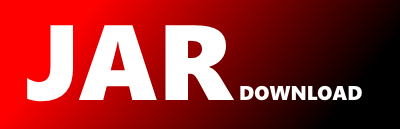
org.bdd.reporting.services.CommonFeatureToFeatureOverviewConsumer.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bdd-reporting-service Show documentation
Show all versions of bdd-reporting-service Show documentation
BDD Enterprise Reporting Server. This server provides the ability to upload reports from tools like Cucumber,
SpecFlow, Pickles and the information is then aggregated into a central view across all projects.
The newest version!
package org.bdd.reporting.services
import org.bdd.reporting.data.CommonFeature
import org.bdd.reporting.data.CommonScenario
import org.bdd.reporting.data.CommonStep
import org.bdd.reporting.events.EventBus
import org.bdd.reporting.repository.elasticsearch.FeatureOverview
import org.bdd.reporting.repository.elasticsearch.FeatureOverviewRepository
import org.springframework.beans.factory.annotation.Qualifier
import org.springframework.stereotype.Service
import javax.annotation.PostConstruct
import javax.annotation.PreDestroy
/**
* Created by Grant Little [email protected]
*/
@Suppress("unused")
@Service
open class CommonFeatureToFeatureOverviewConsumer(@Qualifier("DbEventBus")val eventBus: EventBus,
val featureOverviewRepository: FeatureOverviewRepository) {
@PostConstruct
fun start() {
eventBus.register("common-features", { handle(it)})
}
@PreDestroy
fun stop() {
}
internal fun handle(commonFeature : CommonFeature) {
val featureOverview = toFeatureOverview(commonFeature)
featureOverviewRepository.index(featureOverview)
}
internal fun toFeatureOverview(commonFeature: CommonFeature) : FeatureOverview {
val result = scenarios(commonFeature.scenarios)
return FeatureOverview(
id = commonFeature.id,
timestamp = commonFeature.timestamp,
description = commonFeature.description,
name = commonFeature.name,
passedScenarios = result.first["passed"] ?: 0,
failedScenarios = result.first["failed"] ?: 0,
pendingScenarios = result.first["pending"] ?: 0,
ignoredScenarios = result.first["ignored"] ?: 0,
totalScenarios = result.first["total"] ?: 0,
passedSteps = result.second["passed"] ?: 0,
failedSteps = result.second["failed"] ?: 0,
pendingSteps = result.second["pending"] ?: 0,
ignoredSteps = result.second["ignored"] ?: 0,
totalSteps = result.second["total"] ?: 0,
overallStatus = determineOverallStatus(result.first),
properties = commonFeature.properties ?: mutableSetOf(),
tags = commonFeature.tags
)
}
internal fun scenarios(scenarios : List) : Pair
© 2015 - 2025 Weber Informatics LLC | Privacy Policy