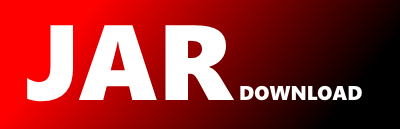
com.hadii.antlr.golang.GoParserVisitor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clarpse Show documentation
Show all versions of clarpse Show documentation
Clarpse is a lightweight polyglot source code analysis tool.
// Generated from com/hadii/antlr/golang/GoParser.g4 by ANTLR 4.4
package com.hadii.antlr.golang;
import org.antlr.v4.runtime.Token;
import org.antlr.v4.runtime.misc.NotNull;
import org.antlr.v4.runtime.tree.ParseTreeVisitor;
/**
* This interface defines a complete generic visitor for a parse tree produced
* by {@link GoParser}.
*
* @param The return type of the visit operation. Use {@link Void} for
* operations with no return type.
*/
public interface GoParserVisitor extends ParseTreeVisitor {
/**
* Visit a parse tree produced by {@link GoParser#shortVarDecl}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitShortVarDecl(@NotNull GoParser.ShortVarDeclContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#eos}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitEos(@NotNull GoParser.EosContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#anonymousField}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitAnonymousField(@NotNull GoParser.AnonymousFieldContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#literalType}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitLiteralType(@NotNull GoParser.LiteralTypeContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#slice}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitSlice(@NotNull GoParser.SliceContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#block}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitBlock(@NotNull GoParser.BlockContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#continueStmt}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitContinueStmt(@NotNull GoParser.ContinueStmtContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#element}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitElement(@NotNull GoParser.ElementContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#arrayType}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitArrayType(@NotNull GoParser.ArrayTypeContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#identifierList}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitIdentifierList(@NotNull GoParser.IdentifierListContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#receiver}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitReceiver(@NotNull GoParser.ReceiverContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#typeSwitchCase}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitTypeSwitchCase(@NotNull GoParser.TypeSwitchCaseContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#index}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitIndex(@NotNull GoParser.IndexContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#sendStmt}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitSendStmt(@NotNull GoParser.SendStmtContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#basicLit}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitBasicLit(@NotNull GoParser.BasicLitContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#simpleStmt}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitSimpleStmt(@NotNull GoParser.SimpleStmtContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#expressionList}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitExpressionList(@NotNull GoParser.ExpressionListContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#receiverType}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitReceiverType(@NotNull GoParser.ReceiverTypeContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#sliceType}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitSliceType(@NotNull GoParser.SliceTypeContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#signature}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitSignature(@NotNull GoParser.SignatureContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#typeName}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitTypeName(@NotNull GoParser.TypeNameContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#importDecl}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitImportDecl(@NotNull GoParser.ImportDeclContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#qualifiedIdent}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitQualifiedIdent(@NotNull GoParser.QualifiedIdentContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#deferStmt}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitDeferStmt(@NotNull GoParser.DeferStmtContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitLiteral(@NotNull GoParser.LiteralContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#compositeLit}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitCompositeLit(@NotNull GoParser.CompositeLitContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#switchStmt}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitSwitchStmt(@NotNull GoParser.SwitchStmtContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#result}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitResult(@NotNull GoParser.ResultContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#unaryExpr}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitUnaryExpr(@NotNull GoParser.UnaryExprContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#typeList}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitTypeList(@NotNull GoParser.TypeListContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#commCase}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitCommCase(@NotNull GoParser.CommCaseContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#primaryExpr}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitPrimaryExpr(@NotNull GoParser.PrimaryExprContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#structType}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitStructType(@NotNull GoParser.StructTypeContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#functionType}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitFunctionType(@NotNull GoParser.FunctionTypeContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#key}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitKey(@NotNull GoParser.KeyContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitExpression(@NotNull GoParser.ExpressionContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#functionLit}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitFunctionLit(@NotNull GoParser.FunctionLitContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#exprSwitchStmt}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitExprSwitchStmt(@NotNull GoParser.ExprSwitchStmtContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#gotoStmt}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitGotoStmt(@NotNull GoParser.GotoStmtContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#mapType}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitMapType(@NotNull GoParser.MapTypeContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#constDecl}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitConstDecl(@NotNull GoParser.ConstDeclContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#declaration}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitDeclaration(@NotNull GoParser.DeclarationContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#typeCaseClause}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitTypeCaseClause(@NotNull GoParser.TypeCaseClauseContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#constSpec}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitConstSpec(@NotNull GoParser.ConstSpecContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#ifStmt}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitIfStmt(@NotNull GoParser.IfStmtContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#forClause}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitForClause(@NotNull GoParser.ForClauseContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#varDecl}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitVarDecl(@NotNull GoParser.VarDeclContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#elementType}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitElementType(@NotNull GoParser.ElementTypeContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#operand}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitOperand(@NotNull GoParser.OperandContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#recvStmt}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitRecvStmt(@NotNull GoParser.RecvStmtContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#labeledStmt}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitLabeledStmt(@NotNull GoParser.LabeledStmtContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#exprCaseClause}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitExprCaseClause(@NotNull GoParser.ExprCaseClauseContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#typeSwitchStmt}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitTypeSwitchStmt(@NotNull GoParser.TypeSwitchStmtContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#channelType}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitChannelType(@NotNull GoParser.ChannelTypeContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#integer}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitInteger(@NotNull GoParser.IntegerContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#sourceFile}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitSourceFile(@NotNull GoParser.SourceFileContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#varSpec}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitVarSpec(@NotNull GoParser.VarSpecContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#statementList}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitStatementList(@NotNull GoParser.StatementListContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#methodExpr}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitMethodExpr(@NotNull GoParser.MethodExprContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#typeSwitchGuard}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitTypeSwitchGuard(@NotNull GoParser.TypeSwitchGuardContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#incDecStmt}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitIncDecStmt(@NotNull GoParser.IncDecStmtContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#keyedElement}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitKeyedElement(@NotNull GoParser.KeyedElementContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#elementList}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitElementList(@NotNull GoParser.ElementListContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#forStmt}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitForStmt(@NotNull GoParser.ForStmtContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#fieldDecl}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitFieldDecl(@NotNull GoParser.FieldDeclContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#parameterDecl}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitParameterDecl(@NotNull GoParser.ParameterDeclContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#returnStmt}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitReturnStmt(@NotNull GoParser.ReturnStmtContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#importSpec}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitImportSpec(@NotNull GoParser.ImportSpecContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#typeDecl}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitTypeDecl(@NotNull GoParser.TypeDeclContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#selectStmt}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitSelectStmt(@NotNull GoParser.SelectStmtContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#breakStmt}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitBreakStmt(@NotNull GoParser.BreakStmtContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#string_}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitString_(@NotNull GoParser.String_Context ctx);
/**
* Visit a parse tree produced by {@link GoParser#typeSpec}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitTypeSpec(@NotNull GoParser.TypeSpecContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#assign_op}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitAssign_op(@NotNull GoParser.Assign_opContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#parameters}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitParameters(@NotNull GoParser.ParametersContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#literalValue}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitLiteralValue(@NotNull GoParser.LiteralValueContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#importPath}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitImportPath(@NotNull GoParser.ImportPathContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#functionDecl}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitFunctionDecl(@NotNull GoParser.FunctionDeclContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#typeAssertion}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitTypeAssertion(@NotNull GoParser.TypeAssertionContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#packageClause}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitPackageClause(@NotNull GoParser.PackageClauseContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#pointerType}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitPointerType(@NotNull GoParser.PointerTypeContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#methodDecl}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitMethodDecl(@NotNull GoParser.MethodDeclContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#exprSwitchCase}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitExprSwitchCase(@NotNull GoParser.ExprSwitchCaseContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#fallthroughStmt}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitFallthroughStmt(@NotNull GoParser.FallthroughStmtContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#interfaceType}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitInterfaceType(@NotNull GoParser.InterfaceTypeContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#goStmt}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitGoStmt(@NotNull GoParser.GoStmtContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#statement}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitStatement(@NotNull GoParser.StatementContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#typeLit}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitTypeLit(@NotNull GoParser.TypeLitContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#methodSpec}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitMethodSpec(@NotNull GoParser.MethodSpecContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#operandName}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitOperandName(@NotNull GoParser.OperandNameContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#conversion}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitConversion(@NotNull GoParser.ConversionContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#assignment}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitAssignment(@NotNull GoParser.AssignmentContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#expressionStmt}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitExpressionStmt(@NotNull GoParser.ExpressionStmtContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#emptyStmt}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitEmptyStmt(@NotNull GoParser.EmptyStmtContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#arrayLength}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitArrayLength(@NotNull GoParser.ArrayLengthContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#commClause}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitCommClause(@NotNull GoParser.CommClauseContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#rangeClause}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitRangeClause(@NotNull GoParser.RangeClauseContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#arguments}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitArguments(@NotNull GoParser.ArgumentsContext ctx);
/**
* Visit a parse tree produced by {@link GoParser#type_}.
* @param ctx the parse tree
* @return the visitor result
*/
Result visitType_(@NotNull GoParser.Type_Context ctx);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy