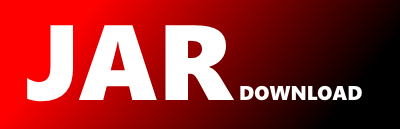
com.github.geko444.im4java.core.IMOps Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of im4java Show documentation
Show all versions of im4java Show documentation
im4java is a pure-java interface to the ImageMagick commandline. It is not meant as a replacement for JMagick, but as an addition. For details about the project, please visit the main project page.
The newest version!
/**************************************************************************
/* This class adds all the IM methods to an Operation.
/*
/* Copyright (c) 2009 by Bernhard Bablok ([email protected])
/*
/* This program is free software; you can redistribute it and/or modify
/* it under the terms of the GNU Library General Public License as published
/* by the Free Software Foundation; either version 2 of the License or
/* (at your option) any later version.
/*
/* This program is distributed in the hope that it will be useful, but
/* WITHOUT ANY WARRANTY; without even the implied warranty of
/* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
/* GNU Library General Public License for more details.
/*
/* You should have received a copy of the GNU Library General Public License
/* along with this program; see the file COPYING.LIB. If not, write to
/* the Free Software Foundation Inc., 59 Temple Place - Suite 330,
/* Boston, MA 02111-1307 USA
/**************************************************************************/
package com.github.geko444.im4java.core;
/**
This class subclasses Operation and adds methods for all commandline
options of ImageMagick.
This class is automatically generated by the source-code generator of
im4java.
@version $Revision: 1.14 $
@author $Author: bablokb $
*/
public class IMOps extends Operation {
//////////////////////////////////////////////////////////////////////////////
/**
The protected Constructor. You should only use subclasses of IMOps.
*/
protected IMOps() {
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -adaptive-blur to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps adaptiveBlur() {
iCmdArgs.add("-adaptive-blur");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -adaptive-blur to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps adaptiveBlur(Double radius) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-adaptive-blur");
if (radius != null) {
buf.append(radius.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -adaptive-blur to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps adaptiveBlur(Double radius, Double sigma) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-adaptive-blur");
if (radius != null) {
buf.append(radius.toString());
}
if (radius != null || sigma != null) {
buf.append("x");
}
if (sigma != null) {
buf.append(sigma.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -adaptive-resize to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps adaptiveResize() {
iCmdArgs.add("-adaptive-resize");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -adaptive-resize to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps adaptiveResize(Integer width) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-adaptive-resize");
if (width != null) {
buf.append(width.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -adaptive-resize to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps adaptiveResize(Integer width, Integer height) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-adaptive-resize");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -adaptive-resize to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps adaptiveResize(Integer width, Integer height, Character special) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-adaptive-resize");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || special != null) {
}
if (special != null) {
buf.append(special.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -adaptive-resize to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps adaptiveResize(Integer width, Integer height, String special) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-adaptive-resize");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || special != null) {
}
if (special != null) {
buf.append(special.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -adaptive-sharpen to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps adaptiveSharpen() {
iCmdArgs.add("-adaptive-sharpen");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -adaptive-sharpen to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps adaptiveSharpen(Double radius) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-adaptive-sharpen");
if (radius != null) {
buf.append(radius.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -adaptive-sharpen to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps adaptiveSharpen(Double radius, Double sigma) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-adaptive-sharpen");
if (radius != null) {
buf.append(radius.toString());
}
if (radius != null || sigma != null) {
buf.append("x");
}
if (sigma != null) {
buf.append(sigma.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -adjoin to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps adjoin() {
iCmdArgs.add("-adjoin");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +adjoin to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_adjoin() {
iCmdArgs.add("+adjoin");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -affine to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps affine() {
iCmdArgs.add("-affine");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -affine to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps affine(Double sx) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-affine");
if (sx != null) {
buf.append(sx.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -affine to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps affine(Double sx, Double rx) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-affine");
if (sx != null) {
buf.append(sx.toString());
}
if (sx != null || rx != null) {
buf.append(",");
}
if (rx != null) {
buf.append(rx.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -affine to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps affine(Double sx, Double rx, Double ry) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-affine");
if (sx != null) {
buf.append(sx.toString());
}
if (sx != null || rx != null) {
buf.append(",");
}
if (rx != null) {
buf.append(rx.toString());
}
if (rx != null || ry != null) {
buf.append(",");
}
if (ry != null) {
buf.append(ry.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -affine to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps affine(Double sx, Double rx, Double ry, Double sy) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-affine");
if (sx != null) {
buf.append(sx.toString());
}
if (sx != null || rx != null) {
buf.append(",");
}
if (rx != null) {
buf.append(rx.toString());
}
if (rx != null || ry != null) {
buf.append(",");
}
if (ry != null) {
buf.append(ry.toString());
}
if (ry != null || sy != null) {
buf.append(",");
}
if (sy != null) {
buf.append(sy.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -affine to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps affine(Double sx, Double rx, Double ry, Double sy, Double tx) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-affine");
if (sx != null) {
buf.append(sx.toString());
}
if (sx != null || rx != null) {
buf.append(",");
}
if (rx != null) {
buf.append(rx.toString());
}
if (rx != null || ry != null) {
buf.append(",");
}
if (ry != null) {
buf.append(ry.toString());
}
if (ry != null || sy != null) {
buf.append(",");
}
if (sy != null) {
buf.append(sy.toString());
}
if (sy != null || tx != null) {
buf.append(",");
}
if (tx != null) {
buf.append(tx.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -affine to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps affine(Double sx, Double rx, Double ry, Double sy, Double tx, Double ty) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-affine");
if (sx != null) {
buf.append(sx.toString());
}
if (sx != null || rx != null) {
buf.append(",");
}
if (rx != null) {
buf.append(rx.toString());
}
if (rx != null || ry != null) {
buf.append(",");
}
if (ry != null) {
buf.append(ry.toString());
}
if (ry != null || sy != null) {
buf.append(",");
}
if (sy != null) {
buf.append(sy.toString());
}
if (sy != null || tx != null) {
buf.append(",");
}
if (tx != null) {
buf.append(tx.toString());
}
if (tx != null || ty != null) {
buf.append(",");
}
if (ty != null) {
buf.append(ty.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -alpha to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps alpha() {
iCmdArgs.add("-alpha");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -alpha to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps alpha(String type) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-alpha");
if (type != null) {
buf.append(type.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -annotate to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps annotate() {
iCmdArgs.add("-annotate");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -annotate to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps annotate(Integer xr) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-annotate");
if (xr != null) {
buf.append(xr.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -annotate to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps annotate(Integer xr, Integer yr) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-annotate");
if (xr != null) {
buf.append(xr.toString());
}
if (xr != null || yr != null) {
buf.append("x");
}
if (yr != null) {
buf.append(yr.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -annotate to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps annotate(Integer xr, Integer yr, Integer x) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-annotate");
if (xr != null) {
buf.append(xr.toString());
}
if (xr != null || yr != null) {
buf.append("x");
}
if (yr != null) {
buf.append(yr.toString());
}
if (yr != null || x != null) {
oper="+";
if (x.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (x != null) {
buf.append(x.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -annotate to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps annotate(Integer xr, Integer yr, Integer x, Integer y) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-annotate");
if (xr != null) {
buf.append(xr.toString());
}
if (xr != null || yr != null) {
buf.append("x");
}
if (yr != null) {
buf.append(yr.toString());
}
if (yr != null || x != null) {
oper="+";
if (x.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (x != null) {
buf.append(x.toString());
}
if (x != null || y != null) {
oper="+";
if (y.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (y != null) {
buf.append(y.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -annotate to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps annotate(Integer xr, Integer yr, Integer x, Integer y, String text) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-annotate");
if (xr != null) {
buf.append(xr.toString());
}
if (xr != null || yr != null) {
buf.append("x");
}
if (yr != null) {
buf.append(yr.toString());
}
if (yr != null || x != null) {
oper="+";
if (x.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (x != null) {
buf.append(x.toString());
}
if (x != null || y != null) {
oper="+";
if (y.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (y != null) {
buf.append(y.toString());
}
if (y != null || text != null) {
iCmdArgs.add(buf.toString());
buf.setLength(0);
}
if (text != null) {
buf.append(text.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -antialias to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps antialias() {
iCmdArgs.add("-antialias");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +antialias to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_antialias() {
iCmdArgs.add("+antialias");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -append to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps append() {
iCmdArgs.add("-append");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +append to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_append() {
iCmdArgs.add("+append");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -attenuate to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps attenuate() {
iCmdArgs.add("-attenuate");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -attenuate to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps attenuate(Double value) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-attenuate");
if (value != null) {
buf.append(value.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -authenticate to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps authenticate() {
iCmdArgs.add("-authenticate");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -authenticate to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps authenticate(String password) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-authenticate");
if (password != null) {
buf.append(password.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -auto-gamma to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps autoGamma() {
iCmdArgs.add("-auto-gamma");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -auto-level to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps autoLevel() {
iCmdArgs.add("-auto-level");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -auto-orient to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps autoOrient() {
iCmdArgs.add("-auto-orient");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -average to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps average() {
iCmdArgs.add("-average");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -backdrop to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps backdrop() {
iCmdArgs.add("-backdrop");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -backdrop to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps backdrop(String color) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-backdrop");
if (color != null) {
buf.append(color.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -background to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps background() {
iCmdArgs.add("-background");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -background to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps background(String color) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-background");
if (color != null) {
buf.append(color.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -bench to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps bench() {
iCmdArgs.add("-bench");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -bench to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps bench(Integer iterations) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-bench");
if (iterations != null) {
buf.append(iterations.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -blend to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps blend() {
iCmdArgs.add("-blend");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -blend to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps blend(Integer srcPercent) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-blend");
if (srcPercent != null) {
buf.append(srcPercent.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -blend to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps blend(Integer srcPercent, Integer dstPercent) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-blend");
if (srcPercent != null) {
buf.append(srcPercent.toString());
}
if (srcPercent != null || dstPercent != null) {
buf.append("x");
}
if (dstPercent != null) {
buf.append(dstPercent.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -bias to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps bias() {
iCmdArgs.add("-bias");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -bias to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps bias(Integer value) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-bias");
if (value != null) {
buf.append(value.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -bias to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps bias(Integer value, Boolean percent) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-bias");
if (value != null) {
buf.append(value.toString());
}
if (value != null || percent != null) {
}
if (percent != null) {
if (percent.booleanValue())
buf.append("%");
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -black-point-compensation to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps blackPointCompensation() {
iCmdArgs.add("-black-point-compensation");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -black-threshold to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps blackThreshold() {
iCmdArgs.add("-black-threshold");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -black-threshold to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps blackThreshold(Double threshold) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-black-threshold");
if (threshold != null) {
buf.append(threshold.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -black-threshold to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps blackThreshold(Double threshold, Boolean percent) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-black-threshold");
if (threshold != null) {
buf.append(threshold.toString());
}
if (threshold != null || percent != null) {
}
if (percent != null) {
if (percent.booleanValue())
buf.append("%");
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -blue-primary to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps bluePrimary() {
iCmdArgs.add("-blue-primary");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -blue-primary to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps bluePrimary(Double x) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-blue-primary");
if (x != null) {
buf.append(x.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -blue-primary to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps bluePrimary(Double x, Double y) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-blue-primary");
if (x != null) {
buf.append(x.toString());
}
if (x != null || y != null) {
buf.append(",");
}
if (y != null) {
buf.append(y.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -blue-shift to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps blueShift() {
iCmdArgs.add("-blue-shift");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -blue-shift to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps blueShift(Double factor) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-blue-shift");
if (factor != null) {
buf.append(factor.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -blur to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps blur() {
iCmdArgs.add("-blur");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -blur to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps blur(Double radius) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-blur");
if (radius != null) {
buf.append(radius.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -blur to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps blur(Double radius, Double sigma) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-blur");
if (radius != null) {
buf.append(radius.toString());
}
if (radius != null || sigma != null) {
buf.append("x");
}
if (sigma != null) {
buf.append(sigma.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -bordercolor to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps bordercolor() {
iCmdArgs.add("-bordercolor");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -bordercolor to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps bordercolor(String color) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-bordercolor");
if (color != null) {
buf.append(color.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -border to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps border() {
iCmdArgs.add("-border");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -border to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps border(Integer width) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-border");
if (width != null) {
buf.append(width.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -border to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps border(Integer width, Integer height) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-border");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -borderwidth to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps borderwidth() {
iCmdArgs.add("-borderwidth");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -borderwidth to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps borderwidth(Integer width) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-borderwidth");
if (width != null) {
buf.append(width.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -borderwidth to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps borderwidth(Integer width, Integer height) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-borderwidth");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -borderwidth to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps borderwidth(Integer width, Integer height, Integer xOffset) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-borderwidth");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || xOffset != null) {
oper="+";
if (xOffset.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (xOffset != null) {
buf.append(xOffset.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -borderwidth to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps borderwidth(Integer width, Integer height, Integer xOffset, Integer yOffset) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-borderwidth");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || xOffset != null) {
oper="+";
if (xOffset.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (xOffset != null) {
buf.append(xOffset.toString());
}
if (xOffset != null || yOffset != null) {
oper="+";
if (yOffset.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (yOffset != null) {
buf.append(yOffset.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -brightness-contrast to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps brightnessContrast() {
iCmdArgs.add("-brightness-contrast");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -brightness-contrast to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps brightnessContrast(Double brightness) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-brightness-contrast");
if (brightness != null) {
buf.append(brightness.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -brightness-contrast to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps brightnessContrast(Double brightness, Double contrast) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-brightness-contrast");
if (brightness != null) {
buf.append(brightness.toString());
}
if (brightness != null || contrast != null) {
buf.append("x");
}
if (contrast != null) {
buf.append(contrast.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -brightness-contrast to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps brightnessContrast(Double brightness, Double contrast, Boolean percent) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-brightness-contrast");
if (brightness != null) {
buf.append(brightness.toString());
}
if (brightness != null || contrast != null) {
buf.append("x");
}
if (contrast != null) {
buf.append(contrast.toString());
}
if (contrast != null || percent != null) {
}
if (percent != null) {
if (percent.booleanValue())
buf.append("%");
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -cache to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps cache() {
iCmdArgs.add("-cache");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -cache to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps cache(Double threshold) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-cache");
if (threshold != null) {
buf.append(threshold.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -caption to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps caption() {
iCmdArgs.add("-caption");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -caption to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps caption(String text) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-caption");
if (text != null) {
buf.append(text.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -cdl to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps cdl() {
iCmdArgs.add("-cdl");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -cdl to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps cdl(String filename) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-cdl");
if (filename != null) {
buf.append(filename.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +channel to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_channel() {
iCmdArgs.add("+channel");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -channel to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps channel() {
iCmdArgs.add("-channel");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -channel to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps channel(String type) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-channel");
if (type != null) {
buf.append(type.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -charcoal to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps charcoal() {
iCmdArgs.add("-charcoal");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -charcoal to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps charcoal(Integer factor) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-charcoal");
if (factor != null) {
buf.append(factor.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -chop to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps chop() {
iCmdArgs.add("-chop");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -chop to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps chop(Integer width) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-chop");
if (width != null) {
buf.append(width.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -chop to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps chop(Integer width, Integer height) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-chop");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -chop to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps chop(Integer width, Integer height, Integer x) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-chop");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || x != null) {
oper="+";
if (x.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (x != null) {
buf.append(x.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -chop to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps chop(Integer width, Integer height, Integer x, Integer y) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-chop");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || x != null) {
oper="+";
if (x.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (x != null) {
buf.append(x.toString());
}
if (x != null || y != null) {
oper="+";
if (y.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (y != null) {
buf.append(y.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -chop to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps chop(Integer width, Integer height, Integer x, Integer y, Boolean percent) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-chop");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || x != null) {
oper="+";
if (x.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (x != null) {
buf.append(x.toString());
}
if (x != null || y != null) {
oper="+";
if (y.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (y != null) {
buf.append(y.toString());
}
if (y != null || percent != null) {
}
if (percent != null) {
if (percent.booleanValue())
buf.append("%");
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -clamp to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps clamp() {
iCmdArgs.add("-clamp");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -clip to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps clip() {
iCmdArgs.add("-clip");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -clip-mask to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps clipMask() {
iCmdArgs.add("-clip-mask");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -clip-path to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps clipPath() {
iCmdArgs.add("-clip-path");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -clip-path to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps clipPath(String id) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-clip-path");
if (id != null) {
buf.append(id.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +clip-path to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_clipPath() {
iCmdArgs.add("+clip-path");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +clip-path to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_clipPath(String id) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("+clip-path");
if (id != null) {
buf.append(id.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +clone to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_clone() {
iCmdArgs.add("+clone");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -clone to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps clone() {
iCmdArgs.add("-clone");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -clone to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps clone(Integer index1) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-clone");
if (index1 != null) {
buf.append(index1.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -clone to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps clone(Integer index1, Integer index2) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-clone");
if (index1 != null) {
buf.append(index1.toString());
}
if (index1 != null || index2 != null) {
buf.append(",");
}
if (index2 != null) {
buf.append(index2.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -clone to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps clone(Integer index1, Integer index2, Integer index3) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-clone");
if (index1 != null) {
buf.append(index1.toString());
}
if (index1 != null || index2 != null) {
buf.append(",");
}
if (index2 != null) {
buf.append(index2.toString());
}
if (index2 != null || index3 != null) {
buf.append(",");
}
if (index3 != null) {
buf.append(index3.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -clone to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps clone(String indexes) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-clone");
if (indexes != null) {
buf.append(indexes.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -clut to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps clut() {
iCmdArgs.add("-clut");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -coalesce to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps coalesce() {
iCmdArgs.add("-coalesce");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -colorize to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps colorize() {
iCmdArgs.add("-colorize");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -colorize to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps colorize(Integer red) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-colorize");
if (red != null) {
buf.append(red.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -colorize to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps colorize(Integer red, Integer blue) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-colorize");
if (red != null) {
buf.append(red.toString());
}
if (red != null || blue != null) {
buf.append(",");
}
if (blue != null) {
buf.append(blue.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -colorize to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps colorize(Integer red, Integer blue, Integer green) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-colorize");
if (red != null) {
buf.append(red.toString());
}
if (red != null || blue != null) {
buf.append(",");
}
if (blue != null) {
buf.append(blue.toString());
}
if (blue != null || green != null) {
buf.append(",");
}
if (green != null) {
buf.append(green.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -colormap to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps colormap() {
iCmdArgs.add("-colormap");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -colormap to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps colormap(String type) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-colormap");
if (type != null) {
buf.append(type.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -color-matrix to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps colorMatrix() {
iCmdArgs.add("-color-matrix");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -color-matrix to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps colorMatrix(String matrix) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-color-matrix");
if (matrix != null) {
buf.append(matrix.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -colors to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps colors() {
iCmdArgs.add("-colors");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -colors to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps colors(Integer value) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-colors");
if (value != null) {
buf.append(value.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -colorspace to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps colorspace() {
iCmdArgs.add("-colorspace");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -colorspace to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps colorspace(String value) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-colorspace");
if (value != null) {
buf.append(value.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -combine to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps combine() {
iCmdArgs.add("-combine");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -comment to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps comment() {
iCmdArgs.add("-comment");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -comment to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps comment(String text) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-comment");
if (text != null) {
buf.append(text.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -compose to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps compose() {
iCmdArgs.add("-compose");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -compose to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps compose(String operator) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-compose");
if (operator != null) {
buf.append(operator.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -composite to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps composite() {
iCmdArgs.add("-composite");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +compress to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_compress() {
iCmdArgs.add("+compress");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -compress to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps compress() {
iCmdArgs.add("-compress");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -compress to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps compress(String type) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-compress");
if (type != null) {
buf.append(type.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -contrast to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps contrast() {
iCmdArgs.add("-contrast");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +contrast to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_contrast() {
iCmdArgs.add("+contrast");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -contrast-stretch to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps contrastStretch() {
iCmdArgs.add("-contrast-stretch");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -contrast-stretch to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps contrastStretch(Integer blackPoint) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-contrast-stretch");
if (blackPoint != null) {
buf.append(blackPoint.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -contrast-stretch to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps contrastStretch(Integer blackPoint, Integer whitePoint) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-contrast-stretch");
if (blackPoint != null) {
buf.append(blackPoint.toString());
}
if (blackPoint != null || whitePoint != null) {
buf.append("x");
}
if (whitePoint != null) {
buf.append(whitePoint.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -contrast-stretch to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps contrastStretch(Integer blackPoint, Integer whitePoint, Boolean percent) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-contrast-stretch");
if (blackPoint != null) {
buf.append(blackPoint.toString());
}
if (blackPoint != null || whitePoint != null) {
buf.append("x");
}
if (whitePoint != null) {
buf.append(whitePoint.toString());
}
if (whitePoint != null || percent != null) {
}
if (percent != null) {
if (percent.booleanValue())
buf.append("%");
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -convolve to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps convolve() {
iCmdArgs.add("-convolve");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -convolve to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps convolve(Integer k1) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-convolve");
if (k1 != null) {
buf.append(k1.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -convolve to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps convolve(Integer k1, Integer k2) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-convolve");
if (k1 != null) {
buf.append(k1.toString());
}
if (k1 != null || k2 != null) {
buf.append(",");
}
if (k2 != null) {
buf.append(k2.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -convolve to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps convolve(Integer k1, Integer k2, Integer k3) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-convolve");
if (k1 != null) {
buf.append(k1.toString());
}
if (k1 != null || k2 != null) {
buf.append(",");
}
if (k2 != null) {
buf.append(k2.toString());
}
if (k2 != null || k3 != null) {
buf.append(",");
}
if (k3 != null) {
buf.append(k3.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -convolve to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps convolve(Integer k1, Integer k2, Integer k3, Integer k4) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-convolve");
if (k1 != null) {
buf.append(k1.toString());
}
if (k1 != null || k2 != null) {
buf.append(",");
}
if (k2 != null) {
buf.append(k2.toString());
}
if (k2 != null || k3 != null) {
buf.append(",");
}
if (k3 != null) {
buf.append(k3.toString());
}
if (k3 != null || k4 != null) {
buf.append(",");
}
if (k4 != null) {
buf.append(k4.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -convolve to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps convolve(Integer k1, Integer k2, Integer k3, Integer k4, Integer k5) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-convolve");
if (k1 != null) {
buf.append(k1.toString());
}
if (k1 != null || k2 != null) {
buf.append(",");
}
if (k2 != null) {
buf.append(k2.toString());
}
if (k2 != null || k3 != null) {
buf.append(",");
}
if (k3 != null) {
buf.append(k3.toString());
}
if (k3 != null || k4 != null) {
buf.append(",");
}
if (k4 != null) {
buf.append(k4.toString());
}
if (k4 != null || k5 != null) {
buf.append(",");
}
if (k5 != null) {
buf.append(k5.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -convolve to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps convolve(Integer k1, Integer k2, Integer k3, Integer k4, Integer k5, Integer k6) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-convolve");
if (k1 != null) {
buf.append(k1.toString());
}
if (k1 != null || k2 != null) {
buf.append(",");
}
if (k2 != null) {
buf.append(k2.toString());
}
if (k2 != null || k3 != null) {
buf.append(",");
}
if (k3 != null) {
buf.append(k3.toString());
}
if (k3 != null || k4 != null) {
buf.append(",");
}
if (k4 != null) {
buf.append(k4.toString());
}
if (k4 != null || k5 != null) {
buf.append(",");
}
if (k5 != null) {
buf.append(k5.toString());
}
if (k5 != null || k6 != null) {
buf.append(",");
}
if (k6 != null) {
buf.append(k6.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -convolve to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps convolve(Integer k1, Integer k2, Integer k3, Integer k4, Integer k5, Integer k6, Integer k7) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-convolve");
if (k1 != null) {
buf.append(k1.toString());
}
if (k1 != null || k2 != null) {
buf.append(",");
}
if (k2 != null) {
buf.append(k2.toString());
}
if (k2 != null || k3 != null) {
buf.append(",");
}
if (k3 != null) {
buf.append(k3.toString());
}
if (k3 != null || k4 != null) {
buf.append(",");
}
if (k4 != null) {
buf.append(k4.toString());
}
if (k4 != null || k5 != null) {
buf.append(",");
}
if (k5 != null) {
buf.append(k5.toString());
}
if (k5 != null || k6 != null) {
buf.append(",");
}
if (k6 != null) {
buf.append(k6.toString());
}
if (k6 != null || k7 != null) {
buf.append(",");
}
if (k7 != null) {
buf.append(k7.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -convolve to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps convolve(Integer k1, Integer k2, Integer k3, Integer k4, Integer k5, Integer k6, Integer k7, Integer k8) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-convolve");
if (k1 != null) {
buf.append(k1.toString());
}
if (k1 != null || k2 != null) {
buf.append(",");
}
if (k2 != null) {
buf.append(k2.toString());
}
if (k2 != null || k3 != null) {
buf.append(",");
}
if (k3 != null) {
buf.append(k3.toString());
}
if (k3 != null || k4 != null) {
buf.append(",");
}
if (k4 != null) {
buf.append(k4.toString());
}
if (k4 != null || k5 != null) {
buf.append(",");
}
if (k5 != null) {
buf.append(k5.toString());
}
if (k5 != null || k6 != null) {
buf.append(",");
}
if (k6 != null) {
buf.append(k6.toString());
}
if (k6 != null || k7 != null) {
buf.append(",");
}
if (k7 != null) {
buf.append(k7.toString());
}
if (k7 != null || k8 != null) {
buf.append(",");
}
if (k8 != null) {
buf.append(k8.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -convolve to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps convolve(Integer k1, Integer k2, Integer k3, Integer k4, Integer k5, Integer k6, Integer k7, Integer k8, Integer k9) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-convolve");
if (k1 != null) {
buf.append(k1.toString());
}
if (k1 != null || k2 != null) {
buf.append(",");
}
if (k2 != null) {
buf.append(k2.toString());
}
if (k2 != null || k3 != null) {
buf.append(",");
}
if (k3 != null) {
buf.append(k3.toString());
}
if (k3 != null || k4 != null) {
buf.append(",");
}
if (k4 != null) {
buf.append(k4.toString());
}
if (k4 != null || k5 != null) {
buf.append(",");
}
if (k5 != null) {
buf.append(k5.toString());
}
if (k5 != null || k6 != null) {
buf.append(",");
}
if (k6 != null) {
buf.append(k6.toString());
}
if (k6 != null || k7 != null) {
buf.append(",");
}
if (k7 != null) {
buf.append(k7.toString());
}
if (k7 != null || k8 != null) {
buf.append(",");
}
if (k8 != null) {
buf.append(k8.toString());
}
if (k8 != null || k9 != null) {
buf.append(",");
}
if (k9 != null) {
buf.append(k9.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -convolve to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps convolve(Integer k1, Integer k2, Integer k3, Integer k4, Integer k5, Integer k6, Integer k7, Integer k8, Integer k9, Integer k10) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-convolve");
if (k1 != null) {
buf.append(k1.toString());
}
if (k1 != null || k2 != null) {
buf.append(",");
}
if (k2 != null) {
buf.append(k2.toString());
}
if (k2 != null || k3 != null) {
buf.append(",");
}
if (k3 != null) {
buf.append(k3.toString());
}
if (k3 != null || k4 != null) {
buf.append(",");
}
if (k4 != null) {
buf.append(k4.toString());
}
if (k4 != null || k5 != null) {
buf.append(",");
}
if (k5 != null) {
buf.append(k5.toString());
}
if (k5 != null || k6 != null) {
buf.append(",");
}
if (k6 != null) {
buf.append(k6.toString());
}
if (k6 != null || k7 != null) {
buf.append(",");
}
if (k7 != null) {
buf.append(k7.toString());
}
if (k7 != null || k8 != null) {
buf.append(",");
}
if (k8 != null) {
buf.append(k8.toString());
}
if (k8 != null || k9 != null) {
buf.append(",");
}
if (k9 != null) {
buf.append(k9.toString());
}
if (k9 != null || k10 != null) {
buf.append(",");
}
if (k10 != null) {
buf.append(k10.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -convolve to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps convolve(Integer k1, Integer k2, Integer k3, Integer k4, Integer k5, Integer k6, Integer k7, Integer k8, Integer k9, Integer k10, Integer k11) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-convolve");
if (k1 != null) {
buf.append(k1.toString());
}
if (k1 != null || k2 != null) {
buf.append(",");
}
if (k2 != null) {
buf.append(k2.toString());
}
if (k2 != null || k3 != null) {
buf.append(",");
}
if (k3 != null) {
buf.append(k3.toString());
}
if (k3 != null || k4 != null) {
buf.append(",");
}
if (k4 != null) {
buf.append(k4.toString());
}
if (k4 != null || k5 != null) {
buf.append(",");
}
if (k5 != null) {
buf.append(k5.toString());
}
if (k5 != null || k6 != null) {
buf.append(",");
}
if (k6 != null) {
buf.append(k6.toString());
}
if (k6 != null || k7 != null) {
buf.append(",");
}
if (k7 != null) {
buf.append(k7.toString());
}
if (k7 != null || k8 != null) {
buf.append(",");
}
if (k8 != null) {
buf.append(k8.toString());
}
if (k8 != null || k9 != null) {
buf.append(",");
}
if (k9 != null) {
buf.append(k9.toString());
}
if (k9 != null || k10 != null) {
buf.append(",");
}
if (k10 != null) {
buf.append(k10.toString());
}
if (k10 != null || k11 != null) {
buf.append(",");
}
if (k11 != null) {
buf.append(k11.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -convolve to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps convolve(Integer k1, Integer k2, Integer k3, Integer k4, Integer k5, Integer k6, Integer k7, Integer k8, Integer k9, Integer k10, Integer k11, Integer k12) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-convolve");
if (k1 != null) {
buf.append(k1.toString());
}
if (k1 != null || k2 != null) {
buf.append(",");
}
if (k2 != null) {
buf.append(k2.toString());
}
if (k2 != null || k3 != null) {
buf.append(",");
}
if (k3 != null) {
buf.append(k3.toString());
}
if (k3 != null || k4 != null) {
buf.append(",");
}
if (k4 != null) {
buf.append(k4.toString());
}
if (k4 != null || k5 != null) {
buf.append(",");
}
if (k5 != null) {
buf.append(k5.toString());
}
if (k5 != null || k6 != null) {
buf.append(",");
}
if (k6 != null) {
buf.append(k6.toString());
}
if (k6 != null || k7 != null) {
buf.append(",");
}
if (k7 != null) {
buf.append(k7.toString());
}
if (k7 != null || k8 != null) {
buf.append(",");
}
if (k8 != null) {
buf.append(k8.toString());
}
if (k8 != null || k9 != null) {
buf.append(",");
}
if (k9 != null) {
buf.append(k9.toString());
}
if (k9 != null || k10 != null) {
buf.append(",");
}
if (k10 != null) {
buf.append(k10.toString());
}
if (k10 != null || k11 != null) {
buf.append(",");
}
if (k11 != null) {
buf.append(k11.toString());
}
if (k11 != null || k12 != null) {
buf.append(",");
}
if (k12 != null) {
buf.append(k12.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -convolve to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps convolve(Integer k1, Integer k2, Integer k3, Integer k4, Integer k5, Integer k6, Integer k7, Integer k8, Integer k9, Integer k10, Integer k11, Integer k12, Integer k13) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-convolve");
if (k1 != null) {
buf.append(k1.toString());
}
if (k1 != null || k2 != null) {
buf.append(",");
}
if (k2 != null) {
buf.append(k2.toString());
}
if (k2 != null || k3 != null) {
buf.append(",");
}
if (k3 != null) {
buf.append(k3.toString());
}
if (k3 != null || k4 != null) {
buf.append(",");
}
if (k4 != null) {
buf.append(k4.toString());
}
if (k4 != null || k5 != null) {
buf.append(",");
}
if (k5 != null) {
buf.append(k5.toString());
}
if (k5 != null || k6 != null) {
buf.append(",");
}
if (k6 != null) {
buf.append(k6.toString());
}
if (k6 != null || k7 != null) {
buf.append(",");
}
if (k7 != null) {
buf.append(k7.toString());
}
if (k7 != null || k8 != null) {
buf.append(",");
}
if (k8 != null) {
buf.append(k8.toString());
}
if (k8 != null || k9 != null) {
buf.append(",");
}
if (k9 != null) {
buf.append(k9.toString());
}
if (k9 != null || k10 != null) {
buf.append(",");
}
if (k10 != null) {
buf.append(k10.toString());
}
if (k10 != null || k11 != null) {
buf.append(",");
}
if (k11 != null) {
buf.append(k11.toString());
}
if (k11 != null || k12 != null) {
buf.append(",");
}
if (k12 != null) {
buf.append(k12.toString());
}
if (k12 != null || k13 != null) {
buf.append(",");
}
if (k13 != null) {
buf.append(k13.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -convolve to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps convolve(Integer k1, Integer k2, Integer k3, Integer k4, Integer k5, Integer k6, Integer k7, Integer k8, Integer k9, Integer k10, Integer k11, Integer k12, Integer k13, Integer k14) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-convolve");
if (k1 != null) {
buf.append(k1.toString());
}
if (k1 != null || k2 != null) {
buf.append(",");
}
if (k2 != null) {
buf.append(k2.toString());
}
if (k2 != null || k3 != null) {
buf.append(",");
}
if (k3 != null) {
buf.append(k3.toString());
}
if (k3 != null || k4 != null) {
buf.append(",");
}
if (k4 != null) {
buf.append(k4.toString());
}
if (k4 != null || k5 != null) {
buf.append(",");
}
if (k5 != null) {
buf.append(k5.toString());
}
if (k5 != null || k6 != null) {
buf.append(",");
}
if (k6 != null) {
buf.append(k6.toString());
}
if (k6 != null || k7 != null) {
buf.append(",");
}
if (k7 != null) {
buf.append(k7.toString());
}
if (k7 != null || k8 != null) {
buf.append(",");
}
if (k8 != null) {
buf.append(k8.toString());
}
if (k8 != null || k9 != null) {
buf.append(",");
}
if (k9 != null) {
buf.append(k9.toString());
}
if (k9 != null || k10 != null) {
buf.append(",");
}
if (k10 != null) {
buf.append(k10.toString());
}
if (k10 != null || k11 != null) {
buf.append(",");
}
if (k11 != null) {
buf.append(k11.toString());
}
if (k11 != null || k12 != null) {
buf.append(",");
}
if (k12 != null) {
buf.append(k12.toString());
}
if (k12 != null || k13 != null) {
buf.append(",");
}
if (k13 != null) {
buf.append(k13.toString());
}
if (k13 != null || k14 != null) {
buf.append(",");
}
if (k14 != null) {
buf.append(k14.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -convolve to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps convolve(Integer k1, Integer k2, Integer k3, Integer k4, Integer k5, Integer k6, Integer k7, Integer k8, Integer k9, Integer k10, Integer k11, Integer k12, Integer k13, Integer k14, Integer k15) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-convolve");
if (k1 != null) {
buf.append(k1.toString());
}
if (k1 != null || k2 != null) {
buf.append(",");
}
if (k2 != null) {
buf.append(k2.toString());
}
if (k2 != null || k3 != null) {
buf.append(",");
}
if (k3 != null) {
buf.append(k3.toString());
}
if (k3 != null || k4 != null) {
buf.append(",");
}
if (k4 != null) {
buf.append(k4.toString());
}
if (k4 != null || k5 != null) {
buf.append(",");
}
if (k5 != null) {
buf.append(k5.toString());
}
if (k5 != null || k6 != null) {
buf.append(",");
}
if (k6 != null) {
buf.append(k6.toString());
}
if (k6 != null || k7 != null) {
buf.append(",");
}
if (k7 != null) {
buf.append(k7.toString());
}
if (k7 != null || k8 != null) {
buf.append(",");
}
if (k8 != null) {
buf.append(k8.toString());
}
if (k8 != null || k9 != null) {
buf.append(",");
}
if (k9 != null) {
buf.append(k9.toString());
}
if (k9 != null || k10 != null) {
buf.append(",");
}
if (k10 != null) {
buf.append(k10.toString());
}
if (k10 != null || k11 != null) {
buf.append(",");
}
if (k11 != null) {
buf.append(k11.toString());
}
if (k11 != null || k12 != null) {
buf.append(",");
}
if (k12 != null) {
buf.append(k12.toString());
}
if (k12 != null || k13 != null) {
buf.append(",");
}
if (k13 != null) {
buf.append(k13.toString());
}
if (k13 != null || k14 != null) {
buf.append(",");
}
if (k14 != null) {
buf.append(k14.toString());
}
if (k14 != null || k15 != null) {
buf.append(",");
}
if (k15 != null) {
buf.append(k15.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -convolve to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps convolve(Integer k1, Integer k2, Integer k3, Integer k4, Integer k5, Integer k6, Integer k7, Integer k8, Integer k9, Integer k10, Integer k11, Integer k12, Integer k13, Integer k14, Integer k15, Integer k16) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-convolve");
if (k1 != null) {
buf.append(k1.toString());
}
if (k1 != null || k2 != null) {
buf.append(",");
}
if (k2 != null) {
buf.append(k2.toString());
}
if (k2 != null || k3 != null) {
buf.append(",");
}
if (k3 != null) {
buf.append(k3.toString());
}
if (k3 != null || k4 != null) {
buf.append(",");
}
if (k4 != null) {
buf.append(k4.toString());
}
if (k4 != null || k5 != null) {
buf.append(",");
}
if (k5 != null) {
buf.append(k5.toString());
}
if (k5 != null || k6 != null) {
buf.append(",");
}
if (k6 != null) {
buf.append(k6.toString());
}
if (k6 != null || k7 != null) {
buf.append(",");
}
if (k7 != null) {
buf.append(k7.toString());
}
if (k7 != null || k8 != null) {
buf.append(",");
}
if (k8 != null) {
buf.append(k8.toString());
}
if (k8 != null || k9 != null) {
buf.append(",");
}
if (k9 != null) {
buf.append(k9.toString());
}
if (k9 != null || k10 != null) {
buf.append(",");
}
if (k10 != null) {
buf.append(k10.toString());
}
if (k10 != null || k11 != null) {
buf.append(",");
}
if (k11 != null) {
buf.append(k11.toString());
}
if (k11 != null || k12 != null) {
buf.append(",");
}
if (k12 != null) {
buf.append(k12.toString());
}
if (k12 != null || k13 != null) {
buf.append(",");
}
if (k13 != null) {
buf.append(k13.toString());
}
if (k13 != null || k14 != null) {
buf.append(",");
}
if (k14 != null) {
buf.append(k14.toString());
}
if (k14 != null || k15 != null) {
buf.append(",");
}
if (k15 != null) {
buf.append(k15.toString());
}
if (k15 != null || k16 != null) {
buf.append(",");
}
if (k16 != null) {
buf.append(k16.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -crop to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps crop() {
iCmdArgs.add("-crop");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -crop to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps crop(Integer width) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-crop");
if (width != null) {
buf.append(width.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -crop to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps crop(Integer width, Integer height) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-crop");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -crop to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps crop(Integer width, Integer height, Integer x) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-crop");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || x != null) {
oper="+";
if (x.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (x != null) {
buf.append(x.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -crop to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps crop(Integer width, Integer height, Integer x, Integer y) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-crop");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || x != null) {
oper="+";
if (x.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (x != null) {
buf.append(x.toString());
}
if (x != null || y != null) {
oper="+";
if (y.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (y != null) {
buf.append(y.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -crop to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps crop(Integer width, Integer height, Integer x, Integer y, Character special) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-crop");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || x != null) {
oper="+";
if (x.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (x != null) {
buf.append(x.toString());
}
if (x != null || y != null) {
oper="+";
if (y.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (y != null) {
buf.append(y.toString());
}
if (y != null || special != null) {
}
if (special != null) {
buf.append(special.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -crop to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps crop(Integer width, Integer height, Integer x, Integer y, String special) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-crop");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || x != null) {
oper="+";
if (x.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (x != null) {
buf.append(x.toString());
}
if (x != null || y != null) {
oper="+";
if (y.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (y != null) {
buf.append(y.toString());
}
if (y != null || special != null) {
}
if (special != null) {
buf.append(special.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -cycle to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps cycle() {
iCmdArgs.add("-cycle");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -cycle to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps cycle(Integer amount) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-cycle");
if (amount != null) {
buf.append(amount.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +debug to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_debug() {
iCmdArgs.add("+debug");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -debug to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps debug() {
iCmdArgs.add("-debug");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -debug to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps debug(String events) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-debug");
if (events != null) {
buf.append(events.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -decipher to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps decipher() {
iCmdArgs.add("-decipher");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -decipher to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps decipher(String filename) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-decipher");
if (filename != null) {
buf.append(filename.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -deconstruct to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps deconstruct() {
iCmdArgs.add("-deconstruct");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +define to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_define() {
iCmdArgs.add("+define");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +define to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_define(String key) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("+define");
if (key != null) {
buf.append(key.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -define to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps define() {
iCmdArgs.add("-define");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -define to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps define(String keyValue) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-define");
if (keyValue != null) {
buf.append(keyValue.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -delay to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps delay() {
iCmdArgs.add("-delay");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -delay to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps delay(Integer ticks) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-delay");
if (ticks != null) {
buf.append(ticks.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -delay to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps delay(Integer ticks, Integer ticksPerSecond) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-delay");
if (ticks != null) {
buf.append(ticks.toString());
}
if (ticks != null || ticksPerSecond != null) {
buf.append("x");
}
if (ticksPerSecond != null) {
buf.append(ticksPerSecond.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -delay to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps delay(Integer ticks, Integer ticksPerSecond, Character special) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-delay");
if (ticks != null) {
buf.append(ticks.toString());
}
if (ticks != null || ticksPerSecond != null) {
buf.append("x");
}
if (ticksPerSecond != null) {
buf.append(ticksPerSecond.toString());
}
if (ticksPerSecond != null || special != null) {
}
if (special != null) {
buf.append(special.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +delete to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_delete() {
iCmdArgs.add("+delete");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -delete to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps delete() {
iCmdArgs.add("-delete");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -delete to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps delete(Integer index1) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-delete");
if (index1 != null) {
buf.append(index1.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -delete to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps delete(Integer index1, Integer index2) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-delete");
if (index1 != null) {
buf.append(index1.toString());
}
if (index1 != null || index2 != null) {
buf.append(",");
}
if (index2 != null) {
buf.append(index2.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -delete to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps delete(Integer index1, Integer index2, Integer index3) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-delete");
if (index1 != null) {
buf.append(index1.toString());
}
if (index1 != null || index2 != null) {
buf.append(",");
}
if (index2 != null) {
buf.append(index2.toString());
}
if (index2 != null || index3 != null) {
buf.append(",");
}
if (index3 != null) {
buf.append(index3.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -delete to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps delete(String indexes) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-delete");
if (indexes != null) {
buf.append(indexes.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -density to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps density() {
iCmdArgs.add("-density");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -density to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps density(Integer width) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-density");
if (width != null) {
buf.append(width.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -density to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps density(Integer width, Integer height) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-density");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -depth to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps depth() {
iCmdArgs.add("-depth");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -depth to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps depth(Integer value) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-depth");
if (value != null) {
buf.append(value.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -descend to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps descend() {
iCmdArgs.add("-descend");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -deskew to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps deskew() {
iCmdArgs.add("-deskew");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -deskew to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps deskew(Double threshold) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-deskew");
if (threshold != null) {
buf.append(threshold.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -despeckle to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps despeckle() {
iCmdArgs.add("-despeckle");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -direction to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps direction() {
iCmdArgs.add("-direction");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -direction to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps direction(String type) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-direction");
if (type != null) {
buf.append(type.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -displace to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps displace() {
iCmdArgs.add("-displace");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -displace to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps displace(Double horizontalScale) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-displace");
if (horizontalScale != null) {
buf.append(horizontalScale.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -displace to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps displace(Double horizontalScale, Double verticalScale) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-displace");
if (horizontalScale != null) {
buf.append(horizontalScale.toString());
}
if (horizontalScale != null || verticalScale != null) {
buf.append("x");
}
if (verticalScale != null) {
buf.append(verticalScale.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -display to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps display() {
iCmdArgs.add("-display");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -display to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps display(String host) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-display");
if (host != null) {
buf.append(host.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -display to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps display(String host, Integer display) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-display");
if (host != null) {
buf.append(host.toString());
}
if (host != null || display != null) {
buf.append(":");
}
if (display != null) {
buf.append(display.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -display to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps display(String host, Integer display, Integer screen) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-display");
if (host != null) {
buf.append(host.toString());
}
if (host != null || display != null) {
buf.append(":");
}
if (display != null) {
buf.append(display.toString());
}
if (display != null || screen != null) {
buf.append(".");
}
if (screen != null) {
buf.append(screen.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +dispose to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_dispose() {
iCmdArgs.add("+dispose");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -dispose to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps dispose() {
iCmdArgs.add("-dispose");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -dispose to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps dispose(String method) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-dispose");
if (method != null) {
buf.append(method.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -dissimilarity-threshold to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps dissimilarityThreshold() {
iCmdArgs.add("-dissimilarity-threshold");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -dissimilarity-threshold to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps dissimilarityThreshold(Double value) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-dissimilarity-threshold");
if (value != null) {
buf.append(value.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -dissolve to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps dissolve() {
iCmdArgs.add("-dissolve");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -dissolve to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps dissolve(Integer percent) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-dissolve");
if (percent != null) {
buf.append(percent.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -distort to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps distort() {
iCmdArgs.add("-distort");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -distort to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps distort(String method) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-distort");
if (method != null) {
buf.append(method.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -distort to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps distort(String method, String arguments) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-distort");
if (method != null) {
buf.append(method.toString());
}
if (method != null || arguments != null) {
iCmdArgs.add(buf.toString());
buf.setLength(0);
}
if (arguments != null) {
buf.append(arguments.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +distort to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_distort() {
iCmdArgs.add("+distort");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +distort to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_distort(String method) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("+distort");
if (method != null) {
buf.append(method.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +distort to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_distort(String method, String arguments) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("+distort");
if (method != null) {
buf.append(method.toString());
}
if (method != null || arguments != null) {
iCmdArgs.add(buf.toString());
buf.setLength(0);
}
if (arguments != null) {
buf.append(arguments.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +dither to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_dither() {
iCmdArgs.add("+dither");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -dither to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps dither() {
iCmdArgs.add("-dither");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -dither to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps dither(String method) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-dither");
if (method != null) {
buf.append(method.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -draw to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps draw() {
iCmdArgs.add("-draw");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -draw to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps draw(String string) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-draw");
if (string != null) {
buf.append(string.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -duplicate to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps duplicate() {
iCmdArgs.add("-duplicate");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -duplicate to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps duplicate(Integer count) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-duplicate");
if (count != null) {
buf.append(count.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -duplicate to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps duplicate(Integer count, String indices) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-duplicate");
if (count != null) {
buf.append(count.toString());
}
if (count != null || indices != null) {
buf.append(",");
}
if (indices != null) {
buf.append(indices.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +duplicate to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_duplicate() {
iCmdArgs.add("+duplicate");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -edge to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps edge() {
iCmdArgs.add("-edge");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -edge to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps edge(Double radius) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-edge");
if (radius != null) {
buf.append(radius.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -emboss to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps emboss() {
iCmdArgs.add("-emboss");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -emboss to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps emboss(Double radius) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-emboss");
if (radius != null) {
buf.append(radius.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -encipher to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps encipher() {
iCmdArgs.add("-encipher");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -encipher to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps encipher(String filename) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-encipher");
if (filename != null) {
buf.append(filename.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -encoding to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps encoding() {
iCmdArgs.add("-encoding");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -encoding to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps encoding(String type) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-encoding");
if (type != null) {
buf.append(type.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +endian to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_endian() {
iCmdArgs.add("+endian");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -endian to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps endian() {
iCmdArgs.add("-endian");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -endian to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps endian(String type) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-endian");
if (type != null) {
buf.append(type.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -enhance to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps enhance() {
iCmdArgs.add("-enhance");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -equalize to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps equalize() {
iCmdArgs.add("-equalize");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -evaluate to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps evaluate() {
iCmdArgs.add("-evaluate");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -evaluate to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps evaluate(String operator) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-evaluate");
if (operator != null) {
buf.append(operator.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -evaluate to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps evaluate(String operator, String constant) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-evaluate");
if (operator != null) {
buf.append(operator.toString());
}
if (operator != null || constant != null) {
iCmdArgs.add(buf.toString());
buf.setLength(0);
}
if (constant != null) {
buf.append(constant.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -evaluate-sequence to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps evaluateSequence() {
iCmdArgs.add("-evaluate-sequence");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -evaluate-sequence to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps evaluateSequence(String operator) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-evaluate-sequence");
if (operator != null) {
buf.append(operator.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -extent to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps extent() {
iCmdArgs.add("-extent");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -extent to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps extent(Integer width) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-extent");
if (width != null) {
buf.append(width.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -extent to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps extent(Integer width, Integer height) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-extent");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -extent to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps extent(Integer width, Integer height, Integer xOffset) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-extent");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || xOffset != null) {
oper="+";
if (xOffset.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (xOffset != null) {
buf.append(xOffset.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -extent to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps extent(Integer width, Integer height, Integer xOffset, Integer yOffset) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-extent");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || xOffset != null) {
oper="+";
if (xOffset.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (xOffset != null) {
buf.append(xOffset.toString());
}
if (xOffset != null || yOffset != null) {
oper="+";
if (yOffset.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (yOffset != null) {
buf.append(yOffset.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -extract to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps extract() {
iCmdArgs.add("-extract");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -extract to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps extract(Integer width) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-extract");
if (width != null) {
buf.append(width.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -extract to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps extract(Integer width, Integer height) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-extract");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -extract to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps extract(Integer width, Integer height, Integer xOffset) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-extract");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || xOffset != null) {
oper="+";
if (xOffset.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (xOffset != null) {
buf.append(xOffset.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -extract to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps extract(Integer width, Integer height, Integer xOffset, Integer yOffset) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-extract");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || xOffset != null) {
oper="+";
if (xOffset.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (xOffset != null) {
buf.append(xOffset.toString());
}
if (xOffset != null || yOffset != null) {
oper="+";
if (yOffset.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (yOffset != null) {
buf.append(yOffset.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -family to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps family() {
iCmdArgs.add("-family");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -family to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps family(String fontFamily) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-family");
if (fontFamily != null) {
buf.append(fontFamily.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -features to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps features() {
iCmdArgs.add("-features");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -features to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps features(String distance) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-features");
if (distance != null) {
buf.append(distance.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -fft to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps fft() {
iCmdArgs.add("-fft");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -fill to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps fill() {
iCmdArgs.add("-fill");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -fill to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps fill(String color) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-fill");
if (color != null) {
buf.append(color.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -filter to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps filter() {
iCmdArgs.add("-filter");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -filter to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps filter(String type) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-filter");
if (type != null) {
buf.append(type.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -flatten to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps flatten() {
iCmdArgs.add("-flatten");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -flip to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps flip() {
iCmdArgs.add("-flip");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -floodfill to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps floodfill() {
iCmdArgs.add("-floodfill");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -floodfill to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps floodfill(Integer x) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-floodfill");
oper="+";
if (x.doubleValue() < 0)
oper="";
buf.append(oper);
if (x != null) {
buf.append(x.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -floodfill to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps floodfill(Integer x, Integer y) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-floodfill");
oper="+";
if (x.doubleValue() < 0)
oper="";
buf.append(oper);
if (x != null) {
buf.append(x.toString());
}
if (x != null || y != null) {
oper="+";
if (y.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (y != null) {
buf.append(y.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -floodfill to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps floodfill(Integer x, Integer y, String color) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-floodfill");
oper="+";
if (x.doubleValue() < 0)
oper="";
buf.append(oper);
if (x != null) {
buf.append(x.toString());
}
if (x != null || y != null) {
oper="+";
if (y.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (y != null) {
buf.append(y.toString());
}
if (y != null || color != null) {
iCmdArgs.add(buf.toString());
buf.setLength(0);
}
if (color != null) {
buf.append(color.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -flop to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps flop() {
iCmdArgs.add("-flop");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -font to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps font() {
iCmdArgs.add("-font");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -font to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps font(String name) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-font");
if (name != null) {
buf.append(name.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -foreground to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps foreground() {
iCmdArgs.add("-foreground");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -foreground to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps foreground(String color) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-foreground");
if (color != null) {
buf.append(color.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -format to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps format() {
iCmdArgs.add("-format");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -format to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps format(String type) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-format");
if (type != null) {
buf.append(type.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -frame to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps frame() {
iCmdArgs.add("-frame");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -frame to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps frame(Integer width) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-frame");
if (width != null) {
buf.append(width.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -frame to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps frame(Integer width, Integer height) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-frame");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -frame to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps frame(Integer width, Integer height, Integer outerBevelWidth) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-frame");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || outerBevelWidth != null) {
oper="+";
if (outerBevelWidth.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (outerBevelWidth != null) {
buf.append(outerBevelWidth.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -frame to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps frame(Integer width, Integer height, Integer outerBevelWidth, Integer innerBevelWidth) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-frame");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || outerBevelWidth != null) {
oper="+";
if (outerBevelWidth.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (outerBevelWidth != null) {
buf.append(outerBevelWidth.toString());
}
if (outerBevelWidth != null || innerBevelWidth != null) {
oper="+";
if (innerBevelWidth.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (innerBevelWidth != null) {
buf.append(innerBevelWidth.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -function to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps function() {
iCmdArgs.add("-function");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -function to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps function(String name) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-function");
if (name != null) {
buf.append(name.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -function to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps function(String name, String parameter) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-function");
if (name != null) {
buf.append(name.toString());
}
if (name != null || parameter != null) {
iCmdArgs.add(buf.toString());
buf.setLength(0);
}
if (parameter != null) {
buf.append(parameter.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -fuzz to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps fuzz() {
iCmdArgs.add("-fuzz");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -fuzz to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps fuzz(Double distance) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-fuzz");
if (distance != null) {
buf.append(distance.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -fuzz to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps fuzz(Double distance, Boolean percent) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-fuzz");
if (distance != null) {
buf.append(distance.toString());
}
if (distance != null || percent != null) {
}
if (percent != null) {
if (percent.booleanValue())
buf.append("%");
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -fx to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps fx() {
iCmdArgs.add("-fx");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -fx to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps fx(String expression) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-fx");
if (expression != null) {
buf.append(expression.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -gamma to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps gamma() {
iCmdArgs.add("-gamma");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -gamma to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps gamma(Double value) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-gamma");
if (value != null) {
buf.append(value.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +gamma to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_gamma() {
iCmdArgs.add("+gamma");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +gamma to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_gamma(Double value) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("+gamma");
if (value != null) {
buf.append(value.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -gaussian-blur to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps gaussianBlur() {
iCmdArgs.add("-gaussian-blur");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -gaussian-blur to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps gaussianBlur(Double radius) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-gaussian-blur");
if (radius != null) {
buf.append(radius.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -gaussian-blur to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps gaussianBlur(Double radius, Double sigma) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-gaussian-blur");
if (radius != null) {
buf.append(radius.toString());
}
if (radius != null || sigma != null) {
buf.append("x");
}
if (sigma != null) {
buf.append(sigma.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -geometry to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps geometry() {
iCmdArgs.add("-geometry");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -geometry to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps geometry(Integer width) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-geometry");
if (width != null) {
buf.append(width.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -geometry to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps geometry(Integer width, Integer height) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-geometry");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -geometry to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps geometry(Integer width, Integer height, Integer x) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-geometry");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || x != null) {
oper="+";
if (x.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (x != null) {
buf.append(x.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -geometry to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps geometry(Integer width, Integer height, Integer x, Integer y) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-geometry");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || x != null) {
oper="+";
if (x.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (x != null) {
buf.append(x.toString());
}
if (x != null || y != null) {
oper="+";
if (y.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (y != null) {
buf.append(y.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -gravity to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps gravity() {
iCmdArgs.add("-gravity");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -gravity to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps gravity(String type) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-gravity");
if (type != null) {
buf.append(type.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -green-primary to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps greenPrimary() {
iCmdArgs.add("-green-primary");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -green-primary to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps greenPrimary(Double x) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-green-primary");
if (x != null) {
buf.append(x.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -green-primary to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps greenPrimary(Double x, Double y) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-green-primary");
if (x != null) {
buf.append(x.toString());
}
if (x != null || y != null) {
buf.append(",");
}
if (y != null) {
buf.append(y.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -help to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps help() {
iCmdArgs.add("-help");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -hald-clut to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps haldClut() {
iCmdArgs.add("-hald-clut");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -highlight-color to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps highlightColor() {
iCmdArgs.add("-highlight-color");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -highlight-color to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps highlightColor(String color) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-highlight-color");
if (color != null) {
buf.append(color.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -iconGeometry to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps iconGeometry() {
iCmdArgs.add("-iconGeometry");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -iconGeometry to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps iconGeometry(Integer width) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-iconGeometry");
if (width != null) {
buf.append(width.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -iconGeometry to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps iconGeometry(Integer width, Integer height) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-iconGeometry");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -iconGeometry to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps iconGeometry(Integer width, Integer height, Integer xOffset) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-iconGeometry");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || xOffset != null) {
oper="+";
if (xOffset.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (xOffset != null) {
buf.append(xOffset.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -iconGeometry to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps iconGeometry(Integer width, Integer height, Integer xOffset, Integer yOffset) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-iconGeometry");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || xOffset != null) {
oper="+";
if (xOffset.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (xOffset != null) {
buf.append(xOffset.toString());
}
if (xOffset != null || yOffset != null) {
oper="+";
if (yOffset.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (yOffset != null) {
buf.append(yOffset.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -iconic to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps iconic() {
iCmdArgs.add("-iconic");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -identify to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps identify() {
iCmdArgs.add("-identify");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -ift to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps ift() {
iCmdArgs.add("-ift");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -immutable to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps immutable() {
iCmdArgs.add("-immutable");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -implode to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps implode() {
iCmdArgs.add("-implode");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -implode to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps implode(Double factor) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-implode");
if (factor != null) {
buf.append(factor.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -insert to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps insert() {
iCmdArgs.add("-insert");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -insert to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps insert(Integer index) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-insert");
if (index != null) {
buf.append(index.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -intent to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps intent() {
iCmdArgs.add("-intent");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -intent to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps intent(String type) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-intent");
if (type != null) {
buf.append(type.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -interlace to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps interlace() {
iCmdArgs.add("-interlace");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -interlace to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps interlace(String type) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-interlace");
if (type != null) {
buf.append(type.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -interline-spacing to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps interlineSpacing() {
iCmdArgs.add("-interline-spacing");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -interline-spacing to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps interlineSpacing(Double value) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-interline-spacing");
if (value != null) {
buf.append(value.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -interpolate to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps interpolate() {
iCmdArgs.add("-interpolate");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -interpolate to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps interpolate(String type) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-interpolate");
if (type != null) {
buf.append(type.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -interword-spacing to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps interwordSpacing() {
iCmdArgs.add("-interword-spacing");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -interword-spacing to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps interwordSpacing(Double value) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-interword-spacing");
if (value != null) {
buf.append(value.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -kerning to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps kerning() {
iCmdArgs.add("-kerning");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -kerning to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps kerning(Integer value) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-kerning");
if (value != null) {
buf.append(value.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +label to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_label() {
iCmdArgs.add("+label");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -label to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps label() {
iCmdArgs.add("-label");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -label to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps label(String name) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-label");
if (name != null) {
buf.append(name.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -lat to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps lat() {
iCmdArgs.add("-lat");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -lat to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps lat(Integer width) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-lat");
if (width != null) {
buf.append(width.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -lat to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps lat(Integer width, Integer height) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-lat");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -lat to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps lat(Integer width, Integer height, Integer offset) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-lat");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || offset != null) {
oper="+";
if (offset.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (offset != null) {
buf.append(offset.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -lat to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps lat(Integer width, Integer height, Integer offset, Boolean percent) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-lat");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || offset != null) {
oper="+";
if (offset.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (offset != null) {
buf.append(offset.toString());
}
if (offset != null || percent != null) {
}
if (percent != null) {
if (percent.booleanValue())
buf.append("%");
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -layers to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps layers() {
iCmdArgs.add("-layers");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -layers to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps layers(String method) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-layers");
if (method != null) {
buf.append(method.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -level-colors to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps levelColors() {
iCmdArgs.add("-level-colors");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -level-colors to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps levelColors(String black_color) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-level-colors");
if (black_color != null) {
buf.append(black_color.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -level-colors to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps levelColors(String black_color, String white_color) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-level-colors");
if (black_color != null) {
buf.append(black_color.toString());
}
if (black_color != null || white_color != null) {
buf.append(",");
}
if (white_color != null) {
buf.append(white_color.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +level-colors to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_levelColors() {
iCmdArgs.add("+level-colors");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +level-colors to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_levelColors(String black_color) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("+level-colors");
if (black_color != null) {
buf.append(black_color.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +level-colors to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_levelColors(String black_color, String white_color) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("+level-colors");
if (black_color != null) {
buf.append(black_color.toString());
}
if (black_color != null || white_color != null) {
buf.append(",");
}
if (white_color != null) {
buf.append(white_color.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -level to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps level() {
iCmdArgs.add("-level");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -level to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps level(Double black_point) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-level");
if (black_point != null) {
buf.append(black_point.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -level to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps level(Double black_point, Double white_point) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-level");
if (black_point != null) {
buf.append(black_point.toString());
}
if (black_point != null || white_point != null) {
buf.append(",");
}
if (white_point != null) {
buf.append(white_point.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -level to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps level(Double black_point, Double white_point, Boolean percent) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-level");
if (black_point != null) {
buf.append(black_point.toString());
}
if (black_point != null || white_point != null) {
buf.append(",");
}
if (white_point != null) {
buf.append(white_point.toString());
}
if (white_point != null || percent != null) {
}
if (percent != null) {
if (percent.booleanValue())
buf.append("%");
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -level to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps level(Double black_point, Double white_point, Boolean percent, Double gamma) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-level");
if (black_point != null) {
buf.append(black_point.toString());
}
if (black_point != null || white_point != null) {
buf.append(",");
}
if (white_point != null) {
buf.append(white_point.toString());
}
if (white_point != null || percent != null) {
}
if (percent != null) {
if (percent.booleanValue())
buf.append("%");
}
if (percent != null || gamma != null) {
buf.append(",");
}
if (gamma != null) {
buf.append(gamma.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +level to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_level() {
iCmdArgs.add("+level");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +level to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_level(Double black_point) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("+level");
if (black_point != null) {
buf.append(black_point.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +level to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_level(Double black_point, Double white_point) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("+level");
if (black_point != null) {
buf.append(black_point.toString());
}
if (black_point != null || white_point != null) {
buf.append(",");
}
if (white_point != null) {
buf.append(white_point.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +level to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_level(Double black_point, Double white_point, Boolean percent) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("+level");
if (black_point != null) {
buf.append(black_point.toString());
}
if (black_point != null || white_point != null) {
buf.append(",");
}
if (white_point != null) {
buf.append(white_point.toString());
}
if (white_point != null || percent != null) {
}
if (percent != null) {
if (percent.booleanValue())
buf.append("%");
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +level to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_level(Double black_point, Double white_point, Boolean percent, Double gamma) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("+level");
if (black_point != null) {
buf.append(black_point.toString());
}
if (black_point != null || white_point != null) {
buf.append(",");
}
if (white_point != null) {
buf.append(white_point.toString());
}
if (white_point != null || percent != null) {
}
if (percent != null) {
if (percent.booleanValue())
buf.append("%");
}
if (percent != null || gamma != null) {
buf.append(",");
}
if (gamma != null) {
buf.append(gamma.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -limit to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps limit() {
iCmdArgs.add("-limit");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -limit to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps limit(String type) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-limit");
if (type != null) {
buf.append(type.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -linear-stretch to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps linearStretch() {
iCmdArgs.add("-linear-stretch");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -linear-stretch to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps linearStretch(Double blackPoint) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-linear-stretch");
if (blackPoint != null) {
buf.append(blackPoint.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -linear-stretch to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps linearStretch(Double blackPoint, Double whitePoint) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-linear-stretch");
if (blackPoint != null) {
buf.append(blackPoint.toString());
}
if (blackPoint != null || whitePoint != null) {
buf.append("x");
}
if (whitePoint != null) {
buf.append(whitePoint.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -linear-stretch to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps linearStretch(Double blackPoint, Double whitePoint, Boolean percent) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-linear-stretch");
if (blackPoint != null) {
buf.append(blackPoint.toString());
}
if (blackPoint != null || whitePoint != null) {
buf.append("x");
}
if (whitePoint != null) {
buf.append(whitePoint.toString());
}
if (whitePoint != null || percent != null) {
}
if (percent != null) {
if (percent.booleanValue())
buf.append("%");
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -linewidth to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps linewidth() {
iCmdArgs.add("-linewidth");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -liquid-rescale to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps liquidRescale() {
iCmdArgs.add("-liquid-rescale");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -liquid-rescale to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps liquidRescale(Integer width) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-liquid-rescale");
if (width != null) {
buf.append(width.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -liquid-rescale to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps liquidRescale(Integer width, Integer height) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-liquid-rescale");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -liquid-rescale to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps liquidRescale(Integer width, Integer height, Integer xOffset) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-liquid-rescale");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || xOffset != null) {
oper="+";
if (xOffset.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (xOffset != null) {
buf.append(xOffset.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -liquid-rescale to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps liquidRescale(Integer width, Integer height, Integer xOffset, Integer yOffset) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-liquid-rescale");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || xOffset != null) {
oper="+";
if (xOffset.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (xOffset != null) {
buf.append(xOffset.toString());
}
if (xOffset != null || yOffset != null) {
oper="+";
if (yOffset.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (yOffset != null) {
buf.append(yOffset.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -list to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps list() {
iCmdArgs.add("-list");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -list to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps list(String type) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-list");
if (type != null) {
buf.append(type.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -log to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps log() {
iCmdArgs.add("-log");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -log to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps log(String text) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-log");
if (text != null) {
buf.append(text.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -loop to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps loop() {
iCmdArgs.add("-loop");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -loop to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps loop(Integer iterations) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-loop");
if (iterations != null) {
buf.append(iterations.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -lowlight-color to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps lowlightColor() {
iCmdArgs.add("-lowlight-color");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -lowlight-color to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps lowlightColor(String color) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-lowlight-color");
if (color != null) {
buf.append(color.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -magnify to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps magnify() {
iCmdArgs.add("-magnify");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -magnify to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps magnify(Double factor) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-magnify");
if (factor != null) {
buf.append(factor.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +map to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_map() {
iCmdArgs.add("+map");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -map to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps map() {
iCmdArgs.add("-map");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -map to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps map(String components) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-map");
if (components != null) {
buf.append(components.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +mask to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_mask() {
iCmdArgs.add("+mask");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -mask to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps mask() {
iCmdArgs.add("-mask");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -mask to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps mask(String filename) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-mask");
if (filename != null) {
buf.append(filename.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -mattecolor to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps mattecolor() {
iCmdArgs.add("-mattecolor");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -mattecolor to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps mattecolor(String color) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-mattecolor");
if (color != null) {
buf.append(color.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -median to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps median() {
iCmdArgs.add("-median");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -median to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps median(Double radius) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-median");
if (radius != null) {
buf.append(radius.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -metric to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps metric() {
iCmdArgs.add("-metric");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -metric to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps metric(String type) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-metric");
if (type != null) {
buf.append(type.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -mode to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps mode() {
iCmdArgs.add("-mode");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -mode to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps mode(String value) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-mode");
if (value != null) {
buf.append(value.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -modulate to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps modulate() {
iCmdArgs.add("-modulate");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -modulate to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps modulate(Double brightness) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-modulate");
if (brightness != null) {
buf.append(brightness.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -modulate to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps modulate(Double brightness, Double saturation) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-modulate");
if (brightness != null) {
buf.append(brightness.toString());
}
if (brightness != null || saturation != null) {
buf.append(",");
}
if (saturation != null) {
buf.append(saturation.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -modulate to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps modulate(Double brightness, Double saturation, Double hue) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-modulate");
if (brightness != null) {
buf.append(brightness.toString());
}
if (brightness != null || saturation != null) {
buf.append(",");
}
if (saturation != null) {
buf.append(saturation.toString());
}
if (saturation != null || hue != null) {
buf.append(",");
}
if (hue != null) {
buf.append(hue.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -monitor to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps monitor() {
iCmdArgs.add("-monitor");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -monochrome to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps monochrome() {
iCmdArgs.add("-monochrome");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -morph to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps morph() {
iCmdArgs.add("-morph");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -morph to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps morph(Integer frames) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-morph");
if (frames != null) {
buf.append(frames.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -morphology to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps morphology() {
iCmdArgs.add("-morphology");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -morphology to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps morphology(String method) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-morphology");
if (method != null) {
buf.append(method.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -morphology to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps morphology(String method, String kernel) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-morphology");
if (method != null) {
buf.append(method.toString());
}
if (method != null || kernel != null) {
iCmdArgs.add(buf.toString());
buf.setLength(0);
}
if (kernel != null) {
buf.append(kernel.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -mosaic to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps mosaic() {
iCmdArgs.add("-mosaic");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -motion-blur to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps motionBlur() {
iCmdArgs.add("-motion-blur");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -motion-blur to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps motionBlur(Double radius) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-motion-blur");
if (radius != null) {
buf.append(radius.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -motion-blur to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps motionBlur(Double radius, Double sigma) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-motion-blur");
if (radius != null) {
buf.append(radius.toString());
}
if (radius != null || sigma != null) {
buf.append("x");
}
if (sigma != null) {
buf.append(sigma.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -motion-blur to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps motionBlur(Double radius, Double sigma, Double angle) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-motion-blur");
if (radius != null) {
buf.append(radius.toString());
}
if (radius != null || sigma != null) {
buf.append("x");
}
if (sigma != null) {
buf.append(sigma.toString());
}
if (sigma != null || angle != null) {
oper="+";
if (angle.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (angle != null) {
buf.append(angle.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -name to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps name() {
iCmdArgs.add("-name");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -negate to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps negate() {
iCmdArgs.add("-negate");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +negate to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_negate() {
iCmdArgs.add("+negate");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -noise to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps noise() {
iCmdArgs.add("-noise");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -noise to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps noise(Double radius) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-noise");
if (radius != null) {
buf.append(radius.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +noise to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_noise() {
iCmdArgs.add("+noise");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +noise to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_noise(String type) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("+noise");
if (type != null) {
buf.append(type.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -normalize to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps normalize() {
iCmdArgs.add("-normalize");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -opaque to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps opaque() {
iCmdArgs.add("-opaque");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -opaque to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps opaque(String color) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-opaque");
if (color != null) {
buf.append(color.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +opaque to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_opaque() {
iCmdArgs.add("+opaque");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +opaque to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_opaque(String color) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("+opaque");
if (color != null) {
buf.append(color.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -ordered-dither to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps orderedDither() {
iCmdArgs.add("-ordered-dither");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -ordered-dither to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps orderedDither(String threshold_map) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-ordered-dither");
if (threshold_map != null) {
buf.append(threshold_map.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -ordered-dither to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps orderedDither(String threshold_map, String level) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-ordered-dither");
if (threshold_map != null) {
buf.append(threshold_map.toString());
}
if (threshold_map != null || level != null) {
buf.append(",");
}
if (level != null) {
buf.append(level.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -orient to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps orient() {
iCmdArgs.add("-orient");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -orient to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps orient(String imageOrientation) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-orient");
if (imageOrientation != null) {
buf.append(imageOrientation.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +page to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_page() {
iCmdArgs.add("+page");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -page to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps page() {
iCmdArgs.add("-page");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -page to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps page(Integer width) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-page");
if (width != null) {
buf.append(width.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -page to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps page(Integer width, Integer height) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-page");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -page to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps page(Integer width, Integer height, Integer x) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-page");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || x != null) {
oper="+";
if (x.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (x != null) {
buf.append(x.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -page to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps page(Integer width, Integer height, Integer x, Integer y) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-page");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || x != null) {
oper="+";
if (x.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (x != null) {
buf.append(x.toString());
}
if (x != null || y != null) {
oper="+";
if (y.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (y != null) {
buf.append(y.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -page to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps page(Integer width, Integer height, Integer x, Integer y, Character special) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-page");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || x != null) {
oper="+";
if (x.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (x != null) {
buf.append(x.toString());
}
if (x != null || y != null) {
oper="+";
if (y.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (y != null) {
buf.append(y.toString());
}
if (y != null || special != null) {
}
if (special != null) {
buf.append(special.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -page to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps page(Integer width, Integer height, Integer x, Integer y, String special) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-page");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || x != null) {
oper="+";
if (x.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (x != null) {
buf.append(x.toString());
}
if (x != null || y != null) {
oper="+";
if (y.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (y != null) {
buf.append(y.toString());
}
if (y != null || special != null) {
}
if (special != null) {
buf.append(special.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -paint to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps paint() {
iCmdArgs.add("-paint");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -paint to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps paint(Double radius) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-paint");
if (radius != null) {
buf.append(radius.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -path to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps path() {
iCmdArgs.add("-path");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -path to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps path(String path) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-path");
if (path != null) {
buf.append(path.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -passphrase to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps passphrase() {
iCmdArgs.add("-passphrase");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -passphrase to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps passphrase(String filename) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-passphrase");
if (filename != null) {
buf.append(filename.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -pause to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps pause() {
iCmdArgs.add("-pause");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -pause to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps pause(Integer seconds) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-pause");
if (seconds != null) {
buf.append(seconds.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -perceptible to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps perceptible() {
iCmdArgs.add("-perceptible");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -perceptible to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps perceptible(Double epsilon) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-perceptible");
if (epsilon != null) {
buf.append(epsilon.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -ping to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps ping() {
iCmdArgs.add("-ping");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -pointsize to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps pointsize() {
iCmdArgs.add("-pointsize");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -pointsize to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps pointsize(Integer value) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-pointsize");
if (value != null) {
buf.append(value.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -polaroid to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps polaroid() {
iCmdArgs.add("-polaroid");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -polaroid to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps polaroid(Double angle) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-polaroid");
if (angle != null) {
buf.append(angle.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +polaroid to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_polaroid() {
iCmdArgs.add("+polaroid");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -poly to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps poly() {
iCmdArgs.add("-poly");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -poly to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps poly(String args) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-poly");
if (args != null) {
buf.append(args.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -posterize to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps posterize() {
iCmdArgs.add("-posterize");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -posterize to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps posterize(Integer levels) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-posterize");
if (levels != null) {
buf.append(levels.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -precision to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps precision() {
iCmdArgs.add("-precision");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -precision to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps precision(Integer digits) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-precision");
if (digits != null) {
buf.append(digits.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -preview to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps preview() {
iCmdArgs.add("-preview");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -preview to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps preview(String type) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-preview");
if (type != null) {
buf.append(type.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -print to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps print() {
iCmdArgs.add("-print");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -print to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps print(String text) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-print");
if (text != null) {
buf.append(text.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -process to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps process() {
iCmdArgs.add("-process");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -process to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps process(String command) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-process");
if (command != null) {
buf.append(command.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -profile to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps profile() {
iCmdArgs.add("-profile");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -profile to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps profile(String filename) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-profile");
if (filename != null) {
buf.append(filename.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +profile to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_profile() {
iCmdArgs.add("+profile");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +profile to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_profile(String profileName) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("+profile");
if (profileName != null) {
buf.append(profileName.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -quality to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps quality() {
iCmdArgs.add("-quality");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -quality to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps quality(Double value) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-quality");
if (value != null) {
buf.append(value.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -quantize to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps quantize() {
iCmdArgs.add("-quantize");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -quantize to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps quantize(String colorspace) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-quantize");
if (colorspace != null) {
buf.append(colorspace.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -quiet to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps quiet() {
iCmdArgs.add("-quiet");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -radial-blur to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps radialBlur() {
iCmdArgs.add("-radial-blur");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -radial-blur to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps radialBlur(Double angle) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-radial-blur");
if (angle != null) {
buf.append(angle.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -raise to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps raise() {
iCmdArgs.add("-raise");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -raise to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps raise(Integer width) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-raise");
if (width != null) {
buf.append(width.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -raise to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps raise(Integer width, Integer height) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-raise");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +raise to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_raise() {
iCmdArgs.add("+raise");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +raise to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_raise(Integer width) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("+raise");
if (width != null) {
buf.append(width.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +raise to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_raise(Integer width, Integer height) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("+raise");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -random-threshold to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps randomThreshold() {
iCmdArgs.add("-random-threshold");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -random-threshold to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps randomThreshold(Double low) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-random-threshold");
if (low != null) {
buf.append(low.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -random-threshold to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps randomThreshold(Double low, Double high) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-random-threshold");
if (low != null) {
buf.append(low.toString());
}
if (low != null || high != null) {
buf.append("x");
}
if (high != null) {
buf.append(high.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -random-threshold to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps randomThreshold(Double low, Double high, Boolean percent) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-random-threshold");
if (low != null) {
buf.append(low.toString());
}
if (low != null || high != null) {
buf.append("x");
}
if (high != null) {
buf.append(high.toString());
}
if (high != null || percent != null) {
}
if (percent != null) {
if (percent.booleanValue())
buf.append("%");
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -recolor to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps recolor() {
iCmdArgs.add("-recolor");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -recolor to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps recolor(String matrix) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-recolor");
if (matrix != null) {
buf.append(matrix.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -red-primary to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps redPrimary() {
iCmdArgs.add("-red-primary");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -red-primary to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps redPrimary(Double x) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-red-primary");
if (x != null) {
buf.append(x.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -red-primary to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps redPrimary(Double x, Double y) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-red-primary");
if (x != null) {
buf.append(x.toString());
}
if (x != null || y != null) {
buf.append(",");
}
if (y != null) {
buf.append(y.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -regard-warnings to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps regardWarnings() {
iCmdArgs.add("-regard-warnings");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -region to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps region() {
iCmdArgs.add("-region");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -region to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps region(Integer width) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-region");
if (width != null) {
buf.append(width.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -region to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps region(Integer width, Integer height) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-region");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -region to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps region(Integer width, Integer height, Integer x) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-region");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || x != null) {
oper="+";
if (x.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (x != null) {
buf.append(x.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -region to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps region(Integer width, Integer height, Integer x, Integer y) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-region");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || x != null) {
oper="+";
if (x.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (x != null) {
buf.append(x.toString());
}
if (x != null || y != null) {
oper="+";
if (y.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (y != null) {
buf.append(y.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +remap to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_remap() {
iCmdArgs.add("+remap");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -remap to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps remap() {
iCmdArgs.add("-remap");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -remap to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps remap(String filename) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-remap");
if (filename != null) {
buf.append(filename.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -remote to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps remote() {
iCmdArgs.add("-remote");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -render to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps render() {
iCmdArgs.add("-render");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +render to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_render() {
iCmdArgs.add("+render");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +repage to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_repage() {
iCmdArgs.add("+repage");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -repage to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps repage() {
iCmdArgs.add("-repage");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -repage to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps repage(Integer width) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-repage");
if (width != null) {
buf.append(width.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -repage to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps repage(Integer width, Integer height) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-repage");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -repage to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps repage(Integer width, Integer height, Integer xOffset) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-repage");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || xOffset != null) {
oper="+";
if (xOffset.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (xOffset != null) {
buf.append(xOffset.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -repage to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps repage(Integer width, Integer height, Integer xOffset, Integer yOffset) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-repage");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || xOffset != null) {
oper="+";
if (xOffset.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (xOffset != null) {
buf.append(xOffset.toString());
}
if (xOffset != null || yOffset != null) {
oper="+";
if (yOffset.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (yOffset != null) {
buf.append(yOffset.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -resample to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps resample() {
iCmdArgs.add("-resample");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -resample to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps resample(Integer horizontal) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-resample");
if (horizontal != null) {
buf.append(horizontal.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -resample to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps resample(Integer horizontal, Integer vertical) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-resample");
if (horizontal != null) {
buf.append(horizontal.toString());
}
if (horizontal != null || vertical != null) {
buf.append("x");
}
if (vertical != null) {
buf.append(vertical.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -resize to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps resize() {
iCmdArgs.add("-resize");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -resize to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps resize(Integer width) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-resize");
if (width != null) {
buf.append(width.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -resize to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps resize(Integer width, Integer height) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-resize");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -resize to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps resize(Integer width, Integer height, Character special) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-resize");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || special != null) {
}
if (special != null) {
buf.append(special.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -resize to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps resize(Integer width, Integer height, String special) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-resize");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || special != null) {
}
if (special != null) {
buf.append(special.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -respect-parentheses to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps respectParentheses() {
iCmdArgs.add("-respect-parentheses");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -respect-parenthesis to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps respectParenthesis() {
iCmdArgs.add("-respect-parenthesis");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -reverse to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps reverse() {
iCmdArgs.add("-reverse");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -roll to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps roll() {
iCmdArgs.add("-roll");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -roll to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps roll(Integer x) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-roll");
if (x != null) {
buf.append(x.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -roll to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps roll(Integer x, Integer y) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-roll");
if (x != null) {
buf.append(x.toString());
}
if (x != null || y != null) {
oper="+";
if (y.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (y != null) {
buf.append(y.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -rotate to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps rotate() {
iCmdArgs.add("-rotate");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -rotate to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps rotate(Double degrees) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-rotate");
if (degrees != null) {
buf.append(degrees.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -rotate to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps rotate(Double degrees, Character special) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-rotate");
if (degrees != null) {
buf.append(degrees.toString());
}
if (degrees != null || special != null) {
}
if (special != null) {
buf.append(special.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -sample to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps sample() {
iCmdArgs.add("-sample");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -sample to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps sample(Integer width) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-sample");
if (width != null) {
buf.append(width.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -sample to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps sample(Integer width, Integer height) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-sample");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -sample to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps sample(Integer width, Integer height, Integer xOffset) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-sample");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || xOffset != null) {
oper="+";
if (xOffset.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (xOffset != null) {
buf.append(xOffset.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -sample to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps sample(Integer width, Integer height, Integer xOffset, Integer yOffset) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-sample");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || xOffset != null) {
oper="+";
if (xOffset.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (xOffset != null) {
buf.append(xOffset.toString());
}
if (xOffset != null || yOffset != null) {
oper="+";
if (yOffset.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (yOffset != null) {
buf.append(yOffset.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -sampling-factor to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps samplingFactor() {
iCmdArgs.add("-sampling-factor");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -sampling-factor to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps samplingFactor(Double horizontalFactor) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-sampling-factor");
if (horizontalFactor != null) {
buf.append(horizontalFactor.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -sampling-factor to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps samplingFactor(Double horizontalFactor, Double verticalFactor) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-sampling-factor");
if (horizontalFactor != null) {
buf.append(horizontalFactor.toString());
}
if (horizontalFactor != null || verticalFactor != null) {
buf.append("x");
}
if (verticalFactor != null) {
buf.append(verticalFactor.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -selective-blur to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps selectiveBlur() {
iCmdArgs.add("-selective-blur");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -selective-blur to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps selectiveBlur(Double radius) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-selective-blur");
if (radius != null) {
buf.append(radius.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -selective-blur to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps selectiveBlur(Double radius, Double sigma) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-selective-blur");
if (radius != null) {
buf.append(radius.toString());
}
if (radius != null || sigma != null) {
buf.append("x");
}
if (sigma != null) {
buf.append(sigma.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -selective-blur to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps selectiveBlur(Double radius, Double sigma, Double threshold) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-selective-blur");
if (radius != null) {
buf.append(radius.toString());
}
if (radius != null || sigma != null) {
buf.append("x");
}
if (sigma != null) {
buf.append(sigma.toString());
}
if (sigma != null || threshold != null) {
oper="+";
if (threshold.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (threshold != null) {
buf.append(threshold.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -sparse-color to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps sparseColor() {
iCmdArgs.add("-sparse-color");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -sparse-color to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps sparseColor(String method) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-sparse-color");
if (method != null) {
buf.append(method.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -sparse-color to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps sparseColor(String method, String cinfo) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-sparse-color");
if (method != null) {
buf.append(method.toString());
}
if (method != null || cinfo != null) {
iCmdArgs.add(buf.toString());
buf.setLength(0);
}
if (cinfo != null) {
buf.append(cinfo.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -scale to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps scale() {
iCmdArgs.add("-scale");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -scale to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps scale(Integer width) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-scale");
if (width != null) {
buf.append(width.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -scale to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps scale(Integer width, Integer height) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-scale");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -scale to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps scale(Integer width, Integer height, Integer xOffset) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-scale");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || xOffset != null) {
oper="+";
if (xOffset.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (xOffset != null) {
buf.append(xOffset.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -scale to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps scale(Integer width, Integer height, Integer xOffset, Integer yOffset) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-scale");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || xOffset != null) {
oper="+";
if (xOffset.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (xOffset != null) {
buf.append(xOffset.toString());
}
if (xOffset != null || yOffset != null) {
oper="+";
if (yOffset.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (yOffset != null) {
buf.append(yOffset.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -scene to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps scene() {
iCmdArgs.add("-scene");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -scene to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps scene(Integer value) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-scene");
if (value != null) {
buf.append(value.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -screen to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps screen() {
iCmdArgs.add("-screen");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -seed to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps seed() {
iCmdArgs.add("-seed");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -segment to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps segment() {
iCmdArgs.add("-segment");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -segment to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps segment(Integer clusterThreshold) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-segment");
if (clusterThreshold != null) {
buf.append(clusterThreshold.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -segment to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps segment(Integer clusterThreshold, Double smoothingThreshold) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-segment");
if (clusterThreshold != null) {
buf.append(clusterThreshold.toString());
}
if (clusterThreshold != null || smoothingThreshold != null) {
buf.append("x");
}
if (smoothingThreshold != null) {
buf.append(smoothingThreshold.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -separate to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps separate() {
iCmdArgs.add("-separate");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -sepia-tone to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps sepiaTone() {
iCmdArgs.add("-sepia-tone");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -sepia-tone to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps sepiaTone(Double threshold) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-sepia-tone");
if (threshold != null) {
buf.append(threshold.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -set to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps set() {
iCmdArgs.add("-set");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -set to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps set(String attribute) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-set");
if (attribute != null) {
buf.append(attribute.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -set to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps set(String attribute, String value) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-set");
if (attribute != null) {
buf.append(attribute.toString());
}
if (attribute != null || value != null) {
iCmdArgs.add(buf.toString());
buf.setLength(0);
}
if (value != null) {
buf.append(value.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -shade to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps shade() {
iCmdArgs.add("-shade");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -shade to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps shade(Double azimuth) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-shade");
if (azimuth != null) {
buf.append(azimuth.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -shade to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps shade(Double azimuth, Double elevation) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-shade");
if (azimuth != null) {
buf.append(azimuth.toString());
}
if (azimuth != null || elevation != null) {
buf.append("x");
}
if (elevation != null) {
buf.append(elevation.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +shade to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_shade() {
iCmdArgs.add("+shade");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +shade to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_shade(Double azimuth) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("+shade");
if (azimuth != null) {
buf.append(azimuth.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +shade to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_shade(Double azimuth, Double elevation) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("+shade");
if (azimuth != null) {
buf.append(azimuth.toString());
}
if (azimuth != null || elevation != null) {
buf.append("x");
}
if (elevation != null) {
buf.append(elevation.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -shadow to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps shadow() {
iCmdArgs.add("-shadow");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -shadow to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps shadow(Integer percentOpacity) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-shadow");
if (percentOpacity != null) {
buf.append(percentOpacity.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -shadow to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps shadow(Integer percentOpacity, Double sigma) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-shadow");
if (percentOpacity != null) {
buf.append(percentOpacity.toString());
}
if (percentOpacity != null || sigma != null) {
buf.append("x");
}
if (sigma != null) {
buf.append(sigma.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -shadow to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps shadow(Integer percentOpacity, Double sigma, Integer x) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-shadow");
if (percentOpacity != null) {
buf.append(percentOpacity.toString());
}
if (percentOpacity != null || sigma != null) {
buf.append("x");
}
if (sigma != null) {
buf.append(sigma.toString());
}
if (sigma != null || x != null) {
oper="+";
if (x.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (x != null) {
buf.append(x.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -shadow to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps shadow(Integer percentOpacity, Double sigma, Integer x, Integer y) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-shadow");
if (percentOpacity != null) {
buf.append(percentOpacity.toString());
}
if (percentOpacity != null || sigma != null) {
buf.append("x");
}
if (sigma != null) {
buf.append(sigma.toString());
}
if (sigma != null || x != null) {
oper="+";
if (x.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (x != null) {
buf.append(x.toString());
}
if (x != null || y != null) {
oper="+";
if (y.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (y != null) {
buf.append(y.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -shadow to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps shadow(Integer percentOpacity, Double sigma, Integer x, Integer y, Boolean percent) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-shadow");
if (percentOpacity != null) {
buf.append(percentOpacity.toString());
}
if (percentOpacity != null || sigma != null) {
buf.append("x");
}
if (sigma != null) {
buf.append(sigma.toString());
}
if (sigma != null || x != null) {
oper="+";
if (x.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (x != null) {
buf.append(x.toString());
}
if (x != null || y != null) {
oper="+";
if (y.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (y != null) {
buf.append(y.toString());
}
if (y != null || percent != null) {
}
if (percent != null) {
if (percent.booleanValue())
buf.append("%");
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -shared-memory to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps sharedMemory() {
iCmdArgs.add("-shared-memory");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -sharpen to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps sharpen() {
iCmdArgs.add("-sharpen");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -sharpen to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps sharpen(Double radius) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-sharpen");
if (radius != null) {
buf.append(radius.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -sharpen to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps sharpen(Double radius, Double sigma) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-sharpen");
if (radius != null) {
buf.append(radius.toString());
}
if (radius != null || sigma != null) {
buf.append("x");
}
if (sigma != null) {
buf.append(sigma.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -shave to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps shave() {
iCmdArgs.add("-shave");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -shave to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps shave(Integer width) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-shave");
if (width != null) {
buf.append(width.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -shave to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps shave(Integer width, Integer height) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-shave");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -shave to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps shave(Integer width, Integer height, Boolean percent) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-shave");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || percent != null) {
}
if (percent != null) {
if (percent.booleanValue())
buf.append("%");
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -shear to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps shear() {
iCmdArgs.add("-shear");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -shear to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps shear(Double xDegrees) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-shear");
if (xDegrees != null) {
buf.append(xDegrees.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -shear to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps shear(Double xDegrees, Double yDegrees) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-shear");
if (xDegrees != null) {
buf.append(xDegrees.toString());
}
if (xDegrees != null || yDegrees != null) {
buf.append("x");
}
if (yDegrees != null) {
buf.append(yDegrees.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -sigmoidal-contrast to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps sigmoidalContrast() {
iCmdArgs.add("-sigmoidal-contrast");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -sigmoidal-contrast to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps sigmoidalContrast(Double contrast) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-sigmoidal-contrast");
if (contrast != null) {
buf.append(contrast.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -sigmoidal-contrast to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps sigmoidalContrast(Double contrast, Double midPoint) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-sigmoidal-contrast");
if (contrast != null) {
buf.append(contrast.toString());
}
if (contrast != null || midPoint != null) {
buf.append("x");
}
if (midPoint != null) {
buf.append(midPoint.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +sigmoidal-contrast to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_sigmoidalContrast() {
iCmdArgs.add("+sigmoidal-contrast");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +sigmoidal-contrast to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_sigmoidalContrast(Double contrast) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("+sigmoidal-contrast");
if (contrast != null) {
buf.append(contrast.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +sigmoidal-contrast to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_sigmoidalContrast(Double contrast, Double midPoint) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("+sigmoidal-contrast");
if (contrast != null) {
buf.append(contrast.toString());
}
if (contrast != null || midPoint != null) {
buf.append("x");
}
if (midPoint != null) {
buf.append(midPoint.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -silent to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps silent() {
iCmdArgs.add("-silent");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -size to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps size() {
iCmdArgs.add("-size");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -size to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps size(Integer width) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-size");
if (width != null) {
buf.append(width.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -size to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps size(Integer width, Integer height) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-size");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -size to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps size(Integer width, Integer height, Integer offset) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-size");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || offset != null) {
oper="+";
if (offset.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (offset != null) {
buf.append(offset.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -sketch to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps sketch() {
iCmdArgs.add("-sketch");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -sketch to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps sketch(Double radius) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-sketch");
if (radius != null) {
buf.append(radius.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -sketch to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps sketch(Double radius, Double sigma) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-sketch");
if (radius != null) {
buf.append(radius.toString());
}
if (radius != null || sigma != null) {
buf.append("x");
}
if (sigma != null) {
buf.append(sigma.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -sketch to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps sketch(Double radius, Double sigma, Double angle) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-sketch");
if (radius != null) {
buf.append(radius.toString());
}
if (radius != null || sigma != null) {
buf.append("x");
}
if (sigma != null) {
buf.append(sigma.toString());
}
if (sigma != null || angle != null) {
oper="+";
if (angle.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (angle != null) {
buf.append(angle.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -smush to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps smush() {
iCmdArgs.add("-smush");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -smush to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps smush(Integer offset) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-smush");
if (offset != null) {
buf.append(offset.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -snaps to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps snaps() {
iCmdArgs.add("-snaps");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -snaps to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps snaps(Integer value) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-snaps");
if (value != null) {
buf.append(value.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -solarize to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps solarize() {
iCmdArgs.add("-solarize");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -solarize to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps solarize(Double threshold) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-solarize");
if (threshold != null) {
buf.append(threshold.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -splice to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps splice() {
iCmdArgs.add("-splice");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -splice to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps splice(Double width) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-splice");
if (width != null) {
buf.append(width.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -splice to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps splice(Double width, Double height) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-splice");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -splice to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps splice(Double width, Double height, Double x) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-splice");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || x != null) {
oper="+";
if (x.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (x != null) {
buf.append(x.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -splice to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps splice(Double width, Double height, Double x, Double y) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-splice");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || x != null) {
oper="+";
if (x.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (x != null) {
buf.append(x.toString());
}
if (x != null || y != null) {
oper="+";
if (y.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (y != null) {
buf.append(y.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -splice to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps splice(Double width, Double height, Double x, Double y, Boolean percent) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-splice");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || x != null) {
oper="+";
if (x.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (x != null) {
buf.append(x.toString());
}
if (x != null || y != null) {
oper="+";
if (y.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (y != null) {
buf.append(y.toString());
}
if (y != null || percent != null) {
}
if (percent != null) {
if (percent.booleanValue())
buf.append("%");
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -spread to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps spread() {
iCmdArgs.add("-spread");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -spread to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps spread(Integer amount) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-spread");
if (amount != null) {
buf.append(amount.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -stegano to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps stegano() {
iCmdArgs.add("-stegano");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -stegano to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps stegano(Integer offset) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-stegano");
if (offset != null) {
buf.append(offset.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -stereo to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps stereo() {
iCmdArgs.add("-stereo");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -stereo to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps stereo(Integer x) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-stereo");
if (x != null) {
buf.append(x.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -stereo to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps stereo(Integer x, Integer y) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-stereo");
if (x != null) {
buf.append(x.toString());
}
if (x != null || y != null) {
oper="+";
if (y.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (y != null) {
buf.append(y.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -statistic to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps statistic() {
iCmdArgs.add("-statistic");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -statistic to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps statistic(String type) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-statistic");
if (type != null) {
buf.append(type.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -statistic to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps statistic(String type, Integer width) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-statistic");
if (type != null) {
buf.append(type.toString());
}
if (type != null || width != null) {
iCmdArgs.add(buf.toString());
buf.setLength(0);
}
if (width != null) {
buf.append(width.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -statistic to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps statistic(String type, Integer width, Integer height) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-statistic");
if (type != null) {
buf.append(type.toString());
}
if (type != null || width != null) {
iCmdArgs.add(buf.toString());
buf.setLength(0);
}
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -statistic to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps statistic(String type, Integer width, Integer height, Integer x) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-statistic");
if (type != null) {
buf.append(type.toString());
}
if (type != null || width != null) {
iCmdArgs.add(buf.toString());
buf.setLength(0);
}
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || x != null) {
oper="+";
if (x.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (x != null) {
buf.append(x.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -statistic to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps statistic(String type, Integer width, Integer height, Integer x, Integer y) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-statistic");
if (type != null) {
buf.append(type.toString());
}
if (type != null || width != null) {
iCmdArgs.add(buf.toString());
buf.setLength(0);
}
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || x != null) {
oper="+";
if (x.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (x != null) {
buf.append(x.toString());
}
if (x != null || y != null) {
oper="+";
if (y.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (y != null) {
buf.append(y.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -statistic to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps statistic(String type, Integer width, Integer height, Integer x, Integer y, String special) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-statistic");
if (type != null) {
buf.append(type.toString());
}
if (type != null || width != null) {
iCmdArgs.add(buf.toString());
buf.setLength(0);
}
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || x != null) {
oper="+";
if (x.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (x != null) {
buf.append(x.toString());
}
if (x != null || y != null) {
oper="+";
if (y.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (y != null) {
buf.append(y.toString());
}
if (y != null || special != null) {
}
if (special != null) {
buf.append(special.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -storage-type to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps storageType() {
iCmdArgs.add("-storage-type");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -storage-type to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps storageType(String type) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-storage-type");
if (type != null) {
buf.append(type.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -stretch to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps stretch() {
iCmdArgs.add("-stretch");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -stretch to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps stretch(String fontStretchType) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-stretch");
if (fontStretchType != null) {
buf.append(fontStretchType.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -strip to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps strip() {
iCmdArgs.add("-strip");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -stroke to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps stroke() {
iCmdArgs.add("-stroke");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -stroke to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps stroke(String color) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-stroke");
if (color != null) {
buf.append(color.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -strokewidth to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps strokewidth() {
iCmdArgs.add("-strokewidth");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -strokewidth to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps strokewidth(Integer value) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-strokewidth");
if (value != null) {
buf.append(value.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -style to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps style() {
iCmdArgs.add("-style");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -style to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps style(String fontStyle) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-style");
if (fontStyle != null) {
buf.append(fontStyle.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -subimage-search to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps subimageSearch() {
iCmdArgs.add("-subimage-search");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +swap to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_swap() {
iCmdArgs.add("+swap");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -swap to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps swap() {
iCmdArgs.add("-swap");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -swap to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps swap(Integer pos1) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-swap");
if (pos1 != null) {
buf.append(pos1.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -swap to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps swap(Integer pos1, Integer pos2) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-swap");
if (pos1 != null) {
buf.append(pos1.toString());
}
if (pos1 != null || pos2 != null) {
buf.append(",");
}
if (pos2 != null) {
buf.append(pos2.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -swirl to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps swirl() {
iCmdArgs.add("-swirl");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -swirl to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps swirl(Double degrees) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-swirl");
if (degrees != null) {
buf.append(degrees.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -synchronize to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps synchronize() {
iCmdArgs.add("-synchronize");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -taint to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps taint() {
iCmdArgs.add("-taint");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -text-font to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps textFont() {
iCmdArgs.add("-text-font");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -text-font to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps textFont(String name) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-text-font");
if (name != null) {
buf.append(name.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -texture to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps texture() {
iCmdArgs.add("-texture");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -texture to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps texture(String filename) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-texture");
if (filename != null) {
buf.append(filename.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -threshold to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps threshold() {
iCmdArgs.add("-threshold");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -threshold to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps threshold(Integer red) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-threshold");
if (red != null) {
buf.append(red.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -threshold to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps threshold(Integer red, Integer green) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-threshold");
if (red != null) {
buf.append(red.toString());
}
if (red != null || green != null) {
buf.append(",");
}
if (green != null) {
buf.append(green.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -threshold to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps threshold(Integer red, Integer green, Integer blue) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-threshold");
if (red != null) {
buf.append(red.toString());
}
if (red != null || green != null) {
buf.append(",");
}
if (green != null) {
buf.append(green.toString());
}
if (green != null || blue != null) {
buf.append(",");
}
if (blue != null) {
buf.append(blue.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -threshold to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps threshold(Integer red, Integer green, Integer blue, Integer opacity) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-threshold");
if (red != null) {
buf.append(red.toString());
}
if (red != null || green != null) {
buf.append(",");
}
if (green != null) {
buf.append(green.toString());
}
if (green != null || blue != null) {
buf.append(",");
}
if (blue != null) {
buf.append(blue.toString());
}
if (blue != null || opacity != null) {
buf.append(",");
}
if (opacity != null) {
buf.append(opacity.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -threshold to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps threshold(Integer red, Integer green, Integer blue, Integer opacity, Boolean percent) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-threshold");
if (red != null) {
buf.append(red.toString());
}
if (red != null || green != null) {
buf.append(",");
}
if (green != null) {
buf.append(green.toString());
}
if (green != null || blue != null) {
buf.append(",");
}
if (blue != null) {
buf.append(blue.toString());
}
if (blue != null || opacity != null) {
buf.append(",");
}
if (opacity != null) {
buf.append(opacity.toString());
}
if (opacity != null || percent != null) {
}
if (percent != null) {
if (percent.booleanValue())
buf.append("%");
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -thumbnail to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps thumbnail() {
iCmdArgs.add("-thumbnail");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -thumbnail to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps thumbnail(Integer width) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-thumbnail");
if (width != null) {
buf.append(width.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -thumbnail to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps thumbnail(Integer width, Integer height) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-thumbnail");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -thumbnail to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps thumbnail(Integer width, Integer height, Character special) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-thumbnail");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || special != null) {
}
if (special != null) {
buf.append(special.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -thumbnail to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps thumbnail(Integer width, Integer height, String special) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-thumbnail");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || special != null) {
}
if (special != null) {
buf.append(special.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -tile to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps tile() {
iCmdArgs.add("-tile");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -tile to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps tile(Integer width) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-tile");
if (width != null) {
buf.append(width.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -tile to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps tile(Integer width, Integer height) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-tile");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -tile to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps tile(Integer width, Integer height, Integer xOffset) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-tile");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || xOffset != null) {
oper="+";
if (xOffset.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (xOffset != null) {
buf.append(xOffset.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -tile to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps tile(Integer width, Integer height, Integer xOffset, Integer yOffset) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-tile");
if (width != null) {
buf.append(width.toString());
}
if (width != null || height != null) {
buf.append("x");
}
if (height != null) {
buf.append(height.toString());
}
if (height != null || xOffset != null) {
oper="+";
if (xOffset.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (xOffset != null) {
buf.append(xOffset.toString());
}
if (xOffset != null || yOffset != null) {
oper="+";
if (yOffset.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (yOffset != null) {
buf.append(yOffset.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -tile-offset to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps tileOffset() {
iCmdArgs.add("-tile-offset");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -tile-offset to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps tileOffset(Integer x) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-tile-offset");
if (x != null) {
buf.append(x.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -tile-offset to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps tileOffset(Integer x, Integer y) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-tile-offset");
if (x != null) {
buf.append(x.toString());
}
if (x != null || y != null) {
oper="+";
if (y.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (y != null) {
buf.append(y.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -tile to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps tile(String filename) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-tile");
if (filename != null) {
buf.append(filename.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -tint to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps tint() {
iCmdArgs.add("-tint");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -tint to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps tint(Double value) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-tint");
if (value != null) {
buf.append(value.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -title to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps title() {
iCmdArgs.add("-title");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -title to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps title(String text) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-title");
if (text != null) {
buf.append(text.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -transform to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps transform() {
iCmdArgs.add("-transform");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -transparent-color to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps transparentColor() {
iCmdArgs.add("-transparent-color");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -transparent-color to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps transparentColor(String color) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-transparent-color");
if (color != null) {
buf.append(color.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -transparent to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps transparent() {
iCmdArgs.add("-transparent");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -transparent to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps transparent(String color) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-transparent");
if (color != null) {
buf.append(color.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -transpose to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps transpose() {
iCmdArgs.add("-transpose");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -transverse to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps transverse() {
iCmdArgs.add("-transverse");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -treedepth to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps treedepth() {
iCmdArgs.add("-treedepth");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -treedepth to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps treedepth(Integer value) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-treedepth");
if (value != null) {
buf.append(value.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -trim to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps trim() {
iCmdArgs.add("-trim");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -type to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps type() {
iCmdArgs.add("-type");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -type to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps type(String type) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-type");
if (type != null) {
buf.append(type.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -undercolor to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps undercolor() {
iCmdArgs.add("-undercolor");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -undercolor to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps undercolor(String color) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-undercolor");
if (color != null) {
buf.append(color.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -unique-colors to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps uniqueColors() {
iCmdArgs.add("-unique-colors");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -units to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps units() {
iCmdArgs.add("-units");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -units to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps units(String type) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-units");
if (type != null) {
buf.append(type.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -unsharp to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps unsharp() {
iCmdArgs.add("-unsharp");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -unsharp to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps unsharp(Double radius) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-unsharp");
if (radius != null) {
buf.append(radius.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -unsharp to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps unsharp(Double radius, Double sigma) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-unsharp");
if (radius != null) {
buf.append(radius.toString());
}
if (radius != null || sigma != null) {
buf.append("x");
}
if (sigma != null) {
buf.append(sigma.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -unsharp to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps unsharp(Double radius, Double sigma, Double amount) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-unsharp");
if (radius != null) {
buf.append(radius.toString());
}
if (radius != null || sigma != null) {
buf.append("x");
}
if (sigma != null) {
buf.append(sigma.toString());
}
if (sigma != null || amount != null) {
oper="+";
if (amount.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (amount != null) {
buf.append(amount.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -unsharp to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps unsharp(Double radius, Double sigma, Double amount, Double threshold) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-unsharp");
if (radius != null) {
buf.append(radius.toString());
}
if (radius != null || sigma != null) {
buf.append("x");
}
if (sigma != null) {
buf.append(sigma.toString());
}
if (sigma != null || amount != null) {
oper="+";
if (amount.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (amount != null) {
buf.append(amount.toString());
}
if (amount != null || threshold != null) {
oper="+";
if (threshold.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (threshold != null) {
buf.append(threshold.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -update to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps update() {
iCmdArgs.add("-update");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -update to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps update(Integer seconds) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-update");
if (seconds != null) {
buf.append(seconds.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -verbose to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps verbose() {
iCmdArgs.add("-verbose");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -version to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps version() {
iCmdArgs.add("-version");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -view to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps view() {
iCmdArgs.add("-view");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -view to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps view(String text) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-view");
if (text != null) {
buf.append(text.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -vignette to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps vignette() {
iCmdArgs.add("-vignette");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -vignette to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps vignette(Double radius) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-vignette");
if (radius != null) {
buf.append(radius.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -vignette to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps vignette(Double radius, Double sigma) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-vignette");
if (radius != null) {
buf.append(radius.toString());
}
if (radius != null || sigma != null) {
buf.append("x");
}
if (sigma != null) {
buf.append(sigma.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -vignette to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps vignette(Double radius, Double sigma, Double x) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-vignette");
if (radius != null) {
buf.append(radius.toString());
}
if (radius != null || sigma != null) {
buf.append("x");
}
if (sigma != null) {
buf.append(sigma.toString());
}
if (sigma != null || x != null) {
oper="+";
if (x.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (x != null) {
buf.append(x.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -vignette to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps vignette(Double radius, Double sigma, Double x, Double y) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-vignette");
if (radius != null) {
buf.append(radius.toString());
}
if (radius != null || sigma != null) {
buf.append("x");
}
if (sigma != null) {
buf.append(sigma.toString());
}
if (sigma != null || x != null) {
oper="+";
if (x.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (x != null) {
buf.append(x.toString());
}
if (x != null || y != null) {
oper="+";
if (y.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (y != null) {
buf.append(y.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -vignette to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps vignette(Double radius, Double sigma, Double x, Double y, Boolean percent) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-vignette");
if (radius != null) {
buf.append(radius.toString());
}
if (radius != null || sigma != null) {
buf.append("x");
}
if (sigma != null) {
buf.append(sigma.toString());
}
if (sigma != null || x != null) {
oper="+";
if (x.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (x != null) {
buf.append(x.toString());
}
if (x != null || y != null) {
oper="+";
if (y.doubleValue() < 0)
oper="";
buf.append(oper);
}
if (y != null) {
buf.append(y.toString());
}
if (y != null || percent != null) {
}
if (percent != null) {
if (percent.booleanValue())
buf.append("%");
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -virtual-pixel to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps virtualPixel() {
iCmdArgs.add("-virtual-pixel");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -virtual-pixel to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps virtualPixel(String method) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-virtual-pixel");
if (method != null) {
buf.append(method.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -visual to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps visual() {
iCmdArgs.add("-visual");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -visual to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps visual(String type) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-visual");
if (type != null) {
buf.append(type.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -watermark to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps watermark() {
iCmdArgs.add("-watermark");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -watermark to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps watermark(Double brightness) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-watermark");
if (brightness != null) {
buf.append(brightness.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -watermark to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps watermark(Double brightness, Double saturation) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-watermark");
if (brightness != null) {
buf.append(brightness.toString());
}
if (brightness != null || saturation != null) {
buf.append("x");
}
if (saturation != null) {
buf.append(saturation.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -wave to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps wave() {
iCmdArgs.add("-wave");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -wave to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps wave(Double amplitude) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-wave");
if (amplitude != null) {
buf.append(amplitude.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -wave to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps wave(Double amplitude, Double wavelength) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-wave");
if (amplitude != null) {
buf.append(amplitude.toString());
}
if (amplitude != null || wavelength != null) {
buf.append("x");
}
if (wavelength != null) {
buf.append(wavelength.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -weight to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps weight() {
iCmdArgs.add("-weight");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -weight to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps weight(String fontWeight) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-weight");
if (fontWeight != null) {
buf.append(fontWeight.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -weight to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps weight(Integer fontWeight) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-weight");
if (fontWeight != null) {
buf.append(fontWeight.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -white-point to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps whitePoint() {
iCmdArgs.add("-white-point");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -white-point to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps whitePoint(Double x) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-white-point");
if (x != null) {
buf.append(x.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -white-point to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps whitePoint(Double x, Double y) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-white-point");
if (x != null) {
buf.append(x.toString());
}
if (x != null || y != null) {
buf.append(",");
}
if (y != null) {
buf.append(y.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -white-threshold to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps whiteThreshold() {
iCmdArgs.add("-white-threshold");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -white-threshold to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps whiteThreshold(Double threshold) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-white-threshold");
if (threshold != null) {
buf.append(threshold.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -white-threshold to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps whiteThreshold(Double threshold, Boolean percent) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-white-threshold");
if (threshold != null) {
buf.append(threshold.toString());
}
if (threshold != null || percent != null) {
}
if (percent != null) {
if (percent.booleanValue())
buf.append("%");
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -window-group to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps windowGroup() {
iCmdArgs.add("-window-group");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -window to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps window() {
iCmdArgs.add("-window");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -window to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps window(String id) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-window");
if (id != null) {
buf.append(id.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -write to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps write() {
iCmdArgs.add("-write");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option -write to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps write(String filename) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("-write");
if (filename != null) {
buf.append(filename.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +write to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_write() {
iCmdArgs.add("+write");
return this;
}
//////////////////////////////////////////////////////////////////////////////
/**
Add option +write to the ImageMagick commandline
(see the documentation of ImageMagick for details).
*/
public IMOps p_write(String filename) {
String oper; // only used in some methods
StringBuffer buf = new StringBuffer(); // local buffer for option-args
iCmdArgs.add("+write");
if (filename != null) {
buf.append(filename.toString());
}
if (buf.length()>0) {
iCmdArgs.add(buf.toString());
}
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy