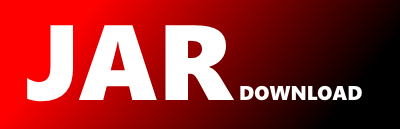
com.ck.tools.TimeUtils Maven / Gradle / Ivy
package com.ck.tools;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
public class TimeUtils {
private static final long m = 60 * 1000L;//分
private static final long hour = 3600 * 1000L;//小时
private static final long day = 24 * hour;//天
/***
* 时间转换成格式化
*
* @param date Date
* @param format TimeFormat
* @return String
*/
public static String timeToString(Date date, TimeFormat format) {
SimpleDateFormat sdf = new SimpleDateFormat(format.getValue());
String dateStr = sdf.format(date.getTime());
return dateStr;
}
/***
* 字符串转日期
* @param time String
* @param format TimeFormat
* @return Date
*/
public static Date stringToDate(String time, TimeFormat format){
SimpleDateFormat sdf = new SimpleDateFormat(format.getValue());
try {
return sdf.parse(time);
} catch (ParseException e) {
e.printStackTrace();
}
return null;
}
/***
* 日期时间转换成文字
* @param date Date
* @return 刚刚, n分钟前, n小时前, n天前
*/
public static String getDateTimeString(Date date) {
if (date == null) {
throw new NullPointerException();
}
Date currentDate = new Date();
long cha = Math.abs(date.getTime() - currentDate.getTime());
long hours = cha / hour;
if (hours < 1) {
if (cha / m <= 0) {
return "刚刚";
}
return cha / m + "分钟前";
}
if (hours < 24) {
return cha / hour + "小时前";
}
if (hours <= 72) {
int nn = Integer.valueOf(cha / day + "");
if (cha % day > 0) {
nn++;
}
return nn + "天前";
}
return timeToString(date, TimeFormat.YYYYMMDDHHMM);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy