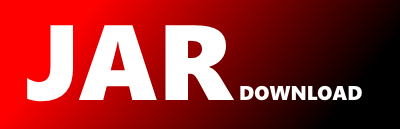
com.gihub.gkutiel.Fbi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fbi Show documentation
Show all versions of fbi Show documentation
Java API for Facebook apps
package com.gihub.gkutiel;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.net.URL;
import java.net.URLEncoder;
import java.util.HashMap;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Scanner;
import com.google.gson.Gson;
import com.google.gson.JsonSyntaxException;
public class Fbi {
private static final Gson gson = new Gson();
final Map params = new HashMap<>();
final String path;
public Fbi(final String path) {
this.path = path;
}
public Fbi accessToken(final String accessToken) {
return param("access_token", accessToken);
}
public String get() throws IOException {
return method("GET").fetch();
}
public T getAs(final Class type) throws IOException {
final String res = get();
try {
return gson.fromJson(res, type);
} catch (final JsonSyntaxException e) {
throw new RuntimeException("failed to parse\n" + res + "\nas " + type, e);
}
}
public Fbi param(final String key, final String val) {
params.put(key, val);
return this;
}
private String buildQuery() {
if (params.isEmpty()) return "";
final StringBuilder query = new StringBuilder("?");
for (final Entry param : params.entrySet())
query.append(encode(param.getKey()) + "=" + encode(param.getValue()) + "&");
query.deleteCharAt(query.length() - 1);
return query.toString();
}
private String encode(final String str) {
try {
return URLEncoder.encode(str, "utf-8");
} catch (final UnsupportedEncodingException e) {
throw new RuntimeException(e);
}
}
String buildUrl() {
final StringBuilder url = new StringBuilder("https://graph.facebook.com/");
url.append(path);
url.append(buildQuery());
return url.toString();
}
String fetch() throws IOException {
try (final Scanner s = new Scanner(new URL(buildUrl()).openStream());) {
return s.useDelimiter("\\A").next();
}
}
Fbi method(final String method) {
return param("method", method);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy