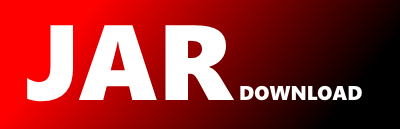
gwt.material.design.incubator.client.keyboard.ScreenKeyboard Maven / Gradle / Ivy
/*
* #%L
* GwtMaterial
* %%
* Copyright (C) 2015 - 2019 GwtMaterialDesign
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
package gwt.material.design.incubator.client.keyboard;
import com.google.gwt.dom.client.Document;
import com.google.gwt.dom.client.Element;
import com.google.gwt.event.shared.HandlerRegistration;
import gwt.material.design.client.MaterialDesign;
import gwt.material.design.client.base.MaterialWidget;
import gwt.material.design.incubator.client.dark.IncubatorDarkThemeReloader;
import gwt.material.design.incubator.client.keyboard.events.*;
import gwt.material.design.incubator.client.keyboard.js.Keyboard;
import gwt.material.design.incubator.client.keyboard.js.KeyboardOptions;
import gwt.material.design.incubator.client.keyboard.js.SimpleKeyboard;
import gwt.material.design.jquery.client.api.Functions;
import gwt.material.design.jquery.client.api.JQuery;
/**
* On Screen Keyboard support
*
* @author kevzlou7979
* @see Website
* @see Documentation
* @see Demos
*/
public class ScreenKeyboard extends MaterialWidget implements HasScreenKeyboardHandlers {
static {
MaterialDesign.injectCss(ScreenKeyboardClientBundle.INSTANCE.screenKeyboardCss());
MaterialDesign.injectCss(ScreenKeyboardClientBundle.INSTANCE.screenKeyboardCustomCss());
MaterialDesign.injectDebugJs(ScreenKeyboardClientBundle.INSTANCE.screenKeyboardJs());
}
private static final String KEYBOARD_PROPERTY = "Keyboard";
private static final String SIMPLE_KEYBOARD_PROPERTY = "SimpleKeyboard";
protected Keyboard keyboard;
protected KeyboardOptions options = new KeyboardOptions();
public ScreenKeyboard() {
super(Document.get().createDivElement(), "simple-keyboard");
}
@Override
protected void onLoad() {
super.onLoad();
load();
}
protected void load() {
// Initial SimpleKeyboard Defaults
SimpleKeyboard simpleKeyboard = (SimpleKeyboard) JQuery.window().getPropertyObject(SIMPLE_KEYBOARD_PROPERTY);
JQuery.window().setPropertyObject(KEYBOARD_PROPERTY, simpleKeyboard._default);
if (options != null) {
// Setup events
options.beforeFirstRender = () -> BeforeFirstRenderEvent.fire(this);
options.beforeRender = () -> BeforeRenderEvent.fire(this);
options.onRender = () -> RenderEvent.fire(this);
options.onInit = () -> InitEvent.fire(this);
options.onKeyPress = button -> KeyPressEvent.fire(this, button);
options.onChange = input -> ChangeEvent.fire(this, input);
options.onChangeAll = inputs -> ChangeAllEvent.fire(this, inputs._default);
// Construct Keyboard with required options
keyboard = new Keyboard(options);
}
IncubatorDarkThemeReloader.get().reload(ScreenKeyboardDarkTheme.class);
}
/**
* Clear the keyboard’s input.
*/
public void clearInput() {
getKeyboard().clearInput();
}
/**
* Clear the keyboard’s specific input
* Must have been previously set using the "inputName" prop.
*/
public void clearInput(String inputName) {
getKeyboard().clearInput(inputName);
}
/**
* Get the keyboard’s input (You can also get it from the onChange prop).
*/
public String getInput() {
return getKeyboard().getInput();
}
/**
* Get the keyboard’s input (You can also get it from the onChange prop).
* Must have been previously set using the "inputName" prop.
*/
public String getInput(String inputName) {
return getKeyboard().getInput(inputName);
}
/**
* Set the keyboard’s input. Useful if you want to track input changes made outside simple-keyboard.
*/
public void setInput(String input) {
getKeyboard().setInput(input);
}
/**
* Set the keyboard’s input. Useful if you want to track input changes made outside simple-keyboard.
* Must have been previously set using the "inputName" prop.
*/
public void setInput(String input, String inputName) {
getKeyboard().setInput(input, inputName);
}
/**
* Replaces the entire internal input object. The difference with “setInput” is that “setInput” only changes a
* single key of the input object, while “replaceInput” replaces the full input object.
*/
public void replaceInput(Object object) {
getKeyboard().replaceInput(object);
}
/**
* Set new option or modify existing ones after initialization. The changes are applied immediately.
*/
public void updateOptions(KeyboardOptions options) {
getKeyboard().setOptions(options);
}
/**
* Send a command to all simple-keyboard instances at once (if you have multiple instances).
*/
public void dispatch(Functions.Func1
© 2015 - 2025 Weber Informatics LLC | Privacy Policy