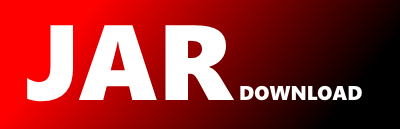
com.helix.kit.HexUtil Maven / Gradle / Ivy
The newest version!
package com.helix.kit;
import java.io.UnsupportedEncodingException;
/**
* Hex工具类,16进制转换算法2
*/
public final class HexUtil {
public static final String CHARSET_NAME_UTF8 = "UTF-8";
public static final char[] digital = "0123456789abcdef".toCharArray();
public static String encodeHexStr(final byte[] bytes) {
if (bytes == null) {
return null;
}
char[] result = new char[bytes.length * 2];
for (int i = 0; i < bytes.length; i++) {
result[i * 2] = digital[(bytes[i] & 0xf0) >> 4];
result[i * 2 + 1] = digital[bytes[i] & 0x0f];
}
return new String(result);
}
public static byte[] decodeHexStr(final String str) {
if (str == null) {
return null;
}
char[] charArray = str.toCharArray();
if (charArray.length % 2 != 0) {
throw new RuntimeException("hex str length must can mod 2, str:" + str);
}
byte[] bytes = new byte[charArray.length / 2];
for (int i = 0; i < charArray.length; i++) {
char c = charArray[i];
int b;
if (c >= '0' && c <= '9') {
b = (c - '0') << 4;
} else if (c >= 'A' && c <= 'F') {
b = (c - 'A' + 10) << 4;
} else {
throw new RuntimeException("unsport hex str:" + str);
}
c = charArray[++i];
if (c >= '0' && c <= '9') {
b |= c - '0';
} else if (c >= 'A' && c <= 'F') {
b |= c - 'A' + 10;
} else {
throw new RuntimeException("unsport hex str:" + str);
}
bytes[i / 2] = (byte) b;
}
return bytes;
}
public static String toString(final byte[] bytes) {
if (bytes == null) {
return null;
}
try {
return new String(bytes, CHARSET_NAME_UTF8);
} catch (final UnsupportedEncodingException e) {
throw new RuntimeException(e.getMessage(), e);
}
}
public static String toString(final byte[] bytes, String charset) {
if (bytes == null) {
return null;
}
try {
return new String(bytes, charset);
} catch (final UnsupportedEncodingException e) {
throw new RuntimeException(e.getMessage(), e);
}
}
public static byte[] toBytes(final String str) {
if (str == null) {
return null;
}
try {
return str.getBytes(CHARSET_NAME_UTF8);
} catch (final UnsupportedEncodingException e) {
throw new RuntimeException(e.getMessage(), e);
}
}
private HexUtil() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy