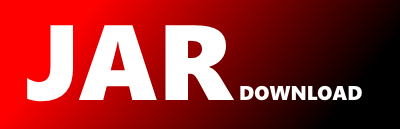
io.aws.lambda.events.LexEvent Maven / Gradle / Ivy
package io.aws.lambda.events;
import lombok.Data;
import lombok.experimental.Accessors;
import org.jetbrains.annotations.NotNull;
import java.io.Serializable;
import java.util.Collections;
import java.util.Map;
/**
* represents a Lex event
*/
@Data
@Accessors(chain = true)
public class LexEvent implements Serializable {
private String messageVersion;
private String invocationSource;
private String userId;
private String outputDialogMode;
private CurrentIntent currentIntent;
private Bot bot;
private Map sessionAttributes;
public @NotNull Map getSessionAttributes() {
return sessionAttributes == null ? Collections.emptyMap() : sessionAttributes;
}
/**
* Represents a Lex bot
*/
@Data
@Accessors(chain = true)
public class Bot implements Serializable {
private String name;
private String alias;
private String version;
}
/**
* models CurrentIntent of Lex event
*/
@Data
@Accessors(chain = true)
public class CurrentIntent implements Serializable {
private String name;
private String confirmationStatus;
private Map slots;
public @NotNull Map getSlots() {
return slots == null ? Collections.emptyMap() : slots;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy