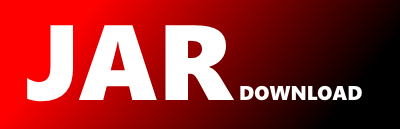
io.github.livr.rules.Modifiers Maven / Gradle / Ivy
package io.github.livr.rules;
import org.json.simple.JSONObject;
import io.github.livr.FunctionKeeper;
import io.github.livr.LIVRUtils;
import java.util.List;
import java.util.function.Function;
import java.util.regex.Pattern;
/**
* Created by vladislavbaluk on 9/28/2017.
*/
public class Modifiers {
public static Function, Function> default1 = objects -> {
final Object defaultValue = objects.get(0);
return (Function) (wrapper) -> {
if (LIVRUtils.isNoValue(wrapper.getValue())) {
wrapper.getFieldResultArr().add(defaultValue);
return "";
}
return "";
};
};
public static Function, Function> trim = objects -> (Function) (wrapper) -> {
if (LIVRUtils.isNoValue(wrapper.getValue()) || wrapper.getValue().getClass() == JSONObject.class) return "";
wrapper.getFieldResultArr().add((wrapper.getValue() + "").trim());
return "";
};
public static Function, Function> to_lc = objects -> (Function) (wrapper) -> {
if (LIVRUtils.isNoValue(wrapper.getValue()) || wrapper.getValue().getClass() == JSONObject.class) return "";
wrapper.getFieldResultArr().add((wrapper.getValue() + "").toLowerCase());
return "";
};
public static Function, Function> to_uc = objects -> (Function) (wrapper) -> {
if (LIVRUtils.isNoValue(wrapper.getValue()) || wrapper.getValue().getClass() == JSONObject.class) return "";
wrapper.getFieldResultArr().add((wrapper.getValue() + "").toUpperCase());
return "";
};
public static Function, Function> remove = objects -> {
String escaped = Pattern.quote(objects.get(0) + "");
String chars = "[" + escaped + "]";
return (Function) (wrapper) -> {
if (LIVRUtils.isNoValue(wrapper.getValue()) || wrapper.getValue().getClass() == JSONObject.class) return "";
wrapper.getFieldResultArr().add((wrapper.getValue() + "").replaceAll(chars, ""));
return "";
};
};
public static Function, Function> leave_only = objects -> {
String escaped = Pattern.quote(objects.get(0) + "");
String chars = "[^" + escaped + "]";
return (Function) (wrapper) -> {
if (LIVRUtils.isNoValue(wrapper.getValue()) || wrapper.getValue().getClass() == JSONObject.class) return "";
wrapper.getFieldResultArr().add((wrapper.getValue() + "").replaceAll(chars, ""));
return "";
};
};
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy