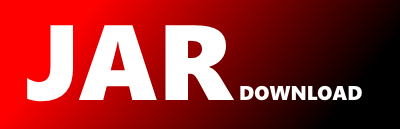
com.hadii.antlr.golang.GolangVisitor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clarpse Show documentation
Show all versions of clarpse Show documentation
Clarpse is a lightweight polyglot source code analysis tool.
// Generated from com/hadii/antlr/golang/Golang.g4 by ANTLR 4.4
package com.hadii.antlr.golang;
import org.antlr.v4.runtime.Token;
import org.antlr.v4.runtime.misc.NotNull;
import org.antlr.v4.runtime.tree.ParseTreeVisitor;
/**
* This interface defines a complete generic visitor for a parse tree produced
* by {@link GolangParser}.
*
* @param The return type of the visit operation. Use {@link Void} for
* operations with no return type.
*/
public interface GolangVisitor extends ParseTreeVisitor {
/**
* Visit a parse tree produced by {@link GolangParser#topLevelDecl}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitTopLevelDecl(@NotNull GolangParser.TopLevelDeclContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#exprCaseClause}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitExprCaseClause(@NotNull GolangParser.ExprCaseClauseContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#eos}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitEos(@NotNull GolangParser.EosContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#channelType}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitChannelType(@NotNull GolangParser.ChannelTypeContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#type}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitType(@NotNull GolangParser.TypeContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#sourceFile}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitSourceFile(@NotNull GolangParser.SourceFileContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#varSpec}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitVarSpec(@NotNull GolangParser.VarSpecContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#anonymousField}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitAnonymousField(@NotNull GolangParser.AnonymousFieldContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#literalType}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitLiteralType(@NotNull GolangParser.LiteralTypeContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#function}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitFunction(@NotNull GolangParser.FunctionContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#statementList}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitStatementList(@NotNull GolangParser.StatementListContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#methodExpr}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitMethodExpr(@NotNull GolangParser.MethodExprContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#block}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitBlock(@NotNull GolangParser.BlockContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#typeSwitchGuard}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitTypeSwitchGuard(@NotNull GolangParser.TypeSwitchGuardContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#keyedElement}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitKeyedElement(@NotNull GolangParser.KeyedElementContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#element}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitElement(@NotNull GolangParser.ElementContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#forStmt}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitForStmt(@NotNull GolangParser.ForStmtContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#identifierList}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitIdentifierList(@NotNull GolangParser.IdentifierListContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#receiver}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitReceiver(@NotNull GolangParser.ReceiverContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#fieldDecl}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitFieldDecl(@NotNull GolangParser.FieldDeclContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#parameterDecl}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitParameterDecl(@NotNull GolangParser.ParameterDeclContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#typeSwitchCase}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitTypeSwitchCase(@NotNull GolangParser.TypeSwitchCaseContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#importSpec}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitImportSpec(@NotNull GolangParser.ImportSpecContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#typeDecl}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitTypeDecl(@NotNull GolangParser.TypeDeclContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#simpleStmt}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitSimpleStmt(@NotNull GolangParser.SimpleStmtContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#expressionList}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitExpressionList(@NotNull GolangParser.ExpressionListContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#receiverType}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitReceiverType(@NotNull GolangParser.ReceiverTypeContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#parameterList}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitParameterList(@NotNull GolangParser.ParameterListContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#typeSpec}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitTypeSpec(@NotNull GolangParser.TypeSpecContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#parameters}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitParameters(@NotNull GolangParser.ParametersContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#importPath}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitImportPath(@NotNull GolangParser.ImportPathContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#functionDecl}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitFunctionDecl(@NotNull GolangParser.FunctionDeclContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#packageClause}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitPackageClause(@NotNull GolangParser.PackageClauseContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#pointerType}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitPointerType(@NotNull GolangParser.PointerTypeContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#signature}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitSignature(@NotNull GolangParser.SignatureContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#typeName}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitTypeName(@NotNull GolangParser.TypeNameContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#importDecl}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitImportDecl(@NotNull GolangParser.ImportDeclContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#methodDecl}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitMethodDecl(@NotNull GolangParser.MethodDeclContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#exprSwitchCase}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitExprSwitchCase(@NotNull GolangParser.ExprSwitchCaseContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#qualifiedIdent}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitQualifiedIdent(@NotNull GolangParser.QualifiedIdentContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitLiteral(@NotNull GolangParser.LiteralContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#result}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitResult(@NotNull GolangParser.ResultContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#interfaceType}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitInterfaceType(@NotNull GolangParser.InterfaceTypeContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#unaryExpr}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitUnaryExpr(@NotNull GolangParser.UnaryExprContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#typeList}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitTypeList(@NotNull GolangParser.TypeListContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#commCase}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitCommCase(@NotNull GolangParser.CommCaseContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#primaryExpr}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitPrimaryExpr(@NotNull GolangParser.PrimaryExprContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#structType}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitStructType(@NotNull GolangParser.StructTypeContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#statement}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitStatement(@NotNull GolangParser.StatementContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#typeLit}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitTypeLit(@NotNull GolangParser.TypeLitContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#methodSpec}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitMethodSpec(@NotNull GolangParser.MethodSpecContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#functionType}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitFunctionType(@NotNull GolangParser.FunctionTypeContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#operandName}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitOperandName(@NotNull GolangParser.OperandNameContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#key}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitKey(@NotNull GolangParser.KeyContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitExpression(@NotNull GolangParser.ExpressionContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#expressionStmt}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitExpressionStmt(@NotNull GolangParser.ExpressionStmtContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#constDecl}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitConstDecl(@NotNull GolangParser.ConstDeclContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#declaration}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitDeclaration(@NotNull GolangParser.DeclarationContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#typeCaseClause}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitTypeCaseClause(@NotNull GolangParser.TypeCaseClauseContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#constSpec}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitConstSpec(@NotNull GolangParser.ConstSpecContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#arrayLength}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitArrayLength(@NotNull GolangParser.ArrayLengthContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#ifStmt}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitIfStmt(@NotNull GolangParser.IfStmtContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#commClause}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitCommClause(@NotNull GolangParser.CommClauseContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#forClause}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitForClause(@NotNull GolangParser.ForClauseContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#rangeClause}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitRangeClause(@NotNull GolangParser.RangeClauseContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#varDecl}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitVarDecl(@NotNull GolangParser.VarDeclContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#elementType}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitElementType(@NotNull GolangParser.ElementTypeContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#operand}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitOperand(@NotNull GolangParser.OperandContext ctx);
/**
* Visit a parse tree produced by {@link GolangParser#recvStmt}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitRecvStmt(@NotNull GolangParser.RecvStmtContext ctx);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy