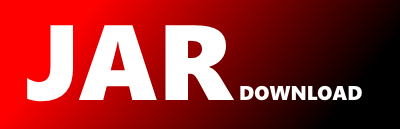
io.aws.lambda.events.system.IamPolicyResponse Maven / Gradle / Ivy
package io.aws.lambda.events.system;
import lombok.Data;
import lombok.experimental.Accessors;
import org.jetbrains.annotations.NotNull;
import java.io.Serializable;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
@Data
@Accessors(chain = true)
public class IamPolicyResponse implements Serializable {
public static final String EXECUTE_API_INVOKE = "execute-api:Invoke";
public static final String VERSION_2012_10_17 = "2012-10-17";
public static final String ALLOW = "Allow";
public static final String DENY = "Deny";
private String principalId;
private PolicyDocument policyDocument;
private Map context;
public @NotNull Map getContext() {
return context == null ? Collections.emptyMap() : context;
}
public Map getPolicyDocument() {
final List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy