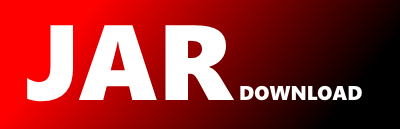
com.zaxxer.sansorm.OrmElf Maven / Gradle / Ivy
Show all versions of q2o Show documentation
/*
Copyright 2012, Brett Wooldridge
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package com.zaxxer.sansorm;
import com.zaxxer.q2o.Q2Obj;
import com.zaxxer.q2o.Q2ObjList;
import com.zaxxer.q2o.Q2Sql;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.List;
import java.util.Set;
/**
* OrmElf
* @deprecated
*/
//CHECKSTYLE:OFF
@SuppressWarnings({"WeakerAccess", "unused"})
public final class OrmElf
{
/**
* Private constructor.
*/
private OrmElf() {
// private constructor
}
// ------------------------------------------------------------------------
// Read Methods
// ------------------------------------------------------------------------
/**
* Load an object by it's ID. The @Id annotated field(s) of the object is used to
* set query parameters.
*
* @param connection a SQL Connection object
* @param clazz the class of the object to load
* @param args the query parameter used to find the object by it's ID
* @param the type of the object to load
* @return the populated object
* @throws SQLException if a {@link SQLException} occurs
* @deprecated
*/
public static T objectById(Connection connection, Class clazz, Object... args) throws SQLException
{
return Q2Obj.byId(connection, clazz, args);
}
/**
* Load an object using the specified clause. If the specified clause contains the text
* "WHERE" or "JOIN", the clause is appended directly to the generated "SELECT .. FROM" SQL.
* However, if the clause contains neither "WHERE" nor "JOIN", it is assumed to be
* just be the conditional portion that would normally appear after the "WHERE", and therefore
* the clause "WHERE" is automatically appended to the generated "SELECT .. FROM" SQL, followed
* by the specified clause. For example:
* {@code User user = OrmElf.objectFromClause(connection, User.class, "username=?", userName);}
*
* @param connection a SQL Connection object
* @param clazz the class of the object to load
* @param clause the conditional part of a SQL where clause
* @param args the query parameters used to find the object
* @param the type of the object to load
* @return the populated object
* @throws SQLException if a {@link SQLException} occurs
* @deprecated
*/
public static T objectFromClause(Connection connection, Class clazz, String clause, Object... args) throws SQLException
{
return Q2Obj.fromClause(connection, clazz, clause, args);
}
/**
* Load a list of objects using the specified where condition. The clause "WHERE" is automatically
* appended, so the {@code where} parameter should just be the conditional portion.
*
* If the {@code where} parameter is {@code null} a select of every object from the
* table mapped for the specified class is executed.
*
* @param connection a SQL Connection object
* @param clazz the class of the object to load
* @param clause the conditional part of a SQL where clause
* @param args the query parameters used to find the list of objects
* @param the type of the object to load
* @return a list of populated objects
* @throws SQLException if a {@link SQLException} occurs
* @deprecated
*/
public static List listFromClause(Connection connection, Class clazz, String clause, Object... args) throws SQLException
{
return Q2ObjList.fromClause(connection, clazz, clause, args);
}
/**
* Counts the number of rows for the given query.
*
* @param connection a SQL connection object.
* @param clazz the class of the object to query.
* @param clause The conditional part of a SQL where clause.
* @param args The query parameters used to find the list of objects.
* @param the type of object to query.
* @return The result count.
* @throws SQLException if a {@link SQLException} occurs
* @deprecated
*/
public static int countObjectsFromClause(Connection connection, Class clazz, String clause, Object... args) throws SQLException
{
return Q2Obj.countFromClause(connection, clazz, clause, args);
}
/**
* This method takes a PreparedStatement, a target class, and optional arguments to set
* as query parameters. It sets the parameters automatically, executes the query, and
* constructs and populates an instance of the target class. The PreparedStatement will be closed.
*
* @param stmt the PreparedStatement to execute to construct an object
* @param clazz the class of the object to instantiate and populate with state
* @param args optional arguments to set as query parameters in the PreparedStatement
* @param the class template
* @return the populated object
* @throws SQLException if a {@link SQLException} occurs
* @deprecated
*/
public static T statementToObject(PreparedStatement stmt, Class clazz, Object... args) throws SQLException
{
return Q2Obj.fromStatement(stmt, clazz, args);
}
/**
* Execute a prepared statement (query) with the supplied args set as query parameters (if specified), and
* return a list of objects as a result. The PreparedStatement will be closed.
*
* @param stmt the PreparedStatement to execute
* @param clazz the class of the objects to instantiate and populate with state
* @param args optional arguments to set as query parameters in the PreparedStatement
* @param the class template
* @return a list of instance of the target class, or an empty list
* @throws SQLException if a {@link SQLException} occurs
* @deprecated
*/
public static List statementToList(PreparedStatement stmt, Class clazz, Object... args) throws SQLException
{
return Q2ObjList.fromStatement(stmt, clazz, args);
}
/**
* Get an object from the specified ResultSet. ResultSet.next() is NOT called,
* this should be done by the caller. The ResultSet is not closed as a result of this
* method.
*
* @param resultSet a {@link ResultSet}
* @param target the target object to set values on
* @param the class template
* @return the populated object
* @throws SQLException if a {@link SQLException} occurs
* @deprecated
*/
public static T resultSetToObject(ResultSet resultSet, T target) throws SQLException
{
return Q2Obj.fromResultSet(resultSet, target);
}
/**
* Get an object from the specified ResultSet. ResultSet.next() is NOT called,
* this should be done by the caller. The ResultSet is not closed as a result of this
* method.
*
* @param resultSet a {@link ResultSet}
* @param target the target object to set values on
* @param ignoredColumns the columns in the result set to ignore. Case as in name element or property name.
* @param the class template
* @return the populated object
* @throws SQLException if a {@link SQLException} occurs
* @deprecated
*/
public static T resultSetToObject(ResultSet resultSet, T target, Set ignoredColumns) throws SQLException
{
return Q2Obj.fromResultSet(resultSet, target, ignoredColumns);
}
/**
* This method will iterate over a ResultSet that contains columns that map to the
* target class and return a list of target instances. Note, this assumes that
* ResultSet.next() has NOT been called before calling this method.
*
* The entire ResultSet will be consumed and closed.
*
* @param resultSet a {@link ResultSet}
* @param targetClass the target class
* @param the class template
* @return a list of instance of the target class, or an empty list
* @throws SQLException if a {@link SQLException} occurs
* @deprecated
*/
public static List resultSetToList(ResultSet resultSet, Class targetClass) throws SQLException
{
return Q2ObjList.fromResultSet(resultSet, targetClass);
}
// ------------------------------------------------------------------------
// Write Methods
// ------------------------------------------------------------------------
/**
* Insert a collection of objects in a non-batched manner (i.e. using iteration and individual INSERTs).
*
* @param connection a SQL connection
* @param iterable a list (or other {@link Iterable} collection) of annotated objects to insert
* @param the class template
* @throws SQLException if a {@link SQLException} occurs
* @deprecated
*/
public static void insertListNotBatched(Connection connection, Iterable iterable) throws SQLException
{
Q2ObjList.insertNotBatched(connection, iterable);
}
/**
* Insert a collection of objects using JDBC batching.
*
* @param connection a SQL connection
* @param iterable a list (or other {@link Iterable} collection) of annotated objects to insert
* @param the class template
* @throws SQLException if a {@link SQLException} occurs
* @deprecated
*/
public static void insertListBatched(Connection connection, Iterable iterable) throws SQLException
{
Q2ObjList.insertBatched(connection, iterable);
}
/**
* Insert an annotated object into the database.
*
* @param connection a SQL connection
* @param target the annotated object to insert
* @param the class template
* @return the same object that was passed in, but with possibly updated @Id field due to auto-generated keys
* @throws SQLException if a {@link SQLException} occurs
* @deprecated
*/
public static T insertObject(Connection connection, T target) throws SQLException
{
return Q2Obj.insert(connection, target);
}
/**
* Update a database row using the specified annotated object, the @Id field(s) is used in the WHERE
* clause of the generated UPDATE statement.
*
* @param connection a SQL connection
* @param target the annotated object to use to update a row in the database
* @param the class template
* @return the same object passed in
* @throws SQLException if a {@link SQLException} occurs
* @deprecated
*/
public static T updateObject(Connection connection, T target) throws SQLException
{
return Q2Obj.update(connection, target);
}
/**
* @deprecated
*/
public static T updateObject(Connection connection, T target, String... excludedColumns) throws SQLException
{
return Q2Obj.updateExcludeColumns(connection, target, excludedColumns);
}
/**
* Delete a database row using the specified annotated object, the @Id field(s) is used in the WHERE
* clause of the generated DELETE statement.
*
* @param connection a SQL connection
* @param target the annotated object to use to delete a row in the database
* @param the class template
* @return 0 if no row was deleted, 1 if the row was deleted
* @throws SQLException if a {@link SQLException} occurs
* @deprecated
*/
public static int deleteObject(Connection connection, T target) throws SQLException
{
return Q2Obj.delete(connection, target);
}
/**
* @deprecated
*/
public static int deleteObjectById(Connection connection, Class clazz, Object... args) throws SQLException
{
return Q2Obj.deleteById(connection, clazz, args);
}
// ------------------------------------------------------------------------
// Utility Methods
// ------------------------------------------------------------------------
/**
* Gets the column name defined for the given property for the given type.
*
* @param clazz The type.
* @param propertyName The object property name.
* @return The database column name.
* @deprecated
*/
public static String getColumnFromProperty(Class> clazz, String propertyName)
{
return Q2Sql.getColumnFromProperty(clazz, propertyName);
}
/**
* Get a comma separated values list of column names for the given class, suitable
* for inclusion into a SQL SELECT statement.
*
* @param clazz the annotated class
* @param tablePrefix an optional table prefix to append to each column
* @param the class template
* @return a CSV of annotated column names
* @deprecated
*/
public static String getColumnsCsv(Class clazz, String... tablePrefix)
{
return Q2Sql.getColumnsCsv(clazz, tablePrefix);
}
/**
* Get a comma separated values list of column names for the given class -- excluding
* the column names specified, suitable for inclusion into a SQL SELECT statement.
* Note the excluded column names must exactly match annotated column names in the class
* in a case-sensitive manner.
*
* @param clazz the annotated class
* @param excludeColumns optional columns to exclude from the returned list of columns
* @param the class template
* @return a CSV of annotated column names
* @deprecated
*/
public static String getColumnsCsvExclude(Class clazz, String... excludeColumns)
{
return Q2Sql.getColumnsCsvExclude(clazz, excludeColumns);
}
/**
* To refresh all fields in case they have changed in database.
*
* @param connection a SQL connection
* @param target an annotated object with at least all @Id fields set.
* @return the target object with all values updated or null if the object was not found anymore.
* @throws SQLException if a {@link SQLException} occurs
* @param the type of the target object
* @deprecated
*/
public static T refresh(Connection connection, T target) throws SQLException {
return Q2Obj.refresh(connection, target);
}
}