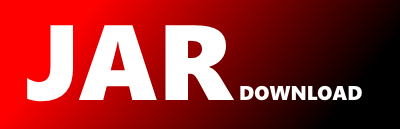
com.ck.email.SimpleMailSender Maven / Gradle / Ivy
package com.ck.email;
import javax.activation.DataHandler;
import javax.activation.FileDataSource;
import javax.mail.*;
import javax.mail.internet.*;
import javax.mail.internet.MimeMessage.RecipientType;
import java.util.Date;
import java.util.Enumeration;
import java.util.List;
import java.util.Properties;
public class SimpleMailSender {
// 发送邮件的props文件
private final transient Properties props = System.getProperties();
// 邮件服务器登录验证
private transient MailAuthenticator authenticator;
// 邮箱session
private transient Session session;
/***
* 初始化邮件发送器
* @param smtpHostName SMTP邮件服务器地址
* @param username 发送邮件的用户名(地址)
* @param password 发送邮件的密码
*/
public SimpleMailSender(final String smtpHostName, final String username, final String password) {
init(username, password, smtpHostName);
}
/**
* 初始化邮件发送器
*
* @param username 发送邮件的用户名(地址),并以此解析SMTP服务器地址(对大多数邮箱都管用)
* @param password 发送邮件的密码
*/
public SimpleMailSender(final String username, final String password) {
// 通过邮箱地址解析出smtp服务器,对大多数邮箱都管用
final String smtpHostName = "smtp." + username.split("@")[1];
init(username, password, smtpHostName);
}
/**
* 初始化
*
* @param username 发送邮件的用户名(地址)
* @param password 密码
* @param smtpHostName SMTP主机地址
*/
private void init(String username, String password, String smtpHostName) {
// 初始化props
props.put("mail.smtp.auth", "true");
props.put("mail.smtp.host", smtpHostName);
// 验证
authenticator = new MailAuthenticator(username, password);
// 创建session
session = Session.getInstance(props, authenticator);
}
/***
* 发送邮件
* @param recipient 收件人邮箱地址
* @param content SimpleMail
* @throws AddressException t
* @throws MessagingException t
*/
private void send(String recipient, SimpleMail content) throws AddressException, MessagingException {
// 创建mime类型邮件
final MimeMessage message = new MimeMessage(session);
// 设置发信人
message.setFrom(new InternetAddress(authenticator.getUsername()));
// 设置收件人
message.setRecipient(RecipientType.TO, new InternetAddress(recipient));
// 设置主题
message.setSubject(content.getSubject());
// 后面的BodyPart将加入到此处创建的Multipart中
//添加HTML正文
MimeMultipart htmlContent = new MimeMultipart("related");
BodyPart msgContent = new MimeBodyPart();
msgContent.setContent(content.getContent(), "text/html;charset=utf-8");
htmlContent.addBodyPart(msgContent);
Multipart mp = new MimeMultipart("subtype");
BodyPart htmlBody = new MimeBodyPart();
htmlBody.setContent(htmlContent);
mp.addBodyPart(htmlBody);
if(content instanceof AttachMail){
// 利用枚举器方便的遍历集合
Enumeration efile = ((AttachMail) content).getFile().elements();
// 检查序列中是否还有更多的对象
while (efile.hasMoreElements()) {
MimeBodyPart mbp = new MimeBodyPart();
// 选择出每一个附件名
String filename = efile.nextElement().toString();
// 得到数据源
FileDataSource fds = new FileDataSource(filename);
// 得到附件本身并至入BodyPart
mbp.setDataHandler(new DataHandler(fds));
// 得到文件名同样至入BodyPart
mbp.setFileName(fds.getName());
mp.addBodyPart(mbp);
}
}
// Multipart加入到信件
message.setContent(mp);
// 设置信件头的发送日期
//message.setSentDate(TimeUtils.stringToDate("2020-05-12 23:12:33", TimeFormat.YYYYMMDDHHMMSS));
// 发送
Transport.send(message);
}
/**
* 群发邮件
*
* @param recipients 收件人们
* @param content 内容
* @throws AddressException 和
* @throws MessagingException 和
*/
private void send(List recipients, SimpleMail content)
throws AddressException, MessagingException {
// 创建mime类型邮件
final MimeMessage message = new MimeMessage(session);
// 设置发信人
message.setFrom(new InternetAddress(authenticator.getUsername()));
// 设置收件人们
final int num = recipients.size();
InternetAddress[] addresses = new InternetAddress[num];
for (int i = 0; i < num; i++) {
addresses[i] = new InternetAddress(recipients.get(i));
}
message.setRecipients(RecipientType.TO, addresses);
// 设置主题
message.setSubject(content.getSubject());
// 设置邮件内容
MimeMultipart htmlContent = new MimeMultipart("related");
BodyPart msgContent = new MimeBodyPart();
msgContent.setContent(content.getContent(), "text/html;charset=utf-8");
htmlContent.addBodyPart(msgContent);
Multipart mp = new MimeMultipart("subtype");
BodyPart htmlBody = new MimeBodyPart();
htmlBody.setContent(htmlContent);
mp.addBodyPart(htmlBody);
if(content instanceof AttachMail){
// 利用枚举器方便的遍历集合
Enumeration efile = ((AttachMail) content).getFile().elements();
// 检查序列中是否还有更多的对象
while (efile.hasMoreElements()) {
MimeBodyPart mbp = new MimeBodyPart();
// 选择出每一个附件名
String filename = efile.nextElement().toString();
// 得到数据源
FileDataSource fds = new FileDataSource(filename);
// 得到附件本身并至入BodyPart
mbp.setDataHandler(new DataHandler(fds));
// 得到文件名同样至入BodyPart
mbp.setFileName(fds.getName());
mp.addBodyPart(mbp);
}
}
// Multipart加入到信件
message.setContent(mp);
// 设置信件头的发送日期
message.setSentDate(new Date());
// 发送
Transport.send(message);
}
/***
* 发送邮件
* @param recipient 收件人邮箱地址
* @param mail 邮件对象
* @return boolean
*/
public boolean sendMsg(String recipient, SimpleMail mail){
try {
send(recipient, mail);
return true;
} catch (MessagingException e) {
e.printStackTrace();
}
return false;
}
/***
* 群发邮件
* @param recipients 收件人们
* @param mail 邮件对 象
* @return boolean
*/
public boolean sendGroupMsg(List recipients, SimpleMail mail){
try {
send(recipients, mail);
return true;
} catch (MessagingException e) {
e.printStackTrace();
}
return false;
}
// public static void main(String[] args) {
// AttachMail simpleMail = new AttachMail();
// simpleMail.setContent("内容.....有附件");
// simpleMail.setSubject("附件标题");
// simpleMail.attachfile("C:\\Users\\ikfan\\Pictures\\Saved Pictures\\1.jfif");
// simpleMail.attachfile("C:\\Users\\ikfan\\Pictures\\Saved Pictures\\2.jpg");
// simpleMail.attachfile("C:\\Users\\ikfan\\Pictures\\Saved Pictures\\3.gif");
// SimpleMailSender sender = new SimpleMailSender("[email protected]","btknwrqmdxwebadf");
// System.out.println(sender.sendMsg("[email protected]",simpleMail));
// }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy