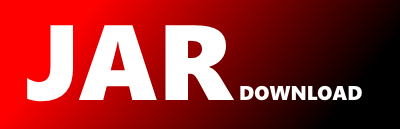
io.aws.lambda.events.SQSEvent Maven / Gradle / Ivy
package io.aws.lambda.events;
import lombok.Data;
import lombok.experimental.Accessors;
import org.jetbrains.annotations.NotNull;
import java.io.Serializable;
import java.util.Collections;
import java.util.List;
import java.util.Map;
/**
* Represents an Amazon SQS event.
*/
@Data
@Accessors(chain = true)
public class SQSEvent implements Serializable {
private List records;
public @NotNull List getRecords() {
return records == null ? Collections.emptyList() : records;
}
@Data
@Accessors(chain = true)
public static class MessageAttribute implements Serializable {
private String stringValue;
private String dataType;
private byte[] binaryValue;
private byte[][] binaryListValues;
private List stringListValues;
public @NotNull List getStringListValues() {
return stringListValues == null ? Collections.emptyList() : stringListValues;
}
}
@Data
@Accessors(chain = true)
public static class SQSMessage implements Serializable {
private String messageId;
private String receiptHandle;
private String body;
private String md5OfBody;
private String md5OfMessageAttributes;
private String eventSourceArn;
private String eventSource;
private String awsRegion;
private Map attributes;
private Map messageAttributes;
public @NotNull Map getAttributes() {
return attributes == null ? Collections.emptyMap() : attributes;
}
public @NotNull Map getMessageAttributes() {
return messageAttributes == null ? Collections.emptyMap() : messageAttributes;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy