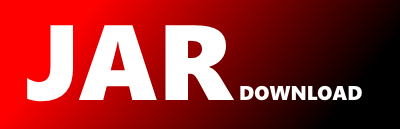
freeseawind.lf.basic.tabbedpane.LuckTabbedPaneUIBundle Maven / Gradle / Ivy
Show all versions of littleluck Show documentation
package freeseawind.lf.basic.tabbedpane;
import java.awt.Color;
import javax.swing.UIDefaults;
import javax.swing.UIManager;
import javax.swing.plaf.InsetsUIResource;
import freeseawind.lf.cfg.LuckResourceBundle;
/**
* TabbedPane资源绑定类。
*
* TabbedPane resource bundle class.
*
* @author freeseawind@github
* @version 1.0
*
*/
public class LuckTabbedPaneUIBundle extends LuckResourceBundle
{
/**
* TabbedPane背景颜色key。
*
* TabbedPane background color property.
*/
public static final String BACKGROUNDCOLOR = "TabbedPane.background";
/**
* 内容面板颜色key。
*
* TabbedPane content area background color property.
*/
public static final String CONTENTAREACOLOR = "TabbedPane.contentAreaColor";
/**
* 选项卡被选中时颜色key。
*
* TabbedPane tab background color properties when tab selected.
*/
public static final String SELECTEDCOLOR = "TabbedPane.selected";
/**
* TabbedPane选中时字体颜色属性key。
*
* TabbedPane tab font color properties when tab selected.
*/
public static final String SELECTEDFOREGROUND = "TabbedPane.selectedForeground";
/**
* 内容面板是否透明key。
*
* TabbedPane content panel is transparent properties.
*/
public static final String CONTENTOPAQUE = "TabbedPane.contentOpaque";
/**
* TabbedPane是否透明key。
*
* TabbedPane is transparent properties.
*/
public static final String OPAQUE = "TabbedPane.opaque";
/**
* 是否使用半透明边框key。
*
* TabbedPane is transparent border properties.
*/
public static final String TABSOVERLAPBORDER = "TabbedPane.tabsOverlapBorder";
/**
* 内容边框间距key。
*
* Content padding properties.
*/
public static final String CONTENTBORDERINSETS = "TabbedPane.contentBorderInsets";
/**
* 选项卡选中时的间距配置key。
*
* TabbedPane tab padding properties when tab selected.
*/
public static final String SELECTEDTABPADINSETS = "TabbedPane.selectedTabPadInsets";
/**
* 选项卡间距配置key。
*
* TabbedPane tab padding properties.
*/
public static final String TABAREAINSETS = "TabbedPane.tabAreaInsets";
/**
* 边框颜色key。
*
* TabbedPane tab border color properties.
*/
public static final String SHADOW = "TabbedPane.shadow";
/**
*
* [LittleLuck属性]选项卡选中时的边框颜色属性key。
*
*
*
* [LittLeLuck Attributes]TabbedPane tab border color
* properties when selected.
*
*/
public static final String SELECTEDSHADOW = "TabbedPane.selectedShadow";
/**
* Tab间距属性key,可通过此属性控制Tab的宽高。
*
* Tab spacing attribute key, you can control the Tab width and height by this property.
*/
public static final String TABINSETS = "TabbedPane.tabInsets";
public void uninitialize()
{
UIManager.put(BACKGROUNDCOLOR, null);
UIManager.put(CONTENTAREACOLOR, null);
UIManager.put(SELECTEDFOREGROUND, null);
UIManager.put(SELECTEDCOLOR, null);
UIManager.put(SHADOW, null);
UIManager.put(SELECTEDSHADOW, null);
UIManager.put(CONTENTOPAQUE, null);
UIManager.put(OPAQUE, null);
UIManager.put(TABSOVERLAPBORDER, null);
UIManager.put(SELECTEDTABPADINSETS, null);
UIManager.put(TABAREAINSETS, null);
UIManager.put(CONTENTBORDERINSETS, null);
UIManager.put(TABINSETS, null);
}
@Override
protected void installColor(UIDefaults table)
{
UIManager.put(BACKGROUNDCOLOR, getColorRes(Color.WHITE));
UIManager.put(CONTENTAREACOLOR, getColorRes(Color.WHITE));
UIManager.put(SELECTEDFOREGROUND, getColorRes(Color.WHITE));
//171, 225, 235
UIManager.put(SELECTEDCOLOR, getColorRes(9, 163, 200));
UIManager.put(SHADOW, getColorRes(221, 220, 227));
UIManager.put(SELECTEDSHADOW, getColorRes(221, 220, 227));
}
@Override
protected void installOther(UIDefaults table)
{
UIManager.put(CONTENTOPAQUE, Boolean.FALSE);
UIManager.put(OPAQUE, Boolean.TRUE);
UIManager.put(TABSOVERLAPBORDER, Boolean.FALSE);
UIManager.put(SELECTEDTABPADINSETS, new InsetsUIResource(0, 0, 0, 0));
UIManager.put(TABAREAINSETS, new InsetsUIResource(3, 2, 0, 2));
UIManager.put(CONTENTBORDERINSETS, new InsetsUIResource(1, 1, 1, 1));
UIManager.put(TABINSETS, new InsetsUIResource(3, 9, 3, 9));
}
}