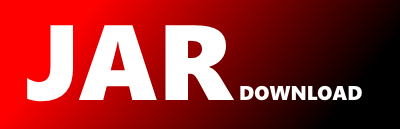
weka.classifiers.immune.airs.AIRS2 Maven / Gradle / Ivy
Show all versions of wekaclassalgos Show documentation
/*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
/*
* Created on 30/12/2004
*
*/
package weka.classifiers.immune.airs;
import weka.classifiers.AbstractClassifier;
import weka.classifiers.immune.airs.algorithm.AIRS2Trainer;
import weka.classifiers.immune.airs.algorithm.AISModelClassifier;
import weka.core.Capabilities;
import weka.core.Capabilities.Capability;
import weka.core.Instance;
import weka.core.Instances;
import weka.core.Option;
import weka.core.Utils;
import java.util.Enumeration;
import java.util.LinkedList;
import java.util.Random;
import java.util.Vector;
/**
* Type: AIRS1
* File: AIRS1.java
* Date: 07/01/2005
*
* Description:
*
* @author Jason Brownlee
*/
public class AIRS2 extends AbstractClassifier
implements AIRSParameterDocumentation {
// paramters
protected long seed;
protected double affinityThresholdScalar;
protected double clonalRate;
protected double hypermutationRate;
protected double totalResources;
protected double stimulationValue;
protected int numInstancesAffinityThreshold;
protected int memInitialPoolSize;
protected int knn;
protected String trainingSummary;
protected String classifierSummary;
private final static String[] PARAMETERS =
{
"S", // seed
"F", // affinity threshold
"C", // clonal rate
"H", // hypermutation
"R", // total resources
"V", // stimulation value
"A", // num affinity threshold instances
"E", // mem pool size
"K" // kNN
};
private final static String[] DESCRIPTIONS =
{
PARAM_SEED,
PARAM_ATS,
PARAM_CLONAL_RATE,
PARAM_HMR,
PARAM_RESOURCES,
PARAM_STIMULATION,
PARAM_AT_INSTANCES,
PARAM_MEM_INSTANCES,
PARAM_KNN
};
/**
* The model
*/
protected AISModelClassifier classifier;
public AIRS2() {
// set default values
seed = 1;
affinityThresholdScalar = 0.2;
totalResources = 150;
stimulationValue = 0.9;
clonalRate = 10;
hypermutationRate = 2.0;
numInstancesAffinityThreshold = -1;
memInitialPoolSize = 1;
knn = 3;
}
/**
* Returns the Capabilities of this classifier.
*
* @return the capabilities of this object
* @see Capabilities
*/
@Override
public Capabilities getCapabilities() {
Capabilities result = super.getCapabilities();
result.disableAll();
// attributes
result.enable(Capability.NUMERIC_ATTRIBUTES);
result.enable(Capability.DATE_ATTRIBUTES);
result.enable(Capability.NOMINAL_ATTRIBUTES);
// class
result.enable(Capability.NOMINAL_CLASS);
result.enable(Capability.MISSING_CLASS_VALUES);
result.setMinimumNumberInstances(1);
return result;
}
/**
* @param data
* @throws java.lang.Exception
*/
public void buildClassifier(Instances data) throws Exception {
Instances trainingInstances = new Instances(data);
trainingInstances.deleteWithMissingClass();
getCapabilities().testWithFail(trainingInstances);
// validate paramters
validateParameters(trainingInstances);
// construct trainer
Random rand = new Random(seed);
AIRS2Trainer trainer = new AIRS2Trainer(
affinityThresholdScalar,
clonalRate,
hypermutationRate,
totalResources,
stimulationValue,
numInstancesAffinityThreshold,
rand,
memInitialPoolSize,
knn);
// prepare classifier
classifier = trainer.train(trainingInstances);
// get summaries
trainingSummary = trainer.getTrainingSummary();
classifierSummary = classifier.getModelSummary(trainingInstances);
}
protected void validateParameters(Instances trainingInstances)
throws Exception {
int numInstances = trainingInstances.numInstances();
if (memInitialPoolSize > numInstances) {
memInitialPoolSize = numInstances;
}
}
public double classifyInstance(Instance instance)
throws Exception {
if (classifier == null) {
throw new Exception("Algorithm has not been prepared.");
}
// TODO: validate of data provided matches training data specs
return classifier.classifyInstance(instance);
}
public String toString() {
StringBuffer buffer = new StringBuffer(1000);
buffer.append("AIRS2 - Artificial Immune Recognition System v2.0\n");
buffer.append("\n");
if (trainingSummary != null) {
buffer.append(trainingSummary);
buffer.append("\n");
}
if (classifierSummary != null) {
buffer.append(classifierSummary);
}
return buffer.toString();
}
public String globalInfo() {
StringBuffer buffer = new StringBuffer(1000);
buffer.append(toString());
buffer.append("A resource limited artifical immune system (AIS) ");
buffer.append("for supervised classification, using clonal selection, ");
buffer.append("affinity maturation and affinity recognition balls (ARBs).");
buffer.append("\n\n");
buffer.append("Andrew Watkins, Jon Timmis, and Lois Boggess, ");
buffer.append("Artificial Immune Recognition System (AIRS): An Immune-Inspired Supervised Learning Algorithm, ");
buffer.append("Genetic Programming and Evolvable Machines, ");
buffer.append("vol. 5, pp. 291-317, Sep, 2004.");
return buffer.toString();
}
public Enumeration listOptions() {
Vector