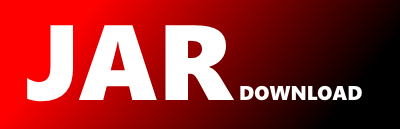
gwt.react.client.components.ReactClassSpec Maven / Gradle / Ivy
package gwt.react.client.components;
import gwt.react.client.api.React;
import gwt.react.client.elements.ReactElement;
import gwt.react.client.proptypes.BaseProps;
import gwt.react.client.utils.ObjLiteral;
import jsinterop.annotations.JsType;
/**
* You can Subclass AbstractReactClassSpec and pass an instance of this class to {@link React#createClass}
*
* In addition to the methods defined in this class, you can also define the following lifecyle
* methods as needed
*
* void componentWillMount()
* void componentDidMount()
* void componentWillReceiveProps(P nextProps)
* boolean shouldComponentUpdate(P nextProps, S nextState)
* void componentWillUpdate(P nextProps, S nextState)
* void componentDidUpdate(P prevProps, S prevState)
* void componentWillUnmount()
*
* @param the type of props this component expects
* @param the type of state this component maintains
*/
@JsType
abstract public class ReactClassSpec
extends ClassicComponentApi
{
/**
* Invoked once before the component is mounted. The return value will be used as the initial value of
* this.state.
*
* @return The state or null if this component maintains no state
*/
abstract public S getInitialState();
/**
* The render() method is required.
*
*
When called, it should examine {@link ClassicComponentApi#getProps} and
* {@link ClassicComponentApi#getState} and return a single child element.This
* child element can be either a virtual representation of a native DOM component
* (such as React.DOM.div()) or another composite component that you've defined
* yourself.
*
* You can also return null to indicate that you don't want anything rendered.
* Behind the scenes, React renders a <noscript> tag to work with our current diffing
* algorithm.
*
* The render() function should be pure, meaning that it does not modify component
* state, it returns the same result each time it's invoked, and it does not read from
* or write to the DOM or otherwise interact with the browser (e.g., by using setTimeout).
* If you need to interact with the browser, perform your work in componentDidMount() or
* the other lifecycle methods instead. Keeping render() pure makes components easier to
* think about.
*
* @return A single {@link ReactElement}
*/
abstract public ReactElement render();
}