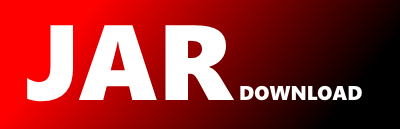
mockit.Injectable Maven / Gradle / Ivy
Show all versions of jmockit Show documentation
/*
* Copyright (c) 2006 JMockit developers
* This file is subject to the terms of the MIT license (see LICENSE.txt).
*/
package mockit;
import static java.lang.annotation.ElementType.FIELD;
import static java.lang.annotation.ElementType.PARAMETER;
import static java.lang.annotation.RetentionPolicy.RUNTIME;
import java.lang.annotation.Retention;
import java.lang.annotation.Target;
/**
* Indicates that the value of a mock field or mock parameter will be an isolated {@linkplain Mocked mocked} instance,
* intended to be passed or injected into the code under test. Such instances can be said to be proper mock
* objects, in contrast to the mocked instances of a regular @Mocked
type.
*
* When the type of the injectable is String
, a primitive wrapper, a {@linkplain Number number type}, or an
* enum, it is not mocked. A non-empty {@link #value} must then be provided, except in the first case where the
* empty string is used by default.
*
* For the duration of each test where the mock field/parameter is in scope, only one injectable instance is
* mocked; other instances of the same mocked type are not affected. For an injectable mocked class, static
* methods and constructors are not mocked; only non-native instance methods are.
*
* When used in combination with {@linkplain Tested @Tested}, the values of injectable fields and parameters will be
* used for automatic injection into the tested object. Additionally, this annotation can be applied to non-mocked
* fields of primitive or array types, which will also be used for injection.
*
* @see #value
* @see Tutorial
*/
@Retention(RUNTIME)
@Target({ FIELD, PARAMETER })
public @interface Injectable {
/**
* Specifies a literal value when the type of the injectable mock field/parameter is String
, a
* primitive or wrapper type, a number type, or an enum type. For a primitive/wrapper/number type, the value
* provided must be convertible to it. For an enum type, the given textual value must equal the name of one of the
* possible enum values.
*
* @return the string
*/
String value() default "";
}