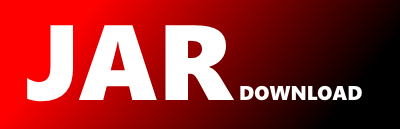
com.github.frtu.simple.infra.reflect.ReflectionMapperUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of simple-infra Show documentation
Show all versions of simple-infra Show documentation
Provide a set of classes for infrastructure and bootstrap that have very few dependencies that you can embedded easily into any application
The newest version!
package com.github.frtu.simple.infra.reflect;
import java.lang.annotation.Annotation;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import org.springframework.core.annotation.AnnotationUtils;
import org.springframework.util.ReflectionUtils;
import org.springframework.util.ReflectionUtils.FieldCallback;
/**
* Scan a source Class or Method and map it into a targetClass where fields has the wanted annotation.
*
* @author Frédéric TU
*/
public class ReflectionMapperUtil {
public static T scanAnnotationSource(final Class> sourceClass, final Class targetClass) {
final T bean = BeanGenerator.generateBean(targetClass);
FieldCallback fc = new FieldCallback() {
@Override
public void doWith(Field field) throws IllegalArgumentException, IllegalAccessException {
Class> type = field.getType();
// If is annotation
if (type.isAnnotation()) {
Class extends Annotation> annotation = type.asSubclass(Annotation.class);
// Fetch the annotation from the sourceClass
Annotation annotationFoundInSource = AnnotationUtils.findAnnotation(sourceClass, annotation);
// Set it back into the target object
field.setAccessible(true);
ReflectionUtils.setField(field, bean, annotationFoundInSource);
}
}
};
ReflectionUtils.doWithFields(targetClass, fc);
return bean;
}
public static T scanAnnotationSource(final Method sourceMethod, final Class targetClass) {
final T bean = BeanGenerator.generateBean(targetClass);
FieldCallback fc = new FieldCallback() {
@Override
public void doWith(Field field) throws IllegalArgumentException, IllegalAccessException {
Class> type = field.getType();
// If is annotation
if (type.isAnnotation()) {
Class extends Annotation> annotation = type.asSubclass(Annotation.class);
// Fetch the annotation from the sourceClass
Annotation annotationFoundInSource = AnnotationUtils.findAnnotation(sourceMethod, annotation);
// Set it back into the target object
field.setAccessible(true);
ReflectionUtils.setField(field, bean, annotationFoundInSource);
}
}
};
ReflectionUtils.doWithFields(targetClass, fc);
return bean;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy