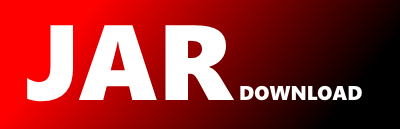
com.github.hekonsek.rxjava.connector.kafka.KafkaEventAdapter Maven / Gradle / Ivy
/**
* Licensed to the RxJava Connector Kafka under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.github.hekonsek.rxjava.connector.kafka;
import com.github.hekonsek.rxjava.event.Event;
import com.google.common.collect.ImmutableMap;
import io.reactivex.functions.Function;
import io.vertx.reactivex.kafka.client.consumer.KafkaConsumerRecord;
import lombok.Data;
import org.apache.kafka.common.serialization.BytesDeserializer;
import org.apache.kafka.common.serialization.Deserializer;
import org.apache.kafka.common.serialization.StringDeserializer;
import org.apache.kafka.common.utils.Bytes;
import java.util.Map;
import static com.github.hekonsek.rxjava.connector.kafka.KafkaHeaders.OFFSET;
import static com.github.hekonsek.rxjava.connector.kafka.KafkaHeaders.PARTITION;
import static com.github.hekonsek.rxjava.event.Headers.ADDRESS;
import static com.github.hekonsek.rxjava.event.Headers.KEY;
import static io.vertx.core.buffer.Buffer.buffer;
import static io.vertx.core.json.Json.decodeValue;
@Data
public class KafkaEventAdapter {
private final Class extends Deserializer> keyDeserializer;
private final Class extends Deserializer> valueDeserializer;
private final Function, Event> mapping;
public static KafkaEventAdapter> stringAndBytesToMap() {
return new KafkaEventAdapter<>(StringDeserializer.class, BytesDeserializer.class,
record -> {
Map value = decodeValue(buffer(((Bytes) record.value()).get()), Map.class);
return new Event<>(headers(record), value);
});
}
public static KafkaEventAdapter simpleMapping(
Class extends Deserializer> keyDeserializer, Class extends Deserializer> valueDeserializer) {
return new KafkaEventAdapter<>(keyDeserializer, valueDeserializer,
record -> new Event<>(headers(record), record.value()));
}
private static Map headers(KafkaConsumerRecord record) {
return ImmutableMap.of(
KEY, record.key(),
ADDRESS, record.topic(),
OFFSET, record.offset(),
PARTITION, record.partition()
);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy