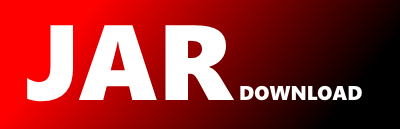
openllet.aterm.pure.ATermApplImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openllet-functions Show documentation
Show all versions of openllet-functions Show documentation
Openllet management of abstracts functions
/*
* Copyright (c) 2002-2007, CWI and INRIA
*
* All rights reserved.
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* * Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* * Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
* * Neither the name of the University of California, Berkeley nor the
* names of its contributors may be used to endorse or promote products
* derived from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE REGENTS AND CONTRIBUTORS ``AS IS'' AND ANY
* EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
* WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL THE REGENTS AND CONTRIBUTORS BE LIABLE FOR ANY
* DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
* (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
* LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
* ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
* SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package openllet.aterm.pure;
import java.io.IOException;
import java.util.List;
import openllet.aterm.AFun;
import openllet.aterm.ATerm;
import openllet.aterm.ATermAppl;
import openllet.aterm.ATermList;
import openllet.aterm.ATermPlaceholder;
import openllet.aterm.Visitor;
import openllet.aterm.stream.BufferedOutputStreamWriter;
import openllet.shared.hash.HashFunctions;
import openllet.shared.hash.SharedObject;
public class ATermApplImpl extends ATermImpl implements ATermAppl
{
private final AFun _fun;
private final ATerm[] _args;
protected ATermApplImpl(final PureFactory factory, final AFun fun, final ATerm[] i_args)
{
super(factory);
_fun = fun;
_args = i_args;
setHashCode(hashFunction());
}
@Override
public int getType()
{
return ATerm.APPL;
}
@Override
public SharedObject duplicate()
{
return this;
}
protected ATermAppl make(final AFun fun, final ATerm[] i_args)
{
return getPureFactory().makeAppl(fun, i_args);
}
@Override
public boolean equivalent(final SharedObject obj)
{
if (obj instanceof ATermAppl)
{
final ATermAppl peer = (ATermAppl) obj;
if (peer.getType() != getType())
return false;
if (peer.getAFun().equals(_fun))
{
for (int i = 0; i < _args.length; i++)
if (!peer.getArgument(i).equals(_args[i]))
return false;
return true;//peer.getAnnotations().equals(getAnnotations());
}
}
return false;
}
@Override
protected boolean match(final ATerm pattern, final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy