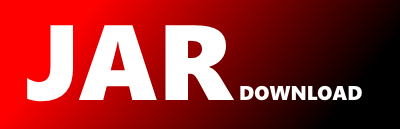
io.aws.lambda.events.dynamodb.AttributeValue Maven / Gradle / Ivy
package io.aws.lambda.events.dynamodb;
import lombok.Data;
import lombok.experimental.Accessors;
import org.jetbrains.annotations.NotNull;
import java.io.Serializable;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* Represents the data for an attribute. Each attribute value is described as a
* name-value pair. The name is the data type, and the value is the data itself.
* For more information, see Data Types in the Amazon DynamoDB Developer Guide.
*
* @see AWS API Documentation
*/
@Data
@Accessors(chain = true)
public class AttributeValue implements Serializable {
/**
* An attribute of type String. For example: "S": "Hello"
*/
private String s;
/**
* An attribute of type Number. For example: "N": "123.45"
Numbers
* are sent across the network to DynamoDB as strings, to maximize compatibility
* across languages and libraries. However, DynamoDB treats them as number type
* attributes for mathematical operations.
*/
private String n;
/**
* An attribute of type Binary. For example:
* "B": "dGhpcyB0ZXh0IGlzIGJhc2U2NC1lbmNvZGVk"
*/
private byte[] b;
/**
* An attribute of type String Set. For example:
* "SS": ["Giraffe", "Hippo" ,"Zebra"]
*/
private List sS;
/**
* An attribute of type Number Set. For example:
* "NS": ["42.2", "-19", "7.5", "3.14"]
Numbers are sent across the
* network to DynamoDB as strings, to maximize compatibility across languages
* and libraries. However, DynamoDB treats them as number type attributes for
* mathematical operations.
*/
private List nS;
/**
* An attribute of type Binary Set. For example:
* "BS": ["U3Vubnk=", "UmFpbnk=", "U25vd3k="]
*/
private byte[][] bS;
/**
* An attribute of type Map. For example:
* "M": {"Name": {"S": "Joe"}, "Age": {"N": "35"}}
*/
private Map m;
/**
* An attribute of type List. For example:
* "L": [ {"S": "Cookies"} , {"S": "Coffee"}, {"N", "3.14159"}]
*/
private List l;
/**
* An attribute of type Null. For example: "NULL": true
*/
private Boolean nULLValue;
/**
* An attribute of type Boolean. For example: "BOOL": true
*/
private Boolean bOOL;
public @NotNull List getsS() {
return sS == null ? Collections.emptyList() : sS;
}
public @NotNull List getnS() {
return nS == null ? Collections.emptyList() : nS;
}
public @NotNull Map getM() {
return m == null ? Collections.emptyMap() : m;
}
public @NotNull List getL() {
return l == null ? Collections.emptyList() : l;
}
public AttributeValue addMEntry(String key, AttributeValue value) {
if (null == this.m)
this.m = new HashMap<>();
if (this.m.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key + ") are provided.");
this.m.put(key, value);
return this;
}
/**
* Removes all the entries added into M.
*
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public AttributeValue clearMEntries() {
this.m = null;
return this;
}
public AttributeValue withL(AttributeValue... l) {
if (this.l == null)
return this;
Collections.addAll(this.l, l);
return this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy