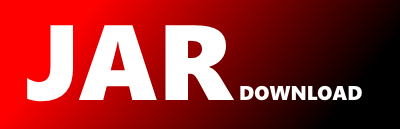
com.github.freegeese.maven.plugin.autocode.metadata.Column Maven / Gradle / Ivy
package com.github.freegeese.maven.plugin.autocode.metadata;
import com.github.freegeese.maven.plugin.autocode.DatabaseMetadataUtils;
/**
* Created by Administrator on 2017/3/29.
*/
public class Column {
private String tableCat;
private String tableSchem;
private String tableName;
private String columnName;
private String dataType;
private String typeName;
private String columnSize;
private String bufferLength;
private String decimalDigits;
private String numPrecRadix;
private boolean nullable;
private String remarks;
private String columnDef;
private String sqlDataType;
private String sqlDatetimeSub;
private String charOctetLength;
private String ordinalPosition;
private String isNullable;
private String scopeCatalog;
private String scopeSchema;
private String scopeTable;
private String sourceDataType;
private boolean autoincrement;
private boolean generatedcolumn;
// 自定义字段 -----------------------------
// 是否是主键列
private boolean primaryKey;
// 列对应的模型属性名
private String property;
// 列对应的java类型
private Class javaType;
// 列对应的jdbc类型
private String jdbcType;
public Column() {
}
public Column(String tableCat, String tableSchem, String tableName, String columnName, String dataType, String typeName, String columnSize, String bufferLength, String decimalDigits, String numPrecRadix, String nullable, String remarks, String columnDef, String sqlDataType, String sqlDatetimeSub, String charOctetLength, String ordinalPosition, String isNullable, String scopeCatalog, String scopeSchema, String scopeTable, String sourceDataType, String isAutoincrement, String isGeneratedcolumn) {
this.tableCat = tableCat;
this.tableSchem = (null == tableSchem) ? tableCat : tableSchem;
this.tableName = tableName;
this.columnName = columnName;
this.dataType = dataType;
this.typeName = typeName;
this.columnSize = columnSize;
this.bufferLength = bufferLength;
this.decimalDigits = decimalDigits;
this.numPrecRadix = numPrecRadix;
this.nullable = "1".equals(nullable);
this.remarks = remarks;
this.columnDef = columnDef;
this.sqlDataType = sqlDataType;
this.sqlDatetimeSub = sqlDatetimeSub;
this.charOctetLength = charOctetLength;
this.ordinalPosition = ordinalPosition;
this.isNullable = isNullable;
this.scopeCatalog = scopeCatalog;
this.scopeSchema = scopeSchema;
this.scopeTable = scopeTable;
this.sourceDataType = sourceDataType;
this.autoincrement = "YES".equals(isAutoincrement);
this.generatedcolumn = "YES".equals(isGeneratedcolumn);
// 初始化自定义字段值--------------------
setPrimaryKey(false);
setProperty(DatabaseMetadataUtils.underlineToCamelHump(getColumnName()));
TypeInfo typeInfo = TypeInfo.valueOf(getTypeName());
setJdbcType(typeInfo.getTypeName());
setJavaType(typeInfo.getJavaType());
}
public String getTableCat() {
return tableCat;
}
public void setTableCat(String tableCat) {
this.tableCat = tableCat;
}
public String getTableSchem() {
return tableSchem;
}
public void setTableSchem(String tableSchem) {
this.tableSchem = tableSchem;
}
public String getTableName() {
return tableName;
}
public void setTableName(String tableName) {
this.tableName = tableName;
}
public String getColumnName() {
return columnName;
}
public void setColumnName(String columnName) {
this.columnName = columnName;
}
public String getDataType() {
return dataType;
}
public void setDataType(String dataType) {
this.dataType = dataType;
}
public String getTypeName() {
return typeName;
}
public void setTypeName(String typeName) {
this.typeName = typeName;
}
public String getColumnSize() {
return columnSize;
}
public void setColumnSize(String columnSize) {
this.columnSize = columnSize;
}
public String getBufferLength() {
return bufferLength;
}
public void setBufferLength(String bufferLength) {
this.bufferLength = bufferLength;
}
public String getDecimalDigits() {
return decimalDigits;
}
public void setDecimalDigits(String decimalDigits) {
this.decimalDigits = decimalDigits;
}
public String getNumPrecRadix() {
return numPrecRadix;
}
public void setNumPrecRadix(String numPrecRadix) {
this.numPrecRadix = numPrecRadix;
}
public boolean isNullable() {
return nullable;
}
public void setNullable(boolean nullable) {
this.nullable = nullable;
}
public String getRemarks() {
return remarks;
}
public void setRemarks(String remarks) {
this.remarks = remarks;
}
public String getColumnDef() {
return columnDef;
}
public void setColumnDef(String columnDef) {
this.columnDef = columnDef;
}
public String getSqlDataType() {
return sqlDataType;
}
public void setSqlDataType(String sqlDataType) {
this.sqlDataType = sqlDataType;
}
public String getSqlDatetimeSub() {
return sqlDatetimeSub;
}
public void setSqlDatetimeSub(String sqlDatetimeSub) {
this.sqlDatetimeSub = sqlDatetimeSub;
}
public String getCharOctetLength() {
return charOctetLength;
}
public void setCharOctetLength(String charOctetLength) {
this.charOctetLength = charOctetLength;
}
public String getOrdinalPosition() {
return ordinalPosition;
}
public void setOrdinalPosition(String ordinalPosition) {
this.ordinalPosition = ordinalPosition;
}
public String getIsNullable() {
return isNullable;
}
public void setIsNullable(String isNullable) {
this.isNullable = isNullable;
}
public String getScopeCatalog() {
return scopeCatalog;
}
public void setScopeCatalog(String scopeCatalog) {
this.scopeCatalog = scopeCatalog;
}
public String getScopeSchema() {
return scopeSchema;
}
public void setScopeSchema(String scopeSchema) {
this.scopeSchema = scopeSchema;
}
public String getScopeTable() {
return scopeTable;
}
public void setScopeTable(String scopeTable) {
this.scopeTable = scopeTable;
}
public String getSourceDataType() {
return sourceDataType;
}
public void setSourceDataType(String sourceDataType) {
this.sourceDataType = sourceDataType;
}
public boolean isPrimaryKey() {
return primaryKey;
}
public void setPrimaryKey(boolean primaryKey) {
this.primaryKey = primaryKey;
}
public boolean isAutoincrement() {
return autoincrement;
}
public void setAutoincrement(boolean autoincrement) {
this.autoincrement = autoincrement;
}
public boolean isGeneratedcolumn() {
return generatedcolumn;
}
public void setGeneratedcolumn(boolean generatedcolumn) {
this.generatedcolumn = generatedcolumn;
}
public String getProperty() {
return property;
}
public void setProperty(String property) {
this.property = property;
}
public Class getJavaType() {
return javaType;
}
public void setJavaType(Class javaType) {
this.javaType = javaType;
}
public String getJdbcType() {
return jdbcType;
}
public void setJdbcType(String jdbcType) {
this.jdbcType = jdbcType;
}
// getter setter method --------------------------------
public String getGetterMethod() {
return "get" + property.substring(0, 1).toUpperCase() + (property.substring(1));
}
public String getSetterMethod() {
return "set" + property.substring(0, 1).toUpperCase() + (property.substring(1));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy