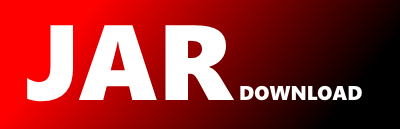
com.github.gwtbootstrap.client.ui.IconCell Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gwt-bootstrap Show documentation
Show all versions of gwt-bootstrap Show documentation
A GWT Library that provides the widgets of Twitter Bootstrap.
The newest version!
package com.github.gwtbootstrap.client.ui;
import com.github.gwtbootstrap.client.ui.constants.Alignment;
import com.github.gwtbootstrap.client.ui.constants.IconFlip;
import com.github.gwtbootstrap.client.ui.constants.IconRotate;
import com.github.gwtbootstrap.client.ui.constants.IconSize;
import com.github.gwtbootstrap.client.ui.constants.IconType;
import com.google.gwt.cell.client.AbstractCell;
import com.google.gwt.safehtml.shared.SafeHtmlBuilder;
/**
* A IconCell supporting icon type, icon size, light, mute, border, spin, alignment, rotate, flip and tooltip
*
* Example
*
*
* {@code
* final IconCell iCell = new IconCell(IconType.INFO_SIGN, IconSize.LARGE);
*
* Column iconColumn = new Column(iCell) {
*
* public Void getValue(OneObject object) {
* if (object.getOneValue() > 0) {
* iCell.setIconType(IconType.PLUS_SIGN);
* iCell.setTooltip("High");
* }
* if (object.getOneValue() < 0) {
* iCell.setIconType(IconType.MINUS_SIGN);
* iCell.setTooltip("Low");
* }
* return null;
* }
* };
* }
*
*
*/
public class IconCell extends AbstractCell {
private IconType iconType;
private IconSize iconSize;
private boolean light;
private boolean muted;
private boolean border;
private boolean spin;
private Alignment alignment;
private IconRotate iconRotate;
private IconFlip iconFlip;
private String tooltip;
/**
* Construct a new {@link IconCell} with the specified icon type
*
* @param iconType
*/
public IconCell(IconType iconType) {
this(iconType, IconSize.DEFAULT);
}
/**
* Construct a new {@link IconCell} with the specified icon type and icon size
*
* @param iconType
* @param iconSize
*/
public IconCell(IconType iconType, IconSize iconSize) {
this.iconType = iconType;
this.iconSize = iconSize;
light = false;
muted = false;
border = false;
spin = false;
alignment = Alignment.NONE;
iconRotate = IconRotate.NONE;
iconFlip = IconFlip.NONE;
}
@Override
public void render(Context context, Void value, SafeHtmlBuilder sb) {
StringBuilder builder = new StringBuilder();
builder.append("");
sb.appendHtmlConstant(builder.toString());
}
public IconType getIconType() {
return iconType;
}
public void setIconType(IconType iconType) {
this.iconType = iconType;
}
public IconSize getIconSize() {
return iconSize;
}
public void setIconSize(IconSize iconSize) {
this.iconSize = iconSize;
}
public boolean isLight() {
return light;
}
public void setLight(boolean light) {
this.light = light;
}
public boolean isMuted() {
return muted;
}
public void setMuted(boolean muted) {
this.muted = muted;
}
public boolean isBorder() {
return border;
}
public void setBorder(boolean border) {
this.border = border;
}
public boolean isSpin() {
return spin;
}
public void setSpin(boolean spin) {
this.spin = spin;
}
public Alignment getAlignment() {
return alignment;
}
public void setAlignment(Alignment alignment) {
this.alignment = alignment;
}
public IconRotate getIconRotate() {
return iconRotate;
}
public void setIconRotate(IconRotate iconRotate) {
this.iconRotate = iconRotate;
}
public IconFlip getIconFlip() {
return iconFlip;
}
public void setIconFlip(IconFlip iconFlip) {
this.iconFlip = iconFlip;
}
public String getTooltip() {
return tooltip;
}
public void setTooltip(String tooltip) {
this.tooltip = tooltip;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy