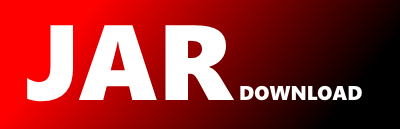
com.github.hammelion.matchers.method.MethodParametersRule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jraml Show documentation
Show all versions of jraml Show documentation
Jraml helps you build your java implementation of REST API, based on specification, described by raml file.
package com.github.hammelion.matchers.method;
import java.beans.Introspector;
import java.lang.annotation.Annotation;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.lang.reflect.Parameter;
import java.util.HashSet;
import java.util.Set;
import javax.inject.Named;
import javax.ws.rs.FormParam;
import javax.ws.rs.HeaderParam;
import javax.ws.rs.PathParam;
import javax.ws.rs.QueryParam;
import org.raml.model.Action;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.google.common.base.CaseFormat;
/**
* All parameters defined in {@link Action} must be in method parameters. It is allowed to have more parameters in method, than
* defined in RAML specification
*/
@Named
class MethodParametersRule {
private static final Logger LOG = LoggerFactory.getLogger(MethodParametersRule.class);
public boolean matches(Method method, Set requiredParameters) {
final HashSet methodParameters = new HashSet();
for (Parameter parameter : method.getParameters()) {
methodParameters.add(Introspector.decapitalize(parameter.getType().getSimpleName()));
try {
final String annotationValue = extractAnnotationValue(parameter);
if (annotationValue != null) {
methodParameters.add(annotationValue);
methodParameters.add(CaseFormat.UPPER_CAMEL.to(CaseFormat.LOWER_UNDERSCORE, annotationValue));
}
} catch (Exception e) {
LOG.error(e.getMessage(), e);
}
}
// TODO Add parameters with same name check
return methodParameters.containsAll(requiredParameters);
}
private String extractAnnotationValue(Parameter parameter) throws NoSuchMethodException, InvocationTargetException,
IllegalAccessException {
for (Annotation annotation : parameter.getAnnotations()) {
if (instanceOfAny(annotation.annotationType(), QueryParam.class, PathParam.class, FormParam.class,
HeaderParam.class)) {
return Introspector.decapitalize((String) annotation.annotationType().getMethod("value").invoke(annotation));
}
}
return null;
}
private static boolean instanceOfAny(Class extends Annotation> annotation, Class... types) {
for (Class type : types) {
if (annotation.equals(type)) {
return true;
}
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy