleW dif, double[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTPCON estimates the reciprocal of the condition number of a packed
* triangular matrix A, in either the 1-norm or the infinity-norm.
*
* The norm of A is computed and an estimate is obtained for
* norm(inv(A)), then the reciprocal of the condition number is
* computed as
* RCOND = 1 / ( norm(A) * norm(inv(A)) ).
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies whether the 1-norm condition number or the
* infinity-norm condition number is required:
* = '1' or 'O': 1-norm;
* = 'I': Infinity-norm.
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The upper or lower triangular matrix A, packed columnwise in
* a linear array. The j-th column of A is stored in the array
* AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
* If DIAG = 'U', the diagonal elements of A are not referenced
* and are assumed to be 1.
*
* RCOND (output) DOUBLE PRECISION
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(norm(A) * norm(inv(A))).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param diag
* @param n
* @param ap
* @param rcond
* @param work
* @param iwork
* @param info
*
*/
abstract public void dtpcon(java.lang.String norm, java.lang.String uplo, java.lang.String diag, int n, double[] ap, org.netlib.util.doubleW rcond, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTPCON estimates the reciprocal of the condition number of a packed
* triangular matrix A, in either the 1-norm or the infinity-norm.
*
* The norm of A is computed and an estimate is obtained for
* norm(inv(A)), then the reciprocal of the condition number is
* computed as
* RCOND = 1 / ( norm(A) * norm(inv(A)) ).
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies whether the 1-norm condition number or the
* infinity-norm condition number is required:
* = '1' or 'O': 1-norm;
* = 'I': Infinity-norm.
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The upper or lower triangular matrix A, packed columnwise in
* a linear array. The j-th column of A is stored in the array
* AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
* If DIAG = 'U', the diagonal elements of A are not referenced
* and are assumed to be 1.
*
* RCOND (output) DOUBLE PRECISION
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(norm(A) * norm(inv(A))).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param diag
* @param n
* @param ap
* @param _ap_offset
* @param rcond
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dtpcon(java.lang.String norm, java.lang.String uplo, java.lang.String diag, int n, double[] ap, int _ap_offset, org.netlib.util.doubleW rcond, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTPRFS provides error bounds and backward error estimates for the
* solution to a system of linear equations with a triangular packed
* coefficient matrix.
*
* The solution matrix X must be computed by DTPTRS or some other
* means before entering this routine. DTPRFS does not do iterative
* refinement because doing so cannot improve the backward error.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The upper or lower triangular matrix A, packed columnwise in
* a linear array. The j-th column of A is stored in the array
* AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
* If DIAG = 'U', the diagonal elements of A are not referenced
* and are assumed to be 1.
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input) DOUBLE PRECISION array, dimension (LDX,NRHS)
* The solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param n
* @param nrhs
* @param ap
* @param b
* @param ldb
* @param x
* @param ldx
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void dtprfs(java.lang.String uplo, java.lang.String trans, java.lang.String diag, int n, int nrhs, double[] ap, double[] b, int ldb, double[] x, int ldx, double[] ferr, double[] berr, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTPRFS provides error bounds and backward error estimates for the
* solution to a system of linear equations with a triangular packed
* coefficient matrix.
*
* The solution matrix X must be computed by DTPTRS or some other
* means before entering this routine. DTPRFS does not do iterative
* refinement because doing so cannot improve the backward error.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The upper or lower triangular matrix A, packed columnwise in
* a linear array. The j-th column of A is stored in the array
* AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
* If DIAG = 'U', the diagonal elements of A are not referenced
* and are assumed to be 1.
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input) DOUBLE PRECISION array, dimension (LDX,NRHS)
* The solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param n
* @param nrhs
* @param ap
* @param _ap_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dtprfs(java.lang.String uplo, java.lang.String trans, java.lang.String diag, int n, int nrhs, double[] ap, int _ap_offset, double[] b, int _b_offset, int ldb, double[] x, int _x_offset, int ldx, double[] ferr, int _ferr_offset, double[] berr, int _berr_offset, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTPTRI computes the inverse of a real upper or lower triangular
* matrix A stored in packed format.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangular matrix A, stored
* columnwise in a linear array. The j-th column of A is stored
* in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*((2*n-j)/2) = A(i,j) for j<=i<=n.
* See below for further details.
* On exit, the (triangular) inverse of the original matrix, in
* the same packed storage format.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, A(i,i) is exactly zero. The triangular
* matrix is singular and its inverse can not be computed.
*
* Further Details
* ===============
*
* A triangular matrix A can be transferred to packed storage using one
* of the following program segments:
*
* UPLO = 'U': UPLO = 'L':
*
* JC = 1 JC = 1
* DO 2 J = 1, N DO 2 J = 1, N
* DO 1 I = 1, J DO 1 I = J, N
* AP(JC+I-1) = A(I,J) AP(JC+I-J) = A(I,J)
* 1 CONTINUE 1 CONTINUE
* JC = JC + J JC = JC + N - J + 1
* 2 CONTINUE 2 CONTINUE
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param diag
* @param n
* @param ap
* @param info
*
*/
abstract public void dtptri(java.lang.String uplo, java.lang.String diag, int n, double[] ap, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTPTRI computes the inverse of a real upper or lower triangular
* matrix A stored in packed format.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangular matrix A, stored
* columnwise in a linear array. The j-th column of A is stored
* in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*((2*n-j)/2) = A(i,j) for j<=i<=n.
* See below for further details.
* On exit, the (triangular) inverse of the original matrix, in
* the same packed storage format.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, A(i,i) is exactly zero. The triangular
* matrix is singular and its inverse can not be computed.
*
* Further Details
* ===============
*
* A triangular matrix A can be transferred to packed storage using one
* of the following program segments:
*
* UPLO = 'U': UPLO = 'L':
*
* JC = 1 JC = 1
* DO 2 J = 1, N DO 2 J = 1, N
* DO 1 I = 1, J DO 1 I = J, N
* AP(JC+I-1) = A(I,J) AP(JC+I-J) = A(I,J)
* 1 CONTINUE 1 CONTINUE
* JC = JC + J JC = JC + N - J + 1
* 2 CONTINUE 2 CONTINUE
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param diag
* @param n
* @param ap
* @param _ap_offset
* @param info
*
*/
abstract public void dtptri(java.lang.String uplo, java.lang.String diag, int n, double[] ap, int _ap_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTPTRS solves a triangular system of the form
*
* A * X = B or A**T * X = B,
*
* where A is a triangular matrix of order N stored in packed format,
* and B is an N-by-NRHS matrix. A check is made to verify that A is
* nonsingular.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The upper or lower triangular matrix A, packed columnwise in
* a linear array. The j-th column of A is stored in the array
* AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, if INFO = 0, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the i-th diagonal element of A is zero,
* indicating that the matrix is singular and the
* solutions X have not been computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param n
* @param nrhs
* @param ap
* @param b
* @param ldb
* @param info
*
*/
abstract public void dtptrs(java.lang.String uplo, java.lang.String trans, java.lang.String diag, int n, int nrhs, double[] ap, double[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTPTRS solves a triangular system of the form
*
* A * X = B or A**T * X = B,
*
* where A is a triangular matrix of order N stored in packed format,
* and B is an N-by-NRHS matrix. A check is made to verify that A is
* nonsingular.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AP (input) DOUBLE PRECISION array, dimension (N*(N+1)/2)
* The upper or lower triangular matrix A, packed columnwise in
* a linear array. The j-th column of A is stored in the array
* AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, if INFO = 0, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the i-th diagonal element of A is zero,
* indicating that the matrix is singular and the
* solutions X have not been computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param n
* @param nrhs
* @param ap
* @param _ap_offset
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void dtptrs(java.lang.String uplo, java.lang.String trans, java.lang.String diag, int n, int nrhs, double[] ap, int _ap_offset, double[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTRCON estimates the reciprocal of the condition number of a
* triangular matrix A, in either the 1-norm or the infinity-norm.
*
* The norm of A is computed and an estimate is obtained for
* norm(inv(A)), then the reciprocal of the condition number is
* computed as
* RCOND = 1 / ( norm(A) * norm(inv(A)) ).
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies whether the 1-norm condition number or the
* infinity-norm condition number is required:
* = '1' or 'O': 1-norm;
* = 'I': Infinity-norm.
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The triangular matrix A. If UPLO = 'U', the leading N-by-N
* upper triangular part of the array A contains the upper
* triangular matrix, and the strictly lower triangular part of
* A is not referenced. If UPLO = 'L', the leading N-by-N lower
* triangular part of the array A contains the lower triangular
* matrix, and the strictly upper triangular part of A is not
* referenced. If DIAG = 'U', the diagonal elements of A are
* also not referenced and are assumed to be 1.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* RCOND (output) DOUBLE PRECISION
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(norm(A) * norm(inv(A))).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param diag
* @param n
* @param a
* @param lda
* @param rcond
* @param work
* @param iwork
* @param info
*
*/
abstract public void dtrcon(java.lang.String norm, java.lang.String uplo, java.lang.String diag, int n, double[] a, int lda, org.netlib.util.doubleW rcond, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTRCON estimates the reciprocal of the condition number of a
* triangular matrix A, in either the 1-norm or the infinity-norm.
*
* The norm of A is computed and an estimate is obtained for
* norm(inv(A)), then the reciprocal of the condition number is
* computed as
* RCOND = 1 / ( norm(A) * norm(inv(A)) ).
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies whether the 1-norm condition number or the
* infinity-norm condition number is required:
* = '1' or 'O': 1-norm;
* = 'I': Infinity-norm.
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The triangular matrix A. If UPLO = 'U', the leading N-by-N
* upper triangular part of the array A contains the upper
* triangular matrix, and the strictly lower triangular part of
* A is not referenced. If UPLO = 'L', the leading N-by-N lower
* triangular part of the array A contains the lower triangular
* matrix, and the strictly upper triangular part of A is not
* referenced. If DIAG = 'U', the diagonal elements of A are
* also not referenced and are assumed to be 1.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* RCOND (output) DOUBLE PRECISION
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(norm(A) * norm(inv(A))).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param diag
* @param n
* @param a
* @param _a_offset
* @param lda
* @param rcond
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dtrcon(java.lang.String norm, java.lang.String uplo, java.lang.String diag, int n, double[] a, int _a_offset, int lda, org.netlib.util.doubleW rcond, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTREVC computes some or all of the right and/or left eigenvectors of
* a real upper quasi-triangular matrix T.
* Matrices of this type are produced by the Schur factorization of
* a real general matrix: A = Q*T*Q**T, as computed by DHSEQR.
*
* The right eigenvector x and the left eigenvector y of T corresponding
* to an eigenvalue w are defined by:
*
* T*x = w*x, (y**H)*T = w*(y**H)
*
* where y**H denotes the conjugate transpose of y.
* The eigenvalues are not input to this routine, but are read directly
* from the diagonal blocks of T.
*
* This routine returns the matrices X and/or Y of right and left
* eigenvectors of T, or the products Q*X and/or Q*Y, where Q is an
* input matrix. If Q is the orthogonal factor that reduces a matrix
* A to Schur form T, then Q*X and Q*Y are the matrices of right and
* left eigenvectors of A.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'R': compute right eigenvectors only;
* = 'L': compute left eigenvectors only;
* = 'B': compute both right and left eigenvectors.
*
* HOWMNY (input) CHARACTER*1
* = 'A': compute all right and/or left eigenvectors;
* = 'B': compute all right and/or left eigenvectors,
* backtransformed by the matrices in VR and/or VL;
* = 'S': compute selected right and/or left eigenvectors,
* as indicated by the logical array SELECT.
*
* SELECT (input/output) LOGICAL array, dimension (N)
* If HOWMNY = 'S', SELECT specifies the eigenvectors to be
* computed.
* If w(j) is a real eigenvalue, the corresponding real
* eigenvector is computed if SELECT(j) is .TRUE..
* If w(j) and w(j+1) are the real and imaginary parts of a
* complex eigenvalue, the corresponding complex eigenvector is
* computed if either SELECT(j) or SELECT(j+1) is .TRUE., and
* on exit SELECT(j) is set to .TRUE. and SELECT(j+1) is set to
* .FALSE..
* Not referenced if HOWMNY = 'A' or 'B'.
*
* N (input) INTEGER
* The order of the matrix T. N >= 0.
*
* T (input) DOUBLE PRECISION array, dimension (LDT,N)
* The upper quasi-triangular matrix T in Schur canonical form.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= max(1,N).
*
* VL (input/output) DOUBLE PRECISION array, dimension (LDVL,MM)
* On entry, if SIDE = 'L' or 'B' and HOWMNY = 'B', VL must
* contain an N-by-N matrix Q (usually the orthogonal matrix Q
* of Schur vectors returned by DHSEQR).
* On exit, if SIDE = 'L' or 'B', VL contains:
* if HOWMNY = 'A', the matrix Y of left eigenvectors of T;
* if HOWMNY = 'B', the matrix Q*Y;
* if HOWMNY = 'S', the left eigenvectors of T specified by
* SELECT, stored consecutively in the columns
* of VL, in the same order as their
* eigenvalues.
* A complex eigenvector corresponding to a complex eigenvalue
* is stored in two consecutive columns, the first holding the
* real part, and the second the imaginary part.
* Not referenced if SIDE = 'R'.
*
* LDVL (input) INTEGER
* The leading dimension of the array VL. LDVL >= 1, and if
* SIDE = 'L' or 'B', LDVL >= N.
*
* VR (input/output) DOUBLE PRECISION array, dimension (LDVR,MM)
* On entry, if SIDE = 'R' or 'B' and HOWMNY = 'B', VR must
* contain an N-by-N matrix Q (usually the orthogonal matrix Q
* of Schur vectors returned by DHSEQR).
* On exit, if SIDE = 'R' or 'B', VR contains:
* if HOWMNY = 'A', the matrix X of right eigenvectors of T;
* if HOWMNY = 'B', the matrix Q*X;
* if HOWMNY = 'S', the right eigenvectors of T specified by
* SELECT, stored consecutively in the columns
* of VR, in the same order as their
* eigenvalues.
* A complex eigenvector corresponding to a complex eigenvalue
* is stored in two consecutive columns, the first holding the
* real part and the second the imaginary part.
* Not referenced if SIDE = 'L'.
*
* LDVR (input) INTEGER
* The leading dimension of the array VR. LDVR >= 1, and if
* SIDE = 'R' or 'B', LDVR >= N.
*
* MM (input) INTEGER
* The number of columns in the arrays VL and/or VR. MM >= M.
*
* M (output) INTEGER
* The number of columns in the arrays VL and/or VR actually
* used to store the eigenvectors.
* If HOWMNY = 'A' or 'B', M is set to N.
* Each selected real eigenvector occupies one column and each
* selected complex eigenvector occupies two columns.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The algorithm used in this program is basically backward (forward)
* substitution, with scaling to make the the code robust against
* possible overflow.
*
* Each eigenvector is normalized so that the element of largest
* magnitude has magnitude 1; here the magnitude of a complex number
* (x,y) is taken to be |x| + |y|.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param howmny
* @param select
* @param n
* @param t
* @param ldt
* @param vl
* @param ldvl
* @param vr
* @param ldvr
* @param mm
* @param m
* @param work
* @param info
*
*/
abstract public void dtrevc(java.lang.String side, java.lang.String howmny, boolean[] select, int n, double[] t, int ldt, double[] vl, int ldvl, double[] vr, int ldvr, int mm, org.netlib.util.intW m, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTREVC computes some or all of the right and/or left eigenvectors of
* a real upper quasi-triangular matrix T.
* Matrices of this type are produced by the Schur factorization of
* a real general matrix: A = Q*T*Q**T, as computed by DHSEQR.
*
* The right eigenvector x and the left eigenvector y of T corresponding
* to an eigenvalue w are defined by:
*
* T*x = w*x, (y**H)*T = w*(y**H)
*
* where y**H denotes the conjugate transpose of y.
* The eigenvalues are not input to this routine, but are read directly
* from the diagonal blocks of T.
*
* This routine returns the matrices X and/or Y of right and left
* eigenvectors of T, or the products Q*X and/or Q*Y, where Q is an
* input matrix. If Q is the orthogonal factor that reduces a matrix
* A to Schur form T, then Q*X and Q*Y are the matrices of right and
* left eigenvectors of A.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'R': compute right eigenvectors only;
* = 'L': compute left eigenvectors only;
* = 'B': compute both right and left eigenvectors.
*
* HOWMNY (input) CHARACTER*1
* = 'A': compute all right and/or left eigenvectors;
* = 'B': compute all right and/or left eigenvectors,
* backtransformed by the matrices in VR and/or VL;
* = 'S': compute selected right and/or left eigenvectors,
* as indicated by the logical array SELECT.
*
* SELECT (input/output) LOGICAL array, dimension (N)
* If HOWMNY = 'S', SELECT specifies the eigenvectors to be
* computed.
* If w(j) is a real eigenvalue, the corresponding real
* eigenvector is computed if SELECT(j) is .TRUE..
* If w(j) and w(j+1) are the real and imaginary parts of a
* complex eigenvalue, the corresponding complex eigenvector is
* computed if either SELECT(j) or SELECT(j+1) is .TRUE., and
* on exit SELECT(j) is set to .TRUE. and SELECT(j+1) is set to
* .FALSE..
* Not referenced if HOWMNY = 'A' or 'B'.
*
* N (input) INTEGER
* The order of the matrix T. N >= 0.
*
* T (input) DOUBLE PRECISION array, dimension (LDT,N)
* The upper quasi-triangular matrix T in Schur canonical form.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= max(1,N).
*
* VL (input/output) DOUBLE PRECISION array, dimension (LDVL,MM)
* On entry, if SIDE = 'L' or 'B' and HOWMNY = 'B', VL must
* contain an N-by-N matrix Q (usually the orthogonal matrix Q
* of Schur vectors returned by DHSEQR).
* On exit, if SIDE = 'L' or 'B', VL contains:
* if HOWMNY = 'A', the matrix Y of left eigenvectors of T;
* if HOWMNY = 'B', the matrix Q*Y;
* if HOWMNY = 'S', the left eigenvectors of T specified by
* SELECT, stored consecutively in the columns
* of VL, in the same order as their
* eigenvalues.
* A complex eigenvector corresponding to a complex eigenvalue
* is stored in two consecutive columns, the first holding the
* real part, and the second the imaginary part.
* Not referenced if SIDE = 'R'.
*
* LDVL (input) INTEGER
* The leading dimension of the array VL. LDVL >= 1, and if
* SIDE = 'L' or 'B', LDVL >= N.
*
* VR (input/output) DOUBLE PRECISION array, dimension (LDVR,MM)
* On entry, if SIDE = 'R' or 'B' and HOWMNY = 'B', VR must
* contain an N-by-N matrix Q (usually the orthogonal matrix Q
* of Schur vectors returned by DHSEQR).
* On exit, if SIDE = 'R' or 'B', VR contains:
* if HOWMNY = 'A', the matrix X of right eigenvectors of T;
* if HOWMNY = 'B', the matrix Q*X;
* if HOWMNY = 'S', the right eigenvectors of T specified by
* SELECT, stored consecutively in the columns
* of VR, in the same order as their
* eigenvalues.
* A complex eigenvector corresponding to a complex eigenvalue
* is stored in two consecutive columns, the first holding the
* real part and the second the imaginary part.
* Not referenced if SIDE = 'L'.
*
* LDVR (input) INTEGER
* The leading dimension of the array VR. LDVR >= 1, and if
* SIDE = 'R' or 'B', LDVR >= N.
*
* MM (input) INTEGER
* The number of columns in the arrays VL and/or VR. MM >= M.
*
* M (output) INTEGER
* The number of columns in the arrays VL and/or VR actually
* used to store the eigenvectors.
* If HOWMNY = 'A' or 'B', M is set to N.
* Each selected real eigenvector occupies one column and each
* selected complex eigenvector occupies two columns.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The algorithm used in this program is basically backward (forward)
* substitution, with scaling to make the the code robust against
* possible overflow.
*
* Each eigenvector is normalized so that the element of largest
* magnitude has magnitude 1; here the magnitude of a complex number
* (x,y) is taken to be |x| + |y|.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param howmny
* @param select
* @param _select_offset
* @param n
* @param t
* @param _t_offset
* @param ldt
* @param vl
* @param _vl_offset
* @param ldvl
* @param vr
* @param _vr_offset
* @param ldvr
* @param mm
* @param m
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dtrevc(java.lang.String side, java.lang.String howmny, boolean[] select, int _select_offset, int n, double[] t, int _t_offset, int ldt, double[] vl, int _vl_offset, int ldvl, double[] vr, int _vr_offset, int ldvr, int mm, org.netlib.util.intW m, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTREXC reorders the real Schur factorization of a real matrix
* A = Q*T*Q**T, so that the diagonal block of T with row index IFST is
* moved to row ILST.
*
* The real Schur form T is reordered by an orthogonal similarity
* transformation Z**T*T*Z, and optionally the matrix Q of Schur vectors
* is updated by postmultiplying it with Z.
*
* T must be in Schur canonical form (as returned by DHSEQR), that is,
* block upper triangular with 1-by-1 and 2-by-2 diagonal blocks; each
* 2-by-2 diagonal block has its diagonal elements equal and its
* off-diagonal elements of opposite sign.
*
* Arguments
* =========
*
* COMPQ (input) CHARACTER*1
* = 'V': update the matrix Q of Schur vectors;
* = 'N': do not update Q.
*
* N (input) INTEGER
* The order of the matrix T. N >= 0.
*
* T (input/output) DOUBLE PRECISION array, dimension (LDT,N)
* On entry, the upper quasi-triangular matrix T, in Schur
* Schur canonical form.
* On exit, the reordered upper quasi-triangular matrix, again
* in Schur canonical form.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= max(1,N).
*
* Q (input/output) DOUBLE PRECISION array, dimension (LDQ,N)
* On entry, if COMPQ = 'V', the matrix Q of Schur vectors.
* On exit, if COMPQ = 'V', Q has been postmultiplied by the
* orthogonal transformation matrix Z which reorders T.
* If COMPQ = 'N', Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N).
*
* IFST (input/output) INTEGER
* ILST (input/output) INTEGER
* Specify the reordering of the diagonal blocks of T.
* The block with row index IFST is moved to row ILST, by a
* sequence of transpositions between adjacent blocks.
* On exit, if IFST pointed on entry to the second row of a
* 2-by-2 block, it is changed to point to the first row; ILST
* always points to the first row of the block in its final
* position (which may differ from its input value by +1 or -1).
* 1 <= IFST <= N; 1 <= ILST <= N.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* = 1: two adjacent blocks were too close to swap (the problem
* is very ill-conditioned); T may have been partially
* reordered, and ILST points to the first row of the
* current position of the block being moved.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param compq
* @param n
* @param t
* @param ldt
* @param q
* @param ldq
* @param ifst
* @param ilst
* @param work
* @param info
*
*/
abstract public void dtrexc(java.lang.String compq, int n, double[] t, int ldt, double[] q, int ldq, org.netlib.util.intW ifst, org.netlib.util.intW ilst, double[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTREXC reorders the real Schur factorization of a real matrix
* A = Q*T*Q**T, so that the diagonal block of T with row index IFST is
* moved to row ILST.
*
* The real Schur form T is reordered by an orthogonal similarity
* transformation Z**T*T*Z, and optionally the matrix Q of Schur vectors
* is updated by postmultiplying it with Z.
*
* T must be in Schur canonical form (as returned by DHSEQR), that is,
* block upper triangular with 1-by-1 and 2-by-2 diagonal blocks; each
* 2-by-2 diagonal block has its diagonal elements equal and its
* off-diagonal elements of opposite sign.
*
* Arguments
* =========
*
* COMPQ (input) CHARACTER*1
* = 'V': update the matrix Q of Schur vectors;
* = 'N': do not update Q.
*
* N (input) INTEGER
* The order of the matrix T. N >= 0.
*
* T (input/output) DOUBLE PRECISION array, dimension (LDT,N)
* On entry, the upper quasi-triangular matrix T, in Schur
* Schur canonical form.
* On exit, the reordered upper quasi-triangular matrix, again
* in Schur canonical form.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= max(1,N).
*
* Q (input/output) DOUBLE PRECISION array, dimension (LDQ,N)
* On entry, if COMPQ = 'V', the matrix Q of Schur vectors.
* On exit, if COMPQ = 'V', Q has been postmultiplied by the
* orthogonal transformation matrix Z which reorders T.
* If COMPQ = 'N', Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N).
*
* IFST (input/output) INTEGER
* ILST (input/output) INTEGER
* Specify the reordering of the diagonal blocks of T.
* The block with row index IFST is moved to row ILST, by a
* sequence of transpositions between adjacent blocks.
* On exit, if IFST pointed on entry to the second row of a
* 2-by-2 block, it is changed to point to the first row; ILST
* always points to the first row of the block in its final
* position (which may differ from its input value by +1 or -1).
* 1 <= IFST <= N; 1 <= ILST <= N.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* = 1: two adjacent blocks were too close to swap (the problem
* is very ill-conditioned); T may have been partially
* reordered, and ILST points to the first row of the
* current position of the block being moved.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param compq
* @param n
* @param t
* @param _t_offset
* @param ldt
* @param q
* @param _q_offset
* @param ldq
* @param ifst
* @param ilst
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void dtrexc(java.lang.String compq, int n, double[] t, int _t_offset, int ldt, double[] q, int _q_offset, int ldq, org.netlib.util.intW ifst, org.netlib.util.intW ilst, double[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTRRFS provides error bounds and backward error estimates for the
* solution to a system of linear equations with a triangular
* coefficient matrix.
*
* The solution matrix X must be computed by DTRTRS or some other
* means before entering this routine. DTRRFS does not do iterative
* refinement because doing so cannot improve the backward error.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The triangular matrix A. If UPLO = 'U', the leading N-by-N
* upper triangular part of the array A contains the upper
* triangular matrix, and the strictly lower triangular part of
* A is not referenced. If UPLO = 'L', the leading N-by-N lower
* triangular part of the array A contains the lower triangular
* matrix, and the strictly upper triangular part of A is not
* referenced. If DIAG = 'U', the diagonal elements of A are
* also not referenced and are assumed to be 1.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input) DOUBLE PRECISION array, dimension (LDX,NRHS)
* The solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param n
* @param nrhs
* @param a
* @param lda
* @param b
* @param ldb
* @param x
* @param ldx
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void dtrrfs(java.lang.String uplo, java.lang.String trans, java.lang.String diag, int n, int nrhs, double[] a, int lda, double[] b, int ldb, double[] x, int ldx, double[] ferr, double[] berr, double[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTRRFS provides error bounds and backward error estimates for the
* solution to a system of linear equations with a triangular
* coefficient matrix.
*
* The solution matrix X must be computed by DTRTRS or some other
* means before entering this routine. DTRRFS does not do iterative
* refinement because doing so cannot improve the backward error.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The triangular matrix A. If UPLO = 'U', the leading N-by-N
* upper triangular part of the array A contains the upper
* triangular matrix, and the strictly lower triangular part of
* A is not referenced. If UPLO = 'L', the leading N-by-N lower
* triangular part of the array A contains the lower triangular
* matrix, and the strictly upper triangular part of A is not
* referenced. If DIAG = 'U', the diagonal elements of A are
* also not referenced and are assumed to be 1.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input) DOUBLE PRECISION array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input) DOUBLE PRECISION array, dimension (LDX,NRHS)
* The solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) DOUBLE PRECISION array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) DOUBLE PRECISION array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dtrrfs(java.lang.String uplo, java.lang.String trans, java.lang.String diag, int n, int nrhs, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, double[] x, int _x_offset, int ldx, double[] ferr, int _ferr_offset, double[] berr, int _berr_offset, double[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTRSEN reorders the real Schur factorization of a real matrix
* A = Q*T*Q**T, so that a selected cluster of eigenvalues appears in
* the leading diagonal blocks of the upper quasi-triangular matrix T,
* and the leading columns of Q form an orthonormal basis of the
* corresponding right invariant subspace.
*
* Optionally the routine computes the reciprocal condition numbers of
* the cluster of eigenvalues and/or the invariant subspace.
*
* T must be in Schur canonical form (as returned by DHSEQR), that is,
* block upper triangular with 1-by-1 and 2-by-2 diagonal blocks; each
* 2-by-2 diagonal block has its diagonal elemnts equal and its
* off-diagonal elements of opposite sign.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* Specifies whether condition numbers are required for the
* cluster of eigenvalues (S) or the invariant subspace (SEP):
* = 'N': none;
* = 'E': for eigenvalues only (S);
* = 'V': for invariant subspace only (SEP);
* = 'B': for both eigenvalues and invariant subspace (S and
* SEP).
*
* COMPQ (input) CHARACTER*1
* = 'V': update the matrix Q of Schur vectors;
* = 'N': do not update Q.
*
* SELECT (input) LOGICAL array, dimension (N)
* SELECT specifies the eigenvalues in the selected cluster. To
* select a real eigenvalue w(j), SELECT(j) must be set to
* .TRUE.. To select a complex conjugate pair of eigenvalues
* w(j) and w(j+1), corresponding to a 2-by-2 diagonal block,
* either SELECT(j) or SELECT(j+1) or both must be set to
* .TRUE.; a complex conjugate pair of eigenvalues must be
* either both included in the cluster or both excluded.
*
* N (input) INTEGER
* The order of the matrix T. N >= 0.
*
* T (input/output) DOUBLE PRECISION array, dimension (LDT,N)
* On entry, the upper quasi-triangular matrix T, in Schur
* canonical form.
* On exit, T is overwritten by the reordered matrix T, again in
* Schur canonical form, with the selected eigenvalues in the
* leading diagonal blocks.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= max(1,N).
*
* Q (input/output) DOUBLE PRECISION array, dimension (LDQ,N)
* On entry, if COMPQ = 'V', the matrix Q of Schur vectors.
* On exit, if COMPQ = 'V', Q has been postmultiplied by the
* orthogonal transformation matrix which reorders T; the
* leading M columns of Q form an orthonormal basis for the
* specified invariant subspace.
* If COMPQ = 'N', Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q.
* LDQ >= 1; and if COMPQ = 'V', LDQ >= N.
*
* WR (output) DOUBLE PRECISION array, dimension (N)
* WI (output) DOUBLE PRECISION array, dimension (N)
* The real and imaginary parts, respectively, of the reordered
* eigenvalues of T. The eigenvalues are stored in the same
* order as on the diagonal of T, with WR(i) = T(i,i) and, if
* T(i:i+1,i:i+1) is a 2-by-2 diagonal block, WI(i) > 0 and
* WI(i+1) = -WI(i). Note that if a complex eigenvalue is
* sufficiently ill-conditioned, then its value may differ
* significantly from its value before reordering.
*
* M (output) INTEGER
* The dimension of the specified invariant subspace.
* 0 < = M <= N.
*
* S (output) DOUBLE PRECISION
* If JOB = 'E' or 'B', S is a lower bound on the reciprocal
* condition number for the selected cluster of eigenvalues.
* S cannot underestimate the true reciprocal condition number
* by more than a factor of sqrt(N). If M = 0 or N, S = 1.
* If JOB = 'N' or 'V', S is not referenced.
*
* SEP (output) DOUBLE PRECISION
* If JOB = 'V' or 'B', SEP is the estimated reciprocal
* condition number of the specified invariant subspace. If
* M = 0 or N, SEP = norm(T).
* If JOB = 'N' or 'E', SEP is not referenced.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If JOB = 'N', LWORK >= max(1,N);
* if JOB = 'E', LWORK >= max(1,M*(N-M));
* if JOB = 'V' or 'B', LWORK >= max(1,2*M*(N-M)).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If JOB = 'N' or 'E', LIWORK >= 1;
* if JOB = 'V' or 'B', LIWORK >= max(1,M*(N-M)).
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal size of the IWORK array,
* returns this value as the first entry of the IWORK array, and
* no error message related to LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* = 1: reordering of T failed because some eigenvalues are too
* close to separate (the problem is very ill-conditioned);
* T may have been partially reordered, and WR and WI
* contain the eigenvalues in the same order as in T; S and
* SEP (if requested) are set to zero.
*
* Further Details
* ===============
*
* DTRSEN first collects the selected eigenvalues by computing an
* orthogonal transformation Z to move them to the top left corner of T.
* In other words, the selected eigenvalues are the eigenvalues of T11
* in:
*
* Z'*T*Z = ( T11 T12 ) n1
* ( 0 T22 ) n2
* n1 n2
*
* where N = n1+n2 and Z' means the transpose of Z. The first n1 columns
* of Z span the specified invariant subspace of T.
*
* If T has been obtained from the real Schur factorization of a matrix
* A = Q*T*Q', then the reordered real Schur factorization of A is given
* by A = (Q*Z)*(Z'*T*Z)*(Q*Z)', and the first n1 columns of Q*Z span
* the corresponding invariant subspace of A.
*
* The reciprocal condition number of the average of the eigenvalues of
* T11 may be returned in S. S lies between 0 (very badly conditioned)
* and 1 (very well conditioned). It is computed as follows. First we
* compute R so that
*
* P = ( I R ) n1
* ( 0 0 ) n2
* n1 n2
*
* is the projector on the invariant subspace associated with T11.
* R is the solution of the Sylvester equation:
*
* T11*R - R*T22 = T12.
*
* Let F-norm(M) denote the Frobenius-norm of M and 2-norm(M) denote
* the two-norm of M. Then S is computed as the lower bound
*
* (1 + F-norm(R)**2)**(-1/2)
*
* on the reciprocal of 2-norm(P), the true reciprocal condition number.
* S cannot underestimate 1 / 2-norm(P) by more than a factor of
* sqrt(N).
*
* An approximate error bound for the computed average of the
* eigenvalues of T11 is
*
* EPS * norm(T) / S
*
* where EPS is the machine precision.
*
* The reciprocal condition number of the right invariant subspace
* spanned by the first n1 columns of Z (or of Q*Z) is returned in SEP.
* SEP is defined as the separation of T11 and T22:
*
* sep( T11, T22 ) = sigma-min( C )
*
* where sigma-min(C) is the smallest singular value of the
* n1*n2-by-n1*n2 matrix
*
* C = kprod( I(n2), T11 ) - kprod( transpose(T22), I(n1) )
*
* I(m) is an m by m identity matrix, and kprod denotes the Kronecker
* product. We estimate sigma-min(C) by the reciprocal of an estimate of
* the 1-norm of inverse(C). The true reciprocal 1-norm of inverse(C)
* cannot differ from sigma-min(C) by more than a factor of sqrt(n1*n2).
*
* When SEP is small, small changes in T can cause large changes in
* the invariant subspace. An approximate bound on the maximum angular
* error in the computed right invariant subspace is
*
* EPS * norm(T) / SEP
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param compq
* @param select
* @param n
* @param t
* @param ldt
* @param q
* @param ldq
* @param wr
* @param wi
* @param m
* @param s
* @param sep
* @param work
* @param lwork
* @param iwork
* @param liwork
* @param info
*
*/
abstract public void dtrsen(java.lang.String job, java.lang.String compq, boolean[] select, int n, double[] t, int ldt, double[] q, int ldq, double[] wr, double[] wi, org.netlib.util.intW m, org.netlib.util.doubleW s, org.netlib.util.doubleW sep, double[] work, int lwork, int[] iwork, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTRSEN reorders the real Schur factorization of a real matrix
* A = Q*T*Q**T, so that a selected cluster of eigenvalues appears in
* the leading diagonal blocks of the upper quasi-triangular matrix T,
* and the leading columns of Q form an orthonormal basis of the
* corresponding right invariant subspace.
*
* Optionally the routine computes the reciprocal condition numbers of
* the cluster of eigenvalues and/or the invariant subspace.
*
* T must be in Schur canonical form (as returned by DHSEQR), that is,
* block upper triangular with 1-by-1 and 2-by-2 diagonal blocks; each
* 2-by-2 diagonal block has its diagonal elemnts equal and its
* off-diagonal elements of opposite sign.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* Specifies whether condition numbers are required for the
* cluster of eigenvalues (S) or the invariant subspace (SEP):
* = 'N': none;
* = 'E': for eigenvalues only (S);
* = 'V': for invariant subspace only (SEP);
* = 'B': for both eigenvalues and invariant subspace (S and
* SEP).
*
* COMPQ (input) CHARACTER*1
* = 'V': update the matrix Q of Schur vectors;
* = 'N': do not update Q.
*
* SELECT (input) LOGICAL array, dimension (N)
* SELECT specifies the eigenvalues in the selected cluster. To
* select a real eigenvalue w(j), SELECT(j) must be set to
* .TRUE.. To select a complex conjugate pair of eigenvalues
* w(j) and w(j+1), corresponding to a 2-by-2 diagonal block,
* either SELECT(j) or SELECT(j+1) or both must be set to
* .TRUE.; a complex conjugate pair of eigenvalues must be
* either both included in the cluster or both excluded.
*
* N (input) INTEGER
* The order of the matrix T. N >= 0.
*
* T (input/output) DOUBLE PRECISION array, dimension (LDT,N)
* On entry, the upper quasi-triangular matrix T, in Schur
* canonical form.
* On exit, T is overwritten by the reordered matrix T, again in
* Schur canonical form, with the selected eigenvalues in the
* leading diagonal blocks.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= max(1,N).
*
* Q (input/output) DOUBLE PRECISION array, dimension (LDQ,N)
* On entry, if COMPQ = 'V', the matrix Q of Schur vectors.
* On exit, if COMPQ = 'V', Q has been postmultiplied by the
* orthogonal transformation matrix which reorders T; the
* leading M columns of Q form an orthonormal basis for the
* specified invariant subspace.
* If COMPQ = 'N', Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q.
* LDQ >= 1; and if COMPQ = 'V', LDQ >= N.
*
* WR (output) DOUBLE PRECISION array, dimension (N)
* WI (output) DOUBLE PRECISION array, dimension (N)
* The real and imaginary parts, respectively, of the reordered
* eigenvalues of T. The eigenvalues are stored in the same
* order as on the diagonal of T, with WR(i) = T(i,i) and, if
* T(i:i+1,i:i+1) is a 2-by-2 diagonal block, WI(i) > 0 and
* WI(i+1) = -WI(i). Note that if a complex eigenvalue is
* sufficiently ill-conditioned, then its value may differ
* significantly from its value before reordering.
*
* M (output) INTEGER
* The dimension of the specified invariant subspace.
* 0 < = M <= N.
*
* S (output) DOUBLE PRECISION
* If JOB = 'E' or 'B', S is a lower bound on the reciprocal
* condition number for the selected cluster of eigenvalues.
* S cannot underestimate the true reciprocal condition number
* by more than a factor of sqrt(N). If M = 0 or N, S = 1.
* If JOB = 'N' or 'V', S is not referenced.
*
* SEP (output) DOUBLE PRECISION
* If JOB = 'V' or 'B', SEP is the estimated reciprocal
* condition number of the specified invariant subspace. If
* M = 0 or N, SEP = norm(T).
* If JOB = 'N' or 'E', SEP is not referenced.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If JOB = 'N', LWORK >= max(1,N);
* if JOB = 'E', LWORK >= max(1,M*(N-M));
* if JOB = 'V' or 'B', LWORK >= max(1,2*M*(N-M)).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If JOB = 'N' or 'E', LIWORK >= 1;
* if JOB = 'V' or 'B', LIWORK >= max(1,M*(N-M)).
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal size of the IWORK array,
* returns this value as the first entry of the IWORK array, and
* no error message related to LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* = 1: reordering of T failed because some eigenvalues are too
* close to separate (the problem is very ill-conditioned);
* T may have been partially reordered, and WR and WI
* contain the eigenvalues in the same order as in T; S and
* SEP (if requested) are set to zero.
*
* Further Details
* ===============
*
* DTRSEN first collects the selected eigenvalues by computing an
* orthogonal transformation Z to move them to the top left corner of T.
* In other words, the selected eigenvalues are the eigenvalues of T11
* in:
*
* Z'*T*Z = ( T11 T12 ) n1
* ( 0 T22 ) n2
* n1 n2
*
* where N = n1+n2 and Z' means the transpose of Z. The first n1 columns
* of Z span the specified invariant subspace of T.
*
* If T has been obtained from the real Schur factorization of a matrix
* A = Q*T*Q', then the reordered real Schur factorization of A is given
* by A = (Q*Z)*(Z'*T*Z)*(Q*Z)', and the first n1 columns of Q*Z span
* the corresponding invariant subspace of A.
*
* The reciprocal condition number of the average of the eigenvalues of
* T11 may be returned in S. S lies between 0 (very badly conditioned)
* and 1 (very well conditioned). It is computed as follows. First we
* compute R so that
*
* P = ( I R ) n1
* ( 0 0 ) n2
* n1 n2
*
* is the projector on the invariant subspace associated with T11.
* R is the solution of the Sylvester equation:
*
* T11*R - R*T22 = T12.
*
* Let F-norm(M) denote the Frobenius-norm of M and 2-norm(M) denote
* the two-norm of M. Then S is computed as the lower bound
*
* (1 + F-norm(R)**2)**(-1/2)
*
* on the reciprocal of 2-norm(P), the true reciprocal condition number.
* S cannot underestimate 1 / 2-norm(P) by more than a factor of
* sqrt(N).
*
* An approximate error bound for the computed average of the
* eigenvalues of T11 is
*
* EPS * norm(T) / S
*
* where EPS is the machine precision.
*
* The reciprocal condition number of the right invariant subspace
* spanned by the first n1 columns of Z (or of Q*Z) is returned in SEP.
* SEP is defined as the separation of T11 and T22:
*
* sep( T11, T22 ) = sigma-min( C )
*
* where sigma-min(C) is the smallest singular value of the
* n1*n2-by-n1*n2 matrix
*
* C = kprod( I(n2), T11 ) - kprod( transpose(T22), I(n1) )
*
* I(m) is an m by m identity matrix, and kprod denotes the Kronecker
* product. We estimate sigma-min(C) by the reciprocal of an estimate of
* the 1-norm of inverse(C). The true reciprocal 1-norm of inverse(C)
* cannot differ from sigma-min(C) by more than a factor of sqrt(n1*n2).
*
* When SEP is small, small changes in T can cause large changes in
* the invariant subspace. An approximate bound on the maximum angular
* error in the computed right invariant subspace is
*
* EPS * norm(T) / SEP
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param compq
* @param select
* @param _select_offset
* @param n
* @param t
* @param _t_offset
* @param ldt
* @param q
* @param _q_offset
* @param ldq
* @param wr
* @param _wr_offset
* @param wi
* @param _wi_offset
* @param m
* @param s
* @param sep
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param liwork
* @param info
*
*/
abstract public void dtrsen(java.lang.String job, java.lang.String compq, boolean[] select, int _select_offset, int n, double[] t, int _t_offset, int ldt, double[] q, int _q_offset, int ldq, double[] wr, int _wr_offset, double[] wi, int _wi_offset, org.netlib.util.intW m, org.netlib.util.doubleW s, org.netlib.util.doubleW sep, double[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTRSNA estimates reciprocal condition numbers for specified
* eigenvalues and/or right eigenvectors of a real upper
* quasi-triangular matrix T (or of any matrix Q*T*Q**T with Q
* orthogonal).
*
* T must be in Schur canonical form (as returned by DHSEQR), that is,
* block upper triangular with 1-by-1 and 2-by-2 diagonal blocks; each
* 2-by-2 diagonal block has its diagonal elements equal and its
* off-diagonal elements of opposite sign.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* Specifies whether condition numbers are required for
* eigenvalues (S) or eigenvectors (SEP):
* = 'E': for eigenvalues only (S);
* = 'V': for eigenvectors only (SEP);
* = 'B': for both eigenvalues and eigenvectors (S and SEP).
*
* HOWMNY (input) CHARACTER*1
* = 'A': compute condition numbers for all eigenpairs;
* = 'S': compute condition numbers for selected eigenpairs
* specified by the array SELECT.
*
* SELECT (input) LOGICAL array, dimension (N)
* If HOWMNY = 'S', SELECT specifies the eigenpairs for which
* condition numbers are required. To select condition numbers
* for the eigenpair corresponding to a real eigenvalue w(j),
* SELECT(j) must be set to .TRUE.. To select condition numbers
* corresponding to a complex conjugate pair of eigenvalues w(j)
* and w(j+1), either SELECT(j) or SELECT(j+1) or both, must be
* set to .TRUE..
* If HOWMNY = 'A', SELECT is not referenced.
*
* N (input) INTEGER
* The order of the matrix T. N >= 0.
*
* T (input) DOUBLE PRECISION array, dimension (LDT,N)
* The upper quasi-triangular matrix T, in Schur canonical form.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= max(1,N).
*
* VL (input) DOUBLE PRECISION array, dimension (LDVL,M)
* If JOB = 'E' or 'B', VL must contain left eigenvectors of T
* (or of any Q*T*Q**T with Q orthogonal), corresponding to the
* eigenpairs specified by HOWMNY and SELECT. The eigenvectors
* must be stored in consecutive columns of VL, as returned by
* DHSEIN or DTREVC.
* If JOB = 'V', VL is not referenced.
*
* LDVL (input) INTEGER
* The leading dimension of the array VL.
* LDVL >= 1; and if JOB = 'E' or 'B', LDVL >= N.
*
* VR (input) DOUBLE PRECISION array, dimension (LDVR,M)
* If JOB = 'E' or 'B', VR must contain right eigenvectors of T
* (or of any Q*T*Q**T with Q orthogonal), corresponding to the
* eigenpairs specified by HOWMNY and SELECT. The eigenvectors
* must be stored in consecutive columns of VR, as returned by
* DHSEIN or DTREVC.
* If JOB = 'V', VR is not referenced.
*
* LDVR (input) INTEGER
* The leading dimension of the array VR.
* LDVR >= 1; and if JOB = 'E' or 'B', LDVR >= N.
*
* S (output) DOUBLE PRECISION array, dimension (MM)
* If JOB = 'E' or 'B', the reciprocal condition numbers of the
* selected eigenvalues, stored in consecutive elements of the
* array. For a complex conjugate pair of eigenvalues two
* consecutive elements of S are set to the same value. Thus
* S(j), SEP(j), and the j-th columns of VL and VR all
* correspond to the same eigenpair (but not in general the
* j-th eigenpair, unless all eigenpairs are selected).
* If JOB = 'V', S is not referenced.
*
* SEP (output) DOUBLE PRECISION array, dimension (MM)
* If JOB = 'V' or 'B', the estimated reciprocal condition
* numbers of the selected eigenvectors, stored in consecutive
* elements of the array. For a complex eigenvector two
* consecutive elements of SEP are set to the same value. If
* the eigenvalues cannot be reordered to compute SEP(j), SEP(j)
* is set to 0; this can only occur when the true value would be
* very small anyway.
* If JOB = 'E', SEP is not referenced.
*
* MM (input) INTEGER
* The number of elements in the arrays S (if JOB = 'E' or 'B')
* and/or SEP (if JOB = 'V' or 'B'). MM >= M.
*
* M (output) INTEGER
* The number of elements of the arrays S and/or SEP actually
* used to store the estimated condition numbers.
* If HOWMNY = 'A', M is set to N.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (LDWORK,N+6)
* If JOB = 'E', WORK is not referenced.
*
* LDWORK (input) INTEGER
* The leading dimension of the array WORK.
* LDWORK >= 1; and if JOB = 'V' or 'B', LDWORK >= N.
*
* IWORK (workspace) INTEGER array, dimension (2*(N-1))
* If JOB = 'E', IWORK is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The reciprocal of the condition number of an eigenvalue lambda is
* defined as
*
* S(lambda) = |v'*u| / (norm(u)*norm(v))
*
* where u and v are the right and left eigenvectors of T corresponding
* to lambda; v' denotes the conjugate-transpose of v, and norm(u)
* denotes the Euclidean norm. These reciprocal condition numbers always
* lie between zero (very badly conditioned) and one (very well
* conditioned). If n = 1, S(lambda) is defined to be 1.
*
* An approximate error bound for a computed eigenvalue W(i) is given by
*
* EPS * norm(T) / S(i)
*
* where EPS is the machine precision.
*
* The reciprocal of the condition number of the right eigenvector u
* corresponding to lambda is defined as follows. Suppose
*
* T = ( lambda c )
* ( 0 T22 )
*
* Then the reciprocal condition number is
*
* SEP( lambda, T22 ) = sigma-min( T22 - lambda*I )
*
* where sigma-min denotes the smallest singular value. We approximate
* the smallest singular value by the reciprocal of an estimate of the
* one-norm of the inverse of T22 - lambda*I. If n = 1, SEP(1) is
* defined to be abs(T(1,1)).
*
* An approximate error bound for a computed right eigenvector VR(i)
* is given by
*
* EPS * norm(T) / SEP(i)
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param howmny
* @param select
* @param n
* @param t
* @param ldt
* @param vl
* @param ldvl
* @param vr
* @param ldvr
* @param s
* @param sep
* @param mm
* @param m
* @param work
* @param ldwork
* @param iwork
* @param info
*
*/
abstract public void dtrsna(java.lang.String job, java.lang.String howmny, boolean[] select, int n, double[] t, int ldt, double[] vl, int ldvl, double[] vr, int ldvr, double[] s, double[] sep, int mm, org.netlib.util.intW m, double[] work, int ldwork, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTRSNA estimates reciprocal condition numbers for specified
* eigenvalues and/or right eigenvectors of a real upper
* quasi-triangular matrix T (or of any matrix Q*T*Q**T with Q
* orthogonal).
*
* T must be in Schur canonical form (as returned by DHSEQR), that is,
* block upper triangular with 1-by-1 and 2-by-2 diagonal blocks; each
* 2-by-2 diagonal block has its diagonal elements equal and its
* off-diagonal elements of opposite sign.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* Specifies whether condition numbers are required for
* eigenvalues (S) or eigenvectors (SEP):
* = 'E': for eigenvalues only (S);
* = 'V': for eigenvectors only (SEP);
* = 'B': for both eigenvalues and eigenvectors (S and SEP).
*
* HOWMNY (input) CHARACTER*1
* = 'A': compute condition numbers for all eigenpairs;
* = 'S': compute condition numbers for selected eigenpairs
* specified by the array SELECT.
*
* SELECT (input) LOGICAL array, dimension (N)
* If HOWMNY = 'S', SELECT specifies the eigenpairs for which
* condition numbers are required. To select condition numbers
* for the eigenpair corresponding to a real eigenvalue w(j),
* SELECT(j) must be set to .TRUE.. To select condition numbers
* corresponding to a complex conjugate pair of eigenvalues w(j)
* and w(j+1), either SELECT(j) or SELECT(j+1) or both, must be
* set to .TRUE..
* If HOWMNY = 'A', SELECT is not referenced.
*
* N (input) INTEGER
* The order of the matrix T. N >= 0.
*
* T (input) DOUBLE PRECISION array, dimension (LDT,N)
* The upper quasi-triangular matrix T, in Schur canonical form.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= max(1,N).
*
* VL (input) DOUBLE PRECISION array, dimension (LDVL,M)
* If JOB = 'E' or 'B', VL must contain left eigenvectors of T
* (or of any Q*T*Q**T with Q orthogonal), corresponding to the
* eigenpairs specified by HOWMNY and SELECT. The eigenvectors
* must be stored in consecutive columns of VL, as returned by
* DHSEIN or DTREVC.
* If JOB = 'V', VL is not referenced.
*
* LDVL (input) INTEGER
* The leading dimension of the array VL.
* LDVL >= 1; and if JOB = 'E' or 'B', LDVL >= N.
*
* VR (input) DOUBLE PRECISION array, dimension (LDVR,M)
* If JOB = 'E' or 'B', VR must contain right eigenvectors of T
* (or of any Q*T*Q**T with Q orthogonal), corresponding to the
* eigenpairs specified by HOWMNY and SELECT. The eigenvectors
* must be stored in consecutive columns of VR, as returned by
* DHSEIN or DTREVC.
* If JOB = 'V', VR is not referenced.
*
* LDVR (input) INTEGER
* The leading dimension of the array VR.
* LDVR >= 1; and if JOB = 'E' or 'B', LDVR >= N.
*
* S (output) DOUBLE PRECISION array, dimension (MM)
* If JOB = 'E' or 'B', the reciprocal condition numbers of the
* selected eigenvalues, stored in consecutive elements of the
* array. For a complex conjugate pair of eigenvalues two
* consecutive elements of S are set to the same value. Thus
* S(j), SEP(j), and the j-th columns of VL and VR all
* correspond to the same eigenpair (but not in general the
* j-th eigenpair, unless all eigenpairs are selected).
* If JOB = 'V', S is not referenced.
*
* SEP (output) DOUBLE PRECISION array, dimension (MM)
* If JOB = 'V' or 'B', the estimated reciprocal condition
* numbers of the selected eigenvectors, stored in consecutive
* elements of the array. For a complex eigenvector two
* consecutive elements of SEP are set to the same value. If
* the eigenvalues cannot be reordered to compute SEP(j), SEP(j)
* is set to 0; this can only occur when the true value would be
* very small anyway.
* If JOB = 'E', SEP is not referenced.
*
* MM (input) INTEGER
* The number of elements in the arrays S (if JOB = 'E' or 'B')
* and/or SEP (if JOB = 'V' or 'B'). MM >= M.
*
* M (output) INTEGER
* The number of elements of the arrays S and/or SEP actually
* used to store the estimated condition numbers.
* If HOWMNY = 'A', M is set to N.
*
* WORK (workspace) DOUBLE PRECISION array, dimension (LDWORK,N+6)
* If JOB = 'E', WORK is not referenced.
*
* LDWORK (input) INTEGER
* The leading dimension of the array WORK.
* LDWORK >= 1; and if JOB = 'V' or 'B', LDWORK >= N.
*
* IWORK (workspace) INTEGER array, dimension (2*(N-1))
* If JOB = 'E', IWORK is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The reciprocal of the condition number of an eigenvalue lambda is
* defined as
*
* S(lambda) = |v'*u| / (norm(u)*norm(v))
*
* where u and v are the right and left eigenvectors of T corresponding
* to lambda; v' denotes the conjugate-transpose of v, and norm(u)
* denotes the Euclidean norm. These reciprocal condition numbers always
* lie between zero (very badly conditioned) and one (very well
* conditioned). If n = 1, S(lambda) is defined to be 1.
*
* An approximate error bound for a computed eigenvalue W(i) is given by
*
* EPS * norm(T) / S(i)
*
* where EPS is the machine precision.
*
* The reciprocal of the condition number of the right eigenvector u
* corresponding to lambda is defined as follows. Suppose
*
* T = ( lambda c )
* ( 0 T22 )
*
* Then the reciprocal condition number is
*
* SEP( lambda, T22 ) = sigma-min( T22 - lambda*I )
*
* where sigma-min denotes the smallest singular value. We approximate
* the smallest singular value by the reciprocal of an estimate of the
* one-norm of the inverse of T22 - lambda*I. If n = 1, SEP(1) is
* defined to be abs(T(1,1)).
*
* An approximate error bound for a computed right eigenvector VR(i)
* is given by
*
* EPS * norm(T) / SEP(i)
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param howmny
* @param select
* @param _select_offset
* @param n
* @param t
* @param _t_offset
* @param ldt
* @param vl
* @param _vl_offset
* @param ldvl
* @param vr
* @param _vr_offset
* @param ldvr
* @param s
* @param _s_offset
* @param sep
* @param _sep_offset
* @param mm
* @param m
* @param work
* @param _work_offset
* @param ldwork
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void dtrsna(java.lang.String job, java.lang.String howmny, boolean[] select, int _select_offset, int n, double[] t, int _t_offset, int ldt, double[] vl, int _vl_offset, int ldvl, double[] vr, int _vr_offset, int ldvr, double[] s, int _s_offset, double[] sep, int _sep_offset, int mm, org.netlib.util.intW m, double[] work, int _work_offset, int ldwork, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTRSYL solves the real Sylvester matrix equation:
*
* op(A)*X + X*op(B) = scale*C or
* op(A)*X - X*op(B) = scale*C,
*
* where op(A) = A or A**T, and A and B are both upper quasi-
* triangular. A is M-by-M and B is N-by-N; the right hand side C and
* the solution X are M-by-N; and scale is an output scale factor, set
* <= 1 to avoid overflow in X.
*
* A and B must be in Schur canonical form (as returned by DHSEQR), that
* is, block upper triangular with 1-by-1 and 2-by-2 diagonal blocks;
* each 2-by-2 diagonal block has its diagonal elements equal and its
* off-diagonal elements of opposite sign.
*
* Arguments
* =========
*
* TRANA (input) CHARACTER*1
* Specifies the option op(A):
* = 'N': op(A) = A (No transpose)
* = 'T': op(A) = A**T (Transpose)
* = 'C': op(A) = A**H (Conjugate transpose = Transpose)
*
* TRANB (input) CHARACTER*1
* Specifies the option op(B):
* = 'N': op(B) = B (No transpose)
* = 'T': op(B) = B**T (Transpose)
* = 'C': op(B) = B**H (Conjugate transpose = Transpose)
*
* ISGN (input) INTEGER
* Specifies the sign in the equation:
* = +1: solve op(A)*X + X*op(B) = scale*C
* = -1: solve op(A)*X - X*op(B) = scale*C
*
* M (input) INTEGER
* The order of the matrix A, and the number of rows in the
* matrices X and C. M >= 0.
*
* N (input) INTEGER
* The order of the matrix B, and the number of columns in the
* matrices X and C. N >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,M)
* The upper quasi-triangular matrix A, in Schur canonical form.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input) DOUBLE PRECISION array, dimension (LDB,N)
* The upper quasi-triangular matrix B, in Schur canonical form.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the M-by-N right hand side matrix C.
* On exit, C is overwritten by the solution matrix X.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M)
*
* SCALE (output) DOUBLE PRECISION
* The scale factor, scale, set <= 1 to avoid overflow in X.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* = 1: A and B have common or very close eigenvalues; perturbed
* values were used to solve the equation (but the matrices
* A and B are unchanged).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param trana
* @param tranb
* @param isgn
* @param m
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param c
* @param Ldc
* @param scale
* @param info
*
*/
abstract public void dtrsyl(java.lang.String trana, java.lang.String tranb, int isgn, int m, int n, double[] a, int lda, double[] b, int ldb, double[] c, int Ldc, org.netlib.util.doubleW scale, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTRSYL solves the real Sylvester matrix equation:
*
* op(A)*X + X*op(B) = scale*C or
* op(A)*X - X*op(B) = scale*C,
*
* where op(A) = A or A**T, and A and B are both upper quasi-
* triangular. A is M-by-M and B is N-by-N; the right hand side C and
* the solution X are M-by-N; and scale is an output scale factor, set
* <= 1 to avoid overflow in X.
*
* A and B must be in Schur canonical form (as returned by DHSEQR), that
* is, block upper triangular with 1-by-1 and 2-by-2 diagonal blocks;
* each 2-by-2 diagonal block has its diagonal elements equal and its
* off-diagonal elements of opposite sign.
*
* Arguments
* =========
*
* TRANA (input) CHARACTER*1
* Specifies the option op(A):
* = 'N': op(A) = A (No transpose)
* = 'T': op(A) = A**T (Transpose)
* = 'C': op(A) = A**H (Conjugate transpose = Transpose)
*
* TRANB (input) CHARACTER*1
* Specifies the option op(B):
* = 'N': op(B) = B (No transpose)
* = 'T': op(B) = B**T (Transpose)
* = 'C': op(B) = B**H (Conjugate transpose = Transpose)
*
* ISGN (input) INTEGER
* Specifies the sign in the equation:
* = +1: solve op(A)*X + X*op(B) = scale*C
* = -1: solve op(A)*X - X*op(B) = scale*C
*
* M (input) INTEGER
* The order of the matrix A, and the number of rows in the
* matrices X and C. M >= 0.
*
* N (input) INTEGER
* The order of the matrix B, and the number of columns in the
* matrices X and C. N >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,M)
* The upper quasi-triangular matrix A, in Schur canonical form.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input) DOUBLE PRECISION array, dimension (LDB,N)
* The upper quasi-triangular matrix B, in Schur canonical form.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* C (input/output) DOUBLE PRECISION array, dimension (LDC,N)
* On entry, the M-by-N right hand side matrix C.
* On exit, C is overwritten by the solution matrix X.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M)
*
* SCALE (output) DOUBLE PRECISION
* The scale factor, scale, set <= 1 to avoid overflow in X.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* = 1: A and B have common or very close eigenvalues; perturbed
* values were used to solve the equation (but the matrices
* A and B are unchanged).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param trana
* @param tranb
* @param isgn
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param c
* @param _c_offset
* @param Ldc
* @param scale
* @param info
*
*/
abstract public void dtrsyl(java.lang.String trana, java.lang.String tranb, int isgn, int m, int n, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, double[] c, int _c_offset, int Ldc, org.netlib.util.doubleW scale, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTRTI2 computes the inverse of a real upper or lower triangular
* matrix.
*
* This is the Level 2 BLAS version of the algorithm.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the matrix A is upper or lower triangular.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* DIAG (input) CHARACTER*1
* Specifies whether or not the matrix A is unit triangular.
* = 'N': Non-unit triangular
* = 'U': Unit triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the triangular matrix A. If UPLO = 'U', the
* leading n by n upper triangular part of the array A contains
* the upper triangular matrix, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading n by n lower triangular part of the array A contains
* the lower triangular matrix, and the strictly upper
* triangular part of A is not referenced. If DIAG = 'U', the
* diagonal elements of A are also not referenced and are
* assumed to be 1.
*
* On exit, the (triangular) inverse of the original matrix, in
* the same storage format.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param diag
* @param n
* @param a
* @param lda
* @param info
*
*/
abstract public void dtrti2(java.lang.String uplo, java.lang.String diag, int n, double[] a, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTRTI2 computes the inverse of a real upper or lower triangular
* matrix.
*
* This is the Level 2 BLAS version of the algorithm.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the matrix A is upper or lower triangular.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* DIAG (input) CHARACTER*1
* Specifies whether or not the matrix A is unit triangular.
* = 'N': Non-unit triangular
* = 'U': Unit triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the triangular matrix A. If UPLO = 'U', the
* leading n by n upper triangular part of the array A contains
* the upper triangular matrix, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading n by n lower triangular part of the array A contains
* the lower triangular matrix, and the strictly upper
* triangular part of A is not referenced. If DIAG = 'U', the
* diagonal elements of A are also not referenced and are
* assumed to be 1.
*
* On exit, the (triangular) inverse of the original matrix, in
* the same storage format.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param diag
* @param n
* @param a
* @param _a_offset
* @param lda
* @param info
*
*/
abstract public void dtrti2(java.lang.String uplo, java.lang.String diag, int n, double[] a, int _a_offset, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTRTRI computes the inverse of a real upper or lower triangular
* matrix A.
*
* This is the Level 3 BLAS version of the algorithm.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the triangular matrix A. If UPLO = 'U', the
* leading N-by-N upper triangular part of the array A contains
* the upper triangular matrix, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading N-by-N lower triangular part of the array A contains
* the lower triangular matrix, and the strictly upper
* triangular part of A is not referenced. If DIAG = 'U', the
* diagonal elements of A are also not referenced and are
* assumed to be 1.
* On exit, the (triangular) inverse of the original matrix, in
* the same storage format.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, A(i,i) is exactly zero. The triangular
* matrix is singular and its inverse can not be computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param diag
* @param n
* @param a
* @param lda
* @param info
*
*/
abstract public void dtrtri(java.lang.String uplo, java.lang.String diag, int n, double[] a, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTRTRI computes the inverse of a real upper or lower triangular
* matrix A.
*
* This is the Level 3 BLAS version of the algorithm.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the triangular matrix A. If UPLO = 'U', the
* leading N-by-N upper triangular part of the array A contains
* the upper triangular matrix, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading N-by-N lower triangular part of the array A contains
* the lower triangular matrix, and the strictly upper
* triangular part of A is not referenced. If DIAG = 'U', the
* diagonal elements of A are also not referenced and are
* assumed to be 1.
* On exit, the (triangular) inverse of the original matrix, in
* the same storage format.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, A(i,i) is exactly zero. The triangular
* matrix is singular and its inverse can not be computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param diag
* @param n
* @param a
* @param _a_offset
* @param lda
* @param info
*
*/
abstract public void dtrtri(java.lang.String uplo, java.lang.String diag, int n, double[] a, int _a_offset, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTRTRS solves a triangular system of the form
*
* A * X = B or A**T * X = B,
*
* where A is a triangular matrix of order N, and B is an N-by-NRHS
* matrix. A check is made to verify that A is nonsingular.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The triangular matrix A. If UPLO = 'U', the leading N-by-N
* upper triangular part of the array A contains the upper
* triangular matrix, and the strictly lower triangular part of
* A is not referenced. If UPLO = 'L', the leading N-by-N lower
* triangular part of the array A contains the lower triangular
* matrix, and the strictly upper triangular part of A is not
* referenced. If DIAG = 'U', the diagonal elements of A are
* also not referenced and are assumed to be 1.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, if INFO = 0, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the i-th diagonal element of A is zero,
* indicating that the matrix is singular and the solutions
* X have not been computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param n
* @param nrhs
* @param a
* @param lda
* @param b
* @param ldb
* @param info
*
*/
abstract public void dtrtrs(java.lang.String uplo, java.lang.String trans, java.lang.String diag, int n, int nrhs, double[] a, int lda, double[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTRTRS solves a triangular system of the form
*
* A * X = B or A**T * X = B,
*
* where A is a triangular matrix of order N, and B is an N-by-NRHS
* matrix. A check is made to verify that A is nonsingular.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* The triangular matrix A. If UPLO = 'U', the leading N-by-N
* upper triangular part of the array A contains the upper
* triangular matrix, and the strictly lower triangular part of
* A is not referenced. If UPLO = 'L', the leading N-by-N lower
* triangular part of the array A contains the lower triangular
* matrix, and the strictly upper triangular part of A is not
* referenced. If DIAG = 'U', the diagonal elements of A are
* also not referenced and are assumed to be 1.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) DOUBLE PRECISION array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, if INFO = 0, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the i-th diagonal element of A is zero,
* indicating that the matrix is singular and the solutions
* X have not been computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void dtrtrs(java.lang.String uplo, java.lang.String trans, java.lang.String diag, int n, int nrhs, double[] a, int _a_offset, int lda, double[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* This routine is deprecated and has been replaced by routine DTZRZF.
*
* DTZRQF reduces the M-by-N ( M<=N ) real upper trapezoidal matrix A
* to upper triangular form by means of orthogonal transformations.
*
* The upper trapezoidal matrix A is factored as
*
* A = ( R 0 ) * Z,
*
* where Z is an N-by-N orthogonal matrix and R is an M-by-M upper
* triangular matrix.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= M.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the leading M-by-N upper trapezoidal part of the
* array A must contain the matrix to be factorized.
* On exit, the leading M-by-M upper triangular part of A
* contains the upper triangular matrix R, and elements M+1 to
* N of the first M rows of A, with the array TAU, represent the
* orthogonal matrix Z as a product of M elementary reflectors.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) DOUBLE PRECISION array, dimension (M)
* The scalar factors of the elementary reflectors.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The factorization is obtained by Householder's method. The kth
* transformation matrix, Z( k ), which is used to introduce zeros into
* the ( m - k + 1 )th row of A, is given in the form
*
* Z( k ) = ( I 0 ),
* ( 0 T( k ) )
*
* where
*
* T( k ) = I - tau*u( k )*u( k )', u( k ) = ( 1 ),
* ( 0 )
* ( z( k ) )
*
* tau is a scalar and z( k ) is an ( n - m ) element vector.
* tau and z( k ) are chosen to annihilate the elements of the kth row
* of X.
*
* The scalar tau is returned in the kth element of TAU and the vector
* u( k ) in the kth row of A, such that the elements of z( k ) are
* in a( k, m + 1 ), ..., a( k, n ). The elements of R are returned in
* the upper triangular part of A.
*
* Z is given by
*
* Z = Z( 1 ) * Z( 2 ) * ... * Z( m ).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param tau
* @param info
*
*/
abstract public void dtzrqf(int m, int n, double[] a, int lda, double[] tau, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* This routine is deprecated and has been replaced by routine DTZRZF.
*
* DTZRQF reduces the M-by-N ( M<=N ) real upper trapezoidal matrix A
* to upper triangular form by means of orthogonal transformations.
*
* The upper trapezoidal matrix A is factored as
*
* A = ( R 0 ) * Z,
*
* where Z is an N-by-N orthogonal matrix and R is an M-by-M upper
* triangular matrix.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= M.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the leading M-by-N upper trapezoidal part of the
* array A must contain the matrix to be factorized.
* On exit, the leading M-by-M upper triangular part of A
* contains the upper triangular matrix R, and elements M+1 to
* N of the first M rows of A, with the array TAU, represent the
* orthogonal matrix Z as a product of M elementary reflectors.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) DOUBLE PRECISION array, dimension (M)
* The scalar factors of the elementary reflectors.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The factorization is obtained by Householder's method. The kth
* transformation matrix, Z( k ), which is used to introduce zeros into
* the ( m - k + 1 )th row of A, is given in the form
*
* Z( k ) = ( I 0 ),
* ( 0 T( k ) )
*
* where
*
* T( k ) = I - tau*u( k )*u( k )', u( k ) = ( 1 ),
* ( 0 )
* ( z( k ) )
*
* tau is a scalar and z( k ) is an ( n - m ) element vector.
* tau and z( k ) are chosen to annihilate the elements of the kth row
* of X.
*
* The scalar tau is returned in the kth element of TAU and the vector
* u( k ) in the kth row of A, such that the elements of z( k ) are
* in a( k, m + 1 ), ..., a( k, n ). The elements of R are returned in
* the upper triangular part of A.
*
* Z is given by
*
* Z = Z( 1 ) * Z( 2 ) * ... * Z( m ).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param info
*
*/
abstract public void dtzrqf(int m, int n, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTZRZF reduces the M-by-N ( M<=N ) real upper trapezoidal matrix A
* to upper triangular form by means of orthogonal transformations.
*
* The upper trapezoidal matrix A is factored as
*
* A = ( R 0 ) * Z,
*
* where Z is an N-by-N orthogonal matrix and R is an M-by-M upper
* triangular matrix.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= M.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the leading M-by-N upper trapezoidal part of the
* array A must contain the matrix to be factorized.
* On exit, the leading M-by-M upper triangular part of A
* contains the upper triangular matrix R, and elements M+1 to
* N of the first M rows of A, with the array TAU, represent the
* orthogonal matrix Z as a product of M elementary reflectors.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) DOUBLE PRECISION array, dimension (M)
* The scalar factors of the elementary reflectors.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,M).
* For optimum performance LWORK >= M*NB, where NB is
* the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* Based on contributions by
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
*
* The factorization is obtained by Householder's method. The kth
* transformation matrix, Z( k ), which is used to introduce zeros into
* the ( m - k + 1 )th row of A, is given in the form
*
* Z( k ) = ( I 0 ),
* ( 0 T( k ) )
*
* where
*
* T( k ) = I - tau*u( k )*u( k )', u( k ) = ( 1 ),
* ( 0 )
* ( z( k ) )
*
* tau is a scalar and z( k ) is an ( n - m ) element vector.
* tau and z( k ) are chosen to annihilate the elements of the kth row
* of X.
*
* The scalar tau is returned in the kth element of TAU and the vector
* u( k ) in the kth row of A, such that the elements of z( k ) are
* in a( k, m + 1 ), ..., a( k, n ). The elements of R are returned in
* the upper triangular part of A.
*
* Z is given by
*
* Z = Z( 1 ) * Z( 2 ) * ... * Z( m ).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param tau
* @param work
* @param lwork
* @param info
*
*/
abstract public void dtzrzf(int m, int n, double[] a, int lda, double[] tau, double[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DTZRZF reduces the M-by-N ( M<=N ) real upper trapezoidal matrix A
* to upper triangular form by means of orthogonal transformations.
*
* The upper trapezoidal matrix A is factored as
*
* A = ( R 0 ) * Z,
*
* where Z is an N-by-N orthogonal matrix and R is an M-by-M upper
* triangular matrix.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= M.
*
* A (input/output) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the leading M-by-N upper trapezoidal part of the
* array A must contain the matrix to be factorized.
* On exit, the leading M-by-M upper triangular part of A
* contains the upper triangular matrix R, and elements M+1 to
* N of the first M rows of A, with the array TAU, represent the
* orthogonal matrix Z as a product of M elementary reflectors.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) DOUBLE PRECISION array, dimension (M)
* The scalar factors of the elementary reflectors.
*
* WORK (workspace/output) DOUBLE PRECISION array, dimension (MAX(1,L
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,M).
* For optimum performance LWORK >= M*NB, where NB is
* the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* Based on contributions by
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
*
* The factorization is obtained by Householder's method. The kth
* transformation matrix, Z( k ), which is used to introduce zeros into
* the ( m - k + 1 )th row of A, is given in the form
*
* Z( k ) = ( I 0 ),
* ( 0 T( k ) )
*
* where
*
* T( k ) = I - tau*u( k )*u( k )', u( k ) = ( 1 ),
* ( 0 )
* ( z( k ) )
*
* tau is a scalar and z( k ) is an ( n - m ) element vector.
* tau and z( k ) are chosen to annihilate the elements of the kth row
* of X.
*
* The scalar tau is returned in the kth element of TAU and the vector
* u( k ) in the kth row of A, such that the elements of z( k ) are
* in a( k, m + 1 ), ..., a( k, n ). The elements of R are returned in
* the upper triangular part of A.
*
* Z is given by
*
* Z = Z( 1 ) * Z( 2 ) * ... * Z( m ).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void dtzrzf(int m, int n, double[] a, int _a_offset, int lda, double[] tau, int _tau_offset, double[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* IEEECK is called from the ILAENV to verify that Infinity and
* possibly NaN arithmetic is safe (i.e. will not trap).
*
* Arguments
* =========
*
* ISPEC (input) INTEGER
* Specifies whether to test just for inifinity arithmetic
* or whether to test for infinity and NaN arithmetic.
* = 0: Verify infinity arithmetic only.
* = 1: Verify infinity and NaN arithmetic.
*
* ZERO (input) REAL
* Must contain the value 0.0
* This is passed to prevent the compiler from optimizing
* away this code.
*
* ONE (input) REAL
* Must contain the value 1.0
* This is passed to prevent the compiler from optimizing
* away this code.
*
* RETURN VALUE: INTEGER
* = 0: Arithmetic failed to produce the correct answers
* = 1: Arithmetic produced the correct answers
*
* .. Local Scalars ..
*
*
* @param ispec
* @param zero
* @param one
* @return
*/
abstract public int ieeeck(int ispec, float zero, float one);
/**
*
* ..
*
* Purpose
* =======
*
* ILAENV is called from the LAPACK routines to choose problem-dependent
* parameters for the local environment. See ISPEC for a description of
* the parameters.
*
* ILAENV returns an INTEGER
* if ILAENV >= 0: ILAENV returns the value of the parameter specified b
* if ILAENV < 0: if ILAENV = -k, the k-th argument had an illegal valu
*
* This version provides a set of parameters which should give good,
* but not optimal, performance on many of the currently available
* computers. Users are encouraged to modify this subroutine to set
* the tuning parameters for their particular machine using the option
* and problem size information in the arguments.
*
* This routine will not function correctly if it is converted to all
* lower case. Converting it to all upper case is allowed.
*
* Arguments
* =========
*
* ISPEC (input) INTEGER
* Specifies the parameter to be returned as the value of
* ILAENV.
* = 1: the optimal blocksize; if this value is 1, an unblocked
* algorithm will give the best performance.
* = 2: the minimum block size for which the block routine
* should be used; if the usable block size is less than
* this value, an unblocked routine should be used.
* = 3: the crossover point (in a block routine, for N less
* than this value, an unblocked routine should be used)
* = 4: the number of shifts, used in the nonsymmetric
* eigenvalue routines (DEPRECATED)
* = 5: the minimum column dimension for blocking to be used;
* rectangular blocks must have dimension at least k by m,
* where k is given by ILAENV(2,...) and m by ILAENV(5,...)
* = 6: the crossover point for the SVD (when reducing an m by n
* matrix to bidiagonal form, if max(m,n)/min(m,n) exceeds
* this value, a QR factorization is used first to reduce
* the matrix to a triangular form.)
* = 7: the number of processors
* = 8: the crossover point for the multishift QR method
* for nonsymmetric eigenvalue problems (DEPRECATED)
* = 9: maximum size of the subproblems at the bottom of the
* computation tree in the divide-and-conquer algorithm
* (used by xGELSD and xGESDD)
* =10: ieee NaN arithmetic can be trusted not to trap
* =11: infinity arithmetic can be trusted not to trap
* 12 <= ISPEC <= 16:
* xHSEQR or one of its subroutines,
* see IPARMQ for detailed explanation
*
* NAME (input) CHARACTER*(*)
* The name of the calling subroutine, in either upper case or
* lower case.
*
* OPTS (input) CHARACTER*(*)
* The character options to the subroutine NAME, concatenated
* into a single character string. For example, UPLO = 'U',
* TRANS = 'T', and DIAG = 'N' for a triangular routine would
* be specified as OPTS = 'UTN'.
*
* N1 (input) INTEGER
* N2 (input) INTEGER
* N3 (input) INTEGER
* N4 (input) INTEGER
* Problem dimensions for the subroutine NAME; these may not all
* be required.
*
* Further Details
* ===============
*
* The following conventions have been used when calling ILAENV from the
* LAPACK routines:
* 1) OPTS is a concatenation of all of the character options to
* subroutine NAME, in the same order that they appear in the
* argument list for NAME, even if they are not used in determining
* the value of the parameter specified by ISPEC.
* 2) The problem dimensions N1, N2, N3, N4 are specified in the order
* that they appear in the argument list for NAME. N1 is used
* first, N2 second, and so on, and unused problem dimensions are
* passed a value of -1.
* 3) The parameter value returned by ILAENV is checked for validity in
* the calling subroutine. For example, ILAENV is used to retrieve
* the optimal blocksize for STRTRI as follows:
*
* NB = ILAENV( 1, 'STRTRI', UPLO // DIAG, N, -1, -1, -1 )
* IF( NB.LE.1 ) NB = MAX( 1, N )
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param ispec
* @param name
* @param opts
* @param n1
* @param n2
* @param n3
* @param n4
* @return
*/
abstract public int ilaenv(int ispec, java.lang.String name, java.lang.String opts, int n1, int n2, int n3, int n4);
/**
*
*
* -- LAPACK routine (version 3.1.1) --
* Univ. of Tennessee, Univ. of California Berkeley and NAG Ltd..
* January 2007
* ..
*
* Purpose
* =======
*
* This subroutine return the Lapack version
*
* Arguments
* =========
* VERS_MAJOR (output) INTEGER
* return the lapack major version
* VERS_MINOR (output) INTEGER
* return the lapack minor version from the major version
* VERS_PATCH (output) INTEGER
* return the lapack patch version from the minor version
* =====================================================================
*
*
*
* @param vers_major
* @param vers_minor
* @param vers_patch
*
*/
abstract public void ilaver(org.netlib.util.intW vers_major, org.netlib.util.intW vers_minor, org.netlib.util.intW vers_patch);
/**
*
*
* Purpose
* =======
*
* This program sets problem and machine dependent parameters
* useful for xHSEQR and its subroutines. It is called whenever
* ILAENV is called with 12 <= ISPEC <= 16
*
* Arguments
* =========
*
* ISPEC (input) integer scalar
* ISPEC specifies which tunable parameter IPARMQ should
* return.
*
* ISPEC=12: (INMIN) Matrices of order nmin or less
* are sent directly to xLAHQR, the implicit
* double shift QR algorithm. NMIN must be
* at least 11.
*
* ISPEC=13: (INWIN) Size of the deflation window.
* This is best set greater than or equal to
* the number of simultaneous shifts NS.
* Larger matrices benefit from larger deflation
* windows.
*
* ISPEC=14: (INIBL) Determines when to stop nibbling and
* invest in an (expensive) multi-shift QR sweep.
* If the aggressive early deflation subroutine
* finds LD converged eigenvalues from an order
* NW deflation window and LD.GT.(NW*NIBBLE)/100,
* then the next QR sweep is skipped and early
* deflation is applied immediately to the
* remaining active diagonal block. Setting
* IPARMQ(ISPEC=14) = 0 causes TTQRE to skip a
* multi-shift QR sweep whenever early deflation
* finds a converged eigenvalue. Setting
* IPARMQ(ISPEC=14) greater than or equal to 100
* prevents TTQRE from skipping a multi-shift
* QR sweep.
*
* ISPEC=15: (NSHFTS) The number of simultaneous shifts in
* a multi-shift QR iteration.
*
* ISPEC=16: (IACC22) IPARMQ is set to 0, 1 or 2 with the
* following meanings.
* 0: During the multi-shift QR sweep,
* xLAQR5 does not accumulate reflections and
* does not use matrix-matrix multiply to
* update the far-from-diagonal matrix
* entries.
* 1: During the multi-shift QR sweep,
* xLAQR5 and/or xLAQRaccumulates reflections
* matrix-matrix multiply to update the
* far-from-diagonal matrix entries.
* 2: During the multi-shift QR sweep.
* xLAQR5 accumulates reflections and takes
* advantage of 2-by-2 block structure during
* matrix-matrix multiplies.
* (If xTRMM is slower than xGEMM, then
* IPARMQ(ISPEC=16)=1 may be more efficient than
* IPARMQ(ISPEC=16)=2 despite the greater level of
* arithmetic work implied by the latter choice.)
*
* NAME (input) character string
* Name of the calling subroutine
*
* OPTS (input) character string
* This is a concatenation of the string arguments to
* TTQRE.
*
* N (input) integer scalar
* N is the order of the Hessenberg matrix H.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* It is assumed that H is already upper triangular
* in rows and columns 1:ILO-1 and IHI+1:N.
*
* LWORK (input) integer scalar
* The amount of workspace available.
*
* Further Details
* ===============
*
* Little is known about how best to choose these parameters.
* It is possible to use different values of the parameters
* for each of CHSEQR, DHSEQR, SHSEQR and ZHSEQR.
*
* It is probably best to choose different parameters for
* different matrices and different parameters at different
* times during the iteration, but this has not been
* implemented --- yet.
*
*
* The best choices of most of the parameters depend
* in an ill-understood way on the relative execution
* rate of xLAQR3 and xLAQR5 and on the nature of each
* particular eigenvalue problem. Experiment may be the
* only practical way to determine which choices are most
* effective.
*
* Following is a list of default values supplied by IPARMQ.
* These defaults may be adjusted in order to attain better
* performance in any particular computational environment.
*
* IPARMQ(ISPEC=12) The xLAHQR vs xLAQR0 crossover point.
* Default: 75. (Must be at least 11.)
*
* IPARMQ(ISPEC=13) Recommended deflation window size.
* This depends on ILO, IHI and NS, the
* number of simultaneous shifts returned
* by IPARMQ(ISPEC=15). The default for
* (IHI-ILO+1).LE.500 is NS. The default
* for (IHI-ILO+1).GT.500 is 3*NS/2.
*
* IPARMQ(ISPEC=14) Nibble crossover point. Default: 14.
*
* IPARMQ(ISPEC=15) Number of simultaneous shifts, NS.
* a multi-shift QR iteration.
*
* If IHI-ILO+1 is ...
*
* greater than ...but less ... the
* or equal to ... than default is
*
* 0 30 NS = 2+
* 30 60 NS = 4+
* 60 150 NS = 10
* 150 590 NS = **
* 590 3000 NS = 64
* 3000 6000 NS = 128
* 6000 infinity NS = 256
*
* (+) By default matrices of this order are
* passed to the implicit double shift routine
* xLAHQR. See IPARMQ(ISPEC=12) above. These
* values of NS are used only in case of a rare
* xLAHQR failure.
*
* (**) The asterisks (**) indicate an ad-hoc
* function increasing from 10 to 64.
*
* IPARMQ(ISPEC=16) Select structured matrix multiply.
* (See ISPEC=16 above for details.)
* Default: 3.
*
* ================================================================
* .. Parameters ..
*
*
* @param ispec
* @param name
* @param opts
* @param n
* @param ilo
* @param ihi
* @param lwork
* @return
*/
abstract public int iparmq(int ispec, java.lang.String name, java.lang.String opts, int n, int ilo, int ihi, int lwork);
/**
*
* ..
*
* Purpose
* =======
*
* LSAMEN tests if the first N letters of CA are the same as the
* first N letters of CB, regardless of case.
* LSAMEN returns .TRUE. if CA and CB are equivalent except for case
* and .FALSE. otherwise. LSAMEN also returns .FALSE. if LEN( CA )
* or LEN( CB ) is less than N.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of characters in CA and CB to be compared.
*
* CA (input) CHARACTER*(*)
* CB (input) CHARACTER*(*)
* CA and CB specify two character strings of length at least N.
* Only the first N characters of each string will be accessed.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n
* @param ca
* @param cb
* @return
*/
abstract public boolean lsamen(int n, java.lang.String ca, java.lang.String cb);
/**
*
* ..
*
* Purpose
* =======
*
* SBDSDC computes the singular value decomposition (SVD) of a real
* N-by-N (upper or lower) bidiagonal matrix B: B = U * S * VT,
* using a divide and conquer method, where S is a diagonal matrix
* with non-negative diagonal elements (the singular values of B), and
* U and VT are orthogonal matrices of left and right singular vectors,
* respectively. SBDSDC can be used to compute all singular values,
* and optionally, singular vectors or singular vectors in compact form.
*
* This code makes very mild assumptions about floating point
* arithmetic. It will work on machines with a guard digit in
* add/subtract, or on those binary machines without guard digits
* which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or Cray-2.
* It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none. See SLASD3 for details.
*
* The code currently calls SLASDQ if singular values only are desired.
* However, it can be slightly modified to compute singular values
* using the divide and conquer method.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': B is upper bidiagonal.
* = 'L': B is lower bidiagonal.
*
* COMPQ (input) CHARACTER*1
* Specifies whether singular vectors are to be computed
* as follows:
* = 'N': Compute singular values only;
* = 'P': Compute singular values and compute singular
* vectors in compact form;
* = 'I': Compute singular values and singular vectors.
*
* N (input) INTEGER
* The order of the matrix B. N >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, the n diagonal elements of the bidiagonal matrix B.
* On exit, if INFO=0, the singular values of B.
*
* E (input/output) REAL array, dimension (N-1)
* On entry, the elements of E contain the offdiagonal
* elements of the bidiagonal matrix whose SVD is desired.
* On exit, E has been destroyed.
*
* U (output) REAL array, dimension (LDU,N)
* If COMPQ = 'I', then:
* On exit, if INFO = 0, U contains the left singular vectors
* of the bidiagonal matrix.
* For other values of COMPQ, U is not referenced.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= 1.
* If singular vectors are desired, then LDU >= max( 1, N ).
*
* VT (output) REAL array, dimension (LDVT,N)
* If COMPQ = 'I', then:
* On exit, if INFO = 0, VT' contains the right singular
* vectors of the bidiagonal matrix.
* For other values of COMPQ, VT is not referenced.
*
* LDVT (input) INTEGER
* The leading dimension of the array VT. LDVT >= 1.
* If singular vectors are desired, then LDVT >= max( 1, N ).
*
* Q (output) REAL array, dimension (LDQ)
* If COMPQ = 'P', then:
* On exit, if INFO = 0, Q and IQ contain the left
* and right singular vectors in a compact form,
* requiring O(N log N) space instead of 2*N**2.
* In particular, Q contains all the REAL data in
* LDQ >= N*(11 + 2*SMLSIZ + 8*INT(LOG_2(N/(SMLSIZ+1))))
* words of memory, where SMLSIZ is returned by ILAENV and
* is equal to the maximum size of the subproblems at the
* bottom of the computation tree (usually about 25).
* For other values of COMPQ, Q is not referenced.
*
* IQ (output) INTEGER array, dimension (LDIQ)
* If COMPQ = 'P', then:
* On exit, if INFO = 0, Q and IQ contain the left
* and right singular vectors in a compact form,
* requiring O(N log N) space instead of 2*N**2.
* In particular, IQ contains all INTEGER data in
* LDIQ >= N*(3 + 3*INT(LOG_2(N/(SMLSIZ+1))))
* words of memory, where SMLSIZ is returned by ILAENV and
* is equal to the maximum size of the subproblems at the
* bottom of the computation tree (usually about 25).
* For other values of COMPQ, IQ is not referenced.
*
* WORK (workspace) REAL array, dimension (MAX(1,LWORK))
* If COMPQ = 'N' then LWORK >= (4 * N).
* If COMPQ = 'P' then LWORK >= (6 * N).
* If COMPQ = 'I' then LWORK >= (3 * N**2 + 4 * N).
*
* IWORK (workspace) INTEGER array, dimension (8*N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: The algorithm failed to compute an singular value.
* The update process of divide and conquer failed.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
* =====================================================================
* Changed dimension statement in comment describing E from (N) to
* (N-1). Sven, 17 Feb 05.
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param compq
* @param n
* @param d
* @param e
* @param u
* @param ldu
* @param vt
* @param ldvt
* @param q
* @param iq
* @param work
* @param iwork
* @param info
*
*/
abstract public void sbdsdc(java.lang.String uplo, java.lang.String compq, int n, float[] d, float[] e, float[] u, int ldu, float[] vt, int ldvt, float[] q, int[] iq, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SBDSDC computes the singular value decomposition (SVD) of a real
* N-by-N (upper or lower) bidiagonal matrix B: B = U * S * VT,
* using a divide and conquer method, where S is a diagonal matrix
* with non-negative diagonal elements (the singular values of B), and
* U and VT are orthogonal matrices of left and right singular vectors,
* respectively. SBDSDC can be used to compute all singular values,
* and optionally, singular vectors or singular vectors in compact form.
*
* This code makes very mild assumptions about floating point
* arithmetic. It will work on machines with a guard digit in
* add/subtract, or on those binary machines without guard digits
* which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or Cray-2.
* It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none. See SLASD3 for details.
*
* The code currently calls SLASDQ if singular values only are desired.
* However, it can be slightly modified to compute singular values
* using the divide and conquer method.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': B is upper bidiagonal.
* = 'L': B is lower bidiagonal.
*
* COMPQ (input) CHARACTER*1
* Specifies whether singular vectors are to be computed
* as follows:
* = 'N': Compute singular values only;
* = 'P': Compute singular values and compute singular
* vectors in compact form;
* = 'I': Compute singular values and singular vectors.
*
* N (input) INTEGER
* The order of the matrix B. N >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, the n diagonal elements of the bidiagonal matrix B.
* On exit, if INFO=0, the singular values of B.
*
* E (input/output) REAL array, dimension (N-1)
* On entry, the elements of E contain the offdiagonal
* elements of the bidiagonal matrix whose SVD is desired.
* On exit, E has been destroyed.
*
* U (output) REAL array, dimension (LDU,N)
* If COMPQ = 'I', then:
* On exit, if INFO = 0, U contains the left singular vectors
* of the bidiagonal matrix.
* For other values of COMPQ, U is not referenced.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= 1.
* If singular vectors are desired, then LDU >= max( 1, N ).
*
* VT (output) REAL array, dimension (LDVT,N)
* If COMPQ = 'I', then:
* On exit, if INFO = 0, VT' contains the right singular
* vectors of the bidiagonal matrix.
* For other values of COMPQ, VT is not referenced.
*
* LDVT (input) INTEGER
* The leading dimension of the array VT. LDVT >= 1.
* If singular vectors are desired, then LDVT >= max( 1, N ).
*
* Q (output) REAL array, dimension (LDQ)
* If COMPQ = 'P', then:
* On exit, if INFO = 0, Q and IQ contain the left
* and right singular vectors in a compact form,
* requiring O(N log N) space instead of 2*N**2.
* In particular, Q contains all the REAL data in
* LDQ >= N*(11 + 2*SMLSIZ + 8*INT(LOG_2(N/(SMLSIZ+1))))
* words of memory, where SMLSIZ is returned by ILAENV and
* is equal to the maximum size of the subproblems at the
* bottom of the computation tree (usually about 25).
* For other values of COMPQ, Q is not referenced.
*
* IQ (output) INTEGER array, dimension (LDIQ)
* If COMPQ = 'P', then:
* On exit, if INFO = 0, Q and IQ contain the left
* and right singular vectors in a compact form,
* requiring O(N log N) space instead of 2*N**2.
* In particular, IQ contains all INTEGER data in
* LDIQ >= N*(3 + 3*INT(LOG_2(N/(SMLSIZ+1))))
* words of memory, where SMLSIZ is returned by ILAENV and
* is equal to the maximum size of the subproblems at the
* bottom of the computation tree (usually about 25).
* For other values of COMPQ, IQ is not referenced.
*
* WORK (workspace) REAL array, dimension (MAX(1,LWORK))
* If COMPQ = 'N' then LWORK >= (4 * N).
* If COMPQ = 'P' then LWORK >= (6 * N).
* If COMPQ = 'I' then LWORK >= (3 * N**2 + 4 * N).
*
* IWORK (workspace) INTEGER array, dimension (8*N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: The algorithm failed to compute an singular value.
* The update process of divide and conquer failed.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
* =====================================================================
* Changed dimension statement in comment describing E from (N) to
* (N-1). Sven, 17 Feb 05.
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param compq
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param u
* @param _u_offset
* @param ldu
* @param vt
* @param _vt_offset
* @param ldvt
* @param q
* @param _q_offset
* @param iq
* @param _iq_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void sbdsdc(java.lang.String uplo, java.lang.String compq, int n, float[] d, int _d_offset, float[] e, int _e_offset, float[] u, int _u_offset, int ldu, float[] vt, int _vt_offset, int ldvt, float[] q, int _q_offset, int[] iq, int _iq_offset, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SBDSQR computes the singular values and, optionally, the right and/or
* left singular vectors from the singular value decomposition (SVD) of
* a real N-by-N (upper or lower) bidiagonal matrix B using the implicit
* zero-shift QR algorithm. The SVD of B has the form
*
* B = Q * S * P**T
*
* where S is the diagonal matrix of singular values, Q is an orthogonal
* matrix of left singular vectors, and P is an orthogonal matrix of
* right singular vectors. If left singular vectors are requested, this
* subroutine actually returns U*Q instead of Q, and, if right singular
* vectors are requested, this subroutine returns P**T*VT instead of
* P**T, for given real input matrices U and VT. When U and VT are the
* orthogonal matrices that reduce a general matrix A to bidiagonal
* form: A = U*B*VT, as computed by SGEBRD, then
*
* A = (U*Q) * S * (P**T*VT)
*
* is the SVD of A. Optionally, the subroutine may also compute Q**T*C
* for a given real input matrix C.
*
* See "Computing Small Singular Values of Bidiagonal Matrices With
* Guaranteed High Relative Accuracy," by J. Demmel and W. Kahan,
* LAPACK Working Note #3 (or SIAM J. Sci. Statist. Comput. vol. 11,
* no. 5, pp. 873-912, Sept 1990) and
* "Accurate singular values and differential qd algorithms," by
* B. Parlett and V. Fernando, Technical Report CPAM-554, Mathematics
* Department, University of California at Berkeley, July 1992
* for a detailed description of the algorithm.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': B is upper bidiagonal;
* = 'L': B is lower bidiagonal.
*
* N (input) INTEGER
* The order of the matrix B. N >= 0.
*
* NCVT (input) INTEGER
* The number of columns of the matrix VT. NCVT >= 0.
*
* NRU (input) INTEGER
* The number of rows of the matrix U. NRU >= 0.
*
* NCC (input) INTEGER
* The number of columns of the matrix C. NCC >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, the n diagonal elements of the bidiagonal matrix B.
* On exit, if INFO=0, the singular values of B in decreasing
* order.
*
* E (input/output) REAL array, dimension (N-1)
* On entry, the N-1 offdiagonal elements of the bidiagonal
* matrix B.
* On exit, if INFO = 0, E is destroyed; if INFO > 0, D and E
* will contain the diagonal and superdiagonal elements of a
* bidiagonal matrix orthogonally equivalent to the one given
* as input.
*
* VT (input/output) REAL array, dimension (LDVT, NCVT)
* On entry, an N-by-NCVT matrix VT.
* On exit, VT is overwritten by P**T * VT.
* Not referenced if NCVT = 0.
*
* LDVT (input) INTEGER
* The leading dimension of the array VT.
* LDVT >= max(1,N) if NCVT > 0; LDVT >= 1 if NCVT = 0.
*
* U (input/output) REAL array, dimension (LDU, N)
* On entry, an NRU-by-N matrix U.
* On exit, U is overwritten by U * Q.
* Not referenced if NRU = 0.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= max(1,NRU).
*
* C (input/output) REAL array, dimension (LDC, NCC)
* On entry, an N-by-NCC matrix C.
* On exit, C is overwritten by Q**T * C.
* Not referenced if NCC = 0.
*
* LDC (input) INTEGER
* The leading dimension of the array C.
* LDC >= max(1,N) if NCC > 0; LDC >=1 if NCC = 0.
*
* WORK (workspace) REAL array, dimension (2*N)
* if NCVT = NRU = NCC = 0, (max(1, 4*N)) otherwise
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: If INFO = -i, the i-th argument had an illegal value
* > 0: the algorithm did not converge; D and E contain the
* elements of a bidiagonal matrix which is orthogonally
* similar to the input matrix B; if INFO = i, i
* elements of E have not converged to zero.
*
* Internal Parameters
* ===================
*
* TOLMUL REAL, default = max(10,min(100,EPS**(-1/8)))
* TOLMUL controls the convergence criterion of the QR loop.
* If it is positive, TOLMUL*EPS is the desired relative
* precision in the computed singular values.
* If it is negative, abs(TOLMUL*EPS*sigma_max) is the
* desired absolute accuracy in the computed singular
* values (corresponds to relative accuracy
* abs(TOLMUL*EPS) in the largest singular value.
* abs(TOLMUL) should be between 1 and 1/EPS, and preferably
* between 10 (for fast convergence) and .1/EPS
* (for there to be some accuracy in the results).
* Default is to lose at either one eighth or 2 of the
* available decimal digits in each computed singular value
* (whichever is smaller).
*
* MAXITR INTEGER, default = 6
* MAXITR controls the maximum number of passes of the
* algorithm through its inner loop. The algorithms stops
* (and so fails to converge) if the number of passes
* through the inner loop exceeds MAXITR*N**2.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ncvt
* @param nru
* @param ncc
* @param d
* @param e
* @param vt
* @param ldvt
* @param u
* @param ldu
* @param c
* @param Ldc
* @param work
* @param info
*
*/
abstract public void sbdsqr(java.lang.String uplo, int n, int ncvt, int nru, int ncc, float[] d, float[] e, float[] vt, int ldvt, float[] u, int ldu, float[] c, int Ldc, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SBDSQR computes the singular values and, optionally, the right and/or
* left singular vectors from the singular value decomposition (SVD) of
* a real N-by-N (upper or lower) bidiagonal matrix B using the implicit
* zero-shift QR algorithm. The SVD of B has the form
*
* B = Q * S * P**T
*
* where S is the diagonal matrix of singular values, Q is an orthogonal
* matrix of left singular vectors, and P is an orthogonal matrix of
* right singular vectors. If left singular vectors are requested, this
* subroutine actually returns U*Q instead of Q, and, if right singular
* vectors are requested, this subroutine returns P**T*VT instead of
* P**T, for given real input matrices U and VT. When U and VT are the
* orthogonal matrices that reduce a general matrix A to bidiagonal
* form: A = U*B*VT, as computed by SGEBRD, then
*
* A = (U*Q) * S * (P**T*VT)
*
* is the SVD of A. Optionally, the subroutine may also compute Q**T*C
* for a given real input matrix C.
*
* See "Computing Small Singular Values of Bidiagonal Matrices With
* Guaranteed High Relative Accuracy," by J. Demmel and W. Kahan,
* LAPACK Working Note #3 (or SIAM J. Sci. Statist. Comput. vol. 11,
* no. 5, pp. 873-912, Sept 1990) and
* "Accurate singular values and differential qd algorithms," by
* B. Parlett and V. Fernando, Technical Report CPAM-554, Mathematics
* Department, University of California at Berkeley, July 1992
* for a detailed description of the algorithm.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': B is upper bidiagonal;
* = 'L': B is lower bidiagonal.
*
* N (input) INTEGER
* The order of the matrix B. N >= 0.
*
* NCVT (input) INTEGER
* The number of columns of the matrix VT. NCVT >= 0.
*
* NRU (input) INTEGER
* The number of rows of the matrix U. NRU >= 0.
*
* NCC (input) INTEGER
* The number of columns of the matrix C. NCC >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, the n diagonal elements of the bidiagonal matrix B.
* On exit, if INFO=0, the singular values of B in decreasing
* order.
*
* E (input/output) REAL array, dimension (N-1)
* On entry, the N-1 offdiagonal elements of the bidiagonal
* matrix B.
* On exit, if INFO = 0, E is destroyed; if INFO > 0, D and E
* will contain the diagonal and superdiagonal elements of a
* bidiagonal matrix orthogonally equivalent to the one given
* as input.
*
* VT (input/output) REAL array, dimension (LDVT, NCVT)
* On entry, an N-by-NCVT matrix VT.
* On exit, VT is overwritten by P**T * VT.
* Not referenced if NCVT = 0.
*
* LDVT (input) INTEGER
* The leading dimension of the array VT.
* LDVT >= max(1,N) if NCVT > 0; LDVT >= 1 if NCVT = 0.
*
* U (input/output) REAL array, dimension (LDU, N)
* On entry, an NRU-by-N matrix U.
* On exit, U is overwritten by U * Q.
* Not referenced if NRU = 0.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= max(1,NRU).
*
* C (input/output) REAL array, dimension (LDC, NCC)
* On entry, an N-by-NCC matrix C.
* On exit, C is overwritten by Q**T * C.
* Not referenced if NCC = 0.
*
* LDC (input) INTEGER
* The leading dimension of the array C.
* LDC >= max(1,N) if NCC > 0; LDC >=1 if NCC = 0.
*
* WORK (workspace) REAL array, dimension (2*N)
* if NCVT = NRU = NCC = 0, (max(1, 4*N)) otherwise
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: If INFO = -i, the i-th argument had an illegal value
* > 0: the algorithm did not converge; D and E contain the
* elements of a bidiagonal matrix which is orthogonally
* similar to the input matrix B; if INFO = i, i
* elements of E have not converged to zero.
*
* Internal Parameters
* ===================
*
* TOLMUL REAL, default = max(10,min(100,EPS**(-1/8)))
* TOLMUL controls the convergence criterion of the QR loop.
* If it is positive, TOLMUL*EPS is the desired relative
* precision in the computed singular values.
* If it is negative, abs(TOLMUL*EPS*sigma_max) is the
* desired absolute accuracy in the computed singular
* values (corresponds to relative accuracy
* abs(TOLMUL*EPS) in the largest singular value.
* abs(TOLMUL) should be between 1 and 1/EPS, and preferably
* between 10 (for fast convergence) and .1/EPS
* (for there to be some accuracy in the results).
* Default is to lose at either one eighth or 2 of the
* available decimal digits in each computed singular value
* (whichever is smaller).
*
* MAXITR INTEGER, default = 6
* MAXITR controls the maximum number of passes of the
* algorithm through its inner loop. The algorithms stops
* (and so fails to converge) if the number of passes
* through the inner loop exceeds MAXITR*N**2.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ncvt
* @param nru
* @param ncc
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param vt
* @param _vt_offset
* @param ldvt
* @param u
* @param _u_offset
* @param ldu
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void sbdsqr(java.lang.String uplo, int n, int ncvt, int nru, int ncc, float[] d, int _d_offset, float[] e, int _e_offset, float[] vt, int _vt_offset, int ldvt, float[] u, int _u_offset, int ldu, float[] c, int _c_offset, int Ldc, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SDISNA computes the reciprocal condition numbers for the eigenvectors
* of a real symmetric or complex Hermitian matrix or for the left or
* right singular vectors of a general m-by-n matrix. The reciprocal
* condition number is the 'gap' between the corresponding eigenvalue or
* singular value and the nearest other one.
*
* The bound on the error, measured by angle in radians, in the I-th
* computed vector is given by
*
* SLAMCH( 'E' ) * ( ANORM / SEP( I ) )
*
* where ANORM = 2-norm(A) = max( abs( D(j) ) ). SEP(I) is not allowed
* to be smaller than SLAMCH( 'E' )*ANORM in order to limit the size of
* the error bound.
*
* SDISNA may also be used to compute error bounds for eigenvectors of
* the generalized symmetric definite eigenproblem.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* Specifies for which problem the reciprocal condition numbers
* should be computed:
* = 'E': the eigenvectors of a symmetric/Hermitian matrix;
* = 'L': the left singular vectors of a general matrix;
* = 'R': the right singular vectors of a general matrix.
*
* M (input) INTEGER
* The number of rows of the matrix. M >= 0.
*
* N (input) INTEGER
* If JOB = 'L' or 'R', the number of columns of the matrix,
* in which case N >= 0. Ignored if JOB = 'E'.
*
* D (input) REAL array, dimension (M) if JOB = 'E'
* dimension (min(M,N)) if JOB = 'L' or 'R'
* The eigenvalues (if JOB = 'E') or singular values (if JOB =
* 'L' or 'R') of the matrix, in either increasing or decreasing
* order. If singular values, they must be non-negative.
*
* SEP (output) REAL array, dimension (M) if JOB = 'E'
* dimension (min(M,N)) if JOB = 'L' or 'R'
* The reciprocal condition numbers of the vectors.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param m
* @param n
* @param d
* @param sep
* @param info
*
*/
abstract public void sdisna(java.lang.String job, int m, int n, float[] d, float[] sep, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SDISNA computes the reciprocal condition numbers for the eigenvectors
* of a real symmetric or complex Hermitian matrix or for the left or
* right singular vectors of a general m-by-n matrix. The reciprocal
* condition number is the 'gap' between the corresponding eigenvalue or
* singular value and the nearest other one.
*
* The bound on the error, measured by angle in radians, in the I-th
* computed vector is given by
*
* SLAMCH( 'E' ) * ( ANORM / SEP( I ) )
*
* where ANORM = 2-norm(A) = max( abs( D(j) ) ). SEP(I) is not allowed
* to be smaller than SLAMCH( 'E' )*ANORM in order to limit the size of
* the error bound.
*
* SDISNA may also be used to compute error bounds for eigenvectors of
* the generalized symmetric definite eigenproblem.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* Specifies for which problem the reciprocal condition numbers
* should be computed:
* = 'E': the eigenvectors of a symmetric/Hermitian matrix;
* = 'L': the left singular vectors of a general matrix;
* = 'R': the right singular vectors of a general matrix.
*
* M (input) INTEGER
* The number of rows of the matrix. M >= 0.
*
* N (input) INTEGER
* If JOB = 'L' or 'R', the number of columns of the matrix,
* in which case N >= 0. Ignored if JOB = 'E'.
*
* D (input) REAL array, dimension (M) if JOB = 'E'
* dimension (min(M,N)) if JOB = 'L' or 'R'
* The eigenvalues (if JOB = 'E') or singular values (if JOB =
* 'L' or 'R') of the matrix, in either increasing or decreasing
* order. If singular values, they must be non-negative.
*
* SEP (output) REAL array, dimension (M) if JOB = 'E'
* dimension (min(M,N)) if JOB = 'L' or 'R'
* The reciprocal condition numbers of the vectors.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param m
* @param n
* @param d
* @param _d_offset
* @param sep
* @param _sep_offset
* @param info
*
*/
abstract public void sdisna(java.lang.String job, int m, int n, float[] d, int _d_offset, float[] sep, int _sep_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGBBRD reduces a real general m-by-n band matrix A to upper
* bidiagonal form B by an orthogonal transformation: Q' * A * P = B.
*
* The routine computes B, and optionally forms Q or P', or computes
* Q'*C for a given matrix C.
*
* Arguments
* =========
*
* VECT (input) CHARACTER*1
* Specifies whether or not the matrices Q and P' are to be
* formed.
* = 'N': do not form Q or P';
* = 'Q': form Q only;
* = 'P': form P' only;
* = 'B': form both.
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* NCC (input) INTEGER
* The number of columns of the matrix C. NCC >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals of the matrix A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals of the matrix A. KU >= 0.
*
* AB (input/output) REAL array, dimension (LDAB,N)
* On entry, the m-by-n band matrix A, stored in rows 1 to
* KL+KU+1. The j-th column of A is stored in the j-th column of
* the array AB as follows:
* AB(ku+1+i-j,j) = A(i,j) for max(1,j-ku)<=i<=min(m,j+kl).
* On exit, A is overwritten by values generated during the
* reduction.
*
* LDAB (input) INTEGER
* The leading dimension of the array A. LDAB >= KL+KU+1.
*
* D (output) REAL array, dimension (min(M,N))
* The diagonal elements of the bidiagonal matrix B.
*
* E (output) REAL array, dimension (min(M,N)-1)
* The superdiagonal elements of the bidiagonal matrix B.
*
* Q (output) REAL array, dimension (LDQ,M)
* If VECT = 'Q' or 'B', the m-by-m orthogonal matrix Q.
* If VECT = 'N' or 'P', the array Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q.
* LDQ >= max(1,M) if VECT = 'Q' or 'B'; LDQ >= 1 otherwise.
*
* PT (output) REAL array, dimension (LDPT,N)
* If VECT = 'P' or 'B', the n-by-n orthogonal matrix P'.
* If VECT = 'N' or 'Q', the array PT is not referenced.
*
* LDPT (input) INTEGER
* The leading dimension of the array PT.
* LDPT >= max(1,N) if VECT = 'P' or 'B'; LDPT >= 1 otherwise.
*
* C (input/output) REAL array, dimension (LDC,NCC)
* On entry, an m-by-ncc matrix C.
* On exit, C is overwritten by Q'*C.
* C is not referenced if NCC = 0.
*
* LDC (input) INTEGER
* The leading dimension of the array C.
* LDC >= max(1,M) if NCC > 0; LDC >= 1 if NCC = 0.
*
* WORK (workspace) REAL array, dimension (2*max(M,N))
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param vect
* @param m
* @param n
* @param ncc
* @param kl
* @param ku
* @param ab
* @param ldab
* @param d
* @param e
* @param q
* @param ldq
* @param pt
* @param ldpt
* @param c
* @param Ldc
* @param work
* @param info
*
*/
abstract public void sgbbrd(java.lang.String vect, int m, int n, int ncc, int kl, int ku, float[] ab, int ldab, float[] d, float[] e, float[] q, int ldq, float[] pt, int ldpt, float[] c, int Ldc, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGBBRD reduces a real general m-by-n band matrix A to upper
* bidiagonal form B by an orthogonal transformation: Q' * A * P = B.
*
* The routine computes B, and optionally forms Q or P', or computes
* Q'*C for a given matrix C.
*
* Arguments
* =========
*
* VECT (input) CHARACTER*1
* Specifies whether or not the matrices Q and P' are to be
* formed.
* = 'N': do not form Q or P';
* = 'Q': form Q only;
* = 'P': form P' only;
* = 'B': form both.
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* NCC (input) INTEGER
* The number of columns of the matrix C. NCC >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals of the matrix A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals of the matrix A. KU >= 0.
*
* AB (input/output) REAL array, dimension (LDAB,N)
* On entry, the m-by-n band matrix A, stored in rows 1 to
* KL+KU+1. The j-th column of A is stored in the j-th column of
* the array AB as follows:
* AB(ku+1+i-j,j) = A(i,j) for max(1,j-ku)<=i<=min(m,j+kl).
* On exit, A is overwritten by values generated during the
* reduction.
*
* LDAB (input) INTEGER
* The leading dimension of the array A. LDAB >= KL+KU+1.
*
* D (output) REAL array, dimension (min(M,N))
* The diagonal elements of the bidiagonal matrix B.
*
* E (output) REAL array, dimension (min(M,N)-1)
* The superdiagonal elements of the bidiagonal matrix B.
*
* Q (output) REAL array, dimension (LDQ,M)
* If VECT = 'Q' or 'B', the m-by-m orthogonal matrix Q.
* If VECT = 'N' or 'P', the array Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q.
* LDQ >= max(1,M) if VECT = 'Q' or 'B'; LDQ >= 1 otherwise.
*
* PT (output) REAL array, dimension (LDPT,N)
* If VECT = 'P' or 'B', the n-by-n orthogonal matrix P'.
* If VECT = 'N' or 'Q', the array PT is not referenced.
*
* LDPT (input) INTEGER
* The leading dimension of the array PT.
* LDPT >= max(1,N) if VECT = 'P' or 'B'; LDPT >= 1 otherwise.
*
* C (input/output) REAL array, dimension (LDC,NCC)
* On entry, an m-by-ncc matrix C.
* On exit, C is overwritten by Q'*C.
* C is not referenced if NCC = 0.
*
* LDC (input) INTEGER
* The leading dimension of the array C.
* LDC >= max(1,M) if NCC > 0; LDC >= 1 if NCC = 0.
*
* WORK (workspace) REAL array, dimension (2*max(M,N))
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param vect
* @param m
* @param n
* @param ncc
* @param kl
* @param ku
* @param ab
* @param _ab_offset
* @param ldab
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param q
* @param _q_offset
* @param ldq
* @param pt
* @param _pt_offset
* @param ldpt
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void sgbbrd(java.lang.String vect, int m, int n, int ncc, int kl, int ku, float[] ab, int _ab_offset, int ldab, float[] d, int _d_offset, float[] e, int _e_offset, float[] q, int _q_offset, int ldq, float[] pt, int _pt_offset, int ldpt, float[] c, int _c_offset, int Ldc, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGBCON estimates the reciprocal of the condition number of a real
* general band matrix A, in either the 1-norm or the infinity-norm,
* using the LU factorization computed by SGBTRF.
*
* An estimate is obtained for norm(inv(A)), and the reciprocal of the
* condition number is computed as
* RCOND = 1 / ( norm(A) * norm(inv(A)) ).
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies whether the 1-norm condition number or the
* infinity-norm condition number is required:
* = '1' or 'O': 1-norm;
* = 'I': Infinity-norm.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* AB (input) REAL array, dimension (LDAB,N)
* Details of the LU factorization of the band matrix A, as
* computed by SGBTRF. U is stored as an upper triangular band
* matrix with KL+KU superdiagonals in rows 1 to KL+KU+1, and
* the multipliers used during the factorization are stored in
* rows KL+KU+2 to 2*KL+KU+1.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= 2*KL+KU+1.
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices; for 1 <= i <= N, row i of the matrix was
* interchanged with row IPIV(i).
*
* ANORM (input) REAL
* If NORM = '1' or 'O', the 1-norm of the original matrix A.
* If NORM = 'I', the infinity-norm of the original matrix A.
*
* RCOND (output) REAL
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(norm(A) * norm(inv(A))).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param n
* @param kl
* @param ku
* @param ab
* @param ldab
* @param ipiv
* @param anorm
* @param rcond
* @param work
* @param iwork
* @param info
*
*/
abstract public void sgbcon(java.lang.String norm, int n, int kl, int ku, float[] ab, int ldab, int[] ipiv, float anorm, org.netlib.util.floatW rcond, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGBCON estimates the reciprocal of the condition number of a real
* general band matrix A, in either the 1-norm or the infinity-norm,
* using the LU factorization computed by SGBTRF.
*
* An estimate is obtained for norm(inv(A)), and the reciprocal of the
* condition number is computed as
* RCOND = 1 / ( norm(A) * norm(inv(A)) ).
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies whether the 1-norm condition number or the
* infinity-norm condition number is required:
* = '1' or 'O': 1-norm;
* = 'I': Infinity-norm.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* AB (input) REAL array, dimension (LDAB,N)
* Details of the LU factorization of the band matrix A, as
* computed by SGBTRF. U is stored as an upper triangular band
* matrix with KL+KU superdiagonals in rows 1 to KL+KU+1, and
* the multipliers used during the factorization are stored in
* rows KL+KU+2 to 2*KL+KU+1.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= 2*KL+KU+1.
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices; for 1 <= i <= N, row i of the matrix was
* interchanged with row IPIV(i).
*
* ANORM (input) REAL
* If NORM = '1' or 'O', the 1-norm of the original matrix A.
* If NORM = 'I', the infinity-norm of the original matrix A.
*
* RCOND (output) REAL
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(norm(A) * norm(inv(A))).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param n
* @param kl
* @param ku
* @param ab
* @param _ab_offset
* @param ldab
* @param ipiv
* @param _ipiv_offset
* @param anorm
* @param rcond
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void sgbcon(java.lang.String norm, int n, int kl, int ku, float[] ab, int _ab_offset, int ldab, int[] ipiv, int _ipiv_offset, float anorm, org.netlib.util.floatW rcond, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGBEQU computes row and column scalings intended to equilibrate an
* M-by-N band matrix A and reduce its condition number. R returns the
* row scale factors and C the column scale factors, chosen to try to
* make the largest element in each row and column of the matrix B with
* elements B(i,j)=R(i)*A(i,j)*C(j) have absolute value 1.
*
* R(i) and C(j) are restricted to be between SMLNUM = smallest safe
* number and BIGNUM = largest safe number. Use of these scaling
* factors is not guaranteed to reduce the condition number of A but
* works well in practice.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* AB (input) REAL array, dimension (LDAB,N)
* The band matrix A, stored in rows 1 to KL+KU+1. The j-th
* column of A is stored in the j-th column of the array AB as
* follows:
* AB(ku+1+i-j,j) = A(i,j) for max(1,j-ku)<=i<=min(m,j+kl).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KL+KU+1.
*
* R (output) REAL array, dimension (M)
* If INFO = 0, or INFO > M, R contains the row scale factors
* for A.
*
* C (output) REAL array, dimension (N)
* If INFO = 0, C contains the column scale factors for A.
*
* ROWCND (output) REAL
* If INFO = 0 or INFO > M, ROWCND contains the ratio of the
* smallest R(i) to the largest R(i). If ROWCND >= 0.1 and
* AMAX is neither too large nor too small, it is not worth
* scaling by R.
*
* COLCND (output) REAL
* If INFO = 0, COLCND contains the ratio of the smallest
* C(i) to the largest C(i). If COLCND >= 0.1, it is not
* worth scaling by C.
*
* AMAX (output) REAL
* Absolute value of largest matrix element. If AMAX is very
* close to overflow or very close to underflow, the matrix
* should be scaled.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= M: the i-th row of A is exactly zero
* > M: the (i-M)-th column of A is exactly zero
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param kl
* @param ku
* @param ab
* @param ldab
* @param r
* @param c
* @param rowcnd
* @param colcnd
* @param amax
* @param info
*
*/
abstract public void sgbequ(int m, int n, int kl, int ku, float[] ab, int ldab, float[] r, float[] c, org.netlib.util.floatW rowcnd, org.netlib.util.floatW colcnd, org.netlib.util.floatW amax, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGBEQU computes row and column scalings intended to equilibrate an
* M-by-N band matrix A and reduce its condition number. R returns the
* row scale factors and C the column scale factors, chosen to try to
* make the largest element in each row and column of the matrix B with
* elements B(i,j)=R(i)*A(i,j)*C(j) have absolute value 1.
*
* R(i) and C(j) are restricted to be between SMLNUM = smallest safe
* number and BIGNUM = largest safe number. Use of these scaling
* factors is not guaranteed to reduce the condition number of A but
* works well in practice.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* AB (input) REAL array, dimension (LDAB,N)
* The band matrix A, stored in rows 1 to KL+KU+1. The j-th
* column of A is stored in the j-th column of the array AB as
* follows:
* AB(ku+1+i-j,j) = A(i,j) for max(1,j-ku)<=i<=min(m,j+kl).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KL+KU+1.
*
* R (output) REAL array, dimension (M)
* If INFO = 0, or INFO > M, R contains the row scale factors
* for A.
*
* C (output) REAL array, dimension (N)
* If INFO = 0, C contains the column scale factors for A.
*
* ROWCND (output) REAL
* If INFO = 0 or INFO > M, ROWCND contains the ratio of the
* smallest R(i) to the largest R(i). If ROWCND >= 0.1 and
* AMAX is neither too large nor too small, it is not worth
* scaling by R.
*
* COLCND (output) REAL
* If INFO = 0, COLCND contains the ratio of the smallest
* C(i) to the largest C(i). If COLCND >= 0.1, it is not
* worth scaling by C.
*
* AMAX (output) REAL
* Absolute value of largest matrix element. If AMAX is very
* close to overflow or very close to underflow, the matrix
* should be scaled.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= M: the i-th row of A is exactly zero
* > M: the (i-M)-th column of A is exactly zero
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param kl
* @param ku
* @param ab
* @param _ab_offset
* @param ldab
* @param r
* @param _r_offset
* @param c
* @param _c_offset
* @param rowcnd
* @param colcnd
* @param amax
* @param info
*
*/
abstract public void sgbequ(int m, int n, int kl, int ku, float[] ab, int _ab_offset, int ldab, float[] r, int _r_offset, float[] c, int _c_offset, org.netlib.util.floatW rowcnd, org.netlib.util.floatW colcnd, org.netlib.util.floatW amax, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGBRFS improves the computed solution to a system of linear
* equations when the coefficient matrix is banded, and provides
* error bounds and backward error estimates for the solution.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AB (input) REAL array, dimension (LDAB,N)
* The original band matrix A, stored in rows 1 to KL+KU+1.
* The j-th column of A is stored in the j-th column of the
* array AB as follows:
* AB(ku+1+i-j,j) = A(i,j) for max(1,j-ku)<=i<=min(n,j+kl).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KL+KU+1.
*
* AFB (input) REAL array, dimension (LDAFB,N)
* Details of the LU factorization of the band matrix A, as
* computed by SGBTRF. U is stored as an upper triangular band
* matrix with KL+KU superdiagonals in rows 1 to KL+KU+1, and
* the multipliers used during the factorization are stored in
* rows KL+KU+2 to 2*KL+KU+1.
*
* LDAFB (input) INTEGER
* The leading dimension of the array AFB. LDAFB >= 2*KL*KU+1.
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices from SGBTRF; for 1<=i<=N, row i of the
* matrix was interchanged with row IPIV(i).
*
* B (input) REAL array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) REAL array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by SGBTRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param trans
* @param n
* @param kl
* @param ku
* @param nrhs
* @param ab
* @param ldab
* @param afb
* @param ldafb
* @param ipiv
* @param b
* @param ldb
* @param x
* @param ldx
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void sgbrfs(java.lang.String trans, int n, int kl, int ku, int nrhs, float[] ab, int ldab, float[] afb, int ldafb, int[] ipiv, float[] b, int ldb, float[] x, int ldx, float[] ferr, float[] berr, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGBRFS improves the computed solution to a system of linear
* equations when the coefficient matrix is banded, and provides
* error bounds and backward error estimates for the solution.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AB (input) REAL array, dimension (LDAB,N)
* The original band matrix A, stored in rows 1 to KL+KU+1.
* The j-th column of A is stored in the j-th column of the
* array AB as follows:
* AB(ku+1+i-j,j) = A(i,j) for max(1,j-ku)<=i<=min(n,j+kl).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KL+KU+1.
*
* AFB (input) REAL array, dimension (LDAFB,N)
* Details of the LU factorization of the band matrix A, as
* computed by SGBTRF. U is stored as an upper triangular band
* matrix with KL+KU superdiagonals in rows 1 to KL+KU+1, and
* the multipliers used during the factorization are stored in
* rows KL+KU+2 to 2*KL+KU+1.
*
* LDAFB (input) INTEGER
* The leading dimension of the array AFB. LDAFB >= 2*KL*KU+1.
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices from SGBTRF; for 1<=i<=N, row i of the
* matrix was interchanged with row IPIV(i).
*
* B (input) REAL array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) REAL array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by SGBTRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param trans
* @param n
* @param kl
* @param ku
* @param nrhs
* @param ab
* @param _ab_offset
* @param ldab
* @param afb
* @param _afb_offset
* @param ldafb
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void sgbrfs(java.lang.String trans, int n, int kl, int ku, int nrhs, float[] ab, int _ab_offset, int ldab, float[] afb, int _afb_offset, int ldafb, int[] ipiv, int _ipiv_offset, float[] b, int _b_offset, int ldb, float[] x, int _x_offset, int ldx, float[] ferr, int _ferr_offset, float[] berr, int _berr_offset, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGBSV computes the solution to a real system of linear equations
* A * X = B, where A is a band matrix of order N with KL subdiagonals
* and KU superdiagonals, and X and B are N-by-NRHS matrices.
*
* The LU decomposition with partial pivoting and row interchanges is
* used to factor A as A = L * U, where L is a product of permutation
* and unit lower triangular matrices with KL subdiagonals, and U is
* upper triangular with KL+KU superdiagonals. The factored form of A
* is then used to solve the system of equations A * X = B.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AB (input/output) REAL array, dimension (LDAB,N)
* On entry, the matrix A in band storage, in rows KL+1 to
* 2*KL+KU+1; rows 1 to KL of the array need not be set.
* The j-th column of A is stored in the j-th column of the
* array AB as follows:
* AB(KL+KU+1+i-j,j) = A(i,j) for max(1,j-KU)<=i<=min(N,j+KL)
* On exit, details of the factorization: U is stored as an
* upper triangular band matrix with KL+KU superdiagonals in
* rows 1 to KL+KU+1, and the multipliers used during the
* factorization are stored in rows KL+KU+2 to 2*KL+KU+1.
* See below for further details.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= 2*KL+KU+1.
*
* IPIV (output) INTEGER array, dimension (N)
* The pivot indices that define the permutation matrix P;
* row i of the matrix was interchanged with row IPIV(i).
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if INFO = 0, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, U(i,i) is exactly zero. The factorization
* has been completed, but the factor U is exactly
* singular, and the solution has not been computed.
*
* Further Details
* ===============
*
* The band storage scheme is illustrated by the following example, when
* M = N = 6, KL = 2, KU = 1:
*
* On entry: On exit:
*
* * * * + + + * * * u14 u25 u36
* * * + + + + * * u13 u24 u35 u46
* * a12 a23 a34 a45 a56 * u12 u23 u34 u45 u56
* a11 a22 a33 a44 a55 a66 u11 u22 u33 u44 u55 u66
* a21 a32 a43 a54 a65 * m21 m32 m43 m54 m65 *
* a31 a42 a53 a64 * * m31 m42 m53 m64 * *
*
* Array elements marked * are not used by the routine; elements marked
* + need not be set on entry, but are required by the routine to store
* elements of U because of fill-in resulting from the row interchanges.
*
* =====================================================================
*
* .. External Subroutines ..
*
*
* @param n
* @param kl
* @param ku
* @param nrhs
* @param ab
* @param ldab
* @param ipiv
* @param b
* @param ldb
* @param info
*
*/
abstract public void sgbsv(int n, int kl, int ku, int nrhs, float[] ab, int ldab, int[] ipiv, float[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGBSV computes the solution to a real system of linear equations
* A * X = B, where A is a band matrix of order N with KL subdiagonals
* and KU superdiagonals, and X and B are N-by-NRHS matrices.
*
* The LU decomposition with partial pivoting and row interchanges is
* used to factor A as A = L * U, where L is a product of permutation
* and unit lower triangular matrices with KL subdiagonals, and U is
* upper triangular with KL+KU superdiagonals. The factored form of A
* is then used to solve the system of equations A * X = B.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AB (input/output) REAL array, dimension (LDAB,N)
* On entry, the matrix A in band storage, in rows KL+1 to
* 2*KL+KU+1; rows 1 to KL of the array need not be set.
* The j-th column of A is stored in the j-th column of the
* array AB as follows:
* AB(KL+KU+1+i-j,j) = A(i,j) for max(1,j-KU)<=i<=min(N,j+KL)
* On exit, details of the factorization: U is stored as an
* upper triangular band matrix with KL+KU superdiagonals in
* rows 1 to KL+KU+1, and the multipliers used during the
* factorization are stored in rows KL+KU+2 to 2*KL+KU+1.
* See below for further details.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= 2*KL+KU+1.
*
* IPIV (output) INTEGER array, dimension (N)
* The pivot indices that define the permutation matrix P;
* row i of the matrix was interchanged with row IPIV(i).
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if INFO = 0, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, U(i,i) is exactly zero. The factorization
* has been completed, but the factor U is exactly
* singular, and the solution has not been computed.
*
* Further Details
* ===============
*
* The band storage scheme is illustrated by the following example, when
* M = N = 6, KL = 2, KU = 1:
*
* On entry: On exit:
*
* * * * + + + * * * u14 u25 u36
* * * + + + + * * u13 u24 u35 u46
* * a12 a23 a34 a45 a56 * u12 u23 u34 u45 u56
* a11 a22 a33 a44 a55 a66 u11 u22 u33 u44 u55 u66
* a21 a32 a43 a54 a65 * m21 m32 m43 m54 m65 *
* a31 a42 a53 a64 * * m31 m42 m53 m64 * *
*
* Array elements marked * are not used by the routine; elements marked
* + need not be set on entry, but are required by the routine to store
* elements of U because of fill-in resulting from the row interchanges.
*
* =====================================================================
*
* .. External Subroutines ..
*
*
* @param n
* @param kl
* @param ku
* @param nrhs
* @param ab
* @param _ab_offset
* @param ldab
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void sgbsv(int n, int kl, int ku, int nrhs, float[] ab, int _ab_offset, int ldab, int[] ipiv, int _ipiv_offset, float[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGBSVX uses the LU factorization to compute the solution to a real
* system of linear equations A * X = B, A**T * X = B, or A**H * X = B,
* where A is a band matrix of order N with KL subdiagonals and KU
* superdiagonals, and X and B are N-by-NRHS matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed by this subroutine:
*
* 1. If FACT = 'E', real scaling factors are computed to equilibrate
* the system:
* TRANS = 'N': diag(R)*A*diag(C) *inv(diag(C))*X = diag(R)*B
* TRANS = 'T': (diag(R)*A*diag(C))**T *inv(diag(R))*X = diag(C)*B
* TRANS = 'C': (diag(R)*A*diag(C))**H *inv(diag(R))*X = diag(C)*B
* Whether or not the system will be equilibrated depends on the
* scaling of the matrix A, but if equilibration is used, A is
* overwritten by diag(R)*A*diag(C) and B by diag(R)*B (if TRANS='N')
* or diag(C)*B (if TRANS = 'T' or 'C').
*
* 2. If FACT = 'N' or 'E', the LU decomposition is used to factor the
* matrix A (after equilibration if FACT = 'E') as
* A = L * U,
* where L is a product of permutation and unit lower triangular
* matrices with KL subdiagonals, and U is upper triangular with
* KL+KU superdiagonals.
*
* 3. If some U(i,i)=0, so that U is exactly singular, then the routine
* returns with INFO = i. Otherwise, the factored form of A is used
* to estimate the condition number of the matrix A. If the
* reciprocal of the condition number is less than machine precision,
* INFO = N+1 is returned as a warning, but the routine still goes on
* to solve for X and compute error bounds as described below.
*
* 4. The system of equations is solved for X using the factored form
* of A.
*
* 5. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* 6. If equilibration was used, the matrix X is premultiplied by
* diag(C) (if TRANS = 'N') or diag(R) (if TRANS = 'T' or 'C') so
* that it solves the original system before equilibration.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of the matrix A is
* supplied on entry, and if not, whether the matrix A should be
* equilibrated before it is factored.
* = 'F': On entry, AFB and IPIV contain the factored form of
* A. If EQUED is not 'N', the matrix A has been
* equilibrated with scaling factors given by R and C.
* AB, AFB, and IPIV are not modified.
* = 'N': The matrix A will be copied to AFB and factored.
* = 'E': The matrix A will be equilibrated if necessary, then
* copied to AFB and factored.
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations.
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Transpose)
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AB (input/output) REAL array, dimension (LDAB,N)
* On entry, the matrix A in band storage, in rows 1 to KL+KU+1.
* The j-th column of A is stored in the j-th column of the
* array AB as follows:
* AB(KU+1+i-j,j) = A(i,j) for max(1,j-KU)<=i<=min(N,j+kl)
*
* If FACT = 'F' and EQUED is not 'N', then A must have been
* equilibrated by the scaling factors in R and/or C. AB is not
* modified if FACT = 'F' or 'N', or if FACT = 'E' and
* EQUED = 'N' on exit.
*
* On exit, if EQUED .ne. 'N', A is scaled as follows:
* EQUED = 'R': A := diag(R) * A
* EQUED = 'C': A := A * diag(C)
* EQUED = 'B': A := diag(R) * A * diag(C).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KL+KU+1.
*
* AFB (input or output) REAL array, dimension (LDAFB,N)
* If FACT = 'F', then AFB is an input argument and on entry
* contains details of the LU factorization of the band matrix
* A, as computed by SGBTRF. U is stored as an upper triangular
* band matrix with KL+KU superdiagonals in rows 1 to KL+KU+1,
* and the multipliers used during the factorization are stored
* in rows KL+KU+2 to 2*KL+KU+1. If EQUED .ne. 'N', then AFB is
* the factored form of the equilibrated matrix A.
*
* If FACT = 'N', then AFB is an output argument and on exit
* returns details of the LU factorization of A.
*
* If FACT = 'E', then AFB is an output argument and on exit
* returns details of the LU factorization of the equilibrated
* matrix A (see the description of AB for the form of the
* equilibrated matrix).
*
* LDAFB (input) INTEGER
* The leading dimension of the array AFB. LDAFB >= 2*KL+KU+1.
*
* IPIV (input or output) INTEGER array, dimension (N)
* If FACT = 'F', then IPIV is an input argument and on entry
* contains the pivot indices from the factorization A = L*U
* as computed by SGBTRF; row i of the matrix was interchanged
* with row IPIV(i).
*
* If FACT = 'N', then IPIV is an output argument and on exit
* contains the pivot indices from the factorization A = L*U
* of the original matrix A.
*
* If FACT = 'E', then IPIV is an output argument and on exit
* contains the pivot indices from the factorization A = L*U
* of the equilibrated matrix A.
*
* EQUED (input or output) CHARACTER*1
* Specifies the form of equilibration that was done.
* = 'N': No equilibration (always true if FACT = 'N').
* = 'R': Row equilibration, i.e., A has been premultiplied by
* diag(R).
* = 'C': Column equilibration, i.e., A has been postmultiplied
* by diag(C).
* = 'B': Both row and column equilibration, i.e., A has been
* replaced by diag(R) * A * diag(C).
* EQUED is an input argument if FACT = 'F'; otherwise, it is an
* output argument.
*
* R (input or output) REAL array, dimension (N)
* The row scale factors for A. If EQUED = 'R' or 'B', A is
* multiplied on the left by diag(R); if EQUED = 'N' or 'C', R
* is not accessed. R is an input argument if FACT = 'F';
* otherwise, R is an output argument. If FACT = 'F' and
* EQUED = 'R' or 'B', each element of R must be positive.
*
* C (input or output) REAL array, dimension (N)
* The column scale factors for A. If EQUED = 'C' or 'B', A is
* multiplied on the right by diag(C); if EQUED = 'N' or 'R', C
* is not accessed. C is an input argument if FACT = 'F';
* otherwise, C is an output argument. If FACT = 'F' and
* EQUED = 'C' or 'B', each element of C must be positive.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit,
* if EQUED = 'N', B is not modified;
* if TRANS = 'N' and EQUED = 'R' or 'B', B is overwritten by
* diag(R)*B;
* if TRANS = 'T' or 'C' and EQUED = 'C' or 'B', B is
* overwritten by diag(C)*B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) REAL array, dimension (LDX,NRHS)
* If INFO = 0 or INFO = N+1, the N-by-NRHS solution matrix X
* to the original system of equations. Note that A and B are
* modified on exit if EQUED .ne. 'N', and the solution to the
* equilibrated system is inv(diag(C))*X if TRANS = 'N' and
* EQUED = 'C' or 'B', or inv(diag(R))*X if TRANS = 'T' or 'C'
* and EQUED = 'R' or 'B'.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) REAL
* The estimate of the reciprocal condition number of the matrix
* A after equilibration (if done). If RCOND is less than the
* machine precision (in particular, if RCOND = 0), the matrix
* is singular to working precision. This condition is
* indicated by a return code of INFO > 0.
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace/output) REAL array, dimension (3*N)
* On exit, WORK(1) contains the reciprocal pivot growth
* factor norm(A)/norm(U). The "max absolute element" norm is
* used. If WORK(1) is much less than 1, then the stability
* of the LU factorization of the (equilibrated) matrix A
* could be poor. This also means that the solution X, condition
* estimator RCOND, and forward error bound FERR could be
* unreliable. If factorization fails with 0 0: if INFO = i, and i is
* <= N: U(i,i) is exactly zero. The factorization
* has been completed, but the factor U is exactly
* singular, so the solution and error bounds
* could not be computed. RCOND = 0 is returned.
* = N+1: U is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
*
* value of RCOND would suggest.
* =====================================================================
* Moved setting of INFO = N+1 so INFO does not subsequently get
* overwritten. Sven, 17 Mar 05.
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param trans
* @param n
* @param kl
* @param ku
* @param nrhs
* @param ab
* @param ldab
* @param afb
* @param ldafb
* @param ipiv
* @param equed
* @param r
* @param c
* @param b
* @param ldb
* @param x
* @param ldx
* @param rcond
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void sgbsvx(java.lang.String fact, java.lang.String trans, int n, int kl, int ku, int nrhs, float[] ab, int ldab, float[] afb, int ldafb, int[] ipiv, org.netlib.util.StringW equed, float[] r, float[] c, float[] b, int ldb, float[] x, int ldx, org.netlib.util.floatW rcond, float[] ferr, float[] berr, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGBSVX uses the LU factorization to compute the solution to a real
* system of linear equations A * X = B, A**T * X = B, or A**H * X = B,
* where A is a band matrix of order N with KL subdiagonals and KU
* superdiagonals, and X and B are N-by-NRHS matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed by this subroutine:
*
* 1. If FACT = 'E', real scaling factors are computed to equilibrate
* the system:
* TRANS = 'N': diag(R)*A*diag(C) *inv(diag(C))*X = diag(R)*B
* TRANS = 'T': (diag(R)*A*diag(C))**T *inv(diag(R))*X = diag(C)*B
* TRANS = 'C': (diag(R)*A*diag(C))**H *inv(diag(R))*X = diag(C)*B
* Whether or not the system will be equilibrated depends on the
* scaling of the matrix A, but if equilibration is used, A is
* overwritten by diag(R)*A*diag(C) and B by diag(R)*B (if TRANS='N')
* or diag(C)*B (if TRANS = 'T' or 'C').
*
* 2. If FACT = 'N' or 'E', the LU decomposition is used to factor the
* matrix A (after equilibration if FACT = 'E') as
* A = L * U,
* where L is a product of permutation and unit lower triangular
* matrices with KL subdiagonals, and U is upper triangular with
* KL+KU superdiagonals.
*
* 3. If some U(i,i)=0, so that U is exactly singular, then the routine
* returns with INFO = i. Otherwise, the factored form of A is used
* to estimate the condition number of the matrix A. If the
* reciprocal of the condition number is less than machine precision,
* INFO = N+1 is returned as a warning, but the routine still goes on
* to solve for X and compute error bounds as described below.
*
* 4. The system of equations is solved for X using the factored form
* of A.
*
* 5. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* 6. If equilibration was used, the matrix X is premultiplied by
* diag(C) (if TRANS = 'N') or diag(R) (if TRANS = 'T' or 'C') so
* that it solves the original system before equilibration.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of the matrix A is
* supplied on entry, and if not, whether the matrix A should be
* equilibrated before it is factored.
* = 'F': On entry, AFB and IPIV contain the factored form of
* A. If EQUED is not 'N', the matrix A has been
* equilibrated with scaling factors given by R and C.
* AB, AFB, and IPIV are not modified.
* = 'N': The matrix A will be copied to AFB and factored.
* = 'E': The matrix A will be equilibrated if necessary, then
* copied to AFB and factored.
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations.
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Transpose)
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AB (input/output) REAL array, dimension (LDAB,N)
* On entry, the matrix A in band storage, in rows 1 to KL+KU+1.
* The j-th column of A is stored in the j-th column of the
* array AB as follows:
* AB(KU+1+i-j,j) = A(i,j) for max(1,j-KU)<=i<=min(N,j+kl)
*
* If FACT = 'F' and EQUED is not 'N', then A must have been
* equilibrated by the scaling factors in R and/or C. AB is not
* modified if FACT = 'F' or 'N', or if FACT = 'E' and
* EQUED = 'N' on exit.
*
* On exit, if EQUED .ne. 'N', A is scaled as follows:
* EQUED = 'R': A := diag(R) * A
* EQUED = 'C': A := A * diag(C)
* EQUED = 'B': A := diag(R) * A * diag(C).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KL+KU+1.
*
* AFB (input or output) REAL array, dimension (LDAFB,N)
* If FACT = 'F', then AFB is an input argument and on entry
* contains details of the LU factorization of the band matrix
* A, as computed by SGBTRF. U is stored as an upper triangular
* band matrix with KL+KU superdiagonals in rows 1 to KL+KU+1,
* and the multipliers used during the factorization are stored
* in rows KL+KU+2 to 2*KL+KU+1. If EQUED .ne. 'N', then AFB is
* the factored form of the equilibrated matrix A.
*
* If FACT = 'N', then AFB is an output argument and on exit
* returns details of the LU factorization of A.
*
* If FACT = 'E', then AFB is an output argument and on exit
* returns details of the LU factorization of the equilibrated
* matrix A (see the description of AB for the form of the
* equilibrated matrix).
*
* LDAFB (input) INTEGER
* The leading dimension of the array AFB. LDAFB >= 2*KL+KU+1.
*
* IPIV (input or output) INTEGER array, dimension (N)
* If FACT = 'F', then IPIV is an input argument and on entry
* contains the pivot indices from the factorization A = L*U
* as computed by SGBTRF; row i of the matrix was interchanged
* with row IPIV(i).
*
* If FACT = 'N', then IPIV is an output argument and on exit
* contains the pivot indices from the factorization A = L*U
* of the original matrix A.
*
* If FACT = 'E', then IPIV is an output argument and on exit
* contains the pivot indices from the factorization A = L*U
* of the equilibrated matrix A.
*
* EQUED (input or output) CHARACTER*1
* Specifies the form of equilibration that was done.
* = 'N': No equilibration (always true if FACT = 'N').
* = 'R': Row equilibration, i.e., A has been premultiplied by
* diag(R).
* = 'C': Column equilibration, i.e., A has been postmultiplied
* by diag(C).
* = 'B': Both row and column equilibration, i.e., A has been
* replaced by diag(R) * A * diag(C).
* EQUED is an input argument if FACT = 'F'; otherwise, it is an
* output argument.
*
* R (input or output) REAL array, dimension (N)
* The row scale factors for A. If EQUED = 'R' or 'B', A is
* multiplied on the left by diag(R); if EQUED = 'N' or 'C', R
* is not accessed. R is an input argument if FACT = 'F';
* otherwise, R is an output argument. If FACT = 'F' and
* EQUED = 'R' or 'B', each element of R must be positive.
*
* C (input or output) REAL array, dimension (N)
* The column scale factors for A. If EQUED = 'C' or 'B', A is
* multiplied on the right by diag(C); if EQUED = 'N' or 'R', C
* is not accessed. C is an input argument if FACT = 'F';
* otherwise, C is an output argument. If FACT = 'F' and
* EQUED = 'C' or 'B', each element of C must be positive.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit,
* if EQUED = 'N', B is not modified;
* if TRANS = 'N' and EQUED = 'R' or 'B', B is overwritten by
* diag(R)*B;
* if TRANS = 'T' or 'C' and EQUED = 'C' or 'B', B is
* overwritten by diag(C)*B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) REAL array, dimension (LDX,NRHS)
* If INFO = 0 or INFO = N+1, the N-by-NRHS solution matrix X
* to the original system of equations. Note that A and B are
* modified on exit if EQUED .ne. 'N', and the solution to the
* equilibrated system is inv(diag(C))*X if TRANS = 'N' and
* EQUED = 'C' or 'B', or inv(diag(R))*X if TRANS = 'T' or 'C'
* and EQUED = 'R' or 'B'.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) REAL
* The estimate of the reciprocal condition number of the matrix
* A after equilibration (if done). If RCOND is less than the
* machine precision (in particular, if RCOND = 0), the matrix
* is singular to working precision. This condition is
* indicated by a return code of INFO > 0.
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace/output) REAL array, dimension (3*N)
* On exit, WORK(1) contains the reciprocal pivot growth
* factor norm(A)/norm(U). The "max absolute element" norm is
* used. If WORK(1) is much less than 1, then the stability
* of the LU factorization of the (equilibrated) matrix A
* could be poor. This also means that the solution X, condition
* estimator RCOND, and forward error bound FERR could be
* unreliable. If factorization fails with 0 0: if INFO = i, and i is
* <= N: U(i,i) is exactly zero. The factorization
* has been completed, but the factor U is exactly
* singular, so the solution and error bounds
* could not be computed. RCOND = 0 is returned.
* = N+1: U is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
*
* value of RCOND would suggest.
* =====================================================================
* Moved setting of INFO = N+1 so INFO does not subsequently get
* overwritten. Sven, 17 Mar 05.
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param trans
* @param n
* @param kl
* @param ku
* @param nrhs
* @param ab
* @param _ab_offset
* @param ldab
* @param afb
* @param _afb_offset
* @param ldafb
* @param ipiv
* @param _ipiv_offset
* @param equed
* @param r
* @param _r_offset
* @param c
* @param _c_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param rcond
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void sgbsvx(java.lang.String fact, java.lang.String trans, int n, int kl, int ku, int nrhs, float[] ab, int _ab_offset, int ldab, float[] afb, int _afb_offset, int ldafb, int[] ipiv, int _ipiv_offset, org.netlib.util.StringW equed, float[] r, int _r_offset, float[] c, int _c_offset, float[] b, int _b_offset, int ldb, float[] x, int _x_offset, int ldx, org.netlib.util.floatW rcond, float[] ferr, int _ferr_offset, float[] berr, int _berr_offset, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGBTF2 computes an LU factorization of a real m-by-n band matrix A
* using partial pivoting with row interchanges.
*
* This is the unblocked version of the algorithm, calling Level 2 BLAS.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* AB (input/output) REAL array, dimension (LDAB,N)
* On entry, the matrix A in band storage, in rows KL+1 to
* 2*KL+KU+1; rows 1 to KL of the array need not be set.
* The j-th column of A is stored in the j-th column of the
* array AB as follows:
* AB(kl+ku+1+i-j,j) = A(i,j) for max(1,j-ku)<=i<=min(m,j+kl)
*
* On exit, details of the factorization: U is stored as an
* upper triangular band matrix with KL+KU superdiagonals in
* rows 1 to KL+KU+1, and the multipliers used during the
* factorization are stored in rows KL+KU+2 to 2*KL+KU+1.
* See below for further details.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= 2*KL+KU+1.
*
* IPIV (output) INTEGER array, dimension (min(M,N))
* The pivot indices; for 1 <= i <= min(M,N), row i of the
* matrix was interchanged with row IPIV(i).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = +i, U(i,i) is exactly zero. The factorization
* has been completed, but the factor U is exactly
* singular, and division by zero will occur if it is used
* to solve a system of equations.
*
* Further Details
* ===============
*
* The band storage scheme is illustrated by the following example, when
* M = N = 6, KL = 2, KU = 1:
*
* On entry: On exit:
*
* * * * + + + * * * u14 u25 u36
* * * + + + + * * u13 u24 u35 u46
* * a12 a23 a34 a45 a56 * u12 u23 u34 u45 u56
* a11 a22 a33 a44 a55 a66 u11 u22 u33 u44 u55 u66
* a21 a32 a43 a54 a65 * m21 m32 m43 m54 m65 *
* a31 a42 a53 a64 * * m31 m42 m53 m64 * *
*
* Array elements marked * are not used by the routine; elements marked
* + need not be set on entry, but are required by the routine to store
* elements of U, because of fill-in resulting from the row
* interchanges.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param kl
* @param ku
* @param ab
* @param ldab
* @param ipiv
* @param info
*
*/
abstract public void sgbtf2(int m, int n, int kl, int ku, float[] ab, int ldab, int[] ipiv, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGBTF2 computes an LU factorization of a real m-by-n band matrix A
* using partial pivoting with row interchanges.
*
* This is the unblocked version of the algorithm, calling Level 2 BLAS.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* AB (input/output) REAL array, dimension (LDAB,N)
* On entry, the matrix A in band storage, in rows KL+1 to
* 2*KL+KU+1; rows 1 to KL of the array need not be set.
* The j-th column of A is stored in the j-th column of the
* array AB as follows:
* AB(kl+ku+1+i-j,j) = A(i,j) for max(1,j-ku)<=i<=min(m,j+kl)
*
* On exit, details of the factorization: U is stored as an
* upper triangular band matrix with KL+KU superdiagonals in
* rows 1 to KL+KU+1, and the multipliers used during the
* factorization are stored in rows KL+KU+2 to 2*KL+KU+1.
* See below for further details.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= 2*KL+KU+1.
*
* IPIV (output) INTEGER array, dimension (min(M,N))
* The pivot indices; for 1 <= i <= min(M,N), row i of the
* matrix was interchanged with row IPIV(i).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = +i, U(i,i) is exactly zero. The factorization
* has been completed, but the factor U is exactly
* singular, and division by zero will occur if it is used
* to solve a system of equations.
*
* Further Details
* ===============
*
* The band storage scheme is illustrated by the following example, when
* M = N = 6, KL = 2, KU = 1:
*
* On entry: On exit:
*
* * * * + + + * * * u14 u25 u36
* * * + + + + * * u13 u24 u35 u46
* * a12 a23 a34 a45 a56 * u12 u23 u34 u45 u56
* a11 a22 a33 a44 a55 a66 u11 u22 u33 u44 u55 u66
* a21 a32 a43 a54 a65 * m21 m32 m43 m54 m65 *
* a31 a42 a53 a64 * * m31 m42 m53 m64 * *
*
* Array elements marked * are not used by the routine; elements marked
* + need not be set on entry, but are required by the routine to store
* elements of U, because of fill-in resulting from the row
* interchanges.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param kl
* @param ku
* @param ab
* @param _ab_offset
* @param ldab
* @param ipiv
* @param _ipiv_offset
* @param info
*
*/
abstract public void sgbtf2(int m, int n, int kl, int ku, float[] ab, int _ab_offset, int ldab, int[] ipiv, int _ipiv_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGBTRF computes an LU factorization of a real m-by-n band matrix A
* using partial pivoting with row interchanges.
*
* This is the blocked version of the algorithm, calling Level 3 BLAS.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* AB (input/output) REAL array, dimension (LDAB,N)
* On entry, the matrix A in band storage, in rows KL+1 to
* 2*KL+KU+1; rows 1 to KL of the array need not be set.
* The j-th column of A is stored in the j-th column of the
* array AB as follows:
* AB(kl+ku+1+i-j,j) = A(i,j) for max(1,j-ku)<=i<=min(m,j+kl)
*
* On exit, details of the factorization: U is stored as an
* upper triangular band matrix with KL+KU superdiagonals in
* rows 1 to KL+KU+1, and the multipliers used during the
* factorization are stored in rows KL+KU+2 to 2*KL+KU+1.
* See below for further details.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= 2*KL+KU+1.
*
* IPIV (output) INTEGER array, dimension (min(M,N))
* The pivot indices; for 1 <= i <= min(M,N), row i of the
* matrix was interchanged with row IPIV(i).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = +i, U(i,i) is exactly zero. The factorization
* has been completed, but the factor U is exactly
* singular, and division by zero will occur if it is used
* to solve a system of equations.
*
* Further Details
* ===============
*
* The band storage scheme is illustrated by the following example, when
* M = N = 6, KL = 2, KU = 1:
*
* On entry: On exit:
*
* * * * + + + * * * u14 u25 u36
* * * + + + + * * u13 u24 u35 u46
* * a12 a23 a34 a45 a56 * u12 u23 u34 u45 u56
* a11 a22 a33 a44 a55 a66 u11 u22 u33 u44 u55 u66
* a21 a32 a43 a54 a65 * m21 m32 m43 m54 m65 *
* a31 a42 a53 a64 * * m31 m42 m53 m64 * *
*
* Array elements marked * are not used by the routine; elements marked
* + need not be set on entry, but are required by the routine to store
* elements of U because of fill-in resulting from the row interchanges.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param kl
* @param ku
* @param ab
* @param ldab
* @param ipiv
* @param info
*
*/
abstract public void sgbtrf(int m, int n, int kl, int ku, float[] ab, int ldab, int[] ipiv, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGBTRF computes an LU factorization of a real m-by-n band matrix A
* using partial pivoting with row interchanges.
*
* This is the blocked version of the algorithm, calling Level 3 BLAS.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* AB (input/output) REAL array, dimension (LDAB,N)
* On entry, the matrix A in band storage, in rows KL+1 to
* 2*KL+KU+1; rows 1 to KL of the array need not be set.
* The j-th column of A is stored in the j-th column of the
* array AB as follows:
* AB(kl+ku+1+i-j,j) = A(i,j) for max(1,j-ku)<=i<=min(m,j+kl)
*
* On exit, details of the factorization: U is stored as an
* upper triangular band matrix with KL+KU superdiagonals in
* rows 1 to KL+KU+1, and the multipliers used during the
* factorization are stored in rows KL+KU+2 to 2*KL+KU+1.
* See below for further details.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= 2*KL+KU+1.
*
* IPIV (output) INTEGER array, dimension (min(M,N))
* The pivot indices; for 1 <= i <= min(M,N), row i of the
* matrix was interchanged with row IPIV(i).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = +i, U(i,i) is exactly zero. The factorization
* has been completed, but the factor U is exactly
* singular, and division by zero will occur if it is used
* to solve a system of equations.
*
* Further Details
* ===============
*
* The band storage scheme is illustrated by the following example, when
* M = N = 6, KL = 2, KU = 1:
*
* On entry: On exit:
*
* * * * + + + * * * u14 u25 u36
* * * + + + + * * u13 u24 u35 u46
* * a12 a23 a34 a45 a56 * u12 u23 u34 u45 u56
* a11 a22 a33 a44 a55 a66 u11 u22 u33 u44 u55 u66
* a21 a32 a43 a54 a65 * m21 m32 m43 m54 m65 *
* a31 a42 a53 a64 * * m31 m42 m53 m64 * *
*
* Array elements marked * are not used by the routine; elements marked
* + need not be set on entry, but are required by the routine to store
* elements of U because of fill-in resulting from the row interchanges.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param kl
* @param ku
* @param ab
* @param _ab_offset
* @param ldab
* @param ipiv
* @param _ipiv_offset
* @param info
*
*/
abstract public void sgbtrf(int m, int n, int kl, int ku, float[] ab, int _ab_offset, int ldab, int[] ipiv, int _ipiv_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGBTRS solves a system of linear equations
* A * X = B or A' * X = B
* with a general band matrix A using the LU factorization computed
* by SGBTRF.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations.
* = 'N': A * X = B (No transpose)
* = 'T': A'* X = B (Transpose)
* = 'C': A'* X = B (Conjugate transpose = Transpose)
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AB (input) REAL array, dimension (LDAB,N)
* Details of the LU factorization of the band matrix A, as
* computed by SGBTRF. U is stored as an upper triangular band
* matrix with KL+KU superdiagonals in rows 1 to KL+KU+1, and
* the multipliers used during the factorization are stored in
* rows KL+KU+2 to 2*KL+KU+1.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= 2*KL+KU+1.
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices; for 1 <= i <= N, row i of the matrix was
* interchanged with row IPIV(i).
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param trans
* @param n
* @param kl
* @param ku
* @param nrhs
* @param ab
* @param ldab
* @param ipiv
* @param b
* @param ldb
* @param info
*
*/
abstract public void sgbtrs(java.lang.String trans, int n, int kl, int ku, int nrhs, float[] ab, int ldab, int[] ipiv, float[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGBTRS solves a system of linear equations
* A * X = B or A' * X = B
* with a general band matrix A using the LU factorization computed
* by SGBTRF.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations.
* = 'N': A * X = B (No transpose)
* = 'T': A'* X = B (Transpose)
* = 'C': A'* X = B (Conjugate transpose = Transpose)
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AB (input) REAL array, dimension (LDAB,N)
* Details of the LU factorization of the band matrix A, as
* computed by SGBTRF. U is stored as an upper triangular band
* matrix with KL+KU superdiagonals in rows 1 to KL+KU+1, and
* the multipliers used during the factorization are stored in
* rows KL+KU+2 to 2*KL+KU+1.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= 2*KL+KU+1.
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices; for 1 <= i <= N, row i of the matrix was
* interchanged with row IPIV(i).
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param trans
* @param n
* @param kl
* @param ku
* @param nrhs
* @param ab
* @param _ab_offset
* @param ldab
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void sgbtrs(java.lang.String trans, int n, int kl, int ku, int nrhs, float[] ab, int _ab_offset, int ldab, int[] ipiv, int _ipiv_offset, float[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGEBAK forms the right or left eigenvectors of a real general matrix
* by backward transformation on the computed eigenvectors of the
* balanced matrix output by SGEBAL.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* Specifies the type of backward transformation required:
* = 'N', do nothing, return immediately;
* = 'P', do backward transformation for permutation only;
* = 'S', do backward transformation for scaling only;
* = 'B', do backward transformations for both permutation and
* scaling.
* JOB must be the same as the argument JOB supplied to SGEBAL.
*
* SIDE (input) CHARACTER*1
* = 'R': V contains right eigenvectors;
* = 'L': V contains left eigenvectors.
*
* N (input) INTEGER
* The number of rows of the matrix V. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* The integers ILO and IHI determined by SGEBAL.
* 1 <= ILO <= IHI <= N, if N > 0; ILO=1 and IHI=0, if N=0.
*
* SCALE (input) REAL array, dimension (N)
* Details of the permutation and scaling factors, as returned
* by SGEBAL.
*
* M (input) INTEGER
* The number of columns of the matrix V. M >= 0.
*
* V (input/output) REAL array, dimension (LDV,M)
* On entry, the matrix of right or left eigenvectors to be
* transformed, as returned by SHSEIN or STREVC.
* On exit, V is overwritten by the transformed eigenvectors.
*
* LDV (input) INTEGER
* The leading dimension of the array V. LDV >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param side
* @param n
* @param ilo
* @param ihi
* @param scale
* @param m
* @param v
* @param ldv
* @param info
*
*/
abstract public void sgebak(java.lang.String job, java.lang.String side, int n, int ilo, int ihi, float[] scale, int m, float[] v, int ldv, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGEBAK forms the right or left eigenvectors of a real general matrix
* by backward transformation on the computed eigenvectors of the
* balanced matrix output by SGEBAL.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* Specifies the type of backward transformation required:
* = 'N', do nothing, return immediately;
* = 'P', do backward transformation for permutation only;
* = 'S', do backward transformation for scaling only;
* = 'B', do backward transformations for both permutation and
* scaling.
* JOB must be the same as the argument JOB supplied to SGEBAL.
*
* SIDE (input) CHARACTER*1
* = 'R': V contains right eigenvectors;
* = 'L': V contains left eigenvectors.
*
* N (input) INTEGER
* The number of rows of the matrix V. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* The integers ILO and IHI determined by SGEBAL.
* 1 <= ILO <= IHI <= N, if N > 0; ILO=1 and IHI=0, if N=0.
*
* SCALE (input) REAL array, dimension (N)
* Details of the permutation and scaling factors, as returned
* by SGEBAL.
*
* M (input) INTEGER
* The number of columns of the matrix V. M >= 0.
*
* V (input/output) REAL array, dimension (LDV,M)
* On entry, the matrix of right or left eigenvectors to be
* transformed, as returned by SHSEIN or STREVC.
* On exit, V is overwritten by the transformed eigenvectors.
*
* LDV (input) INTEGER
* The leading dimension of the array V. LDV >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param side
* @param n
* @param ilo
* @param ihi
* @param scale
* @param _scale_offset
* @param m
* @param v
* @param _v_offset
* @param ldv
* @param info
*
*/
abstract public void sgebak(java.lang.String job, java.lang.String side, int n, int ilo, int ihi, float[] scale, int _scale_offset, int m, float[] v, int _v_offset, int ldv, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGEBAL balances a general real matrix A. This involves, first,
* permuting A by a similarity transformation to isolate eigenvalues
* in the first 1 to ILO-1 and last IHI+1 to N elements on the
* diagonal; and second, applying a diagonal similarity transformation
* to rows and columns ILO to IHI to make the rows and columns as
* close in norm as possible. Both steps are optional.
*
* Balancing may reduce the 1-norm of the matrix, and improve the
* accuracy of the computed eigenvalues and/or eigenvectors.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* Specifies the operations to be performed on A:
* = 'N': none: simply set ILO = 1, IHI = N, SCALE(I) = 1.0
* for i = 1,...,N;
* = 'P': permute only;
* = 'S': scale only;
* = 'B': both permute and scale.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the input matrix A.
* On exit, A is overwritten by the balanced matrix.
* If JOB = 'N', A is not referenced.
* See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* ILO (output) INTEGER
* IHI (output) INTEGER
* ILO and IHI are set to integers such that on exit
* A(i,j) = 0 if i > j and j = 1,...,ILO-1 or I = IHI+1,...,N.
* If JOB = 'N' or 'S', ILO = 1 and IHI = N.
*
* SCALE (output) REAL array, dimension (N)
* Details of the permutations and scaling factors applied to
* A. If P(j) is the index of the row and column interchanged
* with row and column j and D(j) is the scaling factor
* applied to row and column j, then
* SCALE(j) = P(j) for j = 1,...,ILO-1
* = D(j) for j = ILO,...,IHI
* = P(j) for j = IHI+1,...,N.
* The order in which the interchanges are made is N to IHI+1,
* then 1 to ILO-1.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* The permutations consist of row and column interchanges which put
* the matrix in the form
*
* ( T1 X Y )
* P A P = ( 0 B Z )
* ( 0 0 T2 )
*
* where T1 and T2 are upper triangular matrices whose eigenvalues lie
* along the diagonal. The column indices ILO and IHI mark the starting
* and ending columns of the submatrix B. Balancing consists of applying
* a diagonal similarity transformation inv(D) * B * D to make the
* 1-norms of each row of B and its corresponding column nearly equal.
* The output matrix is
*
* ( T1 X*D Y )
* ( 0 inv(D)*B*D inv(D)*Z ).
* ( 0 0 T2 )
*
* Information about the permutations P and the diagonal matrix D is
* returned in the vector SCALE.
*
* This subroutine is based on the EISPACK routine BALANC.
*
* Modified by Tzu-Yi Chen, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param n
* @param a
* @param lda
* @param ilo
* @param ihi
* @param scale
* @param info
*
*/
abstract public void sgebal(java.lang.String job, int n, float[] a, int lda, org.netlib.util.intW ilo, org.netlib.util.intW ihi, float[] scale, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGEBAL balances a general real matrix A. This involves, first,
* permuting A by a similarity transformation to isolate eigenvalues
* in the first 1 to ILO-1 and last IHI+1 to N elements on the
* diagonal; and second, applying a diagonal similarity transformation
* to rows and columns ILO to IHI to make the rows and columns as
* close in norm as possible. Both steps are optional.
*
* Balancing may reduce the 1-norm of the matrix, and improve the
* accuracy of the computed eigenvalues and/or eigenvectors.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* Specifies the operations to be performed on A:
* = 'N': none: simply set ILO = 1, IHI = N, SCALE(I) = 1.0
* for i = 1,...,N;
* = 'P': permute only;
* = 'S': scale only;
* = 'B': both permute and scale.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the input matrix A.
* On exit, A is overwritten by the balanced matrix.
* If JOB = 'N', A is not referenced.
* See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* ILO (output) INTEGER
* IHI (output) INTEGER
* ILO and IHI are set to integers such that on exit
* A(i,j) = 0 if i > j and j = 1,...,ILO-1 or I = IHI+1,...,N.
* If JOB = 'N' or 'S', ILO = 1 and IHI = N.
*
* SCALE (output) REAL array, dimension (N)
* Details of the permutations and scaling factors applied to
* A. If P(j) is the index of the row and column interchanged
* with row and column j and D(j) is the scaling factor
* applied to row and column j, then
* SCALE(j) = P(j) for j = 1,...,ILO-1
* = D(j) for j = ILO,...,IHI
* = P(j) for j = IHI+1,...,N.
* The order in which the interchanges are made is N to IHI+1,
* then 1 to ILO-1.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* The permutations consist of row and column interchanges which put
* the matrix in the form
*
* ( T1 X Y )
* P A P = ( 0 B Z )
* ( 0 0 T2 )
*
* where T1 and T2 are upper triangular matrices whose eigenvalues lie
* along the diagonal. The column indices ILO and IHI mark the starting
* and ending columns of the submatrix B. Balancing consists of applying
* a diagonal similarity transformation inv(D) * B * D to make the
* 1-norms of each row of B and its corresponding column nearly equal.
* The output matrix is
*
* ( T1 X*D Y )
* ( 0 inv(D)*B*D inv(D)*Z ).
* ( 0 0 T2 )
*
* Information about the permutations P and the diagonal matrix D is
* returned in the vector SCALE.
*
* This subroutine is based on the EISPACK routine BALANC.
*
* Modified by Tzu-Yi Chen, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param n
* @param a
* @param _a_offset
* @param lda
* @param ilo
* @param ihi
* @param scale
* @param _scale_offset
* @param info
*
*/
abstract public void sgebal(java.lang.String job, int n, float[] a, int _a_offset, int lda, org.netlib.util.intW ilo, org.netlib.util.intW ihi, float[] scale, int _scale_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGEBD2 reduces a real general m by n matrix A to upper or lower
* bidiagonal form B by an orthogonal transformation: Q' * A * P = B.
*
* If m >= n, B is upper bidiagonal; if m < n, B is lower bidiagonal.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows in the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns in the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the m by n general matrix to be reduced.
* On exit,
* if m >= n, the diagonal and the first superdiagonal are
* overwritten with the upper bidiagonal matrix B; the
* elements below the diagonal, with the array TAUQ, represent
* the orthogonal matrix Q as a product of elementary
* reflectors, and the elements above the first superdiagonal,
* with the array TAUP, represent the orthogonal matrix P as
* a product of elementary reflectors;
* if m < n, the diagonal and the first subdiagonal are
* overwritten with the lower bidiagonal matrix B; the
* elements below the first subdiagonal, with the array TAUQ,
* represent the orthogonal matrix Q as a product of
* elementary reflectors, and the elements above the diagonal,
* with the array TAUP, represent the orthogonal matrix P as
* a product of elementary reflectors.
* See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* D (output) REAL array, dimension (min(M,N))
* The diagonal elements of the bidiagonal matrix B:
* D(i) = A(i,i).
*
* E (output) REAL array, dimension (min(M,N)-1)
* The off-diagonal elements of the bidiagonal matrix B:
* if m >= n, E(i) = A(i,i+1) for i = 1,2,...,n-1;
* if m < n, E(i) = A(i+1,i) for i = 1,2,...,m-1.
*
* TAUQ (output) REAL array dimension (min(M,N))
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix Q. See Further Details.
*
* TAUP (output) REAL array, dimension (min(M,N))
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix P. See Further Details.
*
* WORK (workspace) REAL array, dimension (max(M,N))
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* The matrices Q and P are represented as products of elementary
* reflectors:
*
* If m >= n,
*
* Q = H(1) H(2) . . . H(n) and P = G(1) G(2) . . . G(n-1)
*
* Each H(i) and G(i) has the form:
*
* H(i) = I - tauq * v * v' and G(i) = I - taup * u * u'
*
* where tauq and taup are real scalars, and v and u are real vectors;
* v(1:i-1) = 0, v(i) = 1, and v(i+1:m) is stored on exit in A(i+1:m,i);
* u(1:i) = 0, u(i+1) = 1, and u(i+2:n) is stored on exit in A(i,i+2:n);
* tauq is stored in TAUQ(i) and taup in TAUP(i).
*
* If m < n,
*
* Q = H(1) H(2) . . . H(m-1) and P = G(1) G(2) . . . G(m)
*
* Each H(i) and G(i) has the form:
*
* H(i) = I - tauq * v * v' and G(i) = I - taup * u * u'
*
* where tauq and taup are real scalars, and v and u are real vectors;
* v(1:i) = 0, v(i+1) = 1, and v(i+2:m) is stored on exit in A(i+2:m,i);
* u(1:i-1) = 0, u(i) = 1, and u(i+1:n) is stored on exit in A(i,i+1:n);
* tauq is stored in TAUQ(i) and taup in TAUP(i).
*
* The contents of A on exit are illustrated by the following examples:
*
* m = 6 and n = 5 (m > n): m = 5 and n = 6 (m < n):
*
* ( d e u1 u1 u1 ) ( d u1 u1 u1 u1 u1 )
* ( v1 d e u2 u2 ) ( e d u2 u2 u2 u2 )
* ( v1 v2 d e u3 ) ( v1 e d u3 u3 u3 )
* ( v1 v2 v3 d e ) ( v1 v2 e d u4 u4 )
* ( v1 v2 v3 v4 d ) ( v1 v2 v3 e d u5 )
* ( v1 v2 v3 v4 v5 )
*
* where d and e denote diagonal and off-diagonal elements of B, vi
* denotes an element of the vector defining H(i), and ui an element of
* the vector defining G(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param d
* @param e
* @param tauq
* @param taup
* @param work
* @param info
*
*/
abstract public void sgebd2(int m, int n, float[] a, int lda, float[] d, float[] e, float[] tauq, float[] taup, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGEBD2 reduces a real general m by n matrix A to upper or lower
* bidiagonal form B by an orthogonal transformation: Q' * A * P = B.
*
* If m >= n, B is upper bidiagonal; if m < n, B is lower bidiagonal.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows in the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns in the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the m by n general matrix to be reduced.
* On exit,
* if m >= n, the diagonal and the first superdiagonal are
* overwritten with the upper bidiagonal matrix B; the
* elements below the diagonal, with the array TAUQ, represent
* the orthogonal matrix Q as a product of elementary
* reflectors, and the elements above the first superdiagonal,
* with the array TAUP, represent the orthogonal matrix P as
* a product of elementary reflectors;
* if m < n, the diagonal and the first subdiagonal are
* overwritten with the lower bidiagonal matrix B; the
* elements below the first subdiagonal, with the array TAUQ,
* represent the orthogonal matrix Q as a product of
* elementary reflectors, and the elements above the diagonal,
* with the array TAUP, represent the orthogonal matrix P as
* a product of elementary reflectors.
* See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* D (output) REAL array, dimension (min(M,N))
* The diagonal elements of the bidiagonal matrix B:
* D(i) = A(i,i).
*
* E (output) REAL array, dimension (min(M,N)-1)
* The off-diagonal elements of the bidiagonal matrix B:
* if m >= n, E(i) = A(i,i+1) for i = 1,2,...,n-1;
* if m < n, E(i) = A(i+1,i) for i = 1,2,...,m-1.
*
* TAUQ (output) REAL array dimension (min(M,N))
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix Q. See Further Details.
*
* TAUP (output) REAL array, dimension (min(M,N))
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix P. See Further Details.
*
* WORK (workspace) REAL array, dimension (max(M,N))
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* The matrices Q and P are represented as products of elementary
* reflectors:
*
* If m >= n,
*
* Q = H(1) H(2) . . . H(n) and P = G(1) G(2) . . . G(n-1)
*
* Each H(i) and G(i) has the form:
*
* H(i) = I - tauq * v * v' and G(i) = I - taup * u * u'
*
* where tauq and taup are real scalars, and v and u are real vectors;
* v(1:i-1) = 0, v(i) = 1, and v(i+1:m) is stored on exit in A(i+1:m,i);
* u(1:i) = 0, u(i+1) = 1, and u(i+2:n) is stored on exit in A(i,i+2:n);
* tauq is stored in TAUQ(i) and taup in TAUP(i).
*
* If m < n,
*
* Q = H(1) H(2) . . . H(m-1) and P = G(1) G(2) . . . G(m)
*
* Each H(i) and G(i) has the form:
*
* H(i) = I - tauq * v * v' and G(i) = I - taup * u * u'
*
* where tauq and taup are real scalars, and v and u are real vectors;
* v(1:i) = 0, v(i+1) = 1, and v(i+2:m) is stored on exit in A(i+2:m,i);
* u(1:i-1) = 0, u(i) = 1, and u(i+1:n) is stored on exit in A(i,i+1:n);
* tauq is stored in TAUQ(i) and taup in TAUP(i).
*
* The contents of A on exit are illustrated by the following examples:
*
* m = 6 and n = 5 (m > n): m = 5 and n = 6 (m < n):
*
* ( d e u1 u1 u1 ) ( d u1 u1 u1 u1 u1 )
* ( v1 d e u2 u2 ) ( e d u2 u2 u2 u2 )
* ( v1 v2 d e u3 ) ( v1 e d u3 u3 u3 )
* ( v1 v2 v3 d e ) ( v1 v2 e d u4 u4 )
* ( v1 v2 v3 v4 d ) ( v1 v2 v3 e d u5 )
* ( v1 v2 v3 v4 v5 )
*
* where d and e denote diagonal and off-diagonal elements of B, vi
* denotes an element of the vector defining H(i), and ui an element of
* the vector defining G(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param tauq
* @param _tauq_offset
* @param taup
* @param _taup_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void sgebd2(int m, int n, float[] a, int _a_offset, int lda, float[] d, int _d_offset, float[] e, int _e_offset, float[] tauq, int _tauq_offset, float[] taup, int _taup_offset, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGEBRD reduces a general real M-by-N matrix A to upper or lower
* bidiagonal form B by an orthogonal transformation: Q**T * A * P = B.
*
* If m >= n, B is upper bidiagonal; if m < n, B is lower bidiagonal.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows in the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns in the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N general matrix to be reduced.
* On exit,
* if m >= n, the diagonal and the first superdiagonal are
* overwritten with the upper bidiagonal matrix B; the
* elements below the diagonal, with the array TAUQ, represent
* the orthogonal matrix Q as a product of elementary
* reflectors, and the elements above the first superdiagonal,
* with the array TAUP, represent the orthogonal matrix P as
* a product of elementary reflectors;
* if m < n, the diagonal and the first subdiagonal are
* overwritten with the lower bidiagonal matrix B; the
* elements below the first subdiagonal, with the array TAUQ,
* represent the orthogonal matrix Q as a product of
* elementary reflectors, and the elements above the diagonal,
* with the array TAUP, represent the orthogonal matrix P as
* a product of elementary reflectors.
* See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* D (output) REAL array, dimension (min(M,N))
* The diagonal elements of the bidiagonal matrix B:
* D(i) = A(i,i).
*
* E (output) REAL array, dimension (min(M,N)-1)
* The off-diagonal elements of the bidiagonal matrix B:
* if m >= n, E(i) = A(i,i+1) for i = 1,2,...,n-1;
* if m < n, E(i) = A(i+1,i) for i = 1,2,...,m-1.
*
* TAUQ (output) REAL array dimension (min(M,N))
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix Q. See Further Details.
*
* TAUP (output) REAL array, dimension (min(M,N))
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix P. See Further Details.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of the array WORK. LWORK >= max(1,M,N).
* For optimum performance LWORK >= (M+N)*NB, where NB
* is the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* The matrices Q and P are represented as products of elementary
* reflectors:
*
* If m >= n,
*
* Q = H(1) H(2) . . . H(n) and P = G(1) G(2) . . . G(n-1)
*
* Each H(i) and G(i) has the form:
*
* H(i) = I - tauq * v * v' and G(i) = I - taup * u * u'
*
* where tauq and taup are real scalars, and v and u are real vectors;
* v(1:i-1) = 0, v(i) = 1, and v(i+1:m) is stored on exit in A(i+1:m,i);
* u(1:i) = 0, u(i+1) = 1, and u(i+2:n) is stored on exit in A(i,i+2:n);
* tauq is stored in TAUQ(i) and taup in TAUP(i).
*
* If m < n,
*
* Q = H(1) H(2) . . . H(m-1) and P = G(1) G(2) . . . G(m)
*
* Each H(i) and G(i) has the form:
*
* H(i) = I - tauq * v * v' and G(i) = I - taup * u * u'
*
* where tauq and taup are real scalars, and v and u are real vectors;
* v(1:i) = 0, v(i+1) = 1, and v(i+2:m) is stored on exit in A(i+2:m,i);
* u(1:i-1) = 0, u(i) = 1, and u(i+1:n) is stored on exit in A(i,i+1:n);
* tauq is stored in TAUQ(i) and taup in TAUP(i).
*
* The contents of A on exit are illustrated by the following examples:
*
* m = 6 and n = 5 (m > n): m = 5 and n = 6 (m < n):
*
* ( d e u1 u1 u1 ) ( d u1 u1 u1 u1 u1 )
* ( v1 d e u2 u2 ) ( e d u2 u2 u2 u2 )
* ( v1 v2 d e u3 ) ( v1 e d u3 u3 u3 )
* ( v1 v2 v3 d e ) ( v1 v2 e d u4 u4 )
* ( v1 v2 v3 v4 d ) ( v1 v2 v3 e d u5 )
* ( v1 v2 v3 v4 v5 )
*
* where d and e denote diagonal and off-diagonal elements of B, vi
* denotes an element of the vector defining H(i), and ui an element of
* the vector defining G(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param d
* @param e
* @param tauq
* @param taup
* @param work
* @param lwork
* @param info
*
*/
abstract public void sgebrd(int m, int n, float[] a, int lda, float[] d, float[] e, float[] tauq, float[] taup, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGEBRD reduces a general real M-by-N matrix A to upper or lower
* bidiagonal form B by an orthogonal transformation: Q**T * A * P = B.
*
* If m >= n, B is upper bidiagonal; if m < n, B is lower bidiagonal.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows in the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns in the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N general matrix to be reduced.
* On exit,
* if m >= n, the diagonal and the first superdiagonal are
* overwritten with the upper bidiagonal matrix B; the
* elements below the diagonal, with the array TAUQ, represent
* the orthogonal matrix Q as a product of elementary
* reflectors, and the elements above the first superdiagonal,
* with the array TAUP, represent the orthogonal matrix P as
* a product of elementary reflectors;
* if m < n, the diagonal and the first subdiagonal are
* overwritten with the lower bidiagonal matrix B; the
* elements below the first subdiagonal, with the array TAUQ,
* represent the orthogonal matrix Q as a product of
* elementary reflectors, and the elements above the diagonal,
* with the array TAUP, represent the orthogonal matrix P as
* a product of elementary reflectors.
* See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* D (output) REAL array, dimension (min(M,N))
* The diagonal elements of the bidiagonal matrix B:
* D(i) = A(i,i).
*
* E (output) REAL array, dimension (min(M,N)-1)
* The off-diagonal elements of the bidiagonal matrix B:
* if m >= n, E(i) = A(i,i+1) for i = 1,2,...,n-1;
* if m < n, E(i) = A(i+1,i) for i = 1,2,...,m-1.
*
* TAUQ (output) REAL array dimension (min(M,N))
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix Q. See Further Details.
*
* TAUP (output) REAL array, dimension (min(M,N))
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix P. See Further Details.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of the array WORK. LWORK >= max(1,M,N).
* For optimum performance LWORK >= (M+N)*NB, where NB
* is the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* The matrices Q and P are represented as products of elementary
* reflectors:
*
* If m >= n,
*
* Q = H(1) H(2) . . . H(n) and P = G(1) G(2) . . . G(n-1)
*
* Each H(i) and G(i) has the form:
*
* H(i) = I - tauq * v * v' and G(i) = I - taup * u * u'
*
* where tauq and taup are real scalars, and v and u are real vectors;
* v(1:i-1) = 0, v(i) = 1, and v(i+1:m) is stored on exit in A(i+1:m,i);
* u(1:i) = 0, u(i+1) = 1, and u(i+2:n) is stored on exit in A(i,i+2:n);
* tauq is stored in TAUQ(i) and taup in TAUP(i).
*
* If m < n,
*
* Q = H(1) H(2) . . . H(m-1) and P = G(1) G(2) . . . G(m)
*
* Each H(i) and G(i) has the form:
*
* H(i) = I - tauq * v * v' and G(i) = I - taup * u * u'
*
* where tauq and taup are real scalars, and v and u are real vectors;
* v(1:i) = 0, v(i+1) = 1, and v(i+2:m) is stored on exit in A(i+2:m,i);
* u(1:i-1) = 0, u(i) = 1, and u(i+1:n) is stored on exit in A(i,i+1:n);
* tauq is stored in TAUQ(i) and taup in TAUP(i).
*
* The contents of A on exit are illustrated by the following examples:
*
* m = 6 and n = 5 (m > n): m = 5 and n = 6 (m < n):
*
* ( d e u1 u1 u1 ) ( d u1 u1 u1 u1 u1 )
* ( v1 d e u2 u2 ) ( e d u2 u2 u2 u2 )
* ( v1 v2 d e u3 ) ( v1 e d u3 u3 u3 )
* ( v1 v2 v3 d e ) ( v1 v2 e d u4 u4 )
* ( v1 v2 v3 v4 d ) ( v1 v2 v3 e d u5 )
* ( v1 v2 v3 v4 v5 )
*
* where d and e denote diagonal and off-diagonal elements of B, vi
* denotes an element of the vector defining H(i), and ui an element of
* the vector defining G(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param tauq
* @param _tauq_offset
* @param taup
* @param _taup_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sgebrd(int m, int n, float[] a, int _a_offset, int lda, float[] d, int _d_offset, float[] e, int _e_offset, float[] tauq, int _tauq_offset, float[] taup, int _taup_offset, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGECON estimates the reciprocal of the condition number of a general
* real matrix A, in either the 1-norm or the infinity-norm, using
* the LU factorization computed by SGETRF.
*
* An estimate is obtained for norm(inv(A)), and the reciprocal of the
* condition number is computed as
* RCOND = 1 / ( norm(A) * norm(inv(A)) ).
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies whether the 1-norm condition number or the
* infinity-norm condition number is required:
* = '1' or 'O': 1-norm;
* = 'I': Infinity-norm.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The factors L and U from the factorization A = P*L*U
* as computed by SGETRF.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* ANORM (input) REAL
* If NORM = '1' or 'O', the 1-norm of the original matrix A.
* If NORM = 'I', the infinity-norm of the original matrix A.
*
* RCOND (output) REAL
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(norm(A) * norm(inv(A))).
*
* WORK (workspace) REAL array, dimension (4*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param n
* @param a
* @param lda
* @param anorm
* @param rcond
* @param work
* @param iwork
* @param info
*
*/
abstract public void sgecon(java.lang.String norm, int n, float[] a, int lda, float anorm, org.netlib.util.floatW rcond, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGECON estimates the reciprocal of the condition number of a general
* real matrix A, in either the 1-norm or the infinity-norm, using
* the LU factorization computed by SGETRF.
*
* An estimate is obtained for norm(inv(A)), and the reciprocal of the
* condition number is computed as
* RCOND = 1 / ( norm(A) * norm(inv(A)) ).
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies whether the 1-norm condition number or the
* infinity-norm condition number is required:
* = '1' or 'O': 1-norm;
* = 'I': Infinity-norm.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The factors L and U from the factorization A = P*L*U
* as computed by SGETRF.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* ANORM (input) REAL
* If NORM = '1' or 'O', the 1-norm of the original matrix A.
* If NORM = 'I', the infinity-norm of the original matrix A.
*
* RCOND (output) REAL
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(norm(A) * norm(inv(A))).
*
* WORK (workspace) REAL array, dimension (4*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param n
* @param a
* @param _a_offset
* @param lda
* @param anorm
* @param rcond
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void sgecon(java.lang.String norm, int n, float[] a, int _a_offset, int lda, float anorm, org.netlib.util.floatW rcond, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGEEQU computes row and column scalings intended to equilibrate an
* M-by-N matrix A and reduce its condition number. R returns the row
* scale factors and C the column scale factors, chosen to try to make
* the largest element in each row and column of the matrix B with
* elements B(i,j)=R(i)*A(i,j)*C(j) have absolute value 1.
*
* R(i) and C(j) are restricted to be between SMLNUM = smallest safe
* number and BIGNUM = largest safe number. Use of these scaling
* factors is not guaranteed to reduce the condition number of A but
* works well in practice.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The M-by-N matrix whose equilibration factors are
* to be computed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* R (output) REAL array, dimension (M)
* If INFO = 0 or INFO > M, R contains the row scale factors
* for A.
*
* C (output) REAL array, dimension (N)
* If INFO = 0, C contains the column scale factors for A.
*
* ROWCND (output) REAL
* If INFO = 0 or INFO > M, ROWCND contains the ratio of the
* smallest R(i) to the largest R(i). If ROWCND >= 0.1 and
* AMAX is neither too large nor too small, it is not worth
* scaling by R.
*
* COLCND (output) REAL
* If INFO = 0, COLCND contains the ratio of the smallest
* C(i) to the largest C(i). If COLCND >= 0.1, it is not
* worth scaling by C.
*
* AMAX (output) REAL
* Absolute value of largest matrix element. If AMAX is very
* close to overflow or very close to underflow, the matrix
* should be scaled.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= M: the i-th row of A is exactly zero
* > M: the (i-M)-th column of A is exactly zero
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param r
* @param c
* @param rowcnd
* @param colcnd
* @param amax
* @param info
*
*/
abstract public void sgeequ(int m, int n, float[] a, int lda, float[] r, float[] c, org.netlib.util.floatW rowcnd, org.netlib.util.floatW colcnd, org.netlib.util.floatW amax, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGEEQU computes row and column scalings intended to equilibrate an
* M-by-N matrix A and reduce its condition number. R returns the row
* scale factors and C the column scale factors, chosen to try to make
* the largest element in each row and column of the matrix B with
* elements B(i,j)=R(i)*A(i,j)*C(j) have absolute value 1.
*
* R(i) and C(j) are restricted to be between SMLNUM = smallest safe
* number and BIGNUM = largest safe number. Use of these scaling
* factors is not guaranteed to reduce the condition number of A but
* works well in practice.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The M-by-N matrix whose equilibration factors are
* to be computed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* R (output) REAL array, dimension (M)
* If INFO = 0 or INFO > M, R contains the row scale factors
* for A.
*
* C (output) REAL array, dimension (N)
* If INFO = 0, C contains the column scale factors for A.
*
* ROWCND (output) REAL
* If INFO = 0 or INFO > M, ROWCND contains the ratio of the
* smallest R(i) to the largest R(i). If ROWCND >= 0.1 and
* AMAX is neither too large nor too small, it is not worth
* scaling by R.
*
* COLCND (output) REAL
* If INFO = 0, COLCND contains the ratio of the smallest
* C(i) to the largest C(i). If COLCND >= 0.1, it is not
* worth scaling by C.
*
* AMAX (output) REAL
* Absolute value of largest matrix element. If AMAX is very
* close to overflow or very close to underflow, the matrix
* should be scaled.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= M: the i-th row of A is exactly zero
* > M: the (i-M)-th column of A is exactly zero
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param r
* @param _r_offset
* @param c
* @param _c_offset
* @param rowcnd
* @param colcnd
* @param amax
* @param info
*
*/
abstract public void sgeequ(int m, int n, float[] a, int _a_offset, int lda, float[] r, int _r_offset, float[] c, int _c_offset, org.netlib.util.floatW rowcnd, org.netlib.util.floatW colcnd, org.netlib.util.floatW amax, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGEES computes for an N-by-N real nonsymmetric matrix A, the
* eigenvalues, the real Schur form T, and, optionally, the matrix of
* Schur vectors Z. This gives the Schur factorization A = Z*T*(Z**T).
*
* Optionally, it also orders the eigenvalues on the diagonal of the
* real Schur form so that selected eigenvalues are at the top left.
* The leading columns of Z then form an orthonormal basis for the
* invariant subspace corresponding to the selected eigenvalues.
*
* A matrix is in real Schur form if it is upper quasi-triangular with
* 1-by-1 and 2-by-2 blocks. 2-by-2 blocks will be standardized in the
* form
* [ a b ]
* [ c a ]
*
* where b*c < 0. The eigenvalues of such a block are a +- sqrt(bc).
*
* Arguments
* =========
*
* JOBVS (input) CHARACTER*1
* = 'N': Schur vectors are not computed;
* = 'V': Schur vectors are computed.
*
* SORT (input) CHARACTER*1
* Specifies whether or not to order the eigenvalues on the
* diagonal of the Schur form.
* = 'N': Eigenvalues are not ordered;
* = 'S': Eigenvalues are ordered (see SELECT).
*
* SELECT (external procedure) LOGICAL FUNCTION of two REAL arguments
* SELECT must be declared EXTERNAL in the calling subroutine.
* If SORT = 'S', SELECT is used to select eigenvalues to sort
* to the top left of the Schur form.
* If SORT = 'N', SELECT is not referenced.
* An eigenvalue WR(j)+sqrt(-1)*WI(j) is selected if
* SELECT(WR(j),WI(j)) is true; i.e., if either one of a complex
* conjugate pair of eigenvalues is selected, then both complex
* eigenvalues are selected.
* Note that a selected complex eigenvalue may no longer
* satisfy SELECT(WR(j),WI(j)) = .TRUE. after ordering, since
* ordering may change the value of complex eigenvalues
* (especially if the eigenvalue is ill-conditioned); in this
* case INFO is set to N+2 (see INFO below).
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the N-by-N matrix A.
* On exit, A has been overwritten by its real Schur form T.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* SDIM (output) INTEGER
* If SORT = 'N', SDIM = 0.
* If SORT = 'S', SDIM = number of eigenvalues (after sorting)
* for which SELECT is true. (Complex conjugate
* pairs for which SELECT is true for either
* eigenvalue count as 2.)
*
* WR (output) REAL array, dimension (N)
* WI (output) REAL array, dimension (N)
* WR and WI contain the real and imaginary parts,
* respectively, of the computed eigenvalues in the same order
* that they appear on the diagonal of the output Schur form T.
* Complex conjugate pairs of eigenvalues will appear
* consecutively with the eigenvalue having the positive
* imaginary part first.
*
* VS (output) REAL array, dimension (LDVS,N)
* If JOBVS = 'V', VS contains the orthogonal matrix Z of Schur
* vectors.
* If JOBVS = 'N', VS is not referenced.
*
* LDVS (input) INTEGER
* The leading dimension of the array VS. LDVS >= 1; if
* JOBVS = 'V', LDVS >= N.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) contains the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,3*N).
* For good performance, LWORK must generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* BWORK (workspace) LOGICAL array, dimension (N)
* Not referenced if SORT = 'N'.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, and i is
* <= N: the QR algorithm failed to compute all the
* eigenvalues; elements 1:ILO-1 and i+1:N of WR and WI
* contain those eigenvalues which have converged; if
* JOBVS = 'V', VS contains the matrix which reduces A
* to its partially converged Schur form.
* = N+1: the eigenvalues could not be reordered because some
* eigenvalues were too close to separate (the problem
* is very ill-conditioned);
* = N+2: after reordering, roundoff changed values of some
* complex eigenvalues so that leading eigenvalues in
* the Schur form no longer satisfy SELECT=.TRUE. This
* could also be caused by underflow due to scaling.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobvs
* @param sort
* @param select
* @param n
* @param a
* @param lda
* @param sdim
* @param wr
* @param wi
* @param vs
* @param ldvs
* @param work
* @param lwork
* @param bwork
* @param info
*
*/
abstract public void sgees(java.lang.String jobvs, java.lang.String sort, java.lang.Object select, int n, float[] a, int lda, org.netlib.util.intW sdim, float[] wr, float[] wi, float[] vs, int ldvs, float[] work, int lwork, boolean[] bwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGEES computes for an N-by-N real nonsymmetric matrix A, the
* eigenvalues, the real Schur form T, and, optionally, the matrix of
* Schur vectors Z. This gives the Schur factorization A = Z*T*(Z**T).
*
* Optionally, it also orders the eigenvalues on the diagonal of the
* real Schur form so that selected eigenvalues are at the top left.
* The leading columns of Z then form an orthonormal basis for the
* invariant subspace corresponding to the selected eigenvalues.
*
* A matrix is in real Schur form if it is upper quasi-triangular with
* 1-by-1 and 2-by-2 blocks. 2-by-2 blocks will be standardized in the
* form
* [ a b ]
* [ c a ]
*
* where b*c < 0. The eigenvalues of such a block are a +- sqrt(bc).
*
* Arguments
* =========
*
* JOBVS (input) CHARACTER*1
* = 'N': Schur vectors are not computed;
* = 'V': Schur vectors are computed.
*
* SORT (input) CHARACTER*1
* Specifies whether or not to order the eigenvalues on the
* diagonal of the Schur form.
* = 'N': Eigenvalues are not ordered;
* = 'S': Eigenvalues are ordered (see SELECT).
*
* SELECT (external procedure) LOGICAL FUNCTION of two REAL arguments
* SELECT must be declared EXTERNAL in the calling subroutine.
* If SORT = 'S', SELECT is used to select eigenvalues to sort
* to the top left of the Schur form.
* If SORT = 'N', SELECT is not referenced.
* An eigenvalue WR(j)+sqrt(-1)*WI(j) is selected if
* SELECT(WR(j),WI(j)) is true; i.e., if either one of a complex
* conjugate pair of eigenvalues is selected, then both complex
* eigenvalues are selected.
* Note that a selected complex eigenvalue may no longer
* satisfy SELECT(WR(j),WI(j)) = .TRUE. after ordering, since
* ordering may change the value of complex eigenvalues
* (especially if the eigenvalue is ill-conditioned); in this
* case INFO is set to N+2 (see INFO below).
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the N-by-N matrix A.
* On exit, A has been overwritten by its real Schur form T.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* SDIM (output) INTEGER
* If SORT = 'N', SDIM = 0.
* If SORT = 'S', SDIM = number of eigenvalues (after sorting)
* for which SELECT is true. (Complex conjugate
* pairs for which SELECT is true for either
* eigenvalue count as 2.)
*
* WR (output) REAL array, dimension (N)
* WI (output) REAL array, dimension (N)
* WR and WI contain the real and imaginary parts,
* respectively, of the computed eigenvalues in the same order
* that they appear on the diagonal of the output Schur form T.
* Complex conjugate pairs of eigenvalues will appear
* consecutively with the eigenvalue having the positive
* imaginary part first.
*
* VS (output) REAL array, dimension (LDVS,N)
* If JOBVS = 'V', VS contains the orthogonal matrix Z of Schur
* vectors.
* If JOBVS = 'N', VS is not referenced.
*
* LDVS (input) INTEGER
* The leading dimension of the array VS. LDVS >= 1; if
* JOBVS = 'V', LDVS >= N.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) contains the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,3*N).
* For good performance, LWORK must generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* BWORK (workspace) LOGICAL array, dimension (N)
* Not referenced if SORT = 'N'.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, and i is
* <= N: the QR algorithm failed to compute all the
* eigenvalues; elements 1:ILO-1 and i+1:N of WR and WI
* contain those eigenvalues which have converged; if
* JOBVS = 'V', VS contains the matrix which reduces A
* to its partially converged Schur form.
* = N+1: the eigenvalues could not be reordered because some
* eigenvalues were too close to separate (the problem
* is very ill-conditioned);
* = N+2: after reordering, roundoff changed values of some
* complex eigenvalues so that leading eigenvalues in
* the Schur form no longer satisfy SELECT=.TRUE. This
* could also be caused by underflow due to scaling.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobvs
* @param sort
* @param select
* @param n
* @param a
* @param _a_offset
* @param lda
* @param sdim
* @param wr
* @param _wr_offset
* @param wi
* @param _wi_offset
* @param vs
* @param _vs_offset
* @param ldvs
* @param work
* @param _work_offset
* @param lwork
* @param bwork
* @param _bwork_offset
* @param info
*
*/
abstract public void sgees(java.lang.String jobvs, java.lang.String sort, java.lang.Object select, int n, float[] a, int _a_offset, int lda, org.netlib.util.intW sdim, float[] wr, int _wr_offset, float[] wi, int _wi_offset, float[] vs, int _vs_offset, int ldvs, float[] work, int _work_offset, int lwork, boolean[] bwork, int _bwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGEESX computes for an N-by-N real nonsymmetric matrix A, the
* eigenvalues, the real Schur form T, and, optionally, the matrix of
* Schur vectors Z. This gives the Schur factorization A = Z*T*(Z**T).
*
* Optionally, it also orders the eigenvalues on the diagonal of the
* real Schur form so that selected eigenvalues are at the top left;
* computes a reciprocal condition number for the average of the
* selected eigenvalues (RCONDE); and computes a reciprocal condition
* number for the right invariant subspace corresponding to the
* selected eigenvalues (RCONDV). The leading columns of Z form an
* orthonormal basis for this invariant subspace.
*
* For further explanation of the reciprocal condition numbers RCONDE
* and RCONDV, see Section 4.10 of the LAPACK Users' Guide (where
* these quantities are called s and sep respectively).
*
* A real matrix is in real Schur form if it is upper quasi-triangular
* with 1-by-1 and 2-by-2 blocks. 2-by-2 blocks will be standardized in
* the form
* [ a b ]
* [ c a ]
*
* where b*c < 0. The eigenvalues of such a block are a +- sqrt(bc).
*
* Arguments
* =========
*
* JOBVS (input) CHARACTER*1
* = 'N': Schur vectors are not computed;
* = 'V': Schur vectors are computed.
*
* SORT (input) CHARACTER*1
* Specifies whether or not to order the eigenvalues on the
* diagonal of the Schur form.
* = 'N': Eigenvalues are not ordered;
* = 'S': Eigenvalues are ordered (see SELECT).
*
* SELECT (external procedure) LOGICAL FUNCTION of two REAL arguments
* SELECT must be declared EXTERNAL in the calling subroutine.
* If SORT = 'S', SELECT is used to select eigenvalues to sort
* to the top left of the Schur form.
* If SORT = 'N', SELECT is not referenced.
* An eigenvalue WR(j)+sqrt(-1)*WI(j) is selected if
* SELECT(WR(j),WI(j)) is true; i.e., if either one of a
* complex conjugate pair of eigenvalues is selected, then both
* are. Note that a selected complex eigenvalue may no longer
* satisfy SELECT(WR(j),WI(j)) = .TRUE. after ordering, since
* ordering may change the value of complex eigenvalues
* (especially if the eigenvalue is ill-conditioned); in this
* case INFO may be set to N+3 (see INFO below).
*
* SENSE (input) CHARACTER*1
* Determines which reciprocal condition numbers are computed.
* = 'N': None are computed;
* = 'E': Computed for average of selected eigenvalues only;
* = 'V': Computed for selected right invariant subspace only;
* = 'B': Computed for both.
* If SENSE = 'E', 'V' or 'B', SORT must equal 'S'.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA, N)
* On entry, the N-by-N matrix A.
* On exit, A is overwritten by its real Schur form T.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* SDIM (output) INTEGER
* If SORT = 'N', SDIM = 0.
* If SORT = 'S', SDIM = number of eigenvalues (after sorting)
* for which SELECT is true. (Complex conjugate
* pairs for which SELECT is true for either
* eigenvalue count as 2.)
*
* WR (output) REAL array, dimension (N)
* WI (output) REAL array, dimension (N)
* WR and WI contain the real and imaginary parts, respectively,
* of the computed eigenvalues, in the same order that they
* appear on the diagonal of the output Schur form T. Complex
* conjugate pairs of eigenvalues appear consecutively with the
* eigenvalue having the positive imaginary part first.
*
* VS (output) REAL array, dimension (LDVS,N)
* If JOBVS = 'V', VS contains the orthogonal matrix Z of Schur
* vectors.
* If JOBVS = 'N', VS is not referenced.
*
* LDVS (input) INTEGER
* The leading dimension of the array VS. LDVS >= 1, and if
* JOBVS = 'V', LDVS >= N.
*
* RCONDE (output) REAL
* If SENSE = 'E' or 'B', RCONDE contains the reciprocal
* condition number for the average of the selected eigenvalues.
* Not referenced if SENSE = 'N' or 'V'.
*
* RCONDV (output) REAL
* If SENSE = 'V' or 'B', RCONDV contains the reciprocal
* condition number for the selected right invariant subspace.
* Not referenced if SENSE = 'N' or 'E'.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,3*N).
* Also, if SENSE = 'E' or 'V' or 'B',
* LWORK >= N+2*SDIM*(N-SDIM), where SDIM is the number of
* selected eigenvalues computed by this routine. Note that
* N+2*SDIM*(N-SDIM) <= N+N*N/2. Note also that an error is only
* returned if LWORK < max(1,3*N), but if SENSE = 'E' or 'V' or
* 'B' this may not be large enough.
* For good performance, LWORK must generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates upper bounds on the optimal sizes of the
* arrays WORK and IWORK, returns these values as the first
* entries of the WORK and IWORK arrays, and no error messages
* related to LWORK or LIWORK are issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* LIWORK >= 1; if SENSE = 'V' or 'B', LIWORK >= SDIM*(N-SDIM).
* Note that SDIM*(N-SDIM) <= N*N/4. Note also that an error is
* only returned if LIWORK < 1, but if SENSE = 'V' or 'B' this
* may not be large enough.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates upper bounds on the optimal sizes of
* the arrays WORK and IWORK, returns these values as the first
* entries of the WORK and IWORK arrays, and no error messages
* related to LWORK or LIWORK are issued by XERBLA.
*
* BWORK (workspace) LOGICAL array, dimension (N)
* Not referenced if SORT = 'N'.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, and i is
* <= N: the QR algorithm failed to compute all the
* eigenvalues; elements 1:ILO-1 and i+1:N of WR and WI
* contain those eigenvalues which have converged; if
* JOBVS = 'V', VS contains the transformation which
* reduces A to its partially converged Schur form.
* = N+1: the eigenvalues could not be reordered because some
* eigenvalues were too close to separate (the problem
* is very ill-conditioned);
* = N+2: after reordering, roundoff changed values of some
* complex eigenvalues so that leading eigenvalues in
* the Schur form no longer satisfy SELECT=.TRUE. This
* could also be caused by underflow due to scaling.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobvs
* @param sort
* @param select
* @param sense
* @param n
* @param a
* @param lda
* @param sdim
* @param wr
* @param wi
* @param vs
* @param ldvs
* @param rconde
* @param rcondv
* @param work
* @param lwork
* @param iwork
* @param liwork
* @param bwork
* @param info
*
*/
abstract public void sgeesx(java.lang.String jobvs, java.lang.String sort, java.lang.Object select, java.lang.String sense, int n, float[] a, int lda, org.netlib.util.intW sdim, float[] wr, float[] wi, float[] vs, int ldvs, org.netlib.util.floatW rconde, org.netlib.util.floatW rcondv, float[] work, int lwork, int[] iwork, int liwork, boolean[] bwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGEESX computes for an N-by-N real nonsymmetric matrix A, the
* eigenvalues, the real Schur form T, and, optionally, the matrix of
* Schur vectors Z. This gives the Schur factorization A = Z*T*(Z**T).
*
* Optionally, it also orders the eigenvalues on the diagonal of the
* real Schur form so that selected eigenvalues are at the top left;
* computes a reciprocal condition number for the average of the
* selected eigenvalues (RCONDE); and computes a reciprocal condition
* number for the right invariant subspace corresponding to the
* selected eigenvalues (RCONDV). The leading columns of Z form an
* orthonormal basis for this invariant subspace.
*
* For further explanation of the reciprocal condition numbers RCONDE
* and RCONDV, see Section 4.10 of the LAPACK Users' Guide (where
* these quantities are called s and sep respectively).
*
* A real matrix is in real Schur form if it is upper quasi-triangular
* with 1-by-1 and 2-by-2 blocks. 2-by-2 blocks will be standardized in
* the form
* [ a b ]
* [ c a ]
*
* where b*c < 0. The eigenvalues of such a block are a +- sqrt(bc).
*
* Arguments
* =========
*
* JOBVS (input) CHARACTER*1
* = 'N': Schur vectors are not computed;
* = 'V': Schur vectors are computed.
*
* SORT (input) CHARACTER*1
* Specifies whether or not to order the eigenvalues on the
* diagonal of the Schur form.
* = 'N': Eigenvalues are not ordered;
* = 'S': Eigenvalues are ordered (see SELECT).
*
* SELECT (external procedure) LOGICAL FUNCTION of two REAL arguments
* SELECT must be declared EXTERNAL in the calling subroutine.
* If SORT = 'S', SELECT is used to select eigenvalues to sort
* to the top left of the Schur form.
* If SORT = 'N', SELECT is not referenced.
* An eigenvalue WR(j)+sqrt(-1)*WI(j) is selected if
* SELECT(WR(j),WI(j)) is true; i.e., if either one of a
* complex conjugate pair of eigenvalues is selected, then both
* are. Note that a selected complex eigenvalue may no longer
* satisfy SELECT(WR(j),WI(j)) = .TRUE. after ordering, since
* ordering may change the value of complex eigenvalues
* (especially if the eigenvalue is ill-conditioned); in this
* case INFO may be set to N+3 (see INFO below).
*
* SENSE (input) CHARACTER*1
* Determines which reciprocal condition numbers are computed.
* = 'N': None are computed;
* = 'E': Computed for average of selected eigenvalues only;
* = 'V': Computed for selected right invariant subspace only;
* = 'B': Computed for both.
* If SENSE = 'E', 'V' or 'B', SORT must equal 'S'.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA, N)
* On entry, the N-by-N matrix A.
* On exit, A is overwritten by its real Schur form T.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* SDIM (output) INTEGER
* If SORT = 'N', SDIM = 0.
* If SORT = 'S', SDIM = number of eigenvalues (after sorting)
* for which SELECT is true. (Complex conjugate
* pairs for which SELECT is true for either
* eigenvalue count as 2.)
*
* WR (output) REAL array, dimension (N)
* WI (output) REAL array, dimension (N)
* WR and WI contain the real and imaginary parts, respectively,
* of the computed eigenvalues, in the same order that they
* appear on the diagonal of the output Schur form T. Complex
* conjugate pairs of eigenvalues appear consecutively with the
* eigenvalue having the positive imaginary part first.
*
* VS (output) REAL array, dimension (LDVS,N)
* If JOBVS = 'V', VS contains the orthogonal matrix Z of Schur
* vectors.
* If JOBVS = 'N', VS is not referenced.
*
* LDVS (input) INTEGER
* The leading dimension of the array VS. LDVS >= 1, and if
* JOBVS = 'V', LDVS >= N.
*
* RCONDE (output) REAL
* If SENSE = 'E' or 'B', RCONDE contains the reciprocal
* condition number for the average of the selected eigenvalues.
* Not referenced if SENSE = 'N' or 'V'.
*
* RCONDV (output) REAL
* If SENSE = 'V' or 'B', RCONDV contains the reciprocal
* condition number for the selected right invariant subspace.
* Not referenced if SENSE = 'N' or 'E'.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,3*N).
* Also, if SENSE = 'E' or 'V' or 'B',
* LWORK >= N+2*SDIM*(N-SDIM), where SDIM is the number of
* selected eigenvalues computed by this routine. Note that
* N+2*SDIM*(N-SDIM) <= N+N*N/2. Note also that an error is only
* returned if LWORK < max(1,3*N), but if SENSE = 'E' or 'V' or
* 'B' this may not be large enough.
* For good performance, LWORK must generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates upper bounds on the optimal sizes of the
* arrays WORK and IWORK, returns these values as the first
* entries of the WORK and IWORK arrays, and no error messages
* related to LWORK or LIWORK are issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* LIWORK >= 1; if SENSE = 'V' or 'B', LIWORK >= SDIM*(N-SDIM).
* Note that SDIM*(N-SDIM) <= N*N/4. Note also that an error is
* only returned if LIWORK < 1, but if SENSE = 'V' or 'B' this
* may not be large enough.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates upper bounds on the optimal sizes of
* the arrays WORK and IWORK, returns these values as the first
* entries of the WORK and IWORK arrays, and no error messages
* related to LWORK or LIWORK are issued by XERBLA.
*
* BWORK (workspace) LOGICAL array, dimension (N)
* Not referenced if SORT = 'N'.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, and i is
* <= N: the QR algorithm failed to compute all the
* eigenvalues; elements 1:ILO-1 and i+1:N of WR and WI
* contain those eigenvalues which have converged; if
* JOBVS = 'V', VS contains the transformation which
* reduces A to its partially converged Schur form.
* = N+1: the eigenvalues could not be reordered because some
* eigenvalues were too close to separate (the problem
* is very ill-conditioned);
* = N+2: after reordering, roundoff changed values of some
* complex eigenvalues so that leading eigenvalues in
* the Schur form no longer satisfy SELECT=.TRUE. This
* could also be caused by underflow due to scaling.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobvs
* @param sort
* @param select
* @param sense
* @param n
* @param a
* @param _a_offset
* @param lda
* @param sdim
* @param wr
* @param _wr_offset
* @param wi
* @param _wi_offset
* @param vs
* @param _vs_offset
* @param ldvs
* @param rconde
* @param rcondv
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param liwork
* @param bwork
* @param _bwork_offset
* @param info
*
*/
abstract public void sgeesx(java.lang.String jobvs, java.lang.String sort, java.lang.Object select, java.lang.String sense, int n, float[] a, int _a_offset, int lda, org.netlib.util.intW sdim, float[] wr, int _wr_offset, float[] wi, int _wi_offset, float[] vs, int _vs_offset, int ldvs, org.netlib.util.floatW rconde, org.netlib.util.floatW rcondv, float[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int liwork, boolean[] bwork, int _bwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGEEV computes for an N-by-N real nonsymmetric matrix A, the
* eigenvalues and, optionally, the left and/or right eigenvectors.
*
* The right eigenvector v(j) of A satisfies
* A * v(j) = lambda(j) * v(j)
* where lambda(j) is its eigenvalue.
* The left eigenvector u(j) of A satisfies
* u(j)**H * A = lambda(j) * u(j)**H
* where u(j)**H denotes the conjugate transpose of u(j).
*
* The computed eigenvectors are normalized to have Euclidean norm
* equal to 1 and largest component real.
*
* Arguments
* =========
*
* JOBVL (input) CHARACTER*1
* = 'N': left eigenvectors of A are not computed;
* = 'V': left eigenvectors of A are computed.
*
* JOBVR (input) CHARACTER*1
* = 'N': right eigenvectors of A are not computed;
* = 'V': right eigenvectors of A are computed.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the N-by-N matrix A.
* On exit, A has been overwritten.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* WR (output) REAL array, dimension (N)
* WI (output) REAL array, dimension (N)
* WR and WI contain the real and imaginary parts,
* respectively, of the computed eigenvalues. Complex
* conjugate pairs of eigenvalues appear consecutively
* with the eigenvalue having the positive imaginary part
* first.
*
* VL (output) REAL array, dimension (LDVL,N)
* If JOBVL = 'V', the left eigenvectors u(j) are stored one
* after another in the columns of VL, in the same order
* as their eigenvalues.
* If JOBVL = 'N', VL is not referenced.
* If the j-th eigenvalue is real, then u(j) = VL(:,j),
* the j-th column of VL.
* If the j-th and (j+1)-st eigenvalues form a complex
* conjugate pair, then u(j) = VL(:,j) + i*VL(:,j+1) and
* u(j+1) = VL(:,j) - i*VL(:,j+1).
*
* LDVL (input) INTEGER
* The leading dimension of the array VL. LDVL >= 1; if
* JOBVL = 'V', LDVL >= N.
*
* VR (output) REAL array, dimension (LDVR,N)
* If JOBVR = 'V', the right eigenvectors v(j) are stored one
* after another in the columns of VR, in the same order
* as their eigenvalues.
* If JOBVR = 'N', VR is not referenced.
* If the j-th eigenvalue is real, then v(j) = VR(:,j),
* the j-th column of VR.
* If the j-th and (j+1)-st eigenvalues form a complex
* conjugate pair, then v(j) = VR(:,j) + i*VR(:,j+1) and
* v(j+1) = VR(:,j) - i*VR(:,j+1).
*
* LDVR (input) INTEGER
* The leading dimension of the array VR. LDVR >= 1; if
* JOBVR = 'V', LDVR >= N.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,3*N), and
* if JOBVL = 'V' or JOBVR = 'V', LWORK >= 4*N. For good
* performance, LWORK must generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, the QR algorithm failed to compute all the
* eigenvalues, and no eigenvectors have been computed;
* elements i+1:N of WR and WI contain eigenvalues which
* have converged.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobvl
* @param jobvr
* @param n
* @param a
* @param lda
* @param wr
* @param wi
* @param vl
* @param ldvl
* @param vr
* @param ldvr
* @param work
* @param lwork
* @param info
*
*/
abstract public void sgeev(java.lang.String jobvl, java.lang.String jobvr, int n, float[] a, int lda, float[] wr, float[] wi, float[] vl, int ldvl, float[] vr, int ldvr, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGEEV computes for an N-by-N real nonsymmetric matrix A, the
* eigenvalues and, optionally, the left and/or right eigenvectors.
*
* The right eigenvector v(j) of A satisfies
* A * v(j) = lambda(j) * v(j)
* where lambda(j) is its eigenvalue.
* The left eigenvector u(j) of A satisfies
* u(j)**H * A = lambda(j) * u(j)**H
* where u(j)**H denotes the conjugate transpose of u(j).
*
* The computed eigenvectors are normalized to have Euclidean norm
* equal to 1 and largest component real.
*
* Arguments
* =========
*
* JOBVL (input) CHARACTER*1
* = 'N': left eigenvectors of A are not computed;
* = 'V': left eigenvectors of A are computed.
*
* JOBVR (input) CHARACTER*1
* = 'N': right eigenvectors of A are not computed;
* = 'V': right eigenvectors of A are computed.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the N-by-N matrix A.
* On exit, A has been overwritten.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* WR (output) REAL array, dimension (N)
* WI (output) REAL array, dimension (N)
* WR and WI contain the real and imaginary parts,
* respectively, of the computed eigenvalues. Complex
* conjugate pairs of eigenvalues appear consecutively
* with the eigenvalue having the positive imaginary part
* first.
*
* VL (output) REAL array, dimension (LDVL,N)
* If JOBVL = 'V', the left eigenvectors u(j) are stored one
* after another in the columns of VL, in the same order
* as their eigenvalues.
* If JOBVL = 'N', VL is not referenced.
* If the j-th eigenvalue is real, then u(j) = VL(:,j),
* the j-th column of VL.
* If the j-th and (j+1)-st eigenvalues form a complex
* conjugate pair, then u(j) = VL(:,j) + i*VL(:,j+1) and
* u(j+1) = VL(:,j) - i*VL(:,j+1).
*
* LDVL (input) INTEGER
* The leading dimension of the array VL. LDVL >= 1; if
* JOBVL = 'V', LDVL >= N.
*
* VR (output) REAL array, dimension (LDVR,N)
* If JOBVR = 'V', the right eigenvectors v(j) are stored one
* after another in the columns of VR, in the same order
* as their eigenvalues.
* If JOBVR = 'N', VR is not referenced.
* If the j-th eigenvalue is real, then v(j) = VR(:,j),
* the j-th column of VR.
* If the j-th and (j+1)-st eigenvalues form a complex
* conjugate pair, then v(j) = VR(:,j) + i*VR(:,j+1) and
* v(j+1) = VR(:,j) - i*VR(:,j+1).
*
* LDVR (input) INTEGER
* The leading dimension of the array VR. LDVR >= 1; if
* JOBVR = 'V', LDVR >= N.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,3*N), and
* if JOBVL = 'V' or JOBVR = 'V', LWORK >= 4*N. For good
* performance, LWORK must generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, the QR algorithm failed to compute all the
* eigenvalues, and no eigenvectors have been computed;
* elements i+1:N of WR and WI contain eigenvalues which
* have converged.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobvl
* @param jobvr
* @param n
* @param a
* @param _a_offset
* @param lda
* @param wr
* @param _wr_offset
* @param wi
* @param _wi_offset
* @param vl
* @param _vl_offset
* @param ldvl
* @param vr
* @param _vr_offset
* @param ldvr
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sgeev(java.lang.String jobvl, java.lang.String jobvr, int n, float[] a, int _a_offset, int lda, float[] wr, int _wr_offset, float[] wi, int _wi_offset, float[] vl, int _vl_offset, int ldvl, float[] vr, int _vr_offset, int ldvr, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGEEVX computes for an N-by-N real nonsymmetric matrix A, the
* eigenvalues and, optionally, the left and/or right eigenvectors.
*
* Optionally also, it computes a balancing transformation to improve
* the conditioning of the eigenvalues and eigenvectors (ILO, IHI,
* SCALE, and ABNRM), reciprocal condition numbers for the eigenvalues
* (RCONDE), and reciprocal condition numbers for the right
* eigenvectors (RCONDV).
*
* The right eigenvector v(j) of A satisfies
* A * v(j) = lambda(j) * v(j)
* where lambda(j) is its eigenvalue.
* The left eigenvector u(j) of A satisfies
* u(j)**H * A = lambda(j) * u(j)**H
* where u(j)**H denotes the conjugate transpose of u(j).
*
* The computed eigenvectors are normalized to have Euclidean norm
* equal to 1 and largest component real.
*
* Balancing a matrix means permuting the rows and columns to make it
* more nearly upper triangular, and applying a diagonal similarity
* transformation D * A * D**(-1), where D is a diagonal matrix, to
* make its rows and columns closer in norm and the condition numbers
* of its eigenvalues and eigenvectors smaller. The computed
* reciprocal condition numbers correspond to the balanced matrix.
* Permuting rows and columns will not change the condition numbers
* (in exact arithmetic) but diagonal scaling will. For further
* explanation of balancing, see section 4.10.2 of the LAPACK
* Users' Guide.
*
* Arguments
* =========
*
* BALANC (input) CHARACTER*1
* Indicates how the input matrix should be diagonally scaled
* and/or permuted to improve the conditioning of its
* eigenvalues.
* = 'N': Do not diagonally scale or permute;
* = 'P': Perform permutations to make the matrix more nearly
* upper triangular. Do not diagonally scale;
* = 'S': Diagonally scale the matrix, i.e. replace A by
* D*A*D**(-1), where D is a diagonal matrix chosen
* to make the rows and columns of A more equal in
* norm. Do not permute;
* = 'B': Both diagonally scale and permute A.
*
* Computed reciprocal condition numbers will be for the matrix
* after balancing and/or permuting. Permuting does not change
* condition numbers (in exact arithmetic), but balancing does.
*
* JOBVL (input) CHARACTER*1
* = 'N': left eigenvectors of A are not computed;
* = 'V': left eigenvectors of A are computed.
* If SENSE = 'E' or 'B', JOBVL must = 'V'.
*
* JOBVR (input) CHARACTER*1
* = 'N': right eigenvectors of A are not computed;
* = 'V': right eigenvectors of A are computed.
* If SENSE = 'E' or 'B', JOBVR must = 'V'.
*
* SENSE (input) CHARACTER*1
* Determines which reciprocal condition numbers are computed.
* = 'N': None are computed;
* = 'E': Computed for eigenvalues only;
* = 'V': Computed for right eigenvectors only;
* = 'B': Computed for eigenvalues and right eigenvectors.
*
* If SENSE = 'E' or 'B', both left and right eigenvectors
* must also be computed (JOBVL = 'V' and JOBVR = 'V').
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the N-by-N matrix A.
* On exit, A has been overwritten. If JOBVL = 'V' or
* JOBVR = 'V', A contains the real Schur form of the balanced
* version of the input matrix A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* WR (output) REAL array, dimension (N)
* WI (output) REAL array, dimension (N)
* WR and WI contain the real and imaginary parts,
* respectively, of the computed eigenvalues. Complex
* conjugate pairs of eigenvalues will appear consecutively
* with the eigenvalue having the positive imaginary part
* first.
*
* VL (output) REAL array, dimension (LDVL,N)
* If JOBVL = 'V', the left eigenvectors u(j) are stored one
* after another in the columns of VL, in the same order
* as their eigenvalues.
* If JOBVL = 'N', VL is not referenced.
* If the j-th eigenvalue is real, then u(j) = VL(:,j),
* the j-th column of VL.
* If the j-th and (j+1)-st eigenvalues form a complex
* conjugate pair, then u(j) = VL(:,j) + i*VL(:,j+1) and
* u(j+1) = VL(:,j) - i*VL(:,j+1).
*
* LDVL (input) INTEGER
* The leading dimension of the array VL. LDVL >= 1; if
* JOBVL = 'V', LDVL >= N.
*
* VR (output) REAL array, dimension (LDVR,N)
* If JOBVR = 'V', the right eigenvectors v(j) are stored one
* after another in the columns of VR, in the same order
* as their eigenvalues.
* If JOBVR = 'N', VR is not referenced.
* If the j-th eigenvalue is real, then v(j) = VR(:,j),
* the j-th column of VR.
* If the j-th and (j+1)-st eigenvalues form a complex
* conjugate pair, then v(j) = VR(:,j) + i*VR(:,j+1) and
* v(j+1) = VR(:,j) - i*VR(:,j+1).
*
* LDVR (input) INTEGER
* The leading dimension of the array VR. LDVR >= 1, and if
* JOBVR = 'V', LDVR >= N.
*
* ILO (output) INTEGER
* IHI (output) INTEGER
* ILO and IHI are integer values determined when A was
* balanced. The balanced A(i,j) = 0 if I > J and
* J = 1,...,ILO-1 or I = IHI+1,...,N.
*
* SCALE (output) REAL array, dimension (N)
* Details of the permutations and scaling factors applied
* when balancing A. If P(j) is the index of the row and column
* interchanged with row and column j, and D(j) is the scaling
* factor applied to row and column j, then
* SCALE(J) = P(J), for J = 1,...,ILO-1
* = D(J), for J = ILO,...,IHI
* = P(J) for J = IHI+1,...,N.
* The order in which the interchanges are made is N to IHI+1,
* then 1 to ILO-1.
*
* ABNRM (output) REAL
* The one-norm of the balanced matrix (the maximum
* of the sum of absolute values of elements of any column).
*
* RCONDE (output) REAL array, dimension (N)
* RCONDE(j) is the reciprocal condition number of the j-th
* eigenvalue.
*
* RCONDV (output) REAL array, dimension (N)
* RCONDV(j) is the reciprocal condition number of the j-th
* right eigenvector.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. If SENSE = 'N' or 'E',
* LWORK >= max(1,2*N), and if JOBVL = 'V' or JOBVR = 'V',
* LWORK >= 3*N. If SENSE = 'V' or 'B', LWORK >= N*(N+6).
* For good performance, LWORK must generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (2*N-2)
* If SENSE = 'N' or 'E', not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, the QR algorithm failed to compute all the
* eigenvalues, and no eigenvectors or condition numbers
* have been computed; elements 1:ILO-1 and i+1:N of WR
* and WI contain eigenvalues which have converged.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param balanc
* @param jobvl
* @param jobvr
* @param sense
* @param n
* @param a
* @param lda
* @param wr
* @param wi
* @param vl
* @param ldvl
* @param vr
* @param ldvr
* @param ilo
* @param ihi
* @param scale
* @param abnrm
* @param rconde
* @param rcondv
* @param work
* @param lwork
* @param iwork
* @param info
*
*/
abstract public void sgeevx(java.lang.String balanc, java.lang.String jobvl, java.lang.String jobvr, java.lang.String sense, int n, float[] a, int lda, float[] wr, float[] wi, float[] vl, int ldvl, float[] vr, int ldvr, org.netlib.util.intW ilo, org.netlib.util.intW ihi, float[] scale, org.netlib.util.floatW abnrm, float[] rconde, float[] rcondv, float[] work, int lwork, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGEEVX computes for an N-by-N real nonsymmetric matrix A, the
* eigenvalues and, optionally, the left and/or right eigenvectors.
*
* Optionally also, it computes a balancing transformation to improve
* the conditioning of the eigenvalues and eigenvectors (ILO, IHI,
* SCALE, and ABNRM), reciprocal condition numbers for the eigenvalues
* (RCONDE), and reciprocal condition numbers for the right
* eigenvectors (RCONDV).
*
* The right eigenvector v(j) of A satisfies
* A * v(j) = lambda(j) * v(j)
* where lambda(j) is its eigenvalue.
* The left eigenvector u(j) of A satisfies
* u(j)**H * A = lambda(j) * u(j)**H
* where u(j)**H denotes the conjugate transpose of u(j).
*
* The computed eigenvectors are normalized to have Euclidean norm
* equal to 1 and largest component real.
*
* Balancing a matrix means permuting the rows and columns to make it
* more nearly upper triangular, and applying a diagonal similarity
* transformation D * A * D**(-1), where D is a diagonal matrix, to
* make its rows and columns closer in norm and the condition numbers
* of its eigenvalues and eigenvectors smaller. The computed
* reciprocal condition numbers correspond to the balanced matrix.
* Permuting rows and columns will not change the condition numbers
* (in exact arithmetic) but diagonal scaling will. For further
* explanation of balancing, see section 4.10.2 of the LAPACK
* Users' Guide.
*
* Arguments
* =========
*
* BALANC (input) CHARACTER*1
* Indicates how the input matrix should be diagonally scaled
* and/or permuted to improve the conditioning of its
* eigenvalues.
* = 'N': Do not diagonally scale or permute;
* = 'P': Perform permutations to make the matrix more nearly
* upper triangular. Do not diagonally scale;
* = 'S': Diagonally scale the matrix, i.e. replace A by
* D*A*D**(-1), where D is a diagonal matrix chosen
* to make the rows and columns of A more equal in
* norm. Do not permute;
* = 'B': Both diagonally scale and permute A.
*
* Computed reciprocal condition numbers will be for the matrix
* after balancing and/or permuting. Permuting does not change
* condition numbers (in exact arithmetic), but balancing does.
*
* JOBVL (input) CHARACTER*1
* = 'N': left eigenvectors of A are not computed;
* = 'V': left eigenvectors of A are computed.
* If SENSE = 'E' or 'B', JOBVL must = 'V'.
*
* JOBVR (input) CHARACTER*1
* = 'N': right eigenvectors of A are not computed;
* = 'V': right eigenvectors of A are computed.
* If SENSE = 'E' or 'B', JOBVR must = 'V'.
*
* SENSE (input) CHARACTER*1
* Determines which reciprocal condition numbers are computed.
* = 'N': None are computed;
* = 'E': Computed for eigenvalues only;
* = 'V': Computed for right eigenvectors only;
* = 'B': Computed for eigenvalues and right eigenvectors.
*
* If SENSE = 'E' or 'B', both left and right eigenvectors
* must also be computed (JOBVL = 'V' and JOBVR = 'V').
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the N-by-N matrix A.
* On exit, A has been overwritten. If JOBVL = 'V' or
* JOBVR = 'V', A contains the real Schur form of the balanced
* version of the input matrix A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* WR (output) REAL array, dimension (N)
* WI (output) REAL array, dimension (N)
* WR and WI contain the real and imaginary parts,
* respectively, of the computed eigenvalues. Complex
* conjugate pairs of eigenvalues will appear consecutively
* with the eigenvalue having the positive imaginary part
* first.
*
* VL (output) REAL array, dimension (LDVL,N)
* If JOBVL = 'V', the left eigenvectors u(j) are stored one
* after another in the columns of VL, in the same order
* as their eigenvalues.
* If JOBVL = 'N', VL is not referenced.
* If the j-th eigenvalue is real, then u(j) = VL(:,j),
* the j-th column of VL.
* If the j-th and (j+1)-st eigenvalues form a complex
* conjugate pair, then u(j) = VL(:,j) + i*VL(:,j+1) and
* u(j+1) = VL(:,j) - i*VL(:,j+1).
*
* LDVL (input) INTEGER
* The leading dimension of the array VL. LDVL >= 1; if
* JOBVL = 'V', LDVL >= N.
*
* VR (output) REAL array, dimension (LDVR,N)
* If JOBVR = 'V', the right eigenvectors v(j) are stored one
* after another in the columns of VR, in the same order
* as their eigenvalues.
* If JOBVR = 'N', VR is not referenced.
* If the j-th eigenvalue is real, then v(j) = VR(:,j),
* the j-th column of VR.
* If the j-th and (j+1)-st eigenvalues form a complex
* conjugate pair, then v(j) = VR(:,j) + i*VR(:,j+1) and
* v(j+1) = VR(:,j) - i*VR(:,j+1).
*
* LDVR (input) INTEGER
* The leading dimension of the array VR. LDVR >= 1, and if
* JOBVR = 'V', LDVR >= N.
*
* ILO (output) INTEGER
* IHI (output) INTEGER
* ILO and IHI are integer values determined when A was
* balanced. The balanced A(i,j) = 0 if I > J and
* J = 1,...,ILO-1 or I = IHI+1,...,N.
*
* SCALE (output) REAL array, dimension (N)
* Details of the permutations and scaling factors applied
* when balancing A. If P(j) is the index of the row and column
* interchanged with row and column j, and D(j) is the scaling
* factor applied to row and column j, then
* SCALE(J) = P(J), for J = 1,...,ILO-1
* = D(J), for J = ILO,...,IHI
* = P(J) for J = IHI+1,...,N.
* The order in which the interchanges are made is N to IHI+1,
* then 1 to ILO-1.
*
* ABNRM (output) REAL
* The one-norm of the balanced matrix (the maximum
* of the sum of absolute values of elements of any column).
*
* RCONDE (output) REAL array, dimension (N)
* RCONDE(j) is the reciprocal condition number of the j-th
* eigenvalue.
*
* RCONDV (output) REAL array, dimension (N)
* RCONDV(j) is the reciprocal condition number of the j-th
* right eigenvector.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. If SENSE = 'N' or 'E',
* LWORK >= max(1,2*N), and if JOBVL = 'V' or JOBVR = 'V',
* LWORK >= 3*N. If SENSE = 'V' or 'B', LWORK >= N*(N+6).
* For good performance, LWORK must generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (2*N-2)
* If SENSE = 'N' or 'E', not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, the QR algorithm failed to compute all the
* eigenvalues, and no eigenvectors or condition numbers
* have been computed; elements 1:ILO-1 and i+1:N of WR
* and WI contain eigenvalues which have converged.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param balanc
* @param jobvl
* @param jobvr
* @param sense
* @param n
* @param a
* @param _a_offset
* @param lda
* @param wr
* @param _wr_offset
* @param wi
* @param _wi_offset
* @param vl
* @param _vl_offset
* @param ldvl
* @param vr
* @param _vr_offset
* @param ldvr
* @param ilo
* @param ihi
* @param scale
* @param _scale_offset
* @param abnrm
* @param rconde
* @param _rconde_offset
* @param rcondv
* @param _rcondv_offset
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void sgeevx(java.lang.String balanc, java.lang.String jobvl, java.lang.String jobvr, java.lang.String sense, int n, float[] a, int _a_offset, int lda, float[] wr, int _wr_offset, float[] wi, int _wi_offset, float[] vl, int _vl_offset, int ldvl, float[] vr, int _vr_offset, int ldvr, org.netlib.util.intW ilo, org.netlib.util.intW ihi, float[] scale, int _scale_offset, org.netlib.util.floatW abnrm, float[] rconde, int _rconde_offset, float[] rcondv, int _rcondv_offset, float[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* This routine is deprecated and has been replaced by routine SGGES.
*
* SGEGS computes the eigenvalues, real Schur form, and, optionally,
* left and or/right Schur vectors of a real matrix pair (A,B).
* Given two square matrices A and B, the generalized real Schur
* factorization has the form
*
* A = Q*S*Z**T, B = Q*T*Z**T
*
* where Q and Z are orthogonal matrices, T is upper triangular, and S
* is an upper quasi-triangular matrix with 1-by-1 and 2-by-2 diagonal
* blocks, the 2-by-2 blocks corresponding to complex conjugate pairs
* of eigenvalues of (A,B). The columns of Q are the left Schur vectors
* and the columns of Z are the right Schur vectors.
*
* If only the eigenvalues of (A,B) are needed, the driver routine
* SGEGV should be used instead. See SGEGV for a description of the
* eigenvalues of the generalized nonsymmetric eigenvalue problem
* (GNEP).
*
* Arguments
* =========
*
* JOBVSL (input) CHARACTER*1
* = 'N': do not compute the left Schur vectors;
* = 'V': compute the left Schur vectors (returned in VSL).
*
* JOBVSR (input) CHARACTER*1
* = 'N': do not compute the right Schur vectors;
* = 'V': compute the right Schur vectors (returned in VSR).
*
* N (input) INTEGER
* The order of the matrices A, B, VSL, and VSR. N >= 0.
*
* A (input/output) REAL array, dimension (LDA, N)
* On entry, the matrix A.
* On exit, the upper quasi-triangular matrix S from the
* generalized real Schur factorization.
*
* LDA (input) INTEGER
* The leading dimension of A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension (LDB, N)
* On entry, the matrix B.
* On exit, the upper triangular matrix T from the generalized
* real Schur factorization.
*
* LDB (input) INTEGER
* The leading dimension of B. LDB >= max(1,N).
*
* ALPHAR (output) REAL array, dimension (N)
* The real parts of each scalar alpha defining an eigenvalue
* of GNEP.
*
* ALPHAI (output) REAL array, dimension (N)
* The imaginary parts of each scalar alpha defining an
* eigenvalue of GNEP. If ALPHAI(j) is zero, then the j-th
* eigenvalue is real; if positive, then the j-th and (j+1)-st
* eigenvalues are a complex conjugate pair, with
* ALPHAI(j+1) = -ALPHAI(j).
*
* BETA (output) REAL array, dimension (N)
* The scalars beta that define the eigenvalues of GNEP.
* Together, the quantities alpha = (ALPHAR(j),ALPHAI(j)) and
* beta = BETA(j) represent the j-th eigenvalue of the matrix
* pair (A,B), in one of the forms lambda = alpha/beta or
* mu = beta/alpha. Since either lambda or mu may overflow,
* they should not, in general, be computed.
*
* VSL (output) REAL array, dimension (LDVSL,N)
* If JOBVSL = 'V', the matrix of left Schur vectors Q.
* Not referenced if JOBVSL = 'N'.
*
* LDVSL (input) INTEGER
* The leading dimension of the matrix VSL. LDVSL >=1, and
* if JOBVSL = 'V', LDVSL >= N.
*
* VSR (output) REAL array, dimension (LDVSR,N)
* If JOBVSR = 'V', the matrix of right Schur vectors Z.
* Not referenced if JOBVSR = 'N'.
*
* LDVSR (input) INTEGER
* The leading dimension of the matrix VSR. LDVSR >= 1, and
* if JOBVSR = 'V', LDVSR >= N.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,4*N).
* For good performance, LWORK must generally be larger.
* To compute the optimal value of LWORK, call ILAENV to get
* blocksizes (for SGEQRF, SORMQR, and SORGQR.) Then compute:
* NB -- MAX of the blocksizes for SGEQRF, SORMQR, and SORGQR
* The optimal LWORK is 2*N + N*(NB+1).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1,...,N:
* The QZ iteration failed. (A,B) are not in Schur
* form, but ALPHAR(j), ALPHAI(j), and BETA(j) should
* be correct for j=INFO+1,...,N.
* > N: errors that usually indicate LAPACK problems:
* =N+1: error return from SGGBAL
* =N+2: error return from SGEQRF
* =N+3: error return from SORMQR
* =N+4: error return from SORGQR
* =N+5: error return from SGGHRD
* =N+6: error return from SHGEQZ (other than failed
* iteration)
* =N+7: error return from SGGBAK (computing VSL)
* =N+8: error return from SGGBAK (computing VSR)
* =N+9: error return from SLASCL (various places)
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobvsl
* @param jobvsr
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param alphar
* @param alphai
* @param beta
* @param vsl
* @param ldvsl
* @param vsr
* @param ldvsr
* @param work
* @param lwork
* @param info
*
*/
abstract public void sgegs(java.lang.String jobvsl, java.lang.String jobvsr, int n, float[] a, int lda, float[] b, int ldb, float[] alphar, float[] alphai, float[] beta, float[] vsl, int ldvsl, float[] vsr, int ldvsr, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* This routine is deprecated and has been replaced by routine SGGES.
*
* SGEGS computes the eigenvalues, real Schur form, and, optionally,
* left and or/right Schur vectors of a real matrix pair (A,B).
* Given two square matrices A and B, the generalized real Schur
* factorization has the form
*
* A = Q*S*Z**T, B = Q*T*Z**T
*
* where Q and Z are orthogonal matrices, T is upper triangular, and S
* is an upper quasi-triangular matrix with 1-by-1 and 2-by-2 diagonal
* blocks, the 2-by-2 blocks corresponding to complex conjugate pairs
* of eigenvalues of (A,B). The columns of Q are the left Schur vectors
* and the columns of Z are the right Schur vectors.
*
* If only the eigenvalues of (A,B) are needed, the driver routine
* SGEGV should be used instead. See SGEGV for a description of the
* eigenvalues of the generalized nonsymmetric eigenvalue problem
* (GNEP).
*
* Arguments
* =========
*
* JOBVSL (input) CHARACTER*1
* = 'N': do not compute the left Schur vectors;
* = 'V': compute the left Schur vectors (returned in VSL).
*
* JOBVSR (input) CHARACTER*1
* = 'N': do not compute the right Schur vectors;
* = 'V': compute the right Schur vectors (returned in VSR).
*
* N (input) INTEGER
* The order of the matrices A, B, VSL, and VSR. N >= 0.
*
* A (input/output) REAL array, dimension (LDA, N)
* On entry, the matrix A.
* On exit, the upper quasi-triangular matrix S from the
* generalized real Schur factorization.
*
* LDA (input) INTEGER
* The leading dimension of A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension (LDB, N)
* On entry, the matrix B.
* On exit, the upper triangular matrix T from the generalized
* real Schur factorization.
*
* LDB (input) INTEGER
* The leading dimension of B. LDB >= max(1,N).
*
* ALPHAR (output) REAL array, dimension (N)
* The real parts of each scalar alpha defining an eigenvalue
* of GNEP.
*
* ALPHAI (output) REAL array, dimension (N)
* The imaginary parts of each scalar alpha defining an
* eigenvalue of GNEP. If ALPHAI(j) is zero, then the j-th
* eigenvalue is real; if positive, then the j-th and (j+1)-st
* eigenvalues are a complex conjugate pair, with
* ALPHAI(j+1) = -ALPHAI(j).
*
* BETA (output) REAL array, dimension (N)
* The scalars beta that define the eigenvalues of GNEP.
* Together, the quantities alpha = (ALPHAR(j),ALPHAI(j)) and
* beta = BETA(j) represent the j-th eigenvalue of the matrix
* pair (A,B), in one of the forms lambda = alpha/beta or
* mu = beta/alpha. Since either lambda or mu may overflow,
* they should not, in general, be computed.
*
* VSL (output) REAL array, dimension (LDVSL,N)
* If JOBVSL = 'V', the matrix of left Schur vectors Q.
* Not referenced if JOBVSL = 'N'.
*
* LDVSL (input) INTEGER
* The leading dimension of the matrix VSL. LDVSL >=1, and
* if JOBVSL = 'V', LDVSL >= N.
*
* VSR (output) REAL array, dimension (LDVSR,N)
* If JOBVSR = 'V', the matrix of right Schur vectors Z.
* Not referenced if JOBVSR = 'N'.
*
* LDVSR (input) INTEGER
* The leading dimension of the matrix VSR. LDVSR >= 1, and
* if JOBVSR = 'V', LDVSR >= N.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,4*N).
* For good performance, LWORK must generally be larger.
* To compute the optimal value of LWORK, call ILAENV to get
* blocksizes (for SGEQRF, SORMQR, and SORGQR.) Then compute:
* NB -- MAX of the blocksizes for SGEQRF, SORMQR, and SORGQR
* The optimal LWORK is 2*N + N*(NB+1).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1,...,N:
* The QZ iteration failed. (A,B) are not in Schur
* form, but ALPHAR(j), ALPHAI(j), and BETA(j) should
* be correct for j=INFO+1,...,N.
* > N: errors that usually indicate LAPACK problems:
* =N+1: error return from SGGBAL
* =N+2: error return from SGEQRF
* =N+3: error return from SORMQR
* =N+4: error return from SORGQR
* =N+5: error return from SGGHRD
* =N+6: error return from SHGEQZ (other than failed
* iteration)
* =N+7: error return from SGGBAK (computing VSL)
* =N+8: error return from SGGBAK (computing VSR)
* =N+9: error return from SLASCL (various places)
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobvsl
* @param jobvsr
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param alphar
* @param _alphar_offset
* @param alphai
* @param _alphai_offset
* @param beta
* @param _beta_offset
* @param vsl
* @param _vsl_offset
* @param ldvsl
* @param vsr
* @param _vsr_offset
* @param ldvsr
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sgegs(java.lang.String jobvsl, java.lang.String jobvsr, int n, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, float[] alphar, int _alphar_offset, float[] alphai, int _alphai_offset, float[] beta, int _beta_offset, float[] vsl, int _vsl_offset, int ldvsl, float[] vsr, int _vsr_offset, int ldvsr, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* This routine is deprecated and has been replaced by routine SGGEV.
*
* SGEGV computes the eigenvalues and, optionally, the left and/or right
* eigenvectors of a real matrix pair (A,B).
* Given two square matrices A and B,
* the generalized nonsymmetric eigenvalue problem (GNEP) is to find the
* eigenvalues lambda and corresponding (non-zero) eigenvectors x such
* that
*
* A*x = lambda*B*x.
*
* An alternate form is to find the eigenvalues mu and corresponding
* eigenvectors y such that
*
* mu*A*y = B*y.
*
* These two forms are equivalent with mu = 1/lambda and x = y if
* neither lambda nor mu is zero. In order to deal with the case that
* lambda or mu is zero or small, two values alpha and beta are returned
* for each eigenvalue, such that lambda = alpha/beta and
* mu = beta/alpha.
*
* The vectors x and y in the above equations are right eigenvectors of
* the matrix pair (A,B). Vectors u and v satisfying
*
* u**H*A = lambda*u**H*B or mu*v**H*A = v**H*B
*
* are left eigenvectors of (A,B).
*
* Note: this routine performs "full balancing" on A and B -- see
* "Further Details", below.
*
* Arguments
* =========
*
* JOBVL (input) CHARACTER*1
* = 'N': do not compute the left generalized eigenvectors;
* = 'V': compute the left generalized eigenvectors (returned
* in VL).
*
* JOBVR (input) CHARACTER*1
* = 'N': do not compute the right generalized eigenvectors;
* = 'V': compute the right generalized eigenvectors (returned
* in VR).
*
* N (input) INTEGER
* The order of the matrices A, B, VL, and VR. N >= 0.
*
* A (input/output) REAL array, dimension (LDA, N)
* On entry, the matrix A.
* If JOBVL = 'V' or JOBVR = 'V', then on exit A
* contains the real Schur form of A from the generalized Schur
* factorization of the pair (A,B) after balancing.
* If no eigenvectors were computed, then only the diagonal
* blocks from the Schur form will be correct. See SGGHRD and
* SHGEQZ for details.
*
* LDA (input) INTEGER
* The leading dimension of A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension (LDB, N)
* On entry, the matrix B.
* If JOBVL = 'V' or JOBVR = 'V', then on exit B contains the
* upper triangular matrix obtained from B in the generalized
* Schur factorization of the pair (A,B) after balancing.
* If no eigenvectors were computed, then only those elements of
* B corresponding to the diagonal blocks from the Schur form of
* A will be correct. See SGGHRD and SHGEQZ for details.
*
* LDB (input) INTEGER
* The leading dimension of B. LDB >= max(1,N).
*
* ALPHAR (output) REAL array, dimension (N)
* The real parts of each scalar alpha defining an eigenvalue of
* GNEP.
*
* ALPHAI (output) REAL array, dimension (N)
* The imaginary parts of each scalar alpha defining an
* eigenvalue of GNEP. If ALPHAI(j) is zero, then the j-th
* eigenvalue is real; if positive, then the j-th and
* (j+1)-st eigenvalues are a complex conjugate pair, with
* ALPHAI(j+1) = -ALPHAI(j).
*
* BETA (output) REAL array, dimension (N)
* The scalars beta that define the eigenvalues of GNEP.
*
* Together, the quantities alpha = (ALPHAR(j),ALPHAI(j)) and
* beta = BETA(j) represent the j-th eigenvalue of the matrix
* pair (A,B), in one of the forms lambda = alpha/beta or
* mu = beta/alpha. Since either lambda or mu may overflow,
* they should not, in general, be computed.
*
* VL (output) REAL array, dimension (LDVL,N)
* If JOBVL = 'V', the left eigenvectors u(j) are stored
* in the columns of VL, in the same order as their eigenvalues.
* If the j-th eigenvalue is real, then u(j) = VL(:,j).
* If the j-th and (j+1)-st eigenvalues form a complex conjugate
* pair, then
* u(j) = VL(:,j) + i*VL(:,j+1)
* and
* u(j+1) = VL(:,j) - i*VL(:,j+1).
*
* Each eigenvector is scaled so that its largest component has
* abs(real part) + abs(imag. part) = 1, except for eigenvectors
* corresponding to an eigenvalue with alpha = beta = 0, which
* are set to zero.
* Not referenced if JOBVL = 'N'.
*
* LDVL (input) INTEGER
* The leading dimension of the matrix VL. LDVL >= 1, and
* if JOBVL = 'V', LDVL >= N.
*
* VR (output) REAL array, dimension (LDVR,N)
* If JOBVR = 'V', the right eigenvectors x(j) are stored
* in the columns of VR, in the same order as their eigenvalues.
* If the j-th eigenvalue is real, then x(j) = VR(:,j).
* If the j-th and (j+1)-st eigenvalues form a complex conjugate
* pair, then
* x(j) = VR(:,j) + i*VR(:,j+1)
* and
* x(j+1) = VR(:,j) - i*VR(:,j+1).
*
* Each eigenvector is scaled so that its largest component has
* abs(real part) + abs(imag. part) = 1, except for eigenvalues
* corresponding to an eigenvalue with alpha = beta = 0, which
* are set to zero.
* Not referenced if JOBVR = 'N'.
*
* LDVR (input) INTEGER
* The leading dimension of the matrix VR. LDVR >= 1, and
* if JOBVR = 'V', LDVR >= N.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,8*N).
* For good performance, LWORK must generally be larger.
* To compute the optimal value of LWORK, call ILAENV to get
* blocksizes (for SGEQRF, SORMQR, and SORGQR.) Then compute:
* NB -- MAX of the blocksizes for SGEQRF, SORMQR, and SORGQR;
* The optimal LWORK is:
* 2*N + MAX( 6*N, N*(NB+1) ).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1,...,N:
* The QZ iteration failed. No eigenvectors have been
* calculated, but ALPHAR(j), ALPHAI(j), and BETA(j)
* should be correct for j=INFO+1,...,N.
* > N: errors that usually indicate LAPACK problems:
* =N+1: error return from SGGBAL
* =N+2: error return from SGEQRF
* =N+3: error return from SORMQR
* =N+4: error return from SORGQR
* =N+5: error return from SGGHRD
* =N+6: error return from SHGEQZ (other than failed
* iteration)
* =N+7: error return from STGEVC
* =N+8: error return from SGGBAK (computing VL)
* =N+9: error return from SGGBAK (computing VR)
* =N+10: error return from SLASCL (various calls)
*
* Further Details
* ===============
*
* Balancing
* ---------
*
* This driver calls SGGBAL to both permute and scale rows and columns
* of A and B. The permutations PL and PR are chosen so that PL*A*PR
* and PL*B*R will be upper triangular except for the diagonal blocks
* A(i:j,i:j) and B(i:j,i:j), with i and j as close together as
* possible. The diagonal scaling matrices DL and DR are chosen so
* that the pair DL*PL*A*PR*DR, DL*PL*B*PR*DR have elements close to
* one (except for the elements that start out zero.)
*
* After the eigenvalues and eigenvectors of the balanced matrices
* have been computed, SGGBAK transforms the eigenvectors back to what
* they would have been (in perfect arithmetic) if they had not been
* balanced.
*
* Contents of A and B on Exit
* -------- -- - --- - -- ----
*
* If any eigenvectors are computed (either JOBVL='V' or JOBVR='V' or
* both), then on exit the arrays A and B will contain the real Schur
* form[*] of the "balanced" versions of A and B. If no eigenvectors
* are computed, then only the diagonal blocks will be correct.
*
* [*] See SHGEQZ, SGEGS, or read the book "Matrix Computations",
* by Golub & van Loan, pub. by Johns Hopkins U. Press.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobvl
* @param jobvr
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param alphar
* @param alphai
* @param beta
* @param vl
* @param ldvl
* @param vr
* @param ldvr
* @param work
* @param lwork
* @param info
*
*/
abstract public void sgegv(java.lang.String jobvl, java.lang.String jobvr, int n, float[] a, int lda, float[] b, int ldb, float[] alphar, float[] alphai, float[] beta, float[] vl, int ldvl, float[] vr, int ldvr, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* This routine is deprecated and has been replaced by routine SGGEV.
*
* SGEGV computes the eigenvalues and, optionally, the left and/or right
* eigenvectors of a real matrix pair (A,B).
* Given two square matrices A and B,
* the generalized nonsymmetric eigenvalue problem (GNEP) is to find the
* eigenvalues lambda and corresponding (non-zero) eigenvectors x such
* that
*
* A*x = lambda*B*x.
*
* An alternate form is to find the eigenvalues mu and corresponding
* eigenvectors y such that
*
* mu*A*y = B*y.
*
* These two forms are equivalent with mu = 1/lambda and x = y if
* neither lambda nor mu is zero. In order to deal with the case that
* lambda or mu is zero or small, two values alpha and beta are returned
* for each eigenvalue, such that lambda = alpha/beta and
* mu = beta/alpha.
*
* The vectors x and y in the above equations are right eigenvectors of
* the matrix pair (A,B). Vectors u and v satisfying
*
* u**H*A = lambda*u**H*B or mu*v**H*A = v**H*B
*
* are left eigenvectors of (A,B).
*
* Note: this routine performs "full balancing" on A and B -- see
* "Further Details", below.
*
* Arguments
* =========
*
* JOBVL (input) CHARACTER*1
* = 'N': do not compute the left generalized eigenvectors;
* = 'V': compute the left generalized eigenvectors (returned
* in VL).
*
* JOBVR (input) CHARACTER*1
* = 'N': do not compute the right generalized eigenvectors;
* = 'V': compute the right generalized eigenvectors (returned
* in VR).
*
* N (input) INTEGER
* The order of the matrices A, B, VL, and VR. N >= 0.
*
* A (input/output) REAL array, dimension (LDA, N)
* On entry, the matrix A.
* If JOBVL = 'V' or JOBVR = 'V', then on exit A
* contains the real Schur form of A from the generalized Schur
* factorization of the pair (A,B) after balancing.
* If no eigenvectors were computed, then only the diagonal
* blocks from the Schur form will be correct. See SGGHRD and
* SHGEQZ for details.
*
* LDA (input) INTEGER
* The leading dimension of A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension (LDB, N)
* On entry, the matrix B.
* If JOBVL = 'V' or JOBVR = 'V', then on exit B contains the
* upper triangular matrix obtained from B in the generalized
* Schur factorization of the pair (A,B) after balancing.
* If no eigenvectors were computed, then only those elements of
* B corresponding to the diagonal blocks from the Schur form of
* A will be correct. See SGGHRD and SHGEQZ for details.
*
* LDB (input) INTEGER
* The leading dimension of B. LDB >= max(1,N).
*
* ALPHAR (output) REAL array, dimension (N)
* The real parts of each scalar alpha defining an eigenvalue of
* GNEP.
*
* ALPHAI (output) REAL array, dimension (N)
* The imaginary parts of each scalar alpha defining an
* eigenvalue of GNEP. If ALPHAI(j) is zero, then the j-th
* eigenvalue is real; if positive, then the j-th and
* (j+1)-st eigenvalues are a complex conjugate pair, with
* ALPHAI(j+1) = -ALPHAI(j).
*
* BETA (output) REAL array, dimension (N)
* The scalars beta that define the eigenvalues of GNEP.
*
* Together, the quantities alpha = (ALPHAR(j),ALPHAI(j)) and
* beta = BETA(j) represent the j-th eigenvalue of the matrix
* pair (A,B), in one of the forms lambda = alpha/beta or
* mu = beta/alpha. Since either lambda or mu may overflow,
* they should not, in general, be computed.
*
* VL (output) REAL array, dimension (LDVL,N)
* If JOBVL = 'V', the left eigenvectors u(j) are stored
* in the columns of VL, in the same order as their eigenvalues.
* If the j-th eigenvalue is real, then u(j) = VL(:,j).
* If the j-th and (j+1)-st eigenvalues form a complex conjugate
* pair, then
* u(j) = VL(:,j) + i*VL(:,j+1)
* and
* u(j+1) = VL(:,j) - i*VL(:,j+1).
*
* Each eigenvector is scaled so that its largest component has
* abs(real part) + abs(imag. part) = 1, except for eigenvectors
* corresponding to an eigenvalue with alpha = beta = 0, which
* are set to zero.
* Not referenced if JOBVL = 'N'.
*
* LDVL (input) INTEGER
* The leading dimension of the matrix VL. LDVL >= 1, and
* if JOBVL = 'V', LDVL >= N.
*
* VR (output) REAL array, dimension (LDVR,N)
* If JOBVR = 'V', the right eigenvectors x(j) are stored
* in the columns of VR, in the same order as their eigenvalues.
* If the j-th eigenvalue is real, then x(j) = VR(:,j).
* If the j-th and (j+1)-st eigenvalues form a complex conjugate
* pair, then
* x(j) = VR(:,j) + i*VR(:,j+1)
* and
* x(j+1) = VR(:,j) - i*VR(:,j+1).
*
* Each eigenvector is scaled so that its largest component has
* abs(real part) + abs(imag. part) = 1, except for eigenvalues
* corresponding to an eigenvalue with alpha = beta = 0, which
* are set to zero.
* Not referenced if JOBVR = 'N'.
*
* LDVR (input) INTEGER
* The leading dimension of the matrix VR. LDVR >= 1, and
* if JOBVR = 'V', LDVR >= N.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,8*N).
* For good performance, LWORK must generally be larger.
* To compute the optimal value of LWORK, call ILAENV to get
* blocksizes (for SGEQRF, SORMQR, and SORGQR.) Then compute:
* NB -- MAX of the blocksizes for SGEQRF, SORMQR, and SORGQR;
* The optimal LWORK is:
* 2*N + MAX( 6*N, N*(NB+1) ).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1,...,N:
* The QZ iteration failed. No eigenvectors have been
* calculated, but ALPHAR(j), ALPHAI(j), and BETA(j)
* should be correct for j=INFO+1,...,N.
* > N: errors that usually indicate LAPACK problems:
* =N+1: error return from SGGBAL
* =N+2: error return from SGEQRF
* =N+3: error return from SORMQR
* =N+4: error return from SORGQR
* =N+5: error return from SGGHRD
* =N+6: error return from SHGEQZ (other than failed
* iteration)
* =N+7: error return from STGEVC
* =N+8: error return from SGGBAK (computing VL)
* =N+9: error return from SGGBAK (computing VR)
* =N+10: error return from SLASCL (various calls)
*
* Further Details
* ===============
*
* Balancing
* ---------
*
* This driver calls SGGBAL to both permute and scale rows and columns
* of A and B. The permutations PL and PR are chosen so that PL*A*PR
* and PL*B*R will be upper triangular except for the diagonal blocks
* A(i:j,i:j) and B(i:j,i:j), with i and j as close together as
* possible. The diagonal scaling matrices DL and DR are chosen so
* that the pair DL*PL*A*PR*DR, DL*PL*B*PR*DR have elements close to
* one (except for the elements that start out zero.)
*
* After the eigenvalues and eigenvectors of the balanced matrices
* have been computed, SGGBAK transforms the eigenvectors back to what
* they would have been (in perfect arithmetic) if they had not been
* balanced.
*
* Contents of A and B on Exit
* -------- -- - --- - -- ----
*
* If any eigenvectors are computed (either JOBVL='V' or JOBVR='V' or
* both), then on exit the arrays A and B will contain the real Schur
* form[*] of the "balanced" versions of A and B. If no eigenvectors
* are computed, then only the diagonal blocks will be correct.
*
* [*] See SHGEQZ, SGEGS, or read the book "Matrix Computations",
* by Golub & van Loan, pub. by Johns Hopkins U. Press.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobvl
* @param jobvr
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param alphar
* @param _alphar_offset
* @param alphai
* @param _alphai_offset
* @param beta
* @param _beta_offset
* @param vl
* @param _vl_offset
* @param ldvl
* @param vr
* @param _vr_offset
* @param ldvr
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sgegv(java.lang.String jobvl, java.lang.String jobvr, int n, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, float[] alphar, int _alphar_offset, float[] alphai, int _alphai_offset, float[] beta, int _beta_offset, float[] vl, int _vl_offset, int ldvl, float[] vr, int _vr_offset, int ldvr, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGEHD2 reduces a real general matrix A to upper Hessenberg form H by
* an orthogonal similarity transformation: Q' * A * Q = H .
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* It is assumed that A is already upper triangular in rows
* and columns 1:ILO-1 and IHI+1:N. ILO and IHI are normally
* set by a previous call to SGEBAL; otherwise they should be
* set to 1 and N respectively. See Further Details.
* 1 <= ILO <= IHI <= max(1,N).
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the n by n general matrix to be reduced.
* On exit, the upper triangle and the first subdiagonal of A
* are overwritten with the upper Hessenberg matrix H, and the
* elements below the first subdiagonal, with the array TAU,
* represent the orthogonal matrix Q as a product of elementary
* reflectors. See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* TAU (output) REAL array, dimension (N-1)
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace) REAL array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of (ihi-ilo) elementary
* reflectors
*
* Q = H(ilo) H(ilo+1) . . . H(ihi-1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i) = 0, v(i+1) = 1 and v(ihi+1:n) = 0; v(i+2:ihi) is stored on
* exit in A(i+2:ihi,i), and tau in TAU(i).
*
* The contents of A are illustrated by the following example, with
* n = 7, ilo = 2 and ihi = 6:
*
* on entry, on exit,
*
* ( a a a a a a a ) ( a a h h h h a )
* ( a a a a a a ) ( a h h h h a )
* ( a a a a a a ) ( h h h h h h )
* ( a a a a a a ) ( v2 h h h h h )
* ( a a a a a a ) ( v2 v3 h h h h )
* ( a a a a a a ) ( v2 v3 v4 h h h )
* ( a ) ( a )
*
* where a denotes an element of the original matrix A, h denotes a
* modified element of the upper Hessenberg matrix H, and vi denotes an
* element of the vector defining H(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param ilo
* @param ihi
* @param a
* @param lda
* @param tau
* @param work
* @param info
*
*/
abstract public void sgehd2(int n, int ilo, int ihi, float[] a, int lda, float[] tau, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGEHD2 reduces a real general matrix A to upper Hessenberg form H by
* an orthogonal similarity transformation: Q' * A * Q = H .
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* It is assumed that A is already upper triangular in rows
* and columns 1:ILO-1 and IHI+1:N. ILO and IHI are normally
* set by a previous call to SGEBAL; otherwise they should be
* set to 1 and N respectively. See Further Details.
* 1 <= ILO <= IHI <= max(1,N).
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the n by n general matrix to be reduced.
* On exit, the upper triangle and the first subdiagonal of A
* are overwritten with the upper Hessenberg matrix H, and the
* elements below the first subdiagonal, with the array TAU,
* represent the orthogonal matrix Q as a product of elementary
* reflectors. See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* TAU (output) REAL array, dimension (N-1)
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace) REAL array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of (ihi-ilo) elementary
* reflectors
*
* Q = H(ilo) H(ilo+1) . . . H(ihi-1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i) = 0, v(i+1) = 1 and v(ihi+1:n) = 0; v(i+2:ihi) is stored on
* exit in A(i+2:ihi,i), and tau in TAU(i).
*
* The contents of A are illustrated by the following example, with
* n = 7, ilo = 2 and ihi = 6:
*
* on entry, on exit,
*
* ( a a a a a a a ) ( a a h h h h a )
* ( a a a a a a ) ( a h h h h a )
* ( a a a a a a ) ( h h h h h h )
* ( a a a a a a ) ( v2 h h h h h )
* ( a a a a a a ) ( v2 v3 h h h h )
* ( a a a a a a ) ( v2 v3 v4 h h h )
* ( a ) ( a )
*
* where a denotes an element of the original matrix A, h denotes a
* modified element of the upper Hessenberg matrix H, and vi denotes an
* element of the vector defining H(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param ilo
* @param ihi
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void sgehd2(int n, int ilo, int ihi, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGEHRD reduces a real general matrix A to upper Hessenberg form H by
* an orthogonal similarity transformation: Q' * A * Q = H .
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* It is assumed that A is already upper triangular in rows
* and columns 1:ILO-1 and IHI+1:N. ILO and IHI are normally
* set by a previous call to SGEBAL; otherwise they should be
* set to 1 and N respectively. See Further Details.
* 1 <= ILO <= IHI <= N, if N > 0; ILO=1 and IHI=0, if N=0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the N-by-N general matrix to be reduced.
* On exit, the upper triangle and the first subdiagonal of A
* are overwritten with the upper Hessenberg matrix H, and the
* elements below the first subdiagonal, with the array TAU,
* represent the orthogonal matrix Q as a product of elementary
* reflectors. See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* TAU (output) REAL array, dimension (N-1)
* The scalar factors of the elementary reflectors (see Further
* Details). Elements 1:ILO-1 and IHI:N-1 of TAU are set to
* zero.
*
* WORK (workspace/output) REAL array, dimension (LWORK)
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of the array WORK. LWORK >= max(1,N).
* For optimum performance LWORK >= N*NB, where NB is the
* optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of (ihi-ilo) elementary
* reflectors
*
* Q = H(ilo) H(ilo+1) . . . H(ihi-1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i) = 0, v(i+1) = 1 and v(ihi+1:n) = 0; v(i+2:ihi) is stored on
* exit in A(i+2:ihi,i), and tau in TAU(i).
*
* The contents of A are illustrated by the following example, with
* n = 7, ilo = 2 and ihi = 6:
*
* on entry, on exit,
*
* ( a a a a a a a ) ( a a h h h h a )
* ( a a a a a a ) ( a h h h h a )
* ( a a a a a a ) ( h h h h h h )
* ( a a a a a a ) ( v2 h h h h h )
* ( a a a a a a ) ( v2 v3 h h h h )
* ( a a a a a a ) ( v2 v3 v4 h h h )
* ( a ) ( a )
*
* where a denotes an element of the original matrix A, h denotes a
* modified element of the upper Hessenberg matrix H, and vi denotes an
* element of the vector defining H(i).
*
* This file is a slight modification of LAPACK-3.0's SGEHRD
* subroutine incorporating improvements proposed by Quintana-Orti and
* Van de Geijn (2005).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param ilo
* @param ihi
* @param a
* @param lda
* @param tau
* @param work
* @param lwork
* @param info
*
*/
abstract public void sgehrd(int n, int ilo, int ihi, float[] a, int lda, float[] tau, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGEHRD reduces a real general matrix A to upper Hessenberg form H by
* an orthogonal similarity transformation: Q' * A * Q = H .
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* It is assumed that A is already upper triangular in rows
* and columns 1:ILO-1 and IHI+1:N. ILO and IHI are normally
* set by a previous call to SGEBAL; otherwise they should be
* set to 1 and N respectively. See Further Details.
* 1 <= ILO <= IHI <= N, if N > 0; ILO=1 and IHI=0, if N=0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the N-by-N general matrix to be reduced.
* On exit, the upper triangle and the first subdiagonal of A
* are overwritten with the upper Hessenberg matrix H, and the
* elements below the first subdiagonal, with the array TAU,
* represent the orthogonal matrix Q as a product of elementary
* reflectors. See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* TAU (output) REAL array, dimension (N-1)
* The scalar factors of the elementary reflectors (see Further
* Details). Elements 1:ILO-1 and IHI:N-1 of TAU are set to
* zero.
*
* WORK (workspace/output) REAL array, dimension (LWORK)
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of the array WORK. LWORK >= max(1,N).
* For optimum performance LWORK >= N*NB, where NB is the
* optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of (ihi-ilo) elementary
* reflectors
*
* Q = H(ilo) H(ilo+1) . . . H(ihi-1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i) = 0, v(i+1) = 1 and v(ihi+1:n) = 0; v(i+2:ihi) is stored on
* exit in A(i+2:ihi,i), and tau in TAU(i).
*
* The contents of A are illustrated by the following example, with
* n = 7, ilo = 2 and ihi = 6:
*
* on entry, on exit,
*
* ( a a a a a a a ) ( a a h h h h a )
* ( a a a a a a ) ( a h h h h a )
* ( a a a a a a ) ( h h h h h h )
* ( a a a a a a ) ( v2 h h h h h )
* ( a a a a a a ) ( v2 v3 h h h h )
* ( a a a a a a ) ( v2 v3 v4 h h h )
* ( a ) ( a )
*
* where a denotes an element of the original matrix A, h denotes a
* modified element of the upper Hessenberg matrix H, and vi denotes an
* element of the vector defining H(i).
*
* This file is a slight modification of LAPACK-3.0's SGEHRD
* subroutine incorporating improvements proposed by Quintana-Orti and
* Van de Geijn (2005).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param ilo
* @param ihi
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sgehrd(int n, int ilo, int ihi, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGELQ2 computes an LQ factorization of a real m by n matrix A:
* A = L * Q.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the m by n matrix A.
* On exit, the elements on and below the diagonal of the array
* contain the m by min(m,n) lower trapezoidal matrix L (L is
* lower triangular if m <= n); the elements above the diagonal,
* with the array TAU, represent the orthogonal matrix Q as a
* product of elementary reflectors (see Further Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) REAL array, dimension (min(M,N))
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace) REAL array, dimension (M)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(k) . . . H(2) H(1), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i-1) = 0 and v(i) = 1; v(i+1:n) is stored on exit in A(i,i+1:n),
* and tau in TAU(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param tau
* @param work
* @param info
*
*/
abstract public void sgelq2(int m, int n, float[] a, int lda, float[] tau, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGELQ2 computes an LQ factorization of a real m by n matrix A:
* A = L * Q.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the m by n matrix A.
* On exit, the elements on and below the diagonal of the array
* contain the m by min(m,n) lower trapezoidal matrix L (L is
* lower triangular if m <= n); the elements above the diagonal,
* with the array TAU, represent the orthogonal matrix Q as a
* product of elementary reflectors (see Further Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) REAL array, dimension (min(M,N))
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace) REAL array, dimension (M)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(k) . . . H(2) H(1), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i-1) = 0 and v(i) = 1; v(i+1:n) is stored on exit in A(i,i+1:n),
* and tau in TAU(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void sgelq2(int m, int n, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGELQF computes an LQ factorization of a real M-by-N matrix A:
* A = L * Q.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, the elements on and below the diagonal of the array
* contain the m-by-min(m,n) lower trapezoidal matrix L (L is
* lower triangular if m <= n); the elements above the diagonal,
* with the array TAU, represent the orthogonal matrix Q as a
* product of elementary reflectors (see Further Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) REAL array, dimension (min(M,N))
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,M).
* For optimum performance LWORK >= M*NB, where NB is the
* optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(k) . . . H(2) H(1), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i-1) = 0 and v(i) = 1; v(i+1:n) is stored on exit in A(i,i+1:n),
* and tau in TAU(i).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param tau
* @param work
* @param lwork
* @param info
*
*/
abstract public void sgelqf(int m, int n, float[] a, int lda, float[] tau, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGELQF computes an LQ factorization of a real M-by-N matrix A:
* A = L * Q.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, the elements on and below the diagonal of the array
* contain the m-by-min(m,n) lower trapezoidal matrix L (L is
* lower triangular if m <= n); the elements above the diagonal,
* with the array TAU, represent the orthogonal matrix Q as a
* product of elementary reflectors (see Further Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) REAL array, dimension (min(M,N))
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,M).
* For optimum performance LWORK >= M*NB, where NB is the
* optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(k) . . . H(2) H(1), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i-1) = 0 and v(i) = 1; v(i+1:n) is stored on exit in A(i,i+1:n),
* and tau in TAU(i).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sgelqf(int m, int n, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGELS solves overdetermined or underdetermined real linear systems
* involving an M-by-N matrix A, or its transpose, using a QR or LQ
* factorization of A. It is assumed that A has full rank.
*
* The following options are provided:
*
* 1. If TRANS = 'N' and m >= n: find the least squares solution of
* an overdetermined system, i.e., solve the least squares problem
* minimize || B - A*X ||.
*
* 2. If TRANS = 'N' and m < n: find the minimum norm solution of
* an underdetermined system A * X = B.
*
* 3. If TRANS = 'T' and m >= n: find the minimum norm solution of
* an undetermined system A**T * X = B.
*
* 4. If TRANS = 'T' and m < n: find the least squares solution of
* an overdetermined system, i.e., solve the least squares problem
* minimize || B - A**T * X ||.
*
* Several right hand side vectors b and solution vectors x can be
* handled in a single call; they are stored as the columns of the
* M-by-NRHS right hand side matrix B and the N-by-NRHS solution
* matrix X.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* = 'N': the linear system involves A;
* = 'T': the linear system involves A**T.
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of
* columns of the matrices B and X. NRHS >=0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit,
* if M >= N, A is overwritten by details of its QR
* factorization as returned by SGEQRF;
* if M < N, A is overwritten by details of its LQ
* factorization as returned by SGELQF.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the matrix B of right hand side vectors, stored
* columnwise; B is M-by-NRHS if TRANS = 'N', or N-by-NRHS
* if TRANS = 'T'.
* On exit, if INFO = 0, B is overwritten by the solution
* vectors, stored columnwise:
* if TRANS = 'N' and m >= n, rows 1 to n of B contain the least
* squares solution vectors; the residual sum of squares for the
* solution in each column is given by the sum of squares of
* elements N+1 to M in that column;
* if TRANS = 'N' and m < n, rows 1 to N of B contain the
* minimum norm solution vectors;
* if TRANS = 'T' and m >= n, rows 1 to M of B contain the
* minimum norm solution vectors;
* if TRANS = 'T' and m < n, rows 1 to M of B contain the
* least squares solution vectors; the residual sum of squares
* for the solution in each column is given by the sum of
* squares of elements M+1 to N in that column.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= MAX(1,M,N).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* LWORK >= max( 1, MN + max( MN, NRHS ) ).
* For optimal performance,
* LWORK >= max( 1, MN + max( MN, NRHS )*NB ).
* where MN = min(M,N) and NB is the optimum block size.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the i-th diagonal element of the
* triangular factor of A is zero, so that A does not have
* full rank; the least squares solution could not be
* computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param trans
* @param m
* @param n
* @param nrhs
* @param a
* @param lda
* @param b
* @param ldb
* @param work
* @param lwork
* @param info
*
*/
abstract public void sgels(java.lang.String trans, int m, int n, int nrhs, float[] a, int lda, float[] b, int ldb, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGELS solves overdetermined or underdetermined real linear systems
* involving an M-by-N matrix A, or its transpose, using a QR or LQ
* factorization of A. It is assumed that A has full rank.
*
* The following options are provided:
*
* 1. If TRANS = 'N' and m >= n: find the least squares solution of
* an overdetermined system, i.e., solve the least squares problem
* minimize || B - A*X ||.
*
* 2. If TRANS = 'N' and m < n: find the minimum norm solution of
* an underdetermined system A * X = B.
*
* 3. If TRANS = 'T' and m >= n: find the minimum norm solution of
* an undetermined system A**T * X = B.
*
* 4. If TRANS = 'T' and m < n: find the least squares solution of
* an overdetermined system, i.e., solve the least squares problem
* minimize || B - A**T * X ||.
*
* Several right hand side vectors b and solution vectors x can be
* handled in a single call; they are stored as the columns of the
* M-by-NRHS right hand side matrix B and the N-by-NRHS solution
* matrix X.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* = 'N': the linear system involves A;
* = 'T': the linear system involves A**T.
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of
* columns of the matrices B and X. NRHS >=0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit,
* if M >= N, A is overwritten by details of its QR
* factorization as returned by SGEQRF;
* if M < N, A is overwritten by details of its LQ
* factorization as returned by SGELQF.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the matrix B of right hand side vectors, stored
* columnwise; B is M-by-NRHS if TRANS = 'N', or N-by-NRHS
* if TRANS = 'T'.
* On exit, if INFO = 0, B is overwritten by the solution
* vectors, stored columnwise:
* if TRANS = 'N' and m >= n, rows 1 to n of B contain the least
* squares solution vectors; the residual sum of squares for the
* solution in each column is given by the sum of squares of
* elements N+1 to M in that column;
* if TRANS = 'N' and m < n, rows 1 to N of B contain the
* minimum norm solution vectors;
* if TRANS = 'T' and m >= n, rows 1 to M of B contain the
* minimum norm solution vectors;
* if TRANS = 'T' and m < n, rows 1 to M of B contain the
* least squares solution vectors; the residual sum of squares
* for the solution in each column is given by the sum of
* squares of elements M+1 to N in that column.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= MAX(1,M,N).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* LWORK >= max( 1, MN + max( MN, NRHS ) ).
* For optimal performance,
* LWORK >= max( 1, MN + max( MN, NRHS )*NB ).
* where MN = min(M,N) and NB is the optimum block size.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the i-th diagonal element of the
* triangular factor of A is zero, so that A does not have
* full rank; the least squares solution could not be
* computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param trans
* @param m
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sgels(java.lang.String trans, int m, int n, int nrhs, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGELSD computes the minimum-norm solution to a real linear least
* squares problem:
* minimize 2-norm(| b - A*x |)
* using the singular value decomposition (SVD) of A. A is an M-by-N
* matrix which may be rank-deficient.
*
* Several right hand side vectors b and solution vectors x can be
* handled in a single call; they are stored as the columns of the
* M-by-NRHS right hand side matrix B and the N-by-NRHS solution
* matrix X.
*
* The problem is solved in three steps:
* (1) Reduce the coefficient matrix A to bidiagonal form with
* Householder transformations, reducing the original problem
* into a "bidiagonal least squares problem" (BLS)
* (2) Solve the BLS using a divide and conquer approach.
* (3) Apply back all the Householder tranformations to solve
* the original least squares problem.
*
* The effective rank of A is determined by treating as zero those
* singular values which are less than RCOND times the largest singular
* value.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of A. M >= 0.
*
* N (input) INTEGER
* The number of columns of A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, A has been destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the M-by-NRHS right hand side matrix B.
* On exit, B is overwritten by the N-by-NRHS solution
* matrix X. If m >= n and RANK = n, the residual
* sum-of-squares for the solution in the i-th column is given
* by the sum of squares of elements n+1:m in that column.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,max(M,N)).
*
* S (output) REAL array, dimension (min(M,N))
* The singular values of A in decreasing order.
* The condition number of A in the 2-norm = S(1)/S(min(m,n)).
*
* RCOND (input) REAL
* RCOND is used to determine the effective rank of A.
* Singular values S(i) <= RCOND*S(1) are treated as zero.
* If RCOND < 0, machine precision is used instead.
*
* RANK (output) INTEGER
* The effective rank of A, i.e., the number of singular values
* which are greater than RCOND*S(1).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK must be at least 1.
* The exact minimum amount of workspace needed depends on M,
* N and NRHS. As long as LWORK is at least
* 12*N + 2*N*SMLSIZ + 8*N*NLVL + N*NRHS + (SMLSIZ+1)**2,
* if M is greater than or equal to N or
* 12*M + 2*M*SMLSIZ + 8*M*NLVL + M*NRHS + (SMLSIZ+1)**2,
* if M is less than N, the code will execute correctly.
* SMLSIZ is returned by ILAENV and is equal to the maximum
* size of the subproblems at the bottom of the computation
* tree (usually about 25), and
* NLVL = MAX( 0, INT( LOG_2( MIN( M,N )/(SMLSIZ+1) ) ) + 1 )
* For good performance, LWORK should generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the array WORK and the
* minimum size of the array IWORK, and returns these values as
* the first entries of the WORK and IWORK arrays, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (MAX(1,LIWORK))
* LIWORK >= max(1, 3*MINMN*NLVL + 11*MINMN),
* where MINMN = MIN( M,N ).
* On exit, if INFO = 0, IWORK(1) returns the minimum LIWORK.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: the algorithm for computing the SVD failed to converge;
* if INFO = i, i off-diagonal elements of an intermediate
* bidiagonal form did not converge to zero.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Ren-Cang Li, Computer Science Division, University of
* California at Berkeley, USA
* Osni Marques, LBNL/NERSC, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param nrhs
* @param a
* @param lda
* @param b
* @param ldb
* @param s
* @param rcond
* @param rank
* @param work
* @param lwork
* @param iwork
* @param info
*
*/
abstract public void sgelsd(int m, int n, int nrhs, float[] a, int lda, float[] b, int ldb, float[] s, float rcond, org.netlib.util.intW rank, float[] work, int lwork, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGELSD computes the minimum-norm solution to a real linear least
* squares problem:
* minimize 2-norm(| b - A*x |)
* using the singular value decomposition (SVD) of A. A is an M-by-N
* matrix which may be rank-deficient.
*
* Several right hand side vectors b and solution vectors x can be
* handled in a single call; they are stored as the columns of the
* M-by-NRHS right hand side matrix B and the N-by-NRHS solution
* matrix X.
*
* The problem is solved in three steps:
* (1) Reduce the coefficient matrix A to bidiagonal form with
* Householder transformations, reducing the original problem
* into a "bidiagonal least squares problem" (BLS)
* (2) Solve the BLS using a divide and conquer approach.
* (3) Apply back all the Householder tranformations to solve
* the original least squares problem.
*
* The effective rank of A is determined by treating as zero those
* singular values which are less than RCOND times the largest singular
* value.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of A. M >= 0.
*
* N (input) INTEGER
* The number of columns of A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, A has been destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the M-by-NRHS right hand side matrix B.
* On exit, B is overwritten by the N-by-NRHS solution
* matrix X. If m >= n and RANK = n, the residual
* sum-of-squares for the solution in the i-th column is given
* by the sum of squares of elements n+1:m in that column.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,max(M,N)).
*
* S (output) REAL array, dimension (min(M,N))
* The singular values of A in decreasing order.
* The condition number of A in the 2-norm = S(1)/S(min(m,n)).
*
* RCOND (input) REAL
* RCOND is used to determine the effective rank of A.
* Singular values S(i) <= RCOND*S(1) are treated as zero.
* If RCOND < 0, machine precision is used instead.
*
* RANK (output) INTEGER
* The effective rank of A, i.e., the number of singular values
* which are greater than RCOND*S(1).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK must be at least 1.
* The exact minimum amount of workspace needed depends on M,
* N and NRHS. As long as LWORK is at least
* 12*N + 2*N*SMLSIZ + 8*N*NLVL + N*NRHS + (SMLSIZ+1)**2,
* if M is greater than or equal to N or
* 12*M + 2*M*SMLSIZ + 8*M*NLVL + M*NRHS + (SMLSIZ+1)**2,
* if M is less than N, the code will execute correctly.
* SMLSIZ is returned by ILAENV and is equal to the maximum
* size of the subproblems at the bottom of the computation
* tree (usually about 25), and
* NLVL = MAX( 0, INT( LOG_2( MIN( M,N )/(SMLSIZ+1) ) ) + 1 )
* For good performance, LWORK should generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the array WORK and the
* minimum size of the array IWORK, and returns these values as
* the first entries of the WORK and IWORK arrays, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (MAX(1,LIWORK))
* LIWORK >= max(1, 3*MINMN*NLVL + 11*MINMN),
* where MINMN = MIN( M,N ).
* On exit, if INFO = 0, IWORK(1) returns the minimum LIWORK.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: the algorithm for computing the SVD failed to converge;
* if INFO = i, i off-diagonal elements of an intermediate
* bidiagonal form did not converge to zero.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Ren-Cang Li, Computer Science Division, University of
* California at Berkeley, USA
* Osni Marques, LBNL/NERSC, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param s
* @param _s_offset
* @param rcond
* @param rank
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void sgelsd(int m, int n, int nrhs, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, float[] s, int _s_offset, float rcond, org.netlib.util.intW rank, float[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGELSS computes the minimum norm solution to a real linear least
* squares problem:
*
* Minimize 2-norm(| b - A*x |).
*
* using the singular value decomposition (SVD) of A. A is an M-by-N
* matrix which may be rank-deficient.
*
* Several right hand side vectors b and solution vectors x can be
* handled in a single call; they are stored as the columns of the
* M-by-NRHS right hand side matrix B and the N-by-NRHS solution matrix
* X.
*
* The effective rank of A is determined by treating as zero those
* singular values which are less than RCOND times the largest singular
* value.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, the first min(m,n) rows of A are overwritten with
* its right singular vectors, stored rowwise.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the M-by-NRHS right hand side matrix B.
* On exit, B is overwritten by the N-by-NRHS solution
* matrix X. If m >= n and RANK = n, the residual
* sum-of-squares for the solution in the i-th column is given
* by the sum of squares of elements n+1:m in that column.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,max(M,N)).
*
* S (output) REAL array, dimension (min(M,N))
* The singular values of A in decreasing order.
* The condition number of A in the 2-norm = S(1)/S(min(m,n)).
*
* RCOND (input) REAL
* RCOND is used to determine the effective rank of A.
* Singular values S(i) <= RCOND*S(1) are treated as zero.
* If RCOND < 0, machine precision is used instead.
*
* RANK (output) INTEGER
* The effective rank of A, i.e., the number of singular values
* which are greater than RCOND*S(1).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= 1, and also:
* LWORK >= 3*min(M,N) + max( 2*min(M,N), max(M,N), NRHS )
* For good performance, LWORK should generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: the algorithm for computing the SVD failed to converge;
* if INFO = i, i off-diagonal elements of an intermediate
* bidiagonal form did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param nrhs
* @param a
* @param lda
* @param b
* @param ldb
* @param s
* @param rcond
* @param rank
* @param work
* @param lwork
* @param info
*
*/
abstract public void sgelss(int m, int n, int nrhs, float[] a, int lda, float[] b, int ldb, float[] s, float rcond, org.netlib.util.intW rank, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGELSS computes the minimum norm solution to a real linear least
* squares problem:
*
* Minimize 2-norm(| b - A*x |).
*
* using the singular value decomposition (SVD) of A. A is an M-by-N
* matrix which may be rank-deficient.
*
* Several right hand side vectors b and solution vectors x can be
* handled in a single call; they are stored as the columns of the
* M-by-NRHS right hand side matrix B and the N-by-NRHS solution matrix
* X.
*
* The effective rank of A is determined by treating as zero those
* singular values which are less than RCOND times the largest singular
* value.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, the first min(m,n) rows of A are overwritten with
* its right singular vectors, stored rowwise.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the M-by-NRHS right hand side matrix B.
* On exit, B is overwritten by the N-by-NRHS solution
* matrix X. If m >= n and RANK = n, the residual
* sum-of-squares for the solution in the i-th column is given
* by the sum of squares of elements n+1:m in that column.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,max(M,N)).
*
* S (output) REAL array, dimension (min(M,N))
* The singular values of A in decreasing order.
* The condition number of A in the 2-norm = S(1)/S(min(m,n)).
*
* RCOND (input) REAL
* RCOND is used to determine the effective rank of A.
* Singular values S(i) <= RCOND*S(1) are treated as zero.
* If RCOND < 0, machine precision is used instead.
*
* RANK (output) INTEGER
* The effective rank of A, i.e., the number of singular values
* which are greater than RCOND*S(1).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= 1, and also:
* LWORK >= 3*min(M,N) + max( 2*min(M,N), max(M,N), NRHS )
* For good performance, LWORK should generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: the algorithm for computing the SVD failed to converge;
* if INFO = i, i off-diagonal elements of an intermediate
* bidiagonal form did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param s
* @param _s_offset
* @param rcond
* @param rank
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sgelss(int m, int n, int nrhs, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, float[] s, int _s_offset, float rcond, org.netlib.util.intW rank, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* This routine is deprecated and has been replaced by routine SGELSY.
*
* SGELSX computes the minimum-norm solution to a real linear least
* squares problem:
* minimize || A * X - B ||
* using a complete orthogonal factorization of A. A is an M-by-N
* matrix which may be rank-deficient.
*
* Several right hand side vectors b and solution vectors x can be
* handled in a single call; they are stored as the columns of the
* M-by-NRHS right hand side matrix B and the N-by-NRHS solution
* matrix X.
*
* The routine first computes a QR factorization with column pivoting:
* A * P = Q * [ R11 R12 ]
* [ 0 R22 ]
* with R11 defined as the largest leading submatrix whose estimated
* condition number is less than 1/RCOND. The order of R11, RANK,
* is the effective rank of A.
*
* Then, R22 is considered to be negligible, and R12 is annihilated
* by orthogonal transformations from the right, arriving at the
* complete orthogonal factorization:
* A * P = Q * [ T11 0 ] * Z
* [ 0 0 ]
* The minimum-norm solution is then
* X = P * Z' [ inv(T11)*Q1'*B ]
* [ 0 ]
* where Q1 consists of the first RANK columns of Q.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of
* columns of matrices B and X. NRHS >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, A has been overwritten by details of its
* complete orthogonal factorization.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the M-by-NRHS right hand side matrix B.
* On exit, the N-by-NRHS solution matrix X.
* If m >= n and RANK = n, the residual sum-of-squares for
* the solution in the i-th column is given by the sum of
* squares of elements N+1:M in that column.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,M,N).
*
* JPVT (input/output) INTEGER array, dimension (N)
* On entry, if JPVT(i) .ne. 0, the i-th column of A is an
* initial column, otherwise it is a free column. Before
* the QR factorization of A, all initial columns are
* permuted to the leading positions; only the remaining
* free columns are moved as a result of column pivoting
* during the factorization.
* On exit, if JPVT(i) = k, then the i-th column of A*P
* was the k-th column of A.
*
* RCOND (input) REAL
* RCOND is used to determine the effective rank of A, which
* is defined as the order of the largest leading triangular
* submatrix R11 in the QR factorization with pivoting of A,
* whose estimated condition number < 1/RCOND.
*
* RANK (output) INTEGER
* The effective rank of A, i.e., the order of the submatrix
* R11. This is the same as the order of the submatrix T11
* in the complete orthogonal factorization of A.
*
* WORK (workspace) REAL array, dimension
* (max( min(M,N)+3*N, 2*min(M,N)+NRHS )),
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param nrhs
* @param a
* @param lda
* @param b
* @param ldb
* @param jpvt
* @param rcond
* @param rank
* @param work
* @param info
*
*/
abstract public void sgelsx(int m, int n, int nrhs, float[] a, int lda, float[] b, int ldb, int[] jpvt, float rcond, org.netlib.util.intW rank, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* This routine is deprecated and has been replaced by routine SGELSY.
*
* SGELSX computes the minimum-norm solution to a real linear least
* squares problem:
* minimize || A * X - B ||
* using a complete orthogonal factorization of A. A is an M-by-N
* matrix which may be rank-deficient.
*
* Several right hand side vectors b and solution vectors x can be
* handled in a single call; they are stored as the columns of the
* M-by-NRHS right hand side matrix B and the N-by-NRHS solution
* matrix X.
*
* The routine first computes a QR factorization with column pivoting:
* A * P = Q * [ R11 R12 ]
* [ 0 R22 ]
* with R11 defined as the largest leading submatrix whose estimated
* condition number is less than 1/RCOND. The order of R11, RANK,
* is the effective rank of A.
*
* Then, R22 is considered to be negligible, and R12 is annihilated
* by orthogonal transformations from the right, arriving at the
* complete orthogonal factorization:
* A * P = Q * [ T11 0 ] * Z
* [ 0 0 ]
* The minimum-norm solution is then
* X = P * Z' [ inv(T11)*Q1'*B ]
* [ 0 ]
* where Q1 consists of the first RANK columns of Q.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of
* columns of matrices B and X. NRHS >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, A has been overwritten by details of its
* complete orthogonal factorization.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the M-by-NRHS right hand side matrix B.
* On exit, the N-by-NRHS solution matrix X.
* If m >= n and RANK = n, the residual sum-of-squares for
* the solution in the i-th column is given by the sum of
* squares of elements N+1:M in that column.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,M,N).
*
* JPVT (input/output) INTEGER array, dimension (N)
* On entry, if JPVT(i) .ne. 0, the i-th column of A is an
* initial column, otherwise it is a free column. Before
* the QR factorization of A, all initial columns are
* permuted to the leading positions; only the remaining
* free columns are moved as a result of column pivoting
* during the factorization.
* On exit, if JPVT(i) = k, then the i-th column of A*P
* was the k-th column of A.
*
* RCOND (input) REAL
* RCOND is used to determine the effective rank of A, which
* is defined as the order of the largest leading triangular
* submatrix R11 in the QR factorization with pivoting of A,
* whose estimated condition number < 1/RCOND.
*
* RANK (output) INTEGER
* The effective rank of A, i.e., the order of the submatrix
* R11. This is the same as the order of the submatrix T11
* in the complete orthogonal factorization of A.
*
* WORK (workspace) REAL array, dimension
* (max( min(M,N)+3*N, 2*min(M,N)+NRHS )),
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param jpvt
* @param _jpvt_offset
* @param rcond
* @param rank
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void sgelsx(int m, int n, int nrhs, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, int[] jpvt, int _jpvt_offset, float rcond, org.netlib.util.intW rank, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGELSY computes the minimum-norm solution to a real linear least
* squares problem:
* minimize || A * X - B ||
* using a complete orthogonal factorization of A. A is an M-by-N
* matrix which may be rank-deficient.
*
* Several right hand side vectors b and solution vectors x can be
* handled in a single call; they are stored as the columns of the
* M-by-NRHS right hand side matrix B and the N-by-NRHS solution
* matrix X.
*
* The routine first computes a QR factorization with column pivoting:
* A * P = Q * [ R11 R12 ]
* [ 0 R22 ]
* with R11 defined as the largest leading submatrix whose estimated
* condition number is less than 1/RCOND. The order of R11, RANK,
* is the effective rank of A.
*
* Then, R22 is considered to be negligible, and R12 is annihilated
* by orthogonal transformations from the right, arriving at the
* complete orthogonal factorization:
* A * P = Q * [ T11 0 ] * Z
* [ 0 0 ]
* The minimum-norm solution is then
* X = P * Z' [ inv(T11)*Q1'*B ]
* [ 0 ]
* where Q1 consists of the first RANK columns of Q.
*
* This routine is basically identical to the original xGELSX except
* three differences:
* o The call to the subroutine xGEQPF has been substituted by the
* the call to the subroutine xGEQP3. This subroutine is a Blas-3
* version of the QR factorization with column pivoting.
* o Matrix B (the right hand side) is updated with Blas-3.
* o The permutation of matrix B (the right hand side) is faster and
* more simple.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of
* columns of matrices B and X. NRHS >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, A has been overwritten by details of its
* complete orthogonal factorization.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the M-by-NRHS right hand side matrix B.
* On exit, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,M,N).
*
* JPVT (input/output) INTEGER array, dimension (N)
* On entry, if JPVT(i) .ne. 0, the i-th column of A is permuted
* to the front of AP, otherwise column i is a free column.
* On exit, if JPVT(i) = k, then the i-th column of AP
* was the k-th column of A.
*
* RCOND (input) REAL
* RCOND is used to determine the effective rank of A, which
* is defined as the order of the largest leading triangular
* submatrix R11 in the QR factorization with pivoting of A,
* whose estimated condition number < 1/RCOND.
*
* RANK (output) INTEGER
* The effective rank of A, i.e., the order of the submatrix
* R11. This is the same as the order of the submatrix T11
* in the complete orthogonal factorization of A.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* The unblocked strategy requires that:
* LWORK >= MAX( MN+3*N+1, 2*MN+NRHS ),
* where MN = min( M, N ).
* The block algorithm requires that:
* LWORK >= MAX( MN+2*N+NB*(N+1), 2*MN+NB*NRHS ),
* where NB is an upper bound on the blocksize returned
* by ILAENV for the routines SGEQP3, STZRZF, STZRQF, SORMQR,
* and SORMRZ.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: If INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* Based on contributions by
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
* E. Quintana-Orti, Depto. de Informatica, Universidad Jaime I, Spain
* G. Quintana-Orti, Depto. de Informatica, Universidad Jaime I, Spain
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param nrhs
* @param a
* @param lda
* @param b
* @param ldb
* @param jpvt
* @param rcond
* @param rank
* @param work
* @param lwork
* @param info
*
*/
abstract public void sgelsy(int m, int n, int nrhs, float[] a, int lda, float[] b, int ldb, int[] jpvt, float rcond, org.netlib.util.intW rank, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGELSY computes the minimum-norm solution to a real linear least
* squares problem:
* minimize || A * X - B ||
* using a complete orthogonal factorization of A. A is an M-by-N
* matrix which may be rank-deficient.
*
* Several right hand side vectors b and solution vectors x can be
* handled in a single call; they are stored as the columns of the
* M-by-NRHS right hand side matrix B and the N-by-NRHS solution
* matrix X.
*
* The routine first computes a QR factorization with column pivoting:
* A * P = Q * [ R11 R12 ]
* [ 0 R22 ]
* with R11 defined as the largest leading submatrix whose estimated
* condition number is less than 1/RCOND. The order of R11, RANK,
* is the effective rank of A.
*
* Then, R22 is considered to be negligible, and R12 is annihilated
* by orthogonal transformations from the right, arriving at the
* complete orthogonal factorization:
* A * P = Q * [ T11 0 ] * Z
* [ 0 0 ]
* The minimum-norm solution is then
* X = P * Z' [ inv(T11)*Q1'*B ]
* [ 0 ]
* where Q1 consists of the first RANK columns of Q.
*
* This routine is basically identical to the original xGELSX except
* three differences:
* o The call to the subroutine xGEQPF has been substituted by the
* the call to the subroutine xGEQP3. This subroutine is a Blas-3
* version of the QR factorization with column pivoting.
* o Matrix B (the right hand side) is updated with Blas-3.
* o The permutation of matrix B (the right hand side) is faster and
* more simple.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of
* columns of matrices B and X. NRHS >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, A has been overwritten by details of its
* complete orthogonal factorization.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the M-by-NRHS right hand side matrix B.
* On exit, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,M,N).
*
* JPVT (input/output) INTEGER array, dimension (N)
* On entry, if JPVT(i) .ne. 0, the i-th column of A is permuted
* to the front of AP, otherwise column i is a free column.
* On exit, if JPVT(i) = k, then the i-th column of AP
* was the k-th column of A.
*
* RCOND (input) REAL
* RCOND is used to determine the effective rank of A, which
* is defined as the order of the largest leading triangular
* submatrix R11 in the QR factorization with pivoting of A,
* whose estimated condition number < 1/RCOND.
*
* RANK (output) INTEGER
* The effective rank of A, i.e., the order of the submatrix
* R11. This is the same as the order of the submatrix T11
* in the complete orthogonal factorization of A.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* The unblocked strategy requires that:
* LWORK >= MAX( MN+3*N+1, 2*MN+NRHS ),
* where MN = min( M, N ).
* The block algorithm requires that:
* LWORK >= MAX( MN+2*N+NB*(N+1), 2*MN+NB*NRHS ),
* where NB is an upper bound on the blocksize returned
* by ILAENV for the routines SGEQP3, STZRZF, STZRQF, SORMQR,
* and SORMRZ.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: If INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* Based on contributions by
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
* E. Quintana-Orti, Depto. de Informatica, Universidad Jaime I, Spain
* G. Quintana-Orti, Depto. de Informatica, Universidad Jaime I, Spain
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param jpvt
* @param _jpvt_offset
* @param rcond
* @param rank
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sgelsy(int m, int n, int nrhs, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, int[] jpvt, int _jpvt_offset, float rcond, org.netlib.util.intW rank, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGEQL2 computes a QL factorization of a real m by n matrix A:
* A = Q * L.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the m by n matrix A.
* On exit, if m >= n, the lower triangle of the subarray
* A(m-n+1:m,1:n) contains the n by n lower triangular matrix L;
* if m <= n, the elements on and below the (n-m)-th
* superdiagonal contain the m by n lower trapezoidal matrix L;
* the remaining elements, with the array TAU, represent the
* orthogonal matrix Q as a product of elementary reflectors
* (see Further Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) REAL array, dimension (min(M,N))
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace) REAL array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(k) . . . H(2) H(1), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(m-k+i+1:m) = 0 and v(m-k+i) = 1; v(1:m-k+i-1) is stored on exit in
* A(1:m-k+i-1,n-k+i), and tau in TAU(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param tau
* @param work
* @param info
*
*/
abstract public void sgeql2(int m, int n, float[] a, int lda, float[] tau, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGEQL2 computes a QL factorization of a real m by n matrix A:
* A = Q * L.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the m by n matrix A.
* On exit, if m >= n, the lower triangle of the subarray
* A(m-n+1:m,1:n) contains the n by n lower triangular matrix L;
* if m <= n, the elements on and below the (n-m)-th
* superdiagonal contain the m by n lower trapezoidal matrix L;
* the remaining elements, with the array TAU, represent the
* orthogonal matrix Q as a product of elementary reflectors
* (see Further Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) REAL array, dimension (min(M,N))
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace) REAL array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(k) . . . H(2) H(1), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(m-k+i+1:m) = 0 and v(m-k+i) = 1; v(1:m-k+i-1) is stored on exit in
* A(1:m-k+i-1,n-k+i), and tau in TAU(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void sgeql2(int m, int n, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGEQLF computes a QL factorization of a real M-by-N matrix A:
* A = Q * L.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit,
* if m >= n, the lower triangle of the subarray
* A(m-n+1:m,1:n) contains the N-by-N lower triangular matrix L;
* if m <= n, the elements on and below the (n-m)-th
* superdiagonal contain the M-by-N lower trapezoidal matrix L;
* the remaining elements, with the array TAU, represent the
* orthogonal matrix Q as a product of elementary reflectors
* (see Further Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) REAL array, dimension (min(M,N))
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N).
* For optimum performance LWORK >= N*NB, where NB is the
* optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(k) . . . H(2) H(1), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(m-k+i+1:m) = 0 and v(m-k+i) = 1; v(1:m-k+i-1) is stored on exit in
* A(1:m-k+i-1,n-k+i), and tau in TAU(i).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param tau
* @param work
* @param lwork
* @param info
*
*/
abstract public void sgeqlf(int m, int n, float[] a, int lda, float[] tau, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGEQLF computes a QL factorization of a real M-by-N matrix A:
* A = Q * L.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit,
* if m >= n, the lower triangle of the subarray
* A(m-n+1:m,1:n) contains the N-by-N lower triangular matrix L;
* if m <= n, the elements on and below the (n-m)-th
* superdiagonal contain the M-by-N lower trapezoidal matrix L;
* the remaining elements, with the array TAU, represent the
* orthogonal matrix Q as a product of elementary reflectors
* (see Further Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) REAL array, dimension (min(M,N))
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N).
* For optimum performance LWORK >= N*NB, where NB is the
* optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(k) . . . H(2) H(1), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(m-k+i+1:m) = 0 and v(m-k+i) = 1; v(1:m-k+i-1) is stored on exit in
* A(1:m-k+i-1,n-k+i), and tau in TAU(i).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sgeqlf(int m, int n, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGEQP3 computes a QR factorization with column pivoting of a
* matrix A: A*P = Q*R using Level 3 BLAS.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, the upper triangle of the array contains the
* min(M,N)-by-N upper trapezoidal matrix R; the elements below
* the diagonal, together with the array TAU, represent the
* orthogonal matrix Q as a product of min(M,N) elementary
* reflectors.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* JPVT (input/output) INTEGER array, dimension (N)
* On entry, if JPVT(J).ne.0, the J-th column of A is permuted
* to the front of A*P (a leading column); if JPVT(J)=0,
* the J-th column of A is a free column.
* On exit, if JPVT(J)=K, then the J-th column of A*P was the
* the K-th column of A.
*
* TAU (output) REAL array, dimension (min(M,N))
* The scalar factors of the elementary reflectors.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO=0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= 3*N+1.
* For optimal performance LWORK >= 2*N+( N+1 )*NB, where NB
* is the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(1) H(2) . . . H(k), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real/complex scalar, and v is a real/complex vector
* with v(1:i-1) = 0 and v(i) = 1; v(i+1:m) is stored on exit in
* A(i+1:m,i), and tau in TAU(i).
*
* Based on contributions by
* G. Quintana-Orti, Depto. de Informatica, Universidad Jaime I, Spain
* X. Sun, Computer Science Dept., Duke University, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param jpvt
* @param tau
* @param work
* @param lwork
* @param info
*
*/
abstract public void sgeqp3(int m, int n, float[] a, int lda, int[] jpvt, float[] tau, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGEQP3 computes a QR factorization with column pivoting of a
* matrix A: A*P = Q*R using Level 3 BLAS.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, the upper triangle of the array contains the
* min(M,N)-by-N upper trapezoidal matrix R; the elements below
* the diagonal, together with the array TAU, represent the
* orthogonal matrix Q as a product of min(M,N) elementary
* reflectors.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* JPVT (input/output) INTEGER array, dimension (N)
* On entry, if JPVT(J).ne.0, the J-th column of A is permuted
* to the front of A*P (a leading column); if JPVT(J)=0,
* the J-th column of A is a free column.
* On exit, if JPVT(J)=K, then the J-th column of A*P was the
* the K-th column of A.
*
* TAU (output) REAL array, dimension (min(M,N))
* The scalar factors of the elementary reflectors.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO=0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= 3*N+1.
* For optimal performance LWORK >= 2*N+( N+1 )*NB, where NB
* is the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(1) H(2) . . . H(k), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real/complex scalar, and v is a real/complex vector
* with v(1:i-1) = 0 and v(i) = 1; v(i+1:m) is stored on exit in
* A(i+1:m,i), and tau in TAU(i).
*
* Based on contributions by
* G. Quintana-Orti, Depto. de Informatica, Universidad Jaime I, Spain
* X. Sun, Computer Science Dept., Duke University, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param jpvt
* @param _jpvt_offset
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sgeqp3(int m, int n, float[] a, int _a_offset, int lda, int[] jpvt, int _jpvt_offset, float[] tau, int _tau_offset, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* This routine is deprecated and has been replaced by routine SGEQP3.
*
* SGEQPF computes a QR factorization with column pivoting of a
* real M-by-N matrix A: A*P = Q*R.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, the upper triangle of the array contains the
* min(M,N)-by-N upper triangular matrix R; the elements
* below the diagonal, together with the array TAU,
* represent the orthogonal matrix Q as a product of
* min(m,n) elementary reflectors.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* JPVT (input/output) INTEGER array, dimension (N)
* On entry, if JPVT(i) .ne. 0, the i-th column of A is permuted
* to the front of A*P (a leading column); if JPVT(i) = 0,
* the i-th column of A is a free column.
* On exit, if JPVT(i) = k, then the i-th column of A*P
* was the k-th column of A.
*
* TAU (output) REAL array, dimension (min(M,N))
* The scalar factors of the elementary reflectors.
*
* WORK (workspace) REAL array, dimension (3*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(1) H(2) . . . H(n)
*
* Each H(i) has the form
*
* H = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i-1) = 0 and v(i) = 1; v(i+1:m) is stored on exit in A(i+1:m,i).
*
* The matrix P is represented in jpvt as follows: If
* jpvt(j) = i
* then the jth column of P is the ith canonical unit vector.
*
* Partial column norm updating strategy modified by
* Z. Drmac and Z. Bujanovic, Dept. of Mathematics,
* University of Zagreb, Croatia.
* June 2006.
* For more details see LAPACK Working Note 176.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param jpvt
* @param tau
* @param work
* @param info
*
*/
abstract public void sgeqpf(int m, int n, float[] a, int lda, int[] jpvt, float[] tau, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* This routine is deprecated and has been replaced by routine SGEQP3.
*
* SGEQPF computes a QR factorization with column pivoting of a
* real M-by-N matrix A: A*P = Q*R.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, the upper triangle of the array contains the
* min(M,N)-by-N upper triangular matrix R; the elements
* below the diagonal, together with the array TAU,
* represent the orthogonal matrix Q as a product of
* min(m,n) elementary reflectors.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* JPVT (input/output) INTEGER array, dimension (N)
* On entry, if JPVT(i) .ne. 0, the i-th column of A is permuted
* to the front of A*P (a leading column); if JPVT(i) = 0,
* the i-th column of A is a free column.
* On exit, if JPVT(i) = k, then the i-th column of A*P
* was the k-th column of A.
*
* TAU (output) REAL array, dimension (min(M,N))
* The scalar factors of the elementary reflectors.
*
* WORK (workspace) REAL array, dimension (3*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(1) H(2) . . . H(n)
*
* Each H(i) has the form
*
* H = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i-1) = 0 and v(i) = 1; v(i+1:m) is stored on exit in A(i+1:m,i).
*
* The matrix P is represented in jpvt as follows: If
* jpvt(j) = i
* then the jth column of P is the ith canonical unit vector.
*
* Partial column norm updating strategy modified by
* Z. Drmac and Z. Bujanovic, Dept. of Mathematics,
* University of Zagreb, Croatia.
* June 2006.
* For more details see LAPACK Working Note 176.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param jpvt
* @param _jpvt_offset
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void sgeqpf(int m, int n, float[] a, int _a_offset, int lda, int[] jpvt, int _jpvt_offset, float[] tau, int _tau_offset, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGEQR2 computes a QR factorization of a real m by n matrix A:
* A = Q * R.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the m by n matrix A.
* On exit, the elements on and above the diagonal of the array
* contain the min(m,n) by n upper trapezoidal matrix R (R is
* upper triangular if m >= n); the elements below the diagonal,
* with the array TAU, represent the orthogonal matrix Q as a
* product of elementary reflectors (see Further Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) REAL array, dimension (min(M,N))
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace) REAL array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(1) H(2) . . . H(k), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i-1) = 0 and v(i) = 1; v(i+1:m) is stored on exit in A(i+1:m,i),
* and tau in TAU(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param tau
* @param work
* @param info
*
*/
abstract public void sgeqr2(int m, int n, float[] a, int lda, float[] tau, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGEQR2 computes a QR factorization of a real m by n matrix A:
* A = Q * R.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the m by n matrix A.
* On exit, the elements on and above the diagonal of the array
* contain the min(m,n) by n upper trapezoidal matrix R (R is
* upper triangular if m >= n); the elements below the diagonal,
* with the array TAU, represent the orthogonal matrix Q as a
* product of elementary reflectors (see Further Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) REAL array, dimension (min(M,N))
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace) REAL array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(1) H(2) . . . H(k), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i-1) = 0 and v(i) = 1; v(i+1:m) is stored on exit in A(i+1:m,i),
* and tau in TAU(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void sgeqr2(int m, int n, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGEQRF computes a QR factorization of a real M-by-N matrix A:
* A = Q * R.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, the elements on and above the diagonal of the array
* contain the min(M,N)-by-N upper trapezoidal matrix R (R is
* upper triangular if m >= n); the elements below the diagonal,
* with the array TAU, represent the orthogonal matrix Q as a
* product of min(m,n) elementary reflectors (see Further
* Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) REAL array, dimension (min(M,N))
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N).
* For optimum performance LWORK >= N*NB, where NB is
* the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(1) H(2) . . . H(k), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i-1) = 0 and v(i) = 1; v(i+1:m) is stored on exit in A(i+1:m,i),
* and tau in TAU(i).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param tau
* @param work
* @param lwork
* @param info
*
*/
abstract public void sgeqrf(int m, int n, float[] a, int lda, float[] tau, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGEQRF computes a QR factorization of a real M-by-N matrix A:
* A = Q * R.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, the elements on and above the diagonal of the array
* contain the min(M,N)-by-N upper trapezoidal matrix R (R is
* upper triangular if m >= n); the elements below the diagonal,
* with the array TAU, represent the orthogonal matrix Q as a
* product of min(m,n) elementary reflectors (see Further
* Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) REAL array, dimension (min(M,N))
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N).
* For optimum performance LWORK >= N*NB, where NB is
* the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(1) H(2) . . . H(k), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i-1) = 0 and v(i) = 1; v(i+1:m) is stored on exit in A(i+1:m,i),
* and tau in TAU(i).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sgeqrf(int m, int n, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGERFS improves the computed solution to a system of linear
* equations and provides error bounds and backward error estimates for
* the solution.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The original N-by-N matrix A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* AF (input) REAL array, dimension (LDAF,N)
* The factors L and U from the factorization A = P*L*U
* as computed by SGETRF.
*
* LDAF (input) INTEGER
* The leading dimension of the array AF. LDAF >= max(1,N).
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices from SGETRF; for 1<=i<=N, row i of the
* matrix was interchanged with row IPIV(i).
*
* B (input) REAL array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) REAL array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by SGETRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param trans
* @param n
* @param nrhs
* @param a
* @param lda
* @param af
* @param ldaf
* @param ipiv
* @param b
* @param ldb
* @param x
* @param ldx
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void sgerfs(java.lang.String trans, int n, int nrhs, float[] a, int lda, float[] af, int ldaf, int[] ipiv, float[] b, int ldb, float[] x, int ldx, float[] ferr, float[] berr, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGERFS improves the computed solution to a system of linear
* equations and provides error bounds and backward error estimates for
* the solution.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The original N-by-N matrix A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* AF (input) REAL array, dimension (LDAF,N)
* The factors L and U from the factorization A = P*L*U
* as computed by SGETRF.
*
* LDAF (input) INTEGER
* The leading dimension of the array AF. LDAF >= max(1,N).
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices from SGETRF; for 1<=i<=N, row i of the
* matrix was interchanged with row IPIV(i).
*
* B (input) REAL array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) REAL array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by SGETRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param trans
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param af
* @param _af_offset
* @param ldaf
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void sgerfs(java.lang.String trans, int n, int nrhs, float[] a, int _a_offset, int lda, float[] af, int _af_offset, int ldaf, int[] ipiv, int _ipiv_offset, float[] b, int _b_offset, int ldb, float[] x, int _x_offset, int ldx, float[] ferr, int _ferr_offset, float[] berr, int _berr_offset, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGERQ2 computes an RQ factorization of a real m by n matrix A:
* A = R * Q.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the m by n matrix A.
* On exit, if m <= n, the upper triangle of the subarray
* A(1:m,n-m+1:n) contains the m by m upper triangular matrix R;
* if m >= n, the elements on and above the (m-n)-th subdiagonal
* contain the m by n upper trapezoidal matrix R; the remaining
* elements, with the array TAU, represent the orthogonal matrix
* Q as a product of elementary reflectors (see Further
* Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) REAL array, dimension (min(M,N))
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace) REAL array, dimension (M)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(1) H(2) . . . H(k), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(n-k+i+1:n) = 0 and v(n-k+i) = 1; v(1:n-k+i-1) is stored on exit in
* A(m-k+i,1:n-k+i-1), and tau in TAU(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param tau
* @param work
* @param info
*
*/
abstract public void sgerq2(int m, int n, float[] a, int lda, float[] tau, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGERQ2 computes an RQ factorization of a real m by n matrix A:
* A = R * Q.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the m by n matrix A.
* On exit, if m <= n, the upper triangle of the subarray
* A(1:m,n-m+1:n) contains the m by m upper triangular matrix R;
* if m >= n, the elements on and above the (m-n)-th subdiagonal
* contain the m by n upper trapezoidal matrix R; the remaining
* elements, with the array TAU, represent the orthogonal matrix
* Q as a product of elementary reflectors (see Further
* Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) REAL array, dimension (min(M,N))
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace) REAL array, dimension (M)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(1) H(2) . . . H(k), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(n-k+i+1:n) = 0 and v(n-k+i) = 1; v(1:n-k+i-1) is stored on exit in
* A(m-k+i,1:n-k+i-1), and tau in TAU(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void sgerq2(int m, int n, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGERQF computes an RQ factorization of a real M-by-N matrix A:
* A = R * Q.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit,
* if m <= n, the upper triangle of the subarray
* A(1:m,n-m+1:n) contains the M-by-M upper triangular matrix R;
* if m >= n, the elements on and above the (m-n)-th subdiagonal
* contain the M-by-N upper trapezoidal matrix R;
* the remaining elements, with the array TAU, represent the
* orthogonal matrix Q as a product of min(m,n) elementary
* reflectors (see Further Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) REAL array, dimension (min(M,N))
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,M).
* For optimum performance LWORK >= M*NB, where NB is
* the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(1) H(2) . . . H(k), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(n-k+i+1:n) = 0 and v(n-k+i) = 1; v(1:n-k+i-1) is stored on exit in
* A(m-k+i,1:n-k+i-1), and tau in TAU(i).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param tau
* @param work
* @param lwork
* @param info
*
*/
abstract public void sgerqf(int m, int n, float[] a, int lda, float[] tau, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGERQF computes an RQ factorization of a real M-by-N matrix A:
* A = R * Q.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit,
* if m <= n, the upper triangle of the subarray
* A(1:m,n-m+1:n) contains the M-by-M upper triangular matrix R;
* if m >= n, the elements on and above the (m-n)-th subdiagonal
* contain the M-by-N upper trapezoidal matrix R;
* the remaining elements, with the array TAU, represent the
* orthogonal matrix Q as a product of min(m,n) elementary
* reflectors (see Further Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) REAL array, dimension (min(M,N))
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,M).
* For optimum performance LWORK >= M*NB, where NB is
* the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(1) H(2) . . . H(k), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(n-k+i+1:n) = 0 and v(n-k+i) = 1; v(1:n-k+i-1) is stored on exit in
* A(m-k+i,1:n-k+i-1), and tau in TAU(i).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sgerqf(int m, int n, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGESC2 solves a system of linear equations
*
* A * X = scale* RHS
*
* with a general N-by-N matrix A using the LU factorization with
* complete pivoting computed by SGETC2.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A.
*
* A (input) REAL array, dimension (LDA,N)
* On entry, the LU part of the factorization of the n-by-n
* matrix A computed by SGETC2: A = P * L * U * Q
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1, N).
*
* RHS (input/output) REAL array, dimension (N).
* On entry, the right hand side vector b.
* On exit, the solution vector X.
*
* IPIV (input) INTEGER array, dimension (N).
* The pivot indices; for 1 <= i <= N, row i of the
* matrix has been interchanged with row IPIV(i).
*
* JPIV (input) INTEGER array, dimension (N).
* The pivot indices; for 1 <= j <= N, column j of the
* matrix has been interchanged with column JPIV(j).
*
* SCALE (output) REAL
* On exit, SCALE contains the scale factor. SCALE is chosen
* 0 <= SCALE <= 1 to prevent owerflow in the solution.
*
* Further Details
* ===============
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param a
* @param lda
* @param rhs
* @param ipiv
* @param jpiv
* @param scale
*
*/
abstract public void sgesc2(int n, float[] a, int lda, float[] rhs, int[] ipiv, int[] jpiv, org.netlib.util.floatW scale);
/**
*
* ..
*
* Purpose
* =======
*
* SGESC2 solves a system of linear equations
*
* A * X = scale* RHS
*
* with a general N-by-N matrix A using the LU factorization with
* complete pivoting computed by SGETC2.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A.
*
* A (input) REAL array, dimension (LDA,N)
* On entry, the LU part of the factorization of the n-by-n
* matrix A computed by SGETC2: A = P * L * U * Q
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1, N).
*
* RHS (input/output) REAL array, dimension (N).
* On entry, the right hand side vector b.
* On exit, the solution vector X.
*
* IPIV (input) INTEGER array, dimension (N).
* The pivot indices; for 1 <= i <= N, row i of the
* matrix has been interchanged with row IPIV(i).
*
* JPIV (input) INTEGER array, dimension (N).
* The pivot indices; for 1 <= j <= N, column j of the
* matrix has been interchanged with column JPIV(j).
*
* SCALE (output) REAL
* On exit, SCALE contains the scale factor. SCALE is chosen
* 0 <= SCALE <= 1 to prevent owerflow in the solution.
*
* Further Details
* ===============
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param a
* @param _a_offset
* @param lda
* @param rhs
* @param _rhs_offset
* @param ipiv
* @param _ipiv_offset
* @param jpiv
* @param _jpiv_offset
* @param scale
*
*/
abstract public void sgesc2(int n, float[] a, int _a_offset, int lda, float[] rhs, int _rhs_offset, int[] ipiv, int _ipiv_offset, int[] jpiv, int _jpiv_offset, org.netlib.util.floatW scale);
/**
*
* ..
*
* Purpose
* =======
*
* SGESDD computes the singular value decomposition (SVD) of a real
* M-by-N matrix A, optionally computing the left and right singular
* vectors. If singular vectors are desired, it uses a
* divide-and-conquer algorithm.
*
* The SVD is written
*
* A = U * SIGMA * transpose(V)
*
* where SIGMA is an M-by-N matrix which is zero except for its
* min(m,n) diagonal elements, U is an M-by-M orthogonal matrix, and
* V is an N-by-N orthogonal matrix. The diagonal elements of SIGMA
* are the singular values of A; they are real and non-negative, and
* are returned in descending order. The first min(m,n) columns of
* U and V are the left and right singular vectors of A.
*
* Note that the routine returns VT = V**T, not V.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* Specifies options for computing all or part of the matrix U:
* = 'A': all M columns of U and all N rows of V**T are
* returned in the arrays U and VT;
* = 'S': the first min(M,N) columns of U and the first
* min(M,N) rows of V**T are returned in the arrays U
* and VT;
* = 'O': If M >= N, the first N columns of U are overwritten
* on the array A and all rows of V**T are returned in
* the array VT;
* otherwise, all columns of U are returned in the
* array U and the first M rows of V**T are overwritten
* in the array A;
* = 'N': no columns of U or rows of V**T are computed.
*
* M (input) INTEGER
* The number of rows of the input matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the input matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit,
* if JOBZ = 'O', A is overwritten with the first N columns
* of U (the left singular vectors, stored
* columnwise) if M >= N;
* A is overwritten with the first M rows
* of V**T (the right singular vectors, stored
* rowwise) otherwise.
* if JOBZ .ne. 'O', the contents of A are destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* S (output) REAL array, dimension (min(M,N))
* The singular values of A, sorted so that S(i) >= S(i+1).
*
* U (output) REAL array, dimension (LDU,UCOL)
* UCOL = M if JOBZ = 'A' or JOBZ = 'O' and M < N;
* UCOL = min(M,N) if JOBZ = 'S'.
* If JOBZ = 'A' or JOBZ = 'O' and M < N, U contains the M-by-M
* orthogonal matrix U;
* if JOBZ = 'S', U contains the first min(M,N) columns of U
* (the left singular vectors, stored columnwise);
* if JOBZ = 'O' and M >= N, or JOBZ = 'N', U is not referenced.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= 1; if
* JOBZ = 'S' or 'A' or JOBZ = 'O' and M < N, LDU >= M.
*
* VT (output) REAL array, dimension (LDVT,N)
* If JOBZ = 'A' or JOBZ = 'O' and M >= N, VT contains the
* N-by-N orthogonal matrix V**T;
* if JOBZ = 'S', VT contains the first min(M,N) rows of
* V**T (the right singular vectors, stored rowwise);
* if JOBZ = 'O' and M < N, or JOBZ = 'N', VT is not referenced.
*
* LDVT (input) INTEGER
* The leading dimension of the array VT. LDVT >= 1; if
* JOBZ = 'A' or JOBZ = 'O' and M >= N, LDVT >= N;
* if JOBZ = 'S', LDVT >= min(M,N).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK;
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= 1.
* If JOBZ = 'N',
* LWORK >= 3*min(M,N) + max(max(M,N),6*min(M,N)).
* If JOBZ = 'O',
* LWORK >= 3*min(M,N)*min(M,N) +
* max(max(M,N),5*min(M,N)*min(M,N)+4*min(M,N)).
* If JOBZ = 'S' or 'A'
* LWORK >= 3*min(M,N)*min(M,N) +
* max(max(M,N),4*min(M,N)*min(M,N)+4*min(M,N)).
* For good performance, LWORK should generally be larger.
* If LWORK = -1 but other input arguments are legal, WORK(1)
* returns the optimal LWORK.
*
* IWORK (workspace) INTEGER array, dimension (8*min(M,N))
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: SBDSDC did not converge, updating process failed.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param m
* @param n
* @param a
* @param lda
* @param s
* @param u
* @param ldu
* @param vt
* @param ldvt
* @param work
* @param lwork
* @param iwork
* @param info
*
*/
abstract public void sgesdd(java.lang.String jobz, int m, int n, float[] a, int lda, float[] s, float[] u, int ldu, float[] vt, int ldvt, float[] work, int lwork, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGESDD computes the singular value decomposition (SVD) of a real
* M-by-N matrix A, optionally computing the left and right singular
* vectors. If singular vectors are desired, it uses a
* divide-and-conquer algorithm.
*
* The SVD is written
*
* A = U * SIGMA * transpose(V)
*
* where SIGMA is an M-by-N matrix which is zero except for its
* min(m,n) diagonal elements, U is an M-by-M orthogonal matrix, and
* V is an N-by-N orthogonal matrix. The diagonal elements of SIGMA
* are the singular values of A; they are real and non-negative, and
* are returned in descending order. The first min(m,n) columns of
* U and V are the left and right singular vectors of A.
*
* Note that the routine returns VT = V**T, not V.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* Specifies options for computing all or part of the matrix U:
* = 'A': all M columns of U and all N rows of V**T are
* returned in the arrays U and VT;
* = 'S': the first min(M,N) columns of U and the first
* min(M,N) rows of V**T are returned in the arrays U
* and VT;
* = 'O': If M >= N, the first N columns of U are overwritten
* on the array A and all rows of V**T are returned in
* the array VT;
* otherwise, all columns of U are returned in the
* array U and the first M rows of V**T are overwritten
* in the array A;
* = 'N': no columns of U or rows of V**T are computed.
*
* M (input) INTEGER
* The number of rows of the input matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the input matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit,
* if JOBZ = 'O', A is overwritten with the first N columns
* of U (the left singular vectors, stored
* columnwise) if M >= N;
* A is overwritten with the first M rows
* of V**T (the right singular vectors, stored
* rowwise) otherwise.
* if JOBZ .ne. 'O', the contents of A are destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* S (output) REAL array, dimension (min(M,N))
* The singular values of A, sorted so that S(i) >= S(i+1).
*
* U (output) REAL array, dimension (LDU,UCOL)
* UCOL = M if JOBZ = 'A' or JOBZ = 'O' and M < N;
* UCOL = min(M,N) if JOBZ = 'S'.
* If JOBZ = 'A' or JOBZ = 'O' and M < N, U contains the M-by-M
* orthogonal matrix U;
* if JOBZ = 'S', U contains the first min(M,N) columns of U
* (the left singular vectors, stored columnwise);
* if JOBZ = 'O' and M >= N, or JOBZ = 'N', U is not referenced.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= 1; if
* JOBZ = 'S' or 'A' or JOBZ = 'O' and M < N, LDU >= M.
*
* VT (output) REAL array, dimension (LDVT,N)
* If JOBZ = 'A' or JOBZ = 'O' and M >= N, VT contains the
* N-by-N orthogonal matrix V**T;
* if JOBZ = 'S', VT contains the first min(M,N) rows of
* V**T (the right singular vectors, stored rowwise);
* if JOBZ = 'O' and M < N, or JOBZ = 'N', VT is not referenced.
*
* LDVT (input) INTEGER
* The leading dimension of the array VT. LDVT >= 1; if
* JOBZ = 'A' or JOBZ = 'O' and M >= N, LDVT >= N;
* if JOBZ = 'S', LDVT >= min(M,N).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK;
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= 1.
* If JOBZ = 'N',
* LWORK >= 3*min(M,N) + max(max(M,N),6*min(M,N)).
* If JOBZ = 'O',
* LWORK >= 3*min(M,N)*min(M,N) +
* max(max(M,N),5*min(M,N)*min(M,N)+4*min(M,N)).
* If JOBZ = 'S' or 'A'
* LWORK >= 3*min(M,N)*min(M,N) +
* max(max(M,N),4*min(M,N)*min(M,N)+4*min(M,N)).
* For good performance, LWORK should generally be larger.
* If LWORK = -1 but other input arguments are legal, WORK(1)
* returns the optimal LWORK.
*
* IWORK (workspace) INTEGER array, dimension (8*min(M,N))
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: SBDSDC did not converge, updating process failed.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param s
* @param _s_offset
* @param u
* @param _u_offset
* @param ldu
* @param vt
* @param _vt_offset
* @param ldvt
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void sgesdd(java.lang.String jobz, int m, int n, float[] a, int _a_offset, int lda, float[] s, int _s_offset, float[] u, int _u_offset, int ldu, float[] vt, int _vt_offset, int ldvt, float[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGESV computes the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N matrix and X and B are N-by-NRHS matrices.
*
* The LU decomposition with partial pivoting and row interchanges is
* used to factor A as
* A = P * L * U,
* where P is a permutation matrix, L is unit lower triangular, and U is
* upper triangular. The factored form of A is then used to solve the
* system of equations A * X = B.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the N-by-N coefficient matrix A.
* On exit, the factors L and U from the factorization
* A = P*L*U; the unit diagonal elements of L are not stored.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (output) INTEGER array, dimension (N)
* The pivot indices that define the permutation matrix P;
* row i of the matrix was interchanged with row IPIV(i).
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS matrix of right hand side matrix B.
* On exit, if INFO = 0, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, U(i,i) is exactly zero. The factorization
* has been completed, but the factor U is exactly
* singular, so the solution could not be computed.
*
* =====================================================================
*
* .. External Subroutines ..
*
*
* @param n
* @param nrhs
* @param a
* @param lda
* @param ipiv
* @param b
* @param ldb
* @param info
*
*/
abstract public void sgesv(int n, int nrhs, float[] a, int lda, int[] ipiv, float[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGESV computes the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N matrix and X and B are N-by-NRHS matrices.
*
* The LU decomposition with partial pivoting and row interchanges is
* used to factor A as
* A = P * L * U,
* where P is a permutation matrix, L is unit lower triangular, and U is
* upper triangular. The factored form of A is then used to solve the
* system of equations A * X = B.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the N-by-N coefficient matrix A.
* On exit, the factors L and U from the factorization
* A = P*L*U; the unit diagonal elements of L are not stored.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (output) INTEGER array, dimension (N)
* The pivot indices that define the permutation matrix P;
* row i of the matrix was interchanged with row IPIV(i).
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS matrix of right hand side matrix B.
* On exit, if INFO = 0, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, U(i,i) is exactly zero. The factorization
* has been completed, but the factor U is exactly
* singular, so the solution could not be computed.
*
* =====================================================================
*
* .. External Subroutines ..
*
*
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void sgesv(int n, int nrhs, float[] a, int _a_offset, int lda, int[] ipiv, int _ipiv_offset, float[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGESVD computes the singular value decomposition (SVD) of a real
* M-by-N matrix A, optionally computing the left and/or right singular
* vectors. The SVD is written
*
* A = U * SIGMA * transpose(V)
*
* where SIGMA is an M-by-N matrix which is zero except for its
* min(m,n) diagonal elements, U is an M-by-M orthogonal matrix, and
* V is an N-by-N orthogonal matrix. The diagonal elements of SIGMA
* are the singular values of A; they are real and non-negative, and
* are returned in descending order. The first min(m,n) columns of
* U and V are the left and right singular vectors of A.
*
* Note that the routine returns V**T, not V.
*
* Arguments
* =========
*
* JOBU (input) CHARACTER*1
* Specifies options for computing all or part of the matrix U:
* = 'A': all M columns of U are returned in array U:
* = 'S': the first min(m,n) columns of U (the left singular
* vectors) are returned in the array U;
* = 'O': the first min(m,n) columns of U (the left singular
* vectors) are overwritten on the array A;
* = 'N': no columns of U (no left singular vectors) are
* computed.
*
* JOBVT (input) CHARACTER*1
* Specifies options for computing all or part of the matrix
* V**T:
* = 'A': all N rows of V**T are returned in the array VT;
* = 'S': the first min(m,n) rows of V**T (the right singular
* vectors) are returned in the array VT;
* = 'O': the first min(m,n) rows of V**T (the right singular
* vectors) are overwritten on the array A;
* = 'N': no rows of V**T (no right singular vectors) are
* computed.
*
* JOBVT and JOBU cannot both be 'O'.
*
* M (input) INTEGER
* The number of rows of the input matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the input matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit,
* if JOBU = 'O', A is overwritten with the first min(m,n)
* columns of U (the left singular vectors,
* stored columnwise);
* if JOBVT = 'O', A is overwritten with the first min(m,n)
* rows of V**T (the right singular vectors,
* stored rowwise);
* if JOBU .ne. 'O' and JOBVT .ne. 'O', the contents of A
* are destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* S (output) REAL array, dimension (min(M,N))
* The singular values of A, sorted so that S(i) >= S(i+1).
*
* U (output) REAL array, dimension (LDU,UCOL)
* (LDU,M) if JOBU = 'A' or (LDU,min(M,N)) if JOBU = 'S'.
* If JOBU = 'A', U contains the M-by-M orthogonal matrix U;
* if JOBU = 'S', U contains the first min(m,n) columns of U
* (the left singular vectors, stored columnwise);
* if JOBU = 'N' or 'O', U is not referenced.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= 1; if
* JOBU = 'S' or 'A', LDU >= M.
*
* VT (output) REAL array, dimension (LDVT,N)
* If JOBVT = 'A', VT contains the N-by-N orthogonal matrix
* V**T;
* if JOBVT = 'S', VT contains the first min(m,n) rows of
* V**T (the right singular vectors, stored rowwise);
* if JOBVT = 'N' or 'O', VT is not referenced.
*
* LDVT (input) INTEGER
* The leading dimension of the array VT. LDVT >= 1; if
* JOBVT = 'A', LDVT >= N; if JOBVT = 'S', LDVT >= min(M,N).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK;
* if INFO > 0, WORK(2:MIN(M,N)) contains the unconverged
* superdiagonal elements of an upper bidiagonal matrix B
* whose diagonal is in S (not necessarily sorted). B
* satisfies A = U * B * VT, so it has the same singular values
* as A, and singular vectors related by U and VT.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* LWORK >= MAX(1,3*MIN(M,N)+MAX(M,N),5*MIN(M,N)).
* For good performance, LWORK should generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if SBDSQR did not converge, INFO specifies how many
* superdiagonals of an intermediate bidiagonal form B
* did not converge to zero. See the description of WORK
* above for details.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobu
* @param jobvt
* @param m
* @param n
* @param a
* @param lda
* @param s
* @param u
* @param ldu
* @param vt
* @param ldvt
* @param work
* @param lwork
* @param info
*
*/
abstract public void sgesvd(java.lang.String jobu, java.lang.String jobvt, int m, int n, float[] a, int lda, float[] s, float[] u, int ldu, float[] vt, int ldvt, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGESVD computes the singular value decomposition (SVD) of a real
* M-by-N matrix A, optionally computing the left and/or right singular
* vectors. The SVD is written
*
* A = U * SIGMA * transpose(V)
*
* where SIGMA is an M-by-N matrix which is zero except for its
* min(m,n) diagonal elements, U is an M-by-M orthogonal matrix, and
* V is an N-by-N orthogonal matrix. The diagonal elements of SIGMA
* are the singular values of A; they are real and non-negative, and
* are returned in descending order. The first min(m,n) columns of
* U and V are the left and right singular vectors of A.
*
* Note that the routine returns V**T, not V.
*
* Arguments
* =========
*
* JOBU (input) CHARACTER*1
* Specifies options for computing all or part of the matrix U:
* = 'A': all M columns of U are returned in array U:
* = 'S': the first min(m,n) columns of U (the left singular
* vectors) are returned in the array U;
* = 'O': the first min(m,n) columns of U (the left singular
* vectors) are overwritten on the array A;
* = 'N': no columns of U (no left singular vectors) are
* computed.
*
* JOBVT (input) CHARACTER*1
* Specifies options for computing all or part of the matrix
* V**T:
* = 'A': all N rows of V**T are returned in the array VT;
* = 'S': the first min(m,n) rows of V**T (the right singular
* vectors) are returned in the array VT;
* = 'O': the first min(m,n) rows of V**T (the right singular
* vectors) are overwritten on the array A;
* = 'N': no rows of V**T (no right singular vectors) are
* computed.
*
* JOBVT and JOBU cannot both be 'O'.
*
* M (input) INTEGER
* The number of rows of the input matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the input matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit,
* if JOBU = 'O', A is overwritten with the first min(m,n)
* columns of U (the left singular vectors,
* stored columnwise);
* if JOBVT = 'O', A is overwritten with the first min(m,n)
* rows of V**T (the right singular vectors,
* stored rowwise);
* if JOBU .ne. 'O' and JOBVT .ne. 'O', the contents of A
* are destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* S (output) REAL array, dimension (min(M,N))
* The singular values of A, sorted so that S(i) >= S(i+1).
*
* U (output) REAL array, dimension (LDU,UCOL)
* (LDU,M) if JOBU = 'A' or (LDU,min(M,N)) if JOBU = 'S'.
* If JOBU = 'A', U contains the M-by-M orthogonal matrix U;
* if JOBU = 'S', U contains the first min(m,n) columns of U
* (the left singular vectors, stored columnwise);
* if JOBU = 'N' or 'O', U is not referenced.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= 1; if
* JOBU = 'S' or 'A', LDU >= M.
*
* VT (output) REAL array, dimension (LDVT,N)
* If JOBVT = 'A', VT contains the N-by-N orthogonal matrix
* V**T;
* if JOBVT = 'S', VT contains the first min(m,n) rows of
* V**T (the right singular vectors, stored rowwise);
* if JOBVT = 'N' or 'O', VT is not referenced.
*
* LDVT (input) INTEGER
* The leading dimension of the array VT. LDVT >= 1; if
* JOBVT = 'A', LDVT >= N; if JOBVT = 'S', LDVT >= min(M,N).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK;
* if INFO > 0, WORK(2:MIN(M,N)) contains the unconverged
* superdiagonal elements of an upper bidiagonal matrix B
* whose diagonal is in S (not necessarily sorted). B
* satisfies A = U * B * VT, so it has the same singular values
* as A, and singular vectors related by U and VT.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* LWORK >= MAX(1,3*MIN(M,N)+MAX(M,N),5*MIN(M,N)).
* For good performance, LWORK should generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if SBDSQR did not converge, INFO specifies how many
* superdiagonals of an intermediate bidiagonal form B
* did not converge to zero. See the description of WORK
* above for details.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobu
* @param jobvt
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param s
* @param _s_offset
* @param u
* @param _u_offset
* @param ldu
* @param vt
* @param _vt_offset
* @param ldvt
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sgesvd(java.lang.String jobu, java.lang.String jobvt, int m, int n, float[] a, int _a_offset, int lda, float[] s, int _s_offset, float[] u, int _u_offset, int ldu, float[] vt, int _vt_offset, int ldvt, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGESVX uses the LU factorization to compute the solution to a real
* system of linear equations
* A * X = B,
* where A is an N-by-N matrix and X and B are N-by-NRHS matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed:
*
* 1. If FACT = 'E', real scaling factors are computed to equilibrate
* the system:
* TRANS = 'N': diag(R)*A*diag(C) *inv(diag(C))*X = diag(R)*B
* TRANS = 'T': (diag(R)*A*diag(C))**T *inv(diag(R))*X = diag(C)*B
* TRANS = 'C': (diag(R)*A*diag(C))**H *inv(diag(R))*X = diag(C)*B
* Whether or not the system will be equilibrated depends on the
* scaling of the matrix A, but if equilibration is used, A is
* overwritten by diag(R)*A*diag(C) and B by diag(R)*B (if TRANS='N')
* or diag(C)*B (if TRANS = 'T' or 'C').
*
* 2. If FACT = 'N' or 'E', the LU decomposition is used to factor the
* matrix A (after equilibration if FACT = 'E') as
* A = P * L * U,
* where P is a permutation matrix, L is a unit lower triangular
* matrix, and U is upper triangular.
*
* 3. If some U(i,i)=0, so that U is exactly singular, then the routine
* returns with INFO = i. Otherwise, the factored form of A is used
* to estimate the condition number of the matrix A. If the
* reciprocal of the condition number is less than machine precision,
* INFO = N+1 is returned as a warning, but the routine still goes on
* to solve for X and compute error bounds as described below.
*
* 4. The system of equations is solved for X using the factored form
* of A.
*
* 5. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* 6. If equilibration was used, the matrix X is premultiplied by
* diag(C) (if TRANS = 'N') or diag(R) (if TRANS = 'T' or 'C') so
* that it solves the original system before equilibration.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of the matrix A is
* supplied on entry, and if not, whether the matrix A should be
* equilibrated before it is factored.
* = 'F': On entry, AF and IPIV contain the factored form of A.
* If EQUED is not 'N', the matrix A has been
* equilibrated with scaling factors given by R and C.
* A, AF, and IPIV are not modified.
* = 'N': The matrix A will be copied to AF and factored.
* = 'E': The matrix A will be equilibrated if necessary, then
* copied to AF and factored.
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Transpose)
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the N-by-N matrix A. If FACT = 'F' and EQUED is
* not 'N', then A must have been equilibrated by the scaling
* factors in R and/or C. A is not modified if FACT = 'F' or
* 'N', or if FACT = 'E' and EQUED = 'N' on exit.
*
* On exit, if EQUED .ne. 'N', A is scaled as follows:
* EQUED = 'R': A := diag(R) * A
* EQUED = 'C': A := A * diag(C)
* EQUED = 'B': A := diag(R) * A * diag(C).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* AF (input or output) REAL array, dimension (LDAF,N)
* If FACT = 'F', then AF is an input argument and on entry
* contains the factors L and U from the factorization
* A = P*L*U as computed by SGETRF. If EQUED .ne. 'N', then
* AF is the factored form of the equilibrated matrix A.
*
* If FACT = 'N', then AF is an output argument and on exit
* returns the factors L and U from the factorization A = P*L*U
* of the original matrix A.
*
* If FACT = 'E', then AF is an output argument and on exit
* returns the factors L and U from the factorization A = P*L*U
* of the equilibrated matrix A (see the description of A for
* the form of the equilibrated matrix).
*
* LDAF (input) INTEGER
* The leading dimension of the array AF. LDAF >= max(1,N).
*
* IPIV (input or output) INTEGER array, dimension (N)
* If FACT = 'F', then IPIV is an input argument and on entry
* contains the pivot indices from the factorization A = P*L*U
* as computed by SGETRF; row i of the matrix was interchanged
* with row IPIV(i).
*
* If FACT = 'N', then IPIV is an output argument and on exit
* contains the pivot indices from the factorization A = P*L*U
* of the original matrix A.
*
* If FACT = 'E', then IPIV is an output argument and on exit
* contains the pivot indices from the factorization A = P*L*U
* of the equilibrated matrix A.
*
* EQUED (input or output) CHARACTER*1
* Specifies the form of equilibration that was done.
* = 'N': No equilibration (always true if FACT = 'N').
* = 'R': Row equilibration, i.e., A has been premultiplied by
* diag(R).
* = 'C': Column equilibration, i.e., A has been postmultiplied
* by diag(C).
* = 'B': Both row and column equilibration, i.e., A has been
* replaced by diag(R) * A * diag(C).
* EQUED is an input argument if FACT = 'F'; otherwise, it is an
* output argument.
*
* R (input or output) REAL array, dimension (N)
* The row scale factors for A. If EQUED = 'R' or 'B', A is
* multiplied on the left by diag(R); if EQUED = 'N' or 'C', R
* is not accessed. R is an input argument if FACT = 'F';
* otherwise, R is an output argument. If FACT = 'F' and
* EQUED = 'R' or 'B', each element of R must be positive.
*
* C (input or output) REAL array, dimension (N)
* The column scale factors for A. If EQUED = 'C' or 'B', A is
* multiplied on the right by diag(C); if EQUED = 'N' or 'R', C
* is not accessed. C is an input argument if FACT = 'F';
* otherwise, C is an output argument. If FACT = 'F' and
* EQUED = 'C' or 'B', each element of C must be positive.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit,
* if EQUED = 'N', B is not modified;
* if TRANS = 'N' and EQUED = 'R' or 'B', B is overwritten by
* diag(R)*B;
* if TRANS = 'T' or 'C' and EQUED = 'C' or 'B', B is
* overwritten by diag(C)*B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) REAL array, dimension (LDX,NRHS)
* If INFO = 0 or INFO = N+1, the N-by-NRHS solution matrix X
* to the original system of equations. Note that A and B are
* modified on exit if EQUED .ne. 'N', and the solution to the
* equilibrated system is inv(diag(C))*X if TRANS = 'N' and
* EQUED = 'C' or 'B', or inv(diag(R))*X if TRANS = 'T' or 'C'
* and EQUED = 'R' or 'B'.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) REAL
* The estimate of the reciprocal condition number of the matrix
* A after equilibration (if done). If RCOND is less than the
* machine precision (in particular, if RCOND = 0), the matrix
* is singular to working precision. This condition is
* indicated by a return code of INFO > 0.
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace/output) REAL array, dimension (4*N)
* On exit, WORK(1) contains the reciprocal pivot growth
* factor norm(A)/norm(U). The "max absolute element" norm is
* used. If WORK(1) is much less than 1, then the stability
* of the LU factorization of the (equilibrated) matrix A
* could be poor. This also means that the solution X, condition
* estimator RCOND, and forward error bound FERR could be
* unreliable. If factorization fails with 0 0: if INFO = i, and i is
* <= N: U(i,i) is exactly zero. The factorization has
* been completed, but the factor U is exactly
* singular, so the solution and error bounds
* could not be computed. RCOND = 0 is returned.
* = N+1: U is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param trans
* @param n
* @param nrhs
* @param a
* @param lda
* @param af
* @param ldaf
* @param ipiv
* @param equed
* @param r
* @param c
* @param b
* @param ldb
* @param x
* @param ldx
* @param rcond
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void sgesvx(java.lang.String fact, java.lang.String trans, int n, int nrhs, float[] a, int lda, float[] af, int ldaf, int[] ipiv, org.netlib.util.StringW equed, float[] r, float[] c, float[] b, int ldb, float[] x, int ldx, org.netlib.util.floatW rcond, float[] ferr, float[] berr, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGESVX uses the LU factorization to compute the solution to a real
* system of linear equations
* A * X = B,
* where A is an N-by-N matrix and X and B are N-by-NRHS matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed:
*
* 1. If FACT = 'E', real scaling factors are computed to equilibrate
* the system:
* TRANS = 'N': diag(R)*A*diag(C) *inv(diag(C))*X = diag(R)*B
* TRANS = 'T': (diag(R)*A*diag(C))**T *inv(diag(R))*X = diag(C)*B
* TRANS = 'C': (diag(R)*A*diag(C))**H *inv(diag(R))*X = diag(C)*B
* Whether or not the system will be equilibrated depends on the
* scaling of the matrix A, but if equilibration is used, A is
* overwritten by diag(R)*A*diag(C) and B by diag(R)*B (if TRANS='N')
* or diag(C)*B (if TRANS = 'T' or 'C').
*
* 2. If FACT = 'N' or 'E', the LU decomposition is used to factor the
* matrix A (after equilibration if FACT = 'E') as
* A = P * L * U,
* where P is a permutation matrix, L is a unit lower triangular
* matrix, and U is upper triangular.
*
* 3. If some U(i,i)=0, so that U is exactly singular, then the routine
* returns with INFO = i. Otherwise, the factored form of A is used
* to estimate the condition number of the matrix A. If the
* reciprocal of the condition number is less than machine precision,
* INFO = N+1 is returned as a warning, but the routine still goes on
* to solve for X and compute error bounds as described below.
*
* 4. The system of equations is solved for X using the factored form
* of A.
*
* 5. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* 6. If equilibration was used, the matrix X is premultiplied by
* diag(C) (if TRANS = 'N') or diag(R) (if TRANS = 'T' or 'C') so
* that it solves the original system before equilibration.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of the matrix A is
* supplied on entry, and if not, whether the matrix A should be
* equilibrated before it is factored.
* = 'F': On entry, AF and IPIV contain the factored form of A.
* If EQUED is not 'N', the matrix A has been
* equilibrated with scaling factors given by R and C.
* A, AF, and IPIV are not modified.
* = 'N': The matrix A will be copied to AF and factored.
* = 'E': The matrix A will be equilibrated if necessary, then
* copied to AF and factored.
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Transpose)
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the N-by-N matrix A. If FACT = 'F' and EQUED is
* not 'N', then A must have been equilibrated by the scaling
* factors in R and/or C. A is not modified if FACT = 'F' or
* 'N', or if FACT = 'E' and EQUED = 'N' on exit.
*
* On exit, if EQUED .ne. 'N', A is scaled as follows:
* EQUED = 'R': A := diag(R) * A
* EQUED = 'C': A := A * diag(C)
* EQUED = 'B': A := diag(R) * A * diag(C).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* AF (input or output) REAL array, dimension (LDAF,N)
* If FACT = 'F', then AF is an input argument and on entry
* contains the factors L and U from the factorization
* A = P*L*U as computed by SGETRF. If EQUED .ne. 'N', then
* AF is the factored form of the equilibrated matrix A.
*
* If FACT = 'N', then AF is an output argument and on exit
* returns the factors L and U from the factorization A = P*L*U
* of the original matrix A.
*
* If FACT = 'E', then AF is an output argument and on exit
* returns the factors L and U from the factorization A = P*L*U
* of the equilibrated matrix A (see the description of A for
* the form of the equilibrated matrix).
*
* LDAF (input) INTEGER
* The leading dimension of the array AF. LDAF >= max(1,N).
*
* IPIV (input or output) INTEGER array, dimension (N)
* If FACT = 'F', then IPIV is an input argument and on entry
* contains the pivot indices from the factorization A = P*L*U
* as computed by SGETRF; row i of the matrix was interchanged
* with row IPIV(i).
*
* If FACT = 'N', then IPIV is an output argument and on exit
* contains the pivot indices from the factorization A = P*L*U
* of the original matrix A.
*
* If FACT = 'E', then IPIV is an output argument and on exit
* contains the pivot indices from the factorization A = P*L*U
* of the equilibrated matrix A.
*
* EQUED (input or output) CHARACTER*1
* Specifies the form of equilibration that was done.
* = 'N': No equilibration (always true if FACT = 'N').
* = 'R': Row equilibration, i.e., A has been premultiplied by
* diag(R).
* = 'C': Column equilibration, i.e., A has been postmultiplied
* by diag(C).
* = 'B': Both row and column equilibration, i.e., A has been
* replaced by diag(R) * A * diag(C).
* EQUED is an input argument if FACT = 'F'; otherwise, it is an
* output argument.
*
* R (input or output) REAL array, dimension (N)
* The row scale factors for A. If EQUED = 'R' or 'B', A is
* multiplied on the left by diag(R); if EQUED = 'N' or 'C', R
* is not accessed. R is an input argument if FACT = 'F';
* otherwise, R is an output argument. If FACT = 'F' and
* EQUED = 'R' or 'B', each element of R must be positive.
*
* C (input or output) REAL array, dimension (N)
* The column scale factors for A. If EQUED = 'C' or 'B', A is
* multiplied on the right by diag(C); if EQUED = 'N' or 'R', C
* is not accessed. C is an input argument if FACT = 'F';
* otherwise, C is an output argument. If FACT = 'F' and
* EQUED = 'C' or 'B', each element of C must be positive.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit,
* if EQUED = 'N', B is not modified;
* if TRANS = 'N' and EQUED = 'R' or 'B', B is overwritten by
* diag(R)*B;
* if TRANS = 'T' or 'C' and EQUED = 'C' or 'B', B is
* overwritten by diag(C)*B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) REAL array, dimension (LDX,NRHS)
* If INFO = 0 or INFO = N+1, the N-by-NRHS solution matrix X
* to the original system of equations. Note that A and B are
* modified on exit if EQUED .ne. 'N', and the solution to the
* equilibrated system is inv(diag(C))*X if TRANS = 'N' and
* EQUED = 'C' or 'B', or inv(diag(R))*X if TRANS = 'T' or 'C'
* and EQUED = 'R' or 'B'.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) REAL
* The estimate of the reciprocal condition number of the matrix
* A after equilibration (if done). If RCOND is less than the
* machine precision (in particular, if RCOND = 0), the matrix
* is singular to working precision. This condition is
* indicated by a return code of INFO > 0.
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace/output) REAL array, dimension (4*N)
* On exit, WORK(1) contains the reciprocal pivot growth
* factor norm(A)/norm(U). The "max absolute element" norm is
* used. If WORK(1) is much less than 1, then the stability
* of the LU factorization of the (equilibrated) matrix A
* could be poor. This also means that the solution X, condition
* estimator RCOND, and forward error bound FERR could be
* unreliable. If factorization fails with 0 0: if INFO = i, and i is
* <= N: U(i,i) is exactly zero. The factorization has
* been completed, but the factor U is exactly
* singular, so the solution and error bounds
* could not be computed. RCOND = 0 is returned.
* = N+1: U is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param trans
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param af
* @param _af_offset
* @param ldaf
* @param ipiv
* @param _ipiv_offset
* @param equed
* @param r
* @param _r_offset
* @param c
* @param _c_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param rcond
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void sgesvx(java.lang.String fact, java.lang.String trans, int n, int nrhs, float[] a, int _a_offset, int lda, float[] af, int _af_offset, int ldaf, int[] ipiv, int _ipiv_offset, org.netlib.util.StringW equed, float[] r, int _r_offset, float[] c, int _c_offset, float[] b, int _b_offset, int ldb, float[] x, int _x_offset, int ldx, org.netlib.util.floatW rcond, float[] ferr, int _ferr_offset, float[] berr, int _berr_offset, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGETC2 computes an LU factorization with complete pivoting of the
* n-by-n matrix A. The factorization has the form A = P * L * U * Q,
* where P and Q are permutation matrices, L is lower triangular with
* unit diagonal elements and U is upper triangular.
*
* This is the Level 2 BLAS algorithm.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA, N)
* On entry, the n-by-n matrix A to be factored.
* On exit, the factors L and U from the factorization
* A = P*L*U*Q; the unit diagonal elements of L are not stored.
* If U(k, k) appears to be less than SMIN, U(k, k) is given the
* value of SMIN, i.e., giving a nonsingular perturbed system.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (output) INTEGER array, dimension(N).
* The pivot indices; for 1 <= i <= N, row i of the
* matrix has been interchanged with row IPIV(i).
*
* JPIV (output) INTEGER array, dimension(N).
* The pivot indices; for 1 <= j <= N, column j of the
* matrix has been interchanged with column JPIV(j).
*
* INFO (output) INTEGER
* = 0: successful exit
* > 0: if INFO = k, U(k, k) is likely to produce owerflow if
* we try to solve for x in Ax = b. So U is perturbed to
* avoid the overflow.
*
* Further Details
* ===============
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param a
* @param lda
* @param ipiv
* @param jpiv
* @param info
*
*/
abstract public void sgetc2(int n, float[] a, int lda, int[] ipiv, int[] jpiv, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGETC2 computes an LU factorization with complete pivoting of the
* n-by-n matrix A. The factorization has the form A = P * L * U * Q,
* where P and Q are permutation matrices, L is lower triangular with
* unit diagonal elements and U is upper triangular.
*
* This is the Level 2 BLAS algorithm.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA, N)
* On entry, the n-by-n matrix A to be factored.
* On exit, the factors L and U from the factorization
* A = P*L*U*Q; the unit diagonal elements of L are not stored.
* If U(k, k) appears to be less than SMIN, U(k, k) is given the
* value of SMIN, i.e., giving a nonsingular perturbed system.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (output) INTEGER array, dimension(N).
* The pivot indices; for 1 <= i <= N, row i of the
* matrix has been interchanged with row IPIV(i).
*
* JPIV (output) INTEGER array, dimension(N).
* The pivot indices; for 1 <= j <= N, column j of the
* matrix has been interchanged with column JPIV(j).
*
* INFO (output) INTEGER
* = 0: successful exit
* > 0: if INFO = k, U(k, k) is likely to produce owerflow if
* we try to solve for x in Ax = b. So U is perturbed to
* avoid the overflow.
*
* Further Details
* ===============
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param a
* @param _a_offset
* @param lda
* @param ipiv
* @param _ipiv_offset
* @param jpiv
* @param _jpiv_offset
* @param info
*
*/
abstract public void sgetc2(int n, float[] a, int _a_offset, int lda, int[] ipiv, int _ipiv_offset, int[] jpiv, int _jpiv_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGETF2 computes an LU factorization of a general m-by-n matrix A
* using partial pivoting with row interchanges.
*
* The factorization has the form
* A = P * L * U
* where P is a permutation matrix, L is lower triangular with unit
* diagonal elements (lower trapezoidal if m > n), and U is upper
* triangular (upper trapezoidal if m < n).
*
* This is the right-looking Level 2 BLAS version of the algorithm.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the m by n matrix to be factored.
* On exit, the factors L and U from the factorization
* A = P*L*U; the unit diagonal elements of L are not stored.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* IPIV (output) INTEGER array, dimension (min(M,N))
* The pivot indices; for 1 <= i <= min(M,N), row i of the
* matrix was interchanged with row IPIV(i).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
* > 0: if INFO = k, U(k,k) is exactly zero. The factorization
* has been completed, but the factor U is exactly
* singular, and division by zero will occur if it is used
* to solve a system of equations.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param ipiv
* @param info
*
*/
abstract public void sgetf2(int m, int n, float[] a, int lda, int[] ipiv, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGETF2 computes an LU factorization of a general m-by-n matrix A
* using partial pivoting with row interchanges.
*
* The factorization has the form
* A = P * L * U
* where P is a permutation matrix, L is lower triangular with unit
* diagonal elements (lower trapezoidal if m > n), and U is upper
* triangular (upper trapezoidal if m < n).
*
* This is the right-looking Level 2 BLAS version of the algorithm.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the m by n matrix to be factored.
* On exit, the factors L and U from the factorization
* A = P*L*U; the unit diagonal elements of L are not stored.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* IPIV (output) INTEGER array, dimension (min(M,N))
* The pivot indices; for 1 <= i <= min(M,N), row i of the
* matrix was interchanged with row IPIV(i).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
* > 0: if INFO = k, U(k,k) is exactly zero. The factorization
* has been completed, but the factor U is exactly
* singular, and division by zero will occur if it is used
* to solve a system of equations.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param ipiv
* @param _ipiv_offset
* @param info
*
*/
abstract public void sgetf2(int m, int n, float[] a, int _a_offset, int lda, int[] ipiv, int _ipiv_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGETRF computes an LU factorization of a general M-by-N matrix A
* using partial pivoting with row interchanges.
*
* The factorization has the form
* A = P * L * U
* where P is a permutation matrix, L is lower triangular with unit
* diagonal elements (lower trapezoidal if m > n), and U is upper
* triangular (upper trapezoidal if m < n).
*
* This is the right-looking Level 3 BLAS version of the algorithm.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix to be factored.
* On exit, the factors L and U from the factorization
* A = P*L*U; the unit diagonal elements of L are not stored.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* IPIV (output) INTEGER array, dimension (min(M,N))
* The pivot indices; for 1 <= i <= min(M,N), row i of the
* matrix was interchanged with row IPIV(i).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, U(i,i) is exactly zero. The factorization
* has been completed, but the factor U is exactly
* singular, and division by zero will occur if it is used
* to solve a system of equations.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param ipiv
* @param info
*
*/
abstract public void sgetrf(int m, int n, float[] a, int lda, int[] ipiv, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGETRF computes an LU factorization of a general M-by-N matrix A
* using partial pivoting with row interchanges.
*
* The factorization has the form
* A = P * L * U
* where P is a permutation matrix, L is lower triangular with unit
* diagonal elements (lower trapezoidal if m > n), and U is upper
* triangular (upper trapezoidal if m < n).
*
* This is the right-looking Level 3 BLAS version of the algorithm.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix to be factored.
* On exit, the factors L and U from the factorization
* A = P*L*U; the unit diagonal elements of L are not stored.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* IPIV (output) INTEGER array, dimension (min(M,N))
* The pivot indices; for 1 <= i <= min(M,N), row i of the
* matrix was interchanged with row IPIV(i).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, U(i,i) is exactly zero. The factorization
* has been completed, but the factor U is exactly
* singular, and division by zero will occur if it is used
* to solve a system of equations.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param ipiv
* @param _ipiv_offset
* @param info
*
*/
abstract public void sgetrf(int m, int n, float[] a, int _a_offset, int lda, int[] ipiv, int _ipiv_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGETRI computes the inverse of a matrix using the LU factorization
* computed by SGETRF.
*
* This method inverts U and then computes inv(A) by solving the system
* inv(A)*L = inv(U) for inv(A).
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the factors L and U from the factorization
* A = P*L*U as computed by SGETRF.
* On exit, if INFO = 0, the inverse of the original matrix A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices from SGETRF; for 1<=i<=N, row i of the
* matrix was interchanged with row IPIV(i).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO=0, then WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N).
* For optimal performance LWORK >= N*NB, where NB is
* the optimal blocksize returned by ILAENV.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, U(i,i) is exactly zero; the matrix is
* singular and its inverse could not be computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param a
* @param lda
* @param ipiv
* @param work
* @param lwork
* @param info
*
*/
abstract public void sgetri(int n, float[] a, int lda, int[] ipiv, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGETRI computes the inverse of a matrix using the LU factorization
* computed by SGETRF.
*
* This method inverts U and then computes inv(A) by solving the system
* inv(A)*L = inv(U) for inv(A).
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the factors L and U from the factorization
* A = P*L*U as computed by SGETRF.
* On exit, if INFO = 0, the inverse of the original matrix A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices from SGETRF; for 1<=i<=N, row i of the
* matrix was interchanged with row IPIV(i).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO=0, then WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N).
* For optimal performance LWORK >= N*NB, where NB is
* the optimal blocksize returned by ILAENV.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, U(i,i) is exactly zero; the matrix is
* singular and its inverse could not be computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param a
* @param _a_offset
* @param lda
* @param ipiv
* @param _ipiv_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sgetri(int n, float[] a, int _a_offset, int lda, int[] ipiv, int _ipiv_offset, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGETRS solves a system of linear equations
* A * X = B or A' * X = B
* with a general N-by-N matrix A using the LU factorization computed
* by SGETRF.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A'* X = B (Transpose)
* = 'C': A'* X = B (Conjugate transpose = Transpose)
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The factors L and U from the factorization A = P*L*U
* as computed by SGETRF.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices from SGETRF; for 1<=i<=N, row i of the
* matrix was interchanged with row IPIV(i).
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param trans
* @param n
* @param nrhs
* @param a
* @param lda
* @param ipiv
* @param b
* @param ldb
* @param info
*
*/
abstract public void sgetrs(java.lang.String trans, int n, int nrhs, float[] a, int lda, int[] ipiv, float[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGETRS solves a system of linear equations
* A * X = B or A' * X = B
* with a general N-by-N matrix A using the LU factorization computed
* by SGETRF.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A'* X = B (Transpose)
* = 'C': A'* X = B (Conjugate transpose = Transpose)
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The factors L and U from the factorization A = P*L*U
* as computed by SGETRF.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices from SGETRF; for 1<=i<=N, row i of the
* matrix was interchanged with row IPIV(i).
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param trans
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void sgetrs(java.lang.String trans, int n, int nrhs, float[] a, int _a_offset, int lda, int[] ipiv, int _ipiv_offset, float[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGGBAK forms the right or left eigenvectors of a real generalized
* eigenvalue problem A*x = lambda*B*x, by backward transformation on
* the computed eigenvectors of the balanced pair of matrices output by
* SGGBAL.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* Specifies the type of backward transformation required:
* = 'N': do nothing, return immediately;
* = 'P': do backward transformation for permutation only;
* = 'S': do backward transformation for scaling only;
* = 'B': do backward transformations for both permutation and
* scaling.
* JOB must be the same as the argument JOB supplied to SGGBAL.
*
* SIDE (input) CHARACTER*1
* = 'R': V contains right eigenvectors;
* = 'L': V contains left eigenvectors.
*
* N (input) INTEGER
* The number of rows of the matrix V. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* The integers ILO and IHI determined by SGGBAL.
* 1 <= ILO <= IHI <= N, if N > 0; ILO=1 and IHI=0, if N=0.
*
* LSCALE (input) REAL array, dimension (N)
* Details of the permutations and/or scaling factors applied
* to the left side of A and B, as returned by SGGBAL.
*
* RSCALE (input) REAL array, dimension (N)
* Details of the permutations and/or scaling factors applied
* to the right side of A and B, as returned by SGGBAL.
*
* M (input) INTEGER
* The number of columns of the matrix V. M >= 0.
*
* V (input/output) REAL array, dimension (LDV,M)
* On entry, the matrix of right or left eigenvectors to be
* transformed, as returned by STGEVC.
* On exit, V is overwritten by the transformed eigenvectors.
*
* LDV (input) INTEGER
* The leading dimension of the matrix V. LDV >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* See R.C. Ward, Balancing the generalized eigenvalue problem,
* SIAM J. Sci. Stat. Comp. 2 (1981), 141-152.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param job
* @param side
* @param n
* @param ilo
* @param ihi
* @param lscale
* @param rscale
* @param m
* @param v
* @param ldv
* @param info
*
*/
abstract public void sggbak(java.lang.String job, java.lang.String side, int n, int ilo, int ihi, float[] lscale, float[] rscale, int m, float[] v, int ldv, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGGBAK forms the right or left eigenvectors of a real generalized
* eigenvalue problem A*x = lambda*B*x, by backward transformation on
* the computed eigenvectors of the balanced pair of matrices output by
* SGGBAL.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* Specifies the type of backward transformation required:
* = 'N': do nothing, return immediately;
* = 'P': do backward transformation for permutation only;
* = 'S': do backward transformation for scaling only;
* = 'B': do backward transformations for both permutation and
* scaling.
* JOB must be the same as the argument JOB supplied to SGGBAL.
*
* SIDE (input) CHARACTER*1
* = 'R': V contains right eigenvectors;
* = 'L': V contains left eigenvectors.
*
* N (input) INTEGER
* The number of rows of the matrix V. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* The integers ILO and IHI determined by SGGBAL.
* 1 <= ILO <= IHI <= N, if N > 0; ILO=1 and IHI=0, if N=0.
*
* LSCALE (input) REAL array, dimension (N)
* Details of the permutations and/or scaling factors applied
* to the left side of A and B, as returned by SGGBAL.
*
* RSCALE (input) REAL array, dimension (N)
* Details of the permutations and/or scaling factors applied
* to the right side of A and B, as returned by SGGBAL.
*
* M (input) INTEGER
* The number of columns of the matrix V. M >= 0.
*
* V (input/output) REAL array, dimension (LDV,M)
* On entry, the matrix of right or left eigenvectors to be
* transformed, as returned by STGEVC.
* On exit, V is overwritten by the transformed eigenvectors.
*
* LDV (input) INTEGER
* The leading dimension of the matrix V. LDV >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* See R.C. Ward, Balancing the generalized eigenvalue problem,
* SIAM J. Sci. Stat. Comp. 2 (1981), 141-152.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param job
* @param side
* @param n
* @param ilo
* @param ihi
* @param lscale
* @param _lscale_offset
* @param rscale
* @param _rscale_offset
* @param m
* @param v
* @param _v_offset
* @param ldv
* @param info
*
*/
abstract public void sggbak(java.lang.String job, java.lang.String side, int n, int ilo, int ihi, float[] lscale, int _lscale_offset, float[] rscale, int _rscale_offset, int m, float[] v, int _v_offset, int ldv, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGGBAL balances a pair of general real matrices (A,B). This
* involves, first, permuting A and B by similarity transformations to
* isolate eigenvalues in the first 1 to ILO$-$1 and last IHI+1 to N
* elements on the diagonal; and second, applying a diagonal similarity
* transformation to rows and columns ILO to IHI to make the rows
* and columns as close in norm as possible. Both steps are optional.
*
* Balancing may reduce the 1-norm of the matrices, and improve the
* accuracy of the computed eigenvalues and/or eigenvectors in the
* generalized eigenvalue problem A*x = lambda*B*x.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* Specifies the operations to be performed on A and B:
* = 'N': none: simply set ILO = 1, IHI = N, LSCALE(I) = 1.0
* and RSCALE(I) = 1.0 for i = 1,...,N.
* = 'P': permute only;
* = 'S': scale only;
* = 'B': both permute and scale.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the input matrix A.
* On exit, A is overwritten by the balanced matrix.
* If JOB = 'N', A is not referenced.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension (LDB,N)
* On entry, the input matrix B.
* On exit, B is overwritten by the balanced matrix.
* If JOB = 'N', B is not referenced.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* ILO (output) INTEGER
* IHI (output) INTEGER
* ILO and IHI are set to integers such that on exit
* A(i,j) = 0 and B(i,j) = 0 if i > j and
* j = 1,...,ILO-1 or i = IHI+1,...,N.
* If JOB = 'N' or 'S', ILO = 1 and IHI = N.
*
* LSCALE (output) REAL array, dimension (N)
* Details of the permutations and scaling factors applied
* to the left side of A and B. If P(j) is the index of the
* row interchanged with row j, and D(j)
* is the scaling factor applied to row j, then
* LSCALE(j) = P(j) for J = 1,...,ILO-1
* = D(j) for J = ILO,...,IHI
* = P(j) for J = IHI+1,...,N.
* The order in which the interchanges are made is N to IHI+1,
* then 1 to ILO-1.
*
* RSCALE (output) REAL array, dimension (N)
* Details of the permutations and scaling factors applied
* to the right side of A and B. If P(j) is the index of the
* column interchanged with column j, and D(j)
* is the scaling factor applied to column j, then
* LSCALE(j) = P(j) for J = 1,...,ILO-1
* = D(j) for J = ILO,...,IHI
* = P(j) for J = IHI+1,...,N.
* The order in which the interchanges are made is N to IHI+1,
* then 1 to ILO-1.
*
* WORK (workspace) REAL array, dimension (lwork)
* lwork must be at least max(1,6*N) when JOB = 'S' or 'B', and
* at least 1 when JOB = 'N' or 'P'.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* See R.C. WARD, Balancing the generalized eigenvalue problem,
* SIAM J. Sci. Stat. Comp. 2 (1981), 141-152.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param ilo
* @param ihi
* @param lscale
* @param rscale
* @param work
* @param info
*
*/
abstract public void sggbal(java.lang.String job, int n, float[] a, int lda, float[] b, int ldb, org.netlib.util.intW ilo, org.netlib.util.intW ihi, float[] lscale, float[] rscale, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGGBAL balances a pair of general real matrices (A,B). This
* involves, first, permuting A and B by similarity transformations to
* isolate eigenvalues in the first 1 to ILO$-$1 and last IHI+1 to N
* elements on the diagonal; and second, applying a diagonal similarity
* transformation to rows and columns ILO to IHI to make the rows
* and columns as close in norm as possible. Both steps are optional.
*
* Balancing may reduce the 1-norm of the matrices, and improve the
* accuracy of the computed eigenvalues and/or eigenvectors in the
* generalized eigenvalue problem A*x = lambda*B*x.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* Specifies the operations to be performed on A and B:
* = 'N': none: simply set ILO = 1, IHI = N, LSCALE(I) = 1.0
* and RSCALE(I) = 1.0 for i = 1,...,N.
* = 'P': permute only;
* = 'S': scale only;
* = 'B': both permute and scale.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the input matrix A.
* On exit, A is overwritten by the balanced matrix.
* If JOB = 'N', A is not referenced.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension (LDB,N)
* On entry, the input matrix B.
* On exit, B is overwritten by the balanced matrix.
* If JOB = 'N', B is not referenced.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* ILO (output) INTEGER
* IHI (output) INTEGER
* ILO and IHI are set to integers such that on exit
* A(i,j) = 0 and B(i,j) = 0 if i > j and
* j = 1,...,ILO-1 or i = IHI+1,...,N.
* If JOB = 'N' or 'S', ILO = 1 and IHI = N.
*
* LSCALE (output) REAL array, dimension (N)
* Details of the permutations and scaling factors applied
* to the left side of A and B. If P(j) is the index of the
* row interchanged with row j, and D(j)
* is the scaling factor applied to row j, then
* LSCALE(j) = P(j) for J = 1,...,ILO-1
* = D(j) for J = ILO,...,IHI
* = P(j) for J = IHI+1,...,N.
* The order in which the interchanges are made is N to IHI+1,
* then 1 to ILO-1.
*
* RSCALE (output) REAL array, dimension (N)
* Details of the permutations and scaling factors applied
* to the right side of A and B. If P(j) is the index of the
* column interchanged with column j, and D(j)
* is the scaling factor applied to column j, then
* LSCALE(j) = P(j) for J = 1,...,ILO-1
* = D(j) for J = ILO,...,IHI
* = P(j) for J = IHI+1,...,N.
* The order in which the interchanges are made is N to IHI+1,
* then 1 to ILO-1.
*
* WORK (workspace) REAL array, dimension (lwork)
* lwork must be at least max(1,6*N) when JOB = 'S' or 'B', and
* at least 1 when JOB = 'N' or 'P'.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* See R.C. WARD, Balancing the generalized eigenvalue problem,
* SIAM J. Sci. Stat. Comp. 2 (1981), 141-152.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param ilo
* @param ihi
* @param lscale
* @param _lscale_offset
* @param rscale
* @param _rscale_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void sggbal(java.lang.String job, int n, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, org.netlib.util.intW ilo, org.netlib.util.intW ihi, float[] lscale, int _lscale_offset, float[] rscale, int _rscale_offset, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGGES computes for a pair of N-by-N real nonsymmetric matrices (A,B),
* the generalized eigenvalues, the generalized real Schur form (S,T),
* optionally, the left and/or right matrices of Schur vectors (VSL and
* VSR). This gives the generalized Schur factorization
*
* (A,B) = ( (VSL)*S*(VSR)**T, (VSL)*T*(VSR)**T )
*
* Optionally, it also orders the eigenvalues so that a selected cluster
* of eigenvalues appears in the leading diagonal blocks of the upper
* quasi-triangular matrix S and the upper triangular matrix T.The
* leading columns of VSL and VSR then form an orthonormal basis for the
* corresponding left and right eigenspaces (deflating subspaces).
*
* (If only the generalized eigenvalues are needed, use the driver
* SGGEV instead, which is faster.)
*
* A generalized eigenvalue for a pair of matrices (A,B) is a scalar w
* or a ratio alpha/beta = w, such that A - w*B is singular. It is
* usually represented as the pair (alpha,beta), as there is a
* reasonable interpretation for beta=0 or both being zero.
*
* A pair of matrices (S,T) is in generalized real Schur form if T is
* upper triangular with non-negative diagonal and S is block upper
* triangular with 1-by-1 and 2-by-2 blocks. 1-by-1 blocks correspond
* to real generalized eigenvalues, while 2-by-2 blocks of S will be
* "standardized" by making the corresponding elements of T have the
* form:
* [ a 0 ]
* [ 0 b ]
*
* and the pair of corresponding 2-by-2 blocks in S and T will have a
* complex conjugate pair of generalized eigenvalues.
*
*
* Arguments
* =========
*
* JOBVSL (input) CHARACTER*1
* = 'N': do not compute the left Schur vectors;
* = 'V': compute the left Schur vectors.
*
* JOBVSR (input) CHARACTER*1
* = 'N': do not compute the right Schur vectors;
* = 'V': compute the right Schur vectors.
*
* SORT (input) CHARACTER*1
* Specifies whether or not to order the eigenvalues on the
* diagonal of the generalized Schur form.
* = 'N': Eigenvalues are not ordered;
* = 'S': Eigenvalues are ordered (see SELCTG);
*
* SELCTG (external procedure) LOGICAL FUNCTION of three REAL arguments
* SELCTG must be declared EXTERNAL in the calling subroutine.
* If SORT = 'N', SELCTG is not referenced.
* If SORT = 'S', SELCTG is used to select eigenvalues to sort
* to the top left of the Schur form.
* An eigenvalue (ALPHAR(j)+ALPHAI(j))/BETA(j) is selected if
* SELCTG(ALPHAR(j),ALPHAI(j),BETA(j)) is true; i.e. if either
* one of a complex conjugate pair of eigenvalues is selected,
* then both complex eigenvalues are selected.
*
* Note that in the ill-conditioned case, a selected complex
* eigenvalue may no longer satisfy SELCTG(ALPHAR(j),ALPHAI(j),
* BETA(j)) = .TRUE. after ordering. INFO is to be set to N+2
* in this case.
*
* N (input) INTEGER
* The order of the matrices A, B, VSL, and VSR. N >= 0.
*
* A (input/output) REAL array, dimension (LDA, N)
* On entry, the first of the pair of matrices.
* On exit, A has been overwritten by its generalized Schur
* form S.
*
* LDA (input) INTEGER
* The leading dimension of A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension (LDB, N)
* On entry, the second of the pair of matrices.
* On exit, B has been overwritten by its generalized Schur
* form T.
*
* LDB (input) INTEGER
* The leading dimension of B. LDB >= max(1,N).
*
* SDIM (output) INTEGER
* If SORT = 'N', SDIM = 0.
* If SORT = 'S', SDIM = number of eigenvalues (after sorting)
* for which SELCTG is true. (Complex conjugate pairs for which
* SELCTG is true for either eigenvalue count as 2.)
*
* ALPHAR (output) REAL array, dimension (N)
* ALPHAI (output) REAL array, dimension (N)
* BETA (output) REAL array, dimension (N)
* On exit, (ALPHAR(j) + ALPHAI(j)*i)/BETA(j), j=1,...,N, will
* be the generalized eigenvalues. ALPHAR(j) + ALPHAI(j)*i,
* and BETA(j),j=1,...,N are the diagonals of the complex Schur
* form (S,T) that would result if the 2-by-2 diagonal blocks of
* the real Schur form of (A,B) were further reduced to
* triangular form using 2-by-2 complex unitary transformations.
* If ALPHAI(j) is zero, then the j-th eigenvalue is real; if
* positive, then the j-th and (j+1)-st eigenvalues are a
* complex conjugate pair, with ALPHAI(j+1) negative.
*
* Note: the quotients ALPHAR(j)/BETA(j) and ALPHAI(j)/BETA(j)
* may easily over- or underflow, and BETA(j) may even be zero.
* Thus, the user should avoid naively computing the ratio.
* However, ALPHAR and ALPHAI will be always less than and
* usually comparable with norm(A) in magnitude, and BETA always
* less than and usually comparable with norm(B).
*
* VSL (output) REAL array, dimension (LDVSL,N)
* If JOBVSL = 'V', VSL will contain the left Schur vectors.
* Not referenced if JOBVSL = 'N'.
*
* LDVSL (input) INTEGER
* The leading dimension of the matrix VSL. LDVSL >=1, and
* if JOBVSL = 'V', LDVSL >= N.
*
* VSR (output) REAL array, dimension (LDVSR,N)
* If JOBVSR = 'V', VSR will contain the right Schur vectors.
* Not referenced if JOBVSR = 'N'.
*
* LDVSR (input) INTEGER
* The leading dimension of the matrix VSR. LDVSR >= 1, and
* if JOBVSR = 'V', LDVSR >= N.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If N = 0, LWORK >= 1, else LWORK >= max(8*N,6*N+16).
* For good performance , LWORK must generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* BWORK (workspace) LOGICAL array, dimension (N)
* Not referenced if SORT = 'N'.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1,...,N:
* The QZ iteration failed. (A,B) are not in Schur
* form, but ALPHAR(j), ALPHAI(j), and BETA(j) should
* be correct for j=INFO+1,...,N.
* > N: =N+1: other than QZ iteration failed in SHGEQZ.
* =N+2: after reordering, roundoff changed values of
* some complex eigenvalues so that leading
* eigenvalues in the Generalized Schur form no
* longer satisfy SELCTG=.TRUE. This could also
* be caused due to scaling.
* =N+3: reordering failed in STGSEN.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobvsl
* @param jobvsr
* @param sort
* @param selctg
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param sdim
* @param alphar
* @param alphai
* @param beta
* @param vsl
* @param ldvsl
* @param vsr
* @param ldvsr
* @param work
* @param lwork
* @param bwork
* @param info
*
*/
abstract public void sgges(java.lang.String jobvsl, java.lang.String jobvsr, java.lang.String sort, java.lang.Object selctg, int n, float[] a, int lda, float[] b, int ldb, org.netlib.util.intW sdim, float[] alphar, float[] alphai, float[] beta, float[] vsl, int ldvsl, float[] vsr, int ldvsr, float[] work, int lwork, boolean[] bwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGGES computes for a pair of N-by-N real nonsymmetric matrices (A,B),
* the generalized eigenvalues, the generalized real Schur form (S,T),
* optionally, the left and/or right matrices of Schur vectors (VSL and
* VSR). This gives the generalized Schur factorization
*
* (A,B) = ( (VSL)*S*(VSR)**T, (VSL)*T*(VSR)**T )
*
* Optionally, it also orders the eigenvalues so that a selected cluster
* of eigenvalues appears in the leading diagonal blocks of the upper
* quasi-triangular matrix S and the upper triangular matrix T.The
* leading columns of VSL and VSR then form an orthonormal basis for the
* corresponding left and right eigenspaces (deflating subspaces).
*
* (If only the generalized eigenvalues are needed, use the driver
* SGGEV instead, which is faster.)
*
* A generalized eigenvalue for a pair of matrices (A,B) is a scalar w
* or a ratio alpha/beta = w, such that A - w*B is singular. It is
* usually represented as the pair (alpha,beta), as there is a
* reasonable interpretation for beta=0 or both being zero.
*
* A pair of matrices (S,T) is in generalized real Schur form if T is
* upper triangular with non-negative diagonal and S is block upper
* triangular with 1-by-1 and 2-by-2 blocks. 1-by-1 blocks correspond
* to real generalized eigenvalues, while 2-by-2 blocks of S will be
* "standardized" by making the corresponding elements of T have the
* form:
* [ a 0 ]
* [ 0 b ]
*
* and the pair of corresponding 2-by-2 blocks in S and T will have a
* complex conjugate pair of generalized eigenvalues.
*
*
* Arguments
* =========
*
* JOBVSL (input) CHARACTER*1
* = 'N': do not compute the left Schur vectors;
* = 'V': compute the left Schur vectors.
*
* JOBVSR (input) CHARACTER*1
* = 'N': do not compute the right Schur vectors;
* = 'V': compute the right Schur vectors.
*
* SORT (input) CHARACTER*1
* Specifies whether or not to order the eigenvalues on the
* diagonal of the generalized Schur form.
* = 'N': Eigenvalues are not ordered;
* = 'S': Eigenvalues are ordered (see SELCTG);
*
* SELCTG (external procedure) LOGICAL FUNCTION of three REAL arguments
* SELCTG must be declared EXTERNAL in the calling subroutine.
* If SORT = 'N', SELCTG is not referenced.
* If SORT = 'S', SELCTG is used to select eigenvalues to sort
* to the top left of the Schur form.
* An eigenvalue (ALPHAR(j)+ALPHAI(j))/BETA(j) is selected if
* SELCTG(ALPHAR(j),ALPHAI(j),BETA(j)) is true; i.e. if either
* one of a complex conjugate pair of eigenvalues is selected,
* then both complex eigenvalues are selected.
*
* Note that in the ill-conditioned case, a selected complex
* eigenvalue may no longer satisfy SELCTG(ALPHAR(j),ALPHAI(j),
* BETA(j)) = .TRUE. after ordering. INFO is to be set to N+2
* in this case.
*
* N (input) INTEGER
* The order of the matrices A, B, VSL, and VSR. N >= 0.
*
* A (input/output) REAL array, dimension (LDA, N)
* On entry, the first of the pair of matrices.
* On exit, A has been overwritten by its generalized Schur
* form S.
*
* LDA (input) INTEGER
* The leading dimension of A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension (LDB, N)
* On entry, the second of the pair of matrices.
* On exit, B has been overwritten by its generalized Schur
* form T.
*
* LDB (input) INTEGER
* The leading dimension of B. LDB >= max(1,N).
*
* SDIM (output) INTEGER
* If SORT = 'N', SDIM = 0.
* If SORT = 'S', SDIM = number of eigenvalues (after sorting)
* for which SELCTG is true. (Complex conjugate pairs for which
* SELCTG is true for either eigenvalue count as 2.)
*
* ALPHAR (output) REAL array, dimension (N)
* ALPHAI (output) REAL array, dimension (N)
* BETA (output) REAL array, dimension (N)
* On exit, (ALPHAR(j) + ALPHAI(j)*i)/BETA(j), j=1,...,N, will
* be the generalized eigenvalues. ALPHAR(j) + ALPHAI(j)*i,
* and BETA(j),j=1,...,N are the diagonals of the complex Schur
* form (S,T) that would result if the 2-by-2 diagonal blocks of
* the real Schur form of (A,B) were further reduced to
* triangular form using 2-by-2 complex unitary transformations.
* If ALPHAI(j) is zero, then the j-th eigenvalue is real; if
* positive, then the j-th and (j+1)-st eigenvalues are a
* complex conjugate pair, with ALPHAI(j+1) negative.
*
* Note: the quotients ALPHAR(j)/BETA(j) and ALPHAI(j)/BETA(j)
* may easily over- or underflow, and BETA(j) may even be zero.
* Thus, the user should avoid naively computing the ratio.
* However, ALPHAR and ALPHAI will be always less than and
* usually comparable with norm(A) in magnitude, and BETA always
* less than and usually comparable with norm(B).
*
* VSL (output) REAL array, dimension (LDVSL,N)
* If JOBVSL = 'V', VSL will contain the left Schur vectors.
* Not referenced if JOBVSL = 'N'.
*
* LDVSL (input) INTEGER
* The leading dimension of the matrix VSL. LDVSL >=1, and
* if JOBVSL = 'V', LDVSL >= N.
*
* VSR (output) REAL array, dimension (LDVSR,N)
* If JOBVSR = 'V', VSR will contain the right Schur vectors.
* Not referenced if JOBVSR = 'N'.
*
* LDVSR (input) INTEGER
* The leading dimension of the matrix VSR. LDVSR >= 1, and
* if JOBVSR = 'V', LDVSR >= N.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If N = 0, LWORK >= 1, else LWORK >= max(8*N,6*N+16).
* For good performance , LWORK must generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* BWORK (workspace) LOGICAL array, dimension (N)
* Not referenced if SORT = 'N'.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1,...,N:
* The QZ iteration failed. (A,B) are not in Schur
* form, but ALPHAR(j), ALPHAI(j), and BETA(j) should
* be correct for j=INFO+1,...,N.
* > N: =N+1: other than QZ iteration failed in SHGEQZ.
* =N+2: after reordering, roundoff changed values of
* some complex eigenvalues so that leading
* eigenvalues in the Generalized Schur form no
* longer satisfy SELCTG=.TRUE. This could also
* be caused due to scaling.
* =N+3: reordering failed in STGSEN.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobvsl
* @param jobvsr
* @param sort
* @param selctg
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param sdim
* @param alphar
* @param _alphar_offset
* @param alphai
* @param _alphai_offset
* @param beta
* @param _beta_offset
* @param vsl
* @param _vsl_offset
* @param ldvsl
* @param vsr
* @param _vsr_offset
* @param ldvsr
* @param work
* @param _work_offset
* @param lwork
* @param bwork
* @param _bwork_offset
* @param info
*
*/
abstract public void sgges(java.lang.String jobvsl, java.lang.String jobvsr, java.lang.String sort, java.lang.Object selctg, int n, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, org.netlib.util.intW sdim, float[] alphar, int _alphar_offset, float[] alphai, int _alphai_offset, float[] beta, int _beta_offset, float[] vsl, int _vsl_offset, int ldvsl, float[] vsr, int _vsr_offset, int ldvsr, float[] work, int _work_offset, int lwork, boolean[] bwork, int _bwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGGESX computes for a pair of N-by-N real nonsymmetric matrices
* (A,B), the generalized eigenvalues, the real Schur form (S,T), and,
* optionally, the left and/or right matrices of Schur vectors (VSL and
* VSR). This gives the generalized Schur factorization
*
* (A,B) = ( (VSL) S (VSR)**T, (VSL) T (VSR)**T )
*
* Optionally, it also orders the eigenvalues so that a selected cluster
* of eigenvalues appears in the leading diagonal blocks of the upper
* quasi-triangular matrix S and the upper triangular matrix T; computes
* a reciprocal condition number for the average of the selected
* eigenvalues (RCONDE); and computes a reciprocal condition number for
* the right and left deflating subspaces corresponding to the selected
* eigenvalues (RCONDV). The leading columns of VSL and VSR then form
* an orthonormal basis for the corresponding left and right eigenspaces
* (deflating subspaces).
*
* A generalized eigenvalue for a pair of matrices (A,B) is a scalar w
* or a ratio alpha/beta = w, such that A - w*B is singular. It is
* usually represented as the pair (alpha,beta), as there is a
* reasonable interpretation for beta=0 or for both being zero.
*
* A pair of matrices (S,T) is in generalized real Schur form if T is
* upper triangular with non-negative diagonal and S is block upper
* triangular with 1-by-1 and 2-by-2 blocks. 1-by-1 blocks correspond
* to real generalized eigenvalues, while 2-by-2 blocks of S will be
* "standardized" by making the corresponding elements of T have the
* form:
* [ a 0 ]
* [ 0 b ]
*
* and the pair of corresponding 2-by-2 blocks in S and T will have a
* complex conjugate pair of generalized eigenvalues.
*
*
* Arguments
* =========
*
* JOBVSL (input) CHARACTER*1
* = 'N': do not compute the left Schur vectors;
* = 'V': compute the left Schur vectors.
*
* JOBVSR (input) CHARACTER*1
* = 'N': do not compute the right Schur vectors;
* = 'V': compute the right Schur vectors.
*
* SORT (input) CHARACTER*1
* Specifies whether or not to order the eigenvalues on the
* diagonal of the generalized Schur form.
* = 'N': Eigenvalues are not ordered;
* = 'S': Eigenvalues are ordered (see SELCTG).
*
* SELCTG (external procedure) LOGICAL FUNCTION of three REAL arguments
* SELCTG must be declared EXTERNAL in the calling subroutine.
* If SORT = 'N', SELCTG is not referenced.
* If SORT = 'S', SELCTG is used to select eigenvalues to sort
* to the top left of the Schur form.
* An eigenvalue (ALPHAR(j)+ALPHAI(j))/BETA(j) is selected if
* SELCTG(ALPHAR(j),ALPHAI(j),BETA(j)) is true; i.e. if either
* one of a complex conjugate pair of eigenvalues is selected,
* then both complex eigenvalues are selected.
* Note that a selected complex eigenvalue may no longer satisfy
* SELCTG(ALPHAR(j),ALPHAI(j),BETA(j)) = .TRUE. after ordering,
* since ordering may change the value of complex eigenvalues
* (especially if the eigenvalue is ill-conditioned), in this
* case INFO is set to N+3.
*
* SENSE (input) CHARACTER*1
* Determines which reciprocal condition numbers are computed.
* = 'N' : None are computed;
* = 'E' : Computed for average of selected eigenvalues only;
* = 'V' : Computed for selected deflating subspaces only;
* = 'B' : Computed for both.
* If SENSE = 'E', 'V', or 'B', SORT must equal 'S'.
*
* N (input) INTEGER
* The order of the matrices A, B, VSL, and VSR. N >= 0.
*
* A (input/output) REAL array, dimension (LDA, N)
* On entry, the first of the pair of matrices.
* On exit, A has been overwritten by its generalized Schur
* form S.
*
* LDA (input) INTEGER
* The leading dimension of A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension (LDB, N)
* On entry, the second of the pair of matrices.
* On exit, B has been overwritten by its generalized Schur
* form T.
*
* LDB (input) INTEGER
* The leading dimension of B. LDB >= max(1,N).
*
* SDIM (output) INTEGER
* If SORT = 'N', SDIM = 0.
* If SORT = 'S', SDIM = number of eigenvalues (after sorting)
* for which SELCTG is true. (Complex conjugate pairs for which
* SELCTG is true for either eigenvalue count as 2.)
*
* ALPHAR (output) REAL array, dimension (N)
* ALPHAI (output) REAL array, dimension (N)
* BETA (output) REAL array, dimension (N)
* On exit, (ALPHAR(j) + ALPHAI(j)*i)/BETA(j), j=1,...,N, will
* be the generalized eigenvalues. ALPHAR(j) + ALPHAI(j)*i
* and BETA(j),j=1,...,N are the diagonals of the complex Schur
* form (S,T) that would result if the 2-by-2 diagonal blocks of
* the real Schur form of (A,B) were further reduced to
* triangular form using 2-by-2 complex unitary transformations.
* If ALPHAI(j) is zero, then the j-th eigenvalue is real; if
* positive, then the j-th and (j+1)-st eigenvalues are a
* complex conjugate pair, with ALPHAI(j+1) negative.
*
* Note: the quotients ALPHAR(j)/BETA(j) and ALPHAI(j)/BETA(j)
* may easily over- or underflow, and BETA(j) may even be zero.
* Thus, the user should avoid naively computing the ratio.
* However, ALPHAR and ALPHAI will be always less than and
* usually comparable with norm(A) in magnitude, and BETA always
* less than and usually comparable with norm(B).
*
* VSL (output) REAL array, dimension (LDVSL,N)
* If JOBVSL = 'V', VSL will contain the left Schur vectors.
* Not referenced if JOBVSL = 'N'.
*
* LDVSL (input) INTEGER
* The leading dimension of the matrix VSL. LDVSL >=1, and
* if JOBVSL = 'V', LDVSL >= N.
*
* VSR (output) REAL array, dimension (LDVSR,N)
* If JOBVSR = 'V', VSR will contain the right Schur vectors.
* Not referenced if JOBVSR = 'N'.
*
* LDVSR (input) INTEGER
* The leading dimension of the matrix VSR. LDVSR >= 1, and
* if JOBVSR = 'V', LDVSR >= N.
*
* RCONDE (output) REAL array, dimension ( 2 )
* If SENSE = 'E' or 'B', RCONDE(1) and RCONDE(2) contain the
* reciprocal condition numbers for the average of the selected
* eigenvalues.
* Not referenced if SENSE = 'N' or 'V'.
*
* RCONDV (output) REAL array, dimension ( 2 )
* If SENSE = 'V' or 'B', RCONDV(1) and RCONDV(2) contain the
* reciprocal condition numbers for the selected deflating
* subspaces.
* Not referenced if SENSE = 'N' or 'E'.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If N = 0, LWORK >= 1, else if SENSE = 'E', 'V', or 'B',
* LWORK >= max( 8*N, 6*N+16, 2*SDIM*(N-SDIM) ), else
* LWORK >= max( 8*N, 6*N+16 ).
* Note that 2*SDIM*(N-SDIM) <= N*N/2.
* Note also that an error is only returned if
* LWORK < max( 8*N, 6*N+16), but if SENSE = 'E' or 'V' or 'B'
* this may not be large enough.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the bound on the optimal size of the WORK
* array and the minimum size of the IWORK array, returns these
* values as the first entries of the WORK and IWORK arrays, and
* no error message related to LWORK or LIWORK is issued by
* XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the minimum LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If SENSE = 'N' or N = 0, LIWORK >= 1, otherwise
* LIWORK >= N+6.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the bound on the optimal size of the
* WORK array and the minimum size of the IWORK array, returns
* these values as the first entries of the WORK and IWORK
* arrays, and no error message related to LWORK or LIWORK is
* issued by XERBLA.
*
* BWORK (workspace) LOGICAL array, dimension (N)
* Not referenced if SORT = 'N'.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1,...,N:
* The QZ iteration failed. (A,B) are not in Schur
* form, but ALPHAR(j), ALPHAI(j), and BETA(j) should
* be correct for j=INFO+1,...,N.
* > N: =N+1: other than QZ iteration failed in SHGEQZ
* =N+2: after reordering, roundoff changed values of
* some complex eigenvalues so that leading
* eigenvalues in the Generalized Schur form no
* longer satisfy SELCTG=.TRUE. This could also
* be caused due to scaling.
* =N+3: reordering failed in STGSEN.
*
* Further details
* ===============
*
* An approximate (asymptotic) bound on the average absolute error of
* the selected eigenvalues is
*
* EPS * norm((A, B)) / RCONDE( 1 ).
*
* An approximate (asymptotic) bound on the maximum angular error in
* the computed deflating subspaces is
*
* EPS * norm((A, B)) / RCONDV( 2 ).
*
* See LAPACK User's Guide, section 4.11 for more information.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobvsl
* @param jobvsr
* @param sort
* @param selctg
* @param sense
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param sdim
* @param alphar
* @param alphai
* @param beta
* @param vsl
* @param ldvsl
* @param vsr
* @param ldvsr
* @param rconde
* @param rcondv
* @param work
* @param lwork
* @param iwork
* @param liwork
* @param bwork
* @param info
*
*/
abstract public void sggesx(java.lang.String jobvsl, java.lang.String jobvsr, java.lang.String sort, java.lang.Object selctg, java.lang.String sense, int n, float[] a, int lda, float[] b, int ldb, org.netlib.util.intW sdim, float[] alphar, float[] alphai, float[] beta, float[] vsl, int ldvsl, float[] vsr, int ldvsr, float[] rconde, float[] rcondv, float[] work, int lwork, int[] iwork, int liwork, boolean[] bwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGGESX computes for a pair of N-by-N real nonsymmetric matrices
* (A,B), the generalized eigenvalues, the real Schur form (S,T), and,
* optionally, the left and/or right matrices of Schur vectors (VSL and
* VSR). This gives the generalized Schur factorization
*
* (A,B) = ( (VSL) S (VSR)**T, (VSL) T (VSR)**T )
*
* Optionally, it also orders the eigenvalues so that a selected cluster
* of eigenvalues appears in the leading diagonal blocks of the upper
* quasi-triangular matrix S and the upper triangular matrix T; computes
* a reciprocal condition number for the average of the selected
* eigenvalues (RCONDE); and computes a reciprocal condition number for
* the right and left deflating subspaces corresponding to the selected
* eigenvalues (RCONDV). The leading columns of VSL and VSR then form
* an orthonormal basis for the corresponding left and right eigenspaces
* (deflating subspaces).
*
* A generalized eigenvalue for a pair of matrices (A,B) is a scalar w
* or a ratio alpha/beta = w, such that A - w*B is singular. It is
* usually represented as the pair (alpha,beta), as there is a
* reasonable interpretation for beta=0 or for both being zero.
*
* A pair of matrices (S,T) is in generalized real Schur form if T is
* upper triangular with non-negative diagonal and S is block upper
* triangular with 1-by-1 and 2-by-2 blocks. 1-by-1 blocks correspond
* to real generalized eigenvalues, while 2-by-2 blocks of S will be
* "standardized" by making the corresponding elements of T have the
* form:
* [ a 0 ]
* [ 0 b ]
*
* and the pair of corresponding 2-by-2 blocks in S and T will have a
* complex conjugate pair of generalized eigenvalues.
*
*
* Arguments
* =========
*
* JOBVSL (input) CHARACTER*1
* = 'N': do not compute the left Schur vectors;
* = 'V': compute the left Schur vectors.
*
* JOBVSR (input) CHARACTER*1
* = 'N': do not compute the right Schur vectors;
* = 'V': compute the right Schur vectors.
*
* SORT (input) CHARACTER*1
* Specifies whether or not to order the eigenvalues on the
* diagonal of the generalized Schur form.
* = 'N': Eigenvalues are not ordered;
* = 'S': Eigenvalues are ordered (see SELCTG).
*
* SELCTG (external procedure) LOGICAL FUNCTION of three REAL arguments
* SELCTG must be declared EXTERNAL in the calling subroutine.
* If SORT = 'N', SELCTG is not referenced.
* If SORT = 'S', SELCTG is used to select eigenvalues to sort
* to the top left of the Schur form.
* An eigenvalue (ALPHAR(j)+ALPHAI(j))/BETA(j) is selected if
* SELCTG(ALPHAR(j),ALPHAI(j),BETA(j)) is true; i.e. if either
* one of a complex conjugate pair of eigenvalues is selected,
* then both complex eigenvalues are selected.
* Note that a selected complex eigenvalue may no longer satisfy
* SELCTG(ALPHAR(j),ALPHAI(j),BETA(j)) = .TRUE. after ordering,
* since ordering may change the value of complex eigenvalues
* (especially if the eigenvalue is ill-conditioned), in this
* case INFO is set to N+3.
*
* SENSE (input) CHARACTER*1
* Determines which reciprocal condition numbers are computed.
* = 'N' : None are computed;
* = 'E' : Computed for average of selected eigenvalues only;
* = 'V' : Computed for selected deflating subspaces only;
* = 'B' : Computed for both.
* If SENSE = 'E', 'V', or 'B', SORT must equal 'S'.
*
* N (input) INTEGER
* The order of the matrices A, B, VSL, and VSR. N >= 0.
*
* A (input/output) REAL array, dimension (LDA, N)
* On entry, the first of the pair of matrices.
* On exit, A has been overwritten by its generalized Schur
* form S.
*
* LDA (input) INTEGER
* The leading dimension of A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension (LDB, N)
* On entry, the second of the pair of matrices.
* On exit, B has been overwritten by its generalized Schur
* form T.
*
* LDB (input) INTEGER
* The leading dimension of B. LDB >= max(1,N).
*
* SDIM (output) INTEGER
* If SORT = 'N', SDIM = 0.
* If SORT = 'S', SDIM = number of eigenvalues (after sorting)
* for which SELCTG is true. (Complex conjugate pairs for which
* SELCTG is true for either eigenvalue count as 2.)
*
* ALPHAR (output) REAL array, dimension (N)
* ALPHAI (output) REAL array, dimension (N)
* BETA (output) REAL array, dimension (N)
* On exit, (ALPHAR(j) + ALPHAI(j)*i)/BETA(j), j=1,...,N, will
* be the generalized eigenvalues. ALPHAR(j) + ALPHAI(j)*i
* and BETA(j),j=1,...,N are the diagonals of the complex Schur
* form (S,T) that would result if the 2-by-2 diagonal blocks of
* the real Schur form of (A,B) were further reduced to
* triangular form using 2-by-2 complex unitary transformations.
* If ALPHAI(j) is zero, then the j-th eigenvalue is real; if
* positive, then the j-th and (j+1)-st eigenvalues are a
* complex conjugate pair, with ALPHAI(j+1) negative.
*
* Note: the quotients ALPHAR(j)/BETA(j) and ALPHAI(j)/BETA(j)
* may easily over- or underflow, and BETA(j) may even be zero.
* Thus, the user should avoid naively computing the ratio.
* However, ALPHAR and ALPHAI will be always less than and
* usually comparable with norm(A) in magnitude, and BETA always
* less than and usually comparable with norm(B).
*
* VSL (output) REAL array, dimension (LDVSL,N)
* If JOBVSL = 'V', VSL will contain the left Schur vectors.
* Not referenced if JOBVSL = 'N'.
*
* LDVSL (input) INTEGER
* The leading dimension of the matrix VSL. LDVSL >=1, and
* if JOBVSL = 'V', LDVSL >= N.
*
* VSR (output) REAL array, dimension (LDVSR,N)
* If JOBVSR = 'V', VSR will contain the right Schur vectors.
* Not referenced if JOBVSR = 'N'.
*
* LDVSR (input) INTEGER
* The leading dimension of the matrix VSR. LDVSR >= 1, and
* if JOBVSR = 'V', LDVSR >= N.
*
* RCONDE (output) REAL array, dimension ( 2 )
* If SENSE = 'E' or 'B', RCONDE(1) and RCONDE(2) contain the
* reciprocal condition numbers for the average of the selected
* eigenvalues.
* Not referenced if SENSE = 'N' or 'V'.
*
* RCONDV (output) REAL array, dimension ( 2 )
* If SENSE = 'V' or 'B', RCONDV(1) and RCONDV(2) contain the
* reciprocal condition numbers for the selected deflating
* subspaces.
* Not referenced if SENSE = 'N' or 'E'.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If N = 0, LWORK >= 1, else if SENSE = 'E', 'V', or 'B',
* LWORK >= max( 8*N, 6*N+16, 2*SDIM*(N-SDIM) ), else
* LWORK >= max( 8*N, 6*N+16 ).
* Note that 2*SDIM*(N-SDIM) <= N*N/2.
* Note also that an error is only returned if
* LWORK < max( 8*N, 6*N+16), but if SENSE = 'E' or 'V' or 'B'
* this may not be large enough.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the bound on the optimal size of the WORK
* array and the minimum size of the IWORK array, returns these
* values as the first entries of the WORK and IWORK arrays, and
* no error message related to LWORK or LIWORK is issued by
* XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the minimum LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If SENSE = 'N' or N = 0, LIWORK >= 1, otherwise
* LIWORK >= N+6.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the bound on the optimal size of the
* WORK array and the minimum size of the IWORK array, returns
* these values as the first entries of the WORK and IWORK
* arrays, and no error message related to LWORK or LIWORK is
* issued by XERBLA.
*
* BWORK (workspace) LOGICAL array, dimension (N)
* Not referenced if SORT = 'N'.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1,...,N:
* The QZ iteration failed. (A,B) are not in Schur
* form, but ALPHAR(j), ALPHAI(j), and BETA(j) should
* be correct for j=INFO+1,...,N.
* > N: =N+1: other than QZ iteration failed in SHGEQZ
* =N+2: after reordering, roundoff changed values of
* some complex eigenvalues so that leading
* eigenvalues in the Generalized Schur form no
* longer satisfy SELCTG=.TRUE. This could also
* be caused due to scaling.
* =N+3: reordering failed in STGSEN.
*
* Further details
* ===============
*
* An approximate (asymptotic) bound on the average absolute error of
* the selected eigenvalues is
*
* EPS * norm((A, B)) / RCONDE( 1 ).
*
* An approximate (asymptotic) bound on the maximum angular error in
* the computed deflating subspaces is
*
* EPS * norm((A, B)) / RCONDV( 2 ).
*
* See LAPACK User's Guide, section 4.11 for more information.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobvsl
* @param jobvsr
* @param sort
* @param selctg
* @param sense
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param sdim
* @param alphar
* @param _alphar_offset
* @param alphai
* @param _alphai_offset
* @param beta
* @param _beta_offset
* @param vsl
* @param _vsl_offset
* @param ldvsl
* @param vsr
* @param _vsr_offset
* @param ldvsr
* @param rconde
* @param _rconde_offset
* @param rcondv
* @param _rcondv_offset
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param liwork
* @param bwork
* @param _bwork_offset
* @param info
*
*/
abstract public void sggesx(java.lang.String jobvsl, java.lang.String jobvsr, java.lang.String sort, java.lang.Object selctg, java.lang.String sense, int n, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, org.netlib.util.intW sdim, float[] alphar, int _alphar_offset, float[] alphai, int _alphai_offset, float[] beta, int _beta_offset, float[] vsl, int _vsl_offset, int ldvsl, float[] vsr, int _vsr_offset, int ldvsr, float[] rconde, int _rconde_offset, float[] rcondv, int _rcondv_offset, float[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int liwork, boolean[] bwork, int _bwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGGEV computes for a pair of N-by-N real nonsymmetric matrices (A,B)
* the generalized eigenvalues, and optionally, the left and/or right
* generalized eigenvectors.
*
* A generalized eigenvalue for a pair of matrices (A,B) is a scalar
* lambda or a ratio alpha/beta = lambda, such that A - lambda*B is
* singular. It is usually represented as the pair (alpha,beta), as
* there is a reasonable interpretation for beta=0, and even for both
* being zero.
*
* The right eigenvector v(j) corresponding to the eigenvalue lambda(j)
* of (A,B) satisfies
*
* A * v(j) = lambda(j) * B * v(j).
*
* The left eigenvector u(j) corresponding to the eigenvalue lambda(j)
* of (A,B) satisfies
*
* u(j)**H * A = lambda(j) * u(j)**H * B .
*
* where u(j)**H is the conjugate-transpose of u(j).
*
*
* Arguments
* =========
*
* JOBVL (input) CHARACTER*1
* = 'N': do not compute the left generalized eigenvectors;
* = 'V': compute the left generalized eigenvectors.
*
* JOBVR (input) CHARACTER*1
* = 'N': do not compute the right generalized eigenvectors;
* = 'V': compute the right generalized eigenvectors.
*
* N (input) INTEGER
* The order of the matrices A, B, VL, and VR. N >= 0.
*
* A (input/output) REAL array, dimension (LDA, N)
* On entry, the matrix A in the pair (A,B).
* On exit, A has been overwritten.
*
* LDA (input) INTEGER
* The leading dimension of A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension (LDB, N)
* On entry, the matrix B in the pair (A,B).
* On exit, B has been overwritten.
*
* LDB (input) INTEGER
* The leading dimension of B. LDB >= max(1,N).
*
* ALPHAR (output) REAL array, dimension (N)
* ALPHAI (output) REAL array, dimension (N)
* BETA (output) REAL array, dimension (N)
* On exit, (ALPHAR(j) + ALPHAI(j)*i)/BETA(j), j=1,...,N, will
* be the generalized eigenvalues. If ALPHAI(j) is zero, then
* the j-th eigenvalue is real; if positive, then the j-th and
* (j+1)-st eigenvalues are a complex conjugate pair, with
* ALPHAI(j+1) negative.
*
* Note: the quotients ALPHAR(j)/BETA(j) and ALPHAI(j)/BETA(j)
* may easily over- or underflow, and BETA(j) may even be zero.
* Thus, the user should avoid naively computing the ratio
* alpha/beta. However, ALPHAR and ALPHAI will be always less
* than and usually comparable with norm(A) in magnitude, and
* BETA always less than and usually comparable with norm(B).
*
* VL (output) REAL array, dimension (LDVL,N)
* If JOBVL = 'V', the left eigenvectors u(j) are stored one
* after another in the columns of VL, in the same order as
* their eigenvalues. If the j-th eigenvalue is real, then
* u(j) = VL(:,j), the j-th column of VL. If the j-th and
* (j+1)-th eigenvalues form a complex conjugate pair, then
* u(j) = VL(:,j)+i*VL(:,j+1) and u(j+1) = VL(:,j)-i*VL(:,j+1).
* Each eigenvector is scaled so the largest component has
* abs(real part)+abs(imag. part)=1.
* Not referenced if JOBVL = 'N'.
*
* LDVL (input) INTEGER
* The leading dimension of the matrix VL. LDVL >= 1, and
* if JOBVL = 'V', LDVL >= N.
*
* VR (output) REAL array, dimension (LDVR,N)
* If JOBVR = 'V', the right eigenvectors v(j) are stored one
* after another in the columns of VR, in the same order as
* their eigenvalues. If the j-th eigenvalue is real, then
* v(j) = VR(:,j), the j-th column of VR. If the j-th and
* (j+1)-th eigenvalues form a complex conjugate pair, then
* v(j) = VR(:,j)+i*VR(:,j+1) and v(j+1) = VR(:,j)-i*VR(:,j+1).
* Each eigenvector is scaled so the largest component has
* abs(real part)+abs(imag. part)=1.
* Not referenced if JOBVR = 'N'.
*
* LDVR (input) INTEGER
* The leading dimension of the matrix VR. LDVR >= 1, and
* if JOBVR = 'V', LDVR >= N.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,8*N).
* For good performance, LWORK must generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1,...,N:
* The QZ iteration failed. No eigenvectors have been
* calculated, but ALPHAR(j), ALPHAI(j), and BETA(j)
* should be correct for j=INFO+1,...,N.
* > N: =N+1: other than QZ iteration failed in SHGEQZ.
* =N+2: error return from STGEVC.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobvl
* @param jobvr
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param alphar
* @param alphai
* @param beta
* @param vl
* @param ldvl
* @param vr
* @param ldvr
* @param work
* @param lwork
* @param info
*
*/
abstract public void sggev(java.lang.String jobvl, java.lang.String jobvr, int n, float[] a, int lda, float[] b, int ldb, float[] alphar, float[] alphai, float[] beta, float[] vl, int ldvl, float[] vr, int ldvr, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGGEV computes for a pair of N-by-N real nonsymmetric matrices (A,B)
* the generalized eigenvalues, and optionally, the left and/or right
* generalized eigenvectors.
*
* A generalized eigenvalue for a pair of matrices (A,B) is a scalar
* lambda or a ratio alpha/beta = lambda, such that A - lambda*B is
* singular. It is usually represented as the pair (alpha,beta), as
* there is a reasonable interpretation for beta=0, and even for both
* being zero.
*
* The right eigenvector v(j) corresponding to the eigenvalue lambda(j)
* of (A,B) satisfies
*
* A * v(j) = lambda(j) * B * v(j).
*
* The left eigenvector u(j) corresponding to the eigenvalue lambda(j)
* of (A,B) satisfies
*
* u(j)**H * A = lambda(j) * u(j)**H * B .
*
* where u(j)**H is the conjugate-transpose of u(j).
*
*
* Arguments
* =========
*
* JOBVL (input) CHARACTER*1
* = 'N': do not compute the left generalized eigenvectors;
* = 'V': compute the left generalized eigenvectors.
*
* JOBVR (input) CHARACTER*1
* = 'N': do not compute the right generalized eigenvectors;
* = 'V': compute the right generalized eigenvectors.
*
* N (input) INTEGER
* The order of the matrices A, B, VL, and VR. N >= 0.
*
* A (input/output) REAL array, dimension (LDA, N)
* On entry, the matrix A in the pair (A,B).
* On exit, A has been overwritten.
*
* LDA (input) INTEGER
* The leading dimension of A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension (LDB, N)
* On entry, the matrix B in the pair (A,B).
* On exit, B has been overwritten.
*
* LDB (input) INTEGER
* The leading dimension of B. LDB >= max(1,N).
*
* ALPHAR (output) REAL array, dimension (N)
* ALPHAI (output) REAL array, dimension (N)
* BETA (output) REAL array, dimension (N)
* On exit, (ALPHAR(j) + ALPHAI(j)*i)/BETA(j), j=1,...,N, will
* be the generalized eigenvalues. If ALPHAI(j) is zero, then
* the j-th eigenvalue is real; if positive, then the j-th and
* (j+1)-st eigenvalues are a complex conjugate pair, with
* ALPHAI(j+1) negative.
*
* Note: the quotients ALPHAR(j)/BETA(j) and ALPHAI(j)/BETA(j)
* may easily over- or underflow, and BETA(j) may even be zero.
* Thus, the user should avoid naively computing the ratio
* alpha/beta. However, ALPHAR and ALPHAI will be always less
* than and usually comparable with norm(A) in magnitude, and
* BETA always less than and usually comparable with norm(B).
*
* VL (output) REAL array, dimension (LDVL,N)
* If JOBVL = 'V', the left eigenvectors u(j) are stored one
* after another in the columns of VL, in the same order as
* their eigenvalues. If the j-th eigenvalue is real, then
* u(j) = VL(:,j), the j-th column of VL. If the j-th and
* (j+1)-th eigenvalues form a complex conjugate pair, then
* u(j) = VL(:,j)+i*VL(:,j+1) and u(j+1) = VL(:,j)-i*VL(:,j+1).
* Each eigenvector is scaled so the largest component has
* abs(real part)+abs(imag. part)=1.
* Not referenced if JOBVL = 'N'.
*
* LDVL (input) INTEGER
* The leading dimension of the matrix VL. LDVL >= 1, and
* if JOBVL = 'V', LDVL >= N.
*
* VR (output) REAL array, dimension (LDVR,N)
* If JOBVR = 'V', the right eigenvectors v(j) are stored one
* after another in the columns of VR, in the same order as
* their eigenvalues. If the j-th eigenvalue is real, then
* v(j) = VR(:,j), the j-th column of VR. If the j-th and
* (j+1)-th eigenvalues form a complex conjugate pair, then
* v(j) = VR(:,j)+i*VR(:,j+1) and v(j+1) = VR(:,j)-i*VR(:,j+1).
* Each eigenvector is scaled so the largest component has
* abs(real part)+abs(imag. part)=1.
* Not referenced if JOBVR = 'N'.
*
* LDVR (input) INTEGER
* The leading dimension of the matrix VR. LDVR >= 1, and
* if JOBVR = 'V', LDVR >= N.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,8*N).
* For good performance, LWORK must generally be larger.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1,...,N:
* The QZ iteration failed. No eigenvectors have been
* calculated, but ALPHAR(j), ALPHAI(j), and BETA(j)
* should be correct for j=INFO+1,...,N.
* > N: =N+1: other than QZ iteration failed in SHGEQZ.
* =N+2: error return from STGEVC.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobvl
* @param jobvr
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param alphar
* @param _alphar_offset
* @param alphai
* @param _alphai_offset
* @param beta
* @param _beta_offset
* @param vl
* @param _vl_offset
* @param ldvl
* @param vr
* @param _vr_offset
* @param ldvr
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sggev(java.lang.String jobvl, java.lang.String jobvr, int n, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, float[] alphar, int _alphar_offset, float[] alphai, int _alphai_offset, float[] beta, int _beta_offset, float[] vl, int _vl_offset, int ldvl, float[] vr, int _vr_offset, int ldvr, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGGEVX computes for a pair of N-by-N real nonsymmetric matrices (A,B)
* the generalized eigenvalues, and optionally, the left and/or right
* generalized eigenvectors.
*
* Optionally also, it computes a balancing transformation to improve
* the conditioning of the eigenvalues and eigenvectors (ILO, IHI,
* LSCALE, RSCALE, ABNRM, and BBNRM), reciprocal condition numbers for
* the eigenvalues (RCONDE), and reciprocal condition numbers for the
* right eigenvectors (RCONDV).
*
* A generalized eigenvalue for a pair of matrices (A,B) is a scalar
* lambda or a ratio alpha/beta = lambda, such that A - lambda*B is
* singular. It is usually represented as the pair (alpha,beta), as
* there is a reasonable interpretation for beta=0, and even for both
* being zero.
*
* The right eigenvector v(j) corresponding to the eigenvalue lambda(j)
* of (A,B) satisfies
*
* A * v(j) = lambda(j) * B * v(j) .
*
* The left eigenvector u(j) corresponding to the eigenvalue lambda(j)
* of (A,B) satisfies
*
* u(j)**H * A = lambda(j) * u(j)**H * B.
*
* where u(j)**H is the conjugate-transpose of u(j).
*
*
* Arguments
* =========
*
* BALANC (input) CHARACTER*1
* Specifies the balance option to be performed.
* = 'N': do not diagonally scale or permute;
* = 'P': permute only;
* = 'S': scale only;
* = 'B': both permute and scale.
* Computed reciprocal condition numbers will be for the
* matrices after permuting and/or balancing. Permuting does
* not change condition numbers (in exact arithmetic), but
* balancing does.
*
* JOBVL (input) CHARACTER*1
* = 'N': do not compute the left generalized eigenvectors;
* = 'V': compute the left generalized eigenvectors.
*
* JOBVR (input) CHARACTER*1
* = 'N': do not compute the right generalized eigenvectors;
* = 'V': compute the right generalized eigenvectors.
*
* SENSE (input) CHARACTER*1
* Determines which reciprocal condition numbers are computed.
* = 'N': none are computed;
* = 'E': computed for eigenvalues only;
* = 'V': computed for eigenvectors only;
* = 'B': computed for eigenvalues and eigenvectors.
*
* N (input) INTEGER
* The order of the matrices A, B, VL, and VR. N >= 0.
*
* A (input/output) REAL array, dimension (LDA, N)
* On entry, the matrix A in the pair (A,B).
* On exit, A has been overwritten. If JOBVL='V' or JOBVR='V'
* or both, then A contains the first part of the real Schur
* form of the "balanced" versions of the input A and B.
*
* LDA (input) INTEGER
* The leading dimension of A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension (LDB, N)
* On entry, the matrix B in the pair (A,B).
* On exit, B has been overwritten. If JOBVL='V' or JOBVR='V'
* or both, then B contains the second part of the real Schur
* form of the "balanced" versions of the input A and B.
*
* LDB (input) INTEGER
* The leading dimension of B. LDB >= max(1,N).
*
* ALPHAR (output) REAL array, dimension (N)
* ALPHAI (output) REAL array, dimension (N)
* BETA (output) REAL array, dimension (N)
* On exit, (ALPHAR(j) + ALPHAI(j)*i)/BETA(j), j=1,...,N, will
* be the generalized eigenvalues. If ALPHAI(j) is zero, then
* the j-th eigenvalue is real; if positive, then the j-th and
* (j+1)-st eigenvalues are a complex conjugate pair, with
* ALPHAI(j+1) negative.
*
* Note: the quotients ALPHAR(j)/BETA(j) and ALPHAI(j)/BETA(j)
* may easily over- or underflow, and BETA(j) may even be zero.
* Thus, the user should avoid naively computing the ratio
* ALPHA/BETA. However, ALPHAR and ALPHAI will be always less
* than and usually comparable with norm(A) in magnitude, and
* BETA always less than and usually comparable with norm(B).
*
* VL (output) REAL array, dimension (LDVL,N)
* If JOBVL = 'V', the left eigenvectors u(j) are stored one
* after another in the columns of VL, in the same order as
* their eigenvalues. If the j-th eigenvalue is real, then
* u(j) = VL(:,j), the j-th column of VL. If the j-th and
* (j+1)-th eigenvalues form a complex conjugate pair, then
* u(j) = VL(:,j)+i*VL(:,j+1) and u(j+1) = VL(:,j)-i*VL(:,j+1).
* Each eigenvector will be scaled so the largest component have
* abs(real part) + abs(imag. part) = 1.
* Not referenced if JOBVL = 'N'.
*
* LDVL (input) INTEGER
* The leading dimension of the matrix VL. LDVL >= 1, and
* if JOBVL = 'V', LDVL >= N.
*
* VR (output) REAL array, dimension (LDVR,N)
* If JOBVR = 'V', the right eigenvectors v(j) are stored one
* after another in the columns of VR, in the same order as
* their eigenvalues. If the j-th eigenvalue is real, then
* v(j) = VR(:,j), the j-th column of VR. If the j-th and
* (j+1)-th eigenvalues form a complex conjugate pair, then
* v(j) = VR(:,j)+i*VR(:,j+1) and v(j+1) = VR(:,j)-i*VR(:,j+1).
* Each eigenvector will be scaled so the largest component have
* abs(real part) + abs(imag. part) = 1.
* Not referenced if JOBVR = 'N'.
*
* LDVR (input) INTEGER
* The leading dimension of the matrix VR. LDVR >= 1, and
* if JOBVR = 'V', LDVR >= N.
*
* ILO (output) INTEGER
* IHI (output) INTEGER
* ILO and IHI are integer values such that on exit
* A(i,j) = 0 and B(i,j) = 0 if i > j and
* j = 1,...,ILO-1 or i = IHI+1,...,N.
* If BALANC = 'N' or 'S', ILO = 1 and IHI = N.
*
* LSCALE (output) REAL array, dimension (N)
* Details of the permutations and scaling factors applied
* to the left side of A and B. If PL(j) is the index of the
* row interchanged with row j, and DL(j) is the scaling
* factor applied to row j, then
* LSCALE(j) = PL(j) for j = 1,...,ILO-1
* = DL(j) for j = ILO,...,IHI
* = PL(j) for j = IHI+1,...,N.
* The order in which the interchanges are made is N to IHI+1,
* then 1 to ILO-1.
*
* RSCALE (output) REAL array, dimension (N)
* Details of the permutations and scaling factors applied
* to the right side of A and B. If PR(j) is the index of the
* column interchanged with column j, and DR(j) is the scaling
* factor applied to column j, then
* RSCALE(j) = PR(j) for j = 1,...,ILO-1
* = DR(j) for j = ILO,...,IHI
* = PR(j) for j = IHI+1,...,N
* The order in which the interchanges are made is N to IHI+1,
* then 1 to ILO-1.
*
* ABNRM (output) REAL
* The one-norm of the balanced matrix A.
*
* BBNRM (output) REAL
* The one-norm of the balanced matrix B.
*
* RCONDE (output) REAL array, dimension (N)
* If SENSE = 'E' or 'B', the reciprocal condition numbers of
* the eigenvalues, stored in consecutive elements of the array.
* For a complex conjugate pair of eigenvalues two consecutive
* elements of RCONDE are set to the same value. Thus RCONDE(j),
* RCONDV(j), and the j-th columns of VL and VR all correspond
* to the j-th eigenpair.
* If SENSE = 'N' or 'V', RCONDE is not referenced.
*
* RCONDV (output) REAL array, dimension (N)
* If SENSE = 'V' or 'B', the estimated reciprocal condition
* numbers of the eigenvectors, stored in consecutive elements
* of the array. For a complex eigenvector two consecutive
* elements of RCONDV are set to the same value. If the
* eigenvalues cannot be reordered to compute RCONDV(j),
* RCONDV(j) is set to 0; this can only occur when the true
* value would be very small anyway.
* If SENSE = 'N' or 'E', RCONDV is not referenced.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,2*N).
* If BALANC = 'S' or 'B', or JOBVL = 'V', or JOBVR = 'V',
* LWORK >= max(1,6*N).
* If SENSE = 'E', LWORK >= max(1,10*N).
* If SENSE = 'V' or 'B', LWORK >= 2*N*N+8*N+16.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (N+6)
* If SENSE = 'E', IWORK is not referenced.
*
* BWORK (workspace) LOGICAL array, dimension (N)
* If SENSE = 'N', BWORK is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1,...,N:
* The QZ iteration failed. No eigenvectors have been
* calculated, but ALPHAR(j), ALPHAI(j), and BETA(j)
* should be correct for j=INFO+1,...,N.
* > N: =N+1: other than QZ iteration failed in SHGEQZ.
* =N+2: error return from STGEVC.
*
* Further Details
* ===============
*
* Balancing a matrix pair (A,B) includes, first, permuting rows and
* columns to isolate eigenvalues, second, applying diagonal similarity
* transformation to the rows and columns to make the rows and columns
* as close in norm as possible. The computed reciprocal condition
* numbers correspond to the balanced matrix. Permuting rows and columns
* will not change the condition numbers (in exact arithmetic) but
* diagonal scaling will. For further explanation of balancing, see
* section 4.11.1.2 of LAPACK Users' Guide.
*
* An approximate error bound on the chordal distance between the i-th
* computed generalized eigenvalue w and the corresponding exact
* eigenvalue lambda is
*
* chord(w, lambda) <= EPS * norm(ABNRM, BBNRM) / RCONDE(I)
*
* An approximate error bound for the angle between the i-th computed
* eigenvector VL(i) or VR(i) is given by
*
* EPS * norm(ABNRM, BBNRM) / DIF(i).
*
* For further explanation of the reciprocal condition numbers RCONDE
* and RCONDV, see section 4.11 of LAPACK User's Guide.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param balanc
* @param jobvl
* @param jobvr
* @param sense
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param alphar
* @param alphai
* @param beta
* @param vl
* @param ldvl
* @param vr
* @param ldvr
* @param ilo
* @param ihi
* @param lscale
* @param rscale
* @param abnrm
* @param bbnrm
* @param rconde
* @param rcondv
* @param work
* @param lwork
* @param iwork
* @param bwork
* @param info
*
*/
abstract public void sggevx(java.lang.String balanc, java.lang.String jobvl, java.lang.String jobvr, java.lang.String sense, int n, float[] a, int lda, float[] b, int ldb, float[] alphar, float[] alphai, float[] beta, float[] vl, int ldvl, float[] vr, int ldvr, org.netlib.util.intW ilo, org.netlib.util.intW ihi, float[] lscale, float[] rscale, org.netlib.util.floatW abnrm, org.netlib.util.floatW bbnrm, float[] rconde, float[] rcondv, float[] work, int lwork, int[] iwork, boolean[] bwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGGEVX computes for a pair of N-by-N real nonsymmetric matrices (A,B)
* the generalized eigenvalues, and optionally, the left and/or right
* generalized eigenvectors.
*
* Optionally also, it computes a balancing transformation to improve
* the conditioning of the eigenvalues and eigenvectors (ILO, IHI,
* LSCALE, RSCALE, ABNRM, and BBNRM), reciprocal condition numbers for
* the eigenvalues (RCONDE), and reciprocal condition numbers for the
* right eigenvectors (RCONDV).
*
* A generalized eigenvalue for a pair of matrices (A,B) is a scalar
* lambda or a ratio alpha/beta = lambda, such that A - lambda*B is
* singular. It is usually represented as the pair (alpha,beta), as
* there is a reasonable interpretation for beta=0, and even for both
* being zero.
*
* The right eigenvector v(j) corresponding to the eigenvalue lambda(j)
* of (A,B) satisfies
*
* A * v(j) = lambda(j) * B * v(j) .
*
* The left eigenvector u(j) corresponding to the eigenvalue lambda(j)
* of (A,B) satisfies
*
* u(j)**H * A = lambda(j) * u(j)**H * B.
*
* where u(j)**H is the conjugate-transpose of u(j).
*
*
* Arguments
* =========
*
* BALANC (input) CHARACTER*1
* Specifies the balance option to be performed.
* = 'N': do not diagonally scale or permute;
* = 'P': permute only;
* = 'S': scale only;
* = 'B': both permute and scale.
* Computed reciprocal condition numbers will be for the
* matrices after permuting and/or balancing. Permuting does
* not change condition numbers (in exact arithmetic), but
* balancing does.
*
* JOBVL (input) CHARACTER*1
* = 'N': do not compute the left generalized eigenvectors;
* = 'V': compute the left generalized eigenvectors.
*
* JOBVR (input) CHARACTER*1
* = 'N': do not compute the right generalized eigenvectors;
* = 'V': compute the right generalized eigenvectors.
*
* SENSE (input) CHARACTER*1
* Determines which reciprocal condition numbers are computed.
* = 'N': none are computed;
* = 'E': computed for eigenvalues only;
* = 'V': computed for eigenvectors only;
* = 'B': computed for eigenvalues and eigenvectors.
*
* N (input) INTEGER
* The order of the matrices A, B, VL, and VR. N >= 0.
*
* A (input/output) REAL array, dimension (LDA, N)
* On entry, the matrix A in the pair (A,B).
* On exit, A has been overwritten. If JOBVL='V' or JOBVR='V'
* or both, then A contains the first part of the real Schur
* form of the "balanced" versions of the input A and B.
*
* LDA (input) INTEGER
* The leading dimension of A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension (LDB, N)
* On entry, the matrix B in the pair (A,B).
* On exit, B has been overwritten. If JOBVL='V' or JOBVR='V'
* or both, then B contains the second part of the real Schur
* form of the "balanced" versions of the input A and B.
*
* LDB (input) INTEGER
* The leading dimension of B. LDB >= max(1,N).
*
* ALPHAR (output) REAL array, dimension (N)
* ALPHAI (output) REAL array, dimension (N)
* BETA (output) REAL array, dimension (N)
* On exit, (ALPHAR(j) + ALPHAI(j)*i)/BETA(j), j=1,...,N, will
* be the generalized eigenvalues. If ALPHAI(j) is zero, then
* the j-th eigenvalue is real; if positive, then the j-th and
* (j+1)-st eigenvalues are a complex conjugate pair, with
* ALPHAI(j+1) negative.
*
* Note: the quotients ALPHAR(j)/BETA(j) and ALPHAI(j)/BETA(j)
* may easily over- or underflow, and BETA(j) may even be zero.
* Thus, the user should avoid naively computing the ratio
* ALPHA/BETA. However, ALPHAR and ALPHAI will be always less
* than and usually comparable with norm(A) in magnitude, and
* BETA always less than and usually comparable with norm(B).
*
* VL (output) REAL array, dimension (LDVL,N)
* If JOBVL = 'V', the left eigenvectors u(j) are stored one
* after another in the columns of VL, in the same order as
* their eigenvalues. If the j-th eigenvalue is real, then
* u(j) = VL(:,j), the j-th column of VL. If the j-th and
* (j+1)-th eigenvalues form a complex conjugate pair, then
* u(j) = VL(:,j)+i*VL(:,j+1) and u(j+1) = VL(:,j)-i*VL(:,j+1).
* Each eigenvector will be scaled so the largest component have
* abs(real part) + abs(imag. part) = 1.
* Not referenced if JOBVL = 'N'.
*
* LDVL (input) INTEGER
* The leading dimension of the matrix VL. LDVL >= 1, and
* if JOBVL = 'V', LDVL >= N.
*
* VR (output) REAL array, dimension (LDVR,N)
* If JOBVR = 'V', the right eigenvectors v(j) are stored one
* after another in the columns of VR, in the same order as
* their eigenvalues. If the j-th eigenvalue is real, then
* v(j) = VR(:,j), the j-th column of VR. If the j-th and
* (j+1)-th eigenvalues form a complex conjugate pair, then
* v(j) = VR(:,j)+i*VR(:,j+1) and v(j+1) = VR(:,j)-i*VR(:,j+1).
* Each eigenvector will be scaled so the largest component have
* abs(real part) + abs(imag. part) = 1.
* Not referenced if JOBVR = 'N'.
*
* LDVR (input) INTEGER
* The leading dimension of the matrix VR. LDVR >= 1, and
* if JOBVR = 'V', LDVR >= N.
*
* ILO (output) INTEGER
* IHI (output) INTEGER
* ILO and IHI are integer values such that on exit
* A(i,j) = 0 and B(i,j) = 0 if i > j and
* j = 1,...,ILO-1 or i = IHI+1,...,N.
* If BALANC = 'N' or 'S', ILO = 1 and IHI = N.
*
* LSCALE (output) REAL array, dimension (N)
* Details of the permutations and scaling factors applied
* to the left side of A and B. If PL(j) is the index of the
* row interchanged with row j, and DL(j) is the scaling
* factor applied to row j, then
* LSCALE(j) = PL(j) for j = 1,...,ILO-1
* = DL(j) for j = ILO,...,IHI
* = PL(j) for j = IHI+1,...,N.
* The order in which the interchanges are made is N to IHI+1,
* then 1 to ILO-1.
*
* RSCALE (output) REAL array, dimension (N)
* Details of the permutations and scaling factors applied
* to the right side of A and B. If PR(j) is the index of the
* column interchanged with column j, and DR(j) is the scaling
* factor applied to column j, then
* RSCALE(j) = PR(j) for j = 1,...,ILO-1
* = DR(j) for j = ILO,...,IHI
* = PR(j) for j = IHI+1,...,N
* The order in which the interchanges are made is N to IHI+1,
* then 1 to ILO-1.
*
* ABNRM (output) REAL
* The one-norm of the balanced matrix A.
*
* BBNRM (output) REAL
* The one-norm of the balanced matrix B.
*
* RCONDE (output) REAL array, dimension (N)
* If SENSE = 'E' or 'B', the reciprocal condition numbers of
* the eigenvalues, stored in consecutive elements of the array.
* For a complex conjugate pair of eigenvalues two consecutive
* elements of RCONDE are set to the same value. Thus RCONDE(j),
* RCONDV(j), and the j-th columns of VL and VR all correspond
* to the j-th eigenpair.
* If SENSE = 'N' or 'V', RCONDE is not referenced.
*
* RCONDV (output) REAL array, dimension (N)
* If SENSE = 'V' or 'B', the estimated reciprocal condition
* numbers of the eigenvectors, stored in consecutive elements
* of the array. For a complex eigenvector two consecutive
* elements of RCONDV are set to the same value. If the
* eigenvalues cannot be reordered to compute RCONDV(j),
* RCONDV(j) is set to 0; this can only occur when the true
* value would be very small anyway.
* If SENSE = 'N' or 'E', RCONDV is not referenced.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,2*N).
* If BALANC = 'S' or 'B', or JOBVL = 'V', or JOBVR = 'V',
* LWORK >= max(1,6*N).
* If SENSE = 'E', LWORK >= max(1,10*N).
* If SENSE = 'V' or 'B', LWORK >= 2*N*N+8*N+16.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (N+6)
* If SENSE = 'E', IWORK is not referenced.
*
* BWORK (workspace) LOGICAL array, dimension (N)
* If SENSE = 'N', BWORK is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1,...,N:
* The QZ iteration failed. No eigenvectors have been
* calculated, but ALPHAR(j), ALPHAI(j), and BETA(j)
* should be correct for j=INFO+1,...,N.
* > N: =N+1: other than QZ iteration failed in SHGEQZ.
* =N+2: error return from STGEVC.
*
* Further Details
* ===============
*
* Balancing a matrix pair (A,B) includes, first, permuting rows and
* columns to isolate eigenvalues, second, applying diagonal similarity
* transformation to the rows and columns to make the rows and columns
* as close in norm as possible. The computed reciprocal condition
* numbers correspond to the balanced matrix. Permuting rows and columns
* will not change the condition numbers (in exact arithmetic) but
* diagonal scaling will. For further explanation of balancing, see
* section 4.11.1.2 of LAPACK Users' Guide.
*
* An approximate error bound on the chordal distance between the i-th
* computed generalized eigenvalue w and the corresponding exact
* eigenvalue lambda is
*
* chord(w, lambda) <= EPS * norm(ABNRM, BBNRM) / RCONDE(I)
*
* An approximate error bound for the angle between the i-th computed
* eigenvector VL(i) or VR(i) is given by
*
* EPS * norm(ABNRM, BBNRM) / DIF(i).
*
* For further explanation of the reciprocal condition numbers RCONDE
* and RCONDV, see section 4.11 of LAPACK User's Guide.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param balanc
* @param jobvl
* @param jobvr
* @param sense
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param alphar
* @param _alphar_offset
* @param alphai
* @param _alphai_offset
* @param beta
* @param _beta_offset
* @param vl
* @param _vl_offset
* @param ldvl
* @param vr
* @param _vr_offset
* @param ldvr
* @param ilo
* @param ihi
* @param lscale
* @param _lscale_offset
* @param rscale
* @param _rscale_offset
* @param abnrm
* @param bbnrm
* @param rconde
* @param _rconde_offset
* @param rcondv
* @param _rcondv_offset
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param bwork
* @param _bwork_offset
* @param info
*
*/
abstract public void sggevx(java.lang.String balanc, java.lang.String jobvl, java.lang.String jobvr, java.lang.String sense, int n, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, float[] alphar, int _alphar_offset, float[] alphai, int _alphai_offset, float[] beta, int _beta_offset, float[] vl, int _vl_offset, int ldvl, float[] vr, int _vr_offset, int ldvr, org.netlib.util.intW ilo, org.netlib.util.intW ihi, float[] lscale, int _lscale_offset, float[] rscale, int _rscale_offset, org.netlib.util.floatW abnrm, org.netlib.util.floatW bbnrm, float[] rconde, int _rconde_offset, float[] rcondv, int _rcondv_offset, float[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, boolean[] bwork, int _bwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGGGLM solves a general Gauss-Markov linear model (GLM) problem:
*
* minimize || y ||_2 subject to d = A*x + B*y
* x
*
* where A is an N-by-M matrix, B is an N-by-P matrix, and d is a
* given N-vector. It is assumed that M <= N <= M+P, and
*
* rank(A) = M and rank( A B ) = N.
*
* Under these assumptions, the constrained equation is always
* consistent, and there is a unique solution x and a minimal 2-norm
* solution y, which is obtained using a generalized QR factorization
* of the matrices (A, B) given by
*
* A = Q*(R), B = Q*T*Z.
* (0)
*
* In particular, if matrix B is square nonsingular, then the problem
* GLM is equivalent to the following weighted linear least squares
* problem
*
* minimize || inv(B)*(d-A*x) ||_2
* x
*
* where inv(B) denotes the inverse of B.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of rows of the matrices A and B. N >= 0.
*
* M (input) INTEGER
* The number of columns of the matrix A. 0 <= M <= N.
*
* P (input) INTEGER
* The number of columns of the matrix B. P >= N-M.
*
* A (input/output) REAL array, dimension (LDA,M)
* On entry, the N-by-M matrix A.
* On exit, the upper triangular part of the array A contains
* the M-by-M upper triangular matrix R.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension (LDB,P)
* On entry, the N-by-P matrix B.
* On exit, if N <= P, the upper triangle of the subarray
* B(1:N,P-N+1:P) contains the N-by-N upper triangular matrix T;
* if N > P, the elements on and above the (N-P)th subdiagonal
* contain the N-by-P upper trapezoidal matrix T.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* D (input/output) REAL array, dimension (N)
* On entry, D is the left hand side of the GLM equation.
* On exit, D is destroyed.
*
* X (output) REAL array, dimension (M)
* Y (output) REAL array, dimension (P)
* On exit, X and Y are the solutions of the GLM problem.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N+M+P).
* For optimum performance, LWORK >= M+min(N,P)+max(N,P)*NB,
* where NB is an upper bound for the optimal blocksizes for
* SGEQRF, SGERQF, SORMQR and SORMRQ.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1: the upper triangular factor R associated with A in the
* generalized QR factorization of the pair (A, B) is
* singular, so that rank(A) < M; the least squares
* solution could not be computed.
* = 2: the bottom (N-M) by (N-M) part of the upper trapezoidal
* factor T associated with B in the generalized QR
* factorization of the pair (A, B) is singular, so that
* rank( A B ) < N; the least squares solution could not
* be computed.
*
* ===================================================================
*
* .. Parameters ..
*
*
* @param n
* @param m
* @param p
* @param a
* @param lda
* @param b
* @param ldb
* @param d
* @param x
* @param y
* @param work
* @param lwork
* @param info
*
*/
abstract public void sggglm(int n, int m, int p, float[] a, int lda, float[] b, int ldb, float[] d, float[] x, float[] y, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGGGLM solves a general Gauss-Markov linear model (GLM) problem:
*
* minimize || y ||_2 subject to d = A*x + B*y
* x
*
* where A is an N-by-M matrix, B is an N-by-P matrix, and d is a
* given N-vector. It is assumed that M <= N <= M+P, and
*
* rank(A) = M and rank( A B ) = N.
*
* Under these assumptions, the constrained equation is always
* consistent, and there is a unique solution x and a minimal 2-norm
* solution y, which is obtained using a generalized QR factorization
* of the matrices (A, B) given by
*
* A = Q*(R), B = Q*T*Z.
* (0)
*
* In particular, if matrix B is square nonsingular, then the problem
* GLM is equivalent to the following weighted linear least squares
* problem
*
* minimize || inv(B)*(d-A*x) ||_2
* x
*
* where inv(B) denotes the inverse of B.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of rows of the matrices A and B. N >= 0.
*
* M (input) INTEGER
* The number of columns of the matrix A. 0 <= M <= N.
*
* P (input) INTEGER
* The number of columns of the matrix B. P >= N-M.
*
* A (input/output) REAL array, dimension (LDA,M)
* On entry, the N-by-M matrix A.
* On exit, the upper triangular part of the array A contains
* the M-by-M upper triangular matrix R.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension (LDB,P)
* On entry, the N-by-P matrix B.
* On exit, if N <= P, the upper triangle of the subarray
* B(1:N,P-N+1:P) contains the N-by-N upper triangular matrix T;
* if N > P, the elements on and above the (N-P)th subdiagonal
* contain the N-by-P upper trapezoidal matrix T.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* D (input/output) REAL array, dimension (N)
* On entry, D is the left hand side of the GLM equation.
* On exit, D is destroyed.
*
* X (output) REAL array, dimension (M)
* Y (output) REAL array, dimension (P)
* On exit, X and Y are the solutions of the GLM problem.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N+M+P).
* For optimum performance, LWORK >= M+min(N,P)+max(N,P)*NB,
* where NB is an upper bound for the optimal blocksizes for
* SGEQRF, SGERQF, SORMQR and SORMRQ.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1: the upper triangular factor R associated with A in the
* generalized QR factorization of the pair (A, B) is
* singular, so that rank(A) < M; the least squares
* solution could not be computed.
* = 2: the bottom (N-M) by (N-M) part of the upper trapezoidal
* factor T associated with B in the generalized QR
* factorization of the pair (A, B) is singular, so that
* rank( A B ) < N; the least squares solution could not
* be computed.
*
* ===================================================================
*
* .. Parameters ..
*
*
* @param n
* @param m
* @param p
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param d
* @param _d_offset
* @param x
* @param _x_offset
* @param y
* @param _y_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sggglm(int n, int m, int p, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, float[] d, int _d_offset, float[] x, int _x_offset, float[] y, int _y_offset, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGGHRD reduces a pair of real matrices (A,B) to generalized upper
* Hessenberg form using orthogonal transformations, where A is a
* general matrix and B is upper triangular. The form of the
* generalized eigenvalue problem is
* A*x = lambda*B*x,
* and B is typically made upper triangular by computing its QR
* factorization and moving the orthogonal matrix Q to the left side
* of the equation.
*
* This subroutine simultaneously reduces A to a Hessenberg matrix H:
* Q**T*A*Z = H
* and transforms B to another upper triangular matrix T:
* Q**T*B*Z = T
* in order to reduce the problem to its standard form
* H*y = lambda*T*y
* where y = Z**T*x.
*
* The orthogonal matrices Q and Z are determined as products of Givens
* rotations. They may either be formed explicitly, or they may be
* postmultiplied into input matrices Q1 and Z1, so that
*
* Q1 * A * Z1**T = (Q1*Q) * H * (Z1*Z)**T
*
* Q1 * B * Z1**T = (Q1*Q) * T * (Z1*Z)**T
*
* If Q1 is the orthogonal matrix from the QR factorization of B in the
* original equation A*x = lambda*B*x, then SGGHRD reduces the original
* problem to generalized Hessenberg form.
*
* Arguments
* =========
*
* COMPQ (input) CHARACTER*1
* = 'N': do not compute Q;
* = 'I': Q is initialized to the unit matrix, and the
* orthogonal matrix Q is returned;
* = 'V': Q must contain an orthogonal matrix Q1 on entry,
* and the product Q1*Q is returned.
*
* COMPZ (input) CHARACTER*1
* = 'N': do not compute Z;
* = 'I': Z is initialized to the unit matrix, and the
* orthogonal matrix Z is returned;
* = 'V': Z must contain an orthogonal matrix Z1 on entry,
* and the product Z1*Z is returned.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* ILO and IHI mark the rows and columns of A which are to be
* reduced. It is assumed that A is already upper triangular
* in rows and columns 1:ILO-1 and IHI+1:N. ILO and IHI are
* normally set by a previous call to SGGBAL; otherwise they
* should be set to 1 and N respectively.
* 1 <= ILO <= IHI <= N, if N > 0; ILO=1 and IHI=0, if N=0.
*
* A (input/output) REAL array, dimension (LDA, N)
* On entry, the N-by-N general matrix to be reduced.
* On exit, the upper triangle and the first subdiagonal of A
* are overwritten with the upper Hessenberg matrix H, and the
* rest is set to zero.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension (LDB, N)
* On entry, the N-by-N upper triangular matrix B.
* On exit, the upper triangular matrix T = Q**T B Z. The
* elements below the diagonal are set to zero.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* Q (input/output) REAL array, dimension (LDQ, N)
* On entry, if COMPQ = 'V', the orthogonal matrix Q1,
* typically from the QR factorization of B.
* On exit, if COMPQ='I', the orthogonal matrix Q, and if
* COMPQ = 'V', the product Q1*Q.
* Not referenced if COMPQ='N'.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q.
* LDQ >= N if COMPQ='V' or 'I'; LDQ >= 1 otherwise.
*
* Z (input/output) REAL array, dimension (LDZ, N)
* On entry, if COMPZ = 'V', the orthogonal matrix Z1.
* On exit, if COMPZ='I', the orthogonal matrix Z, and if
* COMPZ = 'V', the product Z1*Z.
* Not referenced if COMPZ='N'.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z.
* LDZ >= N if COMPZ='V' or 'I'; LDZ >= 1 otherwise.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* This routine reduces A to Hessenberg and B to triangular form by
* an unblocked reduction, as described in _Matrix_Computations_,
* by Golub and Van Loan (Johns Hopkins Press.)
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param compq
* @param compz
* @param n
* @param ilo
* @param ihi
* @param a
* @param lda
* @param b
* @param ldb
* @param q
* @param ldq
* @param z
* @param ldz
* @param info
*
*/
abstract public void sgghrd(java.lang.String compq, java.lang.String compz, int n, int ilo, int ihi, float[] a, int lda, float[] b, int ldb, float[] q, int ldq, float[] z, int ldz, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGGHRD reduces a pair of real matrices (A,B) to generalized upper
* Hessenberg form using orthogonal transformations, where A is a
* general matrix and B is upper triangular. The form of the
* generalized eigenvalue problem is
* A*x = lambda*B*x,
* and B is typically made upper triangular by computing its QR
* factorization and moving the orthogonal matrix Q to the left side
* of the equation.
*
* This subroutine simultaneously reduces A to a Hessenberg matrix H:
* Q**T*A*Z = H
* and transforms B to another upper triangular matrix T:
* Q**T*B*Z = T
* in order to reduce the problem to its standard form
* H*y = lambda*T*y
* where y = Z**T*x.
*
* The orthogonal matrices Q and Z are determined as products of Givens
* rotations. They may either be formed explicitly, or they may be
* postmultiplied into input matrices Q1 and Z1, so that
*
* Q1 * A * Z1**T = (Q1*Q) * H * (Z1*Z)**T
*
* Q1 * B * Z1**T = (Q1*Q) * T * (Z1*Z)**T
*
* If Q1 is the orthogonal matrix from the QR factorization of B in the
* original equation A*x = lambda*B*x, then SGGHRD reduces the original
* problem to generalized Hessenberg form.
*
* Arguments
* =========
*
* COMPQ (input) CHARACTER*1
* = 'N': do not compute Q;
* = 'I': Q is initialized to the unit matrix, and the
* orthogonal matrix Q is returned;
* = 'V': Q must contain an orthogonal matrix Q1 on entry,
* and the product Q1*Q is returned.
*
* COMPZ (input) CHARACTER*1
* = 'N': do not compute Z;
* = 'I': Z is initialized to the unit matrix, and the
* orthogonal matrix Z is returned;
* = 'V': Z must contain an orthogonal matrix Z1 on entry,
* and the product Z1*Z is returned.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* ILO and IHI mark the rows and columns of A which are to be
* reduced. It is assumed that A is already upper triangular
* in rows and columns 1:ILO-1 and IHI+1:N. ILO and IHI are
* normally set by a previous call to SGGBAL; otherwise they
* should be set to 1 and N respectively.
* 1 <= ILO <= IHI <= N, if N > 0; ILO=1 and IHI=0, if N=0.
*
* A (input/output) REAL array, dimension (LDA, N)
* On entry, the N-by-N general matrix to be reduced.
* On exit, the upper triangle and the first subdiagonal of A
* are overwritten with the upper Hessenberg matrix H, and the
* rest is set to zero.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension (LDB, N)
* On entry, the N-by-N upper triangular matrix B.
* On exit, the upper triangular matrix T = Q**T B Z. The
* elements below the diagonal are set to zero.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* Q (input/output) REAL array, dimension (LDQ, N)
* On entry, if COMPQ = 'V', the orthogonal matrix Q1,
* typically from the QR factorization of B.
* On exit, if COMPQ='I', the orthogonal matrix Q, and if
* COMPQ = 'V', the product Q1*Q.
* Not referenced if COMPQ='N'.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q.
* LDQ >= N if COMPQ='V' or 'I'; LDQ >= 1 otherwise.
*
* Z (input/output) REAL array, dimension (LDZ, N)
* On entry, if COMPZ = 'V', the orthogonal matrix Z1.
* On exit, if COMPZ='I', the orthogonal matrix Z, and if
* COMPZ = 'V', the product Z1*Z.
* Not referenced if COMPZ='N'.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z.
* LDZ >= N if COMPZ='V' or 'I'; LDZ >= 1 otherwise.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* This routine reduces A to Hessenberg and B to triangular form by
* an unblocked reduction, as described in _Matrix_Computations_,
* by Golub and Van Loan (Johns Hopkins Press.)
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param compq
* @param compz
* @param n
* @param ilo
* @param ihi
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param q
* @param _q_offset
* @param ldq
* @param z
* @param _z_offset
* @param ldz
* @param info
*
*/
abstract public void sgghrd(java.lang.String compq, java.lang.String compz, int n, int ilo, int ihi, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, float[] q, int _q_offset, int ldq, float[] z, int _z_offset, int ldz, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGGLSE solves the linear equality-constrained least squares (LSE)
* problem:
*
* minimize || c - A*x ||_2 subject to B*x = d
*
* where A is an M-by-N matrix, B is a P-by-N matrix, c is a given
* M-vector, and d is a given P-vector. It is assumed that
* P <= N <= M+P, and
*
* rank(B) = P and rank( (A) ) = N.
* ( (B) )
*
* These conditions ensure that the LSE problem has a unique solution,
* which is obtained using a generalized RQ factorization of the
* matrices (B, A) given by
*
* B = (0 R)*Q, A = Z*T*Q.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrices A and B. N >= 0.
*
* P (input) INTEGER
* The number of rows of the matrix B. 0 <= P <= N <= M+P.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, the elements on and above the diagonal of the array
* contain the min(M,N)-by-N upper trapezoidal matrix T.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) REAL array, dimension (LDB,N)
* On entry, the P-by-N matrix B.
* On exit, the upper triangle of the subarray B(1:P,N-P+1:N)
* contains the P-by-P upper triangular matrix R.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,P).
*
* C (input/output) REAL array, dimension (M)
* On entry, C contains the right hand side vector for the
* least squares part of the LSE problem.
* On exit, the residual sum of squares for the solution
* is given by the sum of squares of elements N-P+1 to M of
* vector C.
*
* D (input/output) REAL array, dimension (P)
* On entry, D contains the right hand side vector for the
* constrained equation.
* On exit, D is destroyed.
*
* X (output) REAL array, dimension (N)
* On exit, X is the solution of the LSE problem.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,M+N+P).
* For optimum performance LWORK >= P+min(M,N)+max(M,N)*NB,
* where NB is an upper bound for the optimal blocksizes for
* SGEQRF, SGERQF, SORMQR and SORMRQ.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1: the upper triangular factor R associated with B in the
* generalized RQ factorization of the pair (B, A) is
* singular, so that rank(B) < P; the least squares
* solution could not be computed.
* = 2: the (N-P) by (N-P) part of the upper trapezoidal factor
* T associated with A in the generalized RQ factorization
* of the pair (B, A) is singular, so that
* rank( (A) ) < N; the least squares solution could not
* ( (B) )
* be computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param p
* @param a
* @param lda
* @param b
* @param ldb
* @param c
* @param d
* @param x
* @param work
* @param lwork
* @param info
*
*/
abstract public void sgglse(int m, int n, int p, float[] a, int lda, float[] b, int ldb, float[] c, float[] d, float[] x, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGGLSE solves the linear equality-constrained least squares (LSE)
* problem:
*
* minimize || c - A*x ||_2 subject to B*x = d
*
* where A is an M-by-N matrix, B is a P-by-N matrix, c is a given
* M-vector, and d is a given P-vector. It is assumed that
* P <= N <= M+P, and
*
* rank(B) = P and rank( (A) ) = N.
* ( (B) )
*
* These conditions ensure that the LSE problem has a unique solution,
* which is obtained using a generalized RQ factorization of the
* matrices (B, A) given by
*
* B = (0 R)*Q, A = Z*T*Q.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrices A and B. N >= 0.
*
* P (input) INTEGER
* The number of rows of the matrix B. 0 <= P <= N <= M+P.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, the elements on and above the diagonal of the array
* contain the min(M,N)-by-N upper trapezoidal matrix T.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) REAL array, dimension (LDB,N)
* On entry, the P-by-N matrix B.
* On exit, the upper triangle of the subarray B(1:P,N-P+1:N)
* contains the P-by-P upper triangular matrix R.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,P).
*
* C (input/output) REAL array, dimension (M)
* On entry, C contains the right hand side vector for the
* least squares part of the LSE problem.
* On exit, the residual sum of squares for the solution
* is given by the sum of squares of elements N-P+1 to M of
* vector C.
*
* D (input/output) REAL array, dimension (P)
* On entry, D contains the right hand side vector for the
* constrained equation.
* On exit, D is destroyed.
*
* X (output) REAL array, dimension (N)
* On exit, X is the solution of the LSE problem.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,M+N+P).
* For optimum performance LWORK >= P+min(M,N)+max(M,N)*NB,
* where NB is an upper bound for the optimal blocksizes for
* SGEQRF, SGERQF, SORMQR and SORMRQ.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1: the upper triangular factor R associated with B in the
* generalized RQ factorization of the pair (B, A) is
* singular, so that rank(B) < P; the least squares
* solution could not be computed.
* = 2: the (N-P) by (N-P) part of the upper trapezoidal factor
* T associated with A in the generalized RQ factorization
* of the pair (B, A) is singular, so that
* rank( (A) ) < N; the least squares solution could not
* ( (B) )
* be computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param p
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param c
* @param _c_offset
* @param d
* @param _d_offset
* @param x
* @param _x_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sgglse(int m, int n, int p, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, float[] c, int _c_offset, float[] d, int _d_offset, float[] x, int _x_offset, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGGQRF computes a generalized QR factorization of an N-by-M matrix A
* and an N-by-P matrix B:
*
* A = Q*R, B = Q*T*Z,
*
* where Q is an N-by-N orthogonal matrix, Z is a P-by-P orthogonal
* matrix, and R and T assume one of the forms:
*
* if N >= M, R = ( R11 ) M , or if N < M, R = ( R11 R12 ) N,
* ( 0 ) N-M N M-N
* M
*
* where R11 is upper triangular, and
*
* if N <= P, T = ( 0 T12 ) N, or if N > P, T = ( T11 ) N-P,
* P-N N ( T21 ) P
* P
*
* where T12 or T21 is upper triangular.
*
* In particular, if B is square and nonsingular, the GQR factorization
* of A and B implicitly gives the QR factorization of inv(B)*A:
*
* inv(B)*A = Z'*(inv(T)*R)
*
* where inv(B) denotes the inverse of the matrix B, and Z' denotes the
* transpose of the matrix Z.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of rows of the matrices A and B. N >= 0.
*
* M (input) INTEGER
* The number of columns of the matrix A. M >= 0.
*
* P (input) INTEGER
* The number of columns of the matrix B. P >= 0.
*
* A (input/output) REAL array, dimension (LDA,M)
* On entry, the N-by-M matrix A.
* On exit, the elements on and above the diagonal of the array
* contain the min(N,M)-by-M upper trapezoidal matrix R (R is
* upper triangular if N >= M); the elements below the diagonal,
* with the array TAUA, represent the orthogonal matrix Q as a
* product of min(N,M) elementary reflectors (see Further
* Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* TAUA (output) REAL array, dimension (min(N,M))
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix Q (see Further Details).
*
* B (input/output) REAL array, dimension (LDB,P)
* On entry, the N-by-P matrix B.
* On exit, if N <= P, the upper triangle of the subarray
* B(1:N,P-N+1:P) contains the N-by-N upper triangular matrix T;
* if N > P, the elements on and above the (N-P)-th subdiagonal
* contain the N-by-P upper trapezoidal matrix T; the remaining
* elements, with the array TAUB, represent the orthogonal
* matrix Z as a product of elementary reflectors (see Further
* Details).
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* TAUB (output) REAL array, dimension (min(N,P))
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix Z (see Further Details).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N,M,P).
* For optimum performance LWORK >= max(N,M,P)*max(NB1,NB2,NB3),
* where NB1 is the optimal blocksize for the QR factorization
* of an N-by-M matrix, NB2 is the optimal blocksize for the
* RQ factorization of an N-by-P matrix, and NB3 is the optimal
* blocksize for a call of SORMQR.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(1) H(2) . . . H(k), where k = min(n,m).
*
* Each H(i) has the form
*
* H(i) = I - taua * v * v'
*
* where taua is a real scalar, and v is a real vector with
* v(1:i-1) = 0 and v(i) = 1; v(i+1:n) is stored on exit in A(i+1:n,i),
* and taua in TAUA(i).
* To form Q explicitly, use LAPACK subroutine SORGQR.
* To use Q to update another matrix, use LAPACK subroutine SORMQR.
*
* The matrix Z is represented as a product of elementary reflectors
*
* Z = H(1) H(2) . . . H(k), where k = min(n,p).
*
* Each H(i) has the form
*
* H(i) = I - taub * v * v'
*
* where taub is a real scalar, and v is a real vector with
* v(p-k+i+1:p) = 0 and v(p-k+i) = 1; v(1:p-k+i-1) is stored on exit in
* B(n-k+i,1:p-k+i-1), and taub in TAUB(i).
* To form Z explicitly, use LAPACK subroutine SORGRQ.
* To use Z to update another matrix, use LAPACK subroutine SORMRQ.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n
* @param m
* @param p
* @param a
* @param lda
* @param taua
* @param b
* @param ldb
* @param taub
* @param work
* @param lwork
* @param info
*
*/
abstract public void sggqrf(int n, int m, int p, float[] a, int lda, float[] taua, float[] b, int ldb, float[] taub, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGGQRF computes a generalized QR factorization of an N-by-M matrix A
* and an N-by-P matrix B:
*
* A = Q*R, B = Q*T*Z,
*
* where Q is an N-by-N orthogonal matrix, Z is a P-by-P orthogonal
* matrix, and R and T assume one of the forms:
*
* if N >= M, R = ( R11 ) M , or if N < M, R = ( R11 R12 ) N,
* ( 0 ) N-M N M-N
* M
*
* where R11 is upper triangular, and
*
* if N <= P, T = ( 0 T12 ) N, or if N > P, T = ( T11 ) N-P,
* P-N N ( T21 ) P
* P
*
* where T12 or T21 is upper triangular.
*
* In particular, if B is square and nonsingular, the GQR factorization
* of A and B implicitly gives the QR factorization of inv(B)*A:
*
* inv(B)*A = Z'*(inv(T)*R)
*
* where inv(B) denotes the inverse of the matrix B, and Z' denotes the
* transpose of the matrix Z.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of rows of the matrices A and B. N >= 0.
*
* M (input) INTEGER
* The number of columns of the matrix A. M >= 0.
*
* P (input) INTEGER
* The number of columns of the matrix B. P >= 0.
*
* A (input/output) REAL array, dimension (LDA,M)
* On entry, the N-by-M matrix A.
* On exit, the elements on and above the diagonal of the array
* contain the min(N,M)-by-M upper trapezoidal matrix R (R is
* upper triangular if N >= M); the elements below the diagonal,
* with the array TAUA, represent the orthogonal matrix Q as a
* product of min(N,M) elementary reflectors (see Further
* Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* TAUA (output) REAL array, dimension (min(N,M))
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix Q (see Further Details).
*
* B (input/output) REAL array, dimension (LDB,P)
* On entry, the N-by-P matrix B.
* On exit, if N <= P, the upper triangle of the subarray
* B(1:N,P-N+1:P) contains the N-by-N upper triangular matrix T;
* if N > P, the elements on and above the (N-P)-th subdiagonal
* contain the N-by-P upper trapezoidal matrix T; the remaining
* elements, with the array TAUB, represent the orthogonal
* matrix Z as a product of elementary reflectors (see Further
* Details).
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* TAUB (output) REAL array, dimension (min(N,P))
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix Z (see Further Details).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N,M,P).
* For optimum performance LWORK >= max(N,M,P)*max(NB1,NB2,NB3),
* where NB1 is the optimal blocksize for the QR factorization
* of an N-by-M matrix, NB2 is the optimal blocksize for the
* RQ factorization of an N-by-P matrix, and NB3 is the optimal
* blocksize for a call of SORMQR.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(1) H(2) . . . H(k), where k = min(n,m).
*
* Each H(i) has the form
*
* H(i) = I - taua * v * v'
*
* where taua is a real scalar, and v is a real vector with
* v(1:i-1) = 0 and v(i) = 1; v(i+1:n) is stored on exit in A(i+1:n,i),
* and taua in TAUA(i).
* To form Q explicitly, use LAPACK subroutine SORGQR.
* To use Q to update another matrix, use LAPACK subroutine SORMQR.
*
* The matrix Z is represented as a product of elementary reflectors
*
* Z = H(1) H(2) . . . H(k), where k = min(n,p).
*
* Each H(i) has the form
*
* H(i) = I - taub * v * v'
*
* where taub is a real scalar, and v is a real vector with
* v(p-k+i+1:p) = 0 and v(p-k+i) = 1; v(1:p-k+i-1) is stored on exit in
* B(n-k+i,1:p-k+i-1), and taub in TAUB(i).
* To form Z explicitly, use LAPACK subroutine SORGRQ.
* To use Z to update another matrix, use LAPACK subroutine SORMRQ.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n
* @param m
* @param p
* @param a
* @param _a_offset
* @param lda
* @param taua
* @param _taua_offset
* @param b
* @param _b_offset
* @param ldb
* @param taub
* @param _taub_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sggqrf(int n, int m, int p, float[] a, int _a_offset, int lda, float[] taua, int _taua_offset, float[] b, int _b_offset, int ldb, float[] taub, int _taub_offset, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGGRQF computes a generalized RQ factorization of an M-by-N matrix A
* and a P-by-N matrix B:
*
* A = R*Q, B = Z*T*Q,
*
* where Q is an N-by-N orthogonal matrix, Z is a P-by-P orthogonal
* matrix, and R and T assume one of the forms:
*
* if M <= N, R = ( 0 R12 ) M, or if M > N, R = ( R11 ) M-N,
* N-M M ( R21 ) N
* N
*
* where R12 or R21 is upper triangular, and
*
* if P >= N, T = ( T11 ) N , or if P < N, T = ( T11 T12 ) P,
* ( 0 ) P-N P N-P
* N
*
* where T11 is upper triangular.
*
* In particular, if B is square and nonsingular, the GRQ factorization
* of A and B implicitly gives the RQ factorization of A*inv(B):
*
* A*inv(B) = (R*inv(T))*Z'
*
* where inv(B) denotes the inverse of the matrix B, and Z' denotes the
* transpose of the matrix Z.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* P (input) INTEGER
* The number of rows of the matrix B. P >= 0.
*
* N (input) INTEGER
* The number of columns of the matrices A and B. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, if M <= N, the upper triangle of the subarray
* A(1:M,N-M+1:N) contains the M-by-M upper triangular matrix R;
* if M > N, the elements on and above the (M-N)-th subdiagonal
* contain the M-by-N upper trapezoidal matrix R; the remaining
* elements, with the array TAUA, represent the orthogonal
* matrix Q as a product of elementary reflectors (see Further
* Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAUA (output) REAL array, dimension (min(M,N))
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix Q (see Further Details).
*
* B (input/output) REAL array, dimension (LDB,N)
* On entry, the P-by-N matrix B.
* On exit, the elements on and above the diagonal of the array
* contain the min(P,N)-by-N upper trapezoidal matrix T (T is
* upper triangular if P >= N); the elements below the diagonal,
* with the array TAUB, represent the orthogonal matrix Z as a
* product of elementary reflectors (see Further Details).
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,P).
*
* TAUB (output) REAL array, dimension (min(P,N))
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix Z (see Further Details).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N,M,P).
* For optimum performance LWORK >= max(N,M,P)*max(NB1,NB2,NB3),
* where NB1 is the optimal blocksize for the RQ factorization
* of an M-by-N matrix, NB2 is the optimal blocksize for the
* QR factorization of a P-by-N matrix, and NB3 is the optimal
* blocksize for a call of SORMRQ.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INF0= -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(1) H(2) . . . H(k), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - taua * v * v'
*
* where taua is a real scalar, and v is a real vector with
* v(n-k+i+1:n) = 0 and v(n-k+i) = 1; v(1:n-k+i-1) is stored on exit in
* A(m-k+i,1:n-k+i-1), and taua in TAUA(i).
* To form Q explicitly, use LAPACK subroutine SORGRQ.
* To use Q to update another matrix, use LAPACK subroutine SORMRQ.
*
* The matrix Z is represented as a product of elementary reflectors
*
* Z = H(1) H(2) . . . H(k), where k = min(p,n).
*
* Each H(i) has the form
*
* H(i) = I - taub * v * v'
*
* where taub is a real scalar, and v is a real vector with
* v(1:i-1) = 0 and v(i) = 1; v(i+1:p) is stored on exit in B(i+1:p,i),
* and taub in TAUB(i).
* To form Z explicitly, use LAPACK subroutine SORGQR.
* To use Z to update another matrix, use LAPACK subroutine SORMQR.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param m
* @param p
* @param n
* @param a
* @param lda
* @param taua
* @param b
* @param ldb
* @param taub
* @param work
* @param lwork
* @param info
*
*/
abstract public void sggrqf(int m, int p, int n, float[] a, int lda, float[] taua, float[] b, int ldb, float[] taub, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGGRQF computes a generalized RQ factorization of an M-by-N matrix A
* and a P-by-N matrix B:
*
* A = R*Q, B = Z*T*Q,
*
* where Q is an N-by-N orthogonal matrix, Z is a P-by-P orthogonal
* matrix, and R and T assume one of the forms:
*
* if M <= N, R = ( 0 R12 ) M, or if M > N, R = ( R11 ) M-N,
* N-M M ( R21 ) N
* N
*
* where R12 or R21 is upper triangular, and
*
* if P >= N, T = ( T11 ) N , or if P < N, T = ( T11 T12 ) P,
* ( 0 ) P-N P N-P
* N
*
* where T11 is upper triangular.
*
* In particular, if B is square and nonsingular, the GRQ factorization
* of A and B implicitly gives the RQ factorization of A*inv(B):
*
* A*inv(B) = (R*inv(T))*Z'
*
* where inv(B) denotes the inverse of the matrix B, and Z' denotes the
* transpose of the matrix Z.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* P (input) INTEGER
* The number of rows of the matrix B. P >= 0.
*
* N (input) INTEGER
* The number of columns of the matrices A and B. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, if M <= N, the upper triangle of the subarray
* A(1:M,N-M+1:N) contains the M-by-M upper triangular matrix R;
* if M > N, the elements on and above the (M-N)-th subdiagonal
* contain the M-by-N upper trapezoidal matrix R; the remaining
* elements, with the array TAUA, represent the orthogonal
* matrix Q as a product of elementary reflectors (see Further
* Details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAUA (output) REAL array, dimension (min(M,N))
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix Q (see Further Details).
*
* B (input/output) REAL array, dimension (LDB,N)
* On entry, the P-by-N matrix B.
* On exit, the elements on and above the diagonal of the array
* contain the min(P,N)-by-N upper trapezoidal matrix T (T is
* upper triangular if P >= N); the elements below the diagonal,
* with the array TAUB, represent the orthogonal matrix Z as a
* product of elementary reflectors (see Further Details).
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,P).
*
* TAUB (output) REAL array, dimension (min(P,N))
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix Z (see Further Details).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N,M,P).
* For optimum performance LWORK >= max(N,M,P)*max(NB1,NB2,NB3),
* where NB1 is the optimal blocksize for the RQ factorization
* of an M-by-N matrix, NB2 is the optimal blocksize for the
* QR factorization of a P-by-N matrix, and NB3 is the optimal
* blocksize for a call of SORMRQ.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INF0= -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of elementary reflectors
*
* Q = H(1) H(2) . . . H(k), where k = min(m,n).
*
* Each H(i) has the form
*
* H(i) = I - taua * v * v'
*
* where taua is a real scalar, and v is a real vector with
* v(n-k+i+1:n) = 0 and v(n-k+i) = 1; v(1:n-k+i-1) is stored on exit in
* A(m-k+i,1:n-k+i-1), and taua in TAUA(i).
* To form Q explicitly, use LAPACK subroutine SORGRQ.
* To use Q to update another matrix, use LAPACK subroutine SORMRQ.
*
* The matrix Z is represented as a product of elementary reflectors
*
* Z = H(1) H(2) . . . H(k), where k = min(p,n).
*
* Each H(i) has the form
*
* H(i) = I - taub * v * v'
*
* where taub is a real scalar, and v is a real vector with
* v(1:i-1) = 0 and v(i) = 1; v(i+1:p) is stored on exit in B(i+1:p,i),
* and taub in TAUB(i).
* To form Z explicitly, use LAPACK subroutine SORGQR.
* To use Z to update another matrix, use LAPACK subroutine SORMQR.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param m
* @param p
* @param n
* @param a
* @param _a_offset
* @param lda
* @param taua
* @param _taua_offset
* @param b
* @param _b_offset
* @param ldb
* @param taub
* @param _taub_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sggrqf(int m, int p, int n, float[] a, int _a_offset, int lda, float[] taua, int _taua_offset, float[] b, int _b_offset, int ldb, float[] taub, int _taub_offset, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGGSVD computes the generalized singular value decomposition (GSVD)
* of an M-by-N real matrix A and P-by-N real matrix B:
*
* U'*A*Q = D1*( 0 R ), V'*B*Q = D2*( 0 R )
*
* where U, V and Q are orthogonal matrices, and Z' is the transpose
* of Z. Let K+L = the effective numerical rank of the matrix (A',B')',
* then R is a K+L-by-K+L nonsingular upper triangular matrix, D1 and
* D2 are M-by-(K+L) and P-by-(K+L) "diagonal" matrices and of the
* following structures, respectively:
*
* If M-K-L >= 0,
*
* K L
* D1 = K ( I 0 )
* L ( 0 C )
* M-K-L ( 0 0 )
*
* K L
* D2 = L ( 0 S )
* P-L ( 0 0 )
*
* N-K-L K L
* ( 0 R ) = K ( 0 R11 R12 )
* L ( 0 0 R22 )
*
* where
*
* C = diag( ALPHA(K+1), ... , ALPHA(K+L) ),
* S = diag( BETA(K+1), ... , BETA(K+L) ),
* C**2 + S**2 = I.
*
* R is stored in A(1:K+L,N-K-L+1:N) on exit.
*
* If M-K-L < 0,
*
* K M-K K+L-M
* D1 = K ( I 0 0 )
* M-K ( 0 C 0 )
*
* K M-K K+L-M
* D2 = M-K ( 0 S 0 )
* K+L-M ( 0 0 I )
* P-L ( 0 0 0 )
*
* N-K-L K M-K K+L-M
* ( 0 R ) = K ( 0 R11 R12 R13 )
* M-K ( 0 0 R22 R23 )
* K+L-M ( 0 0 0 R33 )
*
* where
*
* C = diag( ALPHA(K+1), ... , ALPHA(M) ),
* S = diag( BETA(K+1), ... , BETA(M) ),
* C**2 + S**2 = I.
*
* (R11 R12 R13 ) is stored in A(1:M, N-K-L+1:N), and R33 is stored
* ( 0 R22 R23 )
* in B(M-K+1:L,N+M-K-L+1:N) on exit.
*
* The routine computes C, S, R, and optionally the orthogonal
* transformation matrices U, V and Q.
*
* In particular, if B is an N-by-N nonsingular matrix, then the GSVD of
* A and B implicitly gives the SVD of A*inv(B):
* A*inv(B) = U*(D1*inv(D2))*V'.
* If ( A',B')' has orthonormal columns, then the GSVD of A and B is
* also equal to the CS decomposition of A and B. Furthermore, the GSVD
* can be used to derive the solution of the eigenvalue problem:
* A'*A x = lambda* B'*B x.
* In some literature, the GSVD of A and B is presented in the form
* U'*A*X = ( 0 D1 ), V'*B*X = ( 0 D2 )
* where U and V are orthogonal and X is nonsingular, D1 and D2 are
* ``diagonal''. The former GSVD form can be converted to the latter
* form by taking the nonsingular matrix X as
*
* X = Q*( I 0 )
* ( 0 inv(R) ).
*
* Arguments
* =========
*
* JOBU (input) CHARACTER*1
* = 'U': Orthogonal matrix U is computed;
* = 'N': U is not computed.
*
* JOBV (input) CHARACTER*1
* = 'V': Orthogonal matrix V is computed;
* = 'N': V is not computed.
*
* JOBQ (input) CHARACTER*1
* = 'Q': Orthogonal matrix Q is computed;
* = 'N': Q is not computed.
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrices A and B. N >= 0.
*
* P (input) INTEGER
* The number of rows of the matrix B. P >= 0.
*
* K (output) INTEGER
* L (output) INTEGER
* On exit, K and L specify the dimension of the subblocks
* described in the Purpose section.
* K + L = effective numerical rank of (A',B')'.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, A contains the triangular matrix R, or part of R.
* See Purpose for details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) REAL array, dimension (LDB,N)
* On entry, the P-by-N matrix B.
* On exit, B contains the triangular matrix R if M-K-L < 0.
* See Purpose for details.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,P).
*
* ALPHA (output) REAL array, dimension (N)
* BETA (output) REAL array, dimension (N)
* On exit, ALPHA and BETA contain the generalized singular
* value pairs of A and B;
* ALPHA(1:K) = 1,
* BETA(1:K) = 0,
* and if M-K-L >= 0,
* ALPHA(K+1:K+L) = C,
* BETA(K+1:K+L) = S,
* or if M-K-L < 0,
* ALPHA(K+1:M)=C, ALPHA(M+1:K+L)=0
* BETA(K+1:M) =S, BETA(M+1:K+L) =1
* and
* ALPHA(K+L+1:N) = 0
* BETA(K+L+1:N) = 0
*
* U (output) REAL array, dimension (LDU,M)
* If JOBU = 'U', U contains the M-by-M orthogonal matrix U.
* If JOBU = 'N', U is not referenced.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= max(1,M) if
* JOBU = 'U'; LDU >= 1 otherwise.
*
* V (output) REAL array, dimension (LDV,P)
* If JOBV = 'V', V contains the P-by-P orthogonal matrix V.
* If JOBV = 'N', V is not referenced.
*
* LDV (input) INTEGER
* The leading dimension of the array V. LDV >= max(1,P) if
* JOBV = 'V'; LDV >= 1 otherwise.
*
* Q (output) REAL array, dimension (LDQ,N)
* If JOBQ = 'Q', Q contains the N-by-N orthogonal matrix Q.
* If JOBQ = 'N', Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N) if
* JOBQ = 'Q'; LDQ >= 1 otherwise.
*
* WORK (workspace) REAL array,
* dimension (max(3*N,M,P)+N)
*
* IWORK (workspace/output) INTEGER array, dimension (N)
* On exit, IWORK stores the sorting information. More
* precisely, the following loop will sort ALPHA
* for I = K+1, min(M,K+L)
* swap ALPHA(I) and ALPHA(IWORK(I))
* endfor
* such that ALPHA(1) >= ALPHA(2) >= ... >= ALPHA(N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, the Jacobi-type procedure failed to
* converge. For further details, see subroutine STGSJA.
*
* Internal Parameters
* ===================
*
* TOLA REAL
* TOLB REAL
* TOLA and TOLB are the thresholds to determine the effective
* rank of (A',B')'. Generally, they are set to
* TOLA = MAX(M,N)*norm(A)*MACHEPS,
* TOLB = MAX(P,N)*norm(B)*MACHEPS.
* The size of TOLA and TOLB may affect the size of backward
* errors of the decomposition.
*
* Further Details
* ===============
*
* 2-96 Based on modifications by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param jobu
* @param jobv
* @param jobq
* @param m
* @param n
* @param p
* @param k
* @param l
* @param a
* @param lda
* @param b
* @param ldb
* @param alpha
* @param beta
* @param u
* @param ldu
* @param v
* @param ldv
* @param q
* @param ldq
* @param work
* @param iwork
* @param info
*
*/
abstract public void sggsvd(java.lang.String jobu, java.lang.String jobv, java.lang.String jobq, int m, int n, int p, org.netlib.util.intW k, org.netlib.util.intW l, float[] a, int lda, float[] b, int ldb, float[] alpha, float[] beta, float[] u, int ldu, float[] v, int ldv, float[] q, int ldq, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGGSVD computes the generalized singular value decomposition (GSVD)
* of an M-by-N real matrix A and P-by-N real matrix B:
*
* U'*A*Q = D1*( 0 R ), V'*B*Q = D2*( 0 R )
*
* where U, V and Q are orthogonal matrices, and Z' is the transpose
* of Z. Let K+L = the effective numerical rank of the matrix (A',B')',
* then R is a K+L-by-K+L nonsingular upper triangular matrix, D1 and
* D2 are M-by-(K+L) and P-by-(K+L) "diagonal" matrices and of the
* following structures, respectively:
*
* If M-K-L >= 0,
*
* K L
* D1 = K ( I 0 )
* L ( 0 C )
* M-K-L ( 0 0 )
*
* K L
* D2 = L ( 0 S )
* P-L ( 0 0 )
*
* N-K-L K L
* ( 0 R ) = K ( 0 R11 R12 )
* L ( 0 0 R22 )
*
* where
*
* C = diag( ALPHA(K+1), ... , ALPHA(K+L) ),
* S = diag( BETA(K+1), ... , BETA(K+L) ),
* C**2 + S**2 = I.
*
* R is stored in A(1:K+L,N-K-L+1:N) on exit.
*
* If M-K-L < 0,
*
* K M-K K+L-M
* D1 = K ( I 0 0 )
* M-K ( 0 C 0 )
*
* K M-K K+L-M
* D2 = M-K ( 0 S 0 )
* K+L-M ( 0 0 I )
* P-L ( 0 0 0 )
*
* N-K-L K M-K K+L-M
* ( 0 R ) = K ( 0 R11 R12 R13 )
* M-K ( 0 0 R22 R23 )
* K+L-M ( 0 0 0 R33 )
*
* where
*
* C = diag( ALPHA(K+1), ... , ALPHA(M) ),
* S = diag( BETA(K+1), ... , BETA(M) ),
* C**2 + S**2 = I.
*
* (R11 R12 R13 ) is stored in A(1:M, N-K-L+1:N), and R33 is stored
* ( 0 R22 R23 )
* in B(M-K+1:L,N+M-K-L+1:N) on exit.
*
* The routine computes C, S, R, and optionally the orthogonal
* transformation matrices U, V and Q.
*
* In particular, if B is an N-by-N nonsingular matrix, then the GSVD of
* A and B implicitly gives the SVD of A*inv(B):
* A*inv(B) = U*(D1*inv(D2))*V'.
* If ( A',B')' has orthonormal columns, then the GSVD of A and B is
* also equal to the CS decomposition of A and B. Furthermore, the GSVD
* can be used to derive the solution of the eigenvalue problem:
* A'*A x = lambda* B'*B x.
* In some literature, the GSVD of A and B is presented in the form
* U'*A*X = ( 0 D1 ), V'*B*X = ( 0 D2 )
* where U and V are orthogonal and X is nonsingular, D1 and D2 are
* ``diagonal''. The former GSVD form can be converted to the latter
* form by taking the nonsingular matrix X as
*
* X = Q*( I 0 )
* ( 0 inv(R) ).
*
* Arguments
* =========
*
* JOBU (input) CHARACTER*1
* = 'U': Orthogonal matrix U is computed;
* = 'N': U is not computed.
*
* JOBV (input) CHARACTER*1
* = 'V': Orthogonal matrix V is computed;
* = 'N': V is not computed.
*
* JOBQ (input) CHARACTER*1
* = 'Q': Orthogonal matrix Q is computed;
* = 'N': Q is not computed.
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrices A and B. N >= 0.
*
* P (input) INTEGER
* The number of rows of the matrix B. P >= 0.
*
* K (output) INTEGER
* L (output) INTEGER
* On exit, K and L specify the dimension of the subblocks
* described in the Purpose section.
* K + L = effective numerical rank of (A',B')'.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, A contains the triangular matrix R, or part of R.
* See Purpose for details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) REAL array, dimension (LDB,N)
* On entry, the P-by-N matrix B.
* On exit, B contains the triangular matrix R if M-K-L < 0.
* See Purpose for details.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,P).
*
* ALPHA (output) REAL array, dimension (N)
* BETA (output) REAL array, dimension (N)
* On exit, ALPHA and BETA contain the generalized singular
* value pairs of A and B;
* ALPHA(1:K) = 1,
* BETA(1:K) = 0,
* and if M-K-L >= 0,
* ALPHA(K+1:K+L) = C,
* BETA(K+1:K+L) = S,
* or if M-K-L < 0,
* ALPHA(K+1:M)=C, ALPHA(M+1:K+L)=0
* BETA(K+1:M) =S, BETA(M+1:K+L) =1
* and
* ALPHA(K+L+1:N) = 0
* BETA(K+L+1:N) = 0
*
* U (output) REAL array, dimension (LDU,M)
* If JOBU = 'U', U contains the M-by-M orthogonal matrix U.
* If JOBU = 'N', U is not referenced.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= max(1,M) if
* JOBU = 'U'; LDU >= 1 otherwise.
*
* V (output) REAL array, dimension (LDV,P)
* If JOBV = 'V', V contains the P-by-P orthogonal matrix V.
* If JOBV = 'N', V is not referenced.
*
* LDV (input) INTEGER
* The leading dimension of the array V. LDV >= max(1,P) if
* JOBV = 'V'; LDV >= 1 otherwise.
*
* Q (output) REAL array, dimension (LDQ,N)
* If JOBQ = 'Q', Q contains the N-by-N orthogonal matrix Q.
* If JOBQ = 'N', Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N) if
* JOBQ = 'Q'; LDQ >= 1 otherwise.
*
* WORK (workspace) REAL array,
* dimension (max(3*N,M,P)+N)
*
* IWORK (workspace/output) INTEGER array, dimension (N)
* On exit, IWORK stores the sorting information. More
* precisely, the following loop will sort ALPHA
* for I = K+1, min(M,K+L)
* swap ALPHA(I) and ALPHA(IWORK(I))
* endfor
* such that ALPHA(1) >= ALPHA(2) >= ... >= ALPHA(N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, the Jacobi-type procedure failed to
* converge. For further details, see subroutine STGSJA.
*
* Internal Parameters
* ===================
*
* TOLA REAL
* TOLB REAL
* TOLA and TOLB are the thresholds to determine the effective
* rank of (A',B')'. Generally, they are set to
* TOLA = MAX(M,N)*norm(A)*MACHEPS,
* TOLB = MAX(P,N)*norm(B)*MACHEPS.
* The size of TOLA and TOLB may affect the size of backward
* errors of the decomposition.
*
* Further Details
* ===============
*
* 2-96 Based on modifications by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param jobu
* @param jobv
* @param jobq
* @param m
* @param n
* @param p
* @param k
* @param l
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param alpha
* @param _alpha_offset
* @param beta
* @param _beta_offset
* @param u
* @param _u_offset
* @param ldu
* @param v
* @param _v_offset
* @param ldv
* @param q
* @param _q_offset
* @param ldq
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void sggsvd(java.lang.String jobu, java.lang.String jobv, java.lang.String jobq, int m, int n, int p, org.netlib.util.intW k, org.netlib.util.intW l, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, float[] alpha, int _alpha_offset, float[] beta, int _beta_offset, float[] u, int _u_offset, int ldu, float[] v, int _v_offset, int ldv, float[] q, int _q_offset, int ldq, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGGSVP computes orthogonal matrices U, V and Q such that
*
* N-K-L K L
* U'*A*Q = K ( 0 A12 A13 ) if M-K-L >= 0;
* L ( 0 0 A23 )
* M-K-L ( 0 0 0 )
*
* N-K-L K L
* = K ( 0 A12 A13 ) if M-K-L < 0;
* M-K ( 0 0 A23 )
*
* N-K-L K L
* V'*B*Q = L ( 0 0 B13 )
* P-L ( 0 0 0 )
*
* where the K-by-K matrix A12 and L-by-L matrix B13 are nonsingular
* upper triangular; A23 is L-by-L upper triangular if M-K-L >= 0,
* otherwise A23 is (M-K)-by-L upper trapezoidal. K+L = the effective
* numerical rank of the (M+P)-by-N matrix (A',B')'. Z' denotes the
* transpose of Z.
*
* This decomposition is the preprocessing step for computing the
* Generalized Singular Value Decomposition (GSVD), see subroutine
* SGGSVD.
*
* Arguments
* =========
*
* JOBU (input) CHARACTER*1
* = 'U': Orthogonal matrix U is computed;
* = 'N': U is not computed.
*
* JOBV (input) CHARACTER*1
* = 'V': Orthogonal matrix V is computed;
* = 'N': V is not computed.
*
* JOBQ (input) CHARACTER*1
* = 'Q': Orthogonal matrix Q is computed;
* = 'N': Q is not computed.
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* P (input) INTEGER
* The number of rows of the matrix B. P >= 0.
*
* N (input) INTEGER
* The number of columns of the matrices A and B. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, A contains the triangular (or trapezoidal) matrix
* described in the Purpose section.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) REAL array, dimension (LDB,N)
* On entry, the P-by-N matrix B.
* On exit, B contains the triangular matrix described in
* the Purpose section.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,P).
*
* TOLA (input) REAL
* TOLB (input) REAL
* TOLA and TOLB are the thresholds to determine the effective
* numerical rank of matrix B and a subblock of A. Generally,
* they are set to
* TOLA = MAX(M,N)*norm(A)*MACHEPS,
* TOLB = MAX(P,N)*norm(B)*MACHEPS.
* The size of TOLA and TOLB may affect the size of backward
* errors of the decomposition.
*
* K (output) INTEGER
* L (output) INTEGER
* On exit, K and L specify the dimension of the subblocks
* described in Purpose.
* K + L = effective numerical rank of (A',B')'.
*
* U (output) REAL array, dimension (LDU,M)
* If JOBU = 'U', U contains the orthogonal matrix U.
* If JOBU = 'N', U is not referenced.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= max(1,M) if
* JOBU = 'U'; LDU >= 1 otherwise.
*
* V (output) REAL array, dimension (LDV,M)
* If JOBV = 'V', V contains the orthogonal matrix V.
* If JOBV = 'N', V is not referenced.
*
* LDV (input) INTEGER
* The leading dimension of the array V. LDV >= max(1,P) if
* JOBV = 'V'; LDV >= 1 otherwise.
*
* Q (output) REAL array, dimension (LDQ,N)
* If JOBQ = 'Q', Q contains the orthogonal matrix Q.
* If JOBQ = 'N', Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N) if
* JOBQ = 'Q'; LDQ >= 1 otherwise.
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* TAU (workspace) REAL array, dimension (N)
*
* WORK (workspace) REAL array, dimension (max(3*N,M,P))
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
*
* Further Details
* ===============
*
* The subroutine uses LAPACK subroutine SGEQPF for the QR factorization
* with column pivoting to detect the effective numerical rank of the
* a matrix. It may be replaced by a better rank determination strategy.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobu
* @param jobv
* @param jobq
* @param m
* @param p
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param tola
* @param tolb
* @param k
* @param l
* @param u
* @param ldu
* @param v
* @param ldv
* @param q
* @param ldq
* @param iwork
* @param tau
* @param work
* @param info
*
*/
abstract public void sggsvp(java.lang.String jobu, java.lang.String jobv, java.lang.String jobq, int m, int p, int n, float[] a, int lda, float[] b, int ldb, float tola, float tolb, org.netlib.util.intW k, org.netlib.util.intW l, float[] u, int ldu, float[] v, int ldv, float[] q, int ldq, int[] iwork, float[] tau, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGGSVP computes orthogonal matrices U, V and Q such that
*
* N-K-L K L
* U'*A*Q = K ( 0 A12 A13 ) if M-K-L >= 0;
* L ( 0 0 A23 )
* M-K-L ( 0 0 0 )
*
* N-K-L K L
* = K ( 0 A12 A13 ) if M-K-L < 0;
* M-K ( 0 0 A23 )
*
* N-K-L K L
* V'*B*Q = L ( 0 0 B13 )
* P-L ( 0 0 0 )
*
* where the K-by-K matrix A12 and L-by-L matrix B13 are nonsingular
* upper triangular; A23 is L-by-L upper triangular if M-K-L >= 0,
* otherwise A23 is (M-K)-by-L upper trapezoidal. K+L = the effective
* numerical rank of the (M+P)-by-N matrix (A',B')'. Z' denotes the
* transpose of Z.
*
* This decomposition is the preprocessing step for computing the
* Generalized Singular Value Decomposition (GSVD), see subroutine
* SGGSVD.
*
* Arguments
* =========
*
* JOBU (input) CHARACTER*1
* = 'U': Orthogonal matrix U is computed;
* = 'N': U is not computed.
*
* JOBV (input) CHARACTER*1
* = 'V': Orthogonal matrix V is computed;
* = 'N': V is not computed.
*
* JOBQ (input) CHARACTER*1
* = 'Q': Orthogonal matrix Q is computed;
* = 'N': Q is not computed.
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* P (input) INTEGER
* The number of rows of the matrix B. P >= 0.
*
* N (input) INTEGER
* The number of columns of the matrices A and B. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, A contains the triangular (or trapezoidal) matrix
* described in the Purpose section.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) REAL array, dimension (LDB,N)
* On entry, the P-by-N matrix B.
* On exit, B contains the triangular matrix described in
* the Purpose section.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,P).
*
* TOLA (input) REAL
* TOLB (input) REAL
* TOLA and TOLB are the thresholds to determine the effective
* numerical rank of matrix B and a subblock of A. Generally,
* they are set to
* TOLA = MAX(M,N)*norm(A)*MACHEPS,
* TOLB = MAX(P,N)*norm(B)*MACHEPS.
* The size of TOLA and TOLB may affect the size of backward
* errors of the decomposition.
*
* K (output) INTEGER
* L (output) INTEGER
* On exit, K and L specify the dimension of the subblocks
* described in Purpose.
* K + L = effective numerical rank of (A',B')'.
*
* U (output) REAL array, dimension (LDU,M)
* If JOBU = 'U', U contains the orthogonal matrix U.
* If JOBU = 'N', U is not referenced.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= max(1,M) if
* JOBU = 'U'; LDU >= 1 otherwise.
*
* V (output) REAL array, dimension (LDV,M)
* If JOBV = 'V', V contains the orthogonal matrix V.
* If JOBV = 'N', V is not referenced.
*
* LDV (input) INTEGER
* The leading dimension of the array V. LDV >= max(1,P) if
* JOBV = 'V'; LDV >= 1 otherwise.
*
* Q (output) REAL array, dimension (LDQ,N)
* If JOBQ = 'Q', Q contains the orthogonal matrix Q.
* If JOBQ = 'N', Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N) if
* JOBQ = 'Q'; LDQ >= 1 otherwise.
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* TAU (workspace) REAL array, dimension (N)
*
* WORK (workspace) REAL array, dimension (max(3*N,M,P))
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
*
* Further Details
* ===============
*
* The subroutine uses LAPACK subroutine SGEQPF for the QR factorization
* with column pivoting to detect the effective numerical rank of the
* a matrix. It may be replaced by a better rank determination strategy.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobu
* @param jobv
* @param jobq
* @param m
* @param p
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param tola
* @param tolb
* @param k
* @param l
* @param u
* @param _u_offset
* @param ldu
* @param v
* @param _v_offset
* @param ldv
* @param q
* @param _q_offset
* @param ldq
* @param iwork
* @param _iwork_offset
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void sggsvp(java.lang.String jobu, java.lang.String jobv, java.lang.String jobq, int m, int p, int n, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, float tola, float tolb, org.netlib.util.intW k, org.netlib.util.intW l, float[] u, int _u_offset, int ldu, float[] v, int _v_offset, int ldv, float[] q, int _q_offset, int ldq, int[] iwork, int _iwork_offset, float[] tau, int _tau_offset, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGTCON estimates the reciprocal of the condition number of a real
* tridiagonal matrix A using the LU factorization as computed by
* SGTTRF.
*
* An estimate is obtained for norm(inv(A)), and the reciprocal of the
* condition number is computed as RCOND = 1 / (ANORM * norm(inv(A))).
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies whether the 1-norm condition number or the
* infinity-norm condition number is required:
* = '1' or 'O': 1-norm;
* = 'I': Infinity-norm.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* DL (input) REAL array, dimension (N-1)
* The (n-1) multipliers that define the matrix L from the
* LU factorization of A as computed by SGTTRF.
*
* D (input) REAL array, dimension (N)
* The n diagonal elements of the upper triangular matrix U from
* the LU factorization of A.
*
* DU (input) REAL array, dimension (N-1)
* The (n-1) elements of the first superdiagonal of U.
*
* DU2 (input) REAL array, dimension (N-2)
* The (n-2) elements of the second superdiagonal of U.
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices; for 1 <= i <= n, row i of the matrix was
* interchanged with row IPIV(i). IPIV(i) will always be either
* i or i+1; IPIV(i) = i indicates a row interchange was not
* required.
*
* ANORM (input) REAL
* If NORM = '1' or 'O', the 1-norm of the original matrix A.
* If NORM = 'I', the infinity-norm of the original matrix A.
*
* RCOND (output) REAL
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(ANORM * AINVNM), where AINVNM is an
* estimate of the 1-norm of inv(A) computed in this routine.
*
* WORK (workspace) REAL array, dimension (2*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param n
* @param dl
* @param d
* @param du
* @param du2
* @param ipiv
* @param anorm
* @param rcond
* @param work
* @param iwork
* @param info
*
*/
abstract public void sgtcon(java.lang.String norm, int n, float[] dl, float[] d, float[] du, float[] du2, int[] ipiv, float anorm, org.netlib.util.floatW rcond, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGTCON estimates the reciprocal of the condition number of a real
* tridiagonal matrix A using the LU factorization as computed by
* SGTTRF.
*
* An estimate is obtained for norm(inv(A)), and the reciprocal of the
* condition number is computed as RCOND = 1 / (ANORM * norm(inv(A))).
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies whether the 1-norm condition number or the
* infinity-norm condition number is required:
* = '1' or 'O': 1-norm;
* = 'I': Infinity-norm.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* DL (input) REAL array, dimension (N-1)
* The (n-1) multipliers that define the matrix L from the
* LU factorization of A as computed by SGTTRF.
*
* D (input) REAL array, dimension (N)
* The n diagonal elements of the upper triangular matrix U from
* the LU factorization of A.
*
* DU (input) REAL array, dimension (N-1)
* The (n-1) elements of the first superdiagonal of U.
*
* DU2 (input) REAL array, dimension (N-2)
* The (n-2) elements of the second superdiagonal of U.
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices; for 1 <= i <= n, row i of the matrix was
* interchanged with row IPIV(i). IPIV(i) will always be either
* i or i+1; IPIV(i) = i indicates a row interchange was not
* required.
*
* ANORM (input) REAL
* If NORM = '1' or 'O', the 1-norm of the original matrix A.
* If NORM = 'I', the infinity-norm of the original matrix A.
*
* RCOND (output) REAL
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(ANORM * AINVNM), where AINVNM is an
* estimate of the 1-norm of inv(A) computed in this routine.
*
* WORK (workspace) REAL array, dimension (2*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param n
* @param dl
* @param _dl_offset
* @param d
* @param _d_offset
* @param du
* @param _du_offset
* @param du2
* @param _du2_offset
* @param ipiv
* @param _ipiv_offset
* @param anorm
* @param rcond
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void sgtcon(java.lang.String norm, int n, float[] dl, int _dl_offset, float[] d, int _d_offset, float[] du, int _du_offset, float[] du2, int _du2_offset, int[] ipiv, int _ipiv_offset, float anorm, org.netlib.util.floatW rcond, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGTRFS improves the computed solution to a system of linear
* equations when the coefficient matrix is tridiagonal, and provides
* error bounds and backward error estimates for the solution.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* DL (input) REAL array, dimension (N-1)
* The (n-1) subdiagonal elements of A.
*
* D (input) REAL array, dimension (N)
* The diagonal elements of A.
*
* DU (input) REAL array, dimension (N-1)
* The (n-1) superdiagonal elements of A.
*
* DLF (input) REAL array, dimension (N-1)
* The (n-1) multipliers that define the matrix L from the
* LU factorization of A as computed by SGTTRF.
*
* DF (input) REAL array, dimension (N)
* The n diagonal elements of the upper triangular matrix U from
* the LU factorization of A.
*
* DUF (input) REAL array, dimension (N-1)
* The (n-1) elements of the first superdiagonal of U.
*
* DU2 (input) REAL array, dimension (N-2)
* The (n-2) elements of the second superdiagonal of U.
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices; for 1 <= i <= n, row i of the matrix was
* interchanged with row IPIV(i). IPIV(i) will always be either
* i or i+1; IPIV(i) = i indicates a row interchange was not
* required.
*
* B (input) REAL array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) REAL array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by SGTTRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param trans
* @param n
* @param nrhs
* @param dl
* @param d
* @param du
* @param dlf
* @param df
* @param duf
* @param du2
* @param ipiv
* @param b
* @param ldb
* @param x
* @param ldx
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void sgtrfs(java.lang.String trans, int n, int nrhs, float[] dl, float[] d, float[] du, float[] dlf, float[] df, float[] duf, float[] du2, int[] ipiv, float[] b, int ldb, float[] x, int ldx, float[] ferr, float[] berr, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGTRFS improves the computed solution to a system of linear
* equations when the coefficient matrix is tridiagonal, and provides
* error bounds and backward error estimates for the solution.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* DL (input) REAL array, dimension (N-1)
* The (n-1) subdiagonal elements of A.
*
* D (input) REAL array, dimension (N)
* The diagonal elements of A.
*
* DU (input) REAL array, dimension (N-1)
* The (n-1) superdiagonal elements of A.
*
* DLF (input) REAL array, dimension (N-1)
* The (n-1) multipliers that define the matrix L from the
* LU factorization of A as computed by SGTTRF.
*
* DF (input) REAL array, dimension (N)
* The n diagonal elements of the upper triangular matrix U from
* the LU factorization of A.
*
* DUF (input) REAL array, dimension (N-1)
* The (n-1) elements of the first superdiagonal of U.
*
* DU2 (input) REAL array, dimension (N-2)
* The (n-2) elements of the second superdiagonal of U.
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices; for 1 <= i <= n, row i of the matrix was
* interchanged with row IPIV(i). IPIV(i) will always be either
* i or i+1; IPIV(i) = i indicates a row interchange was not
* required.
*
* B (input) REAL array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) REAL array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by SGTTRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param trans
* @param n
* @param nrhs
* @param dl
* @param _dl_offset
* @param d
* @param _d_offset
* @param du
* @param _du_offset
* @param dlf
* @param _dlf_offset
* @param df
* @param _df_offset
* @param duf
* @param _duf_offset
* @param du2
* @param _du2_offset
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void sgtrfs(java.lang.String trans, int n, int nrhs, float[] dl, int _dl_offset, float[] d, int _d_offset, float[] du, int _du_offset, float[] dlf, int _dlf_offset, float[] df, int _df_offset, float[] duf, int _duf_offset, float[] du2, int _du2_offset, int[] ipiv, int _ipiv_offset, float[] b, int _b_offset, int ldb, float[] x, int _x_offset, int ldx, float[] ferr, int _ferr_offset, float[] berr, int _berr_offset, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGTSV solves the equation
*
* A*X = B,
*
* where A is an n by n tridiagonal matrix, by Gaussian elimination with
* partial pivoting.
*
* Note that the equation A'*X = B may be solved by interchanging the
* order of the arguments DU and DL.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* DL (input/output) REAL array, dimension (N-1)
* On entry, DL must contain the (n-1) sub-diagonal elements of
* A.
*
* On exit, DL is overwritten by the (n-2) elements of the
* second super-diagonal of the upper triangular matrix U from
* the LU factorization of A, in DL(1), ..., DL(n-2).
*
* D (input/output) REAL array, dimension (N)
* On entry, D must contain the diagonal elements of A.
*
* On exit, D is overwritten by the n diagonal elements of U.
*
* DU (input/output) REAL array, dimension (N-1)
* On entry, DU must contain the (n-1) super-diagonal elements
* of A.
*
* On exit, DU is overwritten by the (n-1) elements of the first
* super-diagonal of U.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the N by NRHS matrix of right hand side matrix B.
* On exit, if INFO = 0, the N by NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, U(i,i) is exactly zero, and the solution
* has not been computed. The factorization has not been
* completed unless i = N.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param nrhs
* @param dl
* @param d
* @param du
* @param b
* @param ldb
* @param info
*
*/
abstract public void sgtsv(int n, int nrhs, float[] dl, float[] d, float[] du, float[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGTSV solves the equation
*
* A*X = B,
*
* where A is an n by n tridiagonal matrix, by Gaussian elimination with
* partial pivoting.
*
* Note that the equation A'*X = B may be solved by interchanging the
* order of the arguments DU and DL.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* DL (input/output) REAL array, dimension (N-1)
* On entry, DL must contain the (n-1) sub-diagonal elements of
* A.
*
* On exit, DL is overwritten by the (n-2) elements of the
* second super-diagonal of the upper triangular matrix U from
* the LU factorization of A, in DL(1), ..., DL(n-2).
*
* D (input/output) REAL array, dimension (N)
* On entry, D must contain the diagonal elements of A.
*
* On exit, D is overwritten by the n diagonal elements of U.
*
* DU (input/output) REAL array, dimension (N-1)
* On entry, DU must contain the (n-1) super-diagonal elements
* of A.
*
* On exit, DU is overwritten by the (n-1) elements of the first
* super-diagonal of U.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the N by NRHS matrix of right hand side matrix B.
* On exit, if INFO = 0, the N by NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, U(i,i) is exactly zero, and the solution
* has not been computed. The factorization has not been
* completed unless i = N.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param nrhs
* @param dl
* @param _dl_offset
* @param d
* @param _d_offset
* @param du
* @param _du_offset
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void sgtsv(int n, int nrhs, float[] dl, int _dl_offset, float[] d, int _d_offset, float[] du, int _du_offset, float[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGTSVX uses the LU factorization to compute the solution to a real
* system of linear equations A * X = B or A**T * X = B,
* where A is a tridiagonal matrix of order N and X and B are N-by-NRHS
* matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed:
*
* 1. If FACT = 'N', the LU decomposition is used to factor the matrix A
* as A = L * U, where L is a product of permutation and unit lower
* bidiagonal matrices and U is upper triangular with nonzeros in
* only the main diagonal and first two superdiagonals.
*
* 2. If some U(i,i)=0, so that U is exactly singular, then the routine
* returns with INFO = i. Otherwise, the factored form of A is used
* to estimate the condition number of the matrix A. If the
* reciprocal of the condition number is less than machine precision,
* INFO = N+1 is returned as a warning, but the routine still goes on
* to solve for X and compute error bounds as described below.
*
* 3. The system of equations is solved for X using the factored form
* of A.
*
* 4. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of A has been
* supplied on entry.
* = 'F': DLF, DF, DUF, DU2, and IPIV contain the factored
* form of A; DL, D, DU, DLF, DF, DUF, DU2 and IPIV
* will not be modified.
* = 'N': The matrix will be copied to DLF, DF, and DUF
* and factored.
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* DL (input) REAL array, dimension (N-1)
* The (n-1) subdiagonal elements of A.
*
* D (input) REAL array, dimension (N)
* The n diagonal elements of A.
*
* DU (input) REAL array, dimension (N-1)
* The (n-1) superdiagonal elements of A.
*
* DLF (input or output) REAL array, dimension (N-1)
* If FACT = 'F', then DLF is an input argument and on entry
* contains the (n-1) multipliers that define the matrix L from
* the LU factorization of A as computed by SGTTRF.
*
* If FACT = 'N', then DLF is an output argument and on exit
* contains the (n-1) multipliers that define the matrix L from
* the LU factorization of A.
*
* DF (input or output) REAL array, dimension (N)
* If FACT = 'F', then DF is an input argument and on entry
* contains the n diagonal elements of the upper triangular
* matrix U from the LU factorization of A.
*
* If FACT = 'N', then DF is an output argument and on exit
* contains the n diagonal elements of the upper triangular
* matrix U from the LU factorization of A.
*
* DUF (input or output) REAL array, dimension (N-1)
* If FACT = 'F', then DUF is an input argument and on entry
* contains the (n-1) elements of the first superdiagonal of U.
*
* If FACT = 'N', then DUF is an output argument and on exit
* contains the (n-1) elements of the first superdiagonal of U.
*
* DU2 (input or output) REAL array, dimension (N-2)
* If FACT = 'F', then DU2 is an input argument and on entry
* contains the (n-2) elements of the second superdiagonal of
* U.
*
* If FACT = 'N', then DU2 is an output argument and on exit
* contains the (n-2) elements of the second superdiagonal of
* U.
*
* IPIV (input or output) INTEGER array, dimension (N)
* If FACT = 'F', then IPIV is an input argument and on entry
* contains the pivot indices from the LU factorization of A as
* computed by SGTTRF.
*
* If FACT = 'N', then IPIV is an output argument and on exit
* contains the pivot indices from the LU factorization of A;
* row i of the matrix was interchanged with row IPIV(i).
* IPIV(i) will always be either i or i+1; IPIV(i) = i indicates
* a row interchange was not required.
*
* B (input) REAL array, dimension (LDB,NRHS)
* The N-by-NRHS right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) REAL array, dimension (LDX,NRHS)
* If INFO = 0 or INFO = N+1, the N-by-NRHS solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) REAL
* The estimate of the reciprocal condition number of the matrix
* A. If RCOND is less than the machine precision (in
* particular, if RCOND = 0), the matrix is singular to working
* precision. This condition is indicated by a return code of
* INFO > 0.
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= N: U(i,i) is exactly zero. The factorization
* has not been completed unless i = N, but the
* factor U is exactly singular, so the solution
* and error bounds could not be computed.
* RCOND = 0 is returned.
* = N+1: U is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param trans
* @param n
* @param nrhs
* @param dl
* @param d
* @param du
* @param dlf
* @param df
* @param duf
* @param du2
* @param ipiv
* @param b
* @param ldb
* @param x
* @param ldx
* @param rcond
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void sgtsvx(java.lang.String fact, java.lang.String trans, int n, int nrhs, float[] dl, float[] d, float[] du, float[] dlf, float[] df, float[] duf, float[] du2, int[] ipiv, float[] b, int ldb, float[] x, int ldx, org.netlib.util.floatW rcond, float[] ferr, float[] berr, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGTSVX uses the LU factorization to compute the solution to a real
* system of linear equations A * X = B or A**T * X = B,
* where A is a tridiagonal matrix of order N and X and B are N-by-NRHS
* matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed:
*
* 1. If FACT = 'N', the LU decomposition is used to factor the matrix A
* as A = L * U, where L is a product of permutation and unit lower
* bidiagonal matrices and U is upper triangular with nonzeros in
* only the main diagonal and first two superdiagonals.
*
* 2. If some U(i,i)=0, so that U is exactly singular, then the routine
* returns with INFO = i. Otherwise, the factored form of A is used
* to estimate the condition number of the matrix A. If the
* reciprocal of the condition number is less than machine precision,
* INFO = N+1 is returned as a warning, but the routine still goes on
* to solve for X and compute error bounds as described below.
*
* 3. The system of equations is solved for X using the factored form
* of A.
*
* 4. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of A has been
* supplied on entry.
* = 'F': DLF, DF, DUF, DU2, and IPIV contain the factored
* form of A; DL, D, DU, DLF, DF, DUF, DU2 and IPIV
* will not be modified.
* = 'N': The matrix will be copied to DLF, DF, and DUF
* and factored.
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* DL (input) REAL array, dimension (N-1)
* The (n-1) subdiagonal elements of A.
*
* D (input) REAL array, dimension (N)
* The n diagonal elements of A.
*
* DU (input) REAL array, dimension (N-1)
* The (n-1) superdiagonal elements of A.
*
* DLF (input or output) REAL array, dimension (N-1)
* If FACT = 'F', then DLF is an input argument and on entry
* contains the (n-1) multipliers that define the matrix L from
* the LU factorization of A as computed by SGTTRF.
*
* If FACT = 'N', then DLF is an output argument and on exit
* contains the (n-1) multipliers that define the matrix L from
* the LU factorization of A.
*
* DF (input or output) REAL array, dimension (N)
* If FACT = 'F', then DF is an input argument and on entry
* contains the n diagonal elements of the upper triangular
* matrix U from the LU factorization of A.
*
* If FACT = 'N', then DF is an output argument and on exit
* contains the n diagonal elements of the upper triangular
* matrix U from the LU factorization of A.
*
* DUF (input or output) REAL array, dimension (N-1)
* If FACT = 'F', then DUF is an input argument and on entry
* contains the (n-1) elements of the first superdiagonal of U.
*
* If FACT = 'N', then DUF is an output argument and on exit
* contains the (n-1) elements of the first superdiagonal of U.
*
* DU2 (input or output) REAL array, dimension (N-2)
* If FACT = 'F', then DU2 is an input argument and on entry
* contains the (n-2) elements of the second superdiagonal of
* U.
*
* If FACT = 'N', then DU2 is an output argument and on exit
* contains the (n-2) elements of the second superdiagonal of
* U.
*
* IPIV (input or output) INTEGER array, dimension (N)
* If FACT = 'F', then IPIV is an input argument and on entry
* contains the pivot indices from the LU factorization of A as
* computed by SGTTRF.
*
* If FACT = 'N', then IPIV is an output argument and on exit
* contains the pivot indices from the LU factorization of A;
* row i of the matrix was interchanged with row IPIV(i).
* IPIV(i) will always be either i or i+1; IPIV(i) = i indicates
* a row interchange was not required.
*
* B (input) REAL array, dimension (LDB,NRHS)
* The N-by-NRHS right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) REAL array, dimension (LDX,NRHS)
* If INFO = 0 or INFO = N+1, the N-by-NRHS solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) REAL
* The estimate of the reciprocal condition number of the matrix
* A. If RCOND is less than the machine precision (in
* particular, if RCOND = 0), the matrix is singular to working
* precision. This condition is indicated by a return code of
* INFO > 0.
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= N: U(i,i) is exactly zero. The factorization
* has not been completed unless i = N, but the
* factor U is exactly singular, so the solution
* and error bounds could not be computed.
* RCOND = 0 is returned.
* = N+1: U is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param trans
* @param n
* @param nrhs
* @param dl
* @param _dl_offset
* @param d
* @param _d_offset
* @param du
* @param _du_offset
* @param dlf
* @param _dlf_offset
* @param df
* @param _df_offset
* @param duf
* @param _duf_offset
* @param du2
* @param _du2_offset
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param rcond
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void sgtsvx(java.lang.String fact, java.lang.String trans, int n, int nrhs, float[] dl, int _dl_offset, float[] d, int _d_offset, float[] du, int _du_offset, float[] dlf, int _dlf_offset, float[] df, int _df_offset, float[] duf, int _duf_offset, float[] du2, int _du2_offset, int[] ipiv, int _ipiv_offset, float[] b, int _b_offset, int ldb, float[] x, int _x_offset, int ldx, org.netlib.util.floatW rcond, float[] ferr, int _ferr_offset, float[] berr, int _berr_offset, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGTTRF computes an LU factorization of a real tridiagonal matrix A
* using elimination with partial pivoting and row interchanges.
*
* The factorization has the form
* A = L * U
* where L is a product of permutation and unit lower bidiagonal
* matrices and U is upper triangular with nonzeros in only the main
* diagonal and first two superdiagonals.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A.
*
* DL (input/output) REAL array, dimension (N-1)
* On entry, DL must contain the (n-1) sub-diagonal elements of
* A.
*
* On exit, DL is overwritten by the (n-1) multipliers that
* define the matrix L from the LU factorization of A.
*
* D (input/output) REAL array, dimension (N)
* On entry, D must contain the diagonal elements of A.
*
* On exit, D is overwritten by the n diagonal elements of the
* upper triangular matrix U from the LU factorization of A.
*
* DU (input/output) REAL array, dimension (N-1)
* On entry, DU must contain the (n-1) super-diagonal elements
* of A.
*
* On exit, DU is overwritten by the (n-1) elements of the first
* super-diagonal of U.
*
* DU2 (output) REAL array, dimension (N-2)
* On exit, DU2 is overwritten by the (n-2) elements of the
* second super-diagonal of U.
*
* IPIV (output) INTEGER array, dimension (N)
* The pivot indices; for 1 <= i <= n, row i of the matrix was
* interchanged with row IPIV(i). IPIV(i) will always be either
* i or i+1; IPIV(i) = i indicates a row interchange was not
* required.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
* > 0: if INFO = k, U(k,k) is exactly zero. The factorization
* has been completed, but the factor U is exactly
* singular, and division by zero will occur if it is used
* to solve a system of equations.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param dl
* @param d
* @param du
* @param du2
* @param ipiv
* @param info
*
*/
abstract public void sgttrf(int n, float[] dl, float[] d, float[] du, float[] du2, int[] ipiv, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGTTRF computes an LU factorization of a real tridiagonal matrix A
* using elimination with partial pivoting and row interchanges.
*
* The factorization has the form
* A = L * U
* where L is a product of permutation and unit lower bidiagonal
* matrices and U is upper triangular with nonzeros in only the main
* diagonal and first two superdiagonals.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A.
*
* DL (input/output) REAL array, dimension (N-1)
* On entry, DL must contain the (n-1) sub-diagonal elements of
* A.
*
* On exit, DL is overwritten by the (n-1) multipliers that
* define the matrix L from the LU factorization of A.
*
* D (input/output) REAL array, dimension (N)
* On entry, D must contain the diagonal elements of A.
*
* On exit, D is overwritten by the n diagonal elements of the
* upper triangular matrix U from the LU factorization of A.
*
* DU (input/output) REAL array, dimension (N-1)
* On entry, DU must contain the (n-1) super-diagonal elements
* of A.
*
* On exit, DU is overwritten by the (n-1) elements of the first
* super-diagonal of U.
*
* DU2 (output) REAL array, dimension (N-2)
* On exit, DU2 is overwritten by the (n-2) elements of the
* second super-diagonal of U.
*
* IPIV (output) INTEGER array, dimension (N)
* The pivot indices; for 1 <= i <= n, row i of the matrix was
* interchanged with row IPIV(i). IPIV(i) will always be either
* i or i+1; IPIV(i) = i indicates a row interchange was not
* required.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
* > 0: if INFO = k, U(k,k) is exactly zero. The factorization
* has been completed, but the factor U is exactly
* singular, and division by zero will occur if it is used
* to solve a system of equations.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param dl
* @param _dl_offset
* @param d
* @param _d_offset
* @param du
* @param _du_offset
* @param du2
* @param _du2_offset
* @param ipiv
* @param _ipiv_offset
* @param info
*
*/
abstract public void sgttrf(int n, float[] dl, int _dl_offset, float[] d, int _d_offset, float[] du, int _du_offset, float[] du2, int _du2_offset, int[] ipiv, int _ipiv_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGTTRS solves one of the systems of equations
* A*X = B or A'*X = B,
* with a tridiagonal matrix A using the LU factorization computed
* by SGTTRF.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations.
* = 'N': A * X = B (No transpose)
* = 'T': A'* X = B (Transpose)
* = 'C': A'* X = B (Conjugate transpose = Transpose)
*
* N (input) INTEGER
* The order of the matrix A.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* DL (input) REAL array, dimension (N-1)
* The (n-1) multipliers that define the matrix L from the
* LU factorization of A.
*
* D (input) REAL array, dimension (N)
* The n diagonal elements of the upper triangular matrix U from
* the LU factorization of A.
*
* DU (input) REAL array, dimension (N-1)
* The (n-1) elements of the first super-diagonal of U.
*
* DU2 (input) REAL array, dimension (N-2)
* The (n-2) elements of the second super-diagonal of U.
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices; for 1 <= i <= n, row i of the matrix was
* interchanged with row IPIV(i). IPIV(i) will always be either
* i or i+1; IPIV(i) = i indicates a row interchange was not
* required.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the matrix of right hand side vectors B.
* On exit, B is overwritten by the solution vectors X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param trans
* @param n
* @param nrhs
* @param dl
* @param d
* @param du
* @param du2
* @param ipiv
* @param b
* @param ldb
* @param info
*
*/
abstract public void sgttrs(java.lang.String trans, int n, int nrhs, float[] dl, float[] d, float[] du, float[] du2, int[] ipiv, float[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGTTRS solves one of the systems of equations
* A*X = B or A'*X = B,
* with a tridiagonal matrix A using the LU factorization computed
* by SGTTRF.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations.
* = 'N': A * X = B (No transpose)
* = 'T': A'* X = B (Transpose)
* = 'C': A'* X = B (Conjugate transpose = Transpose)
*
* N (input) INTEGER
* The order of the matrix A.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* DL (input) REAL array, dimension (N-1)
* The (n-1) multipliers that define the matrix L from the
* LU factorization of A.
*
* D (input) REAL array, dimension (N)
* The n diagonal elements of the upper triangular matrix U from
* the LU factorization of A.
*
* DU (input) REAL array, dimension (N-1)
* The (n-1) elements of the first super-diagonal of U.
*
* DU2 (input) REAL array, dimension (N-2)
* The (n-2) elements of the second super-diagonal of U.
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices; for 1 <= i <= n, row i of the matrix was
* interchanged with row IPIV(i). IPIV(i) will always be either
* i or i+1; IPIV(i) = i indicates a row interchange was not
* required.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the matrix of right hand side vectors B.
* On exit, B is overwritten by the solution vectors X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param trans
* @param n
* @param nrhs
* @param dl
* @param _dl_offset
* @param d
* @param _d_offset
* @param du
* @param _du_offset
* @param du2
* @param _du2_offset
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void sgttrs(java.lang.String trans, int n, int nrhs, float[] dl, int _dl_offset, float[] d, int _d_offset, float[] du, int _du_offset, float[] du2, int _du2_offset, int[] ipiv, int _ipiv_offset, float[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SGTTS2 solves one of the systems of equations
* A*X = B or A'*X = B,
* with a tridiagonal matrix A using the LU factorization computed
* by SGTTRF.
*
* Arguments
* =========
*
* ITRANS (input) INTEGER
* Specifies the form of the system of equations.
* = 0: A * X = B (No transpose)
* = 1: A'* X = B (Transpose)
* = 2: A'* X = B (Conjugate transpose = Transpose)
*
* N (input) INTEGER
* The order of the matrix A.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* DL (input) REAL array, dimension (N-1)
* The (n-1) multipliers that define the matrix L from the
* LU factorization of A.
*
* D (input) REAL array, dimension (N)
* The n diagonal elements of the upper triangular matrix U from
* the LU factorization of A.
*
* DU (input) REAL array, dimension (N-1)
* The (n-1) elements of the first super-diagonal of U.
*
* DU2 (input) REAL array, dimension (N-2)
* The (n-2) elements of the second super-diagonal of U.
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices; for 1 <= i <= n, row i of the matrix was
* interchanged with row IPIV(i). IPIV(i) will always be either
* i or i+1; IPIV(i) = i indicates a row interchange was not
* required.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the matrix of right hand side vectors B.
* On exit, B is overwritten by the solution vectors X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param itrans
* @param n
* @param nrhs
* @param dl
* @param d
* @param du
* @param du2
* @param ipiv
* @param b
* @param ldb
*
*/
abstract public void sgtts2(int itrans, int n, int nrhs, float[] dl, float[] d, float[] du, float[] du2, int[] ipiv, float[] b, int ldb);
/**
*
* ..
*
* Purpose
* =======
*
* SGTTS2 solves one of the systems of equations
* A*X = B or A'*X = B,
* with a tridiagonal matrix A using the LU factorization computed
* by SGTTRF.
*
* Arguments
* =========
*
* ITRANS (input) INTEGER
* Specifies the form of the system of equations.
* = 0: A * X = B (No transpose)
* = 1: A'* X = B (Transpose)
* = 2: A'* X = B (Conjugate transpose = Transpose)
*
* N (input) INTEGER
* The order of the matrix A.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* DL (input) REAL array, dimension (N-1)
* The (n-1) multipliers that define the matrix L from the
* LU factorization of A.
*
* D (input) REAL array, dimension (N)
* The n diagonal elements of the upper triangular matrix U from
* the LU factorization of A.
*
* DU (input) REAL array, dimension (N-1)
* The (n-1) elements of the first super-diagonal of U.
*
* DU2 (input) REAL array, dimension (N-2)
* The (n-2) elements of the second super-diagonal of U.
*
* IPIV (input) INTEGER array, dimension (N)
* The pivot indices; for 1 <= i <= n, row i of the matrix was
* interchanged with row IPIV(i). IPIV(i) will always be either
* i or i+1; IPIV(i) = i indicates a row interchange was not
* required.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the matrix of right hand side vectors B.
* On exit, B is overwritten by the solution vectors X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param itrans
* @param n
* @param nrhs
* @param dl
* @param _dl_offset
* @param d
* @param _d_offset
* @param du
* @param _du_offset
* @param du2
* @param _du2_offset
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
*
*/
abstract public void sgtts2(int itrans, int n, int nrhs, float[] dl, int _dl_offset, float[] d, int _d_offset, float[] du, int _du_offset, float[] du2, int _du2_offset, int[] ipiv, int _ipiv_offset, float[] b, int _b_offset, int ldb);
/**
*
* ..
*
* Purpose
* =======
*
* SHGEQZ computes the eigenvalues of a real matrix pair (H,T),
* where H is an upper Hessenberg matrix and T is upper triangular,
* using the double-shift QZ method.
* Matrix pairs of this type are produced by the reduction to
* generalized upper Hessenberg form of a real matrix pair (A,B):
*
* A = Q1*H*Z1**T, B = Q1*T*Z1**T,
*
* as computed by SGGHRD.
*
* If JOB='S', then the Hessenberg-triangular pair (H,T) is
* also reduced to generalized Schur form,
*
* H = Q*S*Z**T, T = Q*P*Z**T,
*
* where Q and Z are orthogonal matrices, P is an upper triangular
* matrix, and S is a quasi-triangular matrix with 1-by-1 and 2-by-2
* diagonal blocks.
*
* The 1-by-1 blocks correspond to real eigenvalues of the matrix pair
* (H,T) and the 2-by-2 blocks correspond to complex conjugate pairs of
* eigenvalues.
*
* Additionally, the 2-by-2 upper triangular diagonal blocks of P
* corresponding to 2-by-2 blocks of S are reduced to positive diagonal
* form, i.e., if S(j+1,j) is non-zero, then P(j+1,j) = P(j,j+1) = 0,
* P(j,j) > 0, and P(j+1,j+1) > 0.
*
* Optionally, the orthogonal matrix Q from the generalized Schur
* factorization may be postmultiplied into an input matrix Q1, and the
* orthogonal matrix Z may be postmultiplied into an input matrix Z1.
* If Q1 and Z1 are the orthogonal matrices from SGGHRD that reduced
* the matrix pair (A,B) to generalized upper Hessenberg form, then the
* output matrices Q1*Q and Z1*Z are the orthogonal factors from the
* generalized Schur factorization of (A,B):
*
* A = (Q1*Q)*S*(Z1*Z)**T, B = (Q1*Q)*P*(Z1*Z)**T.
*
* To avoid overflow, eigenvalues of the matrix pair (H,T) (equivalently
* of (A,B)) are computed as a pair of values (alpha,beta), where alpha
* complex and beta real.
* If beta is nonzero, lambda = alpha / beta is an eigenvalue of the
* generalized nonsymmetric eigenvalue problem (GNEP)
* A*x = lambda*B*x
* and if alpha is nonzero, mu = beta / alpha is an eigenvalue of the
* alternate form of the GNEP
* mu*A*y = B*y.
* Real eigenvalues can be read directly from the generalized Schur
* form:
* alpha = S(i,i), beta = P(i,i).
*
* Ref: C.B. Moler & G.W. Stewart, "An Algorithm for Generalized Matrix
* Eigenvalue Problems", SIAM J. Numer. Anal., 10(1973),
* pp. 241--256.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* = 'E': Compute eigenvalues only;
* = 'S': Compute eigenvalues and the Schur form.
*
* COMPQ (input) CHARACTER*1
* = 'N': Left Schur vectors (Q) are not computed;
* = 'I': Q is initialized to the unit matrix and the matrix Q
* of left Schur vectors of (H,T) is returned;
* = 'V': Q must contain an orthogonal matrix Q1 on entry and
* the product Q1*Q is returned.
*
* COMPZ (input) CHARACTER*1
* = 'N': Right Schur vectors (Z) are not computed;
* = 'I': Z is initialized to the unit matrix and the matrix Z
* of right Schur vectors of (H,T) is returned;
* = 'V': Z must contain an orthogonal matrix Z1 on entry and
* the product Z1*Z is returned.
*
* N (input) INTEGER
* The order of the matrices H, T, Q, and Z. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* ILO and IHI mark the rows and columns of H which are in
* Hessenberg form. It is assumed that A is already upper
* triangular in rows and columns 1:ILO-1 and IHI+1:N.
* If N > 0, 1 <= ILO <= IHI <= N; if N = 0, ILO=1 and IHI=0.
*
* H (input/output) REAL array, dimension (LDH, N)
* On entry, the N-by-N upper Hessenberg matrix H.
* On exit, if JOB = 'S', H contains the upper quasi-triangular
* matrix S from the generalized Schur factorization;
* 2-by-2 diagonal blocks (corresponding to complex conjugate
* pairs of eigenvalues) are returned in standard form, with
* H(i,i) = H(i+1,i+1) and H(i+1,i)*H(i,i+1) < 0.
* If JOB = 'E', the diagonal blocks of H match those of S, but
* the rest of H is unspecified.
*
* LDH (input) INTEGER
* The leading dimension of the array H. LDH >= max( 1, N ).
*
* T (input/output) REAL array, dimension (LDT, N)
* On entry, the N-by-N upper triangular matrix T.
* On exit, if JOB = 'S', T contains the upper triangular
* matrix P from the generalized Schur factorization;
* 2-by-2 diagonal blocks of P corresponding to 2-by-2 blocks of
* are reduced to positive diagonal form, i.e., if H(j+1,j) is
* non-zero, then T(j+1,j) = T(j,j+1) = 0, T(j,j) > 0, and
* T(j+1,j+1) > 0.
* If JOB = 'E', the diagonal blocks of T match those of P, but
* the rest of T is unspecified.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= max( 1, N ).
*
* ALPHAR (output) REAL array, dimension (N)
* The real parts of each scalar alpha defining an eigenvalue
* of GNEP.
*
* ALPHAI (output) REAL array, dimension (N)
* The imaginary parts of each scalar alpha defining an
* eigenvalue of GNEP.
* If ALPHAI(j) is zero, then the j-th eigenvalue is real; if
* positive, then the j-th and (j+1)-st eigenvalues are a
* complex conjugate pair, with ALPHAI(j+1) = -ALPHAI(j).
*
* BETA (output) REAL array, dimension (N)
* The scalars beta that define the eigenvalues of GNEP.
* Together, the quantities alpha = (ALPHAR(j),ALPHAI(j)) and
* beta = BETA(j) represent the j-th eigenvalue of the matrix
* pair (A,B), in one of the forms lambda = alpha/beta or
* mu = beta/alpha. Since either lambda or mu may overflow,
* they should not, in general, be computed.
*
* Q (input/output) REAL array, dimension (LDQ, N)
* On entry, if COMPZ = 'V', the orthogonal matrix Q1 used in
* the reduction of (A,B) to generalized Hessenberg form.
* On exit, if COMPZ = 'I', the orthogonal matrix of left Schur
* vectors of (H,T), and if COMPZ = 'V', the orthogonal matrix
* of left Schur vectors of (A,B).
* Not referenced if COMPZ = 'N'.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= 1.
* If COMPQ='V' or 'I', then LDQ >= N.
*
* Z (input/output) REAL array, dimension (LDZ, N)
* On entry, if COMPZ = 'V', the orthogonal matrix Z1 used in
* the reduction of (A,B) to generalized Hessenberg form.
* On exit, if COMPZ = 'I', the orthogonal matrix of
* right Schur vectors of (H,T), and if COMPZ = 'V', the
* orthogonal matrix of right Schur vectors of (A,B).
* Not referenced if COMPZ = 'N'.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1.
* If COMPZ='V' or 'I', then LDZ >= N.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO >= 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* = 1,...,N: the QZ iteration did not converge. (H,T) is not
* in Schur form, but ALPHAR(i), ALPHAI(i), and
* BETA(i), i=INFO+1,...,N should be correct.
* = N+1,...,2*N: the shift calculation failed. (H,T) is not
* in Schur form, but ALPHAR(i), ALPHAI(i), and
* BETA(i), i=INFO-N+1,...,N should be correct.
*
* Further Details
* ===============
*
* Iteration counters:
*
* JITER -- counts iterations.
* IITER -- counts iterations run since ILAST was last
* changed. This is therefore reset only when a 1-by-1 or
* 2-by-2 block deflates off the bottom.
*
* =====================================================================
*
* .. Parameters ..
* $ SAFETY = 1.0E+0 )
*
*
* @param job
* @param compq
* @param compz
* @param n
* @param ilo
* @param ihi
* @param h
* @param ldh
* @param t
* @param ldt
* @param alphar
* @param alphai
* @param beta
* @param q
* @param ldq
* @param z
* @param ldz
* @param work
* @param lwork
* @param info
*
*/
abstract public void shgeqz(java.lang.String job, java.lang.String compq, java.lang.String compz, int n, int ilo, int ihi, float[] h, int ldh, float[] t, int ldt, float[] alphar, float[] alphai, float[] beta, float[] q, int ldq, float[] z, int ldz, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SHGEQZ computes the eigenvalues of a real matrix pair (H,T),
* where H is an upper Hessenberg matrix and T is upper triangular,
* using the double-shift QZ method.
* Matrix pairs of this type are produced by the reduction to
* generalized upper Hessenberg form of a real matrix pair (A,B):
*
* A = Q1*H*Z1**T, B = Q1*T*Z1**T,
*
* as computed by SGGHRD.
*
* If JOB='S', then the Hessenberg-triangular pair (H,T) is
* also reduced to generalized Schur form,
*
* H = Q*S*Z**T, T = Q*P*Z**T,
*
* where Q and Z are orthogonal matrices, P is an upper triangular
* matrix, and S is a quasi-triangular matrix with 1-by-1 and 2-by-2
* diagonal blocks.
*
* The 1-by-1 blocks correspond to real eigenvalues of the matrix pair
* (H,T) and the 2-by-2 blocks correspond to complex conjugate pairs of
* eigenvalues.
*
* Additionally, the 2-by-2 upper triangular diagonal blocks of P
* corresponding to 2-by-2 blocks of S are reduced to positive diagonal
* form, i.e., if S(j+1,j) is non-zero, then P(j+1,j) = P(j,j+1) = 0,
* P(j,j) > 0, and P(j+1,j+1) > 0.
*
* Optionally, the orthogonal matrix Q from the generalized Schur
* factorization may be postmultiplied into an input matrix Q1, and the
* orthogonal matrix Z may be postmultiplied into an input matrix Z1.
* If Q1 and Z1 are the orthogonal matrices from SGGHRD that reduced
* the matrix pair (A,B) to generalized upper Hessenberg form, then the
* output matrices Q1*Q and Z1*Z are the orthogonal factors from the
* generalized Schur factorization of (A,B):
*
* A = (Q1*Q)*S*(Z1*Z)**T, B = (Q1*Q)*P*(Z1*Z)**T.
*
* To avoid overflow, eigenvalues of the matrix pair (H,T) (equivalently
* of (A,B)) are computed as a pair of values (alpha,beta), where alpha
* complex and beta real.
* If beta is nonzero, lambda = alpha / beta is an eigenvalue of the
* generalized nonsymmetric eigenvalue problem (GNEP)
* A*x = lambda*B*x
* and if alpha is nonzero, mu = beta / alpha is an eigenvalue of the
* alternate form of the GNEP
* mu*A*y = B*y.
* Real eigenvalues can be read directly from the generalized Schur
* form:
* alpha = S(i,i), beta = P(i,i).
*
* Ref: C.B. Moler & G.W. Stewart, "An Algorithm for Generalized Matrix
* Eigenvalue Problems", SIAM J. Numer. Anal., 10(1973),
* pp. 241--256.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* = 'E': Compute eigenvalues only;
* = 'S': Compute eigenvalues and the Schur form.
*
* COMPQ (input) CHARACTER*1
* = 'N': Left Schur vectors (Q) are not computed;
* = 'I': Q is initialized to the unit matrix and the matrix Q
* of left Schur vectors of (H,T) is returned;
* = 'V': Q must contain an orthogonal matrix Q1 on entry and
* the product Q1*Q is returned.
*
* COMPZ (input) CHARACTER*1
* = 'N': Right Schur vectors (Z) are not computed;
* = 'I': Z is initialized to the unit matrix and the matrix Z
* of right Schur vectors of (H,T) is returned;
* = 'V': Z must contain an orthogonal matrix Z1 on entry and
* the product Z1*Z is returned.
*
* N (input) INTEGER
* The order of the matrices H, T, Q, and Z. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* ILO and IHI mark the rows and columns of H which are in
* Hessenberg form. It is assumed that A is already upper
* triangular in rows and columns 1:ILO-1 and IHI+1:N.
* If N > 0, 1 <= ILO <= IHI <= N; if N = 0, ILO=1 and IHI=0.
*
* H (input/output) REAL array, dimension (LDH, N)
* On entry, the N-by-N upper Hessenberg matrix H.
* On exit, if JOB = 'S', H contains the upper quasi-triangular
* matrix S from the generalized Schur factorization;
* 2-by-2 diagonal blocks (corresponding to complex conjugate
* pairs of eigenvalues) are returned in standard form, with
* H(i,i) = H(i+1,i+1) and H(i+1,i)*H(i,i+1) < 0.
* If JOB = 'E', the diagonal blocks of H match those of S, but
* the rest of H is unspecified.
*
* LDH (input) INTEGER
* The leading dimension of the array H. LDH >= max( 1, N ).
*
* T (input/output) REAL array, dimension (LDT, N)
* On entry, the N-by-N upper triangular matrix T.
* On exit, if JOB = 'S', T contains the upper triangular
* matrix P from the generalized Schur factorization;
* 2-by-2 diagonal blocks of P corresponding to 2-by-2 blocks of
* are reduced to positive diagonal form, i.e., if H(j+1,j) is
* non-zero, then T(j+1,j) = T(j,j+1) = 0, T(j,j) > 0, and
* T(j+1,j+1) > 0.
* If JOB = 'E', the diagonal blocks of T match those of P, but
* the rest of T is unspecified.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= max( 1, N ).
*
* ALPHAR (output) REAL array, dimension (N)
* The real parts of each scalar alpha defining an eigenvalue
* of GNEP.
*
* ALPHAI (output) REAL array, dimension (N)
* The imaginary parts of each scalar alpha defining an
* eigenvalue of GNEP.
* If ALPHAI(j) is zero, then the j-th eigenvalue is real; if
* positive, then the j-th and (j+1)-st eigenvalues are a
* complex conjugate pair, with ALPHAI(j+1) = -ALPHAI(j).
*
* BETA (output) REAL array, dimension (N)
* The scalars beta that define the eigenvalues of GNEP.
* Together, the quantities alpha = (ALPHAR(j),ALPHAI(j)) and
* beta = BETA(j) represent the j-th eigenvalue of the matrix
* pair (A,B), in one of the forms lambda = alpha/beta or
* mu = beta/alpha. Since either lambda or mu may overflow,
* they should not, in general, be computed.
*
* Q (input/output) REAL array, dimension (LDQ, N)
* On entry, if COMPZ = 'V', the orthogonal matrix Q1 used in
* the reduction of (A,B) to generalized Hessenberg form.
* On exit, if COMPZ = 'I', the orthogonal matrix of left Schur
* vectors of (H,T), and if COMPZ = 'V', the orthogonal matrix
* of left Schur vectors of (A,B).
* Not referenced if COMPZ = 'N'.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= 1.
* If COMPQ='V' or 'I', then LDQ >= N.
*
* Z (input/output) REAL array, dimension (LDZ, N)
* On entry, if COMPZ = 'V', the orthogonal matrix Z1 used in
* the reduction of (A,B) to generalized Hessenberg form.
* On exit, if COMPZ = 'I', the orthogonal matrix of
* right Schur vectors of (H,T), and if COMPZ = 'V', the
* orthogonal matrix of right Schur vectors of (A,B).
* Not referenced if COMPZ = 'N'.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1.
* If COMPZ='V' or 'I', then LDZ >= N.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO >= 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* = 1,...,N: the QZ iteration did not converge. (H,T) is not
* in Schur form, but ALPHAR(i), ALPHAI(i), and
* BETA(i), i=INFO+1,...,N should be correct.
* = N+1,...,2*N: the shift calculation failed. (H,T) is not
* in Schur form, but ALPHAR(i), ALPHAI(i), and
* BETA(i), i=INFO-N+1,...,N should be correct.
*
* Further Details
* ===============
*
* Iteration counters:
*
* JITER -- counts iterations.
* IITER -- counts iterations run since ILAST was last
* changed. This is therefore reset only when a 1-by-1 or
* 2-by-2 block deflates off the bottom.
*
* =====================================================================
*
* .. Parameters ..
* $ SAFETY = 1.0E+0 )
*
*
* @param job
* @param compq
* @param compz
* @param n
* @param ilo
* @param ihi
* @param h
* @param _h_offset
* @param ldh
* @param t
* @param _t_offset
* @param ldt
* @param alphar
* @param _alphar_offset
* @param alphai
* @param _alphai_offset
* @param beta
* @param _beta_offset
* @param q
* @param _q_offset
* @param ldq
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void shgeqz(java.lang.String job, java.lang.String compq, java.lang.String compz, int n, int ilo, int ihi, float[] h, int _h_offset, int ldh, float[] t, int _t_offset, int ldt, float[] alphar, int _alphar_offset, float[] alphai, int _alphai_offset, float[] beta, int _beta_offset, float[] q, int _q_offset, int ldq, float[] z, int _z_offset, int ldz, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SHSEIN uses inverse iteration to find specified right and/or left
* eigenvectors of a real upper Hessenberg matrix H.
*
* The right eigenvector x and the left eigenvector y of the matrix H
* corresponding to an eigenvalue w are defined by:
*
* H * x = w * x, y**h * H = w * y**h
*
* where y**h denotes the conjugate transpose of the vector y.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'R': compute right eigenvectors only;
* = 'L': compute left eigenvectors only;
* = 'B': compute both right and left eigenvectors.
*
* EIGSRC (input) CHARACTER*1
* Specifies the source of eigenvalues supplied in (WR,WI):
* = 'Q': the eigenvalues were found using SHSEQR; thus, if
* H has zero subdiagonal elements, and so is
* block-triangular, then the j-th eigenvalue can be
* assumed to be an eigenvalue of the block containing
* the j-th row/column. This property allows SHSEIN to
* perform inverse iteration on just one diagonal block.
* = 'N': no assumptions are made on the correspondence
* between eigenvalues and diagonal blocks. In this
* case, SHSEIN must always perform inverse iteration
* using the whole matrix H.
*
* INITV (input) CHARACTER*1
* = 'N': no initial vectors are supplied;
* = 'U': user-supplied initial vectors are stored in the arrays
* VL and/or VR.
*
* SELECT (input/output) LOGICAL array, dimension (N)
* Specifies the eigenvectors to be computed. To select the
* real eigenvector corresponding to a real eigenvalue WR(j),
* SELECT(j) must be set to .TRUE.. To select the complex
* eigenvector corresponding to a complex eigenvalue
* (WR(j),WI(j)), with complex conjugate (WR(j+1),WI(j+1)),
* either SELECT(j) or SELECT(j+1) or both must be set to
* .TRUE.; then on exit SELECT(j) is .TRUE. and SELECT(j+1) is
* .FALSE..
*
* N (input) INTEGER
* The order of the matrix H. N >= 0.
*
* H (input) REAL array, dimension (LDH,N)
* The upper Hessenberg matrix H.
*
* LDH (input) INTEGER
* The leading dimension of the array H. LDH >= max(1,N).
*
* WR (input/output) REAL array, dimension (N)
* WI (input) REAL array, dimension (N)
* On entry, the real and imaginary parts of the eigenvalues of
* H; a complex conjugate pair of eigenvalues must be stored in
* consecutive elements of WR and WI.
* On exit, WR may have been altered since close eigenvalues
* are perturbed slightly in searching for independent
* eigenvectors.
*
* VL (input/output) REAL array, dimension (LDVL,MM)
* On entry, if INITV = 'U' and SIDE = 'L' or 'B', VL must
* contain starting vectors for the inverse iteration for the
* left eigenvectors; the starting vector for each eigenvector
* must be in the same column(s) in which the eigenvector will
* be stored.
* On exit, if SIDE = 'L' or 'B', the left eigenvectors
* specified by SELECT will be stored consecutively in the
* columns of VL, in the same order as their eigenvalues. A
* complex eigenvector corresponding to a complex eigenvalue is
* stored in two consecutive columns, the first holding the real
* part and the second the imaginary part.
* If SIDE = 'R', VL is not referenced.
*
* LDVL (input) INTEGER
* The leading dimension of the array VL.
* LDVL >= max(1,N) if SIDE = 'L' or 'B'; LDVL >= 1 otherwise.
*
* VR (input/output) REAL array, dimension (LDVR,MM)
* On entry, if INITV = 'U' and SIDE = 'R' or 'B', VR must
* contain starting vectors for the inverse iteration for the
* right eigenvectors; the starting vector for each eigenvector
* must be in the same column(s) in which the eigenvector will
* be stored.
* On exit, if SIDE = 'R' or 'B', the right eigenvectors
* specified by SELECT will be stored consecutively in the
* columns of VR, in the same order as their eigenvalues. A
* complex eigenvector corresponding to a complex eigenvalue is
* stored in two consecutive columns, the first holding the real
* part and the second the imaginary part.
* If SIDE = 'L', VR is not referenced.
*
* LDVR (input) INTEGER
* The leading dimension of the array VR.
* LDVR >= max(1,N) if SIDE = 'R' or 'B'; LDVR >= 1 otherwise.
*
* MM (input) INTEGER
* The number of columns in the arrays VL and/or VR. MM >= M.
*
* M (output) INTEGER
* The number of columns in the arrays VL and/or VR required to
* store the eigenvectors; each selected real eigenvector
* occupies one column and each selected complex eigenvector
* occupies two columns.
*
* WORK (workspace) REAL array, dimension ((N+2)*N)
*
* IFAILL (output) INTEGER array, dimension (MM)
* If SIDE = 'L' or 'B', IFAILL(i) = j > 0 if the left
* eigenvector in the i-th column of VL (corresponding to the
* eigenvalue w(j)) failed to converge; IFAILL(i) = 0 if the
* eigenvector converged satisfactorily. If the i-th and (i+1)th
* columns of VL hold a complex eigenvector, then IFAILL(i) and
* IFAILL(i+1) are set to the same value.
* If SIDE = 'R', IFAILL is not referenced.
*
* IFAILR (output) INTEGER array, dimension (MM)
* If SIDE = 'R' or 'B', IFAILR(i) = j > 0 if the right
* eigenvector in the i-th column of VR (corresponding to the
* eigenvalue w(j)) failed to converge; IFAILR(i) = 0 if the
* eigenvector converged satisfactorily. If the i-th and (i+1)th
* columns of VR hold a complex eigenvector, then IFAILR(i) and
* IFAILR(i+1) are set to the same value.
* If SIDE = 'L', IFAILR is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, i is the number of eigenvectors which
* failed to converge; see IFAILL and IFAILR for further
* details.
*
* Further Details
* ===============
*
* Each eigenvector is normalized so that the element of largest
* magnitude has magnitude 1; here the magnitude of a complex number
* (x,y) is taken to be |x|+|y|.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param eigsrc
* @param initv
* @param select
* @param n
* @param h
* @param ldh
* @param wr
* @param wi
* @param vl
* @param ldvl
* @param vr
* @param ldvr
* @param mm
* @param m
* @param work
* @param ifaill
* @param ifailr
* @param info
*
*/
abstract public void shsein(java.lang.String side, java.lang.String eigsrc, java.lang.String initv, boolean[] select, int n, float[] h, int ldh, float[] wr, float[] wi, float[] vl, int ldvl, float[] vr, int ldvr, int mm, org.netlib.util.intW m, float[] work, int[] ifaill, int[] ifailr, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SHSEIN uses inverse iteration to find specified right and/or left
* eigenvectors of a real upper Hessenberg matrix H.
*
* The right eigenvector x and the left eigenvector y of the matrix H
* corresponding to an eigenvalue w are defined by:
*
* H * x = w * x, y**h * H = w * y**h
*
* where y**h denotes the conjugate transpose of the vector y.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'R': compute right eigenvectors only;
* = 'L': compute left eigenvectors only;
* = 'B': compute both right and left eigenvectors.
*
* EIGSRC (input) CHARACTER*1
* Specifies the source of eigenvalues supplied in (WR,WI):
* = 'Q': the eigenvalues were found using SHSEQR; thus, if
* H has zero subdiagonal elements, and so is
* block-triangular, then the j-th eigenvalue can be
* assumed to be an eigenvalue of the block containing
* the j-th row/column. This property allows SHSEIN to
* perform inverse iteration on just one diagonal block.
* = 'N': no assumptions are made on the correspondence
* between eigenvalues and diagonal blocks. In this
* case, SHSEIN must always perform inverse iteration
* using the whole matrix H.
*
* INITV (input) CHARACTER*1
* = 'N': no initial vectors are supplied;
* = 'U': user-supplied initial vectors are stored in the arrays
* VL and/or VR.
*
* SELECT (input/output) LOGICAL array, dimension (N)
* Specifies the eigenvectors to be computed. To select the
* real eigenvector corresponding to a real eigenvalue WR(j),
* SELECT(j) must be set to .TRUE.. To select the complex
* eigenvector corresponding to a complex eigenvalue
* (WR(j),WI(j)), with complex conjugate (WR(j+1),WI(j+1)),
* either SELECT(j) or SELECT(j+1) or both must be set to
* .TRUE.; then on exit SELECT(j) is .TRUE. and SELECT(j+1) is
* .FALSE..
*
* N (input) INTEGER
* The order of the matrix H. N >= 0.
*
* H (input) REAL array, dimension (LDH,N)
* The upper Hessenberg matrix H.
*
* LDH (input) INTEGER
* The leading dimension of the array H. LDH >= max(1,N).
*
* WR (input/output) REAL array, dimension (N)
* WI (input) REAL array, dimension (N)
* On entry, the real and imaginary parts of the eigenvalues of
* H; a complex conjugate pair of eigenvalues must be stored in
* consecutive elements of WR and WI.
* On exit, WR may have been altered since close eigenvalues
* are perturbed slightly in searching for independent
* eigenvectors.
*
* VL (input/output) REAL array, dimension (LDVL,MM)
* On entry, if INITV = 'U' and SIDE = 'L' or 'B', VL must
* contain starting vectors for the inverse iteration for the
* left eigenvectors; the starting vector for each eigenvector
* must be in the same column(s) in which the eigenvector will
* be stored.
* On exit, if SIDE = 'L' or 'B', the left eigenvectors
* specified by SELECT will be stored consecutively in the
* columns of VL, in the same order as their eigenvalues. A
* complex eigenvector corresponding to a complex eigenvalue is
* stored in two consecutive columns, the first holding the real
* part and the second the imaginary part.
* If SIDE = 'R', VL is not referenced.
*
* LDVL (input) INTEGER
* The leading dimension of the array VL.
* LDVL >= max(1,N) if SIDE = 'L' or 'B'; LDVL >= 1 otherwise.
*
* VR (input/output) REAL array, dimension (LDVR,MM)
* On entry, if INITV = 'U' and SIDE = 'R' or 'B', VR must
* contain starting vectors for the inverse iteration for the
* right eigenvectors; the starting vector for each eigenvector
* must be in the same column(s) in which the eigenvector will
* be stored.
* On exit, if SIDE = 'R' or 'B', the right eigenvectors
* specified by SELECT will be stored consecutively in the
* columns of VR, in the same order as their eigenvalues. A
* complex eigenvector corresponding to a complex eigenvalue is
* stored in two consecutive columns, the first holding the real
* part and the second the imaginary part.
* If SIDE = 'L', VR is not referenced.
*
* LDVR (input) INTEGER
* The leading dimension of the array VR.
* LDVR >= max(1,N) if SIDE = 'R' or 'B'; LDVR >= 1 otherwise.
*
* MM (input) INTEGER
* The number of columns in the arrays VL and/or VR. MM >= M.
*
* M (output) INTEGER
* The number of columns in the arrays VL and/or VR required to
* store the eigenvectors; each selected real eigenvector
* occupies one column and each selected complex eigenvector
* occupies two columns.
*
* WORK (workspace) REAL array, dimension ((N+2)*N)
*
* IFAILL (output) INTEGER array, dimension (MM)
* If SIDE = 'L' or 'B', IFAILL(i) = j > 0 if the left
* eigenvector in the i-th column of VL (corresponding to the
* eigenvalue w(j)) failed to converge; IFAILL(i) = 0 if the
* eigenvector converged satisfactorily. If the i-th and (i+1)th
* columns of VL hold a complex eigenvector, then IFAILL(i) and
* IFAILL(i+1) are set to the same value.
* If SIDE = 'R', IFAILL is not referenced.
*
* IFAILR (output) INTEGER array, dimension (MM)
* If SIDE = 'R' or 'B', IFAILR(i) = j > 0 if the right
* eigenvector in the i-th column of VR (corresponding to the
* eigenvalue w(j)) failed to converge; IFAILR(i) = 0 if the
* eigenvector converged satisfactorily. If the i-th and (i+1)th
* columns of VR hold a complex eigenvector, then IFAILR(i) and
* IFAILR(i+1) are set to the same value.
* If SIDE = 'L', IFAILR is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, i is the number of eigenvectors which
* failed to converge; see IFAILL and IFAILR for further
* details.
*
* Further Details
* ===============
*
* Each eigenvector is normalized so that the element of largest
* magnitude has magnitude 1; here the magnitude of a complex number
* (x,y) is taken to be |x|+|y|.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param eigsrc
* @param initv
* @param select
* @param _select_offset
* @param n
* @param h
* @param _h_offset
* @param ldh
* @param wr
* @param _wr_offset
* @param wi
* @param _wi_offset
* @param vl
* @param _vl_offset
* @param ldvl
* @param vr
* @param _vr_offset
* @param ldvr
* @param mm
* @param m
* @param work
* @param _work_offset
* @param ifaill
* @param _ifaill_offset
* @param ifailr
* @param _ifailr_offset
* @param info
*
*/
abstract public void shsein(java.lang.String side, java.lang.String eigsrc, java.lang.String initv, boolean[] select, int _select_offset, int n, float[] h, int _h_offset, int ldh, float[] wr, int _wr_offset, float[] wi, int _wi_offset, float[] vl, int _vl_offset, int ldvl, float[] vr, int _vr_offset, int ldvr, int mm, org.netlib.util.intW m, float[] work, int _work_offset, int[] ifaill, int _ifaill_offset, int[] ifailr, int _ifailr_offset, org.netlib.util.intW info);
/**
*
* ..
* Purpose
* =======
*
* SHSEQR computes the eigenvalues of a Hessenberg matrix H
* and, optionally, the matrices T and Z from the Schur decomposition
* H = Z T Z**T, where T is an upper quasi-triangular matrix (the
* Schur form), and Z is the orthogonal matrix of Schur vectors.
*
* Optionally Z may be postmultiplied into an input orthogonal
* matrix Q so that this routine can give the Schur factorization
* of a matrix A which has been reduced to the Hessenberg form H
* by the orthogonal matrix Q: A = Q*H*Q**T = (QZ)*T*(QZ)**T.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* = 'E': compute eigenvalues only;
* = 'S': compute eigenvalues and the Schur form T.
*
* COMPZ (input) CHARACTER*1
* = 'N': no Schur vectors are computed;
* = 'I': Z is initialized to the unit matrix and the matrix Z
* of Schur vectors of H is returned;
* = 'V': Z must contain an orthogonal matrix Q on entry, and
* the product Q*Z is returned.
*
* N (input) INTEGER
* The order of the matrix H. N .GE. 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* It is assumed that H is already upper triangular in rows
* and columns 1:ILO-1 and IHI+1:N. ILO and IHI are normally
* set by a previous call to SGEBAL, and then passed to SGEHRD
* when the matrix output by SGEBAL is reduced to Hessenberg
* form. Otherwise ILO and IHI should be set to 1 and N
* respectively. If N.GT.0, then 1.LE.ILO.LE.IHI.LE.N.
* If N = 0, then ILO = 1 and IHI = 0.
*
* H (input/output) REAL array, dimension (LDH,N)
* On entry, the upper Hessenberg matrix H.
* On exit, if INFO = 0 and JOB = 'S', then H contains the
* upper quasi-triangular matrix T from the Schur decomposition
* (the Schur form); 2-by-2 diagonal blocks (corresponding to
* complex conjugate pairs of eigenvalues) are returned in
* standard form, with H(i,i) = H(i+1,i+1) and
* H(i+1,i)*H(i,i+1).LT.0. If INFO = 0 and JOB = 'E', the
* contents of H are unspecified on exit. (The output value of
* H when INFO.GT.0 is given under the description of INFO
* below.)
*
* Unlike earlier versions of SHSEQR, this subroutine may
* explicitly H(i,j) = 0 for i.GT.j and j = 1, 2, ... ILO-1
* or j = IHI+1, IHI+2, ... N.
*
* LDH (input) INTEGER
* The leading dimension of the array H. LDH .GE. max(1,N).
*
* WR (output) REAL array, dimension (N)
* WI (output) REAL array, dimension (N)
* The real and imaginary parts, respectively, of the computed
* eigenvalues. If two eigenvalues are computed as a complex
* conjugate pair, they are stored in consecutive elements of
* WR and WI, say the i-th and (i+1)th, with WI(i) .GT. 0 and
* WI(i+1) .LT. 0. If JOB = 'S', the eigenvalues are stored in
* the same order as on the diagonal of the Schur form returned
* in H, with WR(i) = H(i,i) and, if H(i:i+1,i:i+1) is a 2-by-2
* diagonal block, WI(i) = sqrt(-H(i+1,i)*H(i,i+1)) and
* WI(i+1) = -WI(i).
*
* Z (input/output) REAL array, dimension (LDZ,N)
* If COMPZ = 'N', Z is not referenced.
* If COMPZ = 'I', on entry Z need not be set and on exit,
* if INFO = 0, Z contains the orthogonal matrix Z of the Schur
* vectors of H. If COMPZ = 'V', on entry Z must contain an
* N-by-N matrix Q, which is assumed to be equal to the unit
* matrix except for the submatrix Z(ILO:IHI,ILO:IHI). On exit,
* if INFO = 0, Z contains Q*Z.
* Normally Q is the orthogonal matrix generated by SORGHR
* after the call to SGEHRD which formed the Hessenberg matrix
* H. (The output value of Z when INFO.GT.0 is given under
* the description of INFO below.)
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. if COMPZ = 'I' or
* COMPZ = 'V', then LDZ.GE.MAX(1,N). Otherwize, LDZ.GE.1.
*
* WORK (workspace/output) REAL array, dimension (LWORK)
* On exit, if INFO = 0, WORK(1) returns an estimate of
* the optimal value for LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK .GE. max(1,N)
* is sufficient, but LWORK typically as large as 6*N may
* be required for optimal performance. A workspace query
* to determine the optimal workspace size is recommended.
*
* If LWORK = -1, then SHSEQR does a workspace query.
* In this case, SHSEQR checks the input parameters and
* estimates the optimal workspace size for the given
* values of N, ILO and IHI. The estimate is returned
* in WORK(1). No error message related to LWORK is
* issued by XERBLA. Neither H nor Z are accessed.
*
*
* INFO (output) INTEGER
* = 0: successful exit
* .LT. 0: if INFO = -i, the i-th argument had an illegal
* value
* .GT. 0: if INFO = i, SHSEQR failed to compute all of
* the eigenvalues. Elements 1:ilo-1 and i+1:n of WR
* and WI contain those eigenvalues which have been
* successfully computed. (Failures are rare.)
*
* If INFO .GT. 0 and JOB = 'E', then on exit, the
* remaining unconverged eigenvalues are the eigen-
* values of the upper Hessenberg matrix rows and
* columns ILO through INFO of the final, output
* value of H.
*
* If INFO .GT. 0 and JOB = 'S', then on exit
*
* (*) (initial value of H)*U = U*(final value of H)
*
* where U is an orthogonal matrix. The final
* value of H is upper Hessenberg and quasi-triangular
* in rows and columns INFO+1 through IHI.
*
* If INFO .GT. 0 and COMPZ = 'V', then on exit
*
* (final value of Z) = (initial value of Z)*U
*
* where U is the orthogonal matrix in (*) (regard-
* less of the value of JOB.)
*
* If INFO .GT. 0 and COMPZ = 'I', then on exit
* (final value of Z) = U
* where U is the orthogonal matrix in (*) (regard-
* less of the value of JOB.)
*
* If INFO .GT. 0 and COMPZ = 'N', then Z is not
* accessed.
*
* ================================================================
* Default values supplied by
* ILAENV(ISPEC,'SHSEQR',JOB(:1)//COMPZ(:1),N,ILO,IHI,LWORK).
* It is suggested that these defaults be adjusted in order
* to attain best performance in each particular
* computational environment.
*
* ISPEC=1: The SLAHQR vs SLAQR0 crossover point.
* Default: 75. (Must be at least 11.)
*
* ISPEC=2: Recommended deflation window size.
* This depends on ILO, IHI and NS. NS is the
* number of simultaneous shifts returned
* by ILAENV(ISPEC=4). (See ISPEC=4 below.)
* The default for (IHI-ILO+1).LE.500 is NS.
* The default for (IHI-ILO+1).GT.500 is 3*NS/2.
*
* ISPEC=3: Nibble crossover point. (See ILAENV for
* details.) Default: 14% of deflation window
* size.
*
* ISPEC=4: Number of simultaneous shifts, NS, in
* a multi-shift QR iteration.
*
* If IHI-ILO+1 is ...
*
* greater than ...but less ... the
* or equal to ... than default is
*
* 1 30 NS - 2(+)
* 30 60 NS - 4(+)
* 60 150 NS = 10(+)
* 150 590 NS = **
* 590 3000 NS = 64
* 3000 6000 NS = 128
* 6000 infinity NS = 256
*
* (+) By default some or all matrices of this order
* are passed to the implicit double shift routine
* SLAHQR and NS is ignored. See ISPEC=1 above
* and comments in IPARM for details.
*
* The asterisks (**) indicate an ad-hoc
* function of N increasing from 10 to 64.
*
* ISPEC=5: Select structured matrix multiply.
* (See ILAENV for details.) Default: 3.
*
* ================================================================
* Based on contributions by
* Karen Braman and Ralph Byers, Department of Mathematics,
* University of Kansas, USA
*
* ================================================================
* References:
* K. Braman, R. Byers and R. Mathias, The Multi-Shift QR
* Algorithm Part I: Maintaining Well Focused Shifts, and Level 3
* Performance, SIAM Journal of Matrix Analysis, volume 23, pages
* 929--947, 2002.
*
* K. Braman, R. Byers and R. Mathias, The Multi-Shift QR
* Algorithm Part II: Aggressive Early Deflation, SIAM Journal
* of Matrix Analysis, volume 23, pages 948--973, 2002.
*
* ================================================================
* .. Parameters ..
*
* ==== Matrices of order NTINY or smaller must be processed by
* . SLAHQR because of insufficient subdiagonal scratch space.
* . (This is a hard limit.) ====
*
* ==== NL allocates some local workspace to help small matrices
* . through a rare SLAHQR failure. NL .GT. NTINY = 11 is
* . required and NL .LE. NMIN = ILAENV(ISPEC=1,...) is recom-
* . mended. (The default value of NMIN is 75.) Using NL = 49
* . allows up to six simultaneous shifts and a 16-by-16
* . deflation window. ====
*
*
*
* @param job
* @param compz
* @param n
* @param ilo
* @param ihi
* @param h
* @param ldh
* @param wr
* @param wi
* @param z
* @param ldz
* @param work
* @param lwork
* @param info
*
*/
abstract public void shseqr(java.lang.String job, java.lang.String compz, int n, int ilo, int ihi, float[] h, int ldh, float[] wr, float[] wi, float[] z, int ldz, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
* Purpose
* =======
*
* SHSEQR computes the eigenvalues of a Hessenberg matrix H
* and, optionally, the matrices T and Z from the Schur decomposition
* H = Z T Z**T, where T is an upper quasi-triangular matrix (the
* Schur form), and Z is the orthogonal matrix of Schur vectors.
*
* Optionally Z may be postmultiplied into an input orthogonal
* matrix Q so that this routine can give the Schur factorization
* of a matrix A which has been reduced to the Hessenberg form H
* by the orthogonal matrix Q: A = Q*H*Q**T = (QZ)*T*(QZ)**T.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* = 'E': compute eigenvalues only;
* = 'S': compute eigenvalues and the Schur form T.
*
* COMPZ (input) CHARACTER*1
* = 'N': no Schur vectors are computed;
* = 'I': Z is initialized to the unit matrix and the matrix Z
* of Schur vectors of H is returned;
* = 'V': Z must contain an orthogonal matrix Q on entry, and
* the product Q*Z is returned.
*
* N (input) INTEGER
* The order of the matrix H. N .GE. 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* It is assumed that H is already upper triangular in rows
* and columns 1:ILO-1 and IHI+1:N. ILO and IHI are normally
* set by a previous call to SGEBAL, and then passed to SGEHRD
* when the matrix output by SGEBAL is reduced to Hessenberg
* form. Otherwise ILO and IHI should be set to 1 and N
* respectively. If N.GT.0, then 1.LE.ILO.LE.IHI.LE.N.
* If N = 0, then ILO = 1 and IHI = 0.
*
* H (input/output) REAL array, dimension (LDH,N)
* On entry, the upper Hessenberg matrix H.
* On exit, if INFO = 0 and JOB = 'S', then H contains the
* upper quasi-triangular matrix T from the Schur decomposition
* (the Schur form); 2-by-2 diagonal blocks (corresponding to
* complex conjugate pairs of eigenvalues) are returned in
* standard form, with H(i,i) = H(i+1,i+1) and
* H(i+1,i)*H(i,i+1).LT.0. If INFO = 0 and JOB = 'E', the
* contents of H are unspecified on exit. (The output value of
* H when INFO.GT.0 is given under the description of INFO
* below.)
*
* Unlike earlier versions of SHSEQR, this subroutine may
* explicitly H(i,j) = 0 for i.GT.j and j = 1, 2, ... ILO-1
* or j = IHI+1, IHI+2, ... N.
*
* LDH (input) INTEGER
* The leading dimension of the array H. LDH .GE. max(1,N).
*
* WR (output) REAL array, dimension (N)
* WI (output) REAL array, dimension (N)
* The real and imaginary parts, respectively, of the computed
* eigenvalues. If two eigenvalues are computed as a complex
* conjugate pair, they are stored in consecutive elements of
* WR and WI, say the i-th and (i+1)th, with WI(i) .GT. 0 and
* WI(i+1) .LT. 0. If JOB = 'S', the eigenvalues are stored in
* the same order as on the diagonal of the Schur form returned
* in H, with WR(i) = H(i,i) and, if H(i:i+1,i:i+1) is a 2-by-2
* diagonal block, WI(i) = sqrt(-H(i+1,i)*H(i,i+1)) and
* WI(i+1) = -WI(i).
*
* Z (input/output) REAL array, dimension (LDZ,N)
* If COMPZ = 'N', Z is not referenced.
* If COMPZ = 'I', on entry Z need not be set and on exit,
* if INFO = 0, Z contains the orthogonal matrix Z of the Schur
* vectors of H. If COMPZ = 'V', on entry Z must contain an
* N-by-N matrix Q, which is assumed to be equal to the unit
* matrix except for the submatrix Z(ILO:IHI,ILO:IHI). On exit,
* if INFO = 0, Z contains Q*Z.
* Normally Q is the orthogonal matrix generated by SORGHR
* after the call to SGEHRD which formed the Hessenberg matrix
* H. (The output value of Z when INFO.GT.0 is given under
* the description of INFO below.)
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. if COMPZ = 'I' or
* COMPZ = 'V', then LDZ.GE.MAX(1,N). Otherwize, LDZ.GE.1.
*
* WORK (workspace/output) REAL array, dimension (LWORK)
* On exit, if INFO = 0, WORK(1) returns an estimate of
* the optimal value for LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK .GE. max(1,N)
* is sufficient, but LWORK typically as large as 6*N may
* be required for optimal performance. A workspace query
* to determine the optimal workspace size is recommended.
*
* If LWORK = -1, then SHSEQR does a workspace query.
* In this case, SHSEQR checks the input parameters and
* estimates the optimal workspace size for the given
* values of N, ILO and IHI. The estimate is returned
* in WORK(1). No error message related to LWORK is
* issued by XERBLA. Neither H nor Z are accessed.
*
*
* INFO (output) INTEGER
* = 0: successful exit
* .LT. 0: if INFO = -i, the i-th argument had an illegal
* value
* .GT. 0: if INFO = i, SHSEQR failed to compute all of
* the eigenvalues. Elements 1:ilo-1 and i+1:n of WR
* and WI contain those eigenvalues which have been
* successfully computed. (Failures are rare.)
*
* If INFO .GT. 0 and JOB = 'E', then on exit, the
* remaining unconverged eigenvalues are the eigen-
* values of the upper Hessenberg matrix rows and
* columns ILO through INFO of the final, output
* value of H.
*
* If INFO .GT. 0 and JOB = 'S', then on exit
*
* (*) (initial value of H)*U = U*(final value of H)
*
* where U is an orthogonal matrix. The final
* value of H is upper Hessenberg and quasi-triangular
* in rows and columns INFO+1 through IHI.
*
* If INFO .GT. 0 and COMPZ = 'V', then on exit
*
* (final value of Z) = (initial value of Z)*U
*
* where U is the orthogonal matrix in (*) (regard-
* less of the value of JOB.)
*
* If INFO .GT. 0 and COMPZ = 'I', then on exit
* (final value of Z) = U
* where U is the orthogonal matrix in (*) (regard-
* less of the value of JOB.)
*
* If INFO .GT. 0 and COMPZ = 'N', then Z is not
* accessed.
*
* ================================================================
* Default values supplied by
* ILAENV(ISPEC,'SHSEQR',JOB(:1)//COMPZ(:1),N,ILO,IHI,LWORK).
* It is suggested that these defaults be adjusted in order
* to attain best performance in each particular
* computational environment.
*
* ISPEC=1: The SLAHQR vs SLAQR0 crossover point.
* Default: 75. (Must be at least 11.)
*
* ISPEC=2: Recommended deflation window size.
* This depends on ILO, IHI and NS. NS is the
* number of simultaneous shifts returned
* by ILAENV(ISPEC=4). (See ISPEC=4 below.)
* The default for (IHI-ILO+1).LE.500 is NS.
* The default for (IHI-ILO+1).GT.500 is 3*NS/2.
*
* ISPEC=3: Nibble crossover point. (See ILAENV for
* details.) Default: 14% of deflation window
* size.
*
* ISPEC=4: Number of simultaneous shifts, NS, in
* a multi-shift QR iteration.
*
* If IHI-ILO+1 is ...
*
* greater than ...but less ... the
* or equal to ... than default is
*
* 1 30 NS - 2(+)
* 30 60 NS - 4(+)
* 60 150 NS = 10(+)
* 150 590 NS = **
* 590 3000 NS = 64
* 3000 6000 NS = 128
* 6000 infinity NS = 256
*
* (+) By default some or all matrices of this order
* are passed to the implicit double shift routine
* SLAHQR and NS is ignored. See ISPEC=1 above
* and comments in IPARM for details.
*
* The asterisks (**) indicate an ad-hoc
* function of N increasing from 10 to 64.
*
* ISPEC=5: Select structured matrix multiply.
* (See ILAENV for details.) Default: 3.
*
* ================================================================
* Based on contributions by
* Karen Braman and Ralph Byers, Department of Mathematics,
* University of Kansas, USA
*
* ================================================================
* References:
* K. Braman, R. Byers and R. Mathias, The Multi-Shift QR
* Algorithm Part I: Maintaining Well Focused Shifts, and Level 3
* Performance, SIAM Journal of Matrix Analysis, volume 23, pages
* 929--947, 2002.
*
* K. Braman, R. Byers and R. Mathias, The Multi-Shift QR
* Algorithm Part II: Aggressive Early Deflation, SIAM Journal
* of Matrix Analysis, volume 23, pages 948--973, 2002.
*
* ================================================================
* .. Parameters ..
*
* ==== Matrices of order NTINY or smaller must be processed by
* . SLAHQR because of insufficient subdiagonal scratch space.
* . (This is a hard limit.) ====
*
* ==== NL allocates some local workspace to help small matrices
* . through a rare SLAHQR failure. NL .GT. NTINY = 11 is
* . required and NL .LE. NMIN = ILAENV(ISPEC=1,...) is recom-
* . mended. (The default value of NMIN is 75.) Using NL = 49
* . allows up to six simultaneous shifts and a 16-by-16
* . deflation window. ====
*
*
*
* @param job
* @param compz
* @param n
* @param ilo
* @param ihi
* @param h
* @param _h_offset
* @param ldh
* @param wr
* @param _wr_offset
* @param wi
* @param _wi_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void shseqr(java.lang.String job, java.lang.String compz, int n, int ilo, int ihi, float[] h, int _h_offset, int ldh, float[] wr, int _wr_offset, float[] wi, int _wi_offset, float[] z, int _z_offset, int ldz, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SISNAN returns .TRUE. if its argument is NaN, and .FALSE.
* otherwise. To be replaced by the Fortran 2003 intrinsic in the
* future.
*
* Arguments
* =========
*
* SIN (input) REAL
* Input to test for NaN.
*
* =====================================================================
*
* .. External Functions ..
*
*
* @param sin
* @return
*/
abstract public boolean sisnan(float sin);
/**
*
* ..
*
* Purpose
* =======
*
* SLABAD takes as input the values computed by SLAMCH for underflow and
* overflow, and returns the square root of each of these values if the
* log of LARGE is sufficiently large. This subroutine is intended to
* identify machines with a large exponent range, such as the Crays, and
* redefine the underflow and overflow limits to be the square roots of
* the values computed by SLAMCH. This subroutine is needed because
* SLAMCH does not compensate for poor arithmetic in the upper half of
* the exponent range, as is found on a Cray.
*
* Arguments
* =========
*
* SMALL (input/output) REAL
* On entry, the underflow threshold as computed by SLAMCH.
* On exit, if LOG10(LARGE) is sufficiently large, the square
* root of SMALL, otherwise unchanged.
*
* LARGE (input/output) REAL
* On entry, the overflow threshold as computed by SLAMCH.
* On exit, if LOG10(LARGE) is sufficiently large, the square
* root of LARGE, otherwise unchanged.
*
* =====================================================================
*
* .. Intrinsic Functions ..
*
*
* @param small
* @param large
*
*/
abstract public void slabad(org.netlib.util.floatW small, org.netlib.util.floatW large);
/**
*
* ..
*
* Purpose
* =======
*
* SLABRD reduces the first NB rows and columns of a real general
* m by n matrix A to upper or lower bidiagonal form by an orthogonal
* transformation Q' * A * P, and returns the matrices X and Y which
* are needed to apply the transformation to the unreduced part of A.
*
* If m >= n, A is reduced to upper bidiagonal form; if m < n, to lower
* bidiagonal form.
*
* This is an auxiliary routine called by SGEBRD
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows in the matrix A.
*
* N (input) INTEGER
* The number of columns in the matrix A.
*
* NB (input) INTEGER
* The number of leading rows and columns of A to be reduced.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the m by n general matrix to be reduced.
* On exit, the first NB rows and columns of the matrix are
* overwritten; the rest of the array is unchanged.
* If m >= n, elements on and below the diagonal in the first NB
* columns, with the array TAUQ, represent the orthogonal
* matrix Q as a product of elementary reflectors; and
* elements above the diagonal in the first NB rows, with the
* array TAUP, represent the orthogonal matrix P as a product
* of elementary reflectors.
* If m < n, elements below the diagonal in the first NB
* columns, with the array TAUQ, represent the orthogonal
* matrix Q as a product of elementary reflectors, and
* elements on and above the diagonal in the first NB rows,
* with the array TAUP, represent the orthogonal matrix P as
* a product of elementary reflectors.
* See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* D (output) REAL array, dimension (NB)
* The diagonal elements of the first NB rows and columns of
* the reduced matrix. D(i) = A(i,i).
*
* E (output) REAL array, dimension (NB)
* The off-diagonal elements of the first NB rows and columns of
* the reduced matrix.
*
* TAUQ (output) REAL array dimension (NB)
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix Q. See Further Details.
*
* TAUP (output) REAL array, dimension (NB)
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix P. See Further Details.
*
* X (output) REAL array, dimension (LDX,NB)
* The m-by-nb matrix X required to update the unreduced part
* of A.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= M.
*
* Y (output) REAL array, dimension (LDY,NB)
* The n-by-nb matrix Y required to update the unreduced part
* of A.
*
* LDY (input) INTEGER
* The leading dimension of the array Y. LDY >= N.
*
* Further Details
* ===============
*
* The matrices Q and P are represented as products of elementary
* reflectors:
*
* Q = H(1) H(2) . . . H(nb) and P = G(1) G(2) . . . G(nb)
*
* Each H(i) and G(i) has the form:
*
* H(i) = I - tauq * v * v' and G(i) = I - taup * u * u'
*
* where tauq and taup are real scalars, and v and u are real vectors.
*
* If m >= n, v(1:i-1) = 0, v(i) = 1, and v(i:m) is stored on exit in
* A(i:m,i); u(1:i) = 0, u(i+1) = 1, and u(i+1:n) is stored on exit in
* A(i,i+1:n); tauq is stored in TAUQ(i) and taup in TAUP(i).
*
* If m < n, v(1:i) = 0, v(i+1) = 1, and v(i+1:m) is stored on exit in
* A(i+2:m,i); u(1:i-1) = 0, u(i) = 1, and u(i:n) is stored on exit in
* A(i,i+1:n); tauq is stored in TAUQ(i) and taup in TAUP(i).
*
* The elements of the vectors v and u together form the m-by-nb matrix
* V and the nb-by-n matrix U' which are needed, with X and Y, to apply
* the transformation to the unreduced part of the matrix, using a block
* update of the form: A := A - V*Y' - X*U'.
*
* The contents of A on exit are illustrated by the following examples
* with nb = 2:
*
* m = 6 and n = 5 (m > n): m = 5 and n = 6 (m < n):
*
* ( 1 1 u1 u1 u1 ) ( 1 u1 u1 u1 u1 u1 )
* ( v1 1 1 u2 u2 ) ( 1 1 u2 u2 u2 u2 )
* ( v1 v2 a a a ) ( v1 1 a a a a )
* ( v1 v2 a a a ) ( v1 v2 a a a a )
* ( v1 v2 a a a ) ( v1 v2 a a a a )
* ( v1 v2 a a a )
*
* where a denotes an element of the original matrix which is unchanged,
* vi denotes an element of the vector defining H(i), and ui an element
* of the vector defining G(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param nb
* @param a
* @param lda
* @param d
* @param e
* @param tauq
* @param taup
* @param x
* @param ldx
* @param y
* @param ldy
*
*/
abstract public void slabrd(int m, int n, int nb, float[] a, int lda, float[] d, float[] e, float[] tauq, float[] taup, float[] x, int ldx, float[] y, int ldy);
/**
*
* ..
*
* Purpose
* =======
*
* SLABRD reduces the first NB rows and columns of a real general
* m by n matrix A to upper or lower bidiagonal form by an orthogonal
* transformation Q' * A * P, and returns the matrices X and Y which
* are needed to apply the transformation to the unreduced part of A.
*
* If m >= n, A is reduced to upper bidiagonal form; if m < n, to lower
* bidiagonal form.
*
* This is an auxiliary routine called by SGEBRD
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows in the matrix A.
*
* N (input) INTEGER
* The number of columns in the matrix A.
*
* NB (input) INTEGER
* The number of leading rows and columns of A to be reduced.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the m by n general matrix to be reduced.
* On exit, the first NB rows and columns of the matrix are
* overwritten; the rest of the array is unchanged.
* If m >= n, elements on and below the diagonal in the first NB
* columns, with the array TAUQ, represent the orthogonal
* matrix Q as a product of elementary reflectors; and
* elements above the diagonal in the first NB rows, with the
* array TAUP, represent the orthogonal matrix P as a product
* of elementary reflectors.
* If m < n, elements below the diagonal in the first NB
* columns, with the array TAUQ, represent the orthogonal
* matrix Q as a product of elementary reflectors, and
* elements on and above the diagonal in the first NB rows,
* with the array TAUP, represent the orthogonal matrix P as
* a product of elementary reflectors.
* See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* D (output) REAL array, dimension (NB)
* The diagonal elements of the first NB rows and columns of
* the reduced matrix. D(i) = A(i,i).
*
* E (output) REAL array, dimension (NB)
* The off-diagonal elements of the first NB rows and columns of
* the reduced matrix.
*
* TAUQ (output) REAL array dimension (NB)
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix Q. See Further Details.
*
* TAUP (output) REAL array, dimension (NB)
* The scalar factors of the elementary reflectors which
* represent the orthogonal matrix P. See Further Details.
*
* X (output) REAL array, dimension (LDX,NB)
* The m-by-nb matrix X required to update the unreduced part
* of A.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= M.
*
* Y (output) REAL array, dimension (LDY,NB)
* The n-by-nb matrix Y required to update the unreduced part
* of A.
*
* LDY (input) INTEGER
* The leading dimension of the array Y. LDY >= N.
*
* Further Details
* ===============
*
* The matrices Q and P are represented as products of elementary
* reflectors:
*
* Q = H(1) H(2) . . . H(nb) and P = G(1) G(2) . . . G(nb)
*
* Each H(i) and G(i) has the form:
*
* H(i) = I - tauq * v * v' and G(i) = I - taup * u * u'
*
* where tauq and taup are real scalars, and v and u are real vectors.
*
* If m >= n, v(1:i-1) = 0, v(i) = 1, and v(i:m) is stored on exit in
* A(i:m,i); u(1:i) = 0, u(i+1) = 1, and u(i+1:n) is stored on exit in
* A(i,i+1:n); tauq is stored in TAUQ(i) and taup in TAUP(i).
*
* If m < n, v(1:i) = 0, v(i+1) = 1, and v(i+1:m) is stored on exit in
* A(i+2:m,i); u(1:i-1) = 0, u(i) = 1, and u(i:n) is stored on exit in
* A(i,i+1:n); tauq is stored in TAUQ(i) and taup in TAUP(i).
*
* The elements of the vectors v and u together form the m-by-nb matrix
* V and the nb-by-n matrix U' which are needed, with X and Y, to apply
* the transformation to the unreduced part of the matrix, using a block
* update of the form: A := A - V*Y' - X*U'.
*
* The contents of A on exit are illustrated by the following examples
* with nb = 2:
*
* m = 6 and n = 5 (m > n): m = 5 and n = 6 (m < n):
*
* ( 1 1 u1 u1 u1 ) ( 1 u1 u1 u1 u1 u1 )
* ( v1 1 1 u2 u2 ) ( 1 1 u2 u2 u2 u2 )
* ( v1 v2 a a a ) ( v1 1 a a a a )
* ( v1 v2 a a a ) ( v1 v2 a a a a )
* ( v1 v2 a a a ) ( v1 v2 a a a a )
* ( v1 v2 a a a )
*
* where a denotes an element of the original matrix which is unchanged,
* vi denotes an element of the vector defining H(i), and ui an element
* of the vector defining G(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param nb
* @param a
* @param _a_offset
* @param lda
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param tauq
* @param _tauq_offset
* @param taup
* @param _taup_offset
* @param x
* @param _x_offset
* @param ldx
* @param y
* @param _y_offset
* @param ldy
*
*/
abstract public void slabrd(int m, int n, int nb, float[] a, int _a_offset, int lda, float[] d, int _d_offset, float[] e, int _e_offset, float[] tauq, int _tauq_offset, float[] taup, int _taup_offset, float[] x, int _x_offset, int ldx, float[] y, int _y_offset, int ldy);
/**
*
* ..
*
* Purpose
* =======
*
* SLACN2 estimates the 1-norm of a square, real matrix A.
* Reverse communication is used for evaluating matrix-vector products.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix. N >= 1.
*
* V (workspace) REAL array, dimension (N)
* On the final return, V = A*W, where EST = norm(V)/norm(W)
* (W is not returned).
*
* X (input/output) REAL array, dimension (N)
* On an intermediate return, X should be overwritten by
* A * X, if KASE=1,
* A' * X, if KASE=2,
* and SLACN2 must be re-called with all the other parameters
* unchanged.
*
* ISGN (workspace) INTEGER array, dimension (N)
*
* EST (input/output) REAL
* On entry with KASE = 1 or 2 and ISAVE(1) = 3, EST should be
* unchanged from the previous call to SLACN2.
* On exit, EST is an estimate (a lower bound) for norm(A).
*
* KASE (input/output) INTEGER
* On the initial call to SLACN2, KASE should be 0.
* On an intermediate return, KASE will be 1 or 2, indicating
* whether X should be overwritten by A * X or A' * X.
* On the final return from SLACN2, KASE will again be 0.
*
* ISAVE (input/output) INTEGER array, dimension (3)
* ISAVE is used to save variables between calls to SLACN2
*
* Further Details
* ======= =======
*
* Contributed by Nick Higham, University of Manchester.
* Originally named SONEST, dated March 16, 1988.
*
* Reference: N.J. Higham, "FORTRAN codes for estimating the one-norm of
* a real or complex matrix, with applications to condition estimation",
* ACM Trans. Math. Soft., vol. 14, no. 4, pp. 381-396, December 1988.
*
* This is a thread safe version of SLACON, which uses the array ISAVE
* in place of a SAVE statement, as follows:
*
* SLACON SLACN2
* JUMP ISAVE(1)
* J ISAVE(2)
* ITER ISAVE(3)
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param v
* @param x
* @param isgn
* @param est
* @param kase
* @param isave
*
*/
abstract public void slacn2(int n, float[] v, float[] x, int[] isgn, org.netlib.util.floatW est, org.netlib.util.intW kase, int[] isave);
/**
*
* ..
*
* Purpose
* =======
*
* SLACN2 estimates the 1-norm of a square, real matrix A.
* Reverse communication is used for evaluating matrix-vector products.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix. N >= 1.
*
* V (workspace) REAL array, dimension (N)
* On the final return, V = A*W, where EST = norm(V)/norm(W)
* (W is not returned).
*
* X (input/output) REAL array, dimension (N)
* On an intermediate return, X should be overwritten by
* A * X, if KASE=1,
* A' * X, if KASE=2,
* and SLACN2 must be re-called with all the other parameters
* unchanged.
*
* ISGN (workspace) INTEGER array, dimension (N)
*
* EST (input/output) REAL
* On entry with KASE = 1 or 2 and ISAVE(1) = 3, EST should be
* unchanged from the previous call to SLACN2.
* On exit, EST is an estimate (a lower bound) for norm(A).
*
* KASE (input/output) INTEGER
* On the initial call to SLACN2, KASE should be 0.
* On an intermediate return, KASE will be 1 or 2, indicating
* whether X should be overwritten by A * X or A' * X.
* On the final return from SLACN2, KASE will again be 0.
*
* ISAVE (input/output) INTEGER array, dimension (3)
* ISAVE is used to save variables between calls to SLACN2
*
* Further Details
* ======= =======
*
* Contributed by Nick Higham, University of Manchester.
* Originally named SONEST, dated March 16, 1988.
*
* Reference: N.J. Higham, "FORTRAN codes for estimating the one-norm of
* a real or complex matrix, with applications to condition estimation",
* ACM Trans. Math. Soft., vol. 14, no. 4, pp. 381-396, December 1988.
*
* This is a thread safe version of SLACON, which uses the array ISAVE
* in place of a SAVE statement, as follows:
*
* SLACON SLACN2
* JUMP ISAVE(1)
* J ISAVE(2)
* ITER ISAVE(3)
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param v
* @param _v_offset
* @param x
* @param _x_offset
* @param isgn
* @param _isgn_offset
* @param est
* @param kase
* @param isave
* @param _isave_offset
*
*/
abstract public void slacn2(int n, float[] v, int _v_offset, float[] x, int _x_offset, int[] isgn, int _isgn_offset, org.netlib.util.floatW est, org.netlib.util.intW kase, int[] isave, int _isave_offset);
/**
*
* ..
*
* Purpose
* =======
*
* SLACON estimates the 1-norm of a square, real matrix A.
* Reverse communication is used for evaluating matrix-vector products.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix. N >= 1.
*
* V (workspace) REAL array, dimension (N)
* On the final return, V = A*W, where EST = norm(V)/norm(W)
* (W is not returned).
*
* X (input/output) REAL array, dimension (N)
* On an intermediate return, X should be overwritten by
* A * X, if KASE=1,
* A' * X, if KASE=2,
* and SLACON must be re-called with all the other parameters
* unchanged.
*
* ISGN (workspace) INTEGER array, dimension (N)
*
* EST (input/output) REAL
* On entry with KASE = 1 or 2 and JUMP = 3, EST should be
* unchanged from the previous call to SLACON.
* On exit, EST is an estimate (a lower bound) for norm(A).
*
* KASE (input/output) INTEGER
* On the initial call to SLACON, KASE should be 0.
* On an intermediate return, KASE will be 1 or 2, indicating
* whether X should be overwritten by A * X or A' * X.
* On the final return from SLACON, KASE will again be 0.
*
* Further Details
* ======= =======
*
* Contributed by Nick Higham, University of Manchester.
* Originally named SONEST, dated March 16, 1988.
*
* Reference: N.J. Higham, "FORTRAN codes for estimating the one-norm of
* a real or complex matrix, with applications to condition estimation",
* ACM Trans. Math. Soft., vol. 14, no. 4, pp. 381-396, December 1988.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param v
* @param x
* @param isgn
* @param est
* @param kase
*
*/
abstract public void slacon(int n, float[] v, float[] x, int[] isgn, org.netlib.util.floatW est, org.netlib.util.intW kase);
/**
*
* ..
*
* Purpose
* =======
*
* SLACON estimates the 1-norm of a square, real matrix A.
* Reverse communication is used for evaluating matrix-vector products.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix. N >= 1.
*
* V (workspace) REAL array, dimension (N)
* On the final return, V = A*W, where EST = norm(V)/norm(W)
* (W is not returned).
*
* X (input/output) REAL array, dimension (N)
* On an intermediate return, X should be overwritten by
* A * X, if KASE=1,
* A' * X, if KASE=2,
* and SLACON must be re-called with all the other parameters
* unchanged.
*
* ISGN (workspace) INTEGER array, dimension (N)
*
* EST (input/output) REAL
* On entry with KASE = 1 or 2 and JUMP = 3, EST should be
* unchanged from the previous call to SLACON.
* On exit, EST is an estimate (a lower bound) for norm(A).
*
* KASE (input/output) INTEGER
* On the initial call to SLACON, KASE should be 0.
* On an intermediate return, KASE will be 1 or 2, indicating
* whether X should be overwritten by A * X or A' * X.
* On the final return from SLACON, KASE will again be 0.
*
* Further Details
* ======= =======
*
* Contributed by Nick Higham, University of Manchester.
* Originally named SONEST, dated March 16, 1988.
*
* Reference: N.J. Higham, "FORTRAN codes for estimating the one-norm of
* a real or complex matrix, with applications to condition estimation",
* ACM Trans. Math. Soft., vol. 14, no. 4, pp. 381-396, December 1988.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param v
* @param _v_offset
* @param x
* @param _x_offset
* @param isgn
* @param _isgn_offset
* @param est
* @param kase
*
*/
abstract public void slacon(int n, float[] v, int _v_offset, float[] x, int _x_offset, int[] isgn, int _isgn_offset, org.netlib.util.floatW est, org.netlib.util.intW kase);
/**
*
* ..
*
* Purpose
* =======
*
* SLACPY copies all or part of a two-dimensional matrix A to another
* matrix B.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies the part of the matrix A to be copied to B.
* = 'U': Upper triangular part
* = 'L': Lower triangular part
* Otherwise: All of the matrix A
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The m by n matrix A. If UPLO = 'U', only the upper triangle
* or trapezoid is accessed; if UPLO = 'L', only the lower
* triangle or trapezoid is accessed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (output) REAL array, dimension (LDB,N)
* On exit, B = A in the locations specified by UPLO.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,M).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param uplo
* @param m
* @param n
* @param a
* @param lda
* @param b
* @param ldb
*
*/
abstract public void slacpy(java.lang.String uplo, int m, int n, float[] a, int lda, float[] b, int ldb);
/**
*
* ..
*
* Purpose
* =======
*
* SLACPY copies all or part of a two-dimensional matrix A to another
* matrix B.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies the part of the matrix A to be copied to B.
* = 'U': Upper triangular part
* = 'L': Lower triangular part
* Otherwise: All of the matrix A
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The m by n matrix A. If UPLO = 'U', only the upper triangle
* or trapezoid is accessed; if UPLO = 'L', only the lower
* triangle or trapezoid is accessed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (output) REAL array, dimension (LDB,N)
* On exit, B = A in the locations specified by UPLO.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,M).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param uplo
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
*
*/
abstract public void slacpy(java.lang.String uplo, int m, int n, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb);
/**
*
* ..
*
* Purpose
* =======
*
* SLADIV performs complex division in real arithmetic
*
* a + i*b
* p + i*q = ---------
* c + i*d
*
* The algorithm is due to Robert L. Smith and can be found
* in D. Knuth, The art of Computer Programming, Vol.2, p.195
*
* Arguments
* =========
*
* A (input) REAL
* B (input) REAL
* C (input) REAL
* D (input) REAL
* The scalars a, b, c, and d in the above expression.
*
* P (output) REAL
* Q (output) REAL
* The scalars p and q in the above expression.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param a
* @param b
* @param c
* @param d
* @param p
* @param q
*
*/
abstract public void sladiv(float a, float b, float c, float d, org.netlib.util.floatW p, org.netlib.util.floatW q);
/**
*
* ..
*
* Purpose
* =======
*
* SLAE2 computes the eigenvalues of a 2-by-2 symmetric matrix
* [ A B ]
* [ B C ].
* On return, RT1 is the eigenvalue of larger absolute value, and RT2
* is the eigenvalue of smaller absolute value.
*
* Arguments
* =========
*
* A (input) REAL
* The (1,1) element of the 2-by-2 matrix.
*
* B (input) REAL
* The (1,2) and (2,1) elements of the 2-by-2 matrix.
*
* C (input) REAL
* The (2,2) element of the 2-by-2 matrix.
*
* RT1 (output) REAL
* The eigenvalue of larger absolute value.
*
* RT2 (output) REAL
* The eigenvalue of smaller absolute value.
*
* Further Details
* ===============
*
* RT1 is accurate to a few ulps barring over/underflow.
*
* RT2 may be inaccurate if there is massive cancellation in the
* determinant A*C-B*B; higher precision or correctly rounded or
* correctly truncated arithmetic would be needed to compute RT2
* accurately in all cases.
*
* Overflow is possible only if RT1 is within a factor of 5 of overflow.
* Underflow is harmless if the input data is 0 or exceeds
* underflow_threshold / macheps.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param a
* @param b
* @param c
* @param rt1
* @param rt2
*
*/
abstract public void slae2(float a, float b, float c, org.netlib.util.floatW rt1, org.netlib.util.floatW rt2);
/**
*
* ..
*
* Purpose
* =======
*
* SLAEBZ contains the iteration loops which compute and use the
* function N(w), which is the count of eigenvalues of a symmetric
* tridiagonal matrix T less than or equal to its argument w. It
* performs a choice of two types of loops:
*
* IJOB=1, followed by
* IJOB=2: It takes as input a list of intervals and returns a list of
* sufficiently small intervals whose union contains the same
* eigenvalues as the union of the original intervals.
* The input intervals are (AB(j,1),AB(j,2)], j=1,...,MINP.
* The output interval (AB(j,1),AB(j,2)] will contain
* eigenvalues NAB(j,1)+1,...,NAB(j,2), where 1 <= j <= MOUT.
*
* IJOB=3: It performs a binary search in each input interval
* (AB(j,1),AB(j,2)] for a point w(j) such that
* N(w(j))=NVAL(j), and uses C(j) as the starting point of
* the search. If such a w(j) is found, then on output
* AB(j,1)=AB(j,2)=w. If no such w(j) is found, then on output
* (AB(j,1),AB(j,2)] will be a small interval containing the
* point where N(w) jumps through NVAL(j), unless that point
* lies outside the initial interval.
*
* Note that the intervals are in all cases half-open intervals,
* i.e., of the form (a,b] , which includes b but not a .
*
* To avoid underflow, the matrix should be scaled so that its largest
* element is no greater than overflow**(1/2) * underflow**(1/4)
* in absolute value. To assure the most accurate computation
* of small eigenvalues, the matrix should be scaled to be
* not much smaller than that, either.
*
* See W. Kahan "Accurate Eigenvalues of a Symmetric Tridiagonal
* Matrix", Report CS41, Computer Science Dept., Stanford
* University, July 21, 1966
*
* Note: the arguments are, in general, *not* checked for unreasonable
* values.
*
* Arguments
* =========
*
* IJOB (input) INTEGER
* Specifies what is to be done:
* = 1: Compute NAB for the initial intervals.
* = 2: Perform bisection iteration to find eigenvalues of T.
* = 3: Perform bisection iteration to invert N(w), i.e.,
* to find a point which has a specified number of
* eigenvalues of T to its left.
* Other values will cause SLAEBZ to return with INFO=-1.
*
* NITMAX (input) INTEGER
* The maximum number of "levels" of bisection to be
* performed, i.e., an interval of width W will not be made
* smaller than 2^(-NITMAX) * W. If not all intervals
* have converged after NITMAX iterations, then INFO is set
* to the number of non-converged intervals.
*
* N (input) INTEGER
* The dimension n of the tridiagonal matrix T. It must be at
* least 1.
*
* MMAX (input) INTEGER
* The maximum number of intervals. If more than MMAX intervals
* are generated, then SLAEBZ will quit with INFO=MMAX+1.
*
* MINP (input) INTEGER
* The initial number of intervals. It may not be greater than
* MMAX.
*
* NBMIN (input) INTEGER
* The smallest number of intervals that should be processed
* using a vector loop. If zero, then only the scalar loop
* will be used.
*
* ABSTOL (input) REAL
* The minimum (absolute) width of an interval. When an
* interval is narrower than ABSTOL, or than RELTOL times the
* larger (in magnitude) endpoint, then it is considered to be
* sufficiently small, i.e., converged. This must be at least
* zero.
*
* RELTOL (input) REAL
* The minimum relative width of an interval. When an interval
* is narrower than ABSTOL, or than RELTOL times the larger (in
* magnitude) endpoint, then it is considered to be
* sufficiently small, i.e., converged. Note: this should
* always be at least radix*machine epsilon.
*
* PIVMIN (input) REAL
* The minimum absolute value of a "pivot" in the Sturm
* sequence loop. This *must* be at least max |e(j)**2| *
* safe_min and at least safe_min, where safe_min is at least
* the smallest number that can divide one without overflow.
*
* D (input) REAL array, dimension (N)
* The diagonal elements of the tridiagonal matrix T.
*
* E (input) REAL array, dimension (N)
* The offdiagonal elements of the tridiagonal matrix T in
* positions 1 through N-1. E(N) is arbitrary.
*
* E2 (input) REAL array, dimension (N)
* The squares of the offdiagonal elements of the tridiagonal
* matrix T. E2(N) is ignored.
*
* NVAL (input/output) INTEGER array, dimension (MINP)
* If IJOB=1 or 2, not referenced.
* If IJOB=3, the desired values of N(w). The elements of NVAL
* will be reordered to correspond with the intervals in AB.
* Thus, NVAL(j) on output will not, in general be the same as
* NVAL(j) on input, but it will correspond with the interval
* (AB(j,1),AB(j,2)] on output.
*
* AB (input/output) REAL array, dimension (MMAX,2)
* The endpoints of the intervals. AB(j,1) is a(j), the left
* endpoint of the j-th interval, and AB(j,2) is b(j), the
* right endpoint of the j-th interval. The input intervals
* will, in general, be modified, split, and reordered by the
* calculation.
*
* C (input/output) REAL array, dimension (MMAX)
* If IJOB=1, ignored.
* If IJOB=2, workspace.
* If IJOB=3, then on input C(j) should be initialized to the
* first search point in the binary search.
*
* MOUT (output) INTEGER
* If IJOB=1, the number of eigenvalues in the intervals.
* If IJOB=2 or 3, the number of intervals output.
* If IJOB=3, MOUT will equal MINP.
*
* NAB (input/output) INTEGER array, dimension (MMAX,2)
* If IJOB=1, then on output NAB(i,j) will be set to N(AB(i,j)).
* If IJOB=2, then on input, NAB(i,j) should be set. It must
* satisfy the condition:
* N(AB(i,1)) <= NAB(i,1) <= NAB(i,2) <= N(AB(i,2)),
* which means that in interval i only eigenvalues
* NAB(i,1)+1,...,NAB(i,2) will be considered. Usually,
* NAB(i,j)=N(AB(i,j)), from a previous call to SLAEBZ with
* IJOB=1.
* On output, NAB(i,j) will contain
* max(na(k),min(nb(k),N(AB(i,j)))), where k is the index of
* the input interval that the output interval
* (AB(j,1),AB(j,2)] came from, and na(k) and nb(k) are the
* the input values of NAB(k,1) and NAB(k,2).
* If IJOB=3, then on output, NAB(i,j) contains N(AB(i,j)),
* unless N(w) > NVAL(i) for all search points w , in which
* case NAB(i,1) will not be modified, i.e., the output
* value will be the same as the input value (modulo
* reorderings -- see NVAL and AB), or unless N(w) < NVAL(i)
* for all search points w , in which case NAB(i,2) will
* not be modified. Normally, NAB should be set to some
* distinctive value(s) before SLAEBZ is called.
*
* WORK (workspace) REAL array, dimension (MMAX)
* Workspace.
*
* IWORK (workspace) INTEGER array, dimension (MMAX)
* Workspace.
*
* INFO (output) INTEGER
* = 0: All intervals converged.
* = 1--MMAX: The last INFO intervals did not converge.
* = MMAX+1: More than MMAX intervals were generated.
*
* Further Details
* ===============
*
* This routine is intended to be called only by other LAPACK
* routines, thus the interface is less user-friendly. It is intended
* for two purposes:
*
* (a) finding eigenvalues. In this case, SLAEBZ should have one or
* more initial intervals set up in AB, and SLAEBZ should be called
* with IJOB=1. This sets up NAB, and also counts the eigenvalues.
* Intervals with no eigenvalues would usually be thrown out at
* this point. Also, if not all the eigenvalues in an interval i
* are desired, NAB(i,1) can be increased or NAB(i,2) decreased.
* For example, set NAB(i,1)=NAB(i,2)-1 to get the largest
* eigenvalue. SLAEBZ is then called with IJOB=2 and MMAX
* no smaller than the value of MOUT returned by the call with
* IJOB=1. After this (IJOB=2) call, eigenvalues NAB(i,1)+1
* through NAB(i,2) are approximately AB(i,1) (or AB(i,2)) to the
* tolerance specified by ABSTOL and RELTOL.
*
* (b) finding an interval (a',b'] containing eigenvalues w(f),...,w(l).
* In this case, start with a Gershgorin interval (a,b). Set up
* AB to contain 2 search intervals, both initially (a,b). One
* NVAL element should contain f-1 and the other should contain l
* , while C should contain a and b, resp. NAB(i,1) should be -1
* and NAB(i,2) should be N+1, to flag an error if the desired
* interval does not lie in (a,b). SLAEBZ is then called with
* IJOB=3. On exit, if w(f-1) < w(f), then one of the intervals --
* j -- will have AB(j,1)=AB(j,2) and NAB(j,1)=NAB(j,2)=f-1, while
* if, to the specified tolerance, w(f-k)=...=w(f+r), k > 0 and r
* >= 0, then the interval will have N(AB(j,1))=NAB(j,1)=f-k and
* N(AB(j,2))=NAB(j,2)=f+r. The cases w(l) < w(l+1) and
* w(l-r)=...=w(l+k) are handled similarly.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param ijob
* @param nitmax
* @param n
* @param mmax
* @param minp
* @param nbmin
* @param abstol
* @param reltol
* @param pivmin
* @param d
* @param e
* @param e2
* @param nval
* @param ab
* @param c
* @param mout
* @param nab
* @param work
* @param iwork
* @param info
*
*/
abstract public void slaebz(int ijob, int nitmax, int n, int mmax, int minp, int nbmin, float abstol, float reltol, float pivmin, float[] d, float[] e, float[] e2, int[] nval, float[] ab, float[] c, org.netlib.util.intW mout, int[] nab, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAEBZ contains the iteration loops which compute and use the
* function N(w), which is the count of eigenvalues of a symmetric
* tridiagonal matrix T less than or equal to its argument w. It
* performs a choice of two types of loops:
*
* IJOB=1, followed by
* IJOB=2: It takes as input a list of intervals and returns a list of
* sufficiently small intervals whose union contains the same
* eigenvalues as the union of the original intervals.
* The input intervals are (AB(j,1),AB(j,2)], j=1,...,MINP.
* The output interval (AB(j,1),AB(j,2)] will contain
* eigenvalues NAB(j,1)+1,...,NAB(j,2), where 1 <= j <= MOUT.
*
* IJOB=3: It performs a binary search in each input interval
* (AB(j,1),AB(j,2)] for a point w(j) such that
* N(w(j))=NVAL(j), and uses C(j) as the starting point of
* the search. If such a w(j) is found, then on output
* AB(j,1)=AB(j,2)=w. If no such w(j) is found, then on output
* (AB(j,1),AB(j,2)] will be a small interval containing the
* point where N(w) jumps through NVAL(j), unless that point
* lies outside the initial interval.
*
* Note that the intervals are in all cases half-open intervals,
* i.e., of the form (a,b] , which includes b but not a .
*
* To avoid underflow, the matrix should be scaled so that its largest
* element is no greater than overflow**(1/2) * underflow**(1/4)
* in absolute value. To assure the most accurate computation
* of small eigenvalues, the matrix should be scaled to be
* not much smaller than that, either.
*
* See W. Kahan "Accurate Eigenvalues of a Symmetric Tridiagonal
* Matrix", Report CS41, Computer Science Dept., Stanford
* University, July 21, 1966
*
* Note: the arguments are, in general, *not* checked for unreasonable
* values.
*
* Arguments
* =========
*
* IJOB (input) INTEGER
* Specifies what is to be done:
* = 1: Compute NAB for the initial intervals.
* = 2: Perform bisection iteration to find eigenvalues of T.
* = 3: Perform bisection iteration to invert N(w), i.e.,
* to find a point which has a specified number of
* eigenvalues of T to its left.
* Other values will cause SLAEBZ to return with INFO=-1.
*
* NITMAX (input) INTEGER
* The maximum number of "levels" of bisection to be
* performed, i.e., an interval of width W will not be made
* smaller than 2^(-NITMAX) * W. If not all intervals
* have converged after NITMAX iterations, then INFO is set
* to the number of non-converged intervals.
*
* N (input) INTEGER
* The dimension n of the tridiagonal matrix T. It must be at
* least 1.
*
* MMAX (input) INTEGER
* The maximum number of intervals. If more than MMAX intervals
* are generated, then SLAEBZ will quit with INFO=MMAX+1.
*
* MINP (input) INTEGER
* The initial number of intervals. It may not be greater than
* MMAX.
*
* NBMIN (input) INTEGER
* The smallest number of intervals that should be processed
* using a vector loop. If zero, then only the scalar loop
* will be used.
*
* ABSTOL (input) REAL
* The minimum (absolute) width of an interval. When an
* interval is narrower than ABSTOL, or than RELTOL times the
* larger (in magnitude) endpoint, then it is considered to be
* sufficiently small, i.e., converged. This must be at least
* zero.
*
* RELTOL (input) REAL
* The minimum relative width of an interval. When an interval
* is narrower than ABSTOL, or than RELTOL times the larger (in
* magnitude) endpoint, then it is considered to be
* sufficiently small, i.e., converged. Note: this should
* always be at least radix*machine epsilon.
*
* PIVMIN (input) REAL
* The minimum absolute value of a "pivot" in the Sturm
* sequence loop. This *must* be at least max |e(j)**2| *
* safe_min and at least safe_min, where safe_min is at least
* the smallest number that can divide one without overflow.
*
* D (input) REAL array, dimension (N)
* The diagonal elements of the tridiagonal matrix T.
*
* E (input) REAL array, dimension (N)
* The offdiagonal elements of the tridiagonal matrix T in
* positions 1 through N-1. E(N) is arbitrary.
*
* E2 (input) REAL array, dimension (N)
* The squares of the offdiagonal elements of the tridiagonal
* matrix T. E2(N) is ignored.
*
* NVAL (input/output) INTEGER array, dimension (MINP)
* If IJOB=1 or 2, not referenced.
* If IJOB=3, the desired values of N(w). The elements of NVAL
* will be reordered to correspond with the intervals in AB.
* Thus, NVAL(j) on output will not, in general be the same as
* NVAL(j) on input, but it will correspond with the interval
* (AB(j,1),AB(j,2)] on output.
*
* AB (input/output) REAL array, dimension (MMAX,2)
* The endpoints of the intervals. AB(j,1) is a(j), the left
* endpoint of the j-th interval, and AB(j,2) is b(j), the
* right endpoint of the j-th interval. The input intervals
* will, in general, be modified, split, and reordered by the
* calculation.
*
* C (input/output) REAL array, dimension (MMAX)
* If IJOB=1, ignored.
* If IJOB=2, workspace.
* If IJOB=3, then on input C(j) should be initialized to the
* first search point in the binary search.
*
* MOUT (output) INTEGER
* If IJOB=1, the number of eigenvalues in the intervals.
* If IJOB=2 or 3, the number of intervals output.
* If IJOB=3, MOUT will equal MINP.
*
* NAB (input/output) INTEGER array, dimension (MMAX,2)
* If IJOB=1, then on output NAB(i,j) will be set to N(AB(i,j)).
* If IJOB=2, then on input, NAB(i,j) should be set. It must
* satisfy the condition:
* N(AB(i,1)) <= NAB(i,1) <= NAB(i,2) <= N(AB(i,2)),
* which means that in interval i only eigenvalues
* NAB(i,1)+1,...,NAB(i,2) will be considered. Usually,
* NAB(i,j)=N(AB(i,j)), from a previous call to SLAEBZ with
* IJOB=1.
* On output, NAB(i,j) will contain
* max(na(k),min(nb(k),N(AB(i,j)))), where k is the index of
* the input interval that the output interval
* (AB(j,1),AB(j,2)] came from, and na(k) and nb(k) are the
* the input values of NAB(k,1) and NAB(k,2).
* If IJOB=3, then on output, NAB(i,j) contains N(AB(i,j)),
* unless N(w) > NVAL(i) for all search points w , in which
* case NAB(i,1) will not be modified, i.e., the output
* value will be the same as the input value (modulo
* reorderings -- see NVAL and AB), or unless N(w) < NVAL(i)
* for all search points w , in which case NAB(i,2) will
* not be modified. Normally, NAB should be set to some
* distinctive value(s) before SLAEBZ is called.
*
* WORK (workspace) REAL array, dimension (MMAX)
* Workspace.
*
* IWORK (workspace) INTEGER array, dimension (MMAX)
* Workspace.
*
* INFO (output) INTEGER
* = 0: All intervals converged.
* = 1--MMAX: The last INFO intervals did not converge.
* = MMAX+1: More than MMAX intervals were generated.
*
* Further Details
* ===============
*
* This routine is intended to be called only by other LAPACK
* routines, thus the interface is less user-friendly. It is intended
* for two purposes:
*
* (a) finding eigenvalues. In this case, SLAEBZ should have one or
* more initial intervals set up in AB, and SLAEBZ should be called
* with IJOB=1. This sets up NAB, and also counts the eigenvalues.
* Intervals with no eigenvalues would usually be thrown out at
* this point. Also, if not all the eigenvalues in an interval i
* are desired, NAB(i,1) can be increased or NAB(i,2) decreased.
* For example, set NAB(i,1)=NAB(i,2)-1 to get the largest
* eigenvalue. SLAEBZ is then called with IJOB=2 and MMAX
* no smaller than the value of MOUT returned by the call with
* IJOB=1. After this (IJOB=2) call, eigenvalues NAB(i,1)+1
* through NAB(i,2) are approximately AB(i,1) (or AB(i,2)) to the
* tolerance specified by ABSTOL and RELTOL.
*
* (b) finding an interval (a',b'] containing eigenvalues w(f),...,w(l).
* In this case, start with a Gershgorin interval (a,b). Set up
* AB to contain 2 search intervals, both initially (a,b). One
* NVAL element should contain f-1 and the other should contain l
* , while C should contain a and b, resp. NAB(i,1) should be -1
* and NAB(i,2) should be N+1, to flag an error if the desired
* interval does not lie in (a,b). SLAEBZ is then called with
* IJOB=3. On exit, if w(f-1) < w(f), then one of the intervals --
* j -- will have AB(j,1)=AB(j,2) and NAB(j,1)=NAB(j,2)=f-1, while
* if, to the specified tolerance, w(f-k)=...=w(f+r), k > 0 and r
* >= 0, then the interval will have N(AB(j,1))=NAB(j,1)=f-k and
* N(AB(j,2))=NAB(j,2)=f+r. The cases w(l) < w(l+1) and
* w(l-r)=...=w(l+k) are handled similarly.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param ijob
* @param nitmax
* @param n
* @param mmax
* @param minp
* @param nbmin
* @param abstol
* @param reltol
* @param pivmin
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param e2
* @param _e2_offset
* @param nval
* @param _nval_offset
* @param ab
* @param _ab_offset
* @param c
* @param _c_offset
* @param mout
* @param nab
* @param _nab_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void slaebz(int ijob, int nitmax, int n, int mmax, int minp, int nbmin, float abstol, float reltol, float pivmin, float[] d, int _d_offset, float[] e, int _e_offset, float[] e2, int _e2_offset, int[] nval, int _nval_offset, float[] ab, int _ab_offset, float[] c, int _c_offset, org.netlib.util.intW mout, int[] nab, int _nab_offset, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAED0 computes all eigenvalues and corresponding eigenvectors of a
* symmetric tridiagonal matrix using the divide and conquer method.
*
* Arguments
* =========
*
* ICOMPQ (input) INTEGER
* = 0: Compute eigenvalues only.
* = 1: Compute eigenvectors of original dense symmetric matrix
* also. On entry, Q contains the orthogonal matrix used
* to reduce the original matrix to tridiagonal form.
* = 2: Compute eigenvalues and eigenvectors of tridiagonal
* matrix.
*
* QSIZ (input) INTEGER
* The dimension of the orthogonal matrix used to reduce
* the full matrix to tridiagonal form. QSIZ >= N if ICOMPQ = 1.
*
* N (input) INTEGER
* The dimension of the symmetric tridiagonal matrix. N >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, the main diagonal of the tridiagonal matrix.
* On exit, its eigenvalues.
*
* E (input) REAL array, dimension (N-1)
* The off-diagonal elements of the tridiagonal matrix.
* On exit, E has been destroyed.
*
* Q (input/output) REAL array, dimension (LDQ, N)
* On entry, Q must contain an N-by-N orthogonal matrix.
* If ICOMPQ = 0 Q is not referenced.
* If ICOMPQ = 1 On entry, Q is a subset of the columns of the
* orthogonal matrix used to reduce the full
* matrix to tridiagonal form corresponding to
* the subset of the full matrix which is being
* decomposed at this time.
* If ICOMPQ = 2 On entry, Q will be the identity matrix.
* On exit, Q contains the eigenvectors of the
* tridiagonal matrix.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. If eigenvectors are
* desired, then LDQ >= max(1,N). In any case, LDQ >= 1.
*
* QSTORE (workspace) REAL array, dimension (LDQS, N)
* Referenced only when ICOMPQ = 1. Used to store parts of
* the eigenvector matrix when the updating matrix multiplies
* take place.
*
* LDQS (input) INTEGER
* The leading dimension of the array QSTORE. If ICOMPQ = 1,
* then LDQS >= max(1,N). In any case, LDQS >= 1.
*
* WORK (workspace) REAL array,
* If ICOMPQ = 0 or 1, the dimension of WORK must be at least
* 1 + 3*N + 2*N*lg N + 2*N**2
* ( lg( N ) = smallest integer k
* such that 2^k >= N )
* If ICOMPQ = 2, the dimension of WORK must be at least
* 4*N + N**2.
*
* IWORK (workspace) INTEGER array,
* If ICOMPQ = 0 or 1, the dimension of IWORK must be at least
* 6 + 6*N + 5*N*lg N.
* ( lg( N ) = smallest integer k
* such that 2^k >= N )
* If ICOMPQ = 2, the dimension of IWORK must be at least
* 3 + 5*N.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: The algorithm failed to compute an eigenvalue while
* working on the submatrix lying in rows and columns
* INFO/(N+1) through mod(INFO,N+1).
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param qsiz
* @param n
* @param d
* @param e
* @param q
* @param ldq
* @param qstore
* @param ldqs
* @param work
* @param iwork
* @param info
*
*/
abstract public void slaed0(int icompq, int qsiz, int n, float[] d, float[] e, float[] q, int ldq, float[] qstore, int ldqs, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAED0 computes all eigenvalues and corresponding eigenvectors of a
* symmetric tridiagonal matrix using the divide and conquer method.
*
* Arguments
* =========
*
* ICOMPQ (input) INTEGER
* = 0: Compute eigenvalues only.
* = 1: Compute eigenvectors of original dense symmetric matrix
* also. On entry, Q contains the orthogonal matrix used
* to reduce the original matrix to tridiagonal form.
* = 2: Compute eigenvalues and eigenvectors of tridiagonal
* matrix.
*
* QSIZ (input) INTEGER
* The dimension of the orthogonal matrix used to reduce
* the full matrix to tridiagonal form. QSIZ >= N if ICOMPQ = 1.
*
* N (input) INTEGER
* The dimension of the symmetric tridiagonal matrix. N >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, the main diagonal of the tridiagonal matrix.
* On exit, its eigenvalues.
*
* E (input) REAL array, dimension (N-1)
* The off-diagonal elements of the tridiagonal matrix.
* On exit, E has been destroyed.
*
* Q (input/output) REAL array, dimension (LDQ, N)
* On entry, Q must contain an N-by-N orthogonal matrix.
* If ICOMPQ = 0 Q is not referenced.
* If ICOMPQ = 1 On entry, Q is a subset of the columns of the
* orthogonal matrix used to reduce the full
* matrix to tridiagonal form corresponding to
* the subset of the full matrix which is being
* decomposed at this time.
* If ICOMPQ = 2 On entry, Q will be the identity matrix.
* On exit, Q contains the eigenvectors of the
* tridiagonal matrix.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. If eigenvectors are
* desired, then LDQ >= max(1,N). In any case, LDQ >= 1.
*
* QSTORE (workspace) REAL array, dimension (LDQS, N)
* Referenced only when ICOMPQ = 1. Used to store parts of
* the eigenvector matrix when the updating matrix multiplies
* take place.
*
* LDQS (input) INTEGER
* The leading dimension of the array QSTORE. If ICOMPQ = 1,
* then LDQS >= max(1,N). In any case, LDQS >= 1.
*
* WORK (workspace) REAL array,
* If ICOMPQ = 0 or 1, the dimension of WORK must be at least
* 1 + 3*N + 2*N*lg N + 2*N**2
* ( lg( N ) = smallest integer k
* such that 2^k >= N )
* If ICOMPQ = 2, the dimension of WORK must be at least
* 4*N + N**2.
*
* IWORK (workspace) INTEGER array,
* If ICOMPQ = 0 or 1, the dimension of IWORK must be at least
* 6 + 6*N + 5*N*lg N.
* ( lg( N ) = smallest integer k
* such that 2^k >= N )
* If ICOMPQ = 2, the dimension of IWORK must be at least
* 3 + 5*N.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: The algorithm failed to compute an eigenvalue while
* working on the submatrix lying in rows and columns
* INFO/(N+1) through mod(INFO,N+1).
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param qsiz
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param q
* @param _q_offset
* @param ldq
* @param qstore
* @param _qstore_offset
* @param ldqs
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void slaed0(int icompq, int qsiz, int n, float[] d, int _d_offset, float[] e, int _e_offset, float[] q, int _q_offset, int ldq, float[] qstore, int _qstore_offset, int ldqs, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAED1 computes the updated eigensystem of a diagonal
* matrix after modification by a rank-one symmetric matrix. This
* routine is used only for the eigenproblem which requires all
* eigenvalues and eigenvectors of a tridiagonal matrix. SLAED7 handles
* the case in which eigenvalues only or eigenvalues and eigenvectors
* of a full symmetric matrix (which was reduced to tridiagonal form)
* are desired.
*
* T = Q(in) ( D(in) + RHO * Z*Z' ) Q'(in) = Q(out) * D(out) * Q'(out)
*
* where Z = Q'u, u is a vector of length N with ones in the
* CUTPNT and CUTPNT + 1 th elements and zeros elsewhere.
*
* The eigenvectors of the original matrix are stored in Q, and the
* eigenvalues are in D. The algorithm consists of three stages:
*
* The first stage consists of deflating the size of the problem
* when there are multiple eigenvalues or if there is a zero in
* the Z vector. For each such occurence the dimension of the
* secular equation problem is reduced by one. This stage is
* performed by the routine SLAED2.
*
* The second stage consists of calculating the updated
* eigenvalues. This is done by finding the roots of the secular
* equation via the routine SLAED4 (as called by SLAED3).
* This routine also calculates the eigenvectors of the current
* problem.
*
* The final stage consists of computing the updated eigenvectors
* directly using the updated eigenvalues. The eigenvectors for
* the current problem are multiplied with the eigenvectors from
* the overall problem.
*
* Arguments
* =========
*
* N (input) INTEGER
* The dimension of the symmetric tridiagonal matrix. N >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, the eigenvalues of the rank-1-perturbed matrix.
* On exit, the eigenvalues of the repaired matrix.
*
* Q (input/output) REAL array, dimension (LDQ,N)
* On entry, the eigenvectors of the rank-1-perturbed matrix.
* On exit, the eigenvectors of the repaired tridiagonal matrix.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N).
*
* INDXQ (input/output) INTEGER array, dimension (N)
* On entry, the permutation which separately sorts the two
* subproblems in D into ascending order.
* On exit, the permutation which will reintegrate the
* subproblems back into sorted order,
* i.e. D( INDXQ( I = 1, N ) ) will be in ascending order.
*
* RHO (input) REAL
* The subdiagonal entry used to create the rank-1 modification.
*
* CUTPNT (input) INTEGER
* The location of the last eigenvalue in the leading sub-matrix.
* min(1,N) <= CUTPNT <= N/2.
*
* WORK (workspace) REAL array, dimension (4*N + N**2)
*
* IWORK (workspace) INTEGER array, dimension (4*N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an eigenvalue did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
* Modified by Francoise Tisseur, University of Tennessee.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n
* @param d
* @param q
* @param ldq
* @param indxq
* @param rho
* @param cutpnt
* @param work
* @param iwork
* @param info
*
*/
abstract public void slaed1(int n, float[] d, float[] q, int ldq, int[] indxq, org.netlib.util.floatW rho, int cutpnt, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAED1 computes the updated eigensystem of a diagonal
* matrix after modification by a rank-one symmetric matrix. This
* routine is used only for the eigenproblem which requires all
* eigenvalues and eigenvectors of a tridiagonal matrix. SLAED7 handles
* the case in which eigenvalues only or eigenvalues and eigenvectors
* of a full symmetric matrix (which was reduced to tridiagonal form)
* are desired.
*
* T = Q(in) ( D(in) + RHO * Z*Z' ) Q'(in) = Q(out) * D(out) * Q'(out)
*
* where Z = Q'u, u is a vector of length N with ones in the
* CUTPNT and CUTPNT + 1 th elements and zeros elsewhere.
*
* The eigenvectors of the original matrix are stored in Q, and the
* eigenvalues are in D. The algorithm consists of three stages:
*
* The first stage consists of deflating the size of the problem
* when there are multiple eigenvalues or if there is a zero in
* the Z vector. For each such occurence the dimension of the
* secular equation problem is reduced by one. This stage is
* performed by the routine SLAED2.
*
* The second stage consists of calculating the updated
* eigenvalues. This is done by finding the roots of the secular
* equation via the routine SLAED4 (as called by SLAED3).
* This routine also calculates the eigenvectors of the current
* problem.
*
* The final stage consists of computing the updated eigenvectors
* directly using the updated eigenvalues. The eigenvectors for
* the current problem are multiplied with the eigenvectors from
* the overall problem.
*
* Arguments
* =========
*
* N (input) INTEGER
* The dimension of the symmetric tridiagonal matrix. N >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, the eigenvalues of the rank-1-perturbed matrix.
* On exit, the eigenvalues of the repaired matrix.
*
* Q (input/output) REAL array, dimension (LDQ,N)
* On entry, the eigenvectors of the rank-1-perturbed matrix.
* On exit, the eigenvectors of the repaired tridiagonal matrix.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N).
*
* INDXQ (input/output) INTEGER array, dimension (N)
* On entry, the permutation which separately sorts the two
* subproblems in D into ascending order.
* On exit, the permutation which will reintegrate the
* subproblems back into sorted order,
* i.e. D( INDXQ( I = 1, N ) ) will be in ascending order.
*
* RHO (input) REAL
* The subdiagonal entry used to create the rank-1 modification.
*
* CUTPNT (input) INTEGER
* The location of the last eigenvalue in the leading sub-matrix.
* min(1,N) <= CUTPNT <= N/2.
*
* WORK (workspace) REAL array, dimension (4*N + N**2)
*
* IWORK (workspace) INTEGER array, dimension (4*N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an eigenvalue did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
* Modified by Francoise Tisseur, University of Tennessee.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n
* @param d
* @param _d_offset
* @param q
* @param _q_offset
* @param ldq
* @param indxq
* @param _indxq_offset
* @param rho
* @param cutpnt
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void slaed1(int n, float[] d, int _d_offset, float[] q, int _q_offset, int ldq, int[] indxq, int _indxq_offset, org.netlib.util.floatW rho, int cutpnt, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAED2 merges the two sets of eigenvalues together into a single
* sorted set. Then it tries to deflate the size of the problem.
* There are two ways in which deflation can occur: when two or more
* eigenvalues are close together or if there is a tiny entry in the
* Z vector. For each such occurrence the order of the related secular
* equation problem is reduced by one.
*
* Arguments
* =========
*
* K (output) INTEGER
* The number of non-deflated eigenvalues, and the order of the
* related secular equation. 0 <= K <=N.
*
* N (input) INTEGER
* The dimension of the symmetric tridiagonal matrix. N >= 0.
*
* N1 (input) INTEGER
* The location of the last eigenvalue in the leading sub-matrix.
* min(1,N) <= N1 <= N/2.
*
* D (input/output) REAL array, dimension (N)
* On entry, D contains the eigenvalues of the two submatrices to
* be combined.
* On exit, D contains the trailing (N-K) updated eigenvalues
* (those which were deflated) sorted into increasing order.
*
* Q (input/output) REAL array, dimension (LDQ, N)
* On entry, Q contains the eigenvectors of two submatrices in
* the two square blocks with corners at (1,1), (N1,N1)
* and (N1+1, N1+1), (N,N).
* On exit, Q contains the trailing (N-K) updated eigenvectors
* (those which were deflated) in its last N-K columns.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N).
*
* INDXQ (input/output) INTEGER array, dimension (N)
* The permutation which separately sorts the two sub-problems
* in D into ascending order. Note that elements in the second
* half of this permutation must first have N1 added to their
* values. Destroyed on exit.
*
* RHO (input/output) REAL
* On entry, the off-diagonal element associated with the rank-1
* cut which originally split the two submatrices which are now
* being recombined.
* On exit, RHO has been modified to the value required by
* SLAED3.
*
* Z (input) REAL array, dimension (N)
* On entry, Z contains the updating vector (the last
* row of the first sub-eigenvector matrix and the first row of
* the second sub-eigenvector matrix).
* On exit, the contents of Z have been destroyed by the updating
* process.
*
* DLAMDA (output) REAL array, dimension (N)
* A copy of the first K eigenvalues which will be used by
* SLAED3 to form the secular equation.
*
* W (output) REAL array, dimension (N)
* The first k values of the final deflation-altered z-vector
* which will be passed to SLAED3.
*
* Q2 (output) REAL array, dimension (N1**2+(N-N1)**2)
* A copy of the first K eigenvectors which will be used by
* SLAED3 in a matrix multiply (SGEMM) to solve for the new
* eigenvectors.
*
* INDX (workspace) INTEGER array, dimension (N)
* The permutation used to sort the contents of DLAMDA into
* ascending order.
*
* INDXC (output) INTEGER array, dimension (N)
* The permutation used to arrange the columns of the deflated
* Q matrix into three groups: the first group contains non-zero
* elements only at and above N1, the second contains
* non-zero elements only below N1, and the third is dense.
*
* INDXP (workspace) INTEGER array, dimension (N)
* The permutation used to place deflated values of D at the end
* of the array. INDXP(1:K) points to the nondeflated D-values
* and INDXP(K+1:N) points to the deflated eigenvalues.
*
* COLTYP (workspace/output) INTEGER array, dimension (N)
* During execution, a label which will indicate which of the
* following types a column in the Q2 matrix is:
* 1 : non-zero in the upper half only;
* 2 : dense;
* 3 : non-zero in the lower half only;
* 4 : deflated.
* On exit, COLTYP(i) is the number of columns of type i,
* for i=1 to 4 only.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
* Modified by Francoise Tisseur, University of Tennessee.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param k
* @param n
* @param n1
* @param d
* @param q
* @param ldq
* @param indxq
* @param rho
* @param z
* @param dlamda
* @param w
* @param q2
* @param indx
* @param indxc
* @param indxp
* @param coltyp
* @param info
*
*/
abstract public void slaed2(org.netlib.util.intW k, int n, int n1, float[] d, float[] q, int ldq, int[] indxq, org.netlib.util.floatW rho, float[] z, float[] dlamda, float[] w, float[] q2, int[] indx, int[] indxc, int[] indxp, int[] coltyp, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAED2 merges the two sets of eigenvalues together into a single
* sorted set. Then it tries to deflate the size of the problem.
* There are two ways in which deflation can occur: when two or more
* eigenvalues are close together or if there is a tiny entry in the
* Z vector. For each such occurrence the order of the related secular
* equation problem is reduced by one.
*
* Arguments
* =========
*
* K (output) INTEGER
* The number of non-deflated eigenvalues, and the order of the
* related secular equation. 0 <= K <=N.
*
* N (input) INTEGER
* The dimension of the symmetric tridiagonal matrix. N >= 0.
*
* N1 (input) INTEGER
* The location of the last eigenvalue in the leading sub-matrix.
* min(1,N) <= N1 <= N/2.
*
* D (input/output) REAL array, dimension (N)
* On entry, D contains the eigenvalues of the two submatrices to
* be combined.
* On exit, D contains the trailing (N-K) updated eigenvalues
* (those which were deflated) sorted into increasing order.
*
* Q (input/output) REAL array, dimension (LDQ, N)
* On entry, Q contains the eigenvectors of two submatrices in
* the two square blocks with corners at (1,1), (N1,N1)
* and (N1+1, N1+1), (N,N).
* On exit, Q contains the trailing (N-K) updated eigenvectors
* (those which were deflated) in its last N-K columns.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N).
*
* INDXQ (input/output) INTEGER array, dimension (N)
* The permutation which separately sorts the two sub-problems
* in D into ascending order. Note that elements in the second
* half of this permutation must first have N1 added to their
* values. Destroyed on exit.
*
* RHO (input/output) REAL
* On entry, the off-diagonal element associated with the rank-1
* cut which originally split the two submatrices which are now
* being recombined.
* On exit, RHO has been modified to the value required by
* SLAED3.
*
* Z (input) REAL array, dimension (N)
* On entry, Z contains the updating vector (the last
* row of the first sub-eigenvector matrix and the first row of
* the second sub-eigenvector matrix).
* On exit, the contents of Z have been destroyed by the updating
* process.
*
* DLAMDA (output) REAL array, dimension (N)
* A copy of the first K eigenvalues which will be used by
* SLAED3 to form the secular equation.
*
* W (output) REAL array, dimension (N)
* The first k values of the final deflation-altered z-vector
* which will be passed to SLAED3.
*
* Q2 (output) REAL array, dimension (N1**2+(N-N1)**2)
* A copy of the first K eigenvectors which will be used by
* SLAED3 in a matrix multiply (SGEMM) to solve for the new
* eigenvectors.
*
* INDX (workspace) INTEGER array, dimension (N)
* The permutation used to sort the contents of DLAMDA into
* ascending order.
*
* INDXC (output) INTEGER array, dimension (N)
* The permutation used to arrange the columns of the deflated
* Q matrix into three groups: the first group contains non-zero
* elements only at and above N1, the second contains
* non-zero elements only below N1, and the third is dense.
*
* INDXP (workspace) INTEGER array, dimension (N)
* The permutation used to place deflated values of D at the end
* of the array. INDXP(1:K) points to the nondeflated D-values
* and INDXP(K+1:N) points to the deflated eigenvalues.
*
* COLTYP (workspace/output) INTEGER array, dimension (N)
* During execution, a label which will indicate which of the
* following types a column in the Q2 matrix is:
* 1 : non-zero in the upper half only;
* 2 : dense;
* 3 : non-zero in the lower half only;
* 4 : deflated.
* On exit, COLTYP(i) is the number of columns of type i,
* for i=1 to 4 only.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
* Modified by Francoise Tisseur, University of Tennessee.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param k
* @param n
* @param n1
* @param d
* @param _d_offset
* @param q
* @param _q_offset
* @param ldq
* @param indxq
* @param _indxq_offset
* @param rho
* @param z
* @param _z_offset
* @param dlamda
* @param _dlamda_offset
* @param w
* @param _w_offset
* @param q2
* @param _q2_offset
* @param indx
* @param _indx_offset
* @param indxc
* @param _indxc_offset
* @param indxp
* @param _indxp_offset
* @param coltyp
* @param _coltyp_offset
* @param info
*
*/
abstract public void slaed2(org.netlib.util.intW k, int n, int n1, float[] d, int _d_offset, float[] q, int _q_offset, int ldq, int[] indxq, int _indxq_offset, org.netlib.util.floatW rho, float[] z, int _z_offset, float[] dlamda, int _dlamda_offset, float[] w, int _w_offset, float[] q2, int _q2_offset, int[] indx, int _indx_offset, int[] indxc, int _indxc_offset, int[] indxp, int _indxp_offset, int[] coltyp, int _coltyp_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAED3 finds the roots of the secular equation, as defined by the
* values in D, W, and RHO, between 1 and K. It makes the
* appropriate calls to SLAED4 and then updates the eigenvectors by
* multiplying the matrix of eigenvectors of the pair of eigensystems
* being combined by the matrix of eigenvectors of the K-by-K system
* which is solved here.
*
* This code makes very mild assumptions about floating point
* arithmetic. It will work on machines with a guard digit in
* add/subtract, or on those binary machines without guard digits
* which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or Cray-2.
* It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* K (input) INTEGER
* The number of terms in the rational function to be solved by
* SLAED4. K >= 0.
*
* N (input) INTEGER
* The number of rows and columns in the Q matrix.
* N >= K (deflation may result in N>K).
*
* N1 (input) INTEGER
* The location of the last eigenvalue in the leading submatrix.
* min(1,N) <= N1 <= N/2.
*
* D (output) REAL array, dimension (N)
* D(I) contains the updated eigenvalues for
* 1 <= I <= K.
*
* Q (output) REAL array, dimension (LDQ,N)
* Initially the first K columns are used as workspace.
* On output the columns 1 to K contain
* the updated eigenvectors.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N).
*
* RHO (input) REAL
* The value of the parameter in the rank one update equation.
* RHO >= 0 required.
*
* DLAMDA (input/output) REAL array, dimension (K)
* The first K elements of this array contain the old roots
* of the deflated updating problem. These are the poles
* of the secular equation. May be changed on output by
* having lowest order bit set to zero on Cray X-MP, Cray Y-MP,
* Cray-2, or Cray C-90, as described above.
*
* Q2 (input) REAL array, dimension (LDQ2, N)
* The first K columns of this matrix contain the non-deflated
* eigenvectors for the split problem.
*
* INDX (input) INTEGER array, dimension (N)
* The permutation used to arrange the columns of the deflated
* Q matrix into three groups (see SLAED2).
* The rows of the eigenvectors found by SLAED4 must be likewise
* permuted before the matrix multiply can take place.
*
* CTOT (input) INTEGER array, dimension (4)
* A count of the total number of the various types of columns
* in Q, as described in INDX. The fourth column type is any
* column which has been deflated.
*
* W (input/output) REAL array, dimension (K)
* The first K elements of this array contain the components
* of the deflation-adjusted updating vector. Destroyed on
* output.
*
* S (workspace) REAL array, dimension (N1 + 1)*K
* Will contain the eigenvectors of the repaired matrix which
* will be multiplied by the previously accumulated eigenvectors
* to update the system.
*
* LDS (input) INTEGER
* The leading dimension of S. LDS >= max(1,K).
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an eigenvalue did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
* Modified by Francoise Tisseur, University of Tennessee.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param k
* @param n
* @param n1
* @param d
* @param q
* @param ldq
* @param rho
* @param dlamda
* @param q2
* @param indx
* @param ctot
* @param w
* @param s
* @param info
*
*/
abstract public void slaed3(int k, int n, int n1, float[] d, float[] q, int ldq, float rho, float[] dlamda, float[] q2, int[] indx, int[] ctot, float[] w, float[] s, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAED3 finds the roots of the secular equation, as defined by the
* values in D, W, and RHO, between 1 and K. It makes the
* appropriate calls to SLAED4 and then updates the eigenvectors by
* multiplying the matrix of eigenvectors of the pair of eigensystems
* being combined by the matrix of eigenvectors of the K-by-K system
* which is solved here.
*
* This code makes very mild assumptions about floating point
* arithmetic. It will work on machines with a guard digit in
* add/subtract, or on those binary machines without guard digits
* which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or Cray-2.
* It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* K (input) INTEGER
* The number of terms in the rational function to be solved by
* SLAED4. K >= 0.
*
* N (input) INTEGER
* The number of rows and columns in the Q matrix.
* N >= K (deflation may result in N>K).
*
* N1 (input) INTEGER
* The location of the last eigenvalue in the leading submatrix.
* min(1,N) <= N1 <= N/2.
*
* D (output) REAL array, dimension (N)
* D(I) contains the updated eigenvalues for
* 1 <= I <= K.
*
* Q (output) REAL array, dimension (LDQ,N)
* Initially the first K columns are used as workspace.
* On output the columns 1 to K contain
* the updated eigenvectors.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N).
*
* RHO (input) REAL
* The value of the parameter in the rank one update equation.
* RHO >= 0 required.
*
* DLAMDA (input/output) REAL array, dimension (K)
* The first K elements of this array contain the old roots
* of the deflated updating problem. These are the poles
* of the secular equation. May be changed on output by
* having lowest order bit set to zero on Cray X-MP, Cray Y-MP,
* Cray-2, or Cray C-90, as described above.
*
* Q2 (input) REAL array, dimension (LDQ2, N)
* The first K columns of this matrix contain the non-deflated
* eigenvectors for the split problem.
*
* INDX (input) INTEGER array, dimension (N)
* The permutation used to arrange the columns of the deflated
* Q matrix into three groups (see SLAED2).
* The rows of the eigenvectors found by SLAED4 must be likewise
* permuted before the matrix multiply can take place.
*
* CTOT (input) INTEGER array, dimension (4)
* A count of the total number of the various types of columns
* in Q, as described in INDX. The fourth column type is any
* column which has been deflated.
*
* W (input/output) REAL array, dimension (K)
* The first K elements of this array contain the components
* of the deflation-adjusted updating vector. Destroyed on
* output.
*
* S (workspace) REAL array, dimension (N1 + 1)*K
* Will contain the eigenvectors of the repaired matrix which
* will be multiplied by the previously accumulated eigenvectors
* to update the system.
*
* LDS (input) INTEGER
* The leading dimension of S. LDS >= max(1,K).
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an eigenvalue did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
* Modified by Francoise Tisseur, University of Tennessee.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param k
* @param n
* @param n1
* @param d
* @param _d_offset
* @param q
* @param _q_offset
* @param ldq
* @param rho
* @param dlamda
* @param _dlamda_offset
* @param q2
* @param _q2_offset
* @param indx
* @param _indx_offset
* @param ctot
* @param _ctot_offset
* @param w
* @param _w_offset
* @param s
* @param _s_offset
* @param info
*
*/
abstract public void slaed3(int k, int n, int n1, float[] d, int _d_offset, float[] q, int _q_offset, int ldq, float rho, float[] dlamda, int _dlamda_offset, float[] q2, int _q2_offset, int[] indx, int _indx_offset, int[] ctot, int _ctot_offset, float[] w, int _w_offset, float[] s, int _s_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* This subroutine computes the I-th updated eigenvalue of a symmetric
* rank-one modification to a diagonal matrix whose elements are
* given in the array d, and that
*
* D(i) < D(j) for i < j
*
* and that RHO > 0. This is arranged by the calling routine, and is
* no loss in generality. The rank-one modified system is thus
*
* diag( D ) + RHO * Z * Z_transpose.
*
* where we assume the Euclidean norm of Z is 1.
*
* The method consists of approximating the rational functions in the
* secular equation by simpler interpolating rational functions.
*
* Arguments
* =========
*
* N (input) INTEGER
* The length of all arrays.
*
* I (input) INTEGER
* The index of the eigenvalue to be computed. 1 <= I <= N.
*
* D (input) REAL array, dimension (N)
* The original eigenvalues. It is assumed that they are in
* order, D(I) < D(J) for I < J.
*
* Z (input) REAL array, dimension (N)
* The components of the updating vector.
*
* DELTA (output) REAL array, dimension (N)
* If N .GT. 2, DELTA contains (D(j) - lambda_I) in its j-th
* component. If N = 1, then DELTA(1) = 1. If N = 2, see SLAED5
* for detail. The vector DELTA contains the information necessar
* to construct the eigenvectors by SLAED3 and SLAED9.
*
* RHO (input) REAL
* The scalar in the symmetric updating formula.
*
* DLAM (output) REAL
* The computed lambda_I, the I-th updated eigenvalue.
*
* INFO (output) INTEGER
* = 0: successful exit
* > 0: if INFO = 1, the updating process failed.
*
* Internal Parameters
* ===================
*
* Logical variable ORGATI (origin-at-i?) is used for distinguishing
* whether D(i) or D(i+1) is treated as the origin.
*
* ORGATI = .true. origin at i
* ORGATI = .false. origin at i+1
*
* Logical variable SWTCH3 (switch-for-3-poles?) is for noting
* if we are working with THREE poles!
*
* MAXIT is the maximum number of iterations allowed for each
* eigenvalue.
*
* Further Details
* ===============
*
* Based on contributions by
* Ren-Cang Li, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param i
* @param d
* @param z
* @param delta
* @param rho
* @param dlam
* @param info
*
*/
abstract public void slaed4(int n, int i, float[] d, float[] z, float[] delta, float rho, org.netlib.util.floatW dlam, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* This subroutine computes the I-th updated eigenvalue of a symmetric
* rank-one modification to a diagonal matrix whose elements are
* given in the array d, and that
*
* D(i) < D(j) for i < j
*
* and that RHO > 0. This is arranged by the calling routine, and is
* no loss in generality. The rank-one modified system is thus
*
* diag( D ) + RHO * Z * Z_transpose.
*
* where we assume the Euclidean norm of Z is 1.
*
* The method consists of approximating the rational functions in the
* secular equation by simpler interpolating rational functions.
*
* Arguments
* =========
*
* N (input) INTEGER
* The length of all arrays.
*
* I (input) INTEGER
* The index of the eigenvalue to be computed. 1 <= I <= N.
*
* D (input) REAL array, dimension (N)
* The original eigenvalues. It is assumed that they are in
* order, D(I) < D(J) for I < J.
*
* Z (input) REAL array, dimension (N)
* The components of the updating vector.
*
* DELTA (output) REAL array, dimension (N)
* If N .GT. 2, DELTA contains (D(j) - lambda_I) in its j-th
* component. If N = 1, then DELTA(1) = 1. If N = 2, see SLAED5
* for detail. The vector DELTA contains the information necessar
* to construct the eigenvectors by SLAED3 and SLAED9.
*
* RHO (input) REAL
* The scalar in the symmetric updating formula.
*
* DLAM (output) REAL
* The computed lambda_I, the I-th updated eigenvalue.
*
* INFO (output) INTEGER
* = 0: successful exit
* > 0: if INFO = 1, the updating process failed.
*
* Internal Parameters
* ===================
*
* Logical variable ORGATI (origin-at-i?) is used for distinguishing
* whether D(i) or D(i+1) is treated as the origin.
*
* ORGATI = .true. origin at i
* ORGATI = .false. origin at i+1
*
* Logical variable SWTCH3 (switch-for-3-poles?) is for noting
* if we are working with THREE poles!
*
* MAXIT is the maximum number of iterations allowed for each
* eigenvalue.
*
* Further Details
* ===============
*
* Based on contributions by
* Ren-Cang Li, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param i
* @param d
* @param _d_offset
* @param z
* @param _z_offset
* @param delta
* @param _delta_offset
* @param rho
* @param dlam
* @param info
*
*/
abstract public void slaed4(int n, int i, float[] d, int _d_offset, float[] z, int _z_offset, float[] delta, int _delta_offset, float rho, org.netlib.util.floatW dlam, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* This subroutine computes the I-th eigenvalue of a symmetric rank-one
* modification of a 2-by-2 diagonal matrix
*
* diag( D ) + RHO * Z * transpose(Z) .
*
* The diagonal elements in the array D are assumed to satisfy
*
* D(i) < D(j) for i < j .
*
* We also assume RHO > 0 and that the Euclidean norm of the vector
* Z is one.
*
* Arguments
* =========
*
* I (input) INTEGER
* The index of the eigenvalue to be computed. I = 1 or I = 2.
*
* D (input) REAL array, dimension (2)
* The original eigenvalues. We assume D(1) < D(2).
*
* Z (input) REAL array, dimension (2)
* The components of the updating vector.
*
* DELTA (output) REAL array, dimension (2)
* The vector DELTA contains the information necessary
* to construct the eigenvectors.
*
* RHO (input) REAL
* The scalar in the symmetric updating formula.
*
* DLAM (output) REAL
* The computed lambda_I, the I-th updated eigenvalue.
*
* Further Details
* ===============
*
* Based on contributions by
* Ren-Cang Li, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param i
* @param d
* @param z
* @param delta
* @param rho
* @param dlam
*
*/
abstract public void slaed5(int i, float[] d, float[] z, float[] delta, float rho, org.netlib.util.floatW dlam);
/**
*
* ..
*
* Purpose
* =======
*
* This subroutine computes the I-th eigenvalue of a symmetric rank-one
* modification of a 2-by-2 diagonal matrix
*
* diag( D ) + RHO * Z * transpose(Z) .
*
* The diagonal elements in the array D are assumed to satisfy
*
* D(i) < D(j) for i < j .
*
* We also assume RHO > 0 and that the Euclidean norm of the vector
* Z is one.
*
* Arguments
* =========
*
* I (input) INTEGER
* The index of the eigenvalue to be computed. I = 1 or I = 2.
*
* D (input) REAL array, dimension (2)
* The original eigenvalues. We assume D(1) < D(2).
*
* Z (input) REAL array, dimension (2)
* The components of the updating vector.
*
* DELTA (output) REAL array, dimension (2)
* The vector DELTA contains the information necessary
* to construct the eigenvectors.
*
* RHO (input) REAL
* The scalar in the symmetric updating formula.
*
* DLAM (output) REAL
* The computed lambda_I, the I-th updated eigenvalue.
*
* Further Details
* ===============
*
* Based on contributions by
* Ren-Cang Li, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param i
* @param d
* @param _d_offset
* @param z
* @param _z_offset
* @param delta
* @param _delta_offset
* @param rho
* @param dlam
*
*/
abstract public void slaed5(int i, float[] d, int _d_offset, float[] z, int _z_offset, float[] delta, int _delta_offset, float rho, org.netlib.util.floatW dlam);
/**
*
* ..
*
* Purpose
* =======
*
* SLAED6 computes the positive or negative root (closest to the origin)
* of
* z(1) z(2) z(3)
* f(x) = rho + --------- + ---------- + ---------
* d(1)-x d(2)-x d(3)-x
*
* It is assumed that
*
* if ORGATI = .true. the root is between d(2) and d(3);
* otherwise it is between d(1) and d(2)
*
* This routine will be called by SLAED4 when necessary. In most cases,
* the root sought is the smallest in magnitude, though it might not be
* in some extremely rare situations.
*
* Arguments
* =========
*
* KNITER (input) INTEGER
* Refer to SLAED4 for its significance.
*
* ORGATI (input) LOGICAL
* If ORGATI is true, the needed root is between d(2) and
* d(3); otherwise it is between d(1) and d(2). See
* SLAED4 for further details.
*
* RHO (input) REAL
* Refer to the equation f(x) above.
*
* D (input) REAL array, dimension (3)
* D satisfies d(1) < d(2) < d(3).
*
* Z (input) REAL array, dimension (3)
* Each of the elements in z must be positive.
*
* FINIT (input) REAL
* The value of f at 0. It is more accurate than the one
* evaluated inside this routine (if someone wants to do
* so).
*
* TAU (output) REAL
* The root of the equation f(x).
*
* INFO (output) INTEGER
* = 0: successful exit
* > 0: if INFO = 1, failure to converge
*
* Further Details
* ===============
*
* 30/06/99: Based on contributions by
* Ren-Cang Li, Computer Science Division, University of California
* at Berkeley, USA
*
* 10/02/03: This version has a few statements commented out for thread
* (machine parameters are computed on each entry). SJH.
*
* 05/10/06: Modified from a new version of Ren-Cang Li, use
* Gragg-Thornton-Warner cubic convergent scheme for better stability
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param kniter
* @param orgati
* @param rho
* @param d
* @param z
* @param finit
* @param tau
* @param info
*
*/
abstract public void slaed6(int kniter, boolean orgati, float rho, float[] d, float[] z, float finit, org.netlib.util.floatW tau, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAED6 computes the positive or negative root (closest to the origin)
* of
* z(1) z(2) z(3)
* f(x) = rho + --------- + ---------- + ---------
* d(1)-x d(2)-x d(3)-x
*
* It is assumed that
*
* if ORGATI = .true. the root is between d(2) and d(3);
* otherwise it is between d(1) and d(2)
*
* This routine will be called by SLAED4 when necessary. In most cases,
* the root sought is the smallest in magnitude, though it might not be
* in some extremely rare situations.
*
* Arguments
* =========
*
* KNITER (input) INTEGER
* Refer to SLAED4 for its significance.
*
* ORGATI (input) LOGICAL
* If ORGATI is true, the needed root is between d(2) and
* d(3); otherwise it is between d(1) and d(2). See
* SLAED4 for further details.
*
* RHO (input) REAL
* Refer to the equation f(x) above.
*
* D (input) REAL array, dimension (3)
* D satisfies d(1) < d(2) < d(3).
*
* Z (input) REAL array, dimension (3)
* Each of the elements in z must be positive.
*
* FINIT (input) REAL
* The value of f at 0. It is more accurate than the one
* evaluated inside this routine (if someone wants to do
* so).
*
* TAU (output) REAL
* The root of the equation f(x).
*
* INFO (output) INTEGER
* = 0: successful exit
* > 0: if INFO = 1, failure to converge
*
* Further Details
* ===============
*
* 30/06/99: Based on contributions by
* Ren-Cang Li, Computer Science Division, University of California
* at Berkeley, USA
*
* 10/02/03: This version has a few statements commented out for thread
* (machine parameters are computed on each entry). SJH.
*
* 05/10/06: Modified from a new version of Ren-Cang Li, use
* Gragg-Thornton-Warner cubic convergent scheme for better stability
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param kniter
* @param orgati
* @param rho
* @param d
* @param _d_offset
* @param z
* @param _z_offset
* @param finit
* @param tau
* @param info
*
*/
abstract public void slaed6(int kniter, boolean orgati, float rho, float[] d, int _d_offset, float[] z, int _z_offset, float finit, org.netlib.util.floatW tau, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAED7 computes the updated eigensystem of a diagonal
* matrix after modification by a rank-one symmetric matrix. This
* routine is used only for the eigenproblem which requires all
* eigenvalues and optionally eigenvectors of a dense symmetric matrix
* that has been reduced to tridiagonal form. SLAED1 handles
* the case in which all eigenvalues and eigenvectors of a symmetric
* tridiagonal matrix are desired.
*
* T = Q(in) ( D(in) + RHO * Z*Z' ) Q'(in) = Q(out) * D(out) * Q'(out)
*
* where Z = Q'u, u is a vector of length N with ones in the
* CUTPNT and CUTPNT + 1 th elements and zeros elsewhere.
*
* The eigenvectors of the original matrix are stored in Q, and the
* eigenvalues are in D. The algorithm consists of three stages:
*
* The first stage consists of deflating the size of the problem
* when there are multiple eigenvalues or if there is a zero in
* the Z vector. For each such occurence the dimension of the
* secular equation problem is reduced by one. This stage is
* performed by the routine SLAED8.
*
* The second stage consists of calculating the updated
* eigenvalues. This is done by finding the roots of the secular
* equation via the routine SLAED4 (as called by SLAED9).
* This routine also calculates the eigenvectors of the current
* problem.
*
* The final stage consists of computing the updated eigenvectors
* directly using the updated eigenvalues. The eigenvectors for
* the current problem are multiplied with the eigenvectors from
* the overall problem.
*
* Arguments
* =========
*
* ICOMPQ (input) INTEGER
* = 0: Compute eigenvalues only.
* = 1: Compute eigenvectors of original dense symmetric matrix
* also. On entry, Q contains the orthogonal matrix used
* to reduce the original matrix to tridiagonal form.
*
* N (input) INTEGER
* The dimension of the symmetric tridiagonal matrix. N >= 0.
*
* QSIZ (input) INTEGER
* The dimension of the orthogonal matrix used to reduce
* the full matrix to tridiagonal form. QSIZ >= N if ICOMPQ = 1.
*
* TLVLS (input) INTEGER
* The total number of merging levels in the overall divide and
* conquer tree.
*
* CURLVL (input) INTEGER
* The current level in the overall merge routine,
* 0 <= CURLVL <= TLVLS.
*
* CURPBM (input) INTEGER
* The current problem in the current level in the overall
* merge routine (counting from upper left to lower right).
*
* D (input/output) REAL array, dimension (N)
* On entry, the eigenvalues of the rank-1-perturbed matrix.
* On exit, the eigenvalues of the repaired matrix.
*
* Q (input/output) REAL array, dimension (LDQ, N)
* On entry, the eigenvectors of the rank-1-perturbed matrix.
* On exit, the eigenvectors of the repaired tridiagonal matrix.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N).
*
* INDXQ (output) INTEGER array, dimension (N)
* The permutation which will reintegrate the subproblem just
* solved back into sorted order, i.e., D( INDXQ( I = 1, N ) )
* will be in ascending order.
*
* RHO (input) REAL
* The subdiagonal element used to create the rank-1
* modification.
*
* CUTPNT (input) INTEGER
* Contains the location of the last eigenvalue in the leading
* sub-matrix. min(1,N) <= CUTPNT <= N.
*
* QSTORE (input/output) REAL array, dimension (N**2+1)
* Stores eigenvectors of submatrices encountered during
* divide and conquer, packed together. QPTR points to
* beginning of the submatrices.
*
* QPTR (input/output) INTEGER array, dimension (N+2)
* List of indices pointing to beginning of submatrices stored
* in QSTORE. The submatrices are numbered starting at the
* bottom left of the divide and conquer tree, from left to
* right and bottom to top.
*
* PRMPTR (input) INTEGER array, dimension (N lg N)
* Contains a list of pointers which indicate where in PERM a
* level's permutation is stored. PRMPTR(i+1) - PRMPTR(i)
* indicates the size of the permutation and also the size of
* the full, non-deflated problem.
*
* PERM (input) INTEGER array, dimension (N lg N)
* Contains the permutations (from deflation and sorting) to be
* applied to each eigenblock.
*
* GIVPTR (input) INTEGER array, dimension (N lg N)
* Contains a list of pointers which indicate where in GIVCOL a
* level's Givens rotations are stored. GIVPTR(i+1) - GIVPTR(i)
* indicates the number of Givens rotations.
*
* GIVCOL (input) INTEGER array, dimension (2, N lg N)
* Each pair of numbers indicates a pair of columns to take place
* in a Givens rotation.
*
* GIVNUM (input) REAL array, dimension (2, N lg N)
* Each number indicates the S value to be used in the
* corresponding Givens rotation.
*
* WORK (workspace) REAL array, dimension (3*N+QSIZ*N)
*
* IWORK (workspace) INTEGER array, dimension (4*N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an eigenvalue did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param n
* @param qsiz
* @param tlvls
* @param curlvl
* @param curpbm
* @param d
* @param q
* @param ldq
* @param indxq
* @param rho
* @param cutpnt
* @param qstore
* @param qptr
* @param prmptr
* @param perm
* @param givptr
* @param givcol
* @param givnum
* @param work
* @param iwork
* @param info
*
*/
abstract public void slaed7(int icompq, int n, int qsiz, int tlvls, int curlvl, int curpbm, float[] d, float[] q, int ldq, int[] indxq, org.netlib.util.floatW rho, int cutpnt, float[] qstore, int[] qptr, int[] prmptr, int[] perm, int[] givptr, int[] givcol, float[] givnum, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAED7 computes the updated eigensystem of a diagonal
* matrix after modification by a rank-one symmetric matrix. This
* routine is used only for the eigenproblem which requires all
* eigenvalues and optionally eigenvectors of a dense symmetric matrix
* that has been reduced to tridiagonal form. SLAED1 handles
* the case in which all eigenvalues and eigenvectors of a symmetric
* tridiagonal matrix are desired.
*
* T = Q(in) ( D(in) + RHO * Z*Z' ) Q'(in) = Q(out) * D(out) * Q'(out)
*
* where Z = Q'u, u is a vector of length N with ones in the
* CUTPNT and CUTPNT + 1 th elements and zeros elsewhere.
*
* The eigenvectors of the original matrix are stored in Q, and the
* eigenvalues are in D. The algorithm consists of three stages:
*
* The first stage consists of deflating the size of the problem
* when there are multiple eigenvalues or if there is a zero in
* the Z vector. For each such occurence the dimension of the
* secular equation problem is reduced by one. This stage is
* performed by the routine SLAED8.
*
* The second stage consists of calculating the updated
* eigenvalues. This is done by finding the roots of the secular
* equation via the routine SLAED4 (as called by SLAED9).
* This routine also calculates the eigenvectors of the current
* problem.
*
* The final stage consists of computing the updated eigenvectors
* directly using the updated eigenvalues. The eigenvectors for
* the current problem are multiplied with the eigenvectors from
* the overall problem.
*
* Arguments
* =========
*
* ICOMPQ (input) INTEGER
* = 0: Compute eigenvalues only.
* = 1: Compute eigenvectors of original dense symmetric matrix
* also. On entry, Q contains the orthogonal matrix used
* to reduce the original matrix to tridiagonal form.
*
* N (input) INTEGER
* The dimension of the symmetric tridiagonal matrix. N >= 0.
*
* QSIZ (input) INTEGER
* The dimension of the orthogonal matrix used to reduce
* the full matrix to tridiagonal form. QSIZ >= N if ICOMPQ = 1.
*
* TLVLS (input) INTEGER
* The total number of merging levels in the overall divide and
* conquer tree.
*
* CURLVL (input) INTEGER
* The current level in the overall merge routine,
* 0 <= CURLVL <= TLVLS.
*
* CURPBM (input) INTEGER
* The current problem in the current level in the overall
* merge routine (counting from upper left to lower right).
*
* D (input/output) REAL array, dimension (N)
* On entry, the eigenvalues of the rank-1-perturbed matrix.
* On exit, the eigenvalues of the repaired matrix.
*
* Q (input/output) REAL array, dimension (LDQ, N)
* On entry, the eigenvectors of the rank-1-perturbed matrix.
* On exit, the eigenvectors of the repaired tridiagonal matrix.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N).
*
* INDXQ (output) INTEGER array, dimension (N)
* The permutation which will reintegrate the subproblem just
* solved back into sorted order, i.e., D( INDXQ( I = 1, N ) )
* will be in ascending order.
*
* RHO (input) REAL
* The subdiagonal element used to create the rank-1
* modification.
*
* CUTPNT (input) INTEGER
* Contains the location of the last eigenvalue in the leading
* sub-matrix. min(1,N) <= CUTPNT <= N.
*
* QSTORE (input/output) REAL array, dimension (N**2+1)
* Stores eigenvectors of submatrices encountered during
* divide and conquer, packed together. QPTR points to
* beginning of the submatrices.
*
* QPTR (input/output) INTEGER array, dimension (N+2)
* List of indices pointing to beginning of submatrices stored
* in QSTORE. The submatrices are numbered starting at the
* bottom left of the divide and conquer tree, from left to
* right and bottom to top.
*
* PRMPTR (input) INTEGER array, dimension (N lg N)
* Contains a list of pointers which indicate where in PERM a
* level's permutation is stored. PRMPTR(i+1) - PRMPTR(i)
* indicates the size of the permutation and also the size of
* the full, non-deflated problem.
*
* PERM (input) INTEGER array, dimension (N lg N)
* Contains the permutations (from deflation and sorting) to be
* applied to each eigenblock.
*
* GIVPTR (input) INTEGER array, dimension (N lg N)
* Contains a list of pointers which indicate where in GIVCOL a
* level's Givens rotations are stored. GIVPTR(i+1) - GIVPTR(i)
* indicates the number of Givens rotations.
*
* GIVCOL (input) INTEGER array, dimension (2, N lg N)
* Each pair of numbers indicates a pair of columns to take place
* in a Givens rotation.
*
* GIVNUM (input) REAL array, dimension (2, N lg N)
* Each number indicates the S value to be used in the
* corresponding Givens rotation.
*
* WORK (workspace) REAL array, dimension (3*N+QSIZ*N)
*
* IWORK (workspace) INTEGER array, dimension (4*N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an eigenvalue did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param n
* @param qsiz
* @param tlvls
* @param curlvl
* @param curpbm
* @param d
* @param _d_offset
* @param q
* @param _q_offset
* @param ldq
* @param indxq
* @param _indxq_offset
* @param rho
* @param cutpnt
* @param qstore
* @param _qstore_offset
* @param qptr
* @param _qptr_offset
* @param prmptr
* @param _prmptr_offset
* @param perm
* @param _perm_offset
* @param givptr
* @param _givptr_offset
* @param givcol
* @param _givcol_offset
* @param givnum
* @param _givnum_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void slaed7(int icompq, int n, int qsiz, int tlvls, int curlvl, int curpbm, float[] d, int _d_offset, float[] q, int _q_offset, int ldq, int[] indxq, int _indxq_offset, org.netlib.util.floatW rho, int cutpnt, float[] qstore, int _qstore_offset, int[] qptr, int _qptr_offset, int[] prmptr, int _prmptr_offset, int[] perm, int _perm_offset, int[] givptr, int _givptr_offset, int[] givcol, int _givcol_offset, float[] givnum, int _givnum_offset, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAED8 merges the two sets of eigenvalues together into a single
* sorted set. Then it tries to deflate the size of the problem.
* There are two ways in which deflation can occur: when two or more
* eigenvalues are close together or if there is a tiny element in the
* Z vector. For each such occurrence the order of the related secular
* equation problem is reduced by one.
*
* Arguments
* =========
*
* ICOMPQ (input) INTEGER
* = 0: Compute eigenvalues only.
* = 1: Compute eigenvectors of original dense symmetric matrix
* also. On entry, Q contains the orthogonal matrix used
* to reduce the original matrix to tridiagonal form.
*
* K (output) INTEGER
* The number of non-deflated eigenvalues, and the order of the
* related secular equation.
*
* N (input) INTEGER
* The dimension of the symmetric tridiagonal matrix. N >= 0.
*
* QSIZ (input) INTEGER
* The dimension of the orthogonal matrix used to reduce
* the full matrix to tridiagonal form. QSIZ >= N if ICOMPQ = 1.
*
* D (input/output) REAL array, dimension (N)
* On entry, the eigenvalues of the two submatrices to be
* combined. On exit, the trailing (N-K) updated eigenvalues
* (those which were deflated) sorted into increasing order.
*
* Q (input/output) REAL array, dimension (LDQ,N)
* If ICOMPQ = 0, Q is not referenced. Otherwise,
* on entry, Q contains the eigenvectors of the partially solved
* system which has been previously updated in matrix
* multiplies with other partially solved eigensystems.
* On exit, Q contains the trailing (N-K) updated eigenvectors
* (those which were deflated) in its last N-K columns.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N).
*
* INDXQ (input) INTEGER array, dimension (N)
* The permutation which separately sorts the two sub-problems
* in D into ascending order. Note that elements in the second
* half of this permutation must first have CUTPNT added to
* their values in order to be accurate.
*
* RHO (input/output) REAL
* On entry, the off-diagonal element associated with the rank-1
* cut which originally split the two submatrices which are now
* being recombined.
* On exit, RHO has been modified to the value required by
* SLAED3.
*
* CUTPNT (input) INTEGER
* The location of the last eigenvalue in the leading
* sub-matrix. min(1,N) <= CUTPNT <= N.
*
* Z (input) REAL array, dimension (N)
* On entry, Z contains the updating vector (the last row of
* the first sub-eigenvector matrix and the first row of the
* second sub-eigenvector matrix).
* On exit, the contents of Z are destroyed by the updating
* process.
*
* DLAMDA (output) REAL array, dimension (N)
* A copy of the first K eigenvalues which will be used by
* SLAED3 to form the secular equation.
*
* Q2 (output) REAL array, dimension (LDQ2,N)
* If ICOMPQ = 0, Q2 is not referenced. Otherwise,
* a copy of the first K eigenvectors which will be used by
* SLAED7 in a matrix multiply (SGEMM) to update the new
* eigenvectors.
*
* LDQ2 (input) INTEGER
* The leading dimension of the array Q2. LDQ2 >= max(1,N).
*
* W (output) REAL array, dimension (N)
* The first k values of the final deflation-altered z-vector and
* will be passed to SLAED3.
*
* PERM (output) INTEGER array, dimension (N)
* The permutations (from deflation and sorting) to be applied
* to each eigenblock.
*
* GIVPTR (output) INTEGER
* The number of Givens rotations which took place in this
* subproblem.
*
* GIVCOL (output) INTEGER array, dimension (2, N)
* Each pair of numbers indicates a pair of columns to take place
* in a Givens rotation.
*
* GIVNUM (output) REAL array, dimension (2, N)
* Each number indicates the S value to be used in the
* corresponding Givens rotation.
*
* INDXP (workspace) INTEGER array, dimension (N)
* The permutation used to place deflated values of D at the end
* of the array. INDXP(1:K) points to the nondeflated D-values
* and INDXP(K+1:N) points to the deflated eigenvalues.
*
* INDX (workspace) INTEGER array, dimension (N)
* The permutation used to sort the contents of D into ascending
* order.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param k
* @param n
* @param qsiz
* @param d
* @param q
* @param ldq
* @param indxq
* @param rho
* @param cutpnt
* @param z
* @param dlamda
* @param q2
* @param ldq2
* @param w
* @param perm
* @param givptr
* @param givcol
* @param givnum
* @param indxp
* @param indx
* @param info
*
*/
abstract public void slaed8(int icompq, org.netlib.util.intW k, int n, int qsiz, float[] d, float[] q, int ldq, int[] indxq, org.netlib.util.floatW rho, int cutpnt, float[] z, float[] dlamda, float[] q2, int ldq2, float[] w, int[] perm, org.netlib.util.intW givptr, int[] givcol, float[] givnum, int[] indxp, int[] indx, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAED8 merges the two sets of eigenvalues together into a single
* sorted set. Then it tries to deflate the size of the problem.
* There are two ways in which deflation can occur: when two or more
* eigenvalues are close together or if there is a tiny element in the
* Z vector. For each such occurrence the order of the related secular
* equation problem is reduced by one.
*
* Arguments
* =========
*
* ICOMPQ (input) INTEGER
* = 0: Compute eigenvalues only.
* = 1: Compute eigenvectors of original dense symmetric matrix
* also. On entry, Q contains the orthogonal matrix used
* to reduce the original matrix to tridiagonal form.
*
* K (output) INTEGER
* The number of non-deflated eigenvalues, and the order of the
* related secular equation.
*
* N (input) INTEGER
* The dimension of the symmetric tridiagonal matrix. N >= 0.
*
* QSIZ (input) INTEGER
* The dimension of the orthogonal matrix used to reduce
* the full matrix to tridiagonal form. QSIZ >= N if ICOMPQ = 1.
*
* D (input/output) REAL array, dimension (N)
* On entry, the eigenvalues of the two submatrices to be
* combined. On exit, the trailing (N-K) updated eigenvalues
* (those which were deflated) sorted into increasing order.
*
* Q (input/output) REAL array, dimension (LDQ,N)
* If ICOMPQ = 0, Q is not referenced. Otherwise,
* on entry, Q contains the eigenvectors of the partially solved
* system which has been previously updated in matrix
* multiplies with other partially solved eigensystems.
* On exit, Q contains the trailing (N-K) updated eigenvectors
* (those which were deflated) in its last N-K columns.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N).
*
* INDXQ (input) INTEGER array, dimension (N)
* The permutation which separately sorts the two sub-problems
* in D into ascending order. Note that elements in the second
* half of this permutation must first have CUTPNT added to
* their values in order to be accurate.
*
* RHO (input/output) REAL
* On entry, the off-diagonal element associated with the rank-1
* cut which originally split the two submatrices which are now
* being recombined.
* On exit, RHO has been modified to the value required by
* SLAED3.
*
* CUTPNT (input) INTEGER
* The location of the last eigenvalue in the leading
* sub-matrix. min(1,N) <= CUTPNT <= N.
*
* Z (input) REAL array, dimension (N)
* On entry, Z contains the updating vector (the last row of
* the first sub-eigenvector matrix and the first row of the
* second sub-eigenvector matrix).
* On exit, the contents of Z are destroyed by the updating
* process.
*
* DLAMDA (output) REAL array, dimension (N)
* A copy of the first K eigenvalues which will be used by
* SLAED3 to form the secular equation.
*
* Q2 (output) REAL array, dimension (LDQ2,N)
* If ICOMPQ = 0, Q2 is not referenced. Otherwise,
* a copy of the first K eigenvectors which will be used by
* SLAED7 in a matrix multiply (SGEMM) to update the new
* eigenvectors.
*
* LDQ2 (input) INTEGER
* The leading dimension of the array Q2. LDQ2 >= max(1,N).
*
* W (output) REAL array, dimension (N)
* The first k values of the final deflation-altered z-vector and
* will be passed to SLAED3.
*
* PERM (output) INTEGER array, dimension (N)
* The permutations (from deflation and sorting) to be applied
* to each eigenblock.
*
* GIVPTR (output) INTEGER
* The number of Givens rotations which took place in this
* subproblem.
*
* GIVCOL (output) INTEGER array, dimension (2, N)
* Each pair of numbers indicates a pair of columns to take place
* in a Givens rotation.
*
* GIVNUM (output) REAL array, dimension (2, N)
* Each number indicates the S value to be used in the
* corresponding Givens rotation.
*
* INDXP (workspace) INTEGER array, dimension (N)
* The permutation used to place deflated values of D at the end
* of the array. INDXP(1:K) points to the nondeflated D-values
* and INDXP(K+1:N) points to the deflated eigenvalues.
*
* INDX (workspace) INTEGER array, dimension (N)
* The permutation used to sort the contents of D into ascending
* order.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param k
* @param n
* @param qsiz
* @param d
* @param _d_offset
* @param q
* @param _q_offset
* @param ldq
* @param indxq
* @param _indxq_offset
* @param rho
* @param cutpnt
* @param z
* @param _z_offset
* @param dlamda
* @param _dlamda_offset
* @param q2
* @param _q2_offset
* @param ldq2
* @param w
* @param _w_offset
* @param perm
* @param _perm_offset
* @param givptr
* @param givcol
* @param _givcol_offset
* @param givnum
* @param _givnum_offset
* @param indxp
* @param _indxp_offset
* @param indx
* @param _indx_offset
* @param info
*
*/
abstract public void slaed8(int icompq, org.netlib.util.intW k, int n, int qsiz, float[] d, int _d_offset, float[] q, int _q_offset, int ldq, int[] indxq, int _indxq_offset, org.netlib.util.floatW rho, int cutpnt, float[] z, int _z_offset, float[] dlamda, int _dlamda_offset, float[] q2, int _q2_offset, int ldq2, float[] w, int _w_offset, int[] perm, int _perm_offset, org.netlib.util.intW givptr, int[] givcol, int _givcol_offset, float[] givnum, int _givnum_offset, int[] indxp, int _indxp_offset, int[] indx, int _indx_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAED9 finds the roots of the secular equation, as defined by the
* values in D, Z, and RHO, between KSTART and KSTOP. It makes the
* appropriate calls to SLAED4 and then stores the new matrix of
* eigenvectors for use in calculating the next level of Z vectors.
*
* Arguments
* =========
*
* K (input) INTEGER
* The number of terms in the rational function to be solved by
* SLAED4. K >= 0.
*
* KSTART (input) INTEGER
* KSTOP (input) INTEGER
* The updated eigenvalues Lambda(I), KSTART <= I <= KSTOP
* are to be computed. 1 <= KSTART <= KSTOP <= K.
*
* N (input) INTEGER
* The number of rows and columns in the Q matrix.
* N >= K (delation may result in N > K).
*
* D (output) REAL array, dimension (N)
* D(I) contains the updated eigenvalues
* for KSTART <= I <= KSTOP.
*
* Q (workspace) REAL array, dimension (LDQ,N)
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max( 1, N ).
*
* RHO (input) REAL
* The value of the parameter in the rank one update equation.
* RHO >= 0 required.
*
* DLAMDA (input) REAL array, dimension (K)
* The first K elements of this array contain the old roots
* of the deflated updating problem. These are the poles
* of the secular equation.
*
* W (input) REAL array, dimension (K)
* The first K elements of this array contain the components
* of the deflation-adjusted updating vector.
*
* S (output) REAL array, dimension (LDS, K)
* Will contain the eigenvectors of the repaired matrix which
* will be stored for subsequent Z vector calculation and
* multiplied by the previously accumulated eigenvectors
* to update the system.
*
* LDS (input) INTEGER
* The leading dimension of S. LDS >= max( 1, K ).
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an eigenvalue did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param k
* @param kstart
* @param kstop
* @param n
* @param d
* @param q
* @param ldq
* @param rho
* @param dlamda
* @param w
* @param s
* @param lds
* @param info
*
*/
abstract public void slaed9(int k, int kstart, int kstop, int n, float[] d, float[] q, int ldq, float rho, float[] dlamda, float[] w, float[] s, int lds, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAED9 finds the roots of the secular equation, as defined by the
* values in D, Z, and RHO, between KSTART and KSTOP. It makes the
* appropriate calls to SLAED4 and then stores the new matrix of
* eigenvectors for use in calculating the next level of Z vectors.
*
* Arguments
* =========
*
* K (input) INTEGER
* The number of terms in the rational function to be solved by
* SLAED4. K >= 0.
*
* KSTART (input) INTEGER
* KSTOP (input) INTEGER
* The updated eigenvalues Lambda(I), KSTART <= I <= KSTOP
* are to be computed. 1 <= KSTART <= KSTOP <= K.
*
* N (input) INTEGER
* The number of rows and columns in the Q matrix.
* N >= K (delation may result in N > K).
*
* D (output) REAL array, dimension (N)
* D(I) contains the updated eigenvalues
* for KSTART <= I <= KSTOP.
*
* Q (workspace) REAL array, dimension (LDQ,N)
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max( 1, N ).
*
* RHO (input) REAL
* The value of the parameter in the rank one update equation.
* RHO >= 0 required.
*
* DLAMDA (input) REAL array, dimension (K)
* The first K elements of this array contain the old roots
* of the deflated updating problem. These are the poles
* of the secular equation.
*
* W (input) REAL array, dimension (K)
* The first K elements of this array contain the components
* of the deflation-adjusted updating vector.
*
* S (output) REAL array, dimension (LDS, K)
* Will contain the eigenvectors of the repaired matrix which
* will be stored for subsequent Z vector calculation and
* multiplied by the previously accumulated eigenvectors
* to update the system.
*
* LDS (input) INTEGER
* The leading dimension of S. LDS >= max( 1, K ).
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an eigenvalue did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param k
* @param kstart
* @param kstop
* @param n
* @param d
* @param _d_offset
* @param q
* @param _q_offset
* @param ldq
* @param rho
* @param dlamda
* @param _dlamda_offset
* @param w
* @param _w_offset
* @param s
* @param _s_offset
* @param lds
* @param info
*
*/
abstract public void slaed9(int k, int kstart, int kstop, int n, float[] d, int _d_offset, float[] q, int _q_offset, int ldq, float rho, float[] dlamda, int _dlamda_offset, float[] w, int _w_offset, float[] s, int _s_offset, int lds, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAEDA computes the Z vector corresponding to the merge step in the
* CURLVLth step of the merge process with TLVLS steps for the CURPBMth
* problem.
*
* Arguments
* =========
*
* N (input) INTEGER
* The dimension of the symmetric tridiagonal matrix. N >= 0.
*
* TLVLS (input) INTEGER
* The total number of merging levels in the overall divide and
* conquer tree.
*
* CURLVL (input) INTEGER
* The current level in the overall merge routine,
* 0 <= curlvl <= tlvls.
*
* CURPBM (input) INTEGER
* The current problem in the current level in the overall
* merge routine (counting from upper left to lower right).
*
* PRMPTR (input) INTEGER array, dimension (N lg N)
* Contains a list of pointers which indicate where in PERM a
* level's permutation is stored. PRMPTR(i+1) - PRMPTR(i)
* indicates the size of the permutation and incidentally the
* size of the full, non-deflated problem.
*
* PERM (input) INTEGER array, dimension (N lg N)
* Contains the permutations (from deflation and sorting) to be
* applied to each eigenblock.
*
* GIVPTR (input) INTEGER array, dimension (N lg N)
* Contains a list of pointers which indicate where in GIVCOL a
* level's Givens rotations are stored. GIVPTR(i+1) - GIVPTR(i)
* indicates the number of Givens rotations.
*
* GIVCOL (input) INTEGER array, dimension (2, N lg N)
* Each pair of numbers indicates a pair of columns to take place
* in a Givens rotation.
*
* GIVNUM (input) REAL array, dimension (2, N lg N)
* Each number indicates the S value to be used in the
* corresponding Givens rotation.
*
* Q (input) REAL array, dimension (N**2)
* Contains the square eigenblocks from previous levels, the
* starting positions for blocks are given by QPTR.
*
* QPTR (input) INTEGER array, dimension (N+2)
* Contains a list of pointers which indicate where in Q an
* eigenblock is stored. SQRT( QPTR(i+1) - QPTR(i) ) indicates
* the size of the block.
*
* Z (output) REAL array, dimension (N)
* On output this vector contains the updating vector (the last
* row of the first sub-eigenvector matrix and the first row of
* the second sub-eigenvector matrix).
*
* ZTEMP (workspace) REAL array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param tlvls
* @param curlvl
* @param curpbm
* @param prmptr
* @param perm
* @param givptr
* @param givcol
* @param givnum
* @param q
* @param qptr
* @param z
* @param ztemp
* @param info
*
*/
abstract public void slaeda(int n, int tlvls, int curlvl, int curpbm, int[] prmptr, int[] perm, int[] givptr, int[] givcol, float[] givnum, float[] q, int[] qptr, float[] z, float[] ztemp, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAEDA computes the Z vector corresponding to the merge step in the
* CURLVLth step of the merge process with TLVLS steps for the CURPBMth
* problem.
*
* Arguments
* =========
*
* N (input) INTEGER
* The dimension of the symmetric tridiagonal matrix. N >= 0.
*
* TLVLS (input) INTEGER
* The total number of merging levels in the overall divide and
* conquer tree.
*
* CURLVL (input) INTEGER
* The current level in the overall merge routine,
* 0 <= curlvl <= tlvls.
*
* CURPBM (input) INTEGER
* The current problem in the current level in the overall
* merge routine (counting from upper left to lower right).
*
* PRMPTR (input) INTEGER array, dimension (N lg N)
* Contains a list of pointers which indicate where in PERM a
* level's permutation is stored. PRMPTR(i+1) - PRMPTR(i)
* indicates the size of the permutation and incidentally the
* size of the full, non-deflated problem.
*
* PERM (input) INTEGER array, dimension (N lg N)
* Contains the permutations (from deflation and sorting) to be
* applied to each eigenblock.
*
* GIVPTR (input) INTEGER array, dimension (N lg N)
* Contains a list of pointers which indicate where in GIVCOL a
* level's Givens rotations are stored. GIVPTR(i+1) - GIVPTR(i)
* indicates the number of Givens rotations.
*
* GIVCOL (input) INTEGER array, dimension (2, N lg N)
* Each pair of numbers indicates a pair of columns to take place
* in a Givens rotation.
*
* GIVNUM (input) REAL array, dimension (2, N lg N)
* Each number indicates the S value to be used in the
* corresponding Givens rotation.
*
* Q (input) REAL array, dimension (N**2)
* Contains the square eigenblocks from previous levels, the
* starting positions for blocks are given by QPTR.
*
* QPTR (input) INTEGER array, dimension (N+2)
* Contains a list of pointers which indicate where in Q an
* eigenblock is stored. SQRT( QPTR(i+1) - QPTR(i) ) indicates
* the size of the block.
*
* Z (output) REAL array, dimension (N)
* On output this vector contains the updating vector (the last
* row of the first sub-eigenvector matrix and the first row of
* the second sub-eigenvector matrix).
*
* ZTEMP (workspace) REAL array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param tlvls
* @param curlvl
* @param curpbm
* @param prmptr
* @param _prmptr_offset
* @param perm
* @param _perm_offset
* @param givptr
* @param _givptr_offset
* @param givcol
* @param _givcol_offset
* @param givnum
* @param _givnum_offset
* @param q
* @param _q_offset
* @param qptr
* @param _qptr_offset
* @param z
* @param _z_offset
* @param ztemp
* @param _ztemp_offset
* @param info
*
*/
abstract public void slaeda(int n, int tlvls, int curlvl, int curpbm, int[] prmptr, int _prmptr_offset, int[] perm, int _perm_offset, int[] givptr, int _givptr_offset, int[] givcol, int _givcol_offset, float[] givnum, int _givnum_offset, float[] q, int _q_offset, int[] qptr, int _qptr_offset, float[] z, int _z_offset, float[] ztemp, int _ztemp_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAEIN uses inverse iteration to find a right or left eigenvector
* corresponding to the eigenvalue (WR,WI) of a real upper Hessenberg
* matrix H.
*
* Arguments
* =========
*
* RIGHTV (input) LOGICAL
* = .TRUE. : compute right eigenvector;
* = .FALSE.: compute left eigenvector.
*
* NOINIT (input) LOGICAL
* = .TRUE. : no initial vector supplied in (VR,VI).
* = .FALSE.: initial vector supplied in (VR,VI).
*
* N (input) INTEGER
* The order of the matrix H. N >= 0.
*
* H (input) REAL array, dimension (LDH,N)
* The upper Hessenberg matrix H.
*
* LDH (input) INTEGER
* The leading dimension of the array H. LDH >= max(1,N).
*
* WR (input) REAL
* WI (input) REAL
* The real and imaginary parts of the eigenvalue of H whose
* corresponding right or left eigenvector is to be computed.
*
* VR (input/output) REAL array, dimension (N)
* VI (input/output) REAL array, dimension (N)
* On entry, if NOINIT = .FALSE. and WI = 0.0, VR must contain
* a real starting vector for inverse iteration using the real
* eigenvalue WR; if NOINIT = .FALSE. and WI.ne.0.0, VR and VI
* must contain the real and imaginary parts of a complex
* starting vector for inverse iteration using the complex
* eigenvalue (WR,WI); otherwise VR and VI need not be set.
* On exit, if WI = 0.0 (real eigenvalue), VR contains the
* computed real eigenvector; if WI.ne.0.0 (complex eigenvalue),
* VR and VI contain the real and imaginary parts of the
* computed complex eigenvector. The eigenvector is normalized
* so that the component of largest magnitude has magnitude 1;
* here the magnitude of a complex number (x,y) is taken to be
* |x| + |y|.
* VI is not referenced if WI = 0.0.
*
* B (workspace) REAL array, dimension (LDB,N)
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= N+1.
*
* WORK (workspace) REAL array, dimension (N)
*
* EPS3 (input) REAL
* A small machine-dependent value which is used to perturb
* close eigenvalues, and to replace zero pivots.
*
* SMLNUM (input) REAL
* A machine-dependent value close to the underflow threshold.
*
* BIGNUM (input) REAL
* A machine-dependent value close to the overflow threshold.
*
* INFO (output) INTEGER
* = 0: successful exit
* = 1: inverse iteration did not converge; VR is set to the
* last iterate, and so is VI if WI.ne.0.0.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param rightv
* @param noinit
* @param n
* @param h
* @param ldh
* @param wr
* @param wi
* @param vr
* @param vi
* @param b
* @param ldb
* @param work
* @param eps3
* @param smlnum
* @param bignum
* @param info
*
*/
abstract public void slaein(boolean rightv, boolean noinit, int n, float[] h, int ldh, float wr, float wi, float[] vr, float[] vi, float[] b, int ldb, float[] work, float eps3, float smlnum, float bignum, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAEIN uses inverse iteration to find a right or left eigenvector
* corresponding to the eigenvalue (WR,WI) of a real upper Hessenberg
* matrix H.
*
* Arguments
* =========
*
* RIGHTV (input) LOGICAL
* = .TRUE. : compute right eigenvector;
* = .FALSE.: compute left eigenvector.
*
* NOINIT (input) LOGICAL
* = .TRUE. : no initial vector supplied in (VR,VI).
* = .FALSE.: initial vector supplied in (VR,VI).
*
* N (input) INTEGER
* The order of the matrix H. N >= 0.
*
* H (input) REAL array, dimension (LDH,N)
* The upper Hessenberg matrix H.
*
* LDH (input) INTEGER
* The leading dimension of the array H. LDH >= max(1,N).
*
* WR (input) REAL
* WI (input) REAL
* The real and imaginary parts of the eigenvalue of H whose
* corresponding right or left eigenvector is to be computed.
*
* VR (input/output) REAL array, dimension (N)
* VI (input/output) REAL array, dimension (N)
* On entry, if NOINIT = .FALSE. and WI = 0.0, VR must contain
* a real starting vector for inverse iteration using the real
* eigenvalue WR; if NOINIT = .FALSE. and WI.ne.0.0, VR and VI
* must contain the real and imaginary parts of a complex
* starting vector for inverse iteration using the complex
* eigenvalue (WR,WI); otherwise VR and VI need not be set.
* On exit, if WI = 0.0 (real eigenvalue), VR contains the
* computed real eigenvector; if WI.ne.0.0 (complex eigenvalue),
* VR and VI contain the real and imaginary parts of the
* computed complex eigenvector. The eigenvector is normalized
* so that the component of largest magnitude has magnitude 1;
* here the magnitude of a complex number (x,y) is taken to be
* |x| + |y|.
* VI is not referenced if WI = 0.0.
*
* B (workspace) REAL array, dimension (LDB,N)
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= N+1.
*
* WORK (workspace) REAL array, dimension (N)
*
* EPS3 (input) REAL
* A small machine-dependent value which is used to perturb
* close eigenvalues, and to replace zero pivots.
*
* SMLNUM (input) REAL
* A machine-dependent value close to the underflow threshold.
*
* BIGNUM (input) REAL
* A machine-dependent value close to the overflow threshold.
*
* INFO (output) INTEGER
* = 0: successful exit
* = 1: inverse iteration did not converge; VR is set to the
* last iterate, and so is VI if WI.ne.0.0.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param rightv
* @param noinit
* @param n
* @param h
* @param _h_offset
* @param ldh
* @param wr
* @param wi
* @param vr
* @param _vr_offset
* @param vi
* @param _vi_offset
* @param b
* @param _b_offset
* @param ldb
* @param work
* @param _work_offset
* @param eps3
* @param smlnum
* @param bignum
* @param info
*
*/
abstract public void slaein(boolean rightv, boolean noinit, int n, float[] h, int _h_offset, int ldh, float wr, float wi, float[] vr, int _vr_offset, float[] vi, int _vi_offset, float[] b, int _b_offset, int ldb, float[] work, int _work_offset, float eps3, float smlnum, float bignum, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAEV2 computes the eigendecomposition of a 2-by-2 symmetric matrix
* [ A B ]
* [ B C ].
* On return, RT1 is the eigenvalue of larger absolute value, RT2 is the
* eigenvalue of smaller absolute value, and (CS1,SN1) is the unit right
* eigenvector for RT1, giving the decomposition
*
* [ CS1 SN1 ] [ A B ] [ CS1 -SN1 ] = [ RT1 0 ]
* [-SN1 CS1 ] [ B C ] [ SN1 CS1 ] [ 0 RT2 ].
*
* Arguments
* =========
*
* A (input) REAL
* The (1,1) element of the 2-by-2 matrix.
*
* B (input) REAL
* The (1,2) element and the conjugate of the (2,1) element of
* the 2-by-2 matrix.
*
* C (input) REAL
* The (2,2) element of the 2-by-2 matrix.
*
* RT1 (output) REAL
* The eigenvalue of larger absolute value.
*
* RT2 (output) REAL
* The eigenvalue of smaller absolute value.
*
* CS1 (output) REAL
* SN1 (output) REAL
* The vector (CS1, SN1) is a unit right eigenvector for RT1.
*
* Further Details
* ===============
*
* RT1 is accurate to a few ulps barring over/underflow.
*
* RT2 may be inaccurate if there is massive cancellation in the
* determinant A*C-B*B; higher precision or correctly rounded or
* correctly truncated arithmetic would be needed to compute RT2
* accurately in all cases.
*
* CS1 and SN1 are accurate to a few ulps barring over/underflow.
*
* Overflow is possible only if RT1 is within a factor of 5 of overflow.
* Underflow is harmless if the input data is 0 or exceeds
* underflow_threshold / macheps.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param a
* @param b
* @param c
* @param rt1
* @param rt2
* @param cs1
* @param sn1
*
*/
abstract public void slaev2(float a, float b, float c, org.netlib.util.floatW rt1, org.netlib.util.floatW rt2, org.netlib.util.floatW cs1, org.netlib.util.floatW sn1);
/**
*
* ..
*
* Purpose
* =======
*
* SLAEXC swaps adjacent diagonal blocks T11 and T22 of order 1 or 2 in
* an upper quasi-triangular matrix T by an orthogonal similarity
* transformation.
*
* T must be in Schur canonical form, that is, block upper triangular
* with 1-by-1 and 2-by-2 diagonal blocks; each 2-by-2 diagonal block
* has its diagonal elemnts equal and its off-diagonal elements of
* opposite sign.
*
* Arguments
* =========
*
* WANTQ (input) LOGICAL
* = .TRUE. : accumulate the transformation in the matrix Q;
* = .FALSE.: do not accumulate the transformation.
*
* N (input) INTEGER
* The order of the matrix T. N >= 0.
*
* T (input/output) REAL array, dimension (LDT,N)
* On entry, the upper quasi-triangular matrix T, in Schur
* canonical form.
* On exit, the updated matrix T, again in Schur canonical form.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= max(1,N).
*
* Q (input/output) REAL array, dimension (LDQ,N)
* On entry, if WANTQ is .TRUE., the orthogonal matrix Q.
* On exit, if WANTQ is .TRUE., the updated matrix Q.
* If WANTQ is .FALSE., Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q.
* LDQ >= 1; and if WANTQ is .TRUE., LDQ >= N.
*
* J1 (input) INTEGER
* The index of the first row of the first block T11.
*
* N1 (input) INTEGER
* The order of the first block T11. N1 = 0, 1 or 2.
*
* N2 (input) INTEGER
* The order of the second block T22. N2 = 0, 1 or 2.
*
* WORK (workspace) REAL array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* = 1: the transformed matrix T would be too far from Schur
* form; the blocks are not swapped and T and Q are
* unchanged.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param wantq
* @param n
* @param t
* @param ldt
* @param q
* @param ldq
* @param j1
* @param n1
* @param n2
* @param work
* @param info
*
*/
abstract public void slaexc(boolean wantq, int n, float[] t, int ldt, float[] q, int ldq, int j1, int n1, int n2, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAEXC swaps adjacent diagonal blocks T11 and T22 of order 1 or 2 in
* an upper quasi-triangular matrix T by an orthogonal similarity
* transformation.
*
* T must be in Schur canonical form, that is, block upper triangular
* with 1-by-1 and 2-by-2 diagonal blocks; each 2-by-2 diagonal block
* has its diagonal elemnts equal and its off-diagonal elements of
* opposite sign.
*
* Arguments
* =========
*
* WANTQ (input) LOGICAL
* = .TRUE. : accumulate the transformation in the matrix Q;
* = .FALSE.: do not accumulate the transformation.
*
* N (input) INTEGER
* The order of the matrix T. N >= 0.
*
* T (input/output) REAL array, dimension (LDT,N)
* On entry, the upper quasi-triangular matrix T, in Schur
* canonical form.
* On exit, the updated matrix T, again in Schur canonical form.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= max(1,N).
*
* Q (input/output) REAL array, dimension (LDQ,N)
* On entry, if WANTQ is .TRUE., the orthogonal matrix Q.
* On exit, if WANTQ is .TRUE., the updated matrix Q.
* If WANTQ is .FALSE., Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q.
* LDQ >= 1; and if WANTQ is .TRUE., LDQ >= N.
*
* J1 (input) INTEGER
* The index of the first row of the first block T11.
*
* N1 (input) INTEGER
* The order of the first block T11. N1 = 0, 1 or 2.
*
* N2 (input) INTEGER
* The order of the second block T22. N2 = 0, 1 or 2.
*
* WORK (workspace) REAL array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* = 1: the transformed matrix T would be too far from Schur
* form; the blocks are not swapped and T and Q are
* unchanged.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param wantq
* @param n
* @param t
* @param _t_offset
* @param ldt
* @param q
* @param _q_offset
* @param ldq
* @param j1
* @param n1
* @param n2
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void slaexc(boolean wantq, int n, float[] t, int _t_offset, int ldt, float[] q, int _q_offset, int ldq, int j1, int n1, int n2, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAG2 computes the eigenvalues of a 2 x 2 generalized eigenvalue
* problem A - w B, with scaling as necessary to avoid over-/underflow.
*
* The scaling factor "s" results in a modified eigenvalue equation
*
* s A - w B
*
* where s is a non-negative scaling factor chosen so that w, w B,
* and s A do not overflow and, if possible, do not underflow, either.
*
* Arguments
* =========
*
* A (input) REAL array, dimension (LDA, 2)
* On entry, the 2 x 2 matrix A. It is assumed that its 1-norm
* is less than 1/SAFMIN. Entries less than
* sqrt(SAFMIN)*norm(A) are subject to being treated as zero.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= 2.
*
* B (input) REAL array, dimension (LDB, 2)
* On entry, the 2 x 2 upper triangular matrix B. It is
* assumed that the one-norm of B is less than 1/SAFMIN. The
* diagonals should be at least sqrt(SAFMIN) times the largest
* element of B (in absolute value); if a diagonal is smaller
* than that, then +/- sqrt(SAFMIN) will be used instead of
* that diagonal.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= 2.
*
* SAFMIN (input) REAL
* The smallest positive number s.t. 1/SAFMIN does not
* overflow. (This should always be SLAMCH('S') -- it is an
* argument in order to avoid having to call SLAMCH frequently.)
*
* SCALE1 (output) REAL
* A scaling factor used to avoid over-/underflow in the
* eigenvalue equation which defines the first eigenvalue. If
* the eigenvalues are complex, then the eigenvalues are
* ( WR1 +/- WI i ) / SCALE1 (which may lie outside the
* exponent range of the machine), SCALE1=SCALE2, and SCALE1
* will always be positive. If the eigenvalues are real, then
* the first (real) eigenvalue is WR1 / SCALE1 , but this may
* overflow or underflow, and in fact, SCALE1 may be zero or
* less than the underflow threshhold if the exact eigenvalue
* is sufficiently large.
*
* SCALE2 (output) REAL
* A scaling factor used to avoid over-/underflow in the
* eigenvalue equation which defines the second eigenvalue. If
* the eigenvalues are complex, then SCALE2=SCALE1. If the
* eigenvalues are real, then the second (real) eigenvalue is
* WR2 / SCALE2 , but this may overflow or underflow, and in
* fact, SCALE2 may be zero or less than the underflow
* threshhold if the exact eigenvalue is sufficiently large.
*
* WR1 (output) REAL
* If the eigenvalue is real, then WR1 is SCALE1 times the
* eigenvalue closest to the (2,2) element of A B**(-1). If the
* eigenvalue is complex, then WR1=WR2 is SCALE1 times the real
* part of the eigenvalues.
*
* WR2 (output) REAL
* If the eigenvalue is real, then WR2 is SCALE2 times the
* other eigenvalue. If the eigenvalue is complex, then
* WR1=WR2 is SCALE1 times the real part of the eigenvalues.
*
* WI (output) REAL
* If the eigenvalue is real, then WI is zero. If the
* eigenvalue is complex, then WI is SCALE1 times the imaginary
* part of the eigenvalues. WI will always be non-negative.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param a
* @param lda
* @param b
* @param ldb
* @param safmin
* @param scale1
* @param scale2
* @param wr1
* @param wr2
* @param wi
*
*/
abstract public void slag2(float[] a, int lda, float[] b, int ldb, float safmin, org.netlib.util.floatW scale1, org.netlib.util.floatW scale2, org.netlib.util.floatW wr1, org.netlib.util.floatW wr2, org.netlib.util.floatW wi);
/**
*
* ..
*
* Purpose
* =======
*
* SLAG2 computes the eigenvalues of a 2 x 2 generalized eigenvalue
* problem A - w B, with scaling as necessary to avoid over-/underflow.
*
* The scaling factor "s" results in a modified eigenvalue equation
*
* s A - w B
*
* where s is a non-negative scaling factor chosen so that w, w B,
* and s A do not overflow and, if possible, do not underflow, either.
*
* Arguments
* =========
*
* A (input) REAL array, dimension (LDA, 2)
* On entry, the 2 x 2 matrix A. It is assumed that its 1-norm
* is less than 1/SAFMIN. Entries less than
* sqrt(SAFMIN)*norm(A) are subject to being treated as zero.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= 2.
*
* B (input) REAL array, dimension (LDB, 2)
* On entry, the 2 x 2 upper triangular matrix B. It is
* assumed that the one-norm of B is less than 1/SAFMIN. The
* diagonals should be at least sqrt(SAFMIN) times the largest
* element of B (in absolute value); if a diagonal is smaller
* than that, then +/- sqrt(SAFMIN) will be used instead of
* that diagonal.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= 2.
*
* SAFMIN (input) REAL
* The smallest positive number s.t. 1/SAFMIN does not
* overflow. (This should always be SLAMCH('S') -- it is an
* argument in order to avoid having to call SLAMCH frequently.)
*
* SCALE1 (output) REAL
* A scaling factor used to avoid over-/underflow in the
* eigenvalue equation which defines the first eigenvalue. If
* the eigenvalues are complex, then the eigenvalues are
* ( WR1 +/- WI i ) / SCALE1 (which may lie outside the
* exponent range of the machine), SCALE1=SCALE2, and SCALE1
* will always be positive. If the eigenvalues are real, then
* the first (real) eigenvalue is WR1 / SCALE1 , but this may
* overflow or underflow, and in fact, SCALE1 may be zero or
* less than the underflow threshhold if the exact eigenvalue
* is sufficiently large.
*
* SCALE2 (output) REAL
* A scaling factor used to avoid over-/underflow in the
* eigenvalue equation which defines the second eigenvalue. If
* the eigenvalues are complex, then SCALE2=SCALE1. If the
* eigenvalues are real, then the second (real) eigenvalue is
* WR2 / SCALE2 , but this may overflow or underflow, and in
* fact, SCALE2 may be zero or less than the underflow
* threshhold if the exact eigenvalue is sufficiently large.
*
* WR1 (output) REAL
* If the eigenvalue is real, then WR1 is SCALE1 times the
* eigenvalue closest to the (2,2) element of A B**(-1). If the
* eigenvalue is complex, then WR1=WR2 is SCALE1 times the real
* part of the eigenvalues.
*
* WR2 (output) REAL
* If the eigenvalue is real, then WR2 is SCALE2 times the
* other eigenvalue. If the eigenvalue is complex, then
* WR1=WR2 is SCALE1 times the real part of the eigenvalues.
*
* WI (output) REAL
* If the eigenvalue is real, then WI is zero. If the
* eigenvalue is complex, then WI is SCALE1 times the imaginary
* part of the eigenvalues. WI will always be non-negative.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param safmin
* @param scale1
* @param scale2
* @param wr1
* @param wr2
* @param wi
*
*/
abstract public void slag2(float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, float safmin, org.netlib.util.floatW scale1, org.netlib.util.floatW scale2, org.netlib.util.floatW wr1, org.netlib.util.floatW wr2, org.netlib.util.floatW wi);
/**
*
* ..
*
* Purpose
* =======
*
* SLAG2D converts a SINGLE PRECISION matrix, SA, to a DOUBLE
* PRECISION matrix, A.
*
* Note that while it is possible to overflow while converting
* from double to single, it is not possible to overflow when
* converting from single to double.
*
* This is a helper routine so there is no argument checking.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of lines of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* SA (output) REAL array, dimension (LDSA,N)
* On exit, the M-by-N coefficient matrix SA.
*
* LDSA (input) INTEGER
* The leading dimension of the array SA. LDSA >= max(1,M).
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N coefficient matrix A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* INFO (output) INTEGER
* = 0: successful exit
* =========
*
* .. Local Scalars ..
*
*
* @param m
* @param n
* @param sa
* @param ldsa
* @param a
* @param lda
* @param info
*
*/
abstract public void slag2d(int m, int n, float[] sa, int ldsa, double[] a, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAG2D converts a SINGLE PRECISION matrix, SA, to a DOUBLE
* PRECISION matrix, A.
*
* Note that while it is possible to overflow while converting
* from double to single, it is not possible to overflow when
* converting from single to double.
*
* This is a helper routine so there is no argument checking.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of lines of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* SA (output) REAL array, dimension (LDSA,N)
* On exit, the M-by-N coefficient matrix SA.
*
* LDSA (input) INTEGER
* The leading dimension of the array SA. LDSA >= max(1,M).
*
* A (input) DOUBLE PRECISION array, dimension (LDA,N)
* On entry, the M-by-N coefficient matrix A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* INFO (output) INTEGER
* = 0: successful exit
* =========
*
* .. Local Scalars ..
*
*
* @param m
* @param n
* @param sa
* @param _sa_offset
* @param ldsa
* @param a
* @param _a_offset
* @param lda
* @param info
*
*/
abstract public void slag2d(int m, int n, float[] sa, int _sa_offset, int ldsa, double[] a, int _a_offset, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAGS2 computes 2-by-2 orthogonal matrices U, V and Q, such
* that if ( UPPER ) then
*
* U'*A*Q = U'*( A1 A2 )*Q = ( x 0 )
* ( 0 A3 ) ( x x )
* and
* V'*B*Q = V'*( B1 B2 )*Q = ( x 0 )
* ( 0 B3 ) ( x x )
*
* or if ( .NOT.UPPER ) then
*
* U'*A*Q = U'*( A1 0 )*Q = ( x x )
* ( A2 A3 ) ( 0 x )
* and
* V'*B*Q = V'*( B1 0 )*Q = ( x x )
* ( B2 B3 ) ( 0 x )
*
* The rows of the transformed A and B are parallel, where
*
* U = ( CSU SNU ), V = ( CSV SNV ), Q = ( CSQ SNQ )
* ( -SNU CSU ) ( -SNV CSV ) ( -SNQ CSQ )
*
* Z' denotes the transpose of Z.
*
*
* Arguments
* =========
*
* UPPER (input) LOGICAL
* = .TRUE.: the input matrices A and B are upper triangular.
* = .FALSE.: the input matrices A and B are lower triangular.
*
* A1 (input) REAL
* A2 (input) REAL
* A3 (input) REAL
* On entry, A1, A2 and A3 are elements of the input 2-by-2
* upper (lower) triangular matrix A.
*
* B1 (input) REAL
* B2 (input) REAL
* B3 (input) REAL
* On entry, B1, B2 and B3 are elements of the input 2-by-2
* upper (lower) triangular matrix B.
*
* CSU (output) REAL
* SNU (output) REAL
* The desired orthogonal matrix U.
*
* CSV (output) REAL
* SNV (output) REAL
* The desired orthogonal matrix V.
*
* CSQ (output) REAL
* SNQ (output) REAL
* The desired orthogonal matrix Q.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param upper
* @param a1
* @param a2
* @param a3
* @param b1
* @param b2
* @param b3
* @param csu
* @param snu
* @param csv
* @param snv
* @param csq
* @param snq
*
*/
abstract public void slags2(boolean upper, float a1, float a2, float a3, float b1, float b2, float b3, org.netlib.util.floatW csu, org.netlib.util.floatW snu, org.netlib.util.floatW csv, org.netlib.util.floatW snv, org.netlib.util.floatW csq, org.netlib.util.floatW snq);
/**
*
* ..
*
* Purpose
* =======
*
* SLAGTF factorizes the matrix (T - lambda*I), where T is an n by n
* tridiagonal matrix and lambda is a scalar, as
*
* T - lambda*I = PLU,
*
* where P is a permutation matrix, L is a unit lower tridiagonal matrix
* with at most one non-zero sub-diagonal elements per column and U is
* an upper triangular matrix with at most two non-zero super-diagonal
* elements per column.
*
* The factorization is obtained by Gaussian elimination with partial
* pivoting and implicit row scaling.
*
* The parameter LAMBDA is included in the routine so that SLAGTF may
* be used, in conjunction with SLAGTS, to obtain eigenvectors of T by
* inverse iteration.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix T.
*
* A (input/output) REAL array, dimension (N)
* On entry, A must contain the diagonal elements of T.
*
* On exit, A is overwritten by the n diagonal elements of the
* upper triangular matrix U of the factorization of T.
*
* LAMBDA (input) REAL
* On entry, the scalar lambda.
*
* B (input/output) REAL array, dimension (N-1)
* On entry, B must contain the (n-1) super-diagonal elements of
* T.
*
* On exit, B is overwritten by the (n-1) super-diagonal
* elements of the matrix U of the factorization of T.
*
* C (input/output) REAL array, dimension (N-1)
* On entry, C must contain the (n-1) sub-diagonal elements of
* T.
*
* On exit, C is overwritten by the (n-1) sub-diagonal elements
* of the matrix L of the factorization of T.
*
* TOL (input) REAL
* On entry, a relative tolerance used to indicate whether or
* not the matrix (T - lambda*I) is nearly singular. TOL should
* normally be chose as approximately the largest relative error
* in the elements of T. For example, if the elements of T are
* correct to about 4 significant figures, then TOL should be
* set to about 5*10**(-4). If TOL is supplied as less than eps,
* where eps is the relative machine precision, then the value
* eps is used in place of TOL.
*
* D (output) REAL array, dimension (N-2)
* On exit, D is overwritten by the (n-2) second super-diagonal
* elements of the matrix U of the factorization of T.
*
* IN (output) INTEGER array, dimension (N)
* On exit, IN contains details of the permutation matrix P. If
* an interchange occurred at the kth step of the elimination,
* then IN(k) = 1, otherwise IN(k) = 0. The element IN(n)
* returns the smallest positive integer j such that
*
* abs( u(j,j) ).le. norm( (T - lambda*I)(j) )*TOL,
*
* where norm( A(j) ) denotes the sum of the absolute values of
* the jth row of the matrix A. If no such j exists then IN(n)
* is returned as zero. If IN(n) is returned as positive, then a
* diagonal element of U is small, indicating that
* (T - lambda*I) is singular or nearly singular,
*
* INFO (output) INTEGER
* = 0 : successful exit
* .lt. 0: if INFO = -k, the kth argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param a
* @param lambda
* @param b
* @param c
* @param tol
* @param d
* @param in
* @param info
*
*/
abstract public void slagtf(int n, float[] a, float lambda, float[] b, float[] c, float tol, float[] d, int[] in, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAGTF factorizes the matrix (T - lambda*I), where T is an n by n
* tridiagonal matrix and lambda is a scalar, as
*
* T - lambda*I = PLU,
*
* where P is a permutation matrix, L is a unit lower tridiagonal matrix
* with at most one non-zero sub-diagonal elements per column and U is
* an upper triangular matrix with at most two non-zero super-diagonal
* elements per column.
*
* The factorization is obtained by Gaussian elimination with partial
* pivoting and implicit row scaling.
*
* The parameter LAMBDA is included in the routine so that SLAGTF may
* be used, in conjunction with SLAGTS, to obtain eigenvectors of T by
* inverse iteration.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix T.
*
* A (input/output) REAL array, dimension (N)
* On entry, A must contain the diagonal elements of T.
*
* On exit, A is overwritten by the n diagonal elements of the
* upper triangular matrix U of the factorization of T.
*
* LAMBDA (input) REAL
* On entry, the scalar lambda.
*
* B (input/output) REAL array, dimension (N-1)
* On entry, B must contain the (n-1) super-diagonal elements of
* T.
*
* On exit, B is overwritten by the (n-1) super-diagonal
* elements of the matrix U of the factorization of T.
*
* C (input/output) REAL array, dimension (N-1)
* On entry, C must contain the (n-1) sub-diagonal elements of
* T.
*
* On exit, C is overwritten by the (n-1) sub-diagonal elements
* of the matrix L of the factorization of T.
*
* TOL (input) REAL
* On entry, a relative tolerance used to indicate whether or
* not the matrix (T - lambda*I) is nearly singular. TOL should
* normally be chose as approximately the largest relative error
* in the elements of T. For example, if the elements of T are
* correct to about 4 significant figures, then TOL should be
* set to about 5*10**(-4). If TOL is supplied as less than eps,
* where eps is the relative machine precision, then the value
* eps is used in place of TOL.
*
* D (output) REAL array, dimension (N-2)
* On exit, D is overwritten by the (n-2) second super-diagonal
* elements of the matrix U of the factorization of T.
*
* IN (output) INTEGER array, dimension (N)
* On exit, IN contains details of the permutation matrix P. If
* an interchange occurred at the kth step of the elimination,
* then IN(k) = 1, otherwise IN(k) = 0. The element IN(n)
* returns the smallest positive integer j such that
*
* abs( u(j,j) ).le. norm( (T - lambda*I)(j) )*TOL,
*
* where norm( A(j) ) denotes the sum of the absolute values of
* the jth row of the matrix A. If no such j exists then IN(n)
* is returned as zero. If IN(n) is returned as positive, then a
* diagonal element of U is small, indicating that
* (T - lambda*I) is singular or nearly singular,
*
* INFO (output) INTEGER
* = 0 : successful exit
* .lt. 0: if INFO = -k, the kth argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param a
* @param _a_offset
* @param lambda
* @param b
* @param _b_offset
* @param c
* @param _c_offset
* @param tol
* @param d
* @param _d_offset
* @param in
* @param _in_offset
* @param info
*
*/
abstract public void slagtf(int n, float[] a, int _a_offset, float lambda, float[] b, int _b_offset, float[] c, int _c_offset, float tol, float[] d, int _d_offset, int[] in, int _in_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAGTM performs a matrix-vector product of the form
*
* B := alpha * A * X + beta * B
*
* where A is a tridiagonal matrix of order N, B and X are N by NRHS
* matrices, and alpha and beta are real scalars, each of which may be
* 0., 1., or -1.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* Specifies the operation applied to A.
* = 'N': No transpose, B := alpha * A * X + beta * B
* = 'T': Transpose, B := alpha * A'* X + beta * B
* = 'C': Conjugate transpose = Transpose
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices X and B.
*
* ALPHA (input) REAL
* The scalar alpha. ALPHA must be 0., 1., or -1.; otherwise,
* it is assumed to be 0.
*
* DL (input) REAL array, dimension (N-1)
* The (n-1) sub-diagonal elements of T.
*
* D (input) REAL array, dimension (N)
* The diagonal elements of T.
*
* DU (input) REAL array, dimension (N-1)
* The (n-1) super-diagonal elements of T.
*
* X (input) REAL array, dimension (LDX,NRHS)
* The N by NRHS matrix X.
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(N,1).
*
* BETA (input) REAL
* The scalar beta. BETA must be 0., 1., or -1.; otherwise,
* it is assumed to be 1.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the N by NRHS matrix B.
* On exit, B is overwritten by the matrix expression
* B := alpha * A * X + beta * B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(N,1).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param trans
* @param n
* @param nrhs
* @param alpha
* @param dl
* @param d
* @param du
* @param x
* @param ldx
* @param beta
* @param b
* @param ldb
*
*/
abstract public void slagtm(java.lang.String trans, int n, int nrhs, float alpha, float[] dl, float[] d, float[] du, float[] x, int ldx, float beta, float[] b, int ldb);
/**
*
* ..
*
* Purpose
* =======
*
* SLAGTM performs a matrix-vector product of the form
*
* B := alpha * A * X + beta * B
*
* where A is a tridiagonal matrix of order N, B and X are N by NRHS
* matrices, and alpha and beta are real scalars, each of which may be
* 0., 1., or -1.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* Specifies the operation applied to A.
* = 'N': No transpose, B := alpha * A * X + beta * B
* = 'T': Transpose, B := alpha * A'* X + beta * B
* = 'C': Conjugate transpose = Transpose
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices X and B.
*
* ALPHA (input) REAL
* The scalar alpha. ALPHA must be 0., 1., or -1.; otherwise,
* it is assumed to be 0.
*
* DL (input) REAL array, dimension (N-1)
* The (n-1) sub-diagonal elements of T.
*
* D (input) REAL array, dimension (N)
* The diagonal elements of T.
*
* DU (input) REAL array, dimension (N-1)
* The (n-1) super-diagonal elements of T.
*
* X (input) REAL array, dimension (LDX,NRHS)
* The N by NRHS matrix X.
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(N,1).
*
* BETA (input) REAL
* The scalar beta. BETA must be 0., 1., or -1.; otherwise,
* it is assumed to be 1.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the N by NRHS matrix B.
* On exit, B is overwritten by the matrix expression
* B := alpha * A * X + beta * B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(N,1).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param trans
* @param n
* @param nrhs
* @param alpha
* @param dl
* @param _dl_offset
* @param d
* @param _d_offset
* @param du
* @param _du_offset
* @param x
* @param _x_offset
* @param ldx
* @param beta
* @param b
* @param _b_offset
* @param ldb
*
*/
abstract public void slagtm(java.lang.String trans, int n, int nrhs, float alpha, float[] dl, int _dl_offset, float[] d, int _d_offset, float[] du, int _du_offset, float[] x, int _x_offset, int ldx, float beta, float[] b, int _b_offset, int ldb);
/**
*
* ..
*
* Purpose
* =======
*
* SLAGTS may be used to solve one of the systems of equations
*
* (T - lambda*I)*x = y or (T - lambda*I)'*x = y,
*
* where T is an n by n tridiagonal matrix, for x, following the
* factorization of (T - lambda*I) as
*
* (T - lambda*I) = P*L*U ,
*
* by routine SLAGTF. The choice of equation to be solved is
* controlled by the argument JOB, and in each case there is an option
* to perturb zero or very small diagonal elements of U, this option
* being intended for use in applications such as inverse iteration.
*
* Arguments
* =========
*
* JOB (input) INTEGER
* Specifies the job to be performed by SLAGTS as follows:
* = 1: The equations (T - lambda*I)x = y are to be solved,
* but diagonal elements of U are not to be perturbed.
* = -1: The equations (T - lambda*I)x = y are to be solved
* and, if overflow would otherwise occur, the diagonal
* elements of U are to be perturbed. See argument TOL
* below.
* = 2: The equations (T - lambda*I)'x = y are to be solved,
* but diagonal elements of U are not to be perturbed.
* = -2: The equations (T - lambda*I)'x = y are to be solved
* and, if overflow would otherwise occur, the diagonal
* elements of U are to be perturbed. See argument TOL
* below.
*
* N (input) INTEGER
* The order of the matrix T.
*
* A (input) REAL array, dimension (N)
* On entry, A must contain the diagonal elements of U as
* returned from SLAGTF.
*
* B (input) REAL array, dimension (N-1)
* On entry, B must contain the first super-diagonal elements of
* U as returned from SLAGTF.
*
* C (input) REAL array, dimension (N-1)
* On entry, C must contain the sub-diagonal elements of L as
* returned from SLAGTF.
*
* D (input) REAL array, dimension (N-2)
* On entry, D must contain the second super-diagonal elements
* of U as returned from SLAGTF.
*
* IN (input) INTEGER array, dimension (N)
* On entry, IN must contain details of the matrix P as returned
* from SLAGTF.
*
* Y (input/output) REAL array, dimension (N)
* On entry, the right hand side vector y.
* On exit, Y is overwritten by the solution vector x.
*
* TOL (input/output) REAL
* On entry, with JOB .lt. 0, TOL should be the minimum
* perturbation to be made to very small diagonal elements of U.
* TOL should normally be chosen as about eps*norm(U), where eps
* is the relative machine precision, but if TOL is supplied as
* non-positive, then it is reset to eps*max( abs( u(i,j) ) ).
* If JOB .gt. 0 then TOL is not referenced.
*
* On exit, TOL is changed as described above, only if TOL is
* non-positive on entry. Otherwise TOL is unchanged.
*
* INFO (output) INTEGER
* = 0 : successful exit
* .lt. 0: if INFO = -i, the i-th argument had an illegal value
* .gt. 0: overflow would occur when computing the INFO(th)
* element of the solution vector x. This can only occur
* when JOB is supplied as positive and either means
* that a diagonal element of U is very small, or that
* the elements of the right-hand side vector y are very
* large.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param n
* @param a
* @param b
* @param c
* @param d
* @param in
* @param y
* @param tol
* @param info
*
*/
abstract public void slagts(int job, int n, float[] a, float[] b, float[] c, float[] d, int[] in, float[] y, org.netlib.util.floatW tol, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAGTS may be used to solve one of the systems of equations
*
* (T - lambda*I)*x = y or (T - lambda*I)'*x = y,
*
* where T is an n by n tridiagonal matrix, for x, following the
* factorization of (T - lambda*I) as
*
* (T - lambda*I) = P*L*U ,
*
* by routine SLAGTF. The choice of equation to be solved is
* controlled by the argument JOB, and in each case there is an option
* to perturb zero or very small diagonal elements of U, this option
* being intended for use in applications such as inverse iteration.
*
* Arguments
* =========
*
* JOB (input) INTEGER
* Specifies the job to be performed by SLAGTS as follows:
* = 1: The equations (T - lambda*I)x = y are to be solved,
* but diagonal elements of U are not to be perturbed.
* = -1: The equations (T - lambda*I)x = y are to be solved
* and, if overflow would otherwise occur, the diagonal
* elements of U are to be perturbed. See argument TOL
* below.
* = 2: The equations (T - lambda*I)'x = y are to be solved,
* but diagonal elements of U are not to be perturbed.
* = -2: The equations (T - lambda*I)'x = y are to be solved
* and, if overflow would otherwise occur, the diagonal
* elements of U are to be perturbed. See argument TOL
* below.
*
* N (input) INTEGER
* The order of the matrix T.
*
* A (input) REAL array, dimension (N)
* On entry, A must contain the diagonal elements of U as
* returned from SLAGTF.
*
* B (input) REAL array, dimension (N-1)
* On entry, B must contain the first super-diagonal elements of
* U as returned from SLAGTF.
*
* C (input) REAL array, dimension (N-1)
* On entry, C must contain the sub-diagonal elements of L as
* returned from SLAGTF.
*
* D (input) REAL array, dimension (N-2)
* On entry, D must contain the second super-diagonal elements
* of U as returned from SLAGTF.
*
* IN (input) INTEGER array, dimension (N)
* On entry, IN must contain details of the matrix P as returned
* from SLAGTF.
*
* Y (input/output) REAL array, dimension (N)
* On entry, the right hand side vector y.
* On exit, Y is overwritten by the solution vector x.
*
* TOL (input/output) REAL
* On entry, with JOB .lt. 0, TOL should be the minimum
* perturbation to be made to very small diagonal elements of U.
* TOL should normally be chosen as about eps*norm(U), where eps
* is the relative machine precision, but if TOL is supplied as
* non-positive, then it is reset to eps*max( abs( u(i,j) ) ).
* If JOB .gt. 0 then TOL is not referenced.
*
* On exit, TOL is changed as described above, only if TOL is
* non-positive on entry. Otherwise TOL is unchanged.
*
* INFO (output) INTEGER
* = 0 : successful exit
* .lt. 0: if INFO = -i, the i-th argument had an illegal value
* .gt. 0: overflow would occur when computing the INFO(th)
* element of the solution vector x. This can only occur
* when JOB is supplied as positive and either means
* that a diagonal element of U is very small, or that
* the elements of the right-hand side vector y are very
* large.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param n
* @param a
* @param _a_offset
* @param b
* @param _b_offset
* @param c
* @param _c_offset
* @param d
* @param _d_offset
* @param in
* @param _in_offset
* @param y
* @param _y_offset
* @param tol
* @param info
*
*/
abstract public void slagts(int job, int n, float[] a, int _a_offset, float[] b, int _b_offset, float[] c, int _c_offset, float[] d, int _d_offset, int[] in, int _in_offset, float[] y, int _y_offset, org.netlib.util.floatW tol, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAGV2 computes the Generalized Schur factorization of a real 2-by-2
* matrix pencil (A,B) where B is upper triangular. This routine
* computes orthogonal (rotation) matrices given by CSL, SNL and CSR,
* SNR such that
*
* 1) if the pencil (A,B) has two real eigenvalues (include 0/0 or 1/0
* types), then
*
* [ a11 a12 ] := [ CSL SNL ] [ a11 a12 ] [ CSR -SNR ]
* [ 0 a22 ] [ -SNL CSL ] [ a21 a22 ] [ SNR CSR ]
*
* [ b11 b12 ] := [ CSL SNL ] [ b11 b12 ] [ CSR -SNR ]
* [ 0 b22 ] [ -SNL CSL ] [ 0 b22 ] [ SNR CSR ],
*
* 2) if the pencil (A,B) has a pair of complex conjugate eigenvalues,
* then
*
* [ a11 a12 ] := [ CSL SNL ] [ a11 a12 ] [ CSR -SNR ]
* [ a21 a22 ] [ -SNL CSL ] [ a21 a22 ] [ SNR CSR ]
*
* [ b11 0 ] := [ CSL SNL ] [ b11 b12 ] [ CSR -SNR ]
* [ 0 b22 ] [ -SNL CSL ] [ 0 b22 ] [ SNR CSR ]
*
* where b11 >= b22 > 0.
*
*
* Arguments
* =========
*
* A (input/output) REAL array, dimension (LDA, 2)
* On entry, the 2 x 2 matrix A.
* On exit, A is overwritten by the ``A-part'' of the
* generalized Schur form.
*
* LDA (input) INTEGER
* THe leading dimension of the array A. LDA >= 2.
*
* B (input/output) REAL array, dimension (LDB, 2)
* On entry, the upper triangular 2 x 2 matrix B.
* On exit, B is overwritten by the ``B-part'' of the
* generalized Schur form.
*
* LDB (input) INTEGER
* THe leading dimension of the array B. LDB >= 2.
*
* ALPHAR (output) REAL array, dimension (2)
* ALPHAI (output) REAL array, dimension (2)
* BETA (output) REAL array, dimension (2)
* (ALPHAR(k)+i*ALPHAI(k))/BETA(k) are the eigenvalues of the
* pencil (A,B), k=1,2, i = sqrt(-1). Note that BETA(k) may
* be zero.
*
* CSL (output) REAL
* The cosine of the left rotation matrix.
*
* SNL (output) REAL
* The sine of the left rotation matrix.
*
* CSR (output) REAL
* The cosine of the right rotation matrix.
*
* SNR (output) REAL
* The sine of the right rotation matrix.
*
* Further Details
* ===============
*
* Based on contributions by
* Mark Fahey, Department of Mathematics, Univ. of Kentucky, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param a
* @param lda
* @param b
* @param ldb
* @param alphar
* @param alphai
* @param beta
* @param csl
* @param snl
* @param csr
* @param snr
*
*/
abstract public void slagv2(float[] a, int lda, float[] b, int ldb, float[] alphar, float[] alphai, float[] beta, org.netlib.util.floatW csl, org.netlib.util.floatW snl, org.netlib.util.floatW csr, org.netlib.util.floatW snr);
/**
*
* ..
*
* Purpose
* =======
*
* SLAGV2 computes the Generalized Schur factorization of a real 2-by-2
* matrix pencil (A,B) where B is upper triangular. This routine
* computes orthogonal (rotation) matrices given by CSL, SNL and CSR,
* SNR such that
*
* 1) if the pencil (A,B) has two real eigenvalues (include 0/0 or 1/0
* types), then
*
* [ a11 a12 ] := [ CSL SNL ] [ a11 a12 ] [ CSR -SNR ]
* [ 0 a22 ] [ -SNL CSL ] [ a21 a22 ] [ SNR CSR ]
*
* [ b11 b12 ] := [ CSL SNL ] [ b11 b12 ] [ CSR -SNR ]
* [ 0 b22 ] [ -SNL CSL ] [ 0 b22 ] [ SNR CSR ],
*
* 2) if the pencil (A,B) has a pair of complex conjugate eigenvalues,
* then
*
* [ a11 a12 ] := [ CSL SNL ] [ a11 a12 ] [ CSR -SNR ]
* [ a21 a22 ] [ -SNL CSL ] [ a21 a22 ] [ SNR CSR ]
*
* [ b11 0 ] := [ CSL SNL ] [ b11 b12 ] [ CSR -SNR ]
* [ 0 b22 ] [ -SNL CSL ] [ 0 b22 ] [ SNR CSR ]
*
* where b11 >= b22 > 0.
*
*
* Arguments
* =========
*
* A (input/output) REAL array, dimension (LDA, 2)
* On entry, the 2 x 2 matrix A.
* On exit, A is overwritten by the ``A-part'' of the
* generalized Schur form.
*
* LDA (input) INTEGER
* THe leading dimension of the array A. LDA >= 2.
*
* B (input/output) REAL array, dimension (LDB, 2)
* On entry, the upper triangular 2 x 2 matrix B.
* On exit, B is overwritten by the ``B-part'' of the
* generalized Schur form.
*
* LDB (input) INTEGER
* THe leading dimension of the array B. LDB >= 2.
*
* ALPHAR (output) REAL array, dimension (2)
* ALPHAI (output) REAL array, dimension (2)
* BETA (output) REAL array, dimension (2)
* (ALPHAR(k)+i*ALPHAI(k))/BETA(k) are the eigenvalues of the
* pencil (A,B), k=1,2, i = sqrt(-1). Note that BETA(k) may
* be zero.
*
* CSL (output) REAL
* The cosine of the left rotation matrix.
*
* SNL (output) REAL
* The sine of the left rotation matrix.
*
* CSR (output) REAL
* The cosine of the right rotation matrix.
*
* SNR (output) REAL
* The sine of the right rotation matrix.
*
* Further Details
* ===============
*
* Based on contributions by
* Mark Fahey, Department of Mathematics, Univ. of Kentucky, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param alphar
* @param _alphar_offset
* @param alphai
* @param _alphai_offset
* @param beta
* @param _beta_offset
* @param csl
* @param snl
* @param csr
* @param snr
*
*/
abstract public void slagv2(float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, float[] alphar, int _alphar_offset, float[] alphai, int _alphai_offset, float[] beta, int _beta_offset, org.netlib.util.floatW csl, org.netlib.util.floatW snl, org.netlib.util.floatW csr, org.netlib.util.floatW snr);
/**
*
* ..
*
* Purpose
* =======
*
* SLAHQR is an auxiliary routine called by SHSEQR to update the
* eigenvalues and Schur decomposition already computed by SHSEQR, by
* dealing with the Hessenberg submatrix in rows and columns ILO to
* IHI.
*
* Arguments
* =========
*
* WANTT (input) LOGICAL
* = .TRUE. : the full Schur form T is required;
* = .FALSE.: only eigenvalues are required.
*
* WANTZ (input) LOGICAL
* = .TRUE. : the matrix of Schur vectors Z is required;
* = .FALSE.: Schur vectors are not required.
*
* N (input) INTEGER
* The order of the matrix H. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* It is assumed that H is already upper quasi-triangular in
* rows and columns IHI+1:N, and that H(ILO,ILO-1) = 0 (unless
* ILO = 1). SLAHQR works primarily with the Hessenberg
* submatrix in rows and columns ILO to IHI, but applies
* transformations to all of H if WANTT is .TRUE..
* 1 <= ILO <= max(1,IHI); IHI <= N.
*
* H (input/output) REAL array, dimension (LDH,N)
* On entry, the upper Hessenberg matrix H.
* On exit, if INFO is zero and if WANTT is .TRUE., H is upper
* quasi-triangular in rows and columns ILO:IHI, with any
* 2-by-2 diagonal blocks in standard form. If INFO is zero
* and WANTT is .FALSE., the contents of H are unspecified on
* exit. The output state of H if INFO is nonzero is given
* below under the description of INFO.
*
* LDH (input) INTEGER
* The leading dimension of the array H. LDH >= max(1,N).
*
* WR (output) REAL array, dimension (N)
* WI (output) REAL array, dimension (N)
* The real and imaginary parts, respectively, of the computed
* eigenvalues ILO to IHI are stored in the corresponding
* elements of WR and WI. If two eigenvalues are computed as a
* complex conjugate pair, they are stored in consecutive
* elements of WR and WI, say the i-th and (i+1)th, with
* WI(i) > 0 and WI(i+1) < 0. If WANTT is .TRUE., the
* eigenvalues are stored in the same order as on the diagonal
* of the Schur form returned in H, with WR(i) = H(i,i), and, if
* H(i:i+1,i:i+1) is a 2-by-2 diagonal block,
* WI(i) = sqrt(H(i+1,i)*H(i,i+1)) and WI(i+1) = -WI(i).
*
* ILOZ (input) INTEGER
* IHIZ (input) INTEGER
* Specify the rows of Z to which transformations must be
* applied if WANTZ is .TRUE..
* 1 <= ILOZ <= ILO; IHI <= IHIZ <= N.
*
* Z (input/output) REAL array, dimension (LDZ,N)
* If WANTZ is .TRUE., on entry Z must contain the current
* matrix Z of transformations accumulated by SHSEQR, and on
* exit Z has been updated; transformations are applied only to
* the submatrix Z(ILOZ:IHIZ,ILO:IHI).
* If WANTZ is .FALSE., Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* .GT. 0: If INFO = i, SLAHQR failed to compute all the
* eigenvalues ILO to IHI in a total of 30 iterations
* per eigenvalue; elements i+1:ihi of WR and WI
* contain those eigenvalues which have been
* successfully computed.
*
* If INFO .GT. 0 and WANTT is .FALSE., then on exit,
* the remaining unconverged eigenvalues are the
* eigenvalues of the upper Hessenberg matrix rows
* and columns ILO thorugh INFO of the final, output
* value of H.
*
* If INFO .GT. 0 and WANTT is .TRUE., then on exit
* (*) (initial value of H)*U = U*(final value of H)
* where U is an orthognal matrix. The final
* value of H is upper Hessenberg and triangular in
* rows and columns INFO+1 through IHI.
*
* If INFO .GT. 0 and WANTZ is .TRUE., then on exit
* (final value of Z) = (initial value of Z)*U
* where U is the orthogonal matrix in (*)
* (regardless of the value of WANTT.)
*
* Further Details
* ===============
*
* 02-96 Based on modifications by
* David Day, Sandia National Laboratory, USA
*
* 12-04 Further modifications by
* Ralph Byers, University of Kansas, USA
*
* This is a modified version of SLAHQR from LAPACK version 3.0.
* It is (1) more robust against overflow and underflow and
* (2) adopts the more conservative Ahues & Tisseur stopping
* criterion (LAWN 122, 1997).
*
* =========================================================
*
* .. Parameters ..
*
*
* @param wantt
* @param wantz
* @param n
* @param ilo
* @param ihi
* @param h
* @param ldh
* @param wr
* @param wi
* @param iloz
* @param ihiz
* @param z
* @param ldz
* @param info
*
*/
abstract public void slahqr(boolean wantt, boolean wantz, int n, int ilo, int ihi, float[] h, int ldh, float[] wr, float[] wi, int iloz, int ihiz, float[] z, int ldz, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAHQR is an auxiliary routine called by SHSEQR to update the
* eigenvalues and Schur decomposition already computed by SHSEQR, by
* dealing with the Hessenberg submatrix in rows and columns ILO to
* IHI.
