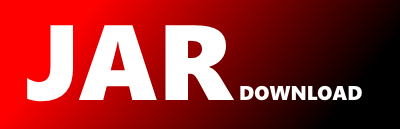
mapper.ResourcesMapper.xml Maven / Gradle / Ivy
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="com.github.freegeese.maven.plugin.autocode.demo.mapper.ResourcesMapper"> <resultMap id="BaseResultMap" type="com.github.freegeese.maven.plugin.autocode.demo.model.Resources"> <id column="id" jdbcType="INTEGER" property="id" /> <result column="id" jdbcType="INTEGER" property="id" /> <result column="name" jdbcType="VARCHAR" property="name" /> <result column="sort" jdbcType="INTEGER" property="sort" /> <result column="parent" jdbcType="INTEGER" property="parent" /> <result column="path" jdbcType="VARCHAR" property="path" /> <result column="attributes" jdbcType="VARCHAR" property="attributes" /> </resultMap> <sql id="Base_Column_List"> id, name, sort, parent, path, attributes </sql> <insert id="insert" keyProperty="id" useGeneratedKeys="true"> insert into t_resources (name, sort, parent, path, attributes) values (#{name,jdbcType=VARCHAR}, #{sort,jdbcType=INTEGER}, #{parent,jdbcType=INTEGER}, #{path,jdbcType=VARCHAR}, #{attributes,jdbcType=VARCHAR}) </insert> <insert id="insertSelective" keyProperty="id" useGeneratedKeys="true"> insert into t_resources <trim prefix="(" suffix=")" suffixOverrides=","> <if test="name != null"> name, </if> <if test="sort != null"> sort, </if> <if test="parent != null"> parent, </if> <if test="path != null"> path, </if> <if test="attributes != null"> attributes, </if> </trim> <trim prefix="values (" suffix=")" suffixOverrides=","> <if test="name != null"> #{name,jdbcType=VARCHAR}, </if> <if test="sort != null"> #{sort,jdbcType=INTEGER}, </if> <if test="parent != null"> #{parent,jdbcType=INTEGER}, </if> <if test="path != null"> #{path,jdbcType=VARCHAR}, </if> <if test="attributes != null"> #{attributes,jdbcType=VARCHAR}, </if> </trim> </insert> <delete id="deleteByPrimaryKey" parameterType="java.lang.Integer"> delete from t_resources where id = #{id,jdbcType=INTEGER} </delete> <delete id="deleteByPrimaryKeys"> delete from t_resources where id in <foreach close=")" collection="list" item="item" open="(" separator=","> #{item} </foreach> </delete> <update id="updateByPrimaryKey"> update t_resources <set> name = #{name,jdbcType=VARCHAR}, sort = #{sort,jdbcType=INTEGER}, parent = #{parent,jdbcType=INTEGER}, path = #{path,jdbcType=VARCHAR}, attributes = #{attributes,jdbcType=VARCHAR} </set> where id = #{id,jdbcType=INTEGER} </update> <update id="updateByPrimaryKeySelective"> update t_resources <set> <if test="name != null"> name = #{name,jdbcType=VARCHAR}, </if> <if test="sort != null"> sort = #{sort,jdbcType=INTEGER}, </if> <if test="parent != null"> parent = #{parent,jdbcType=INTEGER}, </if> <if test="path != null"> path = #{path,jdbcType=VARCHAR}, </if> <if test="attributes != null"> attributes = #{attributes,jdbcType=VARCHAR} </if> </set> where id = #{id,jdbcType=INTEGER} </update> <select id="selectByPrimaryKey" resultMap="BaseResultMap"> select <include refid="Base_Column_List" /> from t_resources where id = #{id,jdbcType=INTEGER} </select> <select id="selectByPrimaryKeys" resultMap="BaseResultMap"> select <include refid="Base_Column_List" /> from t_resources where id in <foreach close=")" collection="list" item="item" open="(" separator=","> #{item} </foreach> </select> <select id="selectByRecord" resultMap="BaseResultMap"> select <include refid="Base_Column_List" /> from t_resources <where> <if test="name != null"> and name = #{name} </if> <if test="sort != null"> and sort = #{sort} </if> <if test="parent != null"> and parent = #{parent} </if> <if test="path != null"> and path = #{path} </if> <if test="attributes != null"> and attributes = #{attributes} </if> </where> order by parent, sort </select> <select id="selectByConditions" resultMap="BaseResultMap"> select <include refid="Base_Column_List" /> from t_resources <where> <if test="name != null"> and name ${name} </if> <if test="sort != null"> and sort ${sort} </if> <if test="parent != null"> and parent ${parent} </if> <if test="path != null"> and path ${path} </if> <if test="attributes != null"> and attributes ${attributes} </if> </where> order by parent, sort </select> <select id="selectAll" resultMap="BaseResultMap"> select <include refid="Base_Column_List" /> from t_resources order by parent, sort </select> <insert id="batchInsert" keyProperty="id" useGeneratedKeys="true"> insert into t_resources (name, sort, parent, path, attributes) values <foreach collection="list" item="item" separator=","> ( #{item.name,jdbcType=VARCHAR}, #{item.sort,jdbcType=INTEGER}, #{item.parent,jdbcType=INTEGER}, #{item.path,jdbcType=VARCHAR}, #{item.attributes,jdbcType=VARCHAR} ) </foreach> </insert> <update id="batchUpdate"> <foreach close="" collection="list" item="item" open="" separator=";"> update t_resources <set> <if test="item.name != null"> name = #{item.name,jdbcType=VARCHAR}, </if> <if test="item.sort != null"> sort = #{item.sort,jdbcType=INTEGER}, </if> <if test="item.parent != null"> parent = #{item.parent,jdbcType=INTEGER}, </if> <if test="item.path != null"> path = #{item.path,jdbcType=VARCHAR}, </if> <if test="item.attributes != null"> attributes = #{item.attributes,jdbcType=VARCHAR} </if> </set> where id = #{item.id,jdbcType=INTEGER} </foreach> </update> <select id="count" resultType="java.lang.Long"> select count(*) from t_resources </select> <update id="exchange"> <if test="source.id != target.id and source.parent == target.parent"> UPDATE t_resources SET sort = #{target.sort} WHERE id = #{source.id}; UPDATE t_resources SET sort = #{source.sort} WHERE id = #{target.id} </if> </update> <update id="move"> <if test="from.id != to.id and from.parent == to.parent"> <if test="from.sort > to.sort"> UPDATE t_resources SET sort = sort + 1 WHERE sort >= #{to.sort} AND parent = #{from.parent} AND sort <![CDATA[ < ]]> #{from.sort}; </if> <if test="to.sort > from.sort"> UPDATE t_resources SET sort = sort - 1 WHERE sort > #{from.sort} AND parent = #{from.parent} AND sort <![CDATA[ <= ]]> #{to.sort}; </if> <if test="to.sort != from.sort"> UPDATE t_resources SET sort = #{to.sort} WHERE id = #{from.id} </if> </if> </update> <update id="updateChildrenPath"> UPDATE t_resources SET path = CONCAT(#{path},SUBSTR(path,LENGTH(#{oldPath}) + 1, LENGTH(path))) WHERE parent = #{id} </update> <select id="selectChildren" resultMap="BaseResultMap"> SELECT <include refid="Base_Column_List" /> FROM t_resources <where> <choose> <when test="deep"> parent like '${entity.parent}%' AND id != #{entity.id} </when> <otherwise> parent = #{entity.id} </otherwise> </choose> </where> order by parent, sort </select> <select id="selectPrevious" resultMap="BaseResultMap"> SELECT <include refid="Base_Column_List" /> FROM t_resources WHERE sort = ( SELECT MAX(sort) FROM t_resources WHERE parent = #{parent} AND sort <![CDATA[ < ]]> #{sort} ) AND parent = #{parent} </select> <select id="selectNext" resultMap="BaseResultMap"> SELECT <include refid="Base_Column_List" /> FROM t_resources WHERE sort = ( SELECT MIN(sort) FROM t_resources WHERE parent = #{parent} AND sort <![CDATA[ > ]]> #{sort} ) AND parent = #{parent} </select> <select id="selectFirst" resultMap="BaseResultMap"> SELECT <include refid="Base_Column_List" /> FROM t_resources WHERE sort = ( SELECT MIN(sort) FROM t_resources WHERE parent = #{parent} ) AND parent = #{parent} </select> <select id="selectLast" resultMap="BaseResultMap"> SELECT <include refid="Base_Column_List" /> FROM t_resources WHERE sort = ( SELECT MAX(sort) FROM t_resources WHERE parent = #{parent} ) AND parent = #{parent} </select> </mapper>
© 2015 - 2025 Weber Informatics LLC | Privacy Policy