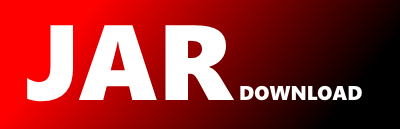
com.google.gwt.angular.client.AngularController Maven / Gradle / Ivy
package com.google.gwt.angular.client;
import com.google.gwt.core.client.JavaScriptObject;
import elemental.util.ArrayOf;
import java.util.Iterator;
/**
* Base class for all implementations of AngularJs conntrollers in GWT. To use,
* subclass {@code AngularController} and define an onInit
method taking at least one
* {@link Scope} object. Each parameter of the onInit
method is assumed to be an injectable type, either
* a builtin type, or a custom injectable type defined by {@link NgInject}.
*
* Any additional public methods defined will automatically be exported to the $scope
object used by
* the controller and callable from the view.
*
*
* public class MyController extends AngularController {
* public void onInit(Scope scope) {
* // publish key "foo" with value "bar" into $scope
* scope.put("foo", "bar");
* }
*
* // automatically published as $scope.doIt
* public void doIt(String arg) {
* Window.alert(scope.getString("foo") + " " + arg);
* }
* }
*
*
* See the documentation for {@link Scope} for how to define custom Scope subtypes with type-safe accessors.
*
* @author Ray Cromwell ([email protected])
*/
public class AngularController {
// convenience variable for accessing scope in published controller methods
protected T scope;
protected AngularController() {
}
protected void setScope(T scope) {
this.scope = scope;
}
protected void register(JavaScriptObject module) {
}
protected Iterable iterable(final ArrayOf array) {
return new Iterable() {
public Iterator iterator() {
return new Iterator() {
int next = 0;
public boolean hasNext() {
return next < array.length();
}
public S next() {
return array.get(next++);
}
public void remove() {
}
};
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy