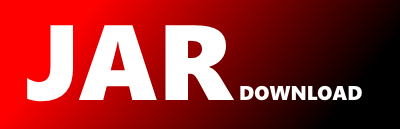
weka.classifiers.immune.clonalg.CLONALG Maven / Gradle / Ivy
Show all versions of wekaclassalgos Show documentation
/*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package weka.classifiers.immune.clonalg;
import weka.classifiers.AbstractClassifier;
import weka.classifiers.Evaluation;
import weka.core.Capabilities;
import weka.core.Capabilities.Capability;
import weka.core.Instance;
import weka.core.Instances;
import weka.core.Option;
import weka.core.UnsupportedClassTypeException;
import weka.core.Utils;
import java.util.Enumeration;
import java.util.LinkedList;
import java.util.Vector;
/**
* Type: CLONALG
* Date: 19/01/2005
*
*
* Description:
*
* @author Jason Brownlee
*/
public class CLONALG extends AbstractClassifier {
protected double clonalFactor; // beta
protected int antibodyPoolSize; // N
protected int selectionPoolSize; // n
protected int totalReplacement; // d
protected int numGenerations; // Ngen
protected long seed; // random number seed
protected double remainderPoolRatio; // typically 5%-8%
protected CLONALGAlgorithm algorithm;
private final static String[] PARAMETERS =
{
"B",
"N",
"n",
"D",
"G",
"S",
"R"
};
private final static String[] DESCRIPTIONS =
{
"Clonal factor (beta). Used to scale the number of clones created by the selected best antibodies.",
"Antibody pool size (N). The total antibodies maintained in the memory pool and remainder pool.",
"Selection pool size (n). The total number of best antibodies selected for cloning and mutation each iteration.",
"Total replacements (d). The total number of antibodies in the remainder pool that are replaced each iteration. Typically 5%-8%",
"Total generations. The total number of times that all antigens are exposed to the system.",
"Random number generator seed. Seed used to initialise the random number generator.",
"Remainder pool percentage. The percentage of the total antibody pool size allocated for the remainder pool."
};
public CLONALG() {
// set defaults
clonalFactor = 0.1; // beta
antibodyPoolSize = 30; // N
selectionPoolSize = 20; // n
totalReplacement = 0; // d
numGenerations = 10;
seed = 1;
remainderPoolRatio = 0.1;
}
/**
* Returns the Capabilities of this classifier.
*
* @return the capabilities of this object
* @see Capabilities
*/
@Override
public Capabilities getCapabilities() {
Capabilities result = super.getCapabilities();
result.disableAll();
// attributes
result.enable(Capability.NUMERIC_ATTRIBUTES);
result.enable(Capability.DATE_ATTRIBUTES);
result.enable(Capability.NOMINAL_ATTRIBUTES);
// class
result.enable(Capability.NOMINAL_CLASS);
result.enable(Capability.MISSING_CLASS_VALUES);
result.setMinimumNumberInstances(1);
return result;
}
public void buildClassifier(Instances data) throws Exception {
Instances trainingInstances = new Instances(data);
trainingInstances.deleteWithMissingClass();
getCapabilities().testWithFail(trainingInstances);
// construct trainer
algorithm = new CLONALGAlgorithm(
clonalFactor,
antibodyPoolSize,
selectionPoolSize,
totalReplacement,
numGenerations,
seed,
remainderPoolRatio);
// train
algorithm.train(trainingInstances);
}
public double classifyInstance(Instance instance) throws Exception {
if (algorithm == null) {
throw new Exception("Algorithm has not been prepared.");
}
return algorithm.classify(instance);
}
public String toString() {
StringBuffer buffer = new StringBuffer(1000);
buffer.append("CLONALG v1.0.\n");
return buffer.toString();
}
public String globalInfo() {
StringBuffer buffer = new StringBuffer(1000);
buffer.append(toString());
buffer.append("CLONALG - Clonal Selection Algorithm for classifiation.");
buffer.append("\n\n");
buffer.append(
"Leandro N. de Castro and Fernando J. Von Zuben. " +
"Learning and Optimization Using the Clonal Selection Principle. " +
"IEEE Transactions on Evolutionary Computation, Special Issue on Artificial Immune Systems. " +
"2002; " +
"6(3): " +
"239-251."
);
return buffer.toString();
}
public Enumeration listOptions() {
Vector