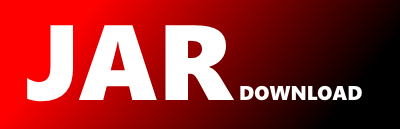
com.github.freegeese.maven.plugin.autocode.Helper Maven / Gradle / Ivy
package com.github.freegeese.maven.plugin.autocode;
import com.google.common.base.Utf8;
import javafx.scene.input.InputMethodTextRun;
import java.io.File;
import java.io.IOException;
import java.nio.charset.Charset;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* Created by Administrator on 2017/4/1.
*/
public abstract class Helper {
/**
* 判断是否是java.lang包下的Class
*
* @param className
* @return
*/
public static boolean isLangPackageClass(String className) {
return className.matches("java\\.lang\\.[A-Z]+[a-zA-Z]*");
}
/**
* 判断是否是java.lang包下的Class
*
* @param cls
* @return
*/
public static boolean isLangPackageClass(Class cls) {
return isLangPackageClass(cls.getName());
}
/**
* 过滤导入包,如果和当前类在同一个包下的import则不需要导入
*
* @param cls
* @param imports
*/
public static void filterImports(Class cls, Collection imports) {
filterImports(cls.getPackage().getName(), imports);
}
/**
* 过滤导入包,如果和当前类在同一个包下的import则不需要导入
*
* @param pkg
* @param imports
*/
public static void filterImports(String pkg, Collection imports) {
Iterator iterator = imports.iterator();
while (iterator.hasNext()) {
if (iterator.next().matches(pkg + "\\.[A-Z]+[a-zA-Z]*")) {
iterator.remove();
}
}
}
public static String findMarkContent(File file, String markStart, String markEnd) {
try {
String content = new String(Files.readAllBytes(Paths.get(file.getAbsolutePath())));
Matcher m = Pattern.compile("(\n|\r\n)[^\n]*" + markStart + ".*" + markEnd + "[^\n\r]*(\n|\r\n)", Pattern.MULTILINE | Pattern.DOTALL).matcher(content);
if (m.find()) {
return m.group().replaceFirst("^\\n+", "").replaceFirst("\\n+$", "");
}
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
/**
* 追加到文件末尾(最后一行上面)
*
* @param file
* @param content
*/
public static void appendToTail(File file, String content) {
try {
Path path = Paths.get(file.getAbsolutePath());
String fileContent = new String(Files.readAllBytes(path));
// 去除文件末尾的空行
fileContent = fileContent.replaceAll("(?s)(\n|\r\n)+$", "");
// 匹配最后一行内容
String regex = "\n.+$";
Matcher m = Pattern.compile(regex).matcher(fileContent);
String matched = "";
if (m.find()) {
matched = m.group();
}
fileContent = fileContent.replaceFirst(regex, content.concat(matched).replace("\\", "\\\\"));
Files.write(path, fileContent.getBytes());
} catch (IOException e) {
e.printStackTrace();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy