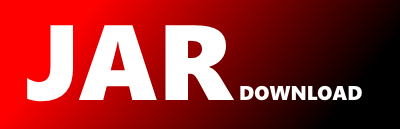
io.api.etherscan.core.IAccountApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-etherscan-api Show documentation
Show all versions of java-etherscan-api Show documentation
Library is a wrapper for EtherScan API.
package io.api.etherscan.core;
import io.api.etherscan.error.ApiException;
import io.api.etherscan.model.*;
import org.jetbrains.annotations.NotNull;
import java.util.List;
/**
* EtherScan - API Descriptions https://etherscan.io/apis#accounts
*
* @author GoodforGod
* @since 28.10.2018
*/
public interface IAccountApi {
/**
* Address ETH balance
*
* @param address get balance for
* @return balance
* @throws ApiException parent exception class
*/
@NotNull
Balance balance(String address) throws ApiException;
/**
* ERC20 token balance for address
*
* @param address get balance for
* @param contract token contract
* @return token balance for address
* @throws ApiException parent exception class
*/
@NotNull
TokenBalance balance(String address, String contract) throws ApiException;
/**
* Maximum 20 address for single batch request If address MORE THAN 20, then there will be more than
* 1 request performed
*
* @param addresses addresses to get balances for
* @return list of balances
* @throws ApiException parent exception class
*/
@NotNull
List balances(List addresses) throws ApiException;
/**
* All txs for given address
*
* @param address get txs for
* @param startBlock tx from this blockNumber
* @param endBlock tx to this blockNumber
* @return txs for address
* @throws ApiException parent exception class
*/
@NotNull
List txs(String address, long startBlock, long endBlock) throws ApiException;
@NotNull
List txs(String address, long startBlock) throws ApiException;
@NotNull
List txs(String address) throws ApiException;
/**
* All internal txs for given address
*
* @param address get txs for
* @param startBlock tx from this blockNumber
* @param endBlock tx to this blockNumber
* @return txs for address
* @throws ApiException parent exception class
*/
@NotNull
List txsInternal(String address, long startBlock, long endBlock) throws ApiException;
@NotNull
List txsInternal(String address, long startBlock) throws ApiException;
@NotNull
List txsInternal(String address) throws ApiException;
/**
* All internal tx for given transaction hash
*
* @param txhash transaction hash
* @return internal txs list
* @throws ApiException parent exception class
*/
@NotNull
List txsInternalByHash(String txhash) throws ApiException;
/**
* All ERC-20 token txs for given address
*
* @param address get txs for
* @param startBlock tx from this blockNumber
* @param endBlock tx to this blockNumber
* @return txs for address
* @throws ApiException parent exception class
*/
@NotNull
List txsToken(String address, long startBlock, long endBlock) throws ApiException;
@NotNull
List txsToken(String address, long startBlock) throws ApiException;
@NotNull
List txsToken(String address) throws ApiException;
/**
* All ERC-721 (NFT) token txs for given address
*
* @param address get txs for
* @param startBlock tx from this blockNumber
* @param endBlock tx to this blockNumber
* @return txs for address
* @throws ApiException parent exception class
*/
@NotNull
List txsNftToken(String address, long startBlock, long endBlock) throws ApiException;
@NotNull
List txsNftToken(String address, long startBlock) throws ApiException;
@NotNull
List txsNftToken(String address) throws ApiException;
/**
* All blocks mined by address
*
* @param address address to search for
* @return blocks mined
* @throws ApiException parent exception class
*/
@NotNull
List minedBlocks(String address) throws ApiException;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy