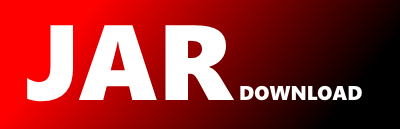
io.api.bloxy.core.ITokenSaleApi.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bloxy-api Show documentation
Show all versions of bloxy-api Show documentation
Kotlin & Java Library for all available Bloxy API endpoints
package io.api.bloxy.core
import io.api.bloxy.model.dto.Address
import io.api.bloxy.model.dto.Tx
import io.api.bloxy.model.dto.tokensale.*
import io.api.bloxy.util.ParamConverter.Companion.MAX_DATETIME
import io.api.bloxy.util.ParamConverter.Companion.MIN_DATETIME
import org.jetbrains.annotations.NotNull
import java.time.LocalDateTime
/**
* API for Analysis of token sales (ICO)
* More information - https://bloxy.info/api_methods#tokensale
*
* @author GoodforGod
* @since 16.11.2018
*/
interface ITokenSaleApi {
/**
* Lists recent token sale aggregated statistics
* @param contracts to filter
* @param limit max result
* @param offset of the list from origin (0)
* @param timeSpanDays get info for period in days from today
*/
@NotNull
fun sales(
contracts: List = emptyList(),
limit: Int = 30,
offset: Int = 0,
timeSpanDays: Int = 30
): List
/**
* Lists recent token sale transactions
* @param contracts to filter
* @param limit max result
* @param offset of the list from origin (0)
* @param timeSpanDays get info for period in days from today
*/
@NotNull
fun saleTxs(
contracts: List = emptyList(),
limit: Int = 30,
offset: Int = 0,
timeSpanDays: Int = 30
): List
/**
* Aggregated statistics for a token sale by days
* @param contract to filter
*/
@NotNull
fun statsDaily(
contract: String
): List
/**
* Token sale smart contracts and wallets addresses
* @param contract to filter
*/
@NotNull
fun statsAddress(
contract: String
): List
/**
* Token sale buyers addresses
* @param contract to filter
* @param limit max result
* @param offset of the list from origin (0)
*/
@NotNull
fun buyers(
contract: String,
limit: Int = 100,
offset: Int = 0
): List
/**
* Shows the wallets, used to collect currency from buyers
* @param contract to filter
* @param withIntermediary
*/
@NotNull
fun wallets(
contract: String,
withIntermediary: Boolean = false
): List
/**
* Calculates money distribution from the tokensale
* @param contract to filter
* @param depth how deep should look in the transaction tree
* @param limit max result
* @param offset of the list from origin (0)
* @param minBalance ignore addresses with this amount or less
* @param minTxAmount minimum amount of transactions
* @param ignoreAddressWithTxs ignore distribution from addresses with txs more than
* @param since timestamp
* @param till timestamp
* @param snapshot take into account only transfers till this time
*/
@NotNull
fun moneyDistribution(
contract: String,
depth: Int = 10,
limit: Int = 100,
offset: Int = 0,
minTxAmount: Int = 0,
minBalance: Double = .001,
ignoreAddressWithTxs: Int = 2000,
since: LocalDateTime = MIN_DATETIME,
till: LocalDateTime = MAX_DATETIME,
snapshot: LocalDateTime = MIN_DATETIME
): List
/**
* Calculates the transactions of money distribution from the tokensale
* @param contract to filter
* @param depth how deep should look in the transaction tree
* @param limit max result
* @param offset of the list from origin (0)
* @param minTxAmount minimum amount of transactions
* @param ignoreAddressWithTxs ignore distribution from addresses with txs more than
* @param since timestamp
* @param till timestamp
* @param snapshot take into account only transfers till this time
*/
@NotNull
fun txsDistribution(
contract: String,
depth: Int = 10,
limit: Int = 5000,
offset: Int = 0,
minTxAmount: Int = 0,
ignoreAddressWithTxs: Int = 2000,
since: LocalDateTime = MIN_DATETIME,
till: LocalDateTime = MAX_DATETIME,
snapshot: LocalDateTime = MIN_DATETIME
): List
/**
* Calculates the money sources for the tokensale by analyzing the graph of transaction for the given depth
* @param contract to filter
* @param depth how deep should look in the transaction tree
* @param limit max result
* @param offset of the list from origin (0)
* @param minBalance ignore addresses with this amount or less
* @param minTxAmount minimum amount of transactions
* @param ignoreAddressWithTxs ignore distribution from addresses with txs more than
* @param since timestamp
* @param till timestamp
* @param snapshot take into account only transfers till this time
*/
@NotNull
fun moneySources(
contract: String,
depth: Int = 5,
limit: Int = 100,
offset: Int = 0,
minTxAmount: Int = 0,
minBalance: Double = .001,
ignoreAddressWithTxs: Int = 2000,
since: LocalDateTime = MIN_DATETIME,
till: LocalDateTime = MAX_DATETIME,
snapshot: LocalDateTime = MIN_DATETIME
): List
/**
* Analyses the full graph of money transactions on tokensale
* @param contract to filter
* @param depth how deep should look in the transaction tree
* @param limit max result
* @param offset of the list from origin (0)
* @param minTxAmount minimum amount of transactions
* @param ignoreAddressWithTxs ignore distribution from addresses with txs more than
* @param since timestamp
* @param till timestamp
* @param snapshot take into account only transfers till this time
*/
@NotNull
fun txsSources(
contract: String,
depth: Int = 5,
limit: Int = 5000,
offset: Int = 0,
minTxAmount: Int = 0,
ignoreAddressWithTxs: Int = 2000,
since: LocalDateTime = MIN_DATETIME,
till: LocalDateTime = MAX_DATETIME,
snapshot: LocalDateTime = MIN_DATETIME
): List
/**
* Builds the graph of the initial token distribution and calculates the list of token holders with the amounts
* @param contract to filter
* @param limit max result
* @param offset of the list from origin (0)
* @param since timestamp
* @param till timestamp
*/
@NotNull
fun tokenDistribution(
contract: String,
limit: Int = 5000,
offset: Int = 0,
since: LocalDateTime = MIN_DATETIME,
till: LocalDateTime = MAX_DATETIME
): List
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy