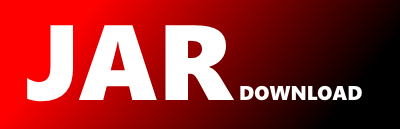
mapper.UserMapper.xml Maven / Gradle / Ivy
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="com.github.freegeese.maven.plugin.autocode.demo.mapper.UserMapper"> <resultMap id="BaseResultMap" type="com.github.freegeese.maven.plugin.autocode.demo.model.User"> <id column="id" jdbcType="INTEGER" property="id" /> <result column="id" jdbcType="INTEGER" property="id" /> <result column="username" jdbcType="VARCHAR" property="username" /> <result column="password" jdbcType="VARCHAR" property="password" /> <result column="email" jdbcType="VARCHAR" property="email" /> <result column="post" jdbcType="VARCHAR" property="post" /> <result column="telephone" jdbcType="VARCHAR" property="telephone" /> <result column="mobilephone" jdbcType="VARCHAR" property="mobilephone" /> <result column="department" jdbcType="VARCHAR" property="department" /> <result column="status" jdbcType="INTEGER" property="status" /> <result column="created_date" jdbcType="TIMESTAMP" property="createdDate" /> <result column="last_modified_date" jdbcType="TIMESTAMP" property="lastModifiedDate" /> </resultMap> <sql id="Base_Column_List"> id, username, password, email, post, telephone, mobilephone, department, status, created_date, last_modified_date </sql> <insert id="insert" keyProperty="id" useGeneratedKeys="true"> insert into t_user (username, password, email, post, telephone, mobilephone, department, status, created_date, last_modified_date) values (#{username,jdbcType=VARCHAR}, #{password,jdbcType=VARCHAR}, #{email,jdbcType=VARCHAR}, #{post,jdbcType=VARCHAR}, #{telephone,jdbcType=VARCHAR}, #{mobilephone,jdbcType=VARCHAR}, #{department,jdbcType=VARCHAR}, #{status,jdbcType=INTEGER}, #{createdDate,jdbcType=TIMESTAMP}, #{lastModifiedDate,jdbcType=TIMESTAMP}) </insert> <insert id="insertSelective" keyProperty="id" useGeneratedKeys="true"> insert into t_user <trim prefix="(" suffix=")" suffixOverrides=","> <if test="username != null"> username, </if> <if test="password != null"> password, </if> <if test="email != null"> email, </if> <if test="post != null"> post, </if> <if test="telephone != null"> telephone, </if> <if test="mobilephone != null"> mobilephone, </if> <if test="department != null"> department, </if> <if test="status != null"> status, </if> <if test="createdDate != null"> created_date, </if> <if test="lastModifiedDate != null"> last_modified_date, </if> </trim> <trim prefix="values (" suffix=")" suffixOverrides=","> <if test="username != null"> #{username,jdbcType=VARCHAR}, </if> <if test="password != null"> #{password,jdbcType=VARCHAR}, </if> <if test="email != null"> #{email,jdbcType=VARCHAR}, </if> <if test="post != null"> #{post,jdbcType=VARCHAR}, </if> <if test="telephone != null"> #{telephone,jdbcType=VARCHAR}, </if> <if test="mobilephone != null"> #{mobilephone,jdbcType=VARCHAR}, </if> <if test="department != null"> #{department,jdbcType=VARCHAR}, </if> <if test="status != null"> #{status,jdbcType=INTEGER}, </if> <if test="createdDate != null"> #{created_date,jdbcType=TIMESTAMP}, </if> <if test="lastModifiedDate != null"> #{last_modified_date,jdbcType=TIMESTAMP}, </if> </trim> </insert> <delete id="deleteByPrimaryKey" parameterType="java.lang.Integer"> delete from t_user where id = #{id,jdbcType=INTEGER} </delete> <delete id="deleteByPrimaryKeys"> delete from t_user where id in <foreach close=")" collection="list" item="item" open="(" separator=","> #{item} </foreach> </delete> <update id="updateByPrimaryKey"> update t_user <set> username = #{username,jdbcType=VARCHAR}, password = #{password,jdbcType=VARCHAR}, email = #{email,jdbcType=VARCHAR}, post = #{post,jdbcType=VARCHAR}, telephone = #{telephone,jdbcType=VARCHAR}, mobilephone = #{mobilephone,jdbcType=VARCHAR}, department = #{department,jdbcType=VARCHAR}, status = #{status,jdbcType=INTEGER}, created_date = #{createdDate,jdbcType=TIMESTAMP}, last_modified_date = #{lastModifiedDate,jdbcType=TIMESTAMP} </set> where id = #{id,jdbcType=INTEGER} </update> <update id="updateByPrimaryKeySelective"> update t_user <set> <if test="username != null"> username = #{username,jdbcType=VARCHAR}, </if> <if test="password != null"> password = #{password,jdbcType=VARCHAR}, </if> <if test="email != null"> email = #{email,jdbcType=VARCHAR}, </if> <if test="post != null"> post = #{post,jdbcType=VARCHAR}, </if> <if test="telephone != null"> telephone = #{telephone,jdbcType=VARCHAR}, </if> <if test="mobilephone != null"> mobilephone = #{mobilephone,jdbcType=VARCHAR}, </if> <if test="department != null"> department = #{department,jdbcType=VARCHAR}, </if> <if test="status != null"> status = #{status,jdbcType=INTEGER}, </if> <if test="createdDate != null"> created_date = #{createdDate,jdbcType=TIMESTAMP}, </if> <if test="lastModifiedDate != null"> last_modified_date = #{lastModifiedDate,jdbcType=TIMESTAMP} </if> </set> where id = #{id,jdbcType=INTEGER} </update> <select id="selectByPrimaryKey" resultMap="BaseResultMap"> select <include refid="Base_Column_List" /> from t_user where id = #{id,jdbcType=INTEGER} </select> <select id="selectByPrimaryKeys" resultMap="BaseResultMap"> select <include refid="Base_Column_List" /> from t_user where id in <foreach close=")" collection="list" item="item" open="(" separator=","> #{item} </foreach> </select> <select id="selectByRecord" resultMap="BaseResultMap"> select <include refid="Base_Column_List" /> from t_user <where> <if test="username != null"> and username = #{username} </if> <if test="password != null"> and password = #{password} </if> <if test="email != null"> and email = #{email} </if> <if test="post != null"> and post = #{post} </if> <if test="telephone != null"> and telephone = #{telephone} </if> <if test="mobilephone != null"> and mobilephone = #{mobilephone} </if> <if test="department != null"> and department = #{department} </if> <if test="status != null"> and status = #{status} </if> <if test="createdDate != null"> and created_date = #{createdDate} </if> <if test="lastModifiedDate != null"> and last_modified_date = #{lastModifiedDate} </if> </where> </select> <select id="selectByConditions" resultMap="BaseResultMap"> select <include refid="Base_Column_List" /> from t_user <where> <if test="username != null"> and username ${username} </if> <if test="password != null"> and password ${password} </if> <if test="email != null"> and email ${email} </if> <if test="post != null"> and post ${post} </if> <if test="telephone != null"> and telephone ${telephone} </if> <if test="mobilephone != null"> and mobilephone ${mobilephone} </if> <if test="department != null"> and department ${department} </if> <if test="status != null"> and status ${status} </if> <if test="createdDate != null"> and created_date ${createdDate} </if> <if test="lastModifiedDate != null"> and last_modified_date ${lastModifiedDate} </if> </where> <if test="orderBy != null"> order by ${orderBy} </if> </select> <select id="selectAll" resultMap="BaseResultMap"> select <include refid="Base_Column_List" /> from t_user </select> <insert id="batchInsert" keyProperty="id" useGeneratedKeys="true"> insert into t_user (username, password, email, post, telephone, mobilephone, department, status, created_date, last_modified_date) values <foreach collection="list" item="item" separator=","> ( #{item.username,jdbcType=VARCHAR}, #{item.password,jdbcType=VARCHAR}, #{item.email,jdbcType=VARCHAR}, #{item.post,jdbcType=VARCHAR}, #{item.telephone,jdbcType=VARCHAR}, #{item.mobilephone,jdbcType=VARCHAR}, #{item.department,jdbcType=VARCHAR}, #{item.status,jdbcType=INTEGER}, #{item.createdDate,jdbcType=TIMESTAMP}, #{item.lastModifiedDate,jdbcType=TIMESTAMP} ) </foreach> </insert> <update id="batchUpdate"> <foreach close="" collection="list" item="item" open="" separator=";"> update t_user <set> <if test="item.username != null"> username = #{item.username,jdbcType=VARCHAR}, </if> <if test="item.password != null"> password = #{item.password,jdbcType=VARCHAR}, </if> <if test="item.email != null"> email = #{item.email,jdbcType=VARCHAR}, </if> <if test="item.post != null"> post = #{item.post,jdbcType=VARCHAR}, </if> <if test="item.telephone != null"> telephone = #{item.telephone,jdbcType=VARCHAR}, </if> <if test="item.mobilephone != null"> mobilephone = #{item.mobilephone,jdbcType=VARCHAR}, </if> <if test="item.department != null"> department = #{item.department,jdbcType=VARCHAR}, </if> <if test="item.status != null"> status = #{item.status,jdbcType=INTEGER}, </if> <if test="item.createdDate != null"> created_date = #{item.createdDate,jdbcType=TIMESTAMP}, </if> <if test="item.lastModifiedDate != null"> last_modified_date = #{item.lastModifiedDate,jdbcType=TIMESTAMP} </if> </set> where id = #{item.id,jdbcType=INTEGER} </foreach> </update> <select id="count" resultType="java.lang.Long"> select count(*) from t_user </select> <!-- CUSTOM_CODE_START --> <select id="about-dialog-button-bar"></select> <!-- CUSTOM_CODE_END --> </mapper>
© 2015 - 2025 Weber Informatics LLC | Privacy Policy