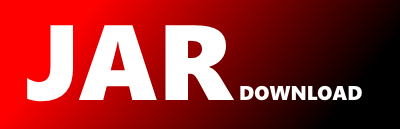
net.zerobuilder.compiler.generate.Generator Maven / Gradle / Ivy
The newest version!
package net.zerobuilder.compiler.generate;
import com.squareup.javapoet.FieldSpec;
import com.squareup.javapoet.TypeSpec;
import net.zerobuilder.compiler.generate.DtoContext.GoalContext;
import net.zerobuilder.compiler.generate.DtoGeneratorInput.AbstractGoalInput;
import net.zerobuilder.compiler.generate.DtoGeneratorInput.GeneratorInput;
import net.zerobuilder.compiler.generate.DtoGeneratorOutput.BuilderMethod;
import net.zerobuilder.compiler.generate.DtoGeneratorOutput.GeneratorOutput;
import net.zerobuilder.compiler.generate.DtoModuleOutput.ModuleOutput;
import java.util.List;
import java.util.function.Function;
import java.util.stream.Collector;
import static java.util.stream.Collectors.toList;
import static net.zerobuilder.compiler.generate.DtoGeneratorInput.goalInputCases;
import static net.zerobuilder.compiler.generate.ZeroUtil.flatList;
import static net.zerobuilder.compiler.generate.ZeroUtil.listCollector;
public final class Generator {
/**
* Entry point for code generation.
*
* @param generatorInput Goal descriptions
* @return a GeneratorOutput
*/
public static GeneratorOutput generate(GeneratorInput generatorInput) {
List goals = generatorInput.goals;
DtoContext.GoalContext context = generatorInput.context;
return goals.stream()
.filter(hasParameters::apply)
.map(process)
.collect(collectOutput(context));
}
private static final Function hasParameters =
goalInputCases(
projected -> !projected.description.parameters.isEmpty(),
regular -> !regular.description.parameters.isEmpty(),
bean -> !bean.description.parameters.isEmpty());
private static Collector, GeneratorOutput> collectOutput(GoalContext context) {
return listCollector(tmpOutputs ->
GeneratorOutput.create(
methods(tmpOutputs),
types(tmpOutputs),
fields(tmpOutputs),
context));
}
private static List methods(List outputs) {
return outputs.stream()
.map(ModuleOutput::method)
.collect(toList());
}
private static List types(List outputs) {
return outputs.stream()
.map(ModuleOutput::typeSpecs)
.collect(flatList());
}
private static List fields(List outputs) {
return outputs.stream()
.map(ModuleOutput::cacheFields)
.collect(flatList());
}
private static final Function process =
goalInputCases(
projected -> projected.module.process(projected.description),
regularSimple -> regularSimple.module.process(regularSimple.description),
bean -> bean.module.process(bean.description));
private Generator() {
throw new UnsupportedOperationException("no instances");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy