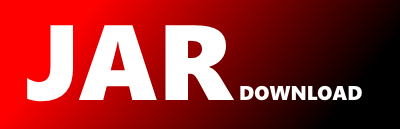
mapper.ConfigurationMapper.xml Maven / Gradle / Ivy
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="com.mocai.merchant.mapper.ConfigurationMapper"> <resultMap id="BaseResultMap" type="com.mocai.merchant.model.Configuration"> <id column="id" jdbcType="INTEGER" property="id" /> <result column="name" jdbcType="VARCHAR" property="name" /> <result column="content" jdbcType="VARCHAR" property="content" /> </resultMap> <sql id="Base_Column_List"> id, name, content </sql> <select id="selectByPrimaryKey" parameterType="java.lang.Integer" resultMap="BaseResultMap"> select <include refid="Base_Column_List" /> from configuration where id = #{id,jdbcType=INTEGER} </select> <delete id="deleteByPrimaryKey" parameterType="java.lang.Integer"> delete from configuration where id = #{id,jdbcType=INTEGER} </delete> <insert id="insert" parameterType="com.mocai.merchant.model.Configuration"> insert into configuration (id, name, content ) values (#{id,jdbcType=INTEGER}, #{name,jdbcType=VARCHAR}, #{content,jdbcType=VARCHAR} ) </insert> <insert id="insertSelective" parameterType="com.mocai.merchant.model.Configuration"> insert into configuration <trim prefix="(" suffix=")" suffixOverrides=","> <if test="id != null"> id, </if> <if test="name != null"> name, </if> <if test="content != null"> content, </if> </trim> <trim prefix="values (" suffix=")" suffixOverrides=","> <if test="id != null"> #{id,jdbcType=INTEGER}, </if> <if test="name != null"> #{name,jdbcType=VARCHAR}, </if> <if test="content != null"> #{content,jdbcType=VARCHAR}, </if> </trim> </insert> <update id="updateByPrimaryKeySelective" parameterType="com.mocai.merchant.model.Configuration"> update configuration <set> <if test="name != null"> name = #{name,jdbcType=VARCHAR}, </if> <if test="content != null"> content = #{content,jdbcType=VARCHAR}, </if> </set> where id = #{id,jdbcType=INTEGER} </update> <update id="updateByPrimaryKey" parameterType="com.mocai.merchant.model.Configuration"> update configuration set name = #{name,jdbcType=VARCHAR}, content = #{content,jdbcType=VARCHAR} where id = #{id,jdbcType=INTEGER} </update> <select id="selectAll" resultMap="BaseResultMap"> select <include refid="Base_Column_List" /> from configuration </select> <select id="selectByPrimaryKeys" resultMap="BaseResultMap"> select <include refid="Base_Column_List" /> from configuration where id in <foreach close=")" collection="list" item="item" open="(" separator=","> #{item} </foreach> </select> <select id="selectByEntity" parameterType="com.mocai.merchant.model.Configuration" resultMap="BaseResultMap"> select <include refid="Base_Column_List" /> from configuration <where> <if test="name != null"> name = #{name,jdbcType=VARCHAR} </if> <if test="content != null"> and content = #{content,jdbcType=VARCHAR} </if> </where> </select> <select id="selectByConditions" parameterType="java.util.Map" resultMap="BaseResultMap"> select <include refid="Base_Column_List" /> from configuration <where> <if test="name != null"> name ${name} </if> <if test="content != null"> and content ${content} </if> <if test="orderBy != null"> order by ${orderBy} </if> </where> </select> <select id="count" resultType="java.lang.Long"> select count(*) from configuration </select> <insert id="batchInsert" keyProperty="id" useGeneratedKeys="true"> insert into configuration (id, name, content) values <foreach collection="list" item="item" separator=","> (#{item.id, jdbcType=INTEGER}, #{item.name, jdbcType=VARCHAR}, #{item.content, jdbcType=VARCHAR}) </foreach> </insert> <update id="batchUpdate"> <foreach close="" collection="list" item="item" open="" separator=";"> update configuration <set> <if test="item.name != null"> name = #{item.name, jdbcType=VARCHAR}, </if> <if test="item.content != null"> content = #{item.content, jdbcType=VARCHAR} </if> </set> where id = #{item.id, jdbcType=INTEGER} </foreach> </update> <delete id="batchDelete"> delete from configuration where id in <foreach close=")" collection="list" item="item" open="(" separator=","> #{item} </foreach> </delete> <!--CUSTOM_CODE_START--> <!--CUSTOM_CODE_END--> </mapper>
© 2015 - 2025 Weber Informatics LLC | Privacy Policy