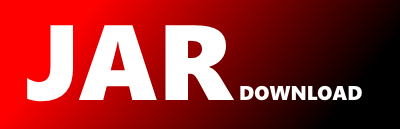
io.api.bloxy.core.IMoneyFlowApi.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bloxy-api Show documentation
Show all versions of bloxy-api Show documentation
Kotlin & Java Library for all available Bloxy API endpoints
package io.api.bloxy.core
import io.api.bloxy.model.dto.Address
import io.api.bloxy.model.dto.Tx
import io.api.bloxy.model.dto.moneyflow.*
import io.api.bloxy.util.ParamConverter.Companion.MAX_DATE
import io.api.bloxy.util.ParamConverter.Companion.MAX_DATETIME
import io.api.bloxy.util.ParamConverter.Companion.MIN_DATE
import io.api.bloxy.util.ParamConverter.Companion.MIN_DATETIME
import org.jetbrains.annotations.NotNull
import java.time.LocalDate
import java.time.LocalDateTime
/**
* API for Money Flow Analysis between wallets. Supports Ether and any tokens
* More information - https://bloxy.info/api_methods#money_flow
*
* @author GoodforGod
* @since 16.11.2018
*/
interface IMoneyFlowApi {
/**
* Aggregates amount of receive and sent amounts for each from the list of addresses
* @param addresses to look for
* @param contract to filter (ETH default)
* @param since timestamp
* @param till timestamp
*/
@NotNull
fun addressVolumes(
addresses: List,
contract: String = "ETH",
since: LocalDateTime = MIN_DATETIME,
till: LocalDateTime = MAX_DATETIME
): List
/**
* Aggregates amount of receive transactions for specific currency and select top senders ( by amount )
* @param address to look for
* @param contract to filter (ETH default)
* @param limit max result
* @param offset of the list from origin (0)
* @param since timestamp
* @param till timestamp
*/
@NotNull
fun topSenders(
address: String,
contract: String = "ETH",
limit: Int = 100,
offset: Int = 0,
since: LocalDateTime = MIN_DATETIME,
till: LocalDateTime = MAX_DATETIME
): List
/**
* Aggregates amount of sent transactions for specific currency and select top receivers ( by amount )
* @param address to look for
* @param contract to filter (ETH default)
* @param limit max result
* @param offset of the list from origin (0)
* @param since timestamp
* @param till timestamp
*/
@NotNull
fun topReceivers(
address: String,
contract: String = "ETH",
limit: Int = 100,
offset: Int = 0,
since: LocalDateTime = MIN_DATETIME,
till: LocalDateTime = MAX_DATETIME
): List
/**
* Analyses the full graph of money transactions and calculates the money distribution from the given address
* @param address to look for
* @param contract to filter (ETH default)
* @param depth how deep should look in the transaction tree
* @param limit max result
* @param offset of the list from origin (0)
* @param minBalance ignore addresses with this amount or less
* @param minTxAmount minimum amount of transactions
* @param ignoreAddressWithTxs ignore distribution from addresses with txs more than
* @param since timestamp
* @param till timestamp
* @param snapshot take into account only transfers till this time
*/
@NotNull
fun moneyDistribution(
address: String,
contract: String = "ETH",
depth: Int = 10,
limit: Int = 1000,
offset: Int = 0,
minTxAmount: Int = 0,
minBalance: Double = .001,
ignoreAddressWithTxs: Int = 2000,
since: LocalDateTime = MIN_DATETIME,
till: LocalDateTime = MAX_DATETIME,
snapshot: LocalDateTime = MIN_DATETIME
): List
/**
* Analyses the full graph of money transactions
* Outputs the transactions of the flow how money distributed from the given address
* @param address to look for
* @param contract to filter (ETH default)
* @param depth how deep should look in the transaction tree
* @param limit max result
* @param offset of the list from origin (0)
* @param minBalance ignore addresses with this amount or less
* @param minTxAmount minimum amount of transactions
* @param ignoreAddressWithTxs ignore distribution from addresses with txs more than
* @param since timestamp
* @param till timestamp
* @param snapshot take into account only transfers till this time
*/
@NotNull
fun txsDistribution(
address: String,
contract: String = "ETH",
depth: Int = 10,
limit: Int = 5000,
offset: Int = 0,
minTxAmount: Int = 0,
minBalance: Double = .001,
ignoreAddressWithTxs: Int = 2000,
since: LocalDateTime = MIN_DATETIME,
till: LocalDateTime = MAX_DATETIME,
snapshot: LocalDateTime = MIN_DATETIME
): List
/**
* Analyses the full graph of money transactions and calculates the money sources for the given address
* @param address to look for
* @param contract to filter (ETH default)
* @param depth how deep should look in the transaction tree
* @param limit max result
* @param offset of the list from origin (0)
* @param minBalance ignore addresses with this amount or less
* @param minTxAmount minimum amount of transactions
* @param ignoreAddressWithTxs ignore distribution from addresses with txs more than
* @param since timestamp
* @param till timestamp
* @param snapshot take into account only transfers till this time
*/
@NotNull
fun moneySource(
address: String,
contract: String = "ETH",
depth: Int = 5,
limit: Int = 1000,
offset: Int = 0,
minTxAmount: Int = 0,
minBalance: Double = .001,
ignoreAddressWithTxs: Int = 1000,
since: LocalDateTime = MIN_DATETIME,
till: LocalDateTime = MAX_DATETIME,
snapshot: LocalDateTime = MIN_DATETIME
): List
/**
* Analyses the full graph of money transactions.
* Outputs the transactions of the flow how money come into given address
* @param address to look for
* @param contract to filter (ETH default)
* @param depth how deep should look in the transaction tree
* @param limit max result
* @param offset of the list from origin (0)
* @param minBalance ignore addresses with this amount or less
* @param minTxAmount minimum amount of transactions
* @param ignoreAddressWithTxs ignore distribution from addresses with txs more than
* @param since timestamp
* @param till timestamp
* @param snapshot take into account only transfers till this time
*/
@NotNull
fun txsSource(
address: String,
contract: String = "ETH",
depth: Int = 5,
limit: Int = 5000,
offset: Int = 0,
minTxAmount: Int = 0,
minBalance: Double = .001,
ignoreAddressWithTxs: Int = 1000,
since: LocalDateTime = MIN_DATETIME,
till: LocalDateTime = MAX_DATETIME,
snapshot: LocalDateTime = MIN_DATETIME
): List
/**
* List of all transfers to/from the given address
* @param addresses to look for
* @param contracts to filter
* @param limit max result
* @param offset of the list from origin (0)
* @param since timestamp
* @param till timestamp
*/
@NotNull
fun transfersAll(
addresses: List,
contracts: List = emptyList(),
limit: Int = 1000,
offset: Int = 0,
since: LocalDate = MIN_DATE,
till: LocalDate = MAX_DATE
): List
/**
* List of transfers to the given address
* @param addresses to look for
* @param contracts to filter
* @param limit max result
* @param offset of the list from origin (0)
* @param since timestamp
* @param till timestamp
*/
@NotNull
fun transfersReceived(
addresses: List,
contracts: List = emptyList(),
limit: Int = 1000,
offset: Int = 0,
since: LocalDate = MIN_DATE,
till: LocalDate = MAX_DATE
): List
/**
* List of transfers from the given address
* @param addresses to look for
* @param contracts to filter
* @param limit max result
* @param offset of the list from origin (0)
* @param since timestamp
* @param till timestamp
*/
@NotNull
fun transfersSend(
addresses: List,
contracts: List = emptyList(),
limit: Int = 1000,
offset: Int = 0,
since: LocalDate = MIN_DATE,
till: LocalDate = MAX_DATE
): List
/**
* Aggregates amount of receive transactions and select top senders ( by Transfer Count )
* @param address to look for
* @param limit max result
* @param offset of the list from origin (0)
* @param since timestamp
* @param till timestamp
*/
@NotNull
fun topSendersCount(
address: String,
limit: Int = 100,
offset: Int = 0,
since: LocalDateTime = MIN_DATETIME,
till: LocalDateTime = MAX_DATETIME
): List
/**
* Aggregates amount of sent transactions and select top receivers ( by Transfer Count )
* @param address to look for
* @param limit max result
* @param offset of the list from origin (0)
* @param since timestamp
* @param till timestamp
*/
@NotNull
fun topReceiversCount(
address: String,
limit: Int = 100,
offset: Int = 0,
since: LocalDateTime = MIN_DATETIME,
till: LocalDateTime = MAX_DATETIME
): List
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy