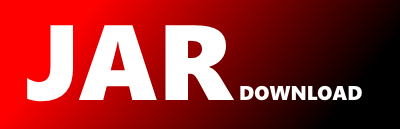
mockit.internal.injection.BeanExporter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jmockit Show documentation
Show all versions of jmockit Show documentation
JMockit is a Java toolkit for automated developer testing.
It contains APIs for the creation of the objects to be tested, for mocking dependencies, and for faking external
APIs; JUnit (4 & 5) and TestNG test runners are supported.
It also contains an advanced code coverage tool.
The newest version!
/*
* Copyright (c) 2006 JMockit developers
* This file is subject to the terms of the MIT license (see LICENSE.txt).
*/
package mockit.internal.injection;
import edu.umd.cs.findbugs.annotations.NonNull;
import edu.umd.cs.findbugs.annotations.Nullable;
import mockit.internal.injection.InjectionPoint.KindOfInjectionPoint;
import mockit.internal.injection.field.FieldInjection;
import mockit.internal.injection.full.FullInjection;
public final class BeanExporter {
@NonNull
private final InjectionState injectionState;
BeanExporter(@NonNull InjectionState injectionState) {
this.injectionState = injectionState;
}
@Nullable
public Object getBean(@NonNull String name) {
InjectionPoint injectionPoint = new InjectionPoint(Object.class, name, true);
return injectionState.getInstantiatedDependency(null, injectionPoint);
}
@Nullable
public T getBean(@NonNull Class beanType) {
TestedClass testedClass = new TestedClass(beanType, beanType);
String beanName = getBeanNameFromType(beanType);
injectionState.injectionProviders.setTypeOfInjectionPoint(beanType, KindOfInjectionPoint.NotAnnotated);
InjectionProvider injectable = injectionState.injectionProviders.findInjectableByTypeAndName(beanName,
testedClass);
if (injectable != null) {
Object testInstance = injectionState.getCurrentTestClassInstance();
return (T) injectable.getValue(testInstance);
}
FullInjection injection = new FullInjection(injectionState, beanType, beanName);
Injector injector = new FieldInjection(injectionState, injection);
return (T) injection.createOrReuseInstance(testedClass, injector, null, beanName);
}
@NonNull
private static String getBeanNameFromType(@NonNull Class> beanType) {
String name = beanType.getSimpleName();
return Character.toLowerCase(name.charAt(0)) + name.substring(1);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy