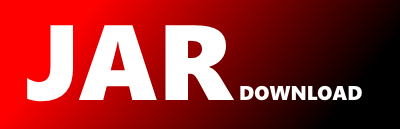
com.ck.tools.FileUtils Maven / Gradle / Ivy
package com.ck.tools;
import java.io.*;
public class FileUtils {
/***
* 生成指定路径文件夹
* @param path String
* @return boolean
*/
public static boolean generatePath(String path) {
if (!Utils.isNull(path)) {
File file = new File(path);
if (!file.exists() && !file.isDirectory()) {
return file.mkdirs();
}
}
return false;
}
/***
* 生成指定路径的文件
* @param path String
* @return boolean
*/
public static boolean generateFile(String path) {
if (!Utils.isNull(path)) {
generatePath(path.substring(0, path.lastIndexOf("/")));
File file = new File(path);
if (!file.exists()) {
try {
return file.createNewFile();
} catch (IOException e) {
e.printStackTrace();
}
}
return true;
}
return false;
}
/***
* 写入内容到指定路径文件(会覆盖原有内容)
* @param filePath String
* @param content String
* @return boolean
*/
public static boolean writeToFile(String filePath, String content) {
if (!Utils.isNull(content) && !Utils.isNull(filePath)) {
try {
if(!generateFile(filePath)) return false;
File file = new File(filePath);// 要写入的文本文件
FileWriter writer = new FileWriter(file);// 获取该文件的输出流
writer.write(content);// 写内容
writer.flush();// 清空缓冲区,立即将输出流里的内容写到文件里
writer.close();// 关闭输出流,施放资源
return true;
} catch (IOException e) {
e.printStackTrace();
}
}
return false;
}
/***
* 追加内容到指定路径文件(不会覆盖原有内容)
* @param filePath String
* @param content String
* @return boolean
*/
public static boolean writeAppendToFile(String filePath,String content) {
if (!Utils.isNull(content) && !Utils.isNull(filePath)) {
try {
if(!generateFile(filePath)) return false;
File file = new File(filePath);// 要写入的文本文件
String origin = readString(filePath,"");
FileWriter writer = new FileWriter(file);// 获取该文件的输出流
writer.write(origin + content);// 写内容
writer.flush();// 清空缓冲区,立即将输出流里的内容写到文件里
writer.close();// 关闭输出流,施放资源
return true;
} catch (IOException e) {
e.printStackTrace();
}
}
return false;
}
/***
* 读取文件内容
* @param path 文件路径
* @param encode 默认是utf-8
* @return String
*/
public static String readString(String path,String encode) {
if(Utils.isNull(encode)) encode = "utf-8";
String str = "";
String temp = "";
File file = new File(path);
try {
FileInputStream in = new FileInputStream(file);
BufferedReader reader = new BufferedReader(new InputStreamReader(in,encode));
while ((temp = reader.readLine()) != null) {
str += temp;
}
reader.close();
} catch (IOException e) {
return null;
}
return str;
}
/***
* 删除文件
* @param filePath String
* @return boolean
*/
public static boolean deleteFile(String filePath){
File file = new File(filePath);
return file.delete();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy