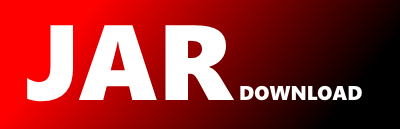
weka.classifiers.immune.airs.algorithm.CellPool Maven / Gradle / Ivy
Show all versions of wekaclassalgos Show documentation
/*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
/*
* Created on 30/12/2004
*
*/
package weka.classifiers.immune.airs.algorithm;
import weka.core.Instance;
import java.io.Serializable;
import java.util.Collections;
import java.util.Comparator;
import java.util.Iterator;
import java.util.LinkedList;
/**
* Type: AISModel
* File: AISModel.java
* Date: 30/12/2004
*
* Description:
*
* @author Jason Brownlee
*/
public class CellPool implements Serializable {
public final static AffinityComparator affinityComparator = new AffinityComparator();
public final static StimulationComparator stimulationComparator = new StimulationComparator();
public final static ResourceComparator resourceComparator = new ResourceComparator();
protected final LinkedList cells;
public CellPool(LinkedList aCells) {
cells = aCells;
}
public LinkedList affinityResponseNormalised(Instance aInstance, AffinityFunction affinity) {
// calculate affinity for all cells
double[] features = aInstance.toDoubleArray();
for (Cell c : cells) {
double aff = affinity.affinityNormalised(features, c);
c.setAffinity(aff);
}
// sort by affinity
Collections.sort(cells, affinityComparator);
// return sorted cells (most similar to least similar)
return cells;
}
public LinkedList affinityResponseUnnormalised(Instance aInstance, AffinityFunction affinity) {
// calculate affinity for all cells
double[] features = aInstance.toDoubleArray();
for (Cell c : cells) {
double aff = affinity.affinityUnnormalised(features, c);
c.setAffinity(aff);
}
// sort by affinity
Collections.sort(cells, affinityComparator);
// return sorted cells (most similar to least similar)
return cells;
}
public LinkedList resourceResponse() {
// sort by resources
Collections.sort(cells, resourceComparator);
return cells;
}
public static class AffinityComparator implements Comparator {
/**
* Compare cells based on affinity. The lower the value the
* higher the affinity.
*
* Orders cells in ascending order, meaning the highest affinity
* members are at the beginning of the array
*
* @param o1
* @param o2
* @return
*/
public int compare(Cell o1, Cell o2) {
if (o1.affinity < o2.affinity) {
return -1;
}
else if (o1.affinity > o2.affinity) {
return +1;
}
return 0;
}
}
public static class StimulationComparator implements Comparator {
/**
* Compare cells based on stimulation. The higher the value the
* higher the stimulation.
*
* Orders cells in decending order, meaning the highest stimulation
* members are at the beginning of the array
*
* @param o1
* @param o2
* @return
*/
public int compare(Cell o1, Cell o2) {
if (o1.stimulation > o2.stimulation) {
return -1;
}
else if (o1.stimulation < o2.stimulation) {
return +1;
}
return 0;
}
}
public static class ResourceComparator implements Comparator {
/**
* Compare cells based on numResources.
*
* Orders cells in decending order, meaning the most resources
* members are at the beginning of the array
*
* @param o1
* @param o2
* @return
*/
public int compare(Cell o1, Cell o2) {
if (o1.numResources > o2.numResources) {
return -1;
}
else if (o1.numResources < o2.numResources) {
return +1;
}
return 0;
}
}
public void add(LinkedList aNewList) {
cells.addAll(aNewList);
}
public void add(Cell aCell) {
cells.add(aCell);
}
public void delete(Cell aCell) {
cells.remove(aCell);
}
public boolean isEmpty() {
return cells.isEmpty();
}
public int size() {
return cells.size();
}
public LinkedList getCells() {
return cells;
}
public Iterator iterator() {
return cells.iterator();
}
public void orderByResources() {
Collections.sort(cells, resourceComparator);
}
public void orderByStimulation() {
Collections.sort(cells, stimulationComparator);
}
public void orderByAffinity() {
Collections.sort(cells, affinityComparator);
}
}
| | | | | | | | | | |