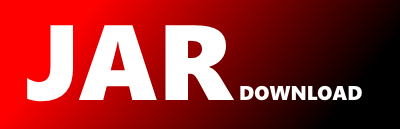
mockit.Mocked Maven / Gradle / Ivy
Show all versions of jmockit Show documentation
/*
* Copyright (c) 2006 JMockit developers
* This file is subject to the terms of the MIT license (see LICENSE.txt).
*/
package mockit;
import static java.lang.annotation.ElementType.FIELD;
import static java.lang.annotation.ElementType.PARAMETER;
import static java.lang.annotation.RetentionPolicy.RUNTIME;
import java.lang.annotation.Retention;
import java.lang.annotation.Target;
/**
* Indicates an instance field of a test class as being a mock field, or a parameter of a test method as a
* mock parameter; in either case, the declared type of the field/parameter is a mocked type, whose
* instances are mocked instances.
*
* Mocked types can also be introduced by other annotations: {@linkplain Injectable @Injectable},
* {@link Capturing @Capturing}. Their effect is to constrain or extend the mocking capabilities here
* specified.
*
* Any type can be mocked, except for primitive and array types. A mocked instance of that type is automatically created
* and assigned to the mock field/parameter, for use when {@linkplain Expectations recording} and/or
* {@linkplain Verifications verifying} expectations. For a mock field, the test itself can provide the
* instance by declaring the field as final
and assigning it the desired instance (or null
).
*
* The effect of declaring a @Mocked
type, by default, is that all new instances of that type, as
* well as those previously created, will also be mocked instances; this will last for the duration of each test where
* the associated mock field/parameter is in scope. All non-private
methods of the mocked type will be
* mocked.
*
* When the mocked type is a class, all super-classes up to but not including java.lang.Object
are also
* mocked. Additionally, static methods and constructors are mocked as well, just like instance
* methods; native methods are also mocked, provided they are public
or protected
.
*
* While a method or constructor is mocked, an invocation does not result in the execution of the original code, but in
* a (generated) call into JMockit, which then responds with either a default or a recorded
* {@linkplain Expectations#result result} (or with a {@linkplain Expectations#times constraint} violation, if the
* invocation is deemed to be unexpected).
*
* Mocking will automatically cascade into the return types of all non-void
methods belonging to
* the mocked type, except for non-eligible ones (primitive wrappers, String
, and collections/maps). When
* needed, such cascaded returns can be overridden by explicitly recording a return value for the mocked method. If
* there is a mock field/parameter with the same type (or a subtype) of some cascaded type, then the original instance
* from that mock field/parameter will be used as the cascaded instance, rather than a new one being created; this
* applies to all cascading levels, and even to the type of the mock field/parameter itself (ie, if a method in
* class/interface "A
" has return type A
, then it will return itself by default). Finally,
* when new cascaded instances are created, {@linkplain Injectable @Injectable} semantics apply.
*
* Static class initializers (including assignments to static fields) of a mocked class are not
* affected, unless {@linkplain #stubOutClassInitialization specified otherwise}.
*
* @see Tutorial
*/
@Retention(RUNTIME)
@Target({ FIELD, PARAMETER })
public @interface Mocked {
/**
* Indicates whether static initialization code in the mocked class should be stubbed out or not. Static
* initialization includes the execution of assignments to static fields of the class and the execution of static
* initialization blocks, if any. (Note that static final fields initialized with compile-time
* constants are not assigned at runtime, remaining unaffected whether the class is stubbed out or not.)
*
* By default, static initialization code in a mocked class is not stubbed out. The JVM will only perform
* static initialization of a class once, so stubbing out the initialization code can have unexpected
* consequences. Stubbing out the static initialization of a class is an unsafe operation, which can cause other
* tests, executed later in the same test run, to unexpectedly fail; instead of resorting to stubbing out a class's
* static initializer, the root cause for wanting to stub it out should be eliminated. Caveat Emptor.
*
* @return true, if successful
*/
boolean stubOutClassInitialization() default false;
}