*
* Arguments
* =========
*
* WANTT (input) LOGICAL
* = .TRUE. : the full Schur form T is required;
* = .FALSE.: only eigenvalues are required.
*
* WANTZ (input) LOGICAL
* = .TRUE. : the matrix of Schur vectors Z is required;
* = .FALSE.: Schur vectors are not required.
*
* N (input) INTEGER
* The order of the matrix H. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* It is assumed that H is already upper quasi-triangular in
* rows and columns IHI+1:N, and that H(ILO,ILO-1) = 0 (unless
* ILO = 1). SLAHQR works primarily with the Hessenberg
* submatrix in rows and columns ILO to IHI, but applies
* transformations to all of H if WANTT is .TRUE..
* 1 <= ILO <= max(1,IHI); IHI <= N.
*
* H (input/output) REAL array, dimension (LDH,N)
* On entry, the upper Hessenberg matrix H.
* On exit, if INFO is zero and if WANTT is .TRUE., H is upper
* quasi-triangular in rows and columns ILO:IHI, with any
* 2-by-2 diagonal blocks in standard form. If INFO is zero
* and WANTT is .FALSE., the contents of H are unspecified on
* exit. The output state of H if INFO is nonzero is given
* below under the description of INFO.
*
* LDH (input) INTEGER
* The leading dimension of the array H. LDH >= max(1,N).
*
* WR (output) REAL array, dimension (N)
* WI (output) REAL array, dimension (N)
* The real and imaginary parts, respectively, of the computed
* eigenvalues ILO to IHI are stored in the corresponding
* elements of WR and WI. If two eigenvalues are computed as a
* complex conjugate pair, they are stored in consecutive
* elements of WR and WI, say the i-th and (i+1)th, with
* WI(i) > 0 and WI(i+1) < 0. If WANTT is .TRUE., the
* eigenvalues are stored in the same order as on the diagonal
* of the Schur form returned in H, with WR(i) = H(i,i), and, if
* H(i:i+1,i:i+1) is a 2-by-2 diagonal block,
* WI(i) = sqrt(H(i+1,i)*H(i,i+1)) and WI(i+1) = -WI(i).
*
* ILOZ (input) INTEGER
* IHIZ (input) INTEGER
* Specify the rows of Z to which transformations must be
* applied if WANTZ is .TRUE..
* 1 <= ILOZ <= ILO; IHI <= IHIZ <= N.
*
* Z (input/output) REAL array, dimension (LDZ,N)
* If WANTZ is .TRUE., on entry Z must contain the current
* matrix Z of transformations accumulated by SHSEQR, and on
* exit Z has been updated; transformations are applied only to
* the submatrix Z(ILOZ:IHIZ,ILO:IHI).
* If WANTZ is .FALSE., Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* .GT. 0: If INFO = i, SLAHQR failed to compute all the
* eigenvalues ILO to IHI in a total of 30 iterations
* per eigenvalue; elements i+1:ihi of WR and WI
* contain those eigenvalues which have been
* successfully computed.
*
* If INFO .GT. 0 and WANTT is .FALSE., then on exit,
* the remaining unconverged eigenvalues are the
* eigenvalues of the upper Hessenberg matrix rows
* and columns ILO thorugh INFO of the final, output
* value of H.
*
* If INFO .GT. 0 and WANTT is .TRUE., then on exit
* (*) (initial value of H)*U = U*(final value of H)
* where U is an orthognal matrix. The final
* value of H is upper Hessenberg and triangular in
* rows and columns INFO+1 through IHI.
*
* If INFO .GT. 0 and WANTZ is .TRUE., then on exit
* (final value of Z) = (initial value of Z)*U
* where U is the orthogonal matrix in (*)
* (regardless of the value of WANTT.)
*
* Further Details
* ===============
*
* 02-96 Based on modifications by
* David Day, Sandia National Laboratory, USA
*
* 12-04 Further modifications by
* Ralph Byers, University of Kansas, USA
*
* This is a modified version of SLAHQR from LAPACK version 3.0.
* It is (1) more robust against overflow and underflow and
* (2) adopts the more conservative Ahues & Tisseur stopping
* criterion (LAWN 122, 1997).
*
* =========================================================
*
* .. Parameters ..
*
*
* @param wantt
* @param wantz
* @param n
* @param ilo
* @param ihi
* @param h
* @param _h_offset
* @param ldh
* @param wr
* @param _wr_offset
* @param wi
* @param _wi_offset
* @param iloz
* @param ihiz
* @param z
* @param _z_offset
* @param ldz
* @param info
*
*/
abstract public void slahqr(boolean wantt, boolean wantz, int n, int ilo, int ihi, float[] h, int _h_offset, int ldh, float[] wr, int _wr_offset, float[] wi, int _wi_offset, int iloz, int ihiz, float[] z, int _z_offset, int ldz, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAHR2 reduces the first NB columns of A real general n-BY-(n-k+1)
* matrix A so that elements below the k-th subdiagonal are zero. The
* reduction is performed by an orthogonal similarity transformation
* Q' * A * Q. The routine returns the matrices V and T which determine
* Q as a block reflector I - V*T*V', and also the matrix Y = A * V * T.
*
* This is an auxiliary routine called by SGEHRD.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A.
*
* K (input) INTEGER
* The offset for the reduction. Elements below the k-th
* subdiagonal in the first NB columns are reduced to zero.
* K < N.
*
* NB (input) INTEGER
* The number of columns to be reduced.
*
* A (input/output) REAL array, dimension (LDA,N-K+1)
* On entry, the n-by-(n-k+1) general matrix A.
* On exit, the elements on and above the k-th subdiagonal in
* the first NB columns are overwritten with the corresponding
* elements of the reduced matrix; the elements below the k-th
* subdiagonal, with the array TAU, represent the matrix Q as a
* product of elementary reflectors. The other columns of A are
* unchanged. See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* TAU (output) REAL array, dimension (NB)
* The scalar factors of the elementary reflectors. See Further
* Details.
*
* T (output) REAL array, dimension (LDT,NB)
* The upper triangular matrix T.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= NB.
*
* Y (output) REAL array, dimension (LDY,NB)
* The n-by-nb matrix Y.
*
* LDY (input) INTEGER
* The leading dimension of the array Y. LDY >= N.
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of nb elementary reflectors
*
* Q = H(1) H(2) . . . H(nb).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i+k-1) = 0, v(i+k) = 1; v(i+k+1:n) is stored on exit in
* A(i+k+1:n,i), and tau in TAU(i).
*
* The elements of the vectors v together form the (n-k+1)-by-nb matrix
* V which is needed, with T and Y, to apply the transformation to the
* unreduced part of the matrix, using an update of the form:
* A := (I - V*T*V') * (A - Y*V').
*
* The contents of A on exit are illustrated by the following example
* with n = 7, k = 3 and nb = 2:
*
* ( a a a a a )
* ( a a a a a )
* ( a a a a a )
* ( h h a a a )
* ( v1 h a a a )
* ( v1 v2 a a a )
* ( v1 v2 a a a )
*
* where a denotes an element of the original matrix A, h denotes a
* modified element of the upper Hessenberg matrix H, and vi denotes an
* element of the vector defining H(i).
*
* This file is a slight modification of LAPACK-3.0's SLAHRD
* incorporating improvements proposed by Quintana-Orti and Van de
* Gejin. Note that the entries of A(1:K,2:NB) differ from those
* returned by the original LAPACK routine. This function is
* not backward compatible with LAPACK3.0.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param k
* @param nb
* @param a
* @param lda
* @param tau
* @param t
* @param ldt
* @param y
* @param ldy
*
*/
abstract public void slahr2(int n, int k, int nb, float[] a, int lda, float[] tau, float[] t, int ldt, float[] y, int ldy);
/**
*
* ..
*
* Purpose
* =======
*
* SLAHR2 reduces the first NB columns of A real general n-BY-(n-k+1)
* matrix A so that elements below the k-th subdiagonal are zero. The
* reduction is performed by an orthogonal similarity transformation
* Q' * A * Q. The routine returns the matrices V and T which determine
* Q as a block reflector I - V*T*V', and also the matrix Y = A * V * T.
*
* This is an auxiliary routine called by SGEHRD.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A.
*
* K (input) INTEGER
* The offset for the reduction. Elements below the k-th
* subdiagonal in the first NB columns are reduced to zero.
* K < N.
*
* NB (input) INTEGER
* The number of columns to be reduced.
*
* A (input/output) REAL array, dimension (LDA,N-K+1)
* On entry, the n-by-(n-k+1) general matrix A.
* On exit, the elements on and above the k-th subdiagonal in
* the first NB columns are overwritten with the corresponding
* elements of the reduced matrix; the elements below the k-th
* subdiagonal, with the array TAU, represent the matrix Q as a
* product of elementary reflectors. The other columns of A are
* unchanged. See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* TAU (output) REAL array, dimension (NB)
* The scalar factors of the elementary reflectors. See Further
* Details.
*
* T (output) REAL array, dimension (LDT,NB)
* The upper triangular matrix T.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= NB.
*
* Y (output) REAL array, dimension (LDY,NB)
* The n-by-nb matrix Y.
*
* LDY (input) INTEGER
* The leading dimension of the array Y. LDY >= N.
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of nb elementary reflectors
*
* Q = H(1) H(2) . . . H(nb).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i+k-1) = 0, v(i+k) = 1; v(i+k+1:n) is stored on exit in
* A(i+k+1:n,i), and tau in TAU(i).
*
* The elements of the vectors v together form the (n-k+1)-by-nb matrix
* V which is needed, with T and Y, to apply the transformation to the
* unreduced part of the matrix, using an update of the form:
* A := (I - V*T*V') * (A - Y*V').
*
* The contents of A on exit are illustrated by the following example
* with n = 7, k = 3 and nb = 2:
*
* ( a a a a a )
* ( a a a a a )
* ( a a a a a )
* ( h h a a a )
* ( v1 h a a a )
* ( v1 v2 a a a )
* ( v1 v2 a a a )
*
* where a denotes an element of the original matrix A, h denotes a
* modified element of the upper Hessenberg matrix H, and vi denotes an
* element of the vector defining H(i).
*
* This file is a slight modification of LAPACK-3.0's SLAHRD
* incorporating improvements proposed by Quintana-Orti and Van de
* Gejin. Note that the entries of A(1:K,2:NB) differ from those
* returned by the original LAPACK routine. This function is
* not backward compatible with LAPACK3.0.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param k
* @param nb
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param t
* @param _t_offset
* @param ldt
* @param y
* @param _y_offset
* @param ldy
*
*/
abstract public void slahr2(int n, int k, int nb, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] t, int _t_offset, int ldt, float[] y, int _y_offset, int ldy);
/**
*
* ..
*
* Purpose
* =======
*
* SLAHRD reduces the first NB columns of a real general n-by-(n-k+1)
* matrix A so that elements below the k-th subdiagonal are zero. The
* reduction is performed by an orthogonal similarity transformation
* Q' * A * Q. The routine returns the matrices V and T which determine
* Q as a block reflector I - V*T*V', and also the matrix Y = A * V * T.
*
* This is an OBSOLETE auxiliary routine.
* This routine will be 'deprecated' in a future release.
* Please use the new routine SLAHR2 instead.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A.
*
* K (input) INTEGER
* The offset for the reduction. Elements below the k-th
* subdiagonal in the first NB columns are reduced to zero.
*
* NB (input) INTEGER
* The number of columns to be reduced.
*
* A (input/output) REAL array, dimension (LDA,N-K+1)
* On entry, the n-by-(n-k+1) general matrix A.
* On exit, the elements on and above the k-th subdiagonal in
* the first NB columns are overwritten with the corresponding
* elements of the reduced matrix; the elements below the k-th
* subdiagonal, with the array TAU, represent the matrix Q as a
* product of elementary reflectors. The other columns of A are
* unchanged. See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* TAU (output) REAL array, dimension (NB)
* The scalar factors of the elementary reflectors. See Further
* Details.
*
* T (output) REAL array, dimension (LDT,NB)
* The upper triangular matrix T.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= NB.
*
* Y (output) REAL array, dimension (LDY,NB)
* The n-by-nb matrix Y.
*
* LDY (input) INTEGER
* The leading dimension of the array Y. LDY >= N.
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of nb elementary reflectors
*
* Q = H(1) H(2) . . . H(nb).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i+k-1) = 0, v(i+k) = 1; v(i+k+1:n) is stored on exit in
* A(i+k+1:n,i), and tau in TAU(i).
*
* The elements of the vectors v together form the (n-k+1)-by-nb matrix
* V which is needed, with T and Y, to apply the transformation to the
* unreduced part of the matrix, using an update of the form:
* A := (I - V*T*V') * (A - Y*V').
*
* The contents of A on exit are illustrated by the following example
* with n = 7, k = 3 and nb = 2:
*
* ( a h a a a )
* ( a h a a a )
* ( a h a a a )
* ( h h a a a )
* ( v1 h a a a )
* ( v1 v2 a a a )
* ( v1 v2 a a a )
*
* where a denotes an element of the original matrix A, h denotes a
* modified element of the upper Hessenberg matrix H, and vi denotes an
* element of the vector defining H(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param k
* @param nb
* @param a
* @param lda
* @param tau
* @param t
* @param ldt
* @param y
* @param ldy
*
*/
abstract public void slahrd(int n, int k, int nb, float[] a, int lda, float[] tau, float[] t, int ldt, float[] y, int ldy);
/**
*
* ..
*
* Purpose
* =======
*
* SLAHRD reduces the first NB columns of a real general n-by-(n-k+1)
* matrix A so that elements below the k-th subdiagonal are zero. The
* reduction is performed by an orthogonal similarity transformation
* Q' * A * Q. The routine returns the matrices V and T which determine
* Q as a block reflector I - V*T*V', and also the matrix Y = A * V * T.
*
* This is an OBSOLETE auxiliary routine.
* This routine will be 'deprecated' in a future release.
* Please use the new routine SLAHR2 instead.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A.
*
* K (input) INTEGER
* The offset for the reduction. Elements below the k-th
* subdiagonal in the first NB columns are reduced to zero.
*
* NB (input) INTEGER
* The number of columns to be reduced.
*
* A (input/output) REAL array, dimension (LDA,N-K+1)
* On entry, the n-by-(n-k+1) general matrix A.
* On exit, the elements on and above the k-th subdiagonal in
* the first NB columns are overwritten with the corresponding
* elements of the reduced matrix; the elements below the k-th
* subdiagonal, with the array TAU, represent the matrix Q as a
* product of elementary reflectors. The other columns of A are
* unchanged. See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* TAU (output) REAL array, dimension (NB)
* The scalar factors of the elementary reflectors. See Further
* Details.
*
* T (output) REAL array, dimension (LDT,NB)
* The upper triangular matrix T.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= NB.
*
* Y (output) REAL array, dimension (LDY,NB)
* The n-by-nb matrix Y.
*
* LDY (input) INTEGER
* The leading dimension of the array Y. LDY >= N.
*
* Further Details
* ===============
*
* The matrix Q is represented as a product of nb elementary reflectors
*
* Q = H(1) H(2) . . . H(nb).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i+k-1) = 0, v(i+k) = 1; v(i+k+1:n) is stored on exit in
* A(i+k+1:n,i), and tau in TAU(i).
*
* The elements of the vectors v together form the (n-k+1)-by-nb matrix
* V which is needed, with T and Y, to apply the transformation to the
* unreduced part of the matrix, using an update of the form:
* A := (I - V*T*V') * (A - Y*V').
*
* The contents of A on exit are illustrated by the following example
* with n = 7, k = 3 and nb = 2:
*
* ( a h a a a )
* ( a h a a a )
* ( a h a a a )
* ( h h a a a )
* ( v1 h a a a )
* ( v1 v2 a a a )
* ( v1 v2 a a a )
*
* where a denotes an element of the original matrix A, h denotes a
* modified element of the upper Hessenberg matrix H, and vi denotes an
* element of the vector defining H(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param k
* @param nb
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param t
* @param _t_offset
* @param ldt
* @param y
* @param _y_offset
* @param ldy
*
*/
abstract public void slahrd(int n, int k, int nb, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] t, int _t_offset, int ldt, float[] y, int _y_offset, int ldy);
/**
*
* ..
*
* Purpose
* =======
*
* SLAIC1 applies one step of incremental condition estimation in
* its simplest version:
*
* Let x, twonorm(x) = 1, be an approximate singular vector of an j-by-j
* lower triangular matrix L, such that
* twonorm(L*x) = sest
* Then SLAIC1 computes sestpr, s, c such that
* the vector
* [ s*x ]
* xhat = [ c ]
* is an approximate singular vector of
* [ L 0 ]
* Lhat = [ w' gamma ]
* in the sense that
* twonorm(Lhat*xhat) = sestpr.
*
* Depending on JOB, an estimate for the largest or smallest singular
* value is computed.
*
* Note that [s c]' and sestpr**2 is an eigenpair of the system
*
* diag(sest*sest, 0) + [alpha gamma] * [ alpha ]
* [ gamma ]
*
* where alpha = x'*w.
*
* Arguments
* =========
*
* JOB (input) INTEGER
* = 1: an estimate for the largest singular value is computed.
* = 2: an estimate for the smallest singular value is computed.
*
* J (input) INTEGER
* Length of X and W
*
* X (input) REAL array, dimension (J)
* The j-vector x.
*
* SEST (input) REAL
* Estimated singular value of j by j matrix L
*
* W (input) REAL array, dimension (J)
* The j-vector w.
*
* GAMMA (input) REAL
* The diagonal element gamma.
*
* SESTPR (output) REAL
* Estimated singular value of (j+1) by (j+1) matrix Lhat.
*
* S (output) REAL
* Sine needed in forming xhat.
*
* C (output) REAL
* Cosine needed in forming xhat.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param j
* @param x
* @param sest
* @param w
* @param gamma
* @param sestpr
* @param s
* @param c
*
*/
abstract public void slaic1(int job, int j, float[] x, float sest, float[] w, float gamma, org.netlib.util.floatW sestpr, org.netlib.util.floatW s, org.netlib.util.floatW c);
/**
*
* ..
*
* Purpose
* =======
*
* SLAIC1 applies one step of incremental condition estimation in
* its simplest version:
*
* Let x, twonorm(x) = 1, be an approximate singular vector of an j-by-j
* lower triangular matrix L, such that
* twonorm(L*x) = sest
* Then SLAIC1 computes sestpr, s, c such that
* the vector
* [ s*x ]
* xhat = [ c ]
* is an approximate singular vector of
* [ L 0 ]
* Lhat = [ w' gamma ]
* in the sense that
* twonorm(Lhat*xhat) = sestpr.
*
* Depending on JOB, an estimate for the largest or smallest singular
* value is computed.
*
* Note that [s c]' and sestpr**2 is an eigenpair of the system
*
* diag(sest*sest, 0) + [alpha gamma] * [ alpha ]
* [ gamma ]
*
* where alpha = x'*w.
*
* Arguments
* =========
*
* JOB (input) INTEGER
* = 1: an estimate for the largest singular value is computed.
* = 2: an estimate for the smallest singular value is computed.
*
* J (input) INTEGER
* Length of X and W
*
* X (input) REAL array, dimension (J)
* The j-vector x.
*
* SEST (input) REAL
* Estimated singular value of j by j matrix L
*
* W (input) REAL array, dimension (J)
* The j-vector w.
*
* GAMMA (input) REAL
* The diagonal element gamma.
*
* SESTPR (output) REAL
* Estimated singular value of (j+1) by (j+1) matrix Lhat.
*
* S (output) REAL
* Sine needed in forming xhat.
*
* C (output) REAL
* Cosine needed in forming xhat.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param j
* @param x
* @param _x_offset
* @param sest
* @param w
* @param _w_offset
* @param gamma
* @param sestpr
* @param s
* @param c
*
*/
abstract public void slaic1(int job, int j, float[] x, int _x_offset, float sest, float[] w, int _w_offset, float gamma, org.netlib.util.floatW sestpr, org.netlib.util.floatW s, org.netlib.util.floatW c);
/**
*
* ..
*
* Purpose
* =======
*
* This routine is not for general use. It exists solely to avoid
* over-optimization in SISNAN.
*
* SLAISNAN checks for NaNs by comparing its two arguments for
* inequality. NaN is the only floating-point value where NaN != NaN
* returns .TRUE. To check for NaNs, pass the same variable as both
* arguments.
*
* Strictly speaking, Fortran does not allow aliasing of function
* arguments. So a compiler must assume that the two arguments are
* not the same variable, and the test will not be optimized away.
* Interprocedural or whole-program optimization may delete this
* test. The ISNAN functions will be replaced by the correct
* Fortran 03 intrinsic once the intrinsic is widely available.
*
* Arguments
* =========
*
* SIN1 (input) REAL
* SIN2 (input) REAL
* Two numbers to compare for inequality.
*
* =====================================================================
*
* .. Executable Statements ..
*
*
* @param sin1
* @param sin2
* @return
*/
abstract public boolean slaisnan(float sin1, float sin2);
/**
*
* ..
*
* Purpose
* =======
*
* SLALN2 solves a system of the form (ca A - w D ) X = s B
* or (ca A' - w D) X = s B with possible scaling ("s") and
* perturbation of A. (A' means A-transpose.)
*
* A is an NA x NA real matrix, ca is a real scalar, D is an NA x NA
* real diagonal matrix, w is a real or complex value, and X and B are
* NA x 1 matrices -- real if w is real, complex if w is complex. NA
* may be 1 or 2.
*
* If w is complex, X and B are represented as NA x 2 matrices,
* the first column of each being the real part and the second
* being the imaginary part.
*
* "s" is a scaling factor (.LE. 1), computed by SLALN2, which is
* so chosen that X can be computed without overflow. X is further
* scaled if necessary to assure that norm(ca A - w D)*norm(X) is less
* than overflow.
*
* If both singular values of (ca A - w D) are less than SMIN,
* SMIN*identity will be used instead of (ca A - w D). If only one
* singular value is less than SMIN, one element of (ca A - w D) will be
* perturbed enough to make the smallest singular value roughly SMIN.
* If both singular values are at least SMIN, (ca A - w D) will not be
* perturbed. In any case, the perturbation will be at most some small
* multiple of max( SMIN, ulp*norm(ca A - w D) ). The singular values
* are computed by infinity-norm approximations, and thus will only be
* correct to a factor of 2 or so.
*
* Note: all input quantities are assumed to be smaller than overflow
* by a reasonable factor. (See BIGNUM.)
*
* Arguments
* ==========
*
* LTRANS (input) LOGICAL
* =.TRUE.: A-transpose will be used.
* =.FALSE.: A will be used (not transposed.)
*
* NA (input) INTEGER
* The size of the matrix A. It may (only) be 1 or 2.
*
* NW (input) INTEGER
* 1 if "w" is real, 2 if "w" is complex. It may only be 1
* or 2.
*
* SMIN (input) REAL
* The desired lower bound on the singular values of A. This
* should be a safe distance away from underflow or overflow,
* say, between (underflow/machine precision) and (machine
* precision * overflow ). (See BIGNUM and ULP.)
*
* CA (input) REAL
* The coefficient c, which A is multiplied by.
*
* A (input) REAL array, dimension (LDA,NA)
* The NA x NA matrix A.
*
* LDA (input) INTEGER
* The leading dimension of A. It must be at least NA.
*
* D1 (input) REAL
* The 1,1 element in the diagonal matrix D.
*
* D2 (input) REAL
* The 2,2 element in the diagonal matrix D. Not used if NW=1.
*
* B (input) REAL array, dimension (LDB,NW)
* The NA x NW matrix B (right-hand side). If NW=2 ("w" is
* complex), column 1 contains the real part of B and column 2
* contains the imaginary part.
*
* LDB (input) INTEGER
* The leading dimension of B. It must be at least NA.
*
* WR (input) REAL
* The real part of the scalar "w".
*
* WI (input) REAL
* The imaginary part of the scalar "w". Not used if NW=1.
*
* X (output) REAL array, dimension (LDX,NW)
* The NA x NW matrix X (unknowns), as computed by SLALN2.
* If NW=2 ("w" is complex), on exit, column 1 will contain
* the real part of X and column 2 will contain the imaginary
* part.
*
* LDX (input) INTEGER
* The leading dimension of X. It must be at least NA.
*
* SCALE (output) REAL
* The scale factor that B must be multiplied by to insure
* that overflow does not occur when computing X. Thus,
* (ca A - w D) X will be SCALE*B, not B (ignoring
* perturbations of A.) It will be at most 1.
*
* XNORM (output) REAL
* The infinity-norm of X, when X is regarded as an NA x NW
* real matrix.
*
* INFO (output) INTEGER
* An error flag. It will be set to zero if no error occurs,
* a negative number if an argument is in error, or a positive
* number if ca A - w D had to be perturbed.
* The possible values are:
* = 0: No error occurred, and (ca A - w D) did not have to be
* perturbed.
* = 1: (ca A - w D) had to be perturbed to make its smallest
* (or only) singular value greater than SMIN.
* NOTE: In the interests of speed, this routine does not
* check the inputs for errors.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param ltrans
* @param na
* @param nw
* @param smin
* @param ca
* @param a
* @param lda
* @param d1
* @param d2
* @param b
* @param ldb
* @param wr
* @param wi
* @param x
* @param ldx
* @param scale
* @param xnorm
* @param info
*
*/
abstract public void slaln2(boolean ltrans, int na, int nw, float smin, float ca, float[] a, int lda, float d1, float d2, float[] b, int ldb, float wr, float wi, float[] x, int ldx, org.netlib.util.floatW scale, org.netlib.util.floatW xnorm, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLALN2 solves a system of the form (ca A - w D ) X = s B
* or (ca A' - w D) X = s B with possible scaling ("s") and
* perturbation of A. (A' means A-transpose.)
*
* A is an NA x NA real matrix, ca is a real scalar, D is an NA x NA
* real diagonal matrix, w is a real or complex value, and X and B are
* NA x 1 matrices -- real if w is real, complex if w is complex. NA
* may be 1 or 2.
*
* If w is complex, X and B are represented as NA x 2 matrices,
* the first column of each being the real part and the second
* being the imaginary part.
*
* "s" is a scaling factor (.LE. 1), computed by SLALN2, which is
* so chosen that X can be computed without overflow. X is further
* scaled if necessary to assure that norm(ca A - w D)*norm(X) is less
* than overflow.
*
* If both singular values of (ca A - w D) are less than SMIN,
* SMIN*identity will be used instead of (ca A - w D). If only one
* singular value is less than SMIN, one element of (ca A - w D) will be
* perturbed enough to make the smallest singular value roughly SMIN.
* If both singular values are at least SMIN, (ca A - w D) will not be
* perturbed. In any case, the perturbation will be at most some small
* multiple of max( SMIN, ulp*norm(ca A - w D) ). The singular values
* are computed by infinity-norm approximations, and thus will only be
* correct to a factor of 2 or so.
*
* Note: all input quantities are assumed to be smaller than overflow
* by a reasonable factor. (See BIGNUM.)
*
* Arguments
* ==========
*
* LTRANS (input) LOGICAL
* =.TRUE.: A-transpose will be used.
* =.FALSE.: A will be used (not transposed.)
*
* NA (input) INTEGER
* The size of the matrix A. It may (only) be 1 or 2.
*
* NW (input) INTEGER
* 1 if "w" is real, 2 if "w" is complex. It may only be 1
* or 2.
*
* SMIN (input) REAL
* The desired lower bound on the singular values of A. This
* should be a safe distance away from underflow or overflow,
* say, between (underflow/machine precision) and (machine
* precision * overflow ). (See BIGNUM and ULP.)
*
* CA (input) REAL
* The coefficient c, which A is multiplied by.
*
* A (input) REAL array, dimension (LDA,NA)
* The NA x NA matrix A.
*
* LDA (input) INTEGER
* The leading dimension of A. It must be at least NA.
*
* D1 (input) REAL
* The 1,1 element in the diagonal matrix D.
*
* D2 (input) REAL
* The 2,2 element in the diagonal matrix D. Not used if NW=1.
*
* B (input) REAL array, dimension (LDB,NW)
* The NA x NW matrix B (right-hand side). If NW=2 ("w" is
* complex), column 1 contains the real part of B and column 2
* contains the imaginary part.
*
* LDB (input) INTEGER
* The leading dimension of B. It must be at least NA.
*
* WR (input) REAL
* The real part of the scalar "w".
*
* WI (input) REAL
* The imaginary part of the scalar "w". Not used if NW=1.
*
* X (output) REAL array, dimension (LDX,NW)
* The NA x NW matrix X (unknowns), as computed by SLALN2.
* If NW=2 ("w" is complex), on exit, column 1 will contain
* the real part of X and column 2 will contain the imaginary
* part.
*
* LDX (input) INTEGER
* The leading dimension of X. It must be at least NA.
*
* SCALE (output) REAL
* The scale factor that B must be multiplied by to insure
* that overflow does not occur when computing X. Thus,
* (ca A - w D) X will be SCALE*B, not B (ignoring
* perturbations of A.) It will be at most 1.
*
* XNORM (output) REAL
* The infinity-norm of X, when X is regarded as an NA x NW
* real matrix.
*
* INFO (output) INTEGER
* An error flag. It will be set to zero if no error occurs,
* a negative number if an argument is in error, or a positive
* number if ca A - w D had to be perturbed.
* The possible values are:
* = 0: No error occurred, and (ca A - w D) did not have to be
* perturbed.
* = 1: (ca A - w D) had to be perturbed to make its smallest
* (or only) singular value greater than SMIN.
* NOTE: In the interests of speed, this routine does not
* check the inputs for errors.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param ltrans
* @param na
* @param nw
* @param smin
* @param ca
* @param a
* @param _a_offset
* @param lda
* @param d1
* @param d2
* @param b
* @param _b_offset
* @param ldb
* @param wr
* @param wi
* @param x
* @param _x_offset
* @param ldx
* @param scale
* @param xnorm
* @param info
*
*/
abstract public void slaln2(boolean ltrans, int na, int nw, float smin, float ca, float[] a, int _a_offset, int lda, float d1, float d2, float[] b, int _b_offset, int ldb, float wr, float wi, float[] x, int _x_offset, int ldx, org.netlib.util.floatW scale, org.netlib.util.floatW xnorm, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLALS0 applies back the multiplying factors of either the left or the
* right singular vector matrix of a diagonal matrix appended by a row
* to the right hand side matrix B in solving the least squares problem
* using the divide-and-conquer SVD approach.
*
* For the left singular vector matrix, three types of orthogonal
* matrices are involved:
*
* (1L) Givens rotations: the number of such rotations is GIVPTR; the
* pairs of columns/rows they were applied to are stored in GIVCOL;
* and the C- and S-values of these rotations are stored in GIVNUM.
*
* (2L) Permutation. The (NL+1)-st row of B is to be moved to the first
* row, and for J=2:N, PERM(J)-th row of B is to be moved to the
* J-th row.
*
* (3L) The left singular vector matrix of the remaining matrix.
*
* For the right singular vector matrix, four types of orthogonal
* matrices are involved:
*
* (1R) The right singular vector matrix of the remaining matrix.
*
* (2R) If SQRE = 1, one extra Givens rotation to generate the right
* null space.
*
* (3R) The inverse transformation of (2L).
*
* (4R) The inverse transformation of (1L).
*
* Arguments
* =========
*
* ICOMPQ (input) INTEGER
* Specifies whether singular vectors are to be computed in
* factored form:
* = 0: Left singular vector matrix.
* = 1: Right singular vector matrix.
*
* NL (input) INTEGER
* The row dimension of the upper block. NL >= 1.
*
* NR (input) INTEGER
* The row dimension of the lower block. NR >= 1.
*
* SQRE (input) INTEGER
* = 0: the lower block is an NR-by-NR square matrix.
* = 1: the lower block is an NR-by-(NR+1) rectangular matrix.
*
* The bidiagonal matrix has row dimension N = NL + NR + 1,
* and column dimension M = N + SQRE.
*
* NRHS (input) INTEGER
* The number of columns of B and BX. NRHS must be at least 1.
*
* B (input/output) REAL array, dimension ( LDB, NRHS )
* On input, B contains the right hand sides of the least
* squares problem in rows 1 through M. On output, B contains
* the solution X in rows 1 through N.
*
* LDB (input) INTEGER
* The leading dimension of B. LDB must be at least
* max(1,MAX( M, N ) ).
*
* BX (workspace) REAL array, dimension ( LDBX, NRHS )
*
* LDBX (input) INTEGER
* The leading dimension of BX.
*
* PERM (input) INTEGER array, dimension ( N )
* The permutations (from deflation and sorting) applied
* to the two blocks.
*
* GIVPTR (input) INTEGER
* The number of Givens rotations which took place in this
* subproblem.
*
* GIVCOL (input) INTEGER array, dimension ( LDGCOL, 2 )
* Each pair of numbers indicates a pair of rows/columns
* involved in a Givens rotation.
*
* LDGCOL (input) INTEGER
* The leading dimension of GIVCOL, must be at least N.
*
* GIVNUM (input) REAL array, dimension ( LDGNUM, 2 )
* Each number indicates the C or S value used in the
* corresponding Givens rotation.
*
* LDGNUM (input) INTEGER
* The leading dimension of arrays DIFR, POLES and
* GIVNUM, must be at least K.
*
* POLES (input) REAL array, dimension ( LDGNUM, 2 )
* On entry, POLES(1:K, 1) contains the new singular
* values obtained from solving the secular equation, and
* POLES(1:K, 2) is an array containing the poles in the secular
* equation.
*
* DIFL (input) REAL array, dimension ( K ).
* On entry, DIFL(I) is the distance between I-th updated
* (undeflated) singular value and the I-th (undeflated) old
* singular value.
*
* DIFR (input) REAL array, dimension ( LDGNUM, 2 ).
* On entry, DIFR(I, 1) contains the distances between I-th
* updated (undeflated) singular value and the I+1-th
* (undeflated) old singular value. And DIFR(I, 2) is the
* normalizing factor for the I-th right singular vector.
*
* Z (input) REAL array, dimension ( K )
* Contain the components of the deflation-adjusted updating row
* vector.
*
* K (input) INTEGER
* Contains the dimension of the non-deflated matrix,
* This is the order of the related secular equation. 1 <= K <=N.
*
* C (input) REAL
* C contains garbage if SQRE =0 and the C-value of a Givens
* rotation related to the right null space if SQRE = 1.
*
* S (input) REAL
* S contains garbage if SQRE =0 and the S-value of a Givens
* rotation related to the right null space if SQRE = 1.
*
* WORK (workspace) REAL array, dimension ( K )
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Ren-Cang Li, Computer Science Division, University of
* California at Berkeley, USA
* Osni Marques, LBNL/NERSC, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param nl
* @param nr
* @param sqre
* @param nrhs
* @param b
* @param ldb
* @param bx
* @param ldbx
* @param perm
* @param givptr
* @param givcol
* @param ldgcol
* @param givnum
* @param ldgnum
* @param poles
* @param difl
* @param difr
* @param z
* @param k
* @param c
* @param s
* @param work
* @param info
*
*/
abstract public void slals0(int icompq, int nl, int nr, int sqre, int nrhs, float[] b, int ldb, float[] bx, int ldbx, int[] perm, int givptr, int[] givcol, int ldgcol, float[] givnum, int ldgnum, float[] poles, float[] difl, float[] difr, float[] z, int k, float c, float s, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLALS0 applies back the multiplying factors of either the left or the
* right singular vector matrix of a diagonal matrix appended by a row
* to the right hand side matrix B in solving the least squares problem
* using the divide-and-conquer SVD approach.
*
* For the left singular vector matrix, three types of orthogonal
* matrices are involved:
*
* (1L) Givens rotations: the number of such rotations is GIVPTR; the
* pairs of columns/rows they were applied to are stored in GIVCOL;
* and the C- and S-values of these rotations are stored in GIVNUM.
*
* (2L) Permutation. The (NL+1)-st row of B is to be moved to the first
* row, and for J=2:N, PERM(J)-th row of B is to be moved to the
* J-th row.
*
* (3L) The left singular vector matrix of the remaining matrix.
*
* For the right singular vector matrix, four types of orthogonal
* matrices are involved:
*
* (1R) The right singular vector matrix of the remaining matrix.
*
* (2R) If SQRE = 1, one extra Givens rotation to generate the right
* null space.
*
* (3R) The inverse transformation of (2L).
*
* (4R) The inverse transformation of (1L).
*
* Arguments
* =========
*
* ICOMPQ (input) INTEGER
* Specifies whether singular vectors are to be computed in
* factored form:
* = 0: Left singular vector matrix.
* = 1: Right singular vector matrix.
*
* NL (input) INTEGER
* The row dimension of the upper block. NL >= 1.
*
* NR (input) INTEGER
* The row dimension of the lower block. NR >= 1.
*
* SQRE (input) INTEGER
* = 0: the lower block is an NR-by-NR square matrix.
* = 1: the lower block is an NR-by-(NR+1) rectangular matrix.
*
* The bidiagonal matrix has row dimension N = NL + NR + 1,
* and column dimension M = N + SQRE.
*
* NRHS (input) INTEGER
* The number of columns of B and BX. NRHS must be at least 1.
*
* B (input/output) REAL array, dimension ( LDB, NRHS )
* On input, B contains the right hand sides of the least
* squares problem in rows 1 through M. On output, B contains
* the solution X in rows 1 through N.
*
* LDB (input) INTEGER
* The leading dimension of B. LDB must be at least
* max(1,MAX( M, N ) ).
*
* BX (workspace) REAL array, dimension ( LDBX, NRHS )
*
* LDBX (input) INTEGER
* The leading dimension of BX.
*
* PERM (input) INTEGER array, dimension ( N )
* The permutations (from deflation and sorting) applied
* to the two blocks.
*
* GIVPTR (input) INTEGER
* The number of Givens rotations which took place in this
* subproblem.
*
* GIVCOL (input) INTEGER array, dimension ( LDGCOL, 2 )
* Each pair of numbers indicates a pair of rows/columns
* involved in a Givens rotation.
*
* LDGCOL (input) INTEGER
* The leading dimension of GIVCOL, must be at least N.
*
* GIVNUM (input) REAL array, dimension ( LDGNUM, 2 )
* Each number indicates the C or S value used in the
* corresponding Givens rotation.
*
* LDGNUM (input) INTEGER
* The leading dimension of arrays DIFR, POLES and
* GIVNUM, must be at least K.
*
* POLES (input) REAL array, dimension ( LDGNUM, 2 )
* On entry, POLES(1:K, 1) contains the new singular
* values obtained from solving the secular equation, and
* POLES(1:K, 2) is an array containing the poles in the secular
* equation.
*
* DIFL (input) REAL array, dimension ( K ).
* On entry, DIFL(I) is the distance between I-th updated
* (undeflated) singular value and the I-th (undeflated) old
* singular value.
*
* DIFR (input) REAL array, dimension ( LDGNUM, 2 ).
* On entry, DIFR(I, 1) contains the distances between I-th
* updated (undeflated) singular value and the I+1-th
* (undeflated) old singular value. And DIFR(I, 2) is the
* normalizing factor for the I-th right singular vector.
*
* Z (input) REAL array, dimension ( K )
* Contain the components of the deflation-adjusted updating row
* vector.
*
* K (input) INTEGER
* Contains the dimension of the non-deflated matrix,
* This is the order of the related secular equation. 1 <= K <=N.
*
* C (input) REAL
* C contains garbage if SQRE =0 and the C-value of a Givens
* rotation related to the right null space if SQRE = 1.
*
* S (input) REAL
* S contains garbage if SQRE =0 and the S-value of a Givens
* rotation related to the right null space if SQRE = 1.
*
* WORK (workspace) REAL array, dimension ( K )
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Ren-Cang Li, Computer Science Division, University of
* California at Berkeley, USA
* Osni Marques, LBNL/NERSC, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param nl
* @param nr
* @param sqre
* @param nrhs
* @param b
* @param _b_offset
* @param ldb
* @param bx
* @param _bx_offset
* @param ldbx
* @param perm
* @param _perm_offset
* @param givptr
* @param givcol
* @param _givcol_offset
* @param ldgcol
* @param givnum
* @param _givnum_offset
* @param ldgnum
* @param poles
* @param _poles_offset
* @param difl
* @param _difl_offset
* @param difr
* @param _difr_offset
* @param z
* @param _z_offset
* @param k
* @param c
* @param s
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void slals0(int icompq, int nl, int nr, int sqre, int nrhs, float[] b, int _b_offset, int ldb, float[] bx, int _bx_offset, int ldbx, int[] perm, int _perm_offset, int givptr, int[] givcol, int _givcol_offset, int ldgcol, float[] givnum, int _givnum_offset, int ldgnum, float[] poles, int _poles_offset, float[] difl, int _difl_offset, float[] difr, int _difr_offset, float[] z, int _z_offset, int k, float c, float s, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLALSA is an itermediate step in solving the least squares problem
* by computing the SVD of the coefficient matrix in compact form (The
* singular vectors are computed as products of simple orthorgonal
* matrices.).
*
* If ICOMPQ = 0, SLALSA applies the inverse of the left singular vector
* matrix of an upper bidiagonal matrix to the right hand side; and if
* ICOMPQ = 1, SLALSA applies the right singular vector matrix to the
* right hand side. The singular vector matrices were generated in
* compact form by SLALSA.
*
* Arguments
* =========
*
*
* ICOMPQ (input) INTEGER
* Specifies whether the left or the right singular vector
* matrix is involved.
* = 0: Left singular vector matrix
* = 1: Right singular vector matrix
*
* SMLSIZ (input) INTEGER
* The maximum size of the subproblems at the bottom of the
* computation tree.
*
* N (input) INTEGER
* The row and column dimensions of the upper bidiagonal matrix.
*
* NRHS (input) INTEGER
* The number of columns of B and BX. NRHS must be at least 1.
*
* B (input/output) REAL array, dimension ( LDB, NRHS )
* On input, B contains the right hand sides of the least
* squares problem in rows 1 through M.
* On output, B contains the solution X in rows 1 through N.
*
* LDB (input) INTEGER
* The leading dimension of B in the calling subprogram.
* LDB must be at least max(1,MAX( M, N ) ).
*
* BX (output) REAL array, dimension ( LDBX, NRHS )
* On exit, the result of applying the left or right singular
* vector matrix to B.
*
* LDBX (input) INTEGER
* The leading dimension of BX.
*
* U (input) REAL array, dimension ( LDU, SMLSIZ ).
* On entry, U contains the left singular vector matrices of all
* subproblems at the bottom level.
*
* LDU (input) INTEGER, LDU = > N.
* The leading dimension of arrays U, VT, DIFL, DIFR,
* POLES, GIVNUM, and Z.
*
* VT (input) REAL array, dimension ( LDU, SMLSIZ+1 ).
* On entry, VT' contains the right singular vector matrices of
* all subproblems at the bottom level.
*
* K (input) INTEGER array, dimension ( N ).
*
* DIFL (input) REAL array, dimension ( LDU, NLVL ).
* where NLVL = INT(log_2 (N/(SMLSIZ+1))) + 1.
*
* DIFR (input) REAL array, dimension ( LDU, 2 * NLVL ).
* On entry, DIFL(*, I) and DIFR(*, 2 * I -1) record
* distances between singular values on the I-th level and
* singular values on the (I -1)-th level, and DIFR(*, 2 * I)
* record the normalizing factors of the right singular vectors
* matrices of subproblems on I-th level.
*
* Z (input) REAL array, dimension ( LDU, NLVL ).
* On entry, Z(1, I) contains the components of the deflation-
* adjusted updating row vector for subproblems on the I-th
* level.
*
* POLES (input) REAL array, dimension ( LDU, 2 * NLVL ).
* On entry, POLES(*, 2 * I -1: 2 * I) contains the new and old
* singular values involved in the secular equations on the I-th
* level.
*
* GIVPTR (input) INTEGER array, dimension ( N ).
* On entry, GIVPTR( I ) records the number of Givens
* rotations performed on the I-th problem on the computation
* tree.
*
* GIVCOL (input) INTEGER array, dimension ( LDGCOL, 2 * NLVL ).
* On entry, for each I, GIVCOL(*, 2 * I - 1: 2 * I) records the
* locations of Givens rotations performed on the I-th level on
* the computation tree.
*
* LDGCOL (input) INTEGER, LDGCOL = > N.
* The leading dimension of arrays GIVCOL and PERM.
*
* PERM (input) INTEGER array, dimension ( LDGCOL, NLVL ).
* On entry, PERM(*, I) records permutations done on the I-th
* level of the computation tree.
*
* GIVNUM (input) REAL array, dimension ( LDU, 2 * NLVL ).
* On entry, GIVNUM(*, 2 *I -1 : 2 * I) records the C- and S-
* values of Givens rotations performed on the I-th level on the
* computation tree.
*
* C (input) REAL array, dimension ( N ).
* On entry, if the I-th subproblem is not square,
* C( I ) contains the C-value of a Givens rotation related to
* the right null space of the I-th subproblem.
*
* S (input) REAL array, dimension ( N ).
* On entry, if the I-th subproblem is not square,
* S( I ) contains the S-value of a Givens rotation related to
* the right null space of the I-th subproblem.
*
* WORK (workspace) REAL array.
* The dimension must be at least N.
*
* IWORK (workspace) INTEGER array.
* The dimension must be at least 3 * N
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Ren-Cang Li, Computer Science Division, University of
* California at Berkeley, USA
* Osni Marques, LBNL/NERSC, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param smlsiz
* @param n
* @param nrhs
* @param b
* @param ldb
* @param bx
* @param ldbx
* @param u
* @param ldu
* @param vt
* @param k
* @param difl
* @param difr
* @param z
* @param poles
* @param givptr
* @param givcol
* @param ldgcol
* @param perm
* @param givnum
* @param c
* @param s
* @param work
* @param iwork
* @param info
*
*/
abstract public void slalsa(int icompq, int smlsiz, int n, int nrhs, float[] b, int ldb, float[] bx, int ldbx, float[] u, int ldu, float[] vt, int[] k, float[] difl, float[] difr, float[] z, float[] poles, int[] givptr, int[] givcol, int ldgcol, int[] perm, float[] givnum, float[] c, float[] s, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLALSA is an itermediate step in solving the least squares problem
* by computing the SVD of the coefficient matrix in compact form (The
* singular vectors are computed as products of simple orthorgonal
* matrices.).
*
* If ICOMPQ = 0, SLALSA applies the inverse of the left singular vector
* matrix of an upper bidiagonal matrix to the right hand side; and if
* ICOMPQ = 1, SLALSA applies the right singular vector matrix to the
* right hand side. The singular vector matrices were generated in
* compact form by SLALSA.
*
* Arguments
* =========
*
*
* ICOMPQ (input) INTEGER
* Specifies whether the left or the right singular vector
* matrix is involved.
* = 0: Left singular vector matrix
* = 1: Right singular vector matrix
*
* SMLSIZ (input) INTEGER
* The maximum size of the subproblems at the bottom of the
* computation tree.
*
* N (input) INTEGER
* The row and column dimensions of the upper bidiagonal matrix.
*
* NRHS (input) INTEGER
* The number of columns of B and BX. NRHS must be at least 1.
*
* B (input/output) REAL array, dimension ( LDB, NRHS )
* On input, B contains the right hand sides of the least
* squares problem in rows 1 through M.
* On output, B contains the solution X in rows 1 through N.
*
* LDB (input) INTEGER
* The leading dimension of B in the calling subprogram.
* LDB must be at least max(1,MAX( M, N ) ).
*
* BX (output) REAL array, dimension ( LDBX, NRHS )
* On exit, the result of applying the left or right singular
* vector matrix to B.
*
* LDBX (input) INTEGER
* The leading dimension of BX.
*
* U (input) REAL array, dimension ( LDU, SMLSIZ ).
* On entry, U contains the left singular vector matrices of all
* subproblems at the bottom level.
*
* LDU (input) INTEGER, LDU = > N.
* The leading dimension of arrays U, VT, DIFL, DIFR,
* POLES, GIVNUM, and Z.
*
* VT (input) REAL array, dimension ( LDU, SMLSIZ+1 ).
* On entry, VT' contains the right singular vector matrices of
* all subproblems at the bottom level.
*
* K (input) INTEGER array, dimension ( N ).
*
* DIFL (input) REAL array, dimension ( LDU, NLVL ).
* where NLVL = INT(log_2 (N/(SMLSIZ+1))) + 1.
*
* DIFR (input) REAL array, dimension ( LDU, 2 * NLVL ).
* On entry, DIFL(*, I) and DIFR(*, 2 * I -1) record
* distances between singular values on the I-th level and
* singular values on the (I -1)-th level, and DIFR(*, 2 * I)
* record the normalizing factors of the right singular vectors
* matrices of subproblems on I-th level.
*
* Z (input) REAL array, dimension ( LDU, NLVL ).
* On entry, Z(1, I) contains the components of the deflation-
* adjusted updating row vector for subproblems on the I-th
* level.
*
* POLES (input) REAL array, dimension ( LDU, 2 * NLVL ).
* On entry, POLES(*, 2 * I -1: 2 * I) contains the new and old
* singular values involved in the secular equations on the I-th
* level.
*
* GIVPTR (input) INTEGER array, dimension ( N ).
* On entry, GIVPTR( I ) records the number of Givens
* rotations performed on the I-th problem on the computation
* tree.
*
* GIVCOL (input) INTEGER array, dimension ( LDGCOL, 2 * NLVL ).
* On entry, for each I, GIVCOL(*, 2 * I - 1: 2 * I) records the
* locations of Givens rotations performed on the I-th level on
* the computation tree.
*
* LDGCOL (input) INTEGER, LDGCOL = > N.
* The leading dimension of arrays GIVCOL and PERM.
*
* PERM (input) INTEGER array, dimension ( LDGCOL, NLVL ).
* On entry, PERM(*, I) records permutations done on the I-th
* level of the computation tree.
*
* GIVNUM (input) REAL array, dimension ( LDU, 2 * NLVL ).
* On entry, GIVNUM(*, 2 *I -1 : 2 * I) records the C- and S-
* values of Givens rotations performed on the I-th level on the
* computation tree.
*
* C (input) REAL array, dimension ( N ).
* On entry, if the I-th subproblem is not square,
* C( I ) contains the C-value of a Givens rotation related to
* the right null space of the I-th subproblem.
*
* S (input) REAL array, dimension ( N ).
* On entry, if the I-th subproblem is not square,
* S( I ) contains the S-value of a Givens rotation related to
* the right null space of the I-th subproblem.
*
* WORK (workspace) REAL array.
* The dimension must be at least N.
*
* IWORK (workspace) INTEGER array.
* The dimension must be at least 3 * N
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Ren-Cang Li, Computer Science Division, University of
* California at Berkeley, USA
* Osni Marques, LBNL/NERSC, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param smlsiz
* @param n
* @param nrhs
* @param b
* @param _b_offset
* @param ldb
* @param bx
* @param _bx_offset
* @param ldbx
* @param u
* @param _u_offset
* @param ldu
* @param vt
* @param _vt_offset
* @param k
* @param _k_offset
* @param difl
* @param _difl_offset
* @param difr
* @param _difr_offset
* @param z
* @param _z_offset
* @param poles
* @param _poles_offset
* @param givptr
* @param _givptr_offset
* @param givcol
* @param _givcol_offset
* @param ldgcol
* @param perm
* @param _perm_offset
* @param givnum
* @param _givnum_offset
* @param c
* @param _c_offset
* @param s
* @param _s_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void slalsa(int icompq, int smlsiz, int n, int nrhs, float[] b, int _b_offset, int ldb, float[] bx, int _bx_offset, int ldbx, float[] u, int _u_offset, int ldu, float[] vt, int _vt_offset, int[] k, int _k_offset, float[] difl, int _difl_offset, float[] difr, int _difr_offset, float[] z, int _z_offset, float[] poles, int _poles_offset, int[] givptr, int _givptr_offset, int[] givcol, int _givcol_offset, int ldgcol, int[] perm, int _perm_offset, float[] givnum, int _givnum_offset, float[] c, int _c_offset, float[] s, int _s_offset, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLALSD uses the singular value decomposition of A to solve the least
* squares problem of finding X to minimize the Euclidean norm of each
* column of A*X-B, where A is N-by-N upper bidiagonal, and X and B
* are N-by-NRHS. The solution X overwrites B.
*
* The singular values of A smaller than RCOND times the largest
* singular value are treated as zero in solving the least squares
* problem; in this case a minimum norm solution is returned.
* The actual singular values are returned in D in ascending order.
*
* This code makes very mild assumptions about floating point
* arithmetic. It will work on machines with a guard digit in
* add/subtract, or on those binary machines without guard digits
* which subtract like the Cray XMP, Cray YMP, Cray C 90, or Cray 2.
* It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': D and E define an upper bidiagonal matrix.
* = 'L': D and E define a lower bidiagonal matrix.
*
* SMLSIZ (input) INTEGER
* The maximum size of the subproblems at the bottom of the
* computation tree.
*
* N (input) INTEGER
* The dimension of the bidiagonal matrix. N >= 0.
*
* NRHS (input) INTEGER
* The number of columns of B. NRHS must be at least 1.
*
* D (input/output) REAL array, dimension (N)
* On entry D contains the main diagonal of the bidiagonal
* matrix. On exit, if INFO = 0, D contains its singular values.
*
* E (input/output) REAL array, dimension (N-1)
* Contains the super-diagonal entries of the bidiagonal matrix.
* On exit, E has been destroyed.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On input, B contains the right hand sides of the least
* squares problem. On output, B contains the solution X.
*
* LDB (input) INTEGER
* The leading dimension of B in the calling subprogram.
* LDB must be at least max(1,N).
*
* RCOND (input) REAL
* The singular values of A less than or equal to RCOND times
* the largest singular value are treated as zero in solving
* the least squares problem. If RCOND is negative,
* machine precision is used instead.
* For example, if diag(S)*X=B were the least squares problem,
* where diag(S) is a diagonal matrix of singular values, the
* solution would be X(i) = B(i) / S(i) if S(i) is greater than
* RCOND*max(S), and X(i) = 0 if S(i) is less than or equal to
* RCOND*max(S).
*
* RANK (output) INTEGER
* The number of singular values of A greater than RCOND times
* the largest singular value.
*
* WORK (workspace) REAL array, dimension at least
* (9*N + 2*N*SMLSIZ + 8*N*NLVL + N*NRHS + (SMLSIZ+1)**2),
* where NLVL = max(0, INT(log_2 (N/(SMLSIZ+1))) + 1).
*
* IWORK (workspace) INTEGER array, dimension at least
* (3*N*NLVL + 11*N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: The algorithm failed to compute an singular value while
* working on the submatrix lying in rows and columns
* INFO/(N+1) through MOD(INFO,N+1).
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Ren-Cang Li, Computer Science Division, University of
* California at Berkeley, USA
* Osni Marques, LBNL/NERSC, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param smlsiz
* @param n
* @param nrhs
* @param d
* @param e
* @param b
* @param ldb
* @param rcond
* @param rank
* @param work
* @param iwork
* @param info
*
*/
abstract public void slalsd(java.lang.String uplo, int smlsiz, int n, int nrhs, float[] d, float[] e, float[] b, int ldb, float rcond, org.netlib.util.intW rank, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLALSD uses the singular value decomposition of A to solve the least
* squares problem of finding X to minimize the Euclidean norm of each
* column of A*X-B, where A is N-by-N upper bidiagonal, and X and B
* are N-by-NRHS. The solution X overwrites B.
*
* The singular values of A smaller than RCOND times the largest
* singular value are treated as zero in solving the least squares
* problem; in this case a minimum norm solution is returned.
* The actual singular values are returned in D in ascending order.
*
* This code makes very mild assumptions about floating point
* arithmetic. It will work on machines with a guard digit in
* add/subtract, or on those binary machines without guard digits
* which subtract like the Cray XMP, Cray YMP, Cray C 90, or Cray 2.
* It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': D and E define an upper bidiagonal matrix.
* = 'L': D and E define a lower bidiagonal matrix.
*
* SMLSIZ (input) INTEGER
* The maximum size of the subproblems at the bottom of the
* computation tree.
*
* N (input) INTEGER
* The dimension of the bidiagonal matrix. N >= 0.
*
* NRHS (input) INTEGER
* The number of columns of B. NRHS must be at least 1.
*
* D (input/output) REAL array, dimension (N)
* On entry D contains the main diagonal of the bidiagonal
* matrix. On exit, if INFO = 0, D contains its singular values.
*
* E (input/output) REAL array, dimension (N-1)
* Contains the super-diagonal entries of the bidiagonal matrix.
* On exit, E has been destroyed.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On input, B contains the right hand sides of the least
* squares problem. On output, B contains the solution X.
*
* LDB (input) INTEGER
* The leading dimension of B in the calling subprogram.
* LDB must be at least max(1,N).
*
* RCOND (input) REAL
* The singular values of A less than or equal to RCOND times
* the largest singular value are treated as zero in solving
* the least squares problem. If RCOND is negative,
* machine precision is used instead.
* For example, if diag(S)*X=B were the least squares problem,
* where diag(S) is a diagonal matrix of singular values, the
* solution would be X(i) = B(i) / S(i) if S(i) is greater than
* RCOND*max(S), and X(i) = 0 if S(i) is less than or equal to
* RCOND*max(S).
*
* RANK (output) INTEGER
* The number of singular values of A greater than RCOND times
* the largest singular value.
*
* WORK (workspace) REAL array, dimension at least
* (9*N + 2*N*SMLSIZ + 8*N*NLVL + N*NRHS + (SMLSIZ+1)**2),
* where NLVL = max(0, INT(log_2 (N/(SMLSIZ+1))) + 1).
*
* IWORK (workspace) INTEGER array, dimension at least
* (3*N*NLVL + 11*N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: The algorithm failed to compute an singular value while
* working on the submatrix lying in rows and columns
* INFO/(N+1) through MOD(INFO,N+1).
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Ren-Cang Li, Computer Science Division, University of
* California at Berkeley, USA
* Osni Marques, LBNL/NERSC, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param smlsiz
* @param n
* @param nrhs
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param b
* @param _b_offset
* @param ldb
* @param rcond
* @param rank
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void slalsd(java.lang.String uplo, int smlsiz, int n, int nrhs, float[] d, int _d_offset, float[] e, int _e_offset, float[] b, int _b_offset, int ldb, float rcond, org.netlib.util.intW rank, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAMRG will create a permutation list which will merge the elements
* of A (which is composed of two independently sorted sets) into a
* single set which is sorted in ascending order.
*
* Arguments
* =========
*
* N1 (input) INTEGER
* N2 (input) INTEGER
* These arguements contain the respective lengths of the two
* sorted lists to be merged.
*
* A (input) REAL array, dimension (N1+N2)
* The first N1 elements of A contain a list of numbers which
* are sorted in either ascending or descending order. Likewise
* for the final N2 elements.
*
* STRD1 (input) INTEGER
* STRD2 (input) INTEGER
* These are the strides to be taken through the array A.
* Allowable strides are 1 and -1. They indicate whether a
* subset of A is sorted in ascending (STRDx = 1) or descending
* (STRDx = -1) order.
*
* INDEX (output) INTEGER array, dimension (N1+N2)
* On exit this array will contain a permutation such that
* if B( I ) = A( INDEX( I ) ) for I=1,N1+N2, then B will be
* sorted in ascending order.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n1
* @param n2
* @param a
* @param strd1
* @param strd2
* @param index
*
*/
abstract public void slamrg(int n1, int n2, float[] a, int strd1, int strd2, int[] index);
/**
*
* ..
*
* Purpose
* =======
*
* SLAMRG will create a permutation list which will merge the elements
* of A (which is composed of two independently sorted sets) into a
* single set which is sorted in ascending order.
*
* Arguments
* =========
*
* N1 (input) INTEGER
* N2 (input) INTEGER
* These arguements contain the respective lengths of the two
* sorted lists to be merged.
*
* A (input) REAL array, dimension (N1+N2)
* The first N1 elements of A contain a list of numbers which
* are sorted in either ascending or descending order. Likewise
* for the final N2 elements.
*
* STRD1 (input) INTEGER
* STRD2 (input) INTEGER
* These are the strides to be taken through the array A.
* Allowable strides are 1 and -1. They indicate whether a
* subset of A is sorted in ascending (STRDx = 1) or descending
* (STRDx = -1) order.
*
* INDEX (output) INTEGER array, dimension (N1+N2)
* On exit this array will contain a permutation such that
* if B( I ) = A( INDEX( I ) ) for I=1,N1+N2, then B will be
* sorted in ascending order.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n1
* @param n2
* @param a
* @param _a_offset
* @param strd1
* @param strd2
* @param index
* @param _index_offset
*
*/
abstract public void slamrg(int n1, int n2, float[] a, int _a_offset, int strd1, int strd2, int[] index, int _index_offset);
/**
*
* ..
*
* Purpose
* =======
*
* SLANEG computes the Sturm count, the number of negative pivots
* encountered while factoring tridiagonal T - sigma I = L D L^T.
* This implementation works directly on the factors without forming
* the tridiagonal matrix T. The Sturm count is also the number of
* eigenvalues of T less than sigma.
*
* This routine is called from SLARRB.
*
* The current routine does not use the PIVMIN parameter but rather
* requires IEEE-754 propagation of Infinities and NaNs. This
* routine also has no input range restrictions but does require
* default exception handling such that x/0 produces Inf when x is
* non-zero, and Inf/Inf produces NaN. For more information, see:
*
* Marques, Riedy, and Voemel, "Benefits of IEEE-754 Features in
* Modern Symmetric Tridiagonal Eigensolvers," SIAM Journal on
* Scientific Computing, v28, n5, 2006. DOI 10.1137/050641624
* (Tech report version in LAWN 172 with the same title.)
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix.
*
* D (input) REAL array, dimension (N)
* The N diagonal elements of the diagonal matrix D.
*
* LLD (input) REAL array, dimension (N-1)
* The (N-1) elements L(i)*L(i)*D(i).
*
* SIGMA (input) REAL
* Shift amount in T - sigma I = L D L^T.
*
* PIVMIN (input) REAL
* The minimum pivot in the Sturm sequence. May be used
* when zero pivots are encountered on non-IEEE-754
* architectures.
*
* R (input) INTEGER
* The twist index for the twisted factorization that is used
* for the negcount.
*
* Further Details
* ===============
*
* Based on contributions by
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
* Jason Riedy, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param lld
* @param sigma
* @param pivmin
* @param r
* @return
*/
abstract public int slaneg(int n, float[] d, float[] lld, float sigma, float pivmin, int r);
/**
*
* ..
*
* Purpose
* =======
*
* SLANEG computes the Sturm count, the number of negative pivots
* encountered while factoring tridiagonal T - sigma I = L D L^T.
* This implementation works directly on the factors without forming
* the tridiagonal matrix T. The Sturm count is also the number of
* eigenvalues of T less than sigma.
*
* This routine is called from SLARRB.
*
* The current routine does not use the PIVMIN parameter but rather
* requires IEEE-754 propagation of Infinities and NaNs. This
* routine also has no input range restrictions but does require
* default exception handling such that x/0 produces Inf when x is
* non-zero, and Inf/Inf produces NaN. For more information, see:
*
* Marques, Riedy, and Voemel, "Benefits of IEEE-754 Features in
* Modern Symmetric Tridiagonal Eigensolvers," SIAM Journal on
* Scientific Computing, v28, n5, 2006. DOI 10.1137/050641624
* (Tech report version in LAWN 172 with the same title.)
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix.
*
* D (input) REAL array, dimension (N)
* The N diagonal elements of the diagonal matrix D.
*
* LLD (input) REAL array, dimension (N-1)
* The (N-1) elements L(i)*L(i)*D(i).
*
* SIGMA (input) REAL
* Shift amount in T - sigma I = L D L^T.
*
* PIVMIN (input) REAL
* The minimum pivot in the Sturm sequence. May be used
* when zero pivots are encountered on non-IEEE-754
* architectures.
*
* R (input) INTEGER
* The twist index for the twisted factorization that is used
* for the negcount.
*
* Further Details
* ===============
*
* Based on contributions by
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
* Jason Riedy, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param _d_offset
* @param lld
* @param _lld_offset
* @param sigma
* @param pivmin
* @param r
* @return
*/
abstract public int slaneg(int n, float[] d, int _d_offset, float[] lld, int _lld_offset, float sigma, float pivmin, int r);
/**
*
* ..
*
* Purpose
* =======
*
* SLANGB returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of an
* n by n band matrix A, with kl sub-diagonals and ku super-diagonals.
*
* Description
* ===========
*
* SLANGB returns the value
*
* SLANGB = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in SLANGB as described
* above.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, SLANGB is
* set to zero.
*
* KL (input) INTEGER
* The number of sub-diagonals of the matrix A. KL >= 0.
*
* KU (input) INTEGER
* The number of super-diagonals of the matrix A. KU >= 0.
*
* AB (input) REAL array, dimension (LDAB,N)
* The band matrix A, stored in rows 1 to KL+KU+1. The j-th
* column of A is stored in the j-th column of the array AB as
* follows:
* AB(ku+1+i-j,j) = A(i,j) for max(1,j-ku)<=i<=min(n,j+kl).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KL+KU+1.
*
* WORK (workspace) REAL array, dimension (MAX(1,LWORK)),
* where LWORK >= N when NORM = 'I'; otherwise, WORK is not
* referenced.
*
* =====================================================================
*
*
* .. Parameters ..
*
*
* @param norm
* @param n
* @param kl
* @param ku
* @param ab
* @param ldab
* @param work
* @return
*/
abstract public float slangb(java.lang.String norm, int n, int kl, int ku, float[] ab, int ldab, float[] work);
/**
*
* ..
*
* Purpose
* =======
*
* SLANGB returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of an
* n by n band matrix A, with kl sub-diagonals and ku super-diagonals.
*
* Description
* ===========
*
* SLANGB returns the value
*
* SLANGB = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in SLANGB as described
* above.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, SLANGB is
* set to zero.
*
* KL (input) INTEGER
* The number of sub-diagonals of the matrix A. KL >= 0.
*
* KU (input) INTEGER
* The number of super-diagonals of the matrix A. KU >= 0.
*
* AB (input) REAL array, dimension (LDAB,N)
* The band matrix A, stored in rows 1 to KL+KU+1. The j-th
* column of A is stored in the j-th column of the array AB as
* follows:
* AB(ku+1+i-j,j) = A(i,j) for max(1,j-ku)<=i<=min(n,j+kl).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KL+KU+1.
*
* WORK (workspace) REAL array, dimension (MAX(1,LWORK)),
* where LWORK >= N when NORM = 'I'; otherwise, WORK is not
* referenced.
*
* =====================================================================
*
*
* .. Parameters ..
*
*
* @param norm
* @param n
* @param kl
* @param ku
* @param ab
* @param _ab_offset
* @param ldab
* @param work
* @param _work_offset
* @return
*/
abstract public float slangb(java.lang.String norm, int n, int kl, int ku, float[] ab, int _ab_offset, int ldab, float[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* SLANGE returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of a
* real matrix A.
*
* Description
* ===========
*
* SLANGE returns the value
*
* SLANGE = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in SLANGE as described
* above.
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0. When M = 0,
* SLANGE is set to zero.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0. When N = 0,
* SLANGE is set to zero.
*
* A (input) REAL array, dimension (LDA,N)
* The m by n matrix A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(M,1).
*
* WORK (workspace) REAL array, dimension (MAX(1,LWORK)),
* where LWORK >= M when NORM = 'I'; otherwise, WORK is not
* referenced.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param m
* @param n
* @param a
* @param lda
* @param work
* @return
*/
abstract public float slange(java.lang.String norm, int m, int n, float[] a, int lda, float[] work);
/**
*
* ..
*
* Purpose
* =======
*
* SLANGE returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of a
* real matrix A.
*
* Description
* ===========
*
* SLANGE returns the value
*
* SLANGE = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in SLANGE as described
* above.
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0. When M = 0,
* SLANGE is set to zero.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0. When N = 0,
* SLANGE is set to zero.
*
* A (input) REAL array, dimension (LDA,N)
* The m by n matrix A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(M,1).
*
* WORK (workspace) REAL array, dimension (MAX(1,LWORK)),
* where LWORK >= M when NORM = 'I'; otherwise, WORK is not
* referenced.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param work
* @param _work_offset
* @return
*/
abstract public float slange(java.lang.String norm, int m, int n, float[] a, int _a_offset, int lda, float[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* SLANGT returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of a
* real tridiagonal matrix A.
*
* Description
* ===========
*
* SLANGT returns the value
*
* SLANGT = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in SLANGT as described
* above.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, SLANGT is
* set to zero.
*
* DL (input) REAL array, dimension (N-1)
* The (n-1) sub-diagonal elements of A.
*
* D (input) REAL array, dimension (N)
* The diagonal elements of A.
*
* DU (input) REAL array, dimension (N-1)
* The (n-1) super-diagonal elements of A.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param n
* @param dl
* @param d
* @param du
* @return
*/
abstract public float slangt(java.lang.String norm, int n, float[] dl, float[] d, float[] du);
/**
*
* ..
*
* Purpose
* =======
*
* SLANGT returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of a
* real tridiagonal matrix A.
*
* Description
* ===========
*
* SLANGT returns the value
*
* SLANGT = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in SLANGT as described
* above.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, SLANGT is
* set to zero.
*
* DL (input) REAL array, dimension (N-1)
* The (n-1) sub-diagonal elements of A.
*
* D (input) REAL array, dimension (N)
* The diagonal elements of A.
*
* DU (input) REAL array, dimension (N-1)
* The (n-1) super-diagonal elements of A.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param n
* @param dl
* @param _dl_offset
* @param d
* @param _d_offset
* @param du
* @param _du_offset
* @return
*/
abstract public float slangt(java.lang.String norm, int n, float[] dl, int _dl_offset, float[] d, int _d_offset, float[] du, int _du_offset);
/**
*
* ..
*
* Purpose
* =======
*
* SLANHS returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of a
* Hessenberg matrix A.
*
* Description
* ===========
*
* SLANHS returns the value
*
* SLANHS = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in SLANHS as described
* above.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, SLANHS is
* set to zero.
*
* A (input) REAL array, dimension (LDA,N)
* The n by n upper Hessenberg matrix A; the part of A below the
* first sub-diagonal is not referenced.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(N,1).
*
* WORK (workspace) REAL array, dimension (MAX(1,LWORK)),
* where LWORK >= N when NORM = 'I'; otherwise, WORK is not
* referenced.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param n
* @param a
* @param lda
* @param work
* @return
*/
abstract public float slanhs(java.lang.String norm, int n, float[] a, int lda, float[] work);
/**
*
* ..
*
* Purpose
* =======
*
* SLANHS returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of a
* Hessenberg matrix A.
*
* Description
* ===========
*
* SLANHS returns the value
*
* SLANHS = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in SLANHS as described
* above.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, SLANHS is
* set to zero.
*
* A (input) REAL array, dimension (LDA,N)
* The n by n upper Hessenberg matrix A; the part of A below the
* first sub-diagonal is not referenced.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(N,1).
*
* WORK (workspace) REAL array, dimension (MAX(1,LWORK)),
* where LWORK >= N when NORM = 'I'; otherwise, WORK is not
* referenced.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param n
* @param a
* @param _a_offset
* @param lda
* @param work
* @param _work_offset
* @return
*/
abstract public float slanhs(java.lang.String norm, int n, float[] a, int _a_offset, int lda, float[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* SLANSB returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of an
* n by n symmetric band matrix A, with k super-diagonals.
*
* Description
* ===========
*
* SLANSB returns the value
*
* SLANSB = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in SLANSB as described
* above.
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* band matrix A is supplied.
* = 'U': Upper triangular part is supplied
* = 'L': Lower triangular part is supplied
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, SLANSB is
* set to zero.
*
* K (input) INTEGER
* The number of super-diagonals or sub-diagonals of the
* band matrix A. K >= 0.
*
* AB (input) REAL array, dimension (LDAB,N)
* The upper or lower triangle of the symmetric band matrix A,
* stored in the first K+1 rows of AB. The j-th column of A is
* stored in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(k+1+i-j,j) = A(i,j) for max(1,j-k)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+k).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= K+1.
*
* WORK (workspace) REAL array, dimension (MAX(1,LWORK)),
* where LWORK >= N when NORM = 'I' or '1' or 'O'; otherwise,
* WORK is not referenced.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param n
* @param k
* @param ab
* @param ldab
* @param work
* @return
*/
abstract public float slansb(java.lang.String norm, java.lang.String uplo, int n, int k, float[] ab, int ldab, float[] work);
/**
*
* ..
*
* Purpose
* =======
*
* SLANSB returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of an
* n by n symmetric band matrix A, with k super-diagonals.
*
* Description
* ===========
*
* SLANSB returns the value
*
* SLANSB = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in SLANSB as described
* above.
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* band matrix A is supplied.
* = 'U': Upper triangular part is supplied
* = 'L': Lower triangular part is supplied
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, SLANSB is
* set to zero.
*
* K (input) INTEGER
* The number of super-diagonals or sub-diagonals of the
* band matrix A. K >= 0.
*
* AB (input) REAL array, dimension (LDAB,N)
* The upper or lower triangle of the symmetric band matrix A,
* stored in the first K+1 rows of AB. The j-th column of A is
* stored in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(k+1+i-j,j) = A(i,j) for max(1,j-k)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+k).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= K+1.
*
* WORK (workspace) REAL array, dimension (MAX(1,LWORK)),
* where LWORK >= N when NORM = 'I' or '1' or 'O'; otherwise,
* WORK is not referenced.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param n
* @param k
* @param ab
* @param _ab_offset
* @param ldab
* @param work
* @param _work_offset
* @return
*/
abstract public float slansb(java.lang.String norm, java.lang.String uplo, int n, int k, float[] ab, int _ab_offset, int ldab, float[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* SLANSP returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of a
* real symmetric matrix A, supplied in packed form.
*
* Description
* ===========
*
* SLANSP returns the value
*
* SLANSP = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in SLANSP as described
* above.
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is supplied.
* = 'U': Upper triangular part of A is supplied
* = 'L': Lower triangular part of A is supplied
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, SLANSP is
* set to zero.
*
* AP (input) REAL array, dimension (N*(N+1)/2)
* The upper or lower triangle of the symmetric matrix A, packed
* columnwise in a linear array. The j-th column of A is stored
* in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
*
* WORK (workspace) REAL array, dimension (MAX(1,LWORK)),
* where LWORK >= N when NORM = 'I' or '1' or 'O'; otherwise,
* WORK is not referenced.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param n
* @param ap
* @param work
* @return
*/
abstract public float slansp(java.lang.String norm, java.lang.String uplo, int n, float[] ap, float[] work);
/**
*
* ..
*
* Purpose
* =======
*
* SLANSP returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of a
* real symmetric matrix A, supplied in packed form.
*
* Description
* ===========
*
* SLANSP returns the value
*
* SLANSP = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in SLANSP as described
* above.
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is supplied.
* = 'U': Upper triangular part of A is supplied
* = 'L': Lower triangular part of A is supplied
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, SLANSP is
* set to zero.
*
* AP (input) REAL array, dimension (N*(N+1)/2)
* The upper or lower triangle of the symmetric matrix A, packed
* columnwise in a linear array. The j-th column of A is stored
* in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
*
* WORK (workspace) REAL array, dimension (MAX(1,LWORK)),
* where LWORK >= N when NORM = 'I' or '1' or 'O'; otherwise,
* WORK is not referenced.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param work
* @param _work_offset
* @return
*/
abstract public float slansp(java.lang.String norm, java.lang.String uplo, int n, float[] ap, int _ap_offset, float[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* SLANST returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of a
* real symmetric tridiagonal matrix A.
*
* Description
* ===========
*
* SLANST returns the value
*
* SLANST = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in SLANST as described
* above.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, SLANST is
* set to zero.
*
* D (input) REAL array, dimension (N)
* The diagonal elements of A.
*
* E (input) REAL array, dimension (N-1)
* The (n-1) sub-diagonal or super-diagonal elements of A.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param n
* @param d
* @param e
* @return
*/
abstract public float slanst(java.lang.String norm, int n, float[] d, float[] e);
/**
*
* ..
*
* Purpose
* =======
*
* SLANST returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of a
* real symmetric tridiagonal matrix A.
*
* Description
* ===========
*
* SLANST returns the value
*
* SLANST = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in SLANST as described
* above.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, SLANST is
* set to zero.
*
* D (input) REAL array, dimension (N)
* The diagonal elements of A.
*
* E (input) REAL array, dimension (N-1)
* The (n-1) sub-diagonal or super-diagonal elements of A.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @return
*/
abstract public float slanst(java.lang.String norm, int n, float[] d, int _d_offset, float[] e, int _e_offset);
/**
*
* ..
*
* Purpose
* =======
*
* SLANSY returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of a
* real symmetric matrix A.
*
* Description
* ===========
*
* SLANSY returns the value
*
* SLANSY = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in SLANSY as described
* above.
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is to be referenced.
* = 'U': Upper triangular part of A is referenced
* = 'L': Lower triangular part of A is referenced
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, SLANSY is
* set to zero.
*
* A (input) REAL array, dimension (LDA,N)
* The symmetric matrix A. If UPLO = 'U', the leading n by n
* upper triangular part of A contains the upper triangular part
* of the matrix A, and the strictly lower triangular part of A
* is not referenced. If UPLO = 'L', the leading n by n lower
* triangular part of A contains the lower triangular part of
* the matrix A, and the strictly upper triangular part of A is
* not referenced.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(N,1).
*
* WORK (workspace) REAL array, dimension (MAX(1,LWORK)),
* where LWORK >= N when NORM = 'I' or '1' or 'O'; otherwise,
* WORK is not referenced.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param n
* @param a
* @param lda
* @param work
* @return
*/
abstract public float slansy(java.lang.String norm, java.lang.String uplo, int n, float[] a, int lda, float[] work);
/**
*
* ..
*
* Purpose
* =======
*
* SLANSY returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of a
* real symmetric matrix A.
*
* Description
* ===========
*
* SLANSY returns the value
*
* SLANSY = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in SLANSY as described
* above.
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is to be referenced.
* = 'U': Upper triangular part of A is referenced
* = 'L': Lower triangular part of A is referenced
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, SLANSY is
* set to zero.
*
* A (input) REAL array, dimension (LDA,N)
* The symmetric matrix A. If UPLO = 'U', the leading n by n
* upper triangular part of A contains the upper triangular part
* of the matrix A, and the strictly lower triangular part of A
* is not referenced. If UPLO = 'L', the leading n by n lower
* triangular part of A contains the lower triangular part of
* the matrix A, and the strictly upper triangular part of A is
* not referenced.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(N,1).
*
* WORK (workspace) REAL array, dimension (MAX(1,LWORK)),
* where LWORK >= N when NORM = 'I' or '1' or 'O'; otherwise,
* WORK is not referenced.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param work
* @param _work_offset
* @return
*/
abstract public float slansy(java.lang.String norm, java.lang.String uplo, int n, float[] a, int _a_offset, int lda, float[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* SLANTB returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of an
* n by n triangular band matrix A, with ( k + 1 ) diagonals.
*
* Description
* ===========
*
* SLANTB returns the value
*
* SLANTB = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in SLANTB as described
* above.
*
* UPLO (input) CHARACTER*1
* Specifies whether the matrix A is upper or lower triangular.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* DIAG (input) CHARACTER*1
* Specifies whether or not the matrix A is unit triangular.
* = 'N': Non-unit triangular
* = 'U': Unit triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, SLANTB is
* set to zero.
*
* K (input) INTEGER
* The number of super-diagonals of the matrix A if UPLO = 'U',
* or the number of sub-diagonals of the matrix A if UPLO = 'L'.
* K >= 0.
*
* AB (input) REAL array, dimension (LDAB,N)
* The upper or lower triangular band matrix A, stored in the
* first k+1 rows of AB. The j-th column of A is stored
* in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(k+1+i-j,j) = A(i,j) for max(1,j-k)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+k).
* Note that when DIAG = 'U', the elements of the array AB
* corresponding to the diagonal elements of the matrix A are
* not referenced, but are assumed to be one.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= K+1.
*
* WORK (workspace) REAL array, dimension (MAX(1,LWORK)),
* where LWORK >= N when NORM = 'I'; otherwise, WORK is not
* referenced.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param diag
* @param n
* @param k
* @param ab
* @param ldab
* @param work
* @return
*/
abstract public float slantb(java.lang.String norm, java.lang.String uplo, java.lang.String diag, int n, int k, float[] ab, int ldab, float[] work);
/**
*
* ..
*
* Purpose
* =======
*
* SLANTB returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of an
* n by n triangular band matrix A, with ( k + 1 ) diagonals.
*
* Description
* ===========
*
* SLANTB returns the value
*
* SLANTB = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in SLANTB as described
* above.
*
* UPLO (input) CHARACTER*1
* Specifies whether the matrix A is upper or lower triangular.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* DIAG (input) CHARACTER*1
* Specifies whether or not the matrix A is unit triangular.
* = 'N': Non-unit triangular
* = 'U': Unit triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, SLANTB is
* set to zero.
*
* K (input) INTEGER
* The number of super-diagonals of the matrix A if UPLO = 'U',
* or the number of sub-diagonals of the matrix A if UPLO = 'L'.
* K >= 0.
*
* AB (input) REAL array, dimension (LDAB,N)
* The upper or lower triangular band matrix A, stored in the
* first k+1 rows of AB. The j-th column of A is stored
* in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(k+1+i-j,j) = A(i,j) for max(1,j-k)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+k).
* Note that when DIAG = 'U', the elements of the array AB
* corresponding to the diagonal elements of the matrix A are
* not referenced, but are assumed to be one.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= K+1.
*
* WORK (workspace) REAL array, dimension (MAX(1,LWORK)),
* where LWORK >= N when NORM = 'I'; otherwise, WORK is not
* referenced.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param diag
* @param n
* @param k
* @param ab
* @param _ab_offset
* @param ldab
* @param work
* @param _work_offset
* @return
*/
abstract public float slantb(java.lang.String norm, java.lang.String uplo, java.lang.String diag, int n, int k, float[] ab, int _ab_offset, int ldab, float[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* SLANTP returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of a
* triangular matrix A, supplied in packed form.
*
* Description
* ===========
*
* SLANTP returns the value
*
* SLANTP = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in SLANTP as described
* above.
*
* UPLO (input) CHARACTER*1
* Specifies whether the matrix A is upper or lower triangular.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* DIAG (input) CHARACTER*1
* Specifies whether or not the matrix A is unit triangular.
* = 'N': Non-unit triangular
* = 'U': Unit triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, SLANTP is
* set to zero.
*
* AP (input) REAL array, dimension (N*(N+1)/2)
* The upper or lower triangular matrix A, packed columnwise in
* a linear array. The j-th column of A is stored in the array
* AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
* Note that when DIAG = 'U', the elements of the array AP
* corresponding to the diagonal elements of the matrix A are
* not referenced, but are assumed to be one.
*
* WORK (workspace) REAL array, dimension (MAX(1,LWORK)),
* where LWORK >= N when NORM = 'I'; otherwise, WORK is not
* referenced.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param diag
* @param n
* @param ap
* @param work
* @return
*/
abstract public float slantp(java.lang.String norm, java.lang.String uplo, java.lang.String diag, int n, float[] ap, float[] work);
/**
*
* ..
*
* Purpose
* =======
*
* SLANTP returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of a
* triangular matrix A, supplied in packed form.
*
* Description
* ===========
*
* SLANTP returns the value
*
* SLANTP = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in SLANTP as described
* above.
*
* UPLO (input) CHARACTER*1
* Specifies whether the matrix A is upper or lower triangular.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* DIAG (input) CHARACTER*1
* Specifies whether or not the matrix A is unit triangular.
* = 'N': Non-unit triangular
* = 'U': Unit triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0. When N = 0, SLANTP is
* set to zero.
*
* AP (input) REAL array, dimension (N*(N+1)/2)
* The upper or lower triangular matrix A, packed columnwise in
* a linear array. The j-th column of A is stored in the array
* AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
* Note that when DIAG = 'U', the elements of the array AP
* corresponding to the diagonal elements of the matrix A are
* not referenced, but are assumed to be one.
*
* WORK (workspace) REAL array, dimension (MAX(1,LWORK)),
* where LWORK >= N when NORM = 'I'; otherwise, WORK is not
* referenced.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param diag
* @param n
* @param ap
* @param _ap_offset
* @param work
* @param _work_offset
* @return
*/
abstract public float slantp(java.lang.String norm, java.lang.String uplo, java.lang.String diag, int n, float[] ap, int _ap_offset, float[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* SLANTR returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of a
* trapezoidal or triangular matrix A.
*
* Description
* ===========
*
* SLANTR returns the value
*
* SLANTR = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in SLANTR as described
* above.
*
* UPLO (input) CHARACTER*1
* Specifies whether the matrix A is upper or lower trapezoidal.
* = 'U': Upper trapezoidal
* = 'L': Lower trapezoidal
* Note that A is triangular instead of trapezoidal if M = N.
*
* DIAG (input) CHARACTER*1
* Specifies whether or not the matrix A has unit diagonal.
* = 'N': Non-unit diagonal
* = 'U': Unit diagonal
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0, and if
* UPLO = 'U', M <= N. When M = 0, SLANTR is set to zero.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0, and if
* UPLO = 'L', N <= M. When N = 0, SLANTR is set to zero.
*
* A (input) REAL array, dimension (LDA,N)
* The trapezoidal matrix A (A is triangular if M = N).
* If UPLO = 'U', the leading m by n upper trapezoidal part of
* the array A contains the upper trapezoidal matrix, and the
* strictly lower triangular part of A is not referenced.
* If UPLO = 'L', the leading m by n lower trapezoidal part of
* the array A contains the lower trapezoidal matrix, and the
* strictly upper triangular part of A is not referenced. Note
* that when DIAG = 'U', the diagonal elements of A are not
* referenced and are assumed to be one.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(M,1).
*
* WORK (workspace) REAL array, dimension (MAX(1,LWORK)),
* where LWORK >= M when NORM = 'I'; otherwise, WORK is not
* referenced.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param diag
* @param m
* @param n
* @param a
* @param lda
* @param work
* @return
*/
abstract public float slantr(java.lang.String norm, java.lang.String uplo, java.lang.String diag, int m, int n, float[] a, int lda, float[] work);
/**
*
* ..
*
* Purpose
* =======
*
* SLANTR returns the value of the one norm, or the Frobenius norm, or
* the infinity norm, or the element of largest absolute value of a
* trapezoidal or triangular matrix A.
*
* Description
* ===========
*
* SLANTR returns the value
*
* SLANTR = ( max(abs(A(i,j))), NORM = 'M' or 'm'
* (
* ( norm1(A), NORM = '1', 'O' or 'o'
* (
* ( normI(A), NORM = 'I' or 'i'
* (
* ( normF(A), NORM = 'F', 'f', 'E' or 'e'
*
* where norm1 denotes the one norm of a matrix (maximum column sum),
* normI denotes the infinity norm of a matrix (maximum row sum) and
* normF denotes the Frobenius norm of a matrix (square root of sum of
* squares). Note that max(abs(A(i,j))) is not a consistent matrix no
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies the value to be returned in SLANTR as described
* above.
*
* UPLO (input) CHARACTER*1
* Specifies whether the matrix A is upper or lower trapezoidal.
* = 'U': Upper trapezoidal
* = 'L': Lower trapezoidal
* Note that A is triangular instead of trapezoidal if M = N.
*
* DIAG (input) CHARACTER*1
* Specifies whether or not the matrix A has unit diagonal.
* = 'N': Non-unit diagonal
* = 'U': Unit diagonal
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0, and if
* UPLO = 'U', M <= N. When M = 0, SLANTR is set to zero.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0, and if
* UPLO = 'L', N <= M. When N = 0, SLANTR is set to zero.
*
* A (input) REAL array, dimension (LDA,N)
* The trapezoidal matrix A (A is triangular if M = N).
* If UPLO = 'U', the leading m by n upper trapezoidal part of
* the array A contains the upper trapezoidal matrix, and the
* strictly lower triangular part of A is not referenced.
* If UPLO = 'L', the leading m by n lower trapezoidal part of
* the array A contains the lower trapezoidal matrix, and the
* strictly upper triangular part of A is not referenced. Note
* that when DIAG = 'U', the diagonal elements of A are not
* referenced and are assumed to be one.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(M,1).
*
* WORK (workspace) REAL array, dimension (MAX(1,LWORK)),
* where LWORK >= M when NORM = 'I'; otherwise, WORK is not
* referenced.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param diag
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param work
* @param _work_offset
* @return
*/
abstract public float slantr(java.lang.String norm, java.lang.String uplo, java.lang.String diag, int m, int n, float[] a, int _a_offset, int lda, float[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* SLANV2 computes the Schur factorization of a real 2-by-2 nonsymmetric
* matrix in standard form:
*
* [ A B ] = [ CS -SN ] [ AA BB ] [ CS SN ]
* [ C D ] [ SN CS ] [ CC DD ] [-SN CS ]
*
* where either
* 1) CC = 0 so that AA and DD are real eigenvalues of the matrix, or
* 2) AA = DD and BB*CC < 0, so that AA + or - sqrt(BB*CC) are complex
* conjugate eigenvalues.
*
* Arguments
* =========
*
* A (input/output) REAL
* B (input/output) REAL
* C (input/output) REAL
* D (input/output) REAL
* On entry, the elements of the input matrix.
* On exit, they are overwritten by the elements of the
* standardised Schur form.
*
* RT1R (output) REAL
* RT1I (output) REAL
* RT2R (output) REAL
* RT2I (output) REAL
* The real and imaginary parts of the eigenvalues. If the
* eigenvalues are a complex conjugate pair, RT1I > 0.
*
* CS (output) REAL
* SN (output) REAL
* Parameters of the rotation matrix.
*
* Further Details
* ===============
*
* Modified by V. Sima, Research Institute for Informatics, Bucharest,
* Romania, to reduce the risk of cancellation errors,
* when computing real eigenvalues, and to ensure, if possible, that
* abs(RT1R) >= abs(RT2R).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param a
* @param b
* @param c
* @param d
* @param rt1r
* @param rt1i
* @param rt2r
* @param rt2i
* @param cs
* @param sn
*
*/
abstract public void slanv2(org.netlib.util.floatW a, org.netlib.util.floatW b, org.netlib.util.floatW c, org.netlib.util.floatW d, org.netlib.util.floatW rt1r, org.netlib.util.floatW rt1i, org.netlib.util.floatW rt2r, org.netlib.util.floatW rt2i, org.netlib.util.floatW cs, org.netlib.util.floatW sn);
/**
*
* ..
*
* Purpose
* =======
*
* Given two column vectors X and Y, let
*
* A = ( X Y ).
*
* The subroutine first computes the QR factorization of A = Q*R,
* and then computes the SVD of the 2-by-2 upper triangular matrix R.
* The smaller singular value of R is returned in SSMIN, which is used
* as the measurement of the linear dependency of the vectors X and Y.
*
* Arguments
* =========
*
* N (input) INTEGER
* The length of the vectors X and Y.
*
* X (input/output) REAL array,
* dimension (1+(N-1)*INCX)
* On entry, X contains the N-vector X.
* On exit, X is overwritten.
*
* INCX (input) INTEGER
* The increment between successive elements of X. INCX > 0.
*
* Y (input/output) REAL array,
* dimension (1+(N-1)*INCY)
* On entry, Y contains the N-vector Y.
* On exit, Y is overwritten.
*
* INCY (input) INTEGER
* The increment between successive elements of Y. INCY > 0.
*
* SSMIN (output) REAL
* The smallest singular value of the N-by-2 matrix A = ( X Y ).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param x
* @param incx
* @param y
* @param incy
* @param ssmin
*
*/
abstract public void slapll(int n, float[] x, int incx, float[] y, int incy, org.netlib.util.floatW ssmin);
/**
*
* ..
*
* Purpose
* =======
*
* Given two column vectors X and Y, let
*
* A = ( X Y ).
*
* The subroutine first computes the QR factorization of A = Q*R,
* and then computes the SVD of the 2-by-2 upper triangular matrix R.
* The smaller singular value of R is returned in SSMIN, which is used
* as the measurement of the linear dependency of the vectors X and Y.
*
* Arguments
* =========
*
* N (input) INTEGER
* The length of the vectors X and Y.
*
* X (input/output) REAL array,
* dimension (1+(N-1)*INCX)
* On entry, X contains the N-vector X.
* On exit, X is overwritten.
*
* INCX (input) INTEGER
* The increment between successive elements of X. INCX > 0.
*
* Y (input/output) REAL array,
* dimension (1+(N-1)*INCY)
* On entry, Y contains the N-vector Y.
* On exit, Y is overwritten.
*
* INCY (input) INTEGER
* The increment between successive elements of Y. INCY > 0.
*
* SSMIN (output) REAL
* The smallest singular value of the N-by-2 matrix A = ( X Y ).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param x
* @param _x_offset
* @param incx
* @param y
* @param _y_offset
* @param incy
* @param ssmin
*
*/
abstract public void slapll(int n, float[] x, int _x_offset, int incx, float[] y, int _y_offset, int incy, org.netlib.util.floatW ssmin);
/**
*
* ..
*
* Purpose
* =======
*
* SLAPMT rearranges the columns of the M by N matrix X as specified
* by the permutation K(1),K(2),...,K(N) of the integers 1,...,N.
* If FORWRD = .TRUE., forward permutation:
*
* X(*,K(J)) is moved X(*,J) for J = 1,2,...,N.
*
* If FORWRD = .FALSE., backward permutation:
*
* X(*,J) is moved to X(*,K(J)) for J = 1,2,...,N.
*
* Arguments
* =========
*
* FORWRD (input) LOGICAL
* = .TRUE., forward permutation
* = .FALSE., backward permutation
*
* M (input) INTEGER
* The number of rows of the matrix X. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix X. N >= 0.
*
* X (input/output) REAL array, dimension (LDX,N)
* On entry, the M by N matrix X.
* On exit, X contains the permuted matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X, LDX >= MAX(1,M).
*
* K (input/output) INTEGER array, dimension (N)
* On entry, K contains the permutation vector. K is used as
* internal workspace, but reset to its original value on
* output.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param forwrd
* @param m
* @param n
* @param x
* @param ldx
* @param k
*
*/
abstract public void slapmt(boolean forwrd, int m, int n, float[] x, int ldx, int[] k);
/**
*
* ..
*
* Purpose
* =======
*
* SLAPMT rearranges the columns of the M by N matrix X as specified
* by the permutation K(1),K(2),...,K(N) of the integers 1,...,N.
* If FORWRD = .TRUE., forward permutation:
*
* X(*,K(J)) is moved X(*,J) for J = 1,2,...,N.
*
* If FORWRD = .FALSE., backward permutation:
*
* X(*,J) is moved to X(*,K(J)) for J = 1,2,...,N.
*
* Arguments
* =========
*
* FORWRD (input) LOGICAL
* = .TRUE., forward permutation
* = .FALSE., backward permutation
*
* M (input) INTEGER
* The number of rows of the matrix X. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix X. N >= 0.
*
* X (input/output) REAL array, dimension (LDX,N)
* On entry, the M by N matrix X.
* On exit, X contains the permuted matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X, LDX >= MAX(1,M).
*
* K (input/output) INTEGER array, dimension (N)
* On entry, K contains the permutation vector. K is used as
* internal workspace, but reset to its original value on
* output.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param forwrd
* @param m
* @param n
* @param x
* @param _x_offset
* @param ldx
* @param k
* @param _k_offset
*
*/
abstract public void slapmt(boolean forwrd, int m, int n, float[] x, int _x_offset, int ldx, int[] k, int _k_offset);
/**
*
* ..
*
* Purpose
* =======
*
* SLAPY2 returns sqrt(x**2+y**2), taking care not to cause unnecessary
* overflow.
*
* Arguments
* =========
*
* X (input) REAL
* Y (input) REAL
* X and Y specify the values x and y.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param x
* @param y
* @return
*/
abstract public float slapy2(float x, float y);
/**
*
* ..
*
* Purpose
* =======
*
* SLAPY3 returns sqrt(x**2+y**2+z**2), taking care not to cause
* unnecessary overflow.
*
* Arguments
* =========
*
* X (input) REAL
* Y (input) REAL
* Z (input) REAL
* X, Y and Z specify the values x, y and z.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param x
* @param y
* @param z
* @return
*/
abstract public float slapy3(float x, float y, float z);
/**
*
* ..
*
* Purpose
* =======
*
* SLAQGB equilibrates a general M by N band matrix A with KL
* subdiagonals and KU superdiagonals using the row and scaling factors
* in the vectors R and C.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* AB (input/output) REAL array, dimension (LDAB,N)
* On entry, the matrix A in band storage, in rows 1 to KL+KU+1.
* The j-th column of A is stored in the j-th column of the
* array AB as follows:
* AB(ku+1+i-j,j) = A(i,j) for max(1,j-ku)<=i<=min(m,j+kl)
*
* On exit, the equilibrated matrix, in the same storage format
* as A. See EQUED for the form of the equilibrated matrix.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDA >= KL+KU+1.
*
* R (input) REAL array, dimension (M)
* The row scale factors for A.
*
* C (input) REAL array, dimension (N)
* The column scale factors for A.
*
* ROWCND (input) REAL
* Ratio of the smallest R(i) to the largest R(i).
*
* COLCND (input) REAL
* Ratio of the smallest C(i) to the largest C(i).
*
* AMAX (input) REAL
* Absolute value of largest matrix entry.
*
* EQUED (output) CHARACTER*1
* Specifies the form of equilibration that was done.
* = 'N': No equilibration
* = 'R': Row equilibration, i.e., A has been premultiplied by
* diag(R).
* = 'C': Column equilibration, i.e., A has been postmultiplied
* by diag(C).
* = 'B': Both row and column equilibration, i.e., A has been
* replaced by diag(R) * A * diag(C).
*
* Internal Parameters
* ===================
*
* THRESH is a threshold value used to decide if row or column scaling
* should be done based on the ratio of the row or column scaling
* factors. If ROWCND < THRESH, row scaling is done, and if
* COLCND < THRESH, column scaling is done.
*
* LARGE and SMALL are threshold values used to decide if row scaling
* should be done based on the absolute size of the largest matrix
* element. If AMAX > LARGE or AMAX < SMALL, row scaling is done.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param kl
* @param ku
* @param ab
* @param ldab
* @param r
* @param c
* @param rowcnd
* @param colcnd
* @param amax
* @param equed
*
*/
abstract public void slaqgb(int m, int n, int kl, int ku, float[] ab, int ldab, float[] r, float[] c, float rowcnd, float colcnd, float amax, org.netlib.util.StringW equed);
/**
*
* ..
*
* Purpose
* =======
*
* SLAQGB equilibrates a general M by N band matrix A with KL
* subdiagonals and KU superdiagonals using the row and scaling factors
* in the vectors R and C.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* KL (input) INTEGER
* The number of subdiagonals within the band of A. KL >= 0.
*
* KU (input) INTEGER
* The number of superdiagonals within the band of A. KU >= 0.
*
* AB (input/output) REAL array, dimension (LDAB,N)
* On entry, the matrix A in band storage, in rows 1 to KL+KU+1.
* The j-th column of A is stored in the j-th column of the
* array AB as follows:
* AB(ku+1+i-j,j) = A(i,j) for max(1,j-ku)<=i<=min(m,j+kl)
*
* On exit, the equilibrated matrix, in the same storage format
* as A. See EQUED for the form of the equilibrated matrix.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDA >= KL+KU+1.
*
* R (input) REAL array, dimension (M)
* The row scale factors for A.
*
* C (input) REAL array, dimension (N)
* The column scale factors for A.
*
* ROWCND (input) REAL
* Ratio of the smallest R(i) to the largest R(i).
*
* COLCND (input) REAL
* Ratio of the smallest C(i) to the largest C(i).
*
* AMAX (input) REAL
* Absolute value of largest matrix entry.
*
* EQUED (output) CHARACTER*1
* Specifies the form of equilibration that was done.
* = 'N': No equilibration
* = 'R': Row equilibration, i.e., A has been premultiplied by
* diag(R).
* = 'C': Column equilibration, i.e., A has been postmultiplied
* by diag(C).
* = 'B': Both row and column equilibration, i.e., A has been
* replaced by diag(R) * A * diag(C).
*
* Internal Parameters
* ===================
*
* THRESH is a threshold value used to decide if row or column scaling
* should be done based on the ratio of the row or column scaling
* factors. If ROWCND < THRESH, row scaling is done, and if
* COLCND < THRESH, column scaling is done.
*
* LARGE and SMALL are threshold values used to decide if row scaling
* should be done based on the absolute size of the largest matrix
* element. If AMAX > LARGE or AMAX < SMALL, row scaling is done.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param kl
* @param ku
* @param ab
* @param _ab_offset
* @param ldab
* @param r
* @param _r_offset
* @param c
* @param _c_offset
* @param rowcnd
* @param colcnd
* @param amax
* @param equed
*
*/
abstract public void slaqgb(int m, int n, int kl, int ku, float[] ab, int _ab_offset, int ldab, float[] r, int _r_offset, float[] c, int _c_offset, float rowcnd, float colcnd, float amax, org.netlib.util.StringW equed);
/**
*
* ..
*
* Purpose
* =======
*
* SLAQGE equilibrates a general M by N matrix A using the row and
* column scaling factors in the vectors R and C.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M by N matrix A.
* On exit, the equilibrated matrix. See EQUED for the form of
* the equilibrated matrix.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(M,1).
*
* R (input) REAL array, dimension (M)
* The row scale factors for A.
*
* C (input) REAL array, dimension (N)
* The column scale factors for A.
*
* ROWCND (input) REAL
* Ratio of the smallest R(i) to the largest R(i).
*
* COLCND (input) REAL
* Ratio of the smallest C(i) to the largest C(i).
*
* AMAX (input) REAL
* Absolute value of largest matrix entry.
*
* EQUED (output) CHARACTER*1
* Specifies the form of equilibration that was done.
* = 'N': No equilibration
* = 'R': Row equilibration, i.e., A has been premultiplied by
* diag(R).
* = 'C': Column equilibration, i.e., A has been postmultiplied
* by diag(C).
* = 'B': Both row and column equilibration, i.e., A has been
* replaced by diag(R) * A * diag(C).
*
* Internal Parameters
* ===================
*
* THRESH is a threshold value used to decide if row or column scaling
* should be done based on the ratio of the row or column scaling
* factors. If ROWCND < THRESH, row scaling is done, and if
* COLCND < THRESH, column scaling is done.
*
* LARGE and SMALL are threshold values used to decide if row scaling
* should be done based on the absolute size of the largest matrix
* element. If AMAX > LARGE or AMAX < SMALL, row scaling is done.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param r
* @param c
* @param rowcnd
* @param colcnd
* @param amax
* @param equed
*
*/
abstract public void slaqge(int m, int n, float[] a, int lda, float[] r, float[] c, float rowcnd, float colcnd, float amax, org.netlib.util.StringW equed);
/**
*
* ..
*
* Purpose
* =======
*
* SLAQGE equilibrates a general M by N matrix A using the row and
* column scaling factors in the vectors R and C.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M by N matrix A.
* On exit, the equilibrated matrix. See EQUED for the form of
* the equilibrated matrix.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(M,1).
*
* R (input) REAL array, dimension (M)
* The row scale factors for A.
*
* C (input) REAL array, dimension (N)
* The column scale factors for A.
*
* ROWCND (input) REAL
* Ratio of the smallest R(i) to the largest R(i).
*
* COLCND (input) REAL
* Ratio of the smallest C(i) to the largest C(i).
*
* AMAX (input) REAL
* Absolute value of largest matrix entry.
*
* EQUED (output) CHARACTER*1
* Specifies the form of equilibration that was done.
* = 'N': No equilibration
* = 'R': Row equilibration, i.e., A has been premultiplied by
* diag(R).
* = 'C': Column equilibration, i.e., A has been postmultiplied
* by diag(C).
* = 'B': Both row and column equilibration, i.e., A has been
* replaced by diag(R) * A * diag(C).
*
* Internal Parameters
* ===================
*
* THRESH is a threshold value used to decide if row or column scaling
* should be done based on the ratio of the row or column scaling
* factors. If ROWCND < THRESH, row scaling is done, and if
* COLCND < THRESH, column scaling is done.
*
* LARGE and SMALL are threshold values used to decide if row scaling
* should be done based on the absolute size of the largest matrix
* element. If AMAX > LARGE or AMAX < SMALL, row scaling is done.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param r
* @param _r_offset
* @param c
* @param _c_offset
* @param rowcnd
* @param colcnd
* @param amax
* @param equed
*
*/
abstract public void slaqge(int m, int n, float[] a, int _a_offset, int lda, float[] r, int _r_offset, float[] c, int _c_offset, float rowcnd, float colcnd, float amax, org.netlib.util.StringW equed);
/**
*
* ..
*
* Purpose
* =======
*
* SLAQP2 computes a QR factorization with column pivoting of
* the block A(OFFSET+1:M,1:N).
* The block A(1:OFFSET,1:N) is accordingly pivoted, but not factorized.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* OFFSET (input) INTEGER
* The number of rows of the matrix A that must be pivoted
* but no factorized. OFFSET >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, the upper triangle of block A(OFFSET+1:M,1:N) is
* the triangular factor obtained; the elements in block
* A(OFFSET+1:M,1:N) below the diagonal, together with the
* array TAU, represent the orthogonal matrix Q as a product of
* elementary reflectors. Block A(1:OFFSET,1:N) has been
* accordingly pivoted, but no factorized.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* JPVT (input/output) INTEGER array, dimension (N)
* On entry, if JPVT(i) .ne. 0, the i-th column of A is permuted
* to the front of A*P (a leading column); if JPVT(i) = 0,
* the i-th column of A is a free column.
* On exit, if JPVT(i) = k, then the i-th column of A*P
* was the k-th column of A.
*
* TAU (output) REAL array, dimension (min(M,N))
* The scalar factors of the elementary reflectors.
*
* VN1 (input/output) REAL array, dimension (N)
* The vector with the partial column norms.
*
* VN2 (input/output) REAL array, dimension (N)
* The vector with the exact column norms.
*
* WORK (workspace) REAL array, dimension (N)
*
* Further Details
* ===============
*
* Based on contributions by
* G. Quintana-Orti, Depto. de Informatica, Universidad Jaime I, Spain
* X. Sun, Computer Science Dept., Duke University, USA
*
* Partial column norm updating strategy modified by
* Z. Drmac and Z. Bujanovic, Dept. of Mathematics,
* University of Zagreb, Croatia.
* June 2006.
* For more details see LAPACK Working Note 176.
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param offset
* @param a
* @param lda
* @param jpvt
* @param tau
* @param vn1
* @param vn2
* @param work
*
*/
abstract public void slaqp2(int m, int n, int offset, float[] a, int lda, int[] jpvt, float[] tau, float[] vn1, float[] vn2, float[] work);
/**
*
* ..
*
* Purpose
* =======
*
* SLAQP2 computes a QR factorization with column pivoting of
* the block A(OFFSET+1:M,1:N).
* The block A(1:OFFSET,1:N) is accordingly pivoted, but not factorized.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* OFFSET (input) INTEGER
* The number of rows of the matrix A that must be pivoted
* but no factorized. OFFSET >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, the upper triangle of block A(OFFSET+1:M,1:N) is
* the triangular factor obtained; the elements in block
* A(OFFSET+1:M,1:N) below the diagonal, together with the
* array TAU, represent the orthogonal matrix Q as a product of
* elementary reflectors. Block A(1:OFFSET,1:N) has been
* accordingly pivoted, but no factorized.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* JPVT (input/output) INTEGER array, dimension (N)
* On entry, if JPVT(i) .ne. 0, the i-th column of A is permuted
* to the front of A*P (a leading column); if JPVT(i) = 0,
* the i-th column of A is a free column.
* On exit, if JPVT(i) = k, then the i-th column of A*P
* was the k-th column of A.
*
* TAU (output) REAL array, dimension (min(M,N))
* The scalar factors of the elementary reflectors.
*
* VN1 (input/output) REAL array, dimension (N)
* The vector with the partial column norms.
*
* VN2 (input/output) REAL array, dimension (N)
* The vector with the exact column norms.
*
* WORK (workspace) REAL array, dimension (N)
*
* Further Details
* ===============
*
* Based on contributions by
* G. Quintana-Orti, Depto. de Informatica, Universidad Jaime I, Spain
* X. Sun, Computer Science Dept., Duke University, USA
*
* Partial column norm updating strategy modified by
* Z. Drmac and Z. Bujanovic, Dept. of Mathematics,
* University of Zagreb, Croatia.
* June 2006.
* For more details see LAPACK Working Note 176.
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param offset
* @param a
* @param _a_offset
* @param lda
* @param jpvt
* @param _jpvt_offset
* @param tau
* @param _tau_offset
* @param vn1
* @param _vn1_offset
* @param vn2
* @param _vn2_offset
* @param work
* @param _work_offset
*
*/
abstract public void slaqp2(int m, int n, int offset, float[] a, int _a_offset, int lda, int[] jpvt, int _jpvt_offset, float[] tau, int _tau_offset, float[] vn1, int _vn1_offset, float[] vn2, int _vn2_offset, float[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* SLAQPS computes a step of QR factorization with column pivoting
* of a real M-by-N matrix A by using Blas-3. It tries to factorize
* NB columns from A starting from the row OFFSET+1, and updates all
* of the matrix with Blas-3 xGEMM.
*
* In some cases, due to catastrophic cancellations, it cannot
* factorize NB columns. Hence, the actual number of factorized
* columns is returned in KB.
*
* Block A(1:OFFSET,1:N) is accordingly pivoted, but not factorized.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0
*
* OFFSET (input) INTEGER
* The number of rows of A that have been factorized in
* previous steps.
*
* NB (input) INTEGER
* The number of columns to factorize.
*
* KB (output) INTEGER
* The number of columns actually factorized.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, block A(OFFSET+1:M,1:KB) is the triangular
* factor obtained and block A(1:OFFSET,1:N) has been
* accordingly pivoted, but no factorized.
* The rest of the matrix, block A(OFFSET+1:M,KB+1:N) has
* been updated.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* JPVT (input/output) INTEGER array, dimension (N)
* JPVT(I) = K <==> Column K of the full matrix A has been
* permuted into position I in AP.
*
* TAU (output) REAL array, dimension (KB)
* The scalar factors of the elementary reflectors.
*
* VN1 (input/output) REAL array, dimension (N)
* The vector with the partial column norms.
*
* VN2 (input/output) REAL array, dimension (N)
* The vector with the exact column norms.
*
* AUXV (input/output) REAL array, dimension (NB)
* Auxiliar vector.
*
* F (input/output) REAL array, dimension (LDF,NB)
* Matrix F' = L*Y'*A.
*
* LDF (input) INTEGER
* The leading dimension of the array F. LDF >= max(1,N).
*
* Further Details
* ===============
*
* Based on contributions by
* G. Quintana-Orti, Depto. de Informatica, Universidad Jaime I, Spain
* X. Sun, Computer Science Dept., Duke University, USA
*
* Partial column norm updating strategy modified by
* Z. Drmac and Z. Bujanovic, Dept. of Mathematics,
* University of Zagreb, Croatia.
* June 2006.
* For more details see LAPACK Working Note 176.
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param offset
* @param nb
* @param kb
* @param a
* @param lda
* @param jpvt
* @param tau
* @param vn1
* @param vn2
* @param auxv
* @param f
* @param ldf
*
*/
abstract public void slaqps(int m, int n, int offset, int nb, org.netlib.util.intW kb, float[] a, int lda, int[] jpvt, float[] tau, float[] vn1, float[] vn2, float[] auxv, float[] f, int ldf);
/**
*
* ..
*
* Purpose
* =======
*
* SLAQPS computes a step of QR factorization with column pivoting
* of a real M-by-N matrix A by using Blas-3. It tries to factorize
* NB columns from A starting from the row OFFSET+1, and updates all
* of the matrix with Blas-3 xGEMM.
*
* In some cases, due to catastrophic cancellations, it cannot
* factorize NB columns. Hence, the actual number of factorized
* columns is returned in KB.
*
* Block A(1:OFFSET,1:N) is accordingly pivoted, but not factorized.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0
*
* OFFSET (input) INTEGER
* The number of rows of A that have been factorized in
* previous steps.
*
* NB (input) INTEGER
* The number of columns to factorize.
*
* KB (output) INTEGER
* The number of columns actually factorized.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, block A(OFFSET+1:M,1:KB) is the triangular
* factor obtained and block A(1:OFFSET,1:N) has been
* accordingly pivoted, but no factorized.
* The rest of the matrix, block A(OFFSET+1:M,KB+1:N) has
* been updated.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* JPVT (input/output) INTEGER array, dimension (N)
* JPVT(I) = K <==> Column K of the full matrix A has been
* permuted into position I in AP.
*
* TAU (output) REAL array, dimension (KB)
* The scalar factors of the elementary reflectors.
*
* VN1 (input/output) REAL array, dimension (N)
* The vector with the partial column norms.
*
* VN2 (input/output) REAL array, dimension (N)
* The vector with the exact column norms.
*
* AUXV (input/output) REAL array, dimension (NB)
* Auxiliar vector.
*
* F (input/output) REAL array, dimension (LDF,NB)
* Matrix F' = L*Y'*A.
*
* LDF (input) INTEGER
* The leading dimension of the array F. LDF >= max(1,N).
*
* Further Details
* ===============
*
* Based on contributions by
* G. Quintana-Orti, Depto. de Informatica, Universidad Jaime I, Spain
* X. Sun, Computer Science Dept., Duke University, USA
*
* Partial column norm updating strategy modified by
* Z. Drmac and Z. Bujanovic, Dept. of Mathematics,
* University of Zagreb, Croatia.
* June 2006.
* For more details see LAPACK Working Note 176.
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param offset
* @param nb
* @param kb
* @param a
* @param _a_offset
* @param lda
* @param jpvt
* @param _jpvt_offset
* @param tau
* @param _tau_offset
* @param vn1
* @param _vn1_offset
* @param vn2
* @param _vn2_offset
* @param auxv
* @param _auxv_offset
* @param f
* @param _f_offset
* @param ldf
*
*/
abstract public void slaqps(int m, int n, int offset, int nb, org.netlib.util.intW kb, float[] a, int _a_offset, int lda, int[] jpvt, int _jpvt_offset, float[] tau, int _tau_offset, float[] vn1, int _vn1_offset, float[] vn2, int _vn2_offset, float[] auxv, int _auxv_offset, float[] f, int _f_offset, int ldf);
/**
*
* ..
*
* Purpose
* =======
*
* SLAQR0 computes the eigenvalues of a Hessenberg matrix H
* and, optionally, the matrices T and Z from the Schur decomposition
* H = Z T Z**T, where T is an upper quasi-triangular matrix (the
* Schur form), and Z is the orthogonal matrix of Schur vectors.
*
* Optionally Z may be postmultiplied into an input orthogonal
* matrix Q so that this routine can give the Schur factorization
* of a matrix A which has been reduced to the Hessenberg form H
* by the orthogonal matrix Q: A = Q*H*Q**T = (QZ)*T*(QZ)**T.
*
* Arguments
* =========
*
* WANTT (input) LOGICAL
* = .TRUE. : the full Schur form T is required;
* = .FALSE.: only eigenvalues are required.
*
* WANTZ (input) LOGICAL
* = .TRUE. : the matrix of Schur vectors Z is required;
* = .FALSE.: Schur vectors are not required.
*
* N (input) INTEGER
* The order of the matrix H. N .GE. 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* It is assumed that H is already upper triangular in rows
* and columns 1:ILO-1 and IHI+1:N and, if ILO.GT.1,
* H(ILO,ILO-1) is zero. ILO and IHI are normally set by a
* previous call to SGEBAL, and then passed to SGEHRD when the
* matrix output by SGEBAL is reduced to Hessenberg form.
* Otherwise, ILO and IHI should be set to 1 and N,
* respectively. If N.GT.0, then 1.LE.ILO.LE.IHI.LE.N.
* If N = 0, then ILO = 1 and IHI = 0.
*
* H (input/output) REAL array, dimension (LDH,N)
* On entry, the upper Hessenberg matrix H.
* On exit, if INFO = 0 and WANTT is .TRUE., then H contains
* the upper quasi-triangular matrix T from the Schur
* decomposition (the Schur form); 2-by-2 diagonal blocks
* (corresponding to complex conjugate pairs of eigenvalues)
* are returned in standard form, with H(i,i) = H(i+1,i+1)
* and H(i+1,i)*H(i,i+1).LT.0. If INFO = 0 and WANTT is
* .FALSE., then the contents of H are unspecified on exit.
* (The output value of H when INFO.GT.0 is given under the
* description of INFO below.)
*
* This subroutine may explicitly set H(i,j) = 0 for i.GT.j and
* j = 1, 2, ... ILO-1 or j = IHI+1, IHI+2, ... N.
*
* LDH (input) INTEGER
* The leading dimension of the array H. LDH .GE. max(1,N).
*
* WR (output) REAL array, dimension (IHI)
* WI (output) REAL array, dimension (IHI)
* The real and imaginary parts, respectively, of the computed
* eigenvalues of H(ILO:IHI,ILO:IHI) are stored WR(ILO:IHI)
* and WI(ILO:IHI). If two eigenvalues are computed as a
* complex conjugate pair, they are stored in consecutive
* elements of WR and WI, say the i-th and (i+1)th, with
* WI(i) .GT. 0 and WI(i+1) .LT. 0. If WANTT is .TRUE., then
* the eigenvalues are stored in the same order as on the
* diagonal of the Schur form returned in H, with
* WR(i) = H(i,i) and, if H(i:i+1,i:i+1) is a 2-by-2 diagonal
* block, WI(i) = sqrt(-H(i+1,i)*H(i,i+1)) and
* WI(i+1) = -WI(i).
*
* ILOZ (input) INTEGER
* IHIZ (input) INTEGER
* Specify the rows of Z to which transformations must be
* applied if WANTZ is .TRUE..
* 1 .LE. ILOZ .LE. ILO; IHI .LE. IHIZ .LE. N.
*
* Z (input/output) REAL array, dimension (LDZ,IHI)
* If WANTZ is .FALSE., then Z is not referenced.
* If WANTZ is .TRUE., then Z(ILO:IHI,ILOZ:IHIZ) is
* replaced by Z(ILO:IHI,ILOZ:IHIZ)*U where U is the
* orthogonal Schur factor of H(ILO:IHI,ILO:IHI).
* (The output value of Z when INFO.GT.0 is given under
* the description of INFO below.)
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. if WANTZ is .TRUE.
* then LDZ.GE.MAX(1,IHIZ). Otherwize, LDZ.GE.1.
*
* WORK (workspace/output) REAL array, dimension LWORK
* On exit, if LWORK = -1, WORK(1) returns an estimate of
* the optimal value for LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK .GE. max(1,N)
* is sufficient, but LWORK typically as large as 6*N may
* be required for optimal performance. A workspace query
* to determine the optimal workspace size is recommended.
*
* If LWORK = -1, then SLAQR0 does a workspace query.
* In this case, SLAQR0 checks the input parameters and
* estimates the optimal workspace size for the given
* values of N, ILO and IHI. The estimate is returned
* in WORK(1). No error message related to LWORK is
* issued by XERBLA. Neither H nor Z are accessed.
*
*
* INFO (output) INTEGER
* = 0: successful exit
* .GT. 0: if INFO = i, SLAQR0 failed to compute all of
* the eigenvalues. Elements 1:ilo-1 and i+1:n of WR
* and WI contain those eigenvalues which have been
* successfully computed. (Failures are rare.)
*
* If INFO .GT. 0 and WANT is .FALSE., then on exit,
* the remaining unconverged eigenvalues are the eigen-
* values of the upper Hessenberg matrix rows and
* columns ILO through INFO of the final, output
* value of H.
*
* If INFO .GT. 0 and WANTT is .TRUE., then on exit
*
* (*) (initial value of H)*U = U*(final value of H)
*
* where U is an orthogonal matrix. The final
* value of H is upper Hessenberg and quasi-triangular
* in rows and columns INFO+1 through IHI.
*
* If INFO .GT. 0 and WANTZ is .TRUE., then on exit
*
* (final value of Z(ILO:IHI,ILOZ:IHIZ)
* = (initial value of Z(ILO:IHI,ILOZ:IHIZ)*U
*
* where U is the orthogonal matrix in (*) (regard-
* less of the value of WANTT.)
*
* If INFO .GT. 0 and WANTZ is .FALSE., then Z is not
* accessed.
*
* ================================================================
* Based on contributions by
* Karen Braman and Ralph Byers, Department of Mathematics,
* University of Kansas, USA
*
* ================================================================
* References:
* K. Braman, R. Byers and R. Mathias, The Multi-Shift QR
* Algorithm Part I: Maintaining Well Focused Shifts, and Level 3
* Performance, SIAM Journal of Matrix Analysis, volume 23, pages
* 929--947, 2002.
*
* K. Braman, R. Byers and R. Mathias, The Multi-Shift QR
* Algorithm Part II: Aggressive Early Deflation, SIAM Journal
* of Matrix Analysis, volume 23, pages 948--973, 2002.
*
* ================================================================
* .. Parameters ..
*
* ==== Matrices of order NTINY or smaller must be processed by
* . SLAHQR because of insufficient subdiagonal scratch space.
* . (This is a hard limit.) ====
*
* ==== Exceptional deflation windows: try to cure rare
* . slow convergence by increasing the size of the
* . deflation window after KEXNW iterations. =====
*
* ==== Exceptional shifts: try to cure rare slow convergence
* . with ad-hoc exceptional shifts every KEXSH iterations.
* . The constants WILK1 and WILK2 are used to form the
* . exceptional shifts. ====
*
*
*
* @param wantt
* @param wantz
* @param n
* @param ilo
* @param ihi
* @param h
* @param ldh
* @param wr
* @param wi
* @param iloz
* @param ihiz
* @param z
* @param ldz
* @param work
* @param lwork
* @param info
*
*/
abstract public void slaqr0(boolean wantt, boolean wantz, int n, int ilo, int ihi, float[] h, int ldh, float[] wr, float[] wi, int iloz, int ihiz, float[] z, int ldz, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAQR0 computes the eigenvalues of a Hessenberg matrix H
* and, optionally, the matrices T and Z from the Schur decomposition
* H = Z T Z**T, where T is an upper quasi-triangular matrix (the
* Schur form), and Z is the orthogonal matrix of Schur vectors.
*
* Optionally Z may be postmultiplied into an input orthogonal
* matrix Q so that this routine can give the Schur factorization
* of a matrix A which has been reduced to the Hessenberg form H
* by the orthogonal matrix Q: A = Q*H*Q**T = (QZ)*T*(QZ)**T.
*
* Arguments
* =========
*
* WANTT (input) LOGICAL
* = .TRUE. : the full Schur form T is required;
* = .FALSE.: only eigenvalues are required.
*
* WANTZ (input) LOGICAL
* = .TRUE. : the matrix of Schur vectors Z is required;
* = .FALSE.: Schur vectors are not required.
*
* N (input) INTEGER
* The order of the matrix H. N .GE. 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* It is assumed that H is already upper triangular in rows
* and columns 1:ILO-1 and IHI+1:N and, if ILO.GT.1,
* H(ILO,ILO-1) is zero. ILO and IHI are normally set by a
* previous call to SGEBAL, and then passed to SGEHRD when the
* matrix output by SGEBAL is reduced to Hessenberg form.
* Otherwise, ILO and IHI should be set to 1 and N,
* respectively. If N.GT.0, then 1.LE.ILO.LE.IHI.LE.N.
* If N = 0, then ILO = 1 and IHI = 0.
*
* H (input/output) REAL array, dimension (LDH,N)
* On entry, the upper Hessenberg matrix H.
* On exit, if INFO = 0 and WANTT is .TRUE., then H contains
* the upper quasi-triangular matrix T from the Schur
* decomposition (the Schur form); 2-by-2 diagonal blocks
* (corresponding to complex conjugate pairs of eigenvalues)
* are returned in standard form, with H(i,i) = H(i+1,i+1)
* and H(i+1,i)*H(i,i+1).LT.0. If INFO = 0 and WANTT is
* .FALSE., then the contents of H are unspecified on exit.
* (The output value of H when INFO.GT.0 is given under the
* description of INFO below.)
*
* This subroutine may explicitly set H(i,j) = 0 for i.GT.j and
* j = 1, 2, ... ILO-1 or j = IHI+1, IHI+2, ... N.
*
* LDH (input) INTEGER
* The leading dimension of the array H. LDH .GE. max(1,N).
*
* WR (output) REAL array, dimension (IHI)
* WI (output) REAL array, dimension (IHI)
* The real and imaginary parts, respectively, of the computed
* eigenvalues of H(ILO:IHI,ILO:IHI) are stored WR(ILO:IHI)
* and WI(ILO:IHI). If two eigenvalues are computed as a
* complex conjugate pair, they are stored in consecutive
* elements of WR and WI, say the i-th and (i+1)th, with
* WI(i) .GT. 0 and WI(i+1) .LT. 0. If WANTT is .TRUE., then
* the eigenvalues are stored in the same order as on the
* diagonal of the Schur form returned in H, with
* WR(i) = H(i,i) and, if H(i:i+1,i:i+1) is a 2-by-2 diagonal
* block, WI(i) = sqrt(-H(i+1,i)*H(i,i+1)) and
* WI(i+1) = -WI(i).
*
* ILOZ (input) INTEGER
* IHIZ (input) INTEGER
* Specify the rows of Z to which transformations must be
* applied if WANTZ is .TRUE..
* 1 .LE. ILOZ .LE. ILO; IHI .LE. IHIZ .LE. N.
*
* Z (input/output) REAL array, dimension (LDZ,IHI)
* If WANTZ is .FALSE., then Z is not referenced.
* If WANTZ is .TRUE., then Z(ILO:IHI,ILOZ:IHIZ) is
* replaced by Z(ILO:IHI,ILOZ:IHIZ)*U where U is the
* orthogonal Schur factor of H(ILO:IHI,ILO:IHI).
* (The output value of Z when INFO.GT.0 is given under
* the description of INFO below.)
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. if WANTZ is .TRUE.
* then LDZ.GE.MAX(1,IHIZ). Otherwize, LDZ.GE.1.
*
* WORK (workspace/output) REAL array, dimension LWORK
* On exit, if LWORK = -1, WORK(1) returns an estimate of
* the optimal value for LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK .GE. max(1,N)
* is sufficient, but LWORK typically as large as 6*N may
* be required for optimal performance. A workspace query
* to determine the optimal workspace size is recommended.
*
* If LWORK = -1, then SLAQR0 does a workspace query.
* In this case, SLAQR0 checks the input parameters and
* estimates the optimal workspace size for the given
* values of N, ILO and IHI. The estimate is returned
* in WORK(1). No error message related to LWORK is
* issued by XERBLA. Neither H nor Z are accessed.
*
*
* INFO (output) INTEGER
* = 0: successful exit
* .GT. 0: if INFO = i, SLAQR0 failed to compute all of
* the eigenvalues. Elements 1:ilo-1 and i+1:n of WR
* and WI contain those eigenvalues which have been
* successfully computed. (Failures are rare.)
*
* If INFO .GT. 0 and WANT is .FALSE., then on exit,
* the remaining unconverged eigenvalues are the eigen-
* values of the upper Hessenberg matrix rows and
* columns ILO through INFO of the final, output
* value of H.
*
* If INFO .GT. 0 and WANTT is .TRUE., then on exit
*
* (*) (initial value of H)*U = U*(final value of H)
*
* where U is an orthogonal matrix. The final
* value of H is upper Hessenberg and quasi-triangular
* in rows and columns INFO+1 through IHI.
*
* If INFO .GT. 0 and WANTZ is .TRUE., then on exit
*
* (final value of Z(ILO:IHI,ILOZ:IHIZ)
* = (initial value of Z(ILO:IHI,ILOZ:IHIZ)*U
*
* where U is the orthogonal matrix in (*) (regard-
* less of the value of WANTT.)
*
* If INFO .GT. 0 and WANTZ is .FALSE., then Z is not
* accessed.
*
* ================================================================
* Based on contributions by
* Karen Braman and Ralph Byers, Department of Mathematics,
* University of Kansas, USA
*
* ================================================================
* References:
* K. Braman, R. Byers and R. Mathias, The Multi-Shift QR
* Algorithm Part I: Maintaining Well Focused Shifts, and Level 3
* Performance, SIAM Journal of Matrix Analysis, volume 23, pages
* 929--947, 2002.
*
* K. Braman, R. Byers and R. Mathias, The Multi-Shift QR
* Algorithm Part II: Aggressive Early Deflation, SIAM Journal
* of Matrix Analysis, volume 23, pages 948--973, 2002.
*
* ================================================================
* .. Parameters ..
*
* ==== Matrices of order NTINY or smaller must be processed by
* . SLAHQR because of insufficient subdiagonal scratch space.
* . (This is a hard limit.) ====
*
* ==== Exceptional deflation windows: try to cure rare
* . slow convergence by increasing the size of the
* . deflation window after KEXNW iterations. =====
*
* ==== Exceptional shifts: try to cure rare slow convergence
* . with ad-hoc exceptional shifts every KEXSH iterations.
* . The constants WILK1 and WILK2 are used to form the
* . exceptional shifts. ====
*
*
*
* @param wantt
* @param wantz
* @param n
* @param ilo
* @param ihi
* @param h
* @param _h_offset
* @param ldh
* @param wr
* @param _wr_offset
* @param wi
* @param _wi_offset
* @param iloz
* @param ihiz
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void slaqr0(boolean wantt, boolean wantz, int n, int ilo, int ihi, float[] h, int _h_offset, int ldh, float[] wr, int _wr_offset, float[] wi, int _wi_offset, int iloz, int ihiz, float[] z, int _z_offset, int ldz, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Given a 2-by-2 or 3-by-3 matrix H, SLAQR1 sets v to a
* scalar multiple of the first column of the product
*
* (*) K = (H - (sr1 + i*si1)*I)*(H - (sr2 + i*si2)*I)
*
* scaling to avoid overflows and most underflows. It
* is assumed that either
*
* 1) sr1 = sr2 and si1 = -si2
* or
* 2) si1 = si2 = 0.
*
* This is useful for starting double implicit shift bulges
* in the QR algorithm.
*
*
* N (input) integer
* Order of the matrix H. N must be either 2 or 3.
*
* H (input) REAL array of dimension (LDH,N)
* The 2-by-2 or 3-by-3 matrix H in (*).
*
* LDH (input) integer
* The leading dimension of H as declared in
* the calling procedure. LDH.GE.N
*
* SR1 (input) REAL
* SI1 The shifts in (*).
* SR2
* SI2
*
* V (output) REAL array of dimension N
* A scalar multiple of the first column of the
* matrix K in (*).
*
* ================================================================
* Based on contributions by
* Karen Braman and Ralph Byers, Department of Mathematics,
* University of Kansas, USA
*
* ================================================================
*
* .. Parameters ..
*
*
* @param n
* @param h
* @param ldh
* @param sr1
* @param si1
* @param sr2
* @param si2
* @param v
*
*/
abstract public void slaqr1(int n, float[] h, int ldh, float sr1, float si1, float sr2, float si2, float[] v);
/**
*
* ..
*
* Given a 2-by-2 or 3-by-3 matrix H, SLAQR1 sets v to a
* scalar multiple of the first column of the product
*
* (*) K = (H - (sr1 + i*si1)*I)*(H - (sr2 + i*si2)*I)
*
* scaling to avoid overflows and most underflows. It
* is assumed that either
*
* 1) sr1 = sr2 and si1 = -si2
* or
* 2) si1 = si2 = 0.
*
* This is useful for starting double implicit shift bulges
* in the QR algorithm.
*
*
* N (input) integer
* Order of the matrix H. N must be either 2 or 3.
*
* H (input) REAL array of dimension (LDH,N)
* The 2-by-2 or 3-by-3 matrix H in (*).
*
* LDH (input) integer
* The leading dimension of H as declared in
* the calling procedure. LDH.GE.N
*
* SR1 (input) REAL
* SI1 The shifts in (*).
* SR2
* SI2
*
* V (output) REAL array of dimension N
* A scalar multiple of the first column of the
* matrix K in (*).
*
* ================================================================
* Based on contributions by
* Karen Braman and Ralph Byers, Department of Mathematics,
* University of Kansas, USA
*
* ================================================================
*
* .. Parameters ..
*
*
* @param n
* @param h
* @param _h_offset
* @param ldh
* @param sr1
* @param si1
* @param sr2
* @param si2
* @param v
* @param _v_offset
*
*/
abstract public void slaqr1(int n, float[] h, int _h_offset, int ldh, float sr1, float si1, float sr2, float si2, float[] v, int _v_offset);
/**
*
* ..
*
* This subroutine is identical to SLAQR3 except that it avoids
* recursion by calling SLAHQR instead of SLAQR4.
*
*
* ******************************************************************
* Aggressive early deflation:
*
* This subroutine accepts as input an upper Hessenberg matrix
* H and performs an orthogonal similarity transformation
* designed to detect and deflate fully converged eigenvalues from
* a trailing principal submatrix. On output H has been over-
* written by a new Hessenberg matrix that is a perturbation of
* an orthogonal similarity transformation of H. It is to be
* hoped that the final version of H has many zero subdiagonal
* entries.
*
* ******************************************************************
* WANTT (input) LOGICAL
* If .TRUE., then the Hessenberg matrix H is fully updated
* so that the quasi-triangular Schur factor may be
* computed (in cooperation with the calling subroutine).
* If .FALSE., then only enough of H is updated to preserve
* the eigenvalues.
*
* WANTZ (input) LOGICAL
* If .TRUE., then the orthogonal matrix Z is updated so
* so that the orthogonal Schur factor may be computed
* (in cooperation with the calling subroutine).
* If .FALSE., then Z is not referenced.
*
* N (input) INTEGER
* The order of the matrix H and (if WANTZ is .TRUE.) the
* order of the orthogonal matrix Z.
*
* KTOP (input) INTEGER
* It is assumed that either KTOP = 1 or H(KTOP,KTOP-1)=0.
* KBOT and KTOP together determine an isolated block
* along the diagonal of the Hessenberg matrix.
*
* KBOT (input) INTEGER
* It is assumed without a check that either
* KBOT = N or H(KBOT+1,KBOT)=0. KBOT and KTOP together
* determine an isolated block along the diagonal of the
* Hessenberg matrix.
*
* NW (input) INTEGER
* Deflation window size. 1 .LE. NW .LE. (KBOT-KTOP+1).
*
* H (input/output) REAL array, dimension (LDH,N)
* On input the initial N-by-N section of H stores the
* Hessenberg matrix undergoing aggressive early deflation.
* On output H has been transformed by an orthogonal
* similarity transformation, perturbed, and the returned
* to Hessenberg form that (it is to be hoped) has some
* zero subdiagonal entries.
*
* LDH (input) integer
* Leading dimension of H just as declared in the calling
* subroutine. N .LE. LDH
*
* ILOZ (input) INTEGER
* IHIZ (input) INTEGER
* Specify the rows of Z to which transformations must be
* applied if WANTZ is .TRUE.. 1 .LE. ILOZ .LE. IHIZ .LE. N.
*
* Z (input/output) REAL array, dimension (LDZ,IHI)
* IF WANTZ is .TRUE., then on output, the orthogonal
* similarity transformation mentioned above has been
* accumulated into Z(ILOZ:IHIZ,ILO:IHI) from the right.
* If WANTZ is .FALSE., then Z is unreferenced.
*
* LDZ (input) integer
* The leading dimension of Z just as declared in the
* calling subroutine. 1 .LE. LDZ.
*
* NS (output) integer
* The number of unconverged (ie approximate) eigenvalues
* returned in SR and SI that may be used as shifts by the
* calling subroutine.
*
* ND (output) integer
* The number of converged eigenvalues uncovered by this
* subroutine.
*
* SR (output) REAL array, dimension KBOT
* SI (output) REAL array, dimension KBOT
* On output, the real and imaginary parts of approximate
* eigenvalues that may be used for shifts are stored in
* SR(KBOT-ND-NS+1) through SR(KBOT-ND) and
* SI(KBOT-ND-NS+1) through SI(KBOT-ND), respectively.
* The real and imaginary parts of converged eigenvalues
* are stored in SR(KBOT-ND+1) through SR(KBOT) and
* SI(KBOT-ND+1) through SI(KBOT), respectively.
*
* V (workspace) REAL array, dimension (LDV,NW)
* An NW-by-NW work array.
*
* LDV (input) integer scalar
* The leading dimension of V just as declared in the
* calling subroutine. NW .LE. LDV
*
* NH (input) integer scalar
* The number of columns of T. NH.GE.NW.
*
* T (workspace) REAL array, dimension (LDT,NW)
*
* LDT (input) integer
* The leading dimension of T just as declared in the
* calling subroutine. NW .LE. LDT
*
* NV (input) integer
* The number of rows of work array WV available for
* workspace. NV.GE.NW.
*
* WV (workspace) REAL array, dimension (LDWV,NW)
*
* LDWV (input) integer
* The leading dimension of W just as declared in the
* calling subroutine. NW .LE. LDV
*
* WORK (workspace) REAL array, dimension LWORK.
* On exit, WORK(1) is set to an estimate of the optimal value
* of LWORK for the given values of N, NW, KTOP and KBOT.
*
* LWORK (input) integer
* The dimension of the work array WORK. LWORK = 2*NW
* suffices, but greater efficiency may result from larger
* values of LWORK.
*
* If LWORK = -1, then a workspace query is assumed; SLAQR2
* only estimates the optimal workspace size for the given
* values of N, NW, KTOP and KBOT. The estimate is returned
* in WORK(1). No error message related to LWORK is issued
* by XERBLA. Neither H nor Z are accessed.
*
* ================================================================
* Based on contributions by
* Karen Braman and Ralph Byers, Department of Mathematics,
* University of Kansas, USA
*
* ==================================================================
* .. Parameters ..
*
*
* @param wantt
* @param wantz
* @param n
* @param ktop
* @param kbot
* @param nw
* @param h
* @param ldh
* @param iloz
* @param ihiz
* @param z
* @param ldz
* @param ns
* @param nd
* @param sr
* @param si
* @param v
* @param ldv
* @param nh
* @param t
* @param ldt
* @param nv
* @param wv
* @param ldwv
* @param work
* @param lwork
*
*/
abstract public void slaqr2(boolean wantt, boolean wantz, int n, int ktop, int kbot, int nw, float[] h, int ldh, int iloz, int ihiz, float[] z, int ldz, org.netlib.util.intW ns, org.netlib.util.intW nd, float[] sr, float[] si, float[] v, int ldv, int nh, float[] t, int ldt, int nv, float[] wv, int ldwv, float[] work, int lwork);
/**
*
* ..
*
* This subroutine is identical to SLAQR3 except that it avoids
* recursion by calling SLAHQR instead of SLAQR4.
*
*
* ******************************************************************
* Aggressive early deflation:
*
* This subroutine accepts as input an upper Hessenberg matrix
* H and performs an orthogonal similarity transformation
* designed to detect and deflate fully converged eigenvalues from
* a trailing principal submatrix. On output H has been over-
* written by a new Hessenberg matrix that is a perturbation of
* an orthogonal similarity transformation of H. It is to be
* hoped that the final version of H has many zero subdiagonal
* entries.
*
* ******************************************************************
* WANTT (input) LOGICAL
* If .TRUE., then the Hessenberg matrix H is fully updated
* so that the quasi-triangular Schur factor may be
* computed (in cooperation with the calling subroutine).
* If .FALSE., then only enough of H is updated to preserve
* the eigenvalues.
*
* WANTZ (input) LOGICAL
* If .TRUE., then the orthogonal matrix Z is updated so
* so that the orthogonal Schur factor may be computed
* (in cooperation with the calling subroutine).
* If .FALSE., then Z is not referenced.
*
* N (input) INTEGER
* The order of the matrix H and (if WANTZ is .TRUE.) the
* order of the orthogonal matrix Z.
*
* KTOP (input) INTEGER
* It is assumed that either KTOP = 1 or H(KTOP,KTOP-1)=0.
* KBOT and KTOP together determine an isolated block
* along the diagonal of the Hessenberg matrix.
*
* KBOT (input) INTEGER
* It is assumed without a check that either
* KBOT = N or H(KBOT+1,KBOT)=0. KBOT and KTOP together
* determine an isolated block along the diagonal of the
* Hessenberg matrix.
*
* NW (input) INTEGER
* Deflation window size. 1 .LE. NW .LE. (KBOT-KTOP+1).
*
* H (input/output) REAL array, dimension (LDH,N)
* On input the initial N-by-N section of H stores the
* Hessenberg matrix undergoing aggressive early deflation.
* On output H has been transformed by an orthogonal
* similarity transformation, perturbed, and the returned
* to Hessenberg form that (it is to be hoped) has some
* zero subdiagonal entries.
*
* LDH (input) integer
* Leading dimension of H just as declared in the calling
* subroutine. N .LE. LDH
*
* ILOZ (input) INTEGER
* IHIZ (input) INTEGER
* Specify the rows of Z to which transformations must be
* applied if WANTZ is .TRUE.. 1 .LE. ILOZ .LE. IHIZ .LE. N.
*
* Z (input/output) REAL array, dimension (LDZ,IHI)
* IF WANTZ is .TRUE., then on output, the orthogonal
* similarity transformation mentioned above has been
* accumulated into Z(ILOZ:IHIZ,ILO:IHI) from the right.
* If WANTZ is .FALSE., then Z is unreferenced.
*
* LDZ (input) integer
* The leading dimension of Z just as declared in the
* calling subroutine. 1 .LE. LDZ.
*
* NS (output) integer
* The number of unconverged (ie approximate) eigenvalues
* returned in SR and SI that may be used as shifts by the
* calling subroutine.
*
* ND (output) integer
* The number of converged eigenvalues uncovered by this
* subroutine.
*
* SR (output) REAL array, dimension KBOT
* SI (output) REAL array, dimension KBOT
* On output, the real and imaginary parts of approximate
* eigenvalues that may be used for shifts are stored in
* SR(KBOT-ND-NS+1) through SR(KBOT-ND) and
* SI(KBOT-ND-NS+1) through SI(KBOT-ND), respectively.
* The real and imaginary parts of converged eigenvalues
* are stored in SR(KBOT-ND+1) through SR(KBOT) and
* SI(KBOT-ND+1) through SI(KBOT), respectively.
*
* V (workspace) REAL array, dimension (LDV,NW)
* An NW-by-NW work array.
*
* LDV (input) integer scalar
* The leading dimension of V just as declared in the
* calling subroutine. NW .LE. LDV
*
* NH (input) integer scalar
* The number of columns of T. NH.GE.NW.
*
* T (workspace) REAL array, dimension (LDT,NW)
*
* LDT (input) integer
* The leading dimension of T just as declared in the
* calling subroutine. NW .LE. LDT
*
* NV (input) integer
* The number of rows of work array WV available for
* workspace. NV.GE.NW.
*
* WV (workspace) REAL array, dimension (LDWV,NW)
*
* LDWV (input) integer
* The leading dimension of W just as declared in the
* calling subroutine. NW .LE. LDV
*
* WORK (workspace) REAL array, dimension LWORK.
* On exit, WORK(1) is set to an estimate of the optimal value
* of LWORK for the given values of N, NW, KTOP and KBOT.
*
* LWORK (input) integer
* The dimension of the work array WORK. LWORK = 2*NW
* suffices, but greater efficiency may result from larger
* values of LWORK.
*
* If LWORK = -1, then a workspace query is assumed; SLAQR2
* only estimates the optimal workspace size for the given
* values of N, NW, KTOP and KBOT. The estimate is returned
* in WORK(1). No error message related to LWORK is issued
* by XERBLA. Neither H nor Z are accessed.
*
* ================================================================
* Based on contributions by
* Karen Braman and Ralph Byers, Department of Mathematics,
* University of Kansas, USA
*
* ==================================================================
* .. Parameters ..
*
*
* @param wantt
* @param wantz
* @param n
* @param ktop
* @param kbot
* @param nw
* @param h
* @param _h_offset
* @param ldh
* @param iloz
* @param ihiz
* @param z
* @param _z_offset
* @param ldz
* @param ns
* @param nd
* @param sr
* @param _sr_offset
* @param si
* @param _si_offset
* @param v
* @param _v_offset
* @param ldv
* @param nh
* @param t
* @param _t_offset
* @param ldt
* @param nv
* @param wv
* @param _wv_offset
* @param ldwv
* @param work
* @param _work_offset
* @param lwork
*
*/
abstract public void slaqr2(boolean wantt, boolean wantz, int n, int ktop, int kbot, int nw, float[] h, int _h_offset, int ldh, int iloz, int ihiz, float[] z, int _z_offset, int ldz, org.netlib.util.intW ns, org.netlib.util.intW nd, float[] sr, int _sr_offset, float[] si, int _si_offset, float[] v, int _v_offset, int ldv, int nh, float[] t, int _t_offset, int ldt, int nv, float[] wv, int _wv_offset, int ldwv, float[] work, int _work_offset, int lwork);
/**
*
* ..
*
* ******************************************************************
* Aggressive early deflation:
*
* This subroutine accepts as input an upper Hessenberg matrix
* H and performs an orthogonal similarity transformation
* designed to detect and deflate fully converged eigenvalues from
* a trailing principal submatrix. On output H has been over-
* written by a new Hessenberg matrix that is a perturbation of
* an orthogonal similarity transformation of H. It is to be
* hoped that the final version of H has many zero subdiagonal
* entries.
*
* ******************************************************************
* WANTT (input) LOGICAL
* If .TRUE., then the Hessenberg matrix H is fully updated
* so that the quasi-triangular Schur factor may be
* computed (in cooperation with the calling subroutine).
* If .FALSE., then only enough of H is updated to preserve
* the eigenvalues.
*
* WANTZ (input) LOGICAL
* If .TRUE., then the orthogonal matrix Z is updated so
* so that the orthogonal Schur factor may be computed
* (in cooperation with the calling subroutine).
* If .FALSE., then Z is not referenced.
*
* N (input) INTEGER
* The order of the matrix H and (if WANTZ is .TRUE.) the
* order of the orthogonal matrix Z.
*
* KTOP (input) INTEGER
* It is assumed that either KTOP = 1 or H(KTOP,KTOP-1)=0.
* KBOT and KTOP together determine an isolated block
* along the diagonal of the Hessenberg matrix.
*
* KBOT (input) INTEGER
* It is assumed without a check that either
* KBOT = N or H(KBOT+1,KBOT)=0. KBOT and KTOP together
* determine an isolated block along the diagonal of the
* Hessenberg matrix.
*
* NW (input) INTEGER
* Deflation window size. 1 .LE. NW .LE. (KBOT-KTOP+1).
*
* H (input/output) REAL array, dimension (LDH,N)
* On input the initial N-by-N section of H stores the
* Hessenberg matrix undergoing aggressive early deflation.
* On output H has been transformed by an orthogonal
* similarity transformation, perturbed, and the returned
* to Hessenberg form that (it is to be hoped) has some
* zero subdiagonal entries.
*
* LDH (input) integer
* Leading dimension of H just as declared in the calling
* subroutine. N .LE. LDH
*
* ILOZ (input) INTEGER
* IHIZ (input) INTEGER
* Specify the rows of Z to which transformations must be
* applied if WANTZ is .TRUE.. 1 .LE. ILOZ .LE. IHIZ .LE. N.
*
* Z (input/output) REAL array, dimension (LDZ,IHI)
* IF WANTZ is .TRUE., then on output, the orthogonal
* similarity transformation mentioned above has been
* accumulated into Z(ILOZ:IHIZ,ILO:IHI) from the right.
* If WANTZ is .FALSE., then Z is unreferenced.
*
* LDZ (input) integer
* The leading dimension of Z just as declared in the
* calling subroutine. 1 .LE. LDZ.
*
* NS (output) integer
* The number of unconverged (ie approximate) eigenvalues
* returned in SR and SI that may be used as shifts by the
* calling subroutine.
*
* ND (output) integer
* The number of converged eigenvalues uncovered by this
* subroutine.
*
* SR (output) REAL array, dimension KBOT
* SI (output) REAL array, dimension KBOT
* On output, the real and imaginary parts of approximate
* eigenvalues that may be used for shifts are stored in
* SR(KBOT-ND-NS+1) through SR(KBOT-ND) and
* SI(KBOT-ND-NS+1) through SI(KBOT-ND), respectively.
* The real and imaginary parts of converged eigenvalues
* are stored in SR(KBOT-ND+1) through SR(KBOT) and
* SI(KBOT-ND+1) through SI(KBOT), respectively.
*
* V (workspace) REAL array, dimension (LDV,NW)
* An NW-by-NW work array.
*
* LDV (input) integer scalar
* The leading dimension of V just as declared in the
* calling subroutine. NW .LE. LDV
*
* NH (input) integer scalar
* The number of columns of T. NH.GE.NW.
*
* T (workspace) REAL array, dimension (LDT,NW)
*
* LDT (input) integer
* The leading dimension of T just as declared in the
* calling subroutine. NW .LE. LDT
*
* NV (input) integer
* The number of rows of work array WV available for
* workspace. NV.GE.NW.
*
* WV (workspace) REAL array, dimension (LDWV,NW)
*
* LDWV (input) integer
* The leading dimension of W just as declared in the
* calling subroutine. NW .LE. LDV
*
* WORK (workspace) REAL array, dimension LWORK.
* On exit, WORK(1) is set to an estimate of the optimal value
* of LWORK for the given values of N, NW, KTOP and KBOT.
*
* LWORK (input) integer
* The dimension of the work array WORK. LWORK = 2*NW
* suffices, but greater efficiency may result from larger
* values of LWORK.
*
* If LWORK = -1, then a workspace query is assumed; SLAQR3
* only estimates the optimal workspace size for the given
* values of N, NW, KTOP and KBOT. The estimate is returned
* in WORK(1). No error message related to LWORK is issued
* by XERBLA. Neither H nor Z are accessed.
*
* ================================================================
* Based on contributions by
* Karen Braman and Ralph Byers, Department of Mathematics,
* University of Kansas, USA
*
* ==================================================================
* .. Parameters ..
*
*
* @param wantt
* @param wantz
* @param n
* @param ktop
* @param kbot
* @param nw
* @param h
* @param ldh
* @param iloz
* @param ihiz
* @param z
* @param ldz
* @param ns
* @param nd
* @param sr
* @param si
* @param v
* @param ldv
* @param nh
* @param t
* @param ldt
* @param nv
* @param wv
* @param ldwv
* @param work
* @param lwork
*
*/
abstract public void slaqr3(boolean wantt, boolean wantz, int n, int ktop, int kbot, int nw, float[] h, int ldh, int iloz, int ihiz, float[] z, int ldz, org.netlib.util.intW ns, org.netlib.util.intW nd, float[] sr, float[] si, float[] v, int ldv, int nh, float[] t, int ldt, int nv, float[] wv, int ldwv, float[] work, int lwork);
/**
*
* ..
*
* ******************************************************************
* Aggressive early deflation:
*
* This subroutine accepts as input an upper Hessenberg matrix
* H and performs an orthogonal similarity transformation
* designed to detect and deflate fully converged eigenvalues from
* a trailing principal submatrix. On output H has been over-
* written by a new Hessenberg matrix that is a perturbation of
* an orthogonal similarity transformation of H. It is to be
* hoped that the final version of H has many zero subdiagonal
* entries.
*
* ******************************************************************
* WANTT (input) LOGICAL
* If .TRUE., then the Hessenberg matrix H is fully updated
* so that the quasi-triangular Schur factor may be
* computed (in cooperation with the calling subroutine).
* If .FALSE., then only enough of H is updated to preserve
* the eigenvalues.
*
* WANTZ (input) LOGICAL
* If .TRUE., then the orthogonal matrix Z is updated so
* so that the orthogonal Schur factor may be computed
* (in cooperation with the calling subroutine).
* If .FALSE., then Z is not referenced.
*
* N (input) INTEGER
* The order of the matrix H and (if WANTZ is .TRUE.) the
* order of the orthogonal matrix Z.
*
* KTOP (input) INTEGER
* It is assumed that either KTOP = 1 or H(KTOP,KTOP-1)=0.
* KBOT and KTOP together determine an isolated block
* along the diagonal of the Hessenberg matrix.
*
* KBOT (input) INTEGER
* It is assumed without a check that either
* KBOT = N or H(KBOT+1,KBOT)=0. KBOT and KTOP together
* determine an isolated block along the diagonal of the
* Hessenberg matrix.
*
* NW (input) INTEGER
* Deflation window size. 1 .LE. NW .LE. (KBOT-KTOP+1).
*
* H (input/output) REAL array, dimension (LDH,N)
* On input the initial N-by-N section of H stores the
* Hessenberg matrix undergoing aggressive early deflation.
* On output H has been transformed by an orthogonal
* similarity transformation, perturbed, and the returned
* to Hessenberg form that (it is to be hoped) has some
* zero subdiagonal entries.
*
* LDH (input) integer
* Leading dimension of H just as declared in the calling
* subroutine. N .LE. LDH
*
* ILOZ (input) INTEGER
* IHIZ (input) INTEGER
* Specify the rows of Z to which transformations must be
* applied if WANTZ is .TRUE.. 1 .LE. ILOZ .LE. IHIZ .LE. N.
*
* Z (input/output) REAL array, dimension (LDZ,IHI)
* IF WANTZ is .TRUE., then on output, the orthogonal
* similarity transformation mentioned above has been
* accumulated into Z(ILOZ:IHIZ,ILO:IHI) from the right.
* If WANTZ is .FALSE., then Z is unreferenced.
*
* LDZ (input) integer
* The leading dimension of Z just as declared in the
* calling subroutine. 1 .LE. LDZ.
*
* NS (output) integer
* The number of unconverged (ie approximate) eigenvalues
* returned in SR and SI that may be used as shifts by the
* calling subroutine.
*
* ND (output) integer
* The number of converged eigenvalues uncovered by this
* subroutine.
*
* SR (output) REAL array, dimension KBOT
* SI (output) REAL array, dimension KBOT
* On output, the real and imaginary parts of approximate
* eigenvalues that may be used for shifts are stored in
* SR(KBOT-ND-NS+1) through SR(KBOT-ND) and
* SI(KBOT-ND-NS+1) through SI(KBOT-ND), respectively.
* The real and imaginary parts of converged eigenvalues
* are stored in SR(KBOT-ND+1) through SR(KBOT) and
* SI(KBOT-ND+1) through SI(KBOT), respectively.
*
* V (workspace) REAL array, dimension (LDV,NW)
* An NW-by-NW work array.
*
* LDV (input) integer scalar
* The leading dimension of V just as declared in the
* calling subroutine. NW .LE. LDV
*
* NH (input) integer scalar
* The number of columns of T. NH.GE.NW.
*
* T (workspace) REAL array, dimension (LDT,NW)
*
* LDT (input) integer
* The leading dimension of T just as declared in the
* calling subroutine. NW .LE. LDT
*
* NV (input) integer
* The number of rows of work array WV available for
* workspace. NV.GE.NW.
*
* WV (workspace) REAL array, dimension (LDWV,NW)
*
* LDWV (input) integer
* The leading dimension of W just as declared in the
* calling subroutine. NW .LE. LDV
*
* WORK (workspace) REAL array, dimension LWORK.
* On exit, WORK(1) is set to an estimate of the optimal value
* of LWORK for the given values of N, NW, KTOP and KBOT.
*
* LWORK (input) integer
* The dimension of the work array WORK. LWORK = 2*NW
* suffices, but greater efficiency may result from larger
* values of LWORK.
*
* If LWORK = -1, then a workspace query is assumed; SLAQR3
* only estimates the optimal workspace size for the given
* values of N, NW, KTOP and KBOT. The estimate is returned
* in WORK(1). No error message related to LWORK is issued
* by XERBLA. Neither H nor Z are accessed.
*
* ================================================================
* Based on contributions by
* Karen Braman and Ralph Byers, Department of Mathematics,
* University of Kansas, USA
*
* ==================================================================
* .. Parameters ..
*
*
* @param wantt
* @param wantz
* @param n
* @param ktop
* @param kbot
* @param nw
* @param h
* @param _h_offset
* @param ldh
* @param iloz
* @param ihiz
* @param z
* @param _z_offset
* @param ldz
* @param ns
* @param nd
* @param sr
* @param _sr_offset
* @param si
* @param _si_offset
* @param v
* @param _v_offset
* @param ldv
* @param nh
* @param t
* @param _t_offset
* @param ldt
* @param nv
* @param wv
* @param _wv_offset
* @param ldwv
* @param work
* @param _work_offset
* @param lwork
*
*/
abstract public void slaqr3(boolean wantt, boolean wantz, int n, int ktop, int kbot, int nw, float[] h, int _h_offset, int ldh, int iloz, int ihiz, float[] z, int _z_offset, int ldz, org.netlib.util.intW ns, org.netlib.util.intW nd, float[] sr, int _sr_offset, float[] si, int _si_offset, float[] v, int _v_offset, int ldv, int nh, float[] t, int _t_offset, int ldt, int nv, float[] wv, int _wv_offset, int ldwv, float[] work, int _work_offset, int lwork);
/**
*
* ..
*
* This subroutine implements one level of recursion for SLAQR0.
* It is a complete implementation of the small bulge multi-shift
* QR algorithm. It may be called by SLAQR0 and, for large enough
* deflation window size, it may be called by SLAQR3. This
* subroutine is identical to SLAQR0 except that it calls SLAQR2
* instead of SLAQR3.
*
* Purpose
* =======
*
* SLAQR4 computes the eigenvalues of a Hessenberg matrix H
* and, optionally, the matrices T and Z from the Schur decomposition
* H = Z T Z**T, where T is an upper quasi-triangular matrix (the
* Schur form), and Z is the orthogonal matrix of Schur vectors.
*
* Optionally Z may be postmultiplied into an input orthogonal
* matrix Q so that this routine can give the Schur factorization
* of a matrix A which has been reduced to the Hessenberg form H
* by the orthogonal matrix Q: A = Q*H*Q**T = (QZ)*T*(QZ)**T.
*
* Arguments
* =========
*
* WANTT (input) LOGICAL
* = .TRUE. : the full Schur form T is required;
* = .FALSE.: only eigenvalues are required.
*
* WANTZ (input) LOGICAL
* = .TRUE. : the matrix of Schur vectors Z is required;
* = .FALSE.: Schur vectors are not required.
*
* N (input) INTEGER
* The order of the matrix H. N .GE. 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* It is assumed that H is already upper triangular in rows
* and columns 1:ILO-1 and IHI+1:N and, if ILO.GT.1,
* H(ILO,ILO-1) is zero. ILO and IHI are normally set by a
* previous call to SGEBAL, and then passed to SGEHRD when the
* matrix output by SGEBAL is reduced to Hessenberg form.
* Otherwise, ILO and IHI should be set to 1 and N,
* respectively. If N.GT.0, then 1.LE.ILO.LE.IHI.LE.N.
* If N = 0, then ILO = 1 and IHI = 0.
*
* H (input/output) REAL array, dimension (LDH,N)
* On entry, the upper Hessenberg matrix H.
* On exit, if INFO = 0 and WANTT is .TRUE., then H contains
* the upper quasi-triangular matrix T from the Schur
* decomposition (the Schur form); 2-by-2 diagonal blocks
* (corresponding to complex conjugate pairs of eigenvalues)
* are returned in standard form, with H(i,i) = H(i+1,i+1)
* and H(i+1,i)*H(i,i+1).LT.0. If INFO = 0 and WANTT is
* .FALSE., then the contents of H are unspecified on exit.
* (The output value of H when INFO.GT.0 is given under the
* description of INFO below.)
*
* This subroutine may explicitly set H(i,j) = 0 for i.GT.j and
* j = 1, 2, ... ILO-1 or j = IHI+1, IHI+2, ... N.
*
* LDH (input) INTEGER
* The leading dimension of the array H. LDH .GE. max(1,N).
*
* WR (output) REAL array, dimension (IHI)
* WI (output) REAL array, dimension (IHI)
* The real and imaginary parts, respectively, of the computed
* eigenvalues of H(ILO:IHI,ILO:IHI) are stored WR(ILO:IHI)
* and WI(ILO:IHI). If two eigenvalues are computed as a
* complex conjugate pair, they are stored in consecutive
* elements of WR and WI, say the i-th and (i+1)th, with
* WI(i) .GT. 0 and WI(i+1) .LT. 0. If WANTT is .TRUE., then
* the eigenvalues are stored in the same order as on the
* diagonal of the Schur form returned in H, with
* WR(i) = H(i,i) and, if H(i:i+1,i:i+1) is a 2-by-2 diagonal
* block, WI(i) = sqrt(-H(i+1,i)*H(i,i+1)) and
* WI(i+1) = -WI(i).
*
* ILOZ (input) INTEGER
* IHIZ (input) INTEGER
* Specify the rows of Z to which transformations must be
* applied if WANTZ is .TRUE..
* 1 .LE. ILOZ .LE. ILO; IHI .LE. IHIZ .LE. N.
*
* Z (input/output) REAL array, dimension (LDZ,IHI)
* If WANTZ is .FALSE., then Z is not referenced.
* If WANTZ is .TRUE., then Z(ILO:IHI,ILOZ:IHIZ) is
* replaced by Z(ILO:IHI,ILOZ:IHIZ)*U where U is the
* orthogonal Schur factor of H(ILO:IHI,ILO:IHI).
* (The output value of Z when INFO.GT.0 is given under
* the description of INFO below.)
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. if WANTZ is .TRUE.
* then LDZ.GE.MAX(1,IHIZ). Otherwize, LDZ.GE.1.
*
* WORK (workspace/output) REAL array, dimension LWORK
* On exit, if LWORK = -1, WORK(1) returns an estimate of
* the optimal value for LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK .GE. max(1,N)
* is sufficient, but LWORK typically as large as 6*N may
* be required for optimal performance. A workspace query
* to determine the optimal workspace size is recommended.
*
* If LWORK = -1, then SLAQR4 does a workspace query.
* In this case, SLAQR4 checks the input parameters and
* estimates the optimal workspace size for the given
* values of N, ILO and IHI. The estimate is returned
* in WORK(1). No error message related to LWORK is
* issued by XERBLA. Neither H nor Z are accessed.
*
*
* INFO (output) INTEGER
* = 0: successful exit
* .GT. 0: if INFO = i, SLAQR4 failed to compute all of
* the eigenvalues. Elements 1:ilo-1 and i+1:n of WR
* and WI contain those eigenvalues which have been
* successfully computed. (Failures are rare.)
*
* If INFO .GT. 0 and WANT is .FALSE., then on exit,
* the remaining unconverged eigenvalues are the eigen-
* values of the upper Hessenberg matrix rows and
* columns ILO through INFO of the final, output
* value of H.
*
* If INFO .GT. 0 and WANTT is .TRUE., then on exit
*
* (*) (initial value of H)*U = U*(final value of H)
*
* where U is an orthogonal matrix. The final
* value of H is upper Hessenberg and quasi-triangular
* in rows and columns INFO+1 through IHI.
*
* If INFO .GT. 0 and WANTZ is .TRUE., then on exit
*
* (final value of Z(ILO:IHI,ILOZ:IHIZ)
* = (initial value of Z(ILO:IHI,ILOZ:IHIZ)*U
*
* where U is the orthogonal matrix in (*) (regard-
* less of the value of WANTT.)
*
* If INFO .GT. 0 and WANTZ is .FALSE., then Z is not
* accessed.
*
* ================================================================
* Based on contributions by
* Karen Braman and Ralph Byers, Department of Mathematics,
* University of Kansas, USA
*
* ================================================================
* References:
* K. Braman, R. Byers and R. Mathias, The Multi-Shift QR
* Algorithm Part I: Maintaining Well Focused Shifts, and Level 3
* Performance, SIAM Journal of Matrix Analysis, volume 23, pages
* 929--947, 2002.
*
* K. Braman, R. Byers and R. Mathias, The Multi-Shift QR
* Algorithm Part II: Aggressive Early Deflation, SIAM Journal
* of Matrix Analysis, volume 23, pages 948--973, 2002.
*
* ================================================================
* .. Parameters ..
*
* ==== Matrices of order NTINY or smaller must be processed by
* . SLAHQR because of insufficient subdiagonal scratch space.
* . (This is a hard limit.) ====
*
* ==== Exceptional deflation windows: try to cure rare
* . slow convergence by increasing the size of the
* . deflation window after KEXNW iterations. =====
*
* ==== Exceptional shifts: try to cure rare slow convergence
* . with ad-hoc exceptional shifts every KEXSH iterations.
* . The constants WILK1 and WILK2 are used to form the
* . exceptional shifts. ====
*
*
*
* @param wantt
* @param wantz
* @param n
* @param ilo
* @param ihi
* @param h
* @param ldh
* @param wr
* @param wi
* @param iloz
* @param ihiz
* @param z
* @param ldz
* @param work
* @param lwork
* @param info
*
*/
abstract public void slaqr4(boolean wantt, boolean wantz, int n, int ilo, int ihi, float[] h, int ldh, float[] wr, float[] wi, int iloz, int ihiz, float[] z, int ldz, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* This subroutine implements one level of recursion for SLAQR0.
* It is a complete implementation of the small bulge multi-shift
* QR algorithm. It may be called by SLAQR0 and, for large enough
* deflation window size, it may be called by SLAQR3. This
* subroutine is identical to SLAQR0 except that it calls SLAQR2
* instead of SLAQR3.
*
* Purpose
* =======
*
* SLAQR4 computes the eigenvalues of a Hessenberg matrix H
* and, optionally, the matrices T and Z from the Schur decomposition
* H = Z T Z**T, where T is an upper quasi-triangular matrix (the
* Schur form), and Z is the orthogonal matrix of Schur vectors.
*
* Optionally Z may be postmultiplied into an input orthogonal
* matrix Q so that this routine can give the Schur factorization
* of a matrix A which has been reduced to the Hessenberg form H
* by the orthogonal matrix Q: A = Q*H*Q**T = (QZ)*T*(QZ)**T.
*
* Arguments
* =========
*
* WANTT (input) LOGICAL
* = .TRUE. : the full Schur form T is required;
* = .FALSE.: only eigenvalues are required.
*
* WANTZ (input) LOGICAL
* = .TRUE. : the matrix of Schur vectors Z is required;
* = .FALSE.: Schur vectors are not required.
*
* N (input) INTEGER
* The order of the matrix H. N .GE. 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* It is assumed that H is already upper triangular in rows
* and columns 1:ILO-1 and IHI+1:N and, if ILO.GT.1,
* H(ILO,ILO-1) is zero. ILO and IHI are normally set by a
* previous call to SGEBAL, and then passed to SGEHRD when the
* matrix output by SGEBAL is reduced to Hessenberg form.
* Otherwise, ILO and IHI should be set to 1 and N,
* respectively. If N.GT.0, then 1.LE.ILO.LE.IHI.LE.N.
* If N = 0, then ILO = 1 and IHI = 0.
*
* H (input/output) REAL array, dimension (LDH,N)
* On entry, the upper Hessenberg matrix H.
* On exit, if INFO = 0 and WANTT is .TRUE., then H contains
* the upper quasi-triangular matrix T from the Schur
* decomposition (the Schur form); 2-by-2 diagonal blocks
* (corresponding to complex conjugate pairs of eigenvalues)
* are returned in standard form, with H(i,i) = H(i+1,i+1)
* and H(i+1,i)*H(i,i+1).LT.0. If INFO = 0 and WANTT is
* .FALSE., then the contents of H are unspecified on exit.
* (The output value of H when INFO.GT.0 is given under the
* description of INFO below.)
*
* This subroutine may explicitly set H(i,j) = 0 for i.GT.j and
* j = 1, 2, ... ILO-1 or j = IHI+1, IHI+2, ... N.
*
* LDH (input) INTEGER
* The leading dimension of the array H. LDH .GE. max(1,N).
*
* WR (output) REAL array, dimension (IHI)
* WI (output) REAL array, dimension (IHI)
* The real and imaginary parts, respectively, of the computed
* eigenvalues of H(ILO:IHI,ILO:IHI) are stored WR(ILO:IHI)
* and WI(ILO:IHI). If two eigenvalues are computed as a
* complex conjugate pair, they are stored in consecutive
* elements of WR and WI, say the i-th and (i+1)th, with
* WI(i) .GT. 0 and WI(i+1) .LT. 0. If WANTT is .TRUE., then
* the eigenvalues are stored in the same order as on the
* diagonal of the Schur form returned in H, with
* WR(i) = H(i,i) and, if H(i:i+1,i:i+1) is a 2-by-2 diagonal
* block, WI(i) = sqrt(-H(i+1,i)*H(i,i+1)) and
* WI(i+1) = -WI(i).
*
* ILOZ (input) INTEGER
* IHIZ (input) INTEGER
* Specify the rows of Z to which transformations must be
* applied if WANTZ is .TRUE..
* 1 .LE. ILOZ .LE. ILO; IHI .LE. IHIZ .LE. N.
*
* Z (input/output) REAL array, dimension (LDZ,IHI)
* If WANTZ is .FALSE., then Z is not referenced.
* If WANTZ is .TRUE., then Z(ILO:IHI,ILOZ:IHIZ) is
* replaced by Z(ILO:IHI,ILOZ:IHIZ)*U where U is the
* orthogonal Schur factor of H(ILO:IHI,ILO:IHI).
* (The output value of Z when INFO.GT.0 is given under
* the description of INFO below.)
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. if WANTZ is .TRUE.
* then LDZ.GE.MAX(1,IHIZ). Otherwize, LDZ.GE.1.
*
* WORK (workspace/output) REAL array, dimension LWORK
* On exit, if LWORK = -1, WORK(1) returns an estimate of
* the optimal value for LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK .GE. max(1,N)
* is sufficient, but LWORK typically as large as 6*N may
* be required for optimal performance. A workspace query
* to determine the optimal workspace size is recommended.
*
* If LWORK = -1, then SLAQR4 does a workspace query.
* In this case, SLAQR4 checks the input parameters and
* estimates the optimal workspace size for the given
* values of N, ILO and IHI. The estimate is returned
* in WORK(1). No error message related to LWORK is
* issued by XERBLA. Neither H nor Z are accessed.
*
*
* INFO (output) INTEGER
* = 0: successful exit
* .GT. 0: if INFO = i, SLAQR4 failed to compute all of
* the eigenvalues. Elements 1:ilo-1 and i+1:n of WR
* and WI contain those eigenvalues which have been
* successfully computed. (Failures are rare.)
*
* If INFO .GT. 0 and WANT is .FALSE., then on exit,
* the remaining unconverged eigenvalues are the eigen-
* values of the upper Hessenberg matrix rows and
* columns ILO through INFO of the final, output
* value of H.
*
* If INFO .GT. 0 and WANTT is .TRUE., then on exit
*
* (*) (initial value of H)*U = U*(final value of H)
*
* where U is an orthogonal matrix. The final
* value of H is upper Hessenberg and quasi-triangular
* in rows and columns INFO+1 through IHI.
*
* If INFO .GT. 0 and WANTZ is .TRUE., then on exit
*
* (final value of Z(ILO:IHI,ILOZ:IHIZ)
* = (initial value of Z(ILO:IHI,ILOZ:IHIZ)*U
*
* where U is the orthogonal matrix in (*) (regard-
* less of the value of WANTT.)
*
* If INFO .GT. 0 and WANTZ is .FALSE., then Z is not
* accessed.
*
* ================================================================
* Based on contributions by
* Karen Braman and Ralph Byers, Department of Mathematics,
* University of Kansas, USA
*
* ================================================================
* References:
* K. Braman, R. Byers and R. Mathias, The Multi-Shift QR
* Algorithm Part I: Maintaining Well Focused Shifts, and Level 3
* Performance, SIAM Journal of Matrix Analysis, volume 23, pages
* 929--947, 2002.
*
* K. Braman, R. Byers and R. Mathias, The Multi-Shift QR
* Algorithm Part II: Aggressive Early Deflation, SIAM Journal
* of Matrix Analysis, volume 23, pages 948--973, 2002.
*
* ================================================================
* .. Parameters ..
*
* ==== Matrices of order NTINY or smaller must be processed by
* . SLAHQR because of insufficient subdiagonal scratch space.
* . (This is a hard limit.) ====
*
* ==== Exceptional deflation windows: try to cure rare
* . slow convergence by increasing the size of the
* . deflation window after KEXNW iterations. =====
*
* ==== Exceptional shifts: try to cure rare slow convergence
* . with ad-hoc exceptional shifts every KEXSH iterations.
* . The constants WILK1 and WILK2 are used to form the
* . exceptional shifts. ====
*
*
*
* @param wantt
* @param wantz
* @param n
* @param ilo
* @param ihi
* @param h
* @param _h_offset
* @param ldh
* @param wr
* @param _wr_offset
* @param wi
* @param _wi_offset
* @param iloz
* @param ihiz
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void slaqr4(boolean wantt, boolean wantz, int n, int ilo, int ihi, float[] h, int _h_offset, int ldh, float[] wr, int _wr_offset, float[] wi, int _wi_offset, int iloz, int ihiz, float[] z, int _z_offset, int ldz, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* This auxiliary subroutine called by SLAQR0 performs a
* single small-bulge multi-shift QR sweep.
*
* WANTT (input) logical scalar
* WANTT = .true. if the quasi-triangular Schur factor
* is being computed. WANTT is set to .false. otherwise.
*
* WANTZ (input) logical scalar
* WANTZ = .true. if the orthogonal Schur factor is being
* computed. WANTZ is set to .false. otherwise.
*
* KACC22 (input) integer with value 0, 1, or 2.
* Specifies the computation mode of far-from-diagonal
* orthogonal updates.
* = 0: SLAQR5 does not accumulate reflections and does not
* use matrix-matrix multiply to update far-from-diagonal
* matrix entries.
* = 1: SLAQR5 accumulates reflections and uses matrix-matrix
* multiply to update the far-from-diagonal matrix entries.
* = 2: SLAQR5 accumulates reflections, uses matrix-matrix
* multiply to update the far-from-diagonal matrix entries,
* and takes advantage of 2-by-2 block structure during
* matrix multiplies.
*
* N (input) integer scalar
* N is the order of the Hessenberg matrix H upon which this
* subroutine operates.
*
* KTOP (input) integer scalar
* KBOT (input) integer scalar
* These are the first and last rows and columns of an
* isolated diagonal block upon which the QR sweep is to be
* applied. It is assumed without a check that
* either KTOP = 1 or H(KTOP,KTOP-1) = 0
* and
* either KBOT = N or H(KBOT+1,KBOT) = 0.
*
* NSHFTS (input) integer scalar
* NSHFTS gives the number of simultaneous shifts. NSHFTS
* must be positive and even.
*
* SR (input) REAL array of size (NSHFTS)
* SI (input) REAL array of size (NSHFTS)
* SR contains the real parts and SI contains the imaginary
* parts of the NSHFTS shifts of origin that define the
* multi-shift QR sweep.
*
* H (input/output) REAL array of size (LDH,N)
* On input H contains a Hessenberg matrix. On output a
* multi-shift QR sweep with shifts SR(J)+i*SI(J) is applied
* to the isolated diagonal block in rows and columns KTOP
* through KBOT.
*
* LDH (input) integer scalar
* LDH is the leading dimension of H just as declared in the
* calling procedure. LDH.GE.MAX(1,N).
*
* ILOZ (input) INTEGER
* IHIZ (input) INTEGER
* Specify the rows of Z to which transformations must be
* applied if WANTZ is .TRUE.. 1 .LE. ILOZ .LE. IHIZ .LE. N
*
* Z (input/output) REAL array of size (LDZ,IHI)
* If WANTZ = .TRUE., then the QR Sweep orthogonal
* similarity transformation is accumulated into
* Z(ILOZ:IHIZ,ILO:IHI) from the right.
* If WANTZ = .FALSE., then Z is unreferenced.
*
* LDZ (input) integer scalar
* LDA is the leading dimension of Z just as declared in
* the calling procedure. LDZ.GE.N.
*
* V (workspace) REAL array of size (LDV,NSHFTS/2)
*
* LDV (input) integer scalar
* LDV is the leading dimension of V as declared in the
* calling procedure. LDV.GE.3.
*
* U (workspace) REAL array of size
* (LDU,3*NSHFTS-3)
*
* LDU (input) integer scalar
* LDU is the leading dimension of U just as declared in the
* in the calling subroutine. LDU.GE.3*NSHFTS-3.
*
* NH (input) integer scalar
* NH is the number of columns in array WH available for
* workspace. NH.GE.1.
*
* WH (workspace) REAL array of size (LDWH,NH)
*
* LDWH (input) integer scalar
* Leading dimension of WH just as declared in the
* calling procedure. LDWH.GE.3*NSHFTS-3.
*
* NV (input) integer scalar
* NV is the number of rows in WV agailable for workspace.
* NV.GE.1.
*
* WV (workspace) REAL array of size
* (LDWV,3*NSHFTS-3)
*
* LDWV (input) integer scalar
* LDWV is the leading dimension of WV as declared in the
* in the calling subroutine. LDWV.GE.NV.
*
*
* ================================================================
* Based on contributions by
* Karen Braman and Ralph Byers, Department of Mathematics,
* University of Kansas, USA
*
* ============================================================
* Reference:
*
* K. Braman, R. Byers and R. Mathias, The Multi-Shift QR
* Algorithm Part I: Maintaining Well Focused Shifts, and
* Level 3 Performance, SIAM Journal of Matrix Analysis,
* volume 23, pages 929--947, 2002.
*
* ============================================================
* .. Parameters ..
*
*
* @param wantt
* @param wantz
* @param kacc22
* @param n
* @param ktop
* @param kbot
* @param nshfts
* @param sr
* @param si
* @param h
* @param ldh
* @param iloz
* @param ihiz
* @param z
* @param ldz
* @param v
* @param ldv
* @param u
* @param ldu
* @param nv
* @param wv
* @param ldwv
* @param nh
* @param wh
* @param ldwh
*
*/
abstract public void slaqr5(boolean wantt, boolean wantz, int kacc22, int n, int ktop, int kbot, int nshfts, float[] sr, float[] si, float[] h, int ldh, int iloz, int ihiz, float[] z, int ldz, float[] v, int ldv, float[] u, int ldu, int nv, float[] wv, int ldwv, int nh, float[] wh, int ldwh);
/**
*
* ..
*
* This auxiliary subroutine called by SLAQR0 performs a
* single small-bulge multi-shift QR sweep.
*
* WANTT (input) logical scalar
* WANTT = .true. if the quasi-triangular Schur factor
* is being computed. WANTT is set to .false. otherwise.
*
* WANTZ (input) logical scalar
* WANTZ = .true. if the orthogonal Schur factor is being
* computed. WANTZ is set to .false. otherwise.
*
* KACC22 (input) integer with value 0, 1, or 2.
* Specifies the computation mode of far-from-diagonal
* orthogonal updates.
* = 0: SLAQR5 does not accumulate reflections and does not
* use matrix-matrix multiply to update far-from-diagonal
* matrix entries.
* = 1: SLAQR5 accumulates reflections and uses matrix-matrix
* multiply to update the far-from-diagonal matrix entries.
* = 2: SLAQR5 accumulates reflections, uses matrix-matrix
* multiply to update the far-from-diagonal matrix entries,
* and takes advantage of 2-by-2 block structure during
* matrix multiplies.
*
* N (input) integer scalar
* N is the order of the Hessenberg matrix H upon which this
* subroutine operates.
*
* KTOP (input) integer scalar
* KBOT (input) integer scalar
* These are the first and last rows and columns of an
* isolated diagonal block upon which the QR sweep is to be
* applied. It is assumed without a check that
* either KTOP = 1 or H(KTOP,KTOP-1) = 0
* and
* either KBOT = N or H(KBOT+1,KBOT) = 0.
*
* NSHFTS (input) integer scalar
* NSHFTS gives the number of simultaneous shifts. NSHFTS
* must be positive and even.
*
* SR (input) REAL array of size (NSHFTS)
* SI (input) REAL array of size (NSHFTS)
* SR contains the real parts and SI contains the imaginary
* parts of the NSHFTS shifts of origin that define the
* multi-shift QR sweep.
*
* H (input/output) REAL array of size (LDH,N)
* On input H contains a Hessenberg matrix. On output a
* multi-shift QR sweep with shifts SR(J)+i*SI(J) is applied
* to the isolated diagonal block in rows and columns KTOP
* through KBOT.
*
* LDH (input) integer scalar
* LDH is the leading dimension of H just as declared in the
* calling procedure. LDH.GE.MAX(1,N).
*
* ILOZ (input) INTEGER
* IHIZ (input) INTEGER
* Specify the rows of Z to which transformations must be
* applied if WANTZ is .TRUE.. 1 .LE. ILOZ .LE. IHIZ .LE. N
*
* Z (input/output) REAL array of size (LDZ,IHI)
* If WANTZ = .TRUE., then the QR Sweep orthogonal
* similarity transformation is accumulated into
* Z(ILOZ:IHIZ,ILO:IHI) from the right.
* If WANTZ = .FALSE., then Z is unreferenced.
*
* LDZ (input) integer scalar
* LDA is the leading dimension of Z just as declared in
* the calling procedure. LDZ.GE.N.
*
* V (workspace) REAL array of size (LDV,NSHFTS/2)
*
* LDV (input) integer scalar
* LDV is the leading dimension of V as declared in the
* calling procedure. LDV.GE.3.
*
* U (workspace) REAL array of size
* (LDU,3*NSHFTS-3)
*
* LDU (input) integer scalar
* LDU is the leading dimension of U just as declared in the
* in the calling subroutine. LDU.GE.3*NSHFTS-3.
*
* NH (input) integer scalar
* NH is the number of columns in array WH available for
* workspace. NH.GE.1.
*
* WH (workspace) REAL array of size (LDWH,NH)
*
* LDWH (input) integer scalar
* Leading dimension of WH just as declared in the
* calling procedure. LDWH.GE.3*NSHFTS-3.
*
* NV (input) integer scalar
* NV is the number of rows in WV agailable for workspace.
* NV.GE.1.
*
* WV (workspace) REAL array of size
* (LDWV,3*NSHFTS-3)
*
* LDWV (input) integer scalar
* LDWV is the leading dimension of WV as declared in the
* in the calling subroutine. LDWV.GE.NV.
*
*
* ================================================================
* Based on contributions by
* Karen Braman and Ralph Byers, Department of Mathematics,
* University of Kansas, USA
*
* ============================================================
* Reference:
*
* K. Braman, R. Byers and R. Mathias, The Multi-Shift QR
* Algorithm Part I: Maintaining Well Focused Shifts, and
* Level 3 Performance, SIAM Journal of Matrix Analysis,
* volume 23, pages 929--947, 2002.
*
* ============================================================
* .. Parameters ..
*
*
* @param wantt
* @param wantz
* @param kacc22
* @param n
* @param ktop
* @param kbot
* @param nshfts
* @param sr
* @param _sr_offset
* @param si
* @param _si_offset
* @param h
* @param _h_offset
* @param ldh
* @param iloz
* @param ihiz
* @param z
* @param _z_offset
* @param ldz
* @param v
* @param _v_offset
* @param ldv
* @param u
* @param _u_offset
* @param ldu
* @param nv
* @param wv
* @param _wv_offset
* @param ldwv
* @param nh
* @param wh
* @param _wh_offset
* @param ldwh
*
*/
abstract public void slaqr5(boolean wantt, boolean wantz, int kacc22, int n, int ktop, int kbot, int nshfts, float[] sr, int _sr_offset, float[] si, int _si_offset, float[] h, int _h_offset, int ldh, int iloz, int ihiz, float[] z, int _z_offset, int ldz, float[] v, int _v_offset, int ldv, float[] u, int _u_offset, int ldu, int nv, float[] wv, int _wv_offset, int ldwv, int nh, float[] wh, int _wh_offset, int ldwh);
/**
*
* ..
*
* Purpose
* =======
*
* SLAQSB equilibrates a symmetric band matrix A using the scaling
* factors in the vector S.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of super-diagonals of the matrix A if UPLO = 'U',
* or the number of sub-diagonals if UPLO = 'L'. KD >= 0.
*
* AB (input/output) REAL array, dimension (LDAB,N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* On exit, if INFO = 0, the triangular factor U or L from the
* Cholesky factorization A = U'*U or A = L*L' of the band
* matrix A, in the same storage format as A.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* S (input) REAL array, dimension (N)
* The scale factors for A.
*
* SCOND (input) REAL
* Ratio of the smallest S(i) to the largest S(i).
*
* AMAX (input) REAL
* Absolute value of largest matrix entry.
*
* EQUED (output) CHARACTER*1
* Specifies whether or not equilibration was done.
* = 'N': No equilibration.
* = 'Y': Equilibration was done, i.e., A has been replaced by
* diag(S) * A * diag(S).
*
* Internal Parameters
* ===================
*
* THRESH is a threshold value used to decide if scaling should be done
* based on the ratio of the scaling factors. If SCOND < THRESH,
* scaling is done.
*
* LARGE and SMALL are threshold values used to decide if scaling should
* be done based on the absolute size of the largest matrix element.
* If AMAX > LARGE or AMAX < SMALL, scaling is done.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param kd
* @param ab
* @param ldab
* @param s
* @param scond
* @param amax
* @param equed
*
*/
abstract public void slaqsb(java.lang.String uplo, int n, int kd, float[] ab, int ldab, float[] s, float scond, float amax, org.netlib.util.StringW equed);
/**
*
* ..
*
* Purpose
* =======
*
* SLAQSB equilibrates a symmetric band matrix A using the scaling
* factors in the vector S.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of super-diagonals of the matrix A if UPLO = 'U',
* or the number of sub-diagonals if UPLO = 'L'. KD >= 0.
*
* AB (input/output) REAL array, dimension (LDAB,N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* On exit, if INFO = 0, the triangular factor U or L from the
* Cholesky factorization A = U'*U or A = L*L' of the band
* matrix A, in the same storage format as A.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* S (input) REAL array, dimension (N)
* The scale factors for A.
*
* SCOND (input) REAL
* Ratio of the smallest S(i) to the largest S(i).
*
* AMAX (input) REAL
* Absolute value of largest matrix entry.
*
* EQUED (output) CHARACTER*1
* Specifies whether or not equilibration was done.
* = 'N': No equilibration.
* = 'Y': Equilibration was done, i.e., A has been replaced by
* diag(S) * A * diag(S).
*
* Internal Parameters
* ===================
*
* THRESH is a threshold value used to decide if scaling should be done
* based on the ratio of the scaling factors. If SCOND < THRESH,
* scaling is done.
*
* LARGE and SMALL are threshold values used to decide if scaling should
* be done based on the absolute size of the largest matrix element.
* If AMAX > LARGE or AMAX < SMALL, scaling is done.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param kd
* @param ab
* @param _ab_offset
* @param ldab
* @param s
* @param _s_offset
* @param scond
* @param amax
* @param equed
*
*/
abstract public void slaqsb(java.lang.String uplo, int n, int kd, float[] ab, int _ab_offset, int ldab, float[] s, int _s_offset, float scond, float amax, org.netlib.util.StringW equed);
/**
*
* ..
*
* Purpose
* =======
*
* SLAQSP equilibrates a symmetric matrix A using the scaling factors
* in the vector S.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, the equilibrated matrix: diag(S) * A * diag(S), in
* the same storage format as A.
*
* S (input) REAL array, dimension (N)
* The scale factors for A.
*
* SCOND (input) REAL
* Ratio of the smallest S(i) to the largest S(i).
*
* AMAX (input) REAL
* Absolute value of largest matrix entry.
*
* EQUED (output) CHARACTER*1
* Specifies whether or not equilibration was done.
* = 'N': No equilibration.
* = 'Y': Equilibration was done, i.e., A has been replaced by
* diag(S) * A * diag(S).
*
* Internal Parameters
* ===================
*
* THRESH is a threshold value used to decide if scaling should be done
* based on the ratio of the scaling factors. If SCOND < THRESH,
* scaling is done.
*
* LARGE and SMALL are threshold values used to decide if scaling should
* be done based on the absolute size of the largest matrix element.
* If AMAX > LARGE or AMAX < SMALL, scaling is done.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param s
* @param scond
* @param amax
* @param equed
*
*/
abstract public void slaqsp(java.lang.String uplo, int n, float[] ap, float[] s, float scond, float amax, org.netlib.util.StringW equed);
/**
*
* ..
*
* Purpose
* =======
*
* SLAQSP equilibrates a symmetric matrix A using the scaling factors
* in the vector S.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, the equilibrated matrix: diag(S) * A * diag(S), in
* the same storage format as A.
*
* S (input) REAL array, dimension (N)
* The scale factors for A.
*
* SCOND (input) REAL
* Ratio of the smallest S(i) to the largest S(i).
*
* AMAX (input) REAL
* Absolute value of largest matrix entry.
*
* EQUED (output) CHARACTER*1
* Specifies whether or not equilibration was done.
* = 'N': No equilibration.
* = 'Y': Equilibration was done, i.e., A has been replaced by
* diag(S) * A * diag(S).
*
* Internal Parameters
* ===================
*
* THRESH is a threshold value used to decide if scaling should be done
* based on the ratio of the scaling factors. If SCOND < THRESH,
* scaling is done.
*
* LARGE and SMALL are threshold values used to decide if scaling should
* be done based on the absolute size of the largest matrix element.
* If AMAX > LARGE or AMAX < SMALL, scaling is done.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param s
* @param _s_offset
* @param scond
* @param amax
* @param equed
*
*/
abstract public void slaqsp(java.lang.String uplo, int n, float[] ap, int _ap_offset, float[] s, int _s_offset, float scond, float amax, org.netlib.util.StringW equed);
/**
*
* ..
*
* Purpose
* =======
*
* SLAQSY equilibrates a symmetric matrix A using the scaling factors
* in the vector S.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* n by n upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading n by n lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, if EQUED = 'Y', the equilibrated matrix:
* diag(S) * A * diag(S).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(N,1).
*
* S (input) REAL array, dimension (N)
* The scale factors for A.
*
* SCOND (input) REAL
* Ratio of the smallest S(i) to the largest S(i).
*
* AMAX (input) REAL
* Absolute value of largest matrix entry.
*
* EQUED (output) CHARACTER*1
* Specifies whether or not equilibration was done.
* = 'N': No equilibration.
* = 'Y': Equilibration was done, i.e., A has been replaced by
* diag(S) * A * diag(S).
*
* Internal Parameters
* ===================
*
* THRESH is a threshold value used to decide if scaling should be done
* based on the ratio of the scaling factors. If SCOND < THRESH,
* scaling is done.
*
* LARGE and SMALL are threshold values used to decide if scaling should
* be done based on the absolute size of the largest matrix element.
* If AMAX > LARGE or AMAX < SMALL, scaling is done.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param lda
* @param s
* @param scond
* @param amax
* @param equed
*
*/
abstract public void slaqsy(java.lang.String uplo, int n, float[] a, int lda, float[] s, float scond, float amax, org.netlib.util.StringW equed);
/**
*
* ..
*
* Purpose
* =======
*
* SLAQSY equilibrates a symmetric matrix A using the scaling factors
* in the vector S.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* n by n upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading n by n lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, if EQUED = 'Y', the equilibrated matrix:
* diag(S) * A * diag(S).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(N,1).
*
* S (input) REAL array, dimension (N)
* The scale factors for A.
*
* SCOND (input) REAL
* Ratio of the smallest S(i) to the largest S(i).
*
* AMAX (input) REAL
* Absolute value of largest matrix entry.
*
* EQUED (output) CHARACTER*1
* Specifies whether or not equilibration was done.
* = 'N': No equilibration.
* = 'Y': Equilibration was done, i.e., A has been replaced by
* diag(S) * A * diag(S).
*
* Internal Parameters
* ===================
*
* THRESH is a threshold value used to decide if scaling should be done
* based on the ratio of the scaling factors. If SCOND < THRESH,
* scaling is done.
*
* LARGE and SMALL are threshold values used to decide if scaling should
* be done based on the absolute size of the largest matrix element.
* If AMAX > LARGE or AMAX < SMALL, scaling is done.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param s
* @param _s_offset
* @param scond
* @param amax
* @param equed
*
*/
abstract public void slaqsy(java.lang.String uplo, int n, float[] a, int _a_offset, int lda, float[] s, int _s_offset, float scond, float amax, org.netlib.util.StringW equed);
/**
*
* ..
*
* Purpose
* =======
*
* SLAQTR solves the real quasi-triangular system
*
* op(T)*p = scale*c, if LREAL = .TRUE.
*
* or the complex quasi-triangular systems
*
* op(T + iB)*(p+iq) = scale*(c+id), if LREAL = .FALSE.
*
* in real arithmetic, where T is upper quasi-triangular.
* If LREAL = .FALSE., then the first diagonal block of T must be
* 1 by 1, B is the specially structured matrix
*
* B = [ b(1) b(2) ... b(n) ]
* [ w ]
* [ w ]
* [ . ]
* [ w ]
*
* op(A) = A or A', A' denotes the conjugate transpose of
* matrix A.
*
* On input, X = [ c ]. On output, X = [ p ].
* [ d ] [ q ]
*
* This subroutine is designed for the condition number estimation
* in routine STRSNA.
*
* Arguments
* =========
*
* LTRAN (input) LOGICAL
* On entry, LTRAN specifies the option of conjugate transpose:
* = .FALSE., op(T+i*B) = T+i*B,
* = .TRUE., op(T+i*B) = (T+i*B)'.
*
* LREAL (input) LOGICAL
* On entry, LREAL specifies the input matrix structure:
* = .FALSE., the input is complex
* = .TRUE., the input is real
*
* N (input) INTEGER
* On entry, N specifies the order of T+i*B. N >= 0.
*
* T (input) REAL array, dimension (LDT,N)
* On entry, T contains a matrix in Schur canonical form.
* If LREAL = .FALSE., then the first diagonal block of T must
* be 1 by 1.
*
* LDT (input) INTEGER
* The leading dimension of the matrix T. LDT >= max(1,N).
*
* B (input) REAL array, dimension (N)
* On entry, B contains the elements to form the matrix
* B as described above.
* If LREAL = .TRUE., B is not referenced.
*
* W (input) REAL
* On entry, W is the diagonal element of the matrix B.
* If LREAL = .TRUE., W is not referenced.
*
* SCALE (output) REAL
* On exit, SCALE is the scale factor.
*
* X (input/output) REAL array, dimension (2*N)
* On entry, X contains the right hand side of the system.
* On exit, X is overwritten by the solution.
*
* WORK (workspace) REAL array, dimension (N)
*
* INFO (output) INTEGER
* On exit, INFO is set to
* 0: successful exit.
* 1: the some diagonal 1 by 1 block has been perturbed by
* a small number SMIN to keep nonsingularity.
* 2: the some diagonal 2 by 2 block has been perturbed by
* a small number in SLALN2 to keep nonsingularity.
* NOTE: In the interests of speed, this routine does not
* check the inputs for errors.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param ltran
* @param lreal
* @param n
* @param t
* @param ldt
* @param b
* @param w
* @param scale
* @param x
* @param work
* @param info
*
*/
abstract public void slaqtr(boolean ltran, boolean lreal, int n, float[] t, int ldt, float[] b, float w, org.netlib.util.floatW scale, float[] x, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAQTR solves the real quasi-triangular system
*
* op(T)*p = scale*c, if LREAL = .TRUE.
*
* or the complex quasi-triangular systems
*
* op(T + iB)*(p+iq) = scale*(c+id), if LREAL = .FALSE.
*
* in real arithmetic, where T is upper quasi-triangular.
* If LREAL = .FALSE., then the first diagonal block of T must be
* 1 by 1, B is the specially structured matrix
*
* B = [ b(1) b(2) ... b(n) ]
* [ w ]
* [ w ]
* [ . ]
* [ w ]
*
* op(A) = A or A', A' denotes the conjugate transpose of
* matrix A.
*
* On input, X = [ c ]. On output, X = [ p ].
* [ d ] [ q ]
*
* This subroutine is designed for the condition number estimation
* in routine STRSNA.
*
* Arguments
* =========
*
* LTRAN (input) LOGICAL
* On entry, LTRAN specifies the option of conjugate transpose:
* = .FALSE., op(T+i*B) = T+i*B,
* = .TRUE., op(T+i*B) = (T+i*B)'.
*
* LREAL (input) LOGICAL
* On entry, LREAL specifies the input matrix structure:
* = .FALSE., the input is complex
* = .TRUE., the input is real
*
* N (input) INTEGER
* On entry, N specifies the order of T+i*B. N >= 0.
*
* T (input) REAL array, dimension (LDT,N)
* On entry, T contains a matrix in Schur canonical form.
* If LREAL = .FALSE., then the first diagonal block of T must
* be 1 by 1.
*
* LDT (input) INTEGER
* The leading dimension of the matrix T. LDT >= max(1,N).
*
* B (input) REAL array, dimension (N)
* On entry, B contains the elements to form the matrix
* B as described above.
* If LREAL = .TRUE., B is not referenced.
*
* W (input) REAL
* On entry, W is the diagonal element of the matrix B.
* If LREAL = .TRUE., W is not referenced.
*
* SCALE (output) REAL
* On exit, SCALE is the scale factor.
*
* X (input/output) REAL array, dimension (2*N)
* On entry, X contains the right hand side of the system.
* On exit, X is overwritten by the solution.
*
* WORK (workspace) REAL array, dimension (N)
*
* INFO (output) INTEGER
* On exit, INFO is set to
* 0: successful exit.
* 1: the some diagonal 1 by 1 block has been perturbed by
* a small number SMIN to keep nonsingularity.
* 2: the some diagonal 2 by 2 block has been perturbed by
* a small number in SLALN2 to keep nonsingularity.
* NOTE: In the interests of speed, this routine does not
* check the inputs for errors.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param ltran
* @param lreal
* @param n
* @param t
* @param _t_offset
* @param ldt
* @param b
* @param _b_offset
* @param w
* @param scale
* @param x
* @param _x_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void slaqtr(boolean ltran, boolean lreal, int n, float[] t, int _t_offset, int ldt, float[] b, int _b_offset, float w, org.netlib.util.floatW scale, float[] x, int _x_offset, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAR1V computes the (scaled) r-th column of the inverse of
* the sumbmatrix in rows B1 through BN of the tridiagonal matrix
* L D L^T - sigma I. When sigma is close to an eigenvalue, the
* computed vector is an accurate eigenvector. Usually, r corresponds
* to the index where the eigenvector is largest in magnitude.
* The following steps accomplish this computation :
* (a) Stationary qd transform, L D L^T - sigma I = L(+) D(+) L(+)^T,
* (b) Progressive qd transform, L D L^T - sigma I = U(-) D(-) U(-)^T,
* (c) Computation of the diagonal elements of the inverse of
* L D L^T - sigma I by combining the above transforms, and choosing
* r as the index where the diagonal of the inverse is (one of the)
* largest in magnitude.
* (d) Computation of the (scaled) r-th column of the inverse using the
* twisted factorization obtained by combining the top part of the
* the stationary and the bottom part of the progressive transform.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix L D L^T.
*
* B1 (input) INTEGER
* First index of the submatrix of L D L^T.
*
* BN (input) INTEGER
* Last index of the submatrix of L D L^T.
*
* LAMBDA (input) REAL
* The shift. In order to compute an accurate eigenvector,
* LAMBDA should be a good approximation to an eigenvalue
* of L D L^T.
*
* L (input) REAL array, dimension (N-1)
* The (n-1) subdiagonal elements of the unit bidiagonal matrix
* L, in elements 1 to N-1.
*
* D (input) REAL array, dimension (N)
* The n diagonal elements of the diagonal matrix D.
*
* LD (input) REAL array, dimension (N-1)
* The n-1 elements L(i)*D(i).
*
* LLD (input) REAL array, dimension (N-1)
* The n-1 elements L(i)*L(i)*D(i).
*
* PIVMIN (input) REAL
* The minimum pivot in the Sturm sequence.
*
* GAPTOL (input) REAL
* Tolerance that indicates when eigenvector entries are neglig
* w.r.t. their contribution to the residual.
*
* Z (input/output) REAL array, dimension (N)
* On input, all entries of Z must be set to 0.
* On output, Z contains the (scaled) r-th column of the
* inverse. The scaling is such that Z(R) equals 1.
*
* WANTNC (input) LOGICAL
* Specifies whether NEGCNT has to be computed.
*
* NEGCNT (output) INTEGER
* If WANTNC is .TRUE. then NEGCNT = the number of pivots < piv
* in the matrix factorization L D L^T, and NEGCNT = -1 otherw
*
* ZTZ (output) REAL
* The square of the 2-norm of Z.
*
* MINGMA (output) REAL
* The reciprocal of the largest (in magnitude) diagonal
* element of the inverse of L D L^T - sigma I.
*
* R (input/output) INTEGER
* The twist index for the twisted factorization used to
* compute Z.
* On input, 0 <= R <= N. If R is input as 0, R is set to
* the index where (L D L^T - sigma I)^{-1} is largest
* in magnitude. If 1 <= R <= N, R is unchanged.
* On output, R contains the twist index used to compute Z.
* Ideally, R designates the position of the maximum entry in t
* eigenvector.
*
* ISUPPZ (output) INTEGER array, dimension (2)
* The support of the vector in Z, i.e., the vector Z is
* nonzero only in elements ISUPPZ(1) through ISUPPZ( 2 ).
*
* NRMINV (output) REAL
* NRMINV = 1/SQRT( ZTZ )
*
* RESID (output) REAL
* The residual of the FP vector.
* RESID = ABS( MINGMA )/SQRT( ZTZ )
*
* RQCORR (output) REAL
* The Rayleigh Quotient correction to LAMBDA.
* RQCORR = MINGMA*TMP
*
* WORK (workspace) REAL array, dimension (4*N)
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param b1
* @param bn
* @param lambda
* @param d
* @param l
* @param ld
* @param lld
* @param pivmin
* @param gaptol
* @param z
* @param wantnc
* @param negcnt
* @param ztz
* @param mingma
* @param r
* @param isuppz
* @param nrminv
* @param resid
* @param rqcorr
* @param work
*
*/
abstract public void slar1v(int n, int b1, int bn, float lambda, float[] d, float[] l, float[] ld, float[] lld, float pivmin, float gaptol, float[] z, boolean wantnc, org.netlib.util.intW negcnt, org.netlib.util.floatW ztz, org.netlib.util.floatW mingma, org.netlib.util.intW r, int[] isuppz, org.netlib.util.floatW nrminv, org.netlib.util.floatW resid, org.netlib.util.floatW rqcorr, float[] work);
/**
*
* ..
*
* Purpose
* =======
*
* SLAR1V computes the (scaled) r-th column of the inverse of
* the sumbmatrix in rows B1 through BN of the tridiagonal matrix
* L D L^T - sigma I. When sigma is close to an eigenvalue, the
* computed vector is an accurate eigenvector. Usually, r corresponds
* to the index where the eigenvector is largest in magnitude.
* The following steps accomplish this computation :
* (a) Stationary qd transform, L D L^T - sigma I = L(+) D(+) L(+)^T,
* (b) Progressive qd transform, L D L^T - sigma I = U(-) D(-) U(-)^T,
* (c) Computation of the diagonal elements of the inverse of
* L D L^T - sigma I by combining the above transforms, and choosing
* r as the index where the diagonal of the inverse is (one of the)
* largest in magnitude.
* (d) Computation of the (scaled) r-th column of the inverse using the
* twisted factorization obtained by combining the top part of the
* the stationary and the bottom part of the progressive transform.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix L D L^T.
*
* B1 (input) INTEGER
* First index of the submatrix of L D L^T.
*
* BN (input) INTEGER
* Last index of the submatrix of L D L^T.
*
* LAMBDA (input) REAL
* The shift. In order to compute an accurate eigenvector,
* LAMBDA should be a good approximation to an eigenvalue
* of L D L^T.
*
* L (input) REAL array, dimension (N-1)
* The (n-1) subdiagonal elements of the unit bidiagonal matrix
* L, in elements 1 to N-1.
*
* D (input) REAL array, dimension (N)
* The n diagonal elements of the diagonal matrix D.
*
* LD (input) REAL array, dimension (N-1)
* The n-1 elements L(i)*D(i).
*
* LLD (input) REAL array, dimension (N-1)
* The n-1 elements L(i)*L(i)*D(i).
*
* PIVMIN (input) REAL
* The minimum pivot in the Sturm sequence.
*
* GAPTOL (input) REAL
* Tolerance that indicates when eigenvector entries are neglig
* w.r.t. their contribution to the residual.
*
* Z (input/output) REAL array, dimension (N)
* On input, all entries of Z must be set to 0.
* On output, Z contains the (scaled) r-th column of the
* inverse. The scaling is such that Z(R) equals 1.
*
* WANTNC (input) LOGICAL
* Specifies whether NEGCNT has to be computed.
*
* NEGCNT (output) INTEGER
* If WANTNC is .TRUE. then NEGCNT = the number of pivots < piv
* in the matrix factorization L D L^T, and NEGCNT = -1 otherw
*
* ZTZ (output) REAL
* The square of the 2-norm of Z.
*
* MINGMA (output) REAL
* The reciprocal of the largest (in magnitude) diagonal
* element of the inverse of L D L^T - sigma I.
*
* R (input/output) INTEGER
* The twist index for the twisted factorization used to
* compute Z.
* On input, 0 <= R <= N. If R is input as 0, R is set to
* the index where (L D L^T - sigma I)^{-1} is largest
* in magnitude. If 1 <= R <= N, R is unchanged.
* On output, R contains the twist index used to compute Z.
* Ideally, R designates the position of the maximum entry in t
* eigenvector.
*
* ISUPPZ (output) INTEGER array, dimension (2)
* The support of the vector in Z, i.e., the vector Z is
* nonzero only in elements ISUPPZ(1) through ISUPPZ( 2 ).
*
* NRMINV (output) REAL
* NRMINV = 1/SQRT( ZTZ )
*
* RESID (output) REAL
* The residual of the FP vector.
* RESID = ABS( MINGMA )/SQRT( ZTZ )
*
* RQCORR (output) REAL
* The Rayleigh Quotient correction to LAMBDA.
* RQCORR = MINGMA*TMP
*
* WORK (workspace) REAL array, dimension (4*N)
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param b1
* @param bn
* @param lambda
* @param d
* @param _d_offset
* @param l
* @param _l_offset
* @param ld
* @param _ld_offset
* @param lld
* @param _lld_offset
* @param pivmin
* @param gaptol
* @param z
* @param _z_offset
* @param wantnc
* @param negcnt
* @param ztz
* @param mingma
* @param r
* @param isuppz
* @param _isuppz_offset
* @param nrminv
* @param resid
* @param rqcorr
* @param work
* @param _work_offset
*
*/
abstract public void slar1v(int n, int b1, int bn, float lambda, float[] d, int _d_offset, float[] l, int _l_offset, float[] ld, int _ld_offset, float[] lld, int _lld_offset, float pivmin, float gaptol, float[] z, int _z_offset, boolean wantnc, org.netlib.util.intW negcnt, org.netlib.util.floatW ztz, org.netlib.util.floatW mingma, org.netlib.util.intW r, int[] isuppz, int _isuppz_offset, org.netlib.util.floatW nrminv, org.netlib.util.floatW resid, org.netlib.util.floatW rqcorr, float[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* SLAR2V applies a vector of real plane rotations from both sides to
* a sequence of 2-by-2 real symmetric matrices, defined by the elements
* of the vectors x, y and z. For i = 1,2,...,n
*
* ( x(i) z(i) ) := ( c(i) s(i) ) ( x(i) z(i) ) ( c(i) -s(i) )
* ( z(i) y(i) ) ( -s(i) c(i) ) ( z(i) y(i) ) ( s(i) c(i) )
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of plane rotations to be applied.
*
* X (input/output) REAL array,
* dimension (1+(N-1)*INCX)
* The vector x.
*
* Y (input/output) REAL array,
* dimension (1+(N-1)*INCX)
* The vector y.
*
* Z (input/output) REAL array,
* dimension (1+(N-1)*INCX)
* The vector z.
*
* INCX (input) INTEGER
* The increment between elements of X, Y and Z. INCX > 0.
*
* C (input) REAL array, dimension (1+(N-1)*INCC)
* The cosines of the plane rotations.
*
* S (input) REAL array, dimension (1+(N-1)*INCC)
* The sines of the plane rotations.
*
* INCC (input) INTEGER
* The increment between elements of C and S. INCC > 0.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n
* @param x
* @param y
* @param z
* @param incx
* @param c
* @param s
* @param incc
*
*/
abstract public void slar2v(int n, float[] x, float[] y, float[] z, int incx, float[] c, float[] s, int incc);
/**
*
* ..
*
* Purpose
* =======
*
* SLAR2V applies a vector of real plane rotations from both sides to
* a sequence of 2-by-2 real symmetric matrices, defined by the elements
* of the vectors x, y and z. For i = 1,2,...,n
*
* ( x(i) z(i) ) := ( c(i) s(i) ) ( x(i) z(i) ) ( c(i) -s(i) )
* ( z(i) y(i) ) ( -s(i) c(i) ) ( z(i) y(i) ) ( s(i) c(i) )
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of plane rotations to be applied.
*
* X (input/output) REAL array,
* dimension (1+(N-1)*INCX)
* The vector x.
*
* Y (input/output) REAL array,
* dimension (1+(N-1)*INCX)
* The vector y.
*
* Z (input/output) REAL array,
* dimension (1+(N-1)*INCX)
* The vector z.
*
* INCX (input) INTEGER
* The increment between elements of X, Y and Z. INCX > 0.
*
* C (input) REAL array, dimension (1+(N-1)*INCC)
* The cosines of the plane rotations.
*
* S (input) REAL array, dimension (1+(N-1)*INCC)
* The sines of the plane rotations.
*
* INCC (input) INTEGER
* The increment between elements of C and S. INCC > 0.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n
* @param x
* @param _x_offset
* @param y
* @param _y_offset
* @param z
* @param _z_offset
* @param incx
* @param c
* @param _c_offset
* @param s
* @param _s_offset
* @param incc
*
*/
abstract public void slar2v(int n, float[] x, int _x_offset, float[] y, int _y_offset, float[] z, int _z_offset, int incx, float[] c, int _c_offset, float[] s, int _s_offset, int incc);
/**
*
* ..
*
* Purpose
* =======
*
* SLARF applies a real elementary reflector H to a real m by n matrix
* C, from either the left or the right. H is represented in the form
*
* H = I - tau * v * v'
*
* where tau is a real scalar and v is a real vector.
*
* If tau = 0, then H is taken to be the unit matrix.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': form H * C
* = 'R': form C * H
*
* M (input) INTEGER
* The number of rows of the matrix C.
*
* N (input) INTEGER
* The number of columns of the matrix C.
*
* V (input) REAL array, dimension
* (1 + (M-1)*abs(INCV)) if SIDE = 'L'
* or (1 + (N-1)*abs(INCV)) if SIDE = 'R'
* The vector v in the representation of H. V is not used if
* TAU = 0.
*
* INCV (input) INTEGER
* The increment between elements of v. INCV <> 0.
*
* TAU (input) REAL
* The value tau in the representation of H.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the m by n matrix C.
* On exit, C is overwritten by the matrix H * C if SIDE = 'L',
* or C * H if SIDE = 'R'.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) REAL array, dimension
* (N) if SIDE = 'L'
* or (M) if SIDE = 'R'
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param m
* @param n
* @param v
* @param incv
* @param tau
* @param c
* @param Ldc
* @param work
*
*/
abstract public void slarf(java.lang.String side, int m, int n, float[] v, int incv, float tau, float[] c, int Ldc, float[] work);
/**
*
* ..
*
* Purpose
* =======
*
* SLARF applies a real elementary reflector H to a real m by n matrix
* C, from either the left or the right. H is represented in the form
*
* H = I - tau * v * v'
*
* where tau is a real scalar and v is a real vector.
*
* If tau = 0, then H is taken to be the unit matrix.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': form H * C
* = 'R': form C * H
*
* M (input) INTEGER
* The number of rows of the matrix C.
*
* N (input) INTEGER
* The number of columns of the matrix C.
*
* V (input) REAL array, dimension
* (1 + (M-1)*abs(INCV)) if SIDE = 'L'
* or (1 + (N-1)*abs(INCV)) if SIDE = 'R'
* The vector v in the representation of H. V is not used if
* TAU = 0.
*
* INCV (input) INTEGER
* The increment between elements of v. INCV <> 0.
*
* TAU (input) REAL
* The value tau in the representation of H.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the m by n matrix C.
* On exit, C is overwritten by the matrix H * C if SIDE = 'L',
* or C * H if SIDE = 'R'.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) REAL array, dimension
* (N) if SIDE = 'L'
* or (M) if SIDE = 'R'
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param m
* @param n
* @param v
* @param _v_offset
* @param incv
* @param tau
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
*
*/
abstract public void slarf(java.lang.String side, int m, int n, float[] v, int _v_offset, int incv, float tau, float[] c, int _c_offset, int Ldc, float[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* SLARFB applies a real block reflector H or its transpose H' to a
* real m by n matrix C, from either the left or the right.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply H or H' from the Left
* = 'R': apply H or H' from the Right
*
* TRANS (input) CHARACTER*1
* = 'N': apply H (No transpose)
* = 'T': apply H' (Transpose)
*
* DIRECT (input) CHARACTER*1
* Indicates how H is formed from a product of elementary
* reflectors
* = 'F': H = H(1) H(2) . . . H(k) (Forward)
* = 'B': H = H(k) . . . H(2) H(1) (Backward)
*
* STOREV (input) CHARACTER*1
* Indicates how the vectors which define the elementary
* reflectors are stored:
* = 'C': Columnwise
* = 'R': Rowwise
*
* M (input) INTEGER
* The number of rows of the matrix C.
*
* N (input) INTEGER
* The number of columns of the matrix C.
*
* K (input) INTEGER
* The order of the matrix T (= the number of elementary
* reflectors whose product defines the block reflector).
*
* V (input) REAL array, dimension
* (LDV,K) if STOREV = 'C'
* (LDV,M) if STOREV = 'R' and SIDE = 'L'
* (LDV,N) if STOREV = 'R' and SIDE = 'R'
* The matrix V. See further details.
*
* LDV (input) INTEGER
* The leading dimension of the array V.
* If STOREV = 'C' and SIDE = 'L', LDV >= max(1,M);
* if STOREV = 'C' and SIDE = 'R', LDV >= max(1,N);
* if STOREV = 'R', LDV >= K.
*
* T (input) REAL array, dimension (LDT,K)
* The triangular k by k matrix T in the representation of the
* block reflector.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= K.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the m by n matrix C.
* On exit, C is overwritten by H*C or H'*C or C*H or C*H'.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDA >= max(1,M).
*
* WORK (workspace) REAL array, dimension (LDWORK,K)
*
* LDWORK (input) INTEGER
* The leading dimension of the array WORK.
* If SIDE = 'L', LDWORK >= max(1,N);
* if SIDE = 'R', LDWORK >= max(1,M).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param direct
* @param storev
* @param m
* @param n
* @param k
* @param v
* @param ldv
* @param t
* @param ldt
* @param c
* @param Ldc
* @param work
* @param ldwork
*
*/
abstract public void slarfb(java.lang.String side, java.lang.String trans, java.lang.String direct, java.lang.String storev, int m, int n, int k, float[] v, int ldv, float[] t, int ldt, float[] c, int Ldc, float[] work, int ldwork);
/**
*
* ..
*
* Purpose
* =======
*
* SLARFB applies a real block reflector H or its transpose H' to a
* real m by n matrix C, from either the left or the right.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply H or H' from the Left
* = 'R': apply H or H' from the Right
*
* TRANS (input) CHARACTER*1
* = 'N': apply H (No transpose)
* = 'T': apply H' (Transpose)
*
* DIRECT (input) CHARACTER*1
* Indicates how H is formed from a product of elementary
* reflectors
* = 'F': H = H(1) H(2) . . . H(k) (Forward)
* = 'B': H = H(k) . . . H(2) H(1) (Backward)
*
* STOREV (input) CHARACTER*1
* Indicates how the vectors which define the elementary
* reflectors are stored:
* = 'C': Columnwise
* = 'R': Rowwise
*
* M (input) INTEGER
* The number of rows of the matrix C.
*
* N (input) INTEGER
* The number of columns of the matrix C.
*
* K (input) INTEGER
* The order of the matrix T (= the number of elementary
* reflectors whose product defines the block reflector).
*
* V (input) REAL array, dimension
* (LDV,K) if STOREV = 'C'
* (LDV,M) if STOREV = 'R' and SIDE = 'L'
* (LDV,N) if STOREV = 'R' and SIDE = 'R'
* The matrix V. See further details.
*
* LDV (input) INTEGER
* The leading dimension of the array V.
* If STOREV = 'C' and SIDE = 'L', LDV >= max(1,M);
* if STOREV = 'C' and SIDE = 'R', LDV >= max(1,N);
* if STOREV = 'R', LDV >= K.
*
* T (input) REAL array, dimension (LDT,K)
* The triangular k by k matrix T in the representation of the
* block reflector.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= K.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the m by n matrix C.
* On exit, C is overwritten by H*C or H'*C or C*H or C*H'.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDA >= max(1,M).
*
* WORK (workspace) REAL array, dimension (LDWORK,K)
*
* LDWORK (input) INTEGER
* The leading dimension of the array WORK.
* If SIDE = 'L', LDWORK >= max(1,N);
* if SIDE = 'R', LDWORK >= max(1,M).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param direct
* @param storev
* @param m
* @param n
* @param k
* @param v
* @param _v_offset
* @param ldv
* @param t
* @param _t_offset
* @param ldt
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param ldwork
*
*/
abstract public void slarfb(java.lang.String side, java.lang.String trans, java.lang.String direct, java.lang.String storev, int m, int n, int k, float[] v, int _v_offset, int ldv, float[] t, int _t_offset, int ldt, float[] c, int _c_offset, int Ldc, float[] work, int _work_offset, int ldwork);
/**
*
* ..
*
* Purpose
* =======
*
* SLARFG generates a real elementary reflector H of order n, such
* that
*
* H * ( alpha ) = ( beta ), H' * H = I.
* ( x ) ( 0 )
*
* where alpha and beta are scalars, and x is an (n-1)-element real
* vector. H is represented in the form
*
* H = I - tau * ( 1 ) * ( 1 v' ) ,
* ( v )
*
* where tau is a real scalar and v is a real (n-1)-element
* vector.
*
* If the elements of x are all zero, then tau = 0 and H is taken to be
* the unit matrix.
*
* Otherwise 1 <= tau <= 2.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the elementary reflector.
*
* ALPHA (input/output) REAL
* On entry, the value alpha.
* On exit, it is overwritten with the value beta.
*
* X (input/output) REAL array, dimension
* (1+(N-2)*abs(INCX))
* On entry, the vector x.
* On exit, it is overwritten with the vector v.
*
* INCX (input) INTEGER
* The increment between elements of X. INCX > 0.
*
* TAU (output) REAL
* The value tau.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param alpha
* @param x
* @param incx
* @param tau
*
*/
abstract public void slarfg(int n, org.netlib.util.floatW alpha, float[] x, int incx, org.netlib.util.floatW tau);
/**
*
* ..
*
* Purpose
* =======
*
* SLARFG generates a real elementary reflector H of order n, such
* that
*
* H * ( alpha ) = ( beta ), H' * H = I.
* ( x ) ( 0 )
*
* where alpha and beta are scalars, and x is an (n-1)-element real
* vector. H is represented in the form
*
* H = I - tau * ( 1 ) * ( 1 v' ) ,
* ( v )
*
* where tau is a real scalar and v is a real (n-1)-element
* vector.
*
* If the elements of x are all zero, then tau = 0 and H is taken to be
* the unit matrix.
*
* Otherwise 1 <= tau <= 2.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the elementary reflector.
*
* ALPHA (input/output) REAL
* On entry, the value alpha.
* On exit, it is overwritten with the value beta.
*
* X (input/output) REAL array, dimension
* (1+(N-2)*abs(INCX))
* On entry, the vector x.
* On exit, it is overwritten with the vector v.
*
* INCX (input) INTEGER
* The increment between elements of X. INCX > 0.
*
* TAU (output) REAL
* The value tau.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param alpha
* @param x
* @param _x_offset
* @param incx
* @param tau
*
*/
abstract public void slarfg(int n, org.netlib.util.floatW alpha, float[] x, int _x_offset, int incx, org.netlib.util.floatW tau);
/**
*
* ..
*
* Purpose
* =======
*
* SLARFT forms the triangular factor T of a real block reflector H
* of order n, which is defined as a product of k elementary reflectors.
*
* If DIRECT = 'F', H = H(1) H(2) . . . H(k) and T is upper triangular;
*
* If DIRECT = 'B', H = H(k) . . . H(2) H(1) and T is lower triangular.
*
* If STOREV = 'C', the vector which defines the elementary reflector
* H(i) is stored in the i-th column of the array V, and
*
* H = I - V * T * V'
*
* If STOREV = 'R', the vector which defines the elementary reflector
* H(i) is stored in the i-th row of the array V, and
*
* H = I - V' * T * V
*
* Arguments
* =========
*
* DIRECT (input) CHARACTER*1
* Specifies the order in which the elementary reflectors are
* multiplied to form the block reflector:
* = 'F': H = H(1) H(2) . . . H(k) (Forward)
* = 'B': H = H(k) . . . H(2) H(1) (Backward)
*
* STOREV (input) CHARACTER*1
* Specifies how the vectors which define the elementary
* reflectors are stored (see also Further Details):
* = 'C': columnwise
* = 'R': rowwise
*
* N (input) INTEGER
* The order of the block reflector H. N >= 0.
*
* K (input) INTEGER
* The order of the triangular factor T (= the number of
* elementary reflectors). K >= 1.
*
* V (input/output) REAL array, dimension
* (LDV,K) if STOREV = 'C'
* (LDV,N) if STOREV = 'R'
* The matrix V. See further details.
*
* LDV (input) INTEGER
* The leading dimension of the array V.
* If STOREV = 'C', LDV >= max(1,N); if STOREV = 'R', LDV >= K.
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i).
*
* T (output) REAL array, dimension (LDT,K)
* The k by k triangular factor T of the block reflector.
* If DIRECT = 'F', T is upper triangular; if DIRECT = 'B', T is
* lower triangular. The rest of the array is not used.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= K.
*
* Further Details
* ===============
*
* The shape of the matrix V and the storage of the vectors which define
* the H(i) is best illustrated by the following example with n = 5 and
* k = 3. The elements equal to 1 are not stored; the corresponding
* array elements are modified but restored on exit. The rest of the
* array is not used.
*
* DIRECT = 'F' and STOREV = 'C': DIRECT = 'F' and STOREV = 'R':
*
* V = ( 1 ) V = ( 1 v1 v1 v1 v1 )
* ( v1 1 ) ( 1 v2 v2 v2 )
* ( v1 v2 1 ) ( 1 v3 v3 )
* ( v1 v2 v3 )
* ( v1 v2 v3 )
*
* DIRECT = 'B' and STOREV = 'C': DIRECT = 'B' and STOREV = 'R':
*
* V = ( v1 v2 v3 ) V = ( v1 v1 1 )
* ( v1 v2 v3 ) ( v2 v2 v2 1 )
* ( 1 v2 v3 ) ( v3 v3 v3 v3 1 )
* ( 1 v3 )
* ( 1 )
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param direct
* @param storev
* @param n
* @param k
* @param v
* @param ldv
* @param tau
* @param t
* @param ldt
*
*/
abstract public void slarft(java.lang.String direct, java.lang.String storev, int n, int k, float[] v, int ldv, float[] tau, float[] t, int ldt);
/**
*
* ..
*
* Purpose
* =======
*
* SLARFT forms the triangular factor T of a real block reflector H
* of order n, which is defined as a product of k elementary reflectors.
*
* If DIRECT = 'F', H = H(1) H(2) . . . H(k) and T is upper triangular;
*
* If DIRECT = 'B', H = H(k) . . . H(2) H(1) and T is lower triangular.
*
* If STOREV = 'C', the vector which defines the elementary reflector
* H(i) is stored in the i-th column of the array V, and
*
* H = I - V * T * V'
*
* If STOREV = 'R', the vector which defines the elementary reflector
* H(i) is stored in the i-th row of the array V, and
*
* H = I - V' * T * V
*
* Arguments
* =========
*
* DIRECT (input) CHARACTER*1
* Specifies the order in which the elementary reflectors are
* multiplied to form the block reflector:
* = 'F': H = H(1) H(2) . . . H(k) (Forward)
* = 'B': H = H(k) . . . H(2) H(1) (Backward)
*
* STOREV (input) CHARACTER*1
* Specifies how the vectors which define the elementary
* reflectors are stored (see also Further Details):
* = 'C': columnwise
* = 'R': rowwise
*
* N (input) INTEGER
* The order of the block reflector H. N >= 0.
*
* K (input) INTEGER
* The order of the triangular factor T (= the number of
* elementary reflectors). K >= 1.
*
* V (input/output) REAL array, dimension
* (LDV,K) if STOREV = 'C'
* (LDV,N) if STOREV = 'R'
* The matrix V. See further details.
*
* LDV (input) INTEGER
* The leading dimension of the array V.
* If STOREV = 'C', LDV >= max(1,N); if STOREV = 'R', LDV >= K.
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i).
*
* T (output) REAL array, dimension (LDT,K)
* The k by k triangular factor T of the block reflector.
* If DIRECT = 'F', T is upper triangular; if DIRECT = 'B', T is
* lower triangular. The rest of the array is not used.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= K.
*
* Further Details
* ===============
*
* The shape of the matrix V and the storage of the vectors which define
* the H(i) is best illustrated by the following example with n = 5 and
* k = 3. The elements equal to 1 are not stored; the corresponding
* array elements are modified but restored on exit. The rest of the
* array is not used.
*
* DIRECT = 'F' and STOREV = 'C': DIRECT = 'F' and STOREV = 'R':
*
* V = ( 1 ) V = ( 1 v1 v1 v1 v1 )
* ( v1 1 ) ( 1 v2 v2 v2 )
* ( v1 v2 1 ) ( 1 v3 v3 )
* ( v1 v2 v3 )
* ( v1 v2 v3 )
*
* DIRECT = 'B' and STOREV = 'C': DIRECT = 'B' and STOREV = 'R':
*
* V = ( v1 v2 v3 ) V = ( v1 v1 1 )
* ( v1 v2 v3 ) ( v2 v2 v2 1 )
* ( 1 v2 v3 ) ( v3 v3 v3 v3 1 )
* ( 1 v3 )
* ( 1 )
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param direct
* @param storev
* @param n
* @param k
* @param v
* @param _v_offset
* @param ldv
* @param tau
* @param _tau_offset
* @param t
* @param _t_offset
* @param ldt
*
*/
abstract public void slarft(java.lang.String direct, java.lang.String storev, int n, int k, float[] v, int _v_offset, int ldv, float[] tau, int _tau_offset, float[] t, int _t_offset, int ldt);
/**
*
* ..
*
* Purpose
* =======
*
* SLARFX applies a real elementary reflector H to a real m by n
* matrix C, from either the left or the right. H is represented in the
* form
*
* H = I - tau * v * v'
*
* where tau is a real scalar and v is a real vector.
*
* If tau = 0, then H is taken to be the unit matrix
*
* This version uses inline code if H has order < 11.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': form H * C
* = 'R': form C * H
*
* M (input) INTEGER
* The number of rows of the matrix C.
*
* N (input) INTEGER
* The number of columns of the matrix C.
*
* V (input) REAL array, dimension (M) if SIDE = 'L'
* or (N) if SIDE = 'R'
* The vector v in the representation of H.
*
* TAU (input) REAL
* The value tau in the representation of H.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the m by n matrix C.
* On exit, C is overwritten by the matrix H * C if SIDE = 'L',
* or C * H if SIDE = 'R'.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDA >= (1,M).
*
* WORK (workspace) REAL array, dimension
* (N) if SIDE = 'L'
* or (M) if SIDE = 'R'
* WORK is not referenced if H has order < 11.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param m
* @param n
* @param v
* @param tau
* @param c
* @param Ldc
* @param work
*
*/
abstract public void slarfx(java.lang.String side, int m, int n, float[] v, float tau, float[] c, int Ldc, float[] work);
/**
*
* ..
*
* Purpose
* =======
*
* SLARFX applies a real elementary reflector H to a real m by n
* matrix C, from either the left or the right. H is represented in the
* form
*
* H = I - tau * v * v'
*
* where tau is a real scalar and v is a real vector.
*
* If tau = 0, then H is taken to be the unit matrix
*
* This version uses inline code if H has order < 11.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': form H * C
* = 'R': form C * H
*
* M (input) INTEGER
* The number of rows of the matrix C.
*
* N (input) INTEGER
* The number of columns of the matrix C.
*
* V (input) REAL array, dimension (M) if SIDE = 'L'
* or (N) if SIDE = 'R'
* The vector v in the representation of H.
*
* TAU (input) REAL
* The value tau in the representation of H.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the m by n matrix C.
* On exit, C is overwritten by the matrix H * C if SIDE = 'L',
* or C * H if SIDE = 'R'.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDA >= (1,M).
*
* WORK (workspace) REAL array, dimension
* (N) if SIDE = 'L'
* or (M) if SIDE = 'R'
* WORK is not referenced if H has order < 11.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param m
* @param n
* @param v
* @param _v_offset
* @param tau
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
*
*/
abstract public void slarfx(java.lang.String side, int m, int n, float[] v, int _v_offset, float tau, float[] c, int _c_offset, int Ldc, float[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* SLARGV generates a vector of real plane rotations, determined by
* elements of the real vectors x and y. For i = 1,2,...,n
*
* ( c(i) s(i) ) ( x(i) ) = ( a(i) )
* ( -s(i) c(i) ) ( y(i) ) = ( 0 )
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of plane rotations to be generated.
*
* X (input/output) REAL array,
* dimension (1+(N-1)*INCX)
* On entry, the vector x.
* On exit, x(i) is overwritten by a(i), for i = 1,...,n.
*
* INCX (input) INTEGER
* The increment between elements of X. INCX > 0.
*
* Y (input/output) REAL array,
* dimension (1+(N-1)*INCY)
* On entry, the vector y.
* On exit, the sines of the plane rotations.
*
* INCY (input) INTEGER
* The increment between elements of Y. INCY > 0.
*
* C (output) REAL array, dimension (1+(N-1)*INCC)
* The cosines of the plane rotations.
*
* INCC (input) INTEGER
* The increment between elements of C. INCC > 0.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param x
* @param incx
* @param y
* @param incy
* @param c
* @param incc
*
*/
abstract public void slargv(int n, float[] x, int incx, float[] y, int incy, float[] c, int incc);
/**
*
* ..
*
* Purpose
* =======
*
* SLARGV generates a vector of real plane rotations, determined by
* elements of the real vectors x and y. For i = 1,2,...,n
*
* ( c(i) s(i) ) ( x(i) ) = ( a(i) )
* ( -s(i) c(i) ) ( y(i) ) = ( 0 )
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of plane rotations to be generated.
*
* X (input/output) REAL array,
* dimension (1+(N-1)*INCX)
* On entry, the vector x.
* On exit, x(i) is overwritten by a(i), for i = 1,...,n.
*
* INCX (input) INTEGER
* The increment between elements of X. INCX > 0.
*
* Y (input/output) REAL array,
* dimension (1+(N-1)*INCY)
* On entry, the vector y.
* On exit, the sines of the plane rotations.
*
* INCY (input) INTEGER
* The increment between elements of Y. INCY > 0.
*
* C (output) REAL array, dimension (1+(N-1)*INCC)
* The cosines of the plane rotations.
*
* INCC (input) INTEGER
* The increment between elements of C. INCC > 0.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param x
* @param _x_offset
* @param incx
* @param y
* @param _y_offset
* @param incy
* @param c
* @param _c_offset
* @param incc
*
*/
abstract public void slargv(int n, float[] x, int _x_offset, int incx, float[] y, int _y_offset, int incy, float[] c, int _c_offset, int incc);
/**
*
* ..
*
* Purpose
* =======
*
* SLARNV returns a vector of n random real numbers from a uniform or
* normal distribution.
*
* Arguments
* =========
*
* IDIST (input) INTEGER
* Specifies the distribution of the random numbers:
* = 1: uniform (0,1)
* = 2: uniform (-1,1)
* = 3: normal (0,1)
*
* ISEED (input/output) INTEGER array, dimension (4)
* On entry, the seed of the random number generator; the array
* elements must be between 0 and 4095, and ISEED(4) must be
* odd.
* On exit, the seed is updated.
*
* N (input) INTEGER
* The number of random numbers to be generated.
*
* X (output) REAL array, dimension (N)
* The generated random numbers.
*
* Further Details
* ===============
*
* This routine calls the auxiliary routine SLARUV to generate random
* real numbers from a uniform (0,1) distribution, in batches of up to
* 128 using vectorisable code. The Box-Muller method is used to
* transform numbers from a uniform to a normal distribution.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param idist
* @param iseed
* @param n
* @param x
*
*/
abstract public void slarnv(int idist, int[] iseed, int n, float[] x);
/**
*
* ..
*
* Purpose
* =======
*
* SLARNV returns a vector of n random real numbers from a uniform or
* normal distribution.
*
* Arguments
* =========
*
* IDIST (input) INTEGER
* Specifies the distribution of the random numbers:
* = 1: uniform (0,1)
* = 2: uniform (-1,1)
* = 3: normal (0,1)
*
* ISEED (input/output) INTEGER array, dimension (4)
* On entry, the seed of the random number generator; the array
* elements must be between 0 and 4095, and ISEED(4) must be
* odd.
* On exit, the seed is updated.
*
* N (input) INTEGER
* The number of random numbers to be generated.
*
* X (output) REAL array, dimension (N)
* The generated random numbers.
*
* Further Details
* ===============
*
* This routine calls the auxiliary routine SLARUV to generate random
* real numbers from a uniform (0,1) distribution, in batches of up to
* 128 using vectorisable code. The Box-Muller method is used to
* transform numbers from a uniform to a normal distribution.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param idist
* @param iseed
* @param _iseed_offset
* @param n
* @param x
* @param _x_offset
*
*/
abstract public void slarnv(int idist, int[] iseed, int _iseed_offset, int n, float[] x, int _x_offset);
/**
*
* ..
*
* Purpose
* =======
*
* Compute the splitting points with threshold SPLTOL.
* SLARRA sets any "small" off-diagonal elements to zero.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix. N > 0.
*
* D (input) REAL array, dimension (N)
* On entry, the N diagonal elements of the tridiagonal
* matrix T.
*
* E (input/output) REAL array, dimension (N)
* On entry, the first (N-1) entries contain the subdiagonal
* elements of the tridiagonal matrix T; E(N) need not be set.
* On exit, the entries E( ISPLIT( I ) ), 1 <= I <= NSPLIT,
* are set to zero, the other entries of E are untouched.
*
* E2 (input/output) REAL array, dimension (N)
* On entry, the first (N-1) entries contain the SQUARES of the
* subdiagonal elements of the tridiagonal matrix T;
* E2(N) need not be set.
* On exit, the entries E2( ISPLIT( I ) ),
* 1 <= I <= NSPLIT, have been set to zero
*
* SPLTOL (input) REAL
* The threshold for splitting. Two criteria can be used:
* SPLTOL<0 : criterion based on absolute off-diagonal value
* SPLTOL>0 : criterion that preserves relative accuracy
*
* TNRM (input) REAL
* The norm of the matrix.
*
* NSPLIT (output) INTEGER
* The number of blocks T splits into. 1 <= NSPLIT <= N.
*
* ISPLIT (output) INTEGER array, dimension (N)
* The splitting points, at which T breaks up into blocks.
* The first block consists of rows/columns 1 to ISPLIT(1),
* the second of rows/columns ISPLIT(1)+1 through ISPLIT(2),
* etc., and the NSPLIT-th consists of rows/columns
* ISPLIT(NSPLIT-1)+1 through ISPLIT(NSPLIT)=N.
*
*
* INFO (output) INTEGER
* = 0: successful exit
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param e
* @param e2
* @param spltol
* @param tnrm
* @param nsplit
* @param isplit
* @param info
*
*/
abstract public void slarra(int n, float[] d, float[] e, float[] e2, float spltol, float tnrm, org.netlib.util.intW nsplit, int[] isplit, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* Compute the splitting points with threshold SPLTOL.
* SLARRA sets any "small" off-diagonal elements to zero.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix. N > 0.
*
* D (input) REAL array, dimension (N)
* On entry, the N diagonal elements of the tridiagonal
* matrix T.
*
* E (input/output) REAL array, dimension (N)
* On entry, the first (N-1) entries contain the subdiagonal
* elements of the tridiagonal matrix T; E(N) need not be set.
* On exit, the entries E( ISPLIT( I ) ), 1 <= I <= NSPLIT,
* are set to zero, the other entries of E are untouched.
*
* E2 (input/output) REAL array, dimension (N)
* On entry, the first (N-1) entries contain the SQUARES of the
* subdiagonal elements of the tridiagonal matrix T;
* E2(N) need not be set.
* On exit, the entries E2( ISPLIT( I ) ),
* 1 <= I <= NSPLIT, have been set to zero
*
* SPLTOL (input) REAL
* The threshold for splitting. Two criteria can be used:
* SPLTOL<0 : criterion based on absolute off-diagonal value
* SPLTOL>0 : criterion that preserves relative accuracy
*
* TNRM (input) REAL
* The norm of the matrix.
*
* NSPLIT (output) INTEGER
* The number of blocks T splits into. 1 <= NSPLIT <= N.
*
* ISPLIT (output) INTEGER array, dimension (N)
* The splitting points, at which T breaks up into blocks.
* The first block consists of rows/columns 1 to ISPLIT(1),
* the second of rows/columns ISPLIT(1)+1 through ISPLIT(2),
* etc., and the NSPLIT-th consists of rows/columns
* ISPLIT(NSPLIT-1)+1 through ISPLIT(NSPLIT)=N.
*
*
* INFO (output) INTEGER
* = 0: successful exit
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param e2
* @param _e2_offset
* @param spltol
* @param tnrm
* @param nsplit
* @param isplit
* @param _isplit_offset
* @param info
*
*/
abstract public void slarra(int n, float[] d, int _d_offset, float[] e, int _e_offset, float[] e2, int _e2_offset, float spltol, float tnrm, org.netlib.util.intW nsplit, int[] isplit, int _isplit_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* Given the relatively robust representation(RRR) L D L^T, SLARRB
* does "limited" bisection to refine the eigenvalues of L D L^T,
* W( IFIRST-OFFSET ) through W( ILAST-OFFSET ), to more accuracy. Initi
* guesses for these eigenvalues are input in W, the corresponding estim
* of the error in these guesses and their gaps are input in WERR
* and WGAP, respectively. During bisection, intervals
* [left, right] are maintained by storing their mid-points and
* semi-widths in the arrays W and WERR respectively.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix.
*
* D (input) REAL array, dimension (N)
* The N diagonal elements of the diagonal matrix D.
*
* LLD (input) REAL array, dimension (N-1)
* The (N-1) elements L(i)*L(i)*D(i).
*
* IFIRST (input) INTEGER
* The index of the first eigenvalue to be computed.
*
* ILAST (input) INTEGER
* The index of the last eigenvalue to be computed.
*
* RTOL1 (input) REAL
* RTOL2 (input) REAL
* Tolerance for the convergence of the bisection intervals.
* An interval [LEFT,RIGHT] has converged if
* RIGHT-LEFT.LT.MAX( RTOL1*GAP, RTOL2*MAX(|LEFT|,|RIGHT|) )
* where GAP is the (estimated) distance to the nearest
* eigenvalue.
*
* OFFSET (input) INTEGER
* Offset for the arrays W, WGAP and WERR, i.e., the IFIRST-OFFS
* through ILAST-OFFSET elements of these arrays are to be used.
*
* W (input/output) REAL array, dimension (N)
* On input, W( IFIRST-OFFSET ) through W( ILAST-OFFSET ) are
* estimates of the eigenvalues of L D L^T indexed IFIRST throug
* ILAST.
* On output, these estimates are refined.
*
* WGAP (input/output) REAL array, dimension (N-1)
* On input, the (estimated) gaps between consecutive
* eigenvalues of L D L^T, i.e., WGAP(I-OFFSET) is the gap betwe
* eigenvalues I and I+1. Note that if IFIRST.EQ.ILAST
* then WGAP(IFIRST-OFFSET) must be set to ZERO.
* On output, these gaps are refined.
*
* WERR (input/output) REAL array, dimension (N)
* On input, WERR( IFIRST-OFFSET ) through WERR( ILAST-OFFSET )
* the errors in the estimates of the corresponding elements in
* On output, these errors are refined.
*
* WORK (workspace) REAL array, dimension (2*N)
* Workspace.
*
* IWORK (workspace) INTEGER array, dimension (2*N)
* Workspace.
*
* PIVMIN (input) DOUBLE PRECISION
* The minimum pivot in the Sturm sequence.
*
* SPDIAM (input) DOUBLE PRECISION
* The spectral diameter of the matrix.
*
* TWIST (input) INTEGER
* The twist index for the twisted factorization that is used
* for the negcount.
* TWIST = N: Compute negcount from L D L^T - LAMBDA I = L+ D+ L
* TWIST = 1: Compute negcount from L D L^T - LAMBDA I = U- D- U
* TWIST = R: Compute negcount from L D L^T - LAMBDA I = N(r) D(
*
* INFO (output) INTEGER
* Error flag.
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param lld
* @param ifirst
* @param ilast
* @param rtol1
* @param rtol2
* @param offset
* @param w
* @param wgap
* @param werr
* @param work
* @param iwork
* @param pivmin
* @param spdiam
* @param twist
* @param info
*
*/
abstract public void slarrb(int n, float[] d, float[] lld, int ifirst, int ilast, float rtol1, float rtol2, int offset, float[] w, float[] wgap, float[] werr, float[] work, int[] iwork, float pivmin, float spdiam, int twist, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* Given the relatively robust representation(RRR) L D L^T, SLARRB
* does "limited" bisection to refine the eigenvalues of L D L^T,
* W( IFIRST-OFFSET ) through W( ILAST-OFFSET ), to more accuracy. Initi
* guesses for these eigenvalues are input in W, the corresponding estim
* of the error in these guesses and their gaps are input in WERR
* and WGAP, respectively. During bisection, intervals
* [left, right] are maintained by storing their mid-points and
* semi-widths in the arrays W and WERR respectively.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix.
*
* D (input) REAL array, dimension (N)
* The N diagonal elements of the diagonal matrix D.
*
* LLD (input) REAL array, dimension (N-1)
* The (N-1) elements L(i)*L(i)*D(i).
*
* IFIRST (input) INTEGER
* The index of the first eigenvalue to be computed.
*
* ILAST (input) INTEGER
* The index of the last eigenvalue to be computed.
*
* RTOL1 (input) REAL
* RTOL2 (input) REAL
* Tolerance for the convergence of the bisection intervals.
* An interval [LEFT,RIGHT] has converged if
* RIGHT-LEFT.LT.MAX( RTOL1*GAP, RTOL2*MAX(|LEFT|,|RIGHT|) )
* where GAP is the (estimated) distance to the nearest
* eigenvalue.
*
* OFFSET (input) INTEGER
* Offset for the arrays W, WGAP and WERR, i.e., the IFIRST-OFFS
* through ILAST-OFFSET elements of these arrays are to be used.
*
* W (input/output) REAL array, dimension (N)
* On input, W( IFIRST-OFFSET ) through W( ILAST-OFFSET ) are
* estimates of the eigenvalues of L D L^T indexed IFIRST throug
* ILAST.
* On output, these estimates are refined.
*
* WGAP (input/output) REAL array, dimension (N-1)
* On input, the (estimated) gaps between consecutive
* eigenvalues of L D L^T, i.e., WGAP(I-OFFSET) is the gap betwe
* eigenvalues I and I+1. Note that if IFIRST.EQ.ILAST
* then WGAP(IFIRST-OFFSET) must be set to ZERO.
* On output, these gaps are refined.
*
* WERR (input/output) REAL array, dimension (N)
* On input, WERR( IFIRST-OFFSET ) through WERR( ILAST-OFFSET )
* the errors in the estimates of the corresponding elements in
* On output, these errors are refined.
*
* WORK (workspace) REAL array, dimension (2*N)
* Workspace.
*
* IWORK (workspace) INTEGER array, dimension (2*N)
* Workspace.
*
* PIVMIN (input) DOUBLE PRECISION
* The minimum pivot in the Sturm sequence.
*
* SPDIAM (input) DOUBLE PRECISION
* The spectral diameter of the matrix.
*
* TWIST (input) INTEGER
* The twist index for the twisted factorization that is used
* for the negcount.
* TWIST = N: Compute negcount from L D L^T - LAMBDA I = L+ D+ L
* TWIST = 1: Compute negcount from L D L^T - LAMBDA I = U- D- U
* TWIST = R: Compute negcount from L D L^T - LAMBDA I = N(r) D(
*
* INFO (output) INTEGER
* Error flag.
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param _d_offset
* @param lld
* @param _lld_offset
* @param ifirst
* @param ilast
* @param rtol1
* @param rtol2
* @param offset
* @param w
* @param _w_offset
* @param wgap
* @param _wgap_offset
* @param werr
* @param _werr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param pivmin
* @param spdiam
* @param twist
* @param info
*
*/
abstract public void slarrb(int n, float[] d, int _d_offset, float[] lld, int _lld_offset, int ifirst, int ilast, float rtol1, float rtol2, int offset, float[] w, int _w_offset, float[] wgap, int _wgap_offset, float[] werr, int _werr_offset, float[] work, int _work_offset, int[] iwork, int _iwork_offset, float pivmin, float spdiam, int twist, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* Find the number of eigenvalues of the symmetric tridiagonal matrix T
* that are in the interval (VL,VU] if JOBT = 'T', and of L D L^T
* if JOBT = 'L'.
*
* Arguments
* =========
*
* JOBT (input) CHARACTER*1
* = 'T': Compute Sturm count for matrix T.
* = 'L': Compute Sturm count for matrix L D L^T.
*
* N (input) INTEGER
* The order of the matrix. N > 0.
*
* VL (input) DOUBLE PRECISION
* VU (input) DOUBLE PRECISION
* The lower and upper bounds for the eigenvalues.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* JOBT = 'T': The N diagonal elements of the tridiagonal matrix
* JOBT = 'L': The N diagonal elements of the diagonal matrix D.
*
* E (input) DOUBLE PRECISION array, dimension (N)
* JOBT = 'T': The N-1 offdiagonal elements of the matrix T.
* JOBT = 'L': The N-1 offdiagonal elements of the matrix L.
*
* PIVMIN (input) DOUBLE PRECISION
* The minimum pivot in the Sturm sequence for T.
*
* EIGCNT (output) INTEGER
* The number of eigenvalues of the symmetric tridiagonal matrix
* that are in the interval (VL,VU]
*
* LCNT (output) INTEGER
* RCNT (output) INTEGER
* The left and right negcounts of the interval.
*
* INFO (output) INTEGER
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobt
* @param n
* @param vl
* @param vu
* @param d
* @param e
* @param pivmin
* @param eigcnt
* @param lcnt
* @param rcnt
* @param info
*
*/
abstract public void slarrc(java.lang.String jobt, int n, float vl, float vu, float[] d, float[] e, float pivmin, org.netlib.util.intW eigcnt, org.netlib.util.intW lcnt, org.netlib.util.intW rcnt, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* Find the number of eigenvalues of the symmetric tridiagonal matrix T
* that are in the interval (VL,VU] if JOBT = 'T', and of L D L^T
* if JOBT = 'L'.
*
* Arguments
* =========
*
* JOBT (input) CHARACTER*1
* = 'T': Compute Sturm count for matrix T.
* = 'L': Compute Sturm count for matrix L D L^T.
*
* N (input) INTEGER
* The order of the matrix. N > 0.
*
* VL (input) DOUBLE PRECISION
* VU (input) DOUBLE PRECISION
* The lower and upper bounds for the eigenvalues.
*
* D (input) DOUBLE PRECISION array, dimension (N)
* JOBT = 'T': The N diagonal elements of the tridiagonal matrix
* JOBT = 'L': The N diagonal elements of the diagonal matrix D.
*
* E (input) DOUBLE PRECISION array, dimension (N)
* JOBT = 'T': The N-1 offdiagonal elements of the matrix T.
* JOBT = 'L': The N-1 offdiagonal elements of the matrix L.
*
* PIVMIN (input) DOUBLE PRECISION
* The minimum pivot in the Sturm sequence for T.
*
* EIGCNT (output) INTEGER
* The number of eigenvalues of the symmetric tridiagonal matrix
* that are in the interval (VL,VU]
*
* LCNT (output) INTEGER
* RCNT (output) INTEGER
* The left and right negcounts of the interval.
*
* INFO (output) INTEGER
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobt
* @param n
* @param vl
* @param vu
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param pivmin
* @param eigcnt
* @param lcnt
* @param rcnt
* @param info
*
*/
abstract public void slarrc(java.lang.String jobt, int n, float vl, float vu, float[] d, int _d_offset, float[] e, int _e_offset, float pivmin, org.netlib.util.intW eigcnt, org.netlib.util.intW lcnt, org.netlib.util.intW rcnt, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLARRD computes the eigenvalues of a symmetric tridiagonal
* matrix T to suitable accuracy. This is an auxiliary code to be
* called from SSTEMR.
* The user may ask for all eigenvalues, all eigenvalues
* in the half-open interval (VL, VU], or the IL-th through IU-th
* eigenvalues.
*
* To avoid overflow, the matrix must be scaled so that its
* largest element is no greater than overflow**(1/2) *
* underflow**(1/4) in absolute value, and for greatest
* accuracy, it should not be much smaller than that.
*
* See W. Kahan "Accurate Eigenvalues of a Symmetric Tridiagonal
* Matrix", Report CS41, Computer Science Dept., Stanford
* University, July 21, 1966.
*
* Arguments
* =========
*
* RANGE (input) CHARACTER
* = 'A': ("All") all eigenvalues will be found.
* = 'V': ("Value") all eigenvalues in the half-open interval
* (VL, VU] will be found.
* = 'I': ("Index") the IL-th through IU-th eigenvalues (of the
* entire matrix) will be found.
*
* ORDER (input) CHARACTER
* = 'B': ("By Block") the eigenvalues will be grouped by
* split-off block (see IBLOCK, ISPLIT) and
* ordered from smallest to largest within
* the block.
* = 'E': ("Entire matrix")
* the eigenvalues for the entire matrix
* will be ordered from smallest to
* largest.
*
* N (input) INTEGER
* The order of the tridiagonal matrix T. N >= 0.
*
* VL (input) REAL
* VU (input) REAL
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. Eigenvalues less than or equal
* to VL, or greater than VU, will not be returned. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* GERS (input) REAL array, dimension (2*N)
* The N Gerschgorin intervals (the i-th Gerschgorin interval
* is (GERS(2*i-1), GERS(2*i)).
*
* RELTOL (input) REAL
* The minimum relative width of an interval. When an interval
* is narrower than RELTOL times the larger (in
* magnitude) endpoint, then it is considered to be
* sufficiently small, i.e., converged. Note: this should
* always be at least radix*machine epsilon.
*
* D (input) REAL array, dimension (N)
* The n diagonal elements of the tridiagonal matrix T.
*
* E (input) REAL array, dimension (N-1)
* The (n-1) off-diagonal elements of the tridiagonal matrix T.
*
* E2 (input) REAL array, dimension (N-1)
* The (n-1) squared off-diagonal elements of the tridiagonal ma
*
* PIVMIN (input) REAL
* The minimum pivot allowed in the Sturm sequence for T.
*
* NSPLIT (input) INTEGER
* The number of diagonal blocks in the matrix T.
* 1 <= NSPLIT <= N.
*
* ISPLIT (input) INTEGER array, dimension (N)
* The splitting points, at which T breaks up into submatrices.
* The first submatrix consists of rows/columns 1 to ISPLIT(1),
* the second of rows/columns ISPLIT(1)+1 through ISPLIT(2),
* etc., and the NSPLIT-th consists of rows/columns
* ISPLIT(NSPLIT-1)+1 through ISPLIT(NSPLIT)=N.
* (Only the first NSPLIT elements will actually be used, but
* since the user cannot know a priori what value NSPLIT will
* have, N words must be reserved for ISPLIT.)
*
* M (output) INTEGER
* The actual number of eigenvalues found. 0 <= M <= N.
* (See also the description of INFO=2,3.)
*
* W (output) REAL array, dimension (N)
* On exit, the first M elements of W will contain the
* eigenvalue approximations. SLARRD computes an interval
* I_j = (a_j, b_j] that includes eigenvalue j. The eigenvalue
* approximation is given as the interval midpoint
* W(j)= ( a_j + b_j)/2. The corresponding error is bounded by
* WERR(j) = abs( a_j - b_j)/2
*
* WERR (output) REAL array, dimension (N)
* The error bound on the corresponding eigenvalue approximation
* in W.
*
* WL (output) REAL
* WU (output) REAL
* The interval (WL, WU] contains all the wanted eigenvalues.
* If RANGE='V', then WL=VL and WU=VU.
* If RANGE='A', then WL and WU are the global Gerschgorin bound
* on the spectrum.
* If RANGE='I', then WL and WU are computed by SLAEBZ from the
* index range specified.
*
* IBLOCK (output) INTEGER array, dimension (N)
* At each row/column j where E(j) is zero or small, the
* matrix T is considered to split into a block diagonal
* matrix. On exit, if INFO = 0, IBLOCK(i) specifies to which
* block (from 1 to the number of blocks) the eigenvalue W(i)
* belongs. (SLARRD may use the remaining N-M elements as
* workspace.)
*
* INDEXW (output) INTEGER array, dimension (N)
* The indices of the eigenvalues within each block (submatrix);
* for example, INDEXW(i)= j and IBLOCK(i)=k imply that the
* i-th eigenvalue W(i) is the j-th eigenvalue in block k.
*
* WORK (workspace) REAL array, dimension (4*N)
*
* IWORK (workspace) INTEGER array, dimension (3*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: some or all of the eigenvalues failed to converge or
* were not computed:
* =1 or 3: Bisection failed to converge for some
* eigenvalues; these eigenvalues are flagged by a
* negative block number. The effect is that the
* eigenvalues may not be as accurate as the
* absolute and relative tolerances. This is
* generally caused by unexpectedly inaccurate
* arithmetic.
* =2 or 3: RANGE='I' only: Not all of the eigenvalues
* IL:IU were found.
* Effect: M < IU+1-IL
* Cause: non-monotonic arithmetic, causing the
* Sturm sequence to be non-monotonic.
* Cure: recalculate, using RANGE='A', and pick
* out eigenvalues IL:IU. In some cases,
* increasing the PARAMETER "FUDGE" may
* make things work.
* = 4: RANGE='I', and the Gershgorin interval
* initially used was too small. No eigenvalues
* were computed.
* Probable cause: your machine has sloppy
* floating-point arithmetic.
* Cure: Increase the PARAMETER "FUDGE",
* recompile, and try again.
*
* Internal Parameters
* ===================
*
* FUDGE REAL , default = 2
* A "fudge factor" to widen the Gershgorin intervals. Ideally,
* a value of 1 should work, but on machines with sloppy
* arithmetic, this needs to be larger. The default for
* publicly released versions should be large enough to handle
* the worst machine around. Note that this has no effect
* on accuracy of the solution.
*
* Based on contributions by
* W. Kahan, University of California, Berkeley, USA
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param range
* @param order
* @param n
* @param vl
* @param vu
* @param il
* @param iu
* @param gers
* @param reltol
* @param d
* @param e
* @param e2
* @param pivmin
* @param nsplit
* @param isplit
* @param m
* @param w
* @param werr
* @param wl
* @param wu
* @param iblock
* @param indexw
* @param work
* @param iwork
* @param info
*
*/
abstract public void slarrd(java.lang.String range, java.lang.String order, int n, float vl, float vu, int il, int iu, float[] gers, float reltol, float[] d, float[] e, float[] e2, float pivmin, int nsplit, int[] isplit, org.netlib.util.intW m, float[] w, float[] werr, org.netlib.util.floatW wl, org.netlib.util.floatW wu, int[] iblock, int[] indexw, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLARRD computes the eigenvalues of a symmetric tridiagonal
* matrix T to suitable accuracy. This is an auxiliary code to be
* called from SSTEMR.
* The user may ask for all eigenvalues, all eigenvalues
* in the half-open interval (VL, VU], or the IL-th through IU-th
* eigenvalues.
*
* To avoid overflow, the matrix must be scaled so that its
* largest element is no greater than overflow**(1/2) *
* underflow**(1/4) in absolute value, and for greatest
* accuracy, it should not be much smaller than that.
*
* See W. Kahan "Accurate Eigenvalues of a Symmetric Tridiagonal
* Matrix", Report CS41, Computer Science Dept., Stanford
* University, July 21, 1966.
*
* Arguments
* =========
*
* RANGE (input) CHARACTER
* = 'A': ("All") all eigenvalues will be found.
* = 'V': ("Value") all eigenvalues in the half-open interval
* (VL, VU] will be found.
* = 'I': ("Index") the IL-th through IU-th eigenvalues (of the
* entire matrix) will be found.
*
* ORDER (input) CHARACTER
* = 'B': ("By Block") the eigenvalues will be grouped by
* split-off block (see IBLOCK, ISPLIT) and
* ordered from smallest to largest within
* the block.
* = 'E': ("Entire matrix")
* the eigenvalues for the entire matrix
* will be ordered from smallest to
* largest.
*
* N (input) INTEGER
* The order of the tridiagonal matrix T. N >= 0.
*
* VL (input) REAL
* VU (input) REAL
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. Eigenvalues less than or equal
* to VL, or greater than VU, will not be returned. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* GERS (input) REAL array, dimension (2*N)
* The N Gerschgorin intervals (the i-th Gerschgorin interval
* is (GERS(2*i-1), GERS(2*i)).
*
* RELTOL (input) REAL
* The minimum relative width of an interval. When an interval
* is narrower than RELTOL times the larger (in
* magnitude) endpoint, then it is considered to be
* sufficiently small, i.e., converged. Note: this should
* always be at least radix*machine epsilon.
*
* D (input) REAL array, dimension (N)
* The n diagonal elements of the tridiagonal matrix T.
*
* E (input) REAL array, dimension (N-1)
* The (n-1) off-diagonal elements of the tridiagonal matrix T.
*
* E2 (input) REAL array, dimension (N-1)
* The (n-1) squared off-diagonal elements of the tridiagonal ma
*
* PIVMIN (input) REAL
* The minimum pivot allowed in the Sturm sequence for T.
*
* NSPLIT (input) INTEGER
* The number of diagonal blocks in the matrix T.
* 1 <= NSPLIT <= N.
*
* ISPLIT (input) INTEGER array, dimension (N)
* The splitting points, at which T breaks up into submatrices.
* The first submatrix consists of rows/columns 1 to ISPLIT(1),
* the second of rows/columns ISPLIT(1)+1 through ISPLIT(2),
* etc., and the NSPLIT-th consists of rows/columns
* ISPLIT(NSPLIT-1)+1 through ISPLIT(NSPLIT)=N.
* (Only the first NSPLIT elements will actually be used, but
* since the user cannot know a priori what value NSPLIT will
* have, N words must be reserved for ISPLIT.)
*
* M (output) INTEGER
* The actual number of eigenvalues found. 0 <= M <= N.
* (See also the description of INFO=2,3.)
*
* W (output) REAL array, dimension (N)
* On exit, the first M elements of W will contain the
* eigenvalue approximations. SLARRD computes an interval
* I_j = (a_j, b_j] that includes eigenvalue j. The eigenvalue
* approximation is given as the interval midpoint
* W(j)= ( a_j + b_j)/2. The corresponding error is bounded by
* WERR(j) = abs( a_j - b_j)/2
*
* WERR (output) REAL array, dimension (N)
* The error bound on the corresponding eigenvalue approximation
* in W.
*
* WL (output) REAL
* WU (output) REAL
* The interval (WL, WU] contains all the wanted eigenvalues.
* If RANGE='V', then WL=VL and WU=VU.
* If RANGE='A', then WL and WU are the global Gerschgorin bound
* on the spectrum.
* If RANGE='I', then WL and WU are computed by SLAEBZ from the
* index range specified.
*
* IBLOCK (output) INTEGER array, dimension (N)
* At each row/column j where E(j) is zero or small, the
* matrix T is considered to split into a block diagonal
* matrix. On exit, if INFO = 0, IBLOCK(i) specifies to which
* block (from 1 to the number of blocks) the eigenvalue W(i)
* belongs. (SLARRD may use the remaining N-M elements as
* workspace.)
*
* INDEXW (output) INTEGER array, dimension (N)
* The indices of the eigenvalues within each block (submatrix);
* for example, INDEXW(i)= j and IBLOCK(i)=k imply that the
* i-th eigenvalue W(i) is the j-th eigenvalue in block k.
*
* WORK (workspace) REAL array, dimension (4*N)
*
* IWORK (workspace) INTEGER array, dimension (3*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: some or all of the eigenvalues failed to converge or
* were not computed:
* =1 or 3: Bisection failed to converge for some
* eigenvalues; these eigenvalues are flagged by a
* negative block number. The effect is that the
* eigenvalues may not be as accurate as the
* absolute and relative tolerances. This is
* generally caused by unexpectedly inaccurate
* arithmetic.
* =2 or 3: RANGE='I' only: Not all of the eigenvalues
* IL:IU were found.
* Effect: M < IU+1-IL
* Cause: non-monotonic arithmetic, causing the
* Sturm sequence to be non-monotonic.
* Cure: recalculate, using RANGE='A', and pick
* out eigenvalues IL:IU. In some cases,
* increasing the PARAMETER "FUDGE" may
* make things work.
* = 4: RANGE='I', and the Gershgorin interval
* initially used was too small. No eigenvalues
* were computed.
* Probable cause: your machine has sloppy
* floating-point arithmetic.
* Cure: Increase the PARAMETER "FUDGE",
* recompile, and try again.
*
* Internal Parameters
* ===================
*
* FUDGE REAL , default = 2
* A "fudge factor" to widen the Gershgorin intervals. Ideally,
* a value of 1 should work, but on machines with sloppy
* arithmetic, this needs to be larger. The default for
* publicly released versions should be large enough to handle
* the worst machine around. Note that this has no effect
* on accuracy of the solution.
*
* Based on contributions by
* W. Kahan, University of California, Berkeley, USA
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param range
* @param order
* @param n
* @param vl
* @param vu
* @param il
* @param iu
* @param gers
* @param _gers_offset
* @param reltol
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param e2
* @param _e2_offset
* @param pivmin
* @param nsplit
* @param isplit
* @param _isplit_offset
* @param m
* @param w
* @param _w_offset
* @param werr
* @param _werr_offset
* @param wl
* @param wu
* @param iblock
* @param _iblock_offset
* @param indexw
* @param _indexw_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void slarrd(java.lang.String range, java.lang.String order, int n, float vl, float vu, int il, int iu, float[] gers, int _gers_offset, float reltol, float[] d, int _d_offset, float[] e, int _e_offset, float[] e2, int _e2_offset, float pivmin, int nsplit, int[] isplit, int _isplit_offset, org.netlib.util.intW m, float[] w, int _w_offset, float[] werr, int _werr_offset, org.netlib.util.floatW wl, org.netlib.util.floatW wu, int[] iblock, int _iblock_offset, int[] indexw, int _indexw_offset, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* To find the desired eigenvalues of a given real symmetric
* tridiagonal matrix T, SLARRE sets any "small" off-diagonal
* elements to zero, and for each unreduced block T_i, it finds
* (a) a suitable shift at one end of the block's spectrum,
* (b) the base representation, T_i - sigma_i I = L_i D_i L_i^T, and
* (c) eigenvalues of each L_i D_i L_i^T.
* The representations and eigenvalues found are then used by
* SSTEMR to compute the eigenvectors of T.
* The accuracy varies depending on whether bisection is used to
* find a few eigenvalues or the dqds algorithm (subroutine SLASQ2) to
* conpute all and then discard any unwanted one.
* As an added benefit, SLARRE also outputs the n
* Gerschgorin intervals for the matrices L_i D_i L_i^T.
*
* Arguments
* =========
*
* RANGE (input) CHARACTER
* = 'A': ("All") all eigenvalues will be found.
* = 'V': ("Value") all eigenvalues in the half-open interval
* (VL, VU] will be found.
* = 'I': ("Index") the IL-th through IU-th eigenvalues (of the
* entire matrix) will be found.
*
* N (input) INTEGER
* The order of the matrix. N > 0.
*
* VL (input/output) REAL
* VU (input/output) REAL
* If RANGE='V', the lower and upper bounds for the eigenvalues.
* Eigenvalues less than or equal to VL, or greater than VU,
* will not be returned. VL < VU.
* If RANGE='I' or ='A', SLARRE computes bounds on the desired
* part of the spectrum.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N.
*
* D (input/output) REAL array, dimension (N)
* On entry, the N diagonal elements of the tridiagonal
* matrix T.
* On exit, the N diagonal elements of the diagonal
* matrices D_i.
*
* E (input/output) REAL array, dimension (N)
* On entry, the first (N-1) entries contain the subdiagonal
* elements of the tridiagonal matrix T; E(N) need not be set.
* On exit, E contains the subdiagonal elements of the unit
* bidiagonal matrices L_i. The entries E( ISPLIT( I ) ),
* 1 <= I <= NSPLIT, contain the base points sigma_i on output.
*
* E2 (input/output) REAL array, dimension (N)
* On entry, the first (N-1) entries contain the SQUARES of the
* subdiagonal elements of the tridiagonal matrix T;
* E2(N) need not be set.
* On exit, the entries E2( ISPLIT( I ) ),
* 1 <= I <= NSPLIT, have been set to zero
*
* RTOL1 (input) REAL
* RTOL2 (input) REAL
* Parameters for bisection.
* An interval [LEFT,RIGHT] has converged if
* RIGHT-LEFT.LT.MAX( RTOL1*GAP, RTOL2*MAX(|LEFT|,|RIGHT|) )
*
* SPLTOL (input) REAL
* The threshold for splitting.
*
* NSPLIT (output) INTEGER
* The number of blocks T splits into. 1 <= NSPLIT <= N.
*
* ISPLIT (output) INTEGER array, dimension (N)
* The splitting points, at which T breaks up into blocks.
* The first block consists of rows/columns 1 to ISPLIT(1),
* the second of rows/columns ISPLIT(1)+1 through ISPLIT(2),
* etc., and the NSPLIT-th consists of rows/columns
* ISPLIT(NSPLIT-1)+1 through ISPLIT(NSPLIT)=N.
*
* M (output) INTEGER
* The total number of eigenvalues (of all L_i D_i L_i^T)
* found.
*
* W (output) REAL array, dimension (N)
* The first M elements contain the eigenvalues. The
* eigenvalues of each of the blocks, L_i D_i L_i^T, are
* sorted in ascending order ( SLARRE may use the
* remaining N-M elements as workspace).
*
* WERR (output) REAL array, dimension (N)
* The error bound on the corresponding eigenvalue in W.
*
* WGAP (output) REAL array, dimension (N)
* The separation from the right neighbor eigenvalue in W.
* The gap is only with respect to the eigenvalues of the same b
* as each block has its own representation tree.
* Exception: at the right end of a block we store the left gap
*
* IBLOCK (output) INTEGER array, dimension (N)
* The indices of the blocks (submatrices) associated with the
* corresponding eigenvalues in W; IBLOCK(i)=1 if eigenvalue
* W(i) belongs to the first block from the top, =2 if W(i)
* belongs to the second block, etc.
*
* INDEXW (output) INTEGER array, dimension (N)
* The indices of the eigenvalues within each block (submatrix);
* for example, INDEXW(i)= 10 and IBLOCK(i)=2 imply that the
* i-th eigenvalue W(i) is the 10-th eigenvalue in block 2
*
* GERS (output) REAL array, dimension (2*N)
* The N Gerschgorin intervals (the i-th Gerschgorin interval
* is (GERS(2*i-1), GERS(2*i)).
*
* PIVMIN (output) DOUBLE PRECISION
* The minimum pivot in the Sturm sequence for T.
*
* WORK (workspace) REAL array, dimension (6*N)
* Workspace.
*
* IWORK (workspace) INTEGER array, dimension (5*N)
* Workspace.
*
* INFO (output) INTEGER
* = 0: successful exit
* > 0: A problem occured in SLARRE.
* < 0: One of the called subroutines signaled an internal prob
* Needs inspection of the corresponding parameter IINFO
* for further information.
*
* =-1: Problem in SLARRD.
* = 2: No base representation could be found in MAXTRY iterati
* Increasing MAXTRY and recompilation might be a remedy.
* =-3: Problem in SLARRB when computing the refined root
* representation for SLASQ2.
* =-4: Problem in SLARRB when preforming bisection on the
* desired part of the spectrum.
* =-5: Problem in SLASQ2.
* =-6: Problem in SLASQ2.
*
* Further Details
* The base representations are required to suffer very little
* element growth and consequently define all their eigenvalues to
* high relative accuracy.
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param range
* @param n
* @param vl
* @param vu
* @param il
* @param iu
* @param d
* @param e
* @param e2
* @param rtol1
* @param rtol2
* @param spltol
* @param nsplit
* @param isplit
* @param m
* @param w
* @param werr
* @param wgap
* @param iblock
* @param indexw
* @param gers
* @param pivmin
* @param work
* @param iwork
* @param info
*
*/
abstract public void slarre(java.lang.String range, int n, org.netlib.util.floatW vl, org.netlib.util.floatW vu, int il, int iu, float[] d, float[] e, float[] e2, float rtol1, float rtol2, float spltol, org.netlib.util.intW nsplit, int[] isplit, org.netlib.util.intW m, float[] w, float[] werr, float[] wgap, int[] iblock, int[] indexw, float[] gers, org.netlib.util.floatW pivmin, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* To find the desired eigenvalues of a given real symmetric
* tridiagonal matrix T, SLARRE sets any "small" off-diagonal
* elements to zero, and for each unreduced block T_i, it finds
* (a) a suitable shift at one end of the block's spectrum,
* (b) the base representation, T_i - sigma_i I = L_i D_i L_i^T, and
* (c) eigenvalues of each L_i D_i L_i^T.
* The representations and eigenvalues found are then used by
* SSTEMR to compute the eigenvectors of T.
* The accuracy varies depending on whether bisection is used to
* find a few eigenvalues or the dqds algorithm (subroutine SLASQ2) to
* conpute all and then discard any unwanted one.
* As an added benefit, SLARRE also outputs the n
* Gerschgorin intervals for the matrices L_i D_i L_i^T.
*
* Arguments
* =========
*
* RANGE (input) CHARACTER
* = 'A': ("All") all eigenvalues will be found.
* = 'V': ("Value") all eigenvalues in the half-open interval
* (VL, VU] will be found.
* = 'I': ("Index") the IL-th through IU-th eigenvalues (of the
* entire matrix) will be found.
*
* N (input) INTEGER
* The order of the matrix. N > 0.
*
* VL (input/output) REAL
* VU (input/output) REAL
* If RANGE='V', the lower and upper bounds for the eigenvalues.
* Eigenvalues less than or equal to VL, or greater than VU,
* will not be returned. VL < VU.
* If RANGE='I' or ='A', SLARRE computes bounds on the desired
* part of the spectrum.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N.
*
* D (input/output) REAL array, dimension (N)
* On entry, the N diagonal elements of the tridiagonal
* matrix T.
* On exit, the N diagonal elements of the diagonal
* matrices D_i.
*
* E (input/output) REAL array, dimension (N)
* On entry, the first (N-1) entries contain the subdiagonal
* elements of the tridiagonal matrix T; E(N) need not be set.
* On exit, E contains the subdiagonal elements of the unit
* bidiagonal matrices L_i. The entries E( ISPLIT( I ) ),
* 1 <= I <= NSPLIT, contain the base points sigma_i on output.
*
* E2 (input/output) REAL array, dimension (N)
* On entry, the first (N-1) entries contain the SQUARES of the
* subdiagonal elements of the tridiagonal matrix T;
* E2(N) need not be set.
* On exit, the entries E2( ISPLIT( I ) ),
* 1 <= I <= NSPLIT, have been set to zero
*
* RTOL1 (input) REAL
* RTOL2 (input) REAL
* Parameters for bisection.
* An interval [LEFT,RIGHT] has converged if
* RIGHT-LEFT.LT.MAX( RTOL1*GAP, RTOL2*MAX(|LEFT|,|RIGHT|) )
*
* SPLTOL (input) REAL
* The threshold for splitting.
*
* NSPLIT (output) INTEGER
* The number of blocks T splits into. 1 <= NSPLIT <= N.
*
* ISPLIT (output) INTEGER array, dimension (N)
* The splitting points, at which T breaks up into blocks.
* The first block consists of rows/columns 1 to ISPLIT(1),
* the second of rows/columns ISPLIT(1)+1 through ISPLIT(2),
* etc., and the NSPLIT-th consists of rows/columns
* ISPLIT(NSPLIT-1)+1 through ISPLIT(NSPLIT)=N.
*
* M (output) INTEGER
* The total number of eigenvalues (of all L_i D_i L_i^T)
* found.
*
* W (output) REAL array, dimension (N)
* The first M elements contain the eigenvalues. The
* eigenvalues of each of the blocks, L_i D_i L_i^T, are
* sorted in ascending order ( SLARRE may use the
* remaining N-M elements as workspace).
*
* WERR (output) REAL array, dimension (N)
* The error bound on the corresponding eigenvalue in W.
*
* WGAP (output) REAL array, dimension (N)
* The separation from the right neighbor eigenvalue in W.
* The gap is only with respect to the eigenvalues of the same b
* as each block has its own representation tree.
* Exception: at the right end of a block we store the left gap
*
* IBLOCK (output) INTEGER array, dimension (N)
* The indices of the blocks (submatrices) associated with the
* corresponding eigenvalues in W; IBLOCK(i)=1 if eigenvalue
* W(i) belongs to the first block from the top, =2 if W(i)
* belongs to the second block, etc.
*
* INDEXW (output) INTEGER array, dimension (N)
* The indices of the eigenvalues within each block (submatrix);
* for example, INDEXW(i)= 10 and IBLOCK(i)=2 imply that the
* i-th eigenvalue W(i) is the 10-th eigenvalue in block 2
*
* GERS (output) REAL array, dimension (2*N)
* The N Gerschgorin intervals (the i-th Gerschgorin interval
* is (GERS(2*i-1), GERS(2*i)).
*
* PIVMIN (output) DOUBLE PRECISION
* The minimum pivot in the Sturm sequence for T.
*
* WORK (workspace) REAL array, dimension (6*N)
* Workspace.
*
* IWORK (workspace) INTEGER array, dimension (5*N)
* Workspace.
*
* INFO (output) INTEGER
* = 0: successful exit
* > 0: A problem occured in SLARRE.
* < 0: One of the called subroutines signaled an internal prob
* Needs inspection of the corresponding parameter IINFO
* for further information.
*
* =-1: Problem in SLARRD.
* = 2: No base representation could be found in MAXTRY iterati
* Increasing MAXTRY and recompilation might be a remedy.
* =-3: Problem in SLARRB when computing the refined root
* representation for SLASQ2.
* =-4: Problem in SLARRB when preforming bisection on the
* desired part of the spectrum.
* =-5: Problem in SLASQ2.
* =-6: Problem in SLASQ2.
*
* Further Details
* The base representations are required to suffer very little
* element growth and consequently define all their eigenvalues to
* high relative accuracy.
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param range
* @param n
* @param vl
* @param vu
* @param il
* @param iu
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param e2
* @param _e2_offset
* @param rtol1
* @param rtol2
* @param spltol
* @param nsplit
* @param isplit
* @param _isplit_offset
* @param m
* @param w
* @param _w_offset
* @param werr
* @param _werr_offset
* @param wgap
* @param _wgap_offset
* @param iblock
* @param _iblock_offset
* @param indexw
* @param _indexw_offset
* @param gers
* @param _gers_offset
* @param pivmin
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void slarre(java.lang.String range, int n, org.netlib.util.floatW vl, org.netlib.util.floatW vu, int il, int iu, float[] d, int _d_offset, float[] e, int _e_offset, float[] e2, int _e2_offset, float rtol1, float rtol2, float spltol, org.netlib.util.intW nsplit, int[] isplit, int _isplit_offset, org.netlib.util.intW m, float[] w, int _w_offset, float[] werr, int _werr_offset, float[] wgap, int _wgap_offset, int[] iblock, int _iblock_offset, int[] indexw, int _indexw_offset, float[] gers, int _gers_offset, org.netlib.util.floatW pivmin, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* Given the initial representation L D L^T and its cluster of close
* eigenvalues (in a relative measure), W( CLSTRT ), W( CLSTRT+1 ), ...
* W( CLEND ), SLARRF finds a new relatively robust representation
* L D L^T - SIGMA I = L(+) D(+) L(+)^T such that at least one of the
* eigenvalues of L(+) D(+) L(+)^T is relatively isolated.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix (subblock, if the matrix splitted).
*
* D (input) REAL array, dimension (N)
* The N diagonal elements of the diagonal matrix D.
*
* L (input) REAL array, dimension (N-1)
* The (N-1) subdiagonal elements of the unit bidiagonal
* matrix L.
*
* LD (input) REAL array, dimension (N-1)
* The (N-1) elements L(i)*D(i).
*
* CLSTRT (input) INTEGER
* The index of the first eigenvalue in the cluster.
*
* CLEND (input) INTEGER
* The index of the last eigenvalue in the cluster.
*
* W (input) REAL array, dimension >= (CLEND-CLSTRT+1
* The eigenvalue APPROXIMATIONS of L D L^T in ascending order.
* W( CLSTRT ) through W( CLEND ) form the cluster of relatively
* close eigenalues.
*
* WGAP (input/output) REAL array, dimension >= (CLEND-C
* The separation from the right neighbor eigenvalue in W.
*
* WERR (input) REAL array, dimension >= (CLEND-CLSTRT+1
* WERR contain the semiwidth of the uncertainty
* interval of the corresponding eigenvalue APPROXIMATION in W
*
* SPDIAM (input) estimate of the spectral diameter obtained from the
* Gerschgorin intervals
*
* CLGAPL, CLGAPR (input) absolute gap on each end of the cluster.
* Set by the calling routine to protect against shifts too clos
* to eigenvalues outside the cluster.
*
* PIVMIN (input) DOUBLE PRECISION
* The minimum pivot allowed in the Sturm sequence.
*
* SIGMA (output) REAL
* The shift used to form L(+) D(+) L(+)^T.
*
* DPLUS (output) REAL array, dimension (N)
* The N diagonal elements of the diagonal matrix D(+).
*
* LPLUS (output) REAL array, dimension (N-1)
* The first (N-1) elements of LPLUS contain the subdiagonal
* elements of the unit bidiagonal matrix L(+).
*
* WORK (workspace) REAL array, dimension (2*N)
* Workspace.
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param l
* @param ld
* @param clstrt
* @param clend
* @param w
* @param wgap
* @param werr
* @param spdiam
* @param clgapl
* @param clgapr
* @param pivmin
* @param sigma
* @param dplus
* @param lplus
* @param work
* @param info
*
*/
abstract public void slarrf(int n, float[] d, float[] l, float[] ld, int clstrt, int clend, float[] w, float[] wgap, float[] werr, float spdiam, float clgapl, float clgapr, float pivmin, org.netlib.util.floatW sigma, float[] dplus, float[] lplus, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* Given the initial representation L D L^T and its cluster of close
* eigenvalues (in a relative measure), W( CLSTRT ), W( CLSTRT+1 ), ...
* W( CLEND ), SLARRF finds a new relatively robust representation
* L D L^T - SIGMA I = L(+) D(+) L(+)^T such that at least one of the
* eigenvalues of L(+) D(+) L(+)^T is relatively isolated.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix (subblock, if the matrix splitted).
*
* D (input) REAL array, dimension (N)
* The N diagonal elements of the diagonal matrix D.
*
* L (input) REAL array, dimension (N-1)
* The (N-1) subdiagonal elements of the unit bidiagonal
* matrix L.
*
* LD (input) REAL array, dimension (N-1)
* The (N-1) elements L(i)*D(i).
*
* CLSTRT (input) INTEGER
* The index of the first eigenvalue in the cluster.
*
* CLEND (input) INTEGER
* The index of the last eigenvalue in the cluster.
*
* W (input) REAL array, dimension >= (CLEND-CLSTRT+1
* The eigenvalue APPROXIMATIONS of L D L^T in ascending order.
* W( CLSTRT ) through W( CLEND ) form the cluster of relatively
* close eigenalues.
*
* WGAP (input/output) REAL array, dimension >= (CLEND-C
* The separation from the right neighbor eigenvalue in W.
*
* WERR (input) REAL array, dimension >= (CLEND-CLSTRT+1
* WERR contain the semiwidth of the uncertainty
* interval of the corresponding eigenvalue APPROXIMATION in W
*
* SPDIAM (input) estimate of the spectral diameter obtained from the
* Gerschgorin intervals
*
* CLGAPL, CLGAPR (input) absolute gap on each end of the cluster.
* Set by the calling routine to protect against shifts too clos
* to eigenvalues outside the cluster.
*
* PIVMIN (input) DOUBLE PRECISION
* The minimum pivot allowed in the Sturm sequence.
*
* SIGMA (output) REAL
* The shift used to form L(+) D(+) L(+)^T.
*
* DPLUS (output) REAL array, dimension (N)
* The N diagonal elements of the diagonal matrix D(+).
*
* LPLUS (output) REAL array, dimension (N-1)
* The first (N-1) elements of LPLUS contain the subdiagonal
* elements of the unit bidiagonal matrix L(+).
*
* WORK (workspace) REAL array, dimension (2*N)
* Workspace.
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param _d_offset
* @param l
* @param _l_offset
* @param ld
* @param _ld_offset
* @param clstrt
* @param clend
* @param w
* @param _w_offset
* @param wgap
* @param _wgap_offset
* @param werr
* @param _werr_offset
* @param spdiam
* @param clgapl
* @param clgapr
* @param pivmin
* @param sigma
* @param dplus
* @param _dplus_offset
* @param lplus
* @param _lplus_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void slarrf(int n, float[] d, int _d_offset, float[] l, int _l_offset, float[] ld, int _ld_offset, int clstrt, int clend, float[] w, int _w_offset, float[] wgap, int _wgap_offset, float[] werr, int _werr_offset, float spdiam, float clgapl, float clgapr, float pivmin, org.netlib.util.floatW sigma, float[] dplus, int _dplus_offset, float[] lplus, int _lplus_offset, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* Given the initial eigenvalue approximations of T, SLARRJ
* does bisection to refine the eigenvalues of T,
* W( IFIRST-OFFSET ) through W( ILAST-OFFSET ), to more accuracy. Initi
* guesses for these eigenvalues are input in W, the corresponding estim
* of the error in these guesses in WERR. During bisection, intervals
* [left, right] are maintained by storing their mid-points and
* semi-widths in the arrays W and WERR respectively.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix.
*
* D (input) REAL array, dimension (N)
* The N diagonal elements of T.
*
* E2 (input) REAL array, dimension (N-1)
* The Squares of the (N-1) subdiagonal elements of T.
*
* IFIRST (input) INTEGER
* The index of the first eigenvalue to be computed.
*
* ILAST (input) INTEGER
* The index of the last eigenvalue to be computed.
*
* RTOL (input) REAL
* Tolerance for the convergence of the bisection intervals.
* An interval [LEFT,RIGHT] has converged if
* RIGHT-LEFT.LT.RTOL*MAX(|LEFT|,|RIGHT|).
*
* OFFSET (input) INTEGER
* Offset for the arrays W and WERR, i.e., the IFIRST-OFFSET
* through ILAST-OFFSET elements of these arrays are to be used.
*
* W (input/output) REAL array, dimension (N)
* On input, W( IFIRST-OFFSET ) through W( ILAST-OFFSET ) are
* estimates of the eigenvalues of L D L^T indexed IFIRST throug
* ILAST.
* On output, these estimates are refined.
*
* WERR (input/output) REAL array, dimension (N)
* On input, WERR( IFIRST-OFFSET ) through WERR( ILAST-OFFSET )
* the errors in the estimates of the corresponding elements in
* On output, these errors are refined.
*
* WORK (workspace) REAL array, dimension (2*N)
* Workspace.
*
* IWORK (workspace) INTEGER array, dimension (2*N)
* Workspace.
*
* PIVMIN (input) DOUBLE PRECISION
* The minimum pivot in the Sturm sequence for T.
*
* SPDIAM (input) DOUBLE PRECISION
* The spectral diameter of T.
*
* INFO (output) INTEGER
* Error flag.
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param e2
* @param ifirst
* @param ilast
* @param rtol
* @param offset
* @param w
* @param werr
* @param work
* @param iwork
* @param pivmin
* @param spdiam
* @param info
*
*/
abstract public void slarrj(int n, float[] d, float[] e2, int ifirst, int ilast, float rtol, int offset, float[] w, float[] werr, float[] work, int[] iwork, float pivmin, float spdiam, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* Given the initial eigenvalue approximations of T, SLARRJ
* does bisection to refine the eigenvalues of T,
* W( IFIRST-OFFSET ) through W( ILAST-OFFSET ), to more accuracy. Initi
* guesses for these eigenvalues are input in W, the corresponding estim
* of the error in these guesses in WERR. During bisection, intervals
* [left, right] are maintained by storing their mid-points and
* semi-widths in the arrays W and WERR respectively.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix.
*
* D (input) REAL array, dimension (N)
* The N diagonal elements of T.
*
* E2 (input) REAL array, dimension (N-1)
* The Squares of the (N-1) subdiagonal elements of T.
*
* IFIRST (input) INTEGER
* The index of the first eigenvalue to be computed.
*
* ILAST (input) INTEGER
* The index of the last eigenvalue to be computed.
*
* RTOL (input) REAL
* Tolerance for the convergence of the bisection intervals.
* An interval [LEFT,RIGHT] has converged if
* RIGHT-LEFT.LT.RTOL*MAX(|LEFT|,|RIGHT|).
*
* OFFSET (input) INTEGER
* Offset for the arrays W and WERR, i.e., the IFIRST-OFFSET
* through ILAST-OFFSET elements of these arrays are to be used.
*
* W (input/output) REAL array, dimension (N)
* On input, W( IFIRST-OFFSET ) through W( ILAST-OFFSET ) are
* estimates of the eigenvalues of L D L^T indexed IFIRST throug
* ILAST.
* On output, these estimates are refined.
*
* WERR (input/output) REAL array, dimension (N)
* On input, WERR( IFIRST-OFFSET ) through WERR( ILAST-OFFSET )
* the errors in the estimates of the corresponding elements in
* On output, these errors are refined.
*
* WORK (workspace) REAL array, dimension (2*N)
* Workspace.
*
* IWORK (workspace) INTEGER array, dimension (2*N)
* Workspace.
*
* PIVMIN (input) DOUBLE PRECISION
* The minimum pivot in the Sturm sequence for T.
*
* SPDIAM (input) DOUBLE PRECISION
* The spectral diameter of T.
*
* INFO (output) INTEGER
* Error flag.
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param _d_offset
* @param e2
* @param _e2_offset
* @param ifirst
* @param ilast
* @param rtol
* @param offset
* @param w
* @param _w_offset
* @param werr
* @param _werr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param pivmin
* @param spdiam
* @param info
*
*/
abstract public void slarrj(int n, float[] d, int _d_offset, float[] e2, int _e2_offset, int ifirst, int ilast, float rtol, int offset, float[] w, int _w_offset, float[] werr, int _werr_offset, float[] work, int _work_offset, int[] iwork, int _iwork_offset, float pivmin, float spdiam, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLARRK computes one eigenvalue of a symmetric tridiagonal
* matrix T to suitable accuracy. This is an auxiliary code to be
* called from SSTEMR.
*
* To avoid overflow, the matrix must be scaled so that its
* largest element is no greater than overflow**(1/2) *
* underflow**(1/4) in absolute value, and for greatest
* accuracy, it should not be much smaller than that.
*
* See W. Kahan "Accurate Eigenvalues of a Symmetric Tridiagonal
* Matrix", Report CS41, Computer Science Dept., Stanford
* University, July 21, 1966.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the tridiagonal matrix T. N >= 0.
*
* IW (input) INTEGER
* The index of the eigenvalues to be returned.
*
* GL (input) REAL
* GU (input) REAL
* An upper and a lower bound on the eigenvalue.
*
* D (input) REAL array, dimension (N)
* The n diagonal elements of the tridiagonal matrix T.
*
* E2 (input) REAL array, dimension (N-1)
* The (n-1) squared off-diagonal elements of the tridiagonal ma
*
* PIVMIN (input) REAL
* The minimum pivot allowed in the Sturm sequence for T.
*
* RELTOL (input) REAL
* The minimum relative width of an interval. When an interval
* is narrower than RELTOL times the larger (in
* magnitude) endpoint, then it is considered to be
* sufficiently small, i.e., converged. Note: this should
* always be at least radix*machine epsilon.
*
* W (output) REAL
*
* WERR (output) REAL
* The error bound on the corresponding eigenvalue approximation
* in W.
*
* INFO (output) INTEGER
* = 0: Eigenvalue converged
* = -1: Eigenvalue did NOT converge
*
* Internal Parameters
* ===================
*
* FUDGE REAL , default = 2
* A "fudge factor" to widen the Gershgorin intervals.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param iw
* @param gl
* @param gu
* @param d
* @param e2
* @param pivmin
* @param reltol
* @param w
* @param werr
* @param info
*
*/
abstract public void slarrk(int n, int iw, float gl, float gu, float[] d, float[] e2, float pivmin, float reltol, org.netlib.util.floatW w, org.netlib.util.floatW werr, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLARRK computes one eigenvalue of a symmetric tridiagonal
* matrix T to suitable accuracy. This is an auxiliary code to be
* called from SSTEMR.
*
* To avoid overflow, the matrix must be scaled so that its
* largest element is no greater than overflow**(1/2) *
* underflow**(1/4) in absolute value, and for greatest
* accuracy, it should not be much smaller than that.
*
* See W. Kahan "Accurate Eigenvalues of a Symmetric Tridiagonal
* Matrix", Report CS41, Computer Science Dept., Stanford
* University, July 21, 1966.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the tridiagonal matrix T. N >= 0.
*
* IW (input) INTEGER
* The index of the eigenvalues to be returned.
*
* GL (input) REAL
* GU (input) REAL
* An upper and a lower bound on the eigenvalue.
*
* D (input) REAL array, dimension (N)
* The n diagonal elements of the tridiagonal matrix T.
*
* E2 (input) REAL array, dimension (N-1)
* The (n-1) squared off-diagonal elements of the tridiagonal ma
*
* PIVMIN (input) REAL
* The minimum pivot allowed in the Sturm sequence for T.
*
* RELTOL (input) REAL
* The minimum relative width of an interval. When an interval
* is narrower than RELTOL times the larger (in
* magnitude) endpoint, then it is considered to be
* sufficiently small, i.e., converged. Note: this should
* always be at least radix*machine epsilon.
*
* W (output) REAL
*
* WERR (output) REAL
* The error bound on the corresponding eigenvalue approximation
* in W.
*
* INFO (output) INTEGER
* = 0: Eigenvalue converged
* = -1: Eigenvalue did NOT converge
*
* Internal Parameters
* ===================
*
* FUDGE REAL , default = 2
* A "fudge factor" to widen the Gershgorin intervals.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param iw
* @param gl
* @param gu
* @param d
* @param _d_offset
* @param e2
* @param _e2_offset
* @param pivmin
* @param reltol
* @param w
* @param werr
* @param info
*
*/
abstract public void slarrk(int n, int iw, float gl, float gu, float[] d, int _d_offset, float[] e2, int _e2_offset, float pivmin, float reltol, org.netlib.util.floatW w, org.netlib.util.floatW werr, org.netlib.util.intW info);
/**
*
* ..
*
*
* Purpose
* =======
*
* Perform tests to decide whether the symmetric tridiagonal matrix T
* warrants expensive computations which guarantee high relative accurac
* in the eigenvalues.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix. N > 0.
*
* D (input) REAL array, dimension (N)
* The N diagonal elements of the tridiagonal matrix T.
*
* E (input/output) REAL array, dimension (N)
* On entry, the first (N-1) entries contain the subdiagonal
* elements of the tridiagonal matrix T; E(N) is set to ZERO.
*
* INFO (output) INTEGER
* INFO = 0(default) : the matrix warrants computations preservi
* relative accuracy.
* INFO = 1 : the matrix warrants computations guarante
* only absolute accuracy.
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param e
* @param info
*
*/
abstract public void slarrr(int n, float[] d, float[] e, org.netlib.util.intW info);
/**
*
* ..
*
*
* Purpose
* =======
*
* Perform tests to decide whether the symmetric tridiagonal matrix T
* warrants expensive computations which guarantee high relative accurac
* in the eigenvalues.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix. N > 0.
*
* D (input) REAL array, dimension (N)
* The N diagonal elements of the tridiagonal matrix T.
*
* E (input/output) REAL array, dimension (N)
* On entry, the first (N-1) entries contain the subdiagonal
* elements of the tridiagonal matrix T; E(N) is set to ZERO.
*
* INFO (output) INTEGER
* INFO = 0(default) : the matrix warrants computations preservi
* relative accuracy.
* INFO = 1 : the matrix warrants computations guarante
* only absolute accuracy.
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param info
*
*/
abstract public void slarrr(int n, float[] d, int _d_offset, float[] e, int _e_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLARRV computes the eigenvectors of the tridiagonal matrix
* T = L D L^T given L, D and APPROXIMATIONS to the eigenvalues of L D L
* The input eigenvalues should have been computed by SLARRE.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* VL (input) REAL
* VU (input) REAL
* Lower and upper bounds of the interval that contains the desi
* eigenvalues. VL < VU. Needed to compute gaps on the left or r
* end of the extremal eigenvalues in the desired RANGE.
*
* D (input/output) REAL array, dimension (N)
* On entry, the N diagonal elements of the diagonal matrix D.
* On exit, D may be overwritten.
*
* L (input/output) REAL array, dimension (N)
* On entry, the (N-1) subdiagonal elements of the unit
* bidiagonal matrix L are in elements 1 to N-1 of L
* (if the matrix is not splitted.) At the end of each block
* is stored the corresponding shift as given by SLARRE.
* On exit, L is overwritten.
*
* PIVMIN (in) DOUBLE PRECISION
* The minimum pivot allowed in the Sturm sequence.
*
* ISPLIT (input) INTEGER array, dimension (N)
* The splitting points, at which T breaks up into blocks.
* The first block consists of rows/columns 1 to
* ISPLIT( 1 ), the second of rows/columns ISPLIT( 1 )+1
* through ISPLIT( 2 ), etc.
*
* M (input) INTEGER
* The total number of input eigenvalues. 0 <= M <= N.
*
* DOL (input) INTEGER
* DOU (input) INTEGER
* If the user wants to compute only selected eigenvectors from
* the eigenvalues supplied, he can specify an index range DOL:D
* Or else the setting DOL=1, DOU=M should be applied.
* Note that DOL and DOU refer to the order in which the eigenva
* are stored in W.
* If the user wants to compute only selected eigenpairs, then
* the columns DOL-1 to DOU+1 of the eigenvector space Z contain
* computed eigenvectors. All other columns of Z are set to zero
*
* MINRGP (input) REAL
*
* RTOL1 (input) REAL
* RTOL2 (input) REAL
* Parameters for bisection.
* An interval [LEFT,RIGHT] has converged if
* RIGHT-LEFT.LT.MAX( RTOL1*GAP, RTOL2*MAX(|LEFT|,|RIGHT|) )
*
* W (input/output) REAL array, dimension (N)
* The first M elements of W contain the APPROXIMATE eigenvalues
* which eigenvectors are to be computed. The eigenvalues
* should be grouped by split-off block and ordered from
* smallest to largest within the block ( The output array
* W from SLARRE is expected here ). Furthermore, they are with
* respect to the shift of the corresponding root representation
* for their block. On exit, W holds the eigenvalues of the
* UNshifted matrix.
*
* WERR (input/output) REAL array, dimension (N)
* The first M elements contain the semiwidth of the uncertainty
* interval of the corresponding eigenvalue in W
*
* WGAP (input/output) REAL array, dimension (N)
* The separation from the right neighbor eigenvalue in W.
*
* IBLOCK (input) INTEGER array, dimension (N)
* The indices of the blocks (submatrices) associated with the
* corresponding eigenvalues in W; IBLOCK(i)=1 if eigenvalue
* W(i) belongs to the first block from the top, =2 if W(i)
* belongs to the second block, etc.
*
* INDEXW (input) INTEGER array, dimension (N)
* The indices of the eigenvalues within each block (submatrix);
* for example, INDEXW(i)= 10 and IBLOCK(i)=2 imply that the
* i-th eigenvalue W(i) is the 10-th eigenvalue in the second bl
*
* GERS (input) REAL array, dimension (2*N)
* The N Gerschgorin intervals (the i-th Gerschgorin interval
* is (GERS(2*i-1), GERS(2*i)). The Gerschgorin intervals should
* be computed from the original UNshifted matrix.
*
* Z (output) REAL array, dimension (LDZ, max(1,M) )
* If INFO = 0, the first M columns of Z contain the
* orthonormal eigenvectors of the matrix T
* corresponding to the input eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* ISUPPZ (output) INTEGER array, dimension ( 2*max(1,M) )
* The support of the eigenvectors in Z, i.e., the indices
* indicating the nonzero elements in Z. The I-th eigenvector
* is nonzero only in elements ISUPPZ( 2*I-1 ) through
* ISUPPZ( 2*I ).
*
* WORK (workspace) REAL array, dimension (12*N)
*
* IWORK (workspace) INTEGER array, dimension (7*N)
*
* INFO (output) INTEGER
* = 0: successful exit
*
* > 0: A problem occured in SLARRV.
* < 0: One of the called subroutines signaled an internal prob
* Needs inspection of the corresponding parameter IINFO
* for further information.
*
* =-1: Problem in SLARRB when refining a child's eigenvalues.
* =-2: Problem in SLARRF when computing the RRR of a child.
* When a child is inside a tight cluster, it can be diffi
* to find an RRR. A partial remedy from the user's point
* view is to make the parameter MINRGP smaller and recomp
* However, as the orthogonality of the computed vectors i
* proportional to 1/MINRGP, the user should be aware that
* he might be trading in precision when he decreases MINR
* =-3: Problem in SLARRB when refining a single eigenvalue
* after the Rayleigh correction was rejected.
* = 5: The Rayleigh Quotient Iteration failed to converge to
* full accuracy in MAXITR steps.
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param vl
* @param vu
* @param d
* @param l
* @param pivmin
* @param isplit
* @param m
* @param dol
* @param dou
* @param minrgp
* @param rtol1
* @param rtol2
* @param w
* @param werr
* @param wgap
* @param iblock
* @param indexw
* @param gers
* @param z
* @param ldz
* @param isuppz
* @param work
* @param iwork
* @param info
*
*/
abstract public void slarrv(int n, float vl, float vu, float[] d, float[] l, float pivmin, int[] isplit, int m, int dol, int dou, float minrgp, org.netlib.util.floatW rtol1, org.netlib.util.floatW rtol2, float[] w, float[] werr, float[] wgap, int[] iblock, int[] indexw, float[] gers, float[] z, int ldz, int[] isuppz, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLARRV computes the eigenvectors of the tridiagonal matrix
* T = L D L^T given L, D and APPROXIMATIONS to the eigenvalues of L D L
* The input eigenvalues should have been computed by SLARRE.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* VL (input) REAL
* VU (input) REAL
* Lower and upper bounds of the interval that contains the desi
* eigenvalues. VL < VU. Needed to compute gaps on the left or r
* end of the extremal eigenvalues in the desired RANGE.
*
* D (input/output) REAL array, dimension (N)
* On entry, the N diagonal elements of the diagonal matrix D.
* On exit, D may be overwritten.
*
* L (input/output) REAL array, dimension (N)
* On entry, the (N-1) subdiagonal elements of the unit
* bidiagonal matrix L are in elements 1 to N-1 of L
* (if the matrix is not splitted.) At the end of each block
* is stored the corresponding shift as given by SLARRE.
* On exit, L is overwritten.
*
* PIVMIN (in) DOUBLE PRECISION
* The minimum pivot allowed in the Sturm sequence.
*
* ISPLIT (input) INTEGER array, dimension (N)
* The splitting points, at which T breaks up into blocks.
* The first block consists of rows/columns 1 to
* ISPLIT( 1 ), the second of rows/columns ISPLIT( 1 )+1
* through ISPLIT( 2 ), etc.
*
* M (input) INTEGER
* The total number of input eigenvalues. 0 <= M <= N.
*
* DOL (input) INTEGER
* DOU (input) INTEGER
* If the user wants to compute only selected eigenvectors from
* the eigenvalues supplied, he can specify an index range DOL:D
* Or else the setting DOL=1, DOU=M should be applied.
* Note that DOL and DOU refer to the order in which the eigenva
* are stored in W.
* If the user wants to compute only selected eigenpairs, then
* the columns DOL-1 to DOU+1 of the eigenvector space Z contain
* computed eigenvectors. All other columns of Z are set to zero
*
* MINRGP (input) REAL
*
* RTOL1 (input) REAL
* RTOL2 (input) REAL
* Parameters for bisection.
* An interval [LEFT,RIGHT] has converged if
* RIGHT-LEFT.LT.MAX( RTOL1*GAP, RTOL2*MAX(|LEFT|,|RIGHT|) )
*
* W (input/output) REAL array, dimension (N)
* The first M elements of W contain the APPROXIMATE eigenvalues
* which eigenvectors are to be computed. The eigenvalues
* should be grouped by split-off block and ordered from
* smallest to largest within the block ( The output array
* W from SLARRE is expected here ). Furthermore, they are with
* respect to the shift of the corresponding root representation
* for their block. On exit, W holds the eigenvalues of the
* UNshifted matrix.
*
* WERR (input/output) REAL array, dimension (N)
* The first M elements contain the semiwidth of the uncertainty
* interval of the corresponding eigenvalue in W
*
* WGAP (input/output) REAL array, dimension (N)
* The separation from the right neighbor eigenvalue in W.
*
* IBLOCK (input) INTEGER array, dimension (N)
* The indices of the blocks (submatrices) associated with the
* corresponding eigenvalues in W; IBLOCK(i)=1 if eigenvalue
* W(i) belongs to the first block from the top, =2 if W(i)
* belongs to the second block, etc.
*
* INDEXW (input) INTEGER array, dimension (N)
* The indices of the eigenvalues within each block (submatrix);
* for example, INDEXW(i)= 10 and IBLOCK(i)=2 imply that the
* i-th eigenvalue W(i) is the 10-th eigenvalue in the second bl
*
* GERS (input) REAL array, dimension (2*N)
* The N Gerschgorin intervals (the i-th Gerschgorin interval
* is (GERS(2*i-1), GERS(2*i)). The Gerschgorin intervals should
* be computed from the original UNshifted matrix.
*
* Z (output) REAL array, dimension (LDZ, max(1,M) )
* If INFO = 0, the first M columns of Z contain the
* orthonormal eigenvectors of the matrix T
* corresponding to the input eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* ISUPPZ (output) INTEGER array, dimension ( 2*max(1,M) )
* The support of the eigenvectors in Z, i.e., the indices
* indicating the nonzero elements in Z. The I-th eigenvector
* is nonzero only in elements ISUPPZ( 2*I-1 ) through
* ISUPPZ( 2*I ).
*
* WORK (workspace) REAL array, dimension (12*N)
*
* IWORK (workspace) INTEGER array, dimension (7*N)
*
* INFO (output) INTEGER
* = 0: successful exit
*
* > 0: A problem occured in SLARRV.
* < 0: One of the called subroutines signaled an internal prob
* Needs inspection of the corresponding parameter IINFO
* for further information.
*
* =-1: Problem in SLARRB when refining a child's eigenvalues.
* =-2: Problem in SLARRF when computing the RRR of a child.
* When a child is inside a tight cluster, it can be diffi
* to find an RRR. A partial remedy from the user's point
* view is to make the parameter MINRGP smaller and recomp
* However, as the orthogonality of the computed vectors i
* proportional to 1/MINRGP, the user should be aware that
* he might be trading in precision when he decreases MINR
* =-3: Problem in SLARRB when refining a single eigenvalue
* after the Rayleigh correction was rejected.
* = 5: The Rayleigh Quotient Iteration failed to converge to
* full accuracy in MAXITR steps.
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param vl
* @param vu
* @param d
* @param _d_offset
* @param l
* @param _l_offset
* @param pivmin
* @param isplit
* @param _isplit_offset
* @param m
* @param dol
* @param dou
* @param minrgp
* @param rtol1
* @param rtol2
* @param w
* @param _w_offset
* @param werr
* @param _werr_offset
* @param wgap
* @param _wgap_offset
* @param iblock
* @param _iblock_offset
* @param indexw
* @param _indexw_offset
* @param gers
* @param _gers_offset
* @param z
* @param _z_offset
* @param ldz
* @param isuppz
* @param _isuppz_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void slarrv(int n, float vl, float vu, float[] d, int _d_offset, float[] l, int _l_offset, float pivmin, int[] isplit, int _isplit_offset, int m, int dol, int dou, float minrgp, org.netlib.util.floatW rtol1, org.netlib.util.floatW rtol2, float[] w, int _w_offset, float[] werr, int _werr_offset, float[] wgap, int _wgap_offset, int[] iblock, int _iblock_offset, int[] indexw, int _indexw_offset, float[] gers, int _gers_offset, float[] z, int _z_offset, int ldz, int[] isuppz, int _isuppz_offset, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLARTG generate a plane rotation so that
*
* [ CS SN ] . [ F ] = [ R ] where CS**2 + SN**2 = 1.
* [ -SN CS ] [ G ] [ 0 ]
*
* This is a slower, more accurate version of the BLAS1 routine SROTG,
* with the following other differences:
* F and G are unchanged on return.
* If G=0, then CS=1 and SN=0.
* If F=0 and (G .ne. 0), then CS=0 and SN=1 without doing any
* floating point operations (saves work in SBDSQR when
* there are zeros on the diagonal).
*
* If F exceeds G in magnitude, CS will be positive.
*
* Arguments
* =========
*
* F (input) REAL
* The first component of vector to be rotated.
*
* G (input) REAL
* The second component of vector to be rotated.
*
* CS (output) REAL
* The cosine of the rotation.
*
* SN (output) REAL
* The sine of the rotation.
*
* R (output) REAL
* The nonzero component of the rotated vector.
*
* This version has a few statements commented out for thread safety
* (machine parameters are computed on each entry). 10 feb 03, SJH.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param f
* @param g
* @param cs
* @param sn
* @param r
*
*/
abstract public void slartg(float f, float g, org.netlib.util.floatW cs, org.netlib.util.floatW sn, org.netlib.util.floatW r);
/**
*
* ..
*
* Purpose
* =======
*
* SLARTV applies a vector of real plane rotations to elements of the
* real vectors x and y. For i = 1,2,...,n
*
* ( x(i) ) := ( c(i) s(i) ) ( x(i) )
* ( y(i) ) ( -s(i) c(i) ) ( y(i) )
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of plane rotations to be applied.
*
* X (input/output) REAL array,
* dimension (1+(N-1)*INCX)
* The vector x.
*
* INCX (input) INTEGER
* The increment between elements of X. INCX > 0.
*
* Y (input/output) REAL array,
* dimension (1+(N-1)*INCY)
* The vector y.
*
* INCY (input) INTEGER
* The increment between elements of Y. INCY > 0.
*
* C (input) REAL array, dimension (1+(N-1)*INCC)
* The cosines of the plane rotations.
*
* S (input) REAL array, dimension (1+(N-1)*INCC)
* The sines of the plane rotations.
*
* INCC (input) INTEGER
* The increment between elements of C and S. INCC > 0.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n
* @param x
* @param incx
* @param y
* @param incy
* @param c
* @param s
* @param incc
*
*/
abstract public void slartv(int n, float[] x, int incx, float[] y, int incy, float[] c, float[] s, int incc);
/**
*
* ..
*
* Purpose
* =======
*
* SLARTV applies a vector of real plane rotations to elements of the
* real vectors x and y. For i = 1,2,...,n
*
* ( x(i) ) := ( c(i) s(i) ) ( x(i) )
* ( y(i) ) ( -s(i) c(i) ) ( y(i) )
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of plane rotations to be applied.
*
* X (input/output) REAL array,
* dimension (1+(N-1)*INCX)
* The vector x.
*
* INCX (input) INTEGER
* The increment between elements of X. INCX > 0.
*
* Y (input/output) REAL array,
* dimension (1+(N-1)*INCY)
* The vector y.
*
* INCY (input) INTEGER
* The increment between elements of Y. INCY > 0.
*
* C (input) REAL array, dimension (1+(N-1)*INCC)
* The cosines of the plane rotations.
*
* S (input) REAL array, dimension (1+(N-1)*INCC)
* The sines of the plane rotations.
*
* INCC (input) INTEGER
* The increment between elements of C and S. INCC > 0.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n
* @param x
* @param _x_offset
* @param incx
* @param y
* @param _y_offset
* @param incy
* @param c
* @param _c_offset
* @param s
* @param _s_offset
* @param incc
*
*/
abstract public void slartv(int n, float[] x, int _x_offset, int incx, float[] y, int _y_offset, int incy, float[] c, int _c_offset, float[] s, int _s_offset, int incc);
/**
*
* ..
*
* Purpose
* =======
*
* SLARUV returns a vector of n random real numbers from a uniform (0,1)
* distribution (n <= 128).
*
* This is an auxiliary routine called by SLARNV and CLARNV.
*
* Arguments
* =========
*
* ISEED (input/output) INTEGER array, dimension (4)
* On entry, the seed of the random number generator; the array
* elements must be between 0 and 4095, and ISEED(4) must be
* odd.
* On exit, the seed is updated.
*
* N (input) INTEGER
* The number of random numbers to be generated. N <= 128.
*
* X (output) REAL array, dimension (N)
* The generated random numbers.
*
* Further Details
* ===============
*
* This routine uses a multiplicative congruential method with modulus
* 2**48 and multiplier 33952834046453 (see G.S.Fishman,
* 'Multiplicative congruential random number generators with modulus
* 2**b: an exhaustive analysis for b = 32 and a partial analysis for
* b = 48', Math. Comp. 189, pp 331-344, 1990).
*
* 48-bit integers are stored in 4 integer array elements with 12 bits
* per element. Hence the routine is portable across machines with
* integers of 32 bits or more.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param iseed
* @param n
* @param x
*
*/
abstract public void slaruv(int[] iseed, int n, float[] x);
/**
*
* ..
*
* Purpose
* =======
*
* SLARUV returns a vector of n random real numbers from a uniform (0,1)
* distribution (n <= 128).
*
* This is an auxiliary routine called by SLARNV and CLARNV.
*
* Arguments
* =========
*
* ISEED (input/output) INTEGER array, dimension (4)
* On entry, the seed of the random number generator; the array
* elements must be between 0 and 4095, and ISEED(4) must be
* odd.
* On exit, the seed is updated.
*
* N (input) INTEGER
* The number of random numbers to be generated. N <= 128.
*
* X (output) REAL array, dimension (N)
* The generated random numbers.
*
* Further Details
* ===============
*
* This routine uses a multiplicative congruential method with modulus
* 2**48 and multiplier 33952834046453 (see G.S.Fishman,
* 'Multiplicative congruential random number generators with modulus
* 2**b: an exhaustive analysis for b = 32 and a partial analysis for
* b = 48', Math. Comp. 189, pp 331-344, 1990).
*
* 48-bit integers are stored in 4 integer array elements with 12 bits
* per element. Hence the routine is portable across machines with
* integers of 32 bits or more.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param iseed
* @param _iseed_offset
* @param n
* @param x
* @param _x_offset
*
*/
abstract public void slaruv(int[] iseed, int _iseed_offset, int n, float[] x, int _x_offset);
/**
*
* ..
*
* Purpose
* =======
*
* SLARZ applies a real elementary reflector H to a real M-by-N
* matrix C, from either the left or the right. H is represented in the
* form
*
* H = I - tau * v * v'
*
* where tau is a real scalar and v is a real vector.
*
* If tau = 0, then H is taken to be the unit matrix.
*
*
* H is a product of k elementary reflectors as returned by STZRZF.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': form H * C
* = 'R': form C * H
*
* M (input) INTEGER
* The number of rows of the matrix C.
*
* N (input) INTEGER
* The number of columns of the matrix C.
*
* L (input) INTEGER
* The number of entries of the vector V containing
* the meaningful part of the Householder vectors.
* If SIDE = 'L', M >= L >= 0, if SIDE = 'R', N >= L >= 0.
*
* V (input) REAL array, dimension (1+(L-1)*abs(INCV))
* The vector v in the representation of H as returned by
* STZRZF. V is not used if TAU = 0.
*
* INCV (input) INTEGER
* The increment between elements of v. INCV <> 0.
*
* TAU (input) REAL
* The value tau in the representation of H.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by the matrix H * C if SIDE = 'L',
* or C * H if SIDE = 'R'.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) REAL array, dimension
* (N) if SIDE = 'L'
* or (M) if SIDE = 'R'
*
* Further Details
* ===============
*
* Based on contributions by
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param m
* @param n
* @param l
* @param v
* @param incv
* @param tau
* @param c
* @param Ldc
* @param work
*
*/
abstract public void slarz(java.lang.String side, int m, int n, int l, float[] v, int incv, float tau, float[] c, int Ldc, float[] work);
/**
*
* ..
*
* Purpose
* =======
*
* SLARZ applies a real elementary reflector H to a real M-by-N
* matrix C, from either the left or the right. H is represented in the
* form
*
* H = I - tau * v * v'
*
* where tau is a real scalar and v is a real vector.
*
* If tau = 0, then H is taken to be the unit matrix.
*
*
* H is a product of k elementary reflectors as returned by STZRZF.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': form H * C
* = 'R': form C * H
*
* M (input) INTEGER
* The number of rows of the matrix C.
*
* N (input) INTEGER
* The number of columns of the matrix C.
*
* L (input) INTEGER
* The number of entries of the vector V containing
* the meaningful part of the Householder vectors.
* If SIDE = 'L', M >= L >= 0, if SIDE = 'R', N >= L >= 0.
*
* V (input) REAL array, dimension (1+(L-1)*abs(INCV))
* The vector v in the representation of H as returned by
* STZRZF. V is not used if TAU = 0.
*
* INCV (input) INTEGER
* The increment between elements of v. INCV <> 0.
*
* TAU (input) REAL
* The value tau in the representation of H.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by the matrix H * C if SIDE = 'L',
* or C * H if SIDE = 'R'.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) REAL array, dimension
* (N) if SIDE = 'L'
* or (M) if SIDE = 'R'
*
* Further Details
* ===============
*
* Based on contributions by
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param m
* @param n
* @param l
* @param v
* @param _v_offset
* @param incv
* @param tau
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
*
*/
abstract public void slarz(java.lang.String side, int m, int n, int l, float[] v, int _v_offset, int incv, float tau, float[] c, int _c_offset, int Ldc, float[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* SLARZB applies a real block reflector H or its transpose H**T to
* a real distributed M-by-N C from the left or the right.
*
* Currently, only STOREV = 'R' and DIRECT = 'B' are supported.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply H or H' from the Left
* = 'R': apply H or H' from the Right
*
* TRANS (input) CHARACTER*1
* = 'N': apply H (No transpose)
* = 'C': apply H' (Transpose)
*
* DIRECT (input) CHARACTER*1
* Indicates how H is formed from a product of elementary
* reflectors
* = 'F': H = H(1) H(2) . . . H(k) (Forward, not supported yet)
* = 'B': H = H(k) . . . H(2) H(1) (Backward)
*
* STOREV (input) CHARACTER*1
* Indicates how the vectors which define the elementary
* reflectors are stored:
* = 'C': Columnwise (not supported yet)
* = 'R': Rowwise
*
* M (input) INTEGER
* The number of rows of the matrix C.
*
* N (input) INTEGER
* The number of columns of the matrix C.
*
* K (input) INTEGER
* The order of the matrix T (= the number of elementary
* reflectors whose product defines the block reflector).
*
* L (input) INTEGER
* The number of columns of the matrix V containing the
* meaningful part of the Householder reflectors.
* If SIDE = 'L', M >= L >= 0, if SIDE = 'R', N >= L >= 0.
*
* V (input) REAL array, dimension (LDV,NV).
* If STOREV = 'C', NV = K; if STOREV = 'R', NV = L.
*
* LDV (input) INTEGER
* The leading dimension of the array V.
* If STOREV = 'C', LDV >= L; if STOREV = 'R', LDV >= K.
*
* T (input) REAL array, dimension (LDT,K)
* The triangular K-by-K matrix T in the representation of the
* block reflector.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= K.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by H*C or H'*C or C*H or C*H'.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) REAL array, dimension (LDWORK,K)
*
* LDWORK (input) INTEGER
* The leading dimension of the array WORK.
* If SIDE = 'L', LDWORK >= max(1,N);
* if SIDE = 'R', LDWORK >= max(1,M).
*
* Further Details
* ===============
*
* Based on contributions by
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param direct
* @param storev
* @param m
* @param n
* @param k
* @param l
* @param v
* @param ldv
* @param t
* @param ldt
* @param c
* @param Ldc
* @param work
* @param ldwork
*
*/
abstract public void slarzb(java.lang.String side, java.lang.String trans, java.lang.String direct, java.lang.String storev, int m, int n, int k, int l, float[] v, int ldv, float[] t, int ldt, float[] c, int Ldc, float[] work, int ldwork);
/**
*
* ..
*
* Purpose
* =======
*
* SLARZB applies a real block reflector H or its transpose H**T to
* a real distributed M-by-N C from the left or the right.
*
* Currently, only STOREV = 'R' and DIRECT = 'B' are supported.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply H or H' from the Left
* = 'R': apply H or H' from the Right
*
* TRANS (input) CHARACTER*1
* = 'N': apply H (No transpose)
* = 'C': apply H' (Transpose)
*
* DIRECT (input) CHARACTER*1
* Indicates how H is formed from a product of elementary
* reflectors
* = 'F': H = H(1) H(2) . . . H(k) (Forward, not supported yet)
* = 'B': H = H(k) . . . H(2) H(1) (Backward)
*
* STOREV (input) CHARACTER*1
* Indicates how the vectors which define the elementary
* reflectors are stored:
* = 'C': Columnwise (not supported yet)
* = 'R': Rowwise
*
* M (input) INTEGER
* The number of rows of the matrix C.
*
* N (input) INTEGER
* The number of columns of the matrix C.
*
* K (input) INTEGER
* The order of the matrix T (= the number of elementary
* reflectors whose product defines the block reflector).
*
* L (input) INTEGER
* The number of columns of the matrix V containing the
* meaningful part of the Householder reflectors.
* If SIDE = 'L', M >= L >= 0, if SIDE = 'R', N >= L >= 0.
*
* V (input) REAL array, dimension (LDV,NV).
* If STOREV = 'C', NV = K; if STOREV = 'R', NV = L.
*
* LDV (input) INTEGER
* The leading dimension of the array V.
* If STOREV = 'C', LDV >= L; if STOREV = 'R', LDV >= K.
*
* T (input) REAL array, dimension (LDT,K)
* The triangular K-by-K matrix T in the representation of the
* block reflector.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= K.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by H*C or H'*C or C*H or C*H'.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) REAL array, dimension (LDWORK,K)
*
* LDWORK (input) INTEGER
* The leading dimension of the array WORK.
* If SIDE = 'L', LDWORK >= max(1,N);
* if SIDE = 'R', LDWORK >= max(1,M).
*
* Further Details
* ===============
*
* Based on contributions by
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param direct
* @param storev
* @param m
* @param n
* @param k
* @param l
* @param v
* @param _v_offset
* @param ldv
* @param t
* @param _t_offset
* @param ldt
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param ldwork
*
*/
abstract public void slarzb(java.lang.String side, java.lang.String trans, java.lang.String direct, java.lang.String storev, int m, int n, int k, int l, float[] v, int _v_offset, int ldv, float[] t, int _t_offset, int ldt, float[] c, int _c_offset, int Ldc, float[] work, int _work_offset, int ldwork);
/**
*
* ..
*
* Purpose
* =======
*
* SLARZT forms the triangular factor T of a real block reflector
* H of order > n, which is defined as a product of k elementary
* reflectors.
*
* If DIRECT = 'F', H = H(1) H(2) . . . H(k) and T is upper triangular;
*
* If DIRECT = 'B', H = H(k) . . . H(2) H(1) and T is lower triangular.
*
* If STOREV = 'C', the vector which defines the elementary reflector
* H(i) is stored in the i-th column of the array V, and
*
* H = I - V * T * V'
*
* If STOREV = 'R', the vector which defines the elementary reflector
* H(i) is stored in the i-th row of the array V, and
*
* H = I - V' * T * V
*
* Currently, only STOREV = 'R' and DIRECT = 'B' are supported.
*
* Arguments
* =========
*
* DIRECT (input) CHARACTER*1
* Specifies the order in which the elementary reflectors are
* multiplied to form the block reflector:
* = 'F': H = H(1) H(2) . . . H(k) (Forward, not supported yet)
* = 'B': H = H(k) . . . H(2) H(1) (Backward)
*
* STOREV (input) CHARACTER*1
* Specifies how the vectors which define the elementary
* reflectors are stored (see also Further Details):
* = 'C': columnwise (not supported yet)
* = 'R': rowwise
*
* N (input) INTEGER
* The order of the block reflector H. N >= 0.
*
* K (input) INTEGER
* The order of the triangular factor T (= the number of
* elementary reflectors). K >= 1.
*
* V (input/output) REAL array, dimension
* (LDV,K) if STOREV = 'C'
* (LDV,N) if STOREV = 'R'
* The matrix V. See further details.
*
* LDV (input) INTEGER
* The leading dimension of the array V.
* If STOREV = 'C', LDV >= max(1,N); if STOREV = 'R', LDV >= K.
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i).
*
* T (output) REAL array, dimension (LDT,K)
* The k by k triangular factor T of the block reflector.
* If DIRECT = 'F', T is upper triangular; if DIRECT = 'B', T is
* lower triangular. The rest of the array is not used.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= K.
*
* Further Details
* ===============
*
* Based on contributions by
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
*
* The shape of the matrix V and the storage of the vectors which define
* the H(i) is best illustrated by the following example with n = 5 and
* k = 3. The elements equal to 1 are not stored; the corresponding
* array elements are modified but restored on exit. The rest of the
* array is not used.
*
* DIRECT = 'F' and STOREV = 'C': DIRECT = 'F' and STOREV = 'R':
*
* ______V_____
* ( v1 v2 v3 ) / \
* ( v1 v2 v3 ) ( v1 v1 v1 v1 v1 . . . . 1 )
* V = ( v1 v2 v3 ) ( v2 v2 v2 v2 v2 . . . 1 )
* ( v1 v2 v3 ) ( v3 v3 v3 v3 v3 . . 1 )
* ( v1 v2 v3 )
* . . .
* . . .
* 1 . .
* 1 .
* 1
*
* DIRECT = 'B' and STOREV = 'C': DIRECT = 'B' and STOREV = 'R':
*
* ______V_____
* 1 / \
* . 1 ( 1 . . . . v1 v1 v1 v1 v1 )
* . . 1 ( . 1 . . . v2 v2 v2 v2 v2 )
* . . . ( . . 1 . . v3 v3 v3 v3 v3 )
* . . .
* ( v1 v2 v3 )
* ( v1 v2 v3 )
* V = ( v1 v2 v3 )
* ( v1 v2 v3 )
* ( v1 v2 v3 )
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param direct
* @param storev
* @param n
* @param k
* @param v
* @param ldv
* @param tau
* @param t
* @param ldt
*
*/
abstract public void slarzt(java.lang.String direct, java.lang.String storev, int n, int k, float[] v, int ldv, float[] tau, float[] t, int ldt);
/**
*
* ..
*
* Purpose
* =======
*
* SLARZT forms the triangular factor T of a real block reflector
* H of order > n, which is defined as a product of k elementary
* reflectors.
*
* If DIRECT = 'F', H = H(1) H(2) . . . H(k) and T is upper triangular;
*
* If DIRECT = 'B', H = H(k) . . . H(2) H(1) and T is lower triangular.
*
* If STOREV = 'C', the vector which defines the elementary reflector
* H(i) is stored in the i-th column of the array V, and
*
* H = I - V * T * V'
*
* If STOREV = 'R', the vector which defines the elementary reflector
* H(i) is stored in the i-th row of the array V, and
*
* H = I - V' * T * V
*
* Currently, only STOREV = 'R' and DIRECT = 'B' are supported.
*
* Arguments
* =========
*
* DIRECT (input) CHARACTER*1
* Specifies the order in which the elementary reflectors are
* multiplied to form the block reflector:
* = 'F': H = H(1) H(2) . . . H(k) (Forward, not supported yet)
* = 'B': H = H(k) . . . H(2) H(1) (Backward)
*
* STOREV (input) CHARACTER*1
* Specifies how the vectors which define the elementary
* reflectors are stored (see also Further Details):
* = 'C': columnwise (not supported yet)
* = 'R': rowwise
*
* N (input) INTEGER
* The order of the block reflector H. N >= 0.
*
* K (input) INTEGER
* The order of the triangular factor T (= the number of
* elementary reflectors). K >= 1.
*
* V (input/output) REAL array, dimension
* (LDV,K) if STOREV = 'C'
* (LDV,N) if STOREV = 'R'
* The matrix V. See further details.
*
* LDV (input) INTEGER
* The leading dimension of the array V.
* If STOREV = 'C', LDV >= max(1,N); if STOREV = 'R', LDV >= K.
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i).
*
* T (output) REAL array, dimension (LDT,K)
* The k by k triangular factor T of the block reflector.
* If DIRECT = 'F', T is upper triangular; if DIRECT = 'B', T is
* lower triangular. The rest of the array is not used.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= K.
*
* Further Details
* ===============
*
* Based on contributions by
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
*
* The shape of the matrix V and the storage of the vectors which define
* the H(i) is best illustrated by the following example with n = 5 and
* k = 3. The elements equal to 1 are not stored; the corresponding
* array elements are modified but restored on exit. The rest of the
* array is not used.
*
* DIRECT = 'F' and STOREV = 'C': DIRECT = 'F' and STOREV = 'R':
*
* ______V_____
* ( v1 v2 v3 ) / \
* ( v1 v2 v3 ) ( v1 v1 v1 v1 v1 . . . . 1 )
* V = ( v1 v2 v3 ) ( v2 v2 v2 v2 v2 . . . 1 )
* ( v1 v2 v3 ) ( v3 v3 v3 v3 v3 . . 1 )
* ( v1 v2 v3 )
* . . .
* . . .
* 1 . .
* 1 .
* 1
*
* DIRECT = 'B' and STOREV = 'C': DIRECT = 'B' and STOREV = 'R':
*
* ______V_____
* 1 / \
* . 1 ( 1 . . . . v1 v1 v1 v1 v1 )
* . . 1 ( . 1 . . . v2 v2 v2 v2 v2 )
* . . . ( . . 1 . . v3 v3 v3 v3 v3 )
* . . .
* ( v1 v2 v3 )
* ( v1 v2 v3 )
* V = ( v1 v2 v3 )
* ( v1 v2 v3 )
* ( v1 v2 v3 )
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param direct
* @param storev
* @param n
* @param k
* @param v
* @param _v_offset
* @param ldv
* @param tau
* @param _tau_offset
* @param t
* @param _t_offset
* @param ldt
*
*/
abstract public void slarzt(java.lang.String direct, java.lang.String storev, int n, int k, float[] v, int _v_offset, int ldv, float[] tau, int _tau_offset, float[] t, int _t_offset, int ldt);
/**
*
* ..
*
* Purpose
* =======
*
* SLAS2 computes the singular values of the 2-by-2 matrix
* [ F G ]
* [ 0 H ].
* On return, SSMIN is the smaller singular value and SSMAX is the
* larger singular value.
*
* Arguments
* =========
*
* F (input) REAL
* The (1,1) element of the 2-by-2 matrix.
*
* G (input) REAL
* The (1,2) element of the 2-by-2 matrix.
*
* H (input) REAL
* The (2,2) element of the 2-by-2 matrix.
*
* SSMIN (output) REAL
* The smaller singular value.
*
* SSMAX (output) REAL
* The larger singular value.
*
* Further Details
* ===============
*
* Barring over/underflow, all output quantities are correct to within
* a few units in the last place (ulps), even in the absence of a guard
* digit in addition/subtraction.
*
* In IEEE arithmetic, the code works correctly if one matrix element is
* infinite.
*
* Overflow will not occur unless the largest singular value itself
* overflows, or is within a few ulps of overflow. (On machines with
* partial overflow, like the Cray, overflow may occur if the largest
* singular value is within a factor of 2 of overflow.)
*
* Underflow is harmless if underflow is gradual. Otherwise, results
* may correspond to a matrix modified by perturbations of size near
* the underflow threshold.
*
* ====================================================================
*
* .. Parameters ..
*
*
* @param f
* @param g
* @param h
* @param ssmin
* @param ssmax
*
*/
abstract public void slas2(float f, float g, float h, org.netlib.util.floatW ssmin, org.netlib.util.floatW ssmax);
/**
*
* ..
*
* Purpose
* =======
*
* SLASCL multiplies the M by N real matrix A by the real scalar
* CTO/CFROM. This is done without over/underflow as long as the final
* result CTO*A(I,J)/CFROM does not over/underflow. TYPE specifies that
* A may be full, upper triangular, lower triangular, upper Hessenberg,
* or banded.
*
* Arguments
* =========
*
* TYPE (input) CHARACTER*1
* TYPE indices the storage type of the input matrix.
* = 'G': A is a full matrix.
* = 'L': A is a lower triangular matrix.
* = 'U': A is an upper triangular matrix.
* = 'H': A is an upper Hessenberg matrix.
* = 'B': A is a symmetric band matrix with lower bandwidth KL
* and upper bandwidth KU and with the only the lower
* half stored.
* = 'Q': A is a symmetric band matrix with lower bandwidth KL
* and upper bandwidth KU and with the only the upper
* half stored.
* = 'Z': A is a band matrix with lower bandwidth KL and upper
* bandwidth KU.
*
* KL (input) INTEGER
* The lower bandwidth of A. Referenced only if TYPE = 'B',
* 'Q' or 'Z'.
*
* KU (input) INTEGER
* The upper bandwidth of A. Referenced only if TYPE = 'B',
* 'Q' or 'Z'.
*
* CFROM (input) REAL
* CTO (input) REAL
* The matrix A is multiplied by CTO/CFROM. A(I,J) is computed
* without over/underflow if the final result CTO*A(I,J)/CFROM
* can be represented without over/underflow. CFROM must be
* nonzero.
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* The matrix to be multiplied by CTO/CFROM. See TYPE for the
* storage type.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* INFO (output) INTEGER
* 0 - successful exit
* <0 - if INFO = -i, the i-th argument had an illegal value.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param type
* @param kl
* @param ku
* @param cfrom
* @param cto
* @param m
* @param n
* @param a
* @param lda
* @param info
*
*/
abstract public void slascl(java.lang.String type, int kl, int ku, float cfrom, float cto, int m, int n, float[] a, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLASCL multiplies the M by N real matrix A by the real scalar
* CTO/CFROM. This is done without over/underflow as long as the final
* result CTO*A(I,J)/CFROM does not over/underflow. TYPE specifies that
* A may be full, upper triangular, lower triangular, upper Hessenberg,
* or banded.
*
* Arguments
* =========
*
* TYPE (input) CHARACTER*1
* TYPE indices the storage type of the input matrix.
* = 'G': A is a full matrix.
* = 'L': A is a lower triangular matrix.
* = 'U': A is an upper triangular matrix.
* = 'H': A is an upper Hessenberg matrix.
* = 'B': A is a symmetric band matrix with lower bandwidth KL
* and upper bandwidth KU and with the only the lower
* half stored.
* = 'Q': A is a symmetric band matrix with lower bandwidth KL
* and upper bandwidth KU and with the only the upper
* half stored.
* = 'Z': A is a band matrix with lower bandwidth KL and upper
* bandwidth KU.
*
* KL (input) INTEGER
* The lower bandwidth of A. Referenced only if TYPE = 'B',
* 'Q' or 'Z'.
*
* KU (input) INTEGER
* The upper bandwidth of A. Referenced only if TYPE = 'B',
* 'Q' or 'Z'.
*
* CFROM (input) REAL
* CTO (input) REAL
* The matrix A is multiplied by CTO/CFROM. A(I,J) is computed
* without over/underflow if the final result CTO*A(I,J)/CFROM
* can be represented without over/underflow. CFROM must be
* nonzero.
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* The matrix to be multiplied by CTO/CFROM. See TYPE for the
* storage type.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* INFO (output) INTEGER
* 0 - successful exit
* <0 - if INFO = -i, the i-th argument had an illegal value.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param type
* @param kl
* @param ku
* @param cfrom
* @param cto
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param info
*
*/
abstract public void slascl(java.lang.String type, int kl, int ku, float cfrom, float cto, int m, int n, float[] a, int _a_offset, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* Using a divide and conquer approach, SLASD0 computes the singular
* value decomposition (SVD) of a real upper bidiagonal N-by-M
* matrix B with diagonal D and offdiagonal E, where M = N + SQRE.
* The algorithm computes orthogonal matrices U and VT such that
* B = U * S * VT. The singular values S are overwritten on D.
*
* A related subroutine, SLASDA, computes only the singular values,
* and optionally, the singular vectors in compact form.
*
* Arguments
* =========
*
* N (input) INTEGER
* On entry, the row dimension of the upper bidiagonal matrix.
* This is also the dimension of the main diagonal array D.
*
* SQRE (input) INTEGER
* Specifies the column dimension of the bidiagonal matrix.
* = 0: The bidiagonal matrix has column dimension M = N;
* = 1: The bidiagonal matrix has column dimension M = N+1;
*
* D (input/output) REAL array, dimension (N)
* On entry D contains the main diagonal of the bidiagonal
* matrix.
* On exit D, if INFO = 0, contains its singular values.
*
* E (input) REAL array, dimension (M-1)
* Contains the subdiagonal entries of the bidiagonal matrix.
* On exit, E has been destroyed.
*
* U (output) REAL array, dimension at least (LDQ, N)
* On exit, U contains the left singular vectors.
*
* LDU (input) INTEGER
* On entry, leading dimension of U.
*
* VT (output) REAL array, dimension at least (LDVT, M)
* On exit, VT' contains the right singular vectors.
*
* LDVT (input) INTEGER
* On entry, leading dimension of VT.
*
* SMLSIZ (input) INTEGER
* On entry, maximum size of the subproblems at the
* bottom of the computation tree.
*
* IWORK (workspace) INTEGER array, dimension (8*N)
*
* WORK (workspace) REAL array, dimension (3*M**2+2*M)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an singular value did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n
* @param sqre
* @param d
* @param e
* @param u
* @param ldu
* @param vt
* @param ldvt
* @param smlsiz
* @param iwork
* @param work
* @param info
*
*/
abstract public void slasd0(int n, int sqre, float[] d, float[] e, float[] u, int ldu, float[] vt, int ldvt, int smlsiz, int[] iwork, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* Using a divide and conquer approach, SLASD0 computes the singular
* value decomposition (SVD) of a real upper bidiagonal N-by-M
* matrix B with diagonal D and offdiagonal E, where M = N + SQRE.
* The algorithm computes orthogonal matrices U and VT such that
* B = U * S * VT. The singular values S are overwritten on D.
*
* A related subroutine, SLASDA, computes only the singular values,
* and optionally, the singular vectors in compact form.
*
* Arguments
* =========
*
* N (input) INTEGER
* On entry, the row dimension of the upper bidiagonal matrix.
* This is also the dimension of the main diagonal array D.
*
* SQRE (input) INTEGER
* Specifies the column dimension of the bidiagonal matrix.
* = 0: The bidiagonal matrix has column dimension M = N;
* = 1: The bidiagonal matrix has column dimension M = N+1;
*
* D (input/output) REAL array, dimension (N)
* On entry D contains the main diagonal of the bidiagonal
* matrix.
* On exit D, if INFO = 0, contains its singular values.
*
* E (input) REAL array, dimension (M-1)
* Contains the subdiagonal entries of the bidiagonal matrix.
* On exit, E has been destroyed.
*
* U (output) REAL array, dimension at least (LDQ, N)
* On exit, U contains the left singular vectors.
*
* LDU (input) INTEGER
* On entry, leading dimension of U.
*
* VT (output) REAL array, dimension at least (LDVT, M)
* On exit, VT' contains the right singular vectors.
*
* LDVT (input) INTEGER
* On entry, leading dimension of VT.
*
* SMLSIZ (input) INTEGER
* On entry, maximum size of the subproblems at the
* bottom of the computation tree.
*
* IWORK (workspace) INTEGER array, dimension (8*N)
*
* WORK (workspace) REAL array, dimension (3*M**2+2*M)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an singular value did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n
* @param sqre
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param u
* @param _u_offset
* @param ldu
* @param vt
* @param _vt_offset
* @param ldvt
* @param smlsiz
* @param iwork
* @param _iwork_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void slasd0(int n, int sqre, float[] d, int _d_offset, float[] e, int _e_offset, float[] u, int _u_offset, int ldu, float[] vt, int _vt_offset, int ldvt, int smlsiz, int[] iwork, int _iwork_offset, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLASD1 computes the SVD of an upper bidiagonal N-by-M matrix B,
* where N = NL + NR + 1 and M = N + SQRE. SLASD1 is called from SLASD0.
*
* A related subroutine SLASD7 handles the case in which the singular
* values (and the singular vectors in factored form) are desired.
*
* SLASD1 computes the SVD as follows:
*
* ( D1(in) 0 0 0 )
* B = U(in) * ( Z1' a Z2' b ) * VT(in)
* ( 0 0 D2(in) 0 )
*
* = U(out) * ( D(out) 0) * VT(out)
*
* where Z' = (Z1' a Z2' b) = u' VT', and u is a vector of dimension M
* with ALPHA and BETA in the NL+1 and NL+2 th entries and zeros
* elsewhere; and the entry b is empty if SQRE = 0.
*
* The left singular vectors of the original matrix are stored in U, and
* the transpose of the right singular vectors are stored in VT, and the
* singular values are in D. The algorithm consists of three stages:
*
* The first stage consists of deflating the size of the problem
* when there are multiple singular values or when there are zeros in
* the Z vector. For each such occurence the dimension of the
* secular equation problem is reduced by one. This stage is
* performed by the routine SLASD2.
*
* The second stage consists of calculating the updated
* singular values. This is done by finding the square roots of the
* roots of the secular equation via the routine SLASD4 (as called
* by SLASD3). This routine also calculates the singular vectors of
* the current problem.
*
* The final stage consists of computing the updated singular vectors
* directly using the updated singular values. The singular vectors
* for the current problem are multiplied with the singular vectors
* from the overall problem.
*
* Arguments
* =========
*
* NL (input) INTEGER
* The row dimension of the upper block. NL >= 1.
*
* NR (input) INTEGER
* The row dimension of the lower block. NR >= 1.
*
* SQRE (input) INTEGER
* = 0: the lower block is an NR-by-NR square matrix.
* = 1: the lower block is an NR-by-(NR+1) rectangular matrix.
*
* The bidiagonal matrix has row dimension N = NL + NR + 1,
* and column dimension M = N + SQRE.
*
* D (input/output) REAL array, dimension (NL+NR+1).
* N = NL+NR+1
* On entry D(1:NL,1:NL) contains the singular values of the
* upper block; and D(NL+2:N) contains the singular values of
* the lower block. On exit D(1:N) contains the singular values
* of the modified matrix.
*
* ALPHA (input/output) REAL
* Contains the diagonal element associated with the added row.
*
* BETA (input/output) REAL
* Contains the off-diagonal element associated with the added
* row.
*
* U (input/output) REAL array, dimension (LDU,N)
* On entry U(1:NL, 1:NL) contains the left singular vectors of
* the upper block; U(NL+2:N, NL+2:N) contains the left singular
* vectors of the lower block. On exit U contains the left
* singular vectors of the bidiagonal matrix.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= max( 1, N ).
*
* VT (input/output) REAL array, dimension (LDVT,M)
* where M = N + SQRE.
* On entry VT(1:NL+1, 1:NL+1)' contains the right singular
* vectors of the upper block; VT(NL+2:M, NL+2:M)' contains
* the right singular vectors of the lower block. On exit
* VT' contains the right singular vectors of the
* bidiagonal matrix.
*
* LDVT (input) INTEGER
* The leading dimension of the array VT. LDVT >= max( 1, M ).
*
* IDXQ (output) INTEGER array, dimension (N)
* This contains the permutation which will reintegrate the
* subproblem just solved back into sorted order, i.e.
* D( IDXQ( I = 1, N ) ) will be in ascending order.
*
* IWORK (workspace) INTEGER array, dimension (4*N)
*
* WORK (workspace) REAL array, dimension (3*M**2+2*M)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an singular value did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
*
* @param nl
* @param nr
* @param sqre
* @param d
* @param alpha
* @param beta
* @param u
* @param ldu
* @param vt
* @param ldvt
* @param idxq
* @param iwork
* @param work
* @param info
*
*/
abstract public void slasd1(int nl, int nr, int sqre, float[] d, org.netlib.util.floatW alpha, org.netlib.util.floatW beta, float[] u, int ldu, float[] vt, int ldvt, int[] idxq, int[] iwork, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLASD1 computes the SVD of an upper bidiagonal N-by-M matrix B,
* where N = NL + NR + 1 and M = N + SQRE. SLASD1 is called from SLASD0.
*
* A related subroutine SLASD7 handles the case in which the singular
* values (and the singular vectors in factored form) are desired.
*
* SLASD1 computes the SVD as follows:
*
* ( D1(in) 0 0 0 )
* B = U(in) * ( Z1' a Z2' b ) * VT(in)
* ( 0 0 D2(in) 0 )
*
* = U(out) * ( D(out) 0) * VT(out)
*
* where Z' = (Z1' a Z2' b) = u' VT', and u is a vector of dimension M
* with ALPHA and BETA in the NL+1 and NL+2 th entries and zeros
* elsewhere; and the entry b is empty if SQRE = 0.
*
* The left singular vectors of the original matrix are stored in U, and
* the transpose of the right singular vectors are stored in VT, and the
* singular values are in D. The algorithm consists of three stages:
*
* The first stage consists of deflating the size of the problem
* when there are multiple singular values or when there are zeros in
* the Z vector. For each such occurence the dimension of the
* secular equation problem is reduced by one. This stage is
* performed by the routine SLASD2.
*
* The second stage consists of calculating the updated
* singular values. This is done by finding the square roots of the
* roots of the secular equation via the routine SLASD4 (as called
* by SLASD3). This routine also calculates the singular vectors of
* the current problem.
*
* The final stage consists of computing the updated singular vectors
* directly using the updated singular values. The singular vectors
* for the current problem are multiplied with the singular vectors
* from the overall problem.
*
* Arguments
* =========
*
* NL (input) INTEGER
* The row dimension of the upper block. NL >= 1.
*
* NR (input) INTEGER
* The row dimension of the lower block. NR >= 1.
*
* SQRE (input) INTEGER
* = 0: the lower block is an NR-by-NR square matrix.
* = 1: the lower block is an NR-by-(NR+1) rectangular matrix.
*
* The bidiagonal matrix has row dimension N = NL + NR + 1,
* and column dimension M = N + SQRE.
*
* D (input/output) REAL array, dimension (NL+NR+1).
* N = NL+NR+1
* On entry D(1:NL,1:NL) contains the singular values of the
* upper block; and D(NL+2:N) contains the singular values of
* the lower block. On exit D(1:N) contains the singular values
* of the modified matrix.
*
* ALPHA (input/output) REAL
* Contains the diagonal element associated with the added row.
*
* BETA (input/output) REAL
* Contains the off-diagonal element associated with the added
* row.
*
* U (input/output) REAL array, dimension (LDU,N)
* On entry U(1:NL, 1:NL) contains the left singular vectors of
* the upper block; U(NL+2:N, NL+2:N) contains the left singular
* vectors of the lower block. On exit U contains the left
* singular vectors of the bidiagonal matrix.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= max( 1, N ).
*
* VT (input/output) REAL array, dimension (LDVT,M)
* where M = N + SQRE.
* On entry VT(1:NL+1, 1:NL+1)' contains the right singular
* vectors of the upper block; VT(NL+2:M, NL+2:M)' contains
* the right singular vectors of the lower block. On exit
* VT' contains the right singular vectors of the
* bidiagonal matrix.
*
* LDVT (input) INTEGER
* The leading dimension of the array VT. LDVT >= max( 1, M ).
*
* IDXQ (output) INTEGER array, dimension (N)
* This contains the permutation which will reintegrate the
* subproblem just solved back into sorted order, i.e.
* D( IDXQ( I = 1, N ) ) will be in ascending order.
*
* IWORK (workspace) INTEGER array, dimension (4*N)
*
* WORK (workspace) REAL array, dimension (3*M**2+2*M)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an singular value did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
*
* @param nl
* @param nr
* @param sqre
* @param d
* @param _d_offset
* @param alpha
* @param beta
* @param u
* @param _u_offset
* @param ldu
* @param vt
* @param _vt_offset
* @param ldvt
* @param idxq
* @param _idxq_offset
* @param iwork
* @param _iwork_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void slasd1(int nl, int nr, int sqre, float[] d, int _d_offset, org.netlib.util.floatW alpha, org.netlib.util.floatW beta, float[] u, int _u_offset, int ldu, float[] vt, int _vt_offset, int ldvt, int[] idxq, int _idxq_offset, int[] iwork, int _iwork_offset, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLASD2 merges the two sets of singular values together into a single
* sorted set. Then it tries to deflate the size of the problem.
* There are two ways in which deflation can occur: when two or more
* singular values are close together or if there is a tiny entry in the
* Z vector. For each such occurrence the order of the related secular
* equation problem is reduced by one.
*
* SLASD2 is called from SLASD1.
*
* Arguments
* =========
*
* NL (input) INTEGER
* The row dimension of the upper block. NL >= 1.
*
* NR (input) INTEGER
* The row dimension of the lower block. NR >= 1.
*
* SQRE (input) INTEGER
* = 0: the lower block is an NR-by-NR square matrix.
* = 1: the lower block is an NR-by-(NR+1) rectangular matrix.
*
* The bidiagonal matrix has N = NL + NR + 1 rows and
* M = N + SQRE >= N columns.
*
* K (output) INTEGER
* Contains the dimension of the non-deflated matrix,
* This is the order of the related secular equation. 1 <= K <=N.
*
* D (input/output) REAL array, dimension (N)
* On entry D contains the singular values of the two submatrices
* to be combined. On exit D contains the trailing (N-K) updated
* singular values (those which were deflated) sorted into
* increasing order.
*
* Z (output) REAL array, dimension (N)
* On exit Z contains the updating row vector in the secular
* equation.
*
* ALPHA (input) REAL
* Contains the diagonal element associated with the added row.
*
* BETA (input) REAL
* Contains the off-diagonal element associated with the added
* row.
*
* U (input/output) REAL array, dimension (LDU,N)
* On entry U contains the left singular vectors of two
* submatrices in the two square blocks with corners at (1,1),
* (NL, NL), and (NL+2, NL+2), (N,N).
* On exit U contains the trailing (N-K) updated left singular
* vectors (those which were deflated) in its last N-K columns.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= N.
*
* VT (input/output) REAL array, dimension (LDVT,M)
* On entry VT' contains the right singular vectors of two
* submatrices in the two square blocks with corners at (1,1),
* (NL+1, NL+1), and (NL+2, NL+2), (M,M).
* On exit VT' contains the trailing (N-K) updated right singular
* vectors (those which were deflated) in its last N-K columns.
* In case SQRE =1, the last row of VT spans the right null
* space.
*
* LDVT (input) INTEGER
* The leading dimension of the array VT. LDVT >= M.
*
* DSIGMA (output) REAL array, dimension (N)
* Contains a copy of the diagonal elements (K-1 singular values
* and one zero) in the secular equation.
*
* U2 (output) REAL array, dimension (LDU2,N)
* Contains a copy of the first K-1 left singular vectors which
* will be used by SLASD3 in a matrix multiply (SGEMM) to solve
* for the new left singular vectors. U2 is arranged into four
* blocks. The first block contains a column with 1 at NL+1 and
* zero everywhere else; the second block contains non-zero
* entries only at and above NL; the third contains non-zero
* entries only below NL+1; and the fourth is dense.
*
* LDU2 (input) INTEGER
* The leading dimension of the array U2. LDU2 >= N.
*
* VT2 (output) REAL array, dimension (LDVT2,N)
* VT2' contains a copy of the first K right singular vectors
* which will be used by SLASD3 in a matrix multiply (SGEMM) to
* solve for the new right singular vectors. VT2 is arranged into
* three blocks. The first block contains a row that corresponds
* to the special 0 diagonal element in SIGMA; the second block
* contains non-zeros only at and before NL +1; the third block
* contains non-zeros only at and after NL +2.
*
* LDVT2 (input) INTEGER
* The leading dimension of the array VT2. LDVT2 >= M.
*
* IDXP (workspace) INTEGER array, dimension (N)
* This will contain the permutation used to place deflated
* values of D at the end of the array. On output IDXP(2:K)
* points to the nondeflated D-values and IDXP(K+1:N)
* points to the deflated singular values.
*
* IDX (workspace) INTEGER array, dimension (N)
* This will contain the permutation used to sort the contents of
* D into ascending order.
*
* IDXC (output) INTEGER array, dimension (N)
* This will contain the permutation used to arrange the columns
* of the deflated U matrix into three groups: the first group
* contains non-zero entries only at and above NL, the second
* contains non-zero entries only below NL+2, and the third is
* dense.
*
* IDXQ (input/output) INTEGER array, dimension (N)
* This contains the permutation which separately sorts the two
* sub-problems in D into ascending order. Note that entries in
* the first hlaf of this permutation must first be moved one
* position backward; and entries in the second half
* must first have NL+1 added to their values.
*
* COLTYP (workspace/output) INTEGER array, dimension (N)
* As workspace, this will contain a label which will indicate
* which of the following types a column in the U2 matrix or a
* row in the VT2 matrix is:
* 1 : non-zero in the upper half only
* 2 : non-zero in the lower half only
* 3 : dense
* 4 : deflated
*
* On exit, it is an array of dimension 4, with COLTYP(I) being
* the dimension of the I-th type columns.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param nl
* @param nr
* @param sqre
* @param k
* @param d
* @param z
* @param alpha
* @param beta
* @param u
* @param ldu
* @param vt
* @param ldvt
* @param dsigma
* @param u2
* @param ldu2
* @param vt2
* @param ldvt2
* @param idxp
* @param idx
* @param idxc
* @param idxq
* @param coltyp
* @param info
*
*/
abstract public void slasd2(int nl, int nr, int sqre, org.netlib.util.intW k, float[] d, float[] z, float alpha, float beta, float[] u, int ldu, float[] vt, int ldvt, float[] dsigma, float[] u2, int ldu2, float[] vt2, int ldvt2, int[] idxp, int[] idx, int[] idxc, int[] idxq, int[] coltyp, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLASD2 merges the two sets of singular values together into a single
* sorted set. Then it tries to deflate the size of the problem.
* There are two ways in which deflation can occur: when two or more
* singular values are close together or if there is a tiny entry in the
* Z vector. For each such occurrence the order of the related secular
* equation problem is reduced by one.
*
* SLASD2 is called from SLASD1.
*
* Arguments
* =========
*
* NL (input) INTEGER
* The row dimension of the upper block. NL >= 1.
*
* NR (input) INTEGER
* The row dimension of the lower block. NR >= 1.
*
* SQRE (input) INTEGER
* = 0: the lower block is an NR-by-NR square matrix.
* = 1: the lower block is an NR-by-(NR+1) rectangular matrix.
*
* The bidiagonal matrix has N = NL + NR + 1 rows and
* M = N + SQRE >= N columns.
*
* K (output) INTEGER
* Contains the dimension of the non-deflated matrix,
* This is the order of the related secular equation. 1 <= K <=N.
*
* D (input/output) REAL array, dimension (N)
* On entry D contains the singular values of the two submatrices
* to be combined. On exit D contains the trailing (N-K) updated
* singular values (those which were deflated) sorted into
* increasing order.
*
* Z (output) REAL array, dimension (N)
* On exit Z contains the updating row vector in the secular
* equation.
*
* ALPHA (input) REAL
* Contains the diagonal element associated with the added row.
*
* BETA (input) REAL
* Contains the off-diagonal element associated with the added
* row.
*
* U (input/output) REAL array, dimension (LDU,N)
* On entry U contains the left singular vectors of two
* submatrices in the two square blocks with corners at (1,1),
* (NL, NL), and (NL+2, NL+2), (N,N).
* On exit U contains the trailing (N-K) updated left singular
* vectors (those which were deflated) in its last N-K columns.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= N.
*
* VT (input/output) REAL array, dimension (LDVT,M)
* On entry VT' contains the right singular vectors of two
* submatrices in the two square blocks with corners at (1,1),
* (NL+1, NL+1), and (NL+2, NL+2), (M,M).
* On exit VT' contains the trailing (N-K) updated right singular
* vectors (those which were deflated) in its last N-K columns.
* In case SQRE =1, the last row of VT spans the right null
* space.
*
* LDVT (input) INTEGER
* The leading dimension of the array VT. LDVT >= M.
*
* DSIGMA (output) REAL array, dimension (N)
* Contains a copy of the diagonal elements (K-1 singular values
* and one zero) in the secular equation.
*
* U2 (output) REAL array, dimension (LDU2,N)
* Contains a copy of the first K-1 left singular vectors which
* will be used by SLASD3 in a matrix multiply (SGEMM) to solve
* for the new left singular vectors. U2 is arranged into four
* blocks. The first block contains a column with 1 at NL+1 and
* zero everywhere else; the second block contains non-zero
* entries only at and above NL; the third contains non-zero
* entries only below NL+1; and the fourth is dense.
*
* LDU2 (input) INTEGER
* The leading dimension of the array U2. LDU2 >= N.
*
* VT2 (output) REAL array, dimension (LDVT2,N)
* VT2' contains a copy of the first K right singular vectors
* which will be used by SLASD3 in a matrix multiply (SGEMM) to
* solve for the new right singular vectors. VT2 is arranged into
* three blocks. The first block contains a row that corresponds
* to the special 0 diagonal element in SIGMA; the second block
* contains non-zeros only at and before NL +1; the third block
* contains non-zeros only at and after NL +2.
*
* LDVT2 (input) INTEGER
* The leading dimension of the array VT2. LDVT2 >= M.
*
* IDXP (workspace) INTEGER array, dimension (N)
* This will contain the permutation used to place deflated
* values of D at the end of the array. On output IDXP(2:K)
* points to the nondeflated D-values and IDXP(K+1:N)
* points to the deflated singular values.
*
* IDX (workspace) INTEGER array, dimension (N)
* This will contain the permutation used to sort the contents of
* D into ascending order.
*
* IDXC (output) INTEGER array, dimension (N)
* This will contain the permutation used to arrange the columns
* of the deflated U matrix into three groups: the first group
* contains non-zero entries only at and above NL, the second
* contains non-zero entries only below NL+2, and the third is
* dense.
*
* IDXQ (input/output) INTEGER array, dimension (N)
* This contains the permutation which separately sorts the two
* sub-problems in D into ascending order. Note that entries in
* the first hlaf of this permutation must first be moved one
* position backward; and entries in the second half
* must first have NL+1 added to their values.
*
* COLTYP (workspace/output) INTEGER array, dimension (N)
* As workspace, this will contain a label which will indicate
* which of the following types a column in the U2 matrix or a
* row in the VT2 matrix is:
* 1 : non-zero in the upper half only
* 2 : non-zero in the lower half only
* 3 : dense
* 4 : deflated
*
* On exit, it is an array of dimension 4, with COLTYP(I) being
* the dimension of the I-th type columns.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param nl
* @param nr
* @param sqre
* @param k
* @param d
* @param _d_offset
* @param z
* @param _z_offset
* @param alpha
* @param beta
* @param u
* @param _u_offset
* @param ldu
* @param vt
* @param _vt_offset
* @param ldvt
* @param dsigma
* @param _dsigma_offset
* @param u2
* @param _u2_offset
* @param ldu2
* @param vt2
* @param _vt2_offset
* @param ldvt2
* @param idxp
* @param _idxp_offset
* @param idx
* @param _idx_offset
* @param idxc
* @param _idxc_offset
* @param idxq
* @param _idxq_offset
* @param coltyp
* @param _coltyp_offset
* @param info
*
*/
abstract public void slasd2(int nl, int nr, int sqre, org.netlib.util.intW k, float[] d, int _d_offset, float[] z, int _z_offset, float alpha, float beta, float[] u, int _u_offset, int ldu, float[] vt, int _vt_offset, int ldvt, float[] dsigma, int _dsigma_offset, float[] u2, int _u2_offset, int ldu2, float[] vt2, int _vt2_offset, int ldvt2, int[] idxp, int _idxp_offset, int[] idx, int _idx_offset, int[] idxc, int _idxc_offset, int[] idxq, int _idxq_offset, int[] coltyp, int _coltyp_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLASD3 finds all the square roots of the roots of the secular
* equation, as defined by the values in D and Z. It makes the
* appropriate calls to SLASD4 and then updates the singular
* vectors by matrix multiplication.
*
* This code makes very mild assumptions about floating point
* arithmetic. It will work on machines with a guard digit in
* add/subtract, or on those binary machines without guard digits
* which subtract like the Cray XMP, Cray YMP, Cray C 90, or Cray 2.
* It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* SLASD3 is called from SLASD1.
*
* Arguments
* =========
*
* NL (input) INTEGER
* The row dimension of the upper block. NL >= 1.
*
* NR (input) INTEGER
* The row dimension of the lower block. NR >= 1.
*
* SQRE (input) INTEGER
* = 0: the lower block is an NR-by-NR square matrix.
* = 1: the lower block is an NR-by-(NR+1) rectangular matrix.
*
* The bidiagonal matrix has N = NL + NR + 1 rows and
* M = N + SQRE >= N columns.
*
* K (input) INTEGER
* The size of the secular equation, 1 =< K = < N.
*
* D (output) REAL array, dimension(K)
* On exit the square roots of the roots of the secular equation,
* in ascending order.
*
* Q (workspace) REAL array,
* dimension at least (LDQ,K).
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= K.
*
* DSIGMA (input/output) REAL array, dimension(K)
* The first K elements of this array contain the old roots
* of the deflated updating problem. These are the poles
* of the secular equation.
*
* U (output) REAL array, dimension (LDU, N)
* The last N - K columns of this matrix contain the deflated
* left singular vectors.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= N.
*
* U2 (input) REAL array, dimension (LDU2, N)
* The first K columns of this matrix contain the non-deflated
* left singular vectors for the split problem.
*
* LDU2 (input) INTEGER
* The leading dimension of the array U2. LDU2 >= N.
*
* VT (output) REAL array, dimension (LDVT, M)
* The last M - K columns of VT' contain the deflated
* right singular vectors.
*
* LDVT (input) INTEGER
* The leading dimension of the array VT. LDVT >= N.
*
* VT2 (input/output) REAL array, dimension (LDVT2, N)
* The first K columns of VT2' contain the non-deflated
* right singular vectors for the split problem.
*
* LDVT2 (input) INTEGER
* The leading dimension of the array VT2. LDVT2 >= N.
*
* IDXC (input) INTEGER array, dimension (N)
* The permutation used to arrange the columns of U (and rows of
* VT) into three groups: the first group contains non-zero
* entries only at and above (or before) NL +1; the second
* contains non-zero entries only at and below (or after) NL+2;
* and the third is dense. The first column of U and the row of
* VT are treated separately, however.
*
* The rows of the singular vectors found by SLASD4
* must be likewise permuted before the matrix multiplies can
* take place.
*
* CTOT (input) INTEGER array, dimension (4)
* A count of the total number of the various types of columns
* in U (or rows in VT), as described in IDXC. The fourth column
* type is any column which has been deflated.
*
* Z (input/output) REAL array, dimension (K)
* The first K elements of this array contain the components
* of the deflation-adjusted updating row vector.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an singular value did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param nl
* @param nr
* @param sqre
* @param k
* @param d
* @param q
* @param ldq
* @param dsigma
* @param u
* @param ldu
* @param u2
* @param ldu2
* @param vt
* @param ldvt
* @param vt2
* @param ldvt2
* @param idxc
* @param ctot
* @param z
* @param info
*
*/
abstract public void slasd3(int nl, int nr, int sqre, int k, float[] d, float[] q, int ldq, float[] dsigma, float[] u, int ldu, float[] u2, int ldu2, float[] vt, int ldvt, float[] vt2, int ldvt2, int[] idxc, int[] ctot, float[] z, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLASD3 finds all the square roots of the roots of the secular
* equation, as defined by the values in D and Z. It makes the
* appropriate calls to SLASD4 and then updates the singular
* vectors by matrix multiplication.
*
* This code makes very mild assumptions about floating point
* arithmetic. It will work on machines with a guard digit in
* add/subtract, or on those binary machines without guard digits
* which subtract like the Cray XMP, Cray YMP, Cray C 90, or Cray 2.
* It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* SLASD3 is called from SLASD1.
*
* Arguments
* =========
*
* NL (input) INTEGER
* The row dimension of the upper block. NL >= 1.
*
* NR (input) INTEGER
* The row dimension of the lower block. NR >= 1.
*
* SQRE (input) INTEGER
* = 0: the lower block is an NR-by-NR square matrix.
* = 1: the lower block is an NR-by-(NR+1) rectangular matrix.
*
* The bidiagonal matrix has N = NL + NR + 1 rows and
* M = N + SQRE >= N columns.
*
* K (input) INTEGER
* The size of the secular equation, 1 =< K = < N.
*
* D (output) REAL array, dimension(K)
* On exit the square roots of the roots of the secular equation,
* in ascending order.
*
* Q (workspace) REAL array,
* dimension at least (LDQ,K).
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= K.
*
* DSIGMA (input/output) REAL array, dimension(K)
* The first K elements of this array contain the old roots
* of the deflated updating problem. These are the poles
* of the secular equation.
*
* U (output) REAL array, dimension (LDU, N)
* The last N - K columns of this matrix contain the deflated
* left singular vectors.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= N.
*
* U2 (input) REAL array, dimension (LDU2, N)
* The first K columns of this matrix contain the non-deflated
* left singular vectors for the split problem.
*
* LDU2 (input) INTEGER
* The leading dimension of the array U2. LDU2 >= N.
*
* VT (output) REAL array, dimension (LDVT, M)
* The last M - K columns of VT' contain the deflated
* right singular vectors.
*
* LDVT (input) INTEGER
* The leading dimension of the array VT. LDVT >= N.
*
* VT2 (input/output) REAL array, dimension (LDVT2, N)
* The first K columns of VT2' contain the non-deflated
* right singular vectors for the split problem.
*
* LDVT2 (input) INTEGER
* The leading dimension of the array VT2. LDVT2 >= N.
*
* IDXC (input) INTEGER array, dimension (N)
* The permutation used to arrange the columns of U (and rows of
* VT) into three groups: the first group contains non-zero
* entries only at and above (or before) NL +1; the second
* contains non-zero entries only at and below (or after) NL+2;
* and the third is dense. The first column of U and the row of
* VT are treated separately, however.
*
* The rows of the singular vectors found by SLASD4
* must be likewise permuted before the matrix multiplies can
* take place.
*
* CTOT (input) INTEGER array, dimension (4)
* A count of the total number of the various types of columns
* in U (or rows in VT), as described in IDXC. The fourth column
* type is any column which has been deflated.
*
* Z (input/output) REAL array, dimension (K)
* The first K elements of this array contain the components
* of the deflation-adjusted updating row vector.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an singular value did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param nl
* @param nr
* @param sqre
* @param k
* @param d
* @param _d_offset
* @param q
* @param _q_offset
* @param ldq
* @param dsigma
* @param _dsigma_offset
* @param u
* @param _u_offset
* @param ldu
* @param u2
* @param _u2_offset
* @param ldu2
* @param vt
* @param _vt_offset
* @param ldvt
* @param vt2
* @param _vt2_offset
* @param ldvt2
* @param idxc
* @param _idxc_offset
* @param ctot
* @param _ctot_offset
* @param z
* @param _z_offset
* @param info
*
*/
abstract public void slasd3(int nl, int nr, int sqre, int k, float[] d, int _d_offset, float[] q, int _q_offset, int ldq, float[] dsigma, int _dsigma_offset, float[] u, int _u_offset, int ldu, float[] u2, int _u2_offset, int ldu2, float[] vt, int _vt_offset, int ldvt, float[] vt2, int _vt2_offset, int ldvt2, int[] idxc, int _idxc_offset, int[] ctot, int _ctot_offset, float[] z, int _z_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* This subroutine computes the square root of the I-th updated
* eigenvalue of a positive symmetric rank-one modification to
* a positive diagonal matrix whose entries are given as the squares
* of the corresponding entries in the array d, and that
*
* 0 <= D(i) < D(j) for i < j
*
* and that RHO > 0. This is arranged by the calling routine, and is
* no loss in generality. The rank-one modified system is thus
*
* diag( D ) * diag( D ) + RHO * Z * Z_transpose.
*
* where we assume the Euclidean norm of Z is 1.
*
* The method consists of approximating the rational functions in the
* secular equation by simpler interpolating rational functions.
*
* Arguments
* =========
*
* N (input) INTEGER
* The length of all arrays.
*
* I (input) INTEGER
* The index of the eigenvalue to be computed. 1 <= I <= N.
*
* D (input) REAL array, dimension ( N )
* The original eigenvalues. It is assumed that they are in
* order, 0 <= D(I) < D(J) for I < J.
*
* Z (input) REAL array, dimension (N)
* The components of the updating vector.
*
* DELTA (output) REAL array, dimension (N)
* If N .ne. 1, DELTA contains (D(j) - sigma_I) in its j-th
* component. If N = 1, then DELTA(1) = 1. The vector DELTA
* contains the information necessary to construct the
* (singular) eigenvectors.
*
* RHO (input) REAL
* The scalar in the symmetric updating formula.
*
* SIGMA (output) REAL
* The computed sigma_I, the I-th updated eigenvalue.
*
* WORK (workspace) REAL array, dimension (N)
* If N .ne. 1, WORK contains (D(j) + sigma_I) in its j-th
* component. If N = 1, then WORK( 1 ) = 1.
*
* INFO (output) INTEGER
* = 0: successful exit
* > 0: if INFO = 1, the updating process failed.
*
* Internal Parameters
* ===================
*
* Logical variable ORGATI (origin-at-i?) is used for distinguishing
* whether D(i) or D(i+1) is treated as the origin.
*
* ORGATI = .true. origin at i
* ORGATI = .false. origin at i+1
*
* Logical variable SWTCH3 (switch-for-3-poles?) is for noting
* if we are working with THREE poles!
*
* MAXIT is the maximum number of iterations allowed for each
* eigenvalue.
*
* Further Details
* ===============
*
* Based on contributions by
* Ren-Cang Li, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param i
* @param d
* @param z
* @param delta
* @param rho
* @param sigma
* @param work
* @param info
*
*/
abstract public void slasd4(int n, int i, float[] d, float[] z, float[] delta, float rho, org.netlib.util.floatW sigma, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* This subroutine computes the square root of the I-th updated
* eigenvalue of a positive symmetric rank-one modification to
* a positive diagonal matrix whose entries are given as the squares
* of the corresponding entries in the array d, and that
*
* 0 <= D(i) < D(j) for i < j
*
* and that RHO > 0. This is arranged by the calling routine, and is
* no loss in generality. The rank-one modified system is thus
*
* diag( D ) * diag( D ) + RHO * Z * Z_transpose.
*
* where we assume the Euclidean norm of Z is 1.
*
* The method consists of approximating the rational functions in the
* secular equation by simpler interpolating rational functions.
*
* Arguments
* =========
*
* N (input) INTEGER
* The length of all arrays.
*
* I (input) INTEGER
* The index of the eigenvalue to be computed. 1 <= I <= N.
*
* D (input) REAL array, dimension ( N )
* The original eigenvalues. It is assumed that they are in
* order, 0 <= D(I) < D(J) for I < J.
*
* Z (input) REAL array, dimension (N)
* The components of the updating vector.
*
* DELTA (output) REAL array, dimension (N)
* If N .ne. 1, DELTA contains (D(j) - sigma_I) in its j-th
* component. If N = 1, then DELTA(1) = 1. The vector DELTA
* contains the information necessary to construct the
* (singular) eigenvectors.
*
* RHO (input) REAL
* The scalar in the symmetric updating formula.
*
* SIGMA (output) REAL
* The computed sigma_I, the I-th updated eigenvalue.
*
* WORK (workspace) REAL array, dimension (N)
* If N .ne. 1, WORK contains (D(j) + sigma_I) in its j-th
* component. If N = 1, then WORK( 1 ) = 1.
*
* INFO (output) INTEGER
* = 0: successful exit
* > 0: if INFO = 1, the updating process failed.
*
* Internal Parameters
* ===================
*
* Logical variable ORGATI (origin-at-i?) is used for distinguishing
* whether D(i) or D(i+1) is treated as the origin.
*
* ORGATI = .true. origin at i
* ORGATI = .false. origin at i+1
*
* Logical variable SWTCH3 (switch-for-3-poles?) is for noting
* if we are working with THREE poles!
*
* MAXIT is the maximum number of iterations allowed for each
* eigenvalue.
*
* Further Details
* ===============
*
* Based on contributions by
* Ren-Cang Li, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param i
* @param d
* @param _d_offset
* @param z
* @param _z_offset
* @param delta
* @param _delta_offset
* @param rho
* @param sigma
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void slasd4(int n, int i, float[] d, int _d_offset, float[] z, int _z_offset, float[] delta, int _delta_offset, float rho, org.netlib.util.floatW sigma, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* This subroutine computes the square root of the I-th eigenvalue
* of a positive symmetric rank-one modification of a 2-by-2 diagonal
* matrix
*
* diag( D ) * diag( D ) + RHO * Z * transpose(Z) .
*
* The diagonal entries in the array D are assumed to satisfy
*
* 0 <= D(i) < D(j) for i < j .
*
* We also assume RHO > 0 and that the Euclidean norm of the vector
* Z is one.
*
* Arguments
* =========
*
* I (input) INTEGER
* The index of the eigenvalue to be computed. I = 1 or I = 2.
*
* D (input) REAL array, dimension (2)
* The original eigenvalues. We assume 0 <= D(1) < D(2).
*
* Z (input) REAL array, dimension (2)
* The components of the updating vector.
*
* DELTA (output) REAL array, dimension (2)
* Contains (D(j) - sigma_I) in its j-th component.
* The vector DELTA contains the information necessary
* to construct the eigenvectors.
*
* RHO (input) REAL
* The scalar in the symmetric updating formula.
*
* DSIGMA (output) REAL
* The computed sigma_I, the I-th updated eigenvalue.
*
* WORK (workspace) REAL array, dimension (2)
* WORK contains (D(j) + sigma_I) in its j-th component.
*
* Further Details
* ===============
*
* Based on contributions by
* Ren-Cang Li, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param i
* @param d
* @param z
* @param delta
* @param rho
* @param dsigma
* @param work
*
*/
abstract public void slasd5(int i, float[] d, float[] z, float[] delta, float rho, org.netlib.util.floatW dsigma, float[] work);
/**
*
* ..
*
* Purpose
* =======
*
* This subroutine computes the square root of the I-th eigenvalue
* of a positive symmetric rank-one modification of a 2-by-2 diagonal
* matrix
*
* diag( D ) * diag( D ) + RHO * Z * transpose(Z) .
*
* The diagonal entries in the array D are assumed to satisfy
*
* 0 <= D(i) < D(j) for i < j .
*
* We also assume RHO > 0 and that the Euclidean norm of the vector
* Z is one.
*
* Arguments
* =========
*
* I (input) INTEGER
* The index of the eigenvalue to be computed. I = 1 or I = 2.
*
* D (input) REAL array, dimension (2)
* The original eigenvalues. We assume 0 <= D(1) < D(2).
*
* Z (input) REAL array, dimension (2)
* The components of the updating vector.
*
* DELTA (output) REAL array, dimension (2)
* Contains (D(j) - sigma_I) in its j-th component.
* The vector DELTA contains the information necessary
* to construct the eigenvectors.
*
* RHO (input) REAL
* The scalar in the symmetric updating formula.
*
* DSIGMA (output) REAL
* The computed sigma_I, the I-th updated eigenvalue.
*
* WORK (workspace) REAL array, dimension (2)
* WORK contains (D(j) + sigma_I) in its j-th component.
*
* Further Details
* ===============
*
* Based on contributions by
* Ren-Cang Li, Computer Science Division, University of California
* at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param i
* @param d
* @param _d_offset
* @param z
* @param _z_offset
* @param delta
* @param _delta_offset
* @param rho
* @param dsigma
* @param work
* @param _work_offset
*
*/
abstract public void slasd5(int i, float[] d, int _d_offset, float[] z, int _z_offset, float[] delta, int _delta_offset, float rho, org.netlib.util.floatW dsigma, float[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* SLASD6 computes the SVD of an updated upper bidiagonal matrix B
* obtained by merging two smaller ones by appending a row. This
* routine is used only for the problem which requires all singular
* values and optionally singular vector matrices in factored form.
* B is an N-by-M matrix with N = NL + NR + 1 and M = N + SQRE.
* A related subroutine, SLASD1, handles the case in which all singular
* values and singular vectors of the bidiagonal matrix are desired.
*
* SLASD6 computes the SVD as follows:
*
* ( D1(in) 0 0 0 )
* B = U(in) * ( Z1' a Z2' b ) * VT(in)
* ( 0 0 D2(in) 0 )
*
* = U(out) * ( D(out) 0) * VT(out)
*
* where Z' = (Z1' a Z2' b) = u' VT', and u is a vector of dimension M
* with ALPHA and BETA in the NL+1 and NL+2 th entries and zeros
* elsewhere; and the entry b is empty if SQRE = 0.
*
* The singular values of B can be computed using D1, D2, the first
* components of all the right singular vectors of the lower block, and
* the last components of all the right singular vectors of the upper
* block. These components are stored and updated in VF and VL,
* respectively, in SLASD6. Hence U and VT are not explicitly
* referenced.
*
* The singular values are stored in D. The algorithm consists of two
* stages:
*
* The first stage consists of deflating the size of the problem
* when there are multiple singular values or if there is a zero
* in the Z vector. For each such occurence the dimension of the
* secular equation problem is reduced by one. This stage is
* performed by the routine SLASD7.
*
* The second stage consists of calculating the updated
* singular values. This is done by finding the roots of the
* secular equation via the routine SLASD4 (as called by SLASD8).
* This routine also updates VF and VL and computes the distances
* between the updated singular values and the old singular
* values.
*
* SLASD6 is called from SLASDA.
*
* Arguments
* =========
*
* ICOMPQ (input) INTEGER
* Specifies whether singular vectors are to be computed in
* factored form:
* = 0: Compute singular values only.
* = 1: Compute singular vectors in factored form as well.
*
* NL (input) INTEGER
* The row dimension of the upper block. NL >= 1.
*
* NR (input) INTEGER
* The row dimension of the lower block. NR >= 1.
*
* SQRE (input) INTEGER
* = 0: the lower block is an NR-by-NR square matrix.
* = 1: the lower block is an NR-by-(NR+1) rectangular matrix.
*
* The bidiagonal matrix has row dimension N = NL + NR + 1,
* and column dimension M = N + SQRE.
*
* D (input/output) REAL array, dimension (NL+NR+1).
* On entry D(1:NL,1:NL) contains the singular values of the
* upper block, and D(NL+2:N) contains the singular values
* of the lower block. On exit D(1:N) contains the singular
* values of the modified matrix.
*
* VF (input/output) REAL array, dimension (M)
* On entry, VF(1:NL+1) contains the first components of all
* right singular vectors of the upper block; and VF(NL+2:M)
* contains the first components of all right singular vectors
* of the lower block. On exit, VF contains the first components
* of all right singular vectors of the bidiagonal matrix.
*
* VL (input/output) REAL array, dimension (M)
* On entry, VL(1:NL+1) contains the last components of all
* right singular vectors of the upper block; and VL(NL+2:M)
* contains the last components of all right singular vectors of
* the lower block. On exit, VL contains the last components of
* all right singular vectors of the bidiagonal matrix.
*
* ALPHA (input/output) REAL
* Contains the diagonal element associated with the added row.
*
* BETA (input/output) REAL
* Contains the off-diagonal element associated with the added
* row.
*
* IDXQ (output) INTEGER array, dimension (N)
* This contains the permutation which will reintegrate the
* subproblem just solved back into sorted order, i.e.
* D( IDXQ( I = 1, N ) ) will be in ascending order.
*
* PERM (output) INTEGER array, dimension ( N )
* The permutations (from deflation and sorting) to be applied
* to each block. Not referenced if ICOMPQ = 0.
*
* GIVPTR (output) INTEGER
* The number of Givens rotations which took place in this
* subproblem. Not referenced if ICOMPQ = 0.
*
* GIVCOL (output) INTEGER array, dimension ( LDGCOL, 2 )
* Each pair of numbers indicates a pair of columns to take place
* in a Givens rotation. Not referenced if ICOMPQ = 0.
*
* LDGCOL (input) INTEGER
* leading dimension of GIVCOL, must be at least N.
*
* GIVNUM (output) REAL array, dimension ( LDGNUM, 2 )
* Each number indicates the C or S value to be used in the
* corresponding Givens rotation. Not referenced if ICOMPQ = 0.
*
* LDGNUM (input) INTEGER
* The leading dimension of GIVNUM and POLES, must be at least N.
*
* POLES (output) REAL array, dimension ( LDGNUM, 2 )
* On exit, POLES(1,*) is an array containing the new singular
* values obtained from solving the secular equation, and
* POLES(2,*) is an array containing the poles in the secular
* equation. Not referenced if ICOMPQ = 0.
*
* DIFL (output) REAL array, dimension ( N )
* On exit, DIFL(I) is the distance between I-th updated
* (undeflated) singular value and the I-th (undeflated) old
* singular value.
*
* DIFR (output) REAL array,
* dimension ( LDGNUM, 2 ) if ICOMPQ = 1 and
* dimension ( N ) if ICOMPQ = 0.
* On exit, DIFR(I, 1) is the distance between I-th updated
* (undeflated) singular value and the I+1-th (undeflated) old
* singular value.
*
* If ICOMPQ = 1, DIFR(1:K,2) is an array containing the
* normalizing factors for the right singular vector matrix.
*
* See SLASD8 for details on DIFL and DIFR.
*
* Z (output) REAL array, dimension ( M )
* The first elements of this array contain the components
* of the deflation-adjusted updating row vector.
*
* K (output) INTEGER
* Contains the dimension of the non-deflated matrix,
* This is the order of the related secular equation. 1 <= K <=N.
*
* C (output) REAL
* C contains garbage if SQRE =0 and the C-value of a Givens
* rotation related to the right null space if SQRE = 1.
*
* S (output) REAL
* S contains garbage if SQRE =0 and the S-value of a Givens
* rotation related to the right null space if SQRE = 1.
*
* WORK (workspace) REAL array, dimension ( 4 * M )
*
* IWORK (workspace) INTEGER array, dimension ( 3 * N )
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an singular value did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param nl
* @param nr
* @param sqre
* @param d
* @param vf
* @param vl
* @param alpha
* @param beta
* @param idxq
* @param perm
* @param givptr
* @param givcol
* @param ldgcol
* @param givnum
* @param ldgnum
* @param poles
* @param difl
* @param difr
* @param z
* @param k
* @param c
* @param s
* @param work
* @param iwork
* @param info
*
*/
abstract public void slasd6(int icompq, int nl, int nr, int sqre, float[] d, float[] vf, float[] vl, org.netlib.util.floatW alpha, org.netlib.util.floatW beta, int[] idxq, int[] perm, org.netlib.util.intW givptr, int[] givcol, int ldgcol, float[] givnum, int ldgnum, float[] poles, float[] difl, float[] difr, float[] z, org.netlib.util.intW k, org.netlib.util.floatW c, org.netlib.util.floatW s, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLASD6 computes the SVD of an updated upper bidiagonal matrix B
* obtained by merging two smaller ones by appending a row. This
* routine is used only for the problem which requires all singular
* values and optionally singular vector matrices in factored form.
* B is an N-by-M matrix with N = NL + NR + 1 and M = N + SQRE.
* A related subroutine, SLASD1, handles the case in which all singular
* values and singular vectors of the bidiagonal matrix are desired.
*
* SLASD6 computes the SVD as follows:
*
* ( D1(in) 0 0 0 )
* B = U(in) * ( Z1' a Z2' b ) * VT(in)
* ( 0 0 D2(in) 0 )
*
* = U(out) * ( D(out) 0) * VT(out)
*
* where Z' = (Z1' a Z2' b) = u' VT', and u is a vector of dimension M
* with ALPHA and BETA in the NL+1 and NL+2 th entries and zeros
* elsewhere; and the entry b is empty if SQRE = 0.
*
* The singular values of B can be computed using D1, D2, the first
* components of all the right singular vectors of the lower block, and
* the last components of all the right singular vectors of the upper
* block. These components are stored and updated in VF and VL,
* respectively, in SLASD6. Hence U and VT are not explicitly
* referenced.
*
* The singular values are stored in D. The algorithm consists of two
* stages:
*
* The first stage consists of deflating the size of the problem
* when there are multiple singular values or if there is a zero
* in the Z vector. For each such occurence the dimension of the
* secular equation problem is reduced by one. This stage is
* performed by the routine SLASD7.
*
* The second stage consists of calculating the updated
* singular values. This is done by finding the roots of the
* secular equation via the routine SLASD4 (as called by SLASD8).
* This routine also updates VF and VL and computes the distances
* between the updated singular values and the old singular
* values.
*
* SLASD6 is called from SLASDA.
*
* Arguments
* =========
*
* ICOMPQ (input) INTEGER
* Specifies whether singular vectors are to be computed in
* factored form:
* = 0: Compute singular values only.
* = 1: Compute singular vectors in factored form as well.
*
* NL (input) INTEGER
* The row dimension of the upper block. NL >= 1.
*
* NR (input) INTEGER
* The row dimension of the lower block. NR >= 1.
*
* SQRE (input) INTEGER
* = 0: the lower block is an NR-by-NR square matrix.
* = 1: the lower block is an NR-by-(NR+1) rectangular matrix.
*
* The bidiagonal matrix has row dimension N = NL + NR + 1,
* and column dimension M = N + SQRE.
*
* D (input/output) REAL array, dimension (NL+NR+1).
* On entry D(1:NL,1:NL) contains the singular values of the
* upper block, and D(NL+2:N) contains the singular values
* of the lower block. On exit D(1:N) contains the singular
* values of the modified matrix.
*
* VF (input/output) REAL array, dimension (M)
* On entry, VF(1:NL+1) contains the first components of all
* right singular vectors of the upper block; and VF(NL+2:M)
* contains the first components of all right singular vectors
* of the lower block. On exit, VF contains the first components
* of all right singular vectors of the bidiagonal matrix.
*
* VL (input/output) REAL array, dimension (M)
* On entry, VL(1:NL+1) contains the last components of all
* right singular vectors of the upper block; and VL(NL+2:M)
* contains the last components of all right singular vectors of
* the lower block. On exit, VL contains the last components of
* all right singular vectors of the bidiagonal matrix.
*
* ALPHA (input/output) REAL
* Contains the diagonal element associated with the added row.
*
* BETA (input/output) REAL
* Contains the off-diagonal element associated with the added
* row.
*
* IDXQ (output) INTEGER array, dimension (N)
* This contains the permutation which will reintegrate the
* subproblem just solved back into sorted order, i.e.
* D( IDXQ( I = 1, N ) ) will be in ascending order.
*
* PERM (output) INTEGER array, dimension ( N )
* The permutations (from deflation and sorting) to be applied
* to each block. Not referenced if ICOMPQ = 0.
*
* GIVPTR (output) INTEGER
* The number of Givens rotations which took place in this
* subproblem. Not referenced if ICOMPQ = 0.
*
* GIVCOL (output) INTEGER array, dimension ( LDGCOL, 2 )
* Each pair of numbers indicates a pair of columns to take place
* in a Givens rotation. Not referenced if ICOMPQ = 0.
*
* LDGCOL (input) INTEGER
* leading dimension of GIVCOL, must be at least N.
*
* GIVNUM (output) REAL array, dimension ( LDGNUM, 2 )
* Each number indicates the C or S value to be used in the
* corresponding Givens rotation. Not referenced if ICOMPQ = 0.
*
* LDGNUM (input) INTEGER
* The leading dimension of GIVNUM and POLES, must be at least N.
*
* POLES (output) REAL array, dimension ( LDGNUM, 2 )
* On exit, POLES(1,*) is an array containing the new singular
* values obtained from solving the secular equation, and
* POLES(2,*) is an array containing the poles in the secular
* equation. Not referenced if ICOMPQ = 0.
*
* DIFL (output) REAL array, dimension ( N )
* On exit, DIFL(I) is the distance between I-th updated
* (undeflated) singular value and the I-th (undeflated) old
* singular value.
*
* DIFR (output) REAL array,
* dimension ( LDGNUM, 2 ) if ICOMPQ = 1 and
* dimension ( N ) if ICOMPQ = 0.
* On exit, DIFR(I, 1) is the distance between I-th updated
* (undeflated) singular value and the I+1-th (undeflated) old
* singular value.
*
* If ICOMPQ = 1, DIFR(1:K,2) is an array containing the
* normalizing factors for the right singular vector matrix.
*
* See SLASD8 for details on DIFL and DIFR.
*
* Z (output) REAL array, dimension ( M )
* The first elements of this array contain the components
* of the deflation-adjusted updating row vector.
*
* K (output) INTEGER
* Contains the dimension of the non-deflated matrix,
* This is the order of the related secular equation. 1 <= K <=N.
*
* C (output) REAL
* C contains garbage if SQRE =0 and the C-value of a Givens
* rotation related to the right null space if SQRE = 1.
*
* S (output) REAL
* S contains garbage if SQRE =0 and the S-value of a Givens
* rotation related to the right null space if SQRE = 1.
*
* WORK (workspace) REAL array, dimension ( 4 * M )
*
* IWORK (workspace) INTEGER array, dimension ( 3 * N )
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an singular value did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param nl
* @param nr
* @param sqre
* @param d
* @param _d_offset
* @param vf
* @param _vf_offset
* @param vl
* @param _vl_offset
* @param alpha
* @param beta
* @param idxq
* @param _idxq_offset
* @param perm
* @param _perm_offset
* @param givptr
* @param givcol
* @param _givcol_offset
* @param ldgcol
* @param givnum
* @param _givnum_offset
* @param ldgnum
* @param poles
* @param _poles_offset
* @param difl
* @param _difl_offset
* @param difr
* @param _difr_offset
* @param z
* @param _z_offset
* @param k
* @param c
* @param s
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void slasd6(int icompq, int nl, int nr, int sqre, float[] d, int _d_offset, float[] vf, int _vf_offset, float[] vl, int _vl_offset, org.netlib.util.floatW alpha, org.netlib.util.floatW beta, int[] idxq, int _idxq_offset, int[] perm, int _perm_offset, org.netlib.util.intW givptr, int[] givcol, int _givcol_offset, int ldgcol, float[] givnum, int _givnum_offset, int ldgnum, float[] poles, int _poles_offset, float[] difl, int _difl_offset, float[] difr, int _difr_offset, float[] z, int _z_offset, org.netlib.util.intW k, org.netlib.util.floatW c, org.netlib.util.floatW s, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLASD7 merges the two sets of singular values together into a single
* sorted set. Then it tries to deflate the size of the problem. There
* are two ways in which deflation can occur: when two or more singular
* values are close together or if there is a tiny entry in the Z
* vector. For each such occurrence the order of the related
* secular equation problem is reduced by one.
*
* SLASD7 is called from SLASD6.
*
* Arguments
* =========
*
* ICOMPQ (input) INTEGER
* Specifies whether singular vectors are to be computed
* in compact form, as follows:
* = 0: Compute singular values only.
* = 1: Compute singular vectors of upper
* bidiagonal matrix in compact form.
*
* NL (input) INTEGER
* The row dimension of the upper block. NL >= 1.
*
* NR (input) INTEGER
* The row dimension of the lower block. NR >= 1.
*
* SQRE (input) INTEGER
* = 0: the lower block is an NR-by-NR square matrix.
* = 1: the lower block is an NR-by-(NR+1) rectangular matrix.
*
* The bidiagonal matrix has
* N = NL + NR + 1 rows and
* M = N + SQRE >= N columns.
*
* K (output) INTEGER
* Contains the dimension of the non-deflated matrix, this is
* the order of the related secular equation. 1 <= K <=N.
*
* D (input/output) REAL array, dimension ( N )
* On entry D contains the singular values of the two submatrices
* to be combined. On exit D contains the trailing (N-K) updated
* singular values (those which were deflated) sorted into
* increasing order.
*
* Z (output) REAL array, dimension ( M )
* On exit Z contains the updating row vector in the secular
* equation.
*
* ZW (workspace) REAL array, dimension ( M )
* Workspace for Z.
*
* VF (input/output) REAL array, dimension ( M )
* On entry, VF(1:NL+1) contains the first components of all
* right singular vectors of the upper block; and VF(NL+2:M)
* contains the first components of all right singular vectors
* of the lower block. On exit, VF contains the first components
* of all right singular vectors of the bidiagonal matrix.
*
* VFW (workspace) REAL array, dimension ( M )
* Workspace for VF.
*
* VL (input/output) REAL array, dimension ( M )
* On entry, VL(1:NL+1) contains the last components of all
* right singular vectors of the upper block; and VL(NL+2:M)
* contains the last components of all right singular vectors
* of the lower block. On exit, VL contains the last components
* of all right singular vectors of the bidiagonal matrix.
*
* VLW (workspace) REAL array, dimension ( M )
* Workspace for VL.
*
* ALPHA (input) REAL
* Contains the diagonal element associated with the added row.
*
* BETA (input) REAL
* Contains the off-diagonal element associated with the added
* row.
*
* DSIGMA (output) REAL array, dimension ( N )
* Contains a copy of the diagonal elements (K-1 singular values
* and one zero) in the secular equation.
*
* IDX (workspace) INTEGER array, dimension ( N )
* This will contain the permutation used to sort the contents of
* D into ascending order.
*
* IDXP (workspace) INTEGER array, dimension ( N )
* This will contain the permutation used to place deflated
* values of D at the end of the array. On output IDXP(2:K)
* points to the nondeflated D-values and IDXP(K+1:N)
* points to the deflated singular values.
*
* IDXQ (input) INTEGER array, dimension ( N )
* This contains the permutation which separately sorts the two
* sub-problems in D into ascending order. Note that entries in
* the first half of this permutation must first be moved one
* position backward; and entries in the second half
* must first have NL+1 added to their values.
*
* PERM (output) INTEGER array, dimension ( N )
* The permutations (from deflation and sorting) to be applied
* to each singular block. Not referenced if ICOMPQ = 0.
*
* GIVPTR (output) INTEGER
* The number of Givens rotations which took place in this
* subproblem. Not referenced if ICOMPQ = 0.
*
* GIVCOL (output) INTEGER array, dimension ( LDGCOL, 2 )
* Each pair of numbers indicates a pair of columns to take place
* in a Givens rotation. Not referenced if ICOMPQ = 0.
*
* LDGCOL (input) INTEGER
* The leading dimension of GIVCOL, must be at least N.
*
* GIVNUM (output) REAL array, dimension ( LDGNUM, 2 )
* Each number indicates the C or S value to be used in the
* corresponding Givens rotation. Not referenced if ICOMPQ = 0.
*
* LDGNUM (input) INTEGER
* The leading dimension of GIVNUM, must be at least N.
*
* C (output) REAL
* C contains garbage if SQRE =0 and the C-value of a Givens
* rotation related to the right null space if SQRE = 1.
*
* S (output) REAL
* S contains garbage if SQRE =0 and the S-value of a Givens
* rotation related to the right null space if SQRE = 1.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param nl
* @param nr
* @param sqre
* @param k
* @param d
* @param z
* @param zw
* @param vf
* @param vfw
* @param vl
* @param vlw
* @param alpha
* @param beta
* @param dsigma
* @param idx
* @param idxp
* @param idxq
* @param perm
* @param givptr
* @param givcol
* @param ldgcol
* @param givnum
* @param ldgnum
* @param c
* @param s
* @param info
*
*/
abstract public void slasd7(int icompq, int nl, int nr, int sqre, org.netlib.util.intW k, float[] d, float[] z, float[] zw, float[] vf, float[] vfw, float[] vl, float[] vlw, float alpha, float beta, float[] dsigma, int[] idx, int[] idxp, int[] idxq, int[] perm, org.netlib.util.intW givptr, int[] givcol, int ldgcol, float[] givnum, int ldgnum, org.netlib.util.floatW c, org.netlib.util.floatW s, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLASD7 merges the two sets of singular values together into a single
* sorted set. Then it tries to deflate the size of the problem. There
* are two ways in which deflation can occur: when two or more singular
* values are close together or if there is a tiny entry in the Z
* vector. For each such occurrence the order of the related
* secular equation problem is reduced by one.
*
* SLASD7 is called from SLASD6.
*
* Arguments
* =========
*
* ICOMPQ (input) INTEGER
* Specifies whether singular vectors are to be computed
* in compact form, as follows:
* = 0: Compute singular values only.
* = 1: Compute singular vectors of upper
* bidiagonal matrix in compact form.
*
* NL (input) INTEGER
* The row dimension of the upper block. NL >= 1.
*
* NR (input) INTEGER
* The row dimension of the lower block. NR >= 1.
*
* SQRE (input) INTEGER
* = 0: the lower block is an NR-by-NR square matrix.
* = 1: the lower block is an NR-by-(NR+1) rectangular matrix.
*
* The bidiagonal matrix has
* N = NL + NR + 1 rows and
* M = N + SQRE >= N columns.
*
* K (output) INTEGER
* Contains the dimension of the non-deflated matrix, this is
* the order of the related secular equation. 1 <= K <=N.
*
* D (input/output) REAL array, dimension ( N )
* On entry D contains the singular values of the two submatrices
* to be combined. On exit D contains the trailing (N-K) updated
* singular values (those which were deflated) sorted into
* increasing order.
*
* Z (output) REAL array, dimension ( M )
* On exit Z contains the updating row vector in the secular
* equation.
*
* ZW (workspace) REAL array, dimension ( M )
* Workspace for Z.
*
* VF (input/output) REAL array, dimension ( M )
* On entry, VF(1:NL+1) contains the first components of all
* right singular vectors of the upper block; and VF(NL+2:M)
* contains the first components of all right singular vectors
* of the lower block. On exit, VF contains the first components
* of all right singular vectors of the bidiagonal matrix.
*
* VFW (workspace) REAL array, dimension ( M )
* Workspace for VF.
*
* VL (input/output) REAL array, dimension ( M )
* On entry, VL(1:NL+1) contains the last components of all
* right singular vectors of the upper block; and VL(NL+2:M)
* contains the last components of all right singular vectors
* of the lower block. On exit, VL contains the last components
* of all right singular vectors of the bidiagonal matrix.
*
* VLW (workspace) REAL array, dimension ( M )
* Workspace for VL.
*
* ALPHA (input) REAL
* Contains the diagonal element associated with the added row.
*
* BETA (input) REAL
* Contains the off-diagonal element associated with the added
* row.
*
* DSIGMA (output) REAL array, dimension ( N )
* Contains a copy of the diagonal elements (K-1 singular values
* and one zero) in the secular equation.
*
* IDX (workspace) INTEGER array, dimension ( N )
* This will contain the permutation used to sort the contents of
* D into ascending order.
*
* IDXP (workspace) INTEGER array, dimension ( N )
* This will contain the permutation used to place deflated
* values of D at the end of the array. On output IDXP(2:K)
* points to the nondeflated D-values and IDXP(K+1:N)
* points to the deflated singular values.
*
* IDXQ (input) INTEGER array, dimension ( N )
* This contains the permutation which separately sorts the two
* sub-problems in D into ascending order. Note that entries in
* the first half of this permutation must first be moved one
* position backward; and entries in the second half
* must first have NL+1 added to their values.
*
* PERM (output) INTEGER array, dimension ( N )
* The permutations (from deflation and sorting) to be applied
* to each singular block. Not referenced if ICOMPQ = 0.
*
* GIVPTR (output) INTEGER
* The number of Givens rotations which took place in this
* subproblem. Not referenced if ICOMPQ = 0.
*
* GIVCOL (output) INTEGER array, dimension ( LDGCOL, 2 )
* Each pair of numbers indicates a pair of columns to take place
* in a Givens rotation. Not referenced if ICOMPQ = 0.
*
* LDGCOL (input) INTEGER
* The leading dimension of GIVCOL, must be at least N.
*
* GIVNUM (output) REAL array, dimension ( LDGNUM, 2 )
* Each number indicates the C or S value to be used in the
* corresponding Givens rotation. Not referenced if ICOMPQ = 0.
*
* LDGNUM (input) INTEGER
* The leading dimension of GIVNUM, must be at least N.
*
* C (output) REAL
* C contains garbage if SQRE =0 and the C-value of a Givens
* rotation related to the right null space if SQRE = 1.
*
* S (output) REAL
* S contains garbage if SQRE =0 and the S-value of a Givens
* rotation related to the right null space if SQRE = 1.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param nl
* @param nr
* @param sqre
* @param k
* @param d
* @param _d_offset
* @param z
* @param _z_offset
* @param zw
* @param _zw_offset
* @param vf
* @param _vf_offset
* @param vfw
* @param _vfw_offset
* @param vl
* @param _vl_offset
* @param vlw
* @param _vlw_offset
* @param alpha
* @param beta
* @param dsigma
* @param _dsigma_offset
* @param idx
* @param _idx_offset
* @param idxp
* @param _idxp_offset
* @param idxq
* @param _idxq_offset
* @param perm
* @param _perm_offset
* @param givptr
* @param givcol
* @param _givcol_offset
* @param ldgcol
* @param givnum
* @param _givnum_offset
* @param ldgnum
* @param c
* @param s
* @param info
*
*/
abstract public void slasd7(int icompq, int nl, int nr, int sqre, org.netlib.util.intW k, float[] d, int _d_offset, float[] z, int _z_offset, float[] zw, int _zw_offset, float[] vf, int _vf_offset, float[] vfw, int _vfw_offset, float[] vl, int _vl_offset, float[] vlw, int _vlw_offset, float alpha, float beta, float[] dsigma, int _dsigma_offset, int[] idx, int _idx_offset, int[] idxp, int _idxp_offset, int[] idxq, int _idxq_offset, int[] perm, int _perm_offset, org.netlib.util.intW givptr, int[] givcol, int _givcol_offset, int ldgcol, float[] givnum, int _givnum_offset, int ldgnum, org.netlib.util.floatW c, org.netlib.util.floatW s, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLASD8 finds the square roots of the roots of the secular equation,
* as defined by the values in DSIGMA and Z. It makes the appropriate
* calls to SLASD4, and stores, for each element in D, the distance
* to its two nearest poles (elements in DSIGMA). It also updates
* the arrays VF and VL, the first and last components of all the
* right singular vectors of the original bidiagonal matrix.
*
* SLASD8 is called from SLASD6.
*
* Arguments
* =========
*
* ICOMPQ (input) INTEGER
* Specifies whether singular vectors are to be computed in
* factored form in the calling routine:
* = 0: Compute singular values only.
* = 1: Compute singular vectors in factored form as well.
*
* K (input) INTEGER
* The number of terms in the rational function to be solved
* by SLASD4. K >= 1.
*
* D (output) REAL array, dimension ( K )
* On output, D contains the updated singular values.
*
* Z (input) REAL array, dimension ( K )
* The first K elements of this array contain the components
* of the deflation-adjusted updating row vector.
*
* VF (input/output) REAL array, dimension ( K )
* On entry, VF contains information passed through DBEDE8.
* On exit, VF contains the first K components of the first
* components of all right singular vectors of the bidiagonal
* matrix.
*
* VL (input/output) REAL array, dimension ( K )
* On entry, VL contains information passed through DBEDE8.
* On exit, VL contains the first K components of the last
* components of all right singular vectors of the bidiagonal
* matrix.
*
* DIFL (output) REAL array, dimension ( K )
* On exit, DIFL(I) = D(I) - DSIGMA(I).
*
* DIFR (output) REAL array,
* dimension ( LDDIFR, 2 ) if ICOMPQ = 1 and
* dimension ( K ) if ICOMPQ = 0.
* On exit, DIFR(I,1) = D(I) - DSIGMA(I+1), DIFR(K,1) is not
* defined and will not be referenced.
*
* If ICOMPQ = 1, DIFR(1:K,2) is an array containing the
* normalizing factors for the right singular vector matrix.
*
* LDDIFR (input) INTEGER
* The leading dimension of DIFR, must be at least K.
*
* DSIGMA (input) REAL array, dimension ( K )
* The first K elements of this array contain the old roots
* of the deflated updating problem. These are the poles
* of the secular equation.
*
* WORK (workspace) REAL array, dimension at least 3 * K
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an singular value did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param k
* @param d
* @param z
* @param vf
* @param vl
* @param difl
* @param difr
* @param lddifr
* @param dsigma
* @param work
* @param info
*
*/
abstract public void slasd8(int icompq, int k, float[] d, float[] z, float[] vf, float[] vl, float[] difl, float[] difr, int lddifr, float[] dsigma, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLASD8 finds the square roots of the roots of the secular equation,
* as defined by the values in DSIGMA and Z. It makes the appropriate
* calls to SLASD4, and stores, for each element in D, the distance
* to its two nearest poles (elements in DSIGMA). It also updates
* the arrays VF and VL, the first and last components of all the
* right singular vectors of the original bidiagonal matrix.
*
* SLASD8 is called from SLASD6.
*
* Arguments
* =========
*
* ICOMPQ (input) INTEGER
* Specifies whether singular vectors are to be computed in
* factored form in the calling routine:
* = 0: Compute singular values only.
* = 1: Compute singular vectors in factored form as well.
*
* K (input) INTEGER
* The number of terms in the rational function to be solved
* by SLASD4. K >= 1.
*
* D (output) REAL array, dimension ( K )
* On output, D contains the updated singular values.
*
* Z (input) REAL array, dimension ( K )
* The first K elements of this array contain the components
* of the deflation-adjusted updating row vector.
*
* VF (input/output) REAL array, dimension ( K )
* On entry, VF contains information passed through DBEDE8.
* On exit, VF contains the first K components of the first
* components of all right singular vectors of the bidiagonal
* matrix.
*
* VL (input/output) REAL array, dimension ( K )
* On entry, VL contains information passed through DBEDE8.
* On exit, VL contains the first K components of the last
* components of all right singular vectors of the bidiagonal
* matrix.
*
* DIFL (output) REAL array, dimension ( K )
* On exit, DIFL(I) = D(I) - DSIGMA(I).
*
* DIFR (output) REAL array,
* dimension ( LDDIFR, 2 ) if ICOMPQ = 1 and
* dimension ( K ) if ICOMPQ = 0.
* On exit, DIFR(I,1) = D(I) - DSIGMA(I+1), DIFR(K,1) is not
* defined and will not be referenced.
*
* If ICOMPQ = 1, DIFR(1:K,2) is an array containing the
* normalizing factors for the right singular vector matrix.
*
* LDDIFR (input) INTEGER
* The leading dimension of DIFR, must be at least K.
*
* DSIGMA (input) REAL array, dimension ( K )
* The first K elements of this array contain the old roots
* of the deflated updating problem. These are the poles
* of the secular equation.
*
* WORK (workspace) REAL array, dimension at least 3 * K
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an singular value did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param k
* @param d
* @param _d_offset
* @param z
* @param _z_offset
* @param vf
* @param _vf_offset
* @param vl
* @param _vl_offset
* @param difl
* @param _difl_offset
* @param difr
* @param _difr_offset
* @param lddifr
* @param dsigma
* @param _dsigma_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void slasd8(int icompq, int k, float[] d, int _d_offset, float[] z, int _z_offset, float[] vf, int _vf_offset, float[] vl, int _vl_offset, float[] difl, int _difl_offset, float[] difr, int _difr_offset, int lddifr, float[] dsigma, int _dsigma_offset, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* Using a divide and conquer approach, SLASDA computes the singular
* value decomposition (SVD) of a real upper bidiagonal N-by-M matrix
* B with diagonal D and offdiagonal E, where M = N + SQRE. The
* algorithm computes the singular values in the SVD B = U * S * VT.
* The orthogonal matrices U and VT are optionally computed in
* compact form.
*
* A related subroutine, SLASD0, computes the singular values and
* the singular vectors in explicit form.
*
* Arguments
* =========
*
* ICOMPQ (input) INTEGER
* Specifies whether singular vectors are to be computed
* in compact form, as follows
* = 0: Compute singular values only.
* = 1: Compute singular vectors of upper bidiagonal
* matrix in compact form.
*
* SMLSIZ (input) INTEGER
* The maximum size of the subproblems at the bottom of the
* computation tree.
*
* N (input) INTEGER
* The row dimension of the upper bidiagonal matrix. This is
* also the dimension of the main diagonal array D.
*
* SQRE (input) INTEGER
* Specifies the column dimension of the bidiagonal matrix.
* = 0: The bidiagonal matrix has column dimension M = N;
* = 1: The bidiagonal matrix has column dimension M = N + 1.
*
* D (input/output) REAL array, dimension ( N )
* On entry D contains the main diagonal of the bidiagonal
* matrix. On exit D, if INFO = 0, contains its singular values.
*
* E (input) REAL array, dimension ( M-1 )
* Contains the subdiagonal entries of the bidiagonal matrix.
* On exit, E has been destroyed.
*
* U (output) REAL array,
* dimension ( LDU, SMLSIZ ) if ICOMPQ = 1, and not referenced
* if ICOMPQ = 0. If ICOMPQ = 1, on exit, U contains the left
* singular vector matrices of all subproblems at the bottom
* level.
*
* LDU (input) INTEGER, LDU = > N.
* The leading dimension of arrays U, VT, DIFL, DIFR, POLES,
* GIVNUM, and Z.
*
* VT (output) REAL array,
* dimension ( LDU, SMLSIZ+1 ) if ICOMPQ = 1, and not referenced
* if ICOMPQ = 0. If ICOMPQ = 1, on exit, VT' contains the right
* singular vector matrices of all subproblems at the bottom
* level.
*
* K (output) INTEGER array, dimension ( N )
* if ICOMPQ = 1 and dimension 1 if ICOMPQ = 0.
* If ICOMPQ = 1, on exit, K(I) is the dimension of the I-th
* secular equation on the computation tree.
*
* DIFL (output) REAL array, dimension ( LDU, NLVL ),
* where NLVL = floor(log_2 (N/SMLSIZ))).
*
* DIFR (output) REAL array,
* dimension ( LDU, 2 * NLVL ) if ICOMPQ = 1 and
* dimension ( N ) if ICOMPQ = 0.
* If ICOMPQ = 1, on exit, DIFL(1:N, I) and DIFR(1:N, 2 * I - 1)
* record distances between singular values on the I-th
* level and singular values on the (I -1)-th level, and
* DIFR(1:N, 2 * I ) contains the normalizing factors for
* the right singular vector matrix. See SLASD8 for details.
*
* Z (output) REAL array,
* dimension ( LDU, NLVL ) if ICOMPQ = 1 and
* dimension ( N ) if ICOMPQ = 0.
* The first K elements of Z(1, I) contain the components of
* the deflation-adjusted updating row vector for subproblems
* on the I-th level.
*
* POLES (output) REAL array,
* dimension ( LDU, 2 * NLVL ) if ICOMPQ = 1, and not referenced
* if ICOMPQ = 0. If ICOMPQ = 1, on exit, POLES(1, 2*I - 1) and
* POLES(1, 2*I) contain the new and old singular values
* involved in the secular equations on the I-th level.
*
* GIVPTR (output) INTEGER array,
* dimension ( N ) if ICOMPQ = 1, and not referenced if
* ICOMPQ = 0. If ICOMPQ = 1, on exit, GIVPTR( I ) records
* the number of Givens rotations performed on the I-th
* problem on the computation tree.
*
* GIVCOL (output) INTEGER array,
* dimension ( LDGCOL, 2 * NLVL ) if ICOMPQ = 1, and not
* referenced if ICOMPQ = 0. If ICOMPQ = 1, on exit, for each I,
* GIVCOL(1, 2 *I - 1) and GIVCOL(1, 2 *I) record the locations
* of Givens rotations performed on the I-th level on the
* computation tree.
*
* LDGCOL (input) INTEGER, LDGCOL = > N.
* The leading dimension of arrays GIVCOL and PERM.
*
* PERM (output) INTEGER array, dimension ( LDGCOL, NLVL )
* if ICOMPQ = 1, and not referenced
* if ICOMPQ = 0. If ICOMPQ = 1, on exit, PERM(1, I) records
* permutations done on the I-th level of the computation tree.
*
* GIVNUM (output) REAL array,
* dimension ( LDU, 2 * NLVL ) if ICOMPQ = 1, and not
* referenced if ICOMPQ = 0. If ICOMPQ = 1, on exit, for each I,
* GIVNUM(1, 2 *I - 1) and GIVNUM(1, 2 *I) record the C- and S-
* values of Givens rotations performed on the I-th level on
* the computation tree.
*
* C (output) REAL array,
* dimension ( N ) if ICOMPQ = 1, and dimension 1 if ICOMPQ = 0.
* If ICOMPQ = 1 and the I-th subproblem is not square, on exit,
* C( I ) contains the C-value of a Givens rotation related to
* the right null space of the I-th subproblem.
*
* S (output) REAL array, dimension ( N ) if
* ICOMPQ = 1, and dimension 1 if ICOMPQ = 0. If ICOMPQ = 1
* and the I-th subproblem is not square, on exit, S( I )
* contains the S-value of a Givens rotation related to
* the right null space of the I-th subproblem.
*
* WORK (workspace) REAL array, dimension
* (6 * N + (SMLSIZ + 1)*(SMLSIZ + 1)).
*
* IWORK (workspace) INTEGER array, dimension (7*N).
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an singular value did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param smlsiz
* @param n
* @param sqre
* @param d
* @param e
* @param u
* @param ldu
* @param vt
* @param k
* @param difl
* @param difr
* @param z
* @param poles
* @param givptr
* @param givcol
* @param ldgcol
* @param perm
* @param givnum
* @param c
* @param s
* @param work
* @param iwork
* @param info
*
*/
abstract public void slasda(int icompq, int smlsiz, int n, int sqre, float[] d, float[] e, float[] u, int ldu, float[] vt, int[] k, float[] difl, float[] difr, float[] z, float[] poles, int[] givptr, int[] givcol, int ldgcol, int[] perm, float[] givnum, float[] c, float[] s, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* Using a divide and conquer approach, SLASDA computes the singular
* value decomposition (SVD) of a real upper bidiagonal N-by-M matrix
* B with diagonal D and offdiagonal E, where M = N + SQRE. The
* algorithm computes the singular values in the SVD B = U * S * VT.
* The orthogonal matrices U and VT are optionally computed in
* compact form.
*
* A related subroutine, SLASD0, computes the singular values and
* the singular vectors in explicit form.
*
* Arguments
* =========
*
* ICOMPQ (input) INTEGER
* Specifies whether singular vectors are to be computed
* in compact form, as follows
* = 0: Compute singular values only.
* = 1: Compute singular vectors of upper bidiagonal
* matrix in compact form.
*
* SMLSIZ (input) INTEGER
* The maximum size of the subproblems at the bottom of the
* computation tree.
*
* N (input) INTEGER
* The row dimension of the upper bidiagonal matrix. This is
* also the dimension of the main diagonal array D.
*
* SQRE (input) INTEGER
* Specifies the column dimension of the bidiagonal matrix.
* = 0: The bidiagonal matrix has column dimension M = N;
* = 1: The bidiagonal matrix has column dimension M = N + 1.
*
* D (input/output) REAL array, dimension ( N )
* On entry D contains the main diagonal of the bidiagonal
* matrix. On exit D, if INFO = 0, contains its singular values.
*
* E (input) REAL array, dimension ( M-1 )
* Contains the subdiagonal entries of the bidiagonal matrix.
* On exit, E has been destroyed.
*
* U (output) REAL array,
* dimension ( LDU, SMLSIZ ) if ICOMPQ = 1, and not referenced
* if ICOMPQ = 0. If ICOMPQ = 1, on exit, U contains the left
* singular vector matrices of all subproblems at the bottom
* level.
*
* LDU (input) INTEGER, LDU = > N.
* The leading dimension of arrays U, VT, DIFL, DIFR, POLES,
* GIVNUM, and Z.
*
* VT (output) REAL array,
* dimension ( LDU, SMLSIZ+1 ) if ICOMPQ = 1, and not referenced
* if ICOMPQ = 0. If ICOMPQ = 1, on exit, VT' contains the right
* singular vector matrices of all subproblems at the bottom
* level.
*
* K (output) INTEGER array, dimension ( N )
* if ICOMPQ = 1 and dimension 1 if ICOMPQ = 0.
* If ICOMPQ = 1, on exit, K(I) is the dimension of the I-th
* secular equation on the computation tree.
*
* DIFL (output) REAL array, dimension ( LDU, NLVL ),
* where NLVL = floor(log_2 (N/SMLSIZ))).
*
* DIFR (output) REAL array,
* dimension ( LDU, 2 * NLVL ) if ICOMPQ = 1 and
* dimension ( N ) if ICOMPQ = 0.
* If ICOMPQ = 1, on exit, DIFL(1:N, I) and DIFR(1:N, 2 * I - 1)
* record distances between singular values on the I-th
* level and singular values on the (I -1)-th level, and
* DIFR(1:N, 2 * I ) contains the normalizing factors for
* the right singular vector matrix. See SLASD8 for details.
*
* Z (output) REAL array,
* dimension ( LDU, NLVL ) if ICOMPQ = 1 and
* dimension ( N ) if ICOMPQ = 0.
* The first K elements of Z(1, I) contain the components of
* the deflation-adjusted updating row vector for subproblems
* on the I-th level.
*
* POLES (output) REAL array,
* dimension ( LDU, 2 * NLVL ) if ICOMPQ = 1, and not referenced
* if ICOMPQ = 0. If ICOMPQ = 1, on exit, POLES(1, 2*I - 1) and
* POLES(1, 2*I) contain the new and old singular values
* involved in the secular equations on the I-th level.
*
* GIVPTR (output) INTEGER array,
* dimension ( N ) if ICOMPQ = 1, and not referenced if
* ICOMPQ = 0. If ICOMPQ = 1, on exit, GIVPTR( I ) records
* the number of Givens rotations performed on the I-th
* problem on the computation tree.
*
* GIVCOL (output) INTEGER array,
* dimension ( LDGCOL, 2 * NLVL ) if ICOMPQ = 1, and not
* referenced if ICOMPQ = 0. If ICOMPQ = 1, on exit, for each I,
* GIVCOL(1, 2 *I - 1) and GIVCOL(1, 2 *I) record the locations
* of Givens rotations performed on the I-th level on the
* computation tree.
*
* LDGCOL (input) INTEGER, LDGCOL = > N.
* The leading dimension of arrays GIVCOL and PERM.
*
* PERM (output) INTEGER array, dimension ( LDGCOL, NLVL )
* if ICOMPQ = 1, and not referenced
* if ICOMPQ = 0. If ICOMPQ = 1, on exit, PERM(1, I) records
* permutations done on the I-th level of the computation tree.
*
* GIVNUM (output) REAL array,
* dimension ( LDU, 2 * NLVL ) if ICOMPQ = 1, and not
* referenced if ICOMPQ = 0. If ICOMPQ = 1, on exit, for each I,
* GIVNUM(1, 2 *I - 1) and GIVNUM(1, 2 *I) record the C- and S-
* values of Givens rotations performed on the I-th level on
* the computation tree.
*
* C (output) REAL array,
* dimension ( N ) if ICOMPQ = 1, and dimension 1 if ICOMPQ = 0.
* If ICOMPQ = 1 and the I-th subproblem is not square, on exit,
* C( I ) contains the C-value of a Givens rotation related to
* the right null space of the I-th subproblem.
*
* S (output) REAL array, dimension ( N ) if
* ICOMPQ = 1, and dimension 1 if ICOMPQ = 0. If ICOMPQ = 1
* and the I-th subproblem is not square, on exit, S( I )
* contains the S-value of a Givens rotation related to
* the right null space of the I-th subproblem.
*
* WORK (workspace) REAL array, dimension
* (6 * N + (SMLSIZ + 1)*(SMLSIZ + 1)).
*
* IWORK (workspace) INTEGER array, dimension (7*N).
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = 1, an singular value did not converge
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param icompq
* @param smlsiz
* @param n
* @param sqre
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param u
* @param _u_offset
* @param ldu
* @param vt
* @param _vt_offset
* @param k
* @param _k_offset
* @param difl
* @param _difl_offset
* @param difr
* @param _difr_offset
* @param z
* @param _z_offset
* @param poles
* @param _poles_offset
* @param givptr
* @param _givptr_offset
* @param givcol
* @param _givcol_offset
* @param ldgcol
* @param perm
* @param _perm_offset
* @param givnum
* @param _givnum_offset
* @param c
* @param _c_offset
* @param s
* @param _s_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void slasda(int icompq, int smlsiz, int n, int sqre, float[] d, int _d_offset, float[] e, int _e_offset, float[] u, int _u_offset, int ldu, float[] vt, int _vt_offset, int[] k, int _k_offset, float[] difl, int _difl_offset, float[] difr, int _difr_offset, float[] z, int _z_offset, float[] poles, int _poles_offset, int[] givptr, int _givptr_offset, int[] givcol, int _givcol_offset, int ldgcol, int[] perm, int _perm_offset, float[] givnum, int _givnum_offset, float[] c, int _c_offset, float[] s, int _s_offset, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLASDQ computes the singular value decomposition (SVD) of a real
* (upper or lower) bidiagonal matrix with diagonal D and offdiagonal
* E, accumulating the transformations if desired. Letting B denote
* the input bidiagonal matrix, the algorithm computes orthogonal
* matrices Q and P such that B = Q * S * P' (P' denotes the transpose
* of P). The singular values S are overwritten on D.
*
* The input matrix U is changed to U * Q if desired.
* The input matrix VT is changed to P' * VT if desired.
* The input matrix C is changed to Q' * C if desired.
*
* See "Computing Small Singular Values of Bidiagonal Matrices With
* Guaranteed High Relative Accuracy," by J. Demmel and W. Kahan,
* LAPACK Working Note #3, for a detailed description of the algorithm.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* On entry, UPLO specifies whether the input bidiagonal matrix
* is upper or lower bidiagonal, and wether it is square are
* not.
* UPLO = 'U' or 'u' B is upper bidiagonal.
* UPLO = 'L' or 'l' B is lower bidiagonal.
*
* SQRE (input) INTEGER
* = 0: then the input matrix is N-by-N.
* = 1: then the input matrix is N-by-(N+1) if UPLU = 'U' and
* (N+1)-by-N if UPLU = 'L'.
*
* The bidiagonal matrix has
* N = NL + NR + 1 rows and
* M = N + SQRE >= N columns.
*
* N (input) INTEGER
* On entry, N specifies the number of rows and columns
* in the matrix. N must be at least 0.
*
* NCVT (input) INTEGER
* On entry, NCVT specifies the number of columns of
* the matrix VT. NCVT must be at least 0.
*
* NRU (input) INTEGER
* On entry, NRU specifies the number of rows of
* the matrix U. NRU must be at least 0.
*
* NCC (input) INTEGER
* On entry, NCC specifies the number of columns of
* the matrix C. NCC must be at least 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, D contains the diagonal entries of the
* bidiagonal matrix whose SVD is desired. On normal exit,
* D contains the singular values in ascending order.
*
* E (input/output) REAL array.
* dimension is (N-1) if SQRE = 0 and N if SQRE = 1.
* On entry, the entries of E contain the offdiagonal entries
* of the bidiagonal matrix whose SVD is desired. On normal
* exit, E will contain 0. If the algorithm does not converge,
* D and E will contain the diagonal and superdiagonal entries
* of a bidiagonal matrix orthogonally equivalent to the one
* given as input.
*
* VT (input/output) REAL array, dimension (LDVT, NCVT)
* On entry, contains a matrix which on exit has been
* premultiplied by P', dimension N-by-NCVT if SQRE = 0
* and (N+1)-by-NCVT if SQRE = 1 (not referenced if NCVT=0).
*
* LDVT (input) INTEGER
* On entry, LDVT specifies the leading dimension of VT as
* declared in the calling (sub) program. LDVT must be at
* least 1. If NCVT is nonzero LDVT must also be at least N.
*
* U (input/output) REAL array, dimension (LDU, N)
* On entry, contains a matrix which on exit has been
* postmultiplied by Q, dimension NRU-by-N if SQRE = 0
* and NRU-by-(N+1) if SQRE = 1 (not referenced if NRU=0).
*
* LDU (input) INTEGER
* On entry, LDU specifies the leading dimension of U as
* declared in the calling (sub) program. LDU must be at
* least max( 1, NRU ) .
*
* C (input/output) REAL array, dimension (LDC, NCC)
* On entry, contains an N-by-NCC matrix which on exit
* has been premultiplied by Q' dimension N-by-NCC if SQRE = 0
* and (N+1)-by-NCC if SQRE = 1 (not referenced if NCC=0).
*
* LDC (input) INTEGER
* On entry, LDC specifies the leading dimension of C as
* declared in the calling (sub) program. LDC must be at
* least 1. If NCC is nonzero, LDC must also be at least N.
*
* WORK (workspace) REAL array, dimension (4*N)
* Workspace. Only referenced if one of NCVT, NRU, or NCC is
* nonzero, and if N is at least 2.
*
* INFO (output) INTEGER
* On exit, a value of 0 indicates a successful exit.
* If INFO < 0, argument number -INFO is illegal.
* If INFO > 0, the algorithm did not converge, and INFO
* specifies how many superdiagonals did not converge.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param sqre
* @param n
* @param ncvt
* @param nru
* @param ncc
* @param d
* @param e
* @param vt
* @param ldvt
* @param u
* @param ldu
* @param c
* @param Ldc
* @param work
* @param info
*
*/
abstract public void slasdq(java.lang.String uplo, int sqre, int n, int ncvt, int nru, int ncc, float[] d, float[] e, float[] vt, int ldvt, float[] u, int ldu, float[] c, int Ldc, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLASDQ computes the singular value decomposition (SVD) of a real
* (upper or lower) bidiagonal matrix with diagonal D and offdiagonal
* E, accumulating the transformations if desired. Letting B denote
* the input bidiagonal matrix, the algorithm computes orthogonal
* matrices Q and P such that B = Q * S * P' (P' denotes the transpose
* of P). The singular values S are overwritten on D.
*
* The input matrix U is changed to U * Q if desired.
* The input matrix VT is changed to P' * VT if desired.
* The input matrix C is changed to Q' * C if desired.
*
* See "Computing Small Singular Values of Bidiagonal Matrices With
* Guaranteed High Relative Accuracy," by J. Demmel and W. Kahan,
* LAPACK Working Note #3, for a detailed description of the algorithm.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* On entry, UPLO specifies whether the input bidiagonal matrix
* is upper or lower bidiagonal, and wether it is square are
* not.
* UPLO = 'U' or 'u' B is upper bidiagonal.
* UPLO = 'L' or 'l' B is lower bidiagonal.
*
* SQRE (input) INTEGER
* = 0: then the input matrix is N-by-N.
* = 1: then the input matrix is N-by-(N+1) if UPLU = 'U' and
* (N+1)-by-N if UPLU = 'L'.
*
* The bidiagonal matrix has
* N = NL + NR + 1 rows and
* M = N + SQRE >= N columns.
*
* N (input) INTEGER
* On entry, N specifies the number of rows and columns
* in the matrix. N must be at least 0.
*
* NCVT (input) INTEGER
* On entry, NCVT specifies the number of columns of
* the matrix VT. NCVT must be at least 0.
*
* NRU (input) INTEGER
* On entry, NRU specifies the number of rows of
* the matrix U. NRU must be at least 0.
*
* NCC (input) INTEGER
* On entry, NCC specifies the number of columns of
* the matrix C. NCC must be at least 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, D contains the diagonal entries of the
* bidiagonal matrix whose SVD is desired. On normal exit,
* D contains the singular values in ascending order.
*
* E (input/output) REAL array.
* dimension is (N-1) if SQRE = 0 and N if SQRE = 1.
* On entry, the entries of E contain the offdiagonal entries
* of the bidiagonal matrix whose SVD is desired. On normal
* exit, E will contain 0. If the algorithm does not converge,
* D and E will contain the diagonal and superdiagonal entries
* of a bidiagonal matrix orthogonally equivalent to the one
* given as input.
*
* VT (input/output) REAL array, dimension (LDVT, NCVT)
* On entry, contains a matrix which on exit has been
* premultiplied by P', dimension N-by-NCVT if SQRE = 0
* and (N+1)-by-NCVT if SQRE = 1 (not referenced if NCVT=0).
*
* LDVT (input) INTEGER
* On entry, LDVT specifies the leading dimension of VT as
* declared in the calling (sub) program. LDVT must be at
* least 1. If NCVT is nonzero LDVT must also be at least N.
*
* U (input/output) REAL array, dimension (LDU, N)
* On entry, contains a matrix which on exit has been
* postmultiplied by Q, dimension NRU-by-N if SQRE = 0
* and NRU-by-(N+1) if SQRE = 1 (not referenced if NRU=0).
*
* LDU (input) INTEGER
* On entry, LDU specifies the leading dimension of U as
* declared in the calling (sub) program. LDU must be at
* least max( 1, NRU ) .
*
* C (input/output) REAL array, dimension (LDC, NCC)
* On entry, contains an N-by-NCC matrix which on exit
* has been premultiplied by Q' dimension N-by-NCC if SQRE = 0
* and (N+1)-by-NCC if SQRE = 1 (not referenced if NCC=0).
*
* LDC (input) INTEGER
* On entry, LDC specifies the leading dimension of C as
* declared in the calling (sub) program. LDC must be at
* least 1. If NCC is nonzero, LDC must also be at least N.
*
* WORK (workspace) REAL array, dimension (4*N)
* Workspace. Only referenced if one of NCVT, NRU, or NCC is
* nonzero, and if N is at least 2.
*
* INFO (output) INTEGER
* On exit, a value of 0 indicates a successful exit.
* If INFO < 0, argument number -INFO is illegal.
* If INFO > 0, the algorithm did not converge, and INFO
* specifies how many superdiagonals did not converge.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param sqre
* @param n
* @param ncvt
* @param nru
* @param ncc
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param vt
* @param _vt_offset
* @param ldvt
* @param u
* @param _u_offset
* @param ldu
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void slasdq(java.lang.String uplo, int sqre, int n, int ncvt, int nru, int ncc, float[] d, int _d_offset, float[] e, int _e_offset, float[] vt, int _vt_offset, int ldvt, float[] u, int _u_offset, int ldu, float[] c, int _c_offset, int Ldc, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLASDT creates a tree of subproblems for bidiagonal divide and
* conquer.
*
* Arguments
* =========
*
* N (input) INTEGER
* On entry, the number of diagonal elements of the
* bidiagonal matrix.
*
* LVL (output) INTEGER
* On exit, the number of levels on the computation tree.
*
* ND (output) INTEGER
* On exit, the number of nodes on the tree.
*
* INODE (output) INTEGER array, dimension ( N )
* On exit, centers of subproblems.
*
* NDIML (output) INTEGER array, dimension ( N )
* On exit, row dimensions of left children.
*
* NDIMR (output) INTEGER array, dimension ( N )
* On exit, row dimensions of right children.
*
* MSUB (input) INTEGER.
* On entry, the maximum row dimension each subproblem at the
* bottom of the tree can be of.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param lvl
* @param nd
* @param inode
* @param ndiml
* @param ndimr
* @param msub
*
*/
abstract public void slasdt(int n, org.netlib.util.intW lvl, org.netlib.util.intW nd, int[] inode, int[] ndiml, int[] ndimr, int msub);
/**
*
* ..
*
* Purpose
* =======
*
* SLASDT creates a tree of subproblems for bidiagonal divide and
* conquer.
*
* Arguments
* =========
*
* N (input) INTEGER
* On entry, the number of diagonal elements of the
* bidiagonal matrix.
*
* LVL (output) INTEGER
* On exit, the number of levels on the computation tree.
*
* ND (output) INTEGER
* On exit, the number of nodes on the tree.
*
* INODE (output) INTEGER array, dimension ( N )
* On exit, centers of subproblems.
*
* NDIML (output) INTEGER array, dimension ( N )
* On exit, row dimensions of left children.
*
* NDIMR (output) INTEGER array, dimension ( N )
* On exit, row dimensions of right children.
*
* MSUB (input) INTEGER.
* On entry, the maximum row dimension each subproblem at the
* bottom of the tree can be of.
*
* Further Details
* ===============
*
* Based on contributions by
* Ming Gu and Huan Ren, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param lvl
* @param nd
* @param inode
* @param _inode_offset
* @param ndiml
* @param _ndiml_offset
* @param ndimr
* @param _ndimr_offset
* @param msub
*
*/
abstract public void slasdt(int n, org.netlib.util.intW lvl, org.netlib.util.intW nd, int[] inode, int _inode_offset, int[] ndiml, int _ndiml_offset, int[] ndimr, int _ndimr_offset, int msub);
/**
*
* ..
*
* Purpose
* =======
*
* SLASET initializes an m-by-n matrix A to BETA on the diagonal and
* ALPHA on the offdiagonals.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies the part of the matrix A to be set.
* = 'U': Upper triangular part is set; the strictly lower
* triangular part of A is not changed.
* = 'L': Lower triangular part is set; the strictly upper
* triangular part of A is not changed.
* Otherwise: All of the matrix A is set.
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* ALPHA (input) REAL
* The constant to which the offdiagonal elements are to be set.
*
* BETA (input) REAL
* The constant to which the diagonal elements are to be set.
*
* A (input/output) REAL array, dimension (LDA,N)
* On exit, the leading m-by-n submatrix of A is set as follows:
*
* if UPLO = 'U', A(i,j) = ALPHA, 1<=i<=j-1, 1<=j<=n,
* if UPLO = 'L', A(i,j) = ALPHA, j+1<=i<=m, 1<=j<=n,
* otherwise, A(i,j) = ALPHA, 1<=i<=m, 1<=j<=n, i.ne.j,
*
* and, for all UPLO, A(i,i) = BETA, 1<=i<=min(m,n).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param uplo
* @param m
* @param n
* @param alpha
* @param beta
* @param a
* @param lda
*
*/
abstract public void slaset(java.lang.String uplo, int m, int n, float alpha, float beta, float[] a, int lda);
/**
*
* ..
*
* Purpose
* =======
*
* SLASET initializes an m-by-n matrix A to BETA on the diagonal and
* ALPHA on the offdiagonals.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies the part of the matrix A to be set.
* = 'U': Upper triangular part is set; the strictly lower
* triangular part of A is not changed.
* = 'L': Lower triangular part is set; the strictly upper
* triangular part of A is not changed.
* Otherwise: All of the matrix A is set.
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* ALPHA (input) REAL
* The constant to which the offdiagonal elements are to be set.
*
* BETA (input) REAL
* The constant to which the diagonal elements are to be set.
*
* A (input/output) REAL array, dimension (LDA,N)
* On exit, the leading m-by-n submatrix of A is set as follows:
*
* if UPLO = 'U', A(i,j) = ALPHA, 1<=i<=j-1, 1<=j<=n,
* if UPLO = 'L', A(i,j) = ALPHA, j+1<=i<=m, 1<=j<=n,
* otherwise, A(i,j) = ALPHA, 1<=i<=m, 1<=j<=n, i.ne.j,
*
* and, for all UPLO, A(i,i) = BETA, 1<=i<=min(m,n).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param uplo
* @param m
* @param n
* @param alpha
* @param beta
* @param a
* @param _a_offset
* @param lda
*
*/
abstract public void slaset(java.lang.String uplo, int m, int n, float alpha, float beta, float[] a, int _a_offset, int lda);
/**
*
* ..
*
* Purpose
* =======
*
* SLASQ1 computes the singular values of a real N-by-N bidiagonal
* matrix with diagonal D and off-diagonal E. The singular values
* are computed to high relative accuracy, in the absence of
* denormalization, underflow and overflow. The algorithm was first
* presented in
*
* "Accurate singular values and differential qd algorithms" by K. V.
* Fernando and B. N. Parlett, Numer. Math., Vol-67, No. 2, pp. 191-230,
* 1994,
*
* and the present implementation is described in "An implementation of
* the dqds Algorithm (Positive Case)", LAPACK Working Note.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of rows and columns in the matrix. N >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, D contains the diagonal elements of the
* bidiagonal matrix whose SVD is desired. On normal exit,
* D contains the singular values in decreasing order.
*
* E (input/output) REAL array, dimension (N)
* On entry, elements E(1:N-1) contain the off-diagonal elements
* of the bidiagonal matrix whose SVD is desired.
* On exit, E is overwritten.
*
* WORK (workspace) REAL array, dimension (4*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: the algorithm failed
* = 1, a split was marked by a positive value in E
* = 2, current block of Z not diagonalized after 30*N
* iterations (in inner while loop)
* = 3, termination criterion of outer while loop not met
* (program created more than N unreduced blocks)
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param e
* @param work
* @param info
*
*/
abstract public void slasq1(int n, float[] d, float[] e, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLASQ1 computes the singular values of a real N-by-N bidiagonal
* matrix with diagonal D and off-diagonal E. The singular values
* are computed to high relative accuracy, in the absence of
* denormalization, underflow and overflow. The algorithm was first
* presented in
*
* "Accurate singular values and differential qd algorithms" by K. V.
* Fernando and B. N. Parlett, Numer. Math., Vol-67, No. 2, pp. 191-230,
* 1994,
*
* and the present implementation is described in "An implementation of
* the dqds Algorithm (Positive Case)", LAPACK Working Note.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of rows and columns in the matrix. N >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, D contains the diagonal elements of the
* bidiagonal matrix whose SVD is desired. On normal exit,
* D contains the singular values in decreasing order.
*
* E (input/output) REAL array, dimension (N)
* On entry, elements E(1:N-1) contain the off-diagonal elements
* of the bidiagonal matrix whose SVD is desired.
* On exit, E is overwritten.
*
* WORK (workspace) REAL array, dimension (4*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: the algorithm failed
* = 1, a split was marked by a positive value in E
* = 2, current block of Z not diagonalized after 30*N
* iterations (in inner while loop)
* = 3, termination criterion of outer while loop not met
* (program created more than N unreduced blocks)
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void slasq1(int n, float[] d, int _d_offset, float[] e, int _e_offset, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLASQ2 computes all the eigenvalues of the symmetric positive
* definite tridiagonal matrix associated with the qd array Z to high
* relative accuracy are computed to high relative accuracy, in the
* absence of denormalization, underflow and overflow.
*
* To see the relation of Z to the tridiagonal matrix, let L be a
* unit lower bidiagonal matrix with subdiagonals Z(2,4,6,,..) and
* let U be an upper bidiagonal matrix with 1's above and diagonal
* Z(1,3,5,,..). The tridiagonal is L*U or, if you prefer, the
* symmetric tridiagonal to which it is similar.
*
* Note : SLASQ2 defines a logical variable, IEEE, which is true
* on machines which follow ieee-754 floating-point standard in their
* handling of infinities and NaNs, and false otherwise. This variable
* is passed to SLAZQ3.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of rows and columns in the matrix. N >= 0.
*
* Z (workspace) REAL array, dimension (4*N)
* On entry Z holds the qd array. On exit, entries 1 to N hold
* the eigenvalues in decreasing order, Z( 2*N+1 ) holds the
* trace, and Z( 2*N+2 ) holds the sum of the eigenvalues. If
* N > 2, then Z( 2*N+3 ) holds the iteration count, Z( 2*N+4 )
* holds NDIVS/NIN^2, and Z( 2*N+5 ) holds the percentage of
* shifts that failed.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if the i-th argument is a scalar and had an illegal
* value, then INFO = -i, if the i-th argument is an
* array and the j-entry had an illegal value, then
* INFO = -(i*100+j)
* > 0: the algorithm failed
* = 1, a split was marked by a positive value in E
* = 2, current block of Z not diagonalized after 30*N
* iterations (in inner while loop)
* = 3, termination criterion of outer while loop not met
* (program created more than N unreduced blocks)
*
* Further Details
* ===============
* Local Variables: I0:N0 defines a current unreduced segment of Z.
* The shifts are accumulated in SIGMA. Iteration count is in ITER.
* Ping-pong is controlled by PP (alternates between 0 and 1).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param z
* @param info
*
*/
abstract public void slasq2(int n, float[] z, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLASQ2 computes all the eigenvalues of the symmetric positive
* definite tridiagonal matrix associated with the qd array Z to high
* relative accuracy are computed to high relative accuracy, in the
* absence of denormalization, underflow and overflow.
*
* To see the relation of Z to the tridiagonal matrix, let L be a
* unit lower bidiagonal matrix with subdiagonals Z(2,4,6,,..) and
* let U be an upper bidiagonal matrix with 1's above and diagonal
* Z(1,3,5,,..). The tridiagonal is L*U or, if you prefer, the
* symmetric tridiagonal to which it is similar.
*
* Note : SLASQ2 defines a logical variable, IEEE, which is true
* on machines which follow ieee-754 floating-point standard in their
* handling of infinities and NaNs, and false otherwise. This variable
* is passed to SLAZQ3.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of rows and columns in the matrix. N >= 0.
*
* Z (workspace) REAL array, dimension (4*N)
* On entry Z holds the qd array. On exit, entries 1 to N hold
* the eigenvalues in decreasing order, Z( 2*N+1 ) holds the
* trace, and Z( 2*N+2 ) holds the sum of the eigenvalues. If
* N > 2, then Z( 2*N+3 ) holds the iteration count, Z( 2*N+4 )
* holds NDIVS/NIN^2, and Z( 2*N+5 ) holds the percentage of
* shifts that failed.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if the i-th argument is a scalar and had an illegal
* value, then INFO = -i, if the i-th argument is an
* array and the j-entry had an illegal value, then
* INFO = -(i*100+j)
* > 0: the algorithm failed
* = 1, a split was marked by a positive value in E
* = 2, current block of Z not diagonalized after 30*N
* iterations (in inner while loop)
* = 3, termination criterion of outer while loop not met
* (program created more than N unreduced blocks)
*
* Further Details
* ===============
* Local Variables: I0:N0 defines a current unreduced segment of Z.
* The shifts are accumulated in SIGMA. Iteration count is in ITER.
* Ping-pong is controlled by PP (alternates between 0 and 1).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param z
* @param _z_offset
* @param info
*
*/
abstract public void slasq2(int n, float[] z, int _z_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLASQ3 checks for deflation, computes a shift (TAU) and calls dqds.
* In case of failure it changes shifts, and tries again until output
* is positive.
*
* Arguments
* =========
*
* I0 (input) INTEGER
* First index.
*
* N0 (input) INTEGER
* Last index.
*
* Z (input) REAL array, dimension ( 4*N )
* Z holds the qd array.
*
* PP (input) INTEGER
* PP=0 for ping, PP=1 for pong.
*
* DMIN (output) REAL
* Minimum value of d.
*
* SIGMA (output) REAL
* Sum of shifts used in current segment.
*
* DESIG (input/output) REAL
* Lower order part of SIGMA
*
* QMAX (input) REAL
* Maximum value of q.
*
* NFAIL (output) INTEGER
* Number of times shift was too big.
*
* ITER (output) INTEGER
* Number of iterations.
*
* NDIV (output) INTEGER
* Number of divisions.
*
* TTYPE (output) INTEGER
* Shift type.
*
* IEEE (input) LOGICAL
* Flag for IEEE or non IEEE arithmetic (passed to SLASQ5).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param i0
* @param n0
* @param z
* @param pp
* @param dmin
* @param sigma
* @param desig
* @param qmax
* @param nfail
* @param iter
* @param ndiv
* @param ieee
*
*/
abstract public void slasq3(int i0, org.netlib.util.intW n0, float[] z, int pp, org.netlib.util.floatW dmin, org.netlib.util.floatW sigma, org.netlib.util.floatW desig, org.netlib.util.floatW qmax, org.netlib.util.intW nfail, org.netlib.util.intW iter, org.netlib.util.intW ndiv, boolean ieee);
/**
*
* ..
*
* Purpose
* =======
*
* SLASQ3 checks for deflation, computes a shift (TAU) and calls dqds.
* In case of failure it changes shifts, and tries again until output
* is positive.
*
* Arguments
* =========
*
* I0 (input) INTEGER
* First index.
*
* N0 (input) INTEGER
* Last index.
*
* Z (input) REAL array, dimension ( 4*N )
* Z holds the qd array.
*
* PP (input) INTEGER
* PP=0 for ping, PP=1 for pong.
*
* DMIN (output) REAL
* Minimum value of d.
*
* SIGMA (output) REAL
* Sum of shifts used in current segment.
*
* DESIG (input/output) REAL
* Lower order part of SIGMA
*
* QMAX (input) REAL
* Maximum value of q.
*
* NFAIL (output) INTEGER
* Number of times shift was too big.
*
* ITER (output) INTEGER
* Number of iterations.
*
* NDIV (output) INTEGER
* Number of divisions.
*
* TTYPE (output) INTEGER
* Shift type.
*
* IEEE (input) LOGICAL
* Flag for IEEE or non IEEE arithmetic (passed to SLASQ5).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param i0
* @param n0
* @param z
* @param _z_offset
* @param pp
* @param dmin
* @param sigma
* @param desig
* @param qmax
* @param nfail
* @param iter
* @param ndiv
* @param ieee
*
*/
abstract public void slasq3(int i0, org.netlib.util.intW n0, float[] z, int _z_offset, int pp, org.netlib.util.floatW dmin, org.netlib.util.floatW sigma, org.netlib.util.floatW desig, org.netlib.util.floatW qmax, org.netlib.util.intW nfail, org.netlib.util.intW iter, org.netlib.util.intW ndiv, boolean ieee);
/**
*
* ..
*
* Purpose
* =======
*
* SLASQ4 computes an approximation TAU to the smallest eigenvalue
* using values of d from the previous transform.
*
* I0 (input) INTEGER
* First index.
*
* N0 (input) INTEGER
* Last index.
*
* Z (input) REAL array, dimension ( 4*N )
* Z holds the qd array.
*
* PP (input) INTEGER
* PP=0 for ping, PP=1 for pong.
*
* N0IN (input) INTEGER
* The value of N0 at start of EIGTEST.
*
* DMIN (input) REAL
* Minimum value of d.
*
* DMIN1 (input) REAL
* Minimum value of d, excluding D( N0 ).
*
* DMIN2 (input) REAL
* Minimum value of d, excluding D( N0 ) and D( N0-1 ).
*
* DN (input) REAL
* d(N)
*
* DN1 (input) REAL
* d(N-1)
*
* DN2 (input) REAL
* d(N-2)
*
* TAU (output) REAL
* This is the shift.
*
* TTYPE (output) INTEGER
* Shift type.
*
* Further Details
* ===============
* CNST1 = 9/16
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param i0
* @param n0
* @param z
* @param pp
* @param n0in
* @param dmin
* @param dmin1
* @param dmin2
* @param dn
* @param dn1
* @param dn2
* @param tau
* @param ttype
*
*/
abstract public void slasq4(int i0, int n0, float[] z, int pp, int n0in, float dmin, float dmin1, float dmin2, float dn, float dn1, float dn2, org.netlib.util.floatW tau, org.netlib.util.intW ttype);
/**
*
* ..
*
* Purpose
* =======
*
* SLASQ4 computes an approximation TAU to the smallest eigenvalue
* using values of d from the previous transform.
*
* I0 (input) INTEGER
* First index.
*
* N0 (input) INTEGER
* Last index.
*
* Z (input) REAL array, dimension ( 4*N )
* Z holds the qd array.
*
* PP (input) INTEGER
* PP=0 for ping, PP=1 for pong.
*
* N0IN (input) INTEGER
* The value of N0 at start of EIGTEST.
*
* DMIN (input) REAL
* Minimum value of d.
*
* DMIN1 (input) REAL
* Minimum value of d, excluding D( N0 ).
*
* DMIN2 (input) REAL
* Minimum value of d, excluding D( N0 ) and D( N0-1 ).
*
* DN (input) REAL
* d(N)
*
* DN1 (input) REAL
* d(N-1)
*
* DN2 (input) REAL
* d(N-2)
*
* TAU (output) REAL
* This is the shift.
*
* TTYPE (output) INTEGER
* Shift type.
*
* Further Details
* ===============
* CNST1 = 9/16
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param i0
* @param n0
* @param z
* @param _z_offset
* @param pp
* @param n0in
* @param dmin
* @param dmin1
* @param dmin2
* @param dn
* @param dn1
* @param dn2
* @param tau
* @param ttype
*
*/
abstract public void slasq4(int i0, int n0, float[] z, int _z_offset, int pp, int n0in, float dmin, float dmin1, float dmin2, float dn, float dn1, float dn2, org.netlib.util.floatW tau, org.netlib.util.intW ttype);
/**
*
* ..
*
* Purpose
* =======
*
* SLASQ5 computes one dqds transform in ping-pong form, one
* version for IEEE machines another for non IEEE machines.
*
* Arguments
* =========
*
* I0 (input) INTEGER
* First index.
*
* N0 (input) INTEGER
* Last index.
*
* Z (input) REAL array, dimension ( 4*N )
* Z holds the qd array. EMIN is stored in Z(4*N0) to avoid
* an extra argument.
*
* PP (input) INTEGER
* PP=0 for ping, PP=1 for pong.
*
* TAU (input) REAL
* This is the shift.
*
* DMIN (output) REAL
* Minimum value of d.
*
* DMIN1 (output) REAL
* Minimum value of d, excluding D( N0 ).
*
* DMIN2 (output) REAL
* Minimum value of d, excluding D( N0 ) and D( N0-1 ).
*
* DN (output) REAL
* d(N0), the last value of d.
*
* DNM1 (output) REAL
* d(N0-1).
*
* DNM2 (output) REAL
* d(N0-2).
*
* IEEE (input) LOGICAL
* Flag for IEEE or non IEEE arithmetic.
*
* =====================================================================
*
* .. Parameter ..
*
*
* @param i0
* @param n0
* @param z
* @param pp
* @param tau
* @param dmin
* @param dmin1
* @param dmin2
* @param dn
* @param dnm1
* @param dnm2
* @param ieee
*
*/
abstract public void slasq5(int i0, int n0, float[] z, int pp, float tau, org.netlib.util.floatW dmin, org.netlib.util.floatW dmin1, org.netlib.util.floatW dmin2, org.netlib.util.floatW dn, org.netlib.util.floatW dnm1, org.netlib.util.floatW dnm2, boolean ieee);
/**
*
* ..
*
* Purpose
* =======
*
* SLASQ5 computes one dqds transform in ping-pong form, one
* version for IEEE machines another for non IEEE machines.
*
* Arguments
* =========
*
* I0 (input) INTEGER
* First index.
*
* N0 (input) INTEGER
* Last index.
*
* Z (input) REAL array, dimension ( 4*N )
* Z holds the qd array. EMIN is stored in Z(4*N0) to avoid
* an extra argument.
*
* PP (input) INTEGER
* PP=0 for ping, PP=1 for pong.
*
* TAU (input) REAL
* This is the shift.
*
* DMIN (output) REAL
* Minimum value of d.
*
* DMIN1 (output) REAL
* Minimum value of d, excluding D( N0 ).
*
* DMIN2 (output) REAL
* Minimum value of d, excluding D( N0 ) and D( N0-1 ).
*
* DN (output) REAL
* d(N0), the last value of d.
*
* DNM1 (output) REAL
* d(N0-1).
*
* DNM2 (output) REAL
* d(N0-2).
*
* IEEE (input) LOGICAL
* Flag for IEEE or non IEEE arithmetic.
*
* =====================================================================
*
* .. Parameter ..
*
*
* @param i0
* @param n0
* @param z
* @param _z_offset
* @param pp
* @param tau
* @param dmin
* @param dmin1
* @param dmin2
* @param dn
* @param dnm1
* @param dnm2
* @param ieee
*
*/
abstract public void slasq5(int i0, int n0, float[] z, int _z_offset, int pp, float tau, org.netlib.util.floatW dmin, org.netlib.util.floatW dmin1, org.netlib.util.floatW dmin2, org.netlib.util.floatW dn, org.netlib.util.floatW dnm1, org.netlib.util.floatW dnm2, boolean ieee);
/**
*
* ..
*
* Purpose
* =======
*
* SLASQ6 computes one dqd (shift equal to zero) transform in
* ping-pong form, with protection against underflow and overflow.
*
* Arguments
* =========
*
* I0 (input) INTEGER
* First index.
*
* N0 (input) INTEGER
* Last index.
*
* Z (input) REAL array, dimension ( 4*N )
* Z holds the qd array. EMIN is stored in Z(4*N0) to avoid
* an extra argument.
*
* PP (input) INTEGER
* PP=0 for ping, PP=1 for pong.
*
* DMIN (output) REAL
* Minimum value of d.
*
* DMIN1 (output) REAL
* Minimum value of d, excluding D( N0 ).
*
* DMIN2 (output) REAL
* Minimum value of d, excluding D( N0 ) and D( N0-1 ).
*
* DN (output) REAL
* d(N0), the last value of d.
*
* DNM1 (output) REAL
* d(N0-1).
*
* DNM2 (output) REAL
* d(N0-2).
*
* =====================================================================
*
* .. Parameter ..
*
*
* @param i0
* @param n0
* @param z
* @param pp
* @param dmin
* @param dmin1
* @param dmin2
* @param dn
* @param dnm1
* @param dnm2
*
*/
abstract public void slasq6(int i0, int n0, float[] z, int pp, org.netlib.util.floatW dmin, org.netlib.util.floatW dmin1, org.netlib.util.floatW dmin2, org.netlib.util.floatW dn, org.netlib.util.floatW dnm1, org.netlib.util.floatW dnm2);
/**
*
* ..
*
* Purpose
* =======
*
* SLASQ6 computes one dqd (shift equal to zero) transform in
* ping-pong form, with protection against underflow and overflow.
*
* Arguments
* =========
*
* I0 (input) INTEGER
* First index.
*
* N0 (input) INTEGER
* Last index.
*
* Z (input) REAL array, dimension ( 4*N )
* Z holds the qd array. EMIN is stored in Z(4*N0) to avoid
* an extra argument.
*
* PP (input) INTEGER
* PP=0 for ping, PP=1 for pong.
*
* DMIN (output) REAL
* Minimum value of d.
*
* DMIN1 (output) REAL
* Minimum value of d, excluding D( N0 ).
*
* DMIN2 (output) REAL
* Minimum value of d, excluding D( N0 ) and D( N0-1 ).
*
* DN (output) REAL
* d(N0), the last value of d.
*
* DNM1 (output) REAL
* d(N0-1).
*
* DNM2 (output) REAL
* d(N0-2).
*
* =====================================================================
*
* .. Parameter ..
*
*
* @param i0
* @param n0
* @param z
* @param _z_offset
* @param pp
* @param dmin
* @param dmin1
* @param dmin2
* @param dn
* @param dnm1
* @param dnm2
*
*/
abstract public void slasq6(int i0, int n0, float[] z, int _z_offset, int pp, org.netlib.util.floatW dmin, org.netlib.util.floatW dmin1, org.netlib.util.floatW dmin2, org.netlib.util.floatW dn, org.netlib.util.floatW dnm1, org.netlib.util.floatW dnm2);
/**
*
* ..
*
* Purpose
* =======
*
* SLASR applies a sequence of plane rotations to a real matrix A,
* from either the left or the right.
*
* When SIDE = 'L', the transformation takes the form
*
* A := P*A
*
* and when SIDE = 'R', the transformation takes the form
*
* A := A*P**T
*
* where P is an orthogonal matrix consisting of a sequence of z plane
* rotations, with z = M when SIDE = 'L' and z = N when SIDE = 'R',
* and P**T is the transpose of P.
*
* When DIRECT = 'F' (Forward sequence), then
*
* P = P(z-1) * ... * P(2) * P(1)
*
* and when DIRECT = 'B' (Backward sequence), then
*
* P = P(1) * P(2) * ... * P(z-1)
*
* where P(k) is a plane rotation matrix defined by the 2-by-2 rotation
*
* R(k) = ( c(k) s(k) )
* = ( -s(k) c(k) ).
*
* When PIVOT = 'V' (Variable pivot), the rotation is performed
* for the plane (k,k+1), i.e., P(k) has the form
*
* P(k) = ( 1 )
* ( ... )
* ( 1 )
* ( c(k) s(k) )
* ( -s(k) c(k) )
* ( 1 )
* ( ... )
* ( 1 )
*
* where R(k) appears as a rank-2 modification to the identity matrix in
* rows and columns k and k+1.
*
* When PIVOT = 'T' (Top pivot), the rotation is performed for the
* plane (1,k+1), so P(k) has the form
*
* P(k) = ( c(k) s(k) )
* ( 1 )
* ( ... )
* ( 1 )
* ( -s(k) c(k) )
* ( 1 )
* ( ... )
* ( 1 )
*
* where R(k) appears in rows and columns 1 and k+1.
*
* Similarly, when PIVOT = 'B' (Bottom pivot), the rotation is
* performed for the plane (k,z), giving P(k) the form
*
* P(k) = ( 1 )
* ( ... )
* ( 1 )
* ( c(k) s(k) )
* ( 1 )
* ( ... )
* ( 1 )
* ( -s(k) c(k) )
*
* where R(k) appears in rows and columns k and z. The rotations are
* performed without ever forming P(k) explicitly.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* Specifies whether the plane rotation matrix P is applied to
* A on the left or the right.
* = 'L': Left, compute A := P*A
* = 'R': Right, compute A:= A*P**T
*
* PIVOT (input) CHARACTER*1
* Specifies the plane for which P(k) is a plane rotation
* matrix.
* = 'V': Variable pivot, the plane (k,k+1)
* = 'T': Top pivot, the plane (1,k+1)
* = 'B': Bottom pivot, the plane (k,z)
*
* DIRECT (input) CHARACTER*1
* Specifies whether P is a forward or backward sequence of
* plane rotations.
* = 'F': Forward, P = P(z-1)*...*P(2)*P(1)
* = 'B': Backward, P = P(1)*P(2)*...*P(z-1)
*
* M (input) INTEGER
* The number of rows of the matrix A. If m <= 1, an immediate
* return is effected.
*
* N (input) INTEGER
* The number of columns of the matrix A. If n <= 1, an
* immediate return is effected.
*
* C (input) REAL array, dimension
* (M-1) if SIDE = 'L'
* (N-1) if SIDE = 'R'
* The cosines c(k) of the plane rotations.
*
* S (input) REAL array, dimension
* (M-1) if SIDE = 'L'
* (N-1) if SIDE = 'R'
* The sines s(k) of the plane rotations. The 2-by-2 plane
* rotation part of the matrix P(k), R(k), has the form
* R(k) = ( c(k) s(k) )
* ( -s(k) c(k) ).
*
* A (input/output) REAL array, dimension (LDA,N)
* The M-by-N matrix A. On exit, A is overwritten by P*A if
* SIDE = 'R' or by A*P**T if SIDE = 'L'.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param pivot
* @param direct
* @param m
* @param n
* @param c
* @param s
* @param a
* @param lda
*
*/
abstract public void slasr(java.lang.String side, java.lang.String pivot, java.lang.String direct, int m, int n, float[] c, float[] s, float[] a, int lda);
/**
*
* ..
*
* Purpose
* =======
*
* SLASR applies a sequence of plane rotations to a real matrix A,
* from either the left or the right.
*
* When SIDE = 'L', the transformation takes the form
*
* A := P*A
*
* and when SIDE = 'R', the transformation takes the form
*
* A := A*P**T
*
* where P is an orthogonal matrix consisting of a sequence of z plane
* rotations, with z = M when SIDE = 'L' and z = N when SIDE = 'R',
* and P**T is the transpose of P.
*
* When DIRECT = 'F' (Forward sequence), then
*
* P = P(z-1) * ... * P(2) * P(1)
*
* and when DIRECT = 'B' (Backward sequence), then
*
* P = P(1) * P(2) * ... * P(z-1)
*
* where P(k) is a plane rotation matrix defined by the 2-by-2 rotation
*
* R(k) = ( c(k) s(k) )
* = ( -s(k) c(k) ).
*
* When PIVOT = 'V' (Variable pivot), the rotation is performed
* for the plane (k,k+1), i.e., P(k) has the form
*
* P(k) = ( 1 )
* ( ... )
* ( 1 )
* ( c(k) s(k) )
* ( -s(k) c(k) )
* ( 1 )
* ( ... )
* ( 1 )
*
* where R(k) appears as a rank-2 modification to the identity matrix in
* rows and columns k and k+1.
*
* When PIVOT = 'T' (Top pivot), the rotation is performed for the
* plane (1,k+1), so P(k) has the form
*
* P(k) = ( c(k) s(k) )
* ( 1 )
* ( ... )
* ( 1 )
* ( -s(k) c(k) )
* ( 1 )
* ( ... )
* ( 1 )
*
* where R(k) appears in rows and columns 1 and k+1.
*
* Similarly, when PIVOT = 'B' (Bottom pivot), the rotation is
* performed for the plane (k,z), giving P(k) the form
*
* P(k) = ( 1 )
* ( ... )
* ( 1 )
* ( c(k) s(k) )
* ( 1 )
* ( ... )
* ( 1 )
* ( -s(k) c(k) )
*
* where R(k) appears in rows and columns k and z. The rotations are
* performed without ever forming P(k) explicitly.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* Specifies whether the plane rotation matrix P is applied to
* A on the left or the right.
* = 'L': Left, compute A := P*A
* = 'R': Right, compute A:= A*P**T
*
* PIVOT (input) CHARACTER*1
* Specifies the plane for which P(k) is a plane rotation
* matrix.
* = 'V': Variable pivot, the plane (k,k+1)
* = 'T': Top pivot, the plane (1,k+1)
* = 'B': Bottom pivot, the plane (k,z)
*
* DIRECT (input) CHARACTER*1
* Specifies whether P is a forward or backward sequence of
* plane rotations.
* = 'F': Forward, P = P(z-1)*...*P(2)*P(1)
* = 'B': Backward, P = P(1)*P(2)*...*P(z-1)
*
* M (input) INTEGER
* The number of rows of the matrix A. If m <= 1, an immediate
* return is effected.
*
* N (input) INTEGER
* The number of columns of the matrix A. If n <= 1, an
* immediate return is effected.
*
* C (input) REAL array, dimension
* (M-1) if SIDE = 'L'
* (N-1) if SIDE = 'R'
* The cosines c(k) of the plane rotations.
*
* S (input) REAL array, dimension
* (M-1) if SIDE = 'L'
* (N-1) if SIDE = 'R'
* The sines s(k) of the plane rotations. The 2-by-2 plane
* rotation part of the matrix P(k), R(k), has the form
* R(k) = ( c(k) s(k) )
* ( -s(k) c(k) ).
*
* A (input/output) REAL array, dimension (LDA,N)
* The M-by-N matrix A. On exit, A is overwritten by P*A if
* SIDE = 'R' or by A*P**T if SIDE = 'L'.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param pivot
* @param direct
* @param m
* @param n
* @param c
* @param _c_offset
* @param s
* @param _s_offset
* @param a
* @param _a_offset
* @param lda
*
*/
abstract public void slasr(java.lang.String side, java.lang.String pivot, java.lang.String direct, int m, int n, float[] c, int _c_offset, float[] s, int _s_offset, float[] a, int _a_offset, int lda);
/**
*
* ..
*
* Purpose
* =======
*
* Sort the numbers in D in increasing order (if ID = 'I') or
* in decreasing order (if ID = 'D' ).
*
* Use Quick Sort, reverting to Insertion sort on arrays of
* size <= 20. Dimension of STACK limits N to about 2**32.
*
* Arguments
* =========
*
* ID (input) CHARACTER*1
* = 'I': sort D in increasing order;
* = 'D': sort D in decreasing order.
*
* N (input) INTEGER
* The length of the array D.
*
* D (input/output) REAL array, dimension (N)
* On entry, the array to be sorted.
* On exit, D has been sorted into increasing order
* (D(1) <= ... <= D(N) ) or into decreasing order
* (D(1) >= ... >= D(N) ), depending on ID.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param id
* @param n
* @param d
* @param info
*
*/
abstract public void slasrt(java.lang.String id, int n, float[] d, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* Sort the numbers in D in increasing order (if ID = 'I') or
* in decreasing order (if ID = 'D' ).
*
* Use Quick Sort, reverting to Insertion sort on arrays of
* size <= 20. Dimension of STACK limits N to about 2**32.
*
* Arguments
* =========
*
* ID (input) CHARACTER*1
* = 'I': sort D in increasing order;
* = 'D': sort D in decreasing order.
*
* N (input) INTEGER
* The length of the array D.
*
* D (input/output) REAL array, dimension (N)
* On entry, the array to be sorted.
* On exit, D has been sorted into increasing order
* (D(1) <= ... <= D(N) ) or into decreasing order
* (D(1) >= ... >= D(N) ), depending on ID.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param id
* @param n
* @param d
* @param _d_offset
* @param info
*
*/
abstract public void slasrt(java.lang.String id, int n, float[] d, int _d_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLASSQ returns the values scl and smsq such that
*
* ( scl**2 )*smsq = x( 1 )**2 +...+ x( n )**2 + ( scale**2 )*sumsq,
*
* where x( i ) = X( 1 + ( i - 1 )*INCX ). The value of sumsq is
* assumed to be non-negative and scl returns the value
*
* scl = max( scale, abs( x( i ) ) ).
*
* scale and sumsq must be supplied in SCALE and SUMSQ and
* scl and smsq are overwritten on SCALE and SUMSQ respectively.
*
* The routine makes only one pass through the vector x.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of elements to be used from the vector X.
*
* X (input) REAL array, dimension (N)
* The vector for which a scaled sum of squares is computed.
* x( i ) = X( 1 + ( i - 1 )*INCX ), 1 <= i <= n.
*
* INCX (input) INTEGER
* The increment between successive values of the vector X.
* INCX > 0.
*
* SCALE (input/output) REAL
* On entry, the value scale in the equation above.
* On exit, SCALE is overwritten with scl , the scaling factor
* for the sum of squares.
*
* SUMSQ (input/output) REAL
* On entry, the value sumsq in the equation above.
* On exit, SUMSQ is overwritten with smsq , the basic sum of
* squares from which scl has been factored out.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param x
* @param incx
* @param scale
* @param sumsq
*
*/
abstract public void slassq(int n, float[] x, int incx, org.netlib.util.floatW scale, org.netlib.util.floatW sumsq);
/**
*
* ..
*
* Purpose
* =======
*
* SLASSQ returns the values scl and smsq such that
*
* ( scl**2 )*smsq = x( 1 )**2 +...+ x( n )**2 + ( scale**2 )*sumsq,
*
* where x( i ) = X( 1 + ( i - 1 )*INCX ). The value of sumsq is
* assumed to be non-negative and scl returns the value
*
* scl = max( scale, abs( x( i ) ) ).
*
* scale and sumsq must be supplied in SCALE and SUMSQ and
* scl and smsq are overwritten on SCALE and SUMSQ respectively.
*
* The routine makes only one pass through the vector x.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of elements to be used from the vector X.
*
* X (input) REAL array, dimension (N)
* The vector for which a scaled sum of squares is computed.
* x( i ) = X( 1 + ( i - 1 )*INCX ), 1 <= i <= n.
*
* INCX (input) INTEGER
* The increment between successive values of the vector X.
* INCX > 0.
*
* SCALE (input/output) REAL
* On entry, the value scale in the equation above.
* On exit, SCALE is overwritten with scl , the scaling factor
* for the sum of squares.
*
* SUMSQ (input/output) REAL
* On entry, the value sumsq in the equation above.
* On exit, SUMSQ is overwritten with smsq , the basic sum of
* squares from which scl has been factored out.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param x
* @param _x_offset
* @param incx
* @param scale
* @param sumsq
*
*/
abstract public void slassq(int n, float[] x, int _x_offset, int incx, org.netlib.util.floatW scale, org.netlib.util.floatW sumsq);
/**
*
* ..
*
* Purpose
* =======
*
* SLASV2 computes the singular value decomposition of a 2-by-2
* triangular matrix
* [ F G ]
* [ 0 H ].
* On return, abs(SSMAX) is the larger singular value, abs(SSMIN) is the
* smaller singular value, and (CSL,SNL) and (CSR,SNR) are the left and
* right singular vectors for abs(SSMAX), giving the decomposition
*
* [ CSL SNL ] [ F G ] [ CSR -SNR ] = [ SSMAX 0 ]
* [-SNL CSL ] [ 0 H ] [ SNR CSR ] [ 0 SSMIN ].
*
* Arguments
* =========
*
* F (input) REAL
* The (1,1) element of the 2-by-2 matrix.
*
* G (input) REAL
* The (1,2) element of the 2-by-2 matrix.
*
* H (input) REAL
* The (2,2) element of the 2-by-2 matrix.
*
* SSMIN (output) REAL
* abs(SSMIN) is the smaller singular value.
*
* SSMAX (output) REAL
* abs(SSMAX) is the larger singular value.
*
* SNL (output) REAL
* CSL (output) REAL
* The vector (CSL, SNL) is a unit left singular vector for the
* singular value abs(SSMAX).
*
* SNR (output) REAL
* CSR (output) REAL
* The vector (CSR, SNR) is a unit right singular vector for the
* singular value abs(SSMAX).
*
* Further Details
* ===============
*
* Any input parameter may be aliased with any output parameter.
*
* Barring over/underflow and assuming a guard digit in subtraction, all
* output quantities are correct to within a few units in the last
* place (ulps).
*
* In IEEE arithmetic, the code works correctly if one matrix element is
* infinite.
*
* Overflow will not occur unless the largest singular value itself
* overflows or is within a few ulps of overflow. (On machines with
* partial overflow, like the Cray, overflow may occur if the largest
* singular value is within a factor of 2 of overflow.)
*
* Underflow is harmless if underflow is gradual. Otherwise, results
* may correspond to a matrix modified by perturbations of size near
* the underflow threshold.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param f
* @param g
* @param h
* @param ssmin
* @param ssmax
* @param snr
* @param csr
* @param snl
* @param csl
*
*/
abstract public void slasv2(float f, float g, float h, org.netlib.util.floatW ssmin, org.netlib.util.floatW ssmax, org.netlib.util.floatW snr, org.netlib.util.floatW csr, org.netlib.util.floatW snl, org.netlib.util.floatW csl);
/**
*
* ..
*
* Purpose
* =======
*
* SLASWP performs a series of row interchanges on the matrix A.
* One row interchange is initiated for each of rows K1 through K2 of A.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of columns of the matrix A.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the matrix of column dimension N to which the row
* interchanges will be applied.
* On exit, the permuted matrix.
*
* LDA (input) INTEGER
* The leading dimension of the array A.
*
* K1 (input) INTEGER
* The first element of IPIV for which a row interchange will
* be done.
*
* K2 (input) INTEGER
* The last element of IPIV for which a row interchange will
* be done.
*
* IPIV (input) INTEGER array, dimension (K2*abs(INCX))
* The vector of pivot indices. Only the elements in positions
* K1 through K2 of IPIV are accessed.
* IPIV(K) = L implies rows K and L are to be interchanged.
*
* INCX (input) INTEGER
* The increment between successive values of IPIV. If IPIV
* is negative, the pivots are applied in reverse order.
*
* Further Details
* ===============
*
* Modified by
* R. C. Whaley, Computer Science Dept., Univ. of Tenn., Knoxville, USA
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n
* @param a
* @param lda
* @param k1
* @param k2
* @param ipiv
* @param incx
*
*/
abstract public void slaswp(int n, float[] a, int lda, int k1, int k2, int[] ipiv, int incx);
/**
*
* ..
*
* Purpose
* =======
*
* SLASWP performs a series of row interchanges on the matrix A.
* One row interchange is initiated for each of rows K1 through K2 of A.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of columns of the matrix A.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the matrix of column dimension N to which the row
* interchanges will be applied.
* On exit, the permuted matrix.
*
* LDA (input) INTEGER
* The leading dimension of the array A.
*
* K1 (input) INTEGER
* The first element of IPIV for which a row interchange will
* be done.
*
* K2 (input) INTEGER
* The last element of IPIV for which a row interchange will
* be done.
*
* IPIV (input) INTEGER array, dimension (K2*abs(INCX))
* The vector of pivot indices. Only the elements in positions
* K1 through K2 of IPIV are accessed.
* IPIV(K) = L implies rows K and L are to be interchanged.
*
* INCX (input) INTEGER
* The increment between successive values of IPIV. If IPIV
* is negative, the pivots are applied in reverse order.
*
* Further Details
* ===============
*
* Modified by
* R. C. Whaley, Computer Science Dept., Univ. of Tenn., Knoxville, USA
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n
* @param a
* @param _a_offset
* @param lda
* @param k1
* @param k2
* @param ipiv
* @param _ipiv_offset
* @param incx
*
*/
abstract public void slaswp(int n, float[] a, int _a_offset, int lda, int k1, int k2, int[] ipiv, int _ipiv_offset, int incx);
/**
*
* ..
*
* Purpose
* =======
*
* SLASY2 solves for the N1 by N2 matrix X, 1 <= N1,N2 <= 2, in
*
* op(TL)*X + ISGN*X*op(TR) = SCALE*B,
*
* where TL is N1 by N1, TR is N2 by N2, B is N1 by N2, and ISGN = 1 or
* -1. op(T) = T or T', where T' denotes the transpose of T.
*
* Arguments
* =========
*
* LTRANL (input) LOGICAL
* On entry, LTRANL specifies the op(TL):
* = .FALSE., op(TL) = TL,
* = .TRUE., op(TL) = TL'.
*
* LTRANR (input) LOGICAL
* On entry, LTRANR specifies the op(TR):
* = .FALSE., op(TR) = TR,
* = .TRUE., op(TR) = TR'.
*
* ISGN (input) INTEGER
* On entry, ISGN specifies the sign of the equation
* as described before. ISGN may only be 1 or -1.
*
* N1 (input) INTEGER
* On entry, N1 specifies the order of matrix TL.
* N1 may only be 0, 1 or 2.
*
* N2 (input) INTEGER
* On entry, N2 specifies the order of matrix TR.
* N2 may only be 0, 1 or 2.
*
* TL (input) REAL array, dimension (LDTL,2)
* On entry, TL contains an N1 by N1 matrix.
*
* LDTL (input) INTEGER
* The leading dimension of the matrix TL. LDTL >= max(1,N1).
*
* TR (input) REAL array, dimension (LDTR,2)
* On entry, TR contains an N2 by N2 matrix.
*
* LDTR (input) INTEGER
* The leading dimension of the matrix TR. LDTR >= max(1,N2).
*
* B (input) REAL array, dimension (LDB,2)
* On entry, the N1 by N2 matrix B contains the right-hand
* side of the equation.
*
* LDB (input) INTEGER
* The leading dimension of the matrix B. LDB >= max(1,N1).
*
* SCALE (output) REAL
* On exit, SCALE contains the scale factor. SCALE is chosen
* less than or equal to 1 to prevent the solution overflowing.
*
* X (output) REAL array, dimension (LDX,2)
* On exit, X contains the N1 by N2 solution.
*
* LDX (input) INTEGER
* The leading dimension of the matrix X. LDX >= max(1,N1).
*
* XNORM (output) REAL
* On exit, XNORM is the infinity-norm of the solution.
*
* INFO (output) INTEGER
* On exit, INFO is set to
* 0: successful exit.
* 1: TL and TR have too close eigenvalues, so TL or
* TR is perturbed to get a nonsingular equation.
* NOTE: In the interests of speed, this routine does not
* check the inputs for errors.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param ltranl
* @param ltranr
* @param isgn
* @param n1
* @param n2
* @param tl
* @param ldtl
* @param tr
* @param ldtr
* @param b
* @param ldb
* @param scale
* @param x
* @param ldx
* @param xnorm
* @param info
*
*/
abstract public void slasy2(boolean ltranl, boolean ltranr, int isgn, int n1, int n2, float[] tl, int ldtl, float[] tr, int ldtr, float[] b, int ldb, org.netlib.util.floatW scale, float[] x, int ldx, org.netlib.util.floatW xnorm, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLASY2 solves for the N1 by N2 matrix X, 1 <= N1,N2 <= 2, in
*
* op(TL)*X + ISGN*X*op(TR) = SCALE*B,
*
* where TL is N1 by N1, TR is N2 by N2, B is N1 by N2, and ISGN = 1 or
* -1. op(T) = T or T', where T' denotes the transpose of T.
*
* Arguments
* =========
*
* LTRANL (input) LOGICAL
* On entry, LTRANL specifies the op(TL):
* = .FALSE., op(TL) = TL,
* = .TRUE., op(TL) = TL'.
*
* LTRANR (input) LOGICAL
* On entry, LTRANR specifies the op(TR):
* = .FALSE., op(TR) = TR,
* = .TRUE., op(TR) = TR'.
*
* ISGN (input) INTEGER
* On entry, ISGN specifies the sign of the equation
* as described before. ISGN may only be 1 or -1.
*
* N1 (input) INTEGER
* On entry, N1 specifies the order of matrix TL.
* N1 may only be 0, 1 or 2.
*
* N2 (input) INTEGER
* On entry, N2 specifies the order of matrix TR.
* N2 may only be 0, 1 or 2.
*
* TL (input) REAL array, dimension (LDTL,2)
* On entry, TL contains an N1 by N1 matrix.
*
* LDTL (input) INTEGER
* The leading dimension of the matrix TL. LDTL >= max(1,N1).
*
* TR (input) REAL array, dimension (LDTR,2)
* On entry, TR contains an N2 by N2 matrix.
*
* LDTR (input) INTEGER
* The leading dimension of the matrix TR. LDTR >= max(1,N2).
*
* B (input) REAL array, dimension (LDB,2)
* On entry, the N1 by N2 matrix B contains the right-hand
* side of the equation.
*
* LDB (input) INTEGER
* The leading dimension of the matrix B. LDB >= max(1,N1).
*
* SCALE (output) REAL
* On exit, SCALE contains the scale factor. SCALE is chosen
* less than or equal to 1 to prevent the solution overflowing.
*
* X (output) REAL array, dimension (LDX,2)
* On exit, X contains the N1 by N2 solution.
*
* LDX (input) INTEGER
* The leading dimension of the matrix X. LDX >= max(1,N1).
*
* XNORM (output) REAL
* On exit, XNORM is the infinity-norm of the solution.
*
* INFO (output) INTEGER
* On exit, INFO is set to
* 0: successful exit.
* 1: TL and TR have too close eigenvalues, so TL or
* TR is perturbed to get a nonsingular equation.
* NOTE: In the interests of speed, this routine does not
* check the inputs for errors.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param ltranl
* @param ltranr
* @param isgn
* @param n1
* @param n2
* @param tl
* @param _tl_offset
* @param ldtl
* @param tr
* @param _tr_offset
* @param ldtr
* @param b
* @param _b_offset
* @param ldb
* @param scale
* @param x
* @param _x_offset
* @param ldx
* @param xnorm
* @param info
*
*/
abstract public void slasy2(boolean ltranl, boolean ltranr, int isgn, int n1, int n2, float[] tl, int _tl_offset, int ldtl, float[] tr, int _tr_offset, int ldtr, float[] b, int _b_offset, int ldb, org.netlib.util.floatW scale, float[] x, int _x_offset, int ldx, org.netlib.util.floatW xnorm, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLASYF computes a partial factorization of a real symmetric matrix A
* using the Bunch-Kaufman diagonal pivoting method. The partial
* factorization has the form:
*
* A = ( I U12 ) ( A11 0 ) ( I 0 ) if UPLO = 'U', or:
* ( 0 U22 ) ( 0 D ) ( U12' U22' )
*
* A = ( L11 0 ) ( D 0 ) ( L11' L21' ) if UPLO = 'L'
* ( L21 I ) ( 0 A22 ) ( 0 I )
*
* where the order of D is at most NB. The actual order is returned in
* the argument KB, and is either NB or NB-1, or N if N <= NB.
*
* SLASYF is an auxiliary routine called by SSYTRF. It uses blocked code
* (calling Level 3 BLAS) to update the submatrix A11 (if UPLO = 'U') or
* A22 (if UPLO = 'L').
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored:
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NB (input) INTEGER
* The maximum number of columns of the matrix A that should be
* factored. NB should be at least 2 to allow for 2-by-2 pivot
* blocks.
*
* KB (output) INTEGER
* The number of columns of A that were actually factored.
* KB is either NB-1 or NB, or N if N <= NB.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* n-by-n upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading n-by-n lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
* On exit, A contains details of the partial factorization.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (output) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D.
* If UPLO = 'U', only the last KB elements of IPIV are set;
* if UPLO = 'L', only the first KB elements are set.
*
* If IPIV(k) > 0, then rows and columns k and IPIV(k) were
* interchanged and D(k,k) is a 1-by-1 diagonal block.
* If UPLO = 'U' and IPIV(k) = IPIV(k-1) < 0, then rows and
* columns k-1 and -IPIV(k) were interchanged and D(k-1:k,k-1:k)
* is a 2-by-2 diagonal block. If UPLO = 'L' and IPIV(k) =
* IPIV(k+1) < 0, then rows and columns k+1 and -IPIV(k) were
* interchanged and D(k:k+1,k:k+1) is a 2-by-2 diagonal block.
*
* W (workspace) REAL array, dimension (LDW,NB)
*
* LDW (input) INTEGER
* The leading dimension of the array W. LDW >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* > 0: if INFO = k, D(k,k) is exactly zero. The factorization
* has been completed, but the block diagonal matrix D is
* exactly singular.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nb
* @param kb
* @param a
* @param lda
* @param ipiv
* @param w
* @param ldw
* @param info
*
*/
abstract public void slasyf(java.lang.String uplo, int n, int nb, org.netlib.util.intW kb, float[] a, int lda, int[] ipiv, float[] w, int ldw, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLASYF computes a partial factorization of a real symmetric matrix A
* using the Bunch-Kaufman diagonal pivoting method. The partial
* factorization has the form:
*
* A = ( I U12 ) ( A11 0 ) ( I 0 ) if UPLO = 'U', or:
* ( 0 U22 ) ( 0 D ) ( U12' U22' )
*
* A = ( L11 0 ) ( D 0 ) ( L11' L21' ) if UPLO = 'L'
* ( L21 I ) ( 0 A22 ) ( 0 I )
*
* where the order of D is at most NB. The actual order is returned in
* the argument KB, and is either NB or NB-1, or N if N <= NB.
*
* SLASYF is an auxiliary routine called by SSYTRF. It uses blocked code
* (calling Level 3 BLAS) to update the submatrix A11 (if UPLO = 'U') or
* A22 (if UPLO = 'L').
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored:
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NB (input) INTEGER
* The maximum number of columns of the matrix A that should be
* factored. NB should be at least 2 to allow for 2-by-2 pivot
* blocks.
*
* KB (output) INTEGER
* The number of columns of A that were actually factored.
* KB is either NB-1 or NB, or N if N <= NB.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* n-by-n upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading n-by-n lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
* On exit, A contains details of the partial factorization.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (output) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D.
* If UPLO = 'U', only the last KB elements of IPIV are set;
* if UPLO = 'L', only the first KB elements are set.
*
* If IPIV(k) > 0, then rows and columns k and IPIV(k) were
* interchanged and D(k,k) is a 1-by-1 diagonal block.
* If UPLO = 'U' and IPIV(k) = IPIV(k-1) < 0, then rows and
* columns k-1 and -IPIV(k) were interchanged and D(k-1:k,k-1:k)
* is a 2-by-2 diagonal block. If UPLO = 'L' and IPIV(k) =
* IPIV(k+1) < 0, then rows and columns k+1 and -IPIV(k) were
* interchanged and D(k:k+1,k:k+1) is a 2-by-2 diagonal block.
*
* W (workspace) REAL array, dimension (LDW,NB)
*
* LDW (input) INTEGER
* The leading dimension of the array W. LDW >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* > 0: if INFO = k, D(k,k) is exactly zero. The factorization
* has been completed, but the block diagonal matrix D is
* exactly singular.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nb
* @param kb
* @param a
* @param _a_offset
* @param lda
* @param ipiv
* @param _ipiv_offset
* @param w
* @param _w_offset
* @param ldw
* @param info
*
*/
abstract public void slasyf(java.lang.String uplo, int n, int nb, org.netlib.util.intW kb, float[] a, int _a_offset, int lda, int[] ipiv, int _ipiv_offset, float[] w, int _w_offset, int ldw, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLATBS solves one of the triangular systems
*
* A *x = s*b or A'*x = s*b
*
* with scaling to prevent overflow, where A is an upper or lower
* triangular band matrix. Here A' denotes the transpose of A, x and b
* are n-element vectors, and s is a scaling factor, usually less than
* or equal to 1, chosen so that the components of x will be less than
* the overflow threshold. If the unscaled problem will not cause
* overflow, the Level 2 BLAS routine STBSV is called. If the matrix A
* is singular (A(j,j) = 0 for some j), then s is set to 0 and a
* non-trivial solution to A*x = 0 is returned.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the matrix A is upper or lower triangular.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* TRANS (input) CHARACTER*1
* Specifies the operation applied to A.
* = 'N': Solve A * x = s*b (No transpose)
* = 'T': Solve A'* x = s*b (Transpose)
* = 'C': Solve A'* x = s*b (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* Specifies whether or not the matrix A is unit triangular.
* = 'N': Non-unit triangular
* = 'U': Unit triangular
*
* NORMIN (input) CHARACTER*1
* Specifies whether CNORM has been set or not.
* = 'Y': CNORM contains the column norms on entry
* = 'N': CNORM is not set on entry. On exit, the norms will
* be computed and stored in CNORM.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of subdiagonals or superdiagonals in the
* triangular matrix A. KD >= 0.
*
* AB (input) REAL array, dimension (LDAB,N)
* The upper or lower triangular band matrix A, stored in the
* first KD+1 rows of the array. The j-th column of A is stored
* in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* X (input/output) REAL array, dimension (N)
* On entry, the right hand side b of the triangular system.
* On exit, X is overwritten by the solution vector x.
*
* SCALE (output) REAL
* The scaling factor s for the triangular system
* A * x = s*b or A'* x = s*b.
* If SCALE = 0, the matrix A is singular or badly scaled, and
* the vector x is an exact or approximate solution to A*x = 0.
*
* CNORM (input or output) REAL array, dimension (N)
*
* If NORMIN = 'Y', CNORM is an input argument and CNORM(j)
* contains the norm of the off-diagonal part of the j-th column
* of A. If TRANS = 'N', CNORM(j) must be greater than or equal
* to the infinity-norm, and if TRANS = 'T' or 'C', CNORM(j)
* must be greater than or equal to the 1-norm.
*
* If NORMIN = 'N', CNORM is an output argument and CNORM(j)
* returns the 1-norm of the offdiagonal part of the j-th column
* of A.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
*
* Further Details
* ======= =======
*
* A rough bound on x is computed; if that is less than overflow, STBSV
* is called, otherwise, specific code is used which checks for possible
* overflow or divide-by-zero at every operation.
*
* A columnwise scheme is used for solving A*x = b. The basic algorithm
* if A is lower triangular is
*
* x[1:n] := b[1:n]
* for j = 1, ..., n
* x(j) := x(j) / A(j,j)
* x[j+1:n] := x[j+1:n] - x(j) * A[j+1:n,j]
* end
*
* Define bounds on the components of x after j iterations of the loop:
* M(j) = bound on x[1:j]
* G(j) = bound on x[j+1:n]
* Initially, let M(0) = 0 and G(0) = max{x(i), i=1,...,n}.
*
* Then for iteration j+1 we have
* M(j+1) <= G(j) / | A(j+1,j+1) |
* G(j+1) <= G(j) + M(j+1) * | A[j+2:n,j+1] |
* <= G(j) ( 1 + CNORM(j+1) / | A(j+1,j+1) | )
*
* where CNORM(j+1) is greater than or equal to the infinity-norm of
* column j+1 of A, not counting the diagonal. Hence
*
* G(j) <= G(0) product ( 1 + CNORM(i) / | A(i,i) | )
* 1<=i<=j
* and
*
* |x(j)| <= ( G(0) / |A(j,j)| ) product ( 1 + CNORM(i) / |A(i,i)| )
* 1<=i< j
*
* Since |x(j)| <= M(j), we use the Level 2 BLAS routine STBSV if the
* reciprocal of the largest M(j), j=1,..,n, is larger than
* max(underflow, 1/overflow).
*
* The bound on x(j) is also used to determine when a step in the
* columnwise method can be performed without fear of overflow. If
* the computed bound is greater than a large constant, x is scaled to
* prevent overflow, but if the bound overflows, x is set to 0, x(j) to
* 1, and scale to 0, and a non-trivial solution to A*x = 0 is found.
*
* Similarly, a row-wise scheme is used to solve A'*x = b. The basic
* algorithm for A upper triangular is
*
* for j = 1, ..., n
* x(j) := ( b(j) - A[1:j-1,j]' * x[1:j-1] ) / A(j,j)
* end
*
* We simultaneously compute two bounds
* G(j) = bound on ( b(i) - A[1:i-1,i]' * x[1:i-1] ), 1<=i<=j
* M(j) = bound on x(i), 1<=i<=j
*
* The initial values are G(0) = 0, M(0) = max{b(i), i=1,..,n}, and we
* add the constraint G(j) >= G(j-1) and M(j) >= M(j-1) for j >= 1.
* Then the bound on x(j) is
*
* M(j) <= M(j-1) * ( 1 + CNORM(j) ) / | A(j,j) |
*
* <= M(0) * product ( ( 1 + CNORM(i) ) / |A(i,i)| )
* 1<=i<=j
*
* and we can safely call STBSV if 1/M(n) and 1/G(n) are both greater
* than max(underflow, 1/overflow).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param normin
* @param n
* @param kd
* @param ab
* @param ldab
* @param x
* @param scale
* @param cnorm
* @param info
*
*/
abstract public void slatbs(java.lang.String uplo, java.lang.String trans, java.lang.String diag, java.lang.String normin, int n, int kd, float[] ab, int ldab, float[] x, org.netlib.util.floatW scale, float[] cnorm, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLATBS solves one of the triangular systems
*
* A *x = s*b or A'*x = s*b
*
* with scaling to prevent overflow, where A is an upper or lower
* triangular band matrix. Here A' denotes the transpose of A, x and b
* are n-element vectors, and s is a scaling factor, usually less than
* or equal to 1, chosen so that the components of x will be less than
* the overflow threshold. If the unscaled problem will not cause
* overflow, the Level 2 BLAS routine STBSV is called. If the matrix A
* is singular (A(j,j) = 0 for some j), then s is set to 0 and a
* non-trivial solution to A*x = 0 is returned.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the matrix A is upper or lower triangular.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* TRANS (input) CHARACTER*1
* Specifies the operation applied to A.
* = 'N': Solve A * x = s*b (No transpose)
* = 'T': Solve A'* x = s*b (Transpose)
* = 'C': Solve A'* x = s*b (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* Specifies whether or not the matrix A is unit triangular.
* = 'N': Non-unit triangular
* = 'U': Unit triangular
*
* NORMIN (input) CHARACTER*1
* Specifies whether CNORM has been set or not.
* = 'Y': CNORM contains the column norms on entry
* = 'N': CNORM is not set on entry. On exit, the norms will
* be computed and stored in CNORM.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of subdiagonals or superdiagonals in the
* triangular matrix A. KD >= 0.
*
* AB (input) REAL array, dimension (LDAB,N)
* The upper or lower triangular band matrix A, stored in the
* first KD+1 rows of the array. The j-th column of A is stored
* in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* X (input/output) REAL array, dimension (N)
* On entry, the right hand side b of the triangular system.
* On exit, X is overwritten by the solution vector x.
*
* SCALE (output) REAL
* The scaling factor s for the triangular system
* A * x = s*b or A'* x = s*b.
* If SCALE = 0, the matrix A is singular or badly scaled, and
* the vector x is an exact or approximate solution to A*x = 0.
*
* CNORM (input or output) REAL array, dimension (N)
*
* If NORMIN = 'Y', CNORM is an input argument and CNORM(j)
* contains the norm of the off-diagonal part of the j-th column
* of A. If TRANS = 'N', CNORM(j) must be greater than or equal
* to the infinity-norm, and if TRANS = 'T' or 'C', CNORM(j)
* must be greater than or equal to the 1-norm.
*
* If NORMIN = 'N', CNORM is an output argument and CNORM(j)
* returns the 1-norm of the offdiagonal part of the j-th column
* of A.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
*
* Further Details
* ======= =======
*
* A rough bound on x is computed; if that is less than overflow, STBSV
* is called, otherwise, specific code is used which checks for possible
* overflow or divide-by-zero at every operation.
*
* A columnwise scheme is used for solving A*x = b. The basic algorithm
* if A is lower triangular is
*
* x[1:n] := b[1:n]
* for j = 1, ..., n
* x(j) := x(j) / A(j,j)
* x[j+1:n] := x[j+1:n] - x(j) * A[j+1:n,j]
* end
*
* Define bounds on the components of x after j iterations of the loop:
* M(j) = bound on x[1:j]
* G(j) = bound on x[j+1:n]
* Initially, let M(0) = 0 and G(0) = max{x(i), i=1,...,n}.
*
* Then for iteration j+1 we have
* M(j+1) <= G(j) / | A(j+1,j+1) |
* G(j+1) <= G(j) + M(j+1) * | A[j+2:n,j+1] |
* <= G(j) ( 1 + CNORM(j+1) / | A(j+1,j+1) | )
*
* where CNORM(j+1) is greater than or equal to the infinity-norm of
* column j+1 of A, not counting the diagonal. Hence
*
* G(j) <= G(0) product ( 1 + CNORM(i) / | A(i,i) | )
* 1<=i<=j
* and
*
* |x(j)| <= ( G(0) / |A(j,j)| ) product ( 1 + CNORM(i) / |A(i,i)| )
* 1<=i< j
*
* Since |x(j)| <= M(j), we use the Level 2 BLAS routine STBSV if the
* reciprocal of the largest M(j), j=1,..,n, is larger than
* max(underflow, 1/overflow).
*
* The bound on x(j) is also used to determine when a step in the
* columnwise method can be performed without fear of overflow. If
* the computed bound is greater than a large constant, x is scaled to
* prevent overflow, but if the bound overflows, x is set to 0, x(j) to
* 1, and scale to 0, and a non-trivial solution to A*x = 0 is found.
*
* Similarly, a row-wise scheme is used to solve A'*x = b. The basic
* algorithm for A upper triangular is
*
* for j = 1, ..., n
* x(j) := ( b(j) - A[1:j-1,j]' * x[1:j-1] ) / A(j,j)
* end
*
* We simultaneously compute two bounds
* G(j) = bound on ( b(i) - A[1:i-1,i]' * x[1:i-1] ), 1<=i<=j
* M(j) = bound on x(i), 1<=i<=j
*
* The initial values are G(0) = 0, M(0) = max{b(i), i=1,..,n}, and we
* add the constraint G(j) >= G(j-1) and M(j) >= M(j-1) for j >= 1.
* Then the bound on x(j) is
*
* M(j) <= M(j-1) * ( 1 + CNORM(j) ) / | A(j,j) |
*
* <= M(0) * product ( ( 1 + CNORM(i) ) / |A(i,i)| )
* 1<=i<=j
*
* and we can safely call STBSV if 1/M(n) and 1/G(n) are both greater
* than max(underflow, 1/overflow).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param normin
* @param n
* @param kd
* @param ab
* @param _ab_offset
* @param ldab
* @param x
* @param _x_offset
* @param scale
* @param cnorm
* @param _cnorm_offset
* @param info
*
*/
abstract public void slatbs(java.lang.String uplo, java.lang.String trans, java.lang.String diag, java.lang.String normin, int n, int kd, float[] ab, int _ab_offset, int ldab, float[] x, int _x_offset, org.netlib.util.floatW scale, float[] cnorm, int _cnorm_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLATDF uses the LU factorization of the n-by-n matrix Z computed by
* SGETC2 and computes a contribution to the reciprocal Dif-estimate
* by solving Z * x = b for x, and choosing the r.h.s. b such that
* the norm of x is as large as possible. On entry RHS = b holds the
* contribution from earlier solved sub-systems, and on return RHS = x.
*
* The factorization of Z returned by SGETC2 has the form Z = P*L*U*Q,
* where P and Q are permutation matrices. L is lower triangular with
* unit diagonal elements and U is upper triangular.
*
* Arguments
* =========
*
* IJOB (input) INTEGER
* IJOB = 2: First compute an approximative null-vector e
* of Z using SGECON, e is normalized and solve for
* Zx = +-e - f with the sign giving the greater value
* of 2-norm(x). About 5 times as expensive as Default.
* IJOB .ne. 2: Local look ahead strategy where all entries of
* the r.h.s. b is choosen as either +1 or -1 (Default).
*
* N (input) INTEGER
* The number of columns of the matrix Z.
*
* Z (input) REAL array, dimension (LDZ, N)
* On entry, the LU part of the factorization of the n-by-n
* matrix Z computed by SGETC2: Z = P * L * U * Q
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDA >= max(1, N).
*
* RHS (input/output) REAL array, dimension N.
* On entry, RHS contains contributions from other subsystems.
* On exit, RHS contains the solution of the subsystem with
* entries acoording to the value of IJOB (see above).
*
* RDSUM (input/output) REAL
* On entry, the sum of squares of computed contributions to
* the Dif-estimate under computation by STGSYL, where the
* scaling factor RDSCAL (see below) has been factored out.
* On exit, the corresponding sum of squares updated with the
* contributions from the current sub-system.
* If TRANS = 'T' RDSUM is not touched.
* NOTE: RDSUM only makes sense when STGSY2 is called by STGSYL.
*
* RDSCAL (input/output) REAL
* On entry, scaling factor used to prevent overflow in RDSUM.
* On exit, RDSCAL is updated w.r.t. the current contributions
* in RDSUM.
* If TRANS = 'T', RDSCAL is not touched.
* NOTE: RDSCAL only makes sense when STGSY2 is called by
* STGSYL.
*
* IPIV (input) INTEGER array, dimension (N).
* The pivot indices; for 1 <= i <= N, row i of the
* matrix has been interchanged with row IPIV(i).
*
* JPIV (input) INTEGER array, dimension (N).
* The pivot indices; for 1 <= j <= N, column j of the
* matrix has been interchanged with column JPIV(j).
*
* Further Details
* ===============
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* This routine is a further developed implementation of algorithm
* BSOLVE in [1] using complete pivoting in the LU factorization.
*
* [1] Bo Kagstrom and Lars Westin,
* Generalized Schur Methods with Condition Estimators for
* Solving the Generalized Sylvester Equation, IEEE Transactions
* on Automatic Control, Vol. 34, No. 7, July 1989, pp 745-751.
*
* [2] Peter Poromaa,
* On Efficient and Robust Estimators for the Separation
* between two Regular Matrix Pairs with Applications in
* Condition Estimation. Report IMINF-95.05, Departement of
* Computing Science, Umea University, S-901 87 Umea, Sweden, 1995.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param ijob
* @param n
* @param z
* @param ldz
* @param rhs
* @param rdsum
* @param rdscal
* @param ipiv
* @param jpiv
*
*/
abstract public void slatdf(int ijob, int n, float[] z, int ldz, float[] rhs, org.netlib.util.floatW rdsum, org.netlib.util.floatW rdscal, int[] ipiv, int[] jpiv);
/**
*
* ..
*
* Purpose
* =======
*
* SLATDF uses the LU factorization of the n-by-n matrix Z computed by
* SGETC2 and computes a contribution to the reciprocal Dif-estimate
* by solving Z * x = b for x, and choosing the r.h.s. b such that
* the norm of x is as large as possible. On entry RHS = b holds the
* contribution from earlier solved sub-systems, and on return RHS = x.
*
* The factorization of Z returned by SGETC2 has the form Z = P*L*U*Q,
* where P and Q are permutation matrices. L is lower triangular with
* unit diagonal elements and U is upper triangular.
*
* Arguments
* =========
*
* IJOB (input) INTEGER
* IJOB = 2: First compute an approximative null-vector e
* of Z using SGECON, e is normalized and solve for
* Zx = +-e - f with the sign giving the greater value
* of 2-norm(x). About 5 times as expensive as Default.
* IJOB .ne. 2: Local look ahead strategy where all entries of
* the r.h.s. b is choosen as either +1 or -1 (Default).
*
* N (input) INTEGER
* The number of columns of the matrix Z.
*
* Z (input) REAL array, dimension (LDZ, N)
* On entry, the LU part of the factorization of the n-by-n
* matrix Z computed by SGETC2: Z = P * L * U * Q
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDA >= max(1, N).
*
* RHS (input/output) REAL array, dimension N.
* On entry, RHS contains contributions from other subsystems.
* On exit, RHS contains the solution of the subsystem with
* entries acoording to the value of IJOB (see above).
*
* RDSUM (input/output) REAL
* On entry, the sum of squares of computed contributions to
* the Dif-estimate under computation by STGSYL, where the
* scaling factor RDSCAL (see below) has been factored out.
* On exit, the corresponding sum of squares updated with the
* contributions from the current sub-system.
* If TRANS = 'T' RDSUM is not touched.
* NOTE: RDSUM only makes sense when STGSY2 is called by STGSYL.
*
* RDSCAL (input/output) REAL
* On entry, scaling factor used to prevent overflow in RDSUM.
* On exit, RDSCAL is updated w.r.t. the current contributions
* in RDSUM.
* If TRANS = 'T', RDSCAL is not touched.
* NOTE: RDSCAL only makes sense when STGSY2 is called by
* STGSYL.
*
* IPIV (input) INTEGER array, dimension (N).
* The pivot indices; for 1 <= i <= N, row i of the
* matrix has been interchanged with row IPIV(i).
*
* JPIV (input) INTEGER array, dimension (N).
* The pivot indices; for 1 <= j <= N, column j of the
* matrix has been interchanged with column JPIV(j).
*
* Further Details
* ===============
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* This routine is a further developed implementation of algorithm
* BSOLVE in [1] using complete pivoting in the LU factorization.
*
* [1] Bo Kagstrom and Lars Westin,
* Generalized Schur Methods with Condition Estimators for
* Solving the Generalized Sylvester Equation, IEEE Transactions
* on Automatic Control, Vol. 34, No. 7, July 1989, pp 745-751.
*
* [2] Peter Poromaa,
* On Efficient and Robust Estimators for the Separation
* between two Regular Matrix Pairs with Applications in
* Condition Estimation. Report IMINF-95.05, Departement of
* Computing Science, Umea University, S-901 87 Umea, Sweden, 1995.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param ijob
* @param n
* @param z
* @param _z_offset
* @param ldz
* @param rhs
* @param _rhs_offset
* @param rdsum
* @param rdscal
* @param ipiv
* @param _ipiv_offset
* @param jpiv
* @param _jpiv_offset
*
*/
abstract public void slatdf(int ijob, int n, float[] z, int _z_offset, int ldz, float[] rhs, int _rhs_offset, org.netlib.util.floatW rdsum, org.netlib.util.floatW rdscal, int[] ipiv, int _ipiv_offset, int[] jpiv, int _jpiv_offset);
/**
*
* ..
*
* Purpose
* =======
*
* SLATPS solves one of the triangular systems
*
* A *x = s*b or A'*x = s*b
*
* with scaling to prevent overflow, where A is an upper or lower
* triangular matrix stored in packed form. Here A' denotes the
* transpose of A, x and b are n-element vectors, and s is a scaling
* factor, usually less than or equal to 1, chosen so that the
* components of x will be less than the overflow threshold. If the
* unscaled problem will not cause overflow, the Level 2 BLAS routine
* STPSV is called. If the matrix A is singular (A(j,j) = 0 for some j),
* then s is set to 0 and a non-trivial solution to A*x = 0 is returned.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the matrix A is upper or lower triangular.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* TRANS (input) CHARACTER*1
* Specifies the operation applied to A.
* = 'N': Solve A * x = s*b (No transpose)
* = 'T': Solve A'* x = s*b (Transpose)
* = 'C': Solve A'* x = s*b (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* Specifies whether or not the matrix A is unit triangular.
* = 'N': Non-unit triangular
* = 'U': Unit triangular
*
* NORMIN (input) CHARACTER*1
* Specifies whether CNORM has been set or not.
* = 'Y': CNORM contains the column norms on entry
* = 'N': CNORM is not set on entry. On exit, the norms will
* be computed and stored in CNORM.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input) REAL array, dimension (N*(N+1)/2)
* The upper or lower triangular matrix A, packed columnwise in
* a linear array. The j-th column of A is stored in the array
* AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
*
* X (input/output) REAL array, dimension (N)
* On entry, the right hand side b of the triangular system.
* On exit, X is overwritten by the solution vector x.
*
* SCALE (output) REAL
* The scaling factor s for the triangular system
* A * x = s*b or A'* x = s*b.
* If SCALE = 0, the matrix A is singular or badly scaled, and
* the vector x is an exact or approximate solution to A*x = 0.
*
* CNORM (input or output) REAL array, dimension (N)
*
* If NORMIN = 'Y', CNORM is an input argument and CNORM(j)
* contains the norm of the off-diagonal part of the j-th column
* of A. If TRANS = 'N', CNORM(j) must be greater than or equal
* to the infinity-norm, and if TRANS = 'T' or 'C', CNORM(j)
* must be greater than or equal to the 1-norm.
*
* If NORMIN = 'N', CNORM is an output argument and CNORM(j)
* returns the 1-norm of the offdiagonal part of the j-th column
* of A.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
*
* Further Details
* ======= =======
*
* A rough bound on x is computed; if that is less than overflow, STPSV
* is called, otherwise, specific code is used which checks for possible
* overflow or divide-by-zero at every operation.
*
* A columnwise scheme is used for solving A*x = b. The basic algorithm
* if A is lower triangular is
*
* x[1:n] := b[1:n]
* for j = 1, ..., n
* x(j) := x(j) / A(j,j)
* x[j+1:n] := x[j+1:n] - x(j) * A[j+1:n,j]
* end
*
* Define bounds on the components of x after j iterations of the loop:
* M(j) = bound on x[1:j]
* G(j) = bound on x[j+1:n]
* Initially, let M(0) = 0 and G(0) = max{x(i), i=1,...,n}.
*
* Then for iteration j+1 we have
* M(j+1) <= G(j) / | A(j+1,j+1) |
* G(j+1) <= G(j) + M(j+1) * | A[j+2:n,j+1] |
* <= G(j) ( 1 + CNORM(j+1) / | A(j+1,j+1) | )
*
* where CNORM(j+1) is greater than or equal to the infinity-norm of
* column j+1 of A, not counting the diagonal. Hence
*
* G(j) <= G(0) product ( 1 + CNORM(i) / | A(i,i) | )
* 1<=i<=j
* and
*
* |x(j)| <= ( G(0) / |A(j,j)| ) product ( 1 + CNORM(i) / |A(i,i)| )
* 1<=i< j
*
* Since |x(j)| <= M(j), we use the Level 2 BLAS routine STPSV if the
* reciprocal of the largest M(j), j=1,..,n, is larger than
* max(underflow, 1/overflow).
*
* The bound on x(j) is also used to determine when a step in the
* columnwise method can be performed without fear of overflow. If
* the computed bound is greater than a large constant, x is scaled to
* prevent overflow, but if the bound overflows, x is set to 0, x(j) to
* 1, and scale to 0, and a non-trivial solution to A*x = 0 is found.
*
* Similarly, a row-wise scheme is used to solve A'*x = b. The basic
* algorithm for A upper triangular is
*
* for j = 1, ..., n
* x(j) := ( b(j) - A[1:j-1,j]' * x[1:j-1] ) / A(j,j)
* end
*
* We simultaneously compute two bounds
* G(j) = bound on ( b(i) - A[1:i-1,i]' * x[1:i-1] ), 1<=i<=j
* M(j) = bound on x(i), 1<=i<=j
*
* The initial values are G(0) = 0, M(0) = max{b(i), i=1,..,n}, and we
* add the constraint G(j) >= G(j-1) and M(j) >= M(j-1) for j >= 1.
* Then the bound on x(j) is
*
* M(j) <= M(j-1) * ( 1 + CNORM(j) ) / | A(j,j) |
*
* <= M(0) * product ( ( 1 + CNORM(i) ) / |A(i,i)| )
* 1<=i<=j
*
* and we can safely call STPSV if 1/M(n) and 1/G(n) are both greater
* than max(underflow, 1/overflow).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param normin
* @param n
* @param ap
* @param x
* @param scale
* @param cnorm
* @param info
*
*/
abstract public void slatps(java.lang.String uplo, java.lang.String trans, java.lang.String diag, java.lang.String normin, int n, float[] ap, float[] x, org.netlib.util.floatW scale, float[] cnorm, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLATPS solves one of the triangular systems
*
* A *x = s*b or A'*x = s*b
*
* with scaling to prevent overflow, where A is an upper or lower
* triangular matrix stored in packed form. Here A' denotes the
* transpose of A, x and b are n-element vectors, and s is a scaling
* factor, usually less than or equal to 1, chosen so that the
* components of x will be less than the overflow threshold. If the
* unscaled problem will not cause overflow, the Level 2 BLAS routine
* STPSV is called. If the matrix A is singular (A(j,j) = 0 for some j),
* then s is set to 0 and a non-trivial solution to A*x = 0 is returned.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the matrix A is upper or lower triangular.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* TRANS (input) CHARACTER*1
* Specifies the operation applied to A.
* = 'N': Solve A * x = s*b (No transpose)
* = 'T': Solve A'* x = s*b (Transpose)
* = 'C': Solve A'* x = s*b (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* Specifies whether or not the matrix A is unit triangular.
* = 'N': Non-unit triangular
* = 'U': Unit triangular
*
* NORMIN (input) CHARACTER*1
* Specifies whether CNORM has been set or not.
* = 'Y': CNORM contains the column norms on entry
* = 'N': CNORM is not set on entry. On exit, the norms will
* be computed and stored in CNORM.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input) REAL array, dimension (N*(N+1)/2)
* The upper or lower triangular matrix A, packed columnwise in
* a linear array. The j-th column of A is stored in the array
* AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
*
* X (input/output) REAL array, dimension (N)
* On entry, the right hand side b of the triangular system.
* On exit, X is overwritten by the solution vector x.
*
* SCALE (output) REAL
* The scaling factor s for the triangular system
* A * x = s*b or A'* x = s*b.
* If SCALE = 0, the matrix A is singular or badly scaled, and
* the vector x is an exact or approximate solution to A*x = 0.
*
* CNORM (input or output) REAL array, dimension (N)
*
* If NORMIN = 'Y', CNORM is an input argument and CNORM(j)
* contains the norm of the off-diagonal part of the j-th column
* of A. If TRANS = 'N', CNORM(j) must be greater than or equal
* to the infinity-norm, and if TRANS = 'T' or 'C', CNORM(j)
* must be greater than or equal to the 1-norm.
*
* If NORMIN = 'N', CNORM is an output argument and CNORM(j)
* returns the 1-norm of the offdiagonal part of the j-th column
* of A.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
*
* Further Details
* ======= =======
*
* A rough bound on x is computed; if that is less than overflow, STPSV
* is called, otherwise, specific code is used which checks for possible
* overflow or divide-by-zero at every operation.
*
* A columnwise scheme is used for solving A*x = b. The basic algorithm
* if A is lower triangular is
*
* x[1:n] := b[1:n]
* for j = 1, ..., n
* x(j) := x(j) / A(j,j)
* x[j+1:n] := x[j+1:n] - x(j) * A[j+1:n,j]
* end
*
* Define bounds on the components of x after j iterations of the loop:
* M(j) = bound on x[1:j]
* G(j) = bound on x[j+1:n]
* Initially, let M(0) = 0 and G(0) = max{x(i), i=1,...,n}.
*
* Then for iteration j+1 we have
* M(j+1) <= G(j) / | A(j+1,j+1) |
* G(j+1) <= G(j) + M(j+1) * | A[j+2:n,j+1] |
* <= G(j) ( 1 + CNORM(j+1) / | A(j+1,j+1) | )
*
* where CNORM(j+1) is greater than or equal to the infinity-norm of
* column j+1 of A, not counting the diagonal. Hence
*
* G(j) <= G(0) product ( 1 + CNORM(i) / | A(i,i) | )
* 1<=i<=j
* and
*
* |x(j)| <= ( G(0) / |A(j,j)| ) product ( 1 + CNORM(i) / |A(i,i)| )
* 1<=i< j
*
* Since |x(j)| <= M(j), we use the Level 2 BLAS routine STPSV if the
* reciprocal of the largest M(j), j=1,..,n, is larger than
* max(underflow, 1/overflow).
*
* The bound on x(j) is also used to determine when a step in the
* columnwise method can be performed without fear of overflow. If
* the computed bound is greater than a large constant, x is scaled to
* prevent overflow, but if the bound overflows, x is set to 0, x(j) to
* 1, and scale to 0, and a non-trivial solution to A*x = 0 is found.
*
* Similarly, a row-wise scheme is used to solve A'*x = b. The basic
* algorithm for A upper triangular is
*
* for j = 1, ..., n
* x(j) := ( b(j) - A[1:j-1,j]' * x[1:j-1] ) / A(j,j)
* end
*
* We simultaneously compute two bounds
* G(j) = bound on ( b(i) - A[1:i-1,i]' * x[1:i-1] ), 1<=i<=j
* M(j) = bound on x(i), 1<=i<=j
*
* The initial values are G(0) = 0, M(0) = max{b(i), i=1,..,n}, and we
* add the constraint G(j) >= G(j-1) and M(j) >= M(j-1) for j >= 1.
* Then the bound on x(j) is
*
* M(j) <= M(j-1) * ( 1 + CNORM(j) ) / | A(j,j) |
*
* <= M(0) * product ( ( 1 + CNORM(i) ) / |A(i,i)| )
* 1<=i<=j
*
* and we can safely call STPSV if 1/M(n) and 1/G(n) are both greater
* than max(underflow, 1/overflow).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param normin
* @param n
* @param ap
* @param _ap_offset
* @param x
* @param _x_offset
* @param scale
* @param cnorm
* @param _cnorm_offset
* @param info
*
*/
abstract public void slatps(java.lang.String uplo, java.lang.String trans, java.lang.String diag, java.lang.String normin, int n, float[] ap, int _ap_offset, float[] x, int _x_offset, org.netlib.util.floatW scale, float[] cnorm, int _cnorm_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLATRD reduces NB rows and columns of a real symmetric matrix A to
* symmetric tridiagonal form by an orthogonal similarity
* transformation Q' * A * Q, and returns the matrices V and W which are
* needed to apply the transformation to the unreduced part of A.
*
* If UPLO = 'U', SLATRD reduces the last NB rows and columns of a
* matrix, of which the upper triangle is supplied;
* if UPLO = 'L', SLATRD reduces the first NB rows and columns of a
* matrix, of which the lower triangle is supplied.
*
* This is an auxiliary routine called by SSYTRD.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored:
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A.
*
* NB (input) INTEGER
* The number of rows and columns to be reduced.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* n-by-n upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading n-by-n lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
* On exit:
* if UPLO = 'U', the last NB columns have been reduced to
* tridiagonal form, with the diagonal elements overwriting
* the diagonal elements of A; the elements above the diagonal
* with the array TAU, represent the orthogonal matrix Q as a
* product of elementary reflectors;
* if UPLO = 'L', the first NB columns have been reduced to
* tridiagonal form, with the diagonal elements overwriting
* the diagonal elements of A; the elements below the diagonal
* with the array TAU, represent the orthogonal matrix Q as a
* product of elementary reflectors.
* See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= (1,N).
*
* E (output) REAL array, dimension (N-1)
* If UPLO = 'U', E(n-nb:n-1) contains the superdiagonal
* elements of the last NB columns of the reduced matrix;
* if UPLO = 'L', E(1:nb) contains the subdiagonal elements of
* the first NB columns of the reduced matrix.
*
* TAU (output) REAL array, dimension (N-1)
* The scalar factors of the elementary reflectors, stored in
* TAU(n-nb:n-1) if UPLO = 'U', and in TAU(1:nb) if UPLO = 'L'.
* See Further Details.
*
* W (output) REAL array, dimension (LDW,NB)
* The n-by-nb matrix W required to update the unreduced part
* of A.
*
* LDW (input) INTEGER
* The leading dimension of the array W. LDW >= max(1,N).
*
* Further Details
* ===============
*
* If UPLO = 'U', the matrix Q is represented as a product of elementary
* reflectors
*
* Q = H(n) H(n-1) . . . H(n-nb+1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(i:n) = 0 and v(i-1) = 1; v(1:i-1) is stored on exit in A(1:i-1,i),
* and tau in TAU(i-1).
*
* If UPLO = 'L', the matrix Q is represented as a product of elementary
* reflectors
*
* Q = H(1) H(2) . . . H(nb).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i) = 0 and v(i+1) = 1; v(i+1:n) is stored on exit in A(i+1:n,i),
* and tau in TAU(i).
*
* The elements of the vectors v together form the n-by-nb matrix V
* which is needed, with W, to apply the transformation to the unreduced
* part of the matrix, using a symmetric rank-2k update of the form:
* A := A - V*W' - W*V'.
*
* The contents of A on exit are illustrated by the following examples
* with n = 5 and nb = 2:
*
* if UPLO = 'U': if UPLO = 'L':
*
* ( a a a v4 v5 ) ( d )
* ( a a v4 v5 ) ( 1 d )
* ( a 1 v5 ) ( v1 1 a )
* ( d 1 ) ( v1 v2 a a )
* ( d ) ( v1 v2 a a a )
*
* where d denotes a diagonal element of the reduced matrix, a denotes
* an element of the original matrix that is unchanged, and vi denotes
* an element of the vector defining H(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nb
* @param a
* @param lda
* @param e
* @param tau
* @param w
* @param ldw
*
*/
abstract public void slatrd(java.lang.String uplo, int n, int nb, float[] a, int lda, float[] e, float[] tau, float[] w, int ldw);
/**
*
* ..
*
* Purpose
* =======
*
* SLATRD reduces NB rows and columns of a real symmetric matrix A to
* symmetric tridiagonal form by an orthogonal similarity
* transformation Q' * A * Q, and returns the matrices V and W which are
* needed to apply the transformation to the unreduced part of A.
*
* If UPLO = 'U', SLATRD reduces the last NB rows and columns of a
* matrix, of which the upper triangle is supplied;
* if UPLO = 'L', SLATRD reduces the first NB rows and columns of a
* matrix, of which the lower triangle is supplied.
*
* This is an auxiliary routine called by SSYTRD.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored:
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A.
*
* NB (input) INTEGER
* The number of rows and columns to be reduced.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* n-by-n upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading n-by-n lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
* On exit:
* if UPLO = 'U', the last NB columns have been reduced to
* tridiagonal form, with the diagonal elements overwriting
* the diagonal elements of A; the elements above the diagonal
* with the array TAU, represent the orthogonal matrix Q as a
* product of elementary reflectors;
* if UPLO = 'L', the first NB columns have been reduced to
* tridiagonal form, with the diagonal elements overwriting
* the diagonal elements of A; the elements below the diagonal
* with the array TAU, represent the orthogonal matrix Q as a
* product of elementary reflectors.
* See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= (1,N).
*
* E (output) REAL array, dimension (N-1)
* If UPLO = 'U', E(n-nb:n-1) contains the superdiagonal
* elements of the last NB columns of the reduced matrix;
* if UPLO = 'L', E(1:nb) contains the subdiagonal elements of
* the first NB columns of the reduced matrix.
*
* TAU (output) REAL array, dimension (N-1)
* The scalar factors of the elementary reflectors, stored in
* TAU(n-nb:n-1) if UPLO = 'U', and in TAU(1:nb) if UPLO = 'L'.
* See Further Details.
*
* W (output) REAL array, dimension (LDW,NB)
* The n-by-nb matrix W required to update the unreduced part
* of A.
*
* LDW (input) INTEGER
* The leading dimension of the array W. LDW >= max(1,N).
*
* Further Details
* ===============
*
* If UPLO = 'U', the matrix Q is represented as a product of elementary
* reflectors
*
* Q = H(n) H(n-1) . . . H(n-nb+1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(i:n) = 0 and v(i-1) = 1; v(1:i-1) is stored on exit in A(1:i-1,i),
* and tau in TAU(i-1).
*
* If UPLO = 'L', the matrix Q is represented as a product of elementary
* reflectors
*
* Q = H(1) H(2) . . . H(nb).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i) = 0 and v(i+1) = 1; v(i+1:n) is stored on exit in A(i+1:n,i),
* and tau in TAU(i).
*
* The elements of the vectors v together form the n-by-nb matrix V
* which is needed, with W, to apply the transformation to the unreduced
* part of the matrix, using a symmetric rank-2k update of the form:
* A := A - V*W' - W*V'.
*
* The contents of A on exit are illustrated by the following examples
* with n = 5 and nb = 2:
*
* if UPLO = 'U': if UPLO = 'L':
*
* ( a a a v4 v5 ) ( d )
* ( a a v4 v5 ) ( 1 d )
* ( a 1 v5 ) ( v1 1 a )
* ( d 1 ) ( v1 v2 a a )
* ( d ) ( v1 v2 a a a )
*
* where d denotes a diagonal element of the reduced matrix, a denotes
* an element of the original matrix that is unchanged, and vi denotes
* an element of the vector defining H(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nb
* @param a
* @param _a_offset
* @param lda
* @param e
* @param _e_offset
* @param tau
* @param _tau_offset
* @param w
* @param _w_offset
* @param ldw
*
*/
abstract public void slatrd(java.lang.String uplo, int n, int nb, float[] a, int _a_offset, int lda, float[] e, int _e_offset, float[] tau, int _tau_offset, float[] w, int _w_offset, int ldw);
/**
*
* ..
*
* Purpose
* =======
*
* SLATRS solves one of the triangular systems
*
* A *x = s*b or A'*x = s*b
*
* with scaling to prevent overflow. Here A is an upper or lower
* triangular matrix, A' denotes the transpose of A, x and b are
* n-element vectors, and s is a scaling factor, usually less than
* or equal to 1, chosen so that the components of x will be less than
* the overflow threshold. If the unscaled problem will not cause
* overflow, the Level 2 BLAS routine STRSV is called. If the matrix A
* is singular (A(j,j) = 0 for some j), then s is set to 0 and a
* non-trivial solution to A*x = 0 is returned.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the matrix A is upper or lower triangular.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* TRANS (input) CHARACTER*1
* Specifies the operation applied to A.
* = 'N': Solve A * x = s*b (No transpose)
* = 'T': Solve A'* x = s*b (Transpose)
* = 'C': Solve A'* x = s*b (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* Specifies whether or not the matrix A is unit triangular.
* = 'N': Non-unit triangular
* = 'U': Unit triangular
*
* NORMIN (input) CHARACTER*1
* Specifies whether CNORM has been set or not.
* = 'Y': CNORM contains the column norms on entry
* = 'N': CNORM is not set on entry. On exit, the norms will
* be computed and stored in CNORM.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The triangular matrix A. If UPLO = 'U', the leading n by n
* upper triangular part of the array A contains the upper
* triangular matrix, and the strictly lower triangular part of
* A is not referenced. If UPLO = 'L', the leading n by n lower
* triangular part of the array A contains the lower triangular
* matrix, and the strictly upper triangular part of A is not
* referenced. If DIAG = 'U', the diagonal elements of A are
* also not referenced and are assumed to be 1.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max (1,N).
*
* X (input/output) REAL array, dimension (N)
* On entry, the right hand side b of the triangular system.
* On exit, X is overwritten by the solution vector x.
*
* SCALE (output) REAL
* The scaling factor s for the triangular system
* A * x = s*b or A'* x = s*b.
* If SCALE = 0, the matrix A is singular or badly scaled, and
* the vector x is an exact or approximate solution to A*x = 0.
*
* CNORM (input or output) REAL array, dimension (N)
*
* If NORMIN = 'Y', CNORM is an input argument and CNORM(j)
* contains the norm of the off-diagonal part of the j-th column
* of A. If TRANS = 'N', CNORM(j) must be greater than or equal
* to the infinity-norm, and if TRANS = 'T' or 'C', CNORM(j)
* must be greater than or equal to the 1-norm.
*
* If NORMIN = 'N', CNORM is an output argument and CNORM(j)
* returns the 1-norm of the offdiagonal part of the j-th column
* of A.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
*
* Further Details
* ======= =======
*
* A rough bound on x is computed; if that is less than overflow, STRSV
* is called, otherwise, specific code is used which checks for possible
* overflow or divide-by-zero at every operation.
*
* A columnwise scheme is used for solving A*x = b. The basic algorithm
* if A is lower triangular is
*
* x[1:n] := b[1:n]
* for j = 1, ..., n
* x(j) := x(j) / A(j,j)
* x[j+1:n] := x[j+1:n] - x(j) * A[j+1:n,j]
* end
*
* Define bounds on the components of x after j iterations of the loop:
* M(j) = bound on x[1:j]
* G(j) = bound on x[j+1:n]
* Initially, let M(0) = 0 and G(0) = max{x(i), i=1,...,n}.
*
* Then for iteration j+1 we have
* M(j+1) <= G(j) / | A(j+1,j+1) |
* G(j+1) <= G(j) + M(j+1) * | A[j+2:n,j+1] |
* <= G(j) ( 1 + CNORM(j+1) / | A(j+1,j+1) | )
*
* where CNORM(j+1) is greater than or equal to the infinity-norm of
* column j+1 of A, not counting the diagonal. Hence
*
* G(j) <= G(0) product ( 1 + CNORM(i) / | A(i,i) | )
* 1<=i<=j
* and
*
* |x(j)| <= ( G(0) / |A(j,j)| ) product ( 1 + CNORM(i) / |A(i,i)| )
* 1<=i< j
*
* Since |x(j)| <= M(j), we use the Level 2 BLAS routine STRSV if the
* reciprocal of the largest M(j), j=1,..,n, is larger than
* max(underflow, 1/overflow).
*
* The bound on x(j) is also used to determine when a step in the
* columnwise method can be performed without fear of overflow. If
* the computed bound is greater than a large constant, x is scaled to
* prevent overflow, but if the bound overflows, x is set to 0, x(j) to
* 1, and scale to 0, and a non-trivial solution to A*x = 0 is found.
*
* Similarly, a row-wise scheme is used to solve A'*x = b. The basic
* algorithm for A upper triangular is
*
* for j = 1, ..., n
* x(j) := ( b(j) - A[1:j-1,j]' * x[1:j-1] ) / A(j,j)
* end
*
* We simultaneously compute two bounds
* G(j) = bound on ( b(i) - A[1:i-1,i]' * x[1:i-1] ), 1<=i<=j
* M(j) = bound on x(i), 1<=i<=j
*
* The initial values are G(0) = 0, M(0) = max{b(i), i=1,..,n}, and we
* add the constraint G(j) >= G(j-1) and M(j) >= M(j-1) for j >= 1.
* Then the bound on x(j) is
*
* M(j) <= M(j-1) * ( 1 + CNORM(j) ) / | A(j,j) |
*
* <= M(0) * product ( ( 1 + CNORM(i) ) / |A(i,i)| )
* 1<=i<=j
*
* and we can safely call STRSV if 1/M(n) and 1/G(n) are both greater
* than max(underflow, 1/overflow).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param normin
* @param n
* @param a
* @param lda
* @param x
* @param scale
* @param cnorm
* @param info
*
*/
abstract public void slatrs(java.lang.String uplo, java.lang.String trans, java.lang.String diag, java.lang.String normin, int n, float[] a, int lda, float[] x, org.netlib.util.floatW scale, float[] cnorm, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLATRS solves one of the triangular systems
*
* A *x = s*b or A'*x = s*b
*
* with scaling to prevent overflow. Here A is an upper or lower
* triangular matrix, A' denotes the transpose of A, x and b are
* n-element vectors, and s is a scaling factor, usually less than
* or equal to 1, chosen so that the components of x will be less than
* the overflow threshold. If the unscaled problem will not cause
* overflow, the Level 2 BLAS routine STRSV is called. If the matrix A
* is singular (A(j,j) = 0 for some j), then s is set to 0 and a
* non-trivial solution to A*x = 0 is returned.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the matrix A is upper or lower triangular.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* TRANS (input) CHARACTER*1
* Specifies the operation applied to A.
* = 'N': Solve A * x = s*b (No transpose)
* = 'T': Solve A'* x = s*b (Transpose)
* = 'C': Solve A'* x = s*b (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* Specifies whether or not the matrix A is unit triangular.
* = 'N': Non-unit triangular
* = 'U': Unit triangular
*
* NORMIN (input) CHARACTER*1
* Specifies whether CNORM has been set or not.
* = 'Y': CNORM contains the column norms on entry
* = 'N': CNORM is not set on entry. On exit, the norms will
* be computed and stored in CNORM.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The triangular matrix A. If UPLO = 'U', the leading n by n
* upper triangular part of the array A contains the upper
* triangular matrix, and the strictly lower triangular part of
* A is not referenced. If UPLO = 'L', the leading n by n lower
* triangular part of the array A contains the lower triangular
* matrix, and the strictly upper triangular part of A is not
* referenced. If DIAG = 'U', the diagonal elements of A are
* also not referenced and are assumed to be 1.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max (1,N).
*
* X (input/output) REAL array, dimension (N)
* On entry, the right hand side b of the triangular system.
* On exit, X is overwritten by the solution vector x.
*
* SCALE (output) REAL
* The scaling factor s for the triangular system
* A * x = s*b or A'* x = s*b.
* If SCALE = 0, the matrix A is singular or badly scaled, and
* the vector x is an exact or approximate solution to A*x = 0.
*
* CNORM (input or output) REAL array, dimension (N)
*
* If NORMIN = 'Y', CNORM is an input argument and CNORM(j)
* contains the norm of the off-diagonal part of the j-th column
* of A. If TRANS = 'N', CNORM(j) must be greater than or equal
* to the infinity-norm, and if TRANS = 'T' or 'C', CNORM(j)
* must be greater than or equal to the 1-norm.
*
* If NORMIN = 'N', CNORM is an output argument and CNORM(j)
* returns the 1-norm of the offdiagonal part of the j-th column
* of A.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
*
* Further Details
* ======= =======
*
* A rough bound on x is computed; if that is less than overflow, STRSV
* is called, otherwise, specific code is used which checks for possible
* overflow or divide-by-zero at every operation.
*
* A columnwise scheme is used for solving A*x = b. The basic algorithm
* if A is lower triangular is
*
* x[1:n] := b[1:n]
* for j = 1, ..., n
* x(j) := x(j) / A(j,j)
* x[j+1:n] := x[j+1:n] - x(j) * A[j+1:n,j]
* end
*
* Define bounds on the components of x after j iterations of the loop:
* M(j) = bound on x[1:j]
* G(j) = bound on x[j+1:n]
* Initially, let M(0) = 0 and G(0) = max{x(i), i=1,...,n}.
*
* Then for iteration j+1 we have
* M(j+1) <= G(j) / | A(j+1,j+1) |
* G(j+1) <= G(j) + M(j+1) * | A[j+2:n,j+1] |
* <= G(j) ( 1 + CNORM(j+1) / | A(j+1,j+1) | )
*
* where CNORM(j+1) is greater than or equal to the infinity-norm of
* column j+1 of A, not counting the diagonal. Hence
*
* G(j) <= G(0) product ( 1 + CNORM(i) / | A(i,i) | )
* 1<=i<=j
* and
*
* |x(j)| <= ( G(0) / |A(j,j)| ) product ( 1 + CNORM(i) / |A(i,i)| )
* 1<=i< j
*
* Since |x(j)| <= M(j), we use the Level 2 BLAS routine STRSV if the
* reciprocal of the largest M(j), j=1,..,n, is larger than
* max(underflow, 1/overflow).
*
* The bound on x(j) is also used to determine when a step in the
* columnwise method can be performed without fear of overflow. If
* the computed bound is greater than a large constant, x is scaled to
* prevent overflow, but if the bound overflows, x is set to 0, x(j) to
* 1, and scale to 0, and a non-trivial solution to A*x = 0 is found.
*
* Similarly, a row-wise scheme is used to solve A'*x = b. The basic
* algorithm for A upper triangular is
*
* for j = 1, ..., n
* x(j) := ( b(j) - A[1:j-1,j]' * x[1:j-1] ) / A(j,j)
* end
*
* We simultaneously compute two bounds
* G(j) = bound on ( b(i) - A[1:i-1,i]' * x[1:i-1] ), 1<=i<=j
* M(j) = bound on x(i), 1<=i<=j
*
* The initial values are G(0) = 0, M(0) = max{b(i), i=1,..,n}, and we
* add the constraint G(j) >= G(j-1) and M(j) >= M(j-1) for j >= 1.
* Then the bound on x(j) is
*
* M(j) <= M(j-1) * ( 1 + CNORM(j) ) / | A(j,j) |
*
* <= M(0) * product ( ( 1 + CNORM(i) ) / |A(i,i)| )
* 1<=i<=j
*
* and we can safely call STRSV if 1/M(n) and 1/G(n) are both greater
* than max(underflow, 1/overflow).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param normin
* @param n
* @param a
* @param _a_offset
* @param lda
* @param x
* @param _x_offset
* @param scale
* @param cnorm
* @param _cnorm_offset
* @param info
*
*/
abstract public void slatrs(java.lang.String uplo, java.lang.String trans, java.lang.String diag, java.lang.String normin, int n, float[] a, int _a_offset, int lda, float[] x, int _x_offset, org.netlib.util.floatW scale, float[] cnorm, int _cnorm_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLATRZ factors the M-by-(M+L) real upper trapezoidal matrix
* [ A1 A2 ] = [ A(1:M,1:M) A(1:M,N-L+1:N) ] as ( R 0 ) * Z, by means
* of orthogonal transformations. Z is an (M+L)-by-(M+L) orthogonal
* matrix and, R and A1 are M-by-M upper triangular matrices.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* L (input) INTEGER
* The number of columns of the matrix A containing the
* meaningful part of the Householder vectors. N-M >= L >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the leading M-by-N upper trapezoidal part of the
* array A must contain the matrix to be factorized.
* On exit, the leading M-by-M upper triangular part of A
* contains the upper triangular matrix R, and elements N-L+1 to
* N of the first M rows of A, with the array TAU, represent the
* orthogonal matrix Z as a product of M elementary reflectors.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) REAL array, dimension (M)
* The scalar factors of the elementary reflectors.
*
* WORK (workspace) REAL array, dimension (M)
*
* Further Details
* ===============
*
* Based on contributions by
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
*
* The factorization is obtained by Householder's method. The kth
* transformation matrix, Z( k ), which is used to introduce zeros into
* the ( m - k + 1 )th row of A, is given in the form
*
* Z( k ) = ( I 0 ),
* ( 0 T( k ) )
*
* where
*
* T( k ) = I - tau*u( k )*u( k )', u( k ) = ( 1 ),
* ( 0 )
* ( z( k ) )
*
* tau is a scalar and z( k ) is an l element vector. tau and z( k )
* are chosen to annihilate the elements of the kth row of A2.
*
* The scalar tau is returned in the kth element of TAU and the vector
* u( k ) in the kth row of A2, such that the elements of z( k ) are
* in a( k, l + 1 ), ..., a( k, n ). The elements of R are returned in
* the upper triangular part of A1.
*
* Z is given by
*
* Z = Z( 1 ) * Z( 2 ) * ... * Z( m ).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param l
* @param a
* @param lda
* @param tau
* @param work
*
*/
abstract public void slatrz(int m, int n, int l, float[] a, int lda, float[] tau, float[] work);
/**
*
* ..
*
* Purpose
* =======
*
* SLATRZ factors the M-by-(M+L) real upper trapezoidal matrix
* [ A1 A2 ] = [ A(1:M,1:M) A(1:M,N-L+1:N) ] as ( R 0 ) * Z, by means
* of orthogonal transformations. Z is an (M+L)-by-(M+L) orthogonal
* matrix and, R and A1 are M-by-M upper triangular matrices.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= 0.
*
* L (input) INTEGER
* The number of columns of the matrix A containing the
* meaningful part of the Householder vectors. N-M >= L >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the leading M-by-N upper trapezoidal part of the
* array A must contain the matrix to be factorized.
* On exit, the leading M-by-M upper triangular part of A
* contains the upper triangular matrix R, and elements N-L+1 to
* N of the first M rows of A, with the array TAU, represent the
* orthogonal matrix Z as a product of M elementary reflectors.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) REAL array, dimension (M)
* The scalar factors of the elementary reflectors.
*
* WORK (workspace) REAL array, dimension (M)
*
* Further Details
* ===============
*
* Based on contributions by
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
*
* The factorization is obtained by Householder's method. The kth
* transformation matrix, Z( k ), which is used to introduce zeros into
* the ( m - k + 1 )th row of A, is given in the form
*
* Z( k ) = ( I 0 ),
* ( 0 T( k ) )
*
* where
*
* T( k ) = I - tau*u( k )*u( k )', u( k ) = ( 1 ),
* ( 0 )
* ( z( k ) )
*
* tau is a scalar and z( k ) is an l element vector. tau and z( k )
* are chosen to annihilate the elements of the kth row of A2.
*
* The scalar tau is returned in the kth element of TAU and the vector
* u( k ) in the kth row of A2, such that the elements of z( k ) are
* in a( k, l + 1 ), ..., a( k, n ). The elements of R are returned in
* the upper triangular part of A1.
*
* Z is given by
*
* Z = Z( 1 ) * Z( 2 ) * ... * Z( m ).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param l
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
*
*/
abstract public void slatrz(int m, int n, int l, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* This routine is deprecated and has been replaced by routine SORMRZ.
*
* SLATZM applies a Householder matrix generated by STZRQF to a matrix.
*
* Let P = I - tau*u*u', u = ( 1 ),
* ( v )
* where v is an (m-1) vector if SIDE = 'L', or a (n-1) vector if
* SIDE = 'R'.
*
* If SIDE equals 'L', let
* C = [ C1 ] 1
* [ C2 ] m-1
* n
* Then C is overwritten by P*C.
*
* If SIDE equals 'R', let
* C = [ C1, C2 ] m
* 1 n-1
* Then C is overwritten by C*P.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': form P * C
* = 'R': form C * P
*
* M (input) INTEGER
* The number of rows of the matrix C.
*
* N (input) INTEGER
* The number of columns of the matrix C.
*
* V (input) REAL array, dimension
* (1 + (M-1)*abs(INCV)) if SIDE = 'L'
* (1 + (N-1)*abs(INCV)) if SIDE = 'R'
* The vector v in the representation of P. V is not used
* if TAU = 0.
*
* INCV (input) INTEGER
* The increment between elements of v. INCV <> 0
*
* TAU (input) REAL
* The value tau in the representation of P.
*
* C1 (input/output) REAL array, dimension
* (LDC,N) if SIDE = 'L'
* (M,1) if SIDE = 'R'
* On entry, the n-vector C1 if SIDE = 'L', or the m-vector C1
* if SIDE = 'R'.
*
* On exit, the first row of P*C if SIDE = 'L', or the first
* column of C*P if SIDE = 'R'.
*
* C2 (input/output) REAL array, dimension
* (LDC, N) if SIDE = 'L'
* (LDC, N-1) if SIDE = 'R'
* On entry, the (m - 1) x n matrix C2 if SIDE = 'L', or the
* m x (n - 1) matrix C2 if SIDE = 'R'.
*
* On exit, rows 2:m of P*C if SIDE = 'L', or columns 2:m of C*P
* if SIDE = 'R'.
*
* LDC (input) INTEGER
* The leading dimension of the arrays C1 and C2. LDC >= (1,M).
*
* WORK (workspace) REAL array, dimension
* (N) if SIDE = 'L'
* (M) if SIDE = 'R'
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param m
* @param n
* @param v
* @param incv
* @param tau
* @param c1
* @param c2
* @param Ldc
* @param work
*
*/
abstract public void slatzm(java.lang.String side, int m, int n, float[] v, int incv, float tau, float[] c1, float[] c2, int Ldc, float[] work);
/**
*
* ..
*
* Purpose
* =======
*
* This routine is deprecated and has been replaced by routine SORMRZ.
*
* SLATZM applies a Householder matrix generated by STZRQF to a matrix.
*
* Let P = I - tau*u*u', u = ( 1 ),
* ( v )
* where v is an (m-1) vector if SIDE = 'L', or a (n-1) vector if
* SIDE = 'R'.
*
* If SIDE equals 'L', let
* C = [ C1 ] 1
* [ C2 ] m-1
* n
* Then C is overwritten by P*C.
*
* If SIDE equals 'R', let
* C = [ C1, C2 ] m
* 1 n-1
* Then C is overwritten by C*P.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': form P * C
* = 'R': form C * P
*
* M (input) INTEGER
* The number of rows of the matrix C.
*
* N (input) INTEGER
* The number of columns of the matrix C.
*
* V (input) REAL array, dimension
* (1 + (M-1)*abs(INCV)) if SIDE = 'L'
* (1 + (N-1)*abs(INCV)) if SIDE = 'R'
* The vector v in the representation of P. V is not used
* if TAU = 0.
*
* INCV (input) INTEGER
* The increment between elements of v. INCV <> 0
*
* TAU (input) REAL
* The value tau in the representation of P.
*
* C1 (input/output) REAL array, dimension
* (LDC,N) if SIDE = 'L'
* (M,1) if SIDE = 'R'
* On entry, the n-vector C1 if SIDE = 'L', or the m-vector C1
* if SIDE = 'R'.
*
* On exit, the first row of P*C if SIDE = 'L', or the first
* column of C*P if SIDE = 'R'.
*
* C2 (input/output) REAL array, dimension
* (LDC, N) if SIDE = 'L'
* (LDC, N-1) if SIDE = 'R'
* On entry, the (m - 1) x n matrix C2 if SIDE = 'L', or the
* m x (n - 1) matrix C2 if SIDE = 'R'.
*
* On exit, rows 2:m of P*C if SIDE = 'L', or columns 2:m of C*P
* if SIDE = 'R'.
*
* LDC (input) INTEGER
* The leading dimension of the arrays C1 and C2. LDC >= (1,M).
*
* WORK (workspace) REAL array, dimension
* (N) if SIDE = 'L'
* (M) if SIDE = 'R'
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param m
* @param n
* @param v
* @param _v_offset
* @param incv
* @param tau
* @param c1
* @param _c1_offset
* @param c2
* @param _c2_offset
* @param Ldc
* @param work
* @param _work_offset
*
*/
abstract public void slatzm(java.lang.String side, int m, int n, float[] v, int _v_offset, int incv, float tau, float[] c1, int _c1_offset, float[] c2, int _c2_offset, int Ldc, float[] work, int _work_offset);
/**
*
* ..
*
* Purpose
* =======
*
* SLAUU2 computes the product U * U' or L' * L, where the triangular
* factor U or L is stored in the upper or lower triangular part of
* the array A.
*
* If UPLO = 'U' or 'u' then the upper triangle of the result is stored,
* overwriting the factor U in A.
* If UPLO = 'L' or 'l' then the lower triangle of the result is stored,
* overwriting the factor L in A.
*
* This is the unblocked form of the algorithm, calling Level 2 BLAS.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the triangular factor stored in the array A
* is upper or lower triangular:
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the triangular factor U or L. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the triangular factor U or L.
* On exit, if UPLO = 'U', the upper triangle of A is
* overwritten with the upper triangle of the product U * U';
* if UPLO = 'L', the lower triangle of A is overwritten with
* the lower triangle of the product L' * L.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param lda
* @param info
*
*/
abstract public void slauu2(java.lang.String uplo, int n, float[] a, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAUU2 computes the product U * U' or L' * L, where the triangular
* factor U or L is stored in the upper or lower triangular part of
* the array A.
*
* If UPLO = 'U' or 'u' then the upper triangle of the result is stored,
* overwriting the factor U in A.
* If UPLO = 'L' or 'l' then the lower triangle of the result is stored,
* overwriting the factor L in A.
*
* This is the unblocked form of the algorithm, calling Level 2 BLAS.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the triangular factor stored in the array A
* is upper or lower triangular:
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the triangular factor U or L. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the triangular factor U or L.
* On exit, if UPLO = 'U', the upper triangle of A is
* overwritten with the upper triangle of the product U * U';
* if UPLO = 'L', the lower triangle of A is overwritten with
* the lower triangle of the product L' * L.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param info
*
*/
abstract public void slauu2(java.lang.String uplo, int n, float[] a, int _a_offset, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAUUM computes the product U * U' or L' * L, where the triangular
* factor U or L is stored in the upper or lower triangular part of
* the array A.
*
* If UPLO = 'U' or 'u' then the upper triangle of the result is stored,
* overwriting the factor U in A.
* If UPLO = 'L' or 'l' then the lower triangle of the result is stored,
* overwriting the factor L in A.
*
* This is the blocked form of the algorithm, calling Level 3 BLAS.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the triangular factor stored in the array A
* is upper or lower triangular:
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the triangular factor U or L. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the triangular factor U or L.
* On exit, if UPLO = 'U', the upper triangle of A is
* overwritten with the upper triangle of the product U * U';
* if UPLO = 'L', the lower triangle of A is overwritten with
* the lower triangle of the product L' * L.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param lda
* @param info
*
*/
abstract public void slauum(java.lang.String uplo, int n, float[] a, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAUUM computes the product U * U' or L' * L, where the triangular
* factor U or L is stored in the upper or lower triangular part of
* the array A.
*
* If UPLO = 'U' or 'u' then the upper triangle of the result is stored,
* overwriting the factor U in A.
* If UPLO = 'L' or 'l' then the lower triangle of the result is stored,
* overwriting the factor L in A.
*
* This is the blocked form of the algorithm, calling Level 3 BLAS.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the triangular factor stored in the array A
* is upper or lower triangular:
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the triangular factor U or L. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the triangular factor U or L.
* On exit, if UPLO = 'U', the upper triangle of A is
* overwritten with the upper triangle of the product U * U';
* if UPLO = 'L', the lower triangle of A is overwritten with
* the lower triangle of the product L' * L.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param info
*
*/
abstract public void slauum(java.lang.String uplo, int n, float[] a, int _a_offset, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SLAZQ3 checks for deflation, computes a shift (TAU) and calls dqds.
* In case of failure it changes shifts, and tries again until output
* is positive.
*
* Arguments
* =========
*
* I0 (input) INTEGER
* First index.
*
* N0 (input) INTEGER
* Last index.
*
* Z (input) REAL array, dimension ( 4*N )
* Z holds the qd array.
*
* PP (input) INTEGER
* PP=0 for ping, PP=1 for pong.
*
* DMIN (output) REAL
* Minimum value of d.
*
* SIGMA (output) REAL
* Sum of shifts used in current segment.
*
* DESIG (input/output) REAL
* Lower order part of SIGMA
*
* QMAX (input) REAL
* Maximum value of q.
*
* NFAIL (output) INTEGER
* Number of times shift was too big.
*
* ITER (output) INTEGER
* Number of iterations.
*
* NDIV (output) INTEGER
* Number of divisions.
*
* IEEE (input) LOGICAL
* Flag for IEEE or non IEEE arithmetic (passed to SLASQ5).
*
* TTYPE (input/output) INTEGER
* Shift type. TTYPE is passed as an argument in order to save
* its value between calls to SLAZQ3
*
* DMIN1 (input/output) REAL
* DMIN2 (input/output) REAL
* DN (input/output) REAL
* DN1 (input/output) REAL
* DN2 (input/output) REAL
* TAU (input/output) REAL
* These are passed as arguments in order to save their values
* between calls to SLAZQ3
*
* This is a thread safe version of SLASQ3, which passes TTYPE, DMIN1,
* DMIN2, DN, DN1. DN2 and TAU through the argument list in place of
* declaring them in a SAVE statment.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param i0
* @param n0
* @param z
* @param pp
* @param dmin
* @param sigma
* @param desig
* @param qmax
* @param nfail
* @param iter
* @param ndiv
* @param ieee
* @param ttype
* @param dmin1
* @param dmin2
* @param dn
* @param dn1
* @param dn2
* @param tau
*
*/
abstract public void slazq3(int i0, org.netlib.util.intW n0, float[] z, int pp, org.netlib.util.floatW dmin, org.netlib.util.floatW sigma, org.netlib.util.floatW desig, org.netlib.util.floatW qmax, org.netlib.util.intW nfail, org.netlib.util.intW iter, org.netlib.util.intW ndiv, boolean ieee, org.netlib.util.intW ttype, org.netlib.util.floatW dmin1, org.netlib.util.floatW dmin2, org.netlib.util.floatW dn, org.netlib.util.floatW dn1, org.netlib.util.floatW dn2, org.netlib.util.floatW tau);
/**
*
* ..
*
* Purpose
* =======
*
* SLAZQ3 checks for deflation, computes a shift (TAU) and calls dqds.
* In case of failure it changes shifts, and tries again until output
* is positive.
*
* Arguments
* =========
*
* I0 (input) INTEGER
* First index.
*
* N0 (input) INTEGER
* Last index.
*
* Z (input) REAL array, dimension ( 4*N )
* Z holds the qd array.
*
* PP (input) INTEGER
* PP=0 for ping, PP=1 for pong.
*
* DMIN (output) REAL
* Minimum value of d.
*
* SIGMA (output) REAL
* Sum of shifts used in current segment.
*
* DESIG (input/output) REAL
* Lower order part of SIGMA
*
* QMAX (input) REAL
* Maximum value of q.
*
* NFAIL (output) INTEGER
* Number of times shift was too big.
*
* ITER (output) INTEGER
* Number of iterations.
*
* NDIV (output) INTEGER
* Number of divisions.
*
* IEEE (input) LOGICAL
* Flag for IEEE or non IEEE arithmetic (passed to SLASQ5).
*
* TTYPE (input/output) INTEGER
* Shift type. TTYPE is passed as an argument in order to save
* its value between calls to SLAZQ3
*
* DMIN1 (input/output) REAL
* DMIN2 (input/output) REAL
* DN (input/output) REAL
* DN1 (input/output) REAL
* DN2 (input/output) REAL
* TAU (input/output) REAL
* These are passed as arguments in order to save their values
* between calls to SLAZQ3
*
* This is a thread safe version of SLASQ3, which passes TTYPE, DMIN1,
* DMIN2, DN, DN1. DN2 and TAU through the argument list in place of
* declaring them in a SAVE statment.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param i0
* @param n0
* @param z
* @param _z_offset
* @param pp
* @param dmin
* @param sigma
* @param desig
* @param qmax
* @param nfail
* @param iter
* @param ndiv
* @param ieee
* @param ttype
* @param dmin1
* @param dmin2
* @param dn
* @param dn1
* @param dn2
* @param tau
*
*/
abstract public void slazq3(int i0, org.netlib.util.intW n0, float[] z, int _z_offset, int pp, org.netlib.util.floatW dmin, org.netlib.util.floatW sigma, org.netlib.util.floatW desig, org.netlib.util.floatW qmax, org.netlib.util.intW nfail, org.netlib.util.intW iter, org.netlib.util.intW ndiv, boolean ieee, org.netlib.util.intW ttype, org.netlib.util.floatW dmin1, org.netlib.util.floatW dmin2, org.netlib.util.floatW dn, org.netlib.util.floatW dn1, org.netlib.util.floatW dn2, org.netlib.util.floatW tau);
/**
*
* ..
*
* Purpose
* =======
*
* SLAZQ4 computes an approximation TAU to the smallest eigenvalue
* using values of d from the previous transform.
*
* I0 (input) INTEGER
* First index.
*
* N0 (input) INTEGER
* Last index.
*
* Z (input) REAL array, dimension ( 4*N )
* Z holds the qd array.
*
* PP (input) INTEGER
* PP=0 for ping, PP=1 for pong.
*
* N0IN (input) INTEGER
* The value of N0 at start of EIGTEST.
*
* DMIN (input) REAL
* Minimum value of d.
*
* DMIN1 (input) REAL
* Minimum value of d, excluding D( N0 ).
*
* DMIN2 (input) REAL
* Minimum value of d, excluding D( N0 ) and D( N0-1 ).
*
* DN (input) REAL
* d(N)
*
* DN1 (input) REAL
* d(N-1)
*
* DN2 (input) REAL
* d(N-2)
*
* TAU (output) REAL
* This is the shift.
*
* TTYPE (output) INTEGER
* Shift type.
*
* G (input/output) REAL
* G is passed as an argument in order to save its value between
* calls to SLAZQ4
*
* Further Details
* ===============
* CNST1 = 9/16
*
* This is a thread safe version of SLASQ4, which passes G through the
* argument list in place of declaring G in a SAVE statment.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param i0
* @param n0
* @param z
* @param pp
* @param n0in
* @param dmin
* @param dmin1
* @param dmin2
* @param dn
* @param dn1
* @param dn2
* @param tau
* @param ttype
* @param g
*
*/
abstract public void slazq4(int i0, int n0, float[] z, int pp, int n0in, float dmin, float dmin1, float dmin2, float dn, float dn1, float dn2, org.netlib.util.floatW tau, org.netlib.util.intW ttype, org.netlib.util.floatW g);
/**
*
* ..
*
* Purpose
* =======
*
* SLAZQ4 computes an approximation TAU to the smallest eigenvalue
* using values of d from the previous transform.
*
* I0 (input) INTEGER
* First index.
*
* N0 (input) INTEGER
* Last index.
*
* Z (input) REAL array, dimension ( 4*N )
* Z holds the qd array.
*
* PP (input) INTEGER
* PP=0 for ping, PP=1 for pong.
*
* N0IN (input) INTEGER
* The value of N0 at start of EIGTEST.
*
* DMIN (input) REAL
* Minimum value of d.
*
* DMIN1 (input) REAL
* Minimum value of d, excluding D( N0 ).
*
* DMIN2 (input) REAL
* Minimum value of d, excluding D( N0 ) and D( N0-1 ).
*
* DN (input) REAL
* d(N)
*
* DN1 (input) REAL
* d(N-1)
*
* DN2 (input) REAL
* d(N-2)
*
* TAU (output) REAL
* This is the shift.
*
* TTYPE (output) INTEGER
* Shift type.
*
* G (input/output) REAL
* G is passed as an argument in order to save its value between
* calls to SLAZQ4
*
* Further Details
* ===============
* CNST1 = 9/16
*
* This is a thread safe version of SLASQ4, which passes G through the
* argument list in place of declaring G in a SAVE statment.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param i0
* @param n0
* @param z
* @param _z_offset
* @param pp
* @param n0in
* @param dmin
* @param dmin1
* @param dmin2
* @param dn
* @param dn1
* @param dn2
* @param tau
* @param ttype
* @param g
*
*/
abstract public void slazq4(int i0, int n0, float[] z, int _z_offset, int pp, int n0in, float dmin, float dmin1, float dmin2, float dn, float dn1, float dn2, org.netlib.util.floatW tau, org.netlib.util.intW ttype, org.netlib.util.floatW g);
/**
*
* ..
*
* Purpose
* =======
*
* SOPGTR generates a real orthogonal matrix Q which is defined as the
* product of n-1 elementary reflectors H(i) of order n, as returned by
* SSPTRD using packed storage:
*
* if UPLO = 'U', Q = H(n-1) . . . H(2) H(1),
*
* if UPLO = 'L', Q = H(1) H(2) . . . H(n-1).
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangular packed storage used in previous
* call to SSPTRD;
* = 'L': Lower triangular packed storage used in previous
* call to SSPTRD.
*
* N (input) INTEGER
* The order of the matrix Q. N >= 0.
*
* AP (input) REAL array, dimension (N*(N+1)/2)
* The vectors which define the elementary reflectors, as
* returned by SSPTRD.
*
* TAU (input) REAL array, dimension (N-1)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SSPTRD.
*
* Q (output) REAL array, dimension (LDQ,N)
* The N-by-N orthogonal matrix Q.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N).
*
* WORK (workspace) REAL array, dimension (N-1)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param tau
* @param q
* @param ldq
* @param work
* @param info
*
*/
abstract public void sopgtr(java.lang.String uplo, int n, float[] ap, float[] tau, float[] q, int ldq, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SOPGTR generates a real orthogonal matrix Q which is defined as the
* product of n-1 elementary reflectors H(i) of order n, as returned by
* SSPTRD using packed storage:
*
* if UPLO = 'U', Q = H(n-1) . . . H(2) H(1),
*
* if UPLO = 'L', Q = H(1) H(2) . . . H(n-1).
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangular packed storage used in previous
* call to SSPTRD;
* = 'L': Lower triangular packed storage used in previous
* call to SSPTRD.
*
* N (input) INTEGER
* The order of the matrix Q. N >= 0.
*
* AP (input) REAL array, dimension (N*(N+1)/2)
* The vectors which define the elementary reflectors, as
* returned by SSPTRD.
*
* TAU (input) REAL array, dimension (N-1)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SSPTRD.
*
* Q (output) REAL array, dimension (LDQ,N)
* The N-by-N orthogonal matrix Q.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N).
*
* WORK (workspace) REAL array, dimension (N-1)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param tau
* @param _tau_offset
* @param q
* @param _q_offset
* @param ldq
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void sopgtr(java.lang.String uplo, int n, float[] ap, int _ap_offset, float[] tau, int _tau_offset, float[] q, int _q_offset, int ldq, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SOPMTR overwrites the general real M-by-N matrix C with
*
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* where Q is a real orthogonal matrix of order nq, with nq = m if
* SIDE = 'L' and nq = n if SIDE = 'R'. Q is defined as the product of
* nq-1 elementary reflectors, as returned by SSPTRD using packed
* storage:
*
* if UPLO = 'U', Q = H(nq-1) . . . H(2) H(1);
*
* if UPLO = 'L', Q = H(1) H(2) . . . H(nq-1).
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q**T from the Left;
* = 'R': apply Q or Q**T from the Right.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangular packed storage used in previous
* call to SSPTRD;
* = 'L': Lower triangular packed storage used in previous
* call to SSPTRD.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q;
* = 'T': Transpose, apply Q**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* AP (input) REAL array, dimension
* (M*(M+1)/2) if SIDE = 'L'
* (N*(N+1)/2) if SIDE = 'R'
* The vectors which define the elementary reflectors, as
* returned by SSPTRD. AP is modified by the routine but
* restored on exit.
*
* TAU (input) REAL array, dimension (M-1) if SIDE = 'L'
* or (N-1) if SIDE = 'R'
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SSPTRD.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**T*C or C*Q**T or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) REAL array, dimension
* (N) if SIDE = 'L'
* (M) if SIDE = 'R'
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param uplo
* @param trans
* @param m
* @param n
* @param ap
* @param tau
* @param c
* @param Ldc
* @param work
* @param info
*
*/
abstract public void sopmtr(java.lang.String side, java.lang.String uplo, java.lang.String trans, int m, int n, float[] ap, float[] tau, float[] c, int Ldc, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SOPMTR overwrites the general real M-by-N matrix C with
*
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* where Q is a real orthogonal matrix of order nq, with nq = m if
* SIDE = 'L' and nq = n if SIDE = 'R'. Q is defined as the product of
* nq-1 elementary reflectors, as returned by SSPTRD using packed
* storage:
*
* if UPLO = 'U', Q = H(nq-1) . . . H(2) H(1);
*
* if UPLO = 'L', Q = H(1) H(2) . . . H(nq-1).
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q**T from the Left;
* = 'R': apply Q or Q**T from the Right.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangular packed storage used in previous
* call to SSPTRD;
* = 'L': Lower triangular packed storage used in previous
* call to SSPTRD.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q;
* = 'T': Transpose, apply Q**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* AP (input) REAL array, dimension
* (M*(M+1)/2) if SIDE = 'L'
* (N*(N+1)/2) if SIDE = 'R'
* The vectors which define the elementary reflectors, as
* returned by SSPTRD. AP is modified by the routine but
* restored on exit.
*
* TAU (input) REAL array, dimension (M-1) if SIDE = 'L'
* or (N-1) if SIDE = 'R'
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SSPTRD.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**T*C or C*Q**T or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) REAL array, dimension
* (N) if SIDE = 'L'
* (M) if SIDE = 'R'
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param uplo
* @param trans
* @param m
* @param n
* @param ap
* @param _ap_offset
* @param tau
* @param _tau_offset
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void sopmtr(java.lang.String side, java.lang.String uplo, java.lang.String trans, int m, int n, float[] ap, int _ap_offset, float[] tau, int _tau_offset, float[] c, int _c_offset, int Ldc, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORG2L generates an m by n real matrix Q with orthonormal columns,
* which is defined as the last n columns of a product of k elementary
* reflectors of order m
*
* Q = H(k) . . . H(2) H(1)
*
* as returned by SGEQLF.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix Q. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q. M >= N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines the
* matrix Q. N >= K >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the (n-k+i)-th column must contain the vector which
* defines the elementary reflector H(i), for i = 1,2,...,k, as
* returned by SGEQLF in the last k columns of its array
* argument A.
* On exit, the m by n matrix Q.
*
* LDA (input) INTEGER
* The first dimension of the array A. LDA >= max(1,M).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGEQLF.
*
* WORK (workspace) REAL array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument has an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param work
* @param info
*
*/
abstract public void sorg2l(int m, int n, int k, float[] a, int lda, float[] tau, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORG2L generates an m by n real matrix Q with orthonormal columns,
* which is defined as the last n columns of a product of k elementary
* reflectors of order m
*
* Q = H(k) . . . H(2) H(1)
*
* as returned by SGEQLF.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix Q. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q. M >= N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines the
* matrix Q. N >= K >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the (n-k+i)-th column must contain the vector which
* defines the elementary reflector H(i), for i = 1,2,...,k, as
* returned by SGEQLF in the last k columns of its array
* argument A.
* On exit, the m by n matrix Q.
*
* LDA (input) INTEGER
* The first dimension of the array A. LDA >= max(1,M).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGEQLF.
*
* WORK (workspace) REAL array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument has an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void sorg2l(int m, int n, int k, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORG2R generates an m by n real matrix Q with orthonormal columns,
* which is defined as the first n columns of a product of k elementary
* reflectors of order m
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by SGEQRF.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix Q. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q. M >= N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines the
* matrix Q. N >= K >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the i-th column must contain the vector which
* defines the elementary reflector H(i), for i = 1,2,...,k, as
* returned by SGEQRF in the first k columns of its array
* argument A.
* On exit, the m-by-n matrix Q.
*
* LDA (input) INTEGER
* The first dimension of the array A. LDA >= max(1,M).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGEQRF.
*
* WORK (workspace) REAL array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument has an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param work
* @param info
*
*/
abstract public void sorg2r(int m, int n, int k, float[] a, int lda, float[] tau, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORG2R generates an m by n real matrix Q with orthonormal columns,
* which is defined as the first n columns of a product of k elementary
* reflectors of order m
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by SGEQRF.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix Q. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q. M >= N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines the
* matrix Q. N >= K >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the i-th column must contain the vector which
* defines the elementary reflector H(i), for i = 1,2,...,k, as
* returned by SGEQRF in the first k columns of its array
* argument A.
* On exit, the m-by-n matrix Q.
*
* LDA (input) INTEGER
* The first dimension of the array A. LDA >= max(1,M).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGEQRF.
*
* WORK (workspace) REAL array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument has an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void sorg2r(int m, int n, int k, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORGBR generates one of the real orthogonal matrices Q or P**T
* determined by SGEBRD when reducing a real matrix A to bidiagonal
* form: A = Q * B * P**T. Q and P**T are defined as products of
* elementary reflectors H(i) or G(i) respectively.
*
* If VECT = 'Q', A is assumed to have been an M-by-K matrix, and Q
* is of order M:
* if m >= k, Q = H(1) H(2) . . . H(k) and SORGBR returns the first n
* columns of Q, where m >= n >= k;
* if m < k, Q = H(1) H(2) . . . H(m-1) and SORGBR returns Q as an
* M-by-M matrix.
*
* If VECT = 'P', A is assumed to have been a K-by-N matrix, and P**T
* is of order N:
* if k < n, P**T = G(k) . . . G(2) G(1) and SORGBR returns the first m
* rows of P**T, where n >= m >= k;
* if k >= n, P**T = G(n-1) . . . G(2) G(1) and SORGBR returns P**T as
* an N-by-N matrix.
*
* Arguments
* =========
*
* VECT (input) CHARACTER*1
* Specifies whether the matrix Q or the matrix P**T is
* required, as defined in the transformation applied by SGEBRD:
* = 'Q': generate Q;
* = 'P': generate P**T.
*
* M (input) INTEGER
* The number of rows of the matrix Q or P**T to be returned.
* M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q or P**T to be returned.
* N >= 0.
* If VECT = 'Q', M >= N >= min(M,K);
* if VECT = 'P', N >= M >= min(N,K).
*
* K (input) INTEGER
* If VECT = 'Q', the number of columns in the original M-by-K
* matrix reduced by SGEBRD.
* If VECT = 'P', the number of rows in the original K-by-N
* matrix reduced by SGEBRD.
* K >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the vectors which define the elementary reflectors,
* as returned by SGEBRD.
* On exit, the M-by-N matrix Q or P**T.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (input) REAL array, dimension
* (min(M,K)) if VECT = 'Q'
* (min(N,K)) if VECT = 'P'
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i) or G(i), which determines Q or P**T, as
* returned by SGEBRD in its array argument TAUQ or TAUP.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,min(M,N)).
* For optimum performance LWORK >= min(M,N)*NB, where NB
* is the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param vect
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param work
* @param lwork
* @param info
*
*/
abstract public void sorgbr(java.lang.String vect, int m, int n, int k, float[] a, int lda, float[] tau, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORGBR generates one of the real orthogonal matrices Q or P**T
* determined by SGEBRD when reducing a real matrix A to bidiagonal
* form: A = Q * B * P**T. Q and P**T are defined as products of
* elementary reflectors H(i) or G(i) respectively.
*
* If VECT = 'Q', A is assumed to have been an M-by-K matrix, and Q
* is of order M:
* if m >= k, Q = H(1) H(2) . . . H(k) and SORGBR returns the first n
* columns of Q, where m >= n >= k;
* if m < k, Q = H(1) H(2) . . . H(m-1) and SORGBR returns Q as an
* M-by-M matrix.
*
* If VECT = 'P', A is assumed to have been a K-by-N matrix, and P**T
* is of order N:
* if k < n, P**T = G(k) . . . G(2) G(1) and SORGBR returns the first m
* rows of P**T, where n >= m >= k;
* if k >= n, P**T = G(n-1) . . . G(2) G(1) and SORGBR returns P**T as
* an N-by-N matrix.
*
* Arguments
* =========
*
* VECT (input) CHARACTER*1
* Specifies whether the matrix Q or the matrix P**T is
* required, as defined in the transformation applied by SGEBRD:
* = 'Q': generate Q;
* = 'P': generate P**T.
*
* M (input) INTEGER
* The number of rows of the matrix Q or P**T to be returned.
* M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q or P**T to be returned.
* N >= 0.
* If VECT = 'Q', M >= N >= min(M,K);
* if VECT = 'P', N >= M >= min(N,K).
*
* K (input) INTEGER
* If VECT = 'Q', the number of columns in the original M-by-K
* matrix reduced by SGEBRD.
* If VECT = 'P', the number of rows in the original K-by-N
* matrix reduced by SGEBRD.
* K >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the vectors which define the elementary reflectors,
* as returned by SGEBRD.
* On exit, the M-by-N matrix Q or P**T.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (input) REAL array, dimension
* (min(M,K)) if VECT = 'Q'
* (min(N,K)) if VECT = 'P'
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i) or G(i), which determines Q or P**T, as
* returned by SGEBRD in its array argument TAUQ or TAUP.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,min(M,N)).
* For optimum performance LWORK >= min(M,N)*NB, where NB
* is the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param vect
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sorgbr(java.lang.String vect, int m, int n, int k, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORGHR generates a real orthogonal matrix Q which is defined as the
* product of IHI-ILO elementary reflectors of order N, as returned by
* SGEHRD:
*
* Q = H(ilo) H(ilo+1) . . . H(ihi-1).
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix Q. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* ILO and IHI must have the same values as in the previous call
* of SGEHRD. Q is equal to the unit matrix except in the
* submatrix Q(ilo+1:ihi,ilo+1:ihi).
* 1 <= ILO <= IHI <= N, if N > 0; ILO=1 and IHI=0, if N=0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the vectors which define the elementary reflectors,
* as returned by SGEHRD.
* On exit, the N-by-N orthogonal matrix Q.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* TAU (input) REAL array, dimension (N-1)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGEHRD.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= IHI-ILO.
* For optimum performance LWORK >= (IHI-ILO)*NB, where NB is
* the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param ilo
* @param ihi
* @param a
* @param lda
* @param tau
* @param work
* @param lwork
* @param info
*
*/
abstract public void sorghr(int n, int ilo, int ihi, float[] a, int lda, float[] tau, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORGHR generates a real orthogonal matrix Q which is defined as the
* product of IHI-ILO elementary reflectors of order N, as returned by
* SGEHRD:
*
* Q = H(ilo) H(ilo+1) . . . H(ihi-1).
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix Q. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* ILO and IHI must have the same values as in the previous call
* of SGEHRD. Q is equal to the unit matrix except in the
* submatrix Q(ilo+1:ihi,ilo+1:ihi).
* 1 <= ILO <= IHI <= N, if N > 0; ILO=1 and IHI=0, if N=0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the vectors which define the elementary reflectors,
* as returned by SGEHRD.
* On exit, the N-by-N orthogonal matrix Q.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* TAU (input) REAL array, dimension (N-1)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGEHRD.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= IHI-ILO.
* For optimum performance LWORK >= (IHI-ILO)*NB, where NB is
* the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param ilo
* @param ihi
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sorghr(int n, int ilo, int ihi, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORGL2 generates an m by n real matrix Q with orthonormal rows,
* which is defined as the first m rows of a product of k elementary
* reflectors of order n
*
* Q = H(k) . . . H(2) H(1)
*
* as returned by SGELQF.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix Q. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q. N >= M.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines the
* matrix Q. M >= K >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the i-th row must contain the vector which defines
* the elementary reflector H(i), for i = 1,2,...,k, as returned
* by SGELQF in the first k rows of its array argument A.
* On exit, the m-by-n matrix Q.
*
* LDA (input) INTEGER
* The first dimension of the array A. LDA >= max(1,M).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGELQF.
*
* WORK (workspace) REAL array, dimension (M)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument has an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param work
* @param info
*
*/
abstract public void sorgl2(int m, int n, int k, float[] a, int lda, float[] tau, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORGL2 generates an m by n real matrix Q with orthonormal rows,
* which is defined as the first m rows of a product of k elementary
* reflectors of order n
*
* Q = H(k) . . . H(2) H(1)
*
* as returned by SGELQF.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix Q. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q. N >= M.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines the
* matrix Q. M >= K >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the i-th row must contain the vector which defines
* the elementary reflector H(i), for i = 1,2,...,k, as returned
* by SGELQF in the first k rows of its array argument A.
* On exit, the m-by-n matrix Q.
*
* LDA (input) INTEGER
* The first dimension of the array A. LDA >= max(1,M).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGELQF.
*
* WORK (workspace) REAL array, dimension (M)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument has an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void sorgl2(int m, int n, int k, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORGLQ generates an M-by-N real matrix Q with orthonormal rows,
* which is defined as the first M rows of a product of K elementary
* reflectors of order N
*
* Q = H(k) . . . H(2) H(1)
*
* as returned by SGELQF.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix Q. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q. N >= M.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines the
* matrix Q. M >= K >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the i-th row must contain the vector which defines
* the elementary reflector H(i), for i = 1,2,...,k, as returned
* by SGELQF in the first k rows of its array argument A.
* On exit, the M-by-N matrix Q.
*
* LDA (input) INTEGER
* The first dimension of the array A. LDA >= max(1,M).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGELQF.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,M).
* For optimum performance LWORK >= M*NB, where NB is
* the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument has an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param work
* @param lwork
* @param info
*
*/
abstract public void sorglq(int m, int n, int k, float[] a, int lda, float[] tau, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORGLQ generates an M-by-N real matrix Q with orthonormal rows,
* which is defined as the first M rows of a product of K elementary
* reflectors of order N
*
* Q = H(k) . . . H(2) H(1)
*
* as returned by SGELQF.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix Q. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q. N >= M.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines the
* matrix Q. M >= K >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the i-th row must contain the vector which defines
* the elementary reflector H(i), for i = 1,2,...,k, as returned
* by SGELQF in the first k rows of its array argument A.
* On exit, the M-by-N matrix Q.
*
* LDA (input) INTEGER
* The first dimension of the array A. LDA >= max(1,M).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGELQF.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,M).
* For optimum performance LWORK >= M*NB, where NB is
* the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument has an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sorglq(int m, int n, int k, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORGQL generates an M-by-N real matrix Q with orthonormal columns,
* which is defined as the last N columns of a product of K elementary
* reflectors of order M
*
* Q = H(k) . . . H(2) H(1)
*
* as returned by SGEQLF.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix Q. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q. M >= N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines the
* matrix Q. N >= K >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the (n-k+i)-th column must contain the vector which
* defines the elementary reflector H(i), for i = 1,2,...,k, as
* returned by SGEQLF in the last k columns of its array
* argument A.
* On exit, the M-by-N matrix Q.
*
* LDA (input) INTEGER
* The first dimension of the array A. LDA >= max(1,M).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGEQLF.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N).
* For optimum performance LWORK >= N*NB, where NB is the
* optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument has an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param work
* @param lwork
* @param info
*
*/
abstract public void sorgql(int m, int n, int k, float[] a, int lda, float[] tau, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORGQL generates an M-by-N real matrix Q with orthonormal columns,
* which is defined as the last N columns of a product of K elementary
* reflectors of order M
*
* Q = H(k) . . . H(2) H(1)
*
* as returned by SGEQLF.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix Q. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q. M >= N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines the
* matrix Q. N >= K >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the (n-k+i)-th column must contain the vector which
* defines the elementary reflector H(i), for i = 1,2,...,k, as
* returned by SGEQLF in the last k columns of its array
* argument A.
* On exit, the M-by-N matrix Q.
*
* LDA (input) INTEGER
* The first dimension of the array A. LDA >= max(1,M).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGEQLF.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N).
* For optimum performance LWORK >= N*NB, where NB is the
* optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument has an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sorgql(int m, int n, int k, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORGQR generates an M-by-N real matrix Q with orthonormal columns,
* which is defined as the first N columns of a product of K elementary
* reflectors of order M
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by SGEQRF.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix Q. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q. M >= N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines the
* matrix Q. N >= K >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the i-th column must contain the vector which
* defines the elementary reflector H(i), for i = 1,2,...,k, as
* returned by SGEQRF in the first k columns of its array
* argument A.
* On exit, the M-by-N matrix Q.
*
* LDA (input) INTEGER
* The first dimension of the array A. LDA >= max(1,M).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGEQRF.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N).
* For optimum performance LWORK >= N*NB, where NB is the
* optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument has an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param work
* @param lwork
* @param info
*
*/
abstract public void sorgqr(int m, int n, int k, float[] a, int lda, float[] tau, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORGQR generates an M-by-N real matrix Q with orthonormal columns,
* which is defined as the first N columns of a product of K elementary
* reflectors of order M
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by SGEQRF.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix Q. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q. M >= N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines the
* matrix Q. N >= K >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the i-th column must contain the vector which
* defines the elementary reflector H(i), for i = 1,2,...,k, as
* returned by SGEQRF in the first k columns of its array
* argument A.
* On exit, the M-by-N matrix Q.
*
* LDA (input) INTEGER
* The first dimension of the array A. LDA >= max(1,M).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGEQRF.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N).
* For optimum performance LWORK >= N*NB, where NB is the
* optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument has an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sorgqr(int m, int n, int k, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORGR2 generates an m by n real matrix Q with orthonormal rows,
* which is defined as the last m rows of a product of k elementary
* reflectors of order n
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by SGERQF.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix Q. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q. N >= M.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines the
* matrix Q. M >= K >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the (m-k+i)-th row must contain the vector which
* defines the elementary reflector H(i), for i = 1,2,...,k, as
* returned by SGERQF in the last k rows of its array argument
* A.
* On exit, the m by n matrix Q.
*
* LDA (input) INTEGER
* The first dimension of the array A. LDA >= max(1,M).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGERQF.
*
* WORK (workspace) REAL array, dimension (M)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument has an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param work
* @param info
*
*/
abstract public void sorgr2(int m, int n, int k, float[] a, int lda, float[] tau, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORGR2 generates an m by n real matrix Q with orthonormal rows,
* which is defined as the last m rows of a product of k elementary
* reflectors of order n
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by SGERQF.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix Q. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q. N >= M.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines the
* matrix Q. M >= K >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the (m-k+i)-th row must contain the vector which
* defines the elementary reflector H(i), for i = 1,2,...,k, as
* returned by SGERQF in the last k rows of its array argument
* A.
* On exit, the m by n matrix Q.
*
* LDA (input) INTEGER
* The first dimension of the array A. LDA >= max(1,M).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGERQF.
*
* WORK (workspace) REAL array, dimension (M)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument has an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void sorgr2(int m, int n, int k, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORGRQ generates an M-by-N real matrix Q with orthonormal rows,
* which is defined as the last M rows of a product of K elementary
* reflectors of order N
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by SGERQF.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix Q. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q. N >= M.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines the
* matrix Q. M >= K >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the (m-k+i)-th row must contain the vector which
* defines the elementary reflector H(i), for i = 1,2,...,k, as
* returned by SGERQF in the last k rows of its array argument
* A.
* On exit, the M-by-N matrix Q.
*
* LDA (input) INTEGER
* The first dimension of the array A. LDA >= max(1,M).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGERQF.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,M).
* For optimum performance LWORK >= M*NB, where NB is the
* optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument has an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param work
* @param lwork
* @param info
*
*/
abstract public void sorgrq(int m, int n, int k, float[] a, int lda, float[] tau, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORGRQ generates an M-by-N real matrix Q with orthonormal rows,
* which is defined as the last M rows of a product of K elementary
* reflectors of order N
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by SGERQF.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix Q. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix Q. N >= M.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines the
* matrix Q. M >= K >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the (m-k+i)-th row must contain the vector which
* defines the elementary reflector H(i), for i = 1,2,...,k, as
* returned by SGERQF in the last k rows of its array argument
* A.
* On exit, the M-by-N matrix Q.
*
* LDA (input) INTEGER
* The first dimension of the array A. LDA >= max(1,M).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGERQF.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,M).
* For optimum performance LWORK >= M*NB, where NB is the
* optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument has an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sorgrq(int m, int n, int k, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORGTR generates a real orthogonal matrix Q which is defined as the
* product of n-1 elementary reflectors of order N, as returned by
* SSYTRD:
*
* if UPLO = 'U', Q = H(n-1) . . . H(2) H(1),
*
* if UPLO = 'L', Q = H(1) H(2) . . . H(n-1).
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A contains elementary reflectors
* from SSYTRD;
* = 'L': Lower triangle of A contains elementary reflectors
* from SSYTRD.
*
* N (input) INTEGER
* The order of the matrix Q. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the vectors which define the elementary reflectors,
* as returned by SSYTRD.
* On exit, the N-by-N orthogonal matrix Q.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* TAU (input) REAL array, dimension (N-1)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SSYTRD.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N-1).
* For optimum performance LWORK >= (N-1)*NB, where NB is
* the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param lda
* @param tau
* @param work
* @param lwork
* @param info
*
*/
abstract public void sorgtr(java.lang.String uplo, int n, float[] a, int lda, float[] tau, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORGTR generates a real orthogonal matrix Q which is defined as the
* product of n-1 elementary reflectors of order N, as returned by
* SSYTRD:
*
* if UPLO = 'U', Q = H(n-1) . . . H(2) H(1),
*
* if UPLO = 'L', Q = H(1) H(2) . . . H(n-1).
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A contains elementary reflectors
* from SSYTRD;
* = 'L': Lower triangle of A contains elementary reflectors
* from SSYTRD.
*
* N (input) INTEGER
* The order of the matrix Q. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the vectors which define the elementary reflectors,
* as returned by SSYTRD.
* On exit, the N-by-N orthogonal matrix Q.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* TAU (input) REAL array, dimension (N-1)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SSYTRD.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N-1).
* For optimum performance LWORK >= (N-1)*NB, where NB is
* the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sorgtr(java.lang.String uplo, int n, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORM2L overwrites the general real m by n matrix C with
*
* Q * C if SIDE = 'L' and TRANS = 'N', or
*
* Q'* C if SIDE = 'L' and TRANS = 'T', or
*
* C * Q if SIDE = 'R' and TRANS = 'N', or
*
* C * Q' if SIDE = 'R' and TRANS = 'T',
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(k) . . . H(2) H(1)
*
* as returned by SGEQLF. Q is of order m if SIDE = 'L' and of order n
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q' from the Left
* = 'R': apply Q or Q' from the Right
*
* TRANS (input) CHARACTER*1
* = 'N': apply Q (No transpose)
* = 'T': apply Q' (Transpose)
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* A (input) REAL array, dimension (LDA,K)
* The i-th column must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* SGEQLF in the last k columns of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A.
* If SIDE = 'L', LDA >= max(1,M);
* if SIDE = 'R', LDA >= max(1,N).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGEQLF.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the m by n matrix C.
* On exit, C is overwritten by Q*C or Q'*C or C*Q' or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) REAL array, dimension
* (N) if SIDE = 'L',
* (M) if SIDE = 'R'
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param c
* @param Ldc
* @param work
* @param info
*
*/
abstract public void sorm2l(java.lang.String side, java.lang.String trans, int m, int n, int k, float[] a, int lda, float[] tau, float[] c, int Ldc, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORM2L overwrites the general real m by n matrix C with
*
* Q * C if SIDE = 'L' and TRANS = 'N', or
*
* Q'* C if SIDE = 'L' and TRANS = 'T', or
*
* C * Q if SIDE = 'R' and TRANS = 'N', or
*
* C * Q' if SIDE = 'R' and TRANS = 'T',
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(k) . . . H(2) H(1)
*
* as returned by SGEQLF. Q is of order m if SIDE = 'L' and of order n
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q' from the Left
* = 'R': apply Q or Q' from the Right
*
* TRANS (input) CHARACTER*1
* = 'N': apply Q (No transpose)
* = 'T': apply Q' (Transpose)
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* A (input) REAL array, dimension (LDA,K)
* The i-th column must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* SGEQLF in the last k columns of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A.
* If SIDE = 'L', LDA >= max(1,M);
* if SIDE = 'R', LDA >= max(1,N).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGEQLF.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the m by n matrix C.
* On exit, C is overwritten by Q*C or Q'*C or C*Q' or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) REAL array, dimension
* (N) if SIDE = 'L',
* (M) if SIDE = 'R'
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void sorm2l(java.lang.String side, java.lang.String trans, int m, int n, int k, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] c, int _c_offset, int Ldc, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORM2R overwrites the general real m by n matrix C with
*
* Q * C if SIDE = 'L' and TRANS = 'N', or
*
* Q'* C if SIDE = 'L' and TRANS = 'T', or
*
* C * Q if SIDE = 'R' and TRANS = 'N', or
*
* C * Q' if SIDE = 'R' and TRANS = 'T',
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by SGEQRF. Q is of order m if SIDE = 'L' and of order n
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q' from the Left
* = 'R': apply Q or Q' from the Right
*
* TRANS (input) CHARACTER*1
* = 'N': apply Q (No transpose)
* = 'T': apply Q' (Transpose)
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* A (input) REAL array, dimension (LDA,K)
* The i-th column must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* SGEQRF in the first k columns of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A.
* If SIDE = 'L', LDA >= max(1,M);
* if SIDE = 'R', LDA >= max(1,N).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGEQRF.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the m by n matrix C.
* On exit, C is overwritten by Q*C or Q'*C or C*Q' or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) REAL array, dimension
* (N) if SIDE = 'L',
* (M) if SIDE = 'R'
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param c
* @param Ldc
* @param work
* @param info
*
*/
abstract public void sorm2r(java.lang.String side, java.lang.String trans, int m, int n, int k, float[] a, int lda, float[] tau, float[] c, int Ldc, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORM2R overwrites the general real m by n matrix C with
*
* Q * C if SIDE = 'L' and TRANS = 'N', or
*
* Q'* C if SIDE = 'L' and TRANS = 'T', or
*
* C * Q if SIDE = 'R' and TRANS = 'N', or
*
* C * Q' if SIDE = 'R' and TRANS = 'T',
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by SGEQRF. Q is of order m if SIDE = 'L' and of order n
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q' from the Left
* = 'R': apply Q or Q' from the Right
*
* TRANS (input) CHARACTER*1
* = 'N': apply Q (No transpose)
* = 'T': apply Q' (Transpose)
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* A (input) REAL array, dimension (LDA,K)
* The i-th column must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* SGEQRF in the first k columns of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A.
* If SIDE = 'L', LDA >= max(1,M);
* if SIDE = 'R', LDA >= max(1,N).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGEQRF.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the m by n matrix C.
* On exit, C is overwritten by Q*C or Q'*C or C*Q' or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) REAL array, dimension
* (N) if SIDE = 'L',
* (M) if SIDE = 'R'
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void sorm2r(java.lang.String side, java.lang.String trans, int m, int n, int k, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] c, int _c_offset, int Ldc, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* If VECT = 'Q', SORMBR overwrites the general real M-by-N matrix C
* with
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* If VECT = 'P', SORMBR overwrites the general real M-by-N matrix C
* with
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': P * C C * P
* TRANS = 'T': P**T * C C * P**T
*
* Here Q and P**T are the orthogonal matrices determined by SGEBRD when
* reducing a real matrix A to bidiagonal form: A = Q * B * P**T. Q and
* P**T are defined as products of elementary reflectors H(i) and G(i)
* respectively.
*
* Let nq = m if SIDE = 'L' and nq = n if SIDE = 'R'. Thus nq is the
* order of the orthogonal matrix Q or P**T that is applied.
*
* If VECT = 'Q', A is assumed to have been an NQ-by-K matrix:
* if nq >= k, Q = H(1) H(2) . . . H(k);
* if nq < k, Q = H(1) H(2) . . . H(nq-1).
*
* If VECT = 'P', A is assumed to have been a K-by-NQ matrix:
* if k < nq, P = G(1) G(2) . . . G(k);
* if k >= nq, P = G(1) G(2) . . . G(nq-1).
*
* Arguments
* =========
*
* VECT (input) CHARACTER*1
* = 'Q': apply Q or Q**T;
* = 'P': apply P or P**T.
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q, Q**T, P or P**T from the Left;
* = 'R': apply Q, Q**T, P or P**T from the Right.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q or P;
* = 'T': Transpose, apply Q**T or P**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* If VECT = 'Q', the number of columns in the original
* matrix reduced by SGEBRD.
* If VECT = 'P', the number of rows in the original
* matrix reduced by SGEBRD.
* K >= 0.
*
* A (input) REAL array, dimension
* (LDA,min(nq,K)) if VECT = 'Q'
* (LDA,nq) if VECT = 'P'
* The vectors which define the elementary reflectors H(i) and
* G(i), whose products determine the matrices Q and P, as
* returned by SGEBRD.
*
* LDA (input) INTEGER
* The leading dimension of the array A.
* If VECT = 'Q', LDA >= max(1,nq);
* if VECT = 'P', LDA >= max(1,min(nq,K)).
*
* TAU (input) REAL array, dimension (min(nq,K))
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i) or G(i) which determines Q or P, as returned
* by SGEBRD in the array argument TAUQ or TAUP.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**T*C or C*Q**T or C*Q
* or P*C or P**T*C or C*P or C*P**T.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If SIDE = 'L', LWORK >= max(1,N);
* if SIDE = 'R', LWORK >= max(1,M).
* For optimum performance LWORK >= N*NB if SIDE = 'L', and
* LWORK >= M*NB if SIDE = 'R', where NB is the optimal
* blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param vect
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param c
* @param Ldc
* @param work
* @param lwork
* @param info
*
*/
abstract public void sormbr(java.lang.String vect, java.lang.String side, java.lang.String trans, int m, int n, int k, float[] a, int lda, float[] tau, float[] c, int Ldc, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* If VECT = 'Q', SORMBR overwrites the general real M-by-N matrix C
* with
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* If VECT = 'P', SORMBR overwrites the general real M-by-N matrix C
* with
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': P * C C * P
* TRANS = 'T': P**T * C C * P**T
*
* Here Q and P**T are the orthogonal matrices determined by SGEBRD when
* reducing a real matrix A to bidiagonal form: A = Q * B * P**T. Q and
* P**T are defined as products of elementary reflectors H(i) and G(i)
* respectively.
*
* Let nq = m if SIDE = 'L' and nq = n if SIDE = 'R'. Thus nq is the
* order of the orthogonal matrix Q or P**T that is applied.
*
* If VECT = 'Q', A is assumed to have been an NQ-by-K matrix:
* if nq >= k, Q = H(1) H(2) . . . H(k);
* if nq < k, Q = H(1) H(2) . . . H(nq-1).
*
* If VECT = 'P', A is assumed to have been a K-by-NQ matrix:
* if k < nq, P = G(1) G(2) . . . G(k);
* if k >= nq, P = G(1) G(2) . . . G(nq-1).
*
* Arguments
* =========
*
* VECT (input) CHARACTER*1
* = 'Q': apply Q or Q**T;
* = 'P': apply P or P**T.
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q, Q**T, P or P**T from the Left;
* = 'R': apply Q, Q**T, P or P**T from the Right.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q or P;
* = 'T': Transpose, apply Q**T or P**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* If VECT = 'Q', the number of columns in the original
* matrix reduced by SGEBRD.
* If VECT = 'P', the number of rows in the original
* matrix reduced by SGEBRD.
* K >= 0.
*
* A (input) REAL array, dimension
* (LDA,min(nq,K)) if VECT = 'Q'
* (LDA,nq) if VECT = 'P'
* The vectors which define the elementary reflectors H(i) and
* G(i), whose products determine the matrices Q and P, as
* returned by SGEBRD.
*
* LDA (input) INTEGER
* The leading dimension of the array A.
* If VECT = 'Q', LDA >= max(1,nq);
* if VECT = 'P', LDA >= max(1,min(nq,K)).
*
* TAU (input) REAL array, dimension (min(nq,K))
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i) or G(i) which determines Q or P, as returned
* by SGEBRD in the array argument TAUQ or TAUP.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**T*C or C*Q**T or C*Q
* or P*C or P**T*C or C*P or C*P**T.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If SIDE = 'L', LWORK >= max(1,N);
* if SIDE = 'R', LWORK >= max(1,M).
* For optimum performance LWORK >= N*NB if SIDE = 'L', and
* LWORK >= M*NB if SIDE = 'R', where NB is the optimal
* blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param vect
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sormbr(java.lang.String vect, java.lang.String side, java.lang.String trans, int m, int n, int k, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] c, int _c_offset, int Ldc, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORMHR overwrites the general real M-by-N matrix C with
*
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* where Q is a real orthogonal matrix of order nq, with nq = m if
* SIDE = 'L' and nq = n if SIDE = 'R'. Q is defined as the product of
* IHI-ILO elementary reflectors, as returned by SGEHRD:
*
* Q = H(ilo) H(ilo+1) . . . H(ihi-1).
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q**T from the Left;
* = 'R': apply Q or Q**T from the Right.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q;
* = 'T': Transpose, apply Q**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* ILO and IHI must have the same values as in the previous call
* of SGEHRD. Q is equal to the unit matrix except in the
* submatrix Q(ilo+1:ihi,ilo+1:ihi).
* If SIDE = 'L', then 1 <= ILO <= IHI <= M, if M > 0, and
* ILO = 1 and IHI = 0, if M = 0;
* if SIDE = 'R', then 1 <= ILO <= IHI <= N, if N > 0, and
* ILO = 1 and IHI = 0, if N = 0.
*
* A (input) REAL array, dimension
* (LDA,M) if SIDE = 'L'
* (LDA,N) if SIDE = 'R'
* The vectors which define the elementary reflectors, as
* returned by SGEHRD.
*
* LDA (input) INTEGER
* The leading dimension of the array A.
* LDA >= max(1,M) if SIDE = 'L'; LDA >= max(1,N) if SIDE = 'R'.
*
* TAU (input) REAL array, dimension
* (M-1) if SIDE = 'L'
* (N-1) if SIDE = 'R'
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGEHRD.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**T*C or C*Q**T or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If SIDE = 'L', LWORK >= max(1,N);
* if SIDE = 'R', LWORK >= max(1,M).
* For optimum performance LWORK >= N*NB if SIDE = 'L', and
* LWORK >= M*NB if SIDE = 'R', where NB is the optimal
* blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param ilo
* @param ihi
* @param a
* @param lda
* @param tau
* @param c
* @param Ldc
* @param work
* @param lwork
* @param info
*
*/
abstract public void sormhr(java.lang.String side, java.lang.String trans, int m, int n, int ilo, int ihi, float[] a, int lda, float[] tau, float[] c, int Ldc, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORMHR overwrites the general real M-by-N matrix C with
*
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* where Q is a real orthogonal matrix of order nq, with nq = m if
* SIDE = 'L' and nq = n if SIDE = 'R'. Q is defined as the product of
* IHI-ILO elementary reflectors, as returned by SGEHRD:
*
* Q = H(ilo) H(ilo+1) . . . H(ihi-1).
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q**T from the Left;
* = 'R': apply Q or Q**T from the Right.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q;
* = 'T': Transpose, apply Q**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* ILO (input) INTEGER
* IHI (input) INTEGER
* ILO and IHI must have the same values as in the previous call
* of SGEHRD. Q is equal to the unit matrix except in the
* submatrix Q(ilo+1:ihi,ilo+1:ihi).
* If SIDE = 'L', then 1 <= ILO <= IHI <= M, if M > 0, and
* ILO = 1 and IHI = 0, if M = 0;
* if SIDE = 'R', then 1 <= ILO <= IHI <= N, if N > 0, and
* ILO = 1 and IHI = 0, if N = 0.
*
* A (input) REAL array, dimension
* (LDA,M) if SIDE = 'L'
* (LDA,N) if SIDE = 'R'
* The vectors which define the elementary reflectors, as
* returned by SGEHRD.
*
* LDA (input) INTEGER
* The leading dimension of the array A.
* LDA >= max(1,M) if SIDE = 'L'; LDA >= max(1,N) if SIDE = 'R'.
*
* TAU (input) REAL array, dimension
* (M-1) if SIDE = 'L'
* (N-1) if SIDE = 'R'
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGEHRD.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**T*C or C*Q**T or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If SIDE = 'L', LWORK >= max(1,N);
* if SIDE = 'R', LWORK >= max(1,M).
* For optimum performance LWORK >= N*NB if SIDE = 'L', and
* LWORK >= M*NB if SIDE = 'R', where NB is the optimal
* blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param ilo
* @param ihi
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sormhr(java.lang.String side, java.lang.String trans, int m, int n, int ilo, int ihi, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] c, int _c_offset, int Ldc, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORML2 overwrites the general real m by n matrix C with
*
* Q * C if SIDE = 'L' and TRANS = 'N', or
*
* Q'* C if SIDE = 'L' and TRANS = 'T', or
*
* C * Q if SIDE = 'R' and TRANS = 'N', or
*
* C * Q' if SIDE = 'R' and TRANS = 'T',
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(k) . . . H(2) H(1)
*
* as returned by SGELQF. Q is of order m if SIDE = 'L' and of order n
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q' from the Left
* = 'R': apply Q or Q' from the Right
*
* TRANS (input) CHARACTER*1
* = 'N': apply Q (No transpose)
* = 'T': apply Q' (Transpose)
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* A (input) REAL array, dimension
* (LDA,M) if SIDE = 'L',
* (LDA,N) if SIDE = 'R'
* The i-th row must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* SGELQF in the first k rows of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,K).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGELQF.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the m by n matrix C.
* On exit, C is overwritten by Q*C or Q'*C or C*Q' or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) REAL array, dimension
* (N) if SIDE = 'L',
* (M) if SIDE = 'R'
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param c
* @param Ldc
* @param work
* @param info
*
*/
abstract public void sorml2(java.lang.String side, java.lang.String trans, int m, int n, int k, float[] a, int lda, float[] tau, float[] c, int Ldc, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORML2 overwrites the general real m by n matrix C with
*
* Q * C if SIDE = 'L' and TRANS = 'N', or
*
* Q'* C if SIDE = 'L' and TRANS = 'T', or
*
* C * Q if SIDE = 'R' and TRANS = 'N', or
*
* C * Q' if SIDE = 'R' and TRANS = 'T',
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(k) . . . H(2) H(1)
*
* as returned by SGELQF. Q is of order m if SIDE = 'L' and of order n
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q' from the Left
* = 'R': apply Q or Q' from the Right
*
* TRANS (input) CHARACTER*1
* = 'N': apply Q (No transpose)
* = 'T': apply Q' (Transpose)
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* A (input) REAL array, dimension
* (LDA,M) if SIDE = 'L',
* (LDA,N) if SIDE = 'R'
* The i-th row must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* SGELQF in the first k rows of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,K).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGELQF.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the m by n matrix C.
* On exit, C is overwritten by Q*C or Q'*C or C*Q' or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) REAL array, dimension
* (N) if SIDE = 'L',
* (M) if SIDE = 'R'
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void sorml2(java.lang.String side, java.lang.String trans, int m, int n, int k, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] c, int _c_offset, int Ldc, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORMLQ overwrites the general real M-by-N matrix C with
*
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(k) . . . H(2) H(1)
*
* as returned by SGELQF. Q is of order M if SIDE = 'L' and of order N
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q**T from the Left;
* = 'R': apply Q or Q**T from the Right.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q;
* = 'T': Transpose, apply Q**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* A (input) REAL array, dimension
* (LDA,M) if SIDE = 'L',
* (LDA,N) if SIDE = 'R'
* The i-th row must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* SGELQF in the first k rows of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,K).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGELQF.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**T*C or C*Q**T or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If SIDE = 'L', LWORK >= max(1,N);
* if SIDE = 'R', LWORK >= max(1,M).
* For optimum performance LWORK >= N*NB if SIDE = 'L', and
* LWORK >= M*NB if SIDE = 'R', where NB is the optimal
* blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param c
* @param Ldc
* @param work
* @param lwork
* @param info
*
*/
abstract public void sormlq(java.lang.String side, java.lang.String trans, int m, int n, int k, float[] a, int lda, float[] tau, float[] c, int Ldc, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORMLQ overwrites the general real M-by-N matrix C with
*
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(k) . . . H(2) H(1)
*
* as returned by SGELQF. Q is of order M if SIDE = 'L' and of order N
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q**T from the Left;
* = 'R': apply Q or Q**T from the Right.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q;
* = 'T': Transpose, apply Q**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* A (input) REAL array, dimension
* (LDA,M) if SIDE = 'L',
* (LDA,N) if SIDE = 'R'
* The i-th row must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* SGELQF in the first k rows of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,K).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGELQF.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**T*C or C*Q**T or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If SIDE = 'L', LWORK >= max(1,N);
* if SIDE = 'R', LWORK >= max(1,M).
* For optimum performance LWORK >= N*NB if SIDE = 'L', and
* LWORK >= M*NB if SIDE = 'R', where NB is the optimal
* blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sormlq(java.lang.String side, java.lang.String trans, int m, int n, int k, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] c, int _c_offset, int Ldc, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORMQL overwrites the general real M-by-N matrix C with
*
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(k) . . . H(2) H(1)
*
* as returned by SGEQLF. Q is of order M if SIDE = 'L' and of order N
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q**T from the Left;
* = 'R': apply Q or Q**T from the Right.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q;
* = 'T': Transpose, apply Q**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* A (input) REAL array, dimension (LDA,K)
* The i-th column must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* SGEQLF in the last k columns of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A.
* If SIDE = 'L', LDA >= max(1,M);
* if SIDE = 'R', LDA >= max(1,N).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGEQLF.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**T*C or C*Q**T or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If SIDE = 'L', LWORK >= max(1,N);
* if SIDE = 'R', LWORK >= max(1,M).
* For optimum performance LWORK >= N*NB if SIDE = 'L', and
* LWORK >= M*NB if SIDE = 'R', where NB is the optimal
* blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param c
* @param Ldc
* @param work
* @param lwork
* @param info
*
*/
abstract public void sormql(java.lang.String side, java.lang.String trans, int m, int n, int k, float[] a, int lda, float[] tau, float[] c, int Ldc, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORMQL overwrites the general real M-by-N matrix C with
*
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(k) . . . H(2) H(1)
*
* as returned by SGEQLF. Q is of order M if SIDE = 'L' and of order N
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q**T from the Left;
* = 'R': apply Q or Q**T from the Right.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q;
* = 'T': Transpose, apply Q**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* A (input) REAL array, dimension (LDA,K)
* The i-th column must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* SGEQLF in the last k columns of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A.
* If SIDE = 'L', LDA >= max(1,M);
* if SIDE = 'R', LDA >= max(1,N).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGEQLF.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**T*C or C*Q**T or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If SIDE = 'L', LWORK >= max(1,N);
* if SIDE = 'R', LWORK >= max(1,M).
* For optimum performance LWORK >= N*NB if SIDE = 'L', and
* LWORK >= M*NB if SIDE = 'R', where NB is the optimal
* blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sormql(java.lang.String side, java.lang.String trans, int m, int n, int k, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] c, int _c_offset, int Ldc, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORMQR overwrites the general real M-by-N matrix C with
*
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by SGEQRF. Q is of order M if SIDE = 'L' and of order N
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q**T from the Left;
* = 'R': apply Q or Q**T from the Right.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q;
* = 'T': Transpose, apply Q**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* A (input) REAL array, dimension (LDA,K)
* The i-th column must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* SGEQRF in the first k columns of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A.
* If SIDE = 'L', LDA >= max(1,M);
* if SIDE = 'R', LDA >= max(1,N).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGEQRF.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**T*C or C*Q**T or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If SIDE = 'L', LWORK >= max(1,N);
* if SIDE = 'R', LWORK >= max(1,M).
* For optimum performance LWORK >= N*NB if SIDE = 'L', and
* LWORK >= M*NB if SIDE = 'R', where NB is the optimal
* blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param c
* @param Ldc
* @param work
* @param lwork
* @param info
*
*/
abstract public void sormqr(java.lang.String side, java.lang.String trans, int m, int n, int k, float[] a, int lda, float[] tau, float[] c, int Ldc, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORMQR overwrites the general real M-by-N matrix C with
*
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by SGEQRF. Q is of order M if SIDE = 'L' and of order N
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q**T from the Left;
* = 'R': apply Q or Q**T from the Right.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q;
* = 'T': Transpose, apply Q**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* A (input) REAL array, dimension (LDA,K)
* The i-th column must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* SGEQRF in the first k columns of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A.
* If SIDE = 'L', LDA >= max(1,M);
* if SIDE = 'R', LDA >= max(1,N).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGEQRF.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**T*C or C*Q**T or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If SIDE = 'L', LWORK >= max(1,N);
* if SIDE = 'R', LWORK >= max(1,M).
* For optimum performance LWORK >= N*NB if SIDE = 'L', and
* LWORK >= M*NB if SIDE = 'R', where NB is the optimal
* blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sormqr(java.lang.String side, java.lang.String trans, int m, int n, int k, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] c, int _c_offset, int Ldc, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORMR2 overwrites the general real m by n matrix C with
*
* Q * C if SIDE = 'L' and TRANS = 'N', or
*
* Q'* C if SIDE = 'L' and TRANS = 'T', or
*
* C * Q if SIDE = 'R' and TRANS = 'N', or
*
* C * Q' if SIDE = 'R' and TRANS = 'T',
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by SGERQF. Q is of order m if SIDE = 'L' and of order n
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q' from the Left
* = 'R': apply Q or Q' from the Right
*
* TRANS (input) CHARACTER*1
* = 'N': apply Q (No transpose)
* = 'T': apply Q' (Transpose)
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* A (input) REAL array, dimension
* (LDA,M) if SIDE = 'L',
* (LDA,N) if SIDE = 'R'
* The i-th row must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* SGERQF in the last k rows of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,K).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGERQF.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the m by n matrix C.
* On exit, C is overwritten by Q*C or Q'*C or C*Q' or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) REAL array, dimension
* (N) if SIDE = 'L',
* (M) if SIDE = 'R'
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param c
* @param Ldc
* @param work
* @param info
*
*/
abstract public void sormr2(java.lang.String side, java.lang.String trans, int m, int n, int k, float[] a, int lda, float[] tau, float[] c, int Ldc, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORMR2 overwrites the general real m by n matrix C with
*
* Q * C if SIDE = 'L' and TRANS = 'N', or
*
* Q'* C if SIDE = 'L' and TRANS = 'T', or
*
* C * Q if SIDE = 'R' and TRANS = 'N', or
*
* C * Q' if SIDE = 'R' and TRANS = 'T',
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by SGERQF. Q is of order m if SIDE = 'L' and of order n
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q' from the Left
* = 'R': apply Q or Q' from the Right
*
* TRANS (input) CHARACTER*1
* = 'N': apply Q (No transpose)
* = 'T': apply Q' (Transpose)
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* A (input) REAL array, dimension
* (LDA,M) if SIDE = 'L',
* (LDA,N) if SIDE = 'R'
* The i-th row must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* SGERQF in the last k rows of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,K).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGERQF.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the m by n matrix C.
* On exit, C is overwritten by Q*C or Q'*C or C*Q' or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) REAL array, dimension
* (N) if SIDE = 'L',
* (M) if SIDE = 'R'
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void sormr2(java.lang.String side, java.lang.String trans, int m, int n, int k, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] c, int _c_offset, int Ldc, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORMR3 overwrites the general real m by n matrix C with
*
* Q * C if SIDE = 'L' and TRANS = 'N', or
*
* Q'* C if SIDE = 'L' and TRANS = 'T', or
*
* C * Q if SIDE = 'R' and TRANS = 'N', or
*
* C * Q' if SIDE = 'R' and TRANS = 'T',
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by STZRZF. Q is of order m if SIDE = 'L' and of order n
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q' from the Left
* = 'R': apply Q or Q' from the Right
*
* TRANS (input) CHARACTER*1
* = 'N': apply Q (No transpose)
* = 'T': apply Q' (Transpose)
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* L (input) INTEGER
* The number of columns of the matrix A containing
* the meaningful part of the Householder reflectors.
* If SIDE = 'L', M >= L >= 0, if SIDE = 'R', N >= L >= 0.
*
* A (input) REAL array, dimension
* (LDA,M) if SIDE = 'L',
* (LDA,N) if SIDE = 'R'
* The i-th row must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* STZRZF in the last k rows of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,K).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by STZRZF.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the m-by-n matrix C.
* On exit, C is overwritten by Q*C or Q'*C or C*Q' or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) REAL array, dimension
* (N) if SIDE = 'L',
* (M) if SIDE = 'R'
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* Based on contributions by
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param l
* @param a
* @param lda
* @param tau
* @param c
* @param Ldc
* @param work
* @param info
*
*/
abstract public void sormr3(java.lang.String side, java.lang.String trans, int m, int n, int k, int l, float[] a, int lda, float[] tau, float[] c, int Ldc, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORMR3 overwrites the general real m by n matrix C with
*
* Q * C if SIDE = 'L' and TRANS = 'N', or
*
* Q'* C if SIDE = 'L' and TRANS = 'T', or
*
* C * Q if SIDE = 'R' and TRANS = 'N', or
*
* C * Q' if SIDE = 'R' and TRANS = 'T',
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by STZRZF. Q is of order m if SIDE = 'L' and of order n
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q' from the Left
* = 'R': apply Q or Q' from the Right
*
* TRANS (input) CHARACTER*1
* = 'N': apply Q (No transpose)
* = 'T': apply Q' (Transpose)
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* L (input) INTEGER
* The number of columns of the matrix A containing
* the meaningful part of the Householder reflectors.
* If SIDE = 'L', M >= L >= 0, if SIDE = 'R', N >= L >= 0.
*
* A (input) REAL array, dimension
* (LDA,M) if SIDE = 'L',
* (LDA,N) if SIDE = 'R'
* The i-th row must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* STZRZF in the last k rows of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,K).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by STZRZF.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the m-by-n matrix C.
* On exit, C is overwritten by Q*C or Q'*C or C*Q' or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace) REAL array, dimension
* (N) if SIDE = 'L',
* (M) if SIDE = 'R'
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* Based on contributions by
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param l
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void sormr3(java.lang.String side, java.lang.String trans, int m, int n, int k, int l, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] c, int _c_offset, int Ldc, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORMRQ overwrites the general real M-by-N matrix C with
*
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by SGERQF. Q is of order M if SIDE = 'L' and of order N
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q**T from the Left;
* = 'R': apply Q or Q**T from the Right.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q;
* = 'T': Transpose, apply Q**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* A (input) REAL array, dimension
* (LDA,M) if SIDE = 'L',
* (LDA,N) if SIDE = 'R'
* The i-th row must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* SGERQF in the last k rows of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,K).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGERQF.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**T*C or C*Q**T or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If SIDE = 'L', LWORK >= max(1,N);
* if SIDE = 'R', LWORK >= max(1,M).
* For optimum performance LWORK >= N*NB if SIDE = 'L', and
* LWORK >= M*NB if SIDE = 'R', where NB is the optimal
* blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param lda
* @param tau
* @param c
* @param Ldc
* @param work
* @param lwork
* @param info
*
*/
abstract public void sormrq(java.lang.String side, java.lang.String trans, int m, int n, int k, float[] a, int lda, float[] tau, float[] c, int Ldc, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORMRQ overwrites the general real M-by-N matrix C with
*
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by SGERQF. Q is of order M if SIDE = 'L' and of order N
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q**T from the Left;
* = 'R': apply Q or Q**T from the Right.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q;
* = 'T': Transpose, apply Q**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* A (input) REAL array, dimension
* (LDA,M) if SIDE = 'L',
* (LDA,N) if SIDE = 'R'
* The i-th row must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* SGERQF in the last k rows of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,K).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SGERQF.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**T*C or C*Q**T or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If SIDE = 'L', LWORK >= max(1,N);
* if SIDE = 'R', LWORK >= max(1,M).
* For optimum performance LWORK >= N*NB if SIDE = 'L', and
* LWORK >= M*NB if SIDE = 'R', where NB is the optimal
* blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sormrq(java.lang.String side, java.lang.String trans, int m, int n, int k, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] c, int _c_offset, int Ldc, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORMRZ overwrites the general real M-by-N matrix C with
*
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by STZRZF. Q is of order M if SIDE = 'L' and of order N
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q**T from the Left;
* = 'R': apply Q or Q**T from the Right.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q;
* = 'T': Transpose, apply Q**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* L (input) INTEGER
* The number of columns of the matrix A containing
* the meaningful part of the Householder reflectors.
* If SIDE = 'L', M >= L >= 0, if SIDE = 'R', N >= L >= 0.
*
* A (input) REAL array, dimension
* (LDA,M) if SIDE = 'L',
* (LDA,N) if SIDE = 'R'
* The i-th row must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* STZRZF in the last k rows of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,K).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by STZRZF.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**H*C or C*Q**H or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If SIDE = 'L', LWORK >= max(1,N);
* if SIDE = 'R', LWORK >= max(1,M).
* For optimum performance LWORK >= N*NB if SIDE = 'L', and
* LWORK >= M*NB if SIDE = 'R', where NB is the optimal
* blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* Based on contributions by
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param l
* @param a
* @param lda
* @param tau
* @param c
* @param Ldc
* @param work
* @param lwork
* @param info
*
*/
abstract public void sormrz(java.lang.String side, java.lang.String trans, int m, int n, int k, int l, float[] a, int lda, float[] tau, float[] c, int Ldc, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORMRZ overwrites the general real M-by-N matrix C with
*
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* where Q is a real orthogonal matrix defined as the product of k
* elementary reflectors
*
* Q = H(1) H(2) . . . H(k)
*
* as returned by STZRZF. Q is of order M if SIDE = 'L' and of order N
* if SIDE = 'R'.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q**T from the Left;
* = 'R': apply Q or Q**T from the Right.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q;
* = 'T': Transpose, apply Q**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* K (input) INTEGER
* The number of elementary reflectors whose product defines
* the matrix Q.
* If SIDE = 'L', M >= K >= 0;
* if SIDE = 'R', N >= K >= 0.
*
* L (input) INTEGER
* The number of columns of the matrix A containing
* the meaningful part of the Householder reflectors.
* If SIDE = 'L', M >= L >= 0, if SIDE = 'R', N >= L >= 0.
*
* A (input) REAL array, dimension
* (LDA,M) if SIDE = 'L',
* (LDA,N) if SIDE = 'R'
* The i-th row must contain the vector which defines the
* elementary reflector H(i), for i = 1,2,...,k, as returned by
* STZRZF in the last k rows of its array argument A.
* A is modified by the routine but restored on exit.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,K).
*
* TAU (input) REAL array, dimension (K)
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by STZRZF.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**H*C or C*Q**H or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If SIDE = 'L', LWORK >= max(1,N);
* if SIDE = 'R', LWORK >= max(1,M).
* For optimum performance LWORK >= N*NB if SIDE = 'L', and
* LWORK >= M*NB if SIDE = 'R', where NB is the optimal
* blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* Based on contributions by
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param trans
* @param m
* @param n
* @param k
* @param l
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sormrz(java.lang.String side, java.lang.String trans, int m, int n, int k, int l, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] c, int _c_offset, int Ldc, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORMTR overwrites the general real M-by-N matrix C with
*
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* where Q is a real orthogonal matrix of order nq, with nq = m if
* SIDE = 'L' and nq = n if SIDE = 'R'. Q is defined as the product of
* nq-1 elementary reflectors, as returned by SSYTRD:
*
* if UPLO = 'U', Q = H(nq-1) . . . H(2) H(1);
*
* if UPLO = 'L', Q = H(1) H(2) . . . H(nq-1).
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q**T from the Left;
* = 'R': apply Q or Q**T from the Right.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A contains elementary reflectors
* from SSYTRD;
* = 'L': Lower triangle of A contains elementary reflectors
* from SSYTRD.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q;
* = 'T': Transpose, apply Q**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* A (input) REAL array, dimension
* (LDA,M) if SIDE = 'L'
* (LDA,N) if SIDE = 'R'
* The vectors which define the elementary reflectors, as
* returned by SSYTRD.
*
* LDA (input) INTEGER
* The leading dimension of the array A.
* LDA >= max(1,M) if SIDE = 'L'; LDA >= max(1,N) if SIDE = 'R'.
*
* TAU (input) REAL array, dimension
* (M-1) if SIDE = 'L'
* (N-1) if SIDE = 'R'
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SSYTRD.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**T*C or C*Q**T or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If SIDE = 'L', LWORK >= max(1,N);
* if SIDE = 'R', LWORK >= max(1,M).
* For optimum performance LWORK >= N*NB if SIDE = 'L', and
* LWORK >= M*NB if SIDE = 'R', where NB is the optimal
* blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param side
* @param uplo
* @param trans
* @param m
* @param n
* @param a
* @param lda
* @param tau
* @param c
* @param Ldc
* @param work
* @param lwork
* @param info
*
*/
abstract public void sormtr(java.lang.String side, java.lang.String uplo, java.lang.String trans, int m, int n, float[] a, int lda, float[] tau, float[] c, int Ldc, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SORMTR overwrites the general real M-by-N matrix C with
*
* SIDE = 'L' SIDE = 'R'
* TRANS = 'N': Q * C C * Q
* TRANS = 'T': Q**T * C C * Q**T
*
* where Q is a real orthogonal matrix of order nq, with nq = m if
* SIDE = 'L' and nq = n if SIDE = 'R'. Q is defined as the product of
* nq-1 elementary reflectors, as returned by SSYTRD:
*
* if UPLO = 'U', Q = H(nq-1) . . . H(2) H(1);
*
* if UPLO = 'L', Q = H(1) H(2) . . . H(nq-1).
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'L': apply Q or Q**T from the Left;
* = 'R': apply Q or Q**T from the Right.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A contains elementary reflectors
* from SSYTRD;
* = 'L': Lower triangle of A contains elementary reflectors
* from SSYTRD.
*
* TRANS (input) CHARACTER*1
* = 'N': No transpose, apply Q;
* = 'T': Transpose, apply Q**T.
*
* M (input) INTEGER
* The number of rows of the matrix C. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix C. N >= 0.
*
* A (input) REAL array, dimension
* (LDA,M) if SIDE = 'L'
* (LDA,N) if SIDE = 'R'
* The vectors which define the elementary reflectors, as
* returned by SSYTRD.
*
* LDA (input) INTEGER
* The leading dimension of the array A.
* LDA >= max(1,M) if SIDE = 'L'; LDA >= max(1,N) if SIDE = 'R'.
*
* TAU (input) REAL array, dimension
* (M-1) if SIDE = 'L'
* (N-1) if SIDE = 'R'
* TAU(i) must contain the scalar factor of the elementary
* reflector H(i), as returned by SSYTRD.
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the M-by-N matrix C.
* On exit, C is overwritten by Q*C or Q**T*C or C*Q**T or C*Q.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If SIDE = 'L', LWORK >= max(1,N);
* if SIDE = 'R', LWORK >= max(1,M).
* For optimum performance LWORK >= N*NB if SIDE = 'L', and
* LWORK >= M*NB if SIDE = 'R', where NB is the optimal
* blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param side
* @param uplo
* @param trans
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param c
* @param _c_offset
* @param Ldc
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void sormtr(java.lang.String side, java.lang.String uplo, java.lang.String trans, int m, int n, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] c, int _c_offset, int Ldc, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPBCON estimates the reciprocal of the condition number (in the
* 1-norm) of a real symmetric positive definite band matrix using the
* Cholesky factorization A = U**T*U or A = L*L**T computed by SPBTRF.
*
* An estimate is obtained for norm(inv(A)), and the reciprocal of the
* condition number is computed as RCOND = 1 / (ANORM * norm(inv(A))).
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangular factor stored in AB;
* = 'L': Lower triangular factor stored in AB.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* AB (input) REAL array, dimension (LDAB,N)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T of the band matrix A, stored in the
* first KD+1 rows of the array. The j-th column of U or L is
* stored in the j-th column of the array AB as follows:
* if UPLO ='U', AB(kd+1+i-j,j) = U(i,j) for max(1,j-kd)<=i<=j;
* if UPLO ='L', AB(1+i-j,j) = L(i,j) for j<=i<=min(n,j+kd).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* ANORM (input) REAL
* The 1-norm (or infinity-norm) of the symmetric band matrix A.
*
* RCOND (output) REAL
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(ANORM * AINVNM), where AINVNM is an
* estimate of the 1-norm of inv(A) computed in this routine.
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param kd
* @param ab
* @param ldab
* @param anorm
* @param rcond
* @param work
* @param iwork
* @param info
*
*/
abstract public void spbcon(java.lang.String uplo, int n, int kd, float[] ab, int ldab, float anorm, org.netlib.util.floatW rcond, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPBCON estimates the reciprocal of the condition number (in the
* 1-norm) of a real symmetric positive definite band matrix using the
* Cholesky factorization A = U**T*U or A = L*L**T computed by SPBTRF.
*
* An estimate is obtained for norm(inv(A)), and the reciprocal of the
* condition number is computed as RCOND = 1 / (ANORM * norm(inv(A))).
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangular factor stored in AB;
* = 'L': Lower triangular factor stored in AB.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* AB (input) REAL array, dimension (LDAB,N)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T of the band matrix A, stored in the
* first KD+1 rows of the array. The j-th column of U or L is
* stored in the j-th column of the array AB as follows:
* if UPLO ='U', AB(kd+1+i-j,j) = U(i,j) for max(1,j-kd)<=i<=j;
* if UPLO ='L', AB(1+i-j,j) = L(i,j) for j<=i<=min(n,j+kd).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* ANORM (input) REAL
* The 1-norm (or infinity-norm) of the symmetric band matrix A.
*
* RCOND (output) REAL
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(ANORM * AINVNM), where AINVNM is an
* estimate of the 1-norm of inv(A) computed in this routine.
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param kd
* @param ab
* @param _ab_offset
* @param ldab
* @param anorm
* @param rcond
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void spbcon(java.lang.String uplo, int n, int kd, float[] ab, int _ab_offset, int ldab, float anorm, org.netlib.util.floatW rcond, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPBEQU computes row and column scalings intended to equilibrate a
* symmetric positive definite band matrix A and reduce its condition
* number (with respect to the two-norm). S contains the scale factors,
* S(i) = 1/sqrt(A(i,i)), chosen so that the scaled matrix B with
* elements B(i,j) = S(i)*A(i,j)*S(j) has ones on the diagonal. This
* choice of S puts the condition number of B within a factor N of the
* smallest possible condition number over all possible diagonal
* scalings.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangular of A is stored;
* = 'L': Lower triangular of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* AB (input) REAL array, dimension (LDAB,N)
* The upper or lower triangle of the symmetric band matrix A,
* stored in the first KD+1 rows of the array. The j-th column
* of A is stored in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* LDAB (input) INTEGER
* The leading dimension of the array A. LDAB >= KD+1.
*
* S (output) REAL array, dimension (N)
* If INFO = 0, S contains the scale factors for A.
*
* SCOND (output) REAL
* If INFO = 0, S contains the ratio of the smallest S(i) to
* the largest S(i). If SCOND >= 0.1 and AMAX is neither too
* large nor too small, it is not worth scaling by S.
*
* AMAX (output) REAL
* Absolute value of largest matrix element. If AMAX is very
* close to overflow or very close to underflow, the matrix
* should be scaled.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, the i-th diagonal element is nonpositive.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param kd
* @param ab
* @param ldab
* @param s
* @param scond
* @param amax
* @param info
*
*/
abstract public void spbequ(java.lang.String uplo, int n, int kd, float[] ab, int ldab, float[] s, org.netlib.util.floatW scond, org.netlib.util.floatW amax, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPBEQU computes row and column scalings intended to equilibrate a
* symmetric positive definite band matrix A and reduce its condition
* number (with respect to the two-norm). S contains the scale factors,
* S(i) = 1/sqrt(A(i,i)), chosen so that the scaled matrix B with
* elements B(i,j) = S(i)*A(i,j)*S(j) has ones on the diagonal. This
* choice of S puts the condition number of B within a factor N of the
* smallest possible condition number over all possible diagonal
* scalings.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangular of A is stored;
* = 'L': Lower triangular of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* AB (input) REAL array, dimension (LDAB,N)
* The upper or lower triangle of the symmetric band matrix A,
* stored in the first KD+1 rows of the array. The j-th column
* of A is stored in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* LDAB (input) INTEGER
* The leading dimension of the array A. LDAB >= KD+1.
*
* S (output) REAL array, dimension (N)
* If INFO = 0, S contains the scale factors for A.
*
* SCOND (output) REAL
* If INFO = 0, S contains the ratio of the smallest S(i) to
* the largest S(i). If SCOND >= 0.1 and AMAX is neither too
* large nor too small, it is not worth scaling by S.
*
* AMAX (output) REAL
* Absolute value of largest matrix element. If AMAX is very
* close to overflow or very close to underflow, the matrix
* should be scaled.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, the i-th diagonal element is nonpositive.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param kd
* @param ab
* @param _ab_offset
* @param ldab
* @param s
* @param _s_offset
* @param scond
* @param amax
* @param info
*
*/
abstract public void spbequ(java.lang.String uplo, int n, int kd, float[] ab, int _ab_offset, int ldab, float[] s, int _s_offset, org.netlib.util.floatW scond, org.netlib.util.floatW amax, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPBRFS improves the computed solution to a system of linear
* equations when the coefficient matrix is symmetric positive definite
* and banded, and provides error bounds and backward error estimates
* for the solution.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AB (input) REAL array, dimension (LDAB,N)
* The upper or lower triangle of the symmetric band matrix A,
* stored in the first KD+1 rows of the array. The j-th column
* of A is stored in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* AFB (input) REAL array, dimension (LDAFB,N)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T of the band matrix A as computed by
* SPBTRF, in the same storage format as A (see AB).
*
* LDAFB (input) INTEGER
* The leading dimension of the array AFB. LDAFB >= KD+1.
*
* B (input) REAL array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) REAL array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by SPBTRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param kd
* @param nrhs
* @param ab
* @param ldab
* @param afb
* @param ldafb
* @param b
* @param ldb
* @param x
* @param ldx
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void spbrfs(java.lang.String uplo, int n, int kd, int nrhs, float[] ab, int ldab, float[] afb, int ldafb, float[] b, int ldb, float[] x, int ldx, float[] ferr, float[] berr, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPBRFS improves the computed solution to a system of linear
* equations when the coefficient matrix is symmetric positive definite
* and banded, and provides error bounds and backward error estimates
* for the solution.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AB (input) REAL array, dimension (LDAB,N)
* The upper or lower triangle of the symmetric band matrix A,
* stored in the first KD+1 rows of the array. The j-th column
* of A is stored in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* AFB (input) REAL array, dimension (LDAFB,N)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T of the band matrix A as computed by
* SPBTRF, in the same storage format as A (see AB).
*
* LDAFB (input) INTEGER
* The leading dimension of the array AFB. LDAFB >= KD+1.
*
* B (input) REAL array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) REAL array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by SPBTRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param kd
* @param nrhs
* @param ab
* @param _ab_offset
* @param ldab
* @param afb
* @param _afb_offset
* @param ldafb
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void spbrfs(java.lang.String uplo, int n, int kd, int nrhs, float[] ab, int _ab_offset, int ldab, float[] afb, int _afb_offset, int ldafb, float[] b, int _b_offset, int ldb, float[] x, int _x_offset, int ldx, float[] ferr, int _ferr_offset, float[] berr, int _berr_offset, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPBSTF computes a split Cholesky factorization of a real
* symmetric positive definite band matrix A.
*
* This routine is designed to be used in conjunction with SSBGST.
*
* The factorization has the form A = S**T*S where S is a band matrix
* of the same bandwidth as A and the following structure:
*
* S = ( U )
* ( M L )
*
* where U is upper triangular of order m = (n+kd)/2, and L is lower
* triangular of order n-m.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* AB (input/output) REAL array, dimension (LDAB,N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first kd+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* On exit, if INFO = 0, the factor S from the split Cholesky
* factorization A = S**T*S. See Further Details.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the factorization could not be completed,
* because the updated element a(i,i) was negative; the
* matrix A is not positive definite.
*
* Further Details
* ===============
*
* The band storage scheme is illustrated by the following example, when
* N = 7, KD = 2:
*
* S = ( s11 s12 s13 )
* ( s22 s23 s24 )
* ( s33 s34 )
* ( s44 )
* ( s53 s54 s55 )
* ( s64 s65 s66 )
* ( s75 s76 s77 )
*
* If UPLO = 'U', the array AB holds:
*
* on entry: on exit:
*
* * * a13 a24 a35 a46 a57 * * s13 s24 s53 s64 s75
* * a12 a23 a34 a45 a56 a67 * s12 s23 s34 s54 s65 s76
* a11 a22 a33 a44 a55 a66 a77 s11 s22 s33 s44 s55 s66 s77
*
* If UPLO = 'L', the array AB holds:
*
* on entry: on exit:
*
* a11 a22 a33 a44 a55 a66 a77 s11 s22 s33 s44 s55 s66 s77
* a21 a32 a43 a54 a65 a76 * s12 s23 s34 s54 s65 s76 *
* a31 a42 a53 a64 a64 * * s13 s24 s53 s64 s75 * *
*
* Array elements marked * are not used by the routine.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param kd
* @param ab
* @param ldab
* @param info
*
*/
abstract public void spbstf(java.lang.String uplo, int n, int kd, float[] ab, int ldab, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPBSTF computes a split Cholesky factorization of a real
* symmetric positive definite band matrix A.
*
* This routine is designed to be used in conjunction with SSBGST.
*
* The factorization has the form A = S**T*S where S is a band matrix
* of the same bandwidth as A and the following structure:
*
* S = ( U )
* ( M L )
*
* where U is upper triangular of order m = (n+kd)/2, and L is lower
* triangular of order n-m.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* AB (input/output) REAL array, dimension (LDAB,N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first kd+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* On exit, if INFO = 0, the factor S from the split Cholesky
* factorization A = S**T*S. See Further Details.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the factorization could not be completed,
* because the updated element a(i,i) was negative; the
* matrix A is not positive definite.
*
* Further Details
* ===============
*
* The band storage scheme is illustrated by the following example, when
* N = 7, KD = 2:
*
* S = ( s11 s12 s13 )
* ( s22 s23 s24 )
* ( s33 s34 )
* ( s44 )
* ( s53 s54 s55 )
* ( s64 s65 s66 )
* ( s75 s76 s77 )
*
* If UPLO = 'U', the array AB holds:
*
* on entry: on exit:
*
* * * a13 a24 a35 a46 a57 * * s13 s24 s53 s64 s75
* * a12 a23 a34 a45 a56 a67 * s12 s23 s34 s54 s65 s76
* a11 a22 a33 a44 a55 a66 a77 s11 s22 s33 s44 s55 s66 s77
*
* If UPLO = 'L', the array AB holds:
*
* on entry: on exit:
*
* a11 a22 a33 a44 a55 a66 a77 s11 s22 s33 s44 s55 s66 s77
* a21 a32 a43 a54 a65 a76 * s12 s23 s34 s54 s65 s76 *
* a31 a42 a53 a64 a64 * * s13 s24 s53 s64 s75 * *
*
* Array elements marked * are not used by the routine.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param kd
* @param ab
* @param _ab_offset
* @param ldab
* @param info
*
*/
abstract public void spbstf(java.lang.String uplo, int n, int kd, float[] ab, int _ab_offset, int ldab, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPBSV computes the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N symmetric positive definite band matrix and X
* and B are N-by-NRHS matrices.
*
* The Cholesky decomposition is used to factor A as
* A = U**T * U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular band matrix, and L is a lower
* triangular band matrix, with the same number of superdiagonals or
* subdiagonals as A. The factored form of A is then used to solve the
* system of equations A * X = B.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AB (input/output) REAL array, dimension (LDAB,N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(KD+1+i-j,j) = A(i,j) for max(1,j-KD)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(N,j+KD).
* See below for further details.
*
* On exit, if INFO = 0, the triangular factor U or L from the
* Cholesky factorization A = U**T*U or A = L*L**T of the band
* matrix A, in the same storage format as A.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if INFO = 0, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the leading minor of order i of A is not
* positive definite, so the factorization could not be
* completed, and the solution has not been computed.
*
* Further Details
* ===============
*
* The band storage scheme is illustrated by the following example, when
* N = 6, KD = 2, and UPLO = 'U':
*
* On entry: On exit:
*
* * * a13 a24 a35 a46 * * u13 u24 u35 u46
* * a12 a23 a34 a45 a56 * u12 u23 u34 u45 u56
* a11 a22 a33 a44 a55 a66 u11 u22 u33 u44 u55 u66
*
* Similarly, if UPLO = 'L' the format of A is as follows:
*
* On entry: On exit:
*
* a11 a22 a33 a44 a55 a66 l11 l22 l33 l44 l55 l66
* a21 a32 a43 a54 a65 * l21 l32 l43 l54 l65 *
* a31 a42 a53 a64 * * l31 l42 l53 l64 * *
*
* Array elements marked * are not used by the routine.
*
* =====================================================================
*
* .. External Functions ..
*
*
* @param uplo
* @param n
* @param kd
* @param nrhs
* @param ab
* @param ldab
* @param b
* @param ldb
* @param info
*
*/
abstract public void spbsv(java.lang.String uplo, int n, int kd, int nrhs, float[] ab, int ldab, float[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPBSV computes the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N symmetric positive definite band matrix and X
* and B are N-by-NRHS matrices.
*
* The Cholesky decomposition is used to factor A as
* A = U**T * U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular band matrix, and L is a lower
* triangular band matrix, with the same number of superdiagonals or
* subdiagonals as A. The factored form of A is then used to solve the
* system of equations A * X = B.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AB (input/output) REAL array, dimension (LDAB,N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(KD+1+i-j,j) = A(i,j) for max(1,j-KD)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(N,j+KD).
* See below for further details.
*
* On exit, if INFO = 0, the triangular factor U or L from the
* Cholesky factorization A = U**T*U or A = L*L**T of the band
* matrix A, in the same storage format as A.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if INFO = 0, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the leading minor of order i of A is not
* positive definite, so the factorization could not be
* completed, and the solution has not been computed.
*
* Further Details
* ===============
*
* The band storage scheme is illustrated by the following example, when
* N = 6, KD = 2, and UPLO = 'U':
*
* On entry: On exit:
*
* * * a13 a24 a35 a46 * * u13 u24 u35 u46
* * a12 a23 a34 a45 a56 * u12 u23 u34 u45 u56
* a11 a22 a33 a44 a55 a66 u11 u22 u33 u44 u55 u66
*
* Similarly, if UPLO = 'L' the format of A is as follows:
*
* On entry: On exit:
*
* a11 a22 a33 a44 a55 a66 l11 l22 l33 l44 l55 l66
* a21 a32 a43 a54 a65 * l21 l32 l43 l54 l65 *
* a31 a42 a53 a64 * * l31 l42 l53 l64 * *
*
* Array elements marked * are not used by the routine.
*
* =====================================================================
*
* .. External Functions ..
*
*
* @param uplo
* @param n
* @param kd
* @param nrhs
* @param ab
* @param _ab_offset
* @param ldab
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void spbsv(java.lang.String uplo, int n, int kd, int nrhs, float[] ab, int _ab_offset, int ldab, float[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPBSVX uses the Cholesky factorization A = U**T*U or A = L*L**T to
* compute the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N symmetric positive definite band matrix and X
* and B are N-by-NRHS matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed:
*
* 1. If FACT = 'E', real scaling factors are computed to equilibrate
* the system:
* diag(S) * A * diag(S) * inv(diag(S)) * X = diag(S) * B
* Whether or not the system will be equilibrated depends on the
* scaling of the matrix A, but if equilibration is used, A is
* overwritten by diag(S)*A*diag(S) and B by diag(S)*B.
*
* 2. If FACT = 'N' or 'E', the Cholesky decomposition is used to
* factor the matrix A (after equilibration if FACT = 'E') as
* A = U**T * U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular band matrix, and L is a lower
* triangular band matrix.
*
* 3. If the leading i-by-i principal minor is not positive definite,
* then the routine returns with INFO = i. Otherwise, the factored
* form of A is used to estimate the condition number of the matrix
* A. If the reciprocal of the condition number is less than machine
* precision, INFO = N+1 is returned as a warning, but the routine
* still goes on to solve for X and compute error bounds as
* described below.
*
* 4. The system of equations is solved for X using the factored form
* of A.
*
* 5. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* 6. If equilibration was used, the matrix X is premultiplied by
* diag(S) so that it solves the original system before
* equilibration.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of the matrix A is
* supplied on entry, and if not, whether the matrix A should be
* equilibrated before it is factored.
* = 'F': On entry, AFB contains the factored form of A.
* If EQUED = 'Y', the matrix A has been equilibrated
* with scaling factors given by S. AB and AFB will not
* be modified.
* = 'N': The matrix A will be copied to AFB and factored.
* = 'E': The matrix A will be equilibrated if necessary, then
* copied to AFB and factored.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* NRHS (input) INTEGER
* The number of right-hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AB (input/output) REAL array, dimension (LDAB,N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array, except
* if FACT = 'F' and EQUED = 'Y', then A must contain the
* equilibrated matrix diag(S)*A*diag(S). The j-th column of A
* is stored in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(KD+1+i-j,j) = A(i,j) for max(1,j-KD)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(N,j+KD).
* See below for further details.
*
* On exit, if FACT = 'E' and EQUED = 'Y', A is overwritten by
* diag(S)*A*diag(S).
*
* LDAB (input) INTEGER
* The leading dimension of the array A. LDAB >= KD+1.
*
* AFB (input or output) REAL array, dimension (LDAFB,N)
* If FACT = 'F', then AFB is an input argument and on entry
* contains the triangular factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T of the band matrix
* A, in the same storage format as A (see AB). If EQUED = 'Y',
* then AFB is the factored form of the equilibrated matrix A.
*
* If FACT = 'N', then AFB is an output argument and on exit
* returns the triangular factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T.
*
* If FACT = 'E', then AFB is an output argument and on exit
* returns the triangular factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T of the equilibrated
* matrix A (see the description of A for the form of the
* equilibrated matrix).
*
* LDAFB (input) INTEGER
* The leading dimension of the array AFB. LDAFB >= KD+1.
*
* EQUED (input or output) CHARACTER*1
* Specifies the form of equilibration that was done.
* = 'N': No equilibration (always true if FACT = 'N').
* = 'Y': Equilibration was done, i.e., A has been replaced by
* diag(S) * A * diag(S).
* EQUED is an input argument if FACT = 'F'; otherwise, it is an
* output argument.
*
* S (input or output) REAL array, dimension (N)
* The scale factors for A; not accessed if EQUED = 'N'. S is
* an input argument if FACT = 'F'; otherwise, S is an output
* argument. If FACT = 'F' and EQUED = 'Y', each element of S
* must be positive.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if EQUED = 'N', B is not modified; if EQUED = 'Y',
* B is overwritten by diag(S) * B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) REAL array, dimension (LDX,NRHS)
* If INFO = 0 or INFO = N+1, the N-by-NRHS solution matrix X to
* the original system of equations. Note that if EQUED = 'Y',
* A and B are modified on exit, and the solution to the
* equilibrated system is inv(diag(S))*X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) REAL
* The estimate of the reciprocal condition number of the matrix
* A after equilibration (if done). If RCOND is less than the
* machine precision (in particular, if RCOND = 0), the matrix
* is singular to working precision. This condition is
* indicated by a return code of INFO > 0.
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= N: the leading minor of order i of A is
* not positive definite, so the factorization
* could not be completed, and the solution has not
* been computed. RCOND = 0 is returned.
* = N+1: U is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* Further Details
* ===============
*
* The band storage scheme is illustrated by the following example, when
* N = 6, KD = 2, and UPLO = 'U':
*
* Two-dimensional storage of the symmetric matrix A:
*
* a11 a12 a13
* a22 a23 a24
* a33 a34 a35
* a44 a45 a46
* a55 a56
* (aij=conjg(aji)) a66
*
* Band storage of the upper triangle of A:
*
* * * a13 a24 a35 a46
* * a12 a23 a34 a45 a56
* a11 a22 a33 a44 a55 a66
*
* Similarly, if UPLO = 'L' the format of A is as follows:
*
* a11 a22 a33 a44 a55 a66
* a21 a32 a43 a54 a65 *
* a31 a42 a53 a64 * *
*
* Array elements marked * are not used by the routine.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param uplo
* @param n
* @param kd
* @param nrhs
* @param ab
* @param ldab
* @param afb
* @param ldafb
* @param equed
* @param s
* @param b
* @param ldb
* @param x
* @param ldx
* @param rcond
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void spbsvx(java.lang.String fact, java.lang.String uplo, int n, int kd, int nrhs, float[] ab, int ldab, float[] afb, int ldafb, org.netlib.util.StringW equed, float[] s, float[] b, int ldb, float[] x, int ldx, org.netlib.util.floatW rcond, float[] ferr, float[] berr, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPBSVX uses the Cholesky factorization A = U**T*U or A = L*L**T to
* compute the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N symmetric positive definite band matrix and X
* and B are N-by-NRHS matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed:
*
* 1. If FACT = 'E', real scaling factors are computed to equilibrate
* the system:
* diag(S) * A * diag(S) * inv(diag(S)) * X = diag(S) * B
* Whether or not the system will be equilibrated depends on the
* scaling of the matrix A, but if equilibration is used, A is
* overwritten by diag(S)*A*diag(S) and B by diag(S)*B.
*
* 2. If FACT = 'N' or 'E', the Cholesky decomposition is used to
* factor the matrix A (after equilibration if FACT = 'E') as
* A = U**T * U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular band matrix, and L is a lower
* triangular band matrix.
*
* 3. If the leading i-by-i principal minor is not positive definite,
* then the routine returns with INFO = i. Otherwise, the factored
* form of A is used to estimate the condition number of the matrix
* A. If the reciprocal of the condition number is less than machine
* precision, INFO = N+1 is returned as a warning, but the routine
* still goes on to solve for X and compute error bounds as
* described below.
*
* 4. The system of equations is solved for X using the factored form
* of A.
*
* 5. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* 6. If equilibration was used, the matrix X is premultiplied by
* diag(S) so that it solves the original system before
* equilibration.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of the matrix A is
* supplied on entry, and if not, whether the matrix A should be
* equilibrated before it is factored.
* = 'F': On entry, AFB contains the factored form of A.
* If EQUED = 'Y', the matrix A has been equilibrated
* with scaling factors given by S. AB and AFB will not
* be modified.
* = 'N': The matrix A will be copied to AFB and factored.
* = 'E': The matrix A will be equilibrated if necessary, then
* copied to AFB and factored.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* NRHS (input) INTEGER
* The number of right-hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AB (input/output) REAL array, dimension (LDAB,N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array, except
* if FACT = 'F' and EQUED = 'Y', then A must contain the
* equilibrated matrix diag(S)*A*diag(S). The j-th column of A
* is stored in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(KD+1+i-j,j) = A(i,j) for max(1,j-KD)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(N,j+KD).
* See below for further details.
*
* On exit, if FACT = 'E' and EQUED = 'Y', A is overwritten by
* diag(S)*A*diag(S).
*
* LDAB (input) INTEGER
* The leading dimension of the array A. LDAB >= KD+1.
*
* AFB (input or output) REAL array, dimension (LDAFB,N)
* If FACT = 'F', then AFB is an input argument and on entry
* contains the triangular factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T of the band matrix
* A, in the same storage format as A (see AB). If EQUED = 'Y',
* then AFB is the factored form of the equilibrated matrix A.
*
* If FACT = 'N', then AFB is an output argument and on exit
* returns the triangular factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T.
*
* If FACT = 'E', then AFB is an output argument and on exit
* returns the triangular factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T of the equilibrated
* matrix A (see the description of A for the form of the
* equilibrated matrix).
*
* LDAFB (input) INTEGER
* The leading dimension of the array AFB. LDAFB >= KD+1.
*
* EQUED (input or output) CHARACTER*1
* Specifies the form of equilibration that was done.
* = 'N': No equilibration (always true if FACT = 'N').
* = 'Y': Equilibration was done, i.e., A has been replaced by
* diag(S) * A * diag(S).
* EQUED is an input argument if FACT = 'F'; otherwise, it is an
* output argument.
*
* S (input or output) REAL array, dimension (N)
* The scale factors for A; not accessed if EQUED = 'N'. S is
* an input argument if FACT = 'F'; otherwise, S is an output
* argument. If FACT = 'F' and EQUED = 'Y', each element of S
* must be positive.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if EQUED = 'N', B is not modified; if EQUED = 'Y',
* B is overwritten by diag(S) * B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) REAL array, dimension (LDX,NRHS)
* If INFO = 0 or INFO = N+1, the N-by-NRHS solution matrix X to
* the original system of equations. Note that if EQUED = 'Y',
* A and B are modified on exit, and the solution to the
* equilibrated system is inv(diag(S))*X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) REAL
* The estimate of the reciprocal condition number of the matrix
* A after equilibration (if done). If RCOND is less than the
* machine precision (in particular, if RCOND = 0), the matrix
* is singular to working precision. This condition is
* indicated by a return code of INFO > 0.
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= N: the leading minor of order i of A is
* not positive definite, so the factorization
* could not be completed, and the solution has not
* been computed. RCOND = 0 is returned.
* = N+1: U is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* Further Details
* ===============
*
* The band storage scheme is illustrated by the following example, when
* N = 6, KD = 2, and UPLO = 'U':
*
* Two-dimensional storage of the symmetric matrix A:
*
* a11 a12 a13
* a22 a23 a24
* a33 a34 a35
* a44 a45 a46
* a55 a56
* (aij=conjg(aji)) a66
*
* Band storage of the upper triangle of A:
*
* * * a13 a24 a35 a46
* * a12 a23 a34 a45 a56
* a11 a22 a33 a44 a55 a66
*
* Similarly, if UPLO = 'L' the format of A is as follows:
*
* a11 a22 a33 a44 a55 a66
* a21 a32 a43 a54 a65 *
* a31 a42 a53 a64 * *
*
* Array elements marked * are not used by the routine.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param uplo
* @param n
* @param kd
* @param nrhs
* @param ab
* @param _ab_offset
* @param ldab
* @param afb
* @param _afb_offset
* @param ldafb
* @param equed
* @param s
* @param _s_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param rcond
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void spbsvx(java.lang.String fact, java.lang.String uplo, int n, int kd, int nrhs, float[] ab, int _ab_offset, int ldab, float[] afb, int _afb_offset, int ldafb, org.netlib.util.StringW equed, float[] s, int _s_offset, float[] b, int _b_offset, int ldb, float[] x, int _x_offset, int ldx, org.netlib.util.floatW rcond, float[] ferr, int _ferr_offset, float[] berr, int _berr_offset, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPBTF2 computes the Cholesky factorization of a real symmetric
* positive definite band matrix A.
*
* The factorization has the form
* A = U' * U , if UPLO = 'U', or
* A = L * L', if UPLO = 'L',
* where U is an upper triangular matrix, U' is the transpose of U, and
* L is lower triangular.
*
* This is the unblocked version of the algorithm, calling Level 2 BLAS.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored:
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of super-diagonals of the matrix A if UPLO = 'U',
* or the number of sub-diagonals if UPLO = 'L'. KD >= 0.
*
* AB (input/output) REAL array, dimension (LDAB,N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* On exit, if INFO = 0, the triangular factor U or L from the
* Cholesky factorization A = U'*U or A = L*L' of the band
* matrix A, in the same storage format as A.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
* > 0: if INFO = k, the leading minor of order k is not
* positive definite, and the factorization could not be
* completed.
*
* Further Details
* ===============
*
* The band storage scheme is illustrated by the following example, when
* N = 6, KD = 2, and UPLO = 'U':
*
* On entry: On exit:
*
* * * a13 a24 a35 a46 * * u13 u24 u35 u46
* * a12 a23 a34 a45 a56 * u12 u23 u34 u45 u56
* a11 a22 a33 a44 a55 a66 u11 u22 u33 u44 u55 u66
*
* Similarly, if UPLO = 'L' the format of A is as follows:
*
* On entry: On exit:
*
* a11 a22 a33 a44 a55 a66 l11 l22 l33 l44 l55 l66
* a21 a32 a43 a54 a65 * l21 l32 l43 l54 l65 *
* a31 a42 a53 a64 * * l31 l42 l53 l64 * *
*
* Array elements marked * are not used by the routine.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param kd
* @param ab
* @param ldab
* @param info
*
*/
abstract public void spbtf2(java.lang.String uplo, int n, int kd, float[] ab, int ldab, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPBTF2 computes the Cholesky factorization of a real symmetric
* positive definite band matrix A.
*
* The factorization has the form
* A = U' * U , if UPLO = 'U', or
* A = L * L', if UPLO = 'L',
* where U is an upper triangular matrix, U' is the transpose of U, and
* L is lower triangular.
*
* This is the unblocked version of the algorithm, calling Level 2 BLAS.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored:
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of super-diagonals of the matrix A if UPLO = 'U',
* or the number of sub-diagonals if UPLO = 'L'. KD >= 0.
*
* AB (input/output) REAL array, dimension (LDAB,N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* On exit, if INFO = 0, the triangular factor U or L from the
* Cholesky factorization A = U'*U or A = L*L' of the band
* matrix A, in the same storage format as A.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
* > 0: if INFO = k, the leading minor of order k is not
* positive definite, and the factorization could not be
* completed.
*
* Further Details
* ===============
*
* The band storage scheme is illustrated by the following example, when
* N = 6, KD = 2, and UPLO = 'U':
*
* On entry: On exit:
*
* * * a13 a24 a35 a46 * * u13 u24 u35 u46
* * a12 a23 a34 a45 a56 * u12 u23 u34 u45 u56
* a11 a22 a33 a44 a55 a66 u11 u22 u33 u44 u55 u66
*
* Similarly, if UPLO = 'L' the format of A is as follows:
*
* On entry: On exit:
*
* a11 a22 a33 a44 a55 a66 l11 l22 l33 l44 l55 l66
* a21 a32 a43 a54 a65 * l21 l32 l43 l54 l65 *
* a31 a42 a53 a64 * * l31 l42 l53 l64 * *
*
* Array elements marked * are not used by the routine.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param kd
* @param ab
* @param _ab_offset
* @param ldab
* @param info
*
*/
abstract public void spbtf2(java.lang.String uplo, int n, int kd, float[] ab, int _ab_offset, int ldab, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPBTRF computes the Cholesky factorization of a real symmetric
* positive definite band matrix A.
*
* The factorization has the form
* A = U**T * U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular matrix and L is lower triangular.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* AB (input/output) REAL array, dimension (LDAB,N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* On exit, if INFO = 0, the triangular factor U or L from the
* Cholesky factorization A = U**T*U or A = L*L**T of the band
* matrix A, in the same storage format as A.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the leading minor of order i is not
* positive definite, and the factorization could not be
* completed.
*
* Further Details
* ===============
*
* The band storage scheme is illustrated by the following example, when
* N = 6, KD = 2, and UPLO = 'U':
*
* On entry: On exit:
*
* * * a13 a24 a35 a46 * * u13 u24 u35 u46
* * a12 a23 a34 a45 a56 * u12 u23 u34 u45 u56
* a11 a22 a33 a44 a55 a66 u11 u22 u33 u44 u55 u66
*
* Similarly, if UPLO = 'L' the format of A is as follows:
*
* On entry: On exit:
*
* a11 a22 a33 a44 a55 a66 l11 l22 l33 l44 l55 l66
* a21 a32 a43 a54 a65 * l21 l32 l43 l54 l65 *
* a31 a42 a53 a64 * * l31 l42 l53 l64 * *
*
* Array elements marked * are not used by the routine.
*
* Contributed by
* Peter Mayes and Giuseppe Radicati, IBM ECSEC, Rome, March 23, 1989
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param kd
* @param ab
* @param ldab
* @param info
*
*/
abstract public void spbtrf(java.lang.String uplo, int n, int kd, float[] ab, int ldab, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPBTRF computes the Cholesky factorization of a real symmetric
* positive definite band matrix A.
*
* The factorization has the form
* A = U**T * U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular matrix and L is lower triangular.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* AB (input/output) REAL array, dimension (LDAB,N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* On exit, if INFO = 0, the triangular factor U or L from the
* Cholesky factorization A = U**T*U or A = L*L**T of the band
* matrix A, in the same storage format as A.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the leading minor of order i is not
* positive definite, and the factorization could not be
* completed.
*
* Further Details
* ===============
*
* The band storage scheme is illustrated by the following example, when
* N = 6, KD = 2, and UPLO = 'U':
*
* On entry: On exit:
*
* * * a13 a24 a35 a46 * * u13 u24 u35 u46
* * a12 a23 a34 a45 a56 * u12 u23 u34 u45 u56
* a11 a22 a33 a44 a55 a66 u11 u22 u33 u44 u55 u66
*
* Similarly, if UPLO = 'L' the format of A is as follows:
*
* On entry: On exit:
*
* a11 a22 a33 a44 a55 a66 l11 l22 l33 l44 l55 l66
* a21 a32 a43 a54 a65 * l21 l32 l43 l54 l65 *
* a31 a42 a53 a64 * * l31 l42 l53 l64 * *
*
* Array elements marked * are not used by the routine.
*
* Contributed by
* Peter Mayes and Giuseppe Radicati, IBM ECSEC, Rome, March 23, 1989
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param kd
* @param ab
* @param _ab_offset
* @param ldab
* @param info
*
*/
abstract public void spbtrf(java.lang.String uplo, int n, int kd, float[] ab, int _ab_offset, int ldab, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPBTRS solves a system of linear equations A*X = B with a symmetric
* positive definite band matrix A using the Cholesky factorization
* A = U**T*U or A = L*L**T computed by SPBTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangular factor stored in AB;
* = 'L': Lower triangular factor stored in AB.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AB (input) REAL array, dimension (LDAB,N)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T of the band matrix A, stored in the
* first KD+1 rows of the array. The j-th column of U or L is
* stored in the j-th column of the array AB as follows:
* if UPLO ='U', AB(kd+1+i-j,j) = U(i,j) for max(1,j-kd)<=i<=j;
* if UPLO ='L', AB(1+i-j,j) = L(i,j) for j<=i<=min(n,j+kd).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param uplo
* @param n
* @param kd
* @param nrhs
* @param ab
* @param ldab
* @param b
* @param ldb
* @param info
*
*/
abstract public void spbtrs(java.lang.String uplo, int n, int kd, int nrhs, float[] ab, int ldab, float[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPBTRS solves a system of linear equations A*X = B with a symmetric
* positive definite band matrix A using the Cholesky factorization
* A = U**T*U or A = L*L**T computed by SPBTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangular factor stored in AB;
* = 'L': Lower triangular factor stored in AB.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AB (input) REAL array, dimension (LDAB,N)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T of the band matrix A, stored in the
* first KD+1 rows of the array. The j-th column of U or L is
* stored in the j-th column of the array AB as follows:
* if UPLO ='U', AB(kd+1+i-j,j) = U(i,j) for max(1,j-kd)<=i<=j;
* if UPLO ='L', AB(1+i-j,j) = L(i,j) for j<=i<=min(n,j+kd).
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param uplo
* @param n
* @param kd
* @param nrhs
* @param ab
* @param _ab_offset
* @param ldab
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void spbtrs(java.lang.String uplo, int n, int kd, int nrhs, float[] ab, int _ab_offset, int ldab, float[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPOCON estimates the reciprocal of the condition number (in the
* 1-norm) of a real symmetric positive definite matrix using the
* Cholesky factorization A = U**T*U or A = L*L**T computed by SPOTRF.
*
* An estimate is obtained for norm(inv(A)), and the reciprocal of the
* condition number is computed as RCOND = 1 / (ANORM * norm(inv(A))).
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T, as computed by SPOTRF.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* ANORM (input) REAL
* The 1-norm (or infinity-norm) of the symmetric matrix A.
*
* RCOND (output) REAL
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(ANORM * AINVNM), where AINVNM is an
* estimate of the 1-norm of inv(A) computed in this routine.
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param lda
* @param anorm
* @param rcond
* @param work
* @param iwork
* @param info
*
*/
abstract public void spocon(java.lang.String uplo, int n, float[] a, int lda, float anorm, org.netlib.util.floatW rcond, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPOCON estimates the reciprocal of the condition number (in the
* 1-norm) of a real symmetric positive definite matrix using the
* Cholesky factorization A = U**T*U or A = L*L**T computed by SPOTRF.
*
* An estimate is obtained for norm(inv(A)), and the reciprocal of the
* condition number is computed as RCOND = 1 / (ANORM * norm(inv(A))).
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T, as computed by SPOTRF.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* ANORM (input) REAL
* The 1-norm (or infinity-norm) of the symmetric matrix A.
*
* RCOND (output) REAL
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(ANORM * AINVNM), where AINVNM is an
* estimate of the 1-norm of inv(A) computed in this routine.
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param anorm
* @param rcond
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void spocon(java.lang.String uplo, int n, float[] a, int _a_offset, int lda, float anorm, org.netlib.util.floatW rcond, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPOEQU computes row and column scalings intended to equilibrate a
* symmetric positive definite matrix A and reduce its condition number
* (with respect to the two-norm). S contains the scale factors,
* S(i) = 1/sqrt(A(i,i)), chosen so that the scaled matrix B with
* elements B(i,j) = S(i)*A(i,j)*S(j) has ones on the diagonal. This
* choice of S puts the condition number of B within a factor N of the
* smallest possible condition number over all possible diagonal
* scalings.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The N-by-N symmetric positive definite matrix whose scaling
* factors are to be computed. Only the diagonal elements of A
* are referenced.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* S (output) REAL array, dimension (N)
* If INFO = 0, S contains the scale factors for A.
*
* SCOND (output) REAL
* If INFO = 0, S contains the ratio of the smallest S(i) to
* the largest S(i). If SCOND >= 0.1 and AMAX is neither too
* large nor too small, it is not worth scaling by S.
*
* AMAX (output) REAL
* Absolute value of largest matrix element. If AMAX is very
* close to overflow or very close to underflow, the matrix
* should be scaled.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the i-th diagonal element is nonpositive.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param a
* @param lda
* @param s
* @param scond
* @param amax
* @param info
*
*/
abstract public void spoequ(int n, float[] a, int lda, float[] s, org.netlib.util.floatW scond, org.netlib.util.floatW amax, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPOEQU computes row and column scalings intended to equilibrate a
* symmetric positive definite matrix A and reduce its condition number
* (with respect to the two-norm). S contains the scale factors,
* S(i) = 1/sqrt(A(i,i)), chosen so that the scaled matrix B with
* elements B(i,j) = S(i)*A(i,j)*S(j) has ones on the diagonal. This
* choice of S puts the condition number of B within a factor N of the
* smallest possible condition number over all possible diagonal
* scalings.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The N-by-N symmetric positive definite matrix whose scaling
* factors are to be computed. Only the diagonal elements of A
* are referenced.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* S (output) REAL array, dimension (N)
* If INFO = 0, S contains the scale factors for A.
*
* SCOND (output) REAL
* If INFO = 0, S contains the ratio of the smallest S(i) to
* the largest S(i). If SCOND >= 0.1 and AMAX is neither too
* large nor too small, it is not worth scaling by S.
*
* AMAX (output) REAL
* Absolute value of largest matrix element. If AMAX is very
* close to overflow or very close to underflow, the matrix
* should be scaled.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the i-th diagonal element is nonpositive.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param a
* @param _a_offset
* @param lda
* @param s
* @param _s_offset
* @param scond
* @param amax
* @param info
*
*/
abstract public void spoequ(int n, float[] a, int _a_offset, int lda, float[] s, int _s_offset, org.netlib.util.floatW scond, org.netlib.util.floatW amax, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPORFS improves the computed solution to a system of linear
* equations when the coefficient matrix is symmetric positive definite,
* and provides error bounds and backward error estimates for the
* solution.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The symmetric matrix A. If UPLO = 'U', the leading N-by-N
* upper triangular part of A contains the upper triangular part
* of the matrix A, and the strictly lower triangular part of A
* is not referenced. If UPLO = 'L', the leading N-by-N lower
* triangular part of A contains the lower triangular part of
* the matrix A, and the strictly upper triangular part of A is
* not referenced.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* AF (input) REAL array, dimension (LDAF,N)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T, as computed by SPOTRF.
*
* LDAF (input) INTEGER
* The leading dimension of the array AF. LDAF >= max(1,N).
*
* B (input) REAL array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) REAL array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by SPOTRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param a
* @param lda
* @param af
* @param ldaf
* @param b
* @param ldb
* @param x
* @param ldx
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void sporfs(java.lang.String uplo, int n, int nrhs, float[] a, int lda, float[] af, int ldaf, float[] b, int ldb, float[] x, int ldx, float[] ferr, float[] berr, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPORFS improves the computed solution to a system of linear
* equations when the coefficient matrix is symmetric positive definite,
* and provides error bounds and backward error estimates for the
* solution.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The symmetric matrix A. If UPLO = 'U', the leading N-by-N
* upper triangular part of A contains the upper triangular part
* of the matrix A, and the strictly lower triangular part of A
* is not referenced. If UPLO = 'L', the leading N-by-N lower
* triangular part of A contains the lower triangular part of
* the matrix A, and the strictly upper triangular part of A is
* not referenced.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* AF (input) REAL array, dimension (LDAF,N)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T, as computed by SPOTRF.
*
* LDAF (input) INTEGER
* The leading dimension of the array AF. LDAF >= max(1,N).
*
* B (input) REAL array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) REAL array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by SPOTRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param af
* @param _af_offset
* @param ldaf
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void sporfs(java.lang.String uplo, int n, int nrhs, float[] a, int _a_offset, int lda, float[] af, int _af_offset, int ldaf, float[] b, int _b_offset, int ldb, float[] x, int _x_offset, int ldx, float[] ferr, int _ferr_offset, float[] berr, int _berr_offset, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPOSV computes the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N symmetric positive definite matrix and X and B
* are N-by-NRHS matrices.
*
* The Cholesky decomposition is used to factor A as
* A = U**T* U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular matrix and L is a lower triangular
* matrix. The factored form of A is then used to solve the system of
* equations A * X = B.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* N-by-N upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading N-by-N lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, if INFO = 0, the factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if INFO = 0, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the leading minor of order i of A is not
* positive definite, so the factorization could not be
* completed, and the solution has not been computed.
*
* =====================================================================
*
* .. External Functions ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param a
* @param lda
* @param b
* @param ldb
* @param info
*
*/
abstract public void sposv(java.lang.String uplo, int n, int nrhs, float[] a, int lda, float[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPOSV computes the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N symmetric positive definite matrix and X and B
* are N-by-NRHS matrices.
*
* The Cholesky decomposition is used to factor A as
* A = U**T* U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular matrix and L is a lower triangular
* matrix. The factored form of A is then used to solve the system of
* equations A * X = B.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* N-by-N upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading N-by-N lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, if INFO = 0, the factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if INFO = 0, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the leading minor of order i of A is not
* positive definite, so the factorization could not be
* completed, and the solution has not been computed.
*
* =====================================================================
*
* .. External Functions ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void sposv(java.lang.String uplo, int n, int nrhs, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPOSVX uses the Cholesky factorization A = U**T*U or A = L*L**T to
* compute the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N symmetric positive definite matrix and X and B
* are N-by-NRHS matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed:
*
* 1. If FACT = 'E', real scaling factors are computed to equilibrate
* the system:
* diag(S) * A * diag(S) * inv(diag(S)) * X = diag(S) * B
* Whether or not the system will be equilibrated depends on the
* scaling of the matrix A, but if equilibration is used, A is
* overwritten by diag(S)*A*diag(S) and B by diag(S)*B.
*
* 2. If FACT = 'N' or 'E', the Cholesky decomposition is used to
* factor the matrix A (after equilibration if FACT = 'E') as
* A = U**T* U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular matrix and L is a lower triangular
* matrix.
*
* 3. If the leading i-by-i principal minor is not positive definite,
* then the routine returns with INFO = i. Otherwise, the factored
* form of A is used to estimate the condition number of the matrix
* A. If the reciprocal of the condition number is less than machine
* precision, INFO = N+1 is returned as a warning, but the routine
* still goes on to solve for X and compute error bounds as
* described below.
*
* 4. The system of equations is solved for X using the factored form
* of A.
*
* 5. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* 6. If equilibration was used, the matrix X is premultiplied by
* diag(S) so that it solves the original system before
* equilibration.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of the matrix A is
* supplied on entry, and if not, whether the matrix A should be
* equilibrated before it is factored.
* = 'F': On entry, AF contains the factored form of A.
* If EQUED = 'Y', the matrix A has been equilibrated
* with scaling factors given by S. A and AF will not
* be modified.
* = 'N': The matrix A will be copied to AF and factored.
* = 'E': The matrix A will be equilibrated if necessary, then
* copied to AF and factored.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the symmetric matrix A, except if FACT = 'F' and
* EQUED = 'Y', then A must contain the equilibrated matrix
* diag(S)*A*diag(S). If UPLO = 'U', the leading
* N-by-N upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading N-by-N lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced. A is not modified if
* FACT = 'F' or 'N', or if FACT = 'E' and EQUED = 'N' on exit.
*
* On exit, if FACT = 'E' and EQUED = 'Y', A is overwritten by
* diag(S)*A*diag(S).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* AF (input or output) REAL array, dimension (LDAF,N)
* If FACT = 'F', then AF is an input argument and on entry
* contains the triangular factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T, in the same storage
* format as A. If EQUED .ne. 'N', then AF is the factored form
* of the equilibrated matrix diag(S)*A*diag(S).
*
* If FACT = 'N', then AF is an output argument and on exit
* returns the triangular factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T of the original
* matrix A.
*
* If FACT = 'E', then AF is an output argument and on exit
* returns the triangular factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T of the equilibrated
* matrix A (see the description of A for the form of the
* equilibrated matrix).
*
* LDAF (input) INTEGER
* The leading dimension of the array AF. LDAF >= max(1,N).
*
* EQUED (input or output) CHARACTER*1
* Specifies the form of equilibration that was done.
* = 'N': No equilibration (always true if FACT = 'N').
* = 'Y': Equilibration was done, i.e., A has been replaced by
* diag(S) * A * diag(S).
* EQUED is an input argument if FACT = 'F'; otherwise, it is an
* output argument.
*
* S (input or output) REAL array, dimension (N)
* The scale factors for A; not accessed if EQUED = 'N'. S is
* an input argument if FACT = 'F'; otherwise, S is an output
* argument. If FACT = 'F' and EQUED = 'Y', each element of S
* must be positive.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if EQUED = 'N', B is not modified; if EQUED = 'Y',
* B is overwritten by diag(S) * B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) REAL array, dimension (LDX,NRHS)
* If INFO = 0 or INFO = N+1, the N-by-NRHS solution matrix X to
* the original system of equations. Note that if EQUED = 'Y',
* A and B are modified on exit, and the solution to the
* equilibrated system is inv(diag(S))*X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) REAL
* The estimate of the reciprocal condition number of the matrix
* A after equilibration (if done). If RCOND is less than the
* machine precision (in particular, if RCOND = 0), the matrix
* is singular to working precision. This condition is
* indicated by a return code of INFO > 0.
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= N: the leading minor of order i of A is
* not positive definite, so the factorization
* could not be completed, and the solution has not
* been computed. RCOND = 0 is returned.
* = N+1: U is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param uplo
* @param n
* @param nrhs
* @param a
* @param lda
* @param af
* @param ldaf
* @param equed
* @param s
* @param b
* @param ldb
* @param x
* @param ldx
* @param rcond
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void sposvx(java.lang.String fact, java.lang.String uplo, int n, int nrhs, float[] a, int lda, float[] af, int ldaf, org.netlib.util.StringW equed, float[] s, float[] b, int ldb, float[] x, int ldx, org.netlib.util.floatW rcond, float[] ferr, float[] berr, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPOSVX uses the Cholesky factorization A = U**T*U or A = L*L**T to
* compute the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N symmetric positive definite matrix and X and B
* are N-by-NRHS matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed:
*
* 1. If FACT = 'E', real scaling factors are computed to equilibrate
* the system:
* diag(S) * A * diag(S) * inv(diag(S)) * X = diag(S) * B
* Whether or not the system will be equilibrated depends on the
* scaling of the matrix A, but if equilibration is used, A is
* overwritten by diag(S)*A*diag(S) and B by diag(S)*B.
*
* 2. If FACT = 'N' or 'E', the Cholesky decomposition is used to
* factor the matrix A (after equilibration if FACT = 'E') as
* A = U**T* U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular matrix and L is a lower triangular
* matrix.
*
* 3. If the leading i-by-i principal minor is not positive definite,
* then the routine returns with INFO = i. Otherwise, the factored
* form of A is used to estimate the condition number of the matrix
* A. If the reciprocal of the condition number is less than machine
* precision, INFO = N+1 is returned as a warning, but the routine
* still goes on to solve for X and compute error bounds as
* described below.
*
* 4. The system of equations is solved for X using the factored form
* of A.
*
* 5. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* 6. If equilibration was used, the matrix X is premultiplied by
* diag(S) so that it solves the original system before
* equilibration.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of the matrix A is
* supplied on entry, and if not, whether the matrix A should be
* equilibrated before it is factored.
* = 'F': On entry, AF contains the factored form of A.
* If EQUED = 'Y', the matrix A has been equilibrated
* with scaling factors given by S. A and AF will not
* be modified.
* = 'N': The matrix A will be copied to AF and factored.
* = 'E': The matrix A will be equilibrated if necessary, then
* copied to AF and factored.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the symmetric matrix A, except if FACT = 'F' and
* EQUED = 'Y', then A must contain the equilibrated matrix
* diag(S)*A*diag(S). If UPLO = 'U', the leading
* N-by-N upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading N-by-N lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced. A is not modified if
* FACT = 'F' or 'N', or if FACT = 'E' and EQUED = 'N' on exit.
*
* On exit, if FACT = 'E' and EQUED = 'Y', A is overwritten by
* diag(S)*A*diag(S).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* AF (input or output) REAL array, dimension (LDAF,N)
* If FACT = 'F', then AF is an input argument and on entry
* contains the triangular factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T, in the same storage
* format as A. If EQUED .ne. 'N', then AF is the factored form
* of the equilibrated matrix diag(S)*A*diag(S).
*
* If FACT = 'N', then AF is an output argument and on exit
* returns the triangular factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T of the original
* matrix A.
*
* If FACT = 'E', then AF is an output argument and on exit
* returns the triangular factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T of the equilibrated
* matrix A (see the description of A for the form of the
* equilibrated matrix).
*
* LDAF (input) INTEGER
* The leading dimension of the array AF. LDAF >= max(1,N).
*
* EQUED (input or output) CHARACTER*1
* Specifies the form of equilibration that was done.
* = 'N': No equilibration (always true if FACT = 'N').
* = 'Y': Equilibration was done, i.e., A has been replaced by
* diag(S) * A * diag(S).
* EQUED is an input argument if FACT = 'F'; otherwise, it is an
* output argument.
*
* S (input or output) REAL array, dimension (N)
* The scale factors for A; not accessed if EQUED = 'N'. S is
* an input argument if FACT = 'F'; otherwise, S is an output
* argument. If FACT = 'F' and EQUED = 'Y', each element of S
* must be positive.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if EQUED = 'N', B is not modified; if EQUED = 'Y',
* B is overwritten by diag(S) * B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) REAL array, dimension (LDX,NRHS)
* If INFO = 0 or INFO = N+1, the N-by-NRHS solution matrix X to
* the original system of equations. Note that if EQUED = 'Y',
* A and B are modified on exit, and the solution to the
* equilibrated system is inv(diag(S))*X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) REAL
* The estimate of the reciprocal condition number of the matrix
* A after equilibration (if done). If RCOND is less than the
* machine precision (in particular, if RCOND = 0), the matrix
* is singular to working precision. This condition is
* indicated by a return code of INFO > 0.
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= N: the leading minor of order i of A is
* not positive definite, so the factorization
* could not be completed, and the solution has not
* been computed. RCOND = 0 is returned.
* = N+1: U is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param uplo
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param af
* @param _af_offset
* @param ldaf
* @param equed
* @param s
* @param _s_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param rcond
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void sposvx(java.lang.String fact, java.lang.String uplo, int n, int nrhs, float[] a, int _a_offset, int lda, float[] af, int _af_offset, int ldaf, org.netlib.util.StringW equed, float[] s, int _s_offset, float[] b, int _b_offset, int ldb, float[] x, int _x_offset, int ldx, org.netlib.util.floatW rcond, float[] ferr, int _ferr_offset, float[] berr, int _berr_offset, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPOTF2 computes the Cholesky factorization of a real symmetric
* positive definite matrix A.
*
* The factorization has the form
* A = U' * U , if UPLO = 'U', or
* A = L * L', if UPLO = 'L',
* where U is an upper triangular matrix and L is lower triangular.
*
* This is the unblocked version of the algorithm, calling Level 2 BLAS.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* n by n upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading n by n lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, if INFO = 0, the factor U or L from the Cholesky
* factorization A = U'*U or A = L*L'.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
* > 0: if INFO = k, the leading minor of order k is not
* positive definite, and the factorization could not be
* completed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param lda
* @param info
*
*/
abstract public void spotf2(java.lang.String uplo, int n, float[] a, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPOTF2 computes the Cholesky factorization of a real symmetric
* positive definite matrix A.
*
* The factorization has the form
* A = U' * U , if UPLO = 'U', or
* A = L * L', if UPLO = 'L',
* where U is an upper triangular matrix and L is lower triangular.
*
* This is the unblocked version of the algorithm, calling Level 2 BLAS.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* n by n upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading n by n lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, if INFO = 0, the factor U or L from the Cholesky
* factorization A = U'*U or A = L*L'.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
* > 0: if INFO = k, the leading minor of order k is not
* positive definite, and the factorization could not be
* completed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param info
*
*/
abstract public void spotf2(java.lang.String uplo, int n, float[] a, int _a_offset, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPOTRF computes the Cholesky factorization of a real symmetric
* positive definite matrix A.
*
* The factorization has the form
* A = U**T * U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular matrix and L is lower triangular.
*
* This is the block version of the algorithm, calling Level 3 BLAS.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* N-by-N upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading N-by-N lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, if INFO = 0, the factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the leading minor of order i is not
* positive definite, and the factorization could not be
* completed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param lda
* @param info
*
*/
abstract public void spotrf(java.lang.String uplo, int n, float[] a, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPOTRF computes the Cholesky factorization of a real symmetric
* positive definite matrix A.
*
* The factorization has the form
* A = U**T * U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular matrix and L is lower triangular.
*
* This is the block version of the algorithm, calling Level 3 BLAS.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* N-by-N upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading N-by-N lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, if INFO = 0, the factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the leading minor of order i is not
* positive definite, and the factorization could not be
* completed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param info
*
*/
abstract public void spotrf(java.lang.String uplo, int n, float[] a, int _a_offset, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPOTRI computes the inverse of a real symmetric positive definite
* matrix A using the Cholesky factorization A = U**T*U or A = L*L**T
* computed by SPOTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the triangular factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T, as computed by
* SPOTRF.
* On exit, the upper or lower triangle of the (symmetric)
* inverse of A, overwriting the input factor U or L.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the (i,i) element of the factor U or L is
* zero, and the inverse could not be computed.
*
* =====================================================================
*
* .. External Functions ..
*
*
* @param uplo
* @param n
* @param a
* @param lda
* @param info
*
*/
abstract public void spotri(java.lang.String uplo, int n, float[] a, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPOTRI computes the inverse of a real symmetric positive definite
* matrix A using the Cholesky factorization A = U**T*U or A = L*L**T
* computed by SPOTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the triangular factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T, as computed by
* SPOTRF.
* On exit, the upper or lower triangle of the (symmetric)
* inverse of A, overwriting the input factor U or L.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the (i,i) element of the factor U or L is
* zero, and the inverse could not be computed.
*
* =====================================================================
*
* .. External Functions ..
*
*
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param info
*
*/
abstract public void spotri(java.lang.String uplo, int n, float[] a, int _a_offset, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPOTRS solves a system of linear equations A*X = B with a symmetric
* positive definite matrix A using the Cholesky factorization
* A = U**T*U or A = L*L**T computed by SPOTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T, as computed by SPOTRF.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param a
* @param lda
* @param b
* @param ldb
* @param info
*
*/
abstract public void spotrs(java.lang.String uplo, int n, int nrhs, float[] a, int lda, float[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPOTRS solves a system of linear equations A*X = B with a symmetric
* positive definite matrix A using the Cholesky factorization
* A = U**T*U or A = L*L**T computed by SPOTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T, as computed by SPOTRF.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void spotrs(java.lang.String uplo, int n, int nrhs, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPPCON estimates the reciprocal of the condition number (in the
* 1-norm) of a real symmetric positive definite packed matrix using
* the Cholesky factorization A = U**T*U or A = L*L**T computed by
* SPPTRF.
*
* An estimate is obtained for norm(inv(A)), and the reciprocal of the
* condition number is computed as RCOND = 1 / (ANORM * norm(inv(A))).
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input) REAL array, dimension (N*(N+1)/2)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T, packed columnwise in a linear
* array. The j-th column of U or L is stored in the array AP
* as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = U(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = L(i,j) for j<=i<=n.
*
* ANORM (input) REAL
* The 1-norm (or infinity-norm) of the symmetric matrix A.
*
* RCOND (output) REAL
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(ANORM * AINVNM), where AINVNM is an
* estimate of the 1-norm of inv(A) computed in this routine.
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param anorm
* @param rcond
* @param work
* @param iwork
* @param info
*
*/
abstract public void sppcon(java.lang.String uplo, int n, float[] ap, float anorm, org.netlib.util.floatW rcond, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPPCON estimates the reciprocal of the condition number (in the
* 1-norm) of a real symmetric positive definite packed matrix using
* the Cholesky factorization A = U**T*U or A = L*L**T computed by
* SPPTRF.
*
* An estimate is obtained for norm(inv(A)), and the reciprocal of the
* condition number is computed as RCOND = 1 / (ANORM * norm(inv(A))).
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input) REAL array, dimension (N*(N+1)/2)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T, packed columnwise in a linear
* array. The j-th column of U or L is stored in the array AP
* as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = U(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = L(i,j) for j<=i<=n.
*
* ANORM (input) REAL
* The 1-norm (or infinity-norm) of the symmetric matrix A.
*
* RCOND (output) REAL
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(ANORM * AINVNM), where AINVNM is an
* estimate of the 1-norm of inv(A) computed in this routine.
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param anorm
* @param rcond
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void sppcon(java.lang.String uplo, int n, float[] ap, int _ap_offset, float anorm, org.netlib.util.floatW rcond, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPPEQU computes row and column scalings intended to equilibrate a
* symmetric positive definite matrix A in packed storage and reduce
* its condition number (with respect to the two-norm). S contains the
* scale factors, S(i)=1/sqrt(A(i,i)), chosen so that the scaled matrix
* B with elements B(i,j)=S(i)*A(i,j)*S(j) has ones on the diagonal.
* This choice of S puts the condition number of B within a factor N of
* the smallest possible condition number over all possible diagonal
* scalings.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input) REAL array, dimension (N*(N+1)/2)
* The upper or lower triangle of the symmetric matrix A, packed
* columnwise in a linear array. The j-th column of A is stored
* in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
*
* S (output) REAL array, dimension (N)
* If INFO = 0, S contains the scale factors for A.
*
* SCOND (output) REAL
* If INFO = 0, S contains the ratio of the smallest S(i) to
* the largest S(i). If SCOND >= 0.1 and AMAX is neither too
* large nor too small, it is not worth scaling by S.
*
* AMAX (output) REAL
* Absolute value of largest matrix element. If AMAX is very
* close to overflow or very close to underflow, the matrix
* should be scaled.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the i-th diagonal element is nonpositive.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param s
* @param scond
* @param amax
* @param info
*
*/
abstract public void sppequ(java.lang.String uplo, int n, float[] ap, float[] s, org.netlib.util.floatW scond, org.netlib.util.floatW amax, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPPEQU computes row and column scalings intended to equilibrate a
* symmetric positive definite matrix A in packed storage and reduce
* its condition number (with respect to the two-norm). S contains the
* scale factors, S(i)=1/sqrt(A(i,i)), chosen so that the scaled matrix
* B with elements B(i,j)=S(i)*A(i,j)*S(j) has ones on the diagonal.
* This choice of S puts the condition number of B within a factor N of
* the smallest possible condition number over all possible diagonal
* scalings.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input) REAL array, dimension (N*(N+1)/2)
* The upper or lower triangle of the symmetric matrix A, packed
* columnwise in a linear array. The j-th column of A is stored
* in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
*
* S (output) REAL array, dimension (N)
* If INFO = 0, S contains the scale factors for A.
*
* SCOND (output) REAL
* If INFO = 0, S contains the ratio of the smallest S(i) to
* the largest S(i). If SCOND >= 0.1 and AMAX is neither too
* large nor too small, it is not worth scaling by S.
*
* AMAX (output) REAL
* Absolute value of largest matrix element. If AMAX is very
* close to overflow or very close to underflow, the matrix
* should be scaled.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the i-th diagonal element is nonpositive.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param s
* @param _s_offset
* @param scond
* @param amax
* @param info
*
*/
abstract public void sppequ(java.lang.String uplo, int n, float[] ap, int _ap_offset, float[] s, int _s_offset, org.netlib.util.floatW scond, org.netlib.util.floatW amax, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPPRFS improves the computed solution to a system of linear
* equations when the coefficient matrix is symmetric positive definite
* and packed, and provides error bounds and backward error estimates
* for the solution.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AP (input) REAL array, dimension (N*(N+1)/2)
* The upper or lower triangle of the symmetric matrix A, packed
* columnwise in a linear array. The j-th column of A is stored
* in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
*
* AFP (input) REAL array, dimension (N*(N+1)/2)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T, as computed by SPPTRF/CPPTRF,
* packed columnwise in a linear array in the same format as A
* (see AP).
*
* B (input) REAL array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) REAL array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by SPPTRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param ap
* @param afp
* @param b
* @param ldb
* @param x
* @param ldx
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void spprfs(java.lang.String uplo, int n, int nrhs, float[] ap, float[] afp, float[] b, int ldb, float[] x, int ldx, float[] ferr, float[] berr, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPPRFS improves the computed solution to a system of linear
* equations when the coefficient matrix is symmetric positive definite
* and packed, and provides error bounds and backward error estimates
* for the solution.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AP (input) REAL array, dimension (N*(N+1)/2)
* The upper or lower triangle of the symmetric matrix A, packed
* columnwise in a linear array. The j-th column of A is stored
* in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
*
* AFP (input) REAL array, dimension (N*(N+1)/2)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T, as computed by SPPTRF/CPPTRF,
* packed columnwise in a linear array in the same format as A
* (see AP).
*
* B (input) REAL array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) REAL array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by SPPTRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param ap
* @param _ap_offset
* @param afp
* @param _afp_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void spprfs(java.lang.String uplo, int n, int nrhs, float[] ap, int _ap_offset, float[] afp, int _afp_offset, float[] b, int _b_offset, int ldb, float[] x, int _x_offset, int ldx, float[] ferr, int _ferr_offset, float[] berr, int _berr_offset, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPPSV computes the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N symmetric positive definite matrix stored in
* packed format and X and B are N-by-NRHS matrices.
*
* The Cholesky decomposition is used to factor A as
* A = U**T* U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular matrix and L is a lower triangular
* matrix. The factored form of A is then used to solve the system of
* equations A * X = B.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
* See below for further details.
*
* On exit, if INFO = 0, the factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T, in the same storage
* format as A.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if INFO = 0, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the leading minor of order i of A is not
* positive definite, so the factorization could not be
* completed, and the solution has not been computed.
*
* Further Details
* ===============
*
* The packed storage scheme is illustrated by the following example
* when N = 4, UPLO = 'U':
*
* Two-dimensional storage of the symmetric matrix A:
*
* a11 a12 a13 a14
* a22 a23 a24
* a33 a34 (aij = conjg(aji))
* a44
*
* Packed storage of the upper triangle of A:
*
* AP = [ a11, a12, a22, a13, a23, a33, a14, a24, a34, a44 ]
*
* =====================================================================
*
* .. External Functions ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param ap
* @param b
* @param ldb
* @param info
*
*/
abstract public void sppsv(java.lang.String uplo, int n, int nrhs, float[] ap, float[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPPSV computes the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N symmetric positive definite matrix stored in
* packed format and X and B are N-by-NRHS matrices.
*
* The Cholesky decomposition is used to factor A as
* A = U**T* U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular matrix and L is a lower triangular
* matrix. The factored form of A is then used to solve the system of
* equations A * X = B.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
* See below for further details.
*
* On exit, if INFO = 0, the factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T, in the same storage
* format as A.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if INFO = 0, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the leading minor of order i of A is not
* positive definite, so the factorization could not be
* completed, and the solution has not been computed.
*
* Further Details
* ===============
*
* The packed storage scheme is illustrated by the following example
* when N = 4, UPLO = 'U':
*
* Two-dimensional storage of the symmetric matrix A:
*
* a11 a12 a13 a14
* a22 a23 a24
* a33 a34 (aij = conjg(aji))
* a44
*
* Packed storage of the upper triangle of A:
*
* AP = [ a11, a12, a22, a13, a23, a33, a14, a24, a34, a44 ]
*
* =====================================================================
*
* .. External Functions ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param ap
* @param _ap_offset
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void sppsv(java.lang.String uplo, int n, int nrhs, float[] ap, int _ap_offset, float[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPPSVX uses the Cholesky factorization A = U**T*U or A = L*L**T to
* compute the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N symmetric positive definite matrix stored in
* packed format and X and B are N-by-NRHS matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed:
*
* 1. If FACT = 'E', real scaling factors are computed to equilibrate
* the system:
* diag(S) * A * diag(S) * inv(diag(S)) * X = diag(S) * B
* Whether or not the system will be equilibrated depends on the
* scaling of the matrix A, but if equilibration is used, A is
* overwritten by diag(S)*A*diag(S) and B by diag(S)*B.
*
* 2. If FACT = 'N' or 'E', the Cholesky decomposition is used to
* factor the matrix A (after equilibration if FACT = 'E') as
* A = U**T* U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular matrix and L is a lower triangular
* matrix.
*
* 3. If the leading i-by-i principal minor is not positive definite,
* then the routine returns with INFO = i. Otherwise, the factored
* form of A is used to estimate the condition number of the matrix
* A. If the reciprocal of the condition number is less than machine
* precision, INFO = N+1 is returned as a warning, but the routine
* still goes on to solve for X and compute error bounds as
* described below.
*
* 4. The system of equations is solved for X using the factored form
* of A.
*
* 5. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* 6. If equilibration was used, the matrix X is premultiplied by
* diag(S) so that it solves the original system before
* equilibration.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of the matrix A is
* supplied on entry, and if not, whether the matrix A should be
* equilibrated before it is factored.
* = 'F': On entry, AFP contains the factored form of A.
* If EQUED = 'Y', the matrix A has been equilibrated
* with scaling factors given by S. AP and AFP will not
* be modified.
* = 'N': The matrix A will be copied to AFP and factored.
* = 'E': The matrix A will be equilibrated if necessary, then
* copied to AFP and factored.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array, except if FACT = 'F'
* and EQUED = 'Y', then A must contain the equilibrated matrix
* diag(S)*A*diag(S). The j-th column of A is stored in the
* array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
* See below for further details. A is not modified if
* FACT = 'F' or 'N', or if FACT = 'E' and EQUED = 'N' on exit.
*
* On exit, if FACT = 'E' and EQUED = 'Y', A is overwritten by
* diag(S)*A*diag(S).
*
* AFP (input or output) REAL array, dimension
* (N*(N+1)/2)
* If FACT = 'F', then AFP is an input argument and on entry
* contains the triangular factor U or L from the Cholesky
* factorization A = U'*U or A = L*L', in the same storage
* format as A. If EQUED .ne. 'N', then AFP is the factored
* form of the equilibrated matrix A.
*
* If FACT = 'N', then AFP is an output argument and on exit
* returns the triangular factor U or L from the Cholesky
* factorization A = U'*U or A = L*L' of the original matrix A.
*
* If FACT = 'E', then AFP is an output argument and on exit
* returns the triangular factor U or L from the Cholesky
* factorization A = U'*U or A = L*L' of the equilibrated
* matrix A (see the description of AP for the form of the
* equilibrated matrix).
*
* EQUED (input or output) CHARACTER*1
* Specifies the form of equilibration that was done.
* = 'N': No equilibration (always true if FACT = 'N').
* = 'Y': Equilibration was done, i.e., A has been replaced by
* diag(S) * A * diag(S).
* EQUED is an input argument if FACT = 'F'; otherwise, it is an
* output argument.
*
* S (input or output) REAL array, dimension (N)
* The scale factors for A; not accessed if EQUED = 'N'. S is
* an input argument if FACT = 'F'; otherwise, S is an output
* argument. If FACT = 'F' and EQUED = 'Y', each element of S
* must be positive.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if EQUED = 'N', B is not modified; if EQUED = 'Y',
* B is overwritten by diag(S) * B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) REAL array, dimension (LDX,NRHS)
* If INFO = 0 or INFO = N+1, the N-by-NRHS solution matrix X to
* the original system of equations. Note that if EQUED = 'Y',
* A and B are modified on exit, and the solution to the
* equilibrated system is inv(diag(S))*X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) REAL
* The estimate of the reciprocal condition number of the matrix
* A after equilibration (if done). If RCOND is less than the
* machine precision (in particular, if RCOND = 0), the matrix
* is singular to working precision. This condition is
* indicated by a return code of INFO > 0.
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= N: the leading minor of order i of A is
* not positive definite, so the factorization
* could not be completed, and the solution has not
* been computed. RCOND = 0 is returned.
* = N+1: U is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* Further Details
* ===============
*
* The packed storage scheme is illustrated by the following example
* when N = 4, UPLO = 'U':
*
* Two-dimensional storage of the symmetric matrix A:
*
* a11 a12 a13 a14
* a22 a23 a24
* a33 a34 (aij = conjg(aji))
* a44
*
* Packed storage of the upper triangle of A:
*
* AP = [ a11, a12, a22, a13, a23, a33, a14, a24, a34, a44 ]
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param uplo
* @param n
* @param nrhs
* @param ap
* @param afp
* @param equed
* @param s
* @param b
* @param ldb
* @param x
* @param ldx
* @param rcond
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void sppsvx(java.lang.String fact, java.lang.String uplo, int n, int nrhs, float[] ap, float[] afp, org.netlib.util.StringW equed, float[] s, float[] b, int ldb, float[] x, int ldx, org.netlib.util.floatW rcond, float[] ferr, float[] berr, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPPSVX uses the Cholesky factorization A = U**T*U or A = L*L**T to
* compute the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N symmetric positive definite matrix stored in
* packed format and X and B are N-by-NRHS matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed:
*
* 1. If FACT = 'E', real scaling factors are computed to equilibrate
* the system:
* diag(S) * A * diag(S) * inv(diag(S)) * X = diag(S) * B
* Whether or not the system will be equilibrated depends on the
* scaling of the matrix A, but if equilibration is used, A is
* overwritten by diag(S)*A*diag(S) and B by diag(S)*B.
*
* 2. If FACT = 'N' or 'E', the Cholesky decomposition is used to
* factor the matrix A (after equilibration if FACT = 'E') as
* A = U**T* U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular matrix and L is a lower triangular
* matrix.
*
* 3. If the leading i-by-i principal minor is not positive definite,
* then the routine returns with INFO = i. Otherwise, the factored
* form of A is used to estimate the condition number of the matrix
* A. If the reciprocal of the condition number is less than machine
* precision, INFO = N+1 is returned as a warning, but the routine
* still goes on to solve for X and compute error bounds as
* described below.
*
* 4. The system of equations is solved for X using the factored form
* of A.
*
* 5. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* 6. If equilibration was used, the matrix X is premultiplied by
* diag(S) so that it solves the original system before
* equilibration.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of the matrix A is
* supplied on entry, and if not, whether the matrix A should be
* equilibrated before it is factored.
* = 'F': On entry, AFP contains the factored form of A.
* If EQUED = 'Y', the matrix A has been equilibrated
* with scaling factors given by S. AP and AFP will not
* be modified.
* = 'N': The matrix A will be copied to AFP and factored.
* = 'E': The matrix A will be equilibrated if necessary, then
* copied to AFP and factored.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array, except if FACT = 'F'
* and EQUED = 'Y', then A must contain the equilibrated matrix
* diag(S)*A*diag(S). The j-th column of A is stored in the
* array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
* See below for further details. A is not modified if
* FACT = 'F' or 'N', or if FACT = 'E' and EQUED = 'N' on exit.
*
* On exit, if FACT = 'E' and EQUED = 'Y', A is overwritten by
* diag(S)*A*diag(S).
*
* AFP (input or output) REAL array, dimension
* (N*(N+1)/2)
* If FACT = 'F', then AFP is an input argument and on entry
* contains the triangular factor U or L from the Cholesky
* factorization A = U'*U or A = L*L', in the same storage
* format as A. If EQUED .ne. 'N', then AFP is the factored
* form of the equilibrated matrix A.
*
* If FACT = 'N', then AFP is an output argument and on exit
* returns the triangular factor U or L from the Cholesky
* factorization A = U'*U or A = L*L' of the original matrix A.
*
* If FACT = 'E', then AFP is an output argument and on exit
* returns the triangular factor U or L from the Cholesky
* factorization A = U'*U or A = L*L' of the equilibrated
* matrix A (see the description of AP for the form of the
* equilibrated matrix).
*
* EQUED (input or output) CHARACTER*1
* Specifies the form of equilibration that was done.
* = 'N': No equilibration (always true if FACT = 'N').
* = 'Y': Equilibration was done, i.e., A has been replaced by
* diag(S) * A * diag(S).
* EQUED is an input argument if FACT = 'F'; otherwise, it is an
* output argument.
*
* S (input or output) REAL array, dimension (N)
* The scale factors for A; not accessed if EQUED = 'N'. S is
* an input argument if FACT = 'F'; otherwise, S is an output
* argument. If FACT = 'F' and EQUED = 'Y', each element of S
* must be positive.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if EQUED = 'N', B is not modified; if EQUED = 'Y',
* B is overwritten by diag(S) * B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) REAL array, dimension (LDX,NRHS)
* If INFO = 0 or INFO = N+1, the N-by-NRHS solution matrix X to
* the original system of equations. Note that if EQUED = 'Y',
* A and B are modified on exit, and the solution to the
* equilibrated system is inv(diag(S))*X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) REAL
* The estimate of the reciprocal condition number of the matrix
* A after equilibration (if done). If RCOND is less than the
* machine precision (in particular, if RCOND = 0), the matrix
* is singular to working precision. This condition is
* indicated by a return code of INFO > 0.
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= N: the leading minor of order i of A is
* not positive definite, so the factorization
* could not be completed, and the solution has not
* been computed. RCOND = 0 is returned.
* = N+1: U is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* Further Details
* ===============
*
* The packed storage scheme is illustrated by the following example
* when N = 4, UPLO = 'U':
*
* Two-dimensional storage of the symmetric matrix A:
*
* a11 a12 a13 a14
* a22 a23 a24
* a33 a34 (aij = conjg(aji))
* a44
*
* Packed storage of the upper triangle of A:
*
* AP = [ a11, a12, a22, a13, a23, a33, a14, a24, a34, a44 ]
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param uplo
* @param n
* @param nrhs
* @param ap
* @param _ap_offset
* @param afp
* @param _afp_offset
* @param equed
* @param s
* @param _s_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param rcond
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void sppsvx(java.lang.String fact, java.lang.String uplo, int n, int nrhs, float[] ap, int _ap_offset, float[] afp, int _afp_offset, org.netlib.util.StringW equed, float[] s, int _s_offset, float[] b, int _b_offset, int ldb, float[] x, int _x_offset, int ldx, org.netlib.util.floatW rcond, float[] ferr, int _ferr_offset, float[] berr, int _berr_offset, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPPTRF computes the Cholesky factorization of a real symmetric
* positive definite matrix A stored in packed format.
*
* The factorization has the form
* A = U**T * U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular matrix and L is lower triangular.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
* See below for further details.
*
* On exit, if INFO = 0, the triangular factor U or L from the
* Cholesky factorization A = U**T*U or A = L*L**T, in the same
* storage format as A.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the leading minor of order i is not
* positive definite, and the factorization could not be
* completed.
*
* Further Details
* ======= =======
*
* The packed storage scheme is illustrated by the following example
* when N = 4, UPLO = 'U':
*
* Two-dimensional storage of the symmetric matrix A:
*
* a11 a12 a13 a14
* a22 a23 a24
* a33 a34 (aij = aji)
* a44
*
* Packed storage of the upper triangle of A:
*
* AP = [ a11, a12, a22, a13, a23, a33, a14, a24, a34, a44 ]
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param info
*
*/
abstract public void spptrf(java.lang.String uplo, int n, float[] ap, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPPTRF computes the Cholesky factorization of a real symmetric
* positive definite matrix A stored in packed format.
*
* The factorization has the form
* A = U**T * U, if UPLO = 'U', or
* A = L * L**T, if UPLO = 'L',
* where U is an upper triangular matrix and L is lower triangular.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
* See below for further details.
*
* On exit, if INFO = 0, the triangular factor U or L from the
* Cholesky factorization A = U**T*U or A = L*L**T, in the same
* storage format as A.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the leading minor of order i is not
* positive definite, and the factorization could not be
* completed.
*
* Further Details
* ======= =======
*
* The packed storage scheme is illustrated by the following example
* when N = 4, UPLO = 'U':
*
* Two-dimensional storage of the symmetric matrix A:
*
* a11 a12 a13 a14
* a22 a23 a24
* a33 a34 (aij = aji)
* a44
*
* Packed storage of the upper triangle of A:
*
* AP = [ a11, a12, a22, a13, a23, a33, a14, a24, a34, a44 ]
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param info
*
*/
abstract public void spptrf(java.lang.String uplo, int n, float[] ap, int _ap_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPPTRI computes the inverse of a real symmetric positive definite
* matrix A using the Cholesky factorization A = U**T*U or A = L*L**T
* computed by SPPTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangular factor is stored in AP;
* = 'L': Lower triangular factor is stored in AP.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the triangular factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T, packed columnwise as
* a linear array. The j-th column of U or L is stored in the
* array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = U(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = L(i,j) for j<=i<=n.
*
* On exit, the upper or lower triangle of the (symmetric)
* inverse of A, overwriting the input factor U or L.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the (i,i) element of the factor U or L is
* zero, and the inverse could not be computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param info
*
*/
abstract public void spptri(java.lang.String uplo, int n, float[] ap, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPPTRI computes the inverse of a real symmetric positive definite
* matrix A using the Cholesky factorization A = U**T*U or A = L*L**T
* computed by SPPTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangular factor is stored in AP;
* = 'L': Lower triangular factor is stored in AP.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the triangular factor U or L from the Cholesky
* factorization A = U**T*U or A = L*L**T, packed columnwise as
* a linear array. The j-th column of U or L is stored in the
* array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = U(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = L(i,j) for j<=i<=n.
*
* On exit, the upper or lower triangle of the (symmetric)
* inverse of A, overwriting the input factor U or L.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the (i,i) element of the factor U or L is
* zero, and the inverse could not be computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param info
*
*/
abstract public void spptri(java.lang.String uplo, int n, float[] ap, int _ap_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPPTRS solves a system of linear equations A*X = B with a symmetric
* positive definite matrix A in packed storage using the Cholesky
* factorization A = U**T*U or A = L*L**T computed by SPPTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AP (input) REAL array, dimension (N*(N+1)/2)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T, packed columnwise in a linear
* array. The j-th column of U or L is stored in the array AP
* as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = U(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = L(i,j) for j<=i<=n.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param ap
* @param b
* @param ldb
* @param info
*
*/
abstract public void spptrs(java.lang.String uplo, int n, int nrhs, float[] ap, float[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPPTRS solves a system of linear equations A*X = B with a symmetric
* positive definite matrix A in packed storage using the Cholesky
* factorization A = U**T*U or A = L*L**T computed by SPPTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AP (input) REAL array, dimension (N*(N+1)/2)
* The triangular factor U or L from the Cholesky factorization
* A = U**T*U or A = L*L**T, packed columnwise in a linear
* array. The j-th column of U or L is stored in the array AP
* as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = U(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = L(i,j) for j<=i<=n.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param ap
* @param _ap_offset
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void spptrs(java.lang.String uplo, int n, int nrhs, float[] ap, int _ap_offset, float[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPTCON computes the reciprocal of the condition number (in the
* 1-norm) of a real symmetric positive definite tridiagonal matrix
* using the factorization A = L*D*L**T or A = U**T*D*U computed by
* SPTTRF.
*
* Norm(inv(A)) is computed by a direct method, and the reciprocal of
* the condition number is computed as
* RCOND = 1 / (ANORM * norm(inv(A))).
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* D (input) REAL array, dimension (N)
* The n diagonal elements of the diagonal matrix D from the
* factorization of A, as computed by SPTTRF.
*
* E (input) REAL array, dimension (N-1)
* The (n-1) off-diagonal elements of the unit bidiagonal factor
* U or L from the factorization of A, as computed by SPTTRF.
*
* ANORM (input) REAL
* The 1-norm of the original matrix A.
*
* RCOND (output) REAL
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(ANORM * AINVNM), where AINVNM is the
* 1-norm of inv(A) computed in this routine.
*
* WORK (workspace) REAL array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The method used is described in Nicholas J. Higham, "Efficient
* Algorithms for Computing the Condition Number of a Tridiagonal
* Matrix", SIAM J. Sci. Stat. Comput., Vol. 7, No. 1, January 1986.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param e
* @param anorm
* @param rcond
* @param work
* @param info
*
*/
abstract public void sptcon(int n, float[] d, float[] e, float anorm, org.netlib.util.floatW rcond, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPTCON computes the reciprocal of the condition number (in the
* 1-norm) of a real symmetric positive definite tridiagonal matrix
* using the factorization A = L*D*L**T or A = U**T*D*U computed by
* SPTTRF.
*
* Norm(inv(A)) is computed by a direct method, and the reciprocal of
* the condition number is computed as
* RCOND = 1 / (ANORM * norm(inv(A))).
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* D (input) REAL array, dimension (N)
* The n diagonal elements of the diagonal matrix D from the
* factorization of A, as computed by SPTTRF.
*
* E (input) REAL array, dimension (N-1)
* The (n-1) off-diagonal elements of the unit bidiagonal factor
* U or L from the factorization of A, as computed by SPTTRF.
*
* ANORM (input) REAL
* The 1-norm of the original matrix A.
*
* RCOND (output) REAL
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(ANORM * AINVNM), where AINVNM is the
* 1-norm of inv(A) computed in this routine.
*
* WORK (workspace) REAL array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The method used is described in Nicholas J. Higham, "Efficient
* Algorithms for Computing the Condition Number of a Tridiagonal
* Matrix", SIAM J. Sci. Stat. Comput., Vol. 7, No. 1, January 1986.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param anorm
* @param rcond
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void sptcon(int n, float[] d, int _d_offset, float[] e, int _e_offset, float anorm, org.netlib.util.floatW rcond, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPTEQR computes all eigenvalues and, optionally, eigenvectors of a
* symmetric positive definite tridiagonal matrix by first factoring the
* matrix using SPTTRF, and then calling SBDSQR to compute the singular
* values of the bidiagonal factor.
*
* This routine computes the eigenvalues of the positive definite
* tridiagonal matrix to high relative accuracy. This means that if the
* eigenvalues range over many orders of magnitude in size, then the
* small eigenvalues and corresponding eigenvectors will be computed
* more accurately than, for example, with the standard QR method.
*
* The eigenvectors of a full or band symmetric positive definite matrix
* can also be found if SSYTRD, SSPTRD, or SSBTRD has been used to
* reduce this matrix to tridiagonal form. (The reduction to tridiagonal
* form, however, may preclude the possibility of obtaining high
* relative accuracy in the small eigenvalues of the original matrix, if
* these eigenvalues range over many orders of magnitude.)
*
* Arguments
* =========
*
* COMPZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only.
* = 'V': Compute eigenvectors of original symmetric
* matrix also. Array Z contains the orthogonal
* matrix used to reduce the original matrix to
* tridiagonal form.
* = 'I': Compute eigenvectors of tridiagonal matrix also.
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, the n diagonal elements of the tridiagonal
* matrix.
* On normal exit, D contains the eigenvalues, in descending
* order.
*
* E (input/output) REAL array, dimension (N-1)
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix.
* On exit, E has been destroyed.
*
* Z (input/output) REAL array, dimension (LDZ, N)
* On entry, if COMPZ = 'V', the orthogonal matrix used in the
* reduction to tridiagonal form.
* On exit, if COMPZ = 'V', the orthonormal eigenvectors of the
* original symmetric matrix;
* if COMPZ = 'I', the orthonormal eigenvectors of the
* tridiagonal matrix.
* If INFO > 0 on exit, Z contains the eigenvectors associated
* with only the stored eigenvalues.
* If COMPZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* COMPZ = 'V' or 'I', LDZ >= max(1,N).
*
* WORK (workspace) REAL array, dimension (4*N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, and i is:
* <= N the Cholesky factorization of the matrix could
* not be performed because the i-th principal minor
* was not positive definite.
* > N the SVD algorithm failed to converge;
* if INFO = N+i, i off-diagonal elements of the
* bidiagonal factor did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param compz
* @param n
* @param d
* @param e
* @param z
* @param ldz
* @param work
* @param info
*
*/
abstract public void spteqr(java.lang.String compz, int n, float[] d, float[] e, float[] z, int ldz, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPTEQR computes all eigenvalues and, optionally, eigenvectors of a
* symmetric positive definite tridiagonal matrix by first factoring the
* matrix using SPTTRF, and then calling SBDSQR to compute the singular
* values of the bidiagonal factor.
*
* This routine computes the eigenvalues of the positive definite
* tridiagonal matrix to high relative accuracy. This means that if the
* eigenvalues range over many orders of magnitude in size, then the
* small eigenvalues and corresponding eigenvectors will be computed
* more accurately than, for example, with the standard QR method.
*
* The eigenvectors of a full or band symmetric positive definite matrix
* can also be found if SSYTRD, SSPTRD, or SSBTRD has been used to
* reduce this matrix to tridiagonal form. (The reduction to tridiagonal
* form, however, may preclude the possibility of obtaining high
* relative accuracy in the small eigenvalues of the original matrix, if
* these eigenvalues range over many orders of magnitude.)
*
* Arguments
* =========
*
* COMPZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only.
* = 'V': Compute eigenvectors of original symmetric
* matrix also. Array Z contains the orthogonal
* matrix used to reduce the original matrix to
* tridiagonal form.
* = 'I': Compute eigenvectors of tridiagonal matrix also.
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, the n diagonal elements of the tridiagonal
* matrix.
* On normal exit, D contains the eigenvalues, in descending
* order.
*
* E (input/output) REAL array, dimension (N-1)
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix.
* On exit, E has been destroyed.
*
* Z (input/output) REAL array, dimension (LDZ, N)
* On entry, if COMPZ = 'V', the orthogonal matrix used in the
* reduction to tridiagonal form.
* On exit, if COMPZ = 'V', the orthonormal eigenvectors of the
* original symmetric matrix;
* if COMPZ = 'I', the orthonormal eigenvectors of the
* tridiagonal matrix.
* If INFO > 0 on exit, Z contains the eigenvectors associated
* with only the stored eigenvalues.
* If COMPZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* COMPZ = 'V' or 'I', LDZ >= max(1,N).
*
* WORK (workspace) REAL array, dimension (4*N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, and i is:
* <= N the Cholesky factorization of the matrix could
* not be performed because the i-th principal minor
* was not positive definite.
* > N the SVD algorithm failed to converge;
* if INFO = N+i, i off-diagonal elements of the
* bidiagonal factor did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param compz
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void spteqr(java.lang.String compz, int n, float[] d, int _d_offset, float[] e, int _e_offset, float[] z, int _z_offset, int ldz, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPTRFS improves the computed solution to a system of linear
* equations when the coefficient matrix is symmetric positive definite
* and tridiagonal, and provides error bounds and backward error
* estimates for the solution.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* D (input) REAL array, dimension (N)
* The n diagonal elements of the tridiagonal matrix A.
*
* E (input) REAL array, dimension (N-1)
* The (n-1) subdiagonal elements of the tridiagonal matrix A.
*
* DF (input) REAL array, dimension (N)
* The n diagonal elements of the diagonal matrix D from the
* factorization computed by SPTTRF.
*
* EF (input) REAL array, dimension (N-1)
* The (n-1) subdiagonal elements of the unit bidiagonal factor
* L from the factorization computed by SPTTRF.
*
* B (input) REAL array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) REAL array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by SPTTRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) REAL array, dimension (NRHS)
* The forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j).
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (2*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param nrhs
* @param d
* @param e
* @param df
* @param ef
* @param b
* @param ldb
* @param x
* @param ldx
* @param ferr
* @param berr
* @param work
* @param info
*
*/
abstract public void sptrfs(int n, int nrhs, float[] d, float[] e, float[] df, float[] ef, float[] b, int ldb, float[] x, int ldx, float[] ferr, float[] berr, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPTRFS improves the computed solution to a system of linear
* equations when the coefficient matrix is symmetric positive definite
* and tridiagonal, and provides error bounds and backward error
* estimates for the solution.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* D (input) REAL array, dimension (N)
* The n diagonal elements of the tridiagonal matrix A.
*
* E (input) REAL array, dimension (N-1)
* The (n-1) subdiagonal elements of the tridiagonal matrix A.
*
* DF (input) REAL array, dimension (N)
* The n diagonal elements of the diagonal matrix D from the
* factorization computed by SPTTRF.
*
* EF (input) REAL array, dimension (N-1)
* The (n-1) subdiagonal elements of the unit bidiagonal factor
* L from the factorization computed by SPTTRF.
*
* B (input) REAL array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) REAL array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by SPTTRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) REAL array, dimension (NRHS)
* The forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j).
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (2*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param nrhs
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param df
* @param _df_offset
* @param ef
* @param _ef_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void sptrfs(int n, int nrhs, float[] d, int _d_offset, float[] e, int _e_offset, float[] df, int _df_offset, float[] ef, int _ef_offset, float[] b, int _b_offset, int ldb, float[] x, int _x_offset, int ldx, float[] ferr, int _ferr_offset, float[] berr, int _berr_offset, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPTSV computes the solution to a real system of linear equations
* A*X = B, where A is an N-by-N symmetric positive definite tridiagonal
* matrix, and X and B are N-by-NRHS matrices.
*
* A is factored as A = L*D*L**T, and the factored form of A is then
* used to solve the system of equations.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, the n diagonal elements of the tridiagonal matrix
* A. On exit, the n diagonal elements of the diagonal matrix
* D from the factorization A = L*D*L**T.
*
* E (input/output) REAL array, dimension (N-1)
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix A. On exit, the (n-1) subdiagonal elements of the
* unit bidiagonal factor L from the L*D*L**T factorization of
* A. (E can also be regarded as the superdiagonal of the unit
* bidiagonal factor U from the U**T*D*U factorization of A.)
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if INFO = 0, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the leading minor of order i is not
* positive definite, and the solution has not been
* computed. The factorization has not been completed
* unless i = N.
*
* =====================================================================
*
* .. External Subroutines ..
*
*
* @param n
* @param nrhs
* @param d
* @param e
* @param b
* @param ldb
* @param info
*
*/
abstract public void sptsv(int n, int nrhs, float[] d, float[] e, float[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPTSV computes the solution to a real system of linear equations
* A*X = B, where A is an N-by-N symmetric positive definite tridiagonal
* matrix, and X and B are N-by-NRHS matrices.
*
* A is factored as A = L*D*L**T, and the factored form of A is then
* used to solve the system of equations.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, the n diagonal elements of the tridiagonal matrix
* A. On exit, the n diagonal elements of the diagonal matrix
* D from the factorization A = L*D*L**T.
*
* E (input/output) REAL array, dimension (N-1)
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix A. On exit, the (n-1) subdiagonal elements of the
* unit bidiagonal factor L from the L*D*L**T factorization of
* A. (E can also be regarded as the superdiagonal of the unit
* bidiagonal factor U from the U**T*D*U factorization of A.)
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if INFO = 0, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the leading minor of order i is not
* positive definite, and the solution has not been
* computed. The factorization has not been completed
* unless i = N.
*
* =====================================================================
*
* .. External Subroutines ..
*
*
* @param n
* @param nrhs
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void sptsv(int n, int nrhs, float[] d, int _d_offset, float[] e, int _e_offset, float[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPTSVX uses the factorization A = L*D*L**T to compute the solution
* to a real system of linear equations A*X = B, where A is an N-by-N
* symmetric positive definite tridiagonal matrix and X and B are
* N-by-NRHS matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed:
*
* 1. If FACT = 'N', the matrix A is factored as A = L*D*L**T, where L
* is a unit lower bidiagonal matrix and D is diagonal. The
* factorization can also be regarded as having the form
* A = U**T*D*U.
*
* 2. If the leading i-by-i principal minor is not positive definite,
* then the routine returns with INFO = i. Otherwise, the factored
* form of A is used to estimate the condition number of the matrix
* A. If the reciprocal of the condition number is less than machine
* precision, INFO = N+1 is returned as a warning, but the routine
* still goes on to solve for X and compute error bounds as
* described below.
*
* 3. The system of equations is solved for X using the factored form
* of A.
*
* 4. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of A has been
* supplied on entry.
* = 'F': On entry, DF and EF contain the factored form of A.
* D, E, DF, and EF will not be modified.
* = 'N': The matrix A will be copied to DF and EF and
* factored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* D (input) REAL array, dimension (N)
* The n diagonal elements of the tridiagonal matrix A.
*
* E (input) REAL array, dimension (N-1)
* The (n-1) subdiagonal elements of the tridiagonal matrix A.
*
* DF (input or output) REAL array, dimension (N)
* If FACT = 'F', then DF is an input argument and on entry
* contains the n diagonal elements of the diagonal matrix D
* from the L*D*L**T factorization of A.
* If FACT = 'N', then DF is an output argument and on exit
* contains the n diagonal elements of the diagonal matrix D
* from the L*D*L**T factorization of A.
*
* EF (input or output) REAL array, dimension (N-1)
* If FACT = 'F', then EF is an input argument and on entry
* contains the (n-1) subdiagonal elements of the unit
* bidiagonal factor L from the L*D*L**T factorization of A.
* If FACT = 'N', then EF is an output argument and on exit
* contains the (n-1) subdiagonal elements of the unit
* bidiagonal factor L from the L*D*L**T factorization of A.
*
* B (input) REAL array, dimension (LDB,NRHS)
* The N-by-NRHS right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) REAL array, dimension (LDX,NRHS)
* If INFO = 0 of INFO = N+1, the N-by-NRHS solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) REAL
* The reciprocal condition number of the matrix A. If RCOND
* is less than the machine precision (in particular, if
* RCOND = 0), the matrix is singular to working precision.
* This condition is indicated by a return code of INFO > 0.
*
* FERR (output) REAL array, dimension (NRHS)
* The forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j).
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in any
* element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (2*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= N: the leading minor of order i of A is
* not positive definite, so the factorization
* could not be completed, and the solution has not
* been computed. RCOND = 0 is returned.
* = N+1: U is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param n
* @param nrhs
* @param d
* @param e
* @param df
* @param ef
* @param b
* @param ldb
* @param x
* @param ldx
* @param rcond
* @param ferr
* @param berr
* @param work
* @param info
*
*/
abstract public void sptsvx(java.lang.String fact, int n, int nrhs, float[] d, float[] e, float[] df, float[] ef, float[] b, int ldb, float[] x, int ldx, org.netlib.util.floatW rcond, float[] ferr, float[] berr, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPTSVX uses the factorization A = L*D*L**T to compute the solution
* to a real system of linear equations A*X = B, where A is an N-by-N
* symmetric positive definite tridiagonal matrix and X and B are
* N-by-NRHS matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed:
*
* 1. If FACT = 'N', the matrix A is factored as A = L*D*L**T, where L
* is a unit lower bidiagonal matrix and D is diagonal. The
* factorization can also be regarded as having the form
* A = U**T*D*U.
*
* 2. If the leading i-by-i principal minor is not positive definite,
* then the routine returns with INFO = i. Otherwise, the factored
* form of A is used to estimate the condition number of the matrix
* A. If the reciprocal of the condition number is less than machine
* precision, INFO = N+1 is returned as a warning, but the routine
* still goes on to solve for X and compute error bounds as
* described below.
*
* 3. The system of equations is solved for X using the factored form
* of A.
*
* 4. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of A has been
* supplied on entry.
* = 'F': On entry, DF and EF contain the factored form of A.
* D, E, DF, and EF will not be modified.
* = 'N': The matrix A will be copied to DF and EF and
* factored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* D (input) REAL array, dimension (N)
* The n diagonal elements of the tridiagonal matrix A.
*
* E (input) REAL array, dimension (N-1)
* The (n-1) subdiagonal elements of the tridiagonal matrix A.
*
* DF (input or output) REAL array, dimension (N)
* If FACT = 'F', then DF is an input argument and on entry
* contains the n diagonal elements of the diagonal matrix D
* from the L*D*L**T factorization of A.
* If FACT = 'N', then DF is an output argument and on exit
* contains the n diagonal elements of the diagonal matrix D
* from the L*D*L**T factorization of A.
*
* EF (input or output) REAL array, dimension (N-1)
* If FACT = 'F', then EF is an input argument and on entry
* contains the (n-1) subdiagonal elements of the unit
* bidiagonal factor L from the L*D*L**T factorization of A.
* If FACT = 'N', then EF is an output argument and on exit
* contains the (n-1) subdiagonal elements of the unit
* bidiagonal factor L from the L*D*L**T factorization of A.
*
* B (input) REAL array, dimension (LDB,NRHS)
* The N-by-NRHS right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) REAL array, dimension (LDX,NRHS)
* If INFO = 0 of INFO = N+1, the N-by-NRHS solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) REAL
* The reciprocal condition number of the matrix A. If RCOND
* is less than the machine precision (in particular, if
* RCOND = 0), the matrix is singular to working precision.
* This condition is indicated by a return code of INFO > 0.
*
* FERR (output) REAL array, dimension (NRHS)
* The forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j).
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in any
* element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (2*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= N: the leading minor of order i of A is
* not positive definite, so the factorization
* could not be completed, and the solution has not
* been computed. RCOND = 0 is returned.
* = N+1: U is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param n
* @param nrhs
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param df
* @param _df_offset
* @param ef
* @param _ef_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param rcond
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void sptsvx(java.lang.String fact, int n, int nrhs, float[] d, int _d_offset, float[] e, int _e_offset, float[] df, int _df_offset, float[] ef, int _ef_offset, float[] b, int _b_offset, int ldb, float[] x, int _x_offset, int ldx, org.netlib.util.floatW rcond, float[] ferr, int _ferr_offset, float[] berr, int _berr_offset, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPTTRF computes the L*D*L' factorization of a real symmetric
* positive definite tridiagonal matrix A. The factorization may also
* be regarded as having the form A = U'*D*U.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, the n diagonal elements of the tridiagonal matrix
* A. On exit, the n diagonal elements of the diagonal matrix
* D from the L*D*L' factorization of A.
*
* E (input/output) REAL array, dimension (N-1)
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix A. On exit, the (n-1) subdiagonal elements of the
* unit bidiagonal factor L from the L*D*L' factorization of A.
* E can also be regarded as the superdiagonal of the unit
* bidiagonal factor U from the U'*D*U factorization of A.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
* > 0: if INFO = k, the leading minor of order k is not
* positive definite; if k < N, the factorization could not
* be completed, while if k = N, the factorization was
* completed, but D(N) <= 0.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param e
* @param info
*
*/
abstract public void spttrf(int n, float[] d, float[] e, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPTTRF computes the L*D*L' factorization of a real symmetric
* positive definite tridiagonal matrix A. The factorization may also
* be regarded as having the form A = U'*D*U.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, the n diagonal elements of the tridiagonal matrix
* A. On exit, the n diagonal elements of the diagonal matrix
* D from the L*D*L' factorization of A.
*
* E (input/output) REAL array, dimension (N-1)
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix A. On exit, the (n-1) subdiagonal elements of the
* unit bidiagonal factor L from the L*D*L' factorization of A.
* E can also be regarded as the superdiagonal of the unit
* bidiagonal factor U from the U'*D*U factorization of A.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
* > 0: if INFO = k, the leading minor of order k is not
* positive definite; if k < N, the factorization could not
* be completed, while if k = N, the factorization was
* completed, but D(N) <= 0.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param info
*
*/
abstract public void spttrf(int n, float[] d, int _d_offset, float[] e, int _e_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPTTRS solves a tridiagonal system of the form
* A * X = B
* using the L*D*L' factorization of A computed by SPTTRF. D is a
* diagonal matrix specified in the vector D, L is a unit bidiagonal
* matrix whose subdiagonal is specified in the vector E, and X and B
* are N by NRHS matrices.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the tridiagonal matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* D (input) REAL array, dimension (N)
* The n diagonal elements of the diagonal matrix D from the
* L*D*L' factorization of A.
*
* E (input) REAL array, dimension (N-1)
* The (n-1) subdiagonal elements of the unit bidiagonal factor
* L from the L*D*L' factorization of A. E can also be regarded
* as the superdiagonal of the unit bidiagonal factor U from the
* factorization A = U'*D*U.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the right hand side vectors B for the system of
* linear equations.
* On exit, the solution vectors, X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n
* @param nrhs
* @param d
* @param e
* @param b
* @param ldb
* @param info
*
*/
abstract public void spttrs(int n, int nrhs, float[] d, float[] e, float[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPTTRS solves a tridiagonal system of the form
* A * X = B
* using the L*D*L' factorization of A computed by SPTTRF. D is a
* diagonal matrix specified in the vector D, L is a unit bidiagonal
* matrix whose subdiagonal is specified in the vector E, and X and B
* are N by NRHS matrices.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the tridiagonal matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* D (input) REAL array, dimension (N)
* The n diagonal elements of the diagonal matrix D from the
* L*D*L' factorization of A.
*
* E (input) REAL array, dimension (N-1)
* The (n-1) subdiagonal elements of the unit bidiagonal factor
* L from the L*D*L' factorization of A. E can also be regarded
* as the superdiagonal of the unit bidiagonal factor U from the
* factorization A = U'*D*U.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the right hand side vectors B for the system of
* linear equations.
* On exit, the solution vectors, X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n
* @param nrhs
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void spttrs(int n, int nrhs, float[] d, int _d_offset, float[] e, int _e_offset, float[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SPTTS2 solves a tridiagonal system of the form
* A * X = B
* using the L*D*L' factorization of A computed by SPTTRF. D is a
* diagonal matrix specified in the vector D, L is a unit bidiagonal
* matrix whose subdiagonal is specified in the vector E, and X and B
* are N by NRHS matrices.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the tridiagonal matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* D (input) REAL array, dimension (N)
* The n diagonal elements of the diagonal matrix D from the
* L*D*L' factorization of A.
*
* E (input) REAL array, dimension (N-1)
* The (n-1) subdiagonal elements of the unit bidiagonal factor
* L from the L*D*L' factorization of A. E can also be regarded
* as the superdiagonal of the unit bidiagonal factor U from the
* factorization A = U'*D*U.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the right hand side vectors B for the system of
* linear equations.
* On exit, the solution vectors, X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n
* @param nrhs
* @param d
* @param e
* @param b
* @param ldb
*
*/
abstract public void sptts2(int n, int nrhs, float[] d, float[] e, float[] b, int ldb);
/**
*
* ..
*
* Purpose
* =======
*
* SPTTS2 solves a tridiagonal system of the form
* A * X = B
* using the L*D*L' factorization of A computed by SPTTRF. D is a
* diagonal matrix specified in the vector D, L is a unit bidiagonal
* matrix whose subdiagonal is specified in the vector E, and X and B
* are N by NRHS matrices.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the tridiagonal matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* D (input) REAL array, dimension (N)
* The n diagonal elements of the diagonal matrix D from the
* L*D*L' factorization of A.
*
* E (input) REAL array, dimension (N-1)
* The (n-1) subdiagonal elements of the unit bidiagonal factor
* L from the L*D*L' factorization of A. E can also be regarded
* as the superdiagonal of the unit bidiagonal factor U from the
* factorization A = U'*D*U.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the right hand side vectors B for the system of
* linear equations.
* On exit, the solution vectors, X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param n
* @param nrhs
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param b
* @param _b_offset
* @param ldb
*
*/
abstract public void sptts2(int n, int nrhs, float[] d, int _d_offset, float[] e, int _e_offset, float[] b, int _b_offset, int ldb);
/**
*
* ..
*
* Purpose
* =======
*
* SRSCL multiplies an n-element real vector x by the real scalar 1/a.
* This is done without overflow or underflow as long as
* the final result x/a does not overflow or underflow.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of components of the vector x.
*
* SA (input) REAL
* The scalar a which is used to divide each component of x.
* SA must be >= 0, or the subroutine will divide by zero.
*
* SX (input/output) REAL array, dimension
* (1+(N-1)*abs(INCX))
* The n-element vector x.
*
* INCX (input) INTEGER
* The increment between successive values of the vector SX.
* > 0: SX(1) = X(1) and SX(1+(i-1)*INCX) = x(i), 1< i<= n
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param sa
* @param sx
* @param incx
*
*/
abstract public void srscl(int n, float sa, float[] sx, int incx);
/**
*
* ..
*
* Purpose
* =======
*
* SRSCL multiplies an n-element real vector x by the real scalar 1/a.
* This is done without overflow or underflow as long as
* the final result x/a does not overflow or underflow.
*
* Arguments
* =========
*
* N (input) INTEGER
* The number of components of the vector x.
*
* SA (input) REAL
* The scalar a which is used to divide each component of x.
* SA must be >= 0, or the subroutine will divide by zero.
*
* SX (input/output) REAL array, dimension
* (1+(N-1)*abs(INCX))
* The n-element vector x.
*
* INCX (input) INTEGER
* The increment between successive values of the vector SX.
* > 0: SX(1) = X(1) and SX(1+(i-1)*INCX) = x(i), 1< i<= n
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param sa
* @param sx
* @param _sx_offset
* @param incx
*
*/
abstract public void srscl(int n, float sa, float[] sx, int _sx_offset, int incx);
/**
*
* ..
*
* Purpose
* =======
*
* SSBEV computes all the eigenvalues and, optionally, eigenvectors of
* a real symmetric band matrix A.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* AB (input/output) REAL array, dimension (LDAB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* On exit, AB is overwritten by values generated during the
* reduction to tridiagonal form. If UPLO = 'U', the first
* superdiagonal and the diagonal of the tridiagonal matrix T
* are returned in rows KD and KD+1 of AB, and if UPLO = 'L',
* the diagonal and first subdiagonal of T are returned in the
* first two rows of AB.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD + 1.
*
* W (output) REAL array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) REAL array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the orthonormal
* eigenvectors of the matrix A, with the i-th column of Z
* holding the eigenvector associated with W(i).
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace) REAL array, dimension (max(1,3*N-2))
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the algorithm failed to converge; i
* off-diagonal elements of an intermediate tridiagonal
* form did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param uplo
* @param n
* @param kd
* @param ab
* @param ldab
* @param w
* @param z
* @param ldz
* @param work
* @param info
*
*/
abstract public void ssbev(java.lang.String jobz, java.lang.String uplo, int n, int kd, float[] ab, int ldab, float[] w, float[] z, int ldz, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSBEV computes all the eigenvalues and, optionally, eigenvectors of
* a real symmetric band matrix A.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* AB (input/output) REAL array, dimension (LDAB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* On exit, AB is overwritten by values generated during the
* reduction to tridiagonal form. If UPLO = 'U', the first
* superdiagonal and the diagonal of the tridiagonal matrix T
* are returned in rows KD and KD+1 of AB, and if UPLO = 'L',
* the diagonal and first subdiagonal of T are returned in the
* first two rows of AB.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD + 1.
*
* W (output) REAL array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) REAL array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the orthonormal
* eigenvectors of the matrix A, with the i-th column of Z
* holding the eigenvector associated with W(i).
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace) REAL array, dimension (max(1,3*N-2))
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the algorithm failed to converge; i
* off-diagonal elements of an intermediate tridiagonal
* form did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param uplo
* @param n
* @param kd
* @param ab
* @param _ab_offset
* @param ldab
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void ssbev(java.lang.String jobz, java.lang.String uplo, int n, int kd, float[] ab, int _ab_offset, int ldab, float[] w, int _w_offset, float[] z, int _z_offset, int ldz, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSBEVD computes all the eigenvalues and, optionally, eigenvectors of
* a real symmetric band matrix A. If eigenvectors are desired, it uses
* a divide and conquer algorithm.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* AB (input/output) REAL array, dimension (LDAB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* On exit, AB is overwritten by values generated during the
* reduction to tridiagonal form. If UPLO = 'U', the first
* superdiagonal and the diagonal of the tridiagonal matrix T
* are returned in rows KD and KD+1 of AB, and if UPLO = 'L',
* the diagonal and first subdiagonal of T are returned in the
* first two rows of AB.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD + 1.
*
* W (output) REAL array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) REAL array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the orthonormal
* eigenvectors of the matrix A, with the i-th column of Z
* holding the eigenvector associated with W(i).
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace/output) REAL array,
* dimension (LWORK)
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* IF N <= 1, LWORK must be at least 1.
* If JOBZ = 'N' and N > 2, LWORK must be at least 2*N.
* If JOBZ = 'V' and N > 2, LWORK must be at least
* ( 1 + 5*N + 2*N**2 ).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array LIWORK.
* If JOBZ = 'N' or N <= 1, LIWORK must be at least 1.
* If JOBZ = 'V' and N > 2, LIWORK must be at least 3 + 5*N.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the algorithm failed to converge; i
* off-diagonal elements of an intermediate tridiagonal
* form did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param uplo
* @param n
* @param kd
* @param ab
* @param ldab
* @param w
* @param z
* @param ldz
* @param work
* @param lwork
* @param iwork
* @param liwork
* @param info
*
*/
abstract public void ssbevd(java.lang.String jobz, java.lang.String uplo, int n, int kd, float[] ab, int ldab, float[] w, float[] z, int ldz, float[] work, int lwork, int[] iwork, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSBEVD computes all the eigenvalues and, optionally, eigenvectors of
* a real symmetric band matrix A. If eigenvectors are desired, it uses
* a divide and conquer algorithm.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* AB (input/output) REAL array, dimension (LDAB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* On exit, AB is overwritten by values generated during the
* reduction to tridiagonal form. If UPLO = 'U', the first
* superdiagonal and the diagonal of the tridiagonal matrix T
* are returned in rows KD and KD+1 of AB, and if UPLO = 'L',
* the diagonal and first subdiagonal of T are returned in the
* first two rows of AB.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD + 1.
*
* W (output) REAL array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) REAL array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the orthonormal
* eigenvectors of the matrix A, with the i-th column of Z
* holding the eigenvector associated with W(i).
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace/output) REAL array,
* dimension (LWORK)
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* IF N <= 1, LWORK must be at least 1.
* If JOBZ = 'N' and N > 2, LWORK must be at least 2*N.
* If JOBZ = 'V' and N > 2, LWORK must be at least
* ( 1 + 5*N + 2*N**2 ).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array LIWORK.
* If JOBZ = 'N' or N <= 1, LIWORK must be at least 1.
* If JOBZ = 'V' and N > 2, LIWORK must be at least 3 + 5*N.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the algorithm failed to converge; i
* off-diagonal elements of an intermediate tridiagonal
* form did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param uplo
* @param n
* @param kd
* @param ab
* @param _ab_offset
* @param ldab
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param liwork
* @param info
*
*/
abstract public void ssbevd(java.lang.String jobz, java.lang.String uplo, int n, int kd, float[] ab, int _ab_offset, int ldab, float[] w, int _w_offset, float[] z, int _z_offset, int ldz, float[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSBEVX computes selected eigenvalues and, optionally, eigenvectors
* of a real symmetric band matrix A. Eigenvalues and eigenvectors can
* be selected by specifying either a range of values or a range of
* indices for the desired eigenvalues.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found;
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found;
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* AB (input/output) REAL array, dimension (LDAB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* On exit, AB is overwritten by values generated during the
* reduction to tridiagonal form. If UPLO = 'U', the first
* superdiagonal and the diagonal of the tridiagonal matrix T
* are returned in rows KD and KD+1 of AB, and if UPLO = 'L',
* the diagonal and first subdiagonal of T are returned in the
* first two rows of AB.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD + 1.
*
* Q (output) REAL array, dimension (LDQ, N)
* If JOBZ = 'V', the N-by-N orthogonal matrix used in the
* reduction to tridiagonal form.
* If JOBZ = 'N', the array Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. If JOBZ = 'V', then
* LDQ >= max(1,N).
*
* VL (input) REAL
* VU (input) REAL
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) REAL
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less than
* or equal to zero, then EPS*|T| will be used in its place,
* where |T| is the 1-norm of the tridiagonal matrix obtained
* by reducing AB to tridiagonal form.
*
* Eigenvalues will be computed most accurately when ABSTOL is
* set to twice the underflow threshold 2*SLAMCH('S'), not zero.
* If this routine returns with INFO>0, indicating that some
* eigenvectors did not converge, try setting ABSTOL to
* 2*SLAMCH('S').
*
* See "Computing Small Singular Values of Bidiagonal Matrices
* with Guaranteed High Relative Accuracy," by Demmel and
* Kahan, LAPACK Working Note #3.
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) REAL array, dimension (N)
* The first M elements contain the selected eigenvalues in
* ascending order.
*
* Z (output) REAL array, dimension (LDZ, max(1,M))
* If JOBZ = 'V', then if INFO = 0, the first M columns of Z
* contain the orthonormal eigenvectors of the matrix A
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* If an eigenvector fails to converge, then that column of Z
* contains the latest approximation to the eigenvector, and the
* index of the eigenvector is returned in IFAIL.
* If JOBZ = 'N', then Z is not referenced.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace) REAL array, dimension (7*N)
*
* IWORK (workspace) INTEGER array, dimension (5*N)
*
* IFAIL (output) INTEGER array, dimension (N)
* If JOBZ = 'V', then if INFO = 0, the first M elements of
* IFAIL are zero. If INFO > 0, then IFAIL contains the
* indices of the eigenvectors that failed to converge.
* If JOBZ = 'N', then IFAIL is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, then i eigenvectors failed to converge.
* Their indices are stored in array IFAIL.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param range
* @param uplo
* @param n
* @param kd
* @param ab
* @param ldab
* @param q
* @param ldq
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param z
* @param ldz
* @param work
* @param iwork
* @param ifail
* @param info
*
*/
abstract public void ssbevx(java.lang.String jobz, java.lang.String range, java.lang.String uplo, int n, int kd, float[] ab, int ldab, float[] q, int ldq, float vl, float vu, int il, int iu, float abstol, org.netlib.util.intW m, float[] w, float[] z, int ldz, float[] work, int[] iwork, int[] ifail, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSBEVX computes selected eigenvalues and, optionally, eigenvectors
* of a real symmetric band matrix A. Eigenvalues and eigenvectors can
* be selected by specifying either a range of values or a range of
* indices for the desired eigenvalues.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found;
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found;
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* AB (input/output) REAL array, dimension (LDAB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
*
* On exit, AB is overwritten by values generated during the
* reduction to tridiagonal form. If UPLO = 'U', the first
* superdiagonal and the diagonal of the tridiagonal matrix T
* are returned in rows KD and KD+1 of AB, and if UPLO = 'L',
* the diagonal and first subdiagonal of T are returned in the
* first two rows of AB.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD + 1.
*
* Q (output) REAL array, dimension (LDQ, N)
* If JOBZ = 'V', the N-by-N orthogonal matrix used in the
* reduction to tridiagonal form.
* If JOBZ = 'N', the array Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. If JOBZ = 'V', then
* LDQ >= max(1,N).
*
* VL (input) REAL
* VU (input) REAL
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) REAL
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less than
* or equal to zero, then EPS*|T| will be used in its place,
* where |T| is the 1-norm of the tridiagonal matrix obtained
* by reducing AB to tridiagonal form.
*
* Eigenvalues will be computed most accurately when ABSTOL is
* set to twice the underflow threshold 2*SLAMCH('S'), not zero.
* If this routine returns with INFO>0, indicating that some
* eigenvectors did not converge, try setting ABSTOL to
* 2*SLAMCH('S').
*
* See "Computing Small Singular Values of Bidiagonal Matrices
* with Guaranteed High Relative Accuracy," by Demmel and
* Kahan, LAPACK Working Note #3.
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) REAL array, dimension (N)
* The first M elements contain the selected eigenvalues in
* ascending order.
*
* Z (output) REAL array, dimension (LDZ, max(1,M))
* If JOBZ = 'V', then if INFO = 0, the first M columns of Z
* contain the orthonormal eigenvectors of the matrix A
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* If an eigenvector fails to converge, then that column of Z
* contains the latest approximation to the eigenvector, and the
* index of the eigenvector is returned in IFAIL.
* If JOBZ = 'N', then Z is not referenced.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace) REAL array, dimension (7*N)
*
* IWORK (workspace) INTEGER array, dimension (5*N)
*
* IFAIL (output) INTEGER array, dimension (N)
* If JOBZ = 'V', then if INFO = 0, the first M elements of
* IFAIL are zero. If INFO > 0, then IFAIL contains the
* indices of the eigenvectors that failed to converge.
* If JOBZ = 'N', then IFAIL is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, then i eigenvectors failed to converge.
* Their indices are stored in array IFAIL.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param range
* @param uplo
* @param n
* @param kd
* @param ab
* @param _ab_offset
* @param ldab
* @param q
* @param _q_offset
* @param ldq
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param ifail
* @param _ifail_offset
* @param info
*
*/
abstract public void ssbevx(java.lang.String jobz, java.lang.String range, java.lang.String uplo, int n, int kd, float[] ab, int _ab_offset, int ldab, float[] q, int _q_offset, int ldq, float vl, float vu, int il, int iu, float abstol, org.netlib.util.intW m, float[] w, int _w_offset, float[] z, int _z_offset, int ldz, float[] work, int _work_offset, int[] iwork, int _iwork_offset, int[] ifail, int _ifail_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSBGST reduces a real symmetric-definite banded generalized
* eigenproblem A*x = lambda*B*x to standard form C*y = lambda*y,
* such that C has the same bandwidth as A.
*
* B must have been previously factorized as S**T*S by SPBSTF, using a
* split Cholesky factorization. A is overwritten by C = X**T*A*X, where
* X = S**(-1)*Q and Q is an orthogonal matrix chosen to preserve the
* bandwidth of A.
*
* Arguments
* =========
*
* VECT (input) CHARACTER*1
* = 'N': do not form the transformation matrix X;
* = 'V': form X.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* KA (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KA >= 0.
*
* KB (input) INTEGER
* The number of superdiagonals of the matrix B if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KA >= KB >= 0.
*
* AB (input/output) REAL array, dimension (LDAB,N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first ka+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(ka+1+i-j,j) = A(i,j) for max(1,j-ka)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+ka).
*
* On exit, the transformed matrix X**T*A*X, stored in the same
* format as A.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KA+1.
*
* BB (input) REAL array, dimension (LDBB,N)
* The banded factor S from the split Cholesky factorization of
* B, as returned by SPBSTF, stored in the first KB+1 rows of
* the array.
*
* LDBB (input) INTEGER
* The leading dimension of the array BB. LDBB >= KB+1.
*
* X (output) REAL array, dimension (LDX,N)
* If VECT = 'V', the n-by-n matrix X.
* If VECT = 'N', the array X is not referenced.
*
* LDX (input) INTEGER
* The leading dimension of the array X.
* LDX >= max(1,N) if VECT = 'V'; LDX >= 1 otherwise.
*
* WORK (workspace) REAL array, dimension (2*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param vect
* @param uplo
* @param n
* @param ka
* @param kb
* @param ab
* @param ldab
* @param bb
* @param ldbb
* @param x
* @param ldx
* @param work
* @param info
*
*/
abstract public void ssbgst(java.lang.String vect, java.lang.String uplo, int n, int ka, int kb, float[] ab, int ldab, float[] bb, int ldbb, float[] x, int ldx, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSBGST reduces a real symmetric-definite banded generalized
* eigenproblem A*x = lambda*B*x to standard form C*y = lambda*y,
* such that C has the same bandwidth as A.
*
* B must have been previously factorized as S**T*S by SPBSTF, using a
* split Cholesky factorization. A is overwritten by C = X**T*A*X, where
* X = S**(-1)*Q and Q is an orthogonal matrix chosen to preserve the
* bandwidth of A.
*
* Arguments
* =========
*
* VECT (input) CHARACTER*1
* = 'N': do not form the transformation matrix X;
* = 'V': form X.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* KA (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KA >= 0.
*
* KB (input) INTEGER
* The number of superdiagonals of the matrix B if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KA >= KB >= 0.
*
* AB (input/output) REAL array, dimension (LDAB,N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first ka+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(ka+1+i-j,j) = A(i,j) for max(1,j-ka)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+ka).
*
* On exit, the transformed matrix X**T*A*X, stored in the same
* format as A.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KA+1.
*
* BB (input) REAL array, dimension (LDBB,N)
* The banded factor S from the split Cholesky factorization of
* B, as returned by SPBSTF, stored in the first KB+1 rows of
* the array.
*
* LDBB (input) INTEGER
* The leading dimension of the array BB. LDBB >= KB+1.
*
* X (output) REAL array, dimension (LDX,N)
* If VECT = 'V', the n-by-n matrix X.
* If VECT = 'N', the array X is not referenced.
*
* LDX (input) INTEGER
* The leading dimension of the array X.
* LDX >= max(1,N) if VECT = 'V'; LDX >= 1 otherwise.
*
* WORK (workspace) REAL array, dimension (2*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param vect
* @param uplo
* @param n
* @param ka
* @param kb
* @param ab
* @param _ab_offset
* @param ldab
* @param bb
* @param _bb_offset
* @param ldbb
* @param x
* @param _x_offset
* @param ldx
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void ssbgst(java.lang.String vect, java.lang.String uplo, int n, int ka, int kb, float[] ab, int _ab_offset, int ldab, float[] bb, int _bb_offset, int ldbb, float[] x, int _x_offset, int ldx, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSBGV computes all the eigenvalues, and optionally, the eigenvectors
* of a real generalized symmetric-definite banded eigenproblem, of
* the form A*x=(lambda)*B*x. Here A and B are assumed to be symmetric
* and banded, and B is also positive definite.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangles of A and B are stored;
* = 'L': Lower triangles of A and B are stored.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* KA (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KA >= 0.
*
* KB (input) INTEGER
* The number of superdiagonals of the matrix B if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KB >= 0.
*
* AB (input/output) REAL array, dimension (LDAB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first ka+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(ka+1+i-j,j) = A(i,j) for max(1,j-ka)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+ka).
*
* On exit, the contents of AB are destroyed.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KA+1.
*
* BB (input/output) REAL array, dimension (LDBB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix B, stored in the first kb+1 rows of the array. The
* j-th column of B is stored in the j-th column of the array BB
* as follows:
* if UPLO = 'U', BB(kb+1+i-j,j) = B(i,j) for max(1,j-kb)<=i<=j;
* if UPLO = 'L', BB(1+i-j,j) = B(i,j) for j<=i<=min(n,j+kb).
*
* On exit, the factor S from the split Cholesky factorization
* B = S**T*S, as returned by SPBSTF.
*
* LDBB (input) INTEGER
* The leading dimension of the array BB. LDBB >= KB+1.
*
* W (output) REAL array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) REAL array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the matrix Z of
* eigenvectors, with the i-th column of Z holding the
* eigenvector associated with W(i). The eigenvectors are
* normalized so that Z**T*B*Z = I.
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= N.
*
* WORK (workspace) REAL array, dimension (3*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is:
* <= N: the algorithm failed to converge:
* i off-diagonal elements of an intermediate
* tridiagonal form did not converge to zero;
* > N: if INFO = N + i, for 1 <= i <= N, then SPBSTF
* returned INFO = i: B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param jobz
* @param uplo
* @param n
* @param ka
* @param kb
* @param ab
* @param ldab
* @param bb
* @param ldbb
* @param w
* @param z
* @param ldz
* @param work
* @param info
*
*/
abstract public void ssbgv(java.lang.String jobz, java.lang.String uplo, int n, int ka, int kb, float[] ab, int ldab, float[] bb, int ldbb, float[] w, float[] z, int ldz, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSBGV computes all the eigenvalues, and optionally, the eigenvectors
* of a real generalized symmetric-definite banded eigenproblem, of
* the form A*x=(lambda)*B*x. Here A and B are assumed to be symmetric
* and banded, and B is also positive definite.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangles of A and B are stored;
* = 'L': Lower triangles of A and B are stored.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* KA (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KA >= 0.
*
* KB (input) INTEGER
* The number of superdiagonals of the matrix B if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KB >= 0.
*
* AB (input/output) REAL array, dimension (LDAB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first ka+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(ka+1+i-j,j) = A(i,j) for max(1,j-ka)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+ka).
*
* On exit, the contents of AB are destroyed.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KA+1.
*
* BB (input/output) REAL array, dimension (LDBB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix B, stored in the first kb+1 rows of the array. The
* j-th column of B is stored in the j-th column of the array BB
* as follows:
* if UPLO = 'U', BB(kb+1+i-j,j) = B(i,j) for max(1,j-kb)<=i<=j;
* if UPLO = 'L', BB(1+i-j,j) = B(i,j) for j<=i<=min(n,j+kb).
*
* On exit, the factor S from the split Cholesky factorization
* B = S**T*S, as returned by SPBSTF.
*
* LDBB (input) INTEGER
* The leading dimension of the array BB. LDBB >= KB+1.
*
* W (output) REAL array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) REAL array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the matrix Z of
* eigenvectors, with the i-th column of Z holding the
* eigenvector associated with W(i). The eigenvectors are
* normalized so that Z**T*B*Z = I.
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= N.
*
* WORK (workspace) REAL array, dimension (3*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is:
* <= N: the algorithm failed to converge:
* i off-diagonal elements of an intermediate
* tridiagonal form did not converge to zero;
* > N: if INFO = N + i, for 1 <= i <= N, then SPBSTF
* returned INFO = i: B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param jobz
* @param uplo
* @param n
* @param ka
* @param kb
* @param ab
* @param _ab_offset
* @param ldab
* @param bb
* @param _bb_offset
* @param ldbb
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void ssbgv(java.lang.String jobz, java.lang.String uplo, int n, int ka, int kb, float[] ab, int _ab_offset, int ldab, float[] bb, int _bb_offset, int ldbb, float[] w, int _w_offset, float[] z, int _z_offset, int ldz, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSBGVD computes all the eigenvalues, and optionally, the eigenvectors
* of a real generalized symmetric-definite banded eigenproblem, of the
* form A*x=(lambda)*B*x. Here A and B are assumed to be symmetric and
* banded, and B is also positive definite. If eigenvectors are
* desired, it uses a divide and conquer algorithm.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangles of A and B are stored;
* = 'L': Lower triangles of A and B are stored.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* KA (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KA >= 0.
*
* KB (input) INTEGER
* The number of superdiagonals of the matrix B if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KB >= 0.
*
* AB (input/output) REAL array, dimension (LDAB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first ka+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(ka+1+i-j,j) = A(i,j) for max(1,j-ka)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+ka).
*
* On exit, the contents of AB are destroyed.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KA+1.
*
* BB (input/output) REAL array, dimension (LDBB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix B, stored in the first kb+1 rows of the array. The
* j-th column of B is stored in the j-th column of the array BB
* as follows:
* if UPLO = 'U', BB(ka+1+i-j,j) = B(i,j) for max(1,j-kb)<=i<=j;
* if UPLO = 'L', BB(1+i-j,j) = B(i,j) for j<=i<=min(n,j+kb).
*
* On exit, the factor S from the split Cholesky factorization
* B = S**T*S, as returned by SPBSTF.
*
* LDBB (input) INTEGER
* The leading dimension of the array BB. LDBB >= KB+1.
*
* W (output) REAL array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) REAL array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the matrix Z of
* eigenvectors, with the i-th column of Z holding the
* eigenvector associated with W(i). The eigenvectors are
* normalized so Z**T*B*Z = I.
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If N <= 1, LWORK >= 1.
* If JOBZ = 'N' and N > 1, LWORK >= 3*N.
* If JOBZ = 'V' and N > 1, LWORK >= 1 + 5*N + 2*N**2.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if LIWORK > 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If JOBZ = 'N' or N <= 1, LIWORK >= 1.
* If JOBZ = 'V' and N > 1, LIWORK >= 3 + 5*N.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is:
* <= N: the algorithm failed to converge:
* i off-diagonal elements of an intermediate
* tridiagonal form did not converge to zero;
* > N: if INFO = N + i, for 1 <= i <= N, then SPBSTF
* returned INFO = i: B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* Further Details
* ===============
*
* Based on contributions by
* Mark Fahey, Department of Mathematics, Univ. of Kentucky, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param uplo
* @param n
* @param ka
* @param kb
* @param ab
* @param ldab
* @param bb
* @param ldbb
* @param w
* @param z
* @param ldz
* @param work
* @param lwork
* @param iwork
* @param liwork
* @param info
*
*/
abstract public void ssbgvd(java.lang.String jobz, java.lang.String uplo, int n, int ka, int kb, float[] ab, int ldab, float[] bb, int ldbb, float[] w, float[] z, int ldz, float[] work, int lwork, int[] iwork, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSBGVD computes all the eigenvalues, and optionally, the eigenvectors
* of a real generalized symmetric-definite banded eigenproblem, of the
* form A*x=(lambda)*B*x. Here A and B are assumed to be symmetric and
* banded, and B is also positive definite. If eigenvectors are
* desired, it uses a divide and conquer algorithm.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangles of A and B are stored;
* = 'L': Lower triangles of A and B are stored.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* KA (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KA >= 0.
*
* KB (input) INTEGER
* The number of superdiagonals of the matrix B if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KB >= 0.
*
* AB (input/output) REAL array, dimension (LDAB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first ka+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(ka+1+i-j,j) = A(i,j) for max(1,j-ka)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+ka).
*
* On exit, the contents of AB are destroyed.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KA+1.
*
* BB (input/output) REAL array, dimension (LDBB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix B, stored in the first kb+1 rows of the array. The
* j-th column of B is stored in the j-th column of the array BB
* as follows:
* if UPLO = 'U', BB(ka+1+i-j,j) = B(i,j) for max(1,j-kb)<=i<=j;
* if UPLO = 'L', BB(1+i-j,j) = B(i,j) for j<=i<=min(n,j+kb).
*
* On exit, the factor S from the split Cholesky factorization
* B = S**T*S, as returned by SPBSTF.
*
* LDBB (input) INTEGER
* The leading dimension of the array BB. LDBB >= KB+1.
*
* W (output) REAL array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) REAL array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the matrix Z of
* eigenvectors, with the i-th column of Z holding the
* eigenvector associated with W(i). The eigenvectors are
* normalized so Z**T*B*Z = I.
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If N <= 1, LWORK >= 1.
* If JOBZ = 'N' and N > 1, LWORK >= 3*N.
* If JOBZ = 'V' and N > 1, LWORK >= 1 + 5*N + 2*N**2.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if LIWORK > 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If JOBZ = 'N' or N <= 1, LIWORK >= 1.
* If JOBZ = 'V' and N > 1, LIWORK >= 3 + 5*N.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is:
* <= N: the algorithm failed to converge:
* i off-diagonal elements of an intermediate
* tridiagonal form did not converge to zero;
* > N: if INFO = N + i, for 1 <= i <= N, then SPBSTF
* returned INFO = i: B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* Further Details
* ===============
*
* Based on contributions by
* Mark Fahey, Department of Mathematics, Univ. of Kentucky, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param uplo
* @param n
* @param ka
* @param kb
* @param ab
* @param _ab_offset
* @param ldab
* @param bb
* @param _bb_offset
* @param ldbb
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param liwork
* @param info
*
*/
abstract public void ssbgvd(java.lang.String jobz, java.lang.String uplo, int n, int ka, int kb, float[] ab, int _ab_offset, int ldab, float[] bb, int _bb_offset, int ldbb, float[] w, int _w_offset, float[] z, int _z_offset, int ldz, float[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSBGVX computes selected eigenvalues, and optionally, eigenvectors
* of a real generalized symmetric-definite banded eigenproblem, of
* the form A*x=(lambda)*B*x. Here A and B are assumed to be symmetric
* and banded, and B is also positive definite. Eigenvalues and
* eigenvectors can be selected by specifying either all eigenvalues,
* a range of values or a range of indices for the desired eigenvalues.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangles of A and B are stored;
* = 'L': Lower triangles of A and B are stored.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* KA (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KA >= 0.
*
* KB (input) INTEGER
* The number of superdiagonals of the matrix B if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KB >= 0.
*
* AB (input/output) REAL array, dimension (LDAB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first ka+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(ka+1+i-j,j) = A(i,j) for max(1,j-ka)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+ka).
*
* On exit, the contents of AB are destroyed.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KA+1.
*
* BB (input/output) REAL array, dimension (LDBB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix B, stored in the first kb+1 rows of the array. The
* j-th column of B is stored in the j-th column of the array BB
* as follows:
* if UPLO = 'U', BB(ka+1+i-j,j) = B(i,j) for max(1,j-kb)<=i<=j;
* if UPLO = 'L', BB(1+i-j,j) = B(i,j) for j<=i<=min(n,j+kb).
*
* On exit, the factor S from the split Cholesky factorization
* B = S**T*S, as returned by SPBSTF.
*
* LDBB (input) INTEGER
* The leading dimension of the array BB. LDBB >= KB+1.
*
* Q (output) REAL array, dimension (LDQ, N)
* If JOBZ = 'V', the n-by-n matrix used in the reduction of
* A*x = (lambda)*B*x to standard form, i.e. C*x = (lambda)*x,
* and consequently C to tridiagonal form.
* If JOBZ = 'N', the array Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. If JOBZ = 'N',
* LDQ >= 1. If JOBZ = 'V', LDQ >= max(1,N).
*
* VL (input) REAL
* VU (input) REAL
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) REAL
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less than
* or equal to zero, then EPS*|T| will be used in its place,
* where |T| is the 1-norm of the tridiagonal matrix obtained
* by reducing A to tridiagonal form.
*
* Eigenvalues will be computed most accurately when ABSTOL is
* set to twice the underflow threshold 2*SLAMCH('S'), not zero.
* If this routine returns with INFO>0, indicating that some
* eigenvectors did not converge, try setting ABSTOL to
* 2*SLAMCH('S').
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) REAL array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) REAL array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the matrix Z of
* eigenvectors, with the i-th column of Z holding the
* eigenvector associated with W(i). The eigenvectors are
* normalized so Z**T*B*Z = I.
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace/output) REAL array, dimension (7N)
*
* IWORK (workspace/output) INTEGER array, dimension (5N)
*
* IFAIL (output) INTEGER array, dimension (M)
* If JOBZ = 'V', then if INFO = 0, the first M elements of
* IFAIL are zero. If INFO > 0, then IFAIL contains the
* indices of the eigenvalues that failed to converge.
* If JOBZ = 'N', then IFAIL is not referenced.
*
* INFO (output) INTEGER
* = 0 : successful exit
* < 0 : if INFO = -i, the i-th argument had an illegal value
* <= N: if INFO = i, then i eigenvectors failed to converge.
* Their indices are stored in IFAIL.
* > N : SPBSTF returned an error code; i.e.,
* if INFO = N + i, for 1 <= i <= N, then the leading
* minor of order i of B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* Further Details
* ===============
*
* Based on contributions by
* Mark Fahey, Department of Mathematics, Univ. of Kentucky, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param range
* @param uplo
* @param n
* @param ka
* @param kb
* @param ab
* @param ldab
* @param bb
* @param ldbb
* @param q
* @param ldq
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param z
* @param ldz
* @param work
* @param iwork
* @param ifail
* @param info
*
*/
abstract public void ssbgvx(java.lang.String jobz, java.lang.String range, java.lang.String uplo, int n, int ka, int kb, float[] ab, int ldab, float[] bb, int ldbb, float[] q, int ldq, float vl, float vu, int il, int iu, float abstol, org.netlib.util.intW m, float[] w, float[] z, int ldz, float[] work, int[] iwork, int[] ifail, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSBGVX computes selected eigenvalues, and optionally, eigenvectors
* of a real generalized symmetric-definite banded eigenproblem, of
* the form A*x=(lambda)*B*x. Here A and B are assumed to be symmetric
* and banded, and B is also positive definite. Eigenvalues and
* eigenvectors can be selected by specifying either all eigenvalues,
* a range of values or a range of indices for the desired eigenvalues.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangles of A and B are stored;
* = 'L': Lower triangles of A and B are stored.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* KA (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KA >= 0.
*
* KB (input) INTEGER
* The number of superdiagonals of the matrix B if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KB >= 0.
*
* AB (input/output) REAL array, dimension (LDAB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first ka+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(ka+1+i-j,j) = A(i,j) for max(1,j-ka)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+ka).
*
* On exit, the contents of AB are destroyed.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KA+1.
*
* BB (input/output) REAL array, dimension (LDBB, N)
* On entry, the upper or lower triangle of the symmetric band
* matrix B, stored in the first kb+1 rows of the array. The
* j-th column of B is stored in the j-th column of the array BB
* as follows:
* if UPLO = 'U', BB(ka+1+i-j,j) = B(i,j) for max(1,j-kb)<=i<=j;
* if UPLO = 'L', BB(1+i-j,j) = B(i,j) for j<=i<=min(n,j+kb).
*
* On exit, the factor S from the split Cholesky factorization
* B = S**T*S, as returned by SPBSTF.
*
* LDBB (input) INTEGER
* The leading dimension of the array BB. LDBB >= KB+1.
*
* Q (output) REAL array, dimension (LDQ, N)
* If JOBZ = 'V', the n-by-n matrix used in the reduction of
* A*x = (lambda)*B*x to standard form, i.e. C*x = (lambda)*x,
* and consequently C to tridiagonal form.
* If JOBZ = 'N', the array Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. If JOBZ = 'N',
* LDQ >= 1. If JOBZ = 'V', LDQ >= max(1,N).
*
* VL (input) REAL
* VU (input) REAL
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) REAL
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less than
* or equal to zero, then EPS*|T| will be used in its place,
* where |T| is the 1-norm of the tridiagonal matrix obtained
* by reducing A to tridiagonal form.
*
* Eigenvalues will be computed most accurately when ABSTOL is
* set to twice the underflow threshold 2*SLAMCH('S'), not zero.
* If this routine returns with INFO>0, indicating that some
* eigenvectors did not converge, try setting ABSTOL to
* 2*SLAMCH('S').
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) REAL array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) REAL array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the matrix Z of
* eigenvectors, with the i-th column of Z holding the
* eigenvector associated with W(i). The eigenvectors are
* normalized so Z**T*B*Z = I.
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace/output) REAL array, dimension (7N)
*
* IWORK (workspace/output) INTEGER array, dimension (5N)
*
* IFAIL (output) INTEGER array, dimension (M)
* If JOBZ = 'V', then if INFO = 0, the first M elements of
* IFAIL are zero. If INFO > 0, then IFAIL contains the
* indices of the eigenvalues that failed to converge.
* If JOBZ = 'N', then IFAIL is not referenced.
*
* INFO (output) INTEGER
* = 0 : successful exit
* < 0 : if INFO = -i, the i-th argument had an illegal value
* <= N: if INFO = i, then i eigenvectors failed to converge.
* Their indices are stored in IFAIL.
* > N : SPBSTF returned an error code; i.e.,
* if INFO = N + i, for 1 <= i <= N, then the leading
* minor of order i of B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* Further Details
* ===============
*
* Based on contributions by
* Mark Fahey, Department of Mathematics, Univ. of Kentucky, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param range
* @param uplo
* @param n
* @param ka
* @param kb
* @param ab
* @param _ab_offset
* @param ldab
* @param bb
* @param _bb_offset
* @param ldbb
* @param q
* @param _q_offset
* @param ldq
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param ifail
* @param _ifail_offset
* @param info
*
*/
abstract public void ssbgvx(java.lang.String jobz, java.lang.String range, java.lang.String uplo, int n, int ka, int kb, float[] ab, int _ab_offset, int ldab, float[] bb, int _bb_offset, int ldbb, float[] q, int _q_offset, int ldq, float vl, float vu, int il, int iu, float abstol, org.netlib.util.intW m, float[] w, int _w_offset, float[] z, int _z_offset, int ldz, float[] work, int _work_offset, int[] iwork, int _iwork_offset, int[] ifail, int _ifail_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSBTRD reduces a real symmetric band matrix A to symmetric
* tridiagonal form T by an orthogonal similarity transformation:
* Q**T * A * Q = T.
*
* Arguments
* =========
*
* VECT (input) CHARACTER*1
* = 'N': do not form Q;
* = 'V': form Q;
* = 'U': update a matrix X, by forming X*Q.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* AB (input/output) REAL array, dimension (LDAB,N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
* On exit, the diagonal elements of AB are overwritten by the
* diagonal elements of the tridiagonal matrix T; if KD > 0, the
* elements on the first superdiagonal (if UPLO = 'U') or the
* first subdiagonal (if UPLO = 'L') are overwritten by the
* off-diagonal elements of T; the rest of AB is overwritten by
* values generated during the reduction.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* D (output) REAL array, dimension (N)
* The diagonal elements of the tridiagonal matrix T.
*
* E (output) REAL array, dimension (N-1)
* The off-diagonal elements of the tridiagonal matrix T:
* E(i) = T(i,i+1) if UPLO = 'U'; E(i) = T(i+1,i) if UPLO = 'L'.
*
* Q (input/output) REAL array, dimension (LDQ,N)
* On entry, if VECT = 'U', then Q must contain an N-by-N
* matrix X; if VECT = 'N' or 'V', then Q need not be set.
*
* On exit:
* if VECT = 'V', Q contains the N-by-N orthogonal matrix Q;
* if VECT = 'U', Q contains the product X*Q;
* if VECT = 'N', the array Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q.
* LDQ >= 1, and LDQ >= N if VECT = 'V' or 'U'.
*
* WORK (workspace) REAL array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* Modified by Linda Kaufman, Bell Labs.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param vect
* @param uplo
* @param n
* @param kd
* @param ab
* @param ldab
* @param d
* @param e
* @param q
* @param ldq
* @param work
* @param info
*
*/
abstract public void ssbtrd(java.lang.String vect, java.lang.String uplo, int n, int kd, float[] ab, int ldab, float[] d, float[] e, float[] q, int ldq, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSBTRD reduces a real symmetric band matrix A to symmetric
* tridiagonal form T by an orthogonal similarity transformation:
* Q**T * A * Q = T.
*
* Arguments
* =========
*
* VECT (input) CHARACTER*1
* = 'N': do not form Q;
* = 'V': form Q;
* = 'U': update a matrix X, by forming X*Q.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals of the matrix A if UPLO = 'U',
* or the number of subdiagonals if UPLO = 'L'. KD >= 0.
*
* AB (input/output) REAL array, dimension (LDAB,N)
* On entry, the upper or lower triangle of the symmetric band
* matrix A, stored in the first KD+1 rows of the array. The
* j-th column of A is stored in the j-th column of the array AB
* as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
* On exit, the diagonal elements of AB are overwritten by the
* diagonal elements of the tridiagonal matrix T; if KD > 0, the
* elements on the first superdiagonal (if UPLO = 'U') or the
* first subdiagonal (if UPLO = 'L') are overwritten by the
* off-diagonal elements of T; the rest of AB is overwritten by
* values generated during the reduction.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* D (output) REAL array, dimension (N)
* The diagonal elements of the tridiagonal matrix T.
*
* E (output) REAL array, dimension (N-1)
* The off-diagonal elements of the tridiagonal matrix T:
* E(i) = T(i,i+1) if UPLO = 'U'; E(i) = T(i+1,i) if UPLO = 'L'.
*
* Q (input/output) REAL array, dimension (LDQ,N)
* On entry, if VECT = 'U', then Q must contain an N-by-N
* matrix X; if VECT = 'N' or 'V', then Q need not be set.
*
* On exit:
* if VECT = 'V', Q contains the N-by-N orthogonal matrix Q;
* if VECT = 'U', Q contains the product X*Q;
* if VECT = 'N', the array Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q.
* LDQ >= 1, and LDQ >= N if VECT = 'V' or 'U'.
*
* WORK (workspace) REAL array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* Modified by Linda Kaufman, Bell Labs.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param vect
* @param uplo
* @param n
* @param kd
* @param ab
* @param _ab_offset
* @param ldab
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param q
* @param _q_offset
* @param ldq
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void ssbtrd(java.lang.String vect, java.lang.String uplo, int n, int kd, float[] ab, int _ab_offset, int ldab, float[] d, int _d_offset, float[] e, int _e_offset, float[] q, int _q_offset, int ldq, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSPCON estimates the reciprocal of the condition number (in the
* 1-norm) of a real symmetric packed matrix A using the factorization
* A = U*D*U**T or A = L*D*L**T computed by SSPTRF.
*
* An estimate is obtained for norm(inv(A)), and the reciprocal of the
* condition number is computed as RCOND = 1 / (ANORM * norm(inv(A))).
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the details of the factorization are stored
* as an upper or lower triangular matrix.
* = 'U': Upper triangular, form is A = U*D*U**T;
* = 'L': Lower triangular, form is A = L*D*L**T.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input) REAL array, dimension (N*(N+1)/2)
* The block diagonal matrix D and the multipliers used to
* obtain the factor U or L as computed by SSPTRF, stored as a
* packed triangular matrix.
*
* IPIV (input) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D
* as determined by SSPTRF.
*
* ANORM (input) REAL
* The 1-norm of the original matrix A.
*
* RCOND (output) REAL
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(ANORM * AINVNM), where AINVNM is an
* estimate of the 1-norm of inv(A) computed in this routine.
*
* WORK (workspace) REAL array, dimension (2*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param ipiv
* @param anorm
* @param rcond
* @param work
* @param iwork
* @param info
*
*/
abstract public void sspcon(java.lang.String uplo, int n, float[] ap, int[] ipiv, float anorm, org.netlib.util.floatW rcond, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSPCON estimates the reciprocal of the condition number (in the
* 1-norm) of a real symmetric packed matrix A using the factorization
* A = U*D*U**T or A = L*D*L**T computed by SSPTRF.
*
* An estimate is obtained for norm(inv(A)), and the reciprocal of the
* condition number is computed as RCOND = 1 / (ANORM * norm(inv(A))).
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the details of the factorization are stored
* as an upper or lower triangular matrix.
* = 'U': Upper triangular, form is A = U*D*U**T;
* = 'L': Lower triangular, form is A = L*D*L**T.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input) REAL array, dimension (N*(N+1)/2)
* The block diagonal matrix D and the multipliers used to
* obtain the factor U or L as computed by SSPTRF, stored as a
* packed triangular matrix.
*
* IPIV (input) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D
* as determined by SSPTRF.
*
* ANORM (input) REAL
* The 1-norm of the original matrix A.
*
* RCOND (output) REAL
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(ANORM * AINVNM), where AINVNM is an
* estimate of the 1-norm of inv(A) computed in this routine.
*
* WORK (workspace) REAL array, dimension (2*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param ipiv
* @param _ipiv_offset
* @param anorm
* @param rcond
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void sspcon(java.lang.String uplo, int n, float[] ap, int _ap_offset, int[] ipiv, int _ipiv_offset, float anorm, org.netlib.util.floatW rcond, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSPEV computes all the eigenvalues and, optionally, eigenvectors of a
* real symmetric matrix A in packed storage.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, AP is overwritten by values generated during the
* reduction to tridiagonal form. If UPLO = 'U', the diagonal
* and first superdiagonal of the tridiagonal matrix T overwrite
* the corresponding elements of A, and if UPLO = 'L', the
* diagonal and first subdiagonal of T overwrite the
* corresponding elements of A.
*
* W (output) REAL array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) REAL array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the orthonormal
* eigenvectors of the matrix A, with the i-th column of Z
* holding the eigenvector associated with W(i).
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, the algorithm failed to converge; i
* off-diagonal elements of an intermediate tridiagonal
* form did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param uplo
* @param n
* @param ap
* @param w
* @param z
* @param ldz
* @param work
* @param info
*
*/
abstract public void sspev(java.lang.String jobz, java.lang.String uplo, int n, float[] ap, float[] w, float[] z, int ldz, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSPEV computes all the eigenvalues and, optionally, eigenvectors of a
* real symmetric matrix A in packed storage.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, AP is overwritten by values generated during the
* reduction to tridiagonal form. If UPLO = 'U', the diagonal
* and first superdiagonal of the tridiagonal matrix T overwrite
* the corresponding elements of A, and if UPLO = 'L', the
* diagonal and first subdiagonal of T overwrite the
* corresponding elements of A.
*
* W (output) REAL array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) REAL array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the orthonormal
* eigenvectors of the matrix A, with the i-th column of Z
* holding the eigenvector associated with W(i).
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, the algorithm failed to converge; i
* off-diagonal elements of an intermediate tridiagonal
* form did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void sspev(java.lang.String jobz, java.lang.String uplo, int n, float[] ap, int _ap_offset, float[] w, int _w_offset, float[] z, int _z_offset, int ldz, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSPEVD computes all the eigenvalues and, optionally, eigenvectors
* of a real symmetric matrix A in packed storage. If eigenvectors are
* desired, it uses a divide and conquer algorithm.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, AP is overwritten by values generated during the
* reduction to tridiagonal form. If UPLO = 'U', the diagonal
* and first superdiagonal of the tridiagonal matrix T overwrite
* the corresponding elements of A, and if UPLO = 'L', the
* diagonal and first subdiagonal of T overwrite the
* corresponding elements of A.
*
* W (output) REAL array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) REAL array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the orthonormal
* eigenvectors of the matrix A, with the i-th column of Z
* holding the eigenvector associated with W(i).
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the required LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If N <= 1, LWORK must be at least 1.
* If JOBZ = 'N' and N > 1, LWORK must be at least 2*N.
* If JOBZ = 'V' and N > 1, LWORK must be at least
* 1 + 6*N + N**2.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the required sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the required LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If JOBZ = 'N' or N <= 1, LIWORK must be at least 1.
* If JOBZ = 'V' and N > 1, LIWORK must be at least 3 + 5*N.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the required sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, the algorithm failed to converge; i
* off-diagonal elements of an intermediate tridiagonal
* form did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param uplo
* @param n
* @param ap
* @param w
* @param z
* @param ldz
* @param work
* @param lwork
* @param iwork
* @param liwork
* @param info
*
*/
abstract public void sspevd(java.lang.String jobz, java.lang.String uplo, int n, float[] ap, float[] w, float[] z, int ldz, float[] work, int lwork, int[] iwork, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSPEVD computes all the eigenvalues and, optionally, eigenvectors
* of a real symmetric matrix A in packed storage. If eigenvectors are
* desired, it uses a divide and conquer algorithm.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, AP is overwritten by values generated during the
* reduction to tridiagonal form. If UPLO = 'U', the diagonal
* and first superdiagonal of the tridiagonal matrix T overwrite
* the corresponding elements of A, and if UPLO = 'L', the
* diagonal and first subdiagonal of T overwrite the
* corresponding elements of A.
*
* W (output) REAL array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) REAL array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the orthonormal
* eigenvectors of the matrix A, with the i-th column of Z
* holding the eigenvector associated with W(i).
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the required LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If N <= 1, LWORK must be at least 1.
* If JOBZ = 'N' and N > 1, LWORK must be at least 2*N.
* If JOBZ = 'V' and N > 1, LWORK must be at least
* 1 + 6*N + N**2.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the required sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the required LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If JOBZ = 'N' or N <= 1, LIWORK must be at least 1.
* If JOBZ = 'V' and N > 1, LIWORK must be at least 3 + 5*N.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the required sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: if INFO = i, the algorithm failed to converge; i
* off-diagonal elements of an intermediate tridiagonal
* form did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param liwork
* @param info
*
*/
abstract public void sspevd(java.lang.String jobz, java.lang.String uplo, int n, float[] ap, int _ap_offset, float[] w, int _w_offset, float[] z, int _z_offset, int ldz, float[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSPEVX computes selected eigenvalues and, optionally, eigenvectors
* of a real symmetric matrix A in packed storage. Eigenvalues/vectors
* can be selected by specifying either a range of values or a range of
* indices for the desired eigenvalues.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found;
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found;
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, AP is overwritten by values generated during the
* reduction to tridiagonal form. If UPLO = 'U', the diagonal
* and first superdiagonal of the tridiagonal matrix T overwrite
* the corresponding elements of A, and if UPLO = 'L', the
* diagonal and first subdiagonal of T overwrite the
* corresponding elements of A.
*
* VL (input) REAL
* VU (input) REAL
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) REAL
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less than
* or equal to zero, then EPS*|T| will be used in its place,
* where |T| is the 1-norm of the tridiagonal matrix obtained
* by reducing AP to tridiagonal form.
*
* Eigenvalues will be computed most accurately when ABSTOL is
* set to twice the underflow threshold 2*SLAMCH('S'), not zero.
* If this routine returns with INFO>0, indicating that some
* eigenvectors did not converge, try setting ABSTOL to
* 2*SLAMCH('S').
*
* See "Computing Small Singular Values of Bidiagonal Matrices
* with Guaranteed High Relative Accuracy," by Demmel and
* Kahan, LAPACK Working Note #3.
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) REAL array, dimension (N)
* If INFO = 0, the selected eigenvalues in ascending order.
*
* Z (output) REAL array, dimension (LDZ, max(1,M))
* If JOBZ = 'V', then if INFO = 0, the first M columns of Z
* contain the orthonormal eigenvectors of the matrix A
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* If an eigenvector fails to converge, then that column of Z
* contains the latest approximation to the eigenvector, and the
* index of the eigenvector is returned in IFAIL.
* If JOBZ = 'N', then Z is not referenced.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace) REAL array, dimension (8*N)
*
* IWORK (workspace) INTEGER array, dimension (5*N)
*
* IFAIL (output) INTEGER array, dimension (N)
* If JOBZ = 'V', then if INFO = 0, the first M elements of
* IFAIL are zero. If INFO > 0, then IFAIL contains the
* indices of the eigenvectors that failed to converge.
* If JOBZ = 'N', then IFAIL is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, then i eigenvectors failed to converge.
* Their indices are stored in array IFAIL.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param range
* @param uplo
* @param n
* @param ap
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param z
* @param ldz
* @param work
* @param iwork
* @param ifail
* @param info
*
*/
abstract public void sspevx(java.lang.String jobz, java.lang.String range, java.lang.String uplo, int n, float[] ap, float vl, float vu, int il, int iu, float abstol, org.netlib.util.intW m, float[] w, float[] z, int ldz, float[] work, int[] iwork, int[] ifail, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSPEVX computes selected eigenvalues and, optionally, eigenvectors
* of a real symmetric matrix A in packed storage. Eigenvalues/vectors
* can be selected by specifying either a range of values or a range of
* indices for the desired eigenvalues.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found;
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found;
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, AP is overwritten by values generated during the
* reduction to tridiagonal form. If UPLO = 'U', the diagonal
* and first superdiagonal of the tridiagonal matrix T overwrite
* the corresponding elements of A, and if UPLO = 'L', the
* diagonal and first subdiagonal of T overwrite the
* corresponding elements of A.
*
* VL (input) REAL
* VU (input) REAL
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) REAL
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less than
* or equal to zero, then EPS*|T| will be used in its place,
* where |T| is the 1-norm of the tridiagonal matrix obtained
* by reducing AP to tridiagonal form.
*
* Eigenvalues will be computed most accurately when ABSTOL is
* set to twice the underflow threshold 2*SLAMCH('S'), not zero.
* If this routine returns with INFO>0, indicating that some
* eigenvectors did not converge, try setting ABSTOL to
* 2*SLAMCH('S').
*
* See "Computing Small Singular Values of Bidiagonal Matrices
* with Guaranteed High Relative Accuracy," by Demmel and
* Kahan, LAPACK Working Note #3.
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) REAL array, dimension (N)
* If INFO = 0, the selected eigenvalues in ascending order.
*
* Z (output) REAL array, dimension (LDZ, max(1,M))
* If JOBZ = 'V', then if INFO = 0, the first M columns of Z
* contain the orthonormal eigenvectors of the matrix A
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* If an eigenvector fails to converge, then that column of Z
* contains the latest approximation to the eigenvector, and the
* index of the eigenvector is returned in IFAIL.
* If JOBZ = 'N', then Z is not referenced.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace) REAL array, dimension (8*N)
*
* IWORK (workspace) INTEGER array, dimension (5*N)
*
* IFAIL (output) INTEGER array, dimension (N)
* If JOBZ = 'V', then if INFO = 0, the first M elements of
* IFAIL are zero. If INFO > 0, then IFAIL contains the
* indices of the eigenvectors that failed to converge.
* If JOBZ = 'N', then IFAIL is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, then i eigenvectors failed to converge.
* Their indices are stored in array IFAIL.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param range
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param ifail
* @param _ifail_offset
* @param info
*
*/
abstract public void sspevx(java.lang.String jobz, java.lang.String range, java.lang.String uplo, int n, float[] ap, int _ap_offset, float vl, float vu, int il, int iu, float abstol, org.netlib.util.intW m, float[] w, int _w_offset, float[] z, int _z_offset, int ldz, float[] work, int _work_offset, int[] iwork, int _iwork_offset, int[] ifail, int _ifail_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSPGST reduces a real symmetric-definite generalized eigenproblem
* to standard form, using packed storage.
*
* If ITYPE = 1, the problem is A*x = lambda*B*x,
* and A is overwritten by inv(U**T)*A*inv(U) or inv(L)*A*inv(L**T)
*
* If ITYPE = 2 or 3, the problem is A*B*x = lambda*x or
* B*A*x = lambda*x, and A is overwritten by U*A*U**T or L**T*A*L.
*
* B must have been previously factorized as U**T*U or L*L**T by SPPTRF.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* = 1: compute inv(U**T)*A*inv(U) or inv(L)*A*inv(L**T);
* = 2 or 3: compute U*A*U**T or L**T*A*L.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored and B is factored as
* U**T*U;
* = 'L': Lower triangle of A is stored and B is factored as
* L*L**T.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* AP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, if INFO = 0, the transformed matrix, stored in the
* same format as A.
*
* BP (input) REAL array, dimension (N*(N+1)/2)
* The triangular factor from the Cholesky factorization of B,
* stored in the same format as A, as returned by SPPTRF.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param itype
* @param uplo
* @param n
* @param ap
* @param bp
* @param info
*
*/
abstract public void sspgst(int itype, java.lang.String uplo, int n, float[] ap, float[] bp, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSPGST reduces a real symmetric-definite generalized eigenproblem
* to standard form, using packed storage.
*
* If ITYPE = 1, the problem is A*x = lambda*B*x,
* and A is overwritten by inv(U**T)*A*inv(U) or inv(L)*A*inv(L**T)
*
* If ITYPE = 2 or 3, the problem is A*B*x = lambda*x or
* B*A*x = lambda*x, and A is overwritten by U*A*U**T or L**T*A*L.
*
* B must have been previously factorized as U**T*U or L*L**T by SPPTRF.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* = 1: compute inv(U**T)*A*inv(U) or inv(L)*A*inv(L**T);
* = 2 or 3: compute U*A*U**T or L**T*A*L.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored and B is factored as
* U**T*U;
* = 'L': Lower triangle of A is stored and B is factored as
* L*L**T.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* AP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, if INFO = 0, the transformed matrix, stored in the
* same format as A.
*
* BP (input) REAL array, dimension (N*(N+1)/2)
* The triangular factor from the Cholesky factorization of B,
* stored in the same format as A, as returned by SPPTRF.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param itype
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param bp
* @param _bp_offset
* @param info
*
*/
abstract public void sspgst(int itype, java.lang.String uplo, int n, float[] ap, int _ap_offset, float[] bp, int _bp_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSPGV computes all the eigenvalues and, optionally, the eigenvectors
* of a real generalized symmetric-definite eigenproblem, of the form
* A*x=(lambda)*B*x, A*Bx=(lambda)*x, or B*A*x=(lambda)*x.
* Here A and B are assumed to be symmetric, stored in packed format,
* and B is also positive definite.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* Specifies the problem type to be solved:
* = 1: A*x = (lambda)*B*x
* = 2: A*B*x = (lambda)*x
* = 3: B*A*x = (lambda)*x
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangles of A and B are stored;
* = 'L': Lower triangles of A and B are stored.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* AP (input/output) REAL array, dimension
* (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, the contents of AP are destroyed.
*
* BP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* B, packed columnwise in a linear array. The j-th column of B
* is stored in the array BP as follows:
* if UPLO = 'U', BP(i + (j-1)*j/2) = B(i,j) for 1<=i<=j;
* if UPLO = 'L', BP(i + (j-1)*(2*n-j)/2) = B(i,j) for j<=i<=n.
*
* On exit, the triangular factor U or L from the Cholesky
* factorization B = U**T*U or B = L*L**T, in the same storage
* format as B.
*
* W (output) REAL array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) REAL array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the matrix Z of
* eigenvectors. The eigenvectors are normalized as follows:
* if ITYPE = 1 or 2, Z**T*B*Z = I;
* if ITYPE = 3, Z**T*inv(B)*Z = I.
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: SPPTRF or SSPEV returned an error code:
* <= N: if INFO = i, SSPEV failed to converge;
* i off-diagonal elements of an intermediate
* tridiagonal form did not converge to zero.
* > N: if INFO = n + i, for 1 <= i <= n, then the leading
* minor of order i of B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param itype
* @param jobz
* @param uplo
* @param n
* @param ap
* @param bp
* @param w
* @param z
* @param ldz
* @param work
* @param info
*
*/
abstract public void sspgv(int itype, java.lang.String jobz, java.lang.String uplo, int n, float[] ap, float[] bp, float[] w, float[] z, int ldz, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSPGV computes all the eigenvalues and, optionally, the eigenvectors
* of a real generalized symmetric-definite eigenproblem, of the form
* A*x=(lambda)*B*x, A*Bx=(lambda)*x, or B*A*x=(lambda)*x.
* Here A and B are assumed to be symmetric, stored in packed format,
* and B is also positive definite.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* Specifies the problem type to be solved:
* = 1: A*x = (lambda)*B*x
* = 2: A*B*x = (lambda)*x
* = 3: B*A*x = (lambda)*x
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangles of A and B are stored;
* = 'L': Lower triangles of A and B are stored.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* AP (input/output) REAL array, dimension
* (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, the contents of AP are destroyed.
*
* BP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* B, packed columnwise in a linear array. The j-th column of B
* is stored in the array BP as follows:
* if UPLO = 'U', BP(i + (j-1)*j/2) = B(i,j) for 1<=i<=j;
* if UPLO = 'L', BP(i + (j-1)*(2*n-j)/2) = B(i,j) for j<=i<=n.
*
* On exit, the triangular factor U or L from the Cholesky
* factorization B = U**T*U or B = L*L**T, in the same storage
* format as B.
*
* W (output) REAL array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) REAL array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the matrix Z of
* eigenvectors. The eigenvectors are normalized as follows:
* if ITYPE = 1 or 2, Z**T*B*Z = I;
* if ITYPE = 3, Z**T*inv(B)*Z = I.
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: SPPTRF or SSPEV returned an error code:
* <= N: if INFO = i, SSPEV failed to converge;
* i off-diagonal elements of an intermediate
* tridiagonal form did not converge to zero.
* > N: if INFO = n + i, for 1 <= i <= n, then the leading
* minor of order i of B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param itype
* @param jobz
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param bp
* @param _bp_offset
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void sspgv(int itype, java.lang.String jobz, java.lang.String uplo, int n, float[] ap, int _ap_offset, float[] bp, int _bp_offset, float[] w, int _w_offset, float[] z, int _z_offset, int ldz, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSPGVD computes all the eigenvalues, and optionally, the eigenvectors
* of a real generalized symmetric-definite eigenproblem, of the form
* A*x=(lambda)*B*x, A*Bx=(lambda)*x, or B*A*x=(lambda)*x. Here A and
* B are assumed to be symmetric, stored in packed format, and B is also
* positive definite.
* If eigenvectors are desired, it uses a divide and conquer algorithm.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* Specifies the problem type to be solved:
* = 1: A*x = (lambda)*B*x
* = 2: A*B*x = (lambda)*x
* = 3: B*A*x = (lambda)*x
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangles of A and B are stored;
* = 'L': Lower triangles of A and B are stored.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* AP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, the contents of AP are destroyed.
*
* BP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* B, packed columnwise in a linear array. The j-th column of B
* is stored in the array BP as follows:
* if UPLO = 'U', BP(i + (j-1)*j/2) = B(i,j) for 1<=i<=j;
* if UPLO = 'L', BP(i + (j-1)*(2*n-j)/2) = B(i,j) for j<=i<=n.
*
* On exit, the triangular factor U or L from the Cholesky
* factorization B = U**T*U or B = L*L**T, in the same storage
* format as B.
*
* W (output) REAL array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) REAL array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the matrix Z of
* eigenvectors. The eigenvectors are normalized as follows:
* if ITYPE = 1 or 2, Z**T*B*Z = I;
* if ITYPE = 3, Z**T*inv(B)*Z = I.
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the required LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If N <= 1, LWORK >= 1.
* If JOBZ = 'N' and N > 1, LWORK >= 2*N.
* If JOBZ = 'V' and N > 1, LWORK >= 1 + 6*N + 2*N**2.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the required sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the required LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If JOBZ = 'N' or N <= 1, LIWORK >= 1.
* If JOBZ = 'V' and N > 1, LIWORK >= 3 + 5*N.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the required sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: SPPTRF or SSPEVD returned an error code:
* <= N: if INFO = i, SSPEVD failed to converge;
* i off-diagonal elements of an intermediate
* tridiagonal form did not converge to zero;
* > N: if INFO = N + i, for 1 <= i <= N, then the leading
* minor of order i of B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* Further Details
* ===============
*
* Based on contributions by
* Mark Fahey, Department of Mathematics, Univ. of Kentucky, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param itype
* @param jobz
* @param uplo
* @param n
* @param ap
* @param bp
* @param w
* @param z
* @param ldz
* @param work
* @param lwork
* @param iwork
* @param liwork
* @param info
*
*/
abstract public void sspgvd(int itype, java.lang.String jobz, java.lang.String uplo, int n, float[] ap, float[] bp, float[] w, float[] z, int ldz, float[] work, int lwork, int[] iwork, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSPGVD computes all the eigenvalues, and optionally, the eigenvectors
* of a real generalized symmetric-definite eigenproblem, of the form
* A*x=(lambda)*B*x, A*Bx=(lambda)*x, or B*A*x=(lambda)*x. Here A and
* B are assumed to be symmetric, stored in packed format, and B is also
* positive definite.
* If eigenvectors are desired, it uses a divide and conquer algorithm.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* Specifies the problem type to be solved:
* = 1: A*x = (lambda)*B*x
* = 2: A*B*x = (lambda)*x
* = 3: B*A*x = (lambda)*x
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangles of A and B are stored;
* = 'L': Lower triangles of A and B are stored.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* AP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, the contents of AP are destroyed.
*
* BP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* B, packed columnwise in a linear array. The j-th column of B
* is stored in the array BP as follows:
* if UPLO = 'U', BP(i + (j-1)*j/2) = B(i,j) for 1<=i<=j;
* if UPLO = 'L', BP(i + (j-1)*(2*n-j)/2) = B(i,j) for j<=i<=n.
*
* On exit, the triangular factor U or L from the Cholesky
* factorization B = U**T*U or B = L*L**T, in the same storage
* format as B.
*
* W (output) REAL array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* Z (output) REAL array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the matrix Z of
* eigenvectors. The eigenvectors are normalized as follows:
* if ITYPE = 1 or 2, Z**T*B*Z = I;
* if ITYPE = 3, Z**T*inv(B)*Z = I.
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the required LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If N <= 1, LWORK >= 1.
* If JOBZ = 'N' and N > 1, LWORK >= 2*N.
* If JOBZ = 'V' and N > 1, LWORK >= 1 + 6*N + 2*N**2.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the required sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the required LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If JOBZ = 'N' or N <= 1, LIWORK >= 1.
* If JOBZ = 'V' and N > 1, LIWORK >= 3 + 5*N.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the required sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: SPPTRF or SSPEVD returned an error code:
* <= N: if INFO = i, SSPEVD failed to converge;
* i off-diagonal elements of an intermediate
* tridiagonal form did not converge to zero;
* > N: if INFO = N + i, for 1 <= i <= N, then the leading
* minor of order i of B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* Further Details
* ===============
*
* Based on contributions by
* Mark Fahey, Department of Mathematics, Univ. of Kentucky, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param itype
* @param jobz
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param bp
* @param _bp_offset
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param liwork
* @param info
*
*/
abstract public void sspgvd(int itype, java.lang.String jobz, java.lang.String uplo, int n, float[] ap, int _ap_offset, float[] bp, int _bp_offset, float[] w, int _w_offset, float[] z, int _z_offset, int ldz, float[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSPGVX computes selected eigenvalues, and optionally, eigenvectors
* of a real generalized symmetric-definite eigenproblem, of the form
* A*x=(lambda)*B*x, A*Bx=(lambda)*x, or B*A*x=(lambda)*x. Here A
* and B are assumed to be symmetric, stored in packed storage, and B
* is also positive definite. Eigenvalues and eigenvectors can be
* selected by specifying either a range of values or a range of indices
* for the desired eigenvalues.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* Specifies the problem type to be solved:
* = 1: A*x = (lambda)*B*x
* = 2: A*B*x = (lambda)*x
* = 3: B*A*x = (lambda)*x
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A and B are stored;
* = 'L': Lower triangle of A and B are stored.
*
* N (input) INTEGER
* The order of the matrix pencil (A,B). N >= 0.
*
* AP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, the contents of AP are destroyed.
*
* BP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* B, packed columnwise in a linear array. The j-th column of B
* is stored in the array BP as follows:
* if UPLO = 'U', BP(i + (j-1)*j/2) = B(i,j) for 1<=i<=j;
* if UPLO = 'L', BP(i + (j-1)*(2*n-j)/2) = B(i,j) for j<=i<=n.
*
* On exit, the triangular factor U or L from the Cholesky
* factorization B = U**T*U or B = L*L**T, in the same storage
* format as B.
*
* VL (input) REAL
* VU (input) REAL
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) REAL
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less than
* or equal to zero, then EPS*|T| will be used in its place,
* where |T| is the 1-norm of the tridiagonal matrix obtained
* by reducing A to tridiagonal form.
*
* Eigenvalues will be computed most accurately when ABSTOL is
* set to twice the underflow threshold 2*SLAMCH('S'), not zero.
* If this routine returns with INFO>0, indicating that some
* eigenvectors did not converge, try setting ABSTOL to
* 2*SLAMCH('S').
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) REAL array, dimension (N)
* On normal exit, the first M elements contain the selected
* eigenvalues in ascending order.
*
* Z (output) REAL array, dimension (LDZ, max(1,M))
* If JOBZ = 'N', then Z is not referenced.
* If JOBZ = 'V', then if INFO = 0, the first M columns of Z
* contain the orthonormal eigenvectors of the matrix A
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* The eigenvectors are normalized as follows:
* if ITYPE = 1 or 2, Z**T*B*Z = I;
* if ITYPE = 3, Z**T*inv(B)*Z = I.
*
* If an eigenvector fails to converge, then that column of Z
* contains the latest approximation to the eigenvector, and the
* index of the eigenvector is returned in IFAIL.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace) REAL array, dimension (8*N)
*
* IWORK (workspace) INTEGER array, dimension (5*N)
*
* IFAIL (output) INTEGER array, dimension (N)
* If JOBZ = 'V', then if INFO = 0, the first M elements of
* IFAIL are zero. If INFO > 0, then IFAIL contains the
* indices of the eigenvectors that failed to converge.
* If JOBZ = 'N', then IFAIL is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: SPPTRF or SSPEVX returned an error code:
* <= N: if INFO = i, SSPEVX failed to converge;
* i eigenvectors failed to converge. Their indices
* are stored in array IFAIL.
* > N: if INFO = N + i, for 1 <= i <= N, then the leading
* minor of order i of B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* Further Details
* ===============
*
* Based on contributions by
* Mark Fahey, Department of Mathematics, Univ. of Kentucky, USA
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param itype
* @param jobz
* @param range
* @param uplo
* @param n
* @param ap
* @param bp
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param z
* @param ldz
* @param work
* @param iwork
* @param ifail
* @param info
*
*/
abstract public void sspgvx(int itype, java.lang.String jobz, java.lang.String range, java.lang.String uplo, int n, float[] ap, float[] bp, float vl, float vu, int il, int iu, float abstol, org.netlib.util.intW m, float[] w, float[] z, int ldz, float[] work, int[] iwork, int[] ifail, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSPGVX computes selected eigenvalues, and optionally, eigenvectors
* of a real generalized symmetric-definite eigenproblem, of the form
* A*x=(lambda)*B*x, A*Bx=(lambda)*x, or B*A*x=(lambda)*x. Here A
* and B are assumed to be symmetric, stored in packed storage, and B
* is also positive definite. Eigenvalues and eigenvectors can be
* selected by specifying either a range of values or a range of indices
* for the desired eigenvalues.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* Specifies the problem type to be solved:
* = 1: A*x = (lambda)*B*x
* = 2: A*B*x = (lambda)*x
* = 3: B*A*x = (lambda)*x
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A and B are stored;
* = 'L': Lower triangle of A and B are stored.
*
* N (input) INTEGER
* The order of the matrix pencil (A,B). N >= 0.
*
* AP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, the contents of AP are destroyed.
*
* BP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* B, packed columnwise in a linear array. The j-th column of B
* is stored in the array BP as follows:
* if UPLO = 'U', BP(i + (j-1)*j/2) = B(i,j) for 1<=i<=j;
* if UPLO = 'L', BP(i + (j-1)*(2*n-j)/2) = B(i,j) for j<=i<=n.
*
* On exit, the triangular factor U or L from the Cholesky
* factorization B = U**T*U or B = L*L**T, in the same storage
* format as B.
*
* VL (input) REAL
* VU (input) REAL
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) REAL
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less than
* or equal to zero, then EPS*|T| will be used in its place,
* where |T| is the 1-norm of the tridiagonal matrix obtained
* by reducing A to tridiagonal form.
*
* Eigenvalues will be computed most accurately when ABSTOL is
* set to twice the underflow threshold 2*SLAMCH('S'), not zero.
* If this routine returns with INFO>0, indicating that some
* eigenvectors did not converge, try setting ABSTOL to
* 2*SLAMCH('S').
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) REAL array, dimension (N)
* On normal exit, the first M elements contain the selected
* eigenvalues in ascending order.
*
* Z (output) REAL array, dimension (LDZ, max(1,M))
* If JOBZ = 'N', then Z is not referenced.
* If JOBZ = 'V', then if INFO = 0, the first M columns of Z
* contain the orthonormal eigenvectors of the matrix A
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* The eigenvectors are normalized as follows:
* if ITYPE = 1 or 2, Z**T*B*Z = I;
* if ITYPE = 3, Z**T*inv(B)*Z = I.
*
* If an eigenvector fails to converge, then that column of Z
* contains the latest approximation to the eigenvector, and the
* index of the eigenvector is returned in IFAIL.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace) REAL array, dimension (8*N)
*
* IWORK (workspace) INTEGER array, dimension (5*N)
*
* IFAIL (output) INTEGER array, dimension (N)
* If JOBZ = 'V', then if INFO = 0, the first M elements of
* IFAIL are zero. If INFO > 0, then IFAIL contains the
* indices of the eigenvectors that failed to converge.
* If JOBZ = 'N', then IFAIL is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: SPPTRF or SSPEVX returned an error code:
* <= N: if INFO = i, SSPEVX failed to converge;
* i eigenvectors failed to converge. Their indices
* are stored in array IFAIL.
* > N: if INFO = N + i, for 1 <= i <= N, then the leading
* minor of order i of B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* Further Details
* ===============
*
* Based on contributions by
* Mark Fahey, Department of Mathematics, Univ. of Kentucky, USA
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param itype
* @param jobz
* @param range
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param bp
* @param _bp_offset
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param ifail
* @param _ifail_offset
* @param info
*
*/
abstract public void sspgvx(int itype, java.lang.String jobz, java.lang.String range, java.lang.String uplo, int n, float[] ap, int _ap_offset, float[] bp, int _bp_offset, float vl, float vu, int il, int iu, float abstol, org.netlib.util.intW m, float[] w, int _w_offset, float[] z, int _z_offset, int ldz, float[] work, int _work_offset, int[] iwork, int _iwork_offset, int[] ifail, int _ifail_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSPRFS improves the computed solution to a system of linear
* equations when the coefficient matrix is symmetric indefinite
* and packed, and provides error bounds and backward error estimates
* for the solution.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AP (input) REAL array, dimension (N*(N+1)/2)
* The upper or lower triangle of the symmetric matrix A, packed
* columnwise in a linear array. The j-th column of A is stored
* in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
*
* AFP (input) REAL array, dimension (N*(N+1)/2)
* The factored form of the matrix A. AFP contains the block
* diagonal matrix D and the multipliers used to obtain the
* factor U or L from the factorization A = U*D*U**T or
* A = L*D*L**T as computed by SSPTRF, stored as a packed
* triangular matrix.
*
* IPIV (input) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D
* as determined by SSPTRF.
*
* B (input) REAL array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) REAL array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by SSPTRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param ap
* @param afp
* @param ipiv
* @param b
* @param ldb
* @param x
* @param ldx
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void ssprfs(java.lang.String uplo, int n, int nrhs, float[] ap, float[] afp, int[] ipiv, float[] b, int ldb, float[] x, int ldx, float[] ferr, float[] berr, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSPRFS improves the computed solution to a system of linear
* equations when the coefficient matrix is symmetric indefinite
* and packed, and provides error bounds and backward error estimates
* for the solution.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AP (input) REAL array, dimension (N*(N+1)/2)
* The upper or lower triangle of the symmetric matrix A, packed
* columnwise in a linear array. The j-th column of A is stored
* in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
*
* AFP (input) REAL array, dimension (N*(N+1)/2)
* The factored form of the matrix A. AFP contains the block
* diagonal matrix D and the multipliers used to obtain the
* factor U or L from the factorization A = U*D*U**T or
* A = L*D*L**T as computed by SSPTRF, stored as a packed
* triangular matrix.
*
* IPIV (input) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D
* as determined by SSPTRF.
*
* B (input) REAL array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) REAL array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by SSPTRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param ap
* @param _ap_offset
* @param afp
* @param _afp_offset
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void ssprfs(java.lang.String uplo, int n, int nrhs, float[] ap, int _ap_offset, float[] afp, int _afp_offset, int[] ipiv, int _ipiv_offset, float[] b, int _b_offset, int ldb, float[] x, int _x_offset, int ldx, float[] ferr, int _ferr_offset, float[] berr, int _berr_offset, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSPSV computes the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N symmetric matrix stored in packed format and X
* and B are N-by-NRHS matrices.
*
* The diagonal pivoting method is used to factor A as
* A = U * D * U**T, if UPLO = 'U', or
* A = L * D * L**T, if UPLO = 'L',
* where U (or L) is a product of permutation and unit upper (lower)
* triangular matrices, D is symmetric and block diagonal with 1-by-1
* and 2-by-2 diagonal blocks. The factored form of A is then used to
* solve the system of equations A * X = B.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
* See below for further details.
*
* On exit, the block diagonal matrix D and the multipliers used
* to obtain the factor U or L from the factorization
* A = U*D*U**T or A = L*D*L**T as computed by SSPTRF, stored as
* a packed triangular matrix in the same storage format as A.
*
* IPIV (output) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D, as
* determined by SSPTRF. If IPIV(k) > 0, then rows and columns
* k and IPIV(k) were interchanged, and D(k,k) is a 1-by-1
* diagonal block. If UPLO = 'U' and IPIV(k) = IPIV(k-1) < 0,
* then rows and columns k-1 and -IPIV(k) were interchanged and
* D(k-1:k,k-1:k) is a 2-by-2 diagonal block. If UPLO = 'L' and
* IPIV(k) = IPIV(k+1) < 0, then rows and columns k+1 and
* -IPIV(k) were interchanged and D(k:k+1,k:k+1) is a 2-by-2
* diagonal block.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if INFO = 0, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, D(i,i) is exactly zero. The factorization
* has been completed, but the block diagonal matrix D is
* exactly singular, so the solution could not be
* computed.
*
* Further Details
* ===============
*
* The packed storage scheme is illustrated by the following example
* when N = 4, UPLO = 'U':
*
* Two-dimensional storage of the symmetric matrix A:
*
* a11 a12 a13 a14
* a22 a23 a24
* a33 a34 (aij = aji)
* a44
*
* Packed storage of the upper triangle of A:
*
* AP = [ a11, a12, a22, a13, a23, a33, a14, a24, a34, a44 ]
*
* =====================================================================
*
* .. External Functions ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param ap
* @param ipiv
* @param b
* @param ldb
* @param info
*
*/
abstract public void sspsv(java.lang.String uplo, int n, int nrhs, float[] ap, int[] ipiv, float[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSPSV computes the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N symmetric matrix stored in packed format and X
* and B are N-by-NRHS matrices.
*
* The diagonal pivoting method is used to factor A as
* A = U * D * U**T, if UPLO = 'U', or
* A = L * D * L**T, if UPLO = 'L',
* where U (or L) is a product of permutation and unit upper (lower)
* triangular matrices, D is symmetric and block diagonal with 1-by-1
* and 2-by-2 diagonal blocks. The factored form of A is then used to
* solve the system of equations A * X = B.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
* See below for further details.
*
* On exit, the block diagonal matrix D and the multipliers used
* to obtain the factor U or L from the factorization
* A = U*D*U**T or A = L*D*L**T as computed by SSPTRF, stored as
* a packed triangular matrix in the same storage format as A.
*
* IPIV (output) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D, as
* determined by SSPTRF. If IPIV(k) > 0, then rows and columns
* k and IPIV(k) were interchanged, and D(k,k) is a 1-by-1
* diagonal block. If UPLO = 'U' and IPIV(k) = IPIV(k-1) < 0,
* then rows and columns k-1 and -IPIV(k) were interchanged and
* D(k-1:k,k-1:k) is a 2-by-2 diagonal block. If UPLO = 'L' and
* IPIV(k) = IPIV(k+1) < 0, then rows and columns k+1 and
* -IPIV(k) were interchanged and D(k:k+1,k:k+1) is a 2-by-2
* diagonal block.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if INFO = 0, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, D(i,i) is exactly zero. The factorization
* has been completed, but the block diagonal matrix D is
* exactly singular, so the solution could not be
* computed.
*
* Further Details
* ===============
*
* The packed storage scheme is illustrated by the following example
* when N = 4, UPLO = 'U':
*
* Two-dimensional storage of the symmetric matrix A:
*
* a11 a12 a13 a14
* a22 a23 a24
* a33 a34 (aij = aji)
* a44
*
* Packed storage of the upper triangle of A:
*
* AP = [ a11, a12, a22, a13, a23, a33, a14, a24, a34, a44 ]
*
* =====================================================================
*
* .. External Functions ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param ap
* @param _ap_offset
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void sspsv(java.lang.String uplo, int n, int nrhs, float[] ap, int _ap_offset, int[] ipiv, int _ipiv_offset, float[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSPSVX uses the diagonal pivoting factorization A = U*D*U**T or
* A = L*D*L**T to compute the solution to a real system of linear
* equations A * X = B, where A is an N-by-N symmetric matrix stored
* in packed format and X and B are N-by-NRHS matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed:
*
* 1. If FACT = 'N', the diagonal pivoting method is used to factor A as
* A = U * D * U**T, if UPLO = 'U', or
* A = L * D * L**T, if UPLO = 'L',
* where U (or L) is a product of permutation and unit upper (lower)
* triangular matrices and D is symmetric and block diagonal with
* 1-by-1 and 2-by-2 diagonal blocks.
*
* 2. If some D(i,i)=0, so that D is exactly singular, then the routine
* returns with INFO = i. Otherwise, the factored form of A is used
* to estimate the condition number of the matrix A. If the
* reciprocal of the condition number is less than machine precision,
* INFO = N+1 is returned as a warning, but the routine still goes on
* to solve for X and compute error bounds as described below.
*
* 3. The system of equations is solved for X using the factored form
* of A.
*
* 4. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of A has been
* supplied on entry.
* = 'F': On entry, AFP and IPIV contain the factored form of
* A. AP, AFP and IPIV will not be modified.
* = 'N': The matrix A will be copied to AFP and factored.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AP (input) REAL array, dimension (N*(N+1)/2)
* The upper or lower triangle of the symmetric matrix A, packed
* columnwise in a linear array. The j-th column of A is stored
* in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
* See below for further details.
*
* AFP (input or output) REAL array, dimension
* (N*(N+1)/2)
* If FACT = 'F', then AFP is an input argument and on entry
* contains the block diagonal matrix D and the multipliers used
* to obtain the factor U or L from the factorization
* A = U*D*U**T or A = L*D*L**T as computed by SSPTRF, stored as
* a packed triangular matrix in the same storage format as A.
*
* If FACT = 'N', then AFP is an output argument and on exit
* contains the block diagonal matrix D and the multipliers used
* to obtain the factor U or L from the factorization
* A = U*D*U**T or A = L*D*L**T as computed by SSPTRF, stored as
* a packed triangular matrix in the same storage format as A.
*
* IPIV (input or output) INTEGER array, dimension (N)
* If FACT = 'F', then IPIV is an input argument and on entry
* contains details of the interchanges and the block structure
* of D, as determined by SSPTRF.
* If IPIV(k) > 0, then rows and columns k and IPIV(k) were
* interchanged and D(k,k) is a 1-by-1 diagonal block.
* If UPLO = 'U' and IPIV(k) = IPIV(k-1) < 0, then rows and
* columns k-1 and -IPIV(k) were interchanged and D(k-1:k,k-1:k)
* is a 2-by-2 diagonal block. If UPLO = 'L' and IPIV(k) =
* IPIV(k+1) < 0, then rows and columns k+1 and -IPIV(k) were
* interchanged and D(k:k+1,k:k+1) is a 2-by-2 diagonal block.
*
* If FACT = 'N', then IPIV is an output argument and on exit
* contains details of the interchanges and the block structure
* of D, as determined by SSPTRF.
*
* B (input) REAL array, dimension (LDB,NRHS)
* The N-by-NRHS right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) REAL array, dimension (LDX,NRHS)
* If INFO = 0 or INFO = N+1, the N-by-NRHS solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) REAL
* The estimate of the reciprocal condition number of the matrix
* A. If RCOND is less than the machine precision (in
* particular, if RCOND = 0), the matrix is singular to working
* precision. This condition is indicated by a return code of
* INFO > 0.
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= N: D(i,i) is exactly zero. The factorization
* has been completed but the factor D is exactly
* singular, so the solution and error bounds could
* not be computed. RCOND = 0 is returned.
* = N+1: D is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* Further Details
* ===============
*
* The packed storage scheme is illustrated by the following example
* when N = 4, UPLO = 'U':
*
* Two-dimensional storage of the symmetric matrix A:
*
* a11 a12 a13 a14
* a22 a23 a24
* a33 a34 (aij = aji)
* a44
*
* Packed storage of the upper triangle of A:
*
* AP = [ a11, a12, a22, a13, a23, a33, a14, a24, a34, a44 ]
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param uplo
* @param n
* @param nrhs
* @param ap
* @param afp
* @param ipiv
* @param b
* @param ldb
* @param x
* @param ldx
* @param rcond
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void sspsvx(java.lang.String fact, java.lang.String uplo, int n, int nrhs, float[] ap, float[] afp, int[] ipiv, float[] b, int ldb, float[] x, int ldx, org.netlib.util.floatW rcond, float[] ferr, float[] berr, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSPSVX uses the diagonal pivoting factorization A = U*D*U**T or
* A = L*D*L**T to compute the solution to a real system of linear
* equations A * X = B, where A is an N-by-N symmetric matrix stored
* in packed format and X and B are N-by-NRHS matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed:
*
* 1. If FACT = 'N', the diagonal pivoting method is used to factor A as
* A = U * D * U**T, if UPLO = 'U', or
* A = L * D * L**T, if UPLO = 'L',
* where U (or L) is a product of permutation and unit upper (lower)
* triangular matrices and D is symmetric and block diagonal with
* 1-by-1 and 2-by-2 diagonal blocks.
*
* 2. If some D(i,i)=0, so that D is exactly singular, then the routine
* returns with INFO = i. Otherwise, the factored form of A is used
* to estimate the condition number of the matrix A. If the
* reciprocal of the condition number is less than machine precision,
* INFO = N+1 is returned as a warning, but the routine still goes on
* to solve for X and compute error bounds as described below.
*
* 3. The system of equations is solved for X using the factored form
* of A.
*
* 4. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of A has been
* supplied on entry.
* = 'F': On entry, AFP and IPIV contain the factored form of
* A. AP, AFP and IPIV will not be modified.
* = 'N': The matrix A will be copied to AFP and factored.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AP (input) REAL array, dimension (N*(N+1)/2)
* The upper or lower triangle of the symmetric matrix A, packed
* columnwise in a linear array. The j-th column of A is stored
* in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
* See below for further details.
*
* AFP (input or output) REAL array, dimension
* (N*(N+1)/2)
* If FACT = 'F', then AFP is an input argument and on entry
* contains the block diagonal matrix D and the multipliers used
* to obtain the factor U or L from the factorization
* A = U*D*U**T or A = L*D*L**T as computed by SSPTRF, stored as
* a packed triangular matrix in the same storage format as A.
*
* If FACT = 'N', then AFP is an output argument and on exit
* contains the block diagonal matrix D and the multipliers used
* to obtain the factor U or L from the factorization
* A = U*D*U**T or A = L*D*L**T as computed by SSPTRF, stored as
* a packed triangular matrix in the same storage format as A.
*
* IPIV (input or output) INTEGER array, dimension (N)
* If FACT = 'F', then IPIV is an input argument and on entry
* contains details of the interchanges and the block structure
* of D, as determined by SSPTRF.
* If IPIV(k) > 0, then rows and columns k and IPIV(k) were
* interchanged and D(k,k) is a 1-by-1 diagonal block.
* If UPLO = 'U' and IPIV(k) = IPIV(k-1) < 0, then rows and
* columns k-1 and -IPIV(k) were interchanged and D(k-1:k,k-1:k)
* is a 2-by-2 diagonal block. If UPLO = 'L' and IPIV(k) =
* IPIV(k+1) < 0, then rows and columns k+1 and -IPIV(k) were
* interchanged and D(k:k+1,k:k+1) is a 2-by-2 diagonal block.
*
* If FACT = 'N', then IPIV is an output argument and on exit
* contains details of the interchanges and the block structure
* of D, as determined by SSPTRF.
*
* B (input) REAL array, dimension (LDB,NRHS)
* The N-by-NRHS right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) REAL array, dimension (LDX,NRHS)
* If INFO = 0 or INFO = N+1, the N-by-NRHS solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) REAL
* The estimate of the reciprocal condition number of the matrix
* A. If RCOND is less than the machine precision (in
* particular, if RCOND = 0), the matrix is singular to working
* precision. This condition is indicated by a return code of
* INFO > 0.
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= N: D(i,i) is exactly zero. The factorization
* has been completed but the factor D is exactly
* singular, so the solution and error bounds could
* not be computed. RCOND = 0 is returned.
* = N+1: D is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* Further Details
* ===============
*
* The packed storage scheme is illustrated by the following example
* when N = 4, UPLO = 'U':
*
* Two-dimensional storage of the symmetric matrix A:
*
* a11 a12 a13 a14
* a22 a23 a24
* a33 a34 (aij = aji)
* a44
*
* Packed storage of the upper triangle of A:
*
* AP = [ a11, a12, a22, a13, a23, a33, a14, a24, a34, a44 ]
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param uplo
* @param n
* @param nrhs
* @param ap
* @param _ap_offset
* @param afp
* @param _afp_offset
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param rcond
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void sspsvx(java.lang.String fact, java.lang.String uplo, int n, int nrhs, float[] ap, int _ap_offset, float[] afp, int _afp_offset, int[] ipiv, int _ipiv_offset, float[] b, int _b_offset, int ldb, float[] x, int _x_offset, int ldx, org.netlib.util.floatW rcond, float[] ferr, int _ferr_offset, float[] berr, int _berr_offset, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSPTRD reduces a real symmetric matrix A stored in packed form to
* symmetric tridiagonal form T by an orthogonal similarity
* transformation: Q**T * A * Q = T.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
* On exit, if UPLO = 'U', the diagonal and first superdiagonal
* of A are overwritten by the corresponding elements of the
* tridiagonal matrix T, and the elements above the first
* superdiagonal, with the array TAU, represent the orthogonal
* matrix Q as a product of elementary reflectors; if UPLO
* = 'L', the diagonal and first subdiagonal of A are over-
* written by the corresponding elements of the tridiagonal
* matrix T, and the elements below the first subdiagonal, with
* the array TAU, represent the orthogonal matrix Q as a product
* of elementary reflectors. See Further Details.
*
* D (output) REAL array, dimension (N)
* The diagonal elements of the tridiagonal matrix T:
* D(i) = A(i,i).
*
* E (output) REAL array, dimension (N-1)
* The off-diagonal elements of the tridiagonal matrix T:
* E(i) = A(i,i+1) if UPLO = 'U', E(i) = A(i+1,i) if UPLO = 'L'.
*
* TAU (output) REAL array, dimension (N-1)
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* If UPLO = 'U', the matrix Q is represented as a product of elementary
* reflectors
*
* Q = H(n-1) . . . H(2) H(1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(i+1:n) = 0 and v(i) = 1; v(1:i-1) is stored on exit in AP,
* overwriting A(1:i-1,i+1), and tau is stored in TAU(i).
*
* If UPLO = 'L', the matrix Q is represented as a product of elementary
* reflectors
*
* Q = H(1) H(2) . . . H(n-1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i) = 0 and v(i+1) = 1; v(i+2:n) is stored on exit in AP,
* overwriting A(i+2:n,i), and tau is stored in TAU(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param d
* @param e
* @param tau
* @param info
*
*/
abstract public void ssptrd(java.lang.String uplo, int n, float[] ap, float[] d, float[] e, float[] tau, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSPTRD reduces a real symmetric matrix A stored in packed form to
* symmetric tridiagonal form T by an orthogonal similarity
* transformation: Q**T * A * Q = T.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
* On exit, if UPLO = 'U', the diagonal and first superdiagonal
* of A are overwritten by the corresponding elements of the
* tridiagonal matrix T, and the elements above the first
* superdiagonal, with the array TAU, represent the orthogonal
* matrix Q as a product of elementary reflectors; if UPLO
* = 'L', the diagonal and first subdiagonal of A are over-
* written by the corresponding elements of the tridiagonal
* matrix T, and the elements below the first subdiagonal, with
* the array TAU, represent the orthogonal matrix Q as a product
* of elementary reflectors. See Further Details.
*
* D (output) REAL array, dimension (N)
* The diagonal elements of the tridiagonal matrix T:
* D(i) = A(i,i).
*
* E (output) REAL array, dimension (N-1)
* The off-diagonal elements of the tridiagonal matrix T:
* E(i) = A(i,i+1) if UPLO = 'U', E(i) = A(i+1,i) if UPLO = 'L'.
*
* TAU (output) REAL array, dimension (N-1)
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* If UPLO = 'U', the matrix Q is represented as a product of elementary
* reflectors
*
* Q = H(n-1) . . . H(2) H(1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(i+1:n) = 0 and v(i) = 1; v(1:i-1) is stored on exit in AP,
* overwriting A(1:i-1,i+1), and tau is stored in TAU(i).
*
* If UPLO = 'L', the matrix Q is represented as a product of elementary
* reflectors
*
* Q = H(1) H(2) . . . H(n-1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i) = 0 and v(i+1) = 1; v(i+2:n) is stored on exit in AP,
* overwriting A(i+2:n,i), and tau is stored in TAU(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param tau
* @param _tau_offset
* @param info
*
*/
abstract public void ssptrd(java.lang.String uplo, int n, float[] ap, int _ap_offset, float[] d, int _d_offset, float[] e, int _e_offset, float[] tau, int _tau_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSPTRF computes the factorization of a real symmetric matrix A stored
* in packed format using the Bunch-Kaufman diagonal pivoting method:
*
* A = U*D*U**T or A = L*D*L**T
*
* where U (or L) is a product of permutation and unit upper (lower)
* triangular matrices, and D is symmetric and block diagonal with
* 1-by-1 and 2-by-2 diagonal blocks.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, the block diagonal matrix D and the multipliers used
* to obtain the factor U or L, stored as a packed triangular
* matrix overwriting A (see below for further details).
*
* IPIV (output) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D.
* If IPIV(k) > 0, then rows and columns k and IPIV(k) were
* interchanged and D(k,k) is a 1-by-1 diagonal block.
* If UPLO = 'U' and IPIV(k) = IPIV(k-1) < 0, then rows and
* columns k-1 and -IPIV(k) were interchanged and D(k-1:k,k-1:k)
* is a 2-by-2 diagonal block. If UPLO = 'L' and IPIV(k) =
* IPIV(k+1) < 0, then rows and columns k+1 and -IPIV(k) were
* interchanged and D(k:k+1,k:k+1) is a 2-by-2 diagonal block.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, D(i,i) is exactly zero. The factorization
* has been completed, but the block diagonal matrix D is
* exactly singular, and division by zero will occur if it
* is used to solve a system of equations.
*
* Further Details
* ===============
*
* 5-96 - Based on modifications by J. Lewis, Boeing Computer Services
* Company
*
* If UPLO = 'U', then A = U*D*U', where
* U = P(n)*U(n)* ... *P(k)U(k)* ...,
* i.e., U is a product of terms P(k)*U(k), where k decreases from n to
* 1 in steps of 1 or 2, and D is a block diagonal matrix with 1-by-1
* and 2-by-2 diagonal blocks D(k). P(k) is a permutation matrix as
* defined by IPIV(k), and U(k) is a unit upper triangular matrix, such
* that if the diagonal block D(k) is of order s (s = 1 or 2), then
*
* ( I v 0 ) k-s
* U(k) = ( 0 I 0 ) s
* ( 0 0 I ) n-k
* k-s s n-k
*
* If s = 1, D(k) overwrites A(k,k), and v overwrites A(1:k-1,k).
* If s = 2, the upper triangle of D(k) overwrites A(k-1,k-1), A(k-1,k),
* and A(k,k), and v overwrites A(1:k-2,k-1:k).
*
* If UPLO = 'L', then A = L*D*L', where
* L = P(1)*L(1)* ... *P(k)*L(k)* ...,
* i.e., L is a product of terms P(k)*L(k), where k increases from 1 to
* n in steps of 1 or 2, and D is a block diagonal matrix with 1-by-1
* and 2-by-2 diagonal blocks D(k). P(k) is a permutation matrix as
* defined by IPIV(k), and L(k) is a unit lower triangular matrix, such
* that if the diagonal block D(k) is of order s (s = 1 or 2), then
*
* ( I 0 0 ) k-1
* L(k) = ( 0 I 0 ) s
* ( 0 v I ) n-k-s+1
* k-1 s n-k-s+1
*
* If s = 1, D(k) overwrites A(k,k), and v overwrites A(k+1:n,k).
* If s = 2, the lower triangle of D(k) overwrites A(k,k), A(k+1,k),
* and A(k+1,k+1), and v overwrites A(k+2:n,k:k+1).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param ipiv
* @param info
*
*/
abstract public void ssptrf(java.lang.String uplo, int n, float[] ap, int[] ipiv, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSPTRF computes the factorization of a real symmetric matrix A stored
* in packed format using the Bunch-Kaufman diagonal pivoting method:
*
* A = U*D*U**T or A = L*D*L**T
*
* where U (or L) is a product of permutation and unit upper (lower)
* triangular matrices, and D is symmetric and block diagonal with
* 1-by-1 and 2-by-2 diagonal blocks.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangle of the symmetric matrix
* A, packed columnwise in a linear array. The j-th column of A
* is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
*
* On exit, the block diagonal matrix D and the multipliers used
* to obtain the factor U or L, stored as a packed triangular
* matrix overwriting A (see below for further details).
*
* IPIV (output) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D.
* If IPIV(k) > 0, then rows and columns k and IPIV(k) were
* interchanged and D(k,k) is a 1-by-1 diagonal block.
* If UPLO = 'U' and IPIV(k) = IPIV(k-1) < 0, then rows and
* columns k-1 and -IPIV(k) were interchanged and D(k-1:k,k-1:k)
* is a 2-by-2 diagonal block. If UPLO = 'L' and IPIV(k) =
* IPIV(k+1) < 0, then rows and columns k+1 and -IPIV(k) were
* interchanged and D(k:k+1,k:k+1) is a 2-by-2 diagonal block.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, D(i,i) is exactly zero. The factorization
* has been completed, but the block diagonal matrix D is
* exactly singular, and division by zero will occur if it
* is used to solve a system of equations.
*
* Further Details
* ===============
*
* 5-96 - Based on modifications by J. Lewis, Boeing Computer Services
* Company
*
* If UPLO = 'U', then A = U*D*U', where
* U = P(n)*U(n)* ... *P(k)U(k)* ...,
* i.e., U is a product of terms P(k)*U(k), where k decreases from n to
* 1 in steps of 1 or 2, and D is a block diagonal matrix with 1-by-1
* and 2-by-2 diagonal blocks D(k). P(k) is a permutation matrix as
* defined by IPIV(k), and U(k) is a unit upper triangular matrix, such
* that if the diagonal block D(k) is of order s (s = 1 or 2), then
*
* ( I v 0 ) k-s
* U(k) = ( 0 I 0 ) s
* ( 0 0 I ) n-k
* k-s s n-k
*
* If s = 1, D(k) overwrites A(k,k), and v overwrites A(1:k-1,k).
* If s = 2, the upper triangle of D(k) overwrites A(k-1,k-1), A(k-1,k),
* and A(k,k), and v overwrites A(1:k-2,k-1:k).
*
* If UPLO = 'L', then A = L*D*L', where
* L = P(1)*L(1)* ... *P(k)*L(k)* ...,
* i.e., L is a product of terms P(k)*L(k), where k increases from 1 to
* n in steps of 1 or 2, and D is a block diagonal matrix with 1-by-1
* and 2-by-2 diagonal blocks D(k). P(k) is a permutation matrix as
* defined by IPIV(k), and L(k) is a unit lower triangular matrix, such
* that if the diagonal block D(k) is of order s (s = 1 or 2), then
*
* ( I 0 0 ) k-1
* L(k) = ( 0 I 0 ) s
* ( 0 v I ) n-k-s+1
* k-1 s n-k-s+1
*
* If s = 1, D(k) overwrites A(k,k), and v overwrites A(k+1:n,k).
* If s = 2, the lower triangle of D(k) overwrites A(k,k), A(k+1,k),
* and A(k+1,k+1), and v overwrites A(k+2:n,k:k+1).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param ipiv
* @param _ipiv_offset
* @param info
*
*/
abstract public void ssptrf(java.lang.String uplo, int n, float[] ap, int _ap_offset, int[] ipiv, int _ipiv_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSPTRI computes the inverse of a real symmetric indefinite matrix
* A in packed storage using the factorization A = U*D*U**T or
* A = L*D*L**T computed by SSPTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the details of the factorization are stored
* as an upper or lower triangular matrix.
* = 'U': Upper triangular, form is A = U*D*U**T;
* = 'L': Lower triangular, form is A = L*D*L**T.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the block diagonal matrix D and the multipliers
* used to obtain the factor U or L as computed by SSPTRF,
* stored as a packed triangular matrix.
*
* On exit, if INFO = 0, the (symmetric) inverse of the original
* matrix, stored as a packed triangular matrix. The j-th column
* of inv(A) is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = inv(A)(i,j) for 1<=i<=j;
* if UPLO = 'L',
* AP(i + (j-1)*(2n-j)/2) = inv(A)(i,j) for j<=i<=n.
*
* IPIV (input) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D
* as determined by SSPTRF.
*
* WORK (workspace) REAL array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, D(i,i) = 0; the matrix is singular and its
* inverse could not be computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param ipiv
* @param work
* @param info
*
*/
abstract public void ssptri(java.lang.String uplo, int n, float[] ap, int[] ipiv, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSPTRI computes the inverse of a real symmetric indefinite matrix
* A in packed storage using the factorization A = U*D*U**T or
* A = L*D*L**T computed by SSPTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the details of the factorization are stored
* as an upper or lower triangular matrix.
* = 'U': Upper triangular, form is A = U*D*U**T;
* = 'L': Lower triangular, form is A = L*D*L**T.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the block diagonal matrix D and the multipliers
* used to obtain the factor U or L as computed by SSPTRF,
* stored as a packed triangular matrix.
*
* On exit, if INFO = 0, the (symmetric) inverse of the original
* matrix, stored as a packed triangular matrix. The j-th column
* of inv(A) is stored in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = inv(A)(i,j) for 1<=i<=j;
* if UPLO = 'L',
* AP(i + (j-1)*(2n-j)/2) = inv(A)(i,j) for j<=i<=n.
*
* IPIV (input) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D
* as determined by SSPTRF.
*
* WORK (workspace) REAL array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, D(i,i) = 0; the matrix is singular and its
* inverse could not be computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param ap
* @param _ap_offset
* @param ipiv
* @param _ipiv_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void ssptri(java.lang.String uplo, int n, float[] ap, int _ap_offset, int[] ipiv, int _ipiv_offset, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSPTRS solves a system of linear equations A*X = B with a real
* symmetric matrix A stored in packed format using the factorization
* A = U*D*U**T or A = L*D*L**T computed by SSPTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the details of the factorization are stored
* as an upper or lower triangular matrix.
* = 'U': Upper triangular, form is A = U*D*U**T;
* = 'L': Lower triangular, form is A = L*D*L**T.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AP (input) REAL array, dimension (N*(N+1)/2)
* The block diagonal matrix D and the multipliers used to
* obtain the factor U or L as computed by SSPTRF, stored as a
* packed triangular matrix.
*
* IPIV (input) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D
* as determined by SSPTRF.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param ap
* @param ipiv
* @param b
* @param ldb
* @param info
*
*/
abstract public void ssptrs(java.lang.String uplo, int n, int nrhs, float[] ap, int[] ipiv, float[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSPTRS solves a system of linear equations A*X = B with a real
* symmetric matrix A stored in packed format using the factorization
* A = U*D*U**T or A = L*D*L**T computed by SSPTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the details of the factorization are stored
* as an upper or lower triangular matrix.
* = 'U': Upper triangular, form is A = U*D*U**T;
* = 'L': Lower triangular, form is A = L*D*L**T.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AP (input) REAL array, dimension (N*(N+1)/2)
* The block diagonal matrix D and the multipliers used to
* obtain the factor U or L as computed by SSPTRF, stored as a
* packed triangular matrix.
*
* IPIV (input) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D
* as determined by SSPTRF.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param ap
* @param _ap_offset
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void ssptrs(java.lang.String uplo, int n, int nrhs, float[] ap, int _ap_offset, int[] ipiv, int _ipiv_offset, float[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSTEBZ computes the eigenvalues of a symmetric tridiagonal
* matrix T. The user may ask for all eigenvalues, all eigenvalues
* in the half-open interval (VL, VU], or the IL-th through IU-th
* eigenvalues.
*
* To avoid overflow, the matrix must be scaled so that its
* largest element is no greater than overflow**(1/2) *
* underflow**(1/4) in absolute value, and for greatest
* accuracy, it should not be much smaller than that.
*
* See W. Kahan "Accurate Eigenvalues of a Symmetric Tridiagonal
* Matrix", Report CS41, Computer Science Dept., Stanford
* University, July 21, 1966.
*
* Arguments
* =========
*
* RANGE (input) CHARACTER*1
* = 'A': ("All") all eigenvalues will be found.
* = 'V': ("Value") all eigenvalues in the half-open interval
* (VL, VU] will be found.
* = 'I': ("Index") the IL-th through IU-th eigenvalues (of the
* entire matrix) will be found.
*
* ORDER (input) CHARACTER*1
* = 'B': ("By Block") the eigenvalues will be grouped by
* split-off block (see IBLOCK, ISPLIT) and
* ordered from smallest to largest within
* the block.
* = 'E': ("Entire matrix")
* the eigenvalues for the entire matrix
* will be ordered from smallest to
* largest.
*
* N (input) INTEGER
* The order of the tridiagonal matrix T. N >= 0.
*
* VL (input) REAL
* VU (input) REAL
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. Eigenvalues less than or equal
* to VL, or greater than VU, will not be returned. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) REAL
* The absolute tolerance for the eigenvalues. An eigenvalue
* (or cluster) is considered to be located if it has been
* determined to lie in an interval whose width is ABSTOL or
* less. If ABSTOL is less than or equal to zero, then ULP*|T|
* will be used, where |T| means the 1-norm of T.
*
* Eigenvalues will be computed most accurately when ABSTOL is
* set to twice the underflow threshold 2*SLAMCH('S'), not zero.
*
* D (input) REAL array, dimension (N)
* The n diagonal elements of the tridiagonal matrix T.
*
* E (input) REAL array, dimension (N-1)
* The (n-1) off-diagonal elements of the tridiagonal matrix T.
*
* M (output) INTEGER
* The actual number of eigenvalues found. 0 <= M <= N.
* (See also the description of INFO=2,3.)
*
* NSPLIT (output) INTEGER
* The number of diagonal blocks in the matrix T.
* 1 <= NSPLIT <= N.
*
* W (output) REAL array, dimension (N)
* On exit, the first M elements of W will contain the
* eigenvalues. (SSTEBZ may use the remaining N-M elements as
* workspace.)
*
* IBLOCK (output) INTEGER array, dimension (N)
* At each row/column j where E(j) is zero or small, the
* matrix T is considered to split into a block diagonal
* matrix. On exit, if INFO = 0, IBLOCK(i) specifies to which
* block (from 1 to the number of blocks) the eigenvalue W(i)
* belongs. (SSTEBZ may use the remaining N-M elements as
* workspace.)
*
* ISPLIT (output) INTEGER array, dimension (N)
* The splitting points, at which T breaks up into submatrices.
* The first submatrix consists of rows/columns 1 to ISPLIT(1),
* the second of rows/columns ISPLIT(1)+1 through ISPLIT(2),
* etc., and the NSPLIT-th consists of rows/columns
* ISPLIT(NSPLIT-1)+1 through ISPLIT(NSPLIT)=N.
* (Only the first NSPLIT elements will actually be used, but
* since the user cannot know a priori what value NSPLIT will
* have, N words must be reserved for ISPLIT.)
*
* WORK (workspace) REAL array, dimension (4*N)
*
* IWORK (workspace) INTEGER array, dimension (3*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: some or all of the eigenvalues failed to converge or
* were not computed:
* =1 or 3: Bisection failed to converge for some
* eigenvalues; these eigenvalues are flagged by a
* negative block number. The effect is that the
* eigenvalues may not be as accurate as the
* absolute and relative tolerances. This is
* generally caused by unexpectedly inaccurate
* arithmetic.
* =2 or 3: RANGE='I' only: Not all of the eigenvalues
* IL:IU were found.
* Effect: M < IU+1-IL
* Cause: non-monotonic arithmetic, causing the
* Sturm sequence to be non-monotonic.
* Cure: recalculate, using RANGE='A', and pick
* out eigenvalues IL:IU. In some cases,
* increasing the PARAMETER "FUDGE" may
* make things work.
* = 4: RANGE='I', and the Gershgorin interval
* initially used was too small. No eigenvalues
* were computed.
* Probable cause: your machine has sloppy
* floating-point arithmetic.
* Cure: Increase the PARAMETER "FUDGE",
* recompile, and try again.
*
* Internal Parameters
* ===================
*
* RELFAC REAL, default = 2.0e0
* The relative tolerance. An interval (a,b] lies within
* "relative tolerance" if b-a < RELFAC*ulp*max(|a|,|b|),
* where "ulp" is the machine precision (distance from 1 to
* the next larger floating point number.)
*
* FUDGE REAL, default = 2
* A "fudge factor" to widen the Gershgorin intervals. Ideally,
* a value of 1 should work, but on machines with sloppy
* arithmetic, this needs to be larger. The default for
* publicly released versions should be large enough to handle
* the worst machine around. Note that this has no effect
* on accuracy of the solution.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param range
* @param order
* @param n
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param d
* @param e
* @param m
* @param nsplit
* @param w
* @param iblock
* @param isplit
* @param work
* @param iwork
* @param info
*
*/
abstract public void sstebz(java.lang.String range, java.lang.String order, int n, float vl, float vu, int il, int iu, float abstol, float[] d, float[] e, org.netlib.util.intW m, org.netlib.util.intW nsplit, float[] w, int[] iblock, int[] isplit, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSTEBZ computes the eigenvalues of a symmetric tridiagonal
* matrix T. The user may ask for all eigenvalues, all eigenvalues
* in the half-open interval (VL, VU], or the IL-th through IU-th
* eigenvalues.
*
* To avoid overflow, the matrix must be scaled so that its
* largest element is no greater than overflow**(1/2) *
* underflow**(1/4) in absolute value, and for greatest
* accuracy, it should not be much smaller than that.
*
* See W. Kahan "Accurate Eigenvalues of a Symmetric Tridiagonal
* Matrix", Report CS41, Computer Science Dept., Stanford
* University, July 21, 1966.
*
* Arguments
* =========
*
* RANGE (input) CHARACTER*1
* = 'A': ("All") all eigenvalues will be found.
* = 'V': ("Value") all eigenvalues in the half-open interval
* (VL, VU] will be found.
* = 'I': ("Index") the IL-th through IU-th eigenvalues (of the
* entire matrix) will be found.
*
* ORDER (input) CHARACTER*1
* = 'B': ("By Block") the eigenvalues will be grouped by
* split-off block (see IBLOCK, ISPLIT) and
* ordered from smallest to largest within
* the block.
* = 'E': ("Entire matrix")
* the eigenvalues for the entire matrix
* will be ordered from smallest to
* largest.
*
* N (input) INTEGER
* The order of the tridiagonal matrix T. N >= 0.
*
* VL (input) REAL
* VU (input) REAL
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. Eigenvalues less than or equal
* to VL, or greater than VU, will not be returned. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) REAL
* The absolute tolerance for the eigenvalues. An eigenvalue
* (or cluster) is considered to be located if it has been
* determined to lie in an interval whose width is ABSTOL or
* less. If ABSTOL is less than or equal to zero, then ULP*|T|
* will be used, where |T| means the 1-norm of T.
*
* Eigenvalues will be computed most accurately when ABSTOL is
* set to twice the underflow threshold 2*SLAMCH('S'), not zero.
*
* D (input) REAL array, dimension (N)
* The n diagonal elements of the tridiagonal matrix T.
*
* E (input) REAL array, dimension (N-1)
* The (n-1) off-diagonal elements of the tridiagonal matrix T.
*
* M (output) INTEGER
* The actual number of eigenvalues found. 0 <= M <= N.
* (See also the description of INFO=2,3.)
*
* NSPLIT (output) INTEGER
* The number of diagonal blocks in the matrix T.
* 1 <= NSPLIT <= N.
*
* W (output) REAL array, dimension (N)
* On exit, the first M elements of W will contain the
* eigenvalues. (SSTEBZ may use the remaining N-M elements as
* workspace.)
*
* IBLOCK (output) INTEGER array, dimension (N)
* At each row/column j where E(j) is zero or small, the
* matrix T is considered to split into a block diagonal
* matrix. On exit, if INFO = 0, IBLOCK(i) specifies to which
* block (from 1 to the number of blocks) the eigenvalue W(i)
* belongs. (SSTEBZ may use the remaining N-M elements as
* workspace.)
*
* ISPLIT (output) INTEGER array, dimension (N)
* The splitting points, at which T breaks up into submatrices.
* The first submatrix consists of rows/columns 1 to ISPLIT(1),
* the second of rows/columns ISPLIT(1)+1 through ISPLIT(2),
* etc., and the NSPLIT-th consists of rows/columns
* ISPLIT(NSPLIT-1)+1 through ISPLIT(NSPLIT)=N.
* (Only the first NSPLIT elements will actually be used, but
* since the user cannot know a priori what value NSPLIT will
* have, N words must be reserved for ISPLIT.)
*
* WORK (workspace) REAL array, dimension (4*N)
*
* IWORK (workspace) INTEGER array, dimension (3*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: some or all of the eigenvalues failed to converge or
* were not computed:
* =1 or 3: Bisection failed to converge for some
* eigenvalues; these eigenvalues are flagged by a
* negative block number. The effect is that the
* eigenvalues may not be as accurate as the
* absolute and relative tolerances. This is
* generally caused by unexpectedly inaccurate
* arithmetic.
* =2 or 3: RANGE='I' only: Not all of the eigenvalues
* IL:IU were found.
* Effect: M < IU+1-IL
* Cause: non-monotonic arithmetic, causing the
* Sturm sequence to be non-monotonic.
* Cure: recalculate, using RANGE='A', and pick
* out eigenvalues IL:IU. In some cases,
* increasing the PARAMETER "FUDGE" may
* make things work.
* = 4: RANGE='I', and the Gershgorin interval
* initially used was too small. No eigenvalues
* were computed.
* Probable cause: your machine has sloppy
* floating-point arithmetic.
* Cure: Increase the PARAMETER "FUDGE",
* recompile, and try again.
*
* Internal Parameters
* ===================
*
* RELFAC REAL, default = 2.0e0
* The relative tolerance. An interval (a,b] lies within
* "relative tolerance" if b-a < RELFAC*ulp*max(|a|,|b|),
* where "ulp" is the machine precision (distance from 1 to
* the next larger floating point number.)
*
* FUDGE REAL, default = 2
* A "fudge factor" to widen the Gershgorin intervals. Ideally,
* a value of 1 should work, but on machines with sloppy
* arithmetic, this needs to be larger. The default for
* publicly released versions should be large enough to handle
* the worst machine around. Note that this has no effect
* on accuracy of the solution.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param range
* @param order
* @param n
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param m
* @param nsplit
* @param w
* @param _w_offset
* @param iblock
* @param _iblock_offset
* @param isplit
* @param _isplit_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void sstebz(java.lang.String range, java.lang.String order, int n, float vl, float vu, int il, int iu, float abstol, float[] d, int _d_offset, float[] e, int _e_offset, org.netlib.util.intW m, org.netlib.util.intW nsplit, float[] w, int _w_offset, int[] iblock, int _iblock_offset, int[] isplit, int _isplit_offset, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSTEDC computes all eigenvalues and, optionally, eigenvectors of a
* symmetric tridiagonal matrix using the divide and conquer method.
* The eigenvectors of a full or band real symmetric matrix can also be
* found if SSYTRD or SSPTRD or SSBTRD has been used to reduce this
* matrix to tridiagonal form.
*
* This code makes very mild assumptions about floating point
* arithmetic. It will work on machines with a guard digit in
* add/subtract, or on those binary machines without guard digits
* which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or Cray-2.
* It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none. See SLAED3 for details.
*
* Arguments
* =========
*
* COMPZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only.
* = 'I': Compute eigenvectors of tridiagonal matrix also.
* = 'V': Compute eigenvectors of original dense symmetric
* matrix also. On entry, Z contains the orthogonal
* matrix used to reduce the original matrix to
* tridiagonal form.
*
* N (input) INTEGER
* The dimension of the symmetric tridiagonal matrix. N >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, the diagonal elements of the tridiagonal matrix.
* On exit, if INFO = 0, the eigenvalues in ascending order.
*
* E (input/output) REAL array, dimension (N-1)
* On entry, the subdiagonal elements of the tridiagonal matrix.
* On exit, E has been destroyed.
*
* Z (input/output) REAL array, dimension (LDZ,N)
* On entry, if COMPZ = 'V', then Z contains the orthogonal
* matrix used in the reduction to tridiagonal form.
* On exit, if INFO = 0, then if COMPZ = 'V', Z contains the
* orthonormal eigenvectors of the original symmetric matrix,
* and if COMPZ = 'I', Z contains the orthonormal eigenvectors
* of the symmetric tridiagonal matrix.
* If COMPZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1.
* If eigenvectors are desired, then LDZ >= max(1,N).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If COMPZ = 'N' or N <= 1 then LWORK must be at least 1.
* If COMPZ = 'V' and N > 1 then LWORK must be at least
* ( 1 + 3*N + 2*N*lg N + 3*N**2 ),
* where lg( N ) = smallest integer k such
* that 2**k >= N.
* If COMPZ = 'I' and N > 1 then LWORK must be at least
* ( 1 + 4*N + N**2 ).
* Note that for COMPZ = 'I' or 'V', then if N is less than or
* equal to the minimum divide size, usually 25, then LWORK need
* only be max(1,2*(N-1)).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If COMPZ = 'N' or N <= 1 then LIWORK must be at least 1.
* If COMPZ = 'V' and N > 1 then LIWORK must be at least
* ( 6 + 6*N + 5*N*lg N ).
* If COMPZ = 'I' and N > 1 then LIWORK must be at least
* ( 3 + 5*N ).
* Note that for COMPZ = 'I' or 'V', then if N is less than or
* equal to the minimum divide size, usually 25, then LIWORK
* need only be 1.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal size of the IWORK array,
* returns this value as the first entry of the IWORK array, and
* no error message related to LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: The algorithm failed to compute an eigenvalue while
* working on the submatrix lying in rows and columns
* INFO/(N+1) through mod(INFO,N+1).
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
* Modified by Francoise Tisseur, University of Tennessee.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param compz
* @param n
* @param d
* @param e
* @param z
* @param ldz
* @param work
* @param lwork
* @param iwork
* @param liwork
* @param info
*
*/
abstract public void sstedc(java.lang.String compz, int n, float[] d, float[] e, float[] z, int ldz, float[] work, int lwork, int[] iwork, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSTEDC computes all eigenvalues and, optionally, eigenvectors of a
* symmetric tridiagonal matrix using the divide and conquer method.
* The eigenvectors of a full or band real symmetric matrix can also be
* found if SSYTRD or SSPTRD or SSBTRD has been used to reduce this
* matrix to tridiagonal form.
*
* This code makes very mild assumptions about floating point
* arithmetic. It will work on machines with a guard digit in
* add/subtract, or on those binary machines without guard digits
* which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or Cray-2.
* It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none. See SLAED3 for details.
*
* Arguments
* =========
*
* COMPZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only.
* = 'I': Compute eigenvectors of tridiagonal matrix also.
* = 'V': Compute eigenvectors of original dense symmetric
* matrix also. On entry, Z contains the orthogonal
* matrix used to reduce the original matrix to
* tridiagonal form.
*
* N (input) INTEGER
* The dimension of the symmetric tridiagonal matrix. N >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, the diagonal elements of the tridiagonal matrix.
* On exit, if INFO = 0, the eigenvalues in ascending order.
*
* E (input/output) REAL array, dimension (N-1)
* On entry, the subdiagonal elements of the tridiagonal matrix.
* On exit, E has been destroyed.
*
* Z (input/output) REAL array, dimension (LDZ,N)
* On entry, if COMPZ = 'V', then Z contains the orthogonal
* matrix used in the reduction to tridiagonal form.
* On exit, if INFO = 0, then if COMPZ = 'V', Z contains the
* orthonormal eigenvectors of the original symmetric matrix,
* and if COMPZ = 'I', Z contains the orthonormal eigenvectors
* of the symmetric tridiagonal matrix.
* If COMPZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1.
* If eigenvectors are desired, then LDZ >= max(1,N).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If COMPZ = 'N' or N <= 1 then LWORK must be at least 1.
* If COMPZ = 'V' and N > 1 then LWORK must be at least
* ( 1 + 3*N + 2*N*lg N + 3*N**2 ),
* where lg( N ) = smallest integer k such
* that 2**k >= N.
* If COMPZ = 'I' and N > 1 then LWORK must be at least
* ( 1 + 4*N + N**2 ).
* Note that for COMPZ = 'I' or 'V', then if N is less than or
* equal to the minimum divide size, usually 25, then LWORK need
* only be max(1,2*(N-1)).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If COMPZ = 'N' or N <= 1 then LIWORK must be at least 1.
* If COMPZ = 'V' and N > 1 then LIWORK must be at least
* ( 6 + 6*N + 5*N*lg N ).
* If COMPZ = 'I' and N > 1 then LIWORK must be at least
* ( 3 + 5*N ).
* Note that for COMPZ = 'I' or 'V', then if N is less than or
* equal to the minimum divide size, usually 25, then LIWORK
* need only be 1.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal size of the IWORK array,
* returns this value as the first entry of the IWORK array, and
* no error message related to LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: The algorithm failed to compute an eigenvalue while
* working on the submatrix lying in rows and columns
* INFO/(N+1) through mod(INFO,N+1).
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
* Modified by Francoise Tisseur, University of Tennessee.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param compz
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param liwork
* @param info
*
*/
abstract public void sstedc(java.lang.String compz, int n, float[] d, int _d_offset, float[] e, int _e_offset, float[] z, int _z_offset, int ldz, float[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSTEGR computes selected eigenvalues and, optionally, eigenvectors
* of a real symmetric tridiagonal matrix T. Any such unreduced matrix h
* a well defined set of pairwise different real eigenvalues, the corres
* real eigenvectors are pairwise orthogonal.
*
* The spectrum may be computed either completely or partially by specif
* either an interval (VL,VU] or a range of indices IL:IU for the desire
* eigenvalues.
*
* SSTEGR is a compatability wrapper around the improved SSTEMR routine.
* See SSTEMR for further details.
*
* One important change is that the ABSTOL parameter no longer provides
* benefit and hence is no longer used.
*
* Note : SSTEGR and SSTEMR work only on machines which follow
* IEEE-754 floating-point standard in their handling of infinities and
* NaNs. Normal execution may create these exceptiona values and hence
* may abort due to a floating point exception in environments which
* do not conform to the IEEE-754 standard.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, the N diagonal elements of the tridiagonal matrix
* T. On exit, D is overwritten.
*
* E (input/output) REAL array, dimension (N)
* On entry, the (N-1) subdiagonal elements of the tridiagonal
* matrix T in elements 1 to N-1 of E. E(N) need not be set on
* input, but is used internally as workspace.
* On exit, E is overwritten.
*
* VL (input) REAL
* VU (input) REAL
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) REAL
* Unused. Was the absolute error tolerance for the
* eigenvalues/eigenvectors in previous versions.
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) REAL array, dimension (N)
* The first M elements contain the selected eigenvalues in
* ascending order.
*
* Z (output) REAL array, dimension (LDZ, max(1,M) )
* If JOBZ = 'V', and if INFO = 0, then the first M columns of Z
* contain the orthonormal eigenvectors of the matrix T
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* If JOBZ = 'N', then Z is not referenced.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
* Supplying N columns is always safe.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', then LDZ >= max(1,N).
*
* ISUPPZ (output) INTEGER ARRAY, dimension ( 2*max(1,M) )
* The support of the eigenvectors in Z, i.e., the indices
* indicating the nonzero elements in Z. The i-th computed eigen
* is nonzero only in elements ISUPPZ( 2*i-1 ) through
* ISUPPZ( 2*i ). This is relevant in the case when the matrix
* is split. ISUPPZ is only accessed when JOBZ is 'V' and N > 0.
*
* WORK (workspace/output) REAL array, dimension (LWORK)
* On exit, if INFO = 0, WORK(1) returns the optimal
* (and minimal) LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,18*N)
* if JOBZ = 'V', and LWORK >= max(1,12*N) if JOBZ = 'N'.
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (LIWORK)
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK. LIWORK >= max(1,10*N)
* if the eigenvectors are desired, and LIWORK >= max(1,8*N)
* if only the eigenvalues are to be computed.
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal size of the IWORK array,
* returns this value as the first entry of the IWORK array, and
* no error message related to LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* On exit, INFO
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = 1X, internal error in SLARRE,
* if INFO = 2X, internal error in SLARRV.
* Here, the digit X = ABS( IINFO ) < 10, where IINFO is
* the nonzero error code returned by SLARRE or
* SLARRV, respectively.
*
* Further Details
* ===============
*
* Based on contributions by
* Inderjit Dhillon, IBM Almaden, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, LBNL/NERSC, USA
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param jobz
* @param range
* @param n
* @param d
* @param e
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param z
* @param ldz
* @param isuppz
* @param work
* @param lwork
* @param iwork
* @param liwork
* @param info
*
*/
abstract public void sstegr(java.lang.String jobz, java.lang.String range, int n, float[] d, float[] e, float vl, float vu, int il, int iu, float abstol, org.netlib.util.intW m, float[] w, float[] z, int ldz, int[] isuppz, float[] work, int lwork, int[] iwork, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSTEGR computes selected eigenvalues and, optionally, eigenvectors
* of a real symmetric tridiagonal matrix T. Any such unreduced matrix h
* a well defined set of pairwise different real eigenvalues, the corres
* real eigenvectors are pairwise orthogonal.
*
* The spectrum may be computed either completely or partially by specif
* either an interval (VL,VU] or a range of indices IL:IU for the desire
* eigenvalues.
*
* SSTEGR is a compatability wrapper around the improved SSTEMR routine.
* See SSTEMR for further details.
*
* One important change is that the ABSTOL parameter no longer provides
* benefit and hence is no longer used.
*
* Note : SSTEGR and SSTEMR work only on machines which follow
* IEEE-754 floating-point standard in their handling of infinities and
* NaNs. Normal execution may create these exceptiona values and hence
* may abort due to a floating point exception in environments which
* do not conform to the IEEE-754 standard.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, the N diagonal elements of the tridiagonal matrix
* T. On exit, D is overwritten.
*
* E (input/output) REAL array, dimension (N)
* On entry, the (N-1) subdiagonal elements of the tridiagonal
* matrix T in elements 1 to N-1 of E. E(N) need not be set on
* input, but is used internally as workspace.
* On exit, E is overwritten.
*
* VL (input) REAL
* VU (input) REAL
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) REAL
* Unused. Was the absolute error tolerance for the
* eigenvalues/eigenvectors in previous versions.
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) REAL array, dimension (N)
* The first M elements contain the selected eigenvalues in
* ascending order.
*
* Z (output) REAL array, dimension (LDZ, max(1,M) )
* If JOBZ = 'V', and if INFO = 0, then the first M columns of Z
* contain the orthonormal eigenvectors of the matrix T
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* If JOBZ = 'N', then Z is not referenced.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
* Supplying N columns is always safe.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', then LDZ >= max(1,N).
*
* ISUPPZ (output) INTEGER ARRAY, dimension ( 2*max(1,M) )
* The support of the eigenvectors in Z, i.e., the indices
* indicating the nonzero elements in Z. The i-th computed eigen
* is nonzero only in elements ISUPPZ( 2*i-1 ) through
* ISUPPZ( 2*i ). This is relevant in the case when the matrix
* is split. ISUPPZ is only accessed when JOBZ is 'V' and N > 0.
*
* WORK (workspace/output) REAL array, dimension (LWORK)
* On exit, if INFO = 0, WORK(1) returns the optimal
* (and minimal) LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,18*N)
* if JOBZ = 'V', and LWORK >= max(1,12*N) if JOBZ = 'N'.
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (LIWORK)
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK. LIWORK >= max(1,10*N)
* if the eigenvectors are desired, and LIWORK >= max(1,8*N)
* if only the eigenvalues are to be computed.
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal size of the IWORK array,
* returns this value as the first entry of the IWORK array, and
* no error message related to LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* On exit, INFO
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = 1X, internal error in SLARRE,
* if INFO = 2X, internal error in SLARRV.
* Here, the digit X = ABS( IINFO ) < 10, where IINFO is
* the nonzero error code returned by SLARRE or
* SLARRV, respectively.
*
* Further Details
* ===============
*
* Based on contributions by
* Inderjit Dhillon, IBM Almaden, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, LBNL/NERSC, USA
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param jobz
* @param range
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param isuppz
* @param _isuppz_offset
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param liwork
* @param info
*
*/
abstract public void sstegr(java.lang.String jobz, java.lang.String range, int n, float[] d, int _d_offset, float[] e, int _e_offset, float vl, float vu, int il, int iu, float abstol, org.netlib.util.intW m, float[] w, int _w_offset, float[] z, int _z_offset, int ldz, int[] isuppz, int _isuppz_offset, float[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSTEIN computes the eigenvectors of a real symmetric tridiagonal
* matrix T corresponding to specified eigenvalues, using inverse
* iteration.
*
* The maximum number of iterations allowed for each eigenvector is
* specified by an internal parameter MAXITS (currently set to 5).
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input) REAL array, dimension (N)
* The n diagonal elements of the tridiagonal matrix T.
*
* E (input) REAL array, dimension (N-1)
* The (n-1) subdiagonal elements of the tridiagonal matrix
* T, in elements 1 to N-1.
*
* M (input) INTEGER
* The number of eigenvectors to be found. 0 <= M <= N.
*
* W (input) REAL array, dimension (N)
* The first M elements of W contain the eigenvalues for
* which eigenvectors are to be computed. The eigenvalues
* should be grouped by split-off block and ordered from
* smallest to largest within the block. ( The output array
* W from SSTEBZ with ORDER = 'B' is expected here. )
*
* IBLOCK (input) INTEGER array, dimension (N)
* The submatrix indices associated with the corresponding
* eigenvalues in W; IBLOCK(i)=1 if eigenvalue W(i) belongs to
* the first submatrix from the top, =2 if W(i) belongs to
* the second submatrix, etc. ( The output array IBLOCK
* from SSTEBZ is expected here. )
*
* ISPLIT (input) INTEGER array, dimension (N)
* The splitting points, at which T breaks up into submatrices.
* The first submatrix consists of rows/columns 1 to
* ISPLIT( 1 ), the second of rows/columns ISPLIT( 1 )+1
* through ISPLIT( 2 ), etc.
* ( The output array ISPLIT from SSTEBZ is expected here. )
*
* Z (output) REAL array, dimension (LDZ, M)
* The computed eigenvectors. The eigenvector associated
* with the eigenvalue W(i) is stored in the i-th column of
* Z. Any vector which fails to converge is set to its current
* iterate after MAXITS iterations.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= max(1,N).
*
* WORK (workspace) REAL array, dimension (5*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* IFAIL (output) INTEGER array, dimension (M)
* On normal exit, all elements of IFAIL are zero.
* If one or more eigenvectors fail to converge after
* MAXITS iterations, then their indices are stored in
* array IFAIL.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, then i eigenvectors failed to converge
* in MAXITS iterations. Their indices are stored in
* array IFAIL.
*
* Internal Parameters
* ===================
*
* MAXITS INTEGER, default = 5
* The maximum number of iterations performed.
*
* EXTRA INTEGER, default = 2
* The number of iterations performed after norm growth
* criterion is satisfied, should be at least 1.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param e
* @param m
* @param w
* @param iblock
* @param isplit
* @param z
* @param ldz
* @param work
* @param iwork
* @param ifail
* @param info
*
*/
abstract public void sstein(int n, float[] d, float[] e, int m, float[] w, int[] iblock, int[] isplit, float[] z, int ldz, float[] work, int[] iwork, int[] ifail, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSTEIN computes the eigenvectors of a real symmetric tridiagonal
* matrix T corresponding to specified eigenvalues, using inverse
* iteration.
*
* The maximum number of iterations allowed for each eigenvector is
* specified by an internal parameter MAXITS (currently set to 5).
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input) REAL array, dimension (N)
* The n diagonal elements of the tridiagonal matrix T.
*
* E (input) REAL array, dimension (N-1)
* The (n-1) subdiagonal elements of the tridiagonal matrix
* T, in elements 1 to N-1.
*
* M (input) INTEGER
* The number of eigenvectors to be found. 0 <= M <= N.
*
* W (input) REAL array, dimension (N)
* The first M elements of W contain the eigenvalues for
* which eigenvectors are to be computed. The eigenvalues
* should be grouped by split-off block and ordered from
* smallest to largest within the block. ( The output array
* W from SSTEBZ with ORDER = 'B' is expected here. )
*
* IBLOCK (input) INTEGER array, dimension (N)
* The submatrix indices associated with the corresponding
* eigenvalues in W; IBLOCK(i)=1 if eigenvalue W(i) belongs to
* the first submatrix from the top, =2 if W(i) belongs to
* the second submatrix, etc. ( The output array IBLOCK
* from SSTEBZ is expected here. )
*
* ISPLIT (input) INTEGER array, dimension (N)
* The splitting points, at which T breaks up into submatrices.
* The first submatrix consists of rows/columns 1 to
* ISPLIT( 1 ), the second of rows/columns ISPLIT( 1 )+1
* through ISPLIT( 2 ), etc.
* ( The output array ISPLIT from SSTEBZ is expected here. )
*
* Z (output) REAL array, dimension (LDZ, M)
* The computed eigenvectors. The eigenvector associated
* with the eigenvalue W(i) is stored in the i-th column of
* Z. Any vector which fails to converge is set to its current
* iterate after MAXITS iterations.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= max(1,N).
*
* WORK (workspace) REAL array, dimension (5*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* IFAIL (output) INTEGER array, dimension (M)
* On normal exit, all elements of IFAIL are zero.
* If one or more eigenvectors fail to converge after
* MAXITS iterations, then their indices are stored in
* array IFAIL.
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, then i eigenvectors failed to converge
* in MAXITS iterations. Their indices are stored in
* array IFAIL.
*
* Internal Parameters
* ===================
*
* MAXITS INTEGER, default = 5
* The maximum number of iterations performed.
*
* EXTRA INTEGER, default = 2
* The number of iterations performed after norm growth
* criterion is satisfied, should be at least 1.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param m
* @param w
* @param _w_offset
* @param iblock
* @param _iblock_offset
* @param isplit
* @param _isplit_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param ifail
* @param _ifail_offset
* @param info
*
*/
abstract public void sstein(int n, float[] d, int _d_offset, float[] e, int _e_offset, int m, float[] w, int _w_offset, int[] iblock, int _iblock_offset, int[] isplit, int _isplit_offset, float[] z, int _z_offset, int ldz, float[] work, int _work_offset, int[] iwork, int _iwork_offset, int[] ifail, int _ifail_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSTEMR computes selected eigenvalues and, optionally, eigenvectors
* of a real symmetric tridiagonal matrix T. Any such unreduced matrix h
* a well defined set of pairwise different real eigenvalues, the corres
* real eigenvectors are pairwise orthogonal.
*
* The spectrum may be computed either completely or partially by specif
* either an interval (VL,VU] or a range of indices IL:IU for the desire
* eigenvalues.
*
* Depending on the number of desired eigenvalues, these are computed ei
* by bisection or the dqds algorithm. Numerically orthogonal eigenvecto
* computed by the use of various suitable L D L^T factorizations near c
* of close eigenvalues (referred to as RRRs, Relatively Robust
* Representations). An informal sketch of the algorithm follows.
*
* For each unreduced block (submatrix) of T,
* (a) Compute T - sigma I = L D L^T, so that L and D
* define all the wanted eigenvalues to high relative accuracy.
* This means that small relative changes in the entries of D and
* cause only small relative changes in the eigenvalues and
* eigenvectors. The standard (unfactored) representation of the
* tridiagonal matrix T does not have this property in general.
* (b) Compute the eigenvalues to suitable accuracy.
* If the eigenvectors are desired, the algorithm attains full
* accuracy of the computed eigenvalues only right before
* the corresponding vectors have to be computed, see steps c) an
* (c) For each cluster of close eigenvalues, select a new
* shift close to the cluster, find a new factorization, and refi
* the shifted eigenvalues to suitable accuracy.
* (d) For each eigenvalue with a large enough relative separation co
* the corresponding eigenvector by forming a rank revealing twis
* factorization. Go back to (c) for any clusters that remain.
*
* For more details, see:
* - Inderjit S. Dhillon and Beresford N. Parlett: "Multiple representat
* to compute orthogonal eigenvectors of symmetric tridiagonal matrice
* Linear Algebra and its Applications, 387(1), pp. 1-28, August 2004.
* - Inderjit Dhillon and Beresford Parlett: "Orthogonal Eigenvectors an
* Relative Gaps," SIAM Journal on Matrix Analysis and Applications, V
* 2004. Also LAPACK Working Note 154.
* - Inderjit Dhillon: "A new O(n^2) algorithm for the symmetric
* tridiagonal eigenvalue/eigenvector problem",
* Computer Science Division Technical Report No. UCB/CSD-97-971,
* UC Berkeley, May 1997.
*
* Notes:
* 1.SSTEMR works only on machines which follow IEEE-754
* floating-point standard in their handling of infinities and NaNs.
* This permits the use of efficient inner loops avoiding a check for
* zero divisors.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, the N diagonal elements of the tridiagonal matrix
* T. On exit, D is overwritten.
*
* E (input/output) REAL array, dimension (N)
* On entry, the (N-1) subdiagonal elements of the tridiagonal
* matrix T in elements 1 to N-1 of E. E(N) need not be set on
* input, but is used internally as workspace.
* On exit, E is overwritten.
*
* VL (input) REAL
* VU (input) REAL
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) REAL array, dimension (N)
* The first M elements contain the selected eigenvalues in
* ascending order.
*
* Z (output) REAL array, dimension (LDZ, max(1,M) )
* If JOBZ = 'V', and if INFO = 0, then the first M columns of Z
* contain the orthonormal eigenvectors of the matrix T
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* If JOBZ = 'N', then Z is not referenced.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and can be computed with a workspace
* query by setting NZC = -1, see below.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', then LDZ >= max(1,N).
*
* NZC (input) INTEGER
* The number of eigenvectors to be held in the array Z.
* If RANGE = 'A', then NZC >= max(1,N).
* If RANGE = 'V', then NZC >= the number of eigenvalues in (VL,
* If RANGE = 'I', then NZC >= IU-IL+1.
* If NZC = -1, then a workspace query is assumed; the
* routine calculates the number of columns of the array Z that
* are needed to hold the eigenvectors.
* This value is returned as the first entry of the Z array, and
* no error message related to NZC is issued by XERBLA.
*
* ISUPPZ (output) INTEGER ARRAY, dimension ( 2*max(1,M) )
* The support of the eigenvectors in Z, i.e., the indices
* indicating the nonzero elements in Z. The i-th computed eigen
* is nonzero only in elements ISUPPZ( 2*i-1 ) through
* ISUPPZ( 2*i ). This is relevant in the case when the matrix
* is split. ISUPPZ is only accessed when JOBZ is 'V' and N > 0.
*
* TRYRAC (input/output) LOGICAL
* If TRYRAC.EQ..TRUE., indicates that the code should check whe
* the tridiagonal matrix defines its eigenvalues to high relati
* accuracy. If so, the code uses relative-accuracy preserving
* algorithms that might be (a bit) slower depending on the matr
* If the matrix does not define its eigenvalues to high relativ
* accuracy, the code can uses possibly faster algorithms.
* If TRYRAC.EQ..FALSE., the code is not required to guarantee
* relatively accurate eigenvalues and can use the fastest possi
* techniques.
* On exit, a .TRUE. TRYRAC will be set to .FALSE. if the matrix
* does not define its eigenvalues to high relative accuracy.
*
* WORK (workspace/output) REAL array, dimension (LWORK)
* On exit, if INFO = 0, WORK(1) returns the optimal
* (and minimal) LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,18*N)
* if JOBZ = 'V', and LWORK >= max(1,12*N) if JOBZ = 'N'.
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (LIWORK)
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK. LIWORK >= max(1,10*N)
* if the eigenvectors are desired, and LIWORK >= max(1,8*N)
* if only the eigenvalues are to be computed.
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal size of the IWORK array,
* returns this value as the first entry of the IWORK array, and
* no error message related to LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* On exit, INFO
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = 1X, internal error in SLARRE,
* if INFO = 2X, internal error in SLARRV.
* Here, the digit X = ABS( IINFO ) < 10, where IINFO is
* the nonzero error code returned by SLARRE or
* SLARRV, respectively.
*
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param range
* @param n
* @param d
* @param e
* @param vl
* @param vu
* @param il
* @param iu
* @param m
* @param w
* @param z
* @param ldz
* @param nzc
* @param isuppz
* @param tryrac
* @param work
* @param lwork
* @param iwork
* @param liwork
* @param info
*
*/
abstract public void sstemr(java.lang.String jobz, java.lang.String range, int n, float[] d, float[] e, float vl, float vu, int il, int iu, org.netlib.util.intW m, float[] w, float[] z, int ldz, int nzc, int[] isuppz, org.netlib.util.booleanW tryrac, float[] work, int lwork, int[] iwork, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSTEMR computes selected eigenvalues and, optionally, eigenvectors
* of a real symmetric tridiagonal matrix T. Any such unreduced matrix h
* a well defined set of pairwise different real eigenvalues, the corres
* real eigenvectors are pairwise orthogonal.
*
* The spectrum may be computed either completely or partially by specif
* either an interval (VL,VU] or a range of indices IL:IU for the desire
* eigenvalues.
*
* Depending on the number of desired eigenvalues, these are computed ei
* by bisection or the dqds algorithm. Numerically orthogonal eigenvecto
* computed by the use of various suitable L D L^T factorizations near c
* of close eigenvalues (referred to as RRRs, Relatively Robust
* Representations). An informal sketch of the algorithm follows.
*
* For each unreduced block (submatrix) of T,
* (a) Compute T - sigma I = L D L^T, so that L and D
* define all the wanted eigenvalues to high relative accuracy.
* This means that small relative changes in the entries of D and
* cause only small relative changes in the eigenvalues and
* eigenvectors. The standard (unfactored) representation of the
* tridiagonal matrix T does not have this property in general.
* (b) Compute the eigenvalues to suitable accuracy.
* If the eigenvectors are desired, the algorithm attains full
* accuracy of the computed eigenvalues only right before
* the corresponding vectors have to be computed, see steps c) an
* (c) For each cluster of close eigenvalues, select a new
* shift close to the cluster, find a new factorization, and refi
* the shifted eigenvalues to suitable accuracy.
* (d) For each eigenvalue with a large enough relative separation co
* the corresponding eigenvector by forming a rank revealing twis
* factorization. Go back to (c) for any clusters that remain.
*
* For more details, see:
* - Inderjit S. Dhillon and Beresford N. Parlett: "Multiple representat
* to compute orthogonal eigenvectors of symmetric tridiagonal matrice
* Linear Algebra and its Applications, 387(1), pp. 1-28, August 2004.
* - Inderjit Dhillon and Beresford Parlett: "Orthogonal Eigenvectors an
* Relative Gaps," SIAM Journal on Matrix Analysis and Applications, V
* 2004. Also LAPACK Working Note 154.
* - Inderjit Dhillon: "A new O(n^2) algorithm for the symmetric
* tridiagonal eigenvalue/eigenvector problem",
* Computer Science Division Technical Report No. UCB/CSD-97-971,
* UC Berkeley, May 1997.
*
* Notes:
* 1.SSTEMR works only on machines which follow IEEE-754
* floating-point standard in their handling of infinities and NaNs.
* This permits the use of efficient inner loops avoiding a check for
* zero divisors.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, the N diagonal elements of the tridiagonal matrix
* T. On exit, D is overwritten.
*
* E (input/output) REAL array, dimension (N)
* On entry, the (N-1) subdiagonal elements of the tridiagonal
* matrix T in elements 1 to N-1 of E. E(N) need not be set on
* input, but is used internally as workspace.
* On exit, E is overwritten.
*
* VL (input) REAL
* VU (input) REAL
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) REAL array, dimension (N)
* The first M elements contain the selected eigenvalues in
* ascending order.
*
* Z (output) REAL array, dimension (LDZ, max(1,M) )
* If JOBZ = 'V', and if INFO = 0, then the first M columns of Z
* contain the orthonormal eigenvectors of the matrix T
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* If JOBZ = 'N', then Z is not referenced.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and can be computed with a workspace
* query by setting NZC = -1, see below.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', then LDZ >= max(1,N).
*
* NZC (input) INTEGER
* The number of eigenvectors to be held in the array Z.
* If RANGE = 'A', then NZC >= max(1,N).
* If RANGE = 'V', then NZC >= the number of eigenvalues in (VL,
* If RANGE = 'I', then NZC >= IU-IL+1.
* If NZC = -1, then a workspace query is assumed; the
* routine calculates the number of columns of the array Z that
* are needed to hold the eigenvectors.
* This value is returned as the first entry of the Z array, and
* no error message related to NZC is issued by XERBLA.
*
* ISUPPZ (output) INTEGER ARRAY, dimension ( 2*max(1,M) )
* The support of the eigenvectors in Z, i.e., the indices
* indicating the nonzero elements in Z. The i-th computed eigen
* is nonzero only in elements ISUPPZ( 2*i-1 ) through
* ISUPPZ( 2*i ). This is relevant in the case when the matrix
* is split. ISUPPZ is only accessed when JOBZ is 'V' and N > 0.
*
* TRYRAC (input/output) LOGICAL
* If TRYRAC.EQ..TRUE., indicates that the code should check whe
* the tridiagonal matrix defines its eigenvalues to high relati
* accuracy. If so, the code uses relative-accuracy preserving
* algorithms that might be (a bit) slower depending on the matr
* If the matrix does not define its eigenvalues to high relativ
* accuracy, the code can uses possibly faster algorithms.
* If TRYRAC.EQ..FALSE., the code is not required to guarantee
* relatively accurate eigenvalues and can use the fastest possi
* techniques.
* On exit, a .TRUE. TRYRAC will be set to .FALSE. if the matrix
* does not define its eigenvalues to high relative accuracy.
*
* WORK (workspace/output) REAL array, dimension (LWORK)
* On exit, if INFO = 0, WORK(1) returns the optimal
* (and minimal) LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,18*N)
* if JOBZ = 'V', and LWORK >= max(1,12*N) if JOBZ = 'N'.
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (LIWORK)
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK. LIWORK >= max(1,10*N)
* if the eigenvectors are desired, and LIWORK >= max(1,8*N)
* if only the eigenvalues are to be computed.
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal size of the IWORK array,
* returns this value as the first entry of the IWORK array, and
* no error message related to LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* On exit, INFO
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = 1X, internal error in SLARRE,
* if INFO = 2X, internal error in SLARRV.
* Here, the digit X = ABS( IINFO ) < 10, where IINFO is
* the nonzero error code returned by SLARRE or
* SLARRV, respectively.
*
*
* Further Details
* ===============
*
* Based on contributions by
* Beresford Parlett, University of California, Berkeley, USA
* Jim Demmel, University of California, Berkeley, USA
* Inderjit Dhillon, University of Texas, Austin, USA
* Osni Marques, LBNL/NERSC, USA
* Christof Voemel, University of California, Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param range
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param vl
* @param vu
* @param il
* @param iu
* @param m
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param nzc
* @param isuppz
* @param _isuppz_offset
* @param tryrac
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param liwork
* @param info
*
*/
abstract public void sstemr(java.lang.String jobz, java.lang.String range, int n, float[] d, int _d_offset, float[] e, int _e_offset, float vl, float vu, int il, int iu, org.netlib.util.intW m, float[] w, int _w_offset, float[] z, int _z_offset, int ldz, int nzc, int[] isuppz, int _isuppz_offset, org.netlib.util.booleanW tryrac, float[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSTEQR computes all eigenvalues and, optionally, eigenvectors of a
* symmetric tridiagonal matrix using the implicit QL or QR method.
* The eigenvectors of a full or band symmetric matrix can also be found
* if SSYTRD or SSPTRD or SSBTRD has been used to reduce this matrix to
* tridiagonal form.
*
* Arguments
* =========
*
* COMPZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only.
* = 'V': Compute eigenvalues and eigenvectors of the original
* symmetric matrix. On entry, Z must contain the
* orthogonal matrix used to reduce the original matrix
* to tridiagonal form.
* = 'I': Compute eigenvalues and eigenvectors of the
* tridiagonal matrix. Z is initialized to the identity
* matrix.
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, the diagonal elements of the tridiagonal matrix.
* On exit, if INFO = 0, the eigenvalues in ascending order.
*
* E (input/output) REAL array, dimension (N-1)
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix.
* On exit, E has been destroyed.
*
* Z (input/output) REAL array, dimension (LDZ, N)
* On entry, if COMPZ = 'V', then Z contains the orthogonal
* matrix used in the reduction to tridiagonal form.
* On exit, if INFO = 0, then if COMPZ = 'V', Z contains the
* orthonormal eigenvectors of the original symmetric matrix,
* and if COMPZ = 'I', Z contains the orthonormal eigenvectors
* of the symmetric tridiagonal matrix.
* If COMPZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* eigenvectors are desired, then LDZ >= max(1,N).
*
* WORK (workspace) REAL array, dimension (max(1,2*N-2))
* If COMPZ = 'N', then WORK is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: the algorithm has failed to find all the eigenvalues in
* a total of 30*N iterations; if INFO = i, then i
* elements of E have not converged to zero; on exit, D
* and E contain the elements of a symmetric tridiagonal
* matrix which is orthogonally similar to the original
* matrix.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param compz
* @param n
* @param d
* @param e
* @param z
* @param ldz
* @param work
* @param info
*
*/
abstract public void ssteqr(java.lang.String compz, int n, float[] d, float[] e, float[] z, int ldz, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSTEQR computes all eigenvalues and, optionally, eigenvectors of a
* symmetric tridiagonal matrix using the implicit QL or QR method.
* The eigenvectors of a full or band symmetric matrix can also be found
* if SSYTRD or SSPTRD or SSBTRD has been used to reduce this matrix to
* tridiagonal form.
*
* Arguments
* =========
*
* COMPZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only.
* = 'V': Compute eigenvalues and eigenvectors of the original
* symmetric matrix. On entry, Z must contain the
* orthogonal matrix used to reduce the original matrix
* to tridiagonal form.
* = 'I': Compute eigenvalues and eigenvectors of the
* tridiagonal matrix. Z is initialized to the identity
* matrix.
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, the diagonal elements of the tridiagonal matrix.
* On exit, if INFO = 0, the eigenvalues in ascending order.
*
* E (input/output) REAL array, dimension (N-1)
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix.
* On exit, E has been destroyed.
*
* Z (input/output) REAL array, dimension (LDZ, N)
* On entry, if COMPZ = 'V', then Z contains the orthogonal
* matrix used in the reduction to tridiagonal form.
* On exit, if INFO = 0, then if COMPZ = 'V', Z contains the
* orthonormal eigenvectors of the original symmetric matrix,
* and if COMPZ = 'I', Z contains the orthonormal eigenvectors
* of the symmetric tridiagonal matrix.
* If COMPZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* eigenvectors are desired, then LDZ >= max(1,N).
*
* WORK (workspace) REAL array, dimension (max(1,2*N-2))
* If COMPZ = 'N', then WORK is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: the algorithm has failed to find all the eigenvalues in
* a total of 30*N iterations; if INFO = i, then i
* elements of E have not converged to zero; on exit, D
* and E contain the elements of a symmetric tridiagonal
* matrix which is orthogonally similar to the original
* matrix.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param compz
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void ssteqr(java.lang.String compz, int n, float[] d, int _d_offset, float[] e, int _e_offset, float[] z, int _z_offset, int ldz, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSTERF computes all eigenvalues of a symmetric tridiagonal matrix
* using the Pal-Walker-Kahan variant of the QL or QR algorithm.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, the n diagonal elements of the tridiagonal matrix.
* On exit, if INFO = 0, the eigenvalues in ascending order.
*
* E (input/output) REAL array, dimension (N-1)
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix.
* On exit, E has been destroyed.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: the algorithm failed to find all of the eigenvalues in
* a total of 30*N iterations; if INFO = i, then i
* elements of E have not converged to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param e
* @param info
*
*/
abstract public void ssterf(int n, float[] d, float[] e, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSTERF computes all eigenvalues of a symmetric tridiagonal matrix
* using the Pal-Walker-Kahan variant of the QL or QR algorithm.
*
* Arguments
* =========
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, the n diagonal elements of the tridiagonal matrix.
* On exit, if INFO = 0, the eigenvalues in ascending order.
*
* E (input/output) REAL array, dimension (N-1)
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix.
* On exit, E has been destroyed.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: the algorithm failed to find all of the eigenvalues in
* a total of 30*N iterations; if INFO = i, then i
* elements of E have not converged to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param info
*
*/
abstract public void ssterf(int n, float[] d, int _d_offset, float[] e, int _e_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSTEV computes all eigenvalues and, optionally, eigenvectors of a
* real symmetric tridiagonal matrix A.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, the n diagonal elements of the tridiagonal matrix
* A.
* On exit, if INFO = 0, the eigenvalues in ascending order.
*
* E (input/output) REAL array, dimension (N-1)
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix A, stored in elements 1 to N-1 of E.
* On exit, the contents of E are destroyed.
*
* Z (output) REAL array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the orthonormal
* eigenvectors of the matrix A, with the i-th column of Z
* holding the eigenvector associated with D(i).
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace) REAL array, dimension (max(1,2*N-2))
* If JOBZ = 'N', WORK is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the algorithm failed to converge; i
* off-diagonal elements of E did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param n
* @param d
* @param e
* @param z
* @param ldz
* @param work
* @param info
*
*/
abstract public void sstev(java.lang.String jobz, int n, float[] d, float[] e, float[] z, int ldz, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSTEV computes all eigenvalues and, optionally, eigenvectors of a
* real symmetric tridiagonal matrix A.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, the n diagonal elements of the tridiagonal matrix
* A.
* On exit, if INFO = 0, the eigenvalues in ascending order.
*
* E (input/output) REAL array, dimension (N-1)
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix A, stored in elements 1 to N-1 of E.
* On exit, the contents of E are destroyed.
*
* Z (output) REAL array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the orthonormal
* eigenvectors of the matrix A, with the i-th column of Z
* holding the eigenvector associated with D(i).
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace) REAL array, dimension (max(1,2*N-2))
* If JOBZ = 'N', WORK is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the algorithm failed to converge; i
* off-diagonal elements of E did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void sstev(java.lang.String jobz, int n, float[] d, int _d_offset, float[] e, int _e_offset, float[] z, int _z_offset, int ldz, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSTEVD computes all eigenvalues and, optionally, eigenvectors of a
* real symmetric tridiagonal matrix. If eigenvectors are desired, it
* uses a divide and conquer algorithm.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, the n diagonal elements of the tridiagonal matrix
* A.
* On exit, if INFO = 0, the eigenvalues in ascending order.
*
* E (input/output) REAL array, dimension (N-1)
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix A, stored in elements 1 to N-1 of E.
* On exit, the contents of E are destroyed.
*
* Z (output) REAL array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the orthonormal
* eigenvectors of the matrix A, with the i-th column of Z
* holding the eigenvector associated with D(i).
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace/output) REAL array,
* dimension (LWORK)
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If JOBZ = 'N' or N <= 1 then LWORK must be at least 1.
* If JOBZ = 'V' and N > 1 then LWORK must be at least
* ( 1 + 4*N + N**2 ).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If JOBZ = 'N' or N <= 1 then LIWORK must be at least 1.
* If JOBZ = 'V' and N > 1 then LIWORK must be at least 3+5*N.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the algorithm failed to converge; i
* off-diagonal elements of E did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param n
* @param d
* @param e
* @param z
* @param ldz
* @param work
* @param lwork
* @param iwork
* @param liwork
* @param info
*
*/
abstract public void sstevd(java.lang.String jobz, int n, float[] d, float[] e, float[] z, int ldz, float[] work, int lwork, int[] iwork, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSTEVD computes all eigenvalues and, optionally, eigenvectors of a
* real symmetric tridiagonal matrix. If eigenvectors are desired, it
* uses a divide and conquer algorithm.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, the n diagonal elements of the tridiagonal matrix
* A.
* On exit, if INFO = 0, the eigenvalues in ascending order.
*
* E (input/output) REAL array, dimension (N-1)
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix A, stored in elements 1 to N-1 of E.
* On exit, the contents of E are destroyed.
*
* Z (output) REAL array, dimension (LDZ, N)
* If JOBZ = 'V', then if INFO = 0, Z contains the orthonormal
* eigenvectors of the matrix A, with the i-th column of Z
* holding the eigenvector associated with D(i).
* If JOBZ = 'N', then Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace/output) REAL array,
* dimension (LWORK)
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If JOBZ = 'N' or N <= 1 then LWORK must be at least 1.
* If JOBZ = 'V' and N > 1 then LWORK must be at least
* ( 1 + 4*N + N**2 ).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If JOBZ = 'N' or N <= 1 then LIWORK must be at least 1.
* If JOBZ = 'V' and N > 1 then LIWORK must be at least 3+5*N.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the algorithm failed to converge; i
* off-diagonal elements of E did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param liwork
* @param info
*
*/
abstract public void sstevd(java.lang.String jobz, int n, float[] d, int _d_offset, float[] e, int _e_offset, float[] z, int _z_offset, int ldz, float[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSTEVR computes selected eigenvalues and, optionally, eigenvectors
* of a real symmetric tridiagonal matrix T. Eigenvalues and
* eigenvectors can be selected by specifying either a range of values
* or a range of indices for the desired eigenvalues.
*
* Whenever possible, SSTEVR calls SSTEMR to compute the
* eigenspectrum using Relatively Robust Representations. SSTEMR
* computes eigenvalues by the dqds algorithm, while orthogonal
* eigenvectors are computed from various "good" L D L^T representations
* (also known as Relatively Robust Representations). Gram-Schmidt
* orthogonalization is avoided as far as possible. More specifically,
* the various steps of the algorithm are as follows. For the i-th
* unreduced block of T,
* (a) Compute T - sigma_i = L_i D_i L_i^T, such that L_i D_i L_i^T
* is a relatively robust representation,
* (b) Compute the eigenvalues, lambda_j, of L_i D_i L_i^T to high
* relative accuracy by the dqds algorithm,
* (c) If there is a cluster of close eigenvalues, "choose" sigma_i
* close to the cluster, and go to step (a),
* (d) Given the approximate eigenvalue lambda_j of L_i D_i L_i^T,
* compute the corresponding eigenvector by forming a
* rank-revealing twisted factorization.
* The desired accuracy of the output can be specified by the input
* parameter ABSTOL.
*
* For more details, see "A new O(n^2) algorithm for the symmetric
* tridiagonal eigenvalue/eigenvector problem", by Inderjit Dhillon,
* Computer Science Division Technical Report No. UCB//CSD-97-971,
* UC Berkeley, May 1997.
*
*
* Note 1 : SSTEVR calls SSTEMR when the full spectrum is requested
* on machines which conform to the ieee-754 floating point standard.
* SSTEVR calls SSTEBZ and SSTEIN on non-ieee machines and
* when partial spectrum requests are made.
*
* Normal execution of SSTEMR may create NaNs and infinities and
* hence may abort due to a floating point exception in environments
* which do not handle NaNs and infinities in the ieee standard default
* manner.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
********** For RANGE = 'V' or 'I' and IU - IL < N - 1, SSTEBZ and
********** SSTEIN are called
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, the n diagonal elements of the tridiagonal matrix
* A.
* On exit, D may be multiplied by a constant factor chosen
* to avoid over/underflow in computing the eigenvalues.
*
* E (input/output) REAL array, dimension (max(1,N-1))
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix A in elements 1 to N-1 of E.
* On exit, E may be multiplied by a constant factor chosen
* to avoid over/underflow in computing the eigenvalues.
*
* VL (input) REAL
* VU (input) REAL
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) REAL
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less than
* or equal to zero, then EPS*|T| will be used in its place,
* where |T| is the 1-norm of the tridiagonal matrix obtained
* by reducing A to tridiagonal form.
*
* See "Computing Small Singular Values of Bidiagonal Matrices
* with Guaranteed High Relative Accuracy," by Demmel and
* Kahan, LAPACK Working Note #3.
*
* If high relative accuracy is important, set ABSTOL to
* SLAMCH( 'Safe minimum' ). Doing so will guarantee that
* eigenvalues are computed to high relative accuracy when
* possible in future releases. The current code does not
* make any guarantees about high relative accuracy, but
* future releases will. See J. Barlow and J. Demmel,
* "Computing Accurate Eigensystems of Scaled Diagonally
* Dominant Matrices", LAPACK Working Note #7, for a discussion
* of which matrices define their eigenvalues to high relative
* accuracy.
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) REAL array, dimension (N)
* The first M elements contain the selected eigenvalues in
* ascending order.
*
* Z (output) REAL array, dimension (LDZ, max(1,M) )
* If JOBZ = 'V', then if INFO = 0, the first M columns of Z
* contain the orthonormal eigenvectors of the matrix A
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* ISUPPZ (output) INTEGER array, dimension ( 2*max(1,M) )
* The support of the eigenvectors in Z, i.e., the indices
* indicating the nonzero elements in Z. The i-th eigenvector
* is nonzero only in elements ISUPPZ( 2*i-1 ) through
* ISUPPZ( 2*i ).
********** Implemented only for RANGE = 'A' or 'I' and IU - IL = N - 1
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal (and
* minimal) LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= 20*N.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal (and
* minimal) LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK. LIWORK >= 10*N.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: Internal error
*
* Further Details
* ===============
*
* Based on contributions by
* Inderjit Dhillon, IBM Almaden, USA
* Osni Marques, LBNL/NERSC, USA
* Ken Stanley, Computer Science Division, University of
* California at Berkeley, USA
* Jason Riedy, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param range
* @param n
* @param d
* @param e
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param z
* @param ldz
* @param isuppz
* @param work
* @param lwork
* @param iwork
* @param liwork
* @param info
*
*/
abstract public void sstevr(java.lang.String jobz, java.lang.String range, int n, float[] d, float[] e, float vl, float vu, int il, int iu, float abstol, org.netlib.util.intW m, float[] w, float[] z, int ldz, int[] isuppz, float[] work, int lwork, int[] iwork, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSTEVR computes selected eigenvalues and, optionally, eigenvectors
* of a real symmetric tridiagonal matrix T. Eigenvalues and
* eigenvectors can be selected by specifying either a range of values
* or a range of indices for the desired eigenvalues.
*
* Whenever possible, SSTEVR calls SSTEMR to compute the
* eigenspectrum using Relatively Robust Representations. SSTEMR
* computes eigenvalues by the dqds algorithm, while orthogonal
* eigenvectors are computed from various "good" L D L^T representations
* (also known as Relatively Robust Representations). Gram-Schmidt
* orthogonalization is avoided as far as possible. More specifically,
* the various steps of the algorithm are as follows. For the i-th
* unreduced block of T,
* (a) Compute T - sigma_i = L_i D_i L_i^T, such that L_i D_i L_i^T
* is a relatively robust representation,
* (b) Compute the eigenvalues, lambda_j, of L_i D_i L_i^T to high
* relative accuracy by the dqds algorithm,
* (c) If there is a cluster of close eigenvalues, "choose" sigma_i
* close to the cluster, and go to step (a),
* (d) Given the approximate eigenvalue lambda_j of L_i D_i L_i^T,
* compute the corresponding eigenvector by forming a
* rank-revealing twisted factorization.
* The desired accuracy of the output can be specified by the input
* parameter ABSTOL.
*
* For more details, see "A new O(n^2) algorithm for the symmetric
* tridiagonal eigenvalue/eigenvector problem", by Inderjit Dhillon,
* Computer Science Division Technical Report No. UCB//CSD-97-971,
* UC Berkeley, May 1997.
*
*
* Note 1 : SSTEVR calls SSTEMR when the full spectrum is requested
* on machines which conform to the ieee-754 floating point standard.
* SSTEVR calls SSTEBZ and SSTEIN on non-ieee machines and
* when partial spectrum requests are made.
*
* Normal execution of SSTEMR may create NaNs and infinities and
* hence may abort due to a floating point exception in environments
* which do not handle NaNs and infinities in the ieee standard default
* manner.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
********** For RANGE = 'V' or 'I' and IU - IL < N - 1, SSTEBZ and
********** SSTEIN are called
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, the n diagonal elements of the tridiagonal matrix
* A.
* On exit, D may be multiplied by a constant factor chosen
* to avoid over/underflow in computing the eigenvalues.
*
* E (input/output) REAL array, dimension (max(1,N-1))
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix A in elements 1 to N-1 of E.
* On exit, E may be multiplied by a constant factor chosen
* to avoid over/underflow in computing the eigenvalues.
*
* VL (input) REAL
* VU (input) REAL
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) REAL
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less than
* or equal to zero, then EPS*|T| will be used in its place,
* where |T| is the 1-norm of the tridiagonal matrix obtained
* by reducing A to tridiagonal form.
*
* See "Computing Small Singular Values of Bidiagonal Matrices
* with Guaranteed High Relative Accuracy," by Demmel and
* Kahan, LAPACK Working Note #3.
*
* If high relative accuracy is important, set ABSTOL to
* SLAMCH( 'Safe minimum' ). Doing so will guarantee that
* eigenvalues are computed to high relative accuracy when
* possible in future releases. The current code does not
* make any guarantees about high relative accuracy, but
* future releases will. See J. Barlow and J. Demmel,
* "Computing Accurate Eigensystems of Scaled Diagonally
* Dominant Matrices", LAPACK Working Note #7, for a discussion
* of which matrices define their eigenvalues to high relative
* accuracy.
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) REAL array, dimension (N)
* The first M elements contain the selected eigenvalues in
* ascending order.
*
* Z (output) REAL array, dimension (LDZ, max(1,M) )
* If JOBZ = 'V', then if INFO = 0, the first M columns of Z
* contain the orthonormal eigenvectors of the matrix A
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* ISUPPZ (output) INTEGER array, dimension ( 2*max(1,M) )
* The support of the eigenvectors in Z, i.e., the indices
* indicating the nonzero elements in Z. The i-th eigenvector
* is nonzero only in elements ISUPPZ( 2*i-1 ) through
* ISUPPZ( 2*i ).
********** Implemented only for RANGE = 'A' or 'I' and IU - IL = N - 1
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal (and
* minimal) LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= 20*N.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal (and
* minimal) LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK. LIWORK >= 10*N.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: Internal error
*
* Further Details
* ===============
*
* Based on contributions by
* Inderjit Dhillon, IBM Almaden, USA
* Osni Marques, LBNL/NERSC, USA
* Ken Stanley, Computer Science Division, University of
* California at Berkeley, USA
* Jason Riedy, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param range
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param isuppz
* @param _isuppz_offset
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param liwork
* @param info
*
*/
abstract public void sstevr(java.lang.String jobz, java.lang.String range, int n, float[] d, int _d_offset, float[] e, int _e_offset, float vl, float vu, int il, int iu, float abstol, org.netlib.util.intW m, float[] w, int _w_offset, float[] z, int _z_offset, int ldz, int[] isuppz, int _isuppz_offset, float[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSTEVX computes selected eigenvalues and, optionally, eigenvectors
* of a real symmetric tridiagonal matrix A. Eigenvalues and
* eigenvectors can be selected by specifying either a range of values
* or a range of indices for the desired eigenvalues.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, the n diagonal elements of the tridiagonal matrix
* A.
* On exit, D may be multiplied by a constant factor chosen
* to avoid over/underflow in computing the eigenvalues.
*
* E (input/output) REAL array, dimension (max(1,N-1))
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix A in elements 1 to N-1 of E.
* On exit, E may be multiplied by a constant factor chosen
* to avoid over/underflow in computing the eigenvalues.
*
* VL (input) REAL
* VU (input) REAL
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) REAL
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less
* than or equal to zero, then EPS*|T| will be used in
* its place, where |T| is the 1-norm of the tridiagonal
* matrix.
*
* Eigenvalues will be computed most accurately when ABSTOL is
* set to twice the underflow threshold 2*SLAMCH('S'), not zero.
* If this routine returns with INFO>0, indicating that some
* eigenvectors did not converge, try setting ABSTOL to
* 2*SLAMCH('S').
*
* See "Computing Small Singular Values of Bidiagonal Matrices
* with Guaranteed High Relative Accuracy," by Demmel and
* Kahan, LAPACK Working Note #3.
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) REAL array, dimension (N)
* The first M elements contain the selected eigenvalues in
* ascending order.
*
* Z (output) REAL array, dimension (LDZ, max(1,M) )
* If JOBZ = 'V', then if INFO = 0, the first M columns of Z
* contain the orthonormal eigenvectors of the matrix A
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* If an eigenvector fails to converge (INFO > 0), then that
* column of Z contains the latest approximation to the
* eigenvector, and the index of the eigenvector is returned
* in IFAIL. If JOBZ = 'N', then Z is not referenced.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace) REAL array, dimension (5*N)
*
* IWORK (workspace) INTEGER array, dimension (5*N)
*
* IFAIL (output) INTEGER array, dimension (N)
* If JOBZ = 'V', then if INFO = 0, the first M elements of
* IFAIL are zero. If INFO > 0, then IFAIL contains the
* indices of the eigenvectors that failed to converge.
* If JOBZ = 'N', then IFAIL is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, then i eigenvectors failed to converge.
* Their indices are stored in array IFAIL.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param range
* @param n
* @param d
* @param e
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param z
* @param ldz
* @param work
* @param iwork
* @param ifail
* @param info
*
*/
abstract public void sstevx(java.lang.String jobz, java.lang.String range, int n, float[] d, float[] e, float vl, float vu, int il, int iu, float abstol, org.netlib.util.intW m, float[] w, float[] z, int ldz, float[] work, int[] iwork, int[] ifail, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSTEVX computes selected eigenvalues and, optionally, eigenvectors
* of a real symmetric tridiagonal matrix A. Eigenvalues and
* eigenvectors can be selected by specifying either a range of values
* or a range of indices for the desired eigenvalues.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* N (input) INTEGER
* The order of the matrix. N >= 0.
*
* D (input/output) REAL array, dimension (N)
* On entry, the n diagonal elements of the tridiagonal matrix
* A.
* On exit, D may be multiplied by a constant factor chosen
* to avoid over/underflow in computing the eigenvalues.
*
* E (input/output) REAL array, dimension (max(1,N-1))
* On entry, the (n-1) subdiagonal elements of the tridiagonal
* matrix A in elements 1 to N-1 of E.
* On exit, E may be multiplied by a constant factor chosen
* to avoid over/underflow in computing the eigenvalues.
*
* VL (input) REAL
* VU (input) REAL
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) REAL
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less
* than or equal to zero, then EPS*|T| will be used in
* its place, where |T| is the 1-norm of the tridiagonal
* matrix.
*
* Eigenvalues will be computed most accurately when ABSTOL is
* set to twice the underflow threshold 2*SLAMCH('S'), not zero.
* If this routine returns with INFO>0, indicating that some
* eigenvectors did not converge, try setting ABSTOL to
* 2*SLAMCH('S').
*
* See "Computing Small Singular Values of Bidiagonal Matrices
* with Guaranteed High Relative Accuracy," by Demmel and
* Kahan, LAPACK Working Note #3.
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) REAL array, dimension (N)
* The first M elements contain the selected eigenvalues in
* ascending order.
*
* Z (output) REAL array, dimension (LDZ, max(1,M) )
* If JOBZ = 'V', then if INFO = 0, the first M columns of Z
* contain the orthonormal eigenvectors of the matrix A
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* If an eigenvector fails to converge (INFO > 0), then that
* column of Z contains the latest approximation to the
* eigenvector, and the index of the eigenvector is returned
* in IFAIL. If JOBZ = 'N', then Z is not referenced.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace) REAL array, dimension (5*N)
*
* IWORK (workspace) INTEGER array, dimension (5*N)
*
* IFAIL (output) INTEGER array, dimension (N)
* If JOBZ = 'V', then if INFO = 0, the first M elements of
* IFAIL are zero. If INFO > 0, then IFAIL contains the
* indices of the eigenvectors that failed to converge.
* If JOBZ = 'N', then IFAIL is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, then i eigenvectors failed to converge.
* Their indices are stored in array IFAIL.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param range
* @param n
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param ifail
* @param _ifail_offset
* @param info
*
*/
abstract public void sstevx(java.lang.String jobz, java.lang.String range, int n, float[] d, int _d_offset, float[] e, int _e_offset, float vl, float vu, int il, int iu, float abstol, org.netlib.util.intW m, float[] w, int _w_offset, float[] z, int _z_offset, int ldz, float[] work, int _work_offset, int[] iwork, int _iwork_offset, int[] ifail, int _ifail_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYCON estimates the reciprocal of the condition number (in the
* 1-norm) of a real symmetric matrix A using the factorization
* A = U*D*U**T or A = L*D*L**T computed by SSYTRF.
*
* An estimate is obtained for norm(inv(A)), and the reciprocal of the
* condition number is computed as RCOND = 1 / (ANORM * norm(inv(A))).
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the details of the factorization are stored
* as an upper or lower triangular matrix.
* = 'U': Upper triangular, form is A = U*D*U**T;
* = 'L': Lower triangular, form is A = L*D*L**T.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The block diagonal matrix D and the multipliers used to
* obtain the factor U or L as computed by SSYTRF.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (input) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D
* as determined by SSYTRF.
*
* ANORM (input) REAL
* The 1-norm of the original matrix A.
*
* RCOND (output) REAL
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(ANORM * AINVNM), where AINVNM is an
* estimate of the 1-norm of inv(A) computed in this routine.
*
* WORK (workspace) REAL array, dimension (2*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param lda
* @param ipiv
* @param anorm
* @param rcond
* @param work
* @param iwork
* @param info
*
*/
abstract public void ssycon(java.lang.String uplo, int n, float[] a, int lda, int[] ipiv, float anorm, org.netlib.util.floatW rcond, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYCON estimates the reciprocal of the condition number (in the
* 1-norm) of a real symmetric matrix A using the factorization
* A = U*D*U**T or A = L*D*L**T computed by SSYTRF.
*
* An estimate is obtained for norm(inv(A)), and the reciprocal of the
* condition number is computed as RCOND = 1 / (ANORM * norm(inv(A))).
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the details of the factorization are stored
* as an upper or lower triangular matrix.
* = 'U': Upper triangular, form is A = U*D*U**T;
* = 'L': Lower triangular, form is A = L*D*L**T.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The block diagonal matrix D and the multipliers used to
* obtain the factor U or L as computed by SSYTRF.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (input) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D
* as determined by SSYTRF.
*
* ANORM (input) REAL
* The 1-norm of the original matrix A.
*
* RCOND (output) REAL
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(ANORM * AINVNM), where AINVNM is an
* estimate of the 1-norm of inv(A) computed in this routine.
*
* WORK (workspace) REAL array, dimension (2*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param ipiv
* @param _ipiv_offset
* @param anorm
* @param rcond
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void ssycon(java.lang.String uplo, int n, float[] a, int _a_offset, int lda, int[] ipiv, int _ipiv_offset, float anorm, org.netlib.util.floatW rcond, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYEV computes all eigenvalues and, optionally, eigenvectors of a
* real symmetric matrix A.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA, N)
* On entry, the symmetric matrix A. If UPLO = 'U', the
* leading N-by-N upper triangular part of A contains the
* upper triangular part of the matrix A. If UPLO = 'L',
* the leading N-by-N lower triangular part of A contains
* the lower triangular part of the matrix A.
* On exit, if JOBZ = 'V', then if INFO = 0, A contains the
* orthonormal eigenvectors of the matrix A.
* If JOBZ = 'N', then on exit the lower triangle (if UPLO='L')
* or the upper triangle (if UPLO='U') of A, including the
* diagonal, is destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* W (output) REAL array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of the array WORK. LWORK >= max(1,3*N-1).
* For optimal efficiency, LWORK >= (NB+2)*N,
* where NB is the blocksize for SSYTRD returned by ILAENV.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the algorithm failed to converge; i
* off-diagonal elements of an intermediate tridiagonal
* form did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param uplo
* @param n
* @param a
* @param lda
* @param w
* @param work
* @param lwork
* @param info
*
*/
abstract public void ssyev(java.lang.String jobz, java.lang.String uplo, int n, float[] a, int lda, float[] w, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYEV computes all eigenvalues and, optionally, eigenvectors of a
* real symmetric matrix A.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA, N)
* On entry, the symmetric matrix A. If UPLO = 'U', the
* leading N-by-N upper triangular part of A contains the
* upper triangular part of the matrix A. If UPLO = 'L',
* the leading N-by-N lower triangular part of A contains
* the lower triangular part of the matrix A.
* On exit, if JOBZ = 'V', then if INFO = 0, A contains the
* orthonormal eigenvectors of the matrix A.
* If JOBZ = 'N', then on exit the lower triangle (if UPLO='L')
* or the upper triangle (if UPLO='U') of A, including the
* diagonal, is destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* W (output) REAL array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of the array WORK. LWORK >= max(1,3*N-1).
* For optimal efficiency, LWORK >= (NB+2)*N,
* where NB is the blocksize for SSYTRD returned by ILAENV.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the algorithm failed to converge; i
* off-diagonal elements of an intermediate tridiagonal
* form did not converge to zero.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param w
* @param _w_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void ssyev(java.lang.String jobz, java.lang.String uplo, int n, float[] a, int _a_offset, int lda, float[] w, int _w_offset, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYEVD computes all eigenvalues and, optionally, eigenvectors of a
* real symmetric matrix A. If eigenvectors are desired, it uses a
* divide and conquer algorithm.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Because of large use of BLAS of level 3, SSYEVD needs N**2 more
* workspace than SSYEVX.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA, N)
* On entry, the symmetric matrix A. If UPLO = 'U', the
* leading N-by-N upper triangular part of A contains the
* upper triangular part of the matrix A. If UPLO = 'L',
* the leading N-by-N lower triangular part of A contains
* the lower triangular part of the matrix A.
* On exit, if JOBZ = 'V', then if INFO = 0, A contains the
* orthonormal eigenvectors of the matrix A.
* If JOBZ = 'N', then on exit the lower triangle (if UPLO='L')
* or the upper triangle (if UPLO='U') of A, including the
* diagonal, is destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* W (output) REAL array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* WORK (workspace/output) REAL array,
* dimension (LWORK)
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If N <= 1, LWORK must be at least 1.
* If JOBZ = 'N' and N > 1, LWORK must be at least 2*N+1.
* If JOBZ = 'V' and N > 1, LWORK must be at least
* 1 + 6*N + 2*N**2.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If N <= 1, LIWORK must be at least 1.
* If JOBZ = 'N' and N > 1, LIWORK must be at least 1.
* If JOBZ = 'V' and N > 1, LIWORK must be at least 3 + 5*N.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i and JOBZ = 'N', then the algorithm failed
* to converge; i off-diagonal elements of an intermediate
* tridiagonal form did not converge to zero;
* if INFO = i and JOBZ = 'V', then the algorithm failed
* to compute an eigenvalue while working on the submatrix
* lying in rows and columns INFO/(N+1) through
* mod(INFO,N+1).
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
* Modified by Francoise Tisseur, University of Tennessee.
*
* Modified description of INFO. Sven, 16 Feb 05.
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param uplo
* @param n
* @param a
* @param lda
* @param w
* @param work
* @param lwork
* @param iwork
* @param liwork
* @param info
*
*/
abstract public void ssyevd(java.lang.String jobz, java.lang.String uplo, int n, float[] a, int lda, float[] w, float[] work, int lwork, int[] iwork, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYEVD computes all eigenvalues and, optionally, eigenvectors of a
* real symmetric matrix A. If eigenvectors are desired, it uses a
* divide and conquer algorithm.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Because of large use of BLAS of level 3, SSYEVD needs N**2 more
* workspace than SSYEVX.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA, N)
* On entry, the symmetric matrix A. If UPLO = 'U', the
* leading N-by-N upper triangular part of A contains the
* upper triangular part of the matrix A. If UPLO = 'L',
* the leading N-by-N lower triangular part of A contains
* the lower triangular part of the matrix A.
* On exit, if JOBZ = 'V', then if INFO = 0, A contains the
* orthonormal eigenvectors of the matrix A.
* If JOBZ = 'N', then on exit the lower triangle (if UPLO='L')
* or the upper triangle (if UPLO='U') of A, including the
* diagonal, is destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* W (output) REAL array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* WORK (workspace/output) REAL array,
* dimension (LWORK)
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If N <= 1, LWORK must be at least 1.
* If JOBZ = 'N' and N > 1, LWORK must be at least 2*N+1.
* If JOBZ = 'V' and N > 1, LWORK must be at least
* 1 + 6*N + 2*N**2.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If N <= 1, LIWORK must be at least 1.
* If JOBZ = 'N' and N > 1, LIWORK must be at least 1.
* If JOBZ = 'V' and N > 1, LIWORK must be at least 3 + 5*N.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i and JOBZ = 'N', then the algorithm failed
* to converge; i off-diagonal elements of an intermediate
* tridiagonal form did not converge to zero;
* if INFO = i and JOBZ = 'V', then the algorithm failed
* to compute an eigenvalue while working on the submatrix
* lying in rows and columns INFO/(N+1) through
* mod(INFO,N+1).
*
* Further Details
* ===============
*
* Based on contributions by
* Jeff Rutter, Computer Science Division, University of California
* at Berkeley, USA
* Modified by Francoise Tisseur, University of Tennessee.
*
* Modified description of INFO. Sven, 16 Feb 05.
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param w
* @param _w_offset
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param liwork
* @param info
*
*/
abstract public void ssyevd(java.lang.String jobz, java.lang.String uplo, int n, float[] a, int _a_offset, int lda, float[] w, int _w_offset, float[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYEVR computes selected eigenvalues and, optionally, eigenvectors
* of a real symmetric matrix A. Eigenvalues and eigenvectors can be
* selected by specifying either a range of values or a range of
* indices for the desired eigenvalues.
*
* SSYEVR first reduces the matrix A to tridiagonal form T with a call
* to SSYTRD. Then, whenever possible, SSYEVR calls SSTEMR to compute
* the eigenspectrum using Relatively Robust Representations. SSTEMR
* computes eigenvalues by the dqds algorithm, while orthogonal
* eigenvectors are computed from various "good" L D L^T representations
* (also known as Relatively Robust Representations). Gram-Schmidt
* orthogonalization is avoided as far as possible. More specifically,
* the various steps of the algorithm are as follows.
*
* For each unreduced block (submatrix) of T,
* (a) Compute T - sigma I = L D L^T, so that L and D
* define all the wanted eigenvalues to high relative accuracy.
* This means that small relative changes in the entries of D and
* cause only small relative changes in the eigenvalues and
* eigenvectors. The standard (unfactored) representation of the
* tridiagonal matrix T does not have this property in general.
* (b) Compute the eigenvalues to suitable accuracy.
* If the eigenvectors are desired, the algorithm attains full
* accuracy of the computed eigenvalues only right before
* the corresponding vectors have to be computed, see steps c) an
* (c) For each cluster of close eigenvalues, select a new
* shift close to the cluster, find a new factorization, and refi
* the shifted eigenvalues to suitable accuracy.
* (d) For each eigenvalue with a large enough relative separation co
* the corresponding eigenvector by forming a rank revealing twis
* factorization. Go back to (c) for any clusters that remain.
*
* The desired accuracy of the output can be specified by the input
* parameter ABSTOL.
*
* For more details, see SSTEMR's documentation and:
* - Inderjit S. Dhillon and Beresford N. Parlett: "Multiple representat
* to compute orthogonal eigenvectors of symmetric tridiagonal matrice
* Linear Algebra and its Applications, 387(1), pp. 1-28, August 2004.
* - Inderjit Dhillon and Beresford Parlett: "Orthogonal Eigenvectors an
* Relative Gaps," SIAM Journal on Matrix Analysis and Applications, V
* 2004. Also LAPACK Working Note 154.
* - Inderjit Dhillon: "A new O(n^2) algorithm for the symmetric
* tridiagonal eigenvalue/eigenvector problem",
* Computer Science Division Technical Report No. UCB/CSD-97-971,
* UC Berkeley, May 1997.
*
*
* Note 1 : SSYEVR calls SSTEMR when the full spectrum is requested
* on machines which conform to the ieee-754 floating point standard.
* SSYEVR calls SSTEBZ and SSTEIN on non-ieee machines and
* when partial spectrum requests are made.
*
* Normal execution of SSTEMR may create NaNs and infinities and
* hence may abort due to a floating point exception in environments
* which do not handle NaNs and infinities in the ieee standard default
* manner.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
********** For RANGE = 'V' or 'I' and IU - IL < N - 1, SSTEBZ and
********** SSTEIN are called
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA, N)
* On entry, the symmetric matrix A. If UPLO = 'U', the
* leading N-by-N upper triangular part of A contains the
* upper triangular part of the matrix A. If UPLO = 'L',
* the leading N-by-N lower triangular part of A contains
* the lower triangular part of the matrix A.
* On exit, the lower triangle (if UPLO='L') or the upper
* triangle (if UPLO='U') of A, including the diagonal, is
* destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* VL (input) REAL
* VU (input) REAL
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) REAL
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less than
* or equal to zero, then EPS*|T| will be used in its place,
* where |T| is the 1-norm of the tridiagonal matrix obtained
* by reducing A to tridiagonal form.
*
* See "Computing Small Singular Values of Bidiagonal Matrices
* with Guaranteed High Relative Accuracy," by Demmel and
* Kahan, LAPACK Working Note #3.
*
* If high relative accuracy is important, set ABSTOL to
* SLAMCH( 'Safe minimum' ). Doing so will guarantee that
* eigenvalues are computed to high relative accuracy when
* possible in future releases. The current code does not
* make any guarantees about high relative accuracy, but
* future releases will. See J. Barlow and J. Demmel,
* "Computing Accurate Eigensystems of Scaled Diagonally
* Dominant Matrices", LAPACK Working Note #7, for a discussion
* of which matrices define their eigenvalues to high relative
* accuracy.
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) REAL array, dimension (N)
* The first M elements contain the selected eigenvalues in
* ascending order.
*
* Z (output) REAL array, dimension (LDZ, max(1,M))
* If JOBZ = 'V', then if INFO = 0, the first M columns of Z
* contain the orthonormal eigenvectors of the matrix A
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* If JOBZ = 'N', then Z is not referenced.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
* Supplying N columns is always safe.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* ISUPPZ (output) INTEGER array, dimension ( 2*max(1,M) )
* The support of the eigenvectors in Z, i.e., the indices
* indicating the nonzero elements in Z. The i-th eigenvector
* is nonzero only in elements ISUPPZ( 2*i-1 ) through
* ISUPPZ( 2*i ).
********** Implemented only for RANGE = 'A' or 'I' and IU - IL = N - 1
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,26*N).
* For optimal efficiency, LWORK >= (NB+6)*N,
* where NB is the max of the blocksize for SSYTRD and SORMTR
* returned by ILAENV.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal LWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK. LIWORK >= max(1,10*N).
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: Internal error
*
* Further Details
* ===============
*
* Based on contributions by
* Inderjit Dhillon, IBM Almaden, USA
* Osni Marques, LBNL/NERSC, USA
* Ken Stanley, Computer Science Division, University of
* California at Berkeley, USA
* Jason Riedy, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param range
* @param uplo
* @param n
* @param a
* @param lda
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param z
* @param ldz
* @param isuppz
* @param work
* @param lwork
* @param iwork
* @param liwork
* @param info
*
*/
abstract public void ssyevr(java.lang.String jobz, java.lang.String range, java.lang.String uplo, int n, float[] a, int lda, float vl, float vu, int il, int iu, float abstol, org.netlib.util.intW m, float[] w, float[] z, int ldz, int[] isuppz, float[] work, int lwork, int[] iwork, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYEVR computes selected eigenvalues and, optionally, eigenvectors
* of a real symmetric matrix A. Eigenvalues and eigenvectors can be
* selected by specifying either a range of values or a range of
* indices for the desired eigenvalues.
*
* SSYEVR first reduces the matrix A to tridiagonal form T with a call
* to SSYTRD. Then, whenever possible, SSYEVR calls SSTEMR to compute
* the eigenspectrum using Relatively Robust Representations. SSTEMR
* computes eigenvalues by the dqds algorithm, while orthogonal
* eigenvectors are computed from various "good" L D L^T representations
* (also known as Relatively Robust Representations). Gram-Schmidt
* orthogonalization is avoided as far as possible. More specifically,
* the various steps of the algorithm are as follows.
*
* For each unreduced block (submatrix) of T,
* (a) Compute T - sigma I = L D L^T, so that L and D
* define all the wanted eigenvalues to high relative accuracy.
* This means that small relative changes in the entries of D and
* cause only small relative changes in the eigenvalues and
* eigenvectors. The standard (unfactored) representation of the
* tridiagonal matrix T does not have this property in general.
* (b) Compute the eigenvalues to suitable accuracy.
* If the eigenvectors are desired, the algorithm attains full
* accuracy of the computed eigenvalues only right before
* the corresponding vectors have to be computed, see steps c) an
* (c) For each cluster of close eigenvalues, select a new
* shift close to the cluster, find a new factorization, and refi
* the shifted eigenvalues to suitable accuracy.
* (d) For each eigenvalue with a large enough relative separation co
* the corresponding eigenvector by forming a rank revealing twis
* factorization. Go back to (c) for any clusters that remain.
*
* The desired accuracy of the output can be specified by the input
* parameter ABSTOL.
*
* For more details, see SSTEMR's documentation and:
* - Inderjit S. Dhillon and Beresford N. Parlett: "Multiple representat
* to compute orthogonal eigenvectors of symmetric tridiagonal matrice
* Linear Algebra and its Applications, 387(1), pp. 1-28, August 2004.
* - Inderjit Dhillon and Beresford Parlett: "Orthogonal Eigenvectors an
* Relative Gaps," SIAM Journal on Matrix Analysis and Applications, V
* 2004. Also LAPACK Working Note 154.
* - Inderjit Dhillon: "A new O(n^2) algorithm for the symmetric
* tridiagonal eigenvalue/eigenvector problem",
* Computer Science Division Technical Report No. UCB/CSD-97-971,
* UC Berkeley, May 1997.
*
*
* Note 1 : SSYEVR calls SSTEMR when the full spectrum is requested
* on machines which conform to the ieee-754 floating point standard.
* SSYEVR calls SSTEBZ and SSTEIN on non-ieee machines and
* when partial spectrum requests are made.
*
* Normal execution of SSTEMR may create NaNs and infinities and
* hence may abort due to a floating point exception in environments
* which do not handle NaNs and infinities in the ieee standard default
* manner.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
********** For RANGE = 'V' or 'I' and IU - IL < N - 1, SSTEBZ and
********** SSTEIN are called
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA, N)
* On entry, the symmetric matrix A. If UPLO = 'U', the
* leading N-by-N upper triangular part of A contains the
* upper triangular part of the matrix A. If UPLO = 'L',
* the leading N-by-N lower triangular part of A contains
* the lower triangular part of the matrix A.
* On exit, the lower triangle (if UPLO='L') or the upper
* triangle (if UPLO='U') of A, including the diagonal, is
* destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* VL (input) REAL
* VU (input) REAL
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) REAL
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less than
* or equal to zero, then EPS*|T| will be used in its place,
* where |T| is the 1-norm of the tridiagonal matrix obtained
* by reducing A to tridiagonal form.
*
* See "Computing Small Singular Values of Bidiagonal Matrices
* with Guaranteed High Relative Accuracy," by Demmel and
* Kahan, LAPACK Working Note #3.
*
* If high relative accuracy is important, set ABSTOL to
* SLAMCH( 'Safe minimum' ). Doing so will guarantee that
* eigenvalues are computed to high relative accuracy when
* possible in future releases. The current code does not
* make any guarantees about high relative accuracy, but
* future releases will. See J. Barlow and J. Demmel,
* "Computing Accurate Eigensystems of Scaled Diagonally
* Dominant Matrices", LAPACK Working Note #7, for a discussion
* of which matrices define their eigenvalues to high relative
* accuracy.
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) REAL array, dimension (N)
* The first M elements contain the selected eigenvalues in
* ascending order.
*
* Z (output) REAL array, dimension (LDZ, max(1,M))
* If JOBZ = 'V', then if INFO = 0, the first M columns of Z
* contain the orthonormal eigenvectors of the matrix A
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* If JOBZ = 'N', then Z is not referenced.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
* Supplying N columns is always safe.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* ISUPPZ (output) INTEGER array, dimension ( 2*max(1,M) )
* The support of the eigenvectors in Z, i.e., the indices
* indicating the nonzero elements in Z. The i-th eigenvector
* is nonzero only in elements ISUPPZ( 2*i-1 ) through
* ISUPPZ( 2*i ).
********** Implemented only for RANGE = 'A' or 'I' and IU - IL = N - 1
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,26*N).
* For optimal efficiency, LWORK >= (NB+6)*N,
* where NB is the max of the blocksize for SSYTRD and SORMTR
* returned by ILAENV.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal LWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK. LIWORK >= max(1,10*N).
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: Internal error
*
* Further Details
* ===============
*
* Based on contributions by
* Inderjit Dhillon, IBM Almaden, USA
* Osni Marques, LBNL/NERSC, USA
* Ken Stanley, Computer Science Division, University of
* California at Berkeley, USA
* Jason Riedy, Computer Science Division, University of
* California at Berkeley, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param range
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param isuppz
* @param _isuppz_offset
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param liwork
* @param info
*
*/
abstract public void ssyevr(java.lang.String jobz, java.lang.String range, java.lang.String uplo, int n, float[] a, int _a_offset, int lda, float vl, float vu, int il, int iu, float abstol, org.netlib.util.intW m, float[] w, int _w_offset, float[] z, int _z_offset, int ldz, int[] isuppz, int _isuppz_offset, float[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYEVX computes selected eigenvalues and, optionally, eigenvectors
* of a real symmetric matrix A. Eigenvalues and eigenvectors can be
* selected by specifying either a range of values or a range of indices
* for the desired eigenvalues.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA, N)
* On entry, the symmetric matrix A. If UPLO = 'U', the
* leading N-by-N upper triangular part of A contains the
* upper triangular part of the matrix A. If UPLO = 'L',
* the leading N-by-N lower triangular part of A contains
* the lower triangular part of the matrix A.
* On exit, the lower triangle (if UPLO='L') or the upper
* triangle (if UPLO='U') of A, including the diagonal, is
* destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* VL (input) REAL
* VU (input) REAL
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) REAL
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less than
* or equal to zero, then EPS*|T| will be used in its place,
* where |T| is the 1-norm of the tridiagonal matrix obtained
* by reducing A to tridiagonal form.
*
* Eigenvalues will be computed most accurately when ABSTOL is
* set to twice the underflow threshold 2*SLAMCH('S'), not zero.
* If this routine returns with INFO>0, indicating that some
* eigenvectors did not converge, try setting ABSTOL to
* 2*SLAMCH('S').
*
* See "Computing Small Singular Values of Bidiagonal Matrices
* with Guaranteed High Relative Accuracy," by Demmel and
* Kahan, LAPACK Working Note #3.
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) REAL array, dimension (N)
* On normal exit, the first M elements contain the selected
* eigenvalues in ascending order.
*
* Z (output) REAL array, dimension (LDZ, max(1,M))
* If JOBZ = 'V', then if INFO = 0, the first M columns of Z
* contain the orthonormal eigenvectors of the matrix A
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* If an eigenvector fails to converge, then that column of Z
* contains the latest approximation to the eigenvector, and the
* index of the eigenvector is returned in IFAIL.
* If JOBZ = 'N', then Z is not referenced.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of the array WORK. LWORK >= 1, when N <= 1;
* otherwise 8*N.
* For optimal efficiency, LWORK >= (NB+3)*N,
* where NB is the max of the blocksize for SSYTRD and SORMTR
* returned by ILAENV.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (5*N)
*
* IFAIL (output) INTEGER array, dimension (N)
* If JOBZ = 'V', then if INFO = 0, the first M elements of
* IFAIL are zero. If INFO > 0, then IFAIL contains the
* indices of the eigenvectors that failed to converge.
* If JOBZ = 'N', then IFAIL is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, then i eigenvectors failed to converge.
* Their indices are stored in array IFAIL.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param range
* @param uplo
* @param n
* @param a
* @param lda
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param z
* @param ldz
* @param work
* @param lwork
* @param iwork
* @param ifail
* @param info
*
*/
abstract public void ssyevx(java.lang.String jobz, java.lang.String range, java.lang.String uplo, int n, float[] a, int lda, float vl, float vu, int il, int iu, float abstol, org.netlib.util.intW m, float[] w, float[] z, int ldz, float[] work, int lwork, int[] iwork, int[] ifail, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYEVX computes selected eigenvalues and, optionally, eigenvectors
* of a real symmetric matrix A. Eigenvalues and eigenvectors can be
* selected by specifying either a range of values or a range of indices
* for the desired eigenvalues.
*
* Arguments
* =========
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA, N)
* On entry, the symmetric matrix A. If UPLO = 'U', the
* leading N-by-N upper triangular part of A contains the
* upper triangular part of the matrix A. If UPLO = 'L',
* the leading N-by-N lower triangular part of A contains
* the lower triangular part of the matrix A.
* On exit, the lower triangle (if UPLO='L') or the upper
* triangle (if UPLO='U') of A, including the diagonal, is
* destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* VL (input) REAL
* VU (input) REAL
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) REAL
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less than
* or equal to zero, then EPS*|T| will be used in its place,
* where |T| is the 1-norm of the tridiagonal matrix obtained
* by reducing A to tridiagonal form.
*
* Eigenvalues will be computed most accurately when ABSTOL is
* set to twice the underflow threshold 2*SLAMCH('S'), not zero.
* If this routine returns with INFO>0, indicating that some
* eigenvectors did not converge, try setting ABSTOL to
* 2*SLAMCH('S').
*
* See "Computing Small Singular Values of Bidiagonal Matrices
* with Guaranteed High Relative Accuracy," by Demmel and
* Kahan, LAPACK Working Note #3.
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) REAL array, dimension (N)
* On normal exit, the first M elements contain the selected
* eigenvalues in ascending order.
*
* Z (output) REAL array, dimension (LDZ, max(1,M))
* If JOBZ = 'V', then if INFO = 0, the first M columns of Z
* contain the orthonormal eigenvectors of the matrix A
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* If an eigenvector fails to converge, then that column of Z
* contains the latest approximation to the eigenvector, and the
* index of the eigenvector is returned in IFAIL.
* If JOBZ = 'N', then Z is not referenced.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of the array WORK. LWORK >= 1, when N <= 1;
* otherwise 8*N.
* For optimal efficiency, LWORK >= (NB+3)*N,
* where NB is the max of the blocksize for SSYTRD and SORMTR
* returned by ILAENV.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (5*N)
*
* IFAIL (output) INTEGER array, dimension (N)
* If JOBZ = 'V', then if INFO = 0, the first M elements of
* IFAIL are zero. If INFO > 0, then IFAIL contains the
* indices of the eigenvectors that failed to converge.
* If JOBZ = 'N', then IFAIL is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, then i eigenvectors failed to converge.
* Their indices are stored in array IFAIL.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobz
* @param range
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param ifail
* @param _ifail_offset
* @param info
*
*/
abstract public void ssyevx(java.lang.String jobz, java.lang.String range, java.lang.String uplo, int n, float[] a, int _a_offset, int lda, float vl, float vu, int il, int iu, float abstol, org.netlib.util.intW m, float[] w, int _w_offset, float[] z, int _z_offset, int ldz, float[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int[] ifail, int _ifail_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYGS2 reduces a real symmetric-definite generalized eigenproblem
* to standard form.
*
* If ITYPE = 1, the problem is A*x = lambda*B*x,
* and A is overwritten by inv(U')*A*inv(U) or inv(L)*A*inv(L')
*
* If ITYPE = 2 or 3, the problem is A*B*x = lambda*x or
* B*A*x = lambda*x, and A is overwritten by U*A*U` or L'*A*L.
*
* B must have been previously factorized as U'*U or L*L' by SPOTRF.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* = 1: compute inv(U')*A*inv(U) or inv(L)*A*inv(L');
* = 2 or 3: compute U*A*U' or L'*A*L.
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored, and how B has been factorized.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* n by n upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading n by n lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, if INFO = 0, the transformed matrix, stored in the
* same format as A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input) REAL array, dimension (LDB,N)
* The triangular factor from the Cholesky factorization of B,
* as returned by SPOTRF.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param itype
* @param uplo
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param info
*
*/
abstract public void ssygs2(int itype, java.lang.String uplo, int n, float[] a, int lda, float[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYGS2 reduces a real symmetric-definite generalized eigenproblem
* to standard form.
*
* If ITYPE = 1, the problem is A*x = lambda*B*x,
* and A is overwritten by inv(U')*A*inv(U) or inv(L)*A*inv(L')
*
* If ITYPE = 2 or 3, the problem is A*B*x = lambda*x or
* B*A*x = lambda*x, and A is overwritten by U*A*U` or L'*A*L.
*
* B must have been previously factorized as U'*U or L*L' by SPOTRF.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* = 1: compute inv(U')*A*inv(U) or inv(L)*A*inv(L');
* = 2 or 3: compute U*A*U' or L'*A*L.
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored, and how B has been factorized.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* n by n upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading n by n lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, if INFO = 0, the transformed matrix, stored in the
* same format as A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input) REAL array, dimension (LDB,N)
* The triangular factor from the Cholesky factorization of B,
* as returned by SPOTRF.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param itype
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void ssygs2(int itype, java.lang.String uplo, int n, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYGST reduces a real symmetric-definite generalized eigenproblem
* to standard form.
*
* If ITYPE = 1, the problem is A*x = lambda*B*x,
* and A is overwritten by inv(U**T)*A*inv(U) or inv(L)*A*inv(L**T)
*
* If ITYPE = 2 or 3, the problem is A*B*x = lambda*x or
* B*A*x = lambda*x, and A is overwritten by U*A*U**T or L**T*A*L.
*
* B must have been previously factorized as U**T*U or L*L**T by SPOTRF.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* = 1: compute inv(U**T)*A*inv(U) or inv(L)*A*inv(L**T);
* = 2 or 3: compute U*A*U**T or L**T*A*L.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored and B is factored as
* U**T*U;
* = 'L': Lower triangle of A is stored and B is factored as
* L*L**T.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* N-by-N upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading N-by-N lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, if INFO = 0, the transformed matrix, stored in the
* same format as A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input) REAL array, dimension (LDB,N)
* The triangular factor from the Cholesky factorization of B,
* as returned by SPOTRF.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param itype
* @param uplo
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param info
*
*/
abstract public void ssygst(int itype, java.lang.String uplo, int n, float[] a, int lda, float[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYGST reduces a real symmetric-definite generalized eigenproblem
* to standard form.
*
* If ITYPE = 1, the problem is A*x = lambda*B*x,
* and A is overwritten by inv(U**T)*A*inv(U) or inv(L)*A*inv(L**T)
*
* If ITYPE = 2 or 3, the problem is A*B*x = lambda*x or
* B*A*x = lambda*x, and A is overwritten by U*A*U**T or L**T*A*L.
*
* B must have been previously factorized as U**T*U or L*L**T by SPOTRF.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* = 1: compute inv(U**T)*A*inv(U) or inv(L)*A*inv(L**T);
* = 2 or 3: compute U*A*U**T or L**T*A*L.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored and B is factored as
* U**T*U;
* = 'L': Lower triangle of A is stored and B is factored as
* L*L**T.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* N-by-N upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading N-by-N lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, if INFO = 0, the transformed matrix, stored in the
* same format as A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input) REAL array, dimension (LDB,N)
* The triangular factor from the Cholesky factorization of B,
* as returned by SPOTRF.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param itype
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void ssygst(int itype, java.lang.String uplo, int n, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYGV computes all the eigenvalues, and optionally, the eigenvectors
* of a real generalized symmetric-definite eigenproblem, of the form
* A*x=(lambda)*B*x, A*Bx=(lambda)*x, or B*A*x=(lambda)*x.
* Here A and B are assumed to be symmetric and B is also
* positive definite.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* Specifies the problem type to be solved:
* = 1: A*x = (lambda)*B*x
* = 2: A*B*x = (lambda)*x
* = 3: B*A*x = (lambda)*x
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangles of A and B are stored;
* = 'L': Lower triangles of A and B are stored.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* A (input/output) REAL array, dimension (LDA, N)
* On entry, the symmetric matrix A. If UPLO = 'U', the
* leading N-by-N upper triangular part of A contains the
* upper triangular part of the matrix A. If UPLO = 'L',
* the leading N-by-N lower triangular part of A contains
* the lower triangular part of the matrix A.
*
* On exit, if JOBZ = 'V', then if INFO = 0, A contains the
* matrix Z of eigenvectors. The eigenvectors are normalized
* as follows:
* if ITYPE = 1 or 2, Z**T*B*Z = I;
* if ITYPE = 3, Z**T*inv(B)*Z = I.
* If JOBZ = 'N', then on exit the upper triangle (if UPLO='U')
* or the lower triangle (if UPLO='L') of A, including the
* diagonal, is destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension (LDB, N)
* On entry, the symmetric positive definite matrix B.
* If UPLO = 'U', the leading N-by-N upper triangular part of B
* contains the upper triangular part of the matrix B.
* If UPLO = 'L', the leading N-by-N lower triangular part of B
* contains the lower triangular part of the matrix B.
*
* On exit, if INFO <= N, the part of B containing the matrix is
* overwritten by the triangular factor U or L from the Cholesky
* factorization B = U**T*U or B = L*L**T.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* W (output) REAL array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of the array WORK. LWORK >= max(1,3*N-1).
* For optimal efficiency, LWORK >= (NB+2)*N,
* where NB is the blocksize for SSYTRD returned by ILAENV.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: SPOTRF or SSYEV returned an error code:
* <= N: if INFO = i, SSYEV failed to converge;
* i off-diagonal elements of an intermediate
* tridiagonal form did not converge to zero;
* > N: if INFO = N + i, for 1 <= i <= N, then the leading
* minor of order i of B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param itype
* @param jobz
* @param uplo
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param w
* @param work
* @param lwork
* @param info
*
*/
abstract public void ssygv(int itype, java.lang.String jobz, java.lang.String uplo, int n, float[] a, int lda, float[] b, int ldb, float[] w, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYGV computes all the eigenvalues, and optionally, the eigenvectors
* of a real generalized symmetric-definite eigenproblem, of the form
* A*x=(lambda)*B*x, A*Bx=(lambda)*x, or B*A*x=(lambda)*x.
* Here A and B are assumed to be symmetric and B is also
* positive definite.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* Specifies the problem type to be solved:
* = 1: A*x = (lambda)*B*x
* = 2: A*B*x = (lambda)*x
* = 3: B*A*x = (lambda)*x
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangles of A and B are stored;
* = 'L': Lower triangles of A and B are stored.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* A (input/output) REAL array, dimension (LDA, N)
* On entry, the symmetric matrix A. If UPLO = 'U', the
* leading N-by-N upper triangular part of A contains the
* upper triangular part of the matrix A. If UPLO = 'L',
* the leading N-by-N lower triangular part of A contains
* the lower triangular part of the matrix A.
*
* On exit, if JOBZ = 'V', then if INFO = 0, A contains the
* matrix Z of eigenvectors. The eigenvectors are normalized
* as follows:
* if ITYPE = 1 or 2, Z**T*B*Z = I;
* if ITYPE = 3, Z**T*inv(B)*Z = I.
* If JOBZ = 'N', then on exit the upper triangle (if UPLO='U')
* or the lower triangle (if UPLO='L') of A, including the
* diagonal, is destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension (LDB, N)
* On entry, the symmetric positive definite matrix B.
* If UPLO = 'U', the leading N-by-N upper triangular part of B
* contains the upper triangular part of the matrix B.
* If UPLO = 'L', the leading N-by-N lower triangular part of B
* contains the lower triangular part of the matrix B.
*
* On exit, if INFO <= N, the part of B containing the matrix is
* overwritten by the triangular factor U or L from the Cholesky
* factorization B = U**T*U or B = L*L**T.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* W (output) REAL array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of the array WORK. LWORK >= max(1,3*N-1).
* For optimal efficiency, LWORK >= (NB+2)*N,
* where NB is the blocksize for SSYTRD returned by ILAENV.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: SPOTRF or SSYEV returned an error code:
* <= N: if INFO = i, SSYEV failed to converge;
* i off-diagonal elements of an intermediate
* tridiagonal form did not converge to zero;
* > N: if INFO = N + i, for 1 <= i <= N, then the leading
* minor of order i of B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param itype
* @param jobz
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param w
* @param _w_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void ssygv(int itype, java.lang.String jobz, java.lang.String uplo, int n, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, float[] w, int _w_offset, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYGVD computes all the eigenvalues, and optionally, the eigenvectors
* of a real generalized symmetric-definite eigenproblem, of the form
* A*x=(lambda)*B*x, A*Bx=(lambda)*x, or B*A*x=(lambda)*x. Here A and
* B are assumed to be symmetric and B is also positive definite.
* If eigenvectors are desired, it uses a divide and conquer algorithm.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* Specifies the problem type to be solved:
* = 1: A*x = (lambda)*B*x
* = 2: A*B*x = (lambda)*x
* = 3: B*A*x = (lambda)*x
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangles of A and B are stored;
* = 'L': Lower triangles of A and B are stored.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* A (input/output) REAL array, dimension (LDA, N)
* On entry, the symmetric matrix A. If UPLO = 'U', the
* leading N-by-N upper triangular part of A contains the
* upper triangular part of the matrix A. If UPLO = 'L',
* the leading N-by-N lower triangular part of A contains
* the lower triangular part of the matrix A.
*
* On exit, if JOBZ = 'V', then if INFO = 0, A contains the
* matrix Z of eigenvectors. The eigenvectors are normalized
* as follows:
* if ITYPE = 1 or 2, Z**T*B*Z = I;
* if ITYPE = 3, Z**T*inv(B)*Z = I.
* If JOBZ = 'N', then on exit the upper triangle (if UPLO='U')
* or the lower triangle (if UPLO='L') of A, including the
* diagonal, is destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension (LDB, N)
* On entry, the symmetric matrix B. If UPLO = 'U', the
* leading N-by-N upper triangular part of B contains the
* upper triangular part of the matrix B. If UPLO = 'L',
* the leading N-by-N lower triangular part of B contains
* the lower triangular part of the matrix B.
*
* On exit, if INFO <= N, the part of B containing the matrix is
* overwritten by the triangular factor U or L from the Cholesky
* factorization B = U**T*U or B = L*L**T.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* W (output) REAL array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If N <= 1, LWORK >= 1.
* If JOBZ = 'N' and N > 1, LWORK >= 2*N+1.
* If JOBZ = 'V' and N > 1, LWORK >= 1 + 6*N + 2*N**2.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If N <= 1, LIWORK >= 1.
* If JOBZ = 'N' and N > 1, LIWORK >= 1.
* If JOBZ = 'V' and N > 1, LIWORK >= 3 + 5*N.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: SPOTRF or SSYEVD returned an error code:
* <= N: if INFO = i and JOBZ = 'N', then the algorithm
* failed to converge; i off-diagonal elements of an
* intermediate tridiagonal form did not converge to
* zero;
* if INFO = i and JOBZ = 'V', then the algorithm
* failed to compute an eigenvalue while working on
* the submatrix lying in rows and columns INFO/(N+1)
* through mod(INFO,N+1);
* > N: if INFO = N + i, for 1 <= i <= N, then the leading
* minor of order i of B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* Further Details
* ===============
*
* Based on contributions by
* Mark Fahey, Department of Mathematics, Univ. of Kentucky, USA
*
* Modified so that no backsubstitution is performed if SSYEVD fails to
* converge (NEIG in old code could be greater than N causing out of
* bounds reference to A - reported by Ralf Meyer). Also corrected the
* description of INFO and the test on ITYPE. Sven, 16 Feb 05.
* =====================================================================
*
* .. Parameters ..
*
*
* @param itype
* @param jobz
* @param uplo
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param w
* @param work
* @param lwork
* @param iwork
* @param liwork
* @param info
*
*/
abstract public void ssygvd(int itype, java.lang.String jobz, java.lang.String uplo, int n, float[] a, int lda, float[] b, int ldb, float[] w, float[] work, int lwork, int[] iwork, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYGVD computes all the eigenvalues, and optionally, the eigenvectors
* of a real generalized symmetric-definite eigenproblem, of the form
* A*x=(lambda)*B*x, A*Bx=(lambda)*x, or B*A*x=(lambda)*x. Here A and
* B are assumed to be symmetric and B is also positive definite.
* If eigenvectors are desired, it uses a divide and conquer algorithm.
*
* The divide and conquer algorithm makes very mild assumptions about
* floating point arithmetic. It will work on machines with a guard
* digit in add/subtract, or on those binary machines without guard
* digits which subtract like the Cray X-MP, Cray Y-MP, Cray C-90, or
* Cray-2. It could conceivably fail on hexadecimal or decimal machines
* without guard digits, but we know of none.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* Specifies the problem type to be solved:
* = 1: A*x = (lambda)*B*x
* = 2: A*B*x = (lambda)*x
* = 3: B*A*x = (lambda)*x
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangles of A and B are stored;
* = 'L': Lower triangles of A and B are stored.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* A (input/output) REAL array, dimension (LDA, N)
* On entry, the symmetric matrix A. If UPLO = 'U', the
* leading N-by-N upper triangular part of A contains the
* upper triangular part of the matrix A. If UPLO = 'L',
* the leading N-by-N lower triangular part of A contains
* the lower triangular part of the matrix A.
*
* On exit, if JOBZ = 'V', then if INFO = 0, A contains the
* matrix Z of eigenvectors. The eigenvectors are normalized
* as follows:
* if ITYPE = 1 or 2, Z**T*B*Z = I;
* if ITYPE = 3, Z**T*inv(B)*Z = I.
* If JOBZ = 'N', then on exit the upper triangle (if UPLO='U')
* or the lower triangle (if UPLO='L') of A, including the
* diagonal, is destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension (LDB, N)
* On entry, the symmetric matrix B. If UPLO = 'U', the
* leading N-by-N upper triangular part of B contains the
* upper triangular part of the matrix B. If UPLO = 'L',
* the leading N-by-N lower triangular part of B contains
* the lower triangular part of the matrix B.
*
* On exit, if INFO <= N, the part of B containing the matrix is
* overwritten by the triangular factor U or L from the Cholesky
* factorization B = U**T*U or B = L*L**T.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* W (output) REAL array, dimension (N)
* If INFO = 0, the eigenvalues in ascending order.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If N <= 1, LWORK >= 1.
* If JOBZ = 'N' and N > 1, LWORK >= 2*N+1.
* If JOBZ = 'V' and N > 1, LWORK >= 1 + 6*N + 2*N**2.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal sizes of the WORK and IWORK
* arrays, returns these values as the first entries of the WORK
* and IWORK arrays, and no error message related to LWORK or
* LIWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If N <= 1, LIWORK >= 1.
* If JOBZ = 'N' and N > 1, LIWORK >= 1.
* If JOBZ = 'V' and N > 1, LIWORK >= 3 + 5*N.
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal sizes of the WORK and
* IWORK arrays, returns these values as the first entries of
* the WORK and IWORK arrays, and no error message related to
* LWORK or LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: SPOTRF or SSYEVD returned an error code:
* <= N: if INFO = i and JOBZ = 'N', then the algorithm
* failed to converge; i off-diagonal elements of an
* intermediate tridiagonal form did not converge to
* zero;
* if INFO = i and JOBZ = 'V', then the algorithm
* failed to compute an eigenvalue while working on
* the submatrix lying in rows and columns INFO/(N+1)
* through mod(INFO,N+1);
* > N: if INFO = N + i, for 1 <= i <= N, then the leading
* minor of order i of B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* Further Details
* ===============
*
* Based on contributions by
* Mark Fahey, Department of Mathematics, Univ. of Kentucky, USA
*
* Modified so that no backsubstitution is performed if SSYEVD fails to
* converge (NEIG in old code could be greater than N causing out of
* bounds reference to A - reported by Ralf Meyer). Also corrected the
* description of INFO and the test on ITYPE. Sven, 16 Feb 05.
* =====================================================================
*
* .. Parameters ..
*
*
* @param itype
* @param jobz
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param w
* @param _w_offset
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param liwork
* @param info
*
*/
abstract public void ssygvd(int itype, java.lang.String jobz, java.lang.String uplo, int n, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, float[] w, int _w_offset, float[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYGVX computes selected eigenvalues, and optionally, eigenvectors
* of a real generalized symmetric-definite eigenproblem, of the form
* A*x=(lambda)*B*x, A*Bx=(lambda)*x, or B*A*x=(lambda)*x. Here A
* and B are assumed to be symmetric and B is also positive definite.
* Eigenvalues and eigenvectors can be selected by specifying either a
* range of values or a range of indices for the desired eigenvalues.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* Specifies the problem type to be solved:
* = 1: A*x = (lambda)*B*x
* = 2: A*B*x = (lambda)*x
* = 3: B*A*x = (lambda)*x
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A and B are stored;
* = 'L': Lower triangle of A and B are stored.
*
* N (input) INTEGER
* The order of the matrix pencil (A,B). N >= 0.
*
* A (input/output) REAL array, dimension (LDA, N)
* On entry, the symmetric matrix A. If UPLO = 'U', the
* leading N-by-N upper triangular part of A contains the
* upper triangular part of the matrix A. If UPLO = 'L',
* the leading N-by-N lower triangular part of A contains
* the lower triangular part of the matrix A.
*
* On exit, the lower triangle (if UPLO='L') or the upper
* triangle (if UPLO='U') of A, including the diagonal, is
* destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension (LDA, N)
* On entry, the symmetric matrix B. If UPLO = 'U', the
* leading N-by-N upper triangular part of B contains the
* upper triangular part of the matrix B. If UPLO = 'L',
* the leading N-by-N lower triangular part of B contains
* the lower triangular part of the matrix B.
*
* On exit, if INFO <= N, the part of B containing the matrix is
* overwritten by the triangular factor U or L from the Cholesky
* factorization B = U**T*U or B = L*L**T.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* VL (input) REAL
* VU (input) REAL
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) REAL
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less than
* or equal to zero, then EPS*|T| will be used in its place,
* where |T| is the 1-norm of the tridiagonal matrix obtained
* by reducing A to tridiagonal form.
*
* Eigenvalues will be computed most accurately when ABSTOL is
* set to twice the underflow threshold 2*DLAMCH('S'), not zero.
* If this routine returns with INFO>0, indicating that some
* eigenvectors did not converge, try setting ABSTOL to
* 2*SLAMCH('S').
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) REAL array, dimension (N)
* On normal exit, the first M elements contain the selected
* eigenvalues in ascending order.
*
* Z (output) REAL array, dimension (LDZ, max(1,M))
* If JOBZ = 'N', then Z is not referenced.
* If JOBZ = 'V', then if INFO = 0, the first M columns of Z
* contain the orthonormal eigenvectors of the matrix A
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* The eigenvectors are normalized as follows:
* if ITYPE = 1 or 2, Z**T*B*Z = I;
* if ITYPE = 3, Z**T*inv(B)*Z = I.
*
* If an eigenvector fails to converge, then that column of Z
* contains the latest approximation to the eigenvector, and the
* index of the eigenvector is returned in IFAIL.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of the array WORK. LWORK >= max(1,8*N).
* For optimal efficiency, LWORK >= (NB+3)*N,
* where NB is the blocksize for SSYTRD returned by ILAENV.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (5*N)
*
* IFAIL (output) INTEGER array, dimension (N)
* If JOBZ = 'V', then if INFO = 0, the first M elements of
* IFAIL are zero. If INFO > 0, then IFAIL contains the
* indices of the eigenvectors that failed to converge.
* If JOBZ = 'N', then IFAIL is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: SPOTRF or SSYEVX returned an error code:
* <= N: if INFO = i, SSYEVX failed to converge;
* i eigenvectors failed to converge. Their indices
* are stored in array IFAIL.
* > N: if INFO = N + i, for 1 <= i <= N, then the leading
* minor of order i of B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* Further Details
* ===============
*
* Based on contributions by
* Mark Fahey, Department of Mathematics, Univ. of Kentucky, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param itype
* @param jobz
* @param range
* @param uplo
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param z
* @param ldz
* @param work
* @param lwork
* @param iwork
* @param ifail
* @param info
*
*/
abstract public void ssygvx(int itype, java.lang.String jobz, java.lang.String range, java.lang.String uplo, int n, float[] a, int lda, float[] b, int ldb, float vl, float vu, int il, int iu, float abstol, org.netlib.util.intW m, float[] w, float[] z, int ldz, float[] work, int lwork, int[] iwork, int[] ifail, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYGVX computes selected eigenvalues, and optionally, eigenvectors
* of a real generalized symmetric-definite eigenproblem, of the form
* A*x=(lambda)*B*x, A*Bx=(lambda)*x, or B*A*x=(lambda)*x. Here A
* and B are assumed to be symmetric and B is also positive definite.
* Eigenvalues and eigenvectors can be selected by specifying either a
* range of values or a range of indices for the desired eigenvalues.
*
* Arguments
* =========
*
* ITYPE (input) INTEGER
* Specifies the problem type to be solved:
* = 1: A*x = (lambda)*B*x
* = 2: A*B*x = (lambda)*x
* = 3: B*A*x = (lambda)*x
*
* JOBZ (input) CHARACTER*1
* = 'N': Compute eigenvalues only;
* = 'V': Compute eigenvalues and eigenvectors.
*
* RANGE (input) CHARACTER*1
* = 'A': all eigenvalues will be found.
* = 'V': all eigenvalues in the half-open interval (VL,VU]
* will be found.
* = 'I': the IL-th through IU-th eigenvalues will be found.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A and B are stored;
* = 'L': Lower triangle of A and B are stored.
*
* N (input) INTEGER
* The order of the matrix pencil (A,B). N >= 0.
*
* A (input/output) REAL array, dimension (LDA, N)
* On entry, the symmetric matrix A. If UPLO = 'U', the
* leading N-by-N upper triangular part of A contains the
* upper triangular part of the matrix A. If UPLO = 'L',
* the leading N-by-N lower triangular part of A contains
* the lower triangular part of the matrix A.
*
* On exit, the lower triangle (if UPLO='L') or the upper
* triangle (if UPLO='U') of A, including the diagonal, is
* destroyed.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension (LDA, N)
* On entry, the symmetric matrix B. If UPLO = 'U', the
* leading N-by-N upper triangular part of B contains the
* upper triangular part of the matrix B. If UPLO = 'L',
* the leading N-by-N lower triangular part of B contains
* the lower triangular part of the matrix B.
*
* On exit, if INFO <= N, the part of B containing the matrix is
* overwritten by the triangular factor U or L from the Cholesky
* factorization B = U**T*U or B = L*L**T.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* VL (input) REAL
* VU (input) REAL
* If RANGE='V', the lower and upper bounds of the interval to
* be searched for eigenvalues. VL < VU.
* Not referenced if RANGE = 'A' or 'I'.
*
* IL (input) INTEGER
* IU (input) INTEGER
* If RANGE='I', the indices (in ascending order) of the
* smallest and largest eigenvalues to be returned.
* 1 <= IL <= IU <= N, if N > 0; IL = 1 and IU = 0 if N = 0.
* Not referenced if RANGE = 'A' or 'V'.
*
* ABSTOL (input) REAL
* The absolute error tolerance for the eigenvalues.
* An approximate eigenvalue is accepted as converged
* when it is determined to lie in an interval [a,b]
* of width less than or equal to
*
* ABSTOL + EPS * max( |a|,|b| ) ,
*
* where EPS is the machine precision. If ABSTOL is less than
* or equal to zero, then EPS*|T| will be used in its place,
* where |T| is the 1-norm of the tridiagonal matrix obtained
* by reducing A to tridiagonal form.
*
* Eigenvalues will be computed most accurately when ABSTOL is
* set to twice the underflow threshold 2*DLAMCH('S'), not zero.
* If this routine returns with INFO>0, indicating that some
* eigenvectors did not converge, try setting ABSTOL to
* 2*SLAMCH('S').
*
* M (output) INTEGER
* The total number of eigenvalues found. 0 <= M <= N.
* If RANGE = 'A', M = N, and if RANGE = 'I', M = IU-IL+1.
*
* W (output) REAL array, dimension (N)
* On normal exit, the first M elements contain the selected
* eigenvalues in ascending order.
*
* Z (output) REAL array, dimension (LDZ, max(1,M))
* If JOBZ = 'N', then Z is not referenced.
* If JOBZ = 'V', then if INFO = 0, the first M columns of Z
* contain the orthonormal eigenvectors of the matrix A
* corresponding to the selected eigenvalues, with the i-th
* column of Z holding the eigenvector associated with W(i).
* The eigenvectors are normalized as follows:
* if ITYPE = 1 or 2, Z**T*B*Z = I;
* if ITYPE = 3, Z**T*inv(B)*Z = I.
*
* If an eigenvector fails to converge, then that column of Z
* contains the latest approximation to the eigenvector, and the
* index of the eigenvector is returned in IFAIL.
* Note: the user must ensure that at least max(1,M) columns are
* supplied in the array Z; if RANGE = 'V', the exact value of M
* is not known in advance and an upper bound must be used.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1, and if
* JOBZ = 'V', LDZ >= max(1,N).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of the array WORK. LWORK >= max(1,8*N).
* For optimal efficiency, LWORK >= (NB+3)*N,
* where NB is the blocksize for SSYTRD returned by ILAENV.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (5*N)
*
* IFAIL (output) INTEGER array, dimension (N)
* If JOBZ = 'V', then if INFO = 0, the first M elements of
* IFAIL are zero. If INFO > 0, then IFAIL contains the
* indices of the eigenvectors that failed to converge.
* If JOBZ = 'N', then IFAIL is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: SPOTRF or SSYEVX returned an error code:
* <= N: if INFO = i, SSYEVX failed to converge;
* i eigenvectors failed to converge. Their indices
* are stored in array IFAIL.
* > N: if INFO = N + i, for 1 <= i <= N, then the leading
* minor of order i of B is not positive definite.
* The factorization of B could not be completed and
* no eigenvalues or eigenvectors were computed.
*
* Further Details
* ===============
*
* Based on contributions by
* Mark Fahey, Department of Mathematics, Univ. of Kentucky, USA
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param itype
* @param jobz
* @param range
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param vl
* @param vu
* @param il
* @param iu
* @param abstol
* @param m
* @param w
* @param _w_offset
* @param z
* @param _z_offset
* @param ldz
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param ifail
* @param _ifail_offset
* @param info
*
*/
abstract public void ssygvx(int itype, java.lang.String jobz, java.lang.String range, java.lang.String uplo, int n, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, float vl, float vu, int il, int iu, float abstol, org.netlib.util.intW m, float[] w, int _w_offset, float[] z, int _z_offset, int ldz, float[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int[] ifail, int _ifail_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYRFS improves the computed solution to a system of linear
* equations when the coefficient matrix is symmetric indefinite, and
* provides error bounds and backward error estimates for the solution.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The symmetric matrix A. If UPLO = 'U', the leading N-by-N
* upper triangular part of A contains the upper triangular part
* of the matrix A, and the strictly lower triangular part of A
* is not referenced. If UPLO = 'L', the leading N-by-N lower
* triangular part of A contains the lower triangular part of
* the matrix A, and the strictly upper triangular part of A is
* not referenced.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* AF (input) REAL array, dimension (LDAF,N)
* The factored form of the matrix A. AF contains the block
* diagonal matrix D and the multipliers used to obtain the
* factor U or L from the factorization A = U*D*U**T or
* A = L*D*L**T as computed by SSYTRF.
*
* LDAF (input) INTEGER
* The leading dimension of the array AF. LDAF >= max(1,N).
*
* IPIV (input) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D
* as determined by SSYTRF.
*
* B (input) REAL array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) REAL array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by SSYTRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param a
* @param lda
* @param af
* @param ldaf
* @param ipiv
* @param b
* @param ldb
* @param x
* @param ldx
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void ssyrfs(java.lang.String uplo, int n, int nrhs, float[] a, int lda, float[] af, int ldaf, int[] ipiv, float[] b, int ldb, float[] x, int ldx, float[] ferr, float[] berr, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYRFS improves the computed solution to a system of linear
* equations when the coefficient matrix is symmetric indefinite, and
* provides error bounds and backward error estimates for the solution.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The symmetric matrix A. If UPLO = 'U', the leading N-by-N
* upper triangular part of A contains the upper triangular part
* of the matrix A, and the strictly lower triangular part of A
* is not referenced. If UPLO = 'L', the leading N-by-N lower
* triangular part of A contains the lower triangular part of
* the matrix A, and the strictly upper triangular part of A is
* not referenced.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* AF (input) REAL array, dimension (LDAF,N)
* The factored form of the matrix A. AF contains the block
* diagonal matrix D and the multipliers used to obtain the
* factor U or L from the factorization A = U*D*U**T or
* A = L*D*L**T as computed by SSYTRF.
*
* LDAF (input) INTEGER
* The leading dimension of the array AF. LDAF >= max(1,N).
*
* IPIV (input) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D
* as determined by SSYTRF.
*
* B (input) REAL array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input/output) REAL array, dimension (LDX,NRHS)
* On entry, the solution matrix X, as computed by SSYTRS.
* On exit, the improved solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Internal Parameters
* ===================
*
* ITMAX is the maximum number of steps of iterative refinement.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param af
* @param _af_offset
* @param ldaf
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void ssyrfs(java.lang.String uplo, int n, int nrhs, float[] a, int _a_offset, int lda, float[] af, int _af_offset, int ldaf, int[] ipiv, int _ipiv_offset, float[] b, int _b_offset, int ldb, float[] x, int _x_offset, int ldx, float[] ferr, int _ferr_offset, float[] berr, int _berr_offset, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYSV computes the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N symmetric matrix and X and B are N-by-NRHS
* matrices.
*
* The diagonal pivoting method is used to factor A as
* A = U * D * U**T, if UPLO = 'U', or
* A = L * D * L**T, if UPLO = 'L',
* where U (or L) is a product of permutation and unit upper (lower)
* triangular matrices, and D is symmetric and block diagonal with
* 1-by-1 and 2-by-2 diagonal blocks. The factored form of A is then
* used to solve the system of equations A * X = B.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* N-by-N upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading N-by-N lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, if INFO = 0, the block diagonal matrix D and the
* multipliers used to obtain the factor U or L from the
* factorization A = U*D*U**T or A = L*D*L**T as computed by
* SSYTRF.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (output) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D, as
* determined by SSYTRF. If IPIV(k) > 0, then rows and columns
* k and IPIV(k) were interchanged, and D(k,k) is a 1-by-1
* diagonal block. If UPLO = 'U' and IPIV(k) = IPIV(k-1) < 0,
* then rows and columns k-1 and -IPIV(k) were interchanged and
* D(k-1:k,k-1:k) is a 2-by-2 diagonal block. If UPLO = 'L' and
* IPIV(k) = IPIV(k+1) < 0, then rows and columns k+1 and
* -IPIV(k) were interchanged and D(k:k+1,k:k+1) is a 2-by-2
* diagonal block.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if INFO = 0, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of WORK. LWORK >= 1, and for best performance
* LWORK >= max(1,N*NB), where NB is the optimal blocksize for
* SSYTRF.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, D(i,i) is exactly zero. The factorization
* has been completed, but the block diagonal matrix D is
* exactly singular, so the solution could not be computed.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param a
* @param lda
* @param ipiv
* @param b
* @param ldb
* @param work
* @param lwork
* @param info
*
*/
abstract public void ssysv(java.lang.String uplo, int n, int nrhs, float[] a, int lda, int[] ipiv, float[] b, int ldb, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYSV computes the solution to a real system of linear equations
* A * X = B,
* where A is an N-by-N symmetric matrix and X and B are N-by-NRHS
* matrices.
*
* The diagonal pivoting method is used to factor A as
* A = U * D * U**T, if UPLO = 'U', or
* A = L * D * L**T, if UPLO = 'L',
* where U (or L) is a product of permutation and unit upper (lower)
* triangular matrices, and D is symmetric and block diagonal with
* 1-by-1 and 2-by-2 diagonal blocks. The factored form of A is then
* used to solve the system of equations A * X = B.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* N-by-N upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading N-by-N lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, if INFO = 0, the block diagonal matrix D and the
* multipliers used to obtain the factor U or L from the
* factorization A = U*D*U**T or A = L*D*L**T as computed by
* SSYTRF.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (output) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D, as
* determined by SSYTRF. If IPIV(k) > 0, then rows and columns
* k and IPIV(k) were interchanged, and D(k,k) is a 1-by-1
* diagonal block. If UPLO = 'U' and IPIV(k) = IPIV(k-1) < 0,
* then rows and columns k-1 and -IPIV(k) were interchanged and
* D(k-1:k,k-1:k) is a 2-by-2 diagonal block. If UPLO = 'L' and
* IPIV(k) = IPIV(k+1) < 0, then rows and columns k+1 and
* -IPIV(k) were interchanged and D(k:k+1,k:k+1) is a 2-by-2
* diagonal block.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the N-by-NRHS right hand side matrix B.
* On exit, if INFO = 0, the N-by-NRHS solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of WORK. LWORK >= 1, and for best performance
* LWORK >= max(1,N*NB), where NB is the optimal blocksize for
* SSYTRF.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, D(i,i) is exactly zero. The factorization
* has been completed, but the block diagonal matrix D is
* exactly singular, so the solution could not be computed.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void ssysv(java.lang.String uplo, int n, int nrhs, float[] a, int _a_offset, int lda, int[] ipiv, int _ipiv_offset, float[] b, int _b_offset, int ldb, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYSVX uses the diagonal pivoting factorization to compute the
* solution to a real system of linear equations A * X = B,
* where A is an N-by-N symmetric matrix and X and B are N-by-NRHS
* matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed:
*
* 1. If FACT = 'N', the diagonal pivoting method is used to factor A.
* The form of the factorization is
* A = U * D * U**T, if UPLO = 'U', or
* A = L * D * L**T, if UPLO = 'L',
* where U (or L) is a product of permutation and unit upper (lower)
* triangular matrices, and D is symmetric and block diagonal with
* 1-by-1 and 2-by-2 diagonal blocks.
*
* 2. If some D(i,i)=0, so that D is exactly singular, then the routine
* returns with INFO = i. Otherwise, the factored form of A is used
* to estimate the condition number of the matrix A. If the
* reciprocal of the condition number is less than machine precision,
* INFO = N+1 is returned as a warning, but the routine still goes on
* to solve for X and compute error bounds as described below.
*
* 3. The system of equations is solved for X using the factored form
* of A.
*
* 4. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of A has been
* supplied on entry.
* = 'F': On entry, AF and IPIV contain the factored form of
* A. AF and IPIV will not be modified.
* = 'N': The matrix A will be copied to AF and factored.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The symmetric matrix A. If UPLO = 'U', the leading N-by-N
* upper triangular part of A contains the upper triangular part
* of the matrix A, and the strictly lower triangular part of A
* is not referenced. If UPLO = 'L', the leading N-by-N lower
* triangular part of A contains the lower triangular part of
* the matrix A, and the strictly upper triangular part of A is
* not referenced.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* AF (input or output) REAL array, dimension (LDAF,N)
* If FACT = 'F', then AF is an input argument and on entry
* contains the block diagonal matrix D and the multipliers used
* to obtain the factor U or L from the factorization
* A = U*D*U**T or A = L*D*L**T as computed by SSYTRF.
*
* If FACT = 'N', then AF is an output argument and on exit
* returns the block diagonal matrix D and the multipliers used
* to obtain the factor U or L from the factorization
* A = U*D*U**T or A = L*D*L**T.
*
* LDAF (input) INTEGER
* The leading dimension of the array AF. LDAF >= max(1,N).
*
* IPIV (input or output) INTEGER array, dimension (N)
* If FACT = 'F', then IPIV is an input argument and on entry
* contains details of the interchanges and the block structure
* of D, as determined by SSYTRF.
* If IPIV(k) > 0, then rows and columns k and IPIV(k) were
* interchanged and D(k,k) is a 1-by-1 diagonal block.
* If UPLO = 'U' and IPIV(k) = IPIV(k-1) < 0, then rows and
* columns k-1 and -IPIV(k) were interchanged and D(k-1:k,k-1:k)
* is a 2-by-2 diagonal block. If UPLO = 'L' and IPIV(k) =
* IPIV(k+1) < 0, then rows and columns k+1 and -IPIV(k) were
* interchanged and D(k:k+1,k:k+1) is a 2-by-2 diagonal block.
*
* If FACT = 'N', then IPIV is an output argument and on exit
* contains details of the interchanges and the block structure
* of D, as determined by SSYTRF.
*
* B (input) REAL array, dimension (LDB,NRHS)
* The N-by-NRHS right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) REAL array, dimension (LDX,NRHS)
* If INFO = 0 or INFO = N+1, the N-by-NRHS solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) REAL
* The estimate of the reciprocal condition number of the matrix
* A. If RCOND is less than the machine precision (in
* particular, if RCOND = 0), the matrix is singular to working
* precision. This condition is indicated by a return code of
* INFO > 0.
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of WORK. LWORK >= max(1,3*N), and for best
* performance, when FACT = 'N', LWORK >= max(1,3*N,N*NB), where
* NB is the optimal blocksize for SSYTRF.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= N: D(i,i) is exactly zero. The factorization
* has been completed but the factor D is exactly
* singular, so the solution and error bounds could
* not be computed. RCOND = 0 is returned.
* = N+1: D is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param uplo
* @param n
* @param nrhs
* @param a
* @param lda
* @param af
* @param ldaf
* @param ipiv
* @param b
* @param ldb
* @param x
* @param ldx
* @param rcond
* @param ferr
* @param berr
* @param work
* @param lwork
* @param iwork
* @param info
*
*/
abstract public void ssysvx(java.lang.String fact, java.lang.String uplo, int n, int nrhs, float[] a, int lda, float[] af, int ldaf, int[] ipiv, float[] b, int ldb, float[] x, int ldx, org.netlib.util.floatW rcond, float[] ferr, float[] berr, float[] work, int lwork, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYSVX uses the diagonal pivoting factorization to compute the
* solution to a real system of linear equations A * X = B,
* where A is an N-by-N symmetric matrix and X and B are N-by-NRHS
* matrices.
*
* Error bounds on the solution and a condition estimate are also
* provided.
*
* Description
* ===========
*
* The following steps are performed:
*
* 1. If FACT = 'N', the diagonal pivoting method is used to factor A.
* The form of the factorization is
* A = U * D * U**T, if UPLO = 'U', or
* A = L * D * L**T, if UPLO = 'L',
* where U (or L) is a product of permutation and unit upper (lower)
* triangular matrices, and D is symmetric and block diagonal with
* 1-by-1 and 2-by-2 diagonal blocks.
*
* 2. If some D(i,i)=0, so that D is exactly singular, then the routine
* returns with INFO = i. Otherwise, the factored form of A is used
* to estimate the condition number of the matrix A. If the
* reciprocal of the condition number is less than machine precision,
* INFO = N+1 is returned as a warning, but the routine still goes on
* to solve for X and compute error bounds as described below.
*
* 3. The system of equations is solved for X using the factored form
* of A.
*
* 4. Iterative refinement is applied to improve the computed solution
* matrix and calculate error bounds and backward error estimates
* for it.
*
* Arguments
* =========
*
* FACT (input) CHARACTER*1
* Specifies whether or not the factored form of A has been
* supplied on entry.
* = 'F': On entry, AF and IPIV contain the factored form of
* A. AF and IPIV will not be modified.
* = 'N': The matrix A will be copied to AF and factored.
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The number of linear equations, i.e., the order of the
* matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The symmetric matrix A. If UPLO = 'U', the leading N-by-N
* upper triangular part of A contains the upper triangular part
* of the matrix A, and the strictly lower triangular part of A
* is not referenced. If UPLO = 'L', the leading N-by-N lower
* triangular part of A contains the lower triangular part of
* the matrix A, and the strictly upper triangular part of A is
* not referenced.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* AF (input or output) REAL array, dimension (LDAF,N)
* If FACT = 'F', then AF is an input argument and on entry
* contains the block diagonal matrix D and the multipliers used
* to obtain the factor U or L from the factorization
* A = U*D*U**T or A = L*D*L**T as computed by SSYTRF.
*
* If FACT = 'N', then AF is an output argument and on exit
* returns the block diagonal matrix D and the multipliers used
* to obtain the factor U or L from the factorization
* A = U*D*U**T or A = L*D*L**T.
*
* LDAF (input) INTEGER
* The leading dimension of the array AF. LDAF >= max(1,N).
*
* IPIV (input or output) INTEGER array, dimension (N)
* If FACT = 'F', then IPIV is an input argument and on entry
* contains details of the interchanges and the block structure
* of D, as determined by SSYTRF.
* If IPIV(k) > 0, then rows and columns k and IPIV(k) were
* interchanged and D(k,k) is a 1-by-1 diagonal block.
* If UPLO = 'U' and IPIV(k) = IPIV(k-1) < 0, then rows and
* columns k-1 and -IPIV(k) were interchanged and D(k-1:k,k-1:k)
* is a 2-by-2 diagonal block. If UPLO = 'L' and IPIV(k) =
* IPIV(k+1) < 0, then rows and columns k+1 and -IPIV(k) were
* interchanged and D(k:k+1,k:k+1) is a 2-by-2 diagonal block.
*
* If FACT = 'N', then IPIV is an output argument and on exit
* contains details of the interchanges and the block structure
* of D, as determined by SSYTRF.
*
* B (input) REAL array, dimension (LDB,NRHS)
* The N-by-NRHS right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (output) REAL array, dimension (LDX,NRHS)
* If INFO = 0 or INFO = N+1, the N-by-NRHS solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* RCOND (output) REAL
* The estimate of the reciprocal condition number of the matrix
* A. If RCOND is less than the machine precision (in
* particular, if RCOND = 0), the matrix is singular to working
* precision. This condition is indicated by a return code of
* INFO > 0.
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of WORK. LWORK >= max(1,3*N), and for best
* performance, when FACT = 'N', LWORK >= max(1,3*N,N*NB), where
* NB is the optimal blocksize for SSYTRF.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, and i is
* <= N: D(i,i) is exactly zero. The factorization
* has been completed but the factor D is exactly
* singular, so the solution and error bounds could
* not be computed. RCOND = 0 is returned.
* = N+1: D is nonsingular, but RCOND is less than machine
* precision, meaning that the matrix is singular
* to working precision. Nevertheless, the
* solution and error bounds are computed because
* there are a number of situations where the
* computed solution can be more accurate than the
* value of RCOND would suggest.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param fact
* @param uplo
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param af
* @param _af_offset
* @param ldaf
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param rcond
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void ssysvx(java.lang.String fact, java.lang.String uplo, int n, int nrhs, float[] a, int _a_offset, int lda, float[] af, int _af_offset, int ldaf, int[] ipiv, int _ipiv_offset, float[] b, int _b_offset, int ldb, float[] x, int _x_offset, int ldx, org.netlib.util.floatW rcond, float[] ferr, int _ferr_offset, float[] berr, int _berr_offset, float[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYTD2 reduces a real symmetric matrix A to symmetric tridiagonal
* form T by an orthogonal similarity transformation: Q' * A * Q = T.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored:
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* n-by-n upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading n-by-n lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
* On exit, if UPLO = 'U', the diagonal and first superdiagonal
* of A are overwritten by the corresponding elements of the
* tridiagonal matrix T, and the elements above the first
* superdiagonal, with the array TAU, represent the orthogonal
* matrix Q as a product of elementary reflectors; if UPLO
* = 'L', the diagonal and first subdiagonal of A are over-
* written by the corresponding elements of the tridiagonal
* matrix T, and the elements below the first subdiagonal, with
* the array TAU, represent the orthogonal matrix Q as a product
* of elementary reflectors. See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* D (output) REAL array, dimension (N)
* The diagonal elements of the tridiagonal matrix T:
* D(i) = A(i,i).
*
* E (output) REAL array, dimension (N-1)
* The off-diagonal elements of the tridiagonal matrix T:
* E(i) = A(i,i+1) if UPLO = 'U', E(i) = A(i+1,i) if UPLO = 'L'.
*
* TAU (output) REAL array, dimension (N-1)
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* If UPLO = 'U', the matrix Q is represented as a product of elementary
* reflectors
*
* Q = H(n-1) . . . H(2) H(1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(i+1:n) = 0 and v(i) = 1; v(1:i-1) is stored on exit in
* A(1:i-1,i+1), and tau in TAU(i).
*
* If UPLO = 'L', the matrix Q is represented as a product of elementary
* reflectors
*
* Q = H(1) H(2) . . . H(n-1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i) = 0 and v(i+1) = 1; v(i+2:n) is stored on exit in A(i+2:n,i),
* and tau in TAU(i).
*
* The contents of A on exit are illustrated by the following examples
* with n = 5:
*
* if UPLO = 'U': if UPLO = 'L':
*
* ( d e v2 v3 v4 ) ( d )
* ( d e v3 v4 ) ( e d )
* ( d e v4 ) ( v1 e d )
* ( d e ) ( v1 v2 e d )
* ( d ) ( v1 v2 v3 e d )
*
* where d and e denote diagonal and off-diagonal elements of T, and vi
* denotes an element of the vector defining H(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param lda
* @param d
* @param e
* @param tau
* @param info
*
*/
abstract public void ssytd2(java.lang.String uplo, int n, float[] a, int lda, float[] d, float[] e, float[] tau, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYTD2 reduces a real symmetric matrix A to symmetric tridiagonal
* form T by an orthogonal similarity transformation: Q' * A * Q = T.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored:
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* n-by-n upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading n-by-n lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
* On exit, if UPLO = 'U', the diagonal and first superdiagonal
* of A are overwritten by the corresponding elements of the
* tridiagonal matrix T, and the elements above the first
* superdiagonal, with the array TAU, represent the orthogonal
* matrix Q as a product of elementary reflectors; if UPLO
* = 'L', the diagonal and first subdiagonal of A are over-
* written by the corresponding elements of the tridiagonal
* matrix T, and the elements below the first subdiagonal, with
* the array TAU, represent the orthogonal matrix Q as a product
* of elementary reflectors. See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* D (output) REAL array, dimension (N)
* The diagonal elements of the tridiagonal matrix T:
* D(i) = A(i,i).
*
* E (output) REAL array, dimension (N-1)
* The off-diagonal elements of the tridiagonal matrix T:
* E(i) = A(i,i+1) if UPLO = 'U', E(i) = A(i+1,i) if UPLO = 'L'.
*
* TAU (output) REAL array, dimension (N-1)
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
*
* Further Details
* ===============
*
* If UPLO = 'U', the matrix Q is represented as a product of elementary
* reflectors
*
* Q = H(n-1) . . . H(2) H(1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(i+1:n) = 0 and v(i) = 1; v(1:i-1) is stored on exit in
* A(1:i-1,i+1), and tau in TAU(i).
*
* If UPLO = 'L', the matrix Q is represented as a product of elementary
* reflectors
*
* Q = H(1) H(2) . . . H(n-1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i) = 0 and v(i+1) = 1; v(i+2:n) is stored on exit in A(i+2:n,i),
* and tau in TAU(i).
*
* The contents of A on exit are illustrated by the following examples
* with n = 5:
*
* if UPLO = 'U': if UPLO = 'L':
*
* ( d e v2 v3 v4 ) ( d )
* ( d e v3 v4 ) ( e d )
* ( d e v4 ) ( v1 e d )
* ( d e ) ( v1 v2 e d )
* ( d ) ( v1 v2 v3 e d )
*
* where d and e denote diagonal and off-diagonal elements of T, and vi
* denotes an element of the vector defining H(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param tau
* @param _tau_offset
* @param info
*
*/
abstract public void ssytd2(java.lang.String uplo, int n, float[] a, int _a_offset, int lda, float[] d, int _d_offset, float[] e, int _e_offset, float[] tau, int _tau_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYTF2 computes the factorization of a real symmetric matrix A using
* the Bunch-Kaufman diagonal pivoting method:
*
* A = U*D*U' or A = L*D*L'
*
* where U (or L) is a product of permutation and unit upper (lower)
* triangular matrices, U' is the transpose of U, and D is symmetric and
* block diagonal with 1-by-1 and 2-by-2 diagonal blocks.
*
* This is the unblocked version of the algorithm, calling Level 2 BLAS.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored:
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* n-by-n upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading n-by-n lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, the block diagonal matrix D and the multipliers used
* to obtain the factor U or L (see below for further details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (output) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D.
* If IPIV(k) > 0, then rows and columns k and IPIV(k) were
* interchanged and D(k,k) is a 1-by-1 diagonal block.
* If UPLO = 'U' and IPIV(k) = IPIV(k-1) < 0, then rows and
* columns k-1 and -IPIV(k) were interchanged and D(k-1:k,k-1:k)
* is a 2-by-2 diagonal block. If UPLO = 'L' and IPIV(k) =
* IPIV(k+1) < 0, then rows and columns k+1 and -IPIV(k) were
* interchanged and D(k:k+1,k:k+1) is a 2-by-2 diagonal block.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
* > 0: if INFO = k, D(k,k) is exactly zero. The factorization
* has been completed, but the block diagonal matrix D is
* exactly singular, and division by zero will occur if it
* is used to solve a system of equations.
*
* Further Details
* ===============
*
* 09-29-06 - patch from
* Bobby Cheng, MathWorks
*
* Replace l.204 and l.372
* IF( MAX( ABSAKK, COLMAX ).EQ.ZERO ) THEN
* by
* IF( (MAX( ABSAKK, COLMAX ).EQ.ZERO) .OR. SISNAN(ABSAKK) ) THEN
*
* 01-01-96 - Based on modifications by
* J. Lewis, Boeing Computer Services Company
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
* 1-96 - Based on modifications by J. Lewis, Boeing Computer Services
* Company
*
* If UPLO = 'U', then A = U*D*U', where
* U = P(n)*U(n)* ... *P(k)U(k)* ...,
* i.e., U is a product of terms P(k)*U(k), where k decreases from n to
* 1 in steps of 1 or 2, and D is a block diagonal matrix with 1-by-1
* and 2-by-2 diagonal blocks D(k). P(k) is a permutation matrix as
* defined by IPIV(k), and U(k) is a unit upper triangular matrix, such
* that if the diagonal block D(k) is of order s (s = 1 or 2), then
*
* ( I v 0 ) k-s
* U(k) = ( 0 I 0 ) s
* ( 0 0 I ) n-k
* k-s s n-k
*
* If s = 1, D(k) overwrites A(k,k), and v overwrites A(1:k-1,k).
* If s = 2, the upper triangle of D(k) overwrites A(k-1,k-1), A(k-1,k),
* and A(k,k), and v overwrites A(1:k-2,k-1:k).
*
* If UPLO = 'L', then A = L*D*L', where
* L = P(1)*L(1)* ... *P(k)*L(k)* ...,
* i.e., L is a product of terms P(k)*L(k), where k increases from 1 to
* n in steps of 1 or 2, and D is a block diagonal matrix with 1-by-1
* and 2-by-2 diagonal blocks D(k). P(k) is a permutation matrix as
* defined by IPIV(k), and L(k) is a unit lower triangular matrix, such
* that if the diagonal block D(k) is of order s (s = 1 or 2), then
*
* ( I 0 0 ) k-1
* L(k) = ( 0 I 0 ) s
* ( 0 v I ) n-k-s+1
* k-1 s n-k-s+1
*
* If s = 1, D(k) overwrites A(k,k), and v overwrites A(k+1:n,k).
* If s = 2, the lower triangle of D(k) overwrites A(k,k), A(k+1,k),
* and A(k+1,k+1), and v overwrites A(k+2:n,k:k+1).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param lda
* @param ipiv
* @param info
*
*/
abstract public void ssytf2(java.lang.String uplo, int n, float[] a, int lda, int[] ipiv, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYTF2 computes the factorization of a real symmetric matrix A using
* the Bunch-Kaufman diagonal pivoting method:
*
* A = U*D*U' or A = L*D*L'
*
* where U (or L) is a product of permutation and unit upper (lower)
* triangular matrices, U' is the transpose of U, and D is symmetric and
* block diagonal with 1-by-1 and 2-by-2 diagonal blocks.
*
* This is the unblocked version of the algorithm, calling Level 2 BLAS.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the upper or lower triangular part of the
* symmetric matrix A is stored:
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* n-by-n upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading n-by-n lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, the block diagonal matrix D and the multipliers used
* to obtain the factor U or L (see below for further details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (output) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D.
* If IPIV(k) > 0, then rows and columns k and IPIV(k) were
* interchanged and D(k,k) is a 1-by-1 diagonal block.
* If UPLO = 'U' and IPIV(k) = IPIV(k-1) < 0, then rows and
* columns k-1 and -IPIV(k) were interchanged and D(k-1:k,k-1:k)
* is a 2-by-2 diagonal block. If UPLO = 'L' and IPIV(k) =
* IPIV(k+1) < 0, then rows and columns k+1 and -IPIV(k) were
* interchanged and D(k:k+1,k:k+1) is a 2-by-2 diagonal block.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
* > 0: if INFO = k, D(k,k) is exactly zero. The factorization
* has been completed, but the block diagonal matrix D is
* exactly singular, and division by zero will occur if it
* is used to solve a system of equations.
*
* Further Details
* ===============
*
* 09-29-06 - patch from
* Bobby Cheng, MathWorks
*
* Replace l.204 and l.372
* IF( MAX( ABSAKK, COLMAX ).EQ.ZERO ) THEN
* by
* IF( (MAX( ABSAKK, COLMAX ).EQ.ZERO) .OR. SISNAN(ABSAKK) ) THEN
*
* 01-01-96 - Based on modifications by
* J. Lewis, Boeing Computer Services Company
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
* 1-96 - Based on modifications by J. Lewis, Boeing Computer Services
* Company
*
* If UPLO = 'U', then A = U*D*U', where
* U = P(n)*U(n)* ... *P(k)U(k)* ...,
* i.e., U is a product of terms P(k)*U(k), where k decreases from n to
* 1 in steps of 1 or 2, and D is a block diagonal matrix with 1-by-1
* and 2-by-2 diagonal blocks D(k). P(k) is a permutation matrix as
* defined by IPIV(k), and U(k) is a unit upper triangular matrix, such
* that if the diagonal block D(k) is of order s (s = 1 or 2), then
*
* ( I v 0 ) k-s
* U(k) = ( 0 I 0 ) s
* ( 0 0 I ) n-k
* k-s s n-k
*
* If s = 1, D(k) overwrites A(k,k), and v overwrites A(1:k-1,k).
* If s = 2, the upper triangle of D(k) overwrites A(k-1,k-1), A(k-1,k),
* and A(k,k), and v overwrites A(1:k-2,k-1:k).
*
* If UPLO = 'L', then A = L*D*L', where
* L = P(1)*L(1)* ... *P(k)*L(k)* ...,
* i.e., L is a product of terms P(k)*L(k), where k increases from 1 to
* n in steps of 1 or 2, and D is a block diagonal matrix with 1-by-1
* and 2-by-2 diagonal blocks D(k). P(k) is a permutation matrix as
* defined by IPIV(k), and L(k) is a unit lower triangular matrix, such
* that if the diagonal block D(k) is of order s (s = 1 or 2), then
*
* ( I 0 0 ) k-1
* L(k) = ( 0 I 0 ) s
* ( 0 v I ) n-k-s+1
* k-1 s n-k-s+1
*
* If s = 1, D(k) overwrites A(k,k), and v overwrites A(k+1:n,k).
* If s = 2, the lower triangle of D(k) overwrites A(k,k), A(k+1,k),
* and A(k+1,k+1), and v overwrites A(k+2:n,k:k+1).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param ipiv
* @param _ipiv_offset
* @param info
*
*/
abstract public void ssytf2(java.lang.String uplo, int n, float[] a, int _a_offset, int lda, int[] ipiv, int _ipiv_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYTRD reduces a real symmetric matrix A to real symmetric
* tridiagonal form T by an orthogonal similarity transformation:
* Q**T * A * Q = T.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* N-by-N upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading N-by-N lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
* On exit, if UPLO = 'U', the diagonal and first superdiagonal
* of A are overwritten by the corresponding elements of the
* tridiagonal matrix T, and the elements above the first
* superdiagonal, with the array TAU, represent the orthogonal
* matrix Q as a product of elementary reflectors; if UPLO
* = 'L', the diagonal and first subdiagonal of A are over-
* written by the corresponding elements of the tridiagonal
* matrix T, and the elements below the first subdiagonal, with
* the array TAU, represent the orthogonal matrix Q as a product
* of elementary reflectors. See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* D (output) REAL array, dimension (N)
* The diagonal elements of the tridiagonal matrix T:
* D(i) = A(i,i).
*
* E (output) REAL array, dimension (N-1)
* The off-diagonal elements of the tridiagonal matrix T:
* E(i) = A(i,i+1) if UPLO = 'U', E(i) = A(i+1,i) if UPLO = 'L'.
*
* TAU (output) REAL array, dimension (N-1)
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= 1.
* For optimum performance LWORK >= N*NB, where NB is the
* optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* If UPLO = 'U', the matrix Q is represented as a product of elementary
* reflectors
*
* Q = H(n-1) . . . H(2) H(1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(i+1:n) = 0 and v(i) = 1; v(1:i-1) is stored on exit in
* A(1:i-1,i+1), and tau in TAU(i).
*
* If UPLO = 'L', the matrix Q is represented as a product of elementary
* reflectors
*
* Q = H(1) H(2) . . . H(n-1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i) = 0 and v(i+1) = 1; v(i+2:n) is stored on exit in A(i+2:n,i),
* and tau in TAU(i).
*
* The contents of A on exit are illustrated by the following examples
* with n = 5:
*
* if UPLO = 'U': if UPLO = 'L':
*
* ( d e v2 v3 v4 ) ( d )
* ( d e v3 v4 ) ( e d )
* ( d e v4 ) ( v1 e d )
* ( d e ) ( v1 v2 e d )
* ( d ) ( v1 v2 v3 e d )
*
* where d and e denote diagonal and off-diagonal elements of T, and vi
* denotes an element of the vector defining H(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param lda
* @param d
* @param e
* @param tau
* @param work
* @param lwork
* @param info
*
*/
abstract public void ssytrd(java.lang.String uplo, int n, float[] a, int lda, float[] d, float[] e, float[] tau, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYTRD reduces a real symmetric matrix A to real symmetric
* tridiagonal form T by an orthogonal similarity transformation:
* Q**T * A * Q = T.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* N-by-N upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading N-by-N lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
* On exit, if UPLO = 'U', the diagonal and first superdiagonal
* of A are overwritten by the corresponding elements of the
* tridiagonal matrix T, and the elements above the first
* superdiagonal, with the array TAU, represent the orthogonal
* matrix Q as a product of elementary reflectors; if UPLO
* = 'L', the diagonal and first subdiagonal of A are over-
* written by the corresponding elements of the tridiagonal
* matrix T, and the elements below the first subdiagonal, with
* the array TAU, represent the orthogonal matrix Q as a product
* of elementary reflectors. See Further Details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* D (output) REAL array, dimension (N)
* The diagonal elements of the tridiagonal matrix T:
* D(i) = A(i,i).
*
* E (output) REAL array, dimension (N-1)
* The off-diagonal elements of the tridiagonal matrix T:
* E(i) = A(i,i+1) if UPLO = 'U', E(i) = A(i+1,i) if UPLO = 'L'.
*
* TAU (output) REAL array, dimension (N-1)
* The scalar factors of the elementary reflectors (see Further
* Details).
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= 1.
* For optimum performance LWORK >= N*NB, where NB is the
* optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* If UPLO = 'U', the matrix Q is represented as a product of elementary
* reflectors
*
* Q = H(n-1) . . . H(2) H(1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(i+1:n) = 0 and v(i) = 1; v(1:i-1) is stored on exit in
* A(1:i-1,i+1), and tau in TAU(i).
*
* If UPLO = 'L', the matrix Q is represented as a product of elementary
* reflectors
*
* Q = H(1) H(2) . . . H(n-1).
*
* Each H(i) has the form
*
* H(i) = I - tau * v * v'
*
* where tau is a real scalar, and v is a real vector with
* v(1:i) = 0 and v(i+1) = 1; v(i+2:n) is stored on exit in A(i+2:n,i),
* and tau in TAU(i).
*
* The contents of A on exit are illustrated by the following examples
* with n = 5:
*
* if UPLO = 'U': if UPLO = 'L':
*
* ( d e v2 v3 v4 ) ( d )
* ( d e v3 v4 ) ( e d )
* ( d e v4 ) ( v1 e d )
* ( d e ) ( v1 v2 e d )
* ( d ) ( v1 v2 v3 e d )
*
* where d and e denote diagonal and off-diagonal elements of T, and vi
* denotes an element of the vector defining H(i).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param d
* @param _d_offset
* @param e
* @param _e_offset
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void ssytrd(java.lang.String uplo, int n, float[] a, int _a_offset, int lda, float[] d, int _d_offset, float[] e, int _e_offset, float[] tau, int _tau_offset, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYTRF computes the factorization of a real symmetric matrix A using
* the Bunch-Kaufman diagonal pivoting method. The form of the
* factorization is
*
* A = U*D*U**T or A = L*D*L**T
*
* where U (or L) is a product of permutation and unit upper (lower)
* triangular matrices, and D is symmetric and block diagonal with
* 1-by-1 and 2-by-2 diagonal blocks.
*
* This is the blocked version of the algorithm, calling Level 3 BLAS.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* N-by-N upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading N-by-N lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, the block diagonal matrix D and the multipliers used
* to obtain the factor U or L (see below for further details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (output) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D.
* If IPIV(k) > 0, then rows and columns k and IPIV(k) were
* interchanged and D(k,k) is a 1-by-1 diagonal block.
* If UPLO = 'U' and IPIV(k) = IPIV(k-1) < 0, then rows and
* columns k-1 and -IPIV(k) were interchanged and D(k-1:k,k-1:k)
* is a 2-by-2 diagonal block. If UPLO = 'L' and IPIV(k) =
* IPIV(k+1) < 0, then rows and columns k+1 and -IPIV(k) were
* interchanged and D(k:k+1,k:k+1) is a 2-by-2 diagonal block.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of WORK. LWORK >=1. For best performance
* LWORK >= N*NB, where NB is the block size returned by ILAENV.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, D(i,i) is exactly zero. The factorization
* has been completed, but the block diagonal matrix D is
* exactly singular, and division by zero will occur if it
* is used to solve a system of equations.
*
* Further Details
* ===============
*
* If UPLO = 'U', then A = U*D*U', where
* U = P(n)*U(n)* ... *P(k)U(k)* ...,
* i.e., U is a product of terms P(k)*U(k), where k decreases from n to
* 1 in steps of 1 or 2, and D is a block diagonal matrix with 1-by-1
* and 2-by-2 diagonal blocks D(k). P(k) is a permutation matrix as
* defined by IPIV(k), and U(k) is a unit upper triangular matrix, such
* that if the diagonal block D(k) is of order s (s = 1 or 2), then
*
* ( I v 0 ) k-s
* U(k) = ( 0 I 0 ) s
* ( 0 0 I ) n-k
* k-s s n-k
*
* If s = 1, D(k) overwrites A(k,k), and v overwrites A(1:k-1,k).
* If s = 2, the upper triangle of D(k) overwrites A(k-1,k-1), A(k-1,k),
* and A(k,k), and v overwrites A(1:k-2,k-1:k).
*
* If UPLO = 'L', then A = L*D*L', where
* L = P(1)*L(1)* ... *P(k)*L(k)* ...,
* i.e., L is a product of terms P(k)*L(k), where k increases from 1 to
* n in steps of 1 or 2, and D is a block diagonal matrix with 1-by-1
* and 2-by-2 diagonal blocks D(k). P(k) is a permutation matrix as
* defined by IPIV(k), and L(k) is a unit lower triangular matrix, such
* that if the diagonal block D(k) is of order s (s = 1 or 2), then
*
* ( I 0 0 ) k-1
* L(k) = ( 0 I 0 ) s
* ( 0 v I ) n-k-s+1
* k-1 s n-k-s+1
*
* If s = 1, D(k) overwrites A(k,k), and v overwrites A(k+1:n,k).
* If s = 2, the lower triangle of D(k) overwrites A(k,k), A(k+1,k),
* and A(k+1,k+1), and v overwrites A(k+2:n,k:k+1).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param uplo
* @param n
* @param a
* @param lda
* @param ipiv
* @param work
* @param lwork
* @param info
*
*/
abstract public void ssytrf(java.lang.String uplo, int n, float[] a, int lda, int[] ipiv, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYTRF computes the factorization of a real symmetric matrix A using
* the Bunch-Kaufman diagonal pivoting method. The form of the
* factorization is
*
* A = U*D*U**T or A = L*D*L**T
*
* where U (or L) is a product of permutation and unit upper (lower)
* triangular matrices, and D is symmetric and block diagonal with
* 1-by-1 and 2-by-2 diagonal blocks.
*
* This is the blocked version of the algorithm, calling Level 3 BLAS.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': Upper triangle of A is stored;
* = 'L': Lower triangle of A is stored.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the symmetric matrix A. If UPLO = 'U', the leading
* N-by-N upper triangular part of A contains the upper
* triangular part of the matrix A, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading N-by-N lower triangular part of A contains the lower
* triangular part of the matrix A, and the strictly upper
* triangular part of A is not referenced.
*
* On exit, the block diagonal matrix D and the multipliers used
* to obtain the factor U or L (see below for further details).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (output) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D.
* If IPIV(k) > 0, then rows and columns k and IPIV(k) were
* interchanged and D(k,k) is a 1-by-1 diagonal block.
* If UPLO = 'U' and IPIV(k) = IPIV(k-1) < 0, then rows and
* columns k-1 and -IPIV(k) were interchanged and D(k-1:k,k-1:k)
* is a 2-by-2 diagonal block. If UPLO = 'L' and IPIV(k) =
* IPIV(k+1) < 0, then rows and columns k+1 and -IPIV(k) were
* interchanged and D(k:k+1,k:k+1) is a 2-by-2 diagonal block.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The length of WORK. LWORK >=1. For best performance
* LWORK >= N*NB, where NB is the block size returned by ILAENV.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, D(i,i) is exactly zero. The factorization
* has been completed, but the block diagonal matrix D is
* exactly singular, and division by zero will occur if it
* is used to solve a system of equations.
*
* Further Details
* ===============
*
* If UPLO = 'U', then A = U*D*U', where
* U = P(n)*U(n)* ... *P(k)U(k)* ...,
* i.e., U is a product of terms P(k)*U(k), where k decreases from n to
* 1 in steps of 1 or 2, and D is a block diagonal matrix with 1-by-1
* and 2-by-2 diagonal blocks D(k). P(k) is a permutation matrix as
* defined by IPIV(k), and U(k) is a unit upper triangular matrix, such
* that if the diagonal block D(k) is of order s (s = 1 or 2), then
*
* ( I v 0 ) k-s
* U(k) = ( 0 I 0 ) s
* ( 0 0 I ) n-k
* k-s s n-k
*
* If s = 1, D(k) overwrites A(k,k), and v overwrites A(1:k-1,k).
* If s = 2, the upper triangle of D(k) overwrites A(k-1,k-1), A(k-1,k),
* and A(k,k), and v overwrites A(1:k-2,k-1:k).
*
* If UPLO = 'L', then A = L*D*L', where
* L = P(1)*L(1)* ... *P(k)*L(k)* ...,
* i.e., L is a product of terms P(k)*L(k), where k increases from 1 to
* n in steps of 1 or 2, and D is a block diagonal matrix with 1-by-1
* and 2-by-2 diagonal blocks D(k). P(k) is a permutation matrix as
* defined by IPIV(k), and L(k) is a unit lower triangular matrix, such
* that if the diagonal block D(k) is of order s (s = 1 or 2), then
*
* ( I 0 0 ) k-1
* L(k) = ( 0 I 0 ) s
* ( 0 v I ) n-k-s+1
* k-1 s n-k-s+1
*
* If s = 1, D(k) overwrites A(k,k), and v overwrites A(k+1:n,k).
* If s = 2, the lower triangle of D(k) overwrites A(k,k), A(k+1,k),
* and A(k+1,k+1), and v overwrites A(k+2:n,k:k+1).
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param ipiv
* @param _ipiv_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void ssytrf(java.lang.String uplo, int n, float[] a, int _a_offset, int lda, int[] ipiv, int _ipiv_offset, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYTRI computes the inverse of a real symmetric indefinite matrix
* A using the factorization A = U*D*U**T or A = L*D*L**T computed by
* SSYTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the details of the factorization are stored
* as an upper or lower triangular matrix.
* = 'U': Upper triangular, form is A = U*D*U**T;
* = 'L': Lower triangular, form is A = L*D*L**T.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the block diagonal matrix D and the multipliers
* used to obtain the factor U or L as computed by SSYTRF.
*
* On exit, if INFO = 0, the (symmetric) inverse of the original
* matrix. If UPLO = 'U', the upper triangular part of the
* inverse is formed and the part of A below the diagonal is not
* referenced; if UPLO = 'L' the lower triangular part of the
* inverse is formed and the part of A above the diagonal is
* not referenced.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (input) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D
* as determined by SSYTRF.
*
* WORK (workspace) REAL array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, D(i,i) = 0; the matrix is singular and its
* inverse could not be computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param lda
* @param ipiv
* @param work
* @param info
*
*/
abstract public void ssytri(java.lang.String uplo, int n, float[] a, int lda, int[] ipiv, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYTRI computes the inverse of a real symmetric indefinite matrix
* A using the factorization A = U*D*U**T or A = L*D*L**T computed by
* SSYTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the details of the factorization are stored
* as an upper or lower triangular matrix.
* = 'U': Upper triangular, form is A = U*D*U**T;
* = 'L': Lower triangular, form is A = L*D*L**T.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the block diagonal matrix D and the multipliers
* used to obtain the factor U or L as computed by SSYTRF.
*
* On exit, if INFO = 0, the (symmetric) inverse of the original
* matrix. If UPLO = 'U', the upper triangular part of the
* inverse is formed and the part of A below the diagonal is not
* referenced; if UPLO = 'L' the lower triangular part of the
* inverse is formed and the part of A above the diagonal is
* not referenced.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (input) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D
* as determined by SSYTRF.
*
* WORK (workspace) REAL array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, D(i,i) = 0; the matrix is singular and its
* inverse could not be computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param a
* @param _a_offset
* @param lda
* @param ipiv
* @param _ipiv_offset
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void ssytri(java.lang.String uplo, int n, float[] a, int _a_offset, int lda, int[] ipiv, int _ipiv_offset, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYTRS solves a system of linear equations A*X = B with a real
* symmetric matrix A using the factorization A = U*D*U**T or
* A = L*D*L**T computed by SSYTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the details of the factorization are stored
* as an upper or lower triangular matrix.
* = 'U': Upper triangular, form is A = U*D*U**T;
* = 'L': Lower triangular, form is A = L*D*L**T.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The block diagonal matrix D and the multipliers used to
* obtain the factor U or L as computed by SSYTRF.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (input) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D
* as determined by SSYTRF.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param a
* @param lda
* @param ipiv
* @param b
* @param ldb
* @param info
*
*/
abstract public void ssytrs(java.lang.String uplo, int n, int nrhs, float[] a, int lda, int[] ipiv, float[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* SSYTRS solves a system of linear equations A*X = B with a real
* symmetric matrix A using the factorization A = U*D*U**T or
* A = L*D*L**T computed by SSYTRF.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the details of the factorization are stored
* as an upper or lower triangular matrix.
* = 'U': Upper triangular, form is A = U*D*U**T;
* = 'L': Lower triangular, form is A = L*D*L**T.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The block diagonal matrix D and the multipliers used to
* obtain the factor U or L as computed by SSYTRF.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* IPIV (input) INTEGER array, dimension (N)
* Details of the interchanges and the block structure of D
* as determined by SSYTRF.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param ipiv
* @param _ipiv_offset
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void ssytrs(java.lang.String uplo, int n, int nrhs, float[] a, int _a_offset, int lda, int[] ipiv, int _ipiv_offset, float[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STBCON estimates the reciprocal of the condition number of a
* triangular band matrix A, in either the 1-norm or the infinity-norm.
*
* The norm of A is computed and an estimate is obtained for
* norm(inv(A)), then the reciprocal of the condition number is
* computed as
* RCOND = 1 / ( norm(A) * norm(inv(A)) ).
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies whether the 1-norm condition number or the
* infinity-norm condition number is required:
* = '1' or 'O': 1-norm;
* = 'I': Infinity-norm.
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals or subdiagonals of the
* triangular band matrix A. KD >= 0.
*
* AB (input) REAL array, dimension (LDAB,N)
* The upper or lower triangular band matrix A, stored in the
* first kd+1 rows of the array. The j-th column of A is stored
* in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
* If DIAG = 'U', the diagonal elements of A are not referenced
* and are assumed to be 1.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* RCOND (output) REAL
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(norm(A) * norm(inv(A))).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param diag
* @param n
* @param kd
* @param ab
* @param ldab
* @param rcond
* @param work
* @param iwork
* @param info
*
*/
abstract public void stbcon(java.lang.String norm, java.lang.String uplo, java.lang.String diag, int n, int kd, float[] ab, int ldab, org.netlib.util.floatW rcond, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STBCON estimates the reciprocal of the condition number of a
* triangular band matrix A, in either the 1-norm or the infinity-norm.
*
* The norm of A is computed and an estimate is obtained for
* norm(inv(A)), then the reciprocal of the condition number is
* computed as
* RCOND = 1 / ( norm(A) * norm(inv(A)) ).
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies whether the 1-norm condition number or the
* infinity-norm condition number is required:
* = '1' or 'O': 1-norm;
* = 'I': Infinity-norm.
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals or subdiagonals of the
* triangular band matrix A. KD >= 0.
*
* AB (input) REAL array, dimension (LDAB,N)
* The upper or lower triangular band matrix A, stored in the
* first kd+1 rows of the array. The j-th column of A is stored
* in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
* If DIAG = 'U', the diagonal elements of A are not referenced
* and are assumed to be 1.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* RCOND (output) REAL
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(norm(A) * norm(inv(A))).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param diag
* @param n
* @param kd
* @param ab
* @param _ab_offset
* @param ldab
* @param rcond
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void stbcon(java.lang.String norm, java.lang.String uplo, java.lang.String diag, int n, int kd, float[] ab, int _ab_offset, int ldab, org.netlib.util.floatW rcond, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STBRFS provides error bounds and backward error estimates for the
* solution to a system of linear equations with a triangular band
* coefficient matrix.
*
* The solution matrix X must be computed by STBTRS or some other
* means before entering this routine. STBRFS does not do iterative
* refinement because doing so cannot improve the backward error.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals or subdiagonals of the
* triangular band matrix A. KD >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AB (input) REAL array, dimension (LDAB,N)
* The upper or lower triangular band matrix A, stored in the
* first kd+1 rows of the array. The j-th column of A is stored
* in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
* If DIAG = 'U', the diagonal elements of A are not referenced
* and are assumed to be 1.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* B (input) REAL array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input) REAL array, dimension (LDX,NRHS)
* The solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param n
* @param kd
* @param nrhs
* @param ab
* @param ldab
* @param b
* @param ldb
* @param x
* @param ldx
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void stbrfs(java.lang.String uplo, java.lang.String trans, java.lang.String diag, int n, int kd, int nrhs, float[] ab, int ldab, float[] b, int ldb, float[] x, int ldx, float[] ferr, float[] berr, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STBRFS provides error bounds and backward error estimates for the
* solution to a system of linear equations with a triangular band
* coefficient matrix.
*
* The solution matrix X must be computed by STBTRS or some other
* means before entering this routine. STBRFS does not do iterative
* refinement because doing so cannot improve the backward error.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals or subdiagonals of the
* triangular band matrix A. KD >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AB (input) REAL array, dimension (LDAB,N)
* The upper or lower triangular band matrix A, stored in the
* first kd+1 rows of the array. The j-th column of A is stored
* in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
* If DIAG = 'U', the diagonal elements of A are not referenced
* and are assumed to be 1.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* B (input) REAL array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input) REAL array, dimension (LDX,NRHS)
* The solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param n
* @param kd
* @param nrhs
* @param ab
* @param _ab_offset
* @param ldab
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void stbrfs(java.lang.String uplo, java.lang.String trans, java.lang.String diag, int n, int kd, int nrhs, float[] ab, int _ab_offset, int ldab, float[] b, int _b_offset, int ldb, float[] x, int _x_offset, int ldx, float[] ferr, int _ferr_offset, float[] berr, int _berr_offset, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STBTRS solves a triangular system of the form
*
* A * X = B or A**T * X = B,
*
* where A is a triangular band matrix of order N, and B is an
* N-by NRHS matrix. A check is made to verify that A is nonsingular.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* TRANS (input) CHARACTER*1
* Specifies the form the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals or subdiagonals of the
* triangular band matrix A. KD >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AB (input) REAL array, dimension (LDAB,N)
* The upper or lower triangular band matrix A, stored in the
* first kd+1 rows of AB. The j-th column of A is stored
* in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
* If DIAG = 'U', the diagonal elements of A are not referenced
* and are assumed to be 1.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, if INFO = 0, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the i-th diagonal element of A is zero,
* indicating that the matrix is singular and the
* solutions X have not been computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param n
* @param kd
* @param nrhs
* @param ab
* @param ldab
* @param b
* @param ldb
* @param info
*
*/
abstract public void stbtrs(java.lang.String uplo, java.lang.String trans, java.lang.String diag, int n, int kd, int nrhs, float[] ab, int ldab, float[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STBTRS solves a triangular system of the form
*
* A * X = B or A**T * X = B,
*
* where A is a triangular band matrix of order N, and B is an
* N-by NRHS matrix. A check is made to verify that A is nonsingular.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* TRANS (input) CHARACTER*1
* Specifies the form the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* KD (input) INTEGER
* The number of superdiagonals or subdiagonals of the
* triangular band matrix A. KD >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AB (input) REAL array, dimension (LDAB,N)
* The upper or lower triangular band matrix A, stored in the
* first kd+1 rows of AB. The j-th column of A is stored
* in the j-th column of the array AB as follows:
* if UPLO = 'U', AB(kd+1+i-j,j) = A(i,j) for max(1,j-kd)<=i<=j;
* if UPLO = 'L', AB(1+i-j,j) = A(i,j) for j<=i<=min(n,j+kd).
* If DIAG = 'U', the diagonal elements of A are not referenced
* and are assumed to be 1.
*
* LDAB (input) INTEGER
* The leading dimension of the array AB. LDAB >= KD+1.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, if INFO = 0, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the i-th diagonal element of A is zero,
* indicating that the matrix is singular and the
* solutions X have not been computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param n
* @param kd
* @param nrhs
* @param ab
* @param _ab_offset
* @param ldab
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void stbtrs(java.lang.String uplo, java.lang.String trans, java.lang.String diag, int n, int kd, int nrhs, float[] ab, int _ab_offset, int ldab, float[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
*
* Purpose
* =======
*
* STGEVC computes some or all of the right and/or left eigenvectors of
* a pair of real matrices (S,P), where S is a quasi-triangular matrix
* and P is upper triangular. Matrix pairs of this type are produced by
* the generalized Schur factorization of a matrix pair (A,B):
*
* A = Q*S*Z**T, B = Q*P*Z**T
*
* as computed by SGGHRD + SHGEQZ.
*
* The right eigenvector x and the left eigenvector y of (S,P)
* corresponding to an eigenvalue w are defined by:
*
* S*x = w*P*x, (y**H)*S = w*(y**H)*P,
*
* where y**H denotes the conjugate tranpose of y.
* The eigenvalues are not input to this routine, but are computed
* directly from the diagonal blocks of S and P.
*
* This routine returns the matrices X and/or Y of right and left
* eigenvectors of (S,P), or the products Z*X and/or Q*Y,
* where Z and Q are input matrices.
* If Q and Z are the orthogonal factors from the generalized Schur
* factorization of a matrix pair (A,B), then Z*X and Q*Y
* are the matrices of right and left eigenvectors of (A,B).
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'R': compute right eigenvectors only;
* = 'L': compute left eigenvectors only;
* = 'B': compute both right and left eigenvectors.
*
* HOWMNY (input) CHARACTER*1
* = 'A': compute all right and/or left eigenvectors;
* = 'B': compute all right and/or left eigenvectors,
* backtransformed by the matrices in VR and/or VL;
* = 'S': compute selected right and/or left eigenvectors,
* specified by the logical array SELECT.
*
* SELECT (input) LOGICAL array, dimension (N)
* If HOWMNY='S', SELECT specifies the eigenvectors to be
* computed. If w(j) is a real eigenvalue, the corresponding
* real eigenvector is computed if SELECT(j) is .TRUE..
* If w(j) and w(j+1) are the real and imaginary parts of a
* complex eigenvalue, the corresponding complex eigenvector
* is computed if either SELECT(j) or SELECT(j+1) is .TRUE.,
* and on exit SELECT(j) is set to .TRUE. and SELECT(j+1) is
* set to .FALSE..
* Not referenced if HOWMNY = 'A' or 'B'.
*
* N (input) INTEGER
* The order of the matrices S and P. N >= 0.
*
* S (input) REAL array, dimension (LDS,N)
* The upper quasi-triangular matrix S from a generalized Schur
* factorization, as computed by SHGEQZ.
*
* LDS (input) INTEGER
* The leading dimension of array S. LDS >= max(1,N).
*
* P (input) REAL array, dimension (LDP,N)
* The upper triangular matrix P from a generalized Schur
* factorization, as computed by SHGEQZ.
* 2-by-2 diagonal blocks of P corresponding to 2-by-2 blocks
* of S must be in positive diagonal form.
*
* LDP (input) INTEGER
* The leading dimension of array P. LDP >= max(1,N).
*
* VL (input/output) REAL array, dimension (LDVL,MM)
* On entry, if SIDE = 'L' or 'B' and HOWMNY = 'B', VL must
* contain an N-by-N matrix Q (usually the orthogonal matrix Q
* of left Schur vectors returned by SHGEQZ).
* On exit, if SIDE = 'L' or 'B', VL contains:
* if HOWMNY = 'A', the matrix Y of left eigenvectors of (S,P);
* if HOWMNY = 'B', the matrix Q*Y;
* if HOWMNY = 'S', the left eigenvectors of (S,P) specified by
* SELECT, stored consecutively in the columns of
* VL, in the same order as their eigenvalues.
*
* A complex eigenvector corresponding to a complex eigenvalue
* is stored in two consecutive columns, the first holding the
* real part, and the second the imaginary part.
*
* Not referenced if SIDE = 'R'.
*
* LDVL (input) INTEGER
* The leading dimension of array VL. LDVL >= 1, and if
* SIDE = 'L' or 'B', LDVL >= N.
*
* VR (input/output) REAL array, dimension (LDVR,MM)
* On entry, if SIDE = 'R' or 'B' and HOWMNY = 'B', VR must
* contain an N-by-N matrix Z (usually the orthogonal matrix Z
* of right Schur vectors returned by SHGEQZ).
*
* On exit, if SIDE = 'R' or 'B', VR contains:
* if HOWMNY = 'A', the matrix X of right eigenvectors of (S,P);
* if HOWMNY = 'B' or 'b', the matrix Z*X;
* if HOWMNY = 'S' or 's', the right eigenvectors of (S,P)
* specified by SELECT, stored consecutively in the
* columns of VR, in the same order as their
* eigenvalues.
*
* A complex eigenvector corresponding to a complex eigenvalue
* is stored in two consecutive columns, the first holding the
* real part and the second the imaginary part.
*
* Not referenced if SIDE = 'L'.
*
* LDVR (input) INTEGER
* The leading dimension of the array VR. LDVR >= 1, and if
* SIDE = 'R' or 'B', LDVR >= N.
*
* MM (input) INTEGER
* The number of columns in the arrays VL and/or VR. MM >= M.
*
* M (output) INTEGER
* The number of columns in the arrays VL and/or VR actually
* used to store the eigenvectors. If HOWMNY = 'A' or 'B', M
* is set to N. Each selected real eigenvector occupies one
* column and each selected complex eigenvector occupies two
* columns.
*
* WORK (workspace) REAL array, dimension (6*N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: the 2-by-2 block (INFO:INFO+1) does not have a complex
* eigenvalue.
*
* Further Details
* ===============
*
* Allocation of workspace:
* ---------- -- ---------
*
* WORK( j ) = 1-norm of j-th column of A, above the diagonal
* WORK( N+j ) = 1-norm of j-th column of B, above the diagonal
* WORK( 2*N+1:3*N ) = real part of eigenvector
* WORK( 3*N+1:4*N ) = imaginary part of eigenvector
* WORK( 4*N+1:5*N ) = real part of back-transformed eigenvector
* WORK( 5*N+1:6*N ) = imaginary part of back-transformed eigenvector
*
* Rowwise vs. columnwise solution methods:
* ------- -- ---------- -------- -------
*
* Finding a generalized eigenvector consists basically of solving the
* singular triangular system
*
* (A - w B) x = 0 (for right) or: (A - w B)**H y = 0 (for left)
*
* Consider finding the i-th right eigenvector (assume all eigenvalues
* are real). The equation to be solved is:
* n i
* 0 = sum C(j,k) v(k) = sum C(j,k) v(k) for j = i,. . .,1
* k=j k=j
*
* where C = (A - w B) (The components v(i+1:n) are 0.)
*
* The "rowwise" method is:
*
* (1) v(i) := 1
* for j = i-1,. . .,1:
* i
* (2) compute s = - sum C(j,k) v(k) and
* k=j+1
*
* (3) v(j) := s / C(j,j)
*
* Step 2 is sometimes called the "dot product" step, since it is an
* inner product between the j-th row and the portion of the eigenvector
* that has been computed so far.
*
* The "columnwise" method consists basically in doing the sums
* for all the rows in parallel. As each v(j) is computed, the
* contribution of v(j) times the j-th column of C is added to the
* partial sums. Since FORTRAN arrays are stored columnwise, this has
* the advantage that at each step, the elements of C that are accessed
* are adjacent to one another, whereas with the rowwise method, the
* elements accessed at a step are spaced LDS (and LDP) words apart.
*
* When finding left eigenvectors, the matrix in question is the
* transpose of the one in storage, so the rowwise method then
* actually accesses columns of A and B at each step, and so is the
* preferred method.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param howmny
* @param select
* @param n
* @param s
* @param lds
* @param p
* @param ldp
* @param vl
* @param ldvl
* @param vr
* @param ldvr
* @param mm
* @param m
* @param work
* @param info
*
*/
abstract public void stgevc(java.lang.String side, java.lang.String howmny, boolean[] select, int n, float[] s, int lds, float[] p, int ldp, float[] vl, int ldvl, float[] vr, int ldvr, int mm, org.netlib.util.intW m, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
*
* Purpose
* =======
*
* STGEVC computes some or all of the right and/or left eigenvectors of
* a pair of real matrices (S,P), where S is a quasi-triangular matrix
* and P is upper triangular. Matrix pairs of this type are produced by
* the generalized Schur factorization of a matrix pair (A,B):
*
* A = Q*S*Z**T, B = Q*P*Z**T
*
* as computed by SGGHRD + SHGEQZ.
*
* The right eigenvector x and the left eigenvector y of (S,P)
* corresponding to an eigenvalue w are defined by:
*
* S*x = w*P*x, (y**H)*S = w*(y**H)*P,
*
* where y**H denotes the conjugate tranpose of y.
* The eigenvalues are not input to this routine, but are computed
* directly from the diagonal blocks of S and P.
*
* This routine returns the matrices X and/or Y of right and left
* eigenvectors of (S,P), or the products Z*X and/or Q*Y,
* where Z and Q are input matrices.
* If Q and Z are the orthogonal factors from the generalized Schur
* factorization of a matrix pair (A,B), then Z*X and Q*Y
* are the matrices of right and left eigenvectors of (A,B).
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'R': compute right eigenvectors only;
* = 'L': compute left eigenvectors only;
* = 'B': compute both right and left eigenvectors.
*
* HOWMNY (input) CHARACTER*1
* = 'A': compute all right and/or left eigenvectors;
* = 'B': compute all right and/or left eigenvectors,
* backtransformed by the matrices in VR and/or VL;
* = 'S': compute selected right and/or left eigenvectors,
* specified by the logical array SELECT.
*
* SELECT (input) LOGICAL array, dimension (N)
* If HOWMNY='S', SELECT specifies the eigenvectors to be
* computed. If w(j) is a real eigenvalue, the corresponding
* real eigenvector is computed if SELECT(j) is .TRUE..
* If w(j) and w(j+1) are the real and imaginary parts of a
* complex eigenvalue, the corresponding complex eigenvector
* is computed if either SELECT(j) or SELECT(j+1) is .TRUE.,
* and on exit SELECT(j) is set to .TRUE. and SELECT(j+1) is
* set to .FALSE..
* Not referenced if HOWMNY = 'A' or 'B'.
*
* N (input) INTEGER
* The order of the matrices S and P. N >= 0.
*
* S (input) REAL array, dimension (LDS,N)
* The upper quasi-triangular matrix S from a generalized Schur
* factorization, as computed by SHGEQZ.
*
* LDS (input) INTEGER
* The leading dimension of array S. LDS >= max(1,N).
*
* P (input) REAL array, dimension (LDP,N)
* The upper triangular matrix P from a generalized Schur
* factorization, as computed by SHGEQZ.
* 2-by-2 diagonal blocks of P corresponding to 2-by-2 blocks
* of S must be in positive diagonal form.
*
* LDP (input) INTEGER
* The leading dimension of array P. LDP >= max(1,N).
*
* VL (input/output) REAL array, dimension (LDVL,MM)
* On entry, if SIDE = 'L' or 'B' and HOWMNY = 'B', VL must
* contain an N-by-N matrix Q (usually the orthogonal matrix Q
* of left Schur vectors returned by SHGEQZ).
* On exit, if SIDE = 'L' or 'B', VL contains:
* if HOWMNY = 'A', the matrix Y of left eigenvectors of (S,P);
* if HOWMNY = 'B', the matrix Q*Y;
* if HOWMNY = 'S', the left eigenvectors of (S,P) specified by
* SELECT, stored consecutively in the columns of
* VL, in the same order as their eigenvalues.
*
* A complex eigenvector corresponding to a complex eigenvalue
* is stored in two consecutive columns, the first holding the
* real part, and the second the imaginary part.
*
* Not referenced if SIDE = 'R'.
*
* LDVL (input) INTEGER
* The leading dimension of array VL. LDVL >= 1, and if
* SIDE = 'L' or 'B', LDVL >= N.
*
* VR (input/output) REAL array, dimension (LDVR,MM)
* On entry, if SIDE = 'R' or 'B' and HOWMNY = 'B', VR must
* contain an N-by-N matrix Z (usually the orthogonal matrix Z
* of right Schur vectors returned by SHGEQZ).
*
* On exit, if SIDE = 'R' or 'B', VR contains:
* if HOWMNY = 'A', the matrix X of right eigenvectors of (S,P);
* if HOWMNY = 'B' or 'b', the matrix Z*X;
* if HOWMNY = 'S' or 's', the right eigenvectors of (S,P)
* specified by SELECT, stored consecutively in the
* columns of VR, in the same order as their
* eigenvalues.
*
* A complex eigenvector corresponding to a complex eigenvalue
* is stored in two consecutive columns, the first holding the
* real part and the second the imaginary part.
*
* Not referenced if SIDE = 'L'.
*
* LDVR (input) INTEGER
* The leading dimension of the array VR. LDVR >= 1, and if
* SIDE = 'R' or 'B', LDVR >= N.
*
* MM (input) INTEGER
* The number of columns in the arrays VL and/or VR. MM >= M.
*
* M (output) INTEGER
* The number of columns in the arrays VL and/or VR actually
* used to store the eigenvectors. If HOWMNY = 'A' or 'B', M
* is set to N. Each selected real eigenvector occupies one
* column and each selected complex eigenvector occupies two
* columns.
*
* WORK (workspace) REAL array, dimension (6*N)
*
* INFO (output) INTEGER
* = 0: successful exit.
* < 0: if INFO = -i, the i-th argument had an illegal value.
* > 0: the 2-by-2 block (INFO:INFO+1) does not have a complex
* eigenvalue.
*
* Further Details
* ===============
*
* Allocation of workspace:
* ---------- -- ---------
*
* WORK( j ) = 1-norm of j-th column of A, above the diagonal
* WORK( N+j ) = 1-norm of j-th column of B, above the diagonal
* WORK( 2*N+1:3*N ) = real part of eigenvector
* WORK( 3*N+1:4*N ) = imaginary part of eigenvector
* WORK( 4*N+1:5*N ) = real part of back-transformed eigenvector
* WORK( 5*N+1:6*N ) = imaginary part of back-transformed eigenvector
*
* Rowwise vs. columnwise solution methods:
* ------- -- ---------- -------- -------
*
* Finding a generalized eigenvector consists basically of solving the
* singular triangular system
*
* (A - w B) x = 0 (for right) or: (A - w B)**H y = 0 (for left)
*
* Consider finding the i-th right eigenvector (assume all eigenvalues
* are real). The equation to be solved is:
* n i
* 0 = sum C(j,k) v(k) = sum C(j,k) v(k) for j = i,. . .,1
* k=j k=j
*
* where C = (A - w B) (The components v(i+1:n) are 0.)
*
* The "rowwise" method is:
*
* (1) v(i) := 1
* for j = i-1,. . .,1:
* i
* (2) compute s = - sum C(j,k) v(k) and
* k=j+1
*
* (3) v(j) := s / C(j,j)
*
* Step 2 is sometimes called the "dot product" step, since it is an
* inner product between the j-th row and the portion of the eigenvector
* that has been computed so far.
*
* The "columnwise" method consists basically in doing the sums
* for all the rows in parallel. As each v(j) is computed, the
* contribution of v(j) times the j-th column of C is added to the
* partial sums. Since FORTRAN arrays are stored columnwise, this has
* the advantage that at each step, the elements of C that are accessed
* are adjacent to one another, whereas with the rowwise method, the
* elements accessed at a step are spaced LDS (and LDP) words apart.
*
* When finding left eigenvectors, the matrix in question is the
* transpose of the one in storage, so the rowwise method then
* actually accesses columns of A and B at each step, and so is the
* preferred method.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param howmny
* @param select
* @param _select_offset
* @param n
* @param s
* @param _s_offset
* @param lds
* @param p
* @param _p_offset
* @param ldp
* @param vl
* @param _vl_offset
* @param ldvl
* @param vr
* @param _vr_offset
* @param ldvr
* @param mm
* @param m
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void stgevc(java.lang.String side, java.lang.String howmny, boolean[] select, int _select_offset, int n, float[] s, int _s_offset, int lds, float[] p, int _p_offset, int ldp, float[] vl, int _vl_offset, int ldvl, float[] vr, int _vr_offset, int ldvr, int mm, org.netlib.util.intW m, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STGEX2 swaps adjacent diagonal blocks (A11, B11) and (A22, B22)
* of size 1-by-1 or 2-by-2 in an upper (quasi) triangular matrix pair
* (A, B) by an orthogonal equivalence transformation.
*
* (A, B) must be in generalized real Schur canonical form (as returned
* by SGGES), i.e. A is block upper triangular with 1-by-1 and 2-by-2
* diagonal blocks. B is upper triangular.
*
* Optionally, the matrices Q and Z of generalized Schur vectors are
* updated.
*
* Q(in) * A(in) * Z(in)' = Q(out) * A(out) * Z(out)'
* Q(in) * B(in) * Z(in)' = Q(out) * B(out) * Z(out)'
*
*
* Arguments
* =========
*
* WANTQ (input) LOGICAL
* .TRUE. : update the left transformation matrix Q;
* .FALSE.: do not update Q.
*
* WANTZ (input) LOGICAL
* .TRUE. : update the right transformation matrix Z;
* .FALSE.: do not update Z.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* A (input/output) REAL arrays, dimensions (LDA,N)
* On entry, the matrix A in the pair (A, B).
* On exit, the updated matrix A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) REAL arrays, dimensions (LDB,N)
* On entry, the matrix B in the pair (A, B).
* On exit, the updated matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* Q (input/output) REAL array, dimension (LDZ,N)
* On entry, if WANTQ = .TRUE., the orthogonal matrix Q.
* On exit, the updated matrix Q.
* Not referenced if WANTQ = .FALSE..
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= 1.
* If WANTQ = .TRUE., LDQ >= N.
*
* Z (input/output) REAL array, dimension (LDZ,N)
* On entry, if WANTZ =.TRUE., the orthogonal matrix Z.
* On exit, the updated matrix Z.
* Not referenced if WANTZ = .FALSE..
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1.
* If WANTZ = .TRUE., LDZ >= N.
*
* J1 (input) INTEGER
* The index to the first block (A11, B11). 1 <= J1 <= N.
*
* N1 (input) INTEGER
* The order of the first block (A11, B11). N1 = 0, 1 or 2.
*
* N2 (input) INTEGER
* The order of the second block (A22, B22). N2 = 0, 1 or 2.
*
* WORK (workspace) REAL array, dimension (MAX(1,LWORK)).
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* LWORK >= MAX( N*(N2+N1), (N2+N1)*(N2+N1)*2 )
*
* INFO (output) INTEGER
* =0: Successful exit
* >0: If INFO = 1, the transformed matrix (A, B) would be
* too far from generalized Schur form; the blocks are
* not swapped and (A, B) and (Q, Z) are unchanged.
* The problem of swapping is too ill-conditioned.
* <0: If INFO = -16: LWORK is too small. Appropriate value
* for LWORK is returned in WORK(1).
*
* Further Details
* ===============
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* In the current code both weak and strong stability tests are
* performed. The user can omit the strong stability test by changing
* the internal logical parameter WANDS to .FALSE.. See ref. [2] for
* details.
*
* [1] B. Kagstrom; A Direct Method for Reordering Eigenvalues in the
* Generalized Real Schur Form of a Regular Matrix Pair (A, B), in
* M.S. Moonen et al (eds), Linear Algebra for Large Scale and
* Real-Time Applications, Kluwer Academic Publ. 1993, pp 195-218.
*
* [2] B. Kagstrom and P. Poromaa; Computing Eigenspaces with Specified
* Eigenvalues of a Regular Matrix Pair (A, B) and Condition
* Estimation: Theory, Algorithms and Software,
* Report UMINF - 94.04, Department of Computing Science, Umea
* University, S-901 87 Umea, Sweden, 1994. Also as LAPACK Working
* Note 87. To appear in Numerical Algorithms, 1996.
*
* =====================================================================
* Replaced various illegal calls to SCOPY by calls to SLASET, or by DO
* loops. Sven Hammarling, 1/5/02.
*
* .. Parameters ..
*
*
* @param wantq
* @param wantz
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param q
* @param ldq
* @param z
* @param ldz
* @param j1
* @param n1
* @param n2
* @param work
* @param lwork
* @param info
*
*/
abstract public void stgex2(boolean wantq, boolean wantz, int n, float[] a, int lda, float[] b, int ldb, float[] q, int ldq, float[] z, int ldz, int j1, int n1, int n2, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STGEX2 swaps adjacent diagonal blocks (A11, B11) and (A22, B22)
* of size 1-by-1 or 2-by-2 in an upper (quasi) triangular matrix pair
* (A, B) by an orthogonal equivalence transformation.
*
* (A, B) must be in generalized real Schur canonical form (as returned
* by SGGES), i.e. A is block upper triangular with 1-by-1 and 2-by-2
* diagonal blocks. B is upper triangular.
*
* Optionally, the matrices Q and Z of generalized Schur vectors are
* updated.
*
* Q(in) * A(in) * Z(in)' = Q(out) * A(out) * Z(out)'
* Q(in) * B(in) * Z(in)' = Q(out) * B(out) * Z(out)'
*
*
* Arguments
* =========
*
* WANTQ (input) LOGICAL
* .TRUE. : update the left transformation matrix Q;
* .FALSE.: do not update Q.
*
* WANTZ (input) LOGICAL
* .TRUE. : update the right transformation matrix Z;
* .FALSE.: do not update Z.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* A (input/output) REAL arrays, dimensions (LDA,N)
* On entry, the matrix A in the pair (A, B).
* On exit, the updated matrix A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) REAL arrays, dimensions (LDB,N)
* On entry, the matrix B in the pair (A, B).
* On exit, the updated matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* Q (input/output) REAL array, dimension (LDZ,N)
* On entry, if WANTQ = .TRUE., the orthogonal matrix Q.
* On exit, the updated matrix Q.
* Not referenced if WANTQ = .FALSE..
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= 1.
* If WANTQ = .TRUE., LDQ >= N.
*
* Z (input/output) REAL array, dimension (LDZ,N)
* On entry, if WANTZ =.TRUE., the orthogonal matrix Z.
* On exit, the updated matrix Z.
* Not referenced if WANTZ = .FALSE..
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1.
* If WANTZ = .TRUE., LDZ >= N.
*
* J1 (input) INTEGER
* The index to the first block (A11, B11). 1 <= J1 <= N.
*
* N1 (input) INTEGER
* The order of the first block (A11, B11). N1 = 0, 1 or 2.
*
* N2 (input) INTEGER
* The order of the second block (A22, B22). N2 = 0, 1 or 2.
*
* WORK (workspace) REAL array, dimension (MAX(1,LWORK)).
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* LWORK >= MAX( N*(N2+N1), (N2+N1)*(N2+N1)*2 )
*
* INFO (output) INTEGER
* =0: Successful exit
* >0: If INFO = 1, the transformed matrix (A, B) would be
* too far from generalized Schur form; the blocks are
* not swapped and (A, B) and (Q, Z) are unchanged.
* The problem of swapping is too ill-conditioned.
* <0: If INFO = -16: LWORK is too small. Appropriate value
* for LWORK is returned in WORK(1).
*
* Further Details
* ===============
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* In the current code both weak and strong stability tests are
* performed. The user can omit the strong stability test by changing
* the internal logical parameter WANDS to .FALSE.. See ref. [2] for
* details.
*
* [1] B. Kagstrom; A Direct Method for Reordering Eigenvalues in the
* Generalized Real Schur Form of a Regular Matrix Pair (A, B), in
* M.S. Moonen et al (eds), Linear Algebra for Large Scale and
* Real-Time Applications, Kluwer Academic Publ. 1993, pp 195-218.
*
* [2] B. Kagstrom and P. Poromaa; Computing Eigenspaces with Specified
* Eigenvalues of a Regular Matrix Pair (A, B) and Condition
* Estimation: Theory, Algorithms and Software,
* Report UMINF - 94.04, Department of Computing Science, Umea
* University, S-901 87 Umea, Sweden, 1994. Also as LAPACK Working
* Note 87. To appear in Numerical Algorithms, 1996.
*
* =====================================================================
* Replaced various illegal calls to SCOPY by calls to SLASET, or by DO
* loops. Sven Hammarling, 1/5/02.
*
* .. Parameters ..
*
*
* @param wantq
* @param wantz
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param q
* @param _q_offset
* @param ldq
* @param z
* @param _z_offset
* @param ldz
* @param j1
* @param n1
* @param n2
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void stgex2(boolean wantq, boolean wantz, int n, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, float[] q, int _q_offset, int ldq, float[] z, int _z_offset, int ldz, int j1, int n1, int n2, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STGEXC reorders the generalized real Schur decomposition of a real
* matrix pair (A,B) using an orthogonal equivalence transformation
*
* (A, B) = Q * (A, B) * Z',
*
* so that the diagonal block of (A, B) with row index IFST is moved
* to row ILST.
*
* (A, B) must be in generalized real Schur canonical form (as returned
* by SGGES), i.e. A is block upper triangular with 1-by-1 and 2-by-2
* diagonal blocks. B is upper triangular.
*
* Optionally, the matrices Q and Z of generalized Schur vectors are
* updated.
*
* Q(in) * A(in) * Z(in)' = Q(out) * A(out) * Z(out)'
* Q(in) * B(in) * Z(in)' = Q(out) * B(out) * Z(out)'
*
*
* Arguments
* =========
*
* WANTQ (input) LOGICAL
* .TRUE. : update the left transformation matrix Q;
* .FALSE.: do not update Q.
*
* WANTZ (input) LOGICAL
* .TRUE. : update the right transformation matrix Z;
* .FALSE.: do not update Z.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the matrix A in generalized real Schur canonical
* form.
* On exit, the updated matrix A, again in generalized
* real Schur canonical form.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension (LDB,N)
* On entry, the matrix B in generalized real Schur canonical
* form (A,B).
* On exit, the updated matrix B, again in generalized
* real Schur canonical form (A,B).
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* Q (input/output) REAL array, dimension (LDZ,N)
* On entry, if WANTQ = .TRUE., the orthogonal matrix Q.
* On exit, the updated matrix Q.
* If WANTQ = .FALSE., Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= 1.
* If WANTQ = .TRUE., LDQ >= N.
*
* Z (input/output) REAL array, dimension (LDZ,N)
* On entry, if WANTZ = .TRUE., the orthogonal matrix Z.
* On exit, the updated matrix Z.
* If WANTZ = .FALSE., Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1.
* If WANTZ = .TRUE., LDZ >= N.
*
* IFST (input/output) INTEGER
* ILST (input/output) INTEGER
* Specify the reordering of the diagonal blocks of (A, B).
* The block with row index IFST is moved to row ILST, by a
* sequence of swapping between adjacent blocks.
* On exit, if IFST pointed on entry to the second row of
* a 2-by-2 block, it is changed to point to the first row;
* ILST always points to the first row of the block in its
* final position (which may differ from its input value by
* +1 or -1). 1 <= IFST, ILST <= N.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* LWORK >= 1 when N <= 1, otherwise LWORK >= 4*N + 16.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* =0: successful exit.
* <0: if INFO = -i, the i-th argument had an illegal value.
* =1: The transformed matrix pair (A, B) would be too far
* from generalized Schur form; the problem is ill-
* conditioned. (A, B) may have been partially reordered,
* and ILST points to the first row of the current
* position of the block being moved.
*
* Further Details
* ===============
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* [1] B. Kagstrom; A Direct Method for Reordering Eigenvalues in the
* Generalized Real Schur Form of a Regular Matrix Pair (A, B), in
* M.S. Moonen et al (eds), Linear Algebra for Large Scale and
* Real-Time Applications, Kluwer Academic Publ. 1993, pp 195-218.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param wantq
* @param wantz
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param q
* @param ldq
* @param z
* @param ldz
* @param ifst
* @param ilst
* @param work
* @param lwork
* @param info
*
*/
abstract public void stgexc(boolean wantq, boolean wantz, int n, float[] a, int lda, float[] b, int ldb, float[] q, int ldq, float[] z, int ldz, org.netlib.util.intW ifst, org.netlib.util.intW ilst, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STGEXC reorders the generalized real Schur decomposition of a real
* matrix pair (A,B) using an orthogonal equivalence transformation
*
* (A, B) = Q * (A, B) * Z',
*
* so that the diagonal block of (A, B) with row index IFST is moved
* to row ILST.
*
* (A, B) must be in generalized real Schur canonical form (as returned
* by SGGES), i.e. A is block upper triangular with 1-by-1 and 2-by-2
* diagonal blocks. B is upper triangular.
*
* Optionally, the matrices Q and Z of generalized Schur vectors are
* updated.
*
* Q(in) * A(in) * Z(in)' = Q(out) * A(out) * Z(out)'
* Q(in) * B(in) * Z(in)' = Q(out) * B(out) * Z(out)'
*
*
* Arguments
* =========
*
* WANTQ (input) LOGICAL
* .TRUE. : update the left transformation matrix Q;
* .FALSE.: do not update Q.
*
* WANTZ (input) LOGICAL
* .TRUE. : update the right transformation matrix Z;
* .FALSE.: do not update Z.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the matrix A in generalized real Schur canonical
* form.
* On exit, the updated matrix A, again in generalized
* real Schur canonical form.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension (LDB,N)
* On entry, the matrix B in generalized real Schur canonical
* form (A,B).
* On exit, the updated matrix B, again in generalized
* real Schur canonical form (A,B).
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* Q (input/output) REAL array, dimension (LDZ,N)
* On entry, if WANTQ = .TRUE., the orthogonal matrix Q.
* On exit, the updated matrix Q.
* If WANTQ = .FALSE., Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= 1.
* If WANTQ = .TRUE., LDQ >= N.
*
* Z (input/output) REAL array, dimension (LDZ,N)
* On entry, if WANTZ = .TRUE., the orthogonal matrix Z.
* On exit, the updated matrix Z.
* If WANTZ = .FALSE., Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1.
* If WANTZ = .TRUE., LDZ >= N.
*
* IFST (input/output) INTEGER
* ILST (input/output) INTEGER
* Specify the reordering of the diagonal blocks of (A, B).
* The block with row index IFST is moved to row ILST, by a
* sequence of swapping between adjacent blocks.
* On exit, if IFST pointed on entry to the second row of
* a 2-by-2 block, it is changed to point to the first row;
* ILST always points to the first row of the block in its
* final position (which may differ from its input value by
* +1 or -1). 1 <= IFST, ILST <= N.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* LWORK >= 1 when N <= 1, otherwise LWORK >= 4*N + 16.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* =0: successful exit.
* <0: if INFO = -i, the i-th argument had an illegal value.
* =1: The transformed matrix pair (A, B) would be too far
* from generalized Schur form; the problem is ill-
* conditioned. (A, B) may have been partially reordered,
* and ILST points to the first row of the current
* position of the block being moved.
*
* Further Details
* ===============
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* [1] B. Kagstrom; A Direct Method for Reordering Eigenvalues in the
* Generalized Real Schur Form of a Regular Matrix Pair (A, B), in
* M.S. Moonen et al (eds), Linear Algebra for Large Scale and
* Real-Time Applications, Kluwer Academic Publ. 1993, pp 195-218.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param wantq
* @param wantz
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param q
* @param _q_offset
* @param ldq
* @param z
* @param _z_offset
* @param ldz
* @param ifst
* @param ilst
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void stgexc(boolean wantq, boolean wantz, int n, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, float[] q, int _q_offset, int ldq, float[] z, int _z_offset, int ldz, org.netlib.util.intW ifst, org.netlib.util.intW ilst, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STGSEN reorders the generalized real Schur decomposition of a real
* matrix pair (A, B) (in terms of an orthonormal equivalence trans-
* formation Q' * (A, B) * Z), so that a selected cluster of eigenvalues
* appears in the leading diagonal blocks of the upper quasi-triangular
* matrix A and the upper triangular B. The leading columns of Q and
* Z form orthonormal bases of the corresponding left and right eigen-
* spaces (deflating subspaces). (A, B) must be in generalized real
* Schur canonical form (as returned by SGGES), i.e. A is block upper
* triangular with 1-by-1 and 2-by-2 diagonal blocks. B is upper
* triangular.
*
* STGSEN also computes the generalized eigenvalues
*
* w(j) = (ALPHAR(j) + i*ALPHAI(j))/BETA(j)
*
* of the reordered matrix pair (A, B).
*
* Optionally, STGSEN computes the estimates of reciprocal condition
* numbers for eigenvalues and eigenspaces. These are Difu[(A11,B11),
* (A22,B22)] and Difl[(A11,B11), (A22,B22)], i.e. the separation(s)
* between the matrix pairs (A11, B11) and (A22,B22) that correspond to
* the selected cluster and the eigenvalues outside the cluster, resp.,
* and norms of "projections" onto left and right eigenspaces w.r.t.
* the selected cluster in the (1,1)-block.
*
* Arguments
* =========
*
* IJOB (input) INTEGER
* Specifies whether condition numbers are required for the
* cluster of eigenvalues (PL and PR) or the deflating subspaces
* (Difu and Difl):
* =0: Only reorder w.r.t. SELECT. No extras.
* =1: Reciprocal of norms of "projections" onto left and right
* eigenspaces w.r.t. the selected cluster (PL and PR).
* =2: Upper bounds on Difu and Difl. F-norm-based estimate
* (DIF(1:2)).
* =3: Estimate of Difu and Difl. 1-norm-based estimate
* (DIF(1:2)).
* About 5 times as expensive as IJOB = 2.
* =4: Compute PL, PR and DIF (i.e. 0, 1 and 2 above): Economic
* version to get it all.
* =5: Compute PL, PR and DIF (i.e. 0, 1 and 3 above)
*
* WANTQ (input) LOGICAL
* .TRUE. : update the left transformation matrix Q;
* .FALSE.: do not update Q.
*
* WANTZ (input) LOGICAL
* .TRUE. : update the right transformation matrix Z;
* .FALSE.: do not update Z.
*
* SELECT (input) LOGICAL array, dimension (N)
* SELECT specifies the eigenvalues in the selected cluster.
* To select a real eigenvalue w(j), SELECT(j) must be set to
* .TRUE.. To select a complex conjugate pair of eigenvalues
* w(j) and w(j+1), corresponding to a 2-by-2 diagonal block,
* either SELECT(j) or SELECT(j+1) or both must be set to
* .TRUE.; a complex conjugate pair of eigenvalues must be
* either both included in the cluster or both excluded.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* A (input/output) REAL array, dimension(LDA,N)
* On entry, the upper quasi-triangular matrix A, with (A, B) in
* generalized real Schur canonical form.
* On exit, A is overwritten by the reordered matrix A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension(LDB,N)
* On entry, the upper triangular matrix B, with (A, B) in
* generalized real Schur canonical form.
* On exit, B is overwritten by the reordered matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* ALPHAR (output) REAL array, dimension (N)
* ALPHAI (output) REAL array, dimension (N)
* BETA (output) REAL array, dimension (N)
* On exit, (ALPHAR(j) + ALPHAI(j)*i)/BETA(j), j=1,...,N, will
* be the generalized eigenvalues. ALPHAR(j) + ALPHAI(j)*i
* and BETA(j),j=1,...,N are the diagonals of the complex Schur
* form (S,T) that would result if the 2-by-2 diagonal blocks of
* the real generalized Schur form of (A,B) were further reduced
* to triangular form using complex unitary transformations.
* If ALPHAI(j) is zero, then the j-th eigenvalue is real; if
* positive, then the j-th and (j+1)-st eigenvalues are a
* complex conjugate pair, with ALPHAI(j+1) negative.
*
* Q (input/output) REAL array, dimension (LDQ,N)
* On entry, if WANTQ = .TRUE., Q is an N-by-N matrix.
* On exit, Q has been postmultiplied by the left orthogonal
* transformation matrix which reorder (A, B); The leading M
* columns of Q form orthonormal bases for the specified pair of
* left eigenspaces (deflating subspaces).
* If WANTQ = .FALSE., Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= 1;
* and if WANTQ = .TRUE., LDQ >= N.
*
* Z (input/output) REAL array, dimension (LDZ,N)
* On entry, if WANTZ = .TRUE., Z is an N-by-N matrix.
* On exit, Z has been postmultiplied by the left orthogonal
* transformation matrix which reorder (A, B); The leading M
* columns of Z form orthonormal bases for the specified pair of
* left eigenspaces (deflating subspaces).
* If WANTZ = .FALSE., Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1;
* If WANTZ = .TRUE., LDZ >= N.
*
* M (output) INTEGER
* The dimension of the specified pair of left and right eigen-
* spaces (deflating subspaces). 0 <= M <= N.
*
* PL (output) REAL
* PR (output) REAL
* If IJOB = 1, 4 or 5, PL, PR are lower bounds on the
* reciprocal of the norm of "projections" onto left and right
* eigenspaces with respect to the selected cluster.
* 0 < PL, PR <= 1.
* If M = 0 or M = N, PL = PR = 1.
* If IJOB = 0, 2 or 3, PL and PR are not referenced.
*
* DIF (output) REAL array, dimension (2).
* If IJOB >= 2, DIF(1:2) store the estimates of Difu and Difl.
* If IJOB = 2 or 4, DIF(1:2) are F-norm-based upper bounds on
* Difu and Difl. If IJOB = 3 or 5, DIF(1:2) are 1-norm-based
* estimates of Difu and Difl.
* If M = 0 or N, DIF(1:2) = F-norm([A, B]).
* If IJOB = 0 or 1, DIF is not referenced.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= 4*N+16.
* If IJOB = 1, 2 or 4, LWORK >= MAX(4*N+16, 2*M*(N-M)).
* If IJOB = 3 or 5, LWORK >= MAX(4*N+16, 4*M*(N-M)).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* IF IJOB = 0, IWORK is not referenced. Otherwise,
* on exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK. LIWORK >= 1.
* If IJOB = 1, 2 or 4, LIWORK >= N+6.
* If IJOB = 3 or 5, LIWORK >= MAX(2*M*(N-M), N+6).
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal size of the IWORK array,
* returns this value as the first entry of the IWORK array, and
* no error message related to LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* =0: Successful exit.
* <0: If INFO = -i, the i-th argument had an illegal value.
* =1: Reordering of (A, B) failed because the transformed
* matrix pair (A, B) would be too far from generalized
* Schur form; the problem is very ill-conditioned.
* (A, B) may have been partially reordered.
* If requested, 0 is returned in DIF(*), PL and PR.
*
* Further Details
* ===============
*
* STGSEN first collects the selected eigenvalues by computing
* orthogonal U and W that move them to the top left corner of (A, B).
* In other words, the selected eigenvalues are the eigenvalues of
* (A11, B11) in:
*
* U'*(A, B)*W = (A11 A12) (B11 B12) n1
* ( 0 A22),( 0 B22) n2
* n1 n2 n1 n2
*
* where N = n1+n2 and U' means the transpose of U. The first n1 columns
* of U and W span the specified pair of left and right eigenspaces
* (deflating subspaces) of (A, B).
*
* If (A, B) has been obtained from the generalized real Schur
* decomposition of a matrix pair (C, D) = Q*(A, B)*Z', then the
* reordered generalized real Schur form of (C, D) is given by
*
* (C, D) = (Q*U)*(U'*(A, B)*W)*(Z*W)',
*
* and the first n1 columns of Q*U and Z*W span the corresponding
* deflating subspaces of (C, D) (Q and Z store Q*U and Z*W, resp.).
*
* Note that if the selected eigenvalue is sufficiently ill-conditioned,
* then its value may differ significantly from its value before
* reordering.
*
* The reciprocal condition numbers of the left and right eigenspaces
* spanned by the first n1 columns of U and W (or Q*U and Z*W) may
* be returned in DIF(1:2), corresponding to Difu and Difl, resp.
*
* The Difu and Difl are defined as:
*
* Difu[(A11, B11), (A22, B22)] = sigma-min( Zu )
* and
* Difl[(A11, B11), (A22, B22)] = Difu[(A22, B22), (A11, B11)],
*
* where sigma-min(Zu) is the smallest singular value of the
* (2*n1*n2)-by-(2*n1*n2) matrix
*
* Zu = [ kron(In2, A11) -kron(A22', In1) ]
* [ kron(In2, B11) -kron(B22', In1) ].
*
* Here, Inx is the identity matrix of size nx and A22' is the
* transpose of A22. kron(X, Y) is the Kronecker product between
* the matrices X and Y.
*
* When DIF(2) is small, small changes in (A, B) can cause large changes
* in the deflating subspace. An approximate (asymptotic) bound on the
* maximum angular error in the computed deflating subspaces is
*
* EPS * norm((A, B)) / DIF(2),
*
* where EPS is the machine precision.
*
* The reciprocal norm of the projectors on the left and right
* eigenspaces associated with (A11, B11) may be returned in PL and PR.
* They are computed as follows. First we compute L and R so that
* P*(A, B)*Q is block diagonal, where
*
* P = ( I -L ) n1 Q = ( I R ) n1
* ( 0 I ) n2 and ( 0 I ) n2
* n1 n2 n1 n2
*
* and (L, R) is the solution to the generalized Sylvester equation
*
* A11*R - L*A22 = -A12
* B11*R - L*B22 = -B12
*
* Then PL = (F-norm(L)**2+1)**(-1/2) and PR = (F-norm(R)**2+1)**(-1/2).
* An approximate (asymptotic) bound on the average absolute error of
* the selected eigenvalues is
*
* EPS * norm((A, B)) / PL.
*
* There are also global error bounds which valid for perturbations up
* to a certain restriction: A lower bound (x) on the smallest
* F-norm(E,F) for which an eigenvalue of (A11, B11) may move and
* coalesce with an eigenvalue of (A22, B22) under perturbation (E,F),
* (i.e. (A + E, B + F), is
*
* x = min(Difu,Difl)/((1/(PL*PL)+1/(PR*PR))**(1/2)+2*max(1/PL,1/PR)).
*
* An approximate bound on x can be computed from DIF(1:2), PL and PR.
*
* If y = ( F-norm(E,F) / x) <= 1, the angles between the perturbed
* (L', R') and unperturbed (L, R) left and right deflating subspaces
* associated with the selected cluster in the (1,1)-blocks can be
* bounded as
*
* max-angle(L, L') <= arctan( y * PL / (1 - y * (1 - PL * PL)**(1/2))
* max-angle(R, R') <= arctan( y * PR / (1 - y * (1 - PR * PR)**(1/2))
*
* See LAPACK User's Guide section 4.11 or the following references
* for more information.
*
* Note that if the default method for computing the Frobenius-norm-
* based estimate DIF is not wanted (see SLATDF), then the parameter
* IDIFJB (see below) should be changed from 3 to 4 (routine SLATDF
* (IJOB = 2 will be used)). See STGSYL for more details.
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* References
* ==========
*
* [1] B. Kagstrom; A Direct Method for Reordering Eigenvalues in the
* Generalized Real Schur Form of a Regular Matrix Pair (A, B), in
* M.S. Moonen et al (eds), Linear Algebra for Large Scale and
* Real-Time Applications, Kluwer Academic Publ. 1993, pp 195-218.
*
* [2] B. Kagstrom and P. Poromaa; Computing Eigenspaces with Specified
* Eigenvalues of a Regular Matrix Pair (A, B) and Condition
* Estimation: Theory, Algorithms and Software,
* Report UMINF - 94.04, Department of Computing Science, Umea
* University, S-901 87 Umea, Sweden, 1994. Also as LAPACK Working
* Note 87. To appear in Numerical Algorithms, 1996.
*
* [3] B. Kagstrom and P. Poromaa, LAPACK-Style Algorithms and Software
* for Solving the Generalized Sylvester Equation and Estimating the
* Separation between Regular Matrix Pairs, Report UMINF - 93.23,
* Department of Computing Science, Umea University, S-901 87 Umea,
* Sweden, December 1993, Revised April 1994, Also as LAPACK Working
* Note 75. To appear in ACM Trans. on Math. Software, Vol 22, No 1,
* 1996.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param ijob
* @param wantq
* @param wantz
* @param select
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param alphar
* @param alphai
* @param beta
* @param q
* @param ldq
* @param z
* @param ldz
* @param m
* @param pl
* @param pr
* @param dif
* @param work
* @param lwork
* @param iwork
* @param liwork
* @param info
*
*/
abstract public void stgsen(int ijob, boolean wantq, boolean wantz, boolean[] select, int n, float[] a, int lda, float[] b, int ldb, float[] alphar, float[] alphai, float[] beta, float[] q, int ldq, float[] z, int ldz, org.netlib.util.intW m, org.netlib.util.floatW pl, org.netlib.util.floatW pr, float[] dif, float[] work, int lwork, int[] iwork, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STGSEN reorders the generalized real Schur decomposition of a real
* matrix pair (A, B) (in terms of an orthonormal equivalence trans-
* formation Q' * (A, B) * Z), so that a selected cluster of eigenvalues
* appears in the leading diagonal blocks of the upper quasi-triangular
* matrix A and the upper triangular B. The leading columns of Q and
* Z form orthonormal bases of the corresponding left and right eigen-
* spaces (deflating subspaces). (A, B) must be in generalized real
* Schur canonical form (as returned by SGGES), i.e. A is block upper
* triangular with 1-by-1 and 2-by-2 diagonal blocks. B is upper
* triangular.
*
* STGSEN also computes the generalized eigenvalues
*
* w(j) = (ALPHAR(j) + i*ALPHAI(j))/BETA(j)
*
* of the reordered matrix pair (A, B).
*
* Optionally, STGSEN computes the estimates of reciprocal condition
* numbers for eigenvalues and eigenspaces. These are Difu[(A11,B11),
* (A22,B22)] and Difl[(A11,B11), (A22,B22)], i.e. the separation(s)
* between the matrix pairs (A11, B11) and (A22,B22) that correspond to
* the selected cluster and the eigenvalues outside the cluster, resp.,
* and norms of "projections" onto left and right eigenspaces w.r.t.
* the selected cluster in the (1,1)-block.
*
* Arguments
* =========
*
* IJOB (input) INTEGER
* Specifies whether condition numbers are required for the
* cluster of eigenvalues (PL and PR) or the deflating subspaces
* (Difu and Difl):
* =0: Only reorder w.r.t. SELECT. No extras.
* =1: Reciprocal of norms of "projections" onto left and right
* eigenspaces w.r.t. the selected cluster (PL and PR).
* =2: Upper bounds on Difu and Difl. F-norm-based estimate
* (DIF(1:2)).
* =3: Estimate of Difu and Difl. 1-norm-based estimate
* (DIF(1:2)).
* About 5 times as expensive as IJOB = 2.
* =4: Compute PL, PR and DIF (i.e. 0, 1 and 2 above): Economic
* version to get it all.
* =5: Compute PL, PR and DIF (i.e. 0, 1 and 3 above)
*
* WANTQ (input) LOGICAL
* .TRUE. : update the left transformation matrix Q;
* .FALSE.: do not update Q.
*
* WANTZ (input) LOGICAL
* .TRUE. : update the right transformation matrix Z;
* .FALSE.: do not update Z.
*
* SELECT (input) LOGICAL array, dimension (N)
* SELECT specifies the eigenvalues in the selected cluster.
* To select a real eigenvalue w(j), SELECT(j) must be set to
* .TRUE.. To select a complex conjugate pair of eigenvalues
* w(j) and w(j+1), corresponding to a 2-by-2 diagonal block,
* either SELECT(j) or SELECT(j+1) or both must be set to
* .TRUE.; a complex conjugate pair of eigenvalues must be
* either both included in the cluster or both excluded.
*
* N (input) INTEGER
* The order of the matrices A and B. N >= 0.
*
* A (input/output) REAL array, dimension(LDA,N)
* On entry, the upper quasi-triangular matrix A, with (A, B) in
* generalized real Schur canonical form.
* On exit, A is overwritten by the reordered matrix A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension(LDB,N)
* On entry, the upper triangular matrix B, with (A, B) in
* generalized real Schur canonical form.
* On exit, B is overwritten by the reordered matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* ALPHAR (output) REAL array, dimension (N)
* ALPHAI (output) REAL array, dimension (N)
* BETA (output) REAL array, dimension (N)
* On exit, (ALPHAR(j) + ALPHAI(j)*i)/BETA(j), j=1,...,N, will
* be the generalized eigenvalues. ALPHAR(j) + ALPHAI(j)*i
* and BETA(j),j=1,...,N are the diagonals of the complex Schur
* form (S,T) that would result if the 2-by-2 diagonal blocks of
* the real generalized Schur form of (A,B) were further reduced
* to triangular form using complex unitary transformations.
* If ALPHAI(j) is zero, then the j-th eigenvalue is real; if
* positive, then the j-th and (j+1)-st eigenvalues are a
* complex conjugate pair, with ALPHAI(j+1) negative.
*
* Q (input/output) REAL array, dimension (LDQ,N)
* On entry, if WANTQ = .TRUE., Q is an N-by-N matrix.
* On exit, Q has been postmultiplied by the left orthogonal
* transformation matrix which reorder (A, B); The leading M
* columns of Q form orthonormal bases for the specified pair of
* left eigenspaces (deflating subspaces).
* If WANTQ = .FALSE., Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= 1;
* and if WANTQ = .TRUE., LDQ >= N.
*
* Z (input/output) REAL array, dimension (LDZ,N)
* On entry, if WANTZ = .TRUE., Z is an N-by-N matrix.
* On exit, Z has been postmultiplied by the left orthogonal
* transformation matrix which reorder (A, B); The leading M
* columns of Z form orthonormal bases for the specified pair of
* left eigenspaces (deflating subspaces).
* If WANTZ = .FALSE., Z is not referenced.
*
* LDZ (input) INTEGER
* The leading dimension of the array Z. LDZ >= 1;
* If WANTZ = .TRUE., LDZ >= N.
*
* M (output) INTEGER
* The dimension of the specified pair of left and right eigen-
* spaces (deflating subspaces). 0 <= M <= N.
*
* PL (output) REAL
* PR (output) REAL
* If IJOB = 1, 4 or 5, PL, PR are lower bounds on the
* reciprocal of the norm of "projections" onto left and right
* eigenspaces with respect to the selected cluster.
* 0 < PL, PR <= 1.
* If M = 0 or M = N, PL = PR = 1.
* If IJOB = 0, 2 or 3, PL and PR are not referenced.
*
* DIF (output) REAL array, dimension (2).
* If IJOB >= 2, DIF(1:2) store the estimates of Difu and Difl.
* If IJOB = 2 or 4, DIF(1:2) are F-norm-based upper bounds on
* Difu and Difl. If IJOB = 3 or 5, DIF(1:2) are 1-norm-based
* estimates of Difu and Difl.
* If M = 0 or N, DIF(1:2) = F-norm([A, B]).
* If IJOB = 0 or 1, DIF is not referenced.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= 4*N+16.
* If IJOB = 1, 2 or 4, LWORK >= MAX(4*N+16, 2*M*(N-M)).
* If IJOB = 3 or 5, LWORK >= MAX(4*N+16, 4*M*(N-M)).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace/output) INTEGER array, dimension (MAX(1,LIWORK))
* IF IJOB = 0, IWORK is not referenced. Otherwise,
* on exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK. LIWORK >= 1.
* If IJOB = 1, 2 or 4, LIWORK >= N+6.
* If IJOB = 3 or 5, LIWORK >= MAX(2*M*(N-M), N+6).
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal size of the IWORK array,
* returns this value as the first entry of the IWORK array, and
* no error message related to LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* =0: Successful exit.
* <0: If INFO = -i, the i-th argument had an illegal value.
* =1: Reordering of (A, B) failed because the transformed
* matrix pair (A, B) would be too far from generalized
* Schur form; the problem is very ill-conditioned.
* (A, B) may have been partially reordered.
* If requested, 0 is returned in DIF(*), PL and PR.
*
* Further Details
* ===============
*
* STGSEN first collects the selected eigenvalues by computing
* orthogonal U and W that move them to the top left corner of (A, B).
* In other words, the selected eigenvalues are the eigenvalues of
* (A11, B11) in:
*
* U'*(A, B)*W = (A11 A12) (B11 B12) n1
* ( 0 A22),( 0 B22) n2
* n1 n2 n1 n2
*
* where N = n1+n2 and U' means the transpose of U. The first n1 columns
* of U and W span the specified pair of left and right eigenspaces
* (deflating subspaces) of (A, B).
*
* If (A, B) has been obtained from the generalized real Schur
* decomposition of a matrix pair (C, D) = Q*(A, B)*Z', then the
* reordered generalized real Schur form of (C, D) is given by
*
* (C, D) = (Q*U)*(U'*(A, B)*W)*(Z*W)',
*
* and the first n1 columns of Q*U and Z*W span the corresponding
* deflating subspaces of (C, D) (Q and Z store Q*U and Z*W, resp.).
*
* Note that if the selected eigenvalue is sufficiently ill-conditioned,
* then its value may differ significantly from its value before
* reordering.
*
* The reciprocal condition numbers of the left and right eigenspaces
* spanned by the first n1 columns of U and W (or Q*U and Z*W) may
* be returned in DIF(1:2), corresponding to Difu and Difl, resp.
*
* The Difu and Difl are defined as:
*
* Difu[(A11, B11), (A22, B22)] = sigma-min( Zu )
* and
* Difl[(A11, B11), (A22, B22)] = Difu[(A22, B22), (A11, B11)],
*
* where sigma-min(Zu) is the smallest singular value of the
* (2*n1*n2)-by-(2*n1*n2) matrix
*
* Zu = [ kron(In2, A11) -kron(A22', In1) ]
* [ kron(In2, B11) -kron(B22', In1) ].
*
* Here, Inx is the identity matrix of size nx and A22' is the
* transpose of A22. kron(X, Y) is the Kronecker product between
* the matrices X and Y.
*
* When DIF(2) is small, small changes in (A, B) can cause large changes
* in the deflating subspace. An approximate (asymptotic) bound on the
* maximum angular error in the computed deflating subspaces is
*
* EPS * norm((A, B)) / DIF(2),
*
* where EPS is the machine precision.
*
* The reciprocal norm of the projectors on the left and right
* eigenspaces associated with (A11, B11) may be returned in PL and PR.
* They are computed as follows. First we compute L and R so that
* P*(A, B)*Q is block diagonal, where
*
* P = ( I -L ) n1 Q = ( I R ) n1
* ( 0 I ) n2 and ( 0 I ) n2
* n1 n2 n1 n2
*
* and (L, R) is the solution to the generalized Sylvester equation
*
* A11*R - L*A22 = -A12
* B11*R - L*B22 = -B12
*
* Then PL = (F-norm(L)**2+1)**(-1/2) and PR = (F-norm(R)**2+1)**(-1/2).
* An approximate (asymptotic) bound on the average absolute error of
* the selected eigenvalues is
*
* EPS * norm((A, B)) / PL.
*
* There are also global error bounds which valid for perturbations up
* to a certain restriction: A lower bound (x) on the smallest
* F-norm(E,F) for which an eigenvalue of (A11, B11) may move and
* coalesce with an eigenvalue of (A22, B22) under perturbation (E,F),
* (i.e. (A + E, B + F), is
*
* x = min(Difu,Difl)/((1/(PL*PL)+1/(PR*PR))**(1/2)+2*max(1/PL,1/PR)).
*
* An approximate bound on x can be computed from DIF(1:2), PL and PR.
*
* If y = ( F-norm(E,F) / x) <= 1, the angles between the perturbed
* (L', R') and unperturbed (L, R) left and right deflating subspaces
* associated with the selected cluster in the (1,1)-blocks can be
* bounded as
*
* max-angle(L, L') <= arctan( y * PL / (1 - y * (1 - PL * PL)**(1/2))
* max-angle(R, R') <= arctan( y * PR / (1 - y * (1 - PR * PR)**(1/2))
*
* See LAPACK User's Guide section 4.11 or the following references
* for more information.
*
* Note that if the default method for computing the Frobenius-norm-
* based estimate DIF is not wanted (see SLATDF), then the parameter
* IDIFJB (see below) should be changed from 3 to 4 (routine SLATDF
* (IJOB = 2 will be used)). See STGSYL for more details.
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* References
* ==========
*
* [1] B. Kagstrom; A Direct Method for Reordering Eigenvalues in the
* Generalized Real Schur Form of a Regular Matrix Pair (A, B), in
* M.S. Moonen et al (eds), Linear Algebra for Large Scale and
* Real-Time Applications, Kluwer Academic Publ. 1993, pp 195-218.
*
* [2] B. Kagstrom and P. Poromaa; Computing Eigenspaces with Specified
* Eigenvalues of a Regular Matrix Pair (A, B) and Condition
* Estimation: Theory, Algorithms and Software,
* Report UMINF - 94.04, Department of Computing Science, Umea
* University, S-901 87 Umea, Sweden, 1994. Also as LAPACK Working
* Note 87. To appear in Numerical Algorithms, 1996.
*
* [3] B. Kagstrom and P. Poromaa, LAPACK-Style Algorithms and Software
* for Solving the Generalized Sylvester Equation and Estimating the
* Separation between Regular Matrix Pairs, Report UMINF - 93.23,
* Department of Computing Science, Umea University, S-901 87 Umea,
* Sweden, December 1993, Revised April 1994, Also as LAPACK Working
* Note 75. To appear in ACM Trans. on Math. Software, Vol 22, No 1,
* 1996.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param ijob
* @param wantq
* @param wantz
* @param select
* @param _select_offset
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param alphar
* @param _alphar_offset
* @param alphai
* @param _alphai_offset
* @param beta
* @param _beta_offset
* @param q
* @param _q_offset
* @param ldq
* @param z
* @param _z_offset
* @param ldz
* @param m
* @param pl
* @param pr
* @param dif
* @param _dif_offset
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param liwork
* @param info
*
*/
abstract public void stgsen(int ijob, boolean wantq, boolean wantz, boolean[] select, int _select_offset, int n, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, float[] alphar, int _alphar_offset, float[] alphai, int _alphai_offset, float[] beta, int _beta_offset, float[] q, int _q_offset, int ldq, float[] z, int _z_offset, int ldz, org.netlib.util.intW m, org.netlib.util.floatW pl, org.netlib.util.floatW pr, float[] dif, int _dif_offset, float[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STGSJA computes the generalized singular value decomposition (GSVD)
* of two real upper triangular (or trapezoidal) matrices A and B.
*
* On entry, it is assumed that matrices A and B have the following
* forms, which may be obtained by the preprocessing subroutine SGGSVP
* from a general M-by-N matrix A and P-by-N matrix B:
*
* N-K-L K L
* A = K ( 0 A12 A13 ) if M-K-L >= 0;
* L ( 0 0 A23 )
* M-K-L ( 0 0 0 )
*
* N-K-L K L
* A = K ( 0 A12 A13 ) if M-K-L < 0;
* M-K ( 0 0 A23 )
*
* N-K-L K L
* B = L ( 0 0 B13 )
* P-L ( 0 0 0 )
*
* where the K-by-K matrix A12 and L-by-L matrix B13 are nonsingular
* upper triangular; A23 is L-by-L upper triangular if M-K-L >= 0,
* otherwise A23 is (M-K)-by-L upper trapezoidal.
*
* On exit,
*
* U'*A*Q = D1*( 0 R ), V'*B*Q = D2*( 0 R ),
*
* where U, V and Q are orthogonal matrices, Z' denotes the transpose
* of Z, R is a nonsingular upper triangular matrix, and D1 and D2 are
* ``diagonal'' matrices, which are of the following structures:
*
* If M-K-L >= 0,
*
* K L
* D1 = K ( I 0 )
* L ( 0 C )
* M-K-L ( 0 0 )
*
* K L
* D2 = L ( 0 S )
* P-L ( 0 0 )
*
* N-K-L K L
* ( 0 R ) = K ( 0 R11 R12 ) K
* L ( 0 0 R22 ) L
*
* where
*
* C = diag( ALPHA(K+1), ... , ALPHA(K+L) ),
* S = diag( BETA(K+1), ... , BETA(K+L) ),
* C**2 + S**2 = I.
*
* R is stored in A(1:K+L,N-K-L+1:N) on exit.
*
* If M-K-L < 0,
*
* K M-K K+L-M
* D1 = K ( I 0 0 )
* M-K ( 0 C 0 )
*
* K M-K K+L-M
* D2 = M-K ( 0 S 0 )
* K+L-M ( 0 0 I )
* P-L ( 0 0 0 )
*
* N-K-L K M-K K+L-M
* ( 0 R ) = K ( 0 R11 R12 R13 )
* M-K ( 0 0 R22 R23 )
* K+L-M ( 0 0 0 R33 )
*
* where
* C = diag( ALPHA(K+1), ... , ALPHA(M) ),
* S = diag( BETA(K+1), ... , BETA(M) ),
* C**2 + S**2 = I.
*
* R = ( R11 R12 R13 ) is stored in A(1:M, N-K-L+1:N) and R33 is stored
* ( 0 R22 R23 )
* in B(M-K+1:L,N+M-K-L+1:N) on exit.
*
* The computation of the orthogonal transformation matrices U, V or Q
* is optional. These matrices may either be formed explicitly, or they
* may be postmultiplied into input matrices U1, V1, or Q1.
*
* Arguments
* =========
*
* JOBU (input) CHARACTER*1
* = 'U': U must contain an orthogonal matrix U1 on entry, and
* the product U1*U is returned;
* = 'I': U is initialized to the unit matrix, and the
* orthogonal matrix U is returned;
* = 'N': U is not computed.
*
* JOBV (input) CHARACTER*1
* = 'V': V must contain an orthogonal matrix V1 on entry, and
* the product V1*V is returned;
* = 'I': V is initialized to the unit matrix, and the
* orthogonal matrix V is returned;
* = 'N': V is not computed.
*
* JOBQ (input) CHARACTER*1
* = 'Q': Q must contain an orthogonal matrix Q1 on entry, and
* the product Q1*Q is returned;
* = 'I': Q is initialized to the unit matrix, and the
* orthogonal matrix Q is returned;
* = 'N': Q is not computed.
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* P (input) INTEGER
* The number of rows of the matrix B. P >= 0.
*
* N (input) INTEGER
* The number of columns of the matrices A and B. N >= 0.
*
* K (input) INTEGER
* L (input) INTEGER
* K and L specify the subblocks in the input matrices A and B:
* A23 = A(K+1:MIN(K+L,M),N-L+1:N) and B13 = B(1:L,N-L+1:N)
* of A and B, whose GSVD is going to be computed by STGSJA.
* See Further details.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, A(N-K+1:N,1:MIN(K+L,M) ) contains the triangular
* matrix R or part of R. See Purpose for details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) REAL array, dimension (LDB,N)
* On entry, the P-by-N matrix B.
* On exit, if necessary, B(M-K+1:L,N+M-K-L+1:N) contains
* a part of R. See Purpose for details.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,P).
*
* TOLA (input) REAL
* TOLB (input) REAL
* TOLA and TOLB are the convergence criteria for the Jacobi-
* Kogbetliantz iteration procedure. Generally, they are the
* same as used in the preprocessing step, say
* TOLA = max(M,N)*norm(A)*MACHEPS,
* TOLB = max(P,N)*norm(B)*MACHEPS.
*
* ALPHA (output) REAL array, dimension (N)
* BETA (output) REAL array, dimension (N)
* On exit, ALPHA and BETA contain the generalized singular
* value pairs of A and B;
* ALPHA(1:K) = 1,
* BETA(1:K) = 0,
* and if M-K-L >= 0,
* ALPHA(K+1:K+L) = diag(C),
* BETA(K+1:K+L) = diag(S),
* or if M-K-L < 0,
* ALPHA(K+1:M)= C, ALPHA(M+1:K+L)= 0
* BETA(K+1:M) = S, BETA(M+1:K+L) = 1.
* Furthermore, if K+L < N,
* ALPHA(K+L+1:N) = 0 and
* BETA(K+L+1:N) = 0.
*
* U (input/output) REAL array, dimension (LDU,M)
* On entry, if JOBU = 'U', U must contain a matrix U1 (usually
* the orthogonal matrix returned by SGGSVP).
* On exit,
* if JOBU = 'I', U contains the orthogonal matrix U;
* if JOBU = 'U', U contains the product U1*U.
* If JOBU = 'N', U is not referenced.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= max(1,M) if
* JOBU = 'U'; LDU >= 1 otherwise.
*
* V (input/output) REAL array, dimension (LDV,P)
* On entry, if JOBV = 'V', V must contain a matrix V1 (usually
* the orthogonal matrix returned by SGGSVP).
* On exit,
* if JOBV = 'I', V contains the orthogonal matrix V;
* if JOBV = 'V', V contains the product V1*V.
* If JOBV = 'N', V is not referenced.
*
* LDV (input) INTEGER
* The leading dimension of the array V. LDV >= max(1,P) if
* JOBV = 'V'; LDV >= 1 otherwise.
*
* Q (input/output) REAL array, dimension (LDQ,N)
* On entry, if JOBQ = 'Q', Q must contain a matrix Q1 (usually
* the orthogonal matrix returned by SGGSVP).
* On exit,
* if JOBQ = 'I', Q contains the orthogonal matrix Q;
* if JOBQ = 'Q', Q contains the product Q1*Q.
* If JOBQ = 'N', Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N) if
* JOBQ = 'Q'; LDQ >= 1 otherwise.
*
* WORK (workspace) REAL array, dimension (2*N)
*
* NCYCLE (output) INTEGER
* The number of cycles required for convergence.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1: the procedure does not converge after MAXIT cycles.
*
* Internal Parameters
* ===================
*
* MAXIT INTEGER
* MAXIT specifies the total loops that the iterative procedure
* may take. If after MAXIT cycles, the routine fails to
* converge, we return INFO = 1.
*
* Further Details
* ===============
*
* STGSJA essentially uses a variant of Kogbetliantz algorithm to reduce
* min(L,M-K)-by-L triangular (or trapezoidal) matrix A23 and L-by-L
* matrix B13 to the form:
*
* U1'*A13*Q1 = C1*R1; V1'*B13*Q1 = S1*R1,
*
* where U1, V1 and Q1 are orthogonal matrix, and Z' is the transpose
* of Z. C1 and S1 are diagonal matrices satisfying
*
* C1**2 + S1**2 = I,
*
* and R1 is an L-by-L nonsingular upper triangular matrix.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobu
* @param jobv
* @param jobq
* @param m
* @param p
* @param n
* @param k
* @param l
* @param a
* @param lda
* @param b
* @param ldb
* @param tola
* @param tolb
* @param alpha
* @param beta
* @param u
* @param ldu
* @param v
* @param ldv
* @param q
* @param ldq
* @param work
* @param ncycle
* @param info
*
*/
abstract public void stgsja(java.lang.String jobu, java.lang.String jobv, java.lang.String jobq, int m, int p, int n, int k, int l, float[] a, int lda, float[] b, int ldb, float tola, float tolb, float[] alpha, float[] beta, float[] u, int ldu, float[] v, int ldv, float[] q, int ldq, float[] work, org.netlib.util.intW ncycle, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STGSJA computes the generalized singular value decomposition (GSVD)
* of two real upper triangular (or trapezoidal) matrices A and B.
*
* On entry, it is assumed that matrices A and B have the following
* forms, which may be obtained by the preprocessing subroutine SGGSVP
* from a general M-by-N matrix A and P-by-N matrix B:
*
* N-K-L K L
* A = K ( 0 A12 A13 ) if M-K-L >= 0;
* L ( 0 0 A23 )
* M-K-L ( 0 0 0 )
*
* N-K-L K L
* A = K ( 0 A12 A13 ) if M-K-L < 0;
* M-K ( 0 0 A23 )
*
* N-K-L K L
* B = L ( 0 0 B13 )
* P-L ( 0 0 0 )
*
* where the K-by-K matrix A12 and L-by-L matrix B13 are nonsingular
* upper triangular; A23 is L-by-L upper triangular if M-K-L >= 0,
* otherwise A23 is (M-K)-by-L upper trapezoidal.
*
* On exit,
*
* U'*A*Q = D1*( 0 R ), V'*B*Q = D2*( 0 R ),
*
* where U, V and Q are orthogonal matrices, Z' denotes the transpose
* of Z, R is a nonsingular upper triangular matrix, and D1 and D2 are
* ``diagonal'' matrices, which are of the following structures:
*
* If M-K-L >= 0,
*
* K L
* D1 = K ( I 0 )
* L ( 0 C )
* M-K-L ( 0 0 )
*
* K L
* D2 = L ( 0 S )
* P-L ( 0 0 )
*
* N-K-L K L
* ( 0 R ) = K ( 0 R11 R12 ) K
* L ( 0 0 R22 ) L
*
* where
*
* C = diag( ALPHA(K+1), ... , ALPHA(K+L) ),
* S = diag( BETA(K+1), ... , BETA(K+L) ),
* C**2 + S**2 = I.
*
* R is stored in A(1:K+L,N-K-L+1:N) on exit.
*
* If M-K-L < 0,
*
* K M-K K+L-M
* D1 = K ( I 0 0 )
* M-K ( 0 C 0 )
*
* K M-K K+L-M
* D2 = M-K ( 0 S 0 )
* K+L-M ( 0 0 I )
* P-L ( 0 0 0 )
*
* N-K-L K M-K K+L-M
* ( 0 R ) = K ( 0 R11 R12 R13 )
* M-K ( 0 0 R22 R23 )
* K+L-M ( 0 0 0 R33 )
*
* where
* C = diag( ALPHA(K+1), ... , ALPHA(M) ),
* S = diag( BETA(K+1), ... , BETA(M) ),
* C**2 + S**2 = I.
*
* R = ( R11 R12 R13 ) is stored in A(1:M, N-K-L+1:N) and R33 is stored
* ( 0 R22 R23 )
* in B(M-K+1:L,N+M-K-L+1:N) on exit.
*
* The computation of the orthogonal transformation matrices U, V or Q
* is optional. These matrices may either be formed explicitly, or they
* may be postmultiplied into input matrices U1, V1, or Q1.
*
* Arguments
* =========
*
* JOBU (input) CHARACTER*1
* = 'U': U must contain an orthogonal matrix U1 on entry, and
* the product U1*U is returned;
* = 'I': U is initialized to the unit matrix, and the
* orthogonal matrix U is returned;
* = 'N': U is not computed.
*
* JOBV (input) CHARACTER*1
* = 'V': V must contain an orthogonal matrix V1 on entry, and
* the product V1*V is returned;
* = 'I': V is initialized to the unit matrix, and the
* orthogonal matrix V is returned;
* = 'N': V is not computed.
*
* JOBQ (input) CHARACTER*1
* = 'Q': Q must contain an orthogonal matrix Q1 on entry, and
* the product Q1*Q is returned;
* = 'I': Q is initialized to the unit matrix, and the
* orthogonal matrix Q is returned;
* = 'N': Q is not computed.
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* P (input) INTEGER
* The number of rows of the matrix B. P >= 0.
*
* N (input) INTEGER
* The number of columns of the matrices A and B. N >= 0.
*
* K (input) INTEGER
* L (input) INTEGER
* K and L specify the subblocks in the input matrices A and B:
* A23 = A(K+1:MIN(K+L,M),N-L+1:N) and B13 = B(1:L,N-L+1:N)
* of A and B, whose GSVD is going to be computed by STGSJA.
* See Further details.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the M-by-N matrix A.
* On exit, A(N-K+1:N,1:MIN(K+L,M) ) contains the triangular
* matrix R or part of R. See Purpose for details.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input/output) REAL array, dimension (LDB,N)
* On entry, the P-by-N matrix B.
* On exit, if necessary, B(M-K+1:L,N+M-K-L+1:N) contains
* a part of R. See Purpose for details.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,P).
*
* TOLA (input) REAL
* TOLB (input) REAL
* TOLA and TOLB are the convergence criteria for the Jacobi-
* Kogbetliantz iteration procedure. Generally, they are the
* same as used in the preprocessing step, say
* TOLA = max(M,N)*norm(A)*MACHEPS,
* TOLB = max(P,N)*norm(B)*MACHEPS.
*
* ALPHA (output) REAL array, dimension (N)
* BETA (output) REAL array, dimension (N)
* On exit, ALPHA and BETA contain the generalized singular
* value pairs of A and B;
* ALPHA(1:K) = 1,
* BETA(1:K) = 0,
* and if M-K-L >= 0,
* ALPHA(K+1:K+L) = diag(C),
* BETA(K+1:K+L) = diag(S),
* or if M-K-L < 0,
* ALPHA(K+1:M)= C, ALPHA(M+1:K+L)= 0
* BETA(K+1:M) = S, BETA(M+1:K+L) = 1.
* Furthermore, if K+L < N,
* ALPHA(K+L+1:N) = 0 and
* BETA(K+L+1:N) = 0.
*
* U (input/output) REAL array, dimension (LDU,M)
* On entry, if JOBU = 'U', U must contain a matrix U1 (usually
* the orthogonal matrix returned by SGGSVP).
* On exit,
* if JOBU = 'I', U contains the orthogonal matrix U;
* if JOBU = 'U', U contains the product U1*U.
* If JOBU = 'N', U is not referenced.
*
* LDU (input) INTEGER
* The leading dimension of the array U. LDU >= max(1,M) if
* JOBU = 'U'; LDU >= 1 otherwise.
*
* V (input/output) REAL array, dimension (LDV,P)
* On entry, if JOBV = 'V', V must contain a matrix V1 (usually
* the orthogonal matrix returned by SGGSVP).
* On exit,
* if JOBV = 'I', V contains the orthogonal matrix V;
* if JOBV = 'V', V contains the product V1*V.
* If JOBV = 'N', V is not referenced.
*
* LDV (input) INTEGER
* The leading dimension of the array V. LDV >= max(1,P) if
* JOBV = 'V'; LDV >= 1 otherwise.
*
* Q (input/output) REAL array, dimension (LDQ,N)
* On entry, if JOBQ = 'Q', Q must contain a matrix Q1 (usually
* the orthogonal matrix returned by SGGSVP).
* On exit,
* if JOBQ = 'I', Q contains the orthogonal matrix Q;
* if JOBQ = 'Q', Q contains the product Q1*Q.
* If JOBQ = 'N', Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N) if
* JOBQ = 'Q'; LDQ >= 1 otherwise.
*
* WORK (workspace) REAL array, dimension (2*N)
*
* NCYCLE (output) INTEGER
* The number of cycles required for convergence.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value.
* = 1: the procedure does not converge after MAXIT cycles.
*
* Internal Parameters
* ===================
*
* MAXIT INTEGER
* MAXIT specifies the total loops that the iterative procedure
* may take. If after MAXIT cycles, the routine fails to
* converge, we return INFO = 1.
*
* Further Details
* ===============
*
* STGSJA essentially uses a variant of Kogbetliantz algorithm to reduce
* min(L,M-K)-by-L triangular (or trapezoidal) matrix A23 and L-by-L
* matrix B13 to the form:
*
* U1'*A13*Q1 = C1*R1; V1'*B13*Q1 = S1*R1,
*
* where U1, V1 and Q1 are orthogonal matrix, and Z' is the transpose
* of Z. C1 and S1 are diagonal matrices satisfying
*
* C1**2 + S1**2 = I,
*
* and R1 is an L-by-L nonsingular upper triangular matrix.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param jobu
* @param jobv
* @param jobq
* @param m
* @param p
* @param n
* @param k
* @param l
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param tola
* @param tolb
* @param alpha
* @param _alpha_offset
* @param beta
* @param _beta_offset
* @param u
* @param _u_offset
* @param ldu
* @param v
* @param _v_offset
* @param ldv
* @param q
* @param _q_offset
* @param ldq
* @param work
* @param _work_offset
* @param ncycle
* @param info
*
*/
abstract public void stgsja(java.lang.String jobu, java.lang.String jobv, java.lang.String jobq, int m, int p, int n, int k, int l, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, float tola, float tolb, float[] alpha, int _alpha_offset, float[] beta, int _beta_offset, float[] u, int _u_offset, int ldu, float[] v, int _v_offset, int ldv, float[] q, int _q_offset, int ldq, float[] work, int _work_offset, org.netlib.util.intW ncycle, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STGSNA estimates reciprocal condition numbers for specified
* eigenvalues and/or eigenvectors of a matrix pair (A, B) in
* generalized real Schur canonical form (or of any matrix pair
* (Q*A*Z', Q*B*Z') with orthogonal matrices Q and Z, where
* Z' denotes the transpose of Z.
*
* (A, B) must be in generalized real Schur form (as returned by SGGES),
* i.e. A is block upper triangular with 1-by-1 and 2-by-2 diagonal
* blocks. B is upper triangular.
*
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* Specifies whether condition numbers are required for
* eigenvalues (S) or eigenvectors (DIF):
* = 'E': for eigenvalues only (S);
* = 'V': for eigenvectors only (DIF);
* = 'B': for both eigenvalues and eigenvectors (S and DIF).
*
* HOWMNY (input) CHARACTER*1
* = 'A': compute condition numbers for all eigenpairs;
* = 'S': compute condition numbers for selected eigenpairs
* specified by the array SELECT.
*
* SELECT (input) LOGICAL array, dimension (N)
* If HOWMNY = 'S', SELECT specifies the eigenpairs for which
* condition numbers are required. To select condition numbers
* for the eigenpair corresponding to a real eigenvalue w(j),
* SELECT(j) must be set to .TRUE.. To select condition numbers
* corresponding to a complex conjugate pair of eigenvalues w(j)
* and w(j+1), either SELECT(j) or SELECT(j+1) or both, must be
* set to .TRUE..
* If HOWMNY = 'A', SELECT is not referenced.
*
* N (input) INTEGER
* The order of the square matrix pair (A, B). N >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The upper quasi-triangular matrix A in the pair (A,B).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input) REAL array, dimension (LDB,N)
* The upper triangular matrix B in the pair (A,B).
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* VL (input) REAL array, dimension (LDVL,M)
* If JOB = 'E' or 'B', VL must contain left eigenvectors of
* (A, B), corresponding to the eigenpairs specified by HOWMNY
* and SELECT. The eigenvectors must be stored in consecutive
* columns of VL, as returned by STGEVC.
* If JOB = 'V', VL is not referenced.
*
* LDVL (input) INTEGER
* The leading dimension of the array VL. LDVL >= 1.
* If JOB = 'E' or 'B', LDVL >= N.
*
* VR (input) REAL array, dimension (LDVR,M)
* If JOB = 'E' or 'B', VR must contain right eigenvectors of
* (A, B), corresponding to the eigenpairs specified by HOWMNY
* and SELECT. The eigenvectors must be stored in consecutive
* columns ov VR, as returned by STGEVC.
* If JOB = 'V', VR is not referenced.
*
* LDVR (input) INTEGER
* The leading dimension of the array VR. LDVR >= 1.
* If JOB = 'E' or 'B', LDVR >= N.
*
* S (output) REAL array, dimension (MM)
* If JOB = 'E' or 'B', the reciprocal condition numbers of the
* selected eigenvalues, stored in consecutive elements of the
* array. For a complex conjugate pair of eigenvalues two
* consecutive elements of S are set to the same value. Thus
* S(j), DIF(j), and the j-th columns of VL and VR all
* correspond to the same eigenpair (but not in general the
* j-th eigenpair, unless all eigenpairs are selected).
* If JOB = 'V', S is not referenced.
*
* DIF (output) REAL array, dimension (MM)
* If JOB = 'V' or 'B', the estimated reciprocal condition
* numbers of the selected eigenvectors, stored in consecutive
* elements of the array. For a complex eigenvector two
* consecutive elements of DIF are set to the same value. If
* the eigenvalues cannot be reordered to compute DIF(j), DIF(j)
* is set to 0; this can only occur when the true value would be
* very small anyway.
* If JOB = 'E', DIF is not referenced.
*
* MM (input) INTEGER
* The number of elements in the arrays S and DIF. MM >= M.
*
* M (output) INTEGER
* The number of elements of the arrays S and DIF used to store
* the specified condition numbers; for each selected real
* eigenvalue one element is used, and for each selected complex
* conjugate pair of eigenvalues, two elements are used.
* If HOWMNY = 'A', M is set to N.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N).
* If JOB = 'V' or 'B' LWORK >= 2*N*(N+2)+16.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (N + 6)
* If JOB = 'E', IWORK is not referenced.
*
* INFO (output) INTEGER
* =0: Successful exit
* <0: If INFO = -i, the i-th argument had an illegal value
*
*
* Further Details
* ===============
*
* The reciprocal of the condition number of a generalized eigenvalue
* w = (a, b) is defined as
*
* S(w) = (|u'Av|**2 + |u'Bv|**2)**(1/2) / (norm(u)*norm(v))
*
* where u and v are the left and right eigenvectors of (A, B)
* corresponding to w; |z| denotes the absolute value of the complex
* number, and norm(u) denotes the 2-norm of the vector u.
* The pair (a, b) corresponds to an eigenvalue w = a/b (= u'Av/u'Bv)
* of the matrix pair (A, B). If both a and b equal zero, then (A B) is
* singular and S(I) = -1 is returned.
*
* An approximate error bound on the chordal distance between the i-th
* computed generalized eigenvalue w and the corresponding exact
* eigenvalue lambda is
*
* chord(w, lambda) <= EPS * norm(A, B) / S(I)
*
* where EPS is the machine precision.
*
* The reciprocal of the condition number DIF(i) of right eigenvector u
* and left eigenvector v corresponding to the generalized eigenvalue w
* is defined as follows:
*
* a) If the i-th eigenvalue w = (a,b) is real
*
* Suppose U and V are orthogonal transformations such that
*
* U'*(A, B)*V = (S, T) = ( a * ) ( b * ) 1
* ( 0 S22 ),( 0 T22 ) n-1
* 1 n-1 1 n-1
*
* Then the reciprocal condition number DIF(i) is
*
* Difl((a, b), (S22, T22)) = sigma-min( Zl ),
*
* where sigma-min(Zl) denotes the smallest singular value of the
* 2(n-1)-by-2(n-1) matrix
*
* Zl = [ kron(a, In-1) -kron(1, S22) ]
* [ kron(b, In-1) -kron(1, T22) ] .
*
* Here In-1 is the identity matrix of size n-1. kron(X, Y) is the
* Kronecker product between the matrices X and Y.
*
* Note that if the default method for computing DIF(i) is wanted
* (see SLATDF), then the parameter DIFDRI (see below) should be
* changed from 3 to 4 (routine SLATDF(IJOB = 2 will be used)).
* See STGSYL for more details.
*
* b) If the i-th and (i+1)-th eigenvalues are complex conjugate pair,
*
* Suppose U and V are orthogonal transformations such that
*
* U'*(A, B)*V = (S, T) = ( S11 * ) ( T11 * ) 2
* ( 0 S22 ),( 0 T22) n-2
* 2 n-2 2 n-2
*
* and (S11, T11) corresponds to the complex conjugate eigenvalue
* pair (w, conjg(w)). There exist unitary matrices U1 and V1 such
* that
*
* U1'*S11*V1 = ( s11 s12 ) and U1'*T11*V1 = ( t11 t12 )
* ( 0 s22 ) ( 0 t22 )
*
* where the generalized eigenvalues w = s11/t11 and
* conjg(w) = s22/t22.
*
* Then the reciprocal condition number DIF(i) is bounded by
*
* min( d1, max( 1, |real(s11)/real(s22)| )*d2 )
*
* where, d1 = Difl((s11, t11), (s22, t22)) = sigma-min(Z1), where
* Z1 is the complex 2-by-2 matrix
*
* Z1 = [ s11 -s22 ]
* [ t11 -t22 ],
*
* This is done by computing (using real arithmetic) the
* roots of the characteristical polynomial det(Z1' * Z1 - lambda I),
* where Z1' denotes the conjugate transpose of Z1 and det(X) denotes
* the determinant of X.
*
* and d2 is an upper bound on Difl((S11, T11), (S22, T22)), i.e. an
* upper bound on sigma-min(Z2), where Z2 is (2n-2)-by-(2n-2)
*
* Z2 = [ kron(S11', In-2) -kron(I2, S22) ]
* [ kron(T11', In-2) -kron(I2, T22) ]
*
* Note that if the default method for computing DIF is wanted (see
* SLATDF), then the parameter DIFDRI (see below) should be changed
* from 3 to 4 (routine SLATDF(IJOB = 2 will be used)). See STGSYL
* for more details.
*
* For each eigenvalue/vector specified by SELECT, DIF stores a
* Frobenius norm-based estimate of Difl.
*
* An approximate error bound for the i-th computed eigenvector VL(i) or
* VR(i) is given by
*
* EPS * norm(A, B) / DIF(i).
*
* See ref. [2-3] for more details and further references.
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* References
* ==========
*
* [1] B. Kagstrom; A Direct Method for Reordering Eigenvalues in the
* Generalized Real Schur Form of a Regular Matrix Pair (A, B), in
* M.S. Moonen et al (eds), Linear Algebra for Large Scale and
* Real-Time Applications, Kluwer Academic Publ. 1993, pp 195-218.
*
* [2] B. Kagstrom and P. Poromaa; Computing Eigenspaces with Specified
* Eigenvalues of a Regular Matrix Pair (A, B) and Condition
* Estimation: Theory, Algorithms and Software,
* Report UMINF - 94.04, Department of Computing Science, Umea
* University, S-901 87 Umea, Sweden, 1994. Also as LAPACK Working
* Note 87. To appear in Numerical Algorithms, 1996.
*
* [3] B. Kagstrom and P. Poromaa, LAPACK-Style Algorithms and Software
* for Solving the Generalized Sylvester Equation and Estimating the
* Separation between Regular Matrix Pairs, Report UMINF - 93.23,
* Department of Computing Science, Umea University, S-901 87 Umea,
* Sweden, December 1993, Revised April 1994, Also as LAPACK Working
* Note 75. To appear in ACM Trans. on Math. Software, Vol 22,
* No 1, 1996.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param howmny
* @param select
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param vl
* @param ldvl
* @param vr
* @param ldvr
* @param s
* @param dif
* @param mm
* @param m
* @param work
* @param lwork
* @param iwork
* @param info
*
*/
abstract public void stgsna(java.lang.String job, java.lang.String howmny, boolean[] select, int n, float[] a, int lda, float[] b, int ldb, float[] vl, int ldvl, float[] vr, int ldvr, float[] s, float[] dif, int mm, org.netlib.util.intW m, float[] work, int lwork, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STGSNA estimates reciprocal condition numbers for specified
* eigenvalues and/or eigenvectors of a matrix pair (A, B) in
* generalized real Schur canonical form (or of any matrix pair
* (Q*A*Z', Q*B*Z') with orthogonal matrices Q and Z, where
* Z' denotes the transpose of Z.
*
* (A, B) must be in generalized real Schur form (as returned by SGGES),
* i.e. A is block upper triangular with 1-by-1 and 2-by-2 diagonal
* blocks. B is upper triangular.
*
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* Specifies whether condition numbers are required for
* eigenvalues (S) or eigenvectors (DIF):
* = 'E': for eigenvalues only (S);
* = 'V': for eigenvectors only (DIF);
* = 'B': for both eigenvalues and eigenvectors (S and DIF).
*
* HOWMNY (input) CHARACTER*1
* = 'A': compute condition numbers for all eigenpairs;
* = 'S': compute condition numbers for selected eigenpairs
* specified by the array SELECT.
*
* SELECT (input) LOGICAL array, dimension (N)
* If HOWMNY = 'S', SELECT specifies the eigenpairs for which
* condition numbers are required. To select condition numbers
* for the eigenpair corresponding to a real eigenvalue w(j),
* SELECT(j) must be set to .TRUE.. To select condition numbers
* corresponding to a complex conjugate pair of eigenvalues w(j)
* and w(j+1), either SELECT(j) or SELECT(j+1) or both, must be
* set to .TRUE..
* If HOWMNY = 'A', SELECT is not referenced.
*
* N (input) INTEGER
* The order of the square matrix pair (A, B). N >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The upper quasi-triangular matrix A in the pair (A,B).
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input) REAL array, dimension (LDB,N)
* The upper triangular matrix B in the pair (A,B).
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* VL (input) REAL array, dimension (LDVL,M)
* If JOB = 'E' or 'B', VL must contain left eigenvectors of
* (A, B), corresponding to the eigenpairs specified by HOWMNY
* and SELECT. The eigenvectors must be stored in consecutive
* columns of VL, as returned by STGEVC.
* If JOB = 'V', VL is not referenced.
*
* LDVL (input) INTEGER
* The leading dimension of the array VL. LDVL >= 1.
* If JOB = 'E' or 'B', LDVL >= N.
*
* VR (input) REAL array, dimension (LDVR,M)
* If JOB = 'E' or 'B', VR must contain right eigenvectors of
* (A, B), corresponding to the eigenpairs specified by HOWMNY
* and SELECT. The eigenvectors must be stored in consecutive
* columns ov VR, as returned by STGEVC.
* If JOB = 'V', VR is not referenced.
*
* LDVR (input) INTEGER
* The leading dimension of the array VR. LDVR >= 1.
* If JOB = 'E' or 'B', LDVR >= N.
*
* S (output) REAL array, dimension (MM)
* If JOB = 'E' or 'B', the reciprocal condition numbers of the
* selected eigenvalues, stored in consecutive elements of the
* array. For a complex conjugate pair of eigenvalues two
* consecutive elements of S are set to the same value. Thus
* S(j), DIF(j), and the j-th columns of VL and VR all
* correspond to the same eigenpair (but not in general the
* j-th eigenpair, unless all eigenpairs are selected).
* If JOB = 'V', S is not referenced.
*
* DIF (output) REAL array, dimension (MM)
* If JOB = 'V' or 'B', the estimated reciprocal condition
* numbers of the selected eigenvectors, stored in consecutive
* elements of the array. For a complex eigenvector two
* consecutive elements of DIF are set to the same value. If
* the eigenvalues cannot be reordered to compute DIF(j), DIF(j)
* is set to 0; this can only occur when the true value would be
* very small anyway.
* If JOB = 'E', DIF is not referenced.
*
* MM (input) INTEGER
* The number of elements in the arrays S and DIF. MM >= M.
*
* M (output) INTEGER
* The number of elements of the arrays S and DIF used to store
* the specified condition numbers; for each selected real
* eigenvalue one element is used, and for each selected complex
* conjugate pair of eigenvalues, two elements are used.
* If HOWMNY = 'A', M is set to N.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,N).
* If JOB = 'V' or 'B' LWORK >= 2*N*(N+2)+16.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (N + 6)
* If JOB = 'E', IWORK is not referenced.
*
* INFO (output) INTEGER
* =0: Successful exit
* <0: If INFO = -i, the i-th argument had an illegal value
*
*
* Further Details
* ===============
*
* The reciprocal of the condition number of a generalized eigenvalue
* w = (a, b) is defined as
*
* S(w) = (|u'Av|**2 + |u'Bv|**2)**(1/2) / (norm(u)*norm(v))
*
* where u and v are the left and right eigenvectors of (A, B)
* corresponding to w; |z| denotes the absolute value of the complex
* number, and norm(u) denotes the 2-norm of the vector u.
* The pair (a, b) corresponds to an eigenvalue w = a/b (= u'Av/u'Bv)
* of the matrix pair (A, B). If both a and b equal zero, then (A B) is
* singular and S(I) = -1 is returned.
*
* An approximate error bound on the chordal distance between the i-th
* computed generalized eigenvalue w and the corresponding exact
* eigenvalue lambda is
*
* chord(w, lambda) <= EPS * norm(A, B) / S(I)
*
* where EPS is the machine precision.
*
* The reciprocal of the condition number DIF(i) of right eigenvector u
* and left eigenvector v corresponding to the generalized eigenvalue w
* is defined as follows:
*
* a) If the i-th eigenvalue w = (a,b) is real
*
* Suppose U and V are orthogonal transformations such that
*
* U'*(A, B)*V = (S, T) = ( a * ) ( b * ) 1
* ( 0 S22 ),( 0 T22 ) n-1
* 1 n-1 1 n-1
*
* Then the reciprocal condition number DIF(i) is
*
* Difl((a, b), (S22, T22)) = sigma-min( Zl ),
*
* where sigma-min(Zl) denotes the smallest singular value of the
* 2(n-1)-by-2(n-1) matrix
*
* Zl = [ kron(a, In-1) -kron(1, S22) ]
* [ kron(b, In-1) -kron(1, T22) ] .
*
* Here In-1 is the identity matrix of size n-1. kron(X, Y) is the
* Kronecker product between the matrices X and Y.
*
* Note that if the default method for computing DIF(i) is wanted
* (see SLATDF), then the parameter DIFDRI (see below) should be
* changed from 3 to 4 (routine SLATDF(IJOB = 2 will be used)).
* See STGSYL for more details.
*
* b) If the i-th and (i+1)-th eigenvalues are complex conjugate pair,
*
* Suppose U and V are orthogonal transformations such that
*
* U'*(A, B)*V = (S, T) = ( S11 * ) ( T11 * ) 2
* ( 0 S22 ),( 0 T22) n-2
* 2 n-2 2 n-2
*
* and (S11, T11) corresponds to the complex conjugate eigenvalue
* pair (w, conjg(w)). There exist unitary matrices U1 and V1 such
* that
*
* U1'*S11*V1 = ( s11 s12 ) and U1'*T11*V1 = ( t11 t12 )
* ( 0 s22 ) ( 0 t22 )
*
* where the generalized eigenvalues w = s11/t11 and
* conjg(w) = s22/t22.
*
* Then the reciprocal condition number DIF(i) is bounded by
*
* min( d1, max( 1, |real(s11)/real(s22)| )*d2 )
*
* where, d1 = Difl((s11, t11), (s22, t22)) = sigma-min(Z1), where
* Z1 is the complex 2-by-2 matrix
*
* Z1 = [ s11 -s22 ]
* [ t11 -t22 ],
*
* This is done by computing (using real arithmetic) the
* roots of the characteristical polynomial det(Z1' * Z1 - lambda I),
* where Z1' denotes the conjugate transpose of Z1 and det(X) denotes
* the determinant of X.
*
* and d2 is an upper bound on Difl((S11, T11), (S22, T22)), i.e. an
* upper bound on sigma-min(Z2), where Z2 is (2n-2)-by-(2n-2)
*
* Z2 = [ kron(S11', In-2) -kron(I2, S22) ]
* [ kron(T11', In-2) -kron(I2, T22) ]
*
* Note that if the default method for computing DIF is wanted (see
* SLATDF), then the parameter DIFDRI (see below) should be changed
* from 3 to 4 (routine SLATDF(IJOB = 2 will be used)). See STGSYL
* for more details.
*
* For each eigenvalue/vector specified by SELECT, DIF stores a
* Frobenius norm-based estimate of Difl.
*
* An approximate error bound for the i-th computed eigenvector VL(i) or
* VR(i) is given by
*
* EPS * norm(A, B) / DIF(i).
*
* See ref. [2-3] for more details and further references.
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* References
* ==========
*
* [1] B. Kagstrom; A Direct Method for Reordering Eigenvalues in the
* Generalized Real Schur Form of a Regular Matrix Pair (A, B), in
* M.S. Moonen et al (eds), Linear Algebra for Large Scale and
* Real-Time Applications, Kluwer Academic Publ. 1993, pp 195-218.
*
* [2] B. Kagstrom and P. Poromaa; Computing Eigenspaces with Specified
* Eigenvalues of a Regular Matrix Pair (A, B) and Condition
* Estimation: Theory, Algorithms and Software,
* Report UMINF - 94.04, Department of Computing Science, Umea
* University, S-901 87 Umea, Sweden, 1994. Also as LAPACK Working
* Note 87. To appear in Numerical Algorithms, 1996.
*
* [3] B. Kagstrom and P. Poromaa, LAPACK-Style Algorithms and Software
* for Solving the Generalized Sylvester Equation and Estimating the
* Separation between Regular Matrix Pairs, Report UMINF - 93.23,
* Department of Computing Science, Umea University, S-901 87 Umea,
* Sweden, December 1993, Revised April 1994, Also as LAPACK Working
* Note 75. To appear in ACM Trans. on Math. Software, Vol 22,
* No 1, 1996.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param howmny
* @param select
* @param _select_offset
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param vl
* @param _vl_offset
* @param ldvl
* @param vr
* @param _vr_offset
* @param ldvr
* @param s
* @param _s_offset
* @param dif
* @param _dif_offset
* @param mm
* @param m
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void stgsna(java.lang.String job, java.lang.String howmny, boolean[] select, int _select_offset, int n, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, float[] vl, int _vl_offset, int ldvl, float[] vr, int _vr_offset, int ldvr, float[] s, int _s_offset, float[] dif, int _dif_offset, int mm, org.netlib.util.intW m, float[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STGSY2 solves the generalized Sylvester equation:
*
* A * R - L * B = scale * C (1)
* D * R - L * E = scale * F,
*
* using Level 1 and 2 BLAS. where R and L are unknown M-by-N matrices,
* (A, D), (B, E) and (C, F) are given matrix pairs of size M-by-M,
* N-by-N and M-by-N, respectively, with real entries. (A, D) and (B, E)
* must be in generalized Schur canonical form, i.e. A, B are upper
* quasi triangular and D, E are upper triangular. The solution (R, L)
* overwrites (C, F). 0 <= SCALE <= 1 is an output scaling factor
* chosen to avoid overflow.
*
* In matrix notation solving equation (1) corresponds to solve
* Z*x = scale*b, where Z is defined as
*
* Z = [ kron(In, A) -kron(B', Im) ] (2)
* [ kron(In, D) -kron(E', Im) ],
*
* Ik is the identity matrix of size k and X' is the transpose of X.
* kron(X, Y) is the Kronecker product between the matrices X and Y.
* In the process of solving (1), we solve a number of such systems
* where Dim(In), Dim(In) = 1 or 2.
*
* If TRANS = 'T', solve the transposed system Z'*y = scale*b for y,
* which is equivalent to solve for R and L in
*
* A' * R + D' * L = scale * C (3)
* R * B' + L * E' = scale * -F
*
* This case is used to compute an estimate of Dif[(A, D), (B, E)] =
* sigma_min(Z) using reverse communicaton with SLACON.
*
* STGSY2 also (IJOB >= 1) contributes to the computation in STGSYL
* of an upper bound on the separation between to matrix pairs. Then
* the input (A, D), (B, E) are sub-pencils of the matrix pair in
* STGSYL. See STGSYL for details.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* = 'N', solve the generalized Sylvester equation (1).
* = 'T': solve the 'transposed' system (3).
*
* IJOB (input) INTEGER
* Specifies what kind of functionality to be performed.
* = 0: solve (1) only.
* = 1: A contribution from this subsystem to a Frobenius
* norm-based estimate of the separation between two matrix
* pairs is computed. (look ahead strategy is used).
* = 2: A contribution from this subsystem to a Frobenius
* norm-based estimate of the separation between two matrix
* pairs is computed. (SGECON on sub-systems is used.)
* Not referenced if TRANS = 'T'.
*
* M (input) INTEGER
* On entry, M specifies the order of A and D, and the row
* dimension of C, F, R and L.
*
* N (input) INTEGER
* On entry, N specifies the order of B and E, and the column
* dimension of C, F, R and L.
*
* A (input) REAL array, dimension (LDA, M)
* On entry, A contains an upper quasi triangular matrix.
*
* LDA (input) INTEGER
* The leading dimension of the matrix A. LDA >= max(1, M).
*
* B (input) REAL array, dimension (LDB, N)
* On entry, B contains an upper quasi triangular matrix.
*
* LDB (input) INTEGER
* The leading dimension of the matrix B. LDB >= max(1, N).
*
* C (input/output) REAL array, dimension (LDC, N)
* On entry, C contains the right-hand-side of the first matrix
* equation in (1).
* On exit, if IJOB = 0, C has been overwritten by the
* solution R.
*
* LDC (input) INTEGER
* The leading dimension of the matrix C. LDC >= max(1, M).
*
* D (input) REAL array, dimension (LDD, M)
* On entry, D contains an upper triangular matrix.
*
* LDD (input) INTEGER
* The leading dimension of the matrix D. LDD >= max(1, M).
*
* E (input) REAL array, dimension (LDE, N)
* On entry, E contains an upper triangular matrix.
*
* LDE (input) INTEGER
* The leading dimension of the matrix E. LDE >= max(1, N).
*
* F (input/output) REAL array, dimension (LDF, N)
* On entry, F contains the right-hand-side of the second matrix
* equation in (1).
* On exit, if IJOB = 0, F has been overwritten by the
* solution L.
*
* LDF (input) INTEGER
* The leading dimension of the matrix F. LDF >= max(1, M).
*
* SCALE (output) REAL
* On exit, 0 <= SCALE <= 1. If 0 < SCALE < 1, the solutions
* R and L (C and F on entry) will hold the solutions to a
* slightly perturbed system but the input matrices A, B, D and
* E have not been changed. If SCALE = 0, R and L will hold the
* solutions to the homogeneous system with C = F = 0. Normally,
* SCALE = 1.
*
* RDSUM (input/output) REAL
* On entry, the sum of squares of computed contributions to
* the Dif-estimate under computation by STGSYL, where the
* scaling factor RDSCAL (see below) has been factored out.
* On exit, the corresponding sum of squares updated with the
* contributions from the current sub-system.
* If TRANS = 'T' RDSUM is not touched.
* NOTE: RDSUM only makes sense when STGSY2 is called by STGSYL.
*
* RDSCAL (input/output) REAL
* On entry, scaling factor used to prevent overflow in RDSUM.
* On exit, RDSCAL is updated w.r.t. the current contributions
* in RDSUM.
* If TRANS = 'T', RDSCAL is not touched.
* NOTE: RDSCAL only makes sense when STGSY2 is called by
* STGSYL.
*
* IWORK (workspace) INTEGER array, dimension (M+N+2)
*
* PQ (output) INTEGER
* On exit, the number of subsystems (of size 2-by-2, 4-by-4 and
* 8-by-8) solved by this routine.
*
* INFO (output) INTEGER
* On exit, if INFO is set to
* =0: Successful exit
* <0: If INFO = -i, the i-th argument had an illegal value.
* >0: The matrix pairs (A, D) and (B, E) have common or very
* close eigenvalues.
*
* Further Details
* ===============
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* =====================================================================
* Replaced various illegal calls to SCOPY by calls to SLASET.
* Sven Hammarling, 27/5/02.
*
* .. Parameters ..
*
*
* @param trans
* @param ijob
* @param m
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param c
* @param Ldc
* @param d
* @param ldd
* @param e
* @param lde
* @param f
* @param ldf
* @param scale
* @param rdsum
* @param rdscal
* @param iwork
* @param pq
* @param info
*
*/
abstract public void stgsy2(java.lang.String trans, int ijob, int m, int n, float[] a, int lda, float[] b, int ldb, float[] c, int Ldc, float[] d, int ldd, float[] e, int lde, float[] f, int ldf, org.netlib.util.floatW scale, org.netlib.util.floatW rdsum, org.netlib.util.floatW rdscal, int[] iwork, org.netlib.util.intW pq, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STGSY2 solves the generalized Sylvester equation:
*
* A * R - L * B = scale * C (1)
* D * R - L * E = scale * F,
*
* using Level 1 and 2 BLAS. where R and L are unknown M-by-N matrices,
* (A, D), (B, E) and (C, F) are given matrix pairs of size M-by-M,
* N-by-N and M-by-N, respectively, with real entries. (A, D) and (B, E)
* must be in generalized Schur canonical form, i.e. A, B are upper
* quasi triangular and D, E are upper triangular. The solution (R, L)
* overwrites (C, F). 0 <= SCALE <= 1 is an output scaling factor
* chosen to avoid overflow.
*
* In matrix notation solving equation (1) corresponds to solve
* Z*x = scale*b, where Z is defined as
*
* Z = [ kron(In, A) -kron(B', Im) ] (2)
* [ kron(In, D) -kron(E', Im) ],
*
* Ik is the identity matrix of size k and X' is the transpose of X.
* kron(X, Y) is the Kronecker product between the matrices X and Y.
* In the process of solving (1), we solve a number of such systems
* where Dim(In), Dim(In) = 1 or 2.
*
* If TRANS = 'T', solve the transposed system Z'*y = scale*b for y,
* which is equivalent to solve for R and L in
*
* A' * R + D' * L = scale * C (3)
* R * B' + L * E' = scale * -F
*
* This case is used to compute an estimate of Dif[(A, D), (B, E)] =
* sigma_min(Z) using reverse communicaton with SLACON.
*
* STGSY2 also (IJOB >= 1) contributes to the computation in STGSYL
* of an upper bound on the separation between to matrix pairs. Then
* the input (A, D), (B, E) are sub-pencils of the matrix pair in
* STGSYL. See STGSYL for details.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* = 'N', solve the generalized Sylvester equation (1).
* = 'T': solve the 'transposed' system (3).
*
* IJOB (input) INTEGER
* Specifies what kind of functionality to be performed.
* = 0: solve (1) only.
* = 1: A contribution from this subsystem to a Frobenius
* norm-based estimate of the separation between two matrix
* pairs is computed. (look ahead strategy is used).
* = 2: A contribution from this subsystem to a Frobenius
* norm-based estimate of the separation between two matrix
* pairs is computed. (SGECON on sub-systems is used.)
* Not referenced if TRANS = 'T'.
*
* M (input) INTEGER
* On entry, M specifies the order of A and D, and the row
* dimension of C, F, R and L.
*
* N (input) INTEGER
* On entry, N specifies the order of B and E, and the column
* dimension of C, F, R and L.
*
* A (input) REAL array, dimension (LDA, M)
* On entry, A contains an upper quasi triangular matrix.
*
* LDA (input) INTEGER
* The leading dimension of the matrix A. LDA >= max(1, M).
*
* B (input) REAL array, dimension (LDB, N)
* On entry, B contains an upper quasi triangular matrix.
*
* LDB (input) INTEGER
* The leading dimension of the matrix B. LDB >= max(1, N).
*
* C (input/output) REAL array, dimension (LDC, N)
* On entry, C contains the right-hand-side of the first matrix
* equation in (1).
* On exit, if IJOB = 0, C has been overwritten by the
* solution R.
*
* LDC (input) INTEGER
* The leading dimension of the matrix C. LDC >= max(1, M).
*
* D (input) REAL array, dimension (LDD, M)
* On entry, D contains an upper triangular matrix.
*
* LDD (input) INTEGER
* The leading dimension of the matrix D. LDD >= max(1, M).
*
* E (input) REAL array, dimension (LDE, N)
* On entry, E contains an upper triangular matrix.
*
* LDE (input) INTEGER
* The leading dimension of the matrix E. LDE >= max(1, N).
*
* F (input/output) REAL array, dimension (LDF, N)
* On entry, F contains the right-hand-side of the second matrix
* equation in (1).
* On exit, if IJOB = 0, F has been overwritten by the
* solution L.
*
* LDF (input) INTEGER
* The leading dimension of the matrix F. LDF >= max(1, M).
*
* SCALE (output) REAL
* On exit, 0 <= SCALE <= 1. If 0 < SCALE < 1, the solutions
* R and L (C and F on entry) will hold the solutions to a
* slightly perturbed system but the input matrices A, B, D and
* E have not been changed. If SCALE = 0, R and L will hold the
* solutions to the homogeneous system with C = F = 0. Normally,
* SCALE = 1.
*
* RDSUM (input/output) REAL
* On entry, the sum of squares of computed contributions to
* the Dif-estimate under computation by STGSYL, where the
* scaling factor RDSCAL (see below) has been factored out.
* On exit, the corresponding sum of squares updated with the
* contributions from the current sub-system.
* If TRANS = 'T' RDSUM is not touched.
* NOTE: RDSUM only makes sense when STGSY2 is called by STGSYL.
*
* RDSCAL (input/output) REAL
* On entry, scaling factor used to prevent overflow in RDSUM.
* On exit, RDSCAL is updated w.r.t. the current contributions
* in RDSUM.
* If TRANS = 'T', RDSCAL is not touched.
* NOTE: RDSCAL only makes sense when STGSY2 is called by
* STGSYL.
*
* IWORK (workspace) INTEGER array, dimension (M+N+2)
*
* PQ (output) INTEGER
* On exit, the number of subsystems (of size 2-by-2, 4-by-4 and
* 8-by-8) solved by this routine.
*
* INFO (output) INTEGER
* On exit, if INFO is set to
* =0: Successful exit
* <0: If INFO = -i, the i-th argument had an illegal value.
* >0: The matrix pairs (A, D) and (B, E) have common or very
* close eigenvalues.
*
* Further Details
* ===============
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* =====================================================================
* Replaced various illegal calls to SCOPY by calls to SLASET.
* Sven Hammarling, 27/5/02.
*
* .. Parameters ..
*
*
* @param trans
* @param ijob
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param c
* @param _c_offset
* @param Ldc
* @param d
* @param _d_offset
* @param ldd
* @param e
* @param _e_offset
* @param lde
* @param f
* @param _f_offset
* @param ldf
* @param scale
* @param rdsum
* @param rdscal
* @param iwork
* @param _iwork_offset
* @param pq
* @param info
*
*/
abstract public void stgsy2(java.lang.String trans, int ijob, int m, int n, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, float[] c, int _c_offset, int Ldc, float[] d, int _d_offset, int ldd, float[] e, int _e_offset, int lde, float[] f, int _f_offset, int ldf, org.netlib.util.floatW scale, org.netlib.util.floatW rdsum, org.netlib.util.floatW rdscal, int[] iwork, int _iwork_offset, org.netlib.util.intW pq, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STGSYL solves the generalized Sylvester equation:
*
* A * R - L * B = scale * C (1)
* D * R - L * E = scale * F
*
* where R and L are unknown m-by-n matrices, (A, D), (B, E) and
* (C, F) are given matrix pairs of size m-by-m, n-by-n and m-by-n,
* respectively, with real entries. (A, D) and (B, E) must be in
* generalized (real) Schur canonical form, i.e. A, B are upper quasi
* triangular and D, E are upper triangular.
*
* The solution (R, L) overwrites (C, F). 0 <= SCALE <= 1 is an output
* scaling factor chosen to avoid overflow.
*
* In matrix notation (1) is equivalent to solve Zx = scale b, where
* Z is defined as
*
* Z = [ kron(In, A) -kron(B', Im) ] (2)
* [ kron(In, D) -kron(E', Im) ].
*
* Here Ik is the identity matrix of size k and X' is the transpose of
* X. kron(X, Y) is the Kronecker product between the matrices X and Y.
*
* If TRANS = 'T', STGSYL solves the transposed system Z'*y = scale*b,
* which is equivalent to solve for R and L in
*
* A' * R + D' * L = scale * C (3)
* R * B' + L * E' = scale * (-F)
*
* This case (TRANS = 'T') is used to compute an one-norm-based estimate
* of Dif[(A,D), (B,E)], the separation between the matrix pairs (A,D)
* and (B,E), using SLACON.
*
* If IJOB >= 1, STGSYL computes a Frobenius norm-based estimate
* of Dif[(A,D),(B,E)]. That is, the reciprocal of a lower bound on the
* reciprocal of the smallest singular value of Z. See [1-2] for more
* information.
*
* This is a level 3 BLAS algorithm.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* = 'N', solve the generalized Sylvester equation (1).
* = 'T', solve the 'transposed' system (3).
*
* IJOB (input) INTEGER
* Specifies what kind of functionality to be performed.
* =0: solve (1) only.
* =1: The functionality of 0 and 3.
* =2: The functionality of 0 and 4.
* =3: Only an estimate of Dif[(A,D), (B,E)] is computed.
* (look ahead strategy IJOB = 1 is used).
* =4: Only an estimate of Dif[(A,D), (B,E)] is computed.
* ( SGECON on sub-systems is used ).
* Not referenced if TRANS = 'T'.
*
* M (input) INTEGER
* The order of the matrices A and D, and the row dimension of
* the matrices C, F, R and L.
*
* N (input) INTEGER
* The order of the matrices B and E, and the column dimension
* of the matrices C, F, R and L.
*
* A (input) REAL array, dimension (LDA, M)
* The upper quasi triangular matrix A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1, M).
*
* B (input) REAL array, dimension (LDB, N)
* The upper quasi triangular matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1, N).
*
* C (input/output) REAL array, dimension (LDC, N)
* On entry, C contains the right-hand-side of the first matrix
* equation in (1) or (3).
* On exit, if IJOB = 0, 1 or 2, C has been overwritten by
* the solution R. If IJOB = 3 or 4 and TRANS = 'N', C holds R,
* the solution achieved during the computation of the
* Dif-estimate.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1, M).
*
* D (input) REAL array, dimension (LDD, M)
* The upper triangular matrix D.
*
* LDD (input) INTEGER
* The leading dimension of the array D. LDD >= max(1, M).
*
* E (input) REAL array, dimension (LDE, N)
* The upper triangular matrix E.
*
* LDE (input) INTEGER
* The leading dimension of the array E. LDE >= max(1, N).
*
* F (input/output) REAL array, dimension (LDF, N)
* On entry, F contains the right-hand-side of the second matrix
* equation in (1) or (3).
* On exit, if IJOB = 0, 1 or 2, F has been overwritten by
* the solution L. If IJOB = 3 or 4 and TRANS = 'N', F holds L,
* the solution achieved during the computation of the
* Dif-estimate.
*
* LDF (input) INTEGER
* The leading dimension of the array F. LDF >= max(1, M).
*
* DIF (output) REAL
* On exit DIF is the reciprocal of a lower bound of the
* reciprocal of the Dif-function, i.e. DIF is an upper bound of
* Dif[(A,D), (B,E)] = sigma_min(Z), where Z as in (2).
* IF IJOB = 0 or TRANS = 'T', DIF is not touched.
*
* SCALE (output) REAL
* On exit SCALE is the scaling factor in (1) or (3).
* If 0 < SCALE < 1, C and F hold the solutions R and L, resp.,
* to a slightly perturbed system but the input matrices A, B, D
* and E have not been changed. If SCALE = 0, C and F hold the
* solutions R and L, respectively, to the homogeneous system
* with C = F = 0. Normally, SCALE = 1.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK > = 1.
* If IJOB = 1 or 2 and TRANS = 'N', LWORK >= max(1,2*M*N).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (M+N+6)
*
* INFO (output) INTEGER
* =0: successful exit
* <0: If INFO = -i, the i-th argument had an illegal value.
* >0: (A, D) and (B, E) have common or close eigenvalues.
*
* Further Details
* ===============
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* [1] B. Kagstrom and P. Poromaa, LAPACK-Style Algorithms and Software
* for Solving the Generalized Sylvester Equation and Estimating the
* Separation between Regular Matrix Pairs, Report UMINF - 93.23,
* Department of Computing Science, Umea University, S-901 87 Umea,
* Sweden, December 1993, Revised April 1994, Also as LAPACK Working
* Note 75. To appear in ACM Trans. on Math. Software, Vol 22,
* No 1, 1996.
*
* [2] B. Kagstrom, A Perturbation Analysis of the Generalized Sylvester
* Equation (AR - LB, DR - LE ) = (C, F), SIAM J. Matrix Anal.
* Appl., 15(4):1045-1060, 1994
*
* [3] B. Kagstrom and L. Westin, Generalized Schur Methods with
* Condition Estimators for Solving the Generalized Sylvester
* Equation, IEEE Transactions on Automatic Control, Vol. 34, No. 7,
* July 1989, pp 745-751.
*
* =====================================================================
* Replaced various illegal calls to SCOPY by calls to SLASET.
* Sven Hammarling, 1/5/02.
*
* .. Parameters ..
*
*
* @param trans
* @param ijob
* @param m
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param c
* @param Ldc
* @param d
* @param ldd
* @param e
* @param lde
* @param f
* @param ldf
* @param scale
* @param dif
* @param work
* @param lwork
* @param iwork
* @param info
*
*/
abstract public void stgsyl(java.lang.String trans, int ijob, int m, int n, float[] a, int lda, float[] b, int ldb, float[] c, int Ldc, float[] d, int ldd, float[] e, int lde, float[] f, int ldf, org.netlib.util.floatW scale, org.netlib.util.floatW dif, float[] work, int lwork, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STGSYL solves the generalized Sylvester equation:
*
* A * R - L * B = scale * C (1)
* D * R - L * E = scale * F
*
* where R and L are unknown m-by-n matrices, (A, D), (B, E) and
* (C, F) are given matrix pairs of size m-by-m, n-by-n and m-by-n,
* respectively, with real entries. (A, D) and (B, E) must be in
* generalized (real) Schur canonical form, i.e. A, B are upper quasi
* triangular and D, E are upper triangular.
*
* The solution (R, L) overwrites (C, F). 0 <= SCALE <= 1 is an output
* scaling factor chosen to avoid overflow.
*
* In matrix notation (1) is equivalent to solve Zx = scale b, where
* Z is defined as
*
* Z = [ kron(In, A) -kron(B', Im) ] (2)
* [ kron(In, D) -kron(E', Im) ].
*
* Here Ik is the identity matrix of size k and X' is the transpose of
* X. kron(X, Y) is the Kronecker product between the matrices X and Y.
*
* If TRANS = 'T', STGSYL solves the transposed system Z'*y = scale*b,
* which is equivalent to solve for R and L in
*
* A' * R + D' * L = scale * C (3)
* R * B' + L * E' = scale * (-F)
*
* This case (TRANS = 'T') is used to compute an one-norm-based estimate
* of Dif[(A,D), (B,E)], the separation between the matrix pairs (A,D)
* and (B,E), using SLACON.
*
* If IJOB >= 1, STGSYL computes a Frobenius norm-based estimate
* of Dif[(A,D),(B,E)]. That is, the reciprocal of a lower bound on the
* reciprocal of the smallest singular value of Z. See [1-2] for more
* information.
*
* This is a level 3 BLAS algorithm.
*
* Arguments
* =========
*
* TRANS (input) CHARACTER*1
* = 'N', solve the generalized Sylvester equation (1).
* = 'T', solve the 'transposed' system (3).
*
* IJOB (input) INTEGER
* Specifies what kind of functionality to be performed.
* =0: solve (1) only.
* =1: The functionality of 0 and 3.
* =2: The functionality of 0 and 4.
* =3: Only an estimate of Dif[(A,D), (B,E)] is computed.
* (look ahead strategy IJOB = 1 is used).
* =4: Only an estimate of Dif[(A,D), (B,E)] is computed.
* ( SGECON on sub-systems is used ).
* Not referenced if TRANS = 'T'.
*
* M (input) INTEGER
* The order of the matrices A and D, and the row dimension of
* the matrices C, F, R and L.
*
* N (input) INTEGER
* The order of the matrices B and E, and the column dimension
* of the matrices C, F, R and L.
*
* A (input) REAL array, dimension (LDA, M)
* The upper quasi triangular matrix A.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1, M).
*
* B (input) REAL array, dimension (LDB, N)
* The upper quasi triangular matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1, N).
*
* C (input/output) REAL array, dimension (LDC, N)
* On entry, C contains the right-hand-side of the first matrix
* equation in (1) or (3).
* On exit, if IJOB = 0, 1 or 2, C has been overwritten by
* the solution R. If IJOB = 3 or 4 and TRANS = 'N', C holds R,
* the solution achieved during the computation of the
* Dif-estimate.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1, M).
*
* D (input) REAL array, dimension (LDD, M)
* The upper triangular matrix D.
*
* LDD (input) INTEGER
* The leading dimension of the array D. LDD >= max(1, M).
*
* E (input) REAL array, dimension (LDE, N)
* The upper triangular matrix E.
*
* LDE (input) INTEGER
* The leading dimension of the array E. LDE >= max(1, N).
*
* F (input/output) REAL array, dimension (LDF, N)
* On entry, F contains the right-hand-side of the second matrix
* equation in (1) or (3).
* On exit, if IJOB = 0, 1 or 2, F has been overwritten by
* the solution L. If IJOB = 3 or 4 and TRANS = 'N', F holds L,
* the solution achieved during the computation of the
* Dif-estimate.
*
* LDF (input) INTEGER
* The leading dimension of the array F. LDF >= max(1, M).
*
* DIF (output) REAL
* On exit DIF is the reciprocal of a lower bound of the
* reciprocal of the Dif-function, i.e. DIF is an upper bound of
* Dif[(A,D), (B,E)] = sigma_min(Z), where Z as in (2).
* IF IJOB = 0 or TRANS = 'T', DIF is not touched.
*
* SCALE (output) REAL
* On exit SCALE is the scaling factor in (1) or (3).
* If 0 < SCALE < 1, C and F hold the solutions R and L, resp.,
* to a slightly perturbed system but the input matrices A, B, D
* and E have not been changed. If SCALE = 0, C and F hold the
* solutions R and L, respectively, to the homogeneous system
* with C = F = 0. Normally, SCALE = 1.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK > = 1.
* If IJOB = 1 or 2 and TRANS = 'N', LWORK >= max(1,2*M*N).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (M+N+6)
*
* INFO (output) INTEGER
* =0: successful exit
* <0: If INFO = -i, the i-th argument had an illegal value.
* >0: (A, D) and (B, E) have common or close eigenvalues.
*
* Further Details
* ===============
*
* Based on contributions by
* Bo Kagstrom and Peter Poromaa, Department of Computing Science,
* Umea University, S-901 87 Umea, Sweden.
*
* [1] B. Kagstrom and P. Poromaa, LAPACK-Style Algorithms and Software
* for Solving the Generalized Sylvester Equation and Estimating the
* Separation between Regular Matrix Pairs, Report UMINF - 93.23,
* Department of Computing Science, Umea University, S-901 87 Umea,
* Sweden, December 1993, Revised April 1994, Also as LAPACK Working
* Note 75. To appear in ACM Trans. on Math. Software, Vol 22,
* No 1, 1996.
*
* [2] B. Kagstrom, A Perturbation Analysis of the Generalized Sylvester
* Equation (AR - LB, DR - LE ) = (C, F), SIAM J. Matrix Anal.
* Appl., 15(4):1045-1060, 1994
*
* [3] B. Kagstrom and L. Westin, Generalized Schur Methods with
* Condition Estimators for Solving the Generalized Sylvester
* Equation, IEEE Transactions on Automatic Control, Vol. 34, No. 7,
* July 1989, pp 745-751.
*
* =====================================================================
* Replaced various illegal calls to SCOPY by calls to SLASET.
* Sven Hammarling, 1/5/02.
*
* .. Parameters ..
*
*
* @param trans
* @param ijob
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param c
* @param _c_offset
* @param Ldc
* @param d
* @param _d_offset
* @param ldd
* @param e
* @param _e_offset
* @param lde
* @param f
* @param _f_offset
* @param ldf
* @param scale
* @param dif
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void stgsyl(java.lang.String trans, int ijob, int m, int n, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, float[] c, int _c_offset, int Ldc, float[] d, int _d_offset, int ldd, float[] e, int _e_offset, int lde, float[] f, int _f_offset, int ldf, org.netlib.util.floatW scale, org.netlib.util.floatW dif, float[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STPCON estimates the reciprocal of the condition number of a packed
* triangular matrix A, in either the 1-norm or the infinity-norm.
*
* The norm of A is computed and an estimate is obtained for
* norm(inv(A)), then the reciprocal of the condition number is
* computed as
* RCOND = 1 / ( norm(A) * norm(inv(A)) ).
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies whether the 1-norm condition number or the
* infinity-norm condition number is required:
* = '1' or 'O': 1-norm;
* = 'I': Infinity-norm.
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input) REAL array, dimension (N*(N+1)/2)
* The upper or lower triangular matrix A, packed columnwise in
* a linear array. The j-th column of A is stored in the array
* AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
* If DIAG = 'U', the diagonal elements of A are not referenced
* and are assumed to be 1.
*
* RCOND (output) REAL
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(norm(A) * norm(inv(A))).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param diag
* @param n
* @param ap
* @param rcond
* @param work
* @param iwork
* @param info
*
*/
abstract public void stpcon(java.lang.String norm, java.lang.String uplo, java.lang.String diag, int n, float[] ap, org.netlib.util.floatW rcond, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STPCON estimates the reciprocal of the condition number of a packed
* triangular matrix A, in either the 1-norm or the infinity-norm.
*
* The norm of A is computed and an estimate is obtained for
* norm(inv(A)), then the reciprocal of the condition number is
* computed as
* RCOND = 1 / ( norm(A) * norm(inv(A)) ).
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies whether the 1-norm condition number or the
* infinity-norm condition number is required:
* = '1' or 'O': 1-norm;
* = 'I': Infinity-norm.
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input) REAL array, dimension (N*(N+1)/2)
* The upper or lower triangular matrix A, packed columnwise in
* a linear array. The j-th column of A is stored in the array
* AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2n-j)/2) = A(i,j) for j<=i<=n.
* If DIAG = 'U', the diagonal elements of A are not referenced
* and are assumed to be 1.
*
* RCOND (output) REAL
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(norm(A) * norm(inv(A))).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param diag
* @param n
* @param ap
* @param _ap_offset
* @param rcond
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void stpcon(java.lang.String norm, java.lang.String uplo, java.lang.String diag, int n, float[] ap, int _ap_offset, org.netlib.util.floatW rcond, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STPRFS provides error bounds and backward error estimates for the
* solution to a system of linear equations with a triangular packed
* coefficient matrix.
*
* The solution matrix X must be computed by STPTRS or some other
* means before entering this routine. STPRFS does not do iterative
* refinement because doing so cannot improve the backward error.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AP (input) REAL array, dimension (N*(N+1)/2)
* The upper or lower triangular matrix A, packed columnwise in
* a linear array. The j-th column of A is stored in the array
* AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
* If DIAG = 'U', the diagonal elements of A are not referenced
* and are assumed to be 1.
*
* B (input) REAL array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input) REAL array, dimension (LDX,NRHS)
* The solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param n
* @param nrhs
* @param ap
* @param b
* @param ldb
* @param x
* @param ldx
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void stprfs(java.lang.String uplo, java.lang.String trans, java.lang.String diag, int n, int nrhs, float[] ap, float[] b, int ldb, float[] x, int ldx, float[] ferr, float[] berr, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STPRFS provides error bounds and backward error estimates for the
* solution to a system of linear equations with a triangular packed
* coefficient matrix.
*
* The solution matrix X must be computed by STPTRS or some other
* means before entering this routine. STPRFS does not do iterative
* refinement because doing so cannot improve the backward error.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* AP (input) REAL array, dimension (N*(N+1)/2)
* The upper or lower triangular matrix A, packed columnwise in
* a linear array. The j-th column of A is stored in the array
* AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
* If DIAG = 'U', the diagonal elements of A are not referenced
* and are assumed to be 1.
*
* B (input) REAL array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input) REAL array, dimension (LDX,NRHS)
* The solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param n
* @param nrhs
* @param ap
* @param _ap_offset
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void stprfs(java.lang.String uplo, java.lang.String trans, java.lang.String diag, int n, int nrhs, float[] ap, int _ap_offset, float[] b, int _b_offset, int ldb, float[] x, int _x_offset, int ldx, float[] ferr, int _ferr_offset, float[] berr, int _berr_offset, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STPTRI computes the inverse of a real upper or lower triangular
* matrix A stored in packed format.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangular matrix A, stored
* columnwise in a linear array. The j-th column of A is stored
* in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*((2*n-j)/2) = A(i,j) for j<=i<=n.
* See below for further details.
* On exit, the (triangular) inverse of the original matrix, in
* the same packed storage format.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, A(i,i) is exactly zero. The triangular
* matrix is singular and its inverse can not be computed.
*
* Further Details
* ===============
*
* A triangular matrix A can be transferred to packed storage using one
* of the following program segments:
*
* UPLO = 'U': UPLO = 'L':
*
* JC = 1 JC = 1
* DO 2 J = 1, N DO 2 J = 1, N
* DO 1 I = 1, J DO 1 I = J, N
* AP(JC+I-1) = A(I,J) AP(JC+I-J) = A(I,J)
* 1 CONTINUE 1 CONTINUE
* JC = JC + J JC = JC + N - J + 1
* 2 CONTINUE 2 CONTINUE
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param diag
* @param n
* @param ap
* @param info
*
*/
abstract public void stptri(java.lang.String uplo, java.lang.String diag, int n, float[] ap, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STPTRI computes the inverse of a real upper or lower triangular
* matrix A stored in packed format.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* AP (input/output) REAL array, dimension (N*(N+1)/2)
* On entry, the upper or lower triangular matrix A, stored
* columnwise in a linear array. The j-th column of A is stored
* in the array AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*((2*n-j)/2) = A(i,j) for j<=i<=n.
* See below for further details.
* On exit, the (triangular) inverse of the original matrix, in
* the same packed storage format.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, A(i,i) is exactly zero. The triangular
* matrix is singular and its inverse can not be computed.
*
* Further Details
* ===============
*
* A triangular matrix A can be transferred to packed storage using one
* of the following program segments:
*
* UPLO = 'U': UPLO = 'L':
*
* JC = 1 JC = 1
* DO 2 J = 1, N DO 2 J = 1, N
* DO 1 I = 1, J DO 1 I = J, N
* AP(JC+I-1) = A(I,J) AP(JC+I-J) = A(I,J)
* 1 CONTINUE 1 CONTINUE
* JC = JC + J JC = JC + N - J + 1
* 2 CONTINUE 2 CONTINUE
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param diag
* @param n
* @param ap
* @param _ap_offset
* @param info
*
*/
abstract public void stptri(java.lang.String uplo, java.lang.String diag, int n, float[] ap, int _ap_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STPTRS solves a triangular system of the form
*
* A * X = B or A**T * X = B,
*
* where A is a triangular matrix of order N stored in packed format,
* and B is an N-by-NRHS matrix. A check is made to verify that A is
* nonsingular.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AP (input) REAL array, dimension (N*(N+1)/2)
* The upper or lower triangular matrix A, packed columnwise in
* a linear array. The j-th column of A is stored in the array
* AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, if INFO = 0, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the i-th diagonal element of A is zero,
* indicating that the matrix is singular and the
* solutions X have not been computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param n
* @param nrhs
* @param ap
* @param b
* @param ldb
* @param info
*
*/
abstract public void stptrs(java.lang.String uplo, java.lang.String trans, java.lang.String diag, int n, int nrhs, float[] ap, float[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STPTRS solves a triangular system of the form
*
* A * X = B or A**T * X = B,
*
* where A is a triangular matrix of order N stored in packed format,
* and B is an N-by-NRHS matrix. A check is made to verify that A is
* nonsingular.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* AP (input) REAL array, dimension (N*(N+1)/2)
* The upper or lower triangular matrix A, packed columnwise in
* a linear array. The j-th column of A is stored in the array
* AP as follows:
* if UPLO = 'U', AP(i + (j-1)*j/2) = A(i,j) for 1<=i<=j;
* if UPLO = 'L', AP(i + (j-1)*(2*n-j)/2) = A(i,j) for j<=i<=n.
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, if INFO = 0, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the i-th diagonal element of A is zero,
* indicating that the matrix is singular and the
* solutions X have not been computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param n
* @param nrhs
* @param ap
* @param _ap_offset
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void stptrs(java.lang.String uplo, java.lang.String trans, java.lang.String diag, int n, int nrhs, float[] ap, int _ap_offset, float[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STRCON estimates the reciprocal of the condition number of a
* triangular matrix A, in either the 1-norm or the infinity-norm.
*
* The norm of A is computed and an estimate is obtained for
* norm(inv(A)), then the reciprocal of the condition number is
* computed as
* RCOND = 1 / ( norm(A) * norm(inv(A)) ).
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies whether the 1-norm condition number or the
* infinity-norm condition number is required:
* = '1' or 'O': 1-norm;
* = 'I': Infinity-norm.
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The triangular matrix A. If UPLO = 'U', the leading N-by-N
* upper triangular part of the array A contains the upper
* triangular matrix, and the strictly lower triangular part of
* A is not referenced. If UPLO = 'L', the leading N-by-N lower
* triangular part of the array A contains the lower triangular
* matrix, and the strictly upper triangular part of A is not
* referenced. If DIAG = 'U', the diagonal elements of A are
* also not referenced and are assumed to be 1.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* RCOND (output) REAL
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(norm(A) * norm(inv(A))).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param diag
* @param n
* @param a
* @param lda
* @param rcond
* @param work
* @param iwork
* @param info
*
*/
abstract public void strcon(java.lang.String norm, java.lang.String uplo, java.lang.String diag, int n, float[] a, int lda, org.netlib.util.floatW rcond, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STRCON estimates the reciprocal of the condition number of a
* triangular matrix A, in either the 1-norm or the infinity-norm.
*
* The norm of A is computed and an estimate is obtained for
* norm(inv(A)), then the reciprocal of the condition number is
* computed as
* RCOND = 1 / ( norm(A) * norm(inv(A)) ).
*
* Arguments
* =========
*
* NORM (input) CHARACTER*1
* Specifies whether the 1-norm condition number or the
* infinity-norm condition number is required:
* = '1' or 'O': 1-norm;
* = 'I': Infinity-norm.
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The triangular matrix A. If UPLO = 'U', the leading N-by-N
* upper triangular part of the array A contains the upper
* triangular matrix, and the strictly lower triangular part of
* A is not referenced. If UPLO = 'L', the leading N-by-N lower
* triangular part of the array A contains the lower triangular
* matrix, and the strictly upper triangular part of A is not
* referenced. If DIAG = 'U', the diagonal elements of A are
* also not referenced and are assumed to be 1.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* RCOND (output) REAL
* The reciprocal of the condition number of the matrix A,
* computed as RCOND = 1/(norm(A) * norm(inv(A))).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param norm
* @param uplo
* @param diag
* @param n
* @param a
* @param _a_offset
* @param lda
* @param rcond
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void strcon(java.lang.String norm, java.lang.String uplo, java.lang.String diag, int n, float[] a, int _a_offset, int lda, org.netlib.util.floatW rcond, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STREVC computes some or all of the right and/or left eigenvectors of
* a real upper quasi-triangular matrix T.
* Matrices of this type are produced by the Schur factorization of
* a real general matrix: A = Q*T*Q**T, as computed by SHSEQR.
*
* The right eigenvector x and the left eigenvector y of T corresponding
* to an eigenvalue w are defined by:
*
* T*x = w*x, (y**H)*T = w*(y**H)
*
* where y**H denotes the conjugate transpose of y.
* The eigenvalues are not input to this routine, but are read directly
* from the diagonal blocks of T.
*
* This routine returns the matrices X and/or Y of right and left
* eigenvectors of T, or the products Q*X and/or Q*Y, where Q is an
* input matrix. If Q is the orthogonal factor that reduces a matrix
* A to Schur form T, then Q*X and Q*Y are the matrices of right and
* left eigenvectors of A.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'R': compute right eigenvectors only;
* = 'L': compute left eigenvectors only;
* = 'B': compute both right and left eigenvectors.
*
* HOWMNY (input) CHARACTER*1
* = 'A': compute all right and/or left eigenvectors;
* = 'B': compute all right and/or left eigenvectors,
* backtransformed by the matrices in VR and/or VL;
* = 'S': compute selected right and/or left eigenvectors,
* as indicated by the logical array SELECT.
*
* SELECT (input/output) LOGICAL array, dimension (N)
* If HOWMNY = 'S', SELECT specifies the eigenvectors to be
* computed.
* If w(j) is a real eigenvalue, the corresponding real
* eigenvector is computed if SELECT(j) is .TRUE..
* If w(j) and w(j+1) are the real and imaginary parts of a
* complex eigenvalue, the corresponding complex eigenvector is
* computed if either SELECT(j) or SELECT(j+1) is .TRUE., and
* on exit SELECT(j) is set to .TRUE. and SELECT(j+1) is set to
* .FALSE..
* Not referenced if HOWMNY = 'A' or 'B'.
*
* N (input) INTEGER
* The order of the matrix T. N >= 0.
*
* T (input) REAL array, dimension (LDT,N)
* The upper quasi-triangular matrix T in Schur canonical form.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= max(1,N).
*
* VL (input/output) REAL array, dimension (LDVL,MM)
* On entry, if SIDE = 'L' or 'B' and HOWMNY = 'B', VL must
* contain an N-by-N matrix Q (usually the orthogonal matrix Q
* of Schur vectors returned by SHSEQR).
* On exit, if SIDE = 'L' or 'B', VL contains:
* if HOWMNY = 'A', the matrix Y of left eigenvectors of T;
* if HOWMNY = 'B', the matrix Q*Y;
* if HOWMNY = 'S', the left eigenvectors of T specified by
* SELECT, stored consecutively in the columns
* of VL, in the same order as their
* eigenvalues.
* A complex eigenvector corresponding to a complex eigenvalue
* is stored in two consecutive columns, the first holding the
* real part, and the second the imaginary part.
* Not referenced if SIDE = 'R'.
*
* LDVL (input) INTEGER
* The leading dimension of the array VL. LDVL >= 1, and if
* SIDE = 'L' or 'B', LDVL >= N.
*
* VR (input/output) REAL array, dimension (LDVR,MM)
* On entry, if SIDE = 'R' or 'B' and HOWMNY = 'B', VR must
* contain an N-by-N matrix Q (usually the orthogonal matrix Q
* of Schur vectors returned by SHSEQR).
* On exit, if SIDE = 'R' or 'B', VR contains:
* if HOWMNY = 'A', the matrix X of right eigenvectors of T;
* if HOWMNY = 'B', the matrix Q*X;
* if HOWMNY = 'S', the right eigenvectors of T specified by
* SELECT, stored consecutively in the columns
* of VR, in the same order as their
* eigenvalues.
* A complex eigenvector corresponding to a complex eigenvalue
* is stored in two consecutive columns, the first holding the
* real part and the second the imaginary part.
* Not referenced if SIDE = 'L'.
*
* LDVR (input) INTEGER
* The leading dimension of the array VR. LDVR >= 1, and if
* SIDE = 'R' or 'B', LDVR >= N.
*
* MM (input) INTEGER
* The number of columns in the arrays VL and/or VR. MM >= M.
*
* M (output) INTEGER
* The number of columns in the arrays VL and/or VR actually
* used to store the eigenvectors.
* If HOWMNY = 'A' or 'B', M is set to N.
* Each selected real eigenvector occupies one column and each
* selected complex eigenvector occupies two columns.
*
* WORK (workspace) REAL array, dimension (3*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The algorithm used in this program is basically backward (forward)
* substitution, with scaling to make the the code robust against
* possible overflow.
*
* Each eigenvector is normalized so that the element of largest
* magnitude has magnitude 1; here the magnitude of a complex number
* (x,y) is taken to be |x| + |y|.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param howmny
* @param select
* @param n
* @param t
* @param ldt
* @param vl
* @param ldvl
* @param vr
* @param ldvr
* @param mm
* @param m
* @param work
* @param info
*
*/
abstract public void strevc(java.lang.String side, java.lang.String howmny, boolean[] select, int n, float[] t, int ldt, float[] vl, int ldvl, float[] vr, int ldvr, int mm, org.netlib.util.intW m, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STREVC computes some or all of the right and/or left eigenvectors of
* a real upper quasi-triangular matrix T.
* Matrices of this type are produced by the Schur factorization of
* a real general matrix: A = Q*T*Q**T, as computed by SHSEQR.
*
* The right eigenvector x and the left eigenvector y of T corresponding
* to an eigenvalue w are defined by:
*
* T*x = w*x, (y**H)*T = w*(y**H)
*
* where y**H denotes the conjugate transpose of y.
* The eigenvalues are not input to this routine, but are read directly
* from the diagonal blocks of T.
*
* This routine returns the matrices X and/or Y of right and left
* eigenvectors of T, or the products Q*X and/or Q*Y, where Q is an
* input matrix. If Q is the orthogonal factor that reduces a matrix
* A to Schur form T, then Q*X and Q*Y are the matrices of right and
* left eigenvectors of A.
*
* Arguments
* =========
*
* SIDE (input) CHARACTER*1
* = 'R': compute right eigenvectors only;
* = 'L': compute left eigenvectors only;
* = 'B': compute both right and left eigenvectors.
*
* HOWMNY (input) CHARACTER*1
* = 'A': compute all right and/or left eigenvectors;
* = 'B': compute all right and/or left eigenvectors,
* backtransformed by the matrices in VR and/or VL;
* = 'S': compute selected right and/or left eigenvectors,
* as indicated by the logical array SELECT.
*
* SELECT (input/output) LOGICAL array, dimension (N)
* If HOWMNY = 'S', SELECT specifies the eigenvectors to be
* computed.
* If w(j) is a real eigenvalue, the corresponding real
* eigenvector is computed if SELECT(j) is .TRUE..
* If w(j) and w(j+1) are the real and imaginary parts of a
* complex eigenvalue, the corresponding complex eigenvector is
* computed if either SELECT(j) or SELECT(j+1) is .TRUE., and
* on exit SELECT(j) is set to .TRUE. and SELECT(j+1) is set to
* .FALSE..
* Not referenced if HOWMNY = 'A' or 'B'.
*
* N (input) INTEGER
* The order of the matrix T. N >= 0.
*
* T (input) REAL array, dimension (LDT,N)
* The upper quasi-triangular matrix T in Schur canonical form.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= max(1,N).
*
* VL (input/output) REAL array, dimension (LDVL,MM)
* On entry, if SIDE = 'L' or 'B' and HOWMNY = 'B', VL must
* contain an N-by-N matrix Q (usually the orthogonal matrix Q
* of Schur vectors returned by SHSEQR).
* On exit, if SIDE = 'L' or 'B', VL contains:
* if HOWMNY = 'A', the matrix Y of left eigenvectors of T;
* if HOWMNY = 'B', the matrix Q*Y;
* if HOWMNY = 'S', the left eigenvectors of T specified by
* SELECT, stored consecutively in the columns
* of VL, in the same order as their
* eigenvalues.
* A complex eigenvector corresponding to a complex eigenvalue
* is stored in two consecutive columns, the first holding the
* real part, and the second the imaginary part.
* Not referenced if SIDE = 'R'.
*
* LDVL (input) INTEGER
* The leading dimension of the array VL. LDVL >= 1, and if
* SIDE = 'L' or 'B', LDVL >= N.
*
* VR (input/output) REAL array, dimension (LDVR,MM)
* On entry, if SIDE = 'R' or 'B' and HOWMNY = 'B', VR must
* contain an N-by-N matrix Q (usually the orthogonal matrix Q
* of Schur vectors returned by SHSEQR).
* On exit, if SIDE = 'R' or 'B', VR contains:
* if HOWMNY = 'A', the matrix X of right eigenvectors of T;
* if HOWMNY = 'B', the matrix Q*X;
* if HOWMNY = 'S', the right eigenvectors of T specified by
* SELECT, stored consecutively in the columns
* of VR, in the same order as their
* eigenvalues.
* A complex eigenvector corresponding to a complex eigenvalue
* is stored in two consecutive columns, the first holding the
* real part and the second the imaginary part.
* Not referenced if SIDE = 'L'.
*
* LDVR (input) INTEGER
* The leading dimension of the array VR. LDVR >= 1, and if
* SIDE = 'R' or 'B', LDVR >= N.
*
* MM (input) INTEGER
* The number of columns in the arrays VL and/or VR. MM >= M.
*
* M (output) INTEGER
* The number of columns in the arrays VL and/or VR actually
* used to store the eigenvectors.
* If HOWMNY = 'A' or 'B', M is set to N.
* Each selected real eigenvector occupies one column and each
* selected complex eigenvector occupies two columns.
*
* WORK (workspace) REAL array, dimension (3*N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The algorithm used in this program is basically backward (forward)
* substitution, with scaling to make the the code robust against
* possible overflow.
*
* Each eigenvector is normalized so that the element of largest
* magnitude has magnitude 1; here the magnitude of a complex number
* (x,y) is taken to be |x| + |y|.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param side
* @param howmny
* @param select
* @param _select_offset
* @param n
* @param t
* @param _t_offset
* @param ldt
* @param vl
* @param _vl_offset
* @param ldvl
* @param vr
* @param _vr_offset
* @param ldvr
* @param mm
* @param m
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void strevc(java.lang.String side, java.lang.String howmny, boolean[] select, int _select_offset, int n, float[] t, int _t_offset, int ldt, float[] vl, int _vl_offset, int ldvl, float[] vr, int _vr_offset, int ldvr, int mm, org.netlib.util.intW m, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STREXC reorders the real Schur factorization of a real matrix
* A = Q*T*Q**T, so that the diagonal block of T with row index IFST is
* moved to row ILST.
*
* The real Schur form T is reordered by an orthogonal similarity
* transformation Z**T*T*Z, and optionally the matrix Q of Schur vectors
* is updated by postmultiplying it with Z.
*
* T must be in Schur canonical form (as returned by SHSEQR), that is,
* block upper triangular with 1-by-1 and 2-by-2 diagonal blocks; each
* 2-by-2 diagonal block has its diagonal elements equal and its
* off-diagonal elements of opposite sign.
*
* Arguments
* =========
*
* COMPQ (input) CHARACTER*1
* = 'V': update the matrix Q of Schur vectors;
* = 'N': do not update Q.
*
* N (input) INTEGER
* The order of the matrix T. N >= 0.
*
* T (input/output) REAL array, dimension (LDT,N)
* On entry, the upper quasi-triangular matrix T, in Schur
* Schur canonical form.
* On exit, the reordered upper quasi-triangular matrix, again
* in Schur canonical form.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= max(1,N).
*
* Q (input/output) REAL array, dimension (LDQ,N)
* On entry, if COMPQ = 'V', the matrix Q of Schur vectors.
* On exit, if COMPQ = 'V', Q has been postmultiplied by the
* orthogonal transformation matrix Z which reorders T.
* If COMPQ = 'N', Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N).
*
* IFST (input/output) INTEGER
* ILST (input/output) INTEGER
* Specify the reordering of the diagonal blocks of T.
* The block with row index IFST is moved to row ILST, by a
* sequence of transpositions between adjacent blocks.
* On exit, if IFST pointed on entry to the second row of a
* 2-by-2 block, it is changed to point to the first row; ILST
* always points to the first row of the block in its final
* position (which may differ from its input value by +1 or -1).
* 1 <= IFST <= N; 1 <= ILST <= N.
*
* WORK (workspace) REAL array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* = 1: two adjacent blocks were too close to swap (the problem
* is very ill-conditioned); T may have been partially
* reordered, and ILST points to the first row of the
* current position of the block being moved.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param compq
* @param n
* @param t
* @param ldt
* @param q
* @param ldq
* @param ifst
* @param ilst
* @param work
* @param info
*
*/
abstract public void strexc(java.lang.String compq, int n, float[] t, int ldt, float[] q, int ldq, org.netlib.util.intW ifst, org.netlib.util.intW ilst, float[] work, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STREXC reorders the real Schur factorization of a real matrix
* A = Q*T*Q**T, so that the diagonal block of T with row index IFST is
* moved to row ILST.
*
* The real Schur form T is reordered by an orthogonal similarity
* transformation Z**T*T*Z, and optionally the matrix Q of Schur vectors
* is updated by postmultiplying it with Z.
*
* T must be in Schur canonical form (as returned by SHSEQR), that is,
* block upper triangular with 1-by-1 and 2-by-2 diagonal blocks; each
* 2-by-2 diagonal block has its diagonal elements equal and its
* off-diagonal elements of opposite sign.
*
* Arguments
* =========
*
* COMPQ (input) CHARACTER*1
* = 'V': update the matrix Q of Schur vectors;
* = 'N': do not update Q.
*
* N (input) INTEGER
* The order of the matrix T. N >= 0.
*
* T (input/output) REAL array, dimension (LDT,N)
* On entry, the upper quasi-triangular matrix T, in Schur
* Schur canonical form.
* On exit, the reordered upper quasi-triangular matrix, again
* in Schur canonical form.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= max(1,N).
*
* Q (input/output) REAL array, dimension (LDQ,N)
* On entry, if COMPQ = 'V', the matrix Q of Schur vectors.
* On exit, if COMPQ = 'V', Q has been postmultiplied by the
* orthogonal transformation matrix Z which reorders T.
* If COMPQ = 'N', Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q. LDQ >= max(1,N).
*
* IFST (input/output) INTEGER
* ILST (input/output) INTEGER
* Specify the reordering of the diagonal blocks of T.
* The block with row index IFST is moved to row ILST, by a
* sequence of transpositions between adjacent blocks.
* On exit, if IFST pointed on entry to the second row of a
* 2-by-2 block, it is changed to point to the first row; ILST
* always points to the first row of the block in its final
* position (which may differ from its input value by +1 or -1).
* 1 <= IFST <= N; 1 <= ILST <= N.
*
* WORK (workspace) REAL array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* = 1: two adjacent blocks were too close to swap (the problem
* is very ill-conditioned); T may have been partially
* reordered, and ILST points to the first row of the
* current position of the block being moved.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param compq
* @param n
* @param t
* @param _t_offset
* @param ldt
* @param q
* @param _q_offset
* @param ldq
* @param ifst
* @param ilst
* @param work
* @param _work_offset
* @param info
*
*/
abstract public void strexc(java.lang.String compq, int n, float[] t, int _t_offset, int ldt, float[] q, int _q_offset, int ldq, org.netlib.util.intW ifst, org.netlib.util.intW ilst, float[] work, int _work_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STRRFS provides error bounds and backward error estimates for the
* solution to a system of linear equations with a triangular
* coefficient matrix.
*
* The solution matrix X must be computed by STRTRS or some other
* means before entering this routine. STRRFS does not do iterative
* refinement because doing so cannot improve the backward error.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The triangular matrix A. If UPLO = 'U', the leading N-by-N
* upper triangular part of the array A contains the upper
* triangular matrix, and the strictly lower triangular part of
* A is not referenced. If UPLO = 'L', the leading N-by-N lower
* triangular part of the array A contains the lower triangular
* matrix, and the strictly upper triangular part of A is not
* referenced. If DIAG = 'U', the diagonal elements of A are
* also not referenced and are assumed to be 1.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input) REAL array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input) REAL array, dimension (LDX,NRHS)
* The solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param n
* @param nrhs
* @param a
* @param lda
* @param b
* @param ldb
* @param x
* @param ldx
* @param ferr
* @param berr
* @param work
* @param iwork
* @param info
*
*/
abstract public void strrfs(java.lang.String uplo, java.lang.String trans, java.lang.String diag, int n, int nrhs, float[] a, int lda, float[] b, int ldb, float[] x, int ldx, float[] ferr, float[] berr, float[] work, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STRRFS provides error bounds and backward error estimates for the
* solution to a system of linear equations with a triangular
* coefficient matrix.
*
* The solution matrix X must be computed by STRTRS or some other
* means before entering this routine. STRRFS does not do iterative
* refinement because doing so cannot improve the backward error.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrices B and X. NRHS >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The triangular matrix A. If UPLO = 'U', the leading N-by-N
* upper triangular part of the array A contains the upper
* triangular matrix, and the strictly lower triangular part of
* A is not referenced. If UPLO = 'L', the leading N-by-N lower
* triangular part of the array A contains the lower triangular
* matrix, and the strictly upper triangular part of A is not
* referenced. If DIAG = 'U', the diagonal elements of A are
* also not referenced and are assumed to be 1.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input) REAL array, dimension (LDB,NRHS)
* The right hand side matrix B.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* X (input) REAL array, dimension (LDX,NRHS)
* The solution matrix X.
*
* LDX (input) INTEGER
* The leading dimension of the array X. LDX >= max(1,N).
*
* FERR (output) REAL array, dimension (NRHS)
* The estimated forward error bound for each solution vector
* X(j) (the j-th column of the solution matrix X).
* If XTRUE is the true solution corresponding to X(j), FERR(j)
* is an estimated upper bound for the magnitude of the largest
* element in (X(j) - XTRUE) divided by the magnitude of the
* largest element in X(j). The estimate is as reliable as
* the estimate for RCOND, and is almost always a slight
* overestimate of the true error.
*
* BERR (output) REAL array, dimension (NRHS)
* The componentwise relative backward error of each solution
* vector X(j) (i.e., the smallest relative change in
* any element of A or B that makes X(j) an exact solution).
*
* WORK (workspace) REAL array, dimension (3*N)
*
* IWORK (workspace) INTEGER array, dimension (N)
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param x
* @param _x_offset
* @param ldx
* @param ferr
* @param _ferr_offset
* @param berr
* @param _berr_offset
* @param work
* @param _work_offset
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void strrfs(java.lang.String uplo, java.lang.String trans, java.lang.String diag, int n, int nrhs, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, float[] x, int _x_offset, int ldx, float[] ferr, int _ferr_offset, float[] berr, int _berr_offset, float[] work, int _work_offset, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STRSEN reorders the real Schur factorization of a real matrix
* A = Q*T*Q**T, so that a selected cluster of eigenvalues appears in
* the leading diagonal blocks of the upper quasi-triangular matrix T,
* and the leading columns of Q form an orthonormal basis of the
* corresponding right invariant subspace.
*
* Optionally the routine computes the reciprocal condition numbers of
* the cluster of eigenvalues and/or the invariant subspace.
*
* T must be in Schur canonical form (as returned by SHSEQR), that is,
* block upper triangular with 1-by-1 and 2-by-2 diagonal blocks; each
* 2-by-2 diagonal block has its diagonal elemnts equal and its
* off-diagonal elements of opposite sign.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* Specifies whether condition numbers are required for the
* cluster of eigenvalues (S) or the invariant subspace (SEP):
* = 'N': none;
* = 'E': for eigenvalues only (S);
* = 'V': for invariant subspace only (SEP);
* = 'B': for both eigenvalues and invariant subspace (S and
* SEP).
*
* COMPQ (input) CHARACTER*1
* = 'V': update the matrix Q of Schur vectors;
* = 'N': do not update Q.
*
* SELECT (input) LOGICAL array, dimension (N)
* SELECT specifies the eigenvalues in the selected cluster. To
* select a real eigenvalue w(j), SELECT(j) must be set to
* .TRUE.. To select a complex conjugate pair of eigenvalues
* w(j) and w(j+1), corresponding to a 2-by-2 diagonal block,
* either SELECT(j) or SELECT(j+1) or both must be set to
* .TRUE.; a complex conjugate pair of eigenvalues must be
* either both included in the cluster or both excluded.
*
* N (input) INTEGER
* The order of the matrix T. N >= 0.
*
* T (input/output) REAL array, dimension (LDT,N)
* On entry, the upper quasi-triangular matrix T, in Schur
* canonical form.
* On exit, T is overwritten by the reordered matrix T, again in
* Schur canonical form, with the selected eigenvalues in the
* leading diagonal blocks.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= max(1,N).
*
* Q (input/output) REAL array, dimension (LDQ,N)
* On entry, if COMPQ = 'V', the matrix Q of Schur vectors.
* On exit, if COMPQ = 'V', Q has been postmultiplied by the
* orthogonal transformation matrix which reorders T; the
* leading M columns of Q form an orthonormal basis for the
* specified invariant subspace.
* If COMPQ = 'N', Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q.
* LDQ >= 1; and if COMPQ = 'V', LDQ >= N.
*
* WR (output) REAL array, dimension (N)
* WI (output) REAL array, dimension (N)
* The real and imaginary parts, respectively, of the reordered
* eigenvalues of T. The eigenvalues are stored in the same
* order as on the diagonal of T, with WR(i) = T(i,i) and, if
* T(i:i+1,i:i+1) is a 2-by-2 diagonal block, WI(i) > 0 and
* WI(i+1) = -WI(i). Note that if a complex eigenvalue is
* sufficiently ill-conditioned, then its value may differ
* significantly from its value before reordering.
*
* M (output) INTEGER
* The dimension of the specified invariant subspace.
* 0 < = M <= N.
*
* S (output) REAL
* If JOB = 'E' or 'B', S is a lower bound on the reciprocal
* condition number for the selected cluster of eigenvalues.
* S cannot underestimate the true reciprocal condition number
* by more than a factor of sqrt(N). If M = 0 or N, S = 1.
* If JOB = 'N' or 'V', S is not referenced.
*
* SEP (output) REAL
* If JOB = 'V' or 'B', SEP is the estimated reciprocal
* condition number of the specified invariant subspace. If
* M = 0 or N, SEP = norm(T).
* If JOB = 'N' or 'E', SEP is not referenced.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If JOB = 'N', LWORK >= max(1,N);
* if JOB = 'E', LWORK >= max(1,M*(N-M));
* if JOB = 'V' or 'B', LWORK >= max(1,2*M*(N-M)).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If JOB = 'N' or 'E', LIWORK >= 1;
* if JOB = 'V' or 'B', LIWORK >= max(1,M*(N-M)).
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal size of the IWORK array,
* returns this value as the first entry of the IWORK array, and
* no error message related to LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* = 1: reordering of T failed because some eigenvalues are too
* close to separate (the problem is very ill-conditioned);
* T may have been partially reordered, and WR and WI
* contain the eigenvalues in the same order as in T; S and
* SEP (if requested) are set to zero.
*
* Further Details
* ===============
*
* STRSEN first collects the selected eigenvalues by computing an
* orthogonal transformation Z to move them to the top left corner of T.
* In other words, the selected eigenvalues are the eigenvalues of T11
* in:
*
* Z'*T*Z = ( T11 T12 ) n1
* ( 0 T22 ) n2
* n1 n2
*
* where N = n1+n2 and Z' means the transpose of Z. The first n1 columns
* of Z span the specified invariant subspace of T.
*
* If T has been obtained from the real Schur factorization of a matrix
* A = Q*T*Q', then the reordered real Schur factorization of A is given
* by A = (Q*Z)*(Z'*T*Z)*(Q*Z)', and the first n1 columns of Q*Z span
* the corresponding invariant subspace of A.
*
* The reciprocal condition number of the average of the eigenvalues of
* T11 may be returned in S. S lies between 0 (very badly conditioned)
* and 1 (very well conditioned). It is computed as follows. First we
* compute R so that
*
* P = ( I R ) n1
* ( 0 0 ) n2
* n1 n2
*
* is the projector on the invariant subspace associated with T11.
* R is the solution of the Sylvester equation:
*
* T11*R - R*T22 = T12.
*
* Let F-norm(M) denote the Frobenius-norm of M and 2-norm(M) denote
* the two-norm of M. Then S is computed as the lower bound
*
* (1 + F-norm(R)**2)**(-1/2)
*
* on the reciprocal of 2-norm(P), the true reciprocal condition number.
* S cannot underestimate 1 / 2-norm(P) by more than a factor of
* sqrt(N).
*
* An approximate error bound for the computed average of the
* eigenvalues of T11 is
*
* EPS * norm(T) / S
*
* where EPS is the machine precision.
*
* The reciprocal condition number of the right invariant subspace
* spanned by the first n1 columns of Z (or of Q*Z) is returned in SEP.
* SEP is defined as the separation of T11 and T22:
*
* sep( T11, T22 ) = sigma-min( C )
*
* where sigma-min(C) is the smallest singular value of the
* n1*n2-by-n1*n2 matrix
*
* C = kprod( I(n2), T11 ) - kprod( transpose(T22), I(n1) )
*
* I(m) is an m by m identity matrix, and kprod denotes the Kronecker
* product. We estimate sigma-min(C) by the reciprocal of an estimate of
* the 1-norm of inverse(C). The true reciprocal 1-norm of inverse(C)
* cannot differ from sigma-min(C) by more than a factor of sqrt(n1*n2).
*
* When SEP is small, small changes in T can cause large changes in
* the invariant subspace. An approximate bound on the maximum angular
* error in the computed right invariant subspace is
*
* EPS * norm(T) / SEP
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param compq
* @param select
* @param n
* @param t
* @param ldt
* @param q
* @param ldq
* @param wr
* @param wi
* @param m
* @param s
* @param sep
* @param work
* @param lwork
* @param iwork
* @param liwork
* @param info
*
*/
abstract public void strsen(java.lang.String job, java.lang.String compq, boolean[] select, int n, float[] t, int ldt, float[] q, int ldq, float[] wr, float[] wi, org.netlib.util.intW m, org.netlib.util.floatW s, org.netlib.util.floatW sep, float[] work, int lwork, int[] iwork, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STRSEN reorders the real Schur factorization of a real matrix
* A = Q*T*Q**T, so that a selected cluster of eigenvalues appears in
* the leading diagonal blocks of the upper quasi-triangular matrix T,
* and the leading columns of Q form an orthonormal basis of the
* corresponding right invariant subspace.
*
* Optionally the routine computes the reciprocal condition numbers of
* the cluster of eigenvalues and/or the invariant subspace.
*
* T must be in Schur canonical form (as returned by SHSEQR), that is,
* block upper triangular with 1-by-1 and 2-by-2 diagonal blocks; each
* 2-by-2 diagonal block has its diagonal elemnts equal and its
* off-diagonal elements of opposite sign.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* Specifies whether condition numbers are required for the
* cluster of eigenvalues (S) or the invariant subspace (SEP):
* = 'N': none;
* = 'E': for eigenvalues only (S);
* = 'V': for invariant subspace only (SEP);
* = 'B': for both eigenvalues and invariant subspace (S and
* SEP).
*
* COMPQ (input) CHARACTER*1
* = 'V': update the matrix Q of Schur vectors;
* = 'N': do not update Q.
*
* SELECT (input) LOGICAL array, dimension (N)
* SELECT specifies the eigenvalues in the selected cluster. To
* select a real eigenvalue w(j), SELECT(j) must be set to
* .TRUE.. To select a complex conjugate pair of eigenvalues
* w(j) and w(j+1), corresponding to a 2-by-2 diagonal block,
* either SELECT(j) or SELECT(j+1) or both must be set to
* .TRUE.; a complex conjugate pair of eigenvalues must be
* either both included in the cluster or both excluded.
*
* N (input) INTEGER
* The order of the matrix T. N >= 0.
*
* T (input/output) REAL array, dimension (LDT,N)
* On entry, the upper quasi-triangular matrix T, in Schur
* canonical form.
* On exit, T is overwritten by the reordered matrix T, again in
* Schur canonical form, with the selected eigenvalues in the
* leading diagonal blocks.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= max(1,N).
*
* Q (input/output) REAL array, dimension (LDQ,N)
* On entry, if COMPQ = 'V', the matrix Q of Schur vectors.
* On exit, if COMPQ = 'V', Q has been postmultiplied by the
* orthogonal transformation matrix which reorders T; the
* leading M columns of Q form an orthonormal basis for the
* specified invariant subspace.
* If COMPQ = 'N', Q is not referenced.
*
* LDQ (input) INTEGER
* The leading dimension of the array Q.
* LDQ >= 1; and if COMPQ = 'V', LDQ >= N.
*
* WR (output) REAL array, dimension (N)
* WI (output) REAL array, dimension (N)
* The real and imaginary parts, respectively, of the reordered
* eigenvalues of T. The eigenvalues are stored in the same
* order as on the diagonal of T, with WR(i) = T(i,i) and, if
* T(i:i+1,i:i+1) is a 2-by-2 diagonal block, WI(i) > 0 and
* WI(i+1) = -WI(i). Note that if a complex eigenvalue is
* sufficiently ill-conditioned, then its value may differ
* significantly from its value before reordering.
*
* M (output) INTEGER
* The dimension of the specified invariant subspace.
* 0 < = M <= N.
*
* S (output) REAL
* If JOB = 'E' or 'B', S is a lower bound on the reciprocal
* condition number for the selected cluster of eigenvalues.
* S cannot underestimate the true reciprocal condition number
* by more than a factor of sqrt(N). If M = 0 or N, S = 1.
* If JOB = 'N' or 'V', S is not referenced.
*
* SEP (output) REAL
* If JOB = 'V' or 'B', SEP is the estimated reciprocal
* condition number of the specified invariant subspace. If
* M = 0 or N, SEP = norm(T).
* If JOB = 'N' or 'E', SEP is not referenced.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK.
* If JOB = 'N', LWORK >= max(1,N);
* if JOB = 'E', LWORK >= max(1,M*(N-M));
* if JOB = 'V' or 'B', LWORK >= max(1,2*M*(N-M)).
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* IWORK (workspace) INTEGER array, dimension (MAX(1,LIWORK))
* On exit, if INFO = 0, IWORK(1) returns the optimal LIWORK.
*
* LIWORK (input) INTEGER
* The dimension of the array IWORK.
* If JOB = 'N' or 'E', LIWORK >= 1;
* if JOB = 'V' or 'B', LIWORK >= max(1,M*(N-M)).
*
* If LIWORK = -1, then a workspace query is assumed; the
* routine only calculates the optimal size of the IWORK array,
* returns this value as the first entry of the IWORK array, and
* no error message related to LIWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* = 1: reordering of T failed because some eigenvalues are too
* close to separate (the problem is very ill-conditioned);
* T may have been partially reordered, and WR and WI
* contain the eigenvalues in the same order as in T; S and
* SEP (if requested) are set to zero.
*
* Further Details
* ===============
*
* STRSEN first collects the selected eigenvalues by computing an
* orthogonal transformation Z to move them to the top left corner of T.
* In other words, the selected eigenvalues are the eigenvalues of T11
* in:
*
* Z'*T*Z = ( T11 T12 ) n1
* ( 0 T22 ) n2
* n1 n2
*
* where N = n1+n2 and Z' means the transpose of Z. The first n1 columns
* of Z span the specified invariant subspace of T.
*
* If T has been obtained from the real Schur factorization of a matrix
* A = Q*T*Q', then the reordered real Schur factorization of A is given
* by A = (Q*Z)*(Z'*T*Z)*(Q*Z)', and the first n1 columns of Q*Z span
* the corresponding invariant subspace of A.
*
* The reciprocal condition number of the average of the eigenvalues of
* T11 may be returned in S. S lies between 0 (very badly conditioned)
* and 1 (very well conditioned). It is computed as follows. First we
* compute R so that
*
* P = ( I R ) n1
* ( 0 0 ) n2
* n1 n2
*
* is the projector on the invariant subspace associated with T11.
* R is the solution of the Sylvester equation:
*
* T11*R - R*T22 = T12.
*
* Let F-norm(M) denote the Frobenius-norm of M and 2-norm(M) denote
* the two-norm of M. Then S is computed as the lower bound
*
* (1 + F-norm(R)**2)**(-1/2)
*
* on the reciprocal of 2-norm(P), the true reciprocal condition number.
* S cannot underestimate 1 / 2-norm(P) by more than a factor of
* sqrt(N).
*
* An approximate error bound for the computed average of the
* eigenvalues of T11 is
*
* EPS * norm(T) / S
*
* where EPS is the machine precision.
*
* The reciprocal condition number of the right invariant subspace
* spanned by the first n1 columns of Z (or of Q*Z) is returned in SEP.
* SEP is defined as the separation of T11 and T22:
*
* sep( T11, T22 ) = sigma-min( C )
*
* where sigma-min(C) is the smallest singular value of the
* n1*n2-by-n1*n2 matrix
*
* C = kprod( I(n2), T11 ) - kprod( transpose(T22), I(n1) )
*
* I(m) is an m by m identity matrix, and kprod denotes the Kronecker
* product. We estimate sigma-min(C) by the reciprocal of an estimate of
* the 1-norm of inverse(C). The true reciprocal 1-norm of inverse(C)
* cannot differ from sigma-min(C) by more than a factor of sqrt(n1*n2).
*
* When SEP is small, small changes in T can cause large changes in
* the invariant subspace. An approximate bound on the maximum angular
* error in the computed right invariant subspace is
*
* EPS * norm(T) / SEP
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param compq
* @param select
* @param _select_offset
* @param n
* @param t
* @param _t_offset
* @param ldt
* @param q
* @param _q_offset
* @param ldq
* @param wr
* @param _wr_offset
* @param wi
* @param _wi_offset
* @param m
* @param s
* @param sep
* @param work
* @param _work_offset
* @param lwork
* @param iwork
* @param _iwork_offset
* @param liwork
* @param info
*
*/
abstract public void strsen(java.lang.String job, java.lang.String compq, boolean[] select, int _select_offset, int n, float[] t, int _t_offset, int ldt, float[] q, int _q_offset, int ldq, float[] wr, int _wr_offset, float[] wi, int _wi_offset, org.netlib.util.intW m, org.netlib.util.floatW s, org.netlib.util.floatW sep, float[] work, int _work_offset, int lwork, int[] iwork, int _iwork_offset, int liwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STRSNA estimates reciprocal condition numbers for specified
* eigenvalues and/or right eigenvectors of a real upper
* quasi-triangular matrix T (or of any matrix Q*T*Q**T with Q
* orthogonal).
*
* T must be in Schur canonical form (as returned by SHSEQR), that is,
* block upper triangular with 1-by-1 and 2-by-2 diagonal blocks; each
* 2-by-2 diagonal block has its diagonal elements equal and its
* off-diagonal elements of opposite sign.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* Specifies whether condition numbers are required for
* eigenvalues (S) or eigenvectors (SEP):
* = 'E': for eigenvalues only (S);
* = 'V': for eigenvectors only (SEP);
* = 'B': for both eigenvalues and eigenvectors (S and SEP).
*
* HOWMNY (input) CHARACTER*1
* = 'A': compute condition numbers for all eigenpairs;
* = 'S': compute condition numbers for selected eigenpairs
* specified by the array SELECT.
*
* SELECT (input) LOGICAL array, dimension (N)
* If HOWMNY = 'S', SELECT specifies the eigenpairs for which
* condition numbers are required. To select condition numbers
* for the eigenpair corresponding to a real eigenvalue w(j),
* SELECT(j) must be set to .TRUE.. To select condition numbers
* corresponding to a complex conjugate pair of eigenvalues w(j)
* and w(j+1), either SELECT(j) or SELECT(j+1) or both, must be
* set to .TRUE..
* If HOWMNY = 'A', SELECT is not referenced.
*
* N (input) INTEGER
* The order of the matrix T. N >= 0.
*
* T (input) REAL array, dimension (LDT,N)
* The upper quasi-triangular matrix T, in Schur canonical form.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= max(1,N).
*
* VL (input) REAL array, dimension (LDVL,M)
* If JOB = 'E' or 'B', VL must contain left eigenvectors of T
* (or of any Q*T*Q**T with Q orthogonal), corresponding to the
* eigenpairs specified by HOWMNY and SELECT. The eigenvectors
* must be stored in consecutive columns of VL, as returned by
* SHSEIN or STREVC.
* If JOB = 'V', VL is not referenced.
*
* LDVL (input) INTEGER
* The leading dimension of the array VL.
* LDVL >= 1; and if JOB = 'E' or 'B', LDVL >= N.
*
* VR (input) REAL array, dimension (LDVR,M)
* If JOB = 'E' or 'B', VR must contain right eigenvectors of T
* (or of any Q*T*Q**T with Q orthogonal), corresponding to the
* eigenpairs specified by HOWMNY and SELECT. The eigenvectors
* must be stored in consecutive columns of VR, as returned by
* SHSEIN or STREVC.
* If JOB = 'V', VR is not referenced.
*
* LDVR (input) INTEGER
* The leading dimension of the array VR.
* LDVR >= 1; and if JOB = 'E' or 'B', LDVR >= N.
*
* S (output) REAL array, dimension (MM)
* If JOB = 'E' or 'B', the reciprocal condition numbers of the
* selected eigenvalues, stored in consecutive elements of the
* array. For a complex conjugate pair of eigenvalues two
* consecutive elements of S are set to the same value. Thus
* S(j), SEP(j), and the j-th columns of VL and VR all
* correspond to the same eigenpair (but not in general the
* j-th eigenpair, unless all eigenpairs are selected).
* If JOB = 'V', S is not referenced.
*
* SEP (output) REAL array, dimension (MM)
* If JOB = 'V' or 'B', the estimated reciprocal condition
* numbers of the selected eigenvectors, stored in consecutive
* elements of the array. For a complex eigenvector two
* consecutive elements of SEP are set to the same value. If
* the eigenvalues cannot be reordered to compute SEP(j), SEP(j)
* is set to 0; this can only occur when the true value would be
* very small anyway.
* If JOB = 'E', SEP is not referenced.
*
* MM (input) INTEGER
* The number of elements in the arrays S (if JOB = 'E' or 'B')
* and/or SEP (if JOB = 'V' or 'B'). MM >= M.
*
* M (output) INTEGER
* The number of elements of the arrays S and/or SEP actually
* used to store the estimated condition numbers.
* If HOWMNY = 'A', M is set to N.
*
* WORK (workspace) REAL array, dimension (LDWORK,N+6)
* If JOB = 'E', WORK is not referenced.
*
* LDWORK (input) INTEGER
* The leading dimension of the array WORK.
* LDWORK >= 1; and if JOB = 'V' or 'B', LDWORK >= N.
*
* IWORK (workspace) INTEGER array, dimension (2*(N-1))
* If JOB = 'E', IWORK is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The reciprocal of the condition number of an eigenvalue lambda is
* defined as
*
* S(lambda) = |v'*u| / (norm(u)*norm(v))
*
* where u and v are the right and left eigenvectors of T corresponding
* to lambda; v' denotes the conjugate-transpose of v, and norm(u)
* denotes the Euclidean norm. These reciprocal condition numbers always
* lie between zero (very badly conditioned) and one (very well
* conditioned). If n = 1, S(lambda) is defined to be 1.
*
* An approximate error bound for a computed eigenvalue W(i) is given by
*
* EPS * norm(T) / S(i)
*
* where EPS is the machine precision.
*
* The reciprocal of the condition number of the right eigenvector u
* corresponding to lambda is defined as follows. Suppose
*
* T = ( lambda c )
* ( 0 T22 )
*
* Then the reciprocal condition number is
*
* SEP( lambda, T22 ) = sigma-min( T22 - lambda*I )
*
* where sigma-min denotes the smallest singular value. We approximate
* the smallest singular value by the reciprocal of an estimate of the
* one-norm of the inverse of T22 - lambda*I. If n = 1, SEP(1) is
* defined to be abs(T(1,1)).
*
* An approximate error bound for a computed right eigenvector VR(i)
* is given by
*
* EPS * norm(T) / SEP(i)
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param howmny
* @param select
* @param n
* @param t
* @param ldt
* @param vl
* @param ldvl
* @param vr
* @param ldvr
* @param s
* @param sep
* @param mm
* @param m
* @param work
* @param ldwork
* @param iwork
* @param info
*
*/
abstract public void strsna(java.lang.String job, java.lang.String howmny, boolean[] select, int n, float[] t, int ldt, float[] vl, int ldvl, float[] vr, int ldvr, float[] s, float[] sep, int mm, org.netlib.util.intW m, float[] work, int ldwork, int[] iwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STRSNA estimates reciprocal condition numbers for specified
* eigenvalues and/or right eigenvectors of a real upper
* quasi-triangular matrix T (or of any matrix Q*T*Q**T with Q
* orthogonal).
*
* T must be in Schur canonical form (as returned by SHSEQR), that is,
* block upper triangular with 1-by-1 and 2-by-2 diagonal blocks; each
* 2-by-2 diagonal block has its diagonal elements equal and its
* off-diagonal elements of opposite sign.
*
* Arguments
* =========
*
* JOB (input) CHARACTER*1
* Specifies whether condition numbers are required for
* eigenvalues (S) or eigenvectors (SEP):
* = 'E': for eigenvalues only (S);
* = 'V': for eigenvectors only (SEP);
* = 'B': for both eigenvalues and eigenvectors (S and SEP).
*
* HOWMNY (input) CHARACTER*1
* = 'A': compute condition numbers for all eigenpairs;
* = 'S': compute condition numbers for selected eigenpairs
* specified by the array SELECT.
*
* SELECT (input) LOGICAL array, dimension (N)
* If HOWMNY = 'S', SELECT specifies the eigenpairs for which
* condition numbers are required. To select condition numbers
* for the eigenpair corresponding to a real eigenvalue w(j),
* SELECT(j) must be set to .TRUE.. To select condition numbers
* corresponding to a complex conjugate pair of eigenvalues w(j)
* and w(j+1), either SELECT(j) or SELECT(j+1) or both, must be
* set to .TRUE..
* If HOWMNY = 'A', SELECT is not referenced.
*
* N (input) INTEGER
* The order of the matrix T. N >= 0.
*
* T (input) REAL array, dimension (LDT,N)
* The upper quasi-triangular matrix T, in Schur canonical form.
*
* LDT (input) INTEGER
* The leading dimension of the array T. LDT >= max(1,N).
*
* VL (input) REAL array, dimension (LDVL,M)
* If JOB = 'E' or 'B', VL must contain left eigenvectors of T
* (or of any Q*T*Q**T with Q orthogonal), corresponding to the
* eigenpairs specified by HOWMNY and SELECT. The eigenvectors
* must be stored in consecutive columns of VL, as returned by
* SHSEIN or STREVC.
* If JOB = 'V', VL is not referenced.
*
* LDVL (input) INTEGER
* The leading dimension of the array VL.
* LDVL >= 1; and if JOB = 'E' or 'B', LDVL >= N.
*
* VR (input) REAL array, dimension (LDVR,M)
* If JOB = 'E' or 'B', VR must contain right eigenvectors of T
* (or of any Q*T*Q**T with Q orthogonal), corresponding to the
* eigenpairs specified by HOWMNY and SELECT. The eigenvectors
* must be stored in consecutive columns of VR, as returned by
* SHSEIN or STREVC.
* If JOB = 'V', VR is not referenced.
*
* LDVR (input) INTEGER
* The leading dimension of the array VR.
* LDVR >= 1; and if JOB = 'E' or 'B', LDVR >= N.
*
* S (output) REAL array, dimension (MM)
* If JOB = 'E' or 'B', the reciprocal condition numbers of the
* selected eigenvalues, stored in consecutive elements of the
* array. For a complex conjugate pair of eigenvalues two
* consecutive elements of S are set to the same value. Thus
* S(j), SEP(j), and the j-th columns of VL and VR all
* correspond to the same eigenpair (but not in general the
* j-th eigenpair, unless all eigenpairs are selected).
* If JOB = 'V', S is not referenced.
*
* SEP (output) REAL array, dimension (MM)
* If JOB = 'V' or 'B', the estimated reciprocal condition
* numbers of the selected eigenvectors, stored in consecutive
* elements of the array. For a complex eigenvector two
* consecutive elements of SEP are set to the same value. If
* the eigenvalues cannot be reordered to compute SEP(j), SEP(j)
* is set to 0; this can only occur when the true value would be
* very small anyway.
* If JOB = 'E', SEP is not referenced.
*
* MM (input) INTEGER
* The number of elements in the arrays S (if JOB = 'E' or 'B')
* and/or SEP (if JOB = 'V' or 'B'). MM >= M.
*
* M (output) INTEGER
* The number of elements of the arrays S and/or SEP actually
* used to store the estimated condition numbers.
* If HOWMNY = 'A', M is set to N.
*
* WORK (workspace) REAL array, dimension (LDWORK,N+6)
* If JOB = 'E', WORK is not referenced.
*
* LDWORK (input) INTEGER
* The leading dimension of the array WORK.
* LDWORK >= 1; and if JOB = 'V' or 'B', LDWORK >= N.
*
* IWORK (workspace) INTEGER array, dimension (2*(N-1))
* If JOB = 'E', IWORK is not referenced.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The reciprocal of the condition number of an eigenvalue lambda is
* defined as
*
* S(lambda) = |v'*u| / (norm(u)*norm(v))
*
* where u and v are the right and left eigenvectors of T corresponding
* to lambda; v' denotes the conjugate-transpose of v, and norm(u)
* denotes the Euclidean norm. These reciprocal condition numbers always
* lie between zero (very badly conditioned) and one (very well
* conditioned). If n = 1, S(lambda) is defined to be 1.
*
* An approximate error bound for a computed eigenvalue W(i) is given by
*
* EPS * norm(T) / S(i)
*
* where EPS is the machine precision.
*
* The reciprocal of the condition number of the right eigenvector u
* corresponding to lambda is defined as follows. Suppose
*
* T = ( lambda c )
* ( 0 T22 )
*
* Then the reciprocal condition number is
*
* SEP( lambda, T22 ) = sigma-min( T22 - lambda*I )
*
* where sigma-min denotes the smallest singular value. We approximate
* the smallest singular value by the reciprocal of an estimate of the
* one-norm of the inverse of T22 - lambda*I. If n = 1, SEP(1) is
* defined to be abs(T(1,1)).
*
* An approximate error bound for a computed right eigenvector VR(i)
* is given by
*
* EPS * norm(T) / SEP(i)
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param job
* @param howmny
* @param select
* @param _select_offset
* @param n
* @param t
* @param _t_offset
* @param ldt
* @param vl
* @param _vl_offset
* @param ldvl
* @param vr
* @param _vr_offset
* @param ldvr
* @param s
* @param _s_offset
* @param sep
* @param _sep_offset
* @param mm
* @param m
* @param work
* @param _work_offset
* @param ldwork
* @param iwork
* @param _iwork_offset
* @param info
*
*/
abstract public void strsna(java.lang.String job, java.lang.String howmny, boolean[] select, int _select_offset, int n, float[] t, int _t_offset, int ldt, float[] vl, int _vl_offset, int ldvl, float[] vr, int _vr_offset, int ldvr, float[] s, int _s_offset, float[] sep, int _sep_offset, int mm, org.netlib.util.intW m, float[] work, int _work_offset, int ldwork, int[] iwork, int _iwork_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STRSYL solves the real Sylvester matrix equation:
*
* op(A)*X + X*op(B) = scale*C or
* op(A)*X - X*op(B) = scale*C,
*
* where op(A) = A or A**T, and A and B are both upper quasi-
* triangular. A is M-by-M and B is N-by-N; the right hand side C and
* the solution X are M-by-N; and scale is an output scale factor, set
* <= 1 to avoid overflow in X.
*
* A and B must be in Schur canonical form (as returned by SHSEQR), that
* is, block upper triangular with 1-by-1 and 2-by-2 diagonal blocks;
* each 2-by-2 diagonal block has its diagonal elements equal and its
* off-diagonal elements of opposite sign.
*
* Arguments
* =========
*
* TRANA (input) CHARACTER*1
* Specifies the option op(A):
* = 'N': op(A) = A (No transpose)
* = 'T': op(A) = A**T (Transpose)
* = 'C': op(A) = A**H (Conjugate transpose = Transpose)
*
* TRANB (input) CHARACTER*1
* Specifies the option op(B):
* = 'N': op(B) = B (No transpose)
* = 'T': op(B) = B**T (Transpose)
* = 'C': op(B) = B**H (Conjugate transpose = Transpose)
*
* ISGN (input) INTEGER
* Specifies the sign in the equation:
* = +1: solve op(A)*X + X*op(B) = scale*C
* = -1: solve op(A)*X - X*op(B) = scale*C
*
* M (input) INTEGER
* The order of the matrix A, and the number of rows in the
* matrices X and C. M >= 0.
*
* N (input) INTEGER
* The order of the matrix B, and the number of columns in the
* matrices X and C. N >= 0.
*
* A (input) REAL array, dimension (LDA,M)
* The upper quasi-triangular matrix A, in Schur canonical form.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input) REAL array, dimension (LDB,N)
* The upper quasi-triangular matrix B, in Schur canonical form.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the M-by-N right hand side matrix C.
* On exit, C is overwritten by the solution matrix X.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M)
*
* SCALE (output) REAL
* The scale factor, scale, set <= 1 to avoid overflow in X.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* = 1: A and B have common or very close eigenvalues; perturbed
* values were used to solve the equation (but the matrices
* A and B are unchanged).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param trana
* @param tranb
* @param isgn
* @param m
* @param n
* @param a
* @param lda
* @param b
* @param ldb
* @param c
* @param Ldc
* @param scale
* @param info
*
*/
abstract public void strsyl(java.lang.String trana, java.lang.String tranb, int isgn, int m, int n, float[] a, int lda, float[] b, int ldb, float[] c, int Ldc, org.netlib.util.floatW scale, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STRSYL solves the real Sylvester matrix equation:
*
* op(A)*X + X*op(B) = scale*C or
* op(A)*X - X*op(B) = scale*C,
*
* where op(A) = A or A**T, and A and B are both upper quasi-
* triangular. A is M-by-M and B is N-by-N; the right hand side C and
* the solution X are M-by-N; and scale is an output scale factor, set
* <= 1 to avoid overflow in X.
*
* A and B must be in Schur canonical form (as returned by SHSEQR), that
* is, block upper triangular with 1-by-1 and 2-by-2 diagonal blocks;
* each 2-by-2 diagonal block has its diagonal elements equal and its
* off-diagonal elements of opposite sign.
*
* Arguments
* =========
*
* TRANA (input) CHARACTER*1
* Specifies the option op(A):
* = 'N': op(A) = A (No transpose)
* = 'T': op(A) = A**T (Transpose)
* = 'C': op(A) = A**H (Conjugate transpose = Transpose)
*
* TRANB (input) CHARACTER*1
* Specifies the option op(B):
* = 'N': op(B) = B (No transpose)
* = 'T': op(B) = B**T (Transpose)
* = 'C': op(B) = B**H (Conjugate transpose = Transpose)
*
* ISGN (input) INTEGER
* Specifies the sign in the equation:
* = +1: solve op(A)*X + X*op(B) = scale*C
* = -1: solve op(A)*X - X*op(B) = scale*C
*
* M (input) INTEGER
* The order of the matrix A, and the number of rows in the
* matrices X and C. M >= 0.
*
* N (input) INTEGER
* The order of the matrix B, and the number of columns in the
* matrices X and C. N >= 0.
*
* A (input) REAL array, dimension (LDA,M)
* The upper quasi-triangular matrix A, in Schur canonical form.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* B (input) REAL array, dimension (LDB,N)
* The upper quasi-triangular matrix B, in Schur canonical form.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* C (input/output) REAL array, dimension (LDC,N)
* On entry, the M-by-N right hand side matrix C.
* On exit, C is overwritten by the solution matrix X.
*
* LDC (input) INTEGER
* The leading dimension of the array C. LDC >= max(1,M)
*
* SCALE (output) REAL
* The scale factor, scale, set <= 1 to avoid overflow in X.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* = 1: A and B have common or very close eigenvalues; perturbed
* values were used to solve the equation (but the matrices
* A and B are unchanged).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param trana
* @param tranb
* @param isgn
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param c
* @param _c_offset
* @param Ldc
* @param scale
* @param info
*
*/
abstract public void strsyl(java.lang.String trana, java.lang.String tranb, int isgn, int m, int n, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, float[] c, int _c_offset, int Ldc, org.netlib.util.floatW scale, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STRTI2 computes the inverse of a real upper or lower triangular
* matrix.
*
* This is the Level 2 BLAS version of the algorithm.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the matrix A is upper or lower triangular.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* DIAG (input) CHARACTER*1
* Specifies whether or not the matrix A is unit triangular.
* = 'N': Non-unit triangular
* = 'U': Unit triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the triangular matrix A. If UPLO = 'U', the
* leading n by n upper triangular part of the array A contains
* the upper triangular matrix, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading n by n lower triangular part of the array A contains
* the lower triangular matrix, and the strictly upper
* triangular part of A is not referenced. If DIAG = 'U', the
* diagonal elements of A are also not referenced and are
* assumed to be 1.
*
* On exit, the (triangular) inverse of the original matrix, in
* the same storage format.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param diag
* @param n
* @param a
* @param lda
* @param info
*
*/
abstract public void strti2(java.lang.String uplo, java.lang.String diag, int n, float[] a, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STRTI2 computes the inverse of a real upper or lower triangular
* matrix.
*
* This is the Level 2 BLAS version of the algorithm.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* Specifies whether the matrix A is upper or lower triangular.
* = 'U': Upper triangular
* = 'L': Lower triangular
*
* DIAG (input) CHARACTER*1
* Specifies whether or not the matrix A is unit triangular.
* = 'N': Non-unit triangular
* = 'U': Unit triangular
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the triangular matrix A. If UPLO = 'U', the
* leading n by n upper triangular part of the array A contains
* the upper triangular matrix, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading n by n lower triangular part of the array A contains
* the lower triangular matrix, and the strictly upper
* triangular part of A is not referenced. If DIAG = 'U', the
* diagonal elements of A are also not referenced and are
* assumed to be 1.
*
* On exit, the (triangular) inverse of the original matrix, in
* the same storage format.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -k, the k-th argument had an illegal value
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param diag
* @param n
* @param a
* @param _a_offset
* @param lda
* @param info
*
*/
abstract public void strti2(java.lang.String uplo, java.lang.String diag, int n, float[] a, int _a_offset, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STRTRI computes the inverse of a real upper or lower triangular
* matrix A.
*
* This is the Level 3 BLAS version of the algorithm.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the triangular matrix A. If UPLO = 'U', the
* leading N-by-N upper triangular part of the array A contains
* the upper triangular matrix, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading N-by-N lower triangular part of the array A contains
* the lower triangular matrix, and the strictly upper
* triangular part of A is not referenced. If DIAG = 'U', the
* diagonal elements of A are also not referenced and are
* assumed to be 1.
* On exit, the (triangular) inverse of the original matrix, in
* the same storage format.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, A(i,i) is exactly zero. The triangular
* matrix is singular and its inverse can not be computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param diag
* @param n
* @param a
* @param lda
* @param info
*
*/
abstract public void strtri(java.lang.String uplo, java.lang.String diag, int n, float[] a, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STRTRI computes the inverse of a real upper or lower triangular
* matrix A.
*
* This is the Level 3 BLAS version of the algorithm.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the triangular matrix A. If UPLO = 'U', the
* leading N-by-N upper triangular part of the array A contains
* the upper triangular matrix, and the strictly lower
* triangular part of A is not referenced. If UPLO = 'L', the
* leading N-by-N lower triangular part of the array A contains
* the lower triangular matrix, and the strictly upper
* triangular part of A is not referenced. If DIAG = 'U', the
* diagonal elements of A are also not referenced and are
* assumed to be 1.
* On exit, the (triangular) inverse of the original matrix, in
* the same storage format.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, A(i,i) is exactly zero. The triangular
* matrix is singular and its inverse can not be computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param diag
* @param n
* @param a
* @param _a_offset
* @param lda
* @param info
*
*/
abstract public void strtri(java.lang.String uplo, java.lang.String diag, int n, float[] a, int _a_offset, int lda, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STRTRS solves a triangular system of the form
*
* A * X = B or A**T * X = B,
*
* where A is a triangular matrix of order N, and B is an N-by-NRHS
* matrix. A check is made to verify that A is nonsingular.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The triangular matrix A. If UPLO = 'U', the leading N-by-N
* upper triangular part of the array A contains the upper
* triangular matrix, and the strictly lower triangular part of
* A is not referenced. If UPLO = 'L', the leading N-by-N lower
* triangular part of the array A contains the lower triangular
* matrix, and the strictly upper triangular part of A is not
* referenced. If DIAG = 'U', the diagonal elements of A are
* also not referenced and are assumed to be 1.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, if INFO = 0, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the i-th diagonal element of A is zero,
* indicating that the matrix is singular and the solutions
* X have not been computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param n
* @param nrhs
* @param a
* @param lda
* @param b
* @param ldb
* @param info
*
*/
abstract public void strtrs(java.lang.String uplo, java.lang.String trans, java.lang.String diag, int n, int nrhs, float[] a, int lda, float[] b, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STRTRS solves a triangular system of the form
*
* A * X = B or A**T * X = B,
*
* where A is a triangular matrix of order N, and B is an N-by-NRHS
* matrix. A check is made to verify that A is nonsingular.
*
* Arguments
* =========
*
* UPLO (input) CHARACTER*1
* = 'U': A is upper triangular;
* = 'L': A is lower triangular.
*
* TRANS (input) CHARACTER*1
* Specifies the form of the system of equations:
* = 'N': A * X = B (No transpose)
* = 'T': A**T * X = B (Transpose)
* = 'C': A**H * X = B (Conjugate transpose = Transpose)
*
* DIAG (input) CHARACTER*1
* = 'N': A is non-unit triangular;
* = 'U': A is unit triangular.
*
* N (input) INTEGER
* The order of the matrix A. N >= 0.
*
* NRHS (input) INTEGER
* The number of right hand sides, i.e., the number of columns
* of the matrix B. NRHS >= 0.
*
* A (input) REAL array, dimension (LDA,N)
* The triangular matrix A. If UPLO = 'U', the leading N-by-N
* upper triangular part of the array A contains the upper
* triangular matrix, and the strictly lower triangular part of
* A is not referenced. If UPLO = 'L', the leading N-by-N lower
* triangular part of the array A contains the lower triangular
* matrix, and the strictly upper triangular part of A is not
* referenced. If DIAG = 'U', the diagonal elements of A are
* also not referenced and are assumed to be 1.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,N).
*
* B (input/output) REAL array, dimension (LDB,NRHS)
* On entry, the right hand side matrix B.
* On exit, if INFO = 0, the solution matrix X.
*
* LDB (input) INTEGER
* The leading dimension of the array B. LDB >= max(1,N).
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
* > 0: if INFO = i, the i-th diagonal element of A is zero,
* indicating that the matrix is singular and the solutions
* X have not been computed.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param uplo
* @param trans
* @param diag
* @param n
* @param nrhs
* @param a
* @param _a_offset
* @param lda
* @param b
* @param _b_offset
* @param ldb
* @param info
*
*/
abstract public void strtrs(java.lang.String uplo, java.lang.String trans, java.lang.String diag, int n, int nrhs, float[] a, int _a_offset, int lda, float[] b, int _b_offset, int ldb, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* This routine is deprecated and has been replaced by routine STZRZF.
*
* STZRQF reduces the M-by-N ( M<=N ) real upper trapezoidal matrix A
* to upper triangular form by means of orthogonal transformations.
*
* The upper trapezoidal matrix A is factored as
*
* A = ( R 0 ) * Z,
*
* where Z is an N-by-N orthogonal matrix and R is an M-by-M upper
* triangular matrix.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= M.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the leading M-by-N upper trapezoidal part of the
* array A must contain the matrix to be factorized.
* On exit, the leading M-by-M upper triangular part of A
* contains the upper triangular matrix R, and elements M+1 to
* N of the first M rows of A, with the array TAU, represent the
* orthogonal matrix Z as a product of M elementary reflectors.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) REAL array, dimension (M)
* The scalar factors of the elementary reflectors.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The factorization is obtained by Householder's method. The kth
* transformation matrix, Z( k ), which is used to introduce zeros into
* the ( m - k + 1 )th row of A, is given in the form
*
* Z( k ) = ( I 0 ),
* ( 0 T( k ) )
*
* where
*
* T( k ) = I - tau*u( k )*u( k )', u( k ) = ( 1 ),
* ( 0 )
* ( z( k ) )
*
* tau is a scalar and z( k ) is an ( n - m ) element vector.
* tau and z( k ) are chosen to annihilate the elements of the kth row
* of X.
*
* The scalar tau is returned in the kth element of TAU and the vector
* u( k ) in the kth row of A, such that the elements of z( k ) are
* in a( k, m + 1 ), ..., a( k, n ). The elements of R are returned in
* the upper triangular part of A.
*
* Z is given by
*
* Z = Z( 1 ) * Z( 2 ) * ... * Z( m ).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param tau
* @param info
*
*/
abstract public void stzrqf(int m, int n, float[] a, int lda, float[] tau, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* This routine is deprecated and has been replaced by routine STZRZF.
*
* STZRQF reduces the M-by-N ( M<=N ) real upper trapezoidal matrix A
* to upper triangular form by means of orthogonal transformations.
*
* The upper trapezoidal matrix A is factored as
*
* A = ( R 0 ) * Z,
*
* where Z is an N-by-N orthogonal matrix and R is an M-by-M upper
* triangular matrix.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= M.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the leading M-by-N upper trapezoidal part of the
* array A must contain the matrix to be factorized.
* On exit, the leading M-by-M upper triangular part of A
* contains the upper triangular matrix R, and elements M+1 to
* N of the first M rows of A, with the array TAU, represent the
* orthogonal matrix Z as a product of M elementary reflectors.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) REAL array, dimension (M)
* The scalar factors of the elementary reflectors.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* The factorization is obtained by Householder's method. The kth
* transformation matrix, Z( k ), which is used to introduce zeros into
* the ( m - k + 1 )th row of A, is given in the form
*
* Z( k ) = ( I 0 ),
* ( 0 T( k ) )
*
* where
*
* T( k ) = I - tau*u( k )*u( k )', u( k ) = ( 1 ),
* ( 0 )
* ( z( k ) )
*
* tau is a scalar and z( k ) is an ( n - m ) element vector.
* tau and z( k ) are chosen to annihilate the elements of the kth row
* of X.
*
* The scalar tau is returned in the kth element of TAU and the vector
* u( k ) in the kth row of A, such that the elements of z( k ) are
* in a( k, m + 1 ), ..., a( k, n ). The elements of R are returned in
* the upper triangular part of A.
*
* Z is given by
*
* Z = Z( 1 ) * Z( 2 ) * ... * Z( m ).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param info
*
*/
abstract public void stzrqf(int m, int n, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STZRZF reduces the M-by-N ( M<=N ) real upper trapezoidal matrix A
* to upper triangular form by means of orthogonal transformations.
*
* The upper trapezoidal matrix A is factored as
*
* A = ( R 0 ) * Z,
*
* where Z is an N-by-N orthogonal matrix and R is an M-by-M upper
* triangular matrix.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= M.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the leading M-by-N upper trapezoidal part of the
* array A must contain the matrix to be factorized.
* On exit, the leading M-by-M upper triangular part of A
* contains the upper triangular matrix R, and elements M+1 to
* N of the first M rows of A, with the array TAU, represent the
* orthogonal matrix Z as a product of M elementary reflectors.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) REAL array, dimension (M)
* The scalar factors of the elementary reflectors.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,M).
* For optimum performance LWORK >= M*NB, where NB is
* the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* Based on contributions by
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
*
* The factorization is obtained by Householder's method. The kth
* transformation matrix, Z( k ), which is used to introduce zeros into
* the ( m - k + 1 )th row of A, is given in the form
*
* Z( k ) = ( I 0 ),
* ( 0 T( k ) )
*
* where
*
* T( k ) = I - tau*u( k )*u( k )', u( k ) = ( 1 ),
* ( 0 )
* ( z( k ) )
*
* tau is a scalar and z( k ) is an ( n - m ) element vector.
* tau and z( k ) are chosen to annihilate the elements of the kth row
* of X.
*
* The scalar tau is returned in the kth element of TAU and the vector
* u( k ) in the kth row of A, such that the elements of z( k ) are
* in a( k, m + 1 ), ..., a( k, n ). The elements of R are returned in
* the upper triangular part of A.
*
* Z is given by
*
* Z = Z( 1 ) * Z( 2 ) * ... * Z( m ).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param lda
* @param tau
* @param work
* @param lwork
* @param info
*
*/
abstract public void stzrzf(int m, int n, float[] a, int lda, float[] tau, float[] work, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* STZRZF reduces the M-by-N ( M<=N ) real upper trapezoidal matrix A
* to upper triangular form by means of orthogonal transformations.
*
* The upper trapezoidal matrix A is factored as
*
* A = ( R 0 ) * Z,
*
* where Z is an N-by-N orthogonal matrix and R is an M-by-M upper
* triangular matrix.
*
* Arguments
* =========
*
* M (input) INTEGER
* The number of rows of the matrix A. M >= 0.
*
* N (input) INTEGER
* The number of columns of the matrix A. N >= M.
*
* A (input/output) REAL array, dimension (LDA,N)
* On entry, the leading M-by-N upper trapezoidal part of the
* array A must contain the matrix to be factorized.
* On exit, the leading M-by-M upper triangular part of A
* contains the upper triangular matrix R, and elements M+1 to
* N of the first M rows of A, with the array TAU, represent the
* orthogonal matrix Z as a product of M elementary reflectors.
*
* LDA (input) INTEGER
* The leading dimension of the array A. LDA >= max(1,M).
*
* TAU (output) REAL array, dimension (M)
* The scalar factors of the elementary reflectors.
*
* WORK (workspace/output) REAL array, dimension (MAX(1,LWORK))
* On exit, if INFO = 0, WORK(1) returns the optimal LWORK.
*
* LWORK (input) INTEGER
* The dimension of the array WORK. LWORK >= max(1,M).
* For optimum performance LWORK >= M*NB, where NB is
* the optimal blocksize.
*
* If LWORK = -1, then a workspace query is assumed; the routine
* only calculates the optimal size of the WORK array, returns
* this value as the first entry of the WORK array, and no error
* message related to LWORK is issued by XERBLA.
*
* INFO (output) INTEGER
* = 0: successful exit
* < 0: if INFO = -i, the i-th argument had an illegal value
*
* Further Details
* ===============
*
* Based on contributions by
* A. Petitet, Computer Science Dept., Univ. of Tenn., Knoxville, USA
*
* The factorization is obtained by Householder's method. The kth
* transformation matrix, Z( k ), which is used to introduce zeros into
* the ( m - k + 1 )th row of A, is given in the form
*
* Z( k ) = ( I 0 ),
* ( 0 T( k ) )
*
* where
*
* T( k ) = I - tau*u( k )*u( k )', u( k ) = ( 1 ),
* ( 0 )
* ( z( k ) )
*
* tau is a scalar and z( k ) is an ( n - m ) element vector.
* tau and z( k ) are chosen to annihilate the elements of the kth row
* of X.
*
* The scalar tau is returned in the kth element of TAU and the vector
* u( k ) in the kth row of A, such that the elements of z( k ) are
* in a( k, m + 1 ), ..., a( k, n ). The elements of R are returned in
* the upper triangular part of A.
*
* Z is given by
*
* Z = Z( 1 ) * Z( 2 ) * ... * Z( m ).
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param m
* @param n
* @param a
* @param _a_offset
* @param lda
* @param tau
* @param _tau_offset
* @param work
* @param _work_offset
* @param lwork
* @param info
*
*/
abstract public void stzrzf(int m, int n, float[] a, int _a_offset, int lda, float[] tau, int _tau_offset, float[] work, int _work_offset, int lwork, org.netlib.util.intW info);
/**
*
* ..
*
* Purpose
* =======
*
* DLAMCH determines double precision machine parameters.
*
* Arguments
* =========
*
* CMACH (input) CHARACTER*1
* Specifies the value to be returned by DLAMCH:
* = 'E' or 'e', DLAMCH := eps
* = 'S' or 's , DLAMCH := sfmin
* = 'B' or 'b', DLAMCH := base
* = 'P' or 'p', DLAMCH := eps*base
* = 'N' or 'n', DLAMCH := t
* = 'R' or 'r', DLAMCH := rnd
* = 'M' or 'm', DLAMCH := emin
* = 'U' or 'u', DLAMCH := rmin
* = 'L' or 'l', DLAMCH := emax
* = 'O' or 'o', DLAMCH := rmax
*
* where
*
* eps = relative machine precision
* sfmin = safe minimum, such that 1/sfmin does not overflow
* base = base of the machine
* prec = eps*base
* t = number of (base) digits in the mantissa
* rnd = 1.0 when rounding occurs in addition, 0.0 otherwise
* emin = minimum exponent before (gradual) underflow
* rmin = underflow threshold - base**(emin-1)
* emax = largest exponent before overflow
* rmax = overflow threshold - (base**emax)*(1-eps)
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param cmach
* @return
*/
abstract public double dlamch(java.lang.String cmach);
/**
*
* ..
*
* Purpose
* =======
*
* DLAMC1 determines the machine parameters given by BETA, T, RND, and
* IEEE1.
*
* Arguments
* =========
*
* BETA (output) INTEGER
* The base of the machine.
*
* T (output) INTEGER
* The number of ( BETA ) digits in the mantissa.
*
* RND (output) LOGICAL
* Specifies whether proper rounding ( RND = .TRUE. ) or
* chopping ( RND = .FALSE. ) occurs in addition. This may not
* be a reliable guide to the way in which the machine performs
* its arithmetic.
*
* IEEE1 (output) LOGICAL
* Specifies whether rounding appears to be done in the IEEE
* 'round to nearest' style.
*
* Further Details
* ===============
*
* The routine is based on the routine ENVRON by Malcolm and
* incorporates suggestions by Gentleman and Marovich. See
*
* Malcolm M. A. (1972) Algorithms to reveal properties of
* floating-point arithmetic. Comms. of the ACM, 15, 949-951.
*
* Gentleman W. M. and Marovich S. B. (1974) More on algorithms
* that reveal properties of floating point arithmetic units.
* Comms. of the ACM, 17, 276-277.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param beta
* @param t
* @param rnd
* @param ieee1
*
*/
abstract public void dlamc1(org.netlib.util.intW beta, org.netlib.util.intW t, org.netlib.util.booleanW rnd, org.netlib.util.booleanW ieee1);
/**
*
* ..
*
* Purpose
* =======
*
* DLAMC2 determines the machine parameters specified in its argument
* list.
*
* Arguments
* =========
*
* BETA (output) INTEGER
* The base of the machine.
*
* T (output) INTEGER
* The number of ( BETA ) digits in the mantissa.
*
* RND (output) LOGICAL
* Specifies whether proper rounding ( RND = .TRUE. ) or
* chopping ( RND = .FALSE. ) occurs in addition. This may not
* be a reliable guide to the way in which the machine performs
* its arithmetic.
*
* EPS (output) DOUBLE PRECISION
* The smallest positive number such that
*
* fl( 1.0 - EPS ) .LT. 1.0,
*
* where fl denotes the computed value.
*
* EMIN (output) INTEGER
* The minimum exponent before (gradual) underflow occurs.
*
* RMIN (output) DOUBLE PRECISION
* The smallest normalized number for the machine, given by
* BASE**( EMIN - 1 ), where BASE is the floating point value
* of BETA.
*
* EMAX (output) INTEGER
* The maximum exponent before overflow occurs.
*
* RMAX (output) DOUBLE PRECISION
* The largest positive number for the machine, given by
* BASE**EMAX * ( 1 - EPS ), where BASE is the floating point
* value of BETA.
*
* Further Details
* ===============
*
* The computation of EPS is based on a routine PARANOIA by
* W. Kahan of the University of California at Berkeley.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param beta
* @param t
* @param rnd
* @param eps
* @param emin
* @param rmin
* @param emax
* @param rmax
*
*/
abstract public void dlamc2(org.netlib.util.intW beta, org.netlib.util.intW t, org.netlib.util.booleanW rnd, org.netlib.util.doubleW eps, org.netlib.util.intW emin, org.netlib.util.doubleW rmin, org.netlib.util.intW emax, org.netlib.util.doubleW rmax);
/**
*
* ..
*
* Purpose
* =======
*
* DLAMC3 is intended to force A and B to be stored prior to doing
* the addition of A and B , for use in situations where optimizers
* might hold one of these in a register.
*
* Arguments
* =========
*
* A (input) DOUBLE PRECISION
* B (input) DOUBLE PRECISION
* The values A and B.
*
* =====================================================================
*
* .. Executable Statements ..
*
*
*
* @param a
* @param b
* @return
*/
abstract public double dlamc3(double a, double b);
/**
*
* ..
*
* Purpose
* =======
*
* DLAMC4 is a service routine for DLAMC2.
*
* Arguments
* =========
*
* EMIN (output) INTEGER
* The minimum exponent before (gradual) underflow, computed by
* setting A = START and dividing by BASE until the previous A
* can not be recovered.
*
* START (input) DOUBLE PRECISION
* The starting point for determining EMIN.
*
* BASE (input) INTEGER
* The base of the machine.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param emin
* @param start
* @param base
*
*/
abstract public void dlamc4(org.netlib.util.intW emin, double start, int base);
/**
*
* ..
*
* Purpose
* =======
*
* DLAMC5 attempts to compute RMAX, the largest machine floating-point
* number, without overflow. It assumes that EMAX + abs(EMIN) sum
* approximately to a power of 2. It will fail on machines where this
* assumption does not hold, for example, the Cyber 205 (EMIN = -28625,
* EMAX = 28718). It will also fail if the value supplied for EMIN is
* too large (i.e. too close to zero), probably with overflow.
*
* Arguments
* =========
*
* BETA (input) INTEGER
* The base of floating-point arithmetic.
*
* P (input) INTEGER
* The number of base BETA digits in the mantissa of a
* floating-point value.
*
* EMIN (input) INTEGER
* The minimum exponent before (gradual) underflow.
*
* IEEE (input) LOGICAL
* A logical flag specifying whether or not the arithmetic
* system is thought to comply with the IEEE standard.
*
* EMAX (output) INTEGER
* The largest exponent before overflow
*
* RMAX (output) DOUBLE PRECISION
* The largest machine floating-point number.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param beta
* @param p
* @param emin
* @param ieee
* @param emax
* @param rmax
*
*/
abstract public void dlamc5(int beta, int p, int emin, boolean ieee, org.netlib.util.intW emax, org.netlib.util.doubleW rmax);
/**
*
*
* -- LAPACK auxiliary routine (version 3.1.1) --
* Univ. of Tennessee, Univ. of California Berkeley and NAG Ltd..
* February 2007
*
* Purpose
* =======
*
* DSECND returns the user time for a process in seconds.
* This version gets the time from the EXTERNAL system function ETIME.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @return
*/
abstract public double dsecnd();
/**
*
* ..
*
* Purpose
* =======
*
* LSAME returns .TRUE. if CA is the same letter as CB regardless of
* case.
*
* Arguments
* =========
*
* CA (input) CHARACTER*1
* CB (input) CHARACTER*1
* CA and CB specify the single characters to be compared.
*
* =====================================================================
*
* .. Intrinsic Functions ..
*
*
* @param ca
* @param cb
* @return
*/
abstract public boolean lsame(java.lang.String ca, java.lang.String cb);
/**
*
*
* -- LAPACK auxiliary routine (version 3.1.1) --
* Univ. of Tennessee, Univ. of California Berkeley and NAG Ltd..
* February 2007
*
* Purpose
* =======
*
* SECOND returns the user time for a process in seconds.
* This version gets the time from the EXTERNAL system function ETIME.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @return
*/
abstract public float second();
/**
*
* ..
*
* Purpose
* =======
*
* SLAMCH determines single precision machine parameters.
*
* Arguments
* =========
*
* CMACH (input) CHARACTER*1
* Specifies the value to be returned by SLAMCH:
* = 'E' or 'e', SLAMCH := eps
* = 'S' or 's , SLAMCH := sfmin
* = 'B' or 'b', SLAMCH := base
* = 'P' or 'p', SLAMCH := eps*base
* = 'N' or 'n', SLAMCH := t
* = 'R' or 'r', SLAMCH := rnd
* = 'M' or 'm', SLAMCH := emin
* = 'U' or 'u', SLAMCH := rmin
* = 'L' or 'l', SLAMCH := emax
* = 'O' or 'o', SLAMCH := rmax
*
* where
*
* eps = relative machine precision
* sfmin = safe minimum, such that 1/sfmin does not overflow
* base = base of the machine
* prec = eps*base
* t = number of (base) digits in the mantissa
* rnd = 1.0 when rounding occurs in addition, 0.0 otherwise
* emin = minimum exponent before (gradual) underflow
* rmin = underflow threshold - base**(emin-1)
* emax = largest exponent before overflow
* rmax = overflow threshold - (base**emax)*(1-eps)
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param cmach
* @return
*/
abstract public float slamch(java.lang.String cmach);
/**
*
* ..
*
* Purpose
* =======
*
* SLAMC1 determines the machine parameters given by BETA, T, RND, and
* IEEE1.
*
* Arguments
* =========
*
* BETA (output) INTEGER
* The base of the machine.
*
* T (output) INTEGER
* The number of ( BETA ) digits in the mantissa.
*
* RND (output) LOGICAL
* Specifies whether proper rounding ( RND = .TRUE. ) or
* chopping ( RND = .FALSE. ) occurs in addition. This may not
* be a reliable guide to the way in which the machine performs
* its arithmetic.
*
* IEEE1 (output) LOGICAL
* Specifies whether rounding appears to be done in the IEEE
* 'round to nearest' style.
*
* Further Details
* ===============
*
* The routine is based on the routine ENVRON by Malcolm and
* incorporates suggestions by Gentleman and Marovich. See
*
* Malcolm M. A. (1972) Algorithms to reveal properties of
* floating-point arithmetic. Comms. of the ACM, 15, 949-951.
*
* Gentleman W. M. and Marovich S. B. (1974) More on algorithms
* that reveal properties of floating point arithmetic units.
* Comms. of the ACM, 17, 276-277.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param beta
* @param t
* @param rnd
* @param ieee1
*
*/
abstract public void slamc1(org.netlib.util.intW beta, org.netlib.util.intW t, org.netlib.util.booleanW rnd, org.netlib.util.booleanW ieee1);
/**
*
* ..
*
* Purpose
* =======
*
* SLAMC2 determines the machine parameters specified in its argument
* list.
*
* Arguments
* =========
*
* BETA (output) INTEGER
* The base of the machine.
*
* T (output) INTEGER
* The number of ( BETA ) digits in the mantissa.
*
* RND (output) LOGICAL
* Specifies whether proper rounding ( RND = .TRUE. ) or
* chopping ( RND = .FALSE. ) occurs in addition. This may not
* be a reliable guide to the way in which the machine performs
* its arithmetic.
*
* EPS (output) REAL
* The smallest positive number such that
*
* fl( 1.0 - EPS ) .LT. 1.0,
*
* where fl denotes the computed value.
*
* EMIN (output) INTEGER
* The minimum exponent before (gradual) underflow occurs.
*
* RMIN (output) REAL
* The smallest normalized number for the machine, given by
* BASE**( EMIN - 1 ), where BASE is the floating point value
* of BETA.
*
* EMAX (output) INTEGER
* The maximum exponent before overflow occurs.
*
* RMAX (output) REAL
* The largest positive number for the machine, given by
* BASE**EMAX * ( 1 - EPS ), where BASE is the floating point
* value of BETA.
*
* Further Details
* ===============
*
* The computation of EPS is based on a routine PARANOIA by
* W. Kahan of the University of California at Berkeley.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param beta
* @param t
* @param rnd
* @param eps
* @param emin
* @param rmin
* @param emax
* @param rmax
*
*/
abstract public void slamc2(org.netlib.util.intW beta, org.netlib.util.intW t, org.netlib.util.booleanW rnd, org.netlib.util.floatW eps, org.netlib.util.intW emin, org.netlib.util.floatW rmin, org.netlib.util.intW emax, org.netlib.util.floatW rmax);
/**
*
* ..
*
* Purpose
* =======
*
* SLAMC3 is intended to force A and B to be stored prior to doing
* the addition of A and B , for use in situations where optimizers
* might hold one of these in a register.
*
* Arguments
* =========
*
* A (input) REAL
* B (input) REAL
* The values A and B.
*
* =====================================================================
*
* .. Executable Statements ..
*
*
*
* @param a
* @param b
* @return
*/
abstract public float slamc3(float a, float b);
/**
*
* ..
*
* Purpose
* =======
*
* SLAMC4 is a service routine for SLAMC2.
*
* Arguments
* =========
*
* EMIN (output) INTEGER
* The minimum exponent before (gradual) underflow, computed by
* setting A = START and dividing by BASE until the previous A
* can not be recovered.
*
* START (input) REAL
* The starting point for determining EMIN.
*
* BASE (input) INTEGER
* The base of the machine.
*
* =====================================================================
*
* .. Local Scalars ..
*
*
* @param emin
* @param start
* @param base
*
*/
abstract public void slamc4(org.netlib.util.intW emin, float start, int base);
/**
*
* ..
*
* Purpose
* =======
*
* SLAMC5 attempts to compute RMAX, the largest machine floating-point
* number, without overflow. It assumes that EMAX + abs(EMIN) sum
* approximately to a power of 2. It will fail on machines where this
* assumption does not hold, for example, the Cyber 205 (EMIN = -28625,
* EMAX = 28718). It will also fail if the value supplied for EMIN is
* too large (i.e. too close to zero), probably with overflow.
*
* Arguments
* =========
*
* BETA (input) INTEGER
* The base of floating-point arithmetic.
*
* P (input) INTEGER
* The number of base BETA digits in the mantissa of a
* floating-point value.
*
* EMIN (input) INTEGER
* The minimum exponent before (gradual) underflow.
*
* IEEE (input) LOGICAL
* A logical flag specifying whether or not the arithmetic
* system is thought to comply with the IEEE standard.
*
* EMAX (output) INTEGER
* The largest exponent before overflow
*
* RMAX (output) REAL
* The largest machine floating-point number.
*
* =====================================================================
*
* .. Parameters ..
*
*
* @param beta
* @param p
* @param emin
* @param ieee
* @param emax
* @param rmax
*
*/
abstract public void slamc5(int beta, int p, int emin, boolean ieee, org.netlib.util.intW emax, org.netlib.util.floatW rmax);
}