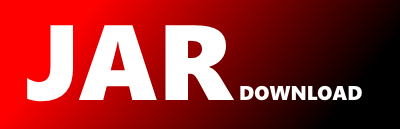
com.github.freegeese.maven.plugin.autocode.demo.base.BaseService Maven / Gradle / Ivy
package com.github.freegeese.maven.plugin.autocode.demo.base;
import com.github.pagehelper.ISelect;
import com.github.pagehelper.PageHelper;
import com.github.pagehelper.PageInfo;
import org.springframework.beans.factory.annotation.Autowired;
import java.util.List;
import java.util.Map;
public class BaseService, E extends BaseEntity, ID> {
@Autowired
M mapper;
public int insert(E entity) {
return mapper.insert(entity);
}
public int insertSelective(E entity) {
return mapper.insertSelective(entity);
}
public int deleteByPrimaryKey(ID id) {
return mapper.deleteByPrimaryKey(id);
}
public int deleteByPrimaryKeys(List ids) {
return mapper.deleteByPrimaryKeys(ids);
}
public int updateByPrimaryKey(E entity) {
return mapper.updateByPrimaryKey(entity);
}
public int updateByPrimaryKeySelective(E entity) {
return mapper.updateByPrimaryKeySelective(entity);
}
public E selectByPrimaryKey(ID id) {
return mapper.selectByPrimaryKey(id);
}
public List selectByPrimaryKeys(List ids) {
return mapper.selectByPrimaryKeys(ids);
}
public List selectAll() {
return mapper.selectAll();
}
public List selectByConditions(Map conditions) {
return mapper.selectByConditions(conditions);
}
public Pageable selectPage(Pageable page) {
PageInfo pageInfo = PageHelper.offsetPage(page.getOffset(), page.getPageSize()).doSelectPageInfo(new ISelect() {
@Override
public void doSelect() {
mapper.selectAll();
}
});
page.setTotalRecords(pageInfo.getTotal());
page.setContent(pageInfo.getList());
return page;
}
public Pageable selectPage(Pageable page, Map conditions) {
PageInfo pageInfo = PageHelper.offsetPage(page.getOffset(), page.getPageSize()).doSelectPageInfo(new ISelect() {
@Override
public void doSelect() {
mapper.selectByConditions(conditions);
}
});
page.setTotalRecords(pageInfo.getTotal());
page.setContent(pageInfo.getList());
return page;
}
public int batchInsert(List entities) {
return mapper.batchInsert(entities);
}
public int batchUpdate(List entities) {
return mapper.batchUpdate(entities);
}
public Long count() {
return mapper.count();
}
public M getMapper() {
return mapper;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy