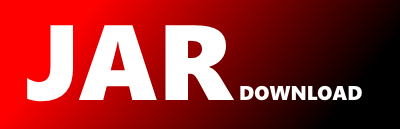
mockit.Mock Maven / Gradle / Ivy
/*
* Copyright (c) 2006 JMockit developers
* This file is subject to the terms of the MIT license (see LICENSE.txt).
*/
package mockit;
import static java.lang.annotation.ElementType.METHOD;
import static java.lang.annotation.RetentionPolicy.RUNTIME;
import java.lang.annotation.Retention;
import java.lang.annotation.Target;
/**
* Used inside a {@linkplain MockUp fake} class to indicate a fake method whose implementation will temporarily
* replace the implementation of one or more matching "real" methods or constructors (these are the "faked"
* methods/constructors).
*
* The fake method must have the same name and the same parameters in order to match a real method, except for an
* optional first parameter of type {@link Invocation}; if this extra parameter is present, the remaining ones
* (if any) must match the parameters in the real method. Alternatively, a single fake method having only the
* Invocation
parameter will match all real methods of the same name, regardless of their parameters. The
* fake method must also have the same return type as the matching real method.
*
* Method modifiers (public
, final
, static
, etc.) between fake and faked methods
* don't have to be the same. It's perfectly fine to have a non-static
fake method for a
* static
faked method (or vice-versa), for example. Checked exceptions in the throws
clause
* (if any) can also differ between the two matching methods.
*
* A fake method can also target a constructor, in which case the previous considerations still apply,
* except for the name of the fake method which must be "$init
".
*
* Another special fake method, "void $clinit()
", will target the static
* initializers of the faked class, if present in the fake class.
*
* Yet another special fake method is "Object $advice(Invocation)
", which if defined will
* match every method in the target class hierarchy.
*
* @see Tutorial
*/
@Retention(RUNTIME)
@Target(METHOD)
public @interface Mock {
/**
* Number of expected invocations of the mock method. If 0 (zero), no invocations will be expected. A negative value
* (the default) means there is no expectation on the number of invocations; that is, the mock can be called any
* number of times or not at all during any test which uses it.
*
* A non-negative value is equivalent to setting {@link #minInvocations minInvocations} and {@link #maxInvocations
* maxInvocations} to that same value.
*
* @return Number of expected invocations of the mock method
*/
int invocations() default -1;
/**
* Minimum number of expected invocations of the mock method, starting from 0 (zero, which is the default).
*
* @return Minimum number of expected invocations of the mock method
*
* @see #invocations
* @see #maxInvocations
*/
int minInvocations() default 0;
/**
* Maximum number of expected invocations of the mock method, if positive. If zero the mock is not expected to be
* called at all. A negative value (the default) means there is no expectation on the maximum number of invocations.
*
* @return Maximum number of expected invocations of the mock method
*
* @see #invocations
* @see #minInvocations
*/
int maxInvocations() default -1;
}