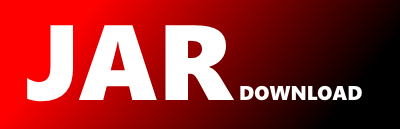
net.ninety.bitmex.model.UserEvent Maven / Gradle / Ivy
/*
* BitMEX API
* ## REST API for the BitMEX Trading Platform [View Changelog](/app/apiChangelog) --- #### Getting Started Base URI: [https://www.bitmex.com/api/v1](/api/v1) ##### Fetching Data All REST endpoints are documented below. You can try out any query right from this interface. Most table queries accept `count`, `start`, and `reverse` params. Set `reverse=true` to get rows newest-first. Additional documentation regarding filters, timestamps, and authentication is available in [the main API documentation](/app/restAPI). _All_ table data is available via the [Websocket](/app/wsAPI). We highly recommend using the socket if you want to have the quickest possible data without being subject to ratelimits. ##### Return Types By default, all data is returned as JSON. Send `?_format=csv` to get CSV data or `?_format=xml` to get XML data. ##### Trade Data Queries _This is only a small subset of what is available, to get you started._ Fill in the parameters and click the `Try it out!` button to try any of these queries. - [Pricing Data](#!/Quote/Quote_get) - [Trade Data](#!/Trade/Trade_get) - [OrderBook Data](#!/OrderBook/OrderBook_getL2) - [Settlement Data](#!/Settlement/Settlement_get) - [Exchange Statistics](#!/Stats/Stats_history) Every function of the BitMEX.com platform is exposed here and documented. Many more functions are available. ##### Swagger Specification [⇩ Download Swagger JSON](swagger.json) --- ## All API Endpoints Click to expand a section.
*
* OpenAPI spec version: 1.2.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package net.ninety.bitmex.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import org.threeten.bp.OffsetDateTime;
/**
* User Events for auditing
*/
@ApiModel(description = "User Events for auditing")
@javax.annotation.Generated(value = "io.swagger.codegen.languages.JavaClientCodegen", date = "2019-11-02T12:22:57.745Z")
public class UserEvent {
@SerializedName("id")
private Double id = null;
/**
* Gets or Sets type
*/
@JsonAdapter(TypeEnum.Adapter.class)
public enum TypeEnum {
APIKEYCREATED("apiKeyCreated"),
DELEVERAGEEXECUTION("deleverageExecution"),
DEPOSITCONFIRMED("depositConfirmed"),
DEPOSITPENDING("depositPending"),
BANZEROVOLUMEAPIUSER("banZeroVolumeApiUser"),
LIQUIDATIONORDERPLACED("liquidationOrderPlaced"),
LOGIN("login"),
PGPMASKEDEMAIL("pgpMaskedEmail"),
PGPTESTEMAIL("pgpTestEmail"),
PASSWORDCHANGED("passwordChanged"),
POSITIONSTATELIQUIDATED("positionStateLiquidated"),
POSITIONSTATEWARNING("positionStateWarning"),
RESETPASSWORDCONFIRMED("resetPasswordConfirmed"),
RESETPASSWORDREQUEST("resetPasswordRequest"),
TRANSFERCANCELED("transferCanceled"),
TRANSFERCOMPLETED("transferCompleted"),
TRANSFERRECEIVED("transferReceived"),
TRANSFERREQUESTED("transferRequested"),
TWOFACTORDISABLED("twoFactorDisabled"),
TWOFACTORENABLED("twoFactorEnabled"),
WITHDRAWALCANCELED("withdrawalCanceled"),
WITHDRAWALCOMPLETED("withdrawalCompleted"),
WITHDRAWALCONFIRMED("withdrawalConfirmed"),
WITHDRAWALREQUESTED("withdrawalRequested"),
VERIFY("verify");
private String value;
TypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static TypeEnum fromValue(String text) {
for (TypeEnum b : TypeEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final TypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public TypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return TypeEnum.fromValue(String.valueOf(value));
}
}
}
@SerializedName("type")
private TypeEnum type = null;
/**
* Gets or Sets status
*/
@JsonAdapter(StatusEnum.Adapter.class)
public enum StatusEnum {
SUCCESS("success"),
FAILURE("failure");
private String value;
StatusEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static StatusEnum fromValue(String text) {
for (StatusEnum b : StatusEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final StatusEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public StatusEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return StatusEnum.fromValue(String.valueOf(value));
}
}
}
@SerializedName("status")
private StatusEnum status = null;
@SerializedName("userId")
private Double userId = null;
@SerializedName("createdById")
private Double createdById = null;
@SerializedName("ip")
private String ip = null;
@SerializedName("geoipCountry")
private String geoipCountry = null;
@SerializedName("geoipRegion")
private String geoipRegion = null;
@SerializedName("geoipSubRegion")
private String geoipSubRegion = null;
@SerializedName("eventMeta")
private Object eventMeta = null;
@SerializedName("created")
private OffsetDateTime created = null;
public UserEvent id(Double id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
**/
@ApiModelProperty(value = "")
public Double getId() {
return id;
}
public void setId(Double id) {
this.id = id;
}
public UserEvent type(TypeEnum type) {
this.type = type;
return this;
}
/**
* Get type
* @return type
**/
@ApiModelProperty(required = true, value = "")
public TypeEnum getType() {
return type;
}
public void setType(TypeEnum type) {
this.type = type;
}
public UserEvent status(StatusEnum status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
**/
@ApiModelProperty(required = true, value = "")
public StatusEnum getStatus() {
return status;
}
public void setStatus(StatusEnum status) {
this.status = status;
}
public UserEvent userId(Double userId) {
this.userId = userId;
return this;
}
/**
* Get userId
* @return userId
**/
@ApiModelProperty(required = true, value = "")
public Double getUserId() {
return userId;
}
public void setUserId(Double userId) {
this.userId = userId;
}
public UserEvent createdById(Double createdById) {
this.createdById = createdById;
return this;
}
/**
* Get createdById
* @return createdById
**/
@ApiModelProperty(required = true, value = "")
public Double getCreatedById() {
return createdById;
}
public void setCreatedById(Double createdById) {
this.createdById = createdById;
}
public UserEvent ip(String ip) {
this.ip = ip;
return this;
}
/**
* Get ip
* @return ip
**/
@ApiModelProperty(value = "")
public String getIp() {
return ip;
}
public void setIp(String ip) {
this.ip = ip;
}
public UserEvent geoipCountry(String geoipCountry) {
this.geoipCountry = geoipCountry;
return this;
}
/**
* Get geoipCountry
* @return geoipCountry
**/
@ApiModelProperty(value = "")
public String getGeoipCountry() {
return geoipCountry;
}
public void setGeoipCountry(String geoipCountry) {
this.geoipCountry = geoipCountry;
}
public UserEvent geoipRegion(String geoipRegion) {
this.geoipRegion = geoipRegion;
return this;
}
/**
* Get geoipRegion
* @return geoipRegion
**/
@ApiModelProperty(value = "")
public String getGeoipRegion() {
return geoipRegion;
}
public void setGeoipRegion(String geoipRegion) {
this.geoipRegion = geoipRegion;
}
public UserEvent geoipSubRegion(String geoipSubRegion) {
this.geoipSubRegion = geoipSubRegion;
return this;
}
/**
* Get geoipSubRegion
* @return geoipSubRegion
**/
@ApiModelProperty(value = "")
public String getGeoipSubRegion() {
return geoipSubRegion;
}
public void setGeoipSubRegion(String geoipSubRegion) {
this.geoipSubRegion = geoipSubRegion;
}
public UserEvent eventMeta(Object eventMeta) {
this.eventMeta = eventMeta;
return this;
}
/**
* Get eventMeta
* @return eventMeta
**/
@ApiModelProperty(value = "")
public Object getEventMeta() {
return eventMeta;
}
public void setEventMeta(Object eventMeta) {
this.eventMeta = eventMeta;
}
public UserEvent created(OffsetDateTime created) {
this.created = created;
return this;
}
/**
* Get created
* @return created
**/
@ApiModelProperty(required = true, value = "")
public OffsetDateTime getCreated() {
return created;
}
public void setCreated(OffsetDateTime created) {
this.created = created;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
UserEvent userEvent = (UserEvent) o;
return Objects.equals(this.id, userEvent.id) &&
Objects.equals(this.type, userEvent.type) &&
Objects.equals(this.status, userEvent.status) &&
Objects.equals(this.userId, userEvent.userId) &&
Objects.equals(this.createdById, userEvent.createdById) &&
Objects.equals(this.ip, userEvent.ip) &&
Objects.equals(this.geoipCountry, userEvent.geoipCountry) &&
Objects.equals(this.geoipRegion, userEvent.geoipRegion) &&
Objects.equals(this.geoipSubRegion, userEvent.geoipSubRegion) &&
Objects.equals(this.eventMeta, userEvent.eventMeta) &&
Objects.equals(this.created, userEvent.created);
}
@Override
public int hashCode() {
return Objects.hash(id, type, status, userId, createdById, ip, geoipCountry, geoipRegion, geoipSubRegion, eventMeta, created);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class UserEvent {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" userId: ").append(toIndentedString(userId)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" ip: ").append(toIndentedString(ip)).append("\n");
sb.append(" geoipCountry: ").append(toIndentedString(geoipCountry)).append("\n");
sb.append(" geoipRegion: ").append(toIndentedString(geoipRegion)).append("\n");
sb.append(" geoipSubRegion: ").append(toIndentedString(geoipSubRegion)).append("\n");
sb.append(" eventMeta: ").append(toIndentedString(eventMeta)).append("\n");
sb.append(" created: ").append(toIndentedString(created)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy