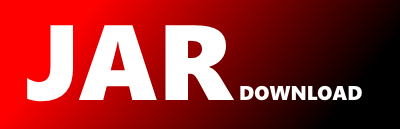
org.sqlite.Backup Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sqlite-javacpp Show documentation
Show all versions of sqlite-javacpp Show documentation
JDBC Driver for SQLite using JavaCPP
The newest version!
/*
* The author disclaims copyright to this source code. In place of
* a legal notice, here is a blessing:
*
* May you do good and not evil.
* May you find forgiveness for yourself and forgive others.
* May you share freely, never taking more than you give.
*/
package org.sqlite;
import static org.sqlite.SQLite.SQLITE_DONE;
import static org.sqlite.SQLite.SQLITE_OK;
import static org.sqlite.SQLite.nativeString;
import static org.sqlite.SQLite.sqlite3_backup;
import static org.sqlite.SQLite.sqlite3_backup_finish;
import static org.sqlite.SQLite.sqlite3_backup_pagecount;
import static org.sqlite.SQLite.sqlite3_backup_remaining;
import static org.sqlite.SQLite.sqlite3_backup_step;
import static org.sqlite.SQLite.sqlite3_log;
/**
* Online Backup Object
* sqlite3_backup
*/
public class Backup implements AutoCloseable {
private sqlite3_backup pBackup;
private final Conn dst, src;
Backup(sqlite3_backup pBackup, Conn dst, Conn src) {
assert pBackup != null && dst != null && src != null;
this.pBackup = pBackup;
this.dst = dst;
this.src = src;
}
/**
* Copy up to N pages between the source and destination databases
* @param nPage number of pages to copy
* @return true
when successfully finishes copying all pages from source to destination.
* @see sqlite3_backup_step
*/
public boolean step(int nPage) throws ConnException {
checkInit();
final int res = sqlite3_backup_step(pBackup, nPage);
if (res == SQLITE_OK || (res&0xFF) == ErrCodes.SQLITE_BUSY || (res&0xFF) == ErrCodes.SQLITE_LOCKED) {
sqlite3_log(-1, nativeString("busy/locked error during backup."));
return true;
} else if (res == SQLITE_DONE) {
return false;
}
throw new ConnException(dst, "backup step failed", res);
}
// TODO progression callback
/**
* Run starts the backup:
*
* - copying up to 'nPage' pages between the source and destination at each step,
* - sleeping 'millis' between steps,
* - closing the backup when done or when an error happens.
*
* Sleeping is disabled if 'sleepNs' is zero or negative.
*/
public void run(int nPage, long millis) throws ConnException, InterruptedException {
try {
while (step(nPage)) {
if (millis > 0L) {
Thread.sleep(millis);
}
}
} finally {
finish();
}
}
/**
* @return the number of pages still to be backed up
* @see sqlite3_backup_remaining
*/
public int remaining() throws ConnException {
checkInit();
return sqlite3_backup_remaining(pBackup);
}
/**
* @return the total number of pages in the source database
* @see sqlite3_backup_pagecount
*/
public int pageCount() throws ConnException {
checkInit();
return sqlite3_backup_pagecount(pBackup);
}
@Override
protected void finalize() throws Throwable {
if (pBackup != null) {
sqlite3_log(-1, nativeString("dangling SQLite backup."));
finish();
}
super.finalize();
}
/**
* Destroy this backup.
* @return result code (No exception is thrown).
* @see sqlite3_backup_finish
*/
public int finish() {
if (pBackup == null) {
return SQLITE_OK;
}
final int res = sqlite3_backup_finish(pBackup); // must be called only once
pBackup = null;
return res;
}
/**
* Destroy this backup and throw an exception if an error occured.
*/
@Override
public void close() throws ConnException {
final int res = finish();
if (res != SQLITE_OK) {
throw new ConnException(dst, "backup finish failed", res);
}
}
/**
* @return whether or not this backup is finished
*/
public boolean isFinished() {
return pBackup == null;
}
void checkInit() throws ConnException {
if (isFinished()) {
throw new ConnException(dst, "backup already finished", ErrCodes.WRAPPER_SPECIFIC);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy