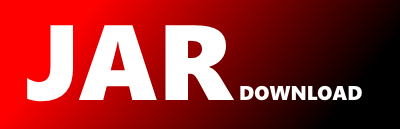
com.github.guang19.cosservice.template.buckettemplate.BaseAliyunOSSBucketTemplate Maven / Gradle / Ivy
package com.github.guang19.cosservice.template.buckettemplate;
import com.aliyun.oss.OSS;
import com.aliyun.oss.OSSClientBuilder;
import com.aliyun.oss.OSSException;
import com.aliyun.oss.model.AccessControlList;
import com.aliyun.oss.model.Bucket;
import com.github.guang19.cosservice.util.COSUtil;
import com.github.guang19.cosservice.config.AliyunOSSClientProperties;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
/**
* @author yangguang
* @date 2020/2/6
* @description 阿里云oss存储桶操作基础模板
*/
public abstract class BaseAliyunOSSBucketTemplate implements AliyunOSSBucketTemplate
{
//阿里云oss客户端
protected final OSS ossClient;
//logger
protected static final Logger log = LoggerFactory.getLogger(BaseAliyunOSSBucketTemplate.class);
/**
* 阿里云OSS客户端属性构造
* @param ossClientProperties OSS客户端属性构造
*/
protected BaseAliyunOSSBucketTemplate(AliyunOSSClientProperties ossClientProperties)
{
this.ossClient = new OSSClientBuilder().build(ossClientProperties.getCname() != null ? ossClientProperties.getCname() : ossClientProperties.getEndpoint(),
ossClientProperties.getCredentialsProvider(),
COSUtil.newAliyunOSSClientConfig(ossClientProperties));
}
/**
* 创建私有读写的存储桶,默认为标准存储桶,本地冗余
*
* @param bucketName 存储桶名(3 - 63个字符)
* @return 存储桶
*/
@Override
public Bucket createPrivateStandardLRSBucket(String bucketName)
{
throw new UnsupportedOperationException("can not create bucket with base template");
}
/**
* 创建私有读写的存储桶:标准存储,同城冗余
*
* @param bucketName 存储桶名
* @return 存储桶
*/
@Override
public Bucket createPrivateStandardZRSBucket(String bucketName)
{
throw new UnsupportedOperationException("can not create bucket with base template");
}
/**
* 创建私有读写的存储桶:低频访问,本地冗余
*
* @param bucketName 存储桶名
* @return 存储桶
*/
@Override
public Bucket createPrivateIALRSBucket(String bucketName)
{
throw new UnsupportedOperationException("can not create bucket with base template");
}
/**
* 创建私有读写的存储桶:低频访问,同城冗余
*
* @param bucketName 存储桶名
* @return 存储桶
*/
@Override
public Bucket createPrivateIAZRSBucket(String bucketName)
{
throw new UnsupportedOperationException("can not create bucket with base template");
}
/**
* 创建私有读写的存储桶:归档存储
*
* @param bucketName 存储桶名
* @return 存储桶
*/
@Override
public Bucket createPrivateArchiveBucket(String bucketName)
{
throw new UnsupportedOperationException("can not create bucket with base template");
}
/**
* 创建公有读写的存储桶:标准存储,本地冗余
*
* @param bucketName 存储桶名
* @return 存储桶
*/
@Override
public Bucket createPublicStandardLRSBucket(String bucketName)
{
throw new UnsupportedOperationException("can not create bucket with base template");
}
/**
* 创建公有读写的存储桶:标准存储,同城冗余
*
* @param bucketName 存储桶名
* @return 存储桶
*/
@Override
public Bucket createPublicStandardZRSBucket(String bucketName)
{
throw new UnsupportedOperationException("can not create bucket with base template");
}
/**
* 创建公有读写的存储桶:低频访问,本地冗余
*
* @param bucketName 存储桶名
* @return 存储桶
*/
@Override
public Bucket createPublicIALRSBucket(String bucketName)
{
throw new UnsupportedOperationException("can not create bucket with base template");
}
/**
* 创建公有读写的存储桶:低频访问,同城冗余
*
* @param bucketName 存储桶名
* @return 存储桶
*/
@Override
public Bucket createPublicIAZRSBucket(String bucketName)
{
throw new UnsupportedOperationException("can not create bucket with base template");
}
/**
* 创建公有读写的存储桶:归档存储
*
* @param bucketName 存储桶名
* @return 存储桶
*/
@Override
public Bucket createPublicArchiveBucket(String bucketName)
{
throw new UnsupportedOperationException("can not create bucket with base template");
}
/**
* 创建公有读私有写的存储桶:标准存储,本地冗余
*
* @param bucketName 存储桶名
* @return 存储桶
*/
@Override
public Bucket createPublicReadStandardLRSBucket(String bucketName)
{
throw new UnsupportedOperationException("can not create bucket with base template");
}
/**
* 创建公有读私有写的存储桶:标准存储,同城冗余
*
* @param bucketName 存储桶名
* @return 存储桶
*/
@Override
public Bucket createPublicReadStandardZRSBucket(String bucketName)
{
throw new UnsupportedOperationException("can not create bucket with base template");
}
/**
* 创建公有读私有写的存储桶:低频访问,本地冗余
*
* @param bucketName 存储桶名
* @return 存储桶
*/
@Override
public Bucket createPublicReadIALRSBucket(String bucketName)
{
throw new UnsupportedOperationException("can not create bucket with base template");
}
/**
* 创建公有读私有写的存储桶:低频访问,同城冗余
*
* @param bucketName 存储桶名
* @return 存储桶
*/
@Override
public Bucket createPublicReadIAZRSBucket(String bucketName)
{
throw new UnsupportedOperationException("can not create bucket with base template");
}
/**
* 创建公有读私有写的存储桶:归档存储
*
* @param bucketName 存储桶名
* @return 存储桶
*/
@Override
public Bucket createPublicReadArchiveBucket(String bucketName)
{
throw new UnsupportedOperationException("can not create bucket with base template");
}
/**
* 获取当前地域下的所有存储桶
*
* @return 存储桶集合
*/
@Override
public List getAllBuckets()
{
List buckets = ossClient.listBuckets().stream().map(Bucket::toString).collect(Collectors.toList());
close();
return buckets;
}
/**
* 获取存储桶的位置
*
* @param bucketName 存储桶名
* @return 存储桶位置, 也就是region {@link com.aliyuncs.regions.Endpoint} , aliyun may be null
*/
@Override
public String getBucketLocation(String bucketName)
{
COSUtil.assertObjectNull(bucketName);
String location = null;
try
{
location = ossClient.getBucketLocation(bucketName);
}
catch (OSSException e)
{
log.error("error during get bucket location".concat(COSUtil.parseAliyunErrorMessage(e)));
}
finally
{
close();
}
return location;
}
/**
* 获取存储桶的访问权限控制列表
*
* @param bucketName 存储桶名
* @return 访问权限控制列表 , aliyun may be null
*
* Map:
* owner: xxx
* grants:xxx
*
*/
@Override
public Map getBucketAccessControllerList(String bucketName)
{
COSUtil.assertObjectNull(bucketName);
Map map = null;
try
{
AccessControlList accessControlList = ossClient.getBucketAcl(bucketName);
map = new HashMap<>();
getBucketAccessControllerList(map,accessControlList);
}
catch (OSSException e)
{
log.error("error during get bucket access controller list : ".concat(COSUtil.parseAliyunErrorMessage(e)));
}
finally
{
close();
}
return map;
}
//获取存储桶权限
private void getBucketAccessControllerList(Map map,AccessControlList accessControlList)
{
if(accessControlList != null)
{
map.put("owner",accessControlList.getOwner());
map.put("grants",accessControlList.getGrants());
}
else
{
map.put("owner",null);
map.put("grants",null);
}
}
/**
* 判断存储桶是否存在
*
* @param bucketName 存储桶名
* @return 存储桶是否存在
*/
@Override
public boolean existBucket(String bucketName)
{
throw new UnsupportedOperationException("aliyun oss service does not support judge exist bucket");
}
/**
* 删除存储桶
*
* @param bucketName 存储桶名
*/
@Override
public void deleteBucket(String bucketName)
{
COSUtil.assertObjectNull(bucketName);
try
{
ossClient.deleteBucket(bucketName);
}
catch (OSSException e)
{
log.error("error during delete bucket : ".concat(COSUtil.parseAliyunErrorMessage(e)));
}
finally
{
close();
}
}
/**
* 关闭cos客户端会话
*/
@Override
public void close()
{
ossClient.shutdown();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy