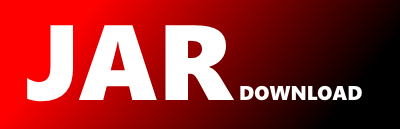
io.aws.lambda.events.s3.S3ObjectLambdaEvent Maven / Gradle / Ivy
package io.aws.lambda.events.s3;
import lombok.Data;
import lombok.experimental.Accessors;
import org.jetbrains.annotations.NotNull;
import java.util.Collections;
import java.util.Map;
/**
* Event to allow transformations to occur before an S3 object is returned to
* the calling service.
*
* Documentation
*
* Writing
* and debugging Lambda functions for S3 Object Lambda Access Points
*
* Example:
*
*
* import com.amazonaws.services.lambda.runtime.Context;
* import com.amazonaws.services.lambda.runtime.events.S3ObjectLambdaEvent;
* import org.apache.http.client.fluent.Request;
* import software.amazon.awssdk.services.s3.S3Client;
* import software.amazon.awssdk.services.s3.model.WriteGetObjectResponseRequest;
*
* import java.io.IOException;
*
* import static software.amazon.awssdk.core.sync.RequestBody.fromString;
*
* public class S3ObjectRequestHandler {
*
* private static final S3Client s3Client = S3Client.create();
*
* public void handleRequest(S3ObjectLambdaEvent event, Context context) throws IOException {
* String s3Body = Request.Get(event.inputS3Url()).execute().returnContent().asString();
*
* String responseBody = s3Body.toUpperCase();
*
* WriteGetObjectResponseRequest request = WriteGetObjectResponseRequest.builder()
* .requestRoute(event.outputRoute())
* .requestToken(event.outputToken())
* .build();
* s3Client.writeGetObjectResponse(request, fromString(responseBody));
* }
* }
*
*
*/
@Data
@Accessors(chain = true)
public class S3ObjectLambdaEvent {
private String xAmzRequestId;
private GetObjectContext getObjectContext;
private Configuration configuration;
private UserRequest userRequest;
private UserIdentity userIdentity;
private String protocolVersion;
/**
* A pre-signed URL that can be used to fetch the original object from Amazon
* S3.
*
* The URL is signed using the original caller's identity, and their permissions
* will apply when the URL is used. If there are signed headers in the URL, the
* Lambda function must include these in the call to Amazon S3, except for the
* Host.
*
* @return A pre-signed URL that can be used to fetch the original object from
* Amazon S3.
*/
public String inputS3Url() {
return getGetObjectContext().getInputS3Url();
}
/**
* A routing token that is added to the S3 Object Lambda URL when the Lambda
* function calls the S3 API WriteGetObjectResponse.
*
* @return the outputRoute
*/
public String outputRoute() {
return getGetObjectContext().getOutputRoute();
}
/**
* An opaque token used by S3 Object Lambda to match the WriteGetObjectResponse
* call with the original caller.
*
* @return the outputToken
*/
public String outputToken() {
return getGetObjectContext().getOutputToken();
}
@Data
@Accessors(chain = true)
public static class GetObjectContext {
private String inputS3Url;
private String outputRoute;
private String outputToken;
}
@Data
@Accessors(chain = true)
public static class Configuration {
private String accessPointArn;
private String supportingAccessPointArn;
private String payload;
}
@Data
@Accessors(chain = true)
public static class UserRequest {
private String url;
private Map headers;
public @NotNull Map getHeaders() {
return headers == null ? Collections.emptyMap() : headers;
}
}
@Data
@Accessors(chain = true)
public static class UserIdentity {
private String type;
private String principalId;
private String arn;
private String accountId;
private String accessKeyId;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy