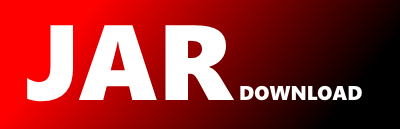
net.ninety.bitmex.model.Instrument Maven / Gradle / Ivy
/*
* BitMEX API
* ## REST API for the BitMEX Trading Platform [View Changelog](/app/apiChangelog) --- #### Getting Started Base URI: [https://www.bitmex.com/api/v1](/api/v1) ##### Fetching Data All REST endpoints are documented below. You can try out any query right from this interface. Most table queries accept `count`, `start`, and `reverse` params. Set `reverse=true` to get rows newest-first. Additional documentation regarding filters, timestamps, and authentication is available in [the main API documentation](/app/restAPI). _All_ table data is available via the [Websocket](/app/wsAPI). We highly recommend using the socket if you want to have the quickest possible data without being subject to ratelimits. ##### Return Types By default, all data is returned as JSON. Send `?_format=csv` to get CSV data or `?_format=xml` to get XML data. ##### Trade Data Queries _This is only a small subset of what is available, to get you started._ Fill in the parameters and click the `Try it out!` button to try any of these queries. - [Pricing Data](#!/Quote/Quote_get) - [Trade Data](#!/Trade/Trade_get) - [OrderBook Data](#!/OrderBook/OrderBook_getL2) - [Settlement Data](#!/Settlement/Settlement_get) - [Exchange Statistics](#!/Stats/Stats_history) Every function of the BitMEX.com platform is exposed here and documented. Many more functions are available. ##### Swagger Specification [⇩ Download Swagger JSON](swagger.json) --- ## All API Endpoints Click to expand a section.
*
* OpenAPI spec version: 1.2.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package net.ninety.bitmex.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.math.BigDecimal;
import org.threeten.bp.OffsetDateTime;
/**
* Tradeable Contracts, Indices, and History
*/
@ApiModel(description = "Tradeable Contracts, Indices, and History")
@javax.annotation.Generated(value = "io.swagger.codegen.languages.JavaClientCodegen", date = "2019-11-02T12:22:57.745Z")
public class Instrument {
@SerializedName("symbol")
private String symbol = null;
@SerializedName("rootSymbol")
private String rootSymbol = null;
@SerializedName("state")
private String state = null;
@SerializedName("typ")
private String typ = null;
@SerializedName("listing")
private OffsetDateTime listing = null;
@SerializedName("front")
private OffsetDateTime front = null;
@SerializedName("expiry")
private OffsetDateTime expiry = null;
@SerializedName("settle")
private OffsetDateTime settle = null;
@SerializedName("relistInterval")
private OffsetDateTime relistInterval = null;
@SerializedName("inverseLeg")
private String inverseLeg = null;
@SerializedName("sellLeg")
private String sellLeg = null;
@SerializedName("buyLeg")
private String buyLeg = null;
@SerializedName("optionStrikePcnt")
private Double optionStrikePcnt = null;
@SerializedName("optionStrikeRound")
private Double optionStrikeRound = null;
@SerializedName("optionStrikePrice")
private Double optionStrikePrice = null;
@SerializedName("optionMultiplier")
private Double optionMultiplier = null;
@SerializedName("positionCurrency")
private String positionCurrency = null;
@SerializedName("underlying")
private String underlying = null;
@SerializedName("quoteCurrency")
private String quoteCurrency = null;
@SerializedName("underlyingSymbol")
private String underlyingSymbol = null;
@SerializedName("reference")
private String reference = null;
@SerializedName("referenceSymbol")
private String referenceSymbol = null;
@SerializedName("calcInterval")
private OffsetDateTime calcInterval = null;
@SerializedName("publishInterval")
private OffsetDateTime publishInterval = null;
@SerializedName("publishTime")
private OffsetDateTime publishTime = null;
@SerializedName("maxOrderQty")
private BigDecimal maxOrderQty = null;
@SerializedName("maxPrice")
private Double maxPrice = null;
@SerializedName("lotSize")
private BigDecimal lotSize = null;
@SerializedName("tickSize")
private Double tickSize = null;
@SerializedName("multiplier")
private BigDecimal multiplier = null;
@SerializedName("settlCurrency")
private String settlCurrency = null;
@SerializedName("underlyingToPositionMultiplier")
private BigDecimal underlyingToPositionMultiplier = null;
@SerializedName("underlyingToSettleMultiplier")
private BigDecimal underlyingToSettleMultiplier = null;
@SerializedName("quoteToSettleMultiplier")
private BigDecimal quoteToSettleMultiplier = null;
@SerializedName("isQuanto")
private Boolean isQuanto = null;
@SerializedName("isInverse")
private Boolean isInverse = null;
@SerializedName("initMargin")
private Double initMargin = null;
@SerializedName("maintMargin")
private Double maintMargin = null;
@SerializedName("riskLimit")
private BigDecimal riskLimit = null;
@SerializedName("riskStep")
private BigDecimal riskStep = null;
@SerializedName("limit")
private Double limit = null;
@SerializedName("capped")
private Boolean capped = null;
@SerializedName("taxed")
private Boolean taxed = null;
@SerializedName("deleverage")
private Boolean deleverage = null;
@SerializedName("makerFee")
private Double makerFee = null;
@SerializedName("takerFee")
private Double takerFee = null;
@SerializedName("settlementFee")
private Double settlementFee = null;
@SerializedName("insuranceFee")
private Double insuranceFee = null;
@SerializedName("fundingBaseSymbol")
private String fundingBaseSymbol = null;
@SerializedName("fundingQuoteSymbol")
private String fundingQuoteSymbol = null;
@SerializedName("fundingPremiumSymbol")
private String fundingPremiumSymbol = null;
@SerializedName("fundingTimestamp")
private OffsetDateTime fundingTimestamp = null;
@SerializedName("fundingInterval")
private OffsetDateTime fundingInterval = null;
@SerializedName("fundingRate")
private Double fundingRate = null;
@SerializedName("indicativeFundingRate")
private Double indicativeFundingRate = null;
@SerializedName("rebalanceTimestamp")
private OffsetDateTime rebalanceTimestamp = null;
@SerializedName("rebalanceInterval")
private OffsetDateTime rebalanceInterval = null;
@SerializedName("openingTimestamp")
private OffsetDateTime openingTimestamp = null;
@SerializedName("closingTimestamp")
private OffsetDateTime closingTimestamp = null;
@SerializedName("sessionInterval")
private OffsetDateTime sessionInterval = null;
@SerializedName("prevClosePrice")
private Double prevClosePrice = null;
@SerializedName("limitDownPrice")
private Double limitDownPrice = null;
@SerializedName("limitUpPrice")
private Double limitUpPrice = null;
@SerializedName("bankruptLimitDownPrice")
private Double bankruptLimitDownPrice = null;
@SerializedName("bankruptLimitUpPrice")
private Double bankruptLimitUpPrice = null;
@SerializedName("prevTotalVolume")
private BigDecimal prevTotalVolume = null;
@SerializedName("totalVolume")
private BigDecimal totalVolume = null;
@SerializedName("volume")
private BigDecimal volume = null;
@SerializedName("volume24h")
private BigDecimal volume24h = null;
@SerializedName("prevTotalTurnover")
private BigDecimal prevTotalTurnover = null;
@SerializedName("totalTurnover")
private BigDecimal totalTurnover = null;
@SerializedName("turnover")
private BigDecimal turnover = null;
@SerializedName("turnover24h")
private BigDecimal turnover24h = null;
@SerializedName("homeNotional24h")
private Double homeNotional24h = null;
@SerializedName("foreignNotional24h")
private Double foreignNotional24h = null;
@SerializedName("prevPrice24h")
private Double prevPrice24h = null;
@SerializedName("vwap")
private Double vwap = null;
@SerializedName("highPrice")
private Double highPrice = null;
@SerializedName("lowPrice")
private Double lowPrice = null;
@SerializedName("lastPrice")
private Double lastPrice = null;
@SerializedName("lastPriceProtected")
private Double lastPriceProtected = null;
@SerializedName("lastTickDirection")
private String lastTickDirection = null;
@SerializedName("lastChangePcnt")
private Double lastChangePcnt = null;
@SerializedName("bidPrice")
private Double bidPrice = null;
@SerializedName("midPrice")
private Double midPrice = null;
@SerializedName("askPrice")
private Double askPrice = null;
@SerializedName("impactBidPrice")
private Double impactBidPrice = null;
@SerializedName("impactMidPrice")
private Double impactMidPrice = null;
@SerializedName("impactAskPrice")
private Double impactAskPrice = null;
@SerializedName("hasLiquidity")
private Boolean hasLiquidity = null;
@SerializedName("openInterest")
private BigDecimal openInterest = null;
@SerializedName("openValue")
private BigDecimal openValue = null;
@SerializedName("fairMethod")
private String fairMethod = null;
@SerializedName("fairBasisRate")
private Double fairBasisRate = null;
@SerializedName("fairBasis")
private Double fairBasis = null;
@SerializedName("fairPrice")
private Double fairPrice = null;
@SerializedName("markMethod")
private String markMethod = null;
@SerializedName("markPrice")
private Double markPrice = null;
@SerializedName("indicativeTaxRate")
private Double indicativeTaxRate = null;
@SerializedName("indicativeSettlePrice")
private Double indicativeSettlePrice = null;
@SerializedName("optionUnderlyingPrice")
private Double optionUnderlyingPrice = null;
@SerializedName("settledPrice")
private Double settledPrice = null;
@SerializedName("timestamp")
private OffsetDateTime timestamp = null;
public Instrument symbol(String symbol) {
this.symbol = symbol;
return this;
}
/**
* Get symbol
* @return symbol
**/
@ApiModelProperty(required = true, value = "")
public String getSymbol() {
return symbol;
}
public void setSymbol(String symbol) {
this.symbol = symbol;
}
public Instrument rootSymbol(String rootSymbol) {
this.rootSymbol = rootSymbol;
return this;
}
/**
* Get rootSymbol
* @return rootSymbol
**/
@ApiModelProperty(value = "")
public String getRootSymbol() {
return rootSymbol;
}
public void setRootSymbol(String rootSymbol) {
this.rootSymbol = rootSymbol;
}
public Instrument state(String state) {
this.state = state;
return this;
}
/**
* Get state
* @return state
**/
@ApiModelProperty(value = "")
public String getState() {
return state;
}
public void setState(String state) {
this.state = state;
}
public Instrument typ(String typ) {
this.typ = typ;
return this;
}
/**
* Get typ
* @return typ
**/
@ApiModelProperty(value = "")
public String getTyp() {
return typ;
}
public void setTyp(String typ) {
this.typ = typ;
}
public Instrument listing(OffsetDateTime listing) {
this.listing = listing;
return this;
}
/**
* Get listing
* @return listing
**/
@ApiModelProperty(value = "")
public OffsetDateTime getListing() {
return listing;
}
public void setListing(OffsetDateTime listing) {
this.listing = listing;
}
public Instrument front(OffsetDateTime front) {
this.front = front;
return this;
}
/**
* Get front
* @return front
**/
@ApiModelProperty(value = "")
public OffsetDateTime getFront() {
return front;
}
public void setFront(OffsetDateTime front) {
this.front = front;
}
public Instrument expiry(OffsetDateTime expiry) {
this.expiry = expiry;
return this;
}
/**
* Get expiry
* @return expiry
**/
@ApiModelProperty(value = "")
public OffsetDateTime getExpiry() {
return expiry;
}
public void setExpiry(OffsetDateTime expiry) {
this.expiry = expiry;
}
public Instrument settle(OffsetDateTime settle) {
this.settle = settle;
return this;
}
/**
* Get settle
* @return settle
**/
@ApiModelProperty(value = "")
public OffsetDateTime getSettle() {
return settle;
}
public void setSettle(OffsetDateTime settle) {
this.settle = settle;
}
public Instrument relistInterval(OffsetDateTime relistInterval) {
this.relistInterval = relistInterval;
return this;
}
/**
* Get relistInterval
* @return relistInterval
**/
@ApiModelProperty(value = "")
public OffsetDateTime getRelistInterval() {
return relistInterval;
}
public void setRelistInterval(OffsetDateTime relistInterval) {
this.relistInterval = relistInterval;
}
public Instrument inverseLeg(String inverseLeg) {
this.inverseLeg = inverseLeg;
return this;
}
/**
* Get inverseLeg
* @return inverseLeg
**/
@ApiModelProperty(value = "")
public String getInverseLeg() {
return inverseLeg;
}
public void setInverseLeg(String inverseLeg) {
this.inverseLeg = inverseLeg;
}
public Instrument sellLeg(String sellLeg) {
this.sellLeg = sellLeg;
return this;
}
/**
* Get sellLeg
* @return sellLeg
**/
@ApiModelProperty(value = "")
public String getSellLeg() {
return sellLeg;
}
public void setSellLeg(String sellLeg) {
this.sellLeg = sellLeg;
}
public Instrument buyLeg(String buyLeg) {
this.buyLeg = buyLeg;
return this;
}
/**
* Get buyLeg
* @return buyLeg
**/
@ApiModelProperty(value = "")
public String getBuyLeg() {
return buyLeg;
}
public void setBuyLeg(String buyLeg) {
this.buyLeg = buyLeg;
}
public Instrument optionStrikePcnt(Double optionStrikePcnt) {
this.optionStrikePcnt = optionStrikePcnt;
return this;
}
/**
* Get optionStrikePcnt
* @return optionStrikePcnt
**/
@ApiModelProperty(value = "")
public Double getOptionStrikePcnt() {
return optionStrikePcnt;
}
public void setOptionStrikePcnt(Double optionStrikePcnt) {
this.optionStrikePcnt = optionStrikePcnt;
}
public Instrument optionStrikeRound(Double optionStrikeRound) {
this.optionStrikeRound = optionStrikeRound;
return this;
}
/**
* Get optionStrikeRound
* @return optionStrikeRound
**/
@ApiModelProperty(value = "")
public Double getOptionStrikeRound() {
return optionStrikeRound;
}
public void setOptionStrikeRound(Double optionStrikeRound) {
this.optionStrikeRound = optionStrikeRound;
}
public Instrument optionStrikePrice(Double optionStrikePrice) {
this.optionStrikePrice = optionStrikePrice;
return this;
}
/**
* Get optionStrikePrice
* @return optionStrikePrice
**/
@ApiModelProperty(value = "")
public Double getOptionStrikePrice() {
return optionStrikePrice;
}
public void setOptionStrikePrice(Double optionStrikePrice) {
this.optionStrikePrice = optionStrikePrice;
}
public Instrument optionMultiplier(Double optionMultiplier) {
this.optionMultiplier = optionMultiplier;
return this;
}
/**
* Get optionMultiplier
* @return optionMultiplier
**/
@ApiModelProperty(value = "")
public Double getOptionMultiplier() {
return optionMultiplier;
}
public void setOptionMultiplier(Double optionMultiplier) {
this.optionMultiplier = optionMultiplier;
}
public Instrument positionCurrency(String positionCurrency) {
this.positionCurrency = positionCurrency;
return this;
}
/**
* Get positionCurrency
* @return positionCurrency
**/
@ApiModelProperty(value = "")
public String getPositionCurrency() {
return positionCurrency;
}
public void setPositionCurrency(String positionCurrency) {
this.positionCurrency = positionCurrency;
}
public Instrument underlying(String underlying) {
this.underlying = underlying;
return this;
}
/**
* Get underlying
* @return underlying
**/
@ApiModelProperty(value = "")
public String getUnderlying() {
return underlying;
}
public void setUnderlying(String underlying) {
this.underlying = underlying;
}
public Instrument quoteCurrency(String quoteCurrency) {
this.quoteCurrency = quoteCurrency;
return this;
}
/**
* Get quoteCurrency
* @return quoteCurrency
**/
@ApiModelProperty(value = "")
public String getQuoteCurrency() {
return quoteCurrency;
}
public void setQuoteCurrency(String quoteCurrency) {
this.quoteCurrency = quoteCurrency;
}
public Instrument underlyingSymbol(String underlyingSymbol) {
this.underlyingSymbol = underlyingSymbol;
return this;
}
/**
* Get underlyingSymbol
* @return underlyingSymbol
**/
@ApiModelProperty(value = "")
public String getUnderlyingSymbol() {
return underlyingSymbol;
}
public void setUnderlyingSymbol(String underlyingSymbol) {
this.underlyingSymbol = underlyingSymbol;
}
public Instrument reference(String reference) {
this.reference = reference;
return this;
}
/**
* Get reference
* @return reference
**/
@ApiModelProperty(value = "")
public String getReference() {
return reference;
}
public void setReference(String reference) {
this.reference = reference;
}
public Instrument referenceSymbol(String referenceSymbol) {
this.referenceSymbol = referenceSymbol;
return this;
}
/**
* Get referenceSymbol
* @return referenceSymbol
**/
@ApiModelProperty(value = "")
public String getReferenceSymbol() {
return referenceSymbol;
}
public void setReferenceSymbol(String referenceSymbol) {
this.referenceSymbol = referenceSymbol;
}
public Instrument calcInterval(OffsetDateTime calcInterval) {
this.calcInterval = calcInterval;
return this;
}
/**
* Get calcInterval
* @return calcInterval
**/
@ApiModelProperty(value = "")
public OffsetDateTime getCalcInterval() {
return calcInterval;
}
public void setCalcInterval(OffsetDateTime calcInterval) {
this.calcInterval = calcInterval;
}
public Instrument publishInterval(OffsetDateTime publishInterval) {
this.publishInterval = publishInterval;
return this;
}
/**
* Get publishInterval
* @return publishInterval
**/
@ApiModelProperty(value = "")
public OffsetDateTime getPublishInterval() {
return publishInterval;
}
public void setPublishInterval(OffsetDateTime publishInterval) {
this.publishInterval = publishInterval;
}
public Instrument publishTime(OffsetDateTime publishTime) {
this.publishTime = publishTime;
return this;
}
/**
* Get publishTime
* @return publishTime
**/
@ApiModelProperty(value = "")
public OffsetDateTime getPublishTime() {
return publishTime;
}
public void setPublishTime(OffsetDateTime publishTime) {
this.publishTime = publishTime;
}
public Instrument maxOrderQty(BigDecimal maxOrderQty) {
this.maxOrderQty = maxOrderQty;
return this;
}
/**
* Get maxOrderQty
* @return maxOrderQty
**/
@ApiModelProperty(value = "")
public BigDecimal getMaxOrderQty() {
return maxOrderQty;
}
public void setMaxOrderQty(BigDecimal maxOrderQty) {
this.maxOrderQty = maxOrderQty;
}
public Instrument maxPrice(Double maxPrice) {
this.maxPrice = maxPrice;
return this;
}
/**
* Get maxPrice
* @return maxPrice
**/
@ApiModelProperty(value = "")
public Double getMaxPrice() {
return maxPrice;
}
public void setMaxPrice(Double maxPrice) {
this.maxPrice = maxPrice;
}
public Instrument lotSize(BigDecimal lotSize) {
this.lotSize = lotSize;
return this;
}
/**
* Get lotSize
* @return lotSize
**/
@ApiModelProperty(value = "")
public BigDecimal getLotSize() {
return lotSize;
}
public void setLotSize(BigDecimal lotSize) {
this.lotSize = lotSize;
}
public Instrument tickSize(Double tickSize) {
this.tickSize = tickSize;
return this;
}
/**
* Get tickSize
* @return tickSize
**/
@ApiModelProperty(value = "")
public Double getTickSize() {
return tickSize;
}
public void setTickSize(Double tickSize) {
this.tickSize = tickSize;
}
public Instrument multiplier(BigDecimal multiplier) {
this.multiplier = multiplier;
return this;
}
/**
* Get multiplier
* @return multiplier
**/
@ApiModelProperty(value = "")
public BigDecimal getMultiplier() {
return multiplier;
}
public void setMultiplier(BigDecimal multiplier) {
this.multiplier = multiplier;
}
public Instrument settlCurrency(String settlCurrency) {
this.settlCurrency = settlCurrency;
return this;
}
/**
* Get settlCurrency
* @return settlCurrency
**/
@ApiModelProperty(value = "")
public String getSettlCurrency() {
return settlCurrency;
}
public void setSettlCurrency(String settlCurrency) {
this.settlCurrency = settlCurrency;
}
public Instrument underlyingToPositionMultiplier(BigDecimal underlyingToPositionMultiplier) {
this.underlyingToPositionMultiplier = underlyingToPositionMultiplier;
return this;
}
/**
* Get underlyingToPositionMultiplier
* @return underlyingToPositionMultiplier
**/
@ApiModelProperty(value = "")
public BigDecimal getUnderlyingToPositionMultiplier() {
return underlyingToPositionMultiplier;
}
public void setUnderlyingToPositionMultiplier(BigDecimal underlyingToPositionMultiplier) {
this.underlyingToPositionMultiplier = underlyingToPositionMultiplier;
}
public Instrument underlyingToSettleMultiplier(BigDecimal underlyingToSettleMultiplier) {
this.underlyingToSettleMultiplier = underlyingToSettleMultiplier;
return this;
}
/**
* Get underlyingToSettleMultiplier
* @return underlyingToSettleMultiplier
**/
@ApiModelProperty(value = "")
public BigDecimal getUnderlyingToSettleMultiplier() {
return underlyingToSettleMultiplier;
}
public void setUnderlyingToSettleMultiplier(BigDecimal underlyingToSettleMultiplier) {
this.underlyingToSettleMultiplier = underlyingToSettleMultiplier;
}
public Instrument quoteToSettleMultiplier(BigDecimal quoteToSettleMultiplier) {
this.quoteToSettleMultiplier = quoteToSettleMultiplier;
return this;
}
/**
* Get quoteToSettleMultiplier
* @return quoteToSettleMultiplier
**/
@ApiModelProperty(value = "")
public BigDecimal getQuoteToSettleMultiplier() {
return quoteToSettleMultiplier;
}
public void setQuoteToSettleMultiplier(BigDecimal quoteToSettleMultiplier) {
this.quoteToSettleMultiplier = quoteToSettleMultiplier;
}
public Instrument isQuanto(Boolean isQuanto) {
this.isQuanto = isQuanto;
return this;
}
/**
* Get isQuanto
* @return isQuanto
**/
@ApiModelProperty(value = "")
public Boolean isIsQuanto() {
return isQuanto;
}
public void setIsQuanto(Boolean isQuanto) {
this.isQuanto = isQuanto;
}
public Instrument isInverse(Boolean isInverse) {
this.isInverse = isInverse;
return this;
}
/**
* Get isInverse
* @return isInverse
**/
@ApiModelProperty(value = "")
public Boolean isIsInverse() {
return isInverse;
}
public void setIsInverse(Boolean isInverse) {
this.isInverse = isInverse;
}
public Instrument initMargin(Double initMargin) {
this.initMargin = initMargin;
return this;
}
/**
* Get initMargin
* @return initMargin
**/
@ApiModelProperty(value = "")
public Double getInitMargin() {
return initMargin;
}
public void setInitMargin(Double initMargin) {
this.initMargin = initMargin;
}
public Instrument maintMargin(Double maintMargin) {
this.maintMargin = maintMargin;
return this;
}
/**
* Get maintMargin
* @return maintMargin
**/
@ApiModelProperty(value = "")
public Double getMaintMargin() {
return maintMargin;
}
public void setMaintMargin(Double maintMargin) {
this.maintMargin = maintMargin;
}
public Instrument riskLimit(BigDecimal riskLimit) {
this.riskLimit = riskLimit;
return this;
}
/**
* Get riskLimit
* @return riskLimit
**/
@ApiModelProperty(value = "")
public BigDecimal getRiskLimit() {
return riskLimit;
}
public void setRiskLimit(BigDecimal riskLimit) {
this.riskLimit = riskLimit;
}
public Instrument riskStep(BigDecimal riskStep) {
this.riskStep = riskStep;
return this;
}
/**
* Get riskStep
* @return riskStep
**/
@ApiModelProperty(value = "")
public BigDecimal getRiskStep() {
return riskStep;
}
public void setRiskStep(BigDecimal riskStep) {
this.riskStep = riskStep;
}
public Instrument limit(Double limit) {
this.limit = limit;
return this;
}
/**
* Get limit
* @return limit
**/
@ApiModelProperty(value = "")
public Double getLimit() {
return limit;
}
public void setLimit(Double limit) {
this.limit = limit;
}
public Instrument capped(Boolean capped) {
this.capped = capped;
return this;
}
/**
* Get capped
* @return capped
**/
@ApiModelProperty(value = "")
public Boolean isCapped() {
return capped;
}
public void setCapped(Boolean capped) {
this.capped = capped;
}
public Instrument taxed(Boolean taxed) {
this.taxed = taxed;
return this;
}
/**
* Get taxed
* @return taxed
**/
@ApiModelProperty(value = "")
public Boolean isTaxed() {
return taxed;
}
public void setTaxed(Boolean taxed) {
this.taxed = taxed;
}
public Instrument deleverage(Boolean deleverage) {
this.deleverage = deleverage;
return this;
}
/**
* Get deleverage
* @return deleverage
**/
@ApiModelProperty(value = "")
public Boolean isDeleverage() {
return deleverage;
}
public void setDeleverage(Boolean deleverage) {
this.deleverage = deleverage;
}
public Instrument makerFee(Double makerFee) {
this.makerFee = makerFee;
return this;
}
/**
* Get makerFee
* @return makerFee
**/
@ApiModelProperty(value = "")
public Double getMakerFee() {
return makerFee;
}
public void setMakerFee(Double makerFee) {
this.makerFee = makerFee;
}
public Instrument takerFee(Double takerFee) {
this.takerFee = takerFee;
return this;
}
/**
* Get takerFee
* @return takerFee
**/
@ApiModelProperty(value = "")
public Double getTakerFee() {
return takerFee;
}
public void setTakerFee(Double takerFee) {
this.takerFee = takerFee;
}
public Instrument settlementFee(Double settlementFee) {
this.settlementFee = settlementFee;
return this;
}
/**
* Get settlementFee
* @return settlementFee
**/
@ApiModelProperty(value = "")
public Double getSettlementFee() {
return settlementFee;
}
public void setSettlementFee(Double settlementFee) {
this.settlementFee = settlementFee;
}
public Instrument insuranceFee(Double insuranceFee) {
this.insuranceFee = insuranceFee;
return this;
}
/**
* Get insuranceFee
* @return insuranceFee
**/
@ApiModelProperty(value = "")
public Double getInsuranceFee() {
return insuranceFee;
}
public void setInsuranceFee(Double insuranceFee) {
this.insuranceFee = insuranceFee;
}
public Instrument fundingBaseSymbol(String fundingBaseSymbol) {
this.fundingBaseSymbol = fundingBaseSymbol;
return this;
}
/**
* Get fundingBaseSymbol
* @return fundingBaseSymbol
**/
@ApiModelProperty(value = "")
public String getFundingBaseSymbol() {
return fundingBaseSymbol;
}
public void setFundingBaseSymbol(String fundingBaseSymbol) {
this.fundingBaseSymbol = fundingBaseSymbol;
}
public Instrument fundingQuoteSymbol(String fundingQuoteSymbol) {
this.fundingQuoteSymbol = fundingQuoteSymbol;
return this;
}
/**
* Get fundingQuoteSymbol
* @return fundingQuoteSymbol
**/
@ApiModelProperty(value = "")
public String getFundingQuoteSymbol() {
return fundingQuoteSymbol;
}
public void setFundingQuoteSymbol(String fundingQuoteSymbol) {
this.fundingQuoteSymbol = fundingQuoteSymbol;
}
public Instrument fundingPremiumSymbol(String fundingPremiumSymbol) {
this.fundingPremiumSymbol = fundingPremiumSymbol;
return this;
}
/**
* Get fundingPremiumSymbol
* @return fundingPremiumSymbol
**/
@ApiModelProperty(value = "")
public String getFundingPremiumSymbol() {
return fundingPremiumSymbol;
}
public void setFundingPremiumSymbol(String fundingPremiumSymbol) {
this.fundingPremiumSymbol = fundingPremiumSymbol;
}
public Instrument fundingTimestamp(OffsetDateTime fundingTimestamp) {
this.fundingTimestamp = fundingTimestamp;
return this;
}
/**
* Get fundingTimestamp
* @return fundingTimestamp
**/
@ApiModelProperty(value = "")
public OffsetDateTime getFundingTimestamp() {
return fundingTimestamp;
}
public void setFundingTimestamp(OffsetDateTime fundingTimestamp) {
this.fundingTimestamp = fundingTimestamp;
}
public Instrument fundingInterval(OffsetDateTime fundingInterval) {
this.fundingInterval = fundingInterval;
return this;
}
/**
* Get fundingInterval
* @return fundingInterval
**/
@ApiModelProperty(value = "")
public OffsetDateTime getFundingInterval() {
return fundingInterval;
}
public void setFundingInterval(OffsetDateTime fundingInterval) {
this.fundingInterval = fundingInterval;
}
public Instrument fundingRate(Double fundingRate) {
this.fundingRate = fundingRate;
return this;
}
/**
* Get fundingRate
* @return fundingRate
**/
@ApiModelProperty(value = "")
public Double getFundingRate() {
return fundingRate;
}
public void setFundingRate(Double fundingRate) {
this.fundingRate = fundingRate;
}
public Instrument indicativeFundingRate(Double indicativeFundingRate) {
this.indicativeFundingRate = indicativeFundingRate;
return this;
}
/**
* Get indicativeFundingRate
* @return indicativeFundingRate
**/
@ApiModelProperty(value = "")
public Double getIndicativeFundingRate() {
return indicativeFundingRate;
}
public void setIndicativeFundingRate(Double indicativeFundingRate) {
this.indicativeFundingRate = indicativeFundingRate;
}
public Instrument rebalanceTimestamp(OffsetDateTime rebalanceTimestamp) {
this.rebalanceTimestamp = rebalanceTimestamp;
return this;
}
/**
* Get rebalanceTimestamp
* @return rebalanceTimestamp
**/
@ApiModelProperty(value = "")
public OffsetDateTime getRebalanceTimestamp() {
return rebalanceTimestamp;
}
public void setRebalanceTimestamp(OffsetDateTime rebalanceTimestamp) {
this.rebalanceTimestamp = rebalanceTimestamp;
}
public Instrument rebalanceInterval(OffsetDateTime rebalanceInterval) {
this.rebalanceInterval = rebalanceInterval;
return this;
}
/**
* Get rebalanceInterval
* @return rebalanceInterval
**/
@ApiModelProperty(value = "")
public OffsetDateTime getRebalanceInterval() {
return rebalanceInterval;
}
public void setRebalanceInterval(OffsetDateTime rebalanceInterval) {
this.rebalanceInterval = rebalanceInterval;
}
public Instrument openingTimestamp(OffsetDateTime openingTimestamp) {
this.openingTimestamp = openingTimestamp;
return this;
}
/**
* Get openingTimestamp
* @return openingTimestamp
**/
@ApiModelProperty(value = "")
public OffsetDateTime getOpeningTimestamp() {
return openingTimestamp;
}
public void setOpeningTimestamp(OffsetDateTime openingTimestamp) {
this.openingTimestamp = openingTimestamp;
}
public Instrument closingTimestamp(OffsetDateTime closingTimestamp) {
this.closingTimestamp = closingTimestamp;
return this;
}
/**
* Get closingTimestamp
* @return closingTimestamp
**/
@ApiModelProperty(value = "")
public OffsetDateTime getClosingTimestamp() {
return closingTimestamp;
}
public void setClosingTimestamp(OffsetDateTime closingTimestamp) {
this.closingTimestamp = closingTimestamp;
}
public Instrument sessionInterval(OffsetDateTime sessionInterval) {
this.sessionInterval = sessionInterval;
return this;
}
/**
* Get sessionInterval
* @return sessionInterval
**/
@ApiModelProperty(value = "")
public OffsetDateTime getSessionInterval() {
return sessionInterval;
}
public void setSessionInterval(OffsetDateTime sessionInterval) {
this.sessionInterval = sessionInterval;
}
public Instrument prevClosePrice(Double prevClosePrice) {
this.prevClosePrice = prevClosePrice;
return this;
}
/**
* Get prevClosePrice
* @return prevClosePrice
**/
@ApiModelProperty(value = "")
public Double getPrevClosePrice() {
return prevClosePrice;
}
public void setPrevClosePrice(Double prevClosePrice) {
this.prevClosePrice = prevClosePrice;
}
public Instrument limitDownPrice(Double limitDownPrice) {
this.limitDownPrice = limitDownPrice;
return this;
}
/**
* Get limitDownPrice
* @return limitDownPrice
**/
@ApiModelProperty(value = "")
public Double getLimitDownPrice() {
return limitDownPrice;
}
public void setLimitDownPrice(Double limitDownPrice) {
this.limitDownPrice = limitDownPrice;
}
public Instrument limitUpPrice(Double limitUpPrice) {
this.limitUpPrice = limitUpPrice;
return this;
}
/**
* Get limitUpPrice
* @return limitUpPrice
**/
@ApiModelProperty(value = "")
public Double getLimitUpPrice() {
return limitUpPrice;
}
public void setLimitUpPrice(Double limitUpPrice) {
this.limitUpPrice = limitUpPrice;
}
public Instrument bankruptLimitDownPrice(Double bankruptLimitDownPrice) {
this.bankruptLimitDownPrice = bankruptLimitDownPrice;
return this;
}
/**
* Get bankruptLimitDownPrice
* @return bankruptLimitDownPrice
**/
@ApiModelProperty(value = "")
public Double getBankruptLimitDownPrice() {
return bankruptLimitDownPrice;
}
public void setBankruptLimitDownPrice(Double bankruptLimitDownPrice) {
this.bankruptLimitDownPrice = bankruptLimitDownPrice;
}
public Instrument bankruptLimitUpPrice(Double bankruptLimitUpPrice) {
this.bankruptLimitUpPrice = bankruptLimitUpPrice;
return this;
}
/**
* Get bankruptLimitUpPrice
* @return bankruptLimitUpPrice
**/
@ApiModelProperty(value = "")
public Double getBankruptLimitUpPrice() {
return bankruptLimitUpPrice;
}
public void setBankruptLimitUpPrice(Double bankruptLimitUpPrice) {
this.bankruptLimitUpPrice = bankruptLimitUpPrice;
}
public Instrument prevTotalVolume(BigDecimal prevTotalVolume) {
this.prevTotalVolume = prevTotalVolume;
return this;
}
/**
* Get prevTotalVolume
* @return prevTotalVolume
**/
@ApiModelProperty(value = "")
public BigDecimal getPrevTotalVolume() {
return prevTotalVolume;
}
public void setPrevTotalVolume(BigDecimal prevTotalVolume) {
this.prevTotalVolume = prevTotalVolume;
}
public Instrument totalVolume(BigDecimal totalVolume) {
this.totalVolume = totalVolume;
return this;
}
/**
* Get totalVolume
* @return totalVolume
**/
@ApiModelProperty(value = "")
public BigDecimal getTotalVolume() {
return totalVolume;
}
public void setTotalVolume(BigDecimal totalVolume) {
this.totalVolume = totalVolume;
}
public Instrument volume(BigDecimal volume) {
this.volume = volume;
return this;
}
/**
* Get volume
* @return volume
**/
@ApiModelProperty(value = "")
public BigDecimal getVolume() {
return volume;
}
public void setVolume(BigDecimal volume) {
this.volume = volume;
}
public Instrument volume24h(BigDecimal volume24h) {
this.volume24h = volume24h;
return this;
}
/**
* Get volume24h
* @return volume24h
**/
@ApiModelProperty(value = "")
public BigDecimal getVolume24h() {
return volume24h;
}
public void setVolume24h(BigDecimal volume24h) {
this.volume24h = volume24h;
}
public Instrument prevTotalTurnover(BigDecimal prevTotalTurnover) {
this.prevTotalTurnover = prevTotalTurnover;
return this;
}
/**
* Get prevTotalTurnover
* @return prevTotalTurnover
**/
@ApiModelProperty(value = "")
public BigDecimal getPrevTotalTurnover() {
return prevTotalTurnover;
}
public void setPrevTotalTurnover(BigDecimal prevTotalTurnover) {
this.prevTotalTurnover = prevTotalTurnover;
}
public Instrument totalTurnover(BigDecimal totalTurnover) {
this.totalTurnover = totalTurnover;
return this;
}
/**
* Get totalTurnover
* @return totalTurnover
**/
@ApiModelProperty(value = "")
public BigDecimal getTotalTurnover() {
return totalTurnover;
}
public void setTotalTurnover(BigDecimal totalTurnover) {
this.totalTurnover = totalTurnover;
}
public Instrument turnover(BigDecimal turnover) {
this.turnover = turnover;
return this;
}
/**
* Get turnover
* @return turnover
**/
@ApiModelProperty(value = "")
public BigDecimal getTurnover() {
return turnover;
}
public void setTurnover(BigDecimal turnover) {
this.turnover = turnover;
}
public Instrument turnover24h(BigDecimal turnover24h) {
this.turnover24h = turnover24h;
return this;
}
/**
* Get turnover24h
* @return turnover24h
**/
@ApiModelProperty(value = "")
public BigDecimal getTurnover24h() {
return turnover24h;
}
public void setTurnover24h(BigDecimal turnover24h) {
this.turnover24h = turnover24h;
}
public Instrument homeNotional24h(Double homeNotional24h) {
this.homeNotional24h = homeNotional24h;
return this;
}
/**
* Get homeNotional24h
* @return homeNotional24h
**/
@ApiModelProperty(value = "")
public Double getHomeNotional24h() {
return homeNotional24h;
}
public void setHomeNotional24h(Double homeNotional24h) {
this.homeNotional24h = homeNotional24h;
}
public Instrument foreignNotional24h(Double foreignNotional24h) {
this.foreignNotional24h = foreignNotional24h;
return this;
}
/**
* Get foreignNotional24h
* @return foreignNotional24h
**/
@ApiModelProperty(value = "")
public Double getForeignNotional24h() {
return foreignNotional24h;
}
public void setForeignNotional24h(Double foreignNotional24h) {
this.foreignNotional24h = foreignNotional24h;
}
public Instrument prevPrice24h(Double prevPrice24h) {
this.prevPrice24h = prevPrice24h;
return this;
}
/**
* Get prevPrice24h
* @return prevPrice24h
**/
@ApiModelProperty(value = "")
public Double getPrevPrice24h() {
return prevPrice24h;
}
public void setPrevPrice24h(Double prevPrice24h) {
this.prevPrice24h = prevPrice24h;
}
public Instrument vwap(Double vwap) {
this.vwap = vwap;
return this;
}
/**
* Get vwap
* @return vwap
**/
@ApiModelProperty(value = "")
public Double getVwap() {
return vwap;
}
public void setVwap(Double vwap) {
this.vwap = vwap;
}
public Instrument highPrice(Double highPrice) {
this.highPrice = highPrice;
return this;
}
/**
* Get highPrice
* @return highPrice
**/
@ApiModelProperty(value = "")
public Double getHighPrice() {
return highPrice;
}
public void setHighPrice(Double highPrice) {
this.highPrice = highPrice;
}
public Instrument lowPrice(Double lowPrice) {
this.lowPrice = lowPrice;
return this;
}
/**
* Get lowPrice
* @return lowPrice
**/
@ApiModelProperty(value = "")
public Double getLowPrice() {
return lowPrice;
}
public void setLowPrice(Double lowPrice) {
this.lowPrice = lowPrice;
}
public Instrument lastPrice(Double lastPrice) {
this.lastPrice = lastPrice;
return this;
}
/**
* Get lastPrice
* @return lastPrice
**/
@ApiModelProperty(value = "")
public Double getLastPrice() {
return lastPrice;
}
public void setLastPrice(Double lastPrice) {
this.lastPrice = lastPrice;
}
public Instrument lastPriceProtected(Double lastPriceProtected) {
this.lastPriceProtected = lastPriceProtected;
return this;
}
/**
* Get lastPriceProtected
* @return lastPriceProtected
**/
@ApiModelProperty(value = "")
public Double getLastPriceProtected() {
return lastPriceProtected;
}
public void setLastPriceProtected(Double lastPriceProtected) {
this.lastPriceProtected = lastPriceProtected;
}
public Instrument lastTickDirection(String lastTickDirection) {
this.lastTickDirection = lastTickDirection;
return this;
}
/**
* Get lastTickDirection
* @return lastTickDirection
**/
@ApiModelProperty(value = "")
public String getLastTickDirection() {
return lastTickDirection;
}
public void setLastTickDirection(String lastTickDirection) {
this.lastTickDirection = lastTickDirection;
}
public Instrument lastChangePcnt(Double lastChangePcnt) {
this.lastChangePcnt = lastChangePcnt;
return this;
}
/**
* Get lastChangePcnt
* @return lastChangePcnt
**/
@ApiModelProperty(value = "")
public Double getLastChangePcnt() {
return lastChangePcnt;
}
public void setLastChangePcnt(Double lastChangePcnt) {
this.lastChangePcnt = lastChangePcnt;
}
public Instrument bidPrice(Double bidPrice) {
this.bidPrice = bidPrice;
return this;
}
/**
* Get bidPrice
* @return bidPrice
**/
@ApiModelProperty(value = "")
public Double getBidPrice() {
return bidPrice;
}
public void setBidPrice(Double bidPrice) {
this.bidPrice = bidPrice;
}
public Instrument midPrice(Double midPrice) {
this.midPrice = midPrice;
return this;
}
/**
* Get midPrice
* @return midPrice
**/
@ApiModelProperty(value = "")
public Double getMidPrice() {
return midPrice;
}
public void setMidPrice(Double midPrice) {
this.midPrice = midPrice;
}
public Instrument askPrice(Double askPrice) {
this.askPrice = askPrice;
return this;
}
/**
* Get askPrice
* @return askPrice
**/
@ApiModelProperty(value = "")
public Double getAskPrice() {
return askPrice;
}
public void setAskPrice(Double askPrice) {
this.askPrice = askPrice;
}
public Instrument impactBidPrice(Double impactBidPrice) {
this.impactBidPrice = impactBidPrice;
return this;
}
/**
* Get impactBidPrice
* @return impactBidPrice
**/
@ApiModelProperty(value = "")
public Double getImpactBidPrice() {
return impactBidPrice;
}
public void setImpactBidPrice(Double impactBidPrice) {
this.impactBidPrice = impactBidPrice;
}
public Instrument impactMidPrice(Double impactMidPrice) {
this.impactMidPrice = impactMidPrice;
return this;
}
/**
* Get impactMidPrice
* @return impactMidPrice
**/
@ApiModelProperty(value = "")
public Double getImpactMidPrice() {
return impactMidPrice;
}
public void setImpactMidPrice(Double impactMidPrice) {
this.impactMidPrice = impactMidPrice;
}
public Instrument impactAskPrice(Double impactAskPrice) {
this.impactAskPrice = impactAskPrice;
return this;
}
/**
* Get impactAskPrice
* @return impactAskPrice
**/
@ApiModelProperty(value = "")
public Double getImpactAskPrice() {
return impactAskPrice;
}
public void setImpactAskPrice(Double impactAskPrice) {
this.impactAskPrice = impactAskPrice;
}
public Instrument hasLiquidity(Boolean hasLiquidity) {
this.hasLiquidity = hasLiquidity;
return this;
}
/**
* Get hasLiquidity
* @return hasLiquidity
**/
@ApiModelProperty(value = "")
public Boolean isHasLiquidity() {
return hasLiquidity;
}
public void setHasLiquidity(Boolean hasLiquidity) {
this.hasLiquidity = hasLiquidity;
}
public Instrument openInterest(BigDecimal openInterest) {
this.openInterest = openInterest;
return this;
}
/**
* Get openInterest
* @return openInterest
**/
@ApiModelProperty(value = "")
public BigDecimal getOpenInterest() {
return openInterest;
}
public void setOpenInterest(BigDecimal openInterest) {
this.openInterest = openInterest;
}
public Instrument openValue(BigDecimal openValue) {
this.openValue = openValue;
return this;
}
/**
* Get openValue
* @return openValue
**/
@ApiModelProperty(value = "")
public BigDecimal getOpenValue() {
return openValue;
}
public void setOpenValue(BigDecimal openValue) {
this.openValue = openValue;
}
public Instrument fairMethod(String fairMethod) {
this.fairMethod = fairMethod;
return this;
}
/**
* Get fairMethod
* @return fairMethod
**/
@ApiModelProperty(value = "")
public String getFairMethod() {
return fairMethod;
}
public void setFairMethod(String fairMethod) {
this.fairMethod = fairMethod;
}
public Instrument fairBasisRate(Double fairBasisRate) {
this.fairBasisRate = fairBasisRate;
return this;
}
/**
* Get fairBasisRate
* @return fairBasisRate
**/
@ApiModelProperty(value = "")
public Double getFairBasisRate() {
return fairBasisRate;
}
public void setFairBasisRate(Double fairBasisRate) {
this.fairBasisRate = fairBasisRate;
}
public Instrument fairBasis(Double fairBasis) {
this.fairBasis = fairBasis;
return this;
}
/**
* Get fairBasis
* @return fairBasis
**/
@ApiModelProperty(value = "")
public Double getFairBasis() {
return fairBasis;
}
public void setFairBasis(Double fairBasis) {
this.fairBasis = fairBasis;
}
public Instrument fairPrice(Double fairPrice) {
this.fairPrice = fairPrice;
return this;
}
/**
* Get fairPrice
* @return fairPrice
**/
@ApiModelProperty(value = "")
public Double getFairPrice() {
return fairPrice;
}
public void setFairPrice(Double fairPrice) {
this.fairPrice = fairPrice;
}
public Instrument markMethod(String markMethod) {
this.markMethod = markMethod;
return this;
}
/**
* Get markMethod
* @return markMethod
**/
@ApiModelProperty(value = "")
public String getMarkMethod() {
return markMethod;
}
public void setMarkMethod(String markMethod) {
this.markMethod = markMethod;
}
public Instrument markPrice(Double markPrice) {
this.markPrice = markPrice;
return this;
}
/**
* Get markPrice
* @return markPrice
**/
@ApiModelProperty(value = "")
public Double getMarkPrice() {
return markPrice;
}
public void setMarkPrice(Double markPrice) {
this.markPrice = markPrice;
}
public Instrument indicativeTaxRate(Double indicativeTaxRate) {
this.indicativeTaxRate = indicativeTaxRate;
return this;
}
/**
* Get indicativeTaxRate
* @return indicativeTaxRate
**/
@ApiModelProperty(value = "")
public Double getIndicativeTaxRate() {
return indicativeTaxRate;
}
public void setIndicativeTaxRate(Double indicativeTaxRate) {
this.indicativeTaxRate = indicativeTaxRate;
}
public Instrument indicativeSettlePrice(Double indicativeSettlePrice) {
this.indicativeSettlePrice = indicativeSettlePrice;
return this;
}
/**
* Get indicativeSettlePrice
* @return indicativeSettlePrice
**/
@ApiModelProperty(value = "")
public Double getIndicativeSettlePrice() {
return indicativeSettlePrice;
}
public void setIndicativeSettlePrice(Double indicativeSettlePrice) {
this.indicativeSettlePrice = indicativeSettlePrice;
}
public Instrument optionUnderlyingPrice(Double optionUnderlyingPrice) {
this.optionUnderlyingPrice = optionUnderlyingPrice;
return this;
}
/**
* Get optionUnderlyingPrice
* @return optionUnderlyingPrice
**/
@ApiModelProperty(value = "")
public Double getOptionUnderlyingPrice() {
return optionUnderlyingPrice;
}
public void setOptionUnderlyingPrice(Double optionUnderlyingPrice) {
this.optionUnderlyingPrice = optionUnderlyingPrice;
}
public Instrument settledPrice(Double settledPrice) {
this.settledPrice = settledPrice;
return this;
}
/**
* Get settledPrice
* @return settledPrice
**/
@ApiModelProperty(value = "")
public Double getSettledPrice() {
return settledPrice;
}
public void setSettledPrice(Double settledPrice) {
this.settledPrice = settledPrice;
}
public Instrument timestamp(OffsetDateTime timestamp) {
this.timestamp = timestamp;
return this;
}
/**
* Get timestamp
* @return timestamp
**/
@ApiModelProperty(value = "")
public OffsetDateTime getTimestamp() {
return timestamp;
}
public void setTimestamp(OffsetDateTime timestamp) {
this.timestamp = timestamp;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Instrument instrument = (Instrument) o;
return Objects.equals(this.symbol, instrument.symbol) &&
Objects.equals(this.rootSymbol, instrument.rootSymbol) &&
Objects.equals(this.state, instrument.state) &&
Objects.equals(this.typ, instrument.typ) &&
Objects.equals(this.listing, instrument.listing) &&
Objects.equals(this.front, instrument.front) &&
Objects.equals(this.expiry, instrument.expiry) &&
Objects.equals(this.settle, instrument.settle) &&
Objects.equals(this.relistInterval, instrument.relistInterval) &&
Objects.equals(this.inverseLeg, instrument.inverseLeg) &&
Objects.equals(this.sellLeg, instrument.sellLeg) &&
Objects.equals(this.buyLeg, instrument.buyLeg) &&
Objects.equals(this.optionStrikePcnt, instrument.optionStrikePcnt) &&
Objects.equals(this.optionStrikeRound, instrument.optionStrikeRound) &&
Objects.equals(this.optionStrikePrice, instrument.optionStrikePrice) &&
Objects.equals(this.optionMultiplier, instrument.optionMultiplier) &&
Objects.equals(this.positionCurrency, instrument.positionCurrency) &&
Objects.equals(this.underlying, instrument.underlying) &&
Objects.equals(this.quoteCurrency, instrument.quoteCurrency) &&
Objects.equals(this.underlyingSymbol, instrument.underlyingSymbol) &&
Objects.equals(this.reference, instrument.reference) &&
Objects.equals(this.referenceSymbol, instrument.referenceSymbol) &&
Objects.equals(this.calcInterval, instrument.calcInterval) &&
Objects.equals(this.publishInterval, instrument.publishInterval) &&
Objects.equals(this.publishTime, instrument.publishTime) &&
Objects.equals(this.maxOrderQty, instrument.maxOrderQty) &&
Objects.equals(this.maxPrice, instrument.maxPrice) &&
Objects.equals(this.lotSize, instrument.lotSize) &&
Objects.equals(this.tickSize, instrument.tickSize) &&
Objects.equals(this.multiplier, instrument.multiplier) &&
Objects.equals(this.settlCurrency, instrument.settlCurrency) &&
Objects.equals(this.underlyingToPositionMultiplier, instrument.underlyingToPositionMultiplier) &&
Objects.equals(this.underlyingToSettleMultiplier, instrument.underlyingToSettleMultiplier) &&
Objects.equals(this.quoteToSettleMultiplier, instrument.quoteToSettleMultiplier) &&
Objects.equals(this.isQuanto, instrument.isQuanto) &&
Objects.equals(this.isInverse, instrument.isInverse) &&
Objects.equals(this.initMargin, instrument.initMargin) &&
Objects.equals(this.maintMargin, instrument.maintMargin) &&
Objects.equals(this.riskLimit, instrument.riskLimit) &&
Objects.equals(this.riskStep, instrument.riskStep) &&
Objects.equals(this.limit, instrument.limit) &&
Objects.equals(this.capped, instrument.capped) &&
Objects.equals(this.taxed, instrument.taxed) &&
Objects.equals(this.deleverage, instrument.deleverage) &&
Objects.equals(this.makerFee, instrument.makerFee) &&
Objects.equals(this.takerFee, instrument.takerFee) &&
Objects.equals(this.settlementFee, instrument.settlementFee) &&
Objects.equals(this.insuranceFee, instrument.insuranceFee) &&
Objects.equals(this.fundingBaseSymbol, instrument.fundingBaseSymbol) &&
Objects.equals(this.fundingQuoteSymbol, instrument.fundingQuoteSymbol) &&
Objects.equals(this.fundingPremiumSymbol, instrument.fundingPremiumSymbol) &&
Objects.equals(this.fundingTimestamp, instrument.fundingTimestamp) &&
Objects.equals(this.fundingInterval, instrument.fundingInterval) &&
Objects.equals(this.fundingRate, instrument.fundingRate) &&
Objects.equals(this.indicativeFundingRate, instrument.indicativeFundingRate) &&
Objects.equals(this.rebalanceTimestamp, instrument.rebalanceTimestamp) &&
Objects.equals(this.rebalanceInterval, instrument.rebalanceInterval) &&
Objects.equals(this.openingTimestamp, instrument.openingTimestamp) &&
Objects.equals(this.closingTimestamp, instrument.closingTimestamp) &&
Objects.equals(this.sessionInterval, instrument.sessionInterval) &&
Objects.equals(this.prevClosePrice, instrument.prevClosePrice) &&
Objects.equals(this.limitDownPrice, instrument.limitDownPrice) &&
Objects.equals(this.limitUpPrice, instrument.limitUpPrice) &&
Objects.equals(this.bankruptLimitDownPrice, instrument.bankruptLimitDownPrice) &&
Objects.equals(this.bankruptLimitUpPrice, instrument.bankruptLimitUpPrice) &&
Objects.equals(this.prevTotalVolume, instrument.prevTotalVolume) &&
Objects.equals(this.totalVolume, instrument.totalVolume) &&
Objects.equals(this.volume, instrument.volume) &&
Objects.equals(this.volume24h, instrument.volume24h) &&
Objects.equals(this.prevTotalTurnover, instrument.prevTotalTurnover) &&
Objects.equals(this.totalTurnover, instrument.totalTurnover) &&
Objects.equals(this.turnover, instrument.turnover) &&
Objects.equals(this.turnover24h, instrument.turnover24h) &&
Objects.equals(this.homeNotional24h, instrument.homeNotional24h) &&
Objects.equals(this.foreignNotional24h, instrument.foreignNotional24h) &&
Objects.equals(this.prevPrice24h, instrument.prevPrice24h) &&
Objects.equals(this.vwap, instrument.vwap) &&
Objects.equals(this.highPrice, instrument.highPrice) &&
Objects.equals(this.lowPrice, instrument.lowPrice) &&
Objects.equals(this.lastPrice, instrument.lastPrice) &&
Objects.equals(this.lastPriceProtected, instrument.lastPriceProtected) &&
Objects.equals(this.lastTickDirection, instrument.lastTickDirection) &&
Objects.equals(this.lastChangePcnt, instrument.lastChangePcnt) &&
Objects.equals(this.bidPrice, instrument.bidPrice) &&
Objects.equals(this.midPrice, instrument.midPrice) &&
Objects.equals(this.askPrice, instrument.askPrice) &&
Objects.equals(this.impactBidPrice, instrument.impactBidPrice) &&
Objects.equals(this.impactMidPrice, instrument.impactMidPrice) &&
Objects.equals(this.impactAskPrice, instrument.impactAskPrice) &&
Objects.equals(this.hasLiquidity, instrument.hasLiquidity) &&
Objects.equals(this.openInterest, instrument.openInterest) &&
Objects.equals(this.openValue, instrument.openValue) &&
Objects.equals(this.fairMethod, instrument.fairMethod) &&
Objects.equals(this.fairBasisRate, instrument.fairBasisRate) &&
Objects.equals(this.fairBasis, instrument.fairBasis) &&
Objects.equals(this.fairPrice, instrument.fairPrice) &&
Objects.equals(this.markMethod, instrument.markMethod) &&
Objects.equals(this.markPrice, instrument.markPrice) &&
Objects.equals(this.indicativeTaxRate, instrument.indicativeTaxRate) &&
Objects.equals(this.indicativeSettlePrice, instrument.indicativeSettlePrice) &&
Objects.equals(this.optionUnderlyingPrice, instrument.optionUnderlyingPrice) &&
Objects.equals(this.settledPrice, instrument.settledPrice) &&
Objects.equals(this.timestamp, instrument.timestamp);
}
@Override
public int hashCode() {
return Objects.hash(symbol, rootSymbol, state, typ, listing, front, expiry, settle, relistInterval, inverseLeg, sellLeg, buyLeg, optionStrikePcnt, optionStrikeRound, optionStrikePrice, optionMultiplier, positionCurrency, underlying, quoteCurrency, underlyingSymbol, reference, referenceSymbol, calcInterval, publishInterval, publishTime, maxOrderQty, maxPrice, lotSize, tickSize, multiplier, settlCurrency, underlyingToPositionMultiplier, underlyingToSettleMultiplier, quoteToSettleMultiplier, isQuanto, isInverse, initMargin, maintMargin, riskLimit, riskStep, limit, capped, taxed, deleverage, makerFee, takerFee, settlementFee, insuranceFee, fundingBaseSymbol, fundingQuoteSymbol, fundingPremiumSymbol, fundingTimestamp, fundingInterval, fundingRate, indicativeFundingRate, rebalanceTimestamp, rebalanceInterval, openingTimestamp, closingTimestamp, sessionInterval, prevClosePrice, limitDownPrice, limitUpPrice, bankruptLimitDownPrice, bankruptLimitUpPrice, prevTotalVolume, totalVolume, volume, volume24h, prevTotalTurnover, totalTurnover, turnover, turnover24h, homeNotional24h, foreignNotional24h, prevPrice24h, vwap, highPrice, lowPrice, lastPrice, lastPriceProtected, lastTickDirection, lastChangePcnt, bidPrice, midPrice, askPrice, impactBidPrice, impactMidPrice, impactAskPrice, hasLiquidity, openInterest, openValue, fairMethod, fairBasisRate, fairBasis, fairPrice, markMethod, markPrice, indicativeTaxRate, indicativeSettlePrice, optionUnderlyingPrice, settledPrice, timestamp);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Instrument {\n");
sb.append(" symbol: ").append(toIndentedString(symbol)).append("\n");
sb.append(" rootSymbol: ").append(toIndentedString(rootSymbol)).append("\n");
sb.append(" state: ").append(toIndentedString(state)).append("\n");
sb.append(" typ: ").append(toIndentedString(typ)).append("\n");
sb.append(" listing: ").append(toIndentedString(listing)).append("\n");
sb.append(" front: ").append(toIndentedString(front)).append("\n");
sb.append(" expiry: ").append(toIndentedString(expiry)).append("\n");
sb.append(" settle: ").append(toIndentedString(settle)).append("\n");
sb.append(" relistInterval: ").append(toIndentedString(relistInterval)).append("\n");
sb.append(" inverseLeg: ").append(toIndentedString(inverseLeg)).append("\n");
sb.append(" sellLeg: ").append(toIndentedString(sellLeg)).append("\n");
sb.append(" buyLeg: ").append(toIndentedString(buyLeg)).append("\n");
sb.append(" optionStrikePcnt: ").append(toIndentedString(optionStrikePcnt)).append("\n");
sb.append(" optionStrikeRound: ").append(toIndentedString(optionStrikeRound)).append("\n");
sb.append(" optionStrikePrice: ").append(toIndentedString(optionStrikePrice)).append("\n");
sb.append(" optionMultiplier: ").append(toIndentedString(optionMultiplier)).append("\n");
sb.append(" positionCurrency: ").append(toIndentedString(positionCurrency)).append("\n");
sb.append(" underlying: ").append(toIndentedString(underlying)).append("\n");
sb.append(" quoteCurrency: ").append(toIndentedString(quoteCurrency)).append("\n");
sb.append(" underlyingSymbol: ").append(toIndentedString(underlyingSymbol)).append("\n");
sb.append(" reference: ").append(toIndentedString(reference)).append("\n");
sb.append(" referenceSymbol: ").append(toIndentedString(referenceSymbol)).append("\n");
sb.append(" calcInterval: ").append(toIndentedString(calcInterval)).append("\n");
sb.append(" publishInterval: ").append(toIndentedString(publishInterval)).append("\n");
sb.append(" publishTime: ").append(toIndentedString(publishTime)).append("\n");
sb.append(" maxOrderQty: ").append(toIndentedString(maxOrderQty)).append("\n");
sb.append(" maxPrice: ").append(toIndentedString(maxPrice)).append("\n");
sb.append(" lotSize: ").append(toIndentedString(lotSize)).append("\n");
sb.append(" tickSize: ").append(toIndentedString(tickSize)).append("\n");
sb.append(" multiplier: ").append(toIndentedString(multiplier)).append("\n");
sb.append(" settlCurrency: ").append(toIndentedString(settlCurrency)).append("\n");
sb.append(" underlyingToPositionMultiplier: ").append(toIndentedString(underlyingToPositionMultiplier)).append("\n");
sb.append(" underlyingToSettleMultiplier: ").append(toIndentedString(underlyingToSettleMultiplier)).append("\n");
sb.append(" quoteToSettleMultiplier: ").append(toIndentedString(quoteToSettleMultiplier)).append("\n");
sb.append(" isQuanto: ").append(toIndentedString(isQuanto)).append("\n");
sb.append(" isInverse: ").append(toIndentedString(isInverse)).append("\n");
sb.append(" initMargin: ").append(toIndentedString(initMargin)).append("\n");
sb.append(" maintMargin: ").append(toIndentedString(maintMargin)).append("\n");
sb.append(" riskLimit: ").append(toIndentedString(riskLimit)).append("\n");
sb.append(" riskStep: ").append(toIndentedString(riskStep)).append("\n");
sb.append(" limit: ").append(toIndentedString(limit)).append("\n");
sb.append(" capped: ").append(toIndentedString(capped)).append("\n");
sb.append(" taxed: ").append(toIndentedString(taxed)).append("\n");
sb.append(" deleverage: ").append(toIndentedString(deleverage)).append("\n");
sb.append(" makerFee: ").append(toIndentedString(makerFee)).append("\n");
sb.append(" takerFee: ").append(toIndentedString(takerFee)).append("\n");
sb.append(" settlementFee: ").append(toIndentedString(settlementFee)).append("\n");
sb.append(" insuranceFee: ").append(toIndentedString(insuranceFee)).append("\n");
sb.append(" fundingBaseSymbol: ").append(toIndentedString(fundingBaseSymbol)).append("\n");
sb.append(" fundingQuoteSymbol: ").append(toIndentedString(fundingQuoteSymbol)).append("\n");
sb.append(" fundingPremiumSymbol: ").append(toIndentedString(fundingPremiumSymbol)).append("\n");
sb.append(" fundingTimestamp: ").append(toIndentedString(fundingTimestamp)).append("\n");
sb.append(" fundingInterval: ").append(toIndentedString(fundingInterval)).append("\n");
sb.append(" fundingRate: ").append(toIndentedString(fundingRate)).append("\n");
sb.append(" indicativeFundingRate: ").append(toIndentedString(indicativeFundingRate)).append("\n");
sb.append(" rebalanceTimestamp: ").append(toIndentedString(rebalanceTimestamp)).append("\n");
sb.append(" rebalanceInterval: ").append(toIndentedString(rebalanceInterval)).append("\n");
sb.append(" openingTimestamp: ").append(toIndentedString(openingTimestamp)).append("\n");
sb.append(" closingTimestamp: ").append(toIndentedString(closingTimestamp)).append("\n");
sb.append(" sessionInterval: ").append(toIndentedString(sessionInterval)).append("\n");
sb.append(" prevClosePrice: ").append(toIndentedString(prevClosePrice)).append("\n");
sb.append(" limitDownPrice: ").append(toIndentedString(limitDownPrice)).append("\n");
sb.append(" limitUpPrice: ").append(toIndentedString(limitUpPrice)).append("\n");
sb.append(" bankruptLimitDownPrice: ").append(toIndentedString(bankruptLimitDownPrice)).append("\n");
sb.append(" bankruptLimitUpPrice: ").append(toIndentedString(bankruptLimitUpPrice)).append("\n");
sb.append(" prevTotalVolume: ").append(toIndentedString(prevTotalVolume)).append("\n");
sb.append(" totalVolume: ").append(toIndentedString(totalVolume)).append("\n");
sb.append(" volume: ").append(toIndentedString(volume)).append("\n");
sb.append(" volume24h: ").append(toIndentedString(volume24h)).append("\n");
sb.append(" prevTotalTurnover: ").append(toIndentedString(prevTotalTurnover)).append("\n");
sb.append(" totalTurnover: ").append(toIndentedString(totalTurnover)).append("\n");
sb.append(" turnover: ").append(toIndentedString(turnover)).append("\n");
sb.append(" turnover24h: ").append(toIndentedString(turnover24h)).append("\n");
sb.append(" homeNotional24h: ").append(toIndentedString(homeNotional24h)).append("\n");
sb.append(" foreignNotional24h: ").append(toIndentedString(foreignNotional24h)).append("\n");
sb.append(" prevPrice24h: ").append(toIndentedString(prevPrice24h)).append("\n");
sb.append(" vwap: ").append(toIndentedString(vwap)).append("\n");
sb.append(" highPrice: ").append(toIndentedString(highPrice)).append("\n");
sb.append(" lowPrice: ").append(toIndentedString(lowPrice)).append("\n");
sb.append(" lastPrice: ").append(toIndentedString(lastPrice)).append("\n");
sb.append(" lastPriceProtected: ").append(toIndentedString(lastPriceProtected)).append("\n");
sb.append(" lastTickDirection: ").append(toIndentedString(lastTickDirection)).append("\n");
sb.append(" lastChangePcnt: ").append(toIndentedString(lastChangePcnt)).append("\n");
sb.append(" bidPrice: ").append(toIndentedString(bidPrice)).append("\n");
sb.append(" midPrice: ").append(toIndentedString(midPrice)).append("\n");
sb.append(" askPrice: ").append(toIndentedString(askPrice)).append("\n");
sb.append(" impactBidPrice: ").append(toIndentedString(impactBidPrice)).append("\n");
sb.append(" impactMidPrice: ").append(toIndentedString(impactMidPrice)).append("\n");
sb.append(" impactAskPrice: ").append(toIndentedString(impactAskPrice)).append("\n");
sb.append(" hasLiquidity: ").append(toIndentedString(hasLiquidity)).append("\n");
sb.append(" openInterest: ").append(toIndentedString(openInterest)).append("\n");
sb.append(" openValue: ").append(toIndentedString(openValue)).append("\n");
sb.append(" fairMethod: ").append(toIndentedString(fairMethod)).append("\n");
sb.append(" fairBasisRate: ").append(toIndentedString(fairBasisRate)).append("\n");
sb.append(" fairBasis: ").append(toIndentedString(fairBasis)).append("\n");
sb.append(" fairPrice: ").append(toIndentedString(fairPrice)).append("\n");
sb.append(" markMethod: ").append(toIndentedString(markMethod)).append("\n");
sb.append(" markPrice: ").append(toIndentedString(markPrice)).append("\n");
sb.append(" indicativeTaxRate: ").append(toIndentedString(indicativeTaxRate)).append("\n");
sb.append(" indicativeSettlePrice: ").append(toIndentedString(indicativeSettlePrice)).append("\n");
sb.append(" optionUnderlyingPrice: ").append(toIndentedString(optionUnderlyingPrice)).append("\n");
sb.append(" settledPrice: ").append(toIndentedString(settledPrice)).append("\n");
sb.append(" timestamp: ").append(toIndentedString(timestamp)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